repo
stringclasses 283
values | pull_number
int64 5
44.1k
| instance_id
stringlengths 13
45
| issue_numbers
sequencelengths 1
4
| base_commit
stringlengths 40
40
| patch
stringlengths 179
224k
| test_patch
stringlengths 94
7.54M
| problem_statement
stringlengths 4
256k
| hints_text
stringlengths 0
294k
| created_at
timestamp[s] | version
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|---|
conda/conda | 620 | conda__conda-620 | [
"599"
] | c453be49e865297bf12858548a6b3e7891a8cb43 | diff --git a/conda/resolve.py b/conda/resolve.py
--- a/conda/resolve.py
+++ b/conda/resolve.py
@@ -30,6 +30,11 @@ def normalized_version(version):
return version
+class NoPackagesFound(RuntimeError):
+ def __init__(self, msg, pkg):
+ super(NoPackagesFound, self).__init__(msg)
+ self.pkg = pkg
+
const_pat = re.compile(r'([=<>!]{1,2})(\S+)$')
def ver_eval(version, constraint):
"""
@@ -243,7 +248,7 @@ def track_features(self, fn):
def get_pkgs(self, ms, max_only=False):
pkgs = [Package(fn, self.index[fn]) for fn in self.find_matches(ms)]
if not pkgs:
- raise RuntimeError("No packages found matching: %s" % ms)
+ raise NoPackagesFound("No packages found matching: %s" % ms, ms.spec)
if max_only:
maxpkg = max(pkgs)
ret = []
@@ -262,7 +267,7 @@ def get_pkgs(self, ms, max_only=False):
def get_max_dists(self, ms):
pkgs = self.get_pkgs(ms, max_only=True)
if not pkgs:
- raise RuntimeError("No packages found matching: %s" % ms)
+ raise NoPackagesFound("No packages found matching: %s" % ms, ms.spec)
for pkg in pkgs:
yield pkg.fn
@@ -371,11 +376,22 @@ def generate_version_eq(self, v, dists, include0=False):
def get_dists(self, specs, max_only=False):
dists = {}
for spec in specs:
+ found = False
+ notfound = []
for pkg in self.get_pkgs(MatchSpec(spec), max_only=max_only):
if pkg.fn in dists:
+ found = True
continue
- dists.update(self.all_deps(pkg.fn, max_only=max_only))
- dists[pkg.fn] = pkg
+ try:
+ dists.update(self.all_deps(pkg.fn, max_only=max_only))
+ except NoPackagesFound as e:
+ # Ignore any package that has nonexisting dependencies.
+ notfound.append(e.pkg)
+ else:
+ dists[pkg.fn] = pkg
+ found = True
+ if not found:
+ raise NoPackagesFound("Could not find some dependencies for %s: %s" % (spec, ', '.join(notfound)), None)
return dists
@@ -387,25 +403,31 @@ def solve2(self, specs, features, guess=True, alg='sorter', returnall=False):
# complicated cases that the pseudo-boolean solver does, but it's also
# much faster when it does work.
- dists = self.get_dists(specs, max_only=True)
-
- v = {} # map fn to variable number
- w = {} # map variable number to fn
- i = -1 # in case the loop doesn't run
- for i, fn in enumerate(sorted(dists)):
- v[fn] = i + 1
- w[i + 1] = fn
- m = i + 1
-
- dotlog.debug("Solving using max dists only")
- clauses = self.gen_clauses(v, dists, specs, features)
- solutions = min_sat(clauses)
-
- if len(solutions) == 1:
- ret = [w[lit] for lit in solutions.pop(0) if 0 < lit]
- if returnall:
- return [ret]
- return ret
+ try:
+ dists = self.get_dists(specs, max_only=True)
+ except NoPackagesFound:
+ # Handle packages that are not included because some dependencies
+ # couldn't be found.
+ pass
+ else:
+ v = {} # map fn to variable number
+ w = {} # map variable number to fn
+ i = -1 # in case the loop doesn't run
+ for i, fn in enumerate(sorted(dists)):
+ v[fn] = i + 1
+ w[i + 1] = fn
+ m = i + 1
+
+ dotlog.debug("Solving using max dists only")
+ clauses = self.gen_clauses(v, dists, specs, features)
+ solutions = min_sat(clauses)
+
+
+ if len(solutions) == 1:
+ ret = [w[lit] for lit in solutions.pop(0) if 0 < lit]
+ if returnall:
+ return [ret]
+ return ret
dists = self.get_dists(specs)
| diff --git a/tests/test_resolve.py b/tests/test_resolve.py
--- a/tests/test_resolve.py
+++ b/tests/test_resolve.py
@@ -3,13 +3,15 @@
import unittest
from os.path import dirname, join
-from conda.resolve import ver_eval, VersionSpec, MatchSpec, Package, Resolve
+from conda.resolve import ver_eval, VersionSpec, MatchSpec, Package, Resolve, NoPackagesFound
from .helpers import raises
with open(join(dirname(__file__), 'index.json')) as fi:
- r = Resolve(json.load(fi))
+ index = json.load(fi)
+
+r = Resolve(index)
f_mkl = set(['mkl'])
@@ -672,9 +674,183 @@ def test_unsat():
def test_nonexistent():
r.msd_cache = {}
- assert raises(RuntimeError, lambda: r.solve(['notarealpackage 2.0*']), 'No packages found')
+ assert raises(NoPackagesFound, lambda: r.solve(['notarealpackage 2.0*']), 'No packages found')
# This exact version of NumPy does not exist
- assert raises(RuntimeError, lambda: r.solve(['numpy 1.5']), 'No packages found')
+ assert raises(NoPackagesFound, lambda: r.solve(['numpy 1.5']), 'No packages found')
+
+def test_nonexistent_deps():
+ index2 = index.copy()
+ index2['mypackage-1.0-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'python 3.3*', 'notarealpackage 2.0*'],
+ 'name': 'mypackage',
+ 'requires': ['nose 1.2.1', 'python 3.3'],
+ 'version': '1.0',
+ }
+ index2['mypackage-1.1-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'python 3.3*'],
+ 'name': 'mypackage',
+ 'requires': ['nose 1.2.1', 'python 3.3'],
+ 'version': '1.1',
+ }
+ r = Resolve(index2)
+
+ assert set(r.find_matches(MatchSpec('mypackage'))) == {
+ 'mypackage-1.0-py33_0.tar.bz2',
+ 'mypackage-1.1-py33_0.tar.bz2',
+ }
+ assert set(r.get_dists(['mypackage']).keys()) == {
+ 'mypackage-1.1-py33_0.tar.bz2',
+ 'nose-1.1.2-py26_0.tar.bz2',
+ 'nose-1.1.2-py27_0.tar.bz2',
+ 'nose-1.1.2-py33_0.tar.bz2',
+ 'nose-1.2.1-py26_0.tar.bz2',
+ 'nose-1.2.1-py27_0.tar.bz2',
+ 'nose-1.2.1-py33_0.tar.bz2',
+ 'nose-1.3.0-py26_0.tar.bz2',
+ 'nose-1.3.0-py27_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-2.6.8-1.tar.bz2',
+ 'python-2.6.8-2.tar.bz2',
+ 'python-2.6.8-3.tar.bz2',
+ 'python-2.6.8-4.tar.bz2',
+ 'python-2.6.8-5.tar.bz2',
+ 'python-2.6.8-6.tar.bz2',
+ 'python-2.7.3-2.tar.bz2',
+ 'python-2.7.3-3.tar.bz2',
+ 'python-2.7.3-4.tar.bz2',
+ 'python-2.7.3-5.tar.bz2',
+ 'python-2.7.3-6.tar.bz2',
+ 'python-2.7.3-7.tar.bz2',
+ 'python-2.7.4-0.tar.bz2',
+ 'python-2.7.5-0.tar.bz2',
+ 'python-3.3.0-2.tar.bz2',
+ 'python-3.3.0-3.tar.bz2',
+ 'python-3.3.0-4.tar.bz2',
+ 'python-3.3.0-pro0.tar.bz2',
+ 'python-3.3.0-pro1.tar.bz2',
+ 'python-3.3.1-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ }
+
+ assert set(r.get_dists(['mypackage'], max_only=True).keys()) == {
+ 'mypackage-1.1-py33_0.tar.bz2',
+ 'nose-1.3.0-py26_0.tar.bz2',
+ 'nose-1.3.0-py27_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-2.6.8-6.tar.bz2',
+ 'python-2.7.5-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ }
+
+ assert r.solve(['mypackage']) == r.solve(['mypackage 1.1']) == [
+ 'mypackage-1.1-py33_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ ]
+ assert raises(RuntimeError, lambda: r.solve(['mypackage 1.0']))
+
+ # This time, the latest version is messed up
+ index3 = index.copy()
+ index3['mypackage-1.1-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'python 3.3*', 'notarealpackage 2.0*'],
+ 'name': 'mypackage',
+ 'requires': ['nose 1.2.1', 'python 3.3'],
+ 'version': '1.1',
+ }
+ index3['mypackage-1.0-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'python 3.3*'],
+ 'name': 'mypackage',
+ 'requires': ['nose 1.2.1', 'python 3.3'],
+ 'version': '1.0',
+ }
+ r = Resolve(index3)
+
+ assert set(r.find_matches(MatchSpec('mypackage'))) == {
+ 'mypackage-1.0-py33_0.tar.bz2',
+ 'mypackage-1.1-py33_0.tar.bz2',
+ }
+ assert set(r.get_dists(['mypackage']).keys()) == {
+ 'mypackage-1.0-py33_0.tar.bz2',
+ 'nose-1.1.2-py26_0.tar.bz2',
+ 'nose-1.1.2-py27_0.tar.bz2',
+ 'nose-1.1.2-py33_0.tar.bz2',
+ 'nose-1.2.1-py26_0.tar.bz2',
+ 'nose-1.2.1-py27_0.tar.bz2',
+ 'nose-1.2.1-py33_0.tar.bz2',
+ 'nose-1.3.0-py26_0.tar.bz2',
+ 'nose-1.3.0-py27_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-2.6.8-1.tar.bz2',
+ 'python-2.6.8-2.tar.bz2',
+ 'python-2.6.8-3.tar.bz2',
+ 'python-2.6.8-4.tar.bz2',
+ 'python-2.6.8-5.tar.bz2',
+ 'python-2.6.8-6.tar.bz2',
+ 'python-2.7.3-2.tar.bz2',
+ 'python-2.7.3-3.tar.bz2',
+ 'python-2.7.3-4.tar.bz2',
+ 'python-2.7.3-5.tar.bz2',
+ 'python-2.7.3-6.tar.bz2',
+ 'python-2.7.3-7.tar.bz2',
+ 'python-2.7.4-0.tar.bz2',
+ 'python-2.7.5-0.tar.bz2',
+ 'python-3.3.0-2.tar.bz2',
+ 'python-3.3.0-3.tar.bz2',
+ 'python-3.3.0-4.tar.bz2',
+ 'python-3.3.0-pro0.tar.bz2',
+ 'python-3.3.0-pro1.tar.bz2',
+ 'python-3.3.1-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ }
+
+ assert raises(RuntimeError, lambda: r.get_dists(['mypackage'], max_only=True))
+
+ assert r.solve(['mypackage']) == r.solve(['mypackage 1.0']) == [
+ 'mypackage-1.0-py33_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ ]
+ assert raises(NoPackagesFound, lambda: r.solve(['mypackage 1.1']))
def test_package_ordering():
sympy_071 = Package('sympy-0.7.1-py27_0.tar.bz2', r.index['sympy-0.7.1-py27_0.tar.bz2'])
| Don't bail when a dependency can't be found
When a dependency for a package can't be found, conda bails completely, but this can happen e.g., just for some old builds of something. So we should just exclude any package like this from the solver.
| It can also happen if someone has a package on their binstar but not all the dependencies for it.
| 2014-03-24T18:23:13 | -1.0 |
conda/conda | 662 | conda__conda-662 | [
"464"
] | 3d4118668fca738984cce13d9235e0fc11a79df4 | diff --git a/conda/cli/main_remove.py b/conda/cli/main_remove.py
--- a/conda/cli/main_remove.py
+++ b/conda/cli/main_remove.py
@@ -63,6 +63,7 @@ def execute(args, parser):
from conda.api import get_index
from conda.cli import pscheck
from conda.install import rm_rf, linked
+ from conda import config
if not (args.all or args.package_names):
sys.exit('Error: no package names supplied,\n'
@@ -71,12 +72,11 @@ def execute(args, parser):
prefix = common.get_prefix(args)
common.check_write('remove', prefix)
- index = None
+ common.ensure_override_channels_requires_channel(args)
+ channel_urls = args.channel or ()
+ index = get_index(channel_urls=channel_urls,
+ prepend=not args.override_channels)
if args.features:
- common.ensure_override_channels_requires_channel(args)
- channel_urls = args.channel or ()
- index = get_index(channel_urls=channel_urls,
- prepend=not args.override_channels)
features = set(args.package_names)
actions = plan.remove_features_actions(prefix, index, features)
diff --git a/conda/plan.py b/conda/plan.py
--- a/conda/plan.py
+++ b/conda/plan.py
@@ -20,7 +20,7 @@
from conda import install
from conda.fetch import fetch_pkg
from conda.history import History
-from conda.resolve import MatchSpec, Resolve
+from conda.resolve import MatchSpec, Resolve, Package
from conda.utils import md5_file, human_bytes
log = getLogger(__name__)
@@ -60,7 +60,7 @@ def split_linkarg(arg):
linktype = install.LINK_HARD
return dist, pkgs_dir, int(linktype)
-def display_actions(actions, index=None):
+def display_actions(actions, index):
if actions.get(FETCH):
print("\nThe following packages will be downloaded:\n")
@@ -79,19 +79,113 @@ def display_actions(actions, index=None):
print(" " * 43 + "Total: %14s" %
human_bytes(sum(index[dist + '.tar.bz2']['size']
for dist in actions[FETCH])))
- if actions.get(UNLINK):
- print("\nThe following packages will be UN-linked:\n")
- print_dists([
- (dist, None)
- for dist in actions[UNLINK]])
- if actions.get(LINK):
- print("\nThe following packages will be linked:\n")
- lst = []
- for arg in actions[LINK]:
- dist, pkgs_dir, lt = split_linkarg(arg)
- extra = ' %s' % install.link_name_map.get(lt)
- lst.append((dist, extra))
- print_dists(lst)
+
+ # package -> [oldver-oldbuild, newver-newbuild]
+ packages = defaultdict(lambda: list(('', '')))
+ features = defaultdict(lambda: list(('', '')))
+
+ # This assumes each package will appear in LINK no more than once.
+ Packages = {}
+ linktypes = {}
+ for arg in actions.get(LINK, []):
+ dist, pkgs_dir, lt = split_linkarg(arg)
+ pkg, ver, build = dist.rsplit('-', 2)
+ packages[pkg][1] = ver + '-' + build
+ Packages[dist] = Package(dist + '.tar.bz2', index[dist + '.tar.bz2'])
+ linktypes[pkg] = lt
+ features[pkg][1] = index[dist + '.tar.bz2'].get('features', '')
+ for arg in actions.get(UNLINK, []):
+ dist, pkgs_dir, lt = split_linkarg(arg)
+ pkg, ver, build = dist.rsplit('-', 2)
+ packages[pkg][0] = ver + '-' + build
+ Packages[dist] = Package(dist + '.tar.bz2', index[dist + '.tar.bz2'])
+ features[pkg][0] = index[dist + '.tar.bz2'].get('features', '')
+
+ # Put a minimum length here---. .--For the :
+ # v v
+ maxpkg = max(len(max(packages or [''], key=len)), 0) + 1
+ maxoldver = len(max(packages.values() or [['']], key=lambda i: len(i[0]))[0])
+ maxnewver = len(max(packages.values() or [['', '']], key=lambda i: len(i[1]))[1])
+ maxoldfeatures = len(max(features.values() or [['']], key=lambda i: len(i[0]))[0])
+ maxnewfeatures = len(max(features.values() or [['', '']], key=lambda i: len(i[1]))[1])
+ maxoldchannel = len(max([config.canonical_channel_name(Packages[pkg + '-' +
+ packages[pkg][0]].channel) for pkg in packages if packages[pkg][0]] or
+ [''], key=len))
+ maxnewchannel = len(max([config.canonical_channel_name(Packages[pkg + '-' +
+ packages[pkg][1]].channel) for pkg in packages if packages[pkg][1]] or
+ [''], key=len))
+ new = {pkg for pkg in packages if not packages[pkg][0]}
+ removed = {pkg for pkg in packages if not packages[pkg][1]}
+ updated = set()
+ downgraded = set()
+ oldfmt = {}
+ newfmt = {}
+ for pkg in packages:
+ # That's right. I'm using old-style string formatting to generate a
+ # string with new-style string formatting.
+ oldfmt[pkg] = '{pkg:<%s} {vers[0]:<%s}' % (maxpkg, maxoldver)
+ if config.show_channel_urls:
+ oldfmt[pkg] += ' {channel[0]:<%s}' % maxoldchannel
+ if packages[pkg][0]:
+ newfmt[pkg] = '{vers[1]:<%s}' % maxnewver
+ else:
+ newfmt[pkg] = '{pkg:<%s} {vers[1]:<%s}' % (maxpkg, maxnewver)
+ if config.show_channel_urls:
+ newfmt[pkg] += ' {channel[1]:<%s}' % maxnewchannel
+ # TODO: Should we also care about the old package's link type?
+ if pkg in linktypes and linktypes[pkg] != install.LINK_HARD:
+ newfmt[pkg] += ' (%s)' % install.link_name_map[linktypes[pkg]]
+
+ if features[pkg][0]:
+ oldfmt[pkg] += ' [{features[0]:<%s}]' % maxoldfeatures
+ if features[pkg][1]:
+ newfmt[pkg] += ' [{features[1]:<%s}]' % maxnewfeatures
+
+ if pkg in new or pkg in removed:
+ continue
+ P0 = Packages[pkg + '-' + packages[pkg][0]]
+ P1 = Packages[pkg + '-' + packages[pkg][1]]
+ try:
+ # <= here means that unchanged packages will be put in updated
+ newer = (P0.name, P0.norm_version, P0.build_number) <= (P1.name, P1.norm_version, P1.build_number)
+ except TypeError:
+ newer = (P0.name, P0.version, P0.build_number) <= (P1.name, P1.version, P1.build_number)
+ if newer:
+ updated.add(pkg)
+ else:
+ downgraded.add(pkg)
+
+ arrow = ' --> '
+ lead = ' '*4
+
+ def format(s, pkg):
+ channel = ['', '']
+ for i in range(2):
+ if packages[pkg][i]:
+ channel[i] = config.canonical_channel_name(Packages[pkg + '-' + packages[pkg][i]].channel)
+ return lead + s.format(pkg=pkg+':', vers=packages[pkg],
+ channel=channel, features=features[pkg])
+
+ if new:
+ print("\nThe following NEW packages will be INSTALLED:\n")
+ for pkg in sorted(new):
+ print(format(newfmt[pkg], pkg))
+
+ if removed:
+ print("\nThe following packages will be REMOVED:\n")
+ for pkg in sorted(removed):
+ print(format(oldfmt[pkg], pkg))
+
+ if updated:
+ print("\nThe following packages will be UPDATED:\n")
+ for pkg in sorted(updated):
+ print(format(oldfmt[pkg] + arrow + newfmt[pkg], pkg))
+
+ if downgraded:
+ print("\nThe following packages will be DOWNGRADED:\n")
+ for pkg in sorted(downgraded):
+ print(format(oldfmt[pkg] + arrow + newfmt[pkg], pkg))
+
print()
# the order matters here, don't change it
| diff --git a/tests/helpers.py b/tests/helpers.py
--- a/tests/helpers.py
+++ b/tests/helpers.py
@@ -5,6 +5,8 @@
import sys
import os
+from contextlib import contextmanager
+
def raises(exception, func, string=None):
try:
a = func()
@@ -35,3 +37,31 @@ def run_conda_command(*args):
stdout, stderr = p.communicate()
return (stdout.decode('utf-8').replace('\r\n', '\n'),
stderr.decode('utf-8').replace('\r\n', '\n'))
+
+class CapturedText(object):
+ pass
+
+@contextmanager
+def captured():
+ """
+ Context manager to capture the printed output of the code in the with block
+
+ Bind the context manager to a variable using `as` and the result will be
+ in the stdout property.
+
+ >>> from tests.helpers import capture
+ >>> with captured() as c:
+ ... print('hello world!')
+ ...
+ >>> c.stdout
+ 'hello world!\n'
+ """
+ from conda.compat import StringIO
+ import sys
+
+ stdout = sys.stdout
+ sys.stdout = file = StringIO()
+ c = CapturedText()
+ yield c
+ c.stdout = file.getvalue()
+ sys.stdout = stdout
diff --git a/tests/test_plan.py b/tests/test_plan.py
--- a/tests/test_plan.py
+++ b/tests/test_plan.py
@@ -1,15 +1,20 @@
import json
import unittest
from os.path import dirname, join
+from collections import defaultdict
from conda.config import default_python, pkgs_dirs
+import conda.config
from conda.install import LINK_HARD
import conda.plan as plan
+from conda.plan import display_actions
from conda.resolve import Resolve
+from tests.helpers import captured
with open(join(dirname(__file__), 'index.json')) as fi:
- r = Resolve(json.load(fi))
+ index = json.load(fi)
+ r = Resolve(index)
def solve(specs):
return [fn[:-8] for fn in r.solve(specs)]
@@ -77,3 +82,685 @@ def test_4(self):
(['anaconda', 'python 3*'], []),
]:
self.check(specs, added)
+
+def test_display_actions():
+ conda.config.show_channel_urls = False
+ actions = defaultdict(list, {"FETCH": ['sympy-0.7.2-py27_0',
+ "numpy-1.7.1-py27_0"]})
+ # The older test index doesn't have the size metadata
+ index['sympy-0.7.2-py27_0.tar.bz2']['size'] = 4374752
+ index["numpy-1.7.1-py27_0.tar.bz2"]['size'] = 5994338
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be downloaded:
+
+ package | build
+ ---------------------------|-----------------
+ sympy-0.7.2 | py27_0 4.2 MB
+ numpy-1.7.1 | py27_0 5.7 MB
+ ------------------------------------------------------------
+ Total: 9.9 MB
+
+"""
+
+ actions = defaultdict(list, {'PREFIX':
+ '/Users/aaronmeurer/anaconda/envs/test', 'SYMLINK_CONDA':
+ ['/Users/aaronmeurer/anaconda'], 'LINK': ['python-3.3.2-0', 'readline-6.2-0 /Users/aaronmeurer/anaconda/pkgs 1', 'sqlite-3.7.13-0 /Users/aaronmeurer/anaconda/pkgs 1', 'tk-8.5.13-0 /Users/aaronmeurer/anaconda/pkgs 1', 'zlib-1.2.7-0 /Users/aaronmeurer/anaconda/pkgs 1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ python: 3.3.2-0 \n\
+ readline: 6.2-0 \n\
+ sqlite: 3.7.13-0
+ tk: 8.5.13-0
+ zlib: 1.2.7-0 \n\
+
+"""
+
+ actions['UNLINK'] = actions['LINK']
+ actions['LINK'] = []
+
+ with captured() as c:
+ display_actions(actions, index)
+
+
+ assert c.stdout == """
+The following packages will be REMOVED:
+
+ python: 3.3.2-0 \n\
+ readline: 6.2-0 \n\
+ sqlite: 3.7.13-0
+ tk: 8.5.13-0
+ zlib: 1.2.7-0 \n\
+
+"""
+
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0'], 'UNLINK':
+ ['cython-0.19-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 --> 0.19.1-py33_0
+
+"""
+
+ actions['LINK'], actions['UNLINK'] = actions['UNLINK'], actions['LINK']
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 --> 0.19-py33_0
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0',
+ 'dateutil-1.5-py33_0', 'numpy-1.7.1-py33_0'], 'UNLINK':
+ ['cython-0.19-py33_0', 'dateutil-2.1-py33_1', 'pip-1.3.1-py33_1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ numpy: 1.7.1-py33_0 \n\
+
+The following packages will be REMOVED:
+
+ pip: 1.3.1-py33_1
+
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 --> 0.19.1-py33_0
+
+The following packages will be DOWNGRADED:
+
+ dateutil: 2.1-py33_1 --> 1.5-py33_0 \n\
+
+"""
+
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0',
+ 'dateutil-2.1-py33_1'], 'UNLINK': ['cython-0.19-py33_0',
+ 'dateutil-1.5-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 --> 0.19.1-py33_0
+ dateutil: 1.5-py33_0 --> 2.1-py33_1 \n\
+
+"""
+
+ actions['LINK'], actions['UNLINK'] = actions['UNLINK'], actions['LINK']
+
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 --> 0.19-py33_0
+ dateutil: 2.1-py33_1 --> 1.5-py33_0 \n\
+
+"""
+
+
+
+def test_display_actions_show_channel_urls():
+ conda.config.show_channel_urls = True
+ actions = defaultdict(list, {"FETCH": ['sympy-0.7.2-py27_0',
+ "numpy-1.7.1-py27_0"]})
+ # The older test index doesn't have the size metadata
+ index['sympy-0.7.2-py27_0.tar.bz2']['size'] = 4374752
+ index["numpy-1.7.1-py27_0.tar.bz2"]['size'] = 5994338
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be downloaded:
+
+ package | build
+ ---------------------------|-----------------
+ sympy-0.7.2 | py27_0 4.2 MB <unknown>
+ numpy-1.7.1 | py27_0 5.7 MB <unknown>
+ ------------------------------------------------------------
+ Total: 9.9 MB
+
+"""
+
+
+ actions = defaultdict(list, {'PREFIX':
+ '/Users/aaronmeurer/anaconda/envs/test', 'SYMLINK_CONDA':
+ ['/Users/aaronmeurer/anaconda'], 'LINK': ['python-3.3.2-0', 'readline-6.2-0 /Users/aaronmeurer/anaconda/pkgs 1', 'sqlite-3.7.13-0 /Users/aaronmeurer/anaconda/pkgs 1', 'tk-8.5.13-0 /Users/aaronmeurer/anaconda/pkgs 1', 'zlib-1.2.7-0 /Users/aaronmeurer/anaconda/pkgs 1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ python: 3.3.2-0 <unknown>
+ readline: 6.2-0 <unknown>
+ sqlite: 3.7.13-0 <unknown>
+ tk: 8.5.13-0 <unknown>
+ zlib: 1.2.7-0 <unknown>
+
+"""
+
+ actions['UNLINK'] = actions['LINK']
+ actions['LINK'] = []
+
+ with captured() as c:
+ display_actions(actions, index)
+
+
+ assert c.stdout == """
+The following packages will be REMOVED:
+
+ python: 3.3.2-0 <unknown>
+ readline: 6.2-0 <unknown>
+ sqlite: 3.7.13-0 <unknown>
+ tk: 8.5.13-0 <unknown>
+ zlib: 1.2.7-0 <unknown>
+
+"""
+
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0'], 'UNLINK':
+ ['cython-0.19-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 <unknown> --> 0.19.1-py33_0 <unknown>
+
+"""
+
+ actions['LINK'], actions['UNLINK'] = actions['UNLINK'], actions['LINK']
+
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 <unknown> --> 0.19-py33_0 <unknown>
+
+"""
+
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0',
+ 'dateutil-1.5-py33_0', 'numpy-1.7.1-py33_0'], 'UNLINK':
+ ['cython-0.19-py33_0', 'dateutil-2.1-py33_1', 'pip-1.3.1-py33_1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ numpy: 1.7.1-py33_0 <unknown>
+
+The following packages will be REMOVED:
+
+ pip: 1.3.1-py33_1 <unknown>
+
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 <unknown> --> 0.19.1-py33_0 <unknown>
+
+The following packages will be DOWNGRADED:
+
+ dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 <unknown>
+
+"""
+
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0',
+ 'dateutil-2.1-py33_1'], 'UNLINK': ['cython-0.19-py33_0',
+ 'dateutil-1.5-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 <unknown> --> 0.19.1-py33_0 <unknown>
+ dateutil: 1.5-py33_0 <unknown> --> 2.1-py33_1 <unknown>
+
+"""
+
+ actions['LINK'], actions['UNLINK'] = actions['UNLINK'], actions['LINK']
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 <unknown> --> 0.19-py33_0 <unknown>
+ dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 <unknown>
+
+"""
+
+ actions['LINK'], actions['UNLINK'] = actions['UNLINK'], actions['LINK']
+
+ index['cython-0.19.1-py33_0.tar.bz2']['channel'] = 'my_channel'
+ index['dateutil-1.5-py33_0.tar.bz2']['channel'] = 'my_channel'
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 <unknown> --> 0.19.1-py33_0 my_channel
+ dateutil: 1.5-py33_0 my_channel --> 2.1-py33_1 <unknown> \n\
+
+"""
+
+ actions['LINK'], actions['UNLINK'] = actions['UNLINK'], actions['LINK']
+
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 my_channel --> 0.19-py33_0 <unknown> \n\
+ dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 my_channel
+
+"""
+
+
+def test_display_actions_link_type():
+ conda.config.show_channel_urls = False
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 2', 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 2',
+ 'numpy-1.7.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 2', 'python-3.3.2-0 /Users/aaronmeurer/anaconda/pkgs 2', 'readline-6.2-0 /Users/aaronmeurer/anaconda/pkgs 2', 'sqlite-3.7.13-0 /Users/aaronmeurer/anaconda/pkgs 2', 'tk-8.5.13-0 /Users/aaronmeurer/anaconda/pkgs 2', 'zlib-1.2.7-0 /Users/aaronmeurer/anaconda/pkgs 2']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ cython: 0.19.1-py33_0 (soft-link)
+ dateutil: 1.5-py33_0 (soft-link)
+ numpy: 1.7.1-py33_0 (soft-link)
+ python: 3.3.2-0 (soft-link)
+ readline: 6.2-0 (soft-link)
+ sqlite: 3.7.13-0 (soft-link)
+ tk: 8.5.13-0 (soft-link)
+ zlib: 1.2.7-0 (soft-link)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 2',
+ 'dateutil-2.1-py33_1 /Users/aaronmeurer/anaconda/pkgs 2'], 'UNLINK': ['cython-0.19-py33_0',
+ 'dateutil-1.5-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 --> 0.19.1-py33_0 (soft-link)
+ dateutil: 1.5-py33_0 --> 2.1-py33_1 (soft-link)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19-py33_0 /Users/aaronmeurer/anaconda/pkgs 2',
+ 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 2'], 'UNLINK': ['cython-0.19.1-py33_0',
+ 'dateutil-2.1-py33_1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 --> 0.19-py33_0 (soft-link)
+ dateutil: 2.1-py33_1 --> 1.5-py33_0 (soft-link)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 1', 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 1',
+ 'numpy-1.7.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 1', 'python-3.3.2-0 /Users/aaronmeurer/anaconda/pkgs 1', 'readline-6.2-0 /Users/aaronmeurer/anaconda/pkgs 1', 'sqlite-3.7.13-0 /Users/aaronmeurer/anaconda/pkgs 1', 'tk-8.5.13-0 /Users/aaronmeurer/anaconda/pkgs 1', 'zlib-1.2.7-0 /Users/aaronmeurer/anaconda/pkgs 1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ cython: 0.19.1-py33_0
+ dateutil: 1.5-py33_0 \n\
+ numpy: 1.7.1-py33_0 \n\
+ python: 3.3.2-0 \n\
+ readline: 6.2-0 \n\
+ sqlite: 3.7.13-0 \n\
+ tk: 8.5.13-0 \n\
+ zlib: 1.2.7-0 \n\
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 1',
+ 'dateutil-2.1-py33_1 /Users/aaronmeurer/anaconda/pkgs 1'], 'UNLINK': ['cython-0.19-py33_0',
+ 'dateutil-1.5-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 --> 0.19.1-py33_0
+ dateutil: 1.5-py33_0 --> 2.1-py33_1 \n\
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19-py33_0 /Users/aaronmeurer/anaconda/pkgs 1',
+ 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 1'], 'UNLINK': ['cython-0.19.1-py33_0',
+ 'dateutil-2.1-py33_1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 --> 0.19-py33_0
+ dateutil: 2.1-py33_1 --> 1.5-py33_0 \n\
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 3', 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 3',
+ 'numpy-1.7.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 3', 'python-3.3.2-0 /Users/aaronmeurer/anaconda/pkgs 3', 'readline-6.2-0 /Users/aaronmeurer/anaconda/pkgs 3', 'sqlite-3.7.13-0 /Users/aaronmeurer/anaconda/pkgs 3', 'tk-8.5.13-0 /Users/aaronmeurer/anaconda/pkgs 3', 'zlib-1.2.7-0 /Users/aaronmeurer/anaconda/pkgs 3']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ cython: 0.19.1-py33_0 (copy)
+ dateutil: 1.5-py33_0 (copy)
+ numpy: 1.7.1-py33_0 (copy)
+ python: 3.3.2-0 (copy)
+ readline: 6.2-0 (copy)
+ sqlite: 3.7.13-0 (copy)
+ tk: 8.5.13-0 (copy)
+ zlib: 1.2.7-0 (copy)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 3',
+ 'dateutil-2.1-py33_1 /Users/aaronmeurer/anaconda/pkgs 3'], 'UNLINK': ['cython-0.19-py33_0',
+ 'dateutil-1.5-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 --> 0.19.1-py33_0 (copy)
+ dateutil: 1.5-py33_0 --> 2.1-py33_1 (copy)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19-py33_0 /Users/aaronmeurer/anaconda/pkgs 3',
+ 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 3'], 'UNLINK': ['cython-0.19.1-py33_0',
+ 'dateutil-2.1-py33_1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 --> 0.19-py33_0 (copy)
+ dateutil: 2.1-py33_1 --> 1.5-py33_0 (copy)
+
+"""
+
+ conda.config.show_channel_urls = True
+
+ index['cython-0.19.1-py33_0.tar.bz2']['channel'] = 'my_channel'
+ index['dateutil-1.5-py33_0.tar.bz2']['channel'] = 'my_channel'
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 3', 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 3',
+ 'numpy-1.7.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 3', 'python-3.3.2-0 /Users/aaronmeurer/anaconda/pkgs 3', 'readline-6.2-0 /Users/aaronmeurer/anaconda/pkgs 3', 'sqlite-3.7.13-0 /Users/aaronmeurer/anaconda/pkgs 3', 'tk-8.5.13-0 /Users/aaronmeurer/anaconda/pkgs 3', 'zlib-1.2.7-0 /Users/aaronmeurer/anaconda/pkgs 3']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ cython: 0.19.1-py33_0 my_channel (copy)
+ dateutil: 1.5-py33_0 my_channel (copy)
+ numpy: 1.7.1-py33_0 <unknown> (copy)
+ python: 3.3.2-0 <unknown> (copy)
+ readline: 6.2-0 <unknown> (copy)
+ sqlite: 3.7.13-0 <unknown> (copy)
+ tk: 8.5.13-0 <unknown> (copy)
+ zlib: 1.2.7-0 <unknown> (copy)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19.1-py33_0 /Users/aaronmeurer/anaconda/pkgs 3',
+ 'dateutil-2.1-py33_1 /Users/aaronmeurer/anaconda/pkgs 3'], 'UNLINK': ['cython-0.19-py33_0',
+ 'dateutil-1.5-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ cython: 0.19-py33_0 <unknown> --> 0.19.1-py33_0 my_channel (copy)
+ dateutil: 1.5-py33_0 my_channel --> 2.1-py33_1 <unknown> (copy)
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['cython-0.19-py33_0 /Users/aaronmeurer/anaconda/pkgs 3',
+ 'dateutil-1.5-py33_0 /Users/aaronmeurer/anaconda/pkgs 3'], 'UNLINK': ['cython-0.19.1-py33_0',
+ 'dateutil-2.1-py33_1']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ cython: 0.19.1-py33_0 my_channel --> 0.19-py33_0 <unknown> (copy)
+ dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 my_channel (copy)
+
+"""
+
+def test_display_actions_features():
+ conda.config.show_channel_urls = False
+
+ actions = defaultdict(list, {'LINK': ['numpy-1.7.1-py33_p0', 'cython-0.19-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ cython: 0.19-py33_0 \n\
+ numpy: 1.7.1-py33_p0 [mkl]
+
+"""
+
+ actions = defaultdict(list, {'UNLINK': ['numpy-1.7.1-py33_p0', 'cython-0.19-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be REMOVED:
+
+ cython: 0.19-py33_0 \n\
+ numpy: 1.7.1-py33_p0 [mkl]
+
+"""
+
+ actions = defaultdict(list, {'UNLINK': ['numpy-1.7.1-py33_p0'], 'LINK': ['numpy-1.7.0-py33_p0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ numpy: 1.7.1-py33_p0 [mkl] --> 1.7.0-py33_p0 [mkl]
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['numpy-1.7.1-py33_p0'], 'UNLINK': ['numpy-1.7.0-py33_p0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ numpy: 1.7.0-py33_p0 [mkl] --> 1.7.1-py33_p0 [mkl]
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['numpy-1.7.1-py33_p0'], 'UNLINK': ['numpy-1.7.1-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ # NB: Packages whose version do not changed are put in UPDATED
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ numpy: 1.7.1-py33_0 --> 1.7.1-py33_p0 [mkl]
+
+"""
+
+ actions = defaultdict(list, {'UNLINK': ['numpy-1.7.1-py33_p0'], 'LINK': ['numpy-1.7.1-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ numpy: 1.7.1-py33_p0 [mkl] --> 1.7.1-py33_0
+
+"""
+
+ conda.config.show_channel_urls = True
+
+ actions = defaultdict(list, {'LINK': ['numpy-1.7.1-py33_p0', 'cython-0.19-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following NEW packages will be INSTALLED:
+
+ cython: 0.19-py33_0 <unknown>
+ numpy: 1.7.1-py33_p0 <unknown> [mkl]
+
+"""
+
+
+ actions = defaultdict(list, {'UNLINK': ['numpy-1.7.1-py33_p0', 'cython-0.19-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be REMOVED:
+
+ cython: 0.19-py33_0 <unknown>
+ numpy: 1.7.1-py33_p0 <unknown> [mkl]
+
+"""
+
+ actions = defaultdict(list, {'UNLINK': ['numpy-1.7.1-py33_p0'], 'LINK': ['numpy-1.7.0-py33_p0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be DOWNGRADED:
+
+ numpy: 1.7.1-py33_p0 <unknown> [mkl] --> 1.7.0-py33_p0 <unknown> [mkl]
+
+"""
+
+ actions = defaultdict(list, {'LINK': ['numpy-1.7.1-py33_p0'], 'UNLINK': ['numpy-1.7.0-py33_p0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ numpy: 1.7.0-py33_p0 <unknown> [mkl] --> 1.7.1-py33_p0 <unknown> [mkl]
+
+"""
+
+
+ actions = defaultdict(list, {'LINK': ['numpy-1.7.1-py33_p0'], 'UNLINK': ['numpy-1.7.1-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ # NB: Packages whose version do not changed are put in UPDATED
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ numpy: 1.7.1-py33_0 <unknown> --> 1.7.1-py33_p0 <unknown> [mkl]
+
+"""
+
+ actions = defaultdict(list, {'UNLINK': ['numpy-1.7.1-py33_p0'], 'LINK': ['numpy-1.7.1-py33_0']})
+
+ with captured() as c:
+ display_actions(actions, index)
+
+ assert c.stdout == """
+The following packages will be UPDATED:
+
+ numpy: 1.7.1-py33_p0 <unknown> [mkl] --> 1.7.1-py33_0 <unknown>
+
+"""
| Make the conda install table easier to read
The table of what packages will be installed and removed is hard to read. For one thing, it's hard to tell easily what packages are not removed but just upgraded or downgraded. Also, the "link" terminology is confusing.
A suggestion by @jklowden:
```
$ conda update conda
Updating Anaconda environment at /usr/local/anaconda
The following packages will be downloaded:
conda-2.2.3-py27_0.tar.bz2
[http://repo.continuum.io/pkgs/free/osx-64/]
The following packages will be upgraded:
Old version Replace with
------------------------- -------------------------
conda-1.4.4 conda-2.2.3
```
> or, if you really want the build (I don't, it's not meaningful to the user)
```
package Old version New version
------------ ------------------ ------------------
conda 1.4.4, py27_0 2.2.3, py27_0
```
I think the build is meaningful as it tells you what Python version is being used. It also tells you if you are using mkl. And also some people might use the build string to put other information which may be useful to users.
<!---
@huboard:{"order":3.3142282405143226e-49,"custom_state":""}
-->
| 2014-04-11T20:19:51 | -1.0 |
|
conda/conda | 667 | conda__conda-667 | [
"666"
] | f2934aea3f32ac94907b742a800d82c1e08757fe | diff --git a/conda/resolve.py b/conda/resolve.py
--- a/conda/resolve.py
+++ b/conda/resolve.py
@@ -278,12 +278,25 @@ def all_deps(self, root_fn, max_only=False):
def add_dependents(fn1, max_only=False):
for ms in self.ms_depends(fn1):
+ found = False
+ notfound = []
for pkg2 in self.get_pkgs(ms, max_only=max_only):
if pkg2.fn in res:
+ found = True
continue
- res[pkg2.fn] = pkg2
- if ms.strictness < 3:
- add_dependents(pkg2.fn, max_only=max_only)
+ try:
+ if ms.strictness < 3:
+ add_dependents(pkg2.fn, max_only=max_only)
+ except NoPackagesFound as e:
+ if e.pkg not in notfound:
+ notfound.append(e.pkg)
+ else:
+ found = True
+ res[pkg2.fn] = pkg2
+
+ if not found:
+ raise NoPackagesFound("Could not find some dependencies "
+ "for %s: %s" % (ms, ', '.join(notfound)), str(ms))
add_dependents(root_fn, max_only=max_only)
return res
@@ -394,7 +407,7 @@ def get_dists(self, specs, max_only=False):
dists[pkg.fn] = pkg
found = True
if not found:
- raise NoPackagesFound("Could not find some dependencies for %s: %s" % (spec, ', '.join(notfound)), None)
+ raise NoPackagesFound("Could not find some dependencies for %s: %s" % (spec, ', '.join(notfound)), spec)
return dists
| diff --git a/tests/test_resolve.py b/tests/test_resolve.py
--- a/tests/test_resolve.py
+++ b/tests/test_resolve.py
@@ -696,6 +696,22 @@ def test_nonexistent_deps():
'requires': ['nose 1.2.1', 'python 3.3'],
'version': '1.1',
}
+ index2['anotherpackage-1.0-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'mypackage 1.1'],
+ 'name': 'anotherpackage',
+ 'requires': ['nose', 'mypackage 1.1'],
+ 'version': '1.0',
+ }
+ index2['anotherpackage-2.0-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'mypackage'],
+ 'name': 'anotherpackage',
+ 'requires': ['nose', 'mypackage'],
+ 'version': '2.0',
+ }
r = Resolve(index2)
assert set(r.find_matches(MatchSpec('mypackage'))) == {
@@ -772,6 +788,32 @@ def test_nonexistent_deps():
]
assert raises(NoPackagesFound, lambda: r.solve(['mypackage 1.0']))
+ assert r.solve(['anotherpackage 1.0']) == [
+ 'anotherpackage-1.0-py33_0.tar.bz2',
+ 'mypackage-1.1-py33_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ ]
+
+ assert r.solve(['anotherpackage']) == [
+ 'anotherpackage-2.0-py33_0.tar.bz2',
+ 'mypackage-1.1-py33_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ ]
+
# This time, the latest version is messed up
index3 = index.copy()
index3['mypackage-1.1-py33_0.tar.bz2'] = {
@@ -790,6 +832,22 @@ def test_nonexistent_deps():
'requires': ['nose 1.2.1', 'python 3.3'],
'version': '1.0',
}
+ index3['anotherpackage-1.0-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'mypackage 1.0'],
+ 'name': 'anotherpackage',
+ 'requires': ['nose', 'mypackage 1.0'],
+ 'version': '1.0',
+ }
+ index3['anotherpackage-2.0-py33_0.tar.bz2'] = {
+ 'build': 'py33_0',
+ 'build_number': 0,
+ 'depends': ['nose', 'mypackage'],
+ 'name': 'anotherpackage',
+ 'requires': ['nose', 'mypackage'],
+ 'version': '2.0',
+ }
r = Resolve(index3)
assert set(r.find_matches(MatchSpec('mypackage'))) == {
@@ -852,6 +910,35 @@ def test_nonexistent_deps():
]
assert raises(NoPackagesFound, lambda: r.solve(['mypackage 1.1']))
+
+ assert r.solve(['anotherpackage 1.0']) == [
+ 'anotherpackage-1.0-py33_0.tar.bz2',
+ 'mypackage-1.0-py33_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ ]
+
+ # If recursive checking is working correctly, this will give
+ # anotherpackage 2.0, not anotherpackage 1.0
+ assert r.solve(['anotherpackage']) == [
+ 'anotherpackage-2.0-py33_0.tar.bz2',
+ 'mypackage-1.0-py33_0.tar.bz2',
+ 'nose-1.3.0-py33_0.tar.bz2',
+ 'openssl-1.0.1c-0.tar.bz2',
+ 'python-3.3.2-0.tar.bz2',
+ 'readline-6.2-0.tar.bz2',
+ 'sqlite-3.7.13-0.tar.bz2',
+ 'system-5.8-1.tar.bz2',
+ 'tk-8.5.13-0.tar.bz2',
+ 'zlib-1.2.7-0.tar.bz2',
+ ]
+
def test_package_ordering():
sympy_071 = Package('sympy-0.7.1-py27_0.tar.bz2', r.index['sympy-0.7.1-py27_0.tar.bz2'])
sympy_072 = Package('sympy-0.7.2-py27_0.tar.bz2', r.index['sympy-0.7.2-py27_0.tar.bz2'])
| NoPackagesFound does not work correctly for missing recursive dependencies
| 2014-04-14T22:05:18 | -1.0 |
|
conda/conda | 1,807 | conda__conda-1807 | [
"1751"
] | f46c73c3cb6353a409449fdba08fdbd0856bdb35 | diff --git a/conda/cli/main_clean.py b/conda/cli/main_clean.py
--- a/conda/cli/main_clean.py
+++ b/conda/cli/main_clean.py
@@ -151,9 +151,13 @@ def rm_tarballs(args, pkgs_dirs, totalsize, verbose=True):
for pkgs_dir in pkgs_dirs:
for fn in pkgs_dirs[pkgs_dir]:
- if verbose:
- print("removing %s" % fn)
- os.unlink(os.path.join(pkgs_dir, fn))
+ if os.access(os.path.join(pkgs_dir, fn), os.W_OK):
+ if verbose:
+ print("Removing %s" % fn)
+ os.unlink(os.path.join(pkgs_dir, fn))
+ else:
+ if verbose:
+ print("WARNING: cannot remove, file permissions: %s" % fn)
def find_pkgs():
diff --git a/conda/install.py b/conda/install.py
--- a/conda/install.py
+++ b/conda/install.py
@@ -138,6 +138,13 @@ def _remove_readonly(func, path, excinfo):
os.chmod(path, stat.S_IWRITE)
func(path)
+def warn_failed_remove(function, path, exc_info):
+ if exc_info[1].errno == errno.EACCES:
+ log.warn("Cannot remove, permission denied: {0}".format(path))
+ elif exc_info[1].errno == errno.ENOTEMPTY:
+ log.warn("Cannot remove, not empty: {0}".format(path))
+ else:
+ log.warn("Cannot remove, unknown reason: {0}".format(path))
def rm_rf(path, max_retries=5, trash=True):
"""
@@ -152,12 +159,15 @@ def rm_rf(path, max_retries=5, trash=True):
# Note that we have to check if the destination is a link because
# exists('/path/to/dead-link') will return False, although
# islink('/path/to/dead-link') is True.
- os.unlink(path)
+ if os.access(path, os.W_OK):
+ os.unlink(path)
+ else:
+ log.warn("Cannot remove, permission denied: {0}".format(path))
elif isdir(path):
for i in range(max_retries):
try:
- shutil.rmtree(path)
+ shutil.rmtree(path, ignore_errors=False, onerror=warn_failed_remove)
return
except OSError as e:
msg = "Unable to delete %s\n%s\n" % (path, e)
@@ -189,7 +199,7 @@ def rm_rf(path, max_retries=5, trash=True):
log.debug(msg + "Retrying after %s seconds..." % i)
time.sleep(i)
# Final time. pass exceptions to caller.
- shutil.rmtree(path)
+ shutil.rmtree(path, ignore_errors=False, onerror=warn_failed_remove)
def rm_empty_dir(path):
"""
| diff --git a/tests/test_install.py b/tests/test_install.py
--- a/tests/test_install.py
+++ b/tests/test_install.py
@@ -8,7 +8,7 @@
from conda import install
-from conda.install import PaddingError, binary_replace, update_prefix
+from conda.install import PaddingError, binary_replace, update_prefix, warn_failed_remove
from .decorators import skip_if_no_mock
from .helpers import mock
@@ -140,6 +140,11 @@ def generate_mock_isfile(self, value):
with patch.object(install, 'isfile', return_value=value) as isfile:
yield isfile
+ @contextmanager
+ def generate_mock_os_access(self, value):
+ with patch.object(install.os, 'access', return_value=value) as os_access:
+ yield os_access
+
@contextmanager
def generate_mock_unlink(self):
with patch.object(install.os, 'unlink') as unlink:
@@ -173,25 +178,27 @@ def generate_mock_check_call(self):
yield check_call
@contextmanager
- def generate_mocks(self, islink=True, isfile=True, isdir=True, on_win=False):
+ def generate_mocks(self, islink=True, isfile=True, isdir=True, on_win=False, os_access=True):
with self.generate_mock_islink(islink) as mock_islink:
with self.generate_mock_isfile(isfile) as mock_isfile:
- with self.generate_mock_isdir(isdir) as mock_isdir:
- with self.generate_mock_unlink() as mock_unlink:
- with self.generate_mock_rmtree() as mock_rmtree:
- with self.generate_mock_sleep() as mock_sleep:
- with self.generate_mock_log() as mock_log:
- with self.generate_mock_on_win(on_win):
- with self.generate_mock_check_call() as check_call:
- yield {
- 'islink': mock_islink,
- 'isfile': mock_isfile,
- 'isdir': mock_isdir,
- 'unlink': mock_unlink,
- 'rmtree': mock_rmtree,
- 'sleep': mock_sleep,
- 'log': mock_log,
- 'check_call': check_call,
+ with self.generate_mock_os_access(os_access) as mock_os_access:
+ with self.generate_mock_isdir(isdir) as mock_isdir:
+ with self.generate_mock_unlink() as mock_unlink:
+ with self.generate_mock_rmtree() as mock_rmtree:
+ with self.generate_mock_sleep() as mock_sleep:
+ with self.generate_mock_log() as mock_log:
+ with self.generate_mock_on_win(on_win):
+ with self.generate_mock_check_call() as check_call:
+ yield {
+ 'islink': mock_islink,
+ 'isfile': mock_isfile,
+ 'isdir': mock_isdir,
+ 'os_access': mock_os_access,
+ 'unlink': mock_unlink,
+ 'rmtree': mock_rmtree,
+ 'sleep': mock_sleep,
+ 'log': mock_log,
+ 'check_call': check_call,
}
def generate_directory_mocks(self, on_win=False):
@@ -219,6 +226,13 @@ def test_calls_unlink_on_true_islink(self):
install.rm_rf(some_path)
mocks['unlink'].assert_called_with(some_path)
+ @skip_if_no_mock
+ def test_does_not_call_unlink_on_os_access_false(self):
+ with self.generate_mocks(os_access=False) as mocks:
+ some_path = self.generate_random_path
+ install.rm_rf(some_path)
+ self.assertFalse(mocks['unlink'].called)
+
@skip_if_no_mock
def test_does_not_call_isfile_if_islink_is_true(self):
with self.generate_mocks() as mocks:
@@ -259,7 +273,8 @@ def test_calls_rmtree_at_least_once_on_isdir_true(self):
with self.generate_directory_mocks() as mocks:
some_path = self.generate_random_path
install.rm_rf(some_path)
- mocks['rmtree'].assert_called_with(some_path)
+ mocks['rmtree'].assert_called_with(
+ some_path, onerror=warn_failed_remove, ignore_errors=False)
@skip_if_no_mock
def test_calls_rmtree_only_once_on_success(self):
@@ -342,7 +357,7 @@ def test_tries_extra_kwarg_on_windows(self):
install.rm_rf(random_path)
expected_call_list = [
- mock.call(random_path),
+ mock.call(random_path, ignore_errors=False, onerror=warn_failed_remove),
mock.call(random_path, onerror=install._remove_readonly)
]
mocks['rmtree'].assert_has_calls(expected_call_list)
| conda clean -pt as non-root user with root anaconda install
I have installed root miniconda at /opt/anaconda. When running
```
conda clean -pt
```
as a lesser user than root, I am seeing errors indicating conda is not checking permissions before attempting to delete package dirs:
```
conda clean -pt
Cache location: /opt/anaconda/pkgs
Will remove the following tarballs:
/opt/anaconda/pkgs
------------------
conda-3.18.3-py27_0.tar.bz2 175 KB
conda-env-2.4.4-py27_0.tar.bz2 24 KB
itsdangerous-0.24-py27_0.tar.bz2 16 KB
markupsafe-0.23-py27_0.tar.bz2 30 KB
flask-0.10.1-py27_1.tar.bz2 129 KB
jinja2-2.8-py27_0.tar.bz2 263 KB
anaconda-build-0.12.0-py27_0.tar.bz2 69 KB
flask-wtf-0.8.4-py27_1.tar.bz2 12 KB
flask-ldap-login-0.3.0-py27_1.tar.bz2 13 KB
---------------------------------------------------
Total: 730 KB
removing conda-3.18.3-py27_0.tar.bz2
An unexpected error has occurred, please consider sending the
following traceback to the conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Include the output of the command 'conda info' in your report.
Traceback (most recent call last):
File "/opt/anaconda/envs/anaconda.org/bin/conda", line 5, in <module>
sys.exit(main())
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main.py", line 195, in main
args_func(args, p)
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main.py", line 202, in args_func
args.func(args, p)
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main_clean.py", line 331, in execute
rm_tarballs(args, pkgs_dirs, totalsize, verbose=not args.json)
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main_clean.py", line 156, in rm_tarballs
os.unlink(os.path.join(pkgs_dir, fn))
OSError: [Errno 13] Permission denied: '/opt/anaconda/pkgs/conda-3.18.3-py27_0.tar.bz2'
```
| Hi @csoja - this issue is blocking some key Build System stability fixes. LMK if this can be prioritized (along with #1752 above)
@stephenakearns and @PeterDSteinberg and @csoja this issue is also now preventing us from moving forward with the anaconda-cluster build scripts.
@csoja - I know you're strapped for resources and may have a new person taking a look at this. Can we bring it up in the platform meeting to discuss a possible solution
| 2015-11-11T20:20:17 | -1.0 |
conda/conda | 1,808 | conda__conda-1808 | [
"1752"
] | f46c73c3cb6353a409449fdba08fdbd0856bdb35 | diff --git a/conda/cli/main_clean.py b/conda/cli/main_clean.py
--- a/conda/cli/main_clean.py
+++ b/conda/cli/main_clean.py
@@ -151,9 +151,13 @@ def rm_tarballs(args, pkgs_dirs, totalsize, verbose=True):
for pkgs_dir in pkgs_dirs:
for fn in pkgs_dirs[pkgs_dir]:
- if verbose:
- print("removing %s" % fn)
- os.unlink(os.path.join(pkgs_dir, fn))
+ if os.access(os.path.join(pkgs_dir, fn), os.W_OK):
+ if verbose:
+ print("Removing %s" % fn)
+ os.unlink(os.path.join(pkgs_dir, fn))
+ else:
+ if verbose:
+ print("WARNING: cannot remove, file permissions: %s" % fn)
def find_pkgs():
@@ -163,6 +167,9 @@ def find_pkgs():
pkgs_dirs = defaultdict(list)
for pkgs_dir in config.pkgs_dirs:
+ if not os.path.exists(pkgs_dir):
+ print("WARNING: {0} does not exist".format(pkgs_dir))
+ continue
pkgs = [i for i in listdir(pkgs_dir) if isdir(join(pkgs_dir, i)) and
# Only include actual packages
isdir(join(pkgs_dir, i, 'info'))]
diff --git a/conda/install.py b/conda/install.py
--- a/conda/install.py
+++ b/conda/install.py
@@ -138,6 +138,13 @@ def _remove_readonly(func, path, excinfo):
os.chmod(path, stat.S_IWRITE)
func(path)
+def warn_failed_remove(function, path, exc_info):
+ if exc_info[1].errno == errno.EACCES:
+ log.warn( "WARNING: cannot remove, permission denied: %s" % path )
+ elif exc_info[1].errno == errno.ENOTEMPTY:
+ log.warn( "WARNING: cannot remove, not empty: %s" % path )
+ else:
+ log.warn( "WARNING: cannot remove, unknown reason: %s" % path )
def rm_rf(path, max_retries=5, trash=True):
"""
@@ -152,12 +159,15 @@ def rm_rf(path, max_retries=5, trash=True):
# Note that we have to check if the destination is a link because
# exists('/path/to/dead-link') will return False, although
# islink('/path/to/dead-link') is True.
- os.unlink(path)
+ if os.access(path, os.W_OK):
+ os.unlink(path)
+ else:
+ log.warn("WARNING: cannot remove, permission denied: %s" % path)
elif isdir(path):
for i in range(max_retries):
try:
- shutil.rmtree(path)
+ shutil.rmtree(path, ignore_errors=False, onerror=warn_failed_remove)
return
except OSError as e:
msg = "Unable to delete %s\n%s\n" % (path, e)
@@ -189,7 +199,7 @@ def rm_rf(path, max_retries=5, trash=True):
log.debug(msg + "Retrying after %s seconds..." % i)
time.sleep(i)
# Final time. pass exceptions to caller.
- shutil.rmtree(path)
+ shutil.rmtree(path, ignore_errors=False, onerror=warn_failed_remove)
def rm_empty_dir(path):
"""
| diff --git a/tests/test_install.py b/tests/test_install.py
--- a/tests/test_install.py
+++ b/tests/test_install.py
@@ -8,7 +8,7 @@
from conda import install
-from conda.install import PaddingError, binary_replace, update_prefix
+from conda.install import PaddingError, binary_replace, update_prefix, warn_failed_remove
from .decorators import skip_if_no_mock
from .helpers import mock
@@ -140,6 +140,11 @@ def generate_mock_isfile(self, value):
with patch.object(install, 'isfile', return_value=value) as isfile:
yield isfile
+ @contextmanager
+ def generate_mock_os_access(self, value):
+ with patch.object(install.os, 'access', return_value=value) as os_access:
+ yield os_access
+
@contextmanager
def generate_mock_unlink(self):
with patch.object(install.os, 'unlink') as unlink:
@@ -173,25 +178,27 @@ def generate_mock_check_call(self):
yield check_call
@contextmanager
- def generate_mocks(self, islink=True, isfile=True, isdir=True, on_win=False):
+ def generate_mocks(self, islink=True, isfile=True, isdir=True, on_win=False, os_access=True):
with self.generate_mock_islink(islink) as mock_islink:
with self.generate_mock_isfile(isfile) as mock_isfile:
- with self.generate_mock_isdir(isdir) as mock_isdir:
- with self.generate_mock_unlink() as mock_unlink:
- with self.generate_mock_rmtree() as mock_rmtree:
- with self.generate_mock_sleep() as mock_sleep:
- with self.generate_mock_log() as mock_log:
- with self.generate_mock_on_win(on_win):
- with self.generate_mock_check_call() as check_call:
- yield {
- 'islink': mock_islink,
- 'isfile': mock_isfile,
- 'isdir': mock_isdir,
- 'unlink': mock_unlink,
- 'rmtree': mock_rmtree,
- 'sleep': mock_sleep,
- 'log': mock_log,
- 'check_call': check_call,
+ with self.generate_mock_os_access(os_access) as mock_os_access:
+ with self.generate_mock_isdir(isdir) as mock_isdir:
+ with self.generate_mock_unlink() as mock_unlink:
+ with self.generate_mock_rmtree() as mock_rmtree:
+ with self.generate_mock_sleep() as mock_sleep:
+ with self.generate_mock_log() as mock_log:
+ with self.generate_mock_on_win(on_win):
+ with self.generate_mock_check_call() as check_call:
+ yield {
+ 'islink': mock_islink,
+ 'isfile': mock_isfile,
+ 'isdir': mock_isdir,
+ 'os_access': mock_os_access,
+ 'unlink': mock_unlink,
+ 'rmtree': mock_rmtree,
+ 'sleep': mock_sleep,
+ 'log': mock_log,
+ 'check_call': check_call,
}
def generate_directory_mocks(self, on_win=False):
@@ -219,6 +226,13 @@ def test_calls_unlink_on_true_islink(self):
install.rm_rf(some_path)
mocks['unlink'].assert_called_with(some_path)
+ @skip_if_no_mock
+ def test_does_not_call_unlink_on_os_access_false(self):
+ with self.generate_mocks(os_access=False) as mocks:
+ some_path = self.generate_random_path
+ install.rm_rf(some_path)
+ self.assertFalse(mocks['unlink'].called)
+
@skip_if_no_mock
def test_does_not_call_isfile_if_islink_is_true(self):
with self.generate_mocks() as mocks:
@@ -259,7 +273,8 @@ def test_calls_rmtree_at_least_once_on_isdir_true(self):
with self.generate_directory_mocks() as mocks:
some_path = self.generate_random_path
install.rm_rf(some_path)
- mocks['rmtree'].assert_called_with(some_path)
+ mocks['rmtree'].assert_called_with(
+ some_path, onerror=warn_failed_remove, ignore_errors=False)
@skip_if_no_mock
def test_calls_rmtree_only_once_on_success(self):
@@ -342,7 +357,7 @@ def test_tries_extra_kwarg_on_windows(self):
install.rm_rf(random_path)
expected_call_list = [
- mock.call(random_path),
+ mock.call(random_path, ignore_errors=False, onerror=warn_failed_remove),
mock.call(random_path, onerror=install._remove_readonly)
]
mocks['rmtree'].assert_has_calls(expected_call_list)
| conda clean -pt with empty package cache and non-root user
I have a root miniconda install at /opt/anaconda. I ran
```
conda clean -pt
```
successfully as root then immediately tried running the same command as a lesser user. I got this error even though the package cache was empty:
```
Cache location:
There are no tarballs to remove
An unexpected error has occurred, please consider sending the
following traceback to the conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Include the output of the command 'conda info' in your report.
Traceback (most recent call last):
File "/opt/anaconda/envs/anaconda.org/bin/conda", line 5, in <module>
sys.exit(main())
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main.py", line 195, in main
args_func(args, p)
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main.py", line 202, in args_func
args.func(args, p)
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main_clean.py", line 340, in execute
pkgs_dirs, warnings, totalsize, pkgsizes = find_pkgs()
File "/opt/anaconda/lib/python2.7/site-packages/conda/cli/main_clean.py", line 166, in find_pkgs
pkgs = [i for i in listdir(pkgs_dir) if isdir(join(pkgs_dir, i)) and
OSError: [Errno 2] No such file or directory: '/home/user5/envs/.pkgs'
```
| Appears somewhat related to #1751. Fixing #1751 and #1752 is necessary for the future needs of anaconda-build.
| 2015-11-11T21:17:30 | -1.0 |
conda/conda | 2,291 | conda__conda-2291 | [
"2284"
] | c3d54a1bd6a15dcea2ce900b0900c369bb848907 | diff --git a/conda/cli/activate.py b/conda/cli/activate.py
--- a/conda/cli/activate.py
+++ b/conda/cli/activate.py
@@ -124,10 +124,13 @@ def main():
if rootpath:
path = path.replace(translate_stream(rootpath, win_path_to_cygwin), "")
else:
- path = translate_stream(path, win_path_to_unix)
+ if sys.platform == 'win32':
+ path = translate_stream(path, win_path_to_unix)
+ if rootpath:
+ rootpath = translate_stream(rootpath, win_path_to_unix)
# Clear the root path if it is present
if rootpath:
- path = path.replace(translate_stream(rootpath, win_path_to_unix), "")
+ path = path.replace(rootpath, "")
elif sys.argv[1] == '..deactivate':
diff --git a/conda/install.py b/conda/install.py
--- a/conda/install.py
+++ b/conda/install.py
@@ -66,11 +66,12 @@ def win_path_to_unix(path, root_prefix=""):
Does not add cygdrive. If you need that, set root_prefix to "/cygdrive"
"""
- import re
- path_re = '[a-zA-Z]:[/\\\\]+(?:[^:*?"<>|]+[\/\\\\]+)*[^:*?"<>|;/\\\\]*'
- converted_paths = [root_prefix + "/" + _path.replace("\\", "/").replace(":", "")
- for _path in re.findall(path_re, path)]
- return ":".join(converted_paths)
+ path_re = '(?<![:/^a-zA-Z])([a-zA-Z]:[\/\\\\]+(?:[^:*?"<>|]+[\/\\\\]+)*[^:*?"<>|;\/\\\\]+?(?![a-zA-Z]:))'
+ translation = lambda found_path: root_prefix + "/" + found_path.groups()[0].replace("\\", "/")\
+ .replace(":", "")
+ translation = re.sub(path_re, translation, path)
+ translation = translation.replace(";/", ":/")
+ return translation
on_win = bool(sys.platform == "win32")
diff --git a/conda/utils.py b/conda/utils.py
--- a/conda/utils.py
+++ b/conda/utils.py
@@ -81,10 +81,12 @@ def win_path_to_unix(path, root_prefix=""):
Does not add cygdrive. If you need that, set root_prefix to "/cygdrive"
"""
- path_re = '(?<![:/])([a-zA-Z]:[\/\\\\]+(?:[^:*?"<>|]+[\/\\\\]+)*[^:*?"<>|;\/\\\\]+?(?![a-zA-Z]:))'
+ path_re = '(?<![:/^a-zA-Z])([a-zA-Z]:[\/\\\\]+(?:[^:*?"<>|]+[\/\\\\]+)*[^:*?"<>|;\/\\\\]+?(?![a-zA-Z]:))'
translation = lambda found_path: root_prefix + "/" + found_path.groups()[0].replace("\\", "/")\
- .replace(":", "").replace(";", ":")
- return re.sub(path_re, translation, path)
+ .replace(":", "")
+ translation = re.sub(path_re, translation, path)
+ translation = translation.replace(";/", ":/")
+ return translation
def unix_path_to_win(path, root_prefix=""):
@@ -100,7 +102,7 @@ def unix_path_to_win(path, root_prefix=""):
translation = lambda found_path: found_path.group(0)[len(root_prefix)+1] + ":" + \
found_path.group(0)[len(root_prefix)+2:].replace("/", "\\")
translation = re.sub(path_re, translation, path)
- translation = re.sub(":([a-zA-Z]):", lambda match: ";" + match.group(0)[1] + ":", translation)
+ translation = re.sub(":?([a-zA-Z]):\\\\", lambda match: ";" + match.group(0)[1] + ":\\", translation)
return translation
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -226,12 +226,12 @@ def _format_vars(shell):
def test_path_translation():
- test_cygwin_path = "/usr/bin:/cygdrive/z/documents (x86)/code/conda/tests/envskhkzts/test1:/cygdrive/z/documents/code/conda/tests/envskhkzts/test1/cmd"
- test_unix_path = "/usr/bin:/z/documents (x86)/code/conda/tests/envskhkzts/test1:/z/documents/code/conda/tests/envskhkzts/test1/cmd"
- test_win_path = "z:\\documents (x86)\\code\\conda\\tests\\envskhkzts\\test1;z:\\documents\\code\\conda\\tests\\envskhkzts\\test1\\cmd"
+ test_cygwin_path = "test dummy text /usr/bin:/cygdrive/z/documents (x86)/code/conda/tests/envskhkzts/test1:/cygdrive/z/documents/code/conda/tests/envskhkzts/test1/cmd more dummy text"
+ test_unix_path = "test dummy text /usr/bin:/z/documents (x86)/code/conda/tests/envskhkzts/test1:/z/documents/code/conda/tests/envskhkzts/test1/cmd more dummy text"
+ test_win_path = "test dummy text /usr/bin;z:\\documents (x86)\\code\\conda\\tests\\envskhkzts\\test1;z:\\documents\\code\\conda\\tests\\envskhkzts\\test1\\cmd more dummy text"
assert_equals(test_win_path, unix_path_to_win(test_unix_path))
- assert_equals(test_unix_path.replace("/usr/bin:", ""), win_path_to_unix(test_win_path))
- assert_equals(test_cygwin_path.replace("/usr/bin:", ""), win_path_to_cygwin(test_win_path))
+ assert_equals(test_unix_path, win_path_to_unix(test_win_path))
+ assert_equals(test_cygwin_path, win_path_to_cygwin(test_win_path))
assert_equals(test_win_path, cygwin_path_to_win(test_cygwin_path))
| activate on master is broken in OSX
@msarahan I'm on master, and I get:
```
$ source activate koo
path:usage: conda [-h] [-V] [--debug] command ...
conda: error: argument command: invalid choice: '..changeps1' (choose from 'info', 'help', 'list', 'search', 'create', 'install', 'update', 'upgrade', 'remove', 'uninstall', 'run', 'config', 'init', 'clean', 'package', 'bundle')
prepending /Users/ilan/python/envs/koo and /Users/ilan/python/envs/koo/cmd and /Users/ilan/python/envs/koo/bin to PATH
-bash: awk: command not found
-bash: dirname: command not found
Traceback (most recent call last):
File "/Users/ilan/python/bin/conda", line 4, in <module>
from conda.cli.main import main
File "/Users/ilan/conda/conda/__init__.py", line 19, in <module>
__version__ = get_version(__file__, __name__)
File "/Users/ilan/python/lib/python2.7/site-packages/auxlib/packaging.py", line 93, in get_version
if is_git_repo(here):
File "/Users/ilan/python/lib/python2.7/site-packages/auxlib/packaging.py", line 70, in is_git_repo
return call(('git', 'rev-parse'), cwd=path) == 0
File "/Users/ilan/python/lib/python2.7/subprocess.py", line 522, in call
return Popen(*popenargs, **kwargs).wait()
File "/Users/ilan/python/lib/python2.7/subprocess.py", line 710, in __init__
errread, errwrite)
File "/Users/ilan/python/lib/python2.7/subprocess.py", line 1335, in _execute_child
raise child_exception
```
It is true that the new activate scripts depend on `awk` and `dirname`?
| > the new activate scripts
The usage of awk and dirname are not new:
https://github.com/conda/conda-env/blame/develop/bin/activate#L32
That's conda-env, of course, but that's where the scripts that are now in conda came from.
Something else is going wrong. It might be a bug in the path translation stuff. It is supposed to just be a pass-through on *nix to *nix, but I did see some issues like this in fixing the tests. I'll look into it and see if I can improve the tests to catch whatever is happening here.
| 2016-03-19T19:56:18 | -1.0 |
conda/conda | 2,445 | conda__conda-2445 | [
"2444"
] | 33e1e45b8aee9df5377931619deacfb250be3986 | diff --git a/conda/cli/main_info.py b/conda/cli/main_info.py
--- a/conda/cli/main_info.py
+++ b/conda/cli/main_info.py
@@ -148,7 +148,7 @@ def execute(args, parser):
import conda.config as config
from conda.resolve import Resolve
from conda.cli.main_init import is_initialized
- from conda.api import get_index, get_package_versions
+ from conda.api import get_index
if args.root:
if args.json:
@@ -158,21 +158,19 @@ def execute(args, parser):
return
if args.packages:
- if args.json:
- results = defaultdict(list)
- for arg in args.packages:
- for pkg in get_package_versions(arg):
- results[arg].append(pkg._asdict())
- common.stdout_json(results)
- return
index = get_index()
r = Resolve(index)
- specs = map(common.arg2spec, args.packages)
-
- for spec in specs:
- versions = r.get_pkgs(spec)
- for pkg in sorted(versions):
- pretty_package(pkg)
+ if args.json:
+ common.stdout_json({
+ package: [p._asdict()
+ for p in sorted(r.get_pkgs(common.arg2spec(package)))]
+ for package in args.packages
+ })
+ else:
+ for package in args.packages:
+ versions = r.get_pkgs(common.arg2spec(package))
+ for pkg in sorted(versions):
+ pretty_package(pkg)
return
options = 'envs', 'system', 'license'
| diff --git a/tests/test_info.py b/tests/test_info.py
--- a/tests/test_info.py
+++ b/tests/test_info.py
@@ -1,6 +1,8 @@
from __future__ import print_function, absolute_import, division
+import json
from conda import config
+from conda.cli import main_info
from tests.helpers import run_conda_command, assert_in, assert_equals
@@ -32,3 +34,21 @@ def test_info():
assert_in(conda_info_out, conda_info_all_out)
assert_in(conda_info_e_out, conda_info_all_out)
assert_in(conda_info_s_out, conda_info_all_out)
+
+
+def test_info_package_json():
+ out, err = run_conda_command("info", "--json", "numpy=1.11.0=py35_0")
+ assert err == ""
+
+ out = json.loads(out)
+ assert set(out.keys()) == {"numpy=1.11.0=py35_0"}
+ assert len(out["numpy=1.11.0=py35_0"]) == 1
+ assert isinstance(out["numpy=1.11.0=py35_0"], list)
+
+ out, err = run_conda_command("info", "--json", "numpy")
+ assert err == ""
+
+ out = json.loads(out)
+ assert set(out.keys()) == {"numpy"}
+ assert len(out["numpy"]) > 1
+ assert isinstance(out["numpy"], list)
| conda info --json and package lookup
If you set the `--json` flag for `conda info` when searching for packages, you sometimes get nothing:
``` bash
$ conda info numpy=1.11.0=py35_0
Fetching package metadata: ....
numpy 1.11.0 py35_0
-------------------
file name : numpy-1.11.0-py35_0.tar.bz2
name : numpy
version : 1.11.0
build number: 0
build string: py35_0
channel : defaults
size : 6.1 MB
date : 2016-03-28
license : BSD
md5 : 1900998c19c5e310687013f95374bba2
installed environments:
dependencies:
mkl 11.3.1
python 3.5*
$ conda info --json numpy=1.11.0=py35_0
{}
```
Things work fine for `conda info --json numpy`, so it's something with the spec format.
conda info:
```
platform : linux-64
conda version : 4.0.5
conda-build version : not installed
python version : 2.7.11.final.0
requests version : 2.9.1
root environment : /opt/conda (writable)
default environment : /opt/conda
envs directories : /opt/conda/envs
package cache : /opt/conda/pkgs
channel URLs : https://repo.continuum.io/pkgs/free/linux-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/linux-64/
https://repo.continuum.io/pkgs/pro/noarch/
config file : None
is foreign system : False
```
| 2016-05-08T06:59:02 | -1.0 |
|
conda/conda | 2,529 | conda__conda-2529 | [
"2527"
] | ff8d814b6a8b075e8a2c4bab5b23691b21d0e902 | diff --git a/conda/install.py b/conda/install.py
--- a/conda/install.py
+++ b/conda/install.py
@@ -85,10 +85,9 @@ def remove_binstar_tokens(url):
# A simpler version of url_channel will do
def url_channel(url):
- return None, 'defaults'
+ return url.rsplit('/', 2)[0] + '/' if url and '/' in url else None, 'defaults'
- # We don't use the package cache or trash logic in the installer
- pkgs_dirs = []
+ pkgs_dirs = [join(sys.prefix, 'pkgs')]
on_win = bool(sys.platform == "win32")
@@ -423,13 +422,14 @@ def create_meta(prefix, dist, info_dir, extra_info):
meta = json.load(fi)
# add extra info, add to our intenral cache
meta.update(extra_info)
+ if 'url' not in meta:
+ meta['url'] = read_url(dist)
# write into <env>/conda-meta/<dist>.json
meta_dir = join(prefix, 'conda-meta')
if not isdir(meta_dir):
os.makedirs(meta_dir)
with open(join(meta_dir, _dist2filename(dist, '.json')), 'w') as fo:
json.dump(meta, fo, indent=2, sort_keys=True)
- # only update the package cache if it is loaded for this prefix.
if prefix in linked_data_:
load_linked_data(prefix, dist, meta)
@@ -821,12 +821,15 @@ def load_linked_data(prefix, dist, rec=None):
rec = json.load(fi)
except IOError:
return None
- _, schannel = url_channel(rec.get('url'))
else:
linked_data(prefix)
+ url = rec.get('url')
+ channel, schannel = url_channel(url)
+ if 'fn' not in rec:
+ rec['fn'] = url.rsplit('/', 1)[-1] if url else dname + '.tar.bz2'
+ rec['channel'] = channel
rec['schannel'] = schannel
cprefix = '' if schannel == 'defaults' else schannel + '::'
- rec['fn'] = dname + '.tar.bz2'
linked_data_[prefix][str(cprefix + dname)] = rec
return rec
@@ -1159,9 +1162,9 @@ def main():
prefix = opts.prefix
pkgs_dir = join(prefix, 'pkgs')
+ pkgs_dirs[0] = [pkgs_dir]
if opts.verbose:
print("prefix: %r" % prefix)
- pkgs_dirs.append(pkgs_dir)
if opts.file:
idists = list(yield_lines(join(prefix, opts.file)))
| diff --git a/tests/test_install.py b/tests/test_install.py
--- a/tests/test_install.py
+++ b/tests/test_install.py
@@ -448,5 +448,20 @@ def test_misc(self):
self.assertEqual(duplicates_to_remove(li, [d1, d2]), [])
+def test_standalone_import():
+ import sys
+ import conda.install
+ tmp_dir = tempfile.mkdtemp()
+ fname = conda.install.__file__.rstrip('co')
+ shutil.copyfile(fname, join(tmp_dir, basename(fname)))
+ opath = [tmp_dir]
+ opath.extend(s for s in sys.path if basename(s) not in ('conda', 'site-packages'))
+ opath, sys.path = sys.path, opath
+ try:
+ import install
+ finally:
+ sys.path = opath
+
+
if __name__ == '__main__':
unittest.main()
| Add test for conda/install.py imports
https://github.com/conda/conda/pull/2526#issuecomment-220751869
Since this type of bug was introduced by at least three people independently, I think it would be good to add a test for this. The test could be something along the lines of:
```
tmp_dir = tempfile.mkdtemp()
shutil.copyfile(conda.install.__file__, tmp_dir)
sys.path = [tmp_dir] # we also need the standard library here
import install
```
Basically, put the `install` module into an empty directory, and test if it can be imported by itself.
| Perhaps it would be sufficient to scan for `(from|import)\s+(conda|[.])`?
| 2016-05-21T21:58:02 | -1.0 |
conda/conda | 2,631 | conda__conda-2631 | [
"2626"
] | 38b4966d5c0cf16ebe310ce82ffef63c926f9f3f | diff --git a/conda/misc.py b/conda/misc.py
--- a/conda/misc.py
+++ b/conda/misc.py
@@ -9,7 +9,7 @@
import sys
from collections import defaultdict
from os.path import (abspath, dirname, expanduser, exists,
- isdir, isfile, islink, join, relpath)
+ isdir, isfile, islink, join, relpath, curdir)
from .install import (name_dist, linked as install_linked, is_fetched, is_extracted, is_linked,
linked_data, find_new_location, cached_url)
@@ -35,8 +35,8 @@ def conda_installed_files(prefix, exclude_self_build=False):
res.update(set(meta['files']))
return res
-
-url_pat = re.compile(r'(?P<url>.+)/(?P<fn>[^/#]+\.tar\.bz2)'
+url_pat = re.compile(r'(?:(?P<url_p>.+)(?:[/\\]))?'
+ r'(?P<fn>[^/\\#]+\.tar\.bz2)'
r'(:?#(?P<md5>[0-9a-f]{32}))?$')
def explicit(specs, prefix, verbose=False, force_extract=True, fetch_args=None):
actions = defaultdict(list)
@@ -55,12 +55,14 @@ def explicit(specs, prefix, verbose=False, force_extract=True, fetch_args=None):
m = url_pat.match(spec)
if m is None:
sys.exit('Could not parse explicit URL: %s' % spec)
- url, md5 = m.group('url') + '/' + m.group('fn'), m.group('md5')
- if not is_url(url):
- if not isfile(url):
- sys.exit('Error: file not found: %s' % url)
- url = utils_url_path(url)
- url_p, fn = url.rsplit('/', 1)
+ url_p, fn, md5 = m.group('url_p'), m.group('fn'), m.group('md5')
+ if not is_url(url_p):
+ if url_p is None:
+ url_p = curdir
+ elif not isdir(url_p):
+ sys.exit('Error: file not found: %s' % join(url_p, fn))
+ url_p = utils_url_path(url_p).rstrip('/')
+ url = "{0}/{1}".format(url_p, fn)
# See if the URL refers to a package in our cache
prefix = pkg_path = dir_path = None
| diff --git a/tests/test_misc.py b/tests/test_misc.py
--- a/tests/test_misc.py
+++ b/tests/test_misc.py
@@ -15,13 +15,13 @@ def test_cache_fn_url(self):
def test_url_pat_1(self):
m = url_pat.match('http://www.cont.io/pkgs/linux-64/foo.tar.bz2'
'#d6918b03927360aa1e57c0188dcb781b')
- self.assertEqual(m.group('url'), 'http://www.cont.io/pkgs/linux-64')
+ self.assertEqual(m.group('url_p'), 'http://www.cont.io/pkgs/linux-64')
self.assertEqual(m.group('fn'), 'foo.tar.bz2')
self.assertEqual(m.group('md5'), 'd6918b03927360aa1e57c0188dcb781b')
def test_url_pat_2(self):
m = url_pat.match('http://www.cont.io/pkgs/linux-64/foo.tar.bz2')
- self.assertEqual(m.group('url'), 'http://www.cont.io/pkgs/linux-64')
+ self.assertEqual(m.group('url_p'), 'http://www.cont.io/pkgs/linux-64')
self.assertEqual(m.group('fn'), 'foo.tar.bz2')
self.assertEqual(m.group('md5'), None)
| Installing packages from files broken in master
```
((p35)) Z:\msarahan\code\conda>conda install --force z:\msarahan\Downloads\numexpr-2.6.0-np110py35_0.tar.bz2
Could not parse explicit URL: z:\msarahan\Downloads\numexpr-2.6.0-np110py35_0.tar.bz2
((p35)) Z:\msarahan\code\conda>conda install --offline z:\msarahan\Downloads\numexpr-2.6.0-np110py35_0.tar.bz2
Could not parse explicit URL: z:\msarahan\Downloads\numexpr-2.6.0-np110py35_0.tar.bz2
((p35)) Z:\msarahan\code\conda>conda install --force ..\..\Downloads\numexpr-2.6.0-np110py35_0.tar.bz2
Could not parse explicit URL: ..\..\Downloads\numexpr-2.6.0-np110py35_0.tar.bz2
```
z: is a mapped network drive - not sure if that makes any difference.
| Looks like this only affects windows. Perhaps the `\`. Will dig further.
https://github.com/conda/conda/blob/master/conda/misc.py#L57
Ok so the regex [here](https://github.com/conda/conda/blob/master/conda/misc.py#L39) needs to be more robust for backslashes I guess?
| 2016-06-09T05:42:02 | -1.0 |
conda/conda | 2,729 | conda__conda-2729 | [
"2642"
] | 07e517865bbb98e333a0ba0d217fc5f60c444aeb | diff --git a/conda/config.py b/conda/config.py
--- a/conda/config.py
+++ b/conda/config.py
@@ -195,7 +195,9 @@ def get_rc_urls():
return rc['channels']
def is_url(url):
- return url and urlparse.urlparse(url).scheme != ""
+ if url:
+ p = urlparse.urlparse(url)
+ return p.netloc != "" or p.scheme == "file"
def binstar_channel_alias(channel_alias):
if channel_alias.startswith('file:/'):
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -5,7 +5,7 @@
from glob import glob
import json
from logging import getLogger, Handler
-from os.path import exists, isdir, isfile, join, relpath
+from os.path import exists, isdir, isfile, join, relpath, basename
import os
from shlex import split
from shutil import rmtree, copyfile
@@ -24,7 +24,7 @@
from conda.cli.main_remove import configure_parser as remove_configure_parser
from conda.cli.main_update import configure_parser as update_configure_parser
from conda.config import pkgs_dirs, bits
-from conda.install import linked as install_linked, linked_data_
+from conda.install import linked as install_linked, linked_data_, dist2dirname
from conda.install import on_win
from conda.compat import PY3, TemporaryDirectory
@@ -63,37 +63,18 @@ def reenable_dotlog(handlers):
dotlogger.handlers = handlers
-@contextmanager
-def make_temp_env(*packages):
- prefix = make_temp_prefix()
- try:
- # try to clear any config that's been set by other tests
- config.rc = config.load_condarc('')
-
- p = conda_argparse.ArgumentParser()
- sub_parsers = p.add_subparsers(metavar='command', dest='cmd')
- create_configure_parser(sub_parsers)
-
- command = "create -y -q -p {0} {1}".format(escape_for_winpath(prefix), " ".join(packages))
-
- args = p.parse_args(split(command))
- args.func(args, p)
-
- yield prefix
- finally:
- rmtree(prefix, ignore_errors=True)
-
-
class Commands:
INSTALL = "install"
UPDATE = "update"
REMOVE = "remove"
+ CREATE = "create"
parser_config = {
Commands.INSTALL: install_configure_parser,
Commands.UPDATE: update_configure_parser,
Commands.REMOVE: remove_configure_parser,
+ Commands.CREATE: create_configure_parser,
}
@@ -102,23 +83,38 @@ def run_command(command, prefix, *arguments):
sub_parsers = p.add_subparsers(metavar='command', dest='cmd')
parser_config[command](sub_parsers)
- command = "{0} -y -q -p {1} {2}".format(command,
- escape_for_winpath(prefix),
- " ".join(arguments))
+ prefix = escape_for_winpath(prefix)
+ arguments = list(map(escape_for_winpath, arguments))
+ command = "{0} -y -q -p {1} {2}".format(command, prefix, " ".join(arguments))
args = p.parse_args(split(command))
args.func(args, p)
+@contextmanager
+def make_temp_env(*packages):
+ prefix = make_temp_prefix()
+ try:
+ # try to clear any config that's been set by other tests
+ config.rc = config.load_condarc('')
+ run_command(Commands.CREATE, prefix, *packages)
+ yield prefix
+ finally:
+ rmtree(prefix, ignore_errors=True)
+
+
def package_is_installed(prefix, package, exact=False):
+ packages = list(install_linked(prefix))
+ if '::' not in package:
+ packages = list(map(dist2dirname, packages))
if exact:
- return any(p == package for p in install_linked(prefix))
- return any(p.startswith(package) for p in install_linked(prefix))
+ return package in packages
+ return any(p.startswith(package) for p in packages)
def assert_package_is_installed(prefix, package, exact=False):
if not package_is_installed(prefix, package, exact):
- print([p for p in install_linked(prefix)])
+ print(list(install_linked(prefix)))
raise AssertionError("package {0} is not in prefix".format(package))
@@ -147,29 +143,29 @@ def test_create_install_update_remove(self):
assert not package_is_installed(prefix, 'flask-0.')
assert_package_is_installed(prefix, 'python-3')
- @pytest.mark.skipif(on_win, reason="windows tarball is broken still")
@pytest.mark.timeout(300)
def test_tarball_install_and_bad_metadata(self):
with make_temp_env("python flask=0.10.1") as prefix:
- assert_package_is_installed(prefix, 'flask-0.')
+ assert_package_is_installed(prefix, 'flask-0.10.1')
run_command(Commands.REMOVE, prefix, 'flask')
- assert not package_is_installed(prefix, 'flask-0.')
+ assert not package_is_installed(prefix, 'flask-0.10.1')
assert_package_is_installed(prefix, 'python')
# regression test for #2626
# install tarball with full path
flask_tar_file = glob(join(pkgs_dirs[0], 'flask-0.*.tar.bz2'))[-1]
- if not on_win:
- run_command(Commands.INSTALL, prefix, flask_tar_file)
- assert_package_is_installed(prefix, 'flask-0.')
+ tar_new_path = join(prefix, basename(flask_tar_file))
+ copyfile(flask_tar_file, tar_new_path)
+ run_command(Commands.INSTALL, prefix, tar_new_path)
+ assert_package_is_installed(prefix, 'flask-0')
- run_command(Commands.REMOVE, prefix, 'flask')
- assert not package_is_installed(prefix, 'flask-0.')
+ run_command(Commands.REMOVE, prefix, 'flask')
+ assert not package_is_installed(prefix, 'flask-0')
# regression test for #2626
# install tarball with relative path
- flask_tar_file = relpath(flask_tar_file)
- run_command(Commands.INSTALL, prefix, flask_tar_file)
+ tar_new_path = relpath(tar_new_path)
+ run_command(Commands.INSTALL, prefix, tar_new_path)
assert_package_is_installed(prefix, 'flask-0.')
# regression test for #2599
| tarball install windows
@msarahan @mingwandroid What _should_ the `file://` url format be on Windows?
```
________________________ IntegrationTests.test_python3 ________________________
Traceback (most recent call last):
File "C:\projects\conda\tests\test_create.py", line 146, in test_python3
run_command(Commands.INSTALL, prefix, flask_tar_file)
File "C:\projects\conda\tests\test_create.py", line 104, in run_command
args.func(args, p)
File "C:\projects\conda\conda\cli\main_install.py", line 62, in execute
install(args, parser, 'install')
File "C:\projects\conda\conda\cli\install.py", line 195, in install
explicit(args.packages, prefix, verbose=not args.quiet)
File "C:\projects\conda\conda\misc.py", line 111, in explicit
index.update(fetch_index(channels, **fetch_args))
File "C:\projects\conda\conda\fetch.py", line 266, in fetch_index
for url in iterkeys(channel_urls)]
File "C:\projects\conda\conda\fetch.py", line 67, in func
res = f(*args, **kwargs)
File "C:\projects\conda\conda\fetch.py", line 149, in fetch_repodata
raise RuntimeError(msg)
RuntimeError: Could not find URL: file:///C|/projects/conda/
---------------------------- Captured stdout call -----------------------------
```
The relevant lines to look at here are line 64 in `conda/misc.py`
```
url_p = utils_url_path(url_p).rstrip('/')
```
and line 147 in `conda/utils.py`
```
def url_path(path):
path = abspath(path)
if sys.platform == 'win32':
path = '/' + path.replace(':', '|').replace('\\', '/')
return 'file://%s' % path
```
Help here is definitely appreciated.
| Maybe this might be useful.
https://blogs.msdn.microsoft.com/ie/2006/12/06/file-uris-in-windows/
Python's (3.4+) pathlib module might give a hint
```
In [1]: import pathlib
In [2]: pathlib.Path(r"C:\projects\conda").as_uri()
Out[2]: 'file:///C:/projects/conda'
```
I'm unable to reproduce this, however maybe the code that interprets the file uri might also be relevant. This would include conda's `LocalFSAdapter()` in connection.py (https://github.com/conda/conda/blob/231e9db898b3d7720b49e9e2050a88be6978fd38/conda/connection.py#L205-L235) which makes a call to `url_to_path()` (https://github.com/conda/conda/blob/231e9db898b3d7720b49e9e2050a88be6978fd38/conda/connection.py#L238-L251). These functions seem to interpret conda's `file:///C|/projects/conda/` format correctly.
One final observation: line 64 in `misc.py` strips the rightmost `/`, yet the uri in the error message seems to still have one. Is that supposed to be happening?
Hi @groutr. thanks for looking at this and good to see you again! @kalefranz yeah, you need to keep the :
@mingwandroid no problem. I hope to keep active here as time permits.
| 2016-06-16T20:23:12 | -1.0 |
conda/conda | 2,734 | conda__conda-2734 | [
"2732"
] | 3c6a9b5827f255735993c433488429e5781d4658 | diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -13,7 +13,7 @@
from ..compat import string_types
from ..config import (rc_bool_keys, rc_string_keys, rc_list_keys, sys_rc_path,
user_rc_path, rc_other)
-from ..utils import yaml_load, yaml_dump
+from ..utils import yaml_load, yaml_dump, yaml_bool
descr = """
Modify configuration values in .condarc. This is modeled after the git
@@ -289,14 +289,14 @@ def execute_config(args, parser):
set_bools, set_strings = set(rc_bool_keys), set(rc_string_keys)
for key, item in args.set:
# Check key and value
- yamlitem = yaml_load(item)
if key in set_bools:
- if not isinstance(yamlitem, bool):
+ itemb = yaml_bool(item)
+ if itemb is None:
error_and_exit("Key: %s; %s is not a YAML boolean." % (key, item),
json=args.json, error_type="TypeError")
- rc_config[key] = yamlitem
+ rc_config[key] = itemb
elif key in set_strings:
- rc_config[key] = yamlitem
+ rc_config[key] = item
else:
error_and_exit("Error key must be one of %s, not %s" %
(', '.join(set_bools | set_strings), key), json=args.json,
diff --git a/conda/connection.py b/conda/connection.py
--- a/conda/connection.py
+++ b/conda/connection.py
@@ -22,7 +22,7 @@
from . import __version__ as VERSION
from .compat import urlparse, StringIO
from .config import platform as config_platform, ssl_verify, get_proxy_servers
-from .utils import gnu_get_libc_version
+from .utils import gnu_get_libc_version, yaml_bool
RETRIES = 3
@@ -110,7 +110,7 @@ def __init__(self, *args, **kwargs):
self.headers['User-Agent'] = user_agent
- self.verify = ssl_verify
+ self.verify = yaml_bool(ssl_verify, ssl_verify)
class NullAuth(requests.auth.AuthBase):
diff --git a/conda/utils.py b/conda/utils.py
--- a/conda/utils.py
+++ b/conda/utils.py
@@ -292,6 +292,20 @@ def get_yaml():
return yaml
+# Restores YAML 1.1 boolean flexibility.
+yaml_bool_ = {
+ 'true': True, 'yes': True, 'on': True,
+ 'false': False, 'no': False, 'off': False
+}
+def yaml_bool(s, passthrough=None):
+ if type(s) is bool:
+ return s
+ try:
+ return yaml_bool_.get(s.lower(), passthrough)
+ except AttributeError:
+ return passthrough
+
+
def yaml_load(filehandle):
yaml = get_yaml()
try:
| diff --git a/tests/test_config.py b/tests/test_config.py
--- a/tests/test_config.py
+++ b/tests/test_config.py
@@ -12,7 +12,7 @@
import pytest
import conda.config as config
-from conda.utils import get_yaml
+from conda.utils import get_yaml, yaml_bool
from tests.helpers import run_conda_command
@@ -441,20 +441,17 @@ def test_invalid_rc():
def test_config_set():
# Test the config set command
- # Make sure it accepts only boolean values for boolean keys and any value for string keys
+ # Make sure it accepts any YAML 1.1 boolean values
+ assert yaml_bool(True) is True
+ assert yaml_bool(False) is False
+ for str in ('yes', 'Yes', 'YES', 'on', 'On', 'ON',
+ 'off', 'Off', 'OFF', 'no', 'No', 'NO'):
+ with make_temp_condarc() as rc:
+ stdout, stderr = run_conda_command('config', '--file', rc,
+ '--set', 'always_yes', str)
+ assert stdout == ''
+ assert stderr == ''
- with make_temp_condarc() as rc:
- stdout, stderr = run_conda_command('config', '--file', rc,
- '--set', 'always_yes', 'yes')
-
- assert stdout == ''
- assert stderr == 'Error: Key: always_yes; yes is not a YAML boolean.'
-
- stdout, stderr = run_conda_command('config', '--file', rc,
- '--set', 'always_yes', 'no')
-
- assert stdout == ''
- assert stderr == 'Error: Key: always_yes; no is not a YAML boolean.'
def test_set_rc_string():
# Test setting string keys in .condarc
| conda config --set show_channel_urls yes doesn't work anymore
This is happening since the latest conda update:
``` bat
λ conda config --set show_channel_urls yes
Error: Key: show_channel_urls; yes is not a YAML boolean.
```
It happens with both conda 4.1.1 (local windows py 3.5) and 4.1.0 (appveyor, https://ci.appveyor.com/project/mdboom/matplotlib/build/1.0.1774) and it worked with 4.0.8 (https://ci.appveyor.com/project/mdboom/matplotlib/build/1.0.1765/job/bkldg98f8p087xmf)
| This one is CC @mcg1969
I didn't mess with the section of code. I don't mind fixing it, don't get me wrong, but I am guessing that this is a difference between our old YAML library and our new one
Try using true/false instead of yes/no... @msarahan?
Ahhhh, interesting. I thought I had tested for that. But we can change
the ruamel_yaml version back to using yaml 1.1 (if it isn't already), and
then yes/no should work for yaml booleans.
On Thu, Jun 16, 2016 at 5:33 PM, Michael C. Grant notifications@github.com
wrote:
> Try using true/false instead of yes/no... @msarahan
> https://github.com/msarahan?
>
> —
> You are receiving this because you commented.
> Reply to this email directly, view it on GitHub
> https://github.com/conda/conda/issues/2732#issuecomment-226633230, or mute
> the thread
> https://github.com/notifications/unsubscribe/ABWks12m0vAh-7R3sO7oVkpihlF1YwIhks5qMc8tgaJpZM4I33Ze
> .
##
_Kale J. Franz, PhD_
_Conda Tech Lead_
_kfranz@continuum.io kfranz@continuum.io_
_@kalefranz https://twitter.com/kalefranz_
http://continuum.io/ http://continuum.io/
http://continuum.io/
221 W 6th St | Suite 1550 | Austin, TX 78701
I think the ship is sailed on the new library. I think we have to work around it
Looks like YAML 1.2 drops Yes/No and On/Off support: http://yaml.readthedocs.io/en/latest/pyyaml.html
Good news, we can fix
Changing `version="1.2"` to `version="1.1"` on line 298 of `utils.py` restores this behavior. I'm concerned we may be breaking other things though by doing this, so my inclination is to create a `yaml_bool` function to wrap around the output of `yaml.load` in cases like this.
Even better: drop the use of `yaml.load` to parse the boolean strings in `cli/main_config.py` in the first place.
| 2016-06-16T23:29:56 | -1.0 |
conda/conda | 2,862 | conda__conda-2862 | [
"2845"
] | b332659482ea5e3b3596dbe89f338f9a7e750d30 | diff --git a/conda/fetch.py b/conda/fetch.py
--- a/conda/fetch.py
+++ b/conda/fetch.py
@@ -98,7 +98,7 @@ def fetch_repodata(url, cache_dir=None, use_cache=False, session=None):
if "_mod" in cache:
headers["If-Modified-Since"] = cache["_mod"]
- if 'repo.continuum.io' in url:
+ if 'repo.continuum.io' in url or url.startswith("file://"):
filename = 'repodata.json.bz2'
else:
headers['Accept-Encoding'] = 'gzip, deflate, compress, identity'
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -260,8 +260,8 @@ def test_tarball_install_and_bad_metadata(self):
os.makedirs(subchan)
tar_new_path = join(subchan, flask_fname)
copyfile(tar_old_path, tar_new_path)
- with open(join(subchan, 'repodata.json'), 'w') as f:
- f.write(json.dumps(repodata))
+ with bz2.BZ2File(join(subchan, 'repodata.json.bz2'), 'w') as f:
+ f.write(json.dumps(repodata).encode('utf-8'))
run_command(Commands.INSTALL, prefix, '-c', channel, 'flask')
assert_package_is_installed(prefix, channel + '::' + 'flask-')
| file:// URLs don't work anymore with conda 4.1.3
Conda 4.1.3 does not work anymore with **file://** URLs:
```
(E:\Anaconda3) C:\Windows\system32>conda update --override-channels --channel file:///A:/pkgs/free --all
Fetching package metadata ....Error: Could not find URL: file:///A:/pkgs/free/win-64/
```
But `A:\pkgs\free\win-64` really exists:
```
(E:\Anaconda3) C:\Windows\system32>dir A:\pkgs\free\win-64
Volume in drive A is Software
Volume Serial Number is 4546-3CD9
Directory of A:\pkgs\free\win-64
06/24/2016 12:31 AM <DIR> .
01/23/2016 06:27 PM <DIR> ..
06/24/2016 12:28 AM 259,605 repodata.json.bz2
07/07/2015 12:54 AM 85,764 argcomplete-0.9.0-py34_0.tar.bz2
```
Before upgrading from 4.0.8-py35_0 everything worked fine. The same happened to the Linux version.
| @mcg1969 I think I was going to write an integration test for this but obviously let it slip.
ARGH! No, actually, we have integration tests for file URLs and for file-based channels. This is madness! :-( @ciupicri, I apologize.
Hold on! @ciupicri, can you please create an _uncompressed_ `repodata.json` in that channel directory?
(You do recall, do you not, @kalefranz, that you removed the `bzip2` support from my test...)
:facepalm:
> On Jun 23, 2016, at 7:09 PM, Michael C. Grant notifications@github.com wrote:
>
> (You do recall, do you not, @kalefranz, that you removed the bzip2 support from my test...)
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub, or mute the thread.
Looks like we need to refer to `bzip2` for file:// base URLs. Should be a simple fix, not quite one-character :-)
@mcg1969, I've uncompresses the `repodata.json.bz2` that I had, and now conda fails at the next stage:
```
unicodecsv: 0.14.1-py35_0 --> 0.14.1-py35_0 file:///A:/pkgs/free
vs2015_runtime: 14.00.23026.0-0 --> 14.00.23026.0-0 file:///A:/pkgs/free
wheel: 0.29.0-py35_0 --> 0.29.0-py35_0 file:///A:/pkgs/free
Proceed ([y]/n)? y
DEBUG:conda.instructions: PREFIX('E:\\Anaconda3')
DEBUG:conda.instructions: PRINT('Fetching packages ...')
Fetching packages ...
INFO:print:Fetching packages ...
DEBUG:conda.instructions: FETCH('file:///A:/pkgs/free::libdynd-0.7.2-0')
DEBUG:requests.packages.urllib3.util.retry:Converted retries value: 3 -> Retry(total=3, connect=None, read=None, redirect=None)
DEBUG:conda.fetch:url='file:///A:/pkgs/free/win-64/libdynd-0.7.2-0.tar.bz2'
DEBUG:conda.fetch:HTTPError: 404 Client Error: None for url: file:///A:/pkgs/free/win-64/libdynd-0.7.2-0.tar.bz2: file:///A:/pkgs/free/win-64/libdynd-0.7.2-0.tar.bz2
Error: HTTPError: 404 Client Error: None for url: file:///A:/pkgs/free/win-64/libdynd-0.7.2-0.tar.bz2: file:///A:/pkgs/free/win-64/libdynd-0.7.2-0.tar.bz2
```
`conda list` show that I already have libdynd-0.7.2.
| 2016-06-24T22:48:06 | -1.0 |
conda/conda | 2,873 | conda__conda-2873 | [
"2754"
] | 895d23dd3c5154b149bdc5f57b1c1e33b3afdd71 | diff --git a/conda/egg_info.py b/conda/egg_info.py
--- a/conda/egg_info.py
+++ b/conda/egg_info.py
@@ -29,14 +29,15 @@ def get_site_packages_dir(installed_pkgs):
def get_egg_info_files(sp_dir):
for fn in os.listdir(sp_dir):
- if not fn.endswith(('.egg', '.egg-info')):
+ if not fn.endswith(('.egg', '.egg-info', '.dist-info')):
continue
path = join(sp_dir, fn)
if isfile(path):
yield path
elif isdir(path):
for path2 in [join(path, 'PKG-INFO'),
- join(path, 'EGG-INFO', 'PKG-INFO')]:
+ join(path, 'EGG-INFO', 'PKG-INFO'),
+ join(path, 'METADATA')]:
if isfile(path2):
yield path2
@@ -54,7 +55,7 @@ def parse_egg_info(path):
key = m.group(1).lower()
info[key] = m.group(2)
try:
- return '%(name)s-%(version)s-<egg_info>' % info
+ return '%(name)s-%(version)s-<pip>' % info
except KeyError:
pass
return None
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -230,11 +230,21 @@ def test_create_install_update_remove(self):
@pytest.mark.timeout(300)
def test_list_with_pip_egg(self):
with make_temp_env("python=3 pip") as prefix:
- check_call(PYTHON_BINARY + " -m pip install --egg --no-use-wheel flask==0.10.1",
+ check_call(PYTHON_BINARY + " -m pip install --egg --no-binary flask flask==0.10.1",
cwd=prefix, shell=True)
stdout, stderr = run_command(Commands.LIST, prefix)
stdout_lines = stdout.split('\n')
- assert any(line.endswith("<egg_info>") for line in stdout_lines
+ assert any(line.endswith("<pip>") for line in stdout_lines
+ if line.lower().startswith("flask"))
+
+ @pytest.mark.timeout(300)
+ def test_list_with_pip_wheel(self):
+ with make_temp_env("python=3 pip") as prefix:
+ check_call(PYTHON_BINARY + " -m pip install flask==0.10.1",
+ cwd=prefix, shell=True)
+ stdout, stderr = run_command(Commands.LIST, prefix)
+ stdout_lines = stdout.split('\n')
+ assert any(line.endswith("<pip>") for line in stdout_lines
if line.lower().startswith("flask"))
@pytest.mark.timeout(300)
| conda list misses pip-installed wheels
As of conda 4.1, `conda list` no longer captures python packages that were pip-installed and were installed from wheels.
https://www.python.org/dev/peps/pep-0427/#id14
CC @ilanschnell
| This is actually a real issue now because the large majority of packages on PyPI are distributed as sdists, and now on install, pip force-compiles sdists to wheels, then installs those wheels.
How about we use something like this:
```
import pkgutil
packages = [p[1] for p in pkgutil.iter_modules()]
```
The problem is that from whatever `pkgutil.iter_modules()` returns, it is hard to tell whether or nor the installed package is a conda package or not. The point of the new `conda.egginfo` module, part from not having to call out to `pip`, is that conda knows which `.egg-info` files are "untracked" (not part of any conda package). I think the best solution is to extend `conda.egginfo` to handle these new meta-data files also.
| 2016-06-26T19:18:01 | -1.0 |
conda/conda | 2,875 | conda__conda-2875 | [
"2841"
] | 8d744a0fab207153da762d615a7e71342fe9a20f | diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -257,13 +257,19 @@ def execute_config(args, parser):
if isinstance(rc_config[key], (bool, string_types)):
print("--set", key, rc_config[key])
- else:
+ else: # assume the key is a list-type
# Note, since conda config --add prepends, these are printed in
# the reverse order so that entering them in this order will
# recreate the same file
- for item in reversed(rc_config.get(key, [])):
+ items = rc_config.get(key, [])
+ numitems = len(items)
+ for q, item in enumerate(reversed(items)):
# Use repr so that it can be pasted back in to conda config --add
- print("--add", key, repr(item))
+ if key == "channels" and q in (0, numitems-1):
+ print("--add", key, repr(item),
+ " # lowest priority" if q == 0 else " # highest priority")
+ else:
+ print("--add", key, repr(item))
# Add, append
for arg, prepend in zip((args.add, args.append), (True, False)):
| diff --git a/tests/test_config.py b/tests/test_config.py
--- a/tests/test_config.py
+++ b/tests/test_config.py
@@ -240,8 +240,8 @@ def test_config_command_get():
--set always_yes True
--set changeps1 False
--set channel_alias http://alpha.conda.anaconda.org
---add channels 'defaults'
---add channels 'test'
+--add channels 'defaults' # lowest priority
+--add channels 'test' # highest priority
--add create_default_packages 'numpy'
--add create_default_packages 'ipython'\
"""
@@ -251,8 +251,8 @@ def test_config_command_get():
'--get', 'channels')
assert stdout == """\
---add channels 'defaults'
---add channels 'test'\
+--add channels 'defaults' # lowest priority
+--add channels 'test' # highest priority\
"""
assert stderr == ""
@@ -269,8 +269,8 @@ def test_config_command_get():
assert stdout == """\
--set changeps1 False
---add channels 'defaults'
---add channels 'test'\
+--add channels 'defaults' # lowest priority
+--add channels 'test' # highest priority\
"""
assert stderr == ""
@@ -326,12 +326,12 @@ def test_config_command_parser():
with make_temp_condarc(condarc) as rc:
stdout, stderr = run_conda_command('config', '--file', rc, '--get')
-
+ print(stdout)
assert stdout == """\
--set always_yes True
--set changeps1 False
---add channels 'defaults'
---add channels 'test'
+--add channels 'defaults' # lowest priority
+--add channels 'test' # highest priority
--add create_default_packages 'numpy'
--add create_default_packages 'ipython'\
"""
| Would be nice if conda config --get channels listed the channels in priority order
As far as I can tell it currently lists them in reverse order.
| Good idea!
Wow, I was about to change this, but the code has a note
```
# Note, since conda config --add prepends, these are printed in
# the reverse order so that entering them in this order will
# recreate the same file
```
It's a good point. And as implemented was intended to be a feature. I don't think this is a bug anymore. And if we change it, would have to go in 4.2.x instead of 4.1.x. I have to think more about it.
I think one problem is that `--add` prepends. It seems more natural to me that `--add` would append, and there should be a separate `--prepend` flag.
As a new feature (maybe not in 4.2.x), we should probably add both `--append` and `--prepend` flags. Keep the `--add` flag as-is for a while, but warn on use, and eventually change the behavior for `--add` from prepend to append.
Good news: we already have append and prepend.
As a stopgap, you could perhaps add `# lowest priority` and `# highest priority` to the first and last lines of the output?
> Good news: we already have append and prepend.
Oh perfect. I thought we had added one of them. Didn't remember if we put in both.
| 2016-06-26T21:50:18 | -1.0 |
conda/conda | 2,908 | conda__conda-2908 | [
"2886"
] | 767c0a9c06e8d37b06ad2a5afce8a25af1eac795 | diff --git a/conda/install.py b/conda/install.py
--- a/conda/install.py
+++ b/conda/install.py
@@ -41,7 +41,6 @@
import tarfile
import tempfile
import time
-import tempfile
import traceback
from os.path import (abspath, basename, dirname, isdir, isfile, islink,
join, normpath)
diff --git a/conda/misc.py b/conda/misc.py
--- a/conda/misc.py
+++ b/conda/misc.py
@@ -69,14 +69,18 @@ def explicit(specs, prefix, verbose=False, force_extract=True, fetch_args=None,
prefix = pkg_path = dir_path = None
if url.startswith('file://'):
prefix = cached_url(url)
+ if prefix is not None:
+ schannel = 'defaults' if prefix == '' else prefix[:-2]
+ is_file = False
# If not, determine the channel name from the URL
if prefix is None:
channel, schannel = url_channel(url)
+ is_file = schannel.startswith('file:') and schannel.endswith('/')
prefix = '' if schannel == 'defaults' else schannel + '::'
+
fn = prefix + fn
dist = fn[:-8]
- is_file = schannel.startswith('file:') and schannel.endswith('/')
# Add explicit file to index so we'll see it later
if is_file:
index[fn] = {'fn': dist2filename(fn), 'url': url, 'md5': None}
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -260,11 +260,32 @@ def test_tarball_install_and_bad_metadata(self):
assert not package_is_installed(prefix, 'flask-0.10.1')
assert_package_is_installed(prefix, 'python')
- # Regression test for 2812
- # install from local channel
from conda.config import pkgs_dirs
flask_fname = flask_data['fn']
tar_old_path = join(pkgs_dirs[0], flask_fname)
+
+ # regression test for #2886 (part 1 of 2)
+ # install tarball from package cache, default channel
+ run_command(Commands.INSTALL, prefix, tar_old_path)
+ assert_package_is_installed(prefix, 'flask-0.')
+
+ # regression test for #2626
+ # install tarball with full path, outside channel
+ tar_new_path = join(prefix, flask_fname)
+ copyfile(tar_old_path, tar_new_path)
+ run_command(Commands.INSTALL, prefix, tar_new_path)
+ assert_package_is_installed(prefix, 'flask-0')
+
+ # regression test for #2626
+ # install tarball with relative path, outside channel
+ run_command(Commands.REMOVE, prefix, 'flask')
+ assert not package_is_installed(prefix, 'flask-0.10.1')
+ tar_new_path = relpath(tar_new_path)
+ run_command(Commands.INSTALL, prefix, tar_new_path)
+ assert_package_is_installed(prefix, 'flask-0.')
+
+ # Regression test for 2812
+ # install from local channel
for field in ('url', 'channel', 'schannel'):
del flask_data[field]
repodata = {'info': {}, 'packages':{flask_fname: flask_data}}
@@ -279,21 +300,12 @@ def test_tarball_install_and_bad_metadata(self):
run_command(Commands.INSTALL, prefix, '-c', channel, 'flask')
assert_package_is_installed(prefix, channel + '::' + 'flask-')
- # regression test for #2626
- # install tarball with full path
- tar_new_path = join(prefix, flask_fname)
- copyfile(tar_old_path, tar_new_path)
- run_command(Commands.INSTALL, prefix, tar_new_path)
- assert_package_is_installed(prefix, 'flask-0')
-
+ # regression test for #2886 (part 2 of 2)
+ # install tarball from package cache, local channel
run_command(Commands.REMOVE, prefix, 'flask')
assert not package_is_installed(prefix, 'flask-0')
-
- # regression test for #2626
- # install tarball with relative path
- tar_new_path = relpath(tar_new_path)
- run_command(Commands.INSTALL, prefix, tar_new_path)
- assert_package_is_installed(prefix, 'flask-0.')
+ run_command(Commands.INSTALL, prefix, tar_old_path)
+ assert_package_is_installed(prefix, channel + '::' + 'flask-')
# regression test for #2599
linked_data_.clear()
| conda install from tarball error?
Running into this issue when trying to install directly from a tarball.
```
Traceback (most recent call last):
File "/usr/local/bin/conda2", line 6, in <module>
sys.exit(main())
An unexpected error has occurred, please consider sending the
following traceback to the conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Include the output of the command 'conda info' in your report.
File "/opt/conda2/lib/python2.7/site-packages/conda/cli/main.py", line 120, in main
exit_code = args_func(args, p)
File "/opt/conda2/lib/python2.7/site-packages/conda/cli/main.py", line 130, in args_func
exit_code = args.func(args, p)
File "/opt/conda2/lib/python2.7/site-packages/conda/cli/main_install.py", line 69, in execute
install(args, parser, 'install')
File "/opt/conda2/lib/python2.7/site-packages/conda/cli/install.py", line 196, in install
explicit(args.packages, prefix, verbose=not args.quiet)
File "/opt/conda2/lib/python2.7/site-packages/conda/misc.py", line 79, in explicit
is_file = schannel.startswith('file:') and schannel.endswith('/')
UnboundLocalError: local variable 'schannel' referenced before assignment
```
| `conda info`?
This is in a docker image.
```
$ conda info
Current conda install:
platform : linux-64
conda version : 4.1.4
conda-env version : 2.5.1
conda-build version : 1.20.0
python version : 2.7.11.final.0
requests version : 2.9.2
root environment : /opt/conda2 (writable)
default environment : /opt/conda2
envs directories : /opt/conda2/envs
package cache : /opt/conda2/pkgs
channel URLs : https://conda.anaconda.org/nanshe/linux-64/
https://conda.anaconda.org/nanshe/noarch/
https://conda.anaconda.org/conda-forge/linux-64/
https://conda.anaconda.org/conda-forge/noarch/
https://repo.continuum.io/pkgs/free/linux-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/linux-64/
https://repo.continuum.io/pkgs/pro/noarch/
config file : /root/.condarc
offline mode : False
is foreign system : False
```
I'm having trouble reproducing this.
I know this should be obvious but can you give us the exact command line?
@jakirkham More details here would be helpful, including the specific package you tried to install by tarball. I'm genuinely having difficulty reproducing.
So, this is coming out of an open sourced Docker image. Though I really should come up with a simpler example. I started to and then other stuff came up. I'll give it another try.
Until I do here is the [Dockerfile](https://github.com/nanshe-org/docker_nanshe/blob/6fa60ad6f221731c17bf2277f5744f8b781095db/Dockerfile). Sorry it is so ugly. I've tried my best to make it readable given the constraints. The line that cause it to fail is this [one](https://github.com/nanshe-org/docker_nanshe/blob/6fa60ad6f221731c17bf2277f5744f8b781095db/Dockerfile#L29).
Basically, what happens is we install everything in the `root` environment of two different `conda`s. One for Python 2 and the other for Python 3. We then remove one package `nanshe` and download the source code matching that version so as to have the test suite. We then remove the package and run the test suite. Once complete we try to reinstall the package from a file which fails.
I was able to reproduce the problem---it is specifically limited to installing tarballs _in the package cache_. As a short-term fix you can copy the package out of the cache and then reinstall, I think. #2907 is the same issue. I'm working on a fix now. cc: @kalefranz
| 2016-06-29T04:29:30 | -1.0 |
conda/conda | 2,915 | conda__conda-2915 | [
"2681"
] | deaccea600d7b80cbcc939f018a5fdfe2a066967 | diff --git a/conda/egg_info.py b/conda/egg_info.py
--- a/conda/egg_info.py
+++ b/conda/egg_info.py
@@ -15,6 +15,7 @@
from .misc import rel_path
+
def get_site_packages_dir(installed_pkgs):
for info in itervalues(installed_pkgs):
if info['name'] == 'python':
diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -8,6 +8,3 @@ class InvalidInstruction(CondaException):
def __init__(self, instruction, *args, **kwargs):
msg = "No handler for instruction: %r" % instruction
super(InvalidInstruction, self).__init__(msg, *args, **kwargs)
-
-class LockError(RuntimeError, CondaException):
- pass
diff --git a/conda/lock.py b/conda/lock.py
--- a/conda/lock.py
+++ b/conda/lock.py
@@ -17,11 +17,11 @@
"""
from __future__ import absolute_import, division, print_function
-import logging
import os
-import time
-
-from .exceptions import LockError
+import logging
+from os.path import join
+import glob
+from time import sleep
LOCKFN = '.conda_lock'
@@ -33,13 +33,15 @@ class Locked(object):
"""
Context manager to handle locks.
"""
- def __init__(self, path, retries=10):
+ def __init__(self, path):
self.path = path
self.end = "-" + str(os.getpid())
- self.lock_path = os.path.join(self.path, LOCKFN + self.end)
- self.retries = retries
+ self.lock_path = join(self.path, LOCKFN + self.end)
+ self.pattern = join(self.path, LOCKFN + '-*')
+ self.remove = True
def __enter__(self):
+ retries = 10
# Keep the string "LOCKERROR" in this string so that external
# programs can look for it.
lockstr = ("""\
@@ -48,24 +50,33 @@ def __enter__(self):
If you are sure that conda is not running, remove it and try again.
You can also use: $ conda clean --lock\n""")
sleeptime = 1
-
- for _ in range(self.retries):
- if os.path.isdir(self.lock_path):
- stdoutlog.info(lockstr % self.lock_path)
+ files = None
+ while retries:
+ files = glob.glob(self.pattern)
+ if files and not files[0].endswith(self.end):
+ stdoutlog.info(lockstr % str(files))
stdoutlog.info("Sleeping for %s seconds\n" % sleeptime)
-
- time.sleep(sleeptime)
+ sleep(sleeptime)
sleeptime *= 2
+ retries -= 1
else:
- os.makedirs(self.lock_path)
- return self
+ break
+ else:
+ stdoutlog.error("Exceeded max retries, giving up")
+ raise RuntimeError(lockstr % str(files))
- stdoutlog.error("Exceeded max retries, giving up")
- raise LockError(lockstr % self.lock_path)
+ if not files:
+ try:
+ os.makedirs(self.lock_path)
+ except OSError:
+ pass
+ else: # PID lock already here --- someone else will remove it.
+ self.remove = False
def __exit__(self, exc_type, exc_value, traceback):
- try:
- os.rmdir(self.lock_path)
- os.rmdir(self.path)
- except OSError:
- pass
+ if self.remove:
+ for path in self.lock_path, self.path:
+ try:
+ os.rmdir(path)
+ except OSError:
+ pass
| diff --git a/tests/test_exceptions.py b/tests/test_exceptions.py
--- a/tests/test_exceptions.py
+++ b/tests/test_exceptions.py
@@ -18,7 +18,3 @@ def test_creates_message_with_instruction_name(self):
e = exceptions.InvalidInstruction(random_instruction)
expected = "No handler for instruction: %s" % random_instruction
self.assertEqual(expected, str(e))
-
-def test_lockerror_hierarchy():
- assert issubclass(exceptions.LockError, exceptions.CondaException)
- assert issubclass(exceptions.LockError, RuntimeError)
diff --git a/tests/test_lock.py b/tests/test_lock.py
deleted file mode 100644
--- a/tests/test_lock.py
+++ /dev/null
@@ -1,32 +0,0 @@
-import os.path
-import pytest
-
-from conda.lock import Locked, LockError
-
-
-def test_lock_passes(tmpdir):
- with Locked(tmpdir.strpath) as lock:
- path = os.path.basename(lock.lock_path)
- assert tmpdir.join(path).exists() and tmpdir.join(path).isdir()
-
- # lock should clean up after itself
- assert not tmpdir.join(path).exists()
- assert not tmpdir.exists()
-
-def test_lock_locks(tmpdir):
- with Locked(tmpdir.strpath) as lock1:
- path = os.path.basename(lock1.lock_path)
- assert tmpdir.join(path).exists() and tmpdir.join(path).isdir()
-
- with pytest.raises(LockError) as execinfo:
- with Locked(tmpdir.strpath, retries=1) as lock2:
- assert False # this should never happen
- assert lock2.lock_path == lock1.lock_path
- assert "LOCKERROR" in str(execinfo)
- assert "conda is already doing something" in str(execinfo)
-
- assert tmpdir.join(path).exists() and tmpdir.join(path).isdir()
-
- # lock should clean up after itself
- assert not tmpdir.join(path).exists()
- assert not tmpdir.exists()
| [Regression] Conda create environment fails on lock if root environment is not under user control
This issue is introduced in Conda 4.1.0 (Conda 4.0.8 works fine).
```
$ conda create -n root2 python=2 [123/1811]
Fetching package metadata .......
Solving package specifications .............
Package plan for installation in environment /home/frol/.conda/envs/root2:
The following NEW packages will be INSTALLED:
openssl: 1.0.2h-1 (soft-link)
pip: 8.1.2-py27_0 (soft-link)
python: 2.7.11-0 (soft-link)
readline: 6.2-2 (soft-link)
setuptools: 23.0.0-py27_0 (soft-link)
sqlite: 3.13.0-0 (soft-link)
tk: 8.5.18-0 (soft-link)
wheel: 0.29.0-py27_0 (soft-link)
zlib: 1.2.8-3 (soft-link)
Proceed ([y]/n)?
Linking packages ...
An unexpected error has occurred, please consider sending the
following traceback to the conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Include the output of the command 'conda info' in your report.
Traceback (most recent call last):
File "/usr/local/miniconda/bin/conda", line 6, in <module>
sys.exit(main())
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/main.py", line 120, in main
args_func(args, p)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/main.py", line 127, in args_func
args.func(args, p)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/main_create.py", line 57, in execute
install(args, parser, 'create')
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/install.py", line 407, in install
execute_actions(actions, index, verbose=not args.quiet)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/plan.py", line 566, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/instructions.py", line 137, in execute_instructions
cmd(state, arg)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/instructions.py", line 80, in LINK_CMD
link(state['prefix'], dist, lt, index=state['index'], shortcuts=shortcuts)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/install.py", line 1035, in link
with Locked(prefix), Locked(pkgs_dir):
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/lock.py", line 60, in __enter__
os.makedirs(self.lock_path)
File "/usr/local/miniconda/lib/python2.7/os.py", line 157, in makedirs
mkdir(name, mode)
OSError: [Errno 13] Permission denied: '/usr/local/miniconda/pkgs/.conda_lock-949'
```
`/usr/local/miniconda/` is a system-wide installation of miniconda, so obviously, users cannot create lock files there.
P.S. I have a dream that updating conda software won't break things on every release...
| It seems that I cannot even do `source activate ...` as a regular user now. It just hangs. Here is what I get when I interrupt it with `^C`:
```
$ source activate root2
^CTraceback (most recent call last):
File "/usr/local/miniconda/bin/conda", line 6, in <module>
sys.exit(main())
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/main.py", line 48, in main
activate.main()
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/activate.py", line 121, in main
path = get_path(shelldict)
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/cli/activate.py", line 108, in get_path
return run_in(shelldict["printpath"], shelldict)[0]
File "/usr/local/miniconda/lib/python2.7/site-packages/conda/utils.py", line 174, in run_in
stdout, stderr = p.communicate()
File "/usr/local/miniconda/lib/python2.7/subprocess.py", line 799, in communicate
return self._communicate(input)
File "/usr/local/miniconda/lib/python2.7/subprocess.py", line 1409, in _communicate
stdout, stderr = self._communicate_with_poll(input)
File "/usr/local/miniconda/lib/python2.7/subprocess.py", line 1463, in _communicate_with_poll
ready = poller.poll()
KeyboardInterrupt
```
It also seems like there is no way to pin Conda version. Installing any package to the root env tries to update Conda to the latest version:
```
$ conda install python
Using Anaconda Cloud api site https://api.anaconda.org
Fetching package metadata: ....
Solving package specifications: .........
Package plan for installation in environment /usr/local/miniconda:
The following packages will be UPDATED:
conda: 4.0.8-py27_0 --> 4.1.0-py27_0
conda-env: 2.4.5-py27_0 --> 2.5.0-py27_0
Proceed ([y]/n)?
```
You can now pin 4.0.9.
```
conda install conda=4.0.9
conda config --set auto_update_conda false
```
`auto_update_conda` setting will be added to 4.1.1 also, which is coming out tonight or tomorrow morning.
Freaking lock on the whole package cache. We're going to get rid of that soon. I can't really tell what initially tripped here. What are the permissions on `/usr/local/miniconda/pkgs/.conda_lock-949`? Are they yours, or root, or does it even exist?
Ohhh I think I get it, from your issue title. I actually thought this was already broken anyway. I plan on having 4.2 out in a couple weeks now, and should be a simple fix at that point. Ok with staying on 4.0.9 for this use case until then?
@kalefranz It seems that I don't have options here but wait. I use Docker containers and this bug doesn't bother me that much. However, I would say that it is not a minor regression to postpone it to the next release. (From my experience, next release will break things in another way, so people will stuck with 4.0.*)
In the last several months our coverage has gone from ~48% to almost 70%. The code base is still far more fragile and brittle than I'd like it to be, but we're making progress I think.
Reverting #2320 fixed the regression. However, it seems that it just fails silently at locking, but at least it works in non-concurrent scenarios.
cc @alanhdu
@frol: Yeah, that's about right. Before #2320, conda would just swallow all `OsError`s (including `PermissionError`s).
For now, we could add a `try ... except` around https://github.com/conda/conda/blob/master/conda/lock.py#L60, catch a `PermissionError` (or whatever the equivalent Python 2 error is), and try to do something smart (or just silently fail... depends how important that lock actually is).
I am seeing this issue as well @kalefranz
After reading this, I thought I would be able to downgrade conda to 4.0.9. However after doing that, I am still unable to activate centrally administered conda environments as a user. Is there a prior version of conda you would reccommend? Or did the 4.1.3 version leave something in my miniconda install that is causing the problem? Do I need to re-install miniconda2 from scratch? Should I just wait for this to be fixed before proceeding with trying to build a central conda install for our users?
@davidslac There are two options you may try:
1. Downgrade conda-env together with conda:
``` bash
$ conda install conda=4.0.9 'conda-env<2.5'
```
2. Patch (revert changes) conda lock in Conda 4.1.x:
``` bash
$ curl -o "/usr/local/miniconda/lib/python"*"/site-packages/conda/lock.py" \
"https://raw.githubusercontent.com/conda/conda/9428ad0b76be55e8070e04dd577c96e7dab571e0/conda/lock.py"
```
Thanks! I tried both, but it sill did not work. It's probably something
I'm overlooking on my part - after the first failure I deleted the
lock.pyc, but still no luck.
I'll just hope for a fix in the future. I suspect though, that central
administration is not as standard of a use case, so one is more likely
to run into problems. It may be we should just provide a channel of our
packages to our users and let them administer their own software stacks,
give them some environments we know work.
best,
David
On 06/27/16 10:58, Vlad Frolov wrote:
> @davidslac https://github.com/davidslac There are two options you
> may try:
>
> 1.
>
> ```
> Downgrade conda-env:
>
> $ conda install'conda-env<2.5'
> ```
>
> 2.
>
> ```
> Patch (revert changes) conda lock:
>
> $ curl -o"/usr/local/miniconda/lib/python"*"/site-packages/conda/lock.py" \
> "https://raw.githubusercontent.com/conda/conda/9428ad0b76be55e8070e04dd577c96e7dab571e0/conda/lock.py"
> ```
| 2016-06-29T17:16:25 | -1.0 |
conda/conda | 3,041 | conda__conda-3041 | [
"3036"
] | 0b4b690e6a3e1b5562307b4bda29f2f7cdbb4632 | diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -151,21 +151,19 @@ def configure_parser(sub_parsers):
choices=BoolOrListKey()
)
action.add_argument(
- "--add",
+ "--append", "--add",
nargs=2,
action="append",
- help="""Add one configuration value to the beginning of a list key.
- To add to the end of the list, use --append.""",
+ help="""Add one configuration value to the end of a list key.""",
default=[],
choices=ListKey(),
metavar=('KEY', 'VALUE'),
)
action.add_argument(
- "--append",
+ "--prepend",
nargs=2,
action="append",
- help="""Add one configuration value to a list key. The default
- behavior is to prepend.""",
+ help="""Add one configuration value to the beginning of a list key.""",
default=[],
choices=ListKey(),
metavar=('KEY', 'VALUE'),
@@ -260,7 +258,7 @@ def execute_config(args, parser):
# recreate the same file
items = rc_config.get(key, [])
numitems = len(items)
- for q, item in enumerate(reversed(items)):
+ for q, item in enumerate(items):
# Use repr so that it can be pasted back in to conda config --add
if key == "channels" and q in (0, numitems-1):
print("--add", key, repr(item),
@@ -268,8 +266,8 @@ def execute_config(args, parser):
else:
print("--add", key, repr(item))
- # Add, append
- for arg, prepend in zip((args.add, args.append), (True, False)):
+ # prepend, append, add
+ for arg, prepend in zip((args.prepend, args.append), (True, False)):
for key, item in arg:
if key == 'channels' and key not in rc_config:
rc_config[key] = ['defaults']
@@ -287,7 +285,7 @@ def execute_config(args, parser):
if item in arglist:
# Right now, all list keys should not contain duplicates
message = "Warning: '%s' already in '%s' list, moving to the %s" % (
- item, key, "front" if prepend else "back")
+ item, key, "top" if prepend else "bottom")
arglist = rc_config[key] = [p for p in arglist if p != item]
if not args.json:
print(message, file=sys.stderr)
diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -197,6 +197,12 @@ def __init__(self, message, *args, **kwargs):
super(PackageNotFoundError, self).__init__(msg, *args, **kwargs)
+class CondaHTTPError(CondaError):
+ def __init__(self, message, *args, **kwargs):
+ msg = 'HTTP Error: %s\n' % message
+ super(CondaHTTPError, self).__init__(msg, *args, **kwargs)
+
+
class NoPackagesFoundError(CondaError, RuntimeError):
'''An exception to report that requested packages are missing.
@@ -352,7 +358,7 @@ def print_exception(exception):
def get_info():
- from StringIO import StringIO
+ from conda.compat import StringIO
from contextlib import contextmanager
from conda.cli import conda_argparse
from conda.cli.main_info import configure_parser
diff --git a/conda/fetch.py b/conda/fetch.py
--- a/conda/fetch.py
+++ b/conda/fetch.py
@@ -27,8 +27,8 @@
rm_rf, exp_backoff_fn)
from .lock import Locked as Locked
from .utils import memoized
-from .exceptions import ProxyError, ChannelNotAllowed, CondaRuntimeError, CondaSignatureError
-
+from .exceptions import ProxyError, ChannelNotAllowed, CondaRuntimeError, CondaSignatureError, \
+ CondaError, CondaHTTPError
log = getLogger(__name__)
dotlog = getLogger('dotupdate')
@@ -159,7 +159,7 @@ def fetch_repodata(url, cache_dir=None, use_cache=False, session=None):
msg = "HTTPError: %s: %s\n" % (e, remove_binstar_tokens(url))
log.debug(msg)
- raise CondaRuntimeError(msg)
+ raise CondaHTTPError(msg)
except requests.exceptions.SSLError as e:
msg = "SSL Error: %s\n" % e
| diff --git a/tests/test_config.py b/tests/test_config.py
--- a/tests/test_config.py
+++ b/tests/test_config.py
@@ -303,19 +303,19 @@ def test_config_command_basics():
assert stdout == stderr == ''
assert _read_test_condarc(rc) == """\
channels:
- - test
- defaults
+ - test
"""
with make_temp_condarc() as rc:
# When defaults is explicitly given, it should not be added
stdout, stderr = run_conda_command('config', '--file', rc, '--add',
'channels', 'test', '--add', 'channels', 'defaults')
assert stdout == ''
- assert stderr == "Warning: 'defaults' already in 'channels' list, moving to the front"
+ assert stderr == "Warning: 'defaults' already in 'channels' list, moving to the bottom"
assert _read_test_condarc(rc) == """\
channels:
- - defaults
- test
+ - defaults
"""
# Duplicate keys should not be added twice
with make_temp_condarc() as rc:
@@ -325,11 +325,11 @@ def test_config_command_basics():
stdout, stderr = run_conda_command('config', '--file', rc, '--add',
'channels', 'test')
assert stdout == ''
- assert stderr == "Warning: 'test' already in 'channels' list, moving to the front"
+ assert stderr == "Warning: 'test' already in 'channels' list, moving to the bottom"
assert _read_test_condarc(rc) == """\
channels:
- - test
- defaults
+ - test
"""
# Test append
@@ -340,7 +340,7 @@ def test_config_command_basics():
stdout, stderr = run_conda_command('config', '--file', rc, '--append',
'channels', 'test')
assert stdout == ''
- assert stderr == "Warning: 'test' already in 'channels' list, moving to the back"
+ assert stderr == "Warning: 'test' already in 'channels' list, moving to the bottom"
assert _read_test_condarc(rc) == """\
channels:
- defaults
@@ -394,10 +394,10 @@ def test_config_command_get():
--set always_yes True
--set changeps1 False
--set channel_alias http://alpha.conda.anaconda.org
---add channels 'defaults' # lowest priority
---add channels 'test' # highest priority
---add create_default_packages 'numpy'
---add create_default_packages 'ipython'\
+--add channels 'test' # lowest priority
+--add channels 'defaults' # highest priority
+--add create_default_packages 'ipython'
+--add create_default_packages 'numpy'\
"""
assert stderr == "unknown key invalid_key"
@@ -405,8 +405,8 @@ def test_config_command_get():
'--get', 'channels')
assert stdout == """\
---add channels 'defaults' # lowest priority
---add channels 'test' # highest priority\
+--add channels 'test' # lowest priority
+--add channels 'defaults' # highest priority\
"""
assert stderr == ""
@@ -423,8 +423,8 @@ def test_config_command_get():
assert stdout == """\
--set changeps1 False
---add channels 'defaults' # lowest priority
---add channels 'test' # highest priority\
+--add channels 'test' # lowest priority
+--add channels 'defaults' # highest priority\
"""
assert stderr == ""
@@ -484,16 +484,23 @@ def test_config_command_parser():
assert stdout == """\
--set always_yes True
--set changeps1 False
---add channels 'defaults' # lowest priority
---add channels 'test' # highest priority
---add create_default_packages 'numpy'
---add create_default_packages 'ipython'\
+--add channels 'test' # lowest priority
+--add channels 'defaults' # highest priority
+--add create_default_packages 'ipython'
+--add create_default_packages 'numpy'\
"""
+ print(">>>>")
+ with open(rc, 'r') as fh:
+ print(fh.read())
- stdout, stderr = run_conda_command('config', '--file', rc, '--add',
- 'channels', 'mychannel')
+
+
+ stdout, stderr = run_conda_command('config', '--file', rc, '--prepend', 'channels', 'mychannel')
assert stdout == stderr == ''
+ with open(rc, 'r') as fh:
+ print(fh.read())
+
assert _read_test_condarc(rc) == """\
channels:
- mychannel
| conda config needs --prepend; change behavior of --add to --append
referencing https://github.com/conda/conda/issues/2841
- conda config needs `--prepend`
- change behavior of `--add` to `--append`
- un-reverse order of `conda config --get channels`
| 2016-07-11T22:03:41 | -1.0 |
|
conda/conda | 3,326 | conda__conda-3326 | [
"3307",
"3307"
] | 550d679e447b02781caeb348aa89370a62ffa400 | diff --git a/conda/lock.py b/conda/lock.py
--- a/conda/lock.py
+++ b/conda/lock.py
@@ -45,8 +45,11 @@ def touch(file_name, times=None):
Examples:
touch("hello_world.py")
"""
- with open(file_name, 'a'):
- os.utime(file_name, times)
+ try:
+ with open(file_name, 'a'):
+ os.utime(file_name, times)
+ except (OSError, IOError) as e:
+ log.warn("Failed to create lock, do not run conda in parallel process\n")
class FileLock(object):
@@ -111,11 +114,13 @@ def __init__(self, directory_path, retries=10):
self.lock_file_path = "%s.pid{0}.%s" % (lock_path_pre, LOCK_EXTENSION)
# e.g. if locking directory `/conda`, lock file will be `/conda/conda.pidXXXX.conda_lock`
self.lock_file_glob_str = "%s.pid*.%s" % (lock_path_pre, LOCK_EXTENSION)
+ # make sure '/' exists
assert isdir(dirname(self.directory_path)), "{0} doesn't exist".format(self.directory_path)
if not isdir(self.directory_path):
- os.makedirs(self.directory_path, exist_ok=True)
- log.debug("forced to create %s", self.directory_path)
- assert os.access(self.directory_path, os.W_OK), "%s not writable" % self.directory_path
-
+ try:
+ os.makedirs(self.directory_path)
+ log.debug("forced to create %s", self.directory_path)
+ except (OSError, IOError) as e:
+ log.warn("Failed to create directory %s" % self.directory_path)
Locked = DirectoryLock
| diff --git a/tests/test_lock.py b/tests/test_lock.py
--- a/tests/test_lock.py
+++ b/tests/test_lock.py
@@ -1,9 +1,12 @@
import pytest
-from os.path import basename, join
-from conda.lock import FileLock, LOCKSTR, LOCK_EXTENSION, LockError
-from conda.install import on_win
+from os.path import basename, join, exists, isfile
+from conda.lock import FileLock, DirectoryLock, LockError
+
def test_filelock_passes(tmpdir):
+ """
+ Normal test on file lock
+ """
package_name = "conda_file1"
tmpfile = join(tmpdir.strpath, package_name)
with FileLock(tmpfile) as lock:
@@ -15,7 +18,10 @@ def test_filelock_passes(tmpdir):
def test_filelock_locks(tmpdir):
-
+ """
+ Test on file lock, multiple lock on same file
+ Lock error should raised
+ """
package_name = "conda_file_2"
tmpfile = join(tmpdir.strpath, package_name)
with FileLock(tmpfile) as lock1:
@@ -27,48 +33,42 @@ def test_filelock_locks(tmpdir):
assert False # this should never happen
assert lock2.path_to_lock == lock1.path_to_lock
- if not on_win:
- assert "LOCKERROR" in str(execinfo.value)
- assert "conda is already doing something" in str(execinfo.value)
assert tmpdir.join(path).exists() and tmpdir.join(path).isfile()
# lock should clean up after itself
assert not tmpdir.join(path).exists()
-def test_filelock_folderlocks(tmpdir):
- import os
- package_name = "conda_file_2"
+def test_folder_locks(tmpdir):
+ """
+ Test on Directory lock
+ """
+ package_name = "dir_1"
tmpfile = join(tmpdir.strpath, package_name)
- os.makedirs(tmpfile)
- with FileLock(tmpfile) as lock1:
- path = basename(lock1.lock_file_path)
- assert tmpdir.join(path).exists() and tmpdir.join(path).isfile()
+ with DirectoryLock(tmpfile) as lock1:
+
+ assert exists(lock1.lock_file_path) and isfile(lock1.lock_file_path)
with pytest.raises(LockError) as execinfo:
- with FileLock(tmpfile, retries=1) as lock2:
+ with DirectoryLock(tmpfile, retries=1) as lock2:
assert False # this should never happen
- assert lock2.path_to_lock == lock1.path_to_lock
-
- if not on_win:
- assert "LOCKERROR" in str(execinfo.value)
- assert "conda is already doing something" in str(execinfo.value)
- assert lock1.path_to_lock in str(execinfo.value)
- assert tmpdir.join(path).exists() and tmpdir.join(path).isfile()
+ assert exists(lock1.lock_file_path) and isfile(lock1.lock_file_path)
# lock should clean up after itself
- assert not tmpdir.join(path).exists()
-
-
-def lock_thread(tmpdir, file_path):
- with FileLock(file_path) as lock1:
- path = basename(lock1.lock_file_path)
- assert tmpdir.join(path).exists() and tmpdir.join(path).isfile()
- assert not tmpdir.join(path).exists()
+ assert not exists(lock1.lock_file_path)
def test_lock_thread(tmpdir):
+ """
+ 2 thread want to lock a file
+ One thread will have LockError Raised
+ """
+ def lock_thread(tmpdir, file_path):
+ with FileLock(file_path) as lock1:
+ path = basename(lock1.lock_file_path)
+ assert tmpdir.join(path).exists() and tmpdir.join(path).isfile()
+ assert not tmpdir.join(path).exists()
from threading import Thread
package_name = "conda_file_3"
@@ -85,13 +85,17 @@ def test_lock_thread(tmpdir):
assert not tmpdir.join(path).exists()
-def lock_thread_retries(tmpdir, file_path):
- with pytest.raises(LockError) as execinfo:
- with FileLock(file_path, retries=0):
- assert False # should never enter here, since max_tires is 0
- assert "LOCKERROR" in str(execinfo.value)
-
def test_lock_retries(tmpdir):
+ """
+ 2 thread want to lock a same file
+ Lock has zero retries
+ One thread will have LockError raised
+ """
+ def lock_thread_retries(tmpdir, file_path):
+ with pytest.raises(LockError) as execinfo:
+ with FileLock(file_path, retries=0):
+ assert False # should never enter here, since max_tires is 0
+ assert "LOCKERROR" in str(execinfo.value)
from threading import Thread
package_name = "conda_file_3"
@@ -106,3 +110,19 @@ def test_lock_retries(tmpdir):
t.join()
# lock should clean up after itself
assert not tmpdir.join(path).exists()
+
+
+def test_permission_file():
+ """
+ Test when lock cannot be created due to permission
+ Make sure no exception raised
+ """
+ import tempfile
+ from conda.compat import text_type
+ with tempfile.NamedTemporaryFile(mode='r') as f:
+ if not isinstance(f.name, text_type):
+ return
+ with FileLock(f.name) as lock:
+
+ path = basename(lock.lock_file_path)
+ assert not exists(join(f.name, path))
| [Regression] Conda create environment fails on lock if root environment is not under user control
This is "funny", but it seems that Conda managed to break this thing the second time in a month... #2681 was the previous one.
This time, I get the following error:
```
$ conda create -n _root --yes --use-index-cache python=3
...
Traceback (most recent call last):
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/exceptions.py", line 442, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/cli/main_create.py", line 66, in execute
install(args, parser, 'create')
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/cli/install.py", line 399, in install
execute_actions(actions, index, verbose=not args.quiet)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/plan.py", line 640, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/instructions.py", line 132, in execute_instructions
cmd(state, arg)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/instructions.py", line 77, in LINK_CMD
link(state['prefix'], dist, lt, index=state['index'])
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/install.py", line 1060, in link
with DirectoryLock(prefix), FileLock(source_dir):
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/lock.py", line 86, in __enter__
touch(self.lock_file_path)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/lock.py", line 48, in touch
with open(file_name, 'a'):
PermissionError: [Errno 13] Permission denied: '/usr/local/miniconda/pkgs/openssl-1.0.2h-1.pid34.conda_lock'
```
```
Current conda install:
platform : linux-64
conda version : 4.2.2
conda is private : False
conda-env version : 2.6.0
conda-build version : 1.21.11+0.g5b44ab3.dirty
python version : 3.5.2.final.0
requests version : 2.10.0
root environment : /usr/local/miniconda (read only)
default environment : /usr/local/miniconda
envs directories : /home/gitlab-ci/.conda/envs
/usr/local/miniconda/envs
package cache : /home/gitlab-ci/.conda/envs/.pkgs
/usr/local/miniconda/pkgs
channel URLs : defaults
config file : None
offline mode : False
```
/CC @kalefranz
[Regression] Conda create environment fails on lock if root environment is not under user control
This is "funny", but it seems that Conda managed to break this thing the second time in a month... #2681 was the previous one.
This time, I get the following error:
```
$ conda create -n _root --yes --use-index-cache python=3
...
Traceback (most recent call last):
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/exceptions.py", line 442, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/cli/main_create.py", line 66, in execute
install(args, parser, 'create')
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/cli/install.py", line 399, in install
execute_actions(actions, index, verbose=not args.quiet)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/plan.py", line 640, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/instructions.py", line 132, in execute_instructions
cmd(state, arg)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/instructions.py", line 77, in LINK_CMD
link(state['prefix'], dist, lt, index=state['index'])
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/install.py", line 1060, in link
with DirectoryLock(prefix), FileLock(source_dir):
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/lock.py", line 86, in __enter__
touch(self.lock_file_path)
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/lock.py", line 48, in touch
with open(file_name, 'a'):
PermissionError: [Errno 13] Permission denied: '/usr/local/miniconda/pkgs/openssl-1.0.2h-1.pid34.conda_lock'
```
```
Current conda install:
platform : linux-64
conda version : 4.2.2
conda is private : False
conda-env version : 2.6.0
conda-build version : 1.21.11+0.g5b44ab3.dirty
python version : 3.5.2.final.0
requests version : 2.10.0
root environment : /usr/local/miniconda (read only)
default environment : /usr/local/miniconda
envs directories : /home/gitlab-ci/.conda/envs
/usr/local/miniconda/envs
package cache : /home/gitlab-ci/.conda/envs/.pkgs
/usr/local/miniconda/pkgs
channel URLs : defaults
config file : None
offline mode : False
```
/CC @kalefranz
| @frol, First, **thanks for using canary**!! Do you know if a previous run of conda crashed, exited prematurely, or you sent a signal (i.e. ctrl-c) for it to exit early? Something made that lock file stick around...
```
conda clean --yes --lock
```
should take care of it for you. If it doesn't, that's definitely a bug.
We're still working on tuning the locking in conda. It's (hopefully) better in 4.2 than it was in 4.1, and I know for sure it will be better yet in 4.3 with @HugoTian's work in #3197.
@kalefranz The error message is quite informative:
```
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/lock.py", line 48, in touch
with open(file_name, 'a'):
PermissionError: [Errno 13] Permission denied: '/usr/local/miniconda/pkgs/openssl-1.0.2h-1.pid34.conda_lock'
```
`/usr/local/miniconda` is a read-only directory, so there is no way `open(mode='a')` will ever succeed there.
Ahh. This then is what I generally call "multi-user support." That is, install conda as one user, and use the executable as another but without write permissions to the conda executable's root environment.
This used case has always been problematic, and it's s high priority to tackle and "get right" in the next several months.
I think I just ran into this, created a env in a central install area, then from a user account tried to clone the environment, but it failed with something similar, doing
`conda create --offline -n myrel --clone anarel-1.0.0
`
caused
```
...
WARNING conda.install:warn_failed_remove(178): Cannot remove, permission denied: /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs/psana-conda-1.0.0-py27_1/bin
WARNING conda.install:warn_failed_remove(178): Cannot remove, permission denied: /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs/psana-conda-1.0.0-py27_1
Pruning fetched packages from the cache ...
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : linux-64
conda version : 4.2.3
conda is private : False
conda-env version : 2.6.0
conda-build version : 1.21.11+0.g5b44ab3.dirty
python version : 2.7.12.final.0
requests version : 2.10.0
root environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7 (read only)
default environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs/anarel-1.0.0
envs directories : /reg/neh/home/davidsch/.conda/envs
/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs
package cache : /reg/neh/home/davidsch/.conda/envs/.pkgs
/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs
channel URLs : file:///reg/g/psdm/sw/conda/channels/system-rhel7
file:///reg/g/psdm/sw/conda/channels/external-rhel7
defaults
file:///reg/g/psdm/sw/conda/channels/psana-rhel7
scikit-beam
file:///reg/g/psdm/sw/conda/channels/testing-rhel7
config file : /reg/neh/home/davidsch/.condarc
offline mode : False
`$ /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs/anarel-1.0.0/bin/conda create --offline -n myrel --clone anarel-1.0.0`
Traceback (most recent call last):
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/exceptions.py", line 442, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/main_create.py", line 66, in execute
install(args, parser, 'create')
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/install.py", line 225, in install
clone(args.clone, prefix, json=args.json, quiet=args.quiet, index_args=index_args)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/install.py", line 90, in clone
index_args=index_args)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/misc.py", line 388, in clone_env
force_extract=False, index_args=index_args)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/misc.py", line 188, in explicit
execute_actions(actions, index=index, verbose=verbose)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/plan.py", line 639, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 132, in execute_instructions
cmd(state, arg)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 66, in RM_FETCHED_CMD
rm_fetched(arg)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/install.py", line 801, in rm_fetched
with FileLock(fname):
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/lock.py", line 86, in __enter__
touch(self.lock_file_path)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/lock.py", line 48, in touch
with open(file_name, 'a'):
IOError: [Errno 13] Permission denied: u'/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs/openmpi-1.10.3-lsf_verbs_100.tar.bz2.pid17825.conda_lock'
```
@HugoTian As part of the 4.2 release, I think we need a lock cleanup step in the exception handler that just attempts to clean up any lock files for the current pid before it ultimately exits. Do you agree?
@kalefranz , Moreover, I think some how we need to bring back the try/except block when we create the lock file.
Something like :
```
try:
touch(****)
except OSError:
pass
```
@frol, First, **thanks for using canary**!! Do you know if a previous run of conda crashed, exited prematurely, or you sent a signal (i.e. ctrl-c) for it to exit early? Something made that lock file stick around...
```
conda clean --yes --lock
```
should take care of it for you. If it doesn't, that's definitely a bug.
We're still working on tuning the locking in conda. It's (hopefully) better in 4.2 than it was in 4.1, and I know for sure it will be better yet in 4.3 with @HugoTian's work in #3197.
@kalefranz The error message is quite informative:
```
File "/usr/local/miniconda/lib/python3.5/site-packages/conda/lock.py", line 48, in touch
with open(file_name, 'a'):
PermissionError: [Errno 13] Permission denied: '/usr/local/miniconda/pkgs/openssl-1.0.2h-1.pid34.conda_lock'
```
`/usr/local/miniconda` is a read-only directory, so there is no way `open(mode='a')` will ever succeed there.
Ahh. This then is what I generally call "multi-user support." That is, install conda as one user, and use the executable as another but without write permissions to the conda executable's root environment.
This used case has always been problematic, and it's s high priority to tackle and "get right" in the next several months.
I think I just ran into this, created a env in a central install area, then from a user account tried to clone the environment, but it failed with something similar, doing
`conda create --offline -n myrel --clone anarel-1.0.0
`
caused
```
...
WARNING conda.install:warn_failed_remove(178): Cannot remove, permission denied: /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs/psana-conda-1.0.0-py27_1/bin
WARNING conda.install:warn_failed_remove(178): Cannot remove, permission denied: /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs/psana-conda-1.0.0-py27_1
Pruning fetched packages from the cache ...
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : linux-64
conda version : 4.2.3
conda is private : False
conda-env version : 2.6.0
conda-build version : 1.21.11+0.g5b44ab3.dirty
python version : 2.7.12.final.0
requests version : 2.10.0
root environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7 (read only)
default environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs/anarel-1.0.0
envs directories : /reg/neh/home/davidsch/.conda/envs
/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs
package cache : /reg/neh/home/davidsch/.conda/envs/.pkgs
/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs
channel URLs : file:///reg/g/psdm/sw/conda/channels/system-rhel7
file:///reg/g/psdm/sw/conda/channels/external-rhel7
defaults
file:///reg/g/psdm/sw/conda/channels/psana-rhel7
scikit-beam
file:///reg/g/psdm/sw/conda/channels/testing-rhel7
config file : /reg/neh/home/davidsch/.condarc
offline mode : False
`$ /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs/anarel-1.0.0/bin/conda create --offline -n myrel --clone anarel-1.0.0`
Traceback (most recent call last):
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/exceptions.py", line 442, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/main_create.py", line 66, in execute
install(args, parser, 'create')
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/install.py", line 225, in install
clone(args.clone, prefix, json=args.json, quiet=args.quiet, index_args=index_args)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/cli/install.py", line 90, in clone
index_args=index_args)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/misc.py", line 388, in clone_env
force_extract=False, index_args=index_args)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/misc.py", line 188, in explicit
execute_actions(actions, index=index, verbose=verbose)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/plan.py", line 639, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 132, in execute_instructions
cmd(state, arg)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 66, in RM_FETCHED_CMD
rm_fetched(arg)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/install.py", line 801, in rm_fetched
with FileLock(fname):
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/lock.py", line 86, in __enter__
touch(self.lock_file_path)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/lock.py", line 48, in touch
with open(file_name, 'a'):
IOError: [Errno 13] Permission denied: u'/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs/openmpi-1.10.3-lsf_verbs_100.tar.bz2.pid17825.conda_lock'
```
@HugoTian As part of the 4.2 release, I think we need a lock cleanup step in the exception handler that just attempts to clean up any lock files for the current pid before it ultimately exits. Do you agree?
@kalefranz , Moreover, I think some how we need to bring back the try/except block when we create the lock file.
Something like :
```
try:
touch(****)
except OSError:
pass
```
| 2016-08-20T01:22:54 | -1.0 |
conda/conda | 3,390 | conda__conda-3390 | [
"3282"
] | 35c14b82aa582f17f37042ae15bcac9d9c0761df | diff --git a/conda/common/configuration.py b/conda/common/configuration.py
--- a/conda/common/configuration.py
+++ b/conda/common/configuration.py
@@ -188,7 +188,7 @@ def __repr__(self):
return text_type(vars(self))
@abstractmethod
- def value(self, parameter_type):
+ def value(self, parameter_obj):
raise NotImplementedError()
@abstractmethod
@@ -196,7 +196,7 @@ def keyflag(self):
raise NotImplementedError()
@abstractmethod
- def valueflags(self, parameter_type):
+ def valueflags(self, parameter_obj):
raise NotImplementedError()
@classmethod
@@ -209,14 +209,29 @@ def make_raw_parameters(cls, source, from_map):
class EnvRawParameter(RawParameter):
source = 'envvars'
- def value(self, parameter_type):
- return self.__important_split_value[0].strip()
+ def value(self, parameter_obj):
+ if hasattr(parameter_obj, 'string_delimiter'):
+ string_delimiter = getattr(parameter_obj, 'string_delimiter')
+ # TODO: add stripping of !important, !top, and !bottom
+ raw_value = self._raw_value
+ if string_delimiter in raw_value:
+ value = raw_value.split(string_delimiter)
+ else:
+ value = [raw_value]
+ return tuple(v.strip() for v in value)
+ else:
+ return self.__important_split_value[0].strip()
def keyflag(self):
return ParameterFlag.final if len(self.__important_split_value) >= 2 else None
- def valueflags(self, parameter_type):
- return None
+ def valueflags(self, parameter_obj):
+ if hasattr(parameter_obj, 'string_delimiter'):
+ string_delimiter = getattr(parameter_obj, 'string_delimiter')
+ # TODO: add stripping of !important, !top, and !bottom
+ return tuple('' for _ in self._raw_value.split(string_delimiter))
+ else:
+ return self.__important_split_value[0].strip()
@property
def __important_split_value(self):
@@ -233,13 +248,13 @@ def make_raw_parameters(cls, appname):
class ArgParseRawParameter(RawParameter):
source = 'cmd_line'
- def value(self, parameter_type):
+ def value(self, parameter_obj):
return make_immutable(self._raw_value)
def keyflag(self):
return None
- def valueflags(self, parameter_type):
+ def valueflags(self, parameter_obj):
return None
@classmethod
@@ -255,18 +270,18 @@ def __init__(self, source, key, raw_value, keycomment):
self._keycomment = keycomment
super(YamlRawParameter, self).__init__(source, key, raw_value)
- def value(self, parameter_type):
- self.__process(parameter_type)
+ def value(self, parameter_obj):
+ self.__process(parameter_obj)
return self._value
def keyflag(self):
return ParameterFlag.from_string(self._keycomment)
- def valueflags(self, parameter_type):
- self.__process(parameter_type)
+ def valueflags(self, parameter_obj):
+ self.__process(parameter_obj)
return self._valueflags
- def __process(self, parameter_type):
+ def __process(self, parameter_obj):
if hasattr(self, '_value'):
return
elif isinstance(self._raw_value, CommentedSeq):
@@ -511,18 +526,17 @@ def __init__(self, default, aliases=(), validation=None, parameter_type=None):
def _merge(self, matches):
important_match = first(matches, self._match_key_is_important, default=None)
if important_match is not None:
- return important_match.value(self.__class__)
+ return important_match.value(self)
last_match = last(matches, lambda x: x is not None, default=None)
if last_match is not None:
- return last_match.value(self.__class__)
+ return last_match.value(self)
raise ThisShouldNeverHappenError() # pragma: no cover
- @classmethod
- def repr_raw(cls, raw_parameter):
+ def repr_raw(self, raw_parameter):
return "%s: %s%s" % (raw_parameter.key,
- cls._str_format_value(raw_parameter.value(cls)),
- cls._str_format_flag(raw_parameter.keyflag()))
+ self._str_format_value(raw_parameter.value(self)),
+ self._str_format_flag(raw_parameter.keyflag()))
class SequenceParameter(Parameter):
@@ -531,7 +545,8 @@ class SequenceParameter(Parameter):
"""
_type = tuple
- def __init__(self, element_type, default=(), aliases=(), validation=None):
+ def __init__(self, element_type, default=(), aliases=(), validation=None,
+ string_delimiter=','):
"""
Args:
element_type (type or Iterable[type]): The generic type of each element in
@@ -543,6 +558,7 @@ def __init__(self, element_type, default=(), aliases=(), validation=None):
"""
self._element_type = element_type
+ self.string_delimiter = string_delimiter
super(SequenceParameter, self).__init__(default, aliases, validation)
def collect_errors(self, instance, value, source="<<merged>>"):
@@ -562,21 +578,21 @@ def _merge(self, matches):
# get individual lines from important_matches that were marked important
# these will be prepended to the final result
- def get_marked_lines(match, marker):
+ def get_marked_lines(match, marker, parameter_obj):
return tuple(line
- for line, flag in zip(match.value(self.__class__),
- match.valueflags(self.__class__))
+ for line, flag in zip(match.value(parameter_obj),
+ match.valueflags(parameter_obj))
if flag is marker)
- top_lines = concat(get_marked_lines(m, ParameterFlag.top) for m in relevant_matches)
+ top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m in relevant_matches)
# also get lines that were marked as bottom, but reverse the match order so that lines
# coming earlier will ultimately be last
- bottom_lines = concat(get_marked_lines(m, ParameterFlag.bottom) for m in
+ bottom_lines = concat(get_marked_lines(m, ParameterFlag.bottom, self) for m in
reversed(relevant_matches))
# now, concat all lines, while reversing the matches
# reverse because elements closer to the end of search path take precedence
- all_lines = concat(m.value(self.__class__) for m in reversed(relevant_matches))
+ all_lines = concat(m.value(self) for m in reversed(relevant_matches))
# stack top_lines + all_lines, then de-dupe
top_deduped = tuple(unique(concatv(top_lines, all_lines)))
@@ -586,19 +602,17 @@ def get_marked_lines(match, marker):
# NOTE: for a line value marked both top and bottom, the bottom marker will win out
# for the top marker to win out, we'd need one additional de-dupe step
bottom_deduped = unique(concatv(reversed(tuple(bottom_lines)), reversed(top_deduped)))
-
# just reverse, and we're good to go
return tuple(reversed(tuple(bottom_deduped)))
- @classmethod
- def repr_raw(cls, raw_parameter):
+ def repr_raw(self, raw_parameter):
lines = list()
lines.append("%s:%s" % (raw_parameter.key,
- cls._str_format_flag(raw_parameter.keyflag())))
- for q, value in enumerate(raw_parameter.value(cls)):
- valueflag = raw_parameter.valueflags(cls)[q]
- lines.append(" - %s%s" % (cls._str_format_value(value),
- cls._str_format_flag(valueflag)))
+ self._str_format_flag(raw_parameter.keyflag())))
+ for q, value in enumerate(raw_parameter.value(self)):
+ valueflag = raw_parameter.valueflags(self)[q]
+ lines.append(" - %s%s" % (self._str_format_value(value),
+ self._str_format_flag(valueflag)))
return '\n'.join(lines)
@@ -635,25 +649,24 @@ def _merge(self, matches):
# mapkeys with important matches
def key_is_important(match, key):
- return match.valueflags(self.__class__).get(key) is ParameterFlag.final
+ return match.valueflags(self).get(key) is ParameterFlag.final
important_maps = tuple(dict((k, v)
- for k, v in iteritems(match.value(self.__class__))
+ for k, v in iteritems(match.value(self))
if key_is_important(match, k))
for match in relevant_matches)
# dump all matches in a dict
# then overwrite with important matches
- return merge(concatv((m.value(self.__class__) for m in relevant_matches),
+ return merge(concatv((m.value(self) for m in relevant_matches),
reversed(important_maps)))
- @classmethod
- def repr_raw(cls, raw_parameter):
+ def repr_raw(self, raw_parameter):
lines = list()
lines.append("%s:%s" % (raw_parameter.key,
- cls._str_format_flag(raw_parameter.keyflag())))
- for valuekey, value in iteritems(raw_parameter.value(cls)):
- valueflag = raw_parameter.valueflags(cls).get(valuekey)
- lines.append(" %s: %s%s" % (valuekey, cls._str_format_value(value),
- cls._str_format_flag(valueflag)))
+ self._str_format_flag(raw_parameter.keyflag())))
+ for valuekey, value in iteritems(raw_parameter.value(self)):
+ valueflag = raw_parameter.valueflags(self).get(valuekey)
+ lines.append(" %s: %s%s" % (valuekey, self._str_format_value(value),
+ self._str_format_flag(valueflag)))
return '\n'.join(lines)
@@ -717,7 +730,7 @@ def check_source(self, source):
if match is not None:
try:
- typed_value = typify_data_structure(match.value(parameter.__class__),
+ typed_value = typify_data_structure(match.value(parameter),
parameter._element_type)
except TypeCoercionError as e:
validation_errors.append(CustomValidationError(match.key, e.value,
| diff --git a/tests/common/test_configuration.py b/tests/common/test_configuration.py
--- a/tests/common/test_configuration.py
+++ b/tests/common/test_configuration.py
@@ -128,7 +128,7 @@
}
-class TestConfiguration(Configuration):
+class SampleConfiguration(Configuration):
always_yes = PrimitiveParameter(False, aliases=('always_yes_altname1', 'yes',
'always_yes_altname2'))
changeps1 = PrimitiveParameter(True)
@@ -148,13 +148,13 @@ def load_from_string_data(*seq):
class ConfigurationTests(TestCase):
def test_simple_merges_and_caching(self):
- config = TestConfiguration()._add_raw_data(load_from_string_data('file1', 'file2'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('file1', 'file2'))
assert config.changeps1 is False
assert config.always_yes is True
assert config.channels == ('porky', 'bugs', 'elmer', 'daffy', 'tweety')
assert config.proxy_servers == {'http': 'marv', 'https': 'sam', 's3': 'pepé'}
- config = TestConfiguration()._add_raw_data(load_from_string_data('file2', 'file1'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('file2', 'file1'))
assert len(config._cache) == 0
assert config.changeps1 is False
assert len(config._cache) == 1
@@ -166,7 +166,7 @@ def test_simple_merges_and_caching(self):
assert config.proxy_servers == {'http': 'taz', 'https': 'sly', 's3': 'pepé'}
def test_default_values(self):
- config = TestConfiguration()
+ config = SampleConfiguration()
assert config.channels == ()
assert config.always_yes is False
assert config.proxy_servers == {}
@@ -183,8 +183,7 @@ def make_key(appname, key):
try:
environ.update(test_dict)
assert 'MYAPP_ALWAYS_YES' in environ
- raw_data = load_from_string_data('file1', 'file2')
- config = TestConfiguration(app_name=appname)
+ config = SampleConfiguration(app_name=appname)
assert config.changeps1 is False
assert config.always_yes is True
finally:
@@ -201,13 +200,42 @@ def make_key(appname, key):
try:
environ.update(test_dict)
assert 'MYAPP_YES' in environ
- raw_data = load_from_string_data('file1', 'file2')
- config = TestConfiguration()._add_env_vars(appname)
+ config = SampleConfiguration()._add_env_vars(appname)
assert config.always_yes is True
assert config.changeps1 is False
finally:
[environ.pop(key) for key in test_dict]
+ def test_env_var_config_split_sequence(self):
+ def make_key(appname, key):
+ return "{0}_{1}".format(appname.upper(), key.upper())
+ appname = "myapp"
+ test_dict = {}
+ test_dict[make_key(appname, 'channels')] = 'channel1,channel2'
+
+ try:
+ environ.update(test_dict)
+ assert 'MYAPP_CHANNELS' in environ
+ config = SampleConfiguration()._add_env_vars(appname)
+ assert config.channels == ('channel1', 'channel2')
+ finally:
+ [environ.pop(key) for key in test_dict]
+
+ def test_env_var_config_no_split_sequence(self):
+ def make_key(appname, key):
+ return "{0}_{1}".format(appname.upper(), key.upper())
+ appname = "myapp"
+ test_dict = {}
+ test_dict[make_key(appname, 'channels')] = 'channel1'
+
+ try:
+ environ.update(test_dict)
+ assert 'MYAPP_CHANNELS' in environ
+ config = SampleConfiguration()._add_env_vars(appname)
+ assert config.channels == ('channel1',)
+ finally:
+ [environ.pop(key) for key in test_dict]
+
def test_load_raw_configs(self):
try:
tempdir = mkdtemp()
@@ -232,7 +260,7 @@ def test_load_raw_configs(self):
assert raw_data[f1]['always_yes'].value(None) == "no"
assert raw_data[f2]['proxy_servers'].value(None)['http'] == "marv"
- config = TestConfiguration(search_path)
+ config = SampleConfiguration(search_path)
assert config.channels == ('wile', 'porky', 'bugs', 'elmer', 'daffy',
'tweety', 'foghorn')
@@ -241,105 +269,105 @@ def test_load_raw_configs(self):
def test_important_primitive_map_merges(self):
raw_data = load_from_string_data('file1', 'file3', 'file2')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is False
assert config.always_yes is True
assert config.channels == ('wile', 'porky', 'bugs', 'elmer', 'daffy', 'foghorn', 'tweety')
assert config.proxy_servers == {'http': 'foghorn', 'https': 'sam', 's3': 'porky'}
raw_data = load_from_string_data('file3', 'file2', 'file1')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is False
assert config.always_yes is True
assert config.channels == ('wile', 'bugs', 'daffy', 'tweety', 'porky', 'elmer', 'foghorn')
assert config.proxy_servers == {'http': 'foghorn', 'https': 'sly', 's3': 'pepé'}
raw_data = load_from_string_data('file4', 'file3', 'file1')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is False
assert config.always_yes is True
assert config.proxy_servers == {'https': 'daffy', 'http': 'bugs'}
raw_data = load_from_string_data('file1', 'file4', 'file3', 'file2')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is False
assert config.always_yes is True
assert config.proxy_servers == {'http': 'bugs', 'https': 'daffy', 's3': 'pepé'}
raw_data = load_from_string_data('file1', 'file2', 'file3', 'file4')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is False
assert config.always_yes is True
assert config.proxy_servers == {'https': 'daffy', 'http': 'foghorn', 's3': 'porky'}
raw_data = load_from_string_data('file3', 'file1')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is True
assert config.always_yes is True
assert config.proxy_servers == {'https': 'sly', 'http': 'foghorn', 's3': 'pepé'}
raw_data = load_from_string_data('file4', 'file3')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.changeps1 is False
assert config.always_yes is True
assert config.proxy_servers == {'http': 'bugs', 'https': 'daffy'}
def test_list_merges(self):
raw_data = load_from_string_data('file5', 'file3')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('marv', 'wile', 'daffy', 'foghorn', 'pepé', 'sam')
raw_data = load_from_string_data('file6', 'file5', 'file4', 'file3')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('pepé', 'sam', 'elmer', 'bugs', 'marv')
raw_data = load_from_string_data('file3', 'file4', 'file5', 'file6')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('wile', 'pepé', 'marv', 'sam', 'daffy', 'foghorn')
raw_data = load_from_string_data('file6', 'file3', 'file4', 'file5')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('wile', 'pepé', 'sam', 'daffy', 'foghorn',
'elmer', 'bugs', 'marv')
raw_data = load_from_string_data('file7', 'file8', 'file9')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('sam', 'marv', 'wile', 'foghorn', 'daffy', 'pepé')
raw_data = load_from_string_data('file7', 'file9', 'file8')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('sam', 'marv', 'pepé', 'wile', 'foghorn', 'daffy')
raw_data = load_from_string_data('file8', 'file7', 'file9')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('marv', 'sam', 'wile', 'foghorn', 'daffy', 'pepé')
raw_data = load_from_string_data('file8', 'file9', 'file7')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('marv', 'sam', 'wile', 'daffy', 'pepé')
raw_data = load_from_string_data('file9', 'file7', 'file8')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('marv', 'sam', 'pepé', 'daffy')
raw_data = load_from_string_data('file9', 'file8', 'file7')
- config = TestConfiguration()._add_raw_data(raw_data)
+ config = SampleConfiguration()._add_raw_data(raw_data)
assert config.channels == ('marv', 'sam', 'pepé', 'daffy')
def test_validation(self):
- config = TestConfiguration()._add_raw_data(load_from_string_data('bad_boolean'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('bad_boolean'))
raises(ValidationError, lambda: config.always_yes)
- config = TestConfiguration()._add_raw_data(load_from_string_data('too_many_aliases'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('too_many_aliases'))
raises(ValidationError, lambda: config.always_yes)
- config = TestConfiguration()._add_raw_data(load_from_string_data('not_an_int'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('not_an_int'))
raises(ValidationError, lambda: config.always_an_int)
- config = TestConfiguration()._add_raw_data(load_from_string_data('bad_boolean_map'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('bad_boolean_map'))
raises(ValidationError, lambda: config.boolean_map)
- config = TestConfiguration()._add_raw_data(load_from_string_data('good_boolean_map'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('good_boolean_map'))
assert config.boolean_map['a_true'] is True
assert config.boolean_map['a_yes'] is True
assert config.boolean_map['a_1'] is True
@@ -351,10 +379,10 @@ def test_parameter(self):
assert ParameterFlag.from_name('top') is ParameterFlag.top
def test_validate_all(self):
- config = TestConfiguration()._add_raw_data(load_from_string_data('file1'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('file1'))
config.validate_all()
- config = TestConfiguration()._add_raw_data(load_from_string_data('bad_boolean_map'))
+ config = SampleConfiguration()._add_raw_data(load_from_string_data('bad_boolean_map'))
raises(ValidationError, config.validate_all)
def test_cross_parameter_validation(self):
diff --git a/tests/conda_env/support/notebook.ipynb b/tests/conda_env/support/notebook.ipynb
--- a/tests/conda_env/support/notebook.ipynb
+++ b/tests/conda_env/support/notebook.ipynb
@@ -1 +1 @@
-{"nbformat": 3, "nbformat_minor": 0, "worksheets": [{"cells": []}], "metadata": {"name": "", "signature": ""}}
\ No newline at end of file
+{"nbformat": 3, "worksheets": [{"cells": []}], "metadata": {"signature": "", "name": ""}, "nbformat_minor": 0}
\ No newline at end of file
| CONDA_CHANNELS environment variable doesn't work
fixed with #3390
| 2016-09-06T21:54:02 | -1.0 |
|
conda/conda | 3,416 | conda__conda-3416 | [
"3407",
"3407"
] | f1a591d5a5afd707dc38386d7504ebcf974f22c0 | diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -128,8 +128,9 @@ def __init__(self, *args, **kwargs):
class PaddingError(CondaError):
- def __init__(self, *args):
- msg = 'Padding error: %s' % ' '.join(text_type(arg) for arg in self.args)
+ def __init__(self, dist, placeholder, placeholder_length):
+ msg = ("Placeholder of length '%d' too short in package %s.\n"
+ "The package must be rebuilt with conda-build > 2.0." % (placeholder_length, dist))
super(PaddingError, self).__init__(msg)
diff --git a/conda/install.py b/conda/install.py
--- a/conda/install.py
+++ b/conda/install.py
@@ -248,6 +248,10 @@ def parse_line(line):
return {pr.filepath: (pr.placeholder, pr.filemode) for pr in parsed_lines}
+class _PaddingError(Exception):
+ pass
+
+
def binary_replace(data, a, b):
"""
Perform a binary replacement of `data`, where the placeholder `a` is
@@ -261,7 +265,7 @@ def replace(match):
occurances = match.group().count(a)
padding = (len(a) - len(b))*occurances
if padding < 0:
- raise PaddingError(a, b, padding)
+ raise _PaddingError
return match.group().replace(a, b) + b'\0' * padding
original_data_len = len(data)
@@ -968,9 +972,8 @@ def link(prefix, dist, linktype=LINK_HARD, index=None):
placeholder, mode = has_prefix_files[filepath]
try:
update_prefix(join(prefix, filepath), prefix, placeholder, mode)
- except PaddingError:
- raise PaddingError("ERROR: placeholder '%s' too short in: %s\n" %
- (placeholder, dist))
+ except _PaddingError:
+ raise PaddingError(dist, placeholder, len(placeholder))
# make sure that the child environment behaves like the parent,
# wrt user/system install on win
| diff --git a/tests/test_install.py b/tests/test_install.py
--- a/tests/test_install.py
+++ b/tests/test_install.py
@@ -18,7 +18,8 @@
from conda.fetch import download
from conda.install import (FileMode, PaddingError, binary_replace, dist2dirname, dist2filename,
dist2name, dist2pair, dist2quad, name_dist, on_win,
- read_no_link, update_prefix, warn_failed_remove, yield_lines)
+ read_no_link, update_prefix, warn_failed_remove, yield_lines,
+ _PaddingError)
from contextlib import contextmanager
from os import chdir, getcwd, makedirs
from os.path import dirname, exists, join, relpath
@@ -46,7 +47,7 @@ def test_shorter(self):
b'xxxbbbbxyz\x00\x00zz')
def test_too_long(self):
- self.assertRaises(PaddingError, binary_replace,
+ self.assertRaises(_PaddingError, binary_replace,
b'xxxaaaaaxyz\x00zz', b'aaaaa', b'bbbbbbbb')
def test_no_extra(self):
@@ -71,7 +72,7 @@ def test_multiple(self):
self.assertEqual(
binary_replace(b'aaaacaaaa\x00', b'aaaa', b'bbb'),
b'bbbcbbb\x00\x00\x00')
- self.assertRaises(PaddingError, binary_replace,
+ self.assertRaises(_PaddingError, binary_replace,
b'aaaacaaaa\x00', b'aaaa', b'bbbbb')
@pytest.mark.skipif(not on_win, reason="exe entry points only necessary on win")
| conda build - exception - padding error
I just updated to the lastest conda-build in defaults, and latest conda conda-env in conda canary, when I run conda build I am getting a 'package error'. Here is my conda environemnt:
```
platform : linux-64
conda version : 4.2.5
conda is private : False
conda-env version : 2.6.0
conda-build version : 2.0.1
python version : 2.7.12.final.0
requests version : 2.10.0
root environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7 (writable)
default environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7
envs directories : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs
package cache : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs
channel URLs : file:///reg/g/psdm/sw/conda/channels/system-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/system-rhel7/noarch/
file:///reg/g/psdm/sw/conda/channels/psana-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/psana-rhel7/noarch/
file:///reg/g/psdm/sw/conda/channels/external-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/external-rhel7/noarch/
https://repo.continuum.io/pkgs/free/linux-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/linux-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://conda.anaconda.org/scikit-beam/linux-64/
https://conda.anaconda.org/scikit-beam/noarch/
file:///reg/g/psdm/sw/conda/channels/testing-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/testing-rhel7/noarch/
config file : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/.condarc
offline mode : False
```
and here is the build output
```
SIT_ARCH=x86_64-rhel7-gcc48-opt conda build -c file:///reg/g/psdm/sw/conda/channels/system-rhel7 -c file:///reg/g/psdm/sw/conda/channels/psana-rhel7 -c file:///reg/g/psdm/sw/conda/channels/external-rhel7 --quiet recipes/psana/psana-conda-opt 2>&1 | tee -a /reg/g/psdm/sw/conda/logs/conda_build_psana_psana-conda-1.0.2-py27_1_rhel7_linux-64.log
/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/environ.py:303: UserWarning: The environment variable 'SIT_ROOT' is undefined.
UserWarning
BUILD START: psana-conda-1.0.2-py27_1
(actual version deferred until further download or env creation)
The following NEW packages will be INSTALLED:
boost: 1.57.0-4
cairo: 1.12.18-6
cycler: 0.10.0-py27_0
cython: 0.24.1-py27_0
fontconfig: 2.11.1-6
freetype: 2.5.5-1
h5py: 2.5.0-py27_hdf518_mpi4py2_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
hdf5: 1.8.17-openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
icu: 54.1-0
libgfortran: 3.0.0-1
libpng: 1.6.22-0
libsodium: 1.0.10-0
libxml2: 2.9.2-0
matplotlib: 1.5.1-np111py27_0
mkl: 11.3.3-0
mpi4py: 2.0.0-py27_openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
mysql: 5.5.24-0
ndarray: 1.1.5-0 file:///reg/g/psdm/sw/conda/channels/psana-rhel7
numexpr: 2.6.1-np111py27_0
numpy: 1.11.1-py27_0
openmpi: 1.10.3-lsf_verbs_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
openssl: 1.0.2h-1
pip: 8.1.2-py27_0
pixman: 0.32.6-0
pycairo: 1.10.0-py27_0
pyparsing: 2.1.4-py27_0
pyqt: 4.11.4-py27_4
python: 2.7.12-1
python-dateutil: 2.5.3-py27_0
pytz: 2016.6.1-py27_0
pyzmq: 15.4.0-py27_0
qt: 4.8.5-0
readline: 6.2-2
scipy: 0.18.0-np111py27_0
scons: 2.3.0-py27_0
setuptools: 26.1.1-py27_0
sip: 4.18-py27_0
six: 1.10.0-py27_0
sqlite: 3.13.0-0
szip: 2.1-100 file:///reg/g/psdm/sw/conda/channels/external-rhel7
tables: 3.2.3.1-py27_hdf18_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
tk: 8.5.18-0
wheel: 0.29.0-py27_0
zeromq: 4.1.4-0
zlib: 1.2.8-3
The following NEW packages will be INSTALLED:
boost: 1.57.0-4
cairo: 1.12.18-6
cycler: 0.10.0-py27_0
cython: 0.24.1-py27_0
fontconfig: 2.11.1-6
freetype: 2.5.5-1
h5py: 2.5.0-py27_hdf518_mpi4py2_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
hdf5: 1.8.17-openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
icu: 54.1-0
libgfortran: 3.0.0-1
libpng: 1.6.22-0
libsodium: 1.0.10-0
libxml2: 2.9.2-0
matplotlib: 1.5.1-np111py27_0
mkl: 11.3.3-0
mpi4py: 2.0.0-py27_openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
mysql: 5.5.24-0
ndarray: 1.1.5-0 file:///reg/g/psdm/sw/conda/channels/psana-rhel7
numexpr: 2.6.1-np111py27_0
numpy: 1.11.1-py27_0
openmpi: 1.10.3-lsf_verbs_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
openssl: 1.0.2h-1
pip: 8.1.2-py27_0
pixman: 0.32.6-0
pycairo: 1.10.0-py27_0
pyparsing: 2.1.4-py27_0
pyqt: 4.11.4-py27_4
python: 2.7.12-1
python-dateutil: 2.5.3-py27_0
pytz: 2016.6.1-py27_0
pyzmq: 15.4.0-py27_0
qt: 4.8.5-0
readline: 6.2-2
scipy: 0.18.0-np111py27_0
scons: 2.3.0-py27_0
setuptools: 26.1.1-py27_0
sip: 4.18-py27_0
six: 1.10.0-py27_0
sqlite: 3.13.0-0
szip: 2.1-100 file:///reg/g/psdm/sw/conda/channels/external-rhel7
tables: 3.2.3.1-py27_hdf18_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
tk: 8.5.18-0
wheel: 0.29.0-py27_0
zeromq: 4.1.4-0
zlib: 1.2.8-3
Traceback (most recent call last):
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/bin/conda-build", line 6, in <module>
sys.exit(conda_build.cli.main_build.main())
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/cli/main_build.py", line 239, in main
execute(sys.argv[1:])
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/cli/main_build.py", line 231, in execute
already_built=None, config=config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/api.py", line 83, in build
need_source_download=need_source_download, config=config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 998, in build_tree
config=recipe_config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 558, in build
create_env(config.build_prefix, specs, config=config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 451, in create_env
clear_cache=clear_cache)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 427, in create_env
plan.execute_actions(actions, index, verbose=config.debug)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/plan.py", line 643, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 132, in execute_instructions
cmd(state, arg)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 77, in LINK_CMD
link(state['prefix'], dist, lt, index=state['index'])
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/install.py", line 973, in link
(placeholder, dist))
conda.exceptions.PaddingError: Padding error:
finished running conda build. logfile: /reg/g/psdm/sw/conda/logs/conda_build_psana_psana-conda-1.0.2-py27_1_rhel7_linux-64.log
sh: -c: line 0: unexpected EOF while looking for matching `''
sh: -c: line 1: syntax error: unexpected end of file
```
An older version of the recipe can be found here
https://github.com/davidslac/manage-lcls-conda-build-system/tree/master/recipes/psana/psana-conda-opt
After backing conda-build back down to 1.19, things look like they are working again, at least conda made the build environment and is running my build script
conda build - exception - padding error
I just updated to the lastest conda-build in defaults, and latest conda conda-env in conda canary, when I run conda build I am getting a 'package error'. Here is my conda environemnt:
```
platform : linux-64
conda version : 4.2.5
conda is private : False
conda-env version : 2.6.0
conda-build version : 2.0.1
python version : 2.7.12.final.0
requests version : 2.10.0
root environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7 (writable)
default environment : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7
envs directories : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/envs
package cache : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/pkgs
channel URLs : file:///reg/g/psdm/sw/conda/channels/system-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/system-rhel7/noarch/
file:///reg/g/psdm/sw/conda/channels/psana-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/psana-rhel7/noarch/
file:///reg/g/psdm/sw/conda/channels/external-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/external-rhel7/noarch/
https://repo.continuum.io/pkgs/free/linux-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/linux-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://conda.anaconda.org/scikit-beam/linux-64/
https://conda.anaconda.org/scikit-beam/noarch/
file:///reg/g/psdm/sw/conda/channels/testing-rhel7/linux-64/
file:///reg/g/psdm/sw/conda/channels/testing-rhel7/noarch/
config file : /reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/.condarc
offline mode : False
```
and here is the build output
```
SIT_ARCH=x86_64-rhel7-gcc48-opt conda build -c file:///reg/g/psdm/sw/conda/channels/system-rhel7 -c file:///reg/g/psdm/sw/conda/channels/psana-rhel7 -c file:///reg/g/psdm/sw/conda/channels/external-rhel7 --quiet recipes/psana/psana-conda-opt 2>&1 | tee -a /reg/g/psdm/sw/conda/logs/conda_build_psana_psana-conda-1.0.2-py27_1_rhel7_linux-64.log
/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/environ.py:303: UserWarning: The environment variable 'SIT_ROOT' is undefined.
UserWarning
BUILD START: psana-conda-1.0.2-py27_1
(actual version deferred until further download or env creation)
The following NEW packages will be INSTALLED:
boost: 1.57.0-4
cairo: 1.12.18-6
cycler: 0.10.0-py27_0
cython: 0.24.1-py27_0
fontconfig: 2.11.1-6
freetype: 2.5.5-1
h5py: 2.5.0-py27_hdf518_mpi4py2_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
hdf5: 1.8.17-openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
icu: 54.1-0
libgfortran: 3.0.0-1
libpng: 1.6.22-0
libsodium: 1.0.10-0
libxml2: 2.9.2-0
matplotlib: 1.5.1-np111py27_0
mkl: 11.3.3-0
mpi4py: 2.0.0-py27_openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
mysql: 5.5.24-0
ndarray: 1.1.5-0 file:///reg/g/psdm/sw/conda/channels/psana-rhel7
numexpr: 2.6.1-np111py27_0
numpy: 1.11.1-py27_0
openmpi: 1.10.3-lsf_verbs_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
openssl: 1.0.2h-1
pip: 8.1.2-py27_0
pixman: 0.32.6-0
pycairo: 1.10.0-py27_0
pyparsing: 2.1.4-py27_0
pyqt: 4.11.4-py27_4
python: 2.7.12-1
python-dateutil: 2.5.3-py27_0
pytz: 2016.6.1-py27_0
pyzmq: 15.4.0-py27_0
qt: 4.8.5-0
readline: 6.2-2
scipy: 0.18.0-np111py27_0
scons: 2.3.0-py27_0
setuptools: 26.1.1-py27_0
sip: 4.18-py27_0
six: 1.10.0-py27_0
sqlite: 3.13.0-0
szip: 2.1-100 file:///reg/g/psdm/sw/conda/channels/external-rhel7
tables: 3.2.3.1-py27_hdf18_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
tk: 8.5.18-0
wheel: 0.29.0-py27_0
zeromq: 4.1.4-0
zlib: 1.2.8-3
The following NEW packages will be INSTALLED:
boost: 1.57.0-4
cairo: 1.12.18-6
cycler: 0.10.0-py27_0
cython: 0.24.1-py27_0
fontconfig: 2.11.1-6
freetype: 2.5.5-1
h5py: 2.5.0-py27_hdf518_mpi4py2_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
hdf5: 1.8.17-openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
icu: 54.1-0
libgfortran: 3.0.0-1
libpng: 1.6.22-0
libsodium: 1.0.10-0
libxml2: 2.9.2-0
matplotlib: 1.5.1-np111py27_0
mkl: 11.3.3-0
mpi4py: 2.0.0-py27_openmpi_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
mysql: 5.5.24-0
ndarray: 1.1.5-0 file:///reg/g/psdm/sw/conda/channels/psana-rhel7
numexpr: 2.6.1-np111py27_0
numpy: 1.11.1-py27_0
openmpi: 1.10.3-lsf_verbs_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
openssl: 1.0.2h-1
pip: 8.1.2-py27_0
pixman: 0.32.6-0
pycairo: 1.10.0-py27_0
pyparsing: 2.1.4-py27_0
pyqt: 4.11.4-py27_4
python: 2.7.12-1
python-dateutil: 2.5.3-py27_0
pytz: 2016.6.1-py27_0
pyzmq: 15.4.0-py27_0
qt: 4.8.5-0
readline: 6.2-2
scipy: 0.18.0-np111py27_0
scons: 2.3.0-py27_0
setuptools: 26.1.1-py27_0
sip: 4.18-py27_0
six: 1.10.0-py27_0
sqlite: 3.13.0-0
szip: 2.1-100 file:///reg/g/psdm/sw/conda/channels/external-rhel7
tables: 3.2.3.1-py27_hdf18_100 file:///reg/g/psdm/sw/conda/channels/system-rhel7
tk: 8.5.18-0
wheel: 0.29.0-py27_0
zeromq: 4.1.4-0
zlib: 1.2.8-3
Traceback (most recent call last):
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/bin/conda-build", line 6, in <module>
sys.exit(conda_build.cli.main_build.main())
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/cli/main_build.py", line 239, in main
execute(sys.argv[1:])
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/cli/main_build.py", line 231, in execute
already_built=None, config=config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/api.py", line 83, in build
need_source_download=need_source_download, config=config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 998, in build_tree
config=recipe_config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 558, in build
create_env(config.build_prefix, specs, config=config)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 451, in create_env
clear_cache=clear_cache)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda_build/build.py", line 427, in create_env
plan.execute_actions(actions, index, verbose=config.debug)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/plan.py", line 643, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 132, in execute_instructions
cmd(state, arg)
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/instructions.py", line 77, in LINK_CMD
link(state['prefix'], dist, lt, index=state['index'])
File "/reg/g/psdm/sw/conda/inst/miniconda2-dev-rhel7/lib/python2.7/site-packages/conda/install.py", line 973, in link
(placeholder, dist))
conda.exceptions.PaddingError: Padding error:
finished running conda build. logfile: /reg/g/psdm/sw/conda/logs/conda_build_psana_psana-conda-1.0.2-py27_1_rhel7_linux-64.log
sh: -c: line 0: unexpected EOF while looking for matching `''
sh: -c: line 1: syntax error: unexpected end of file
```
An older version of the recipe can be found here
https://github.com/davidslac/manage-lcls-conda-build-system/tree/master/recipes/psana/psana-conda-opt
After backing conda-build back down to 1.19, things look like they are working again, at least conda made the build environment and is running my build script
| @davidslac Thanks so much for all of these bug reports. Will investigate and fix them all.
You're welcome!
From: Kale Franz <notifications@github.com<mailto:notifications@github.com>>
Reply-To: conda/conda <reply@reply.github.com<mailto:reply@reply.github.com>>
Date: Monday, September 12, 2016 at 8:28 AM
To: conda/conda <conda@noreply.github.com<mailto:conda@noreply.github.com>>
Cc: David A Schneider <davidsch@slac.stanford.edu<mailto:davidsch@slac.stanford.edu>>, Mention <mention@noreply.github.com<mailto:mention@noreply.github.com>>
Subject: Re: [conda/conda] conda build - exception - padding error (#3407)
@davidslachttps://github.com/davidslac Thanks so much for all of these bug reports. Will investigate and fix them all.
##
You are receiving this because you were mentioned.
Reply to this email directly, view it on GitHubhttps://github.com/conda/conda/issues/3407#issuecomment-246385470, or mute the threadhttps://github.com/notifications/unsubscribe-auth/AQAThWBmDYIZa-Nx_0FK7EluKsLyuG6wks5qpW-HgaJpZM4J6Hdo.
@davidslac I've fixed the other two issues you filed. And I'll make the messaging better on this one.
The issue here is that around conda-build 2.0, we changed the length of the binary path placeholder. Before, it was arbitrarily set to 80 characters, and now we've extended it to the max value we can while still maintaining broad interoperability (255). The downside is that dependencies sometimes have to be rebuilt with the longer padding.
I think we've rebuilt most of the packages in defaults that needed it, but we could have missed some. My guess is it's one of the packages in one of your local file:// channels.
Regardless, I'll make the messaging better so you know exactly which one is the culprit.
@davidslac Thanks so much for all of these bug reports. Will investigate and fix them all.
You're welcome!
From: Kale Franz <notifications@github.com<mailto:notifications@github.com>>
Reply-To: conda/conda <reply@reply.github.com<mailto:reply@reply.github.com>>
Date: Monday, September 12, 2016 at 8:28 AM
To: conda/conda <conda@noreply.github.com<mailto:conda@noreply.github.com>>
Cc: David A Schneider <davidsch@slac.stanford.edu<mailto:davidsch@slac.stanford.edu>>, Mention <mention@noreply.github.com<mailto:mention@noreply.github.com>>
Subject: Re: [conda/conda] conda build - exception - padding error (#3407)
@davidslachttps://github.com/davidslac Thanks so much for all of these bug reports. Will investigate and fix them all.
##
You are receiving this because you were mentioned.
Reply to this email directly, view it on GitHubhttps://github.com/conda/conda/issues/3407#issuecomment-246385470, or mute the threadhttps://github.com/notifications/unsubscribe-auth/AQAThWBmDYIZa-Nx_0FK7EluKsLyuG6wks5qpW-HgaJpZM4J6Hdo.
@davidslac I've fixed the other two issues you filed. And I'll make the messaging better on this one.
The issue here is that around conda-build 2.0, we changed the length of the binary path placeholder. Before, it was arbitrarily set to 80 characters, and now we've extended it to the max value we can while still maintaining broad interoperability (255). The downside is that dependencies sometimes have to be rebuilt with the longer padding.
I think we've rebuilt most of the packages in defaults that needed it, but we could have missed some. My guess is it's one of the packages in one of your local file:// channels.
Regardless, I'll make the messaging better so you know exactly which one is the culprit.
| 2016-09-13T10:29:01 | -1.0 |
conda/conda | 3,454 | conda__conda-3454 | [
"3453"
] | 5980241daa95ec60af005ad1764bbadfa1d244a3 | diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -63,7 +63,7 @@ def check_prefix(prefix, json=False):
if name == ROOT_ENV_NAME:
error = "'%s' is a reserved environment name" % name
if exists(prefix):
- if isdir(prefix) and not os.listdir(prefix):
+ if isdir(prefix) and 'conda-meta' not in os.listdir(prefix):
return None
error = "prefix already exists: %s" % prefix
@@ -164,7 +164,7 @@ def install(args, parser, command='install'):
(name, prefix))
if newenv and not args.no_default_packages:
- default_packages = context.create_default_packages[:]
+ default_packages = list(context.create_default_packages)
# Override defaults if they are specified at the command line
for default_pkg in context.create_default_packages:
if any(pkg.split('=')[0] == default_pkg for pkg in args.packages):
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -19,6 +19,7 @@
from conda.cli.main_update import configure_parser as update_configure_parser
from conda.common.io import captured, disable_logger, stderr_log_level
from conda.common.url import path_to_url
+from conda.common.yaml import yaml_load
from conda.compat import itervalues
from conda.connection import LocalFSAdapter
from conda.install import dist2dirname, linked as install_linked, linked_data, linked_data_, on_win
@@ -46,12 +47,15 @@ def escape_for_winpath(p):
return p.replace('\\', '\\\\')
-def make_temp_prefix(name=None):
+def make_temp_prefix(name=None, create_directory=True):
tempdir = gettempdir()
dirname = str(uuid4())[:8] if name is None else name
prefix = join(tempdir, dirname)
os.makedirs(prefix)
- assert isdir(prefix)
+ if create_directory:
+ assert isdir(prefix)
+ else:
+ os.removedirs(prefix)
return prefix
@@ -591,3 +595,52 @@ def test_shortcut_absent_when_condarc_set(self):
rmtree(prefix, ignore_errors=True)
if isfile(shortcut_file):
os.remove(shortcut_file)
+
+ def test_create_default_packages(self):
+ # Regression test for #3453
+ try:
+ prefix = make_temp_prefix(str(uuid4())[:7])
+
+ # set packages
+ run_command(Commands.CONFIG, prefix, "--add create_default_packages python")
+ run_command(Commands.CONFIG, prefix, "--add create_default_packages pip")
+ run_command(Commands.CONFIG, prefix, "--add create_default_packages flask")
+ stdout, stderr = run_command(Commands.CONFIG, prefix, "--show")
+ yml_obj = yaml_load(stdout)
+ assert yml_obj['create_default_packages'] == ['flask', 'pip', 'python']
+
+ assert not package_is_installed(prefix, 'python-2')
+ assert not package_is_installed(prefix, 'numpy')
+ assert not package_is_installed(prefix, 'flask')
+
+ with make_temp_env("python=2", "numpy", prefix=prefix):
+ assert_package_is_installed(prefix, 'python-2')
+ assert_package_is_installed(prefix, 'numpy')
+ assert_package_is_installed(prefix, 'flask')
+
+ finally:
+ rmtree(prefix, ignore_errors=True)
+
+ def test_create_default_packages_no_default_packages(self):
+ try:
+ prefix = make_temp_prefix(str(uuid4())[:7])
+
+ # set packages
+ run_command(Commands.CONFIG, prefix, "--add create_default_packages python")
+ run_command(Commands.CONFIG, prefix, "--add create_default_packages pip")
+ run_command(Commands.CONFIG, prefix, "--add create_default_packages flask")
+ stdout, stderr = run_command(Commands.CONFIG, prefix, "--show")
+ yml_obj = yaml_load(stdout)
+ assert yml_obj['create_default_packages'] == ['flask', 'pip', 'python']
+
+ assert not package_is_installed(prefix, 'python-2')
+ assert not package_is_installed(prefix, 'numpy')
+ assert not package_is_installed(prefix, 'flask')
+
+ with make_temp_env("python=2", "numpy", "--no-default-packages", prefix=prefix):
+ assert_package_is_installed(prefix, 'python-2')
+ assert_package_is_installed(prefix, 'numpy')
+ assert not package_is_installed(prefix, 'flask')
+
+ finally:
+ rmtree(prefix, ignore_errors=True)
| Conda Fails with create_default_packages
When using `create_default_packages`, `conda create...` fails.
## Sample condarc:
```
channels:
- defaults
create_default_packages:
- python
- pip
```
## Command
`conda create -n test_conda_update python=2 numpy`
## Error
```
Traceback (most recent call last):
File "/opt/a/b/c/muunitnoc/anaconda/lib/python2.7/site-packages/conda/exceptions.py", line 472, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/opt/a/b/c/muunitnoc/anaconda/lib/python2.7/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/opt/a/b/c/muunitnoc/anaconda/lib/python2.7/site-packages/conda/cli/main_create.py", line 68, in execute
install(args, parser, 'create')
File "/opt/a/b/c/muunitnoc/anaconda/lib/python2.7/site-packages/conda/cli/install.py", line 171, in install
default_packages.remove(default_pkg)
AttributeError: 'tuple' object has no attribute 'remove'
```
| 2016-09-16T18:43:46 | -1.0 |
|
conda/conda | 3,521 | conda__conda-3521 | [
"3467",
"3467"
] | 8fa70193de809a03208a1f807e701ee29ddac145 | diff --git a/conda/common/configuration.py b/conda/common/configuration.py
--- a/conda/common/configuration.py
+++ b/conda/common/configuration.py
@@ -637,9 +637,11 @@ def __init__(self, element_type, default=None, aliases=(), validation=None):
def collect_errors(self, instance, value, source="<<merged>>"):
errors = super(MapParameter, self).collect_errors(instance, value)
- element_type = self._element_type
- errors.extend(InvalidElementTypeError(self.name, val, source, type(val), element_type, key)
- for key, val in iteritems(value) if not isinstance(val, element_type))
+ if isinstance(value, Mapping):
+ element_type = self._element_type
+ errors.extend(InvalidElementTypeError(self.name, val, source, type(val),
+ element_type, key)
+ for key, val in iteritems(value) if not isinstance(val, element_type))
return errors
def _merge(self, matches):
| diff --git a/tests/common/test_configuration.py b/tests/common/test_configuration.py
--- a/tests/common/test_configuration.py
+++ b/tests/common/test_configuration.py
@@ -5,7 +5,7 @@
from conda.common.compat import odict, string_types
from conda.common.configuration import (Configuration, MapParameter, ParameterFlag,
PrimitiveParameter, SequenceParameter, YamlRawParameter,
- load_file_configs, MultiValidationError)
+ load_file_configs, MultiValidationError, InvalidTypeError)
from conda.common.yaml import yaml_load
from conda.common.configuration import ValidationError
from os import environ, mkdir
@@ -388,3 +388,12 @@ def test_validate_all(self):
def test_cross_parameter_validation(self):
pass
# test primitive can't be list; list can't be map, etc
+
+ def test_map_parameter_must_be_map(self):
+ # regression test for conda/conda#3467
+ string = dals("""
+ proxy_servers: bad values
+ """)
+ data = odict(s1=YamlRawParameter.make_raw_parameters('s1', yaml_load(string)))
+ config = SampleConfiguration()._add_raw_data(data)
+ raises(InvalidTypeError, config.validate_all)
| Unable to conda update --all
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
```
https://github.com/conda/conda/issues
```
Current conda install:
```
platform : win-64
conda version : 4.2.6
conda is private : False
conda-env version : 4.2.6
conda-build version : 2.0.1
python version : 3.5.2.final.0
requests version : 2.11.1
root environment : C:\Anaconda3 (writable)
default environment : C:\Anaconda3
envs directories : C:\Anaconda3\envs
package cache : C:\Anaconda3\pkgs
channel URLs : https://repo.continuum.io/pkgs/free/win-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/win-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://repo.continuum.io/pkgs/msys2/win-64/
https://repo.continuum.io/pkgs/msys2/noarch/
config file : c:\users\gvdeynde\.condarc
offline mode : False
```
`$ C:\Anaconda3\Scripts\conda-script.py update --all`
```
Traceback (most recent call last):
File "C:\Anaconda3\lib\site-packages\conda\exceptions.py", line 472, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Anaconda3\lib\site-packages\conda\cli\main.py", line 144, in _main
exit_code = args.func(args, p)
File "C:\Anaconda3\lib\site-packages\conda\cli\main_update.py", line 65, in execute
install(args, parser, 'update')
File "C:\Anaconda3\lib\site-packages\conda\cli\install.py", line 139, in install
context.validate_all()
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 752, in validate_all
for source in self.raw_data))
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 752, in <genexpr>
for source in self.raw_data))
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 739, in check_source
collected_errors = parameter.collect_errors(self, typed_value, match.source)
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 642, in collect_errors
for key, val in iteritems(value) if not isinstance(val, element_type))
File "C:\Anaconda3\lib\site-packages\conda\compat.py", line 148, in iteritems
return iter(getattr(d, _iteritems)())
AttributeError: 'str' object has no attribute 'items'
```
Unable to conda update --all
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
```
https://github.com/conda/conda/issues
```
Current conda install:
```
platform : win-64
conda version : 4.2.6
conda is private : False
conda-env version : 4.2.6
conda-build version : 2.0.1
python version : 3.5.2.final.0
requests version : 2.11.1
root environment : C:\Anaconda3 (writable)
default environment : C:\Anaconda3
envs directories : C:\Anaconda3\envs
package cache : C:\Anaconda3\pkgs
channel URLs : https://repo.continuum.io/pkgs/free/win-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/win-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://repo.continuum.io/pkgs/msys2/win-64/
https://repo.continuum.io/pkgs/msys2/noarch/
config file : c:\users\gvdeynde\.condarc
offline mode : False
```
`$ C:\Anaconda3\Scripts\conda-script.py update --all`
```
Traceback (most recent call last):
File "C:\Anaconda3\lib\site-packages\conda\exceptions.py", line 472, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Anaconda3\lib\site-packages\conda\cli\main.py", line 144, in _main
exit_code = args.func(args, p)
File "C:\Anaconda3\lib\site-packages\conda\cli\main_update.py", line 65, in execute
install(args, parser, 'update')
File "C:\Anaconda3\lib\site-packages\conda\cli\install.py", line 139, in install
context.validate_all()
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 752, in validate_all
for source in self.raw_data))
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 752, in <genexpr>
for source in self.raw_data))
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 739, in check_source
collected_errors = parameter.collect_errors(self, typed_value, match.source)
File "C:\Anaconda3\lib\site-packages\conda\common\configuration.py", line 642, in collect_errors
for key, val in iteritems(value) if not isinstance(val, element_type))
File "C:\Anaconda3\lib\site-packages\conda\compat.py", line 148, in iteritems
return iter(getattr(d, _iteritems)())
AttributeError: 'str' object has no attribute 'items'
```
| 2016-09-26T20:38:04 | -1.0 |
|
conda/conda | 3,524 | conda__conda-3524 | [
"3492"
] | 4f96dbd1d29ad9b9476be67217f63edd066b26b0 | diff --git a/conda/base/constants.py b/conda/base/constants.py
--- a/conda/base/constants.py
+++ b/conda/base/constants.py
@@ -99,6 +99,9 @@ class _Null(object):
def __nonzero__(self):
return False
+ def __bool__(self):
+ return False
+
NULL = _Null()
UTF8 = 'UTF-8'
| diff --git a/tests/base/test_constants.py b/tests/base/test_constants.py
new file mode 100644
--- /dev/null
+++ b/tests/base/test_constants.py
@@ -0,0 +1,11 @@
+# -*- coding: utf-8 -*-
+from __future__ import absolute_import, division, print_function, unicode_literals
+
+from conda.base.constants import NULL
+from logging import getLogger
+
+log = getLogger(__name__)
+
+
+def test_null_is_falsey():
+ assert not NULL
| Progress bar broken

```
C:\Users\Korijn\dev\myproject>conda info
Current conda install:
platform : win-64
conda version : 4.2.7
conda is private : False
conda-env version : 4.2.7
conda-build version : 2.0.1
python version : 3.5.1.final.0
requests version : 2.9.1
root environment : C:\Users\Korijn\Miniconda3 (writable)
default environment : C:\Users\Korijn\Miniconda3
envs directories : C:\Users\Korijn\Miniconda3\envs
package cache : C:\Users\Korijn\Miniconda3\pkgs
channel URLs : https://repo.continuum.io/pkgs/free/win-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/win-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://repo.continuum.io/pkgs/msys2/win-64/
https://repo.continuum.io/pkgs/msys2/noarch/
config file : C:\Users\Korijn\.condarc
offline mode : False
```
| I am also having this problem, although only with `conda env` commands (e.g. `conda env create` or `conda env update`). `conda install` works fine.
``` bash
$ conda info
Current conda install:
platform : linux-64
conda version : 4.2.7
conda is private : False
conda-env version : 4.2.7
conda-build version : 2.0.1
python version : 3.5.2.final.0
requests version : 2.11.1
root environment : /home/alan/anaconda (writable)
default environment : /home/alan/anaconda/envs/test
envs directories : /home/alan/anaconda/envs
package cache : /home/alan/anaconda/pkgs
channel URLs : https://conda.anaconda.org/conda-forge/linux-64/
https://conda.anaconda.org/conda-forge/noarch/
https://conda.anaconda.org/conda-canary/linux-64/
https://conda.anaconda.org/conda-canary/noarch/
https://repo.continuum.io/pkgs/free/linux-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/linux-64/
https://repo.continuum.io/pkgs/pro/noarch/
config file : /home/alan/.condarc
offline mode : False
```
| 2016-09-26T22:02:12 | -1.0 |
conda/conda | 3,625 | conda__conda-3625 | [
"3600"
] | 63c67adc0fd9cd187bec79abdd5ca23f8ff73297 | diff --git a/conda/common/disk.py b/conda/common/disk.py
--- a/conda/common/disk.py
+++ b/conda/common/disk.py
@@ -6,7 +6,7 @@
from itertools import chain
from logging import getLogger
from os import W_OK, access, chmod, getpid, listdir, lstat, makedirs, rename, unlink, walk
-from os.path import abspath, basename, dirname, isdir, join, lexists
+from os.path import abspath, basename, dirname, isdir, isfile, islink, join, lexists
from shutil import rmtree
from stat import S_IEXEC, S_IMODE, S_ISDIR, S_ISLNK, S_ISREG, S_IWRITE
from time import sleep
@@ -192,10 +192,10 @@ def rm_rf(path, max_retries=5, trash=True):
backoff_rmdir(path)
finally:
# If path was removed, ensure it's not in linked_data_
- if not isdir(path):
+ if islink(path) or isfile(path):
from conda.install import delete_linked_data_any
delete_linked_data_any(path)
- elif lexists(path):
+ if lexists(path):
try:
backoff_unlink(path)
return True
| diff --git a/tests/common/test_disk.py b/tests/common/test_disk.py
new file mode 100644
--- /dev/null
+++ b/tests/common/test_disk.py
@@ -0,0 +1,58 @@
+import unittest
+import pytest
+from os.path import join, abspath
+import os
+
+from conda.utils import on_win
+from conda.compat import text_type
+from conda.common.disk import rm_rf
+from conda.base.context import context
+
+
+def can_not_symlink():
+ return on_win and context.default_python[0] == '2'
+
+
+def _write_file(path, content):
+ with open(path, "w") as fh:
+ fh.write(content)
+
+
+def test_remove_file(tmpdir):
+ test_file = "test.txt"
+ path = join(text_type(tmpdir), test_file)
+ _write_file(path, "welcome to the ministry of silly walks")
+ assert rm_rf(path) is True
+
+
+@pytest.mark.skipif(not on_win, reason="Testing case for windows is different then Unix")
+def test_remove_file_to_trash(tmpdir):
+ test_file = "test.txt"
+ path = join(text_type(tmpdir), test_file)
+ _write_file(path, "welcome to the ministry of silly walks")
+ assert rm_rf(path) is True
+
+
+def test_remove_dir(tmpdir):
+ test_dir = "test"
+ tmpdir.mkdir(test_dir)
+ path = join(text_type(tmpdir), test_dir)
+ assert rm_rf(path) is True
+
+
+@pytest.mark.skipif(can_not_symlink(), reason="symlink function not available")
+def test_remove_link_to_file(tmpdir):
+ dst_link = join(text_type(tmpdir), "test_link")
+ src_file = join(text_type(tmpdir), "test_file")
+ _write_file(src_file, "welcome to the ministry of silly walks")
+ os.symlink(src_file, dst_link)
+ assert rm_rf(dst_link) is True
+
+
+@pytest.mark.skipif(can_not_symlink(), reason="symlink function not available")
+def test_remove_link_to_dir(tmpdir):
+ dst_link = join(text_type(tmpdir), "test_link")
+ src_dir = join(text_type(tmpdir), "test_dir")
+ tmpdir.mkdir("test_dir")
+ os.symlink(src_dir, dst_link)
+ assert rm_rf(dst_link) is True
| conda update icu (54.1-0 --> 56.1-4 conda-forge)
In a new installation, it appears that going from icu 54 to 56 will fail unless the following is done (at least on linux):
bash Anaconda2-4.2.0-Linux-x86_64.sh
conda remove icu
rm -r $HOME/anaconda2/lib/icu
conda install -c conda-forge icu=56.1
In other words, using the first and fourth lines alone fails with:
CondaOSError: OS error: failed to link (src=u'/home/anaconda2/pkgs/icu-56.1-4/lib/icu/current', dst='/home/anaconda2/lib/icu/current', type=3, error=OSError(17, 'File exists'))
| @rickedanielson could you share the output from `conda info` and the full stack trace for the case when you get the error?
```
> conda info
Current conda install:
platform : linux-64
conda version : 4.2.9
conda is private : False
conda-env version : 4.2.9
conda-build version : 2.0.2
python version : 2.7.12.final.0
requests version : 2.11.1
root environment : $HOME/anaconda2 (writable)
default environment : $HOME/anaconda2
envs directories : $HOME/anaconda2/envs
package cache : $HOME/anaconda2/pkgs
channel URLs : https://conda.anaconda.org/conda-forge/linux-64/
https://conda.anaconda.org/conda-forge/noarch/
https://repo.continuum.io/pkgs/free/linux-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/linux-64/
https://repo.continuum.io/pkgs/pro/noarch/
config file : $HOME/.condarc
offline mode : False
> conda install -c conda-forge icu=56.1
Fetching package metadata .........
Solving package specifications: ..........
Package plan for installation in environment $HOME/anaconda2:
The following packages will be downloaded:
package | build
---------------------------|-----------------
icu-56.1 | 4 21.9 MB conda-forge
conda-env-2.5.2 | py27_0 26 KB conda-forge
conda-4.1.11 | py27_1 204 KB conda-forge
------------------------------------------------------------
Total: 22.1 MB
The following NEW packages will be INSTALLED:
conda-env: 2.5.2-py27_0 conda-forge
The following packages will be UPDATED:
icu: 54.1-0 --> 56.1-4 conda-forge
The following packages will be SUPERCEDED by a higher-priority channel:
conda: 4.2.9-py27_0 --> 4.1.11-py27_1 conda-forge
Proceed ([y]/n)?
Fetching packages ...
icu-56.1-4.tar 100% |#############################################################################################################################################| Time: 0:00:39 583.39 kB/s
conda-env-2.5. 100% |#############################################################################################################################################| Time: 0:00:00 164.50 kB/s
conda-4.1.11-p 100% |#############################################################################################################################################| Time: 0:00:00 303.18 kB/s
Extracting packages ...
[ COMPLETE ]|################################################################################################################################################################| 100%
Unlinking packages ...
[ COMPLETE ]|################################################################################################################################################################| 100%
Linking packages ...
CondaOSError: OS error: failed to link (src=u'$HOME/anaconda2/pkgs/icu-56.1-4/lib/icu/current', dst='$HOME/anaconda2/lib/icu/current', type=3, error=OSError(17, 'File exists'))
> ls -al $HOME/anaconda2/pkgs/icu-56.1-4/lib/icu/current $HOME/anaconda2/lib/icu/current
lrwxrwxrwx 1 here now 4 okt. 10 22:14 $HOME/anaconda2/lib/icu/current -> 54.1
lrwxrwxrwx 1 here now 4 okt. 10 22:16 $HOME/anaconda2/pkgs/icu-56.1-4/lib/icu/current -> 56.1
> cat $HOME/.condarc
channels:
- conda-forge
- defaults
```
| 2016-10-13T23:53:09 | -1.0 |
conda/conda | 3,654 | conda__conda-3654 | [
"3651"
] | 08fcffebd379914df4e3ba781d100d48cde11650 | diff --git a/conda/exports.py b/conda/exports.py
new file mode 100644
--- /dev/null
+++ b/conda/exports.py
@@ -0,0 +1,96 @@
+# -*- coding: utf-8 -*-
+from __future__ import absolute_import, division, print_function, unicode_literals
+
+from functools import partial
+from warnings import warn
+
+from conda import compat, plan
+compat = compat
+plan = plan
+
+from conda.api import get_index # NOQA
+get_index = get_index
+
+from conda.cli.common import (Completer, InstalledPackages, add_parser_channels, add_parser_prefix, # NOQA
+ specs_from_args, spec_from_line, specs_from_url) # NOQA
+Completer, InstalledPackages = Completer, InstalledPackages
+add_parser_channels, add_parser_prefix = add_parser_channels, add_parser_prefix
+specs_from_args, spec_from_line = specs_from_args, spec_from_line
+specs_from_url = specs_from_url
+
+from conda.cli.conda_argparse import ArgumentParser # NOQA
+ArgumentParser = ArgumentParser
+
+from conda.compat import (PY3, StringIO, configparser, input, iteritems, lchmod, string_types, # NOQA
+ text_type, TemporaryDirectory) # NOQA
+PY3, StringIO, configparser, input = PY3, StringIO, configparser, input
+iteritems, lchmod, string_types = iteritems, lchmod, string_types
+text_type, TemporaryDirectory = text_type, TemporaryDirectory
+
+from conda.connection import CondaSession # NOQA
+CondaSession = CondaSession
+
+from conda.fetch import TmpDownload, download, fetch_index # NOQA
+TmpDownload, download, fetch_index = TmpDownload, download, fetch_index
+handle_proxy_407 = lambda x, y: warn("handle_proxy_407 is deprecated. "
+ "Now handled by CondaSession.")
+
+from conda.install import (delete_trash, is_linked, linked, linked_data, move_to_trash, # NOQA
+ prefix_placeholder, rm_rf, symlink_conda, rm_fetched, package_cache) # NOQA
+delete_trash, is_linked, linked = delete_trash, is_linked, linked
+linked_data, move_to_trash = linked_data, move_to_trash
+prefix_placeholder, rm_rf, symlink_conda = prefix_placeholder, rm_rf, symlink_conda
+rm_fetched, package_cache = rm_fetched, package_cache
+
+from conda.lock import Locked # NOQA
+Locked = Locked
+
+from conda.misc import untracked, walk_prefix # NOQA
+untracked, walk_prefix = untracked, walk_prefix
+
+from conda.resolve import MatchSpec, NoPackagesFound, Resolve, Unsatisfiable, normalized_version # NOQA
+MatchSpec, NoPackagesFound, Resolve = MatchSpec, NoPackagesFound, Resolve
+Unsatisfiable, normalized_version = Unsatisfiable, normalized_version
+
+from conda.signature import KEYS, KEYS_DIR, hash_file, verify # NOQA
+KEYS, KEYS_DIR = KEYS, KEYS_DIR
+hash_file, verify = hash_file, verify
+
+from conda.utils import (human_bytes, hashsum_file, md5_file, memoized, unix_path_to_win, # NOQA
+ win_path_to_unix, url_path) # NOQA
+human_bytes, hashsum_file, md5_file = human_bytes, hashsum_file, md5_file
+memoized, unix_path_to_win = memoized, unix_path_to_win
+win_path_to_unix, url_path = win_path_to_unix, url_path
+
+from conda.config import sys_rc_path # NOQA
+sys_rc_path = sys_rc_path
+
+from conda.version import VersionOrder # NOQA
+VersionOrder = VersionOrder
+
+
+import conda.base.context # NOQA
+import conda.exceptions # NOQA
+from conda.base.context import get_prefix as context_get_prefix, non_x86_linux_machines # NOQA
+non_x86_linux_machines = non_x86_linux_machines
+
+from conda.base.constants import DEFAULT_CHANNELS # NOQA
+get_prefix = partial(context_get_prefix, conda.base.context.context)
+get_default_urls = lambda: DEFAULT_CHANNELS
+
+arch_name = conda.base.context.context.arch_name
+binstar_upload = conda.base.context.context.binstar_upload
+bits = conda.base.context.context.bits
+default_prefix = conda.base.context.context.default_prefix
+default_python = conda.base.context.context.default_python
+envs_dirs = conda.base.context.context.envs_dirs
+pkgs_dirs = conda.base.context.context.pkgs_dirs
+platform = conda.base.context.context.platform
+root_dir = conda.base.context.context.root_dir
+root_writable = conda.base.context.context.root_writable
+subdir = conda.base.context.context.subdir
+from conda.models.channel import get_conda_build_local_url # NOQA
+get_rc_urls = lambda: list(conda.base.context.context.channels)
+get_local_urls = lambda: list(get_conda_build_local_url()) or []
+load_condarc = lambda fn: conda.base.context.reset_context([fn])
+PaddingError = conda.exceptions.PaddingError
| diff --git a/tests/test_exports.py b/tests/test_exports.py
new file mode 100644
--- /dev/null
+++ b/tests/test_exports.py
@@ -0,0 +1,7 @@
+# -*- coding: utf-8 -*-
+from __future__ import absolute_import, division, print_function, unicode_literals
+
+
+def test_exports():
+ import conda.exports
+ assert conda.exports.PaddingError
| Removal of handle_proxy_407 breaks conda-build
Elevating as issue from https://github.com/conda/conda/commit/b993816b39a48d807e7a9659246266f6035c3dcd#commitcomment-19452072 so that it does not get lost.
Removal of handle_proxy_407 breaks conda-build (it is used in pypi skeleton code for some reason.) Is there an alternative that I can use instead? Please provide example code.
| 2016-10-17T17:47:56 | -1.0 |
|
conda/conda | 3,684 | conda__conda-3684 | [
"3517",
"3517"
] | 94e75d401e415e17d20a0b789a03f1f0bc85b7dc | diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -142,7 +142,7 @@ def install(args, parser, command='install'):
isinstall = bool(command == 'install')
if newenv:
common.ensure_name_or_prefix(args, command)
- prefix = context.prefix if newenv else context.prefix_w_legacy_search
+ prefix = context.prefix if newenv or args.mkdir else context.prefix_w_legacy_search
if newenv:
check_prefix(prefix, json=context.json)
if context.force_32bit and is_root_prefix(prefix):
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -20,6 +20,7 @@
from conda.cli.main_remove import configure_parser as remove_configure_parser
from conda.cli.main_search import configure_parser as search_configure_parser
from conda.cli.main_update import configure_parser as update_configure_parser
+from conda.common.disk import rm_rf
from conda.common.io import captured, disable_logger, replace_log_streams, stderr_log_level
from conda.common.url import path_to_url
from conda.common.yaml import yaml_load
@@ -801,3 +802,18 @@ def test_clean_index_cache(self):
# now clear it
run_command(Commands.CLEAN, prefix, "--index-cache")
assert not glob(join(index_cache_dir, "*.json"))
+
+ def test_install_mkdir(self):
+ try:
+ prefix = make_temp_prefix()
+ assert isdir(prefix)
+ run_command(Commands.INSTALL, prefix, "python=3.5", "--mkdir")
+ assert_package_is_installed(prefix, "python-3.5")
+
+ rm_rf(prefix)
+ assert not isdir(prefix)
+ run_command(Commands.INSTALL, prefix, "python=3.5", "--mkdir")
+ assert_package_is_installed(prefix, "python-3.5")
+
+ finally:
+ rmtree(prefix, ignore_errors=True)
| --mkdir no longer works
After upgrading to `conda=4.2`:
```
C:\> conda install --mkdir -n name python=3.5
CondaEnvironmentNotFoundError: Could not find environment: name .
You can list all discoverable environments with `conda info --envs`.
```
--mkdir no longer works
After upgrading to `conda=4.2`:
```
C:\> conda install --mkdir -n name python=3.5
CondaEnvironmentNotFoundError: Could not find environment: name .
You can list all discoverable environments with `conda info --envs`.
```
| 2016-10-20T20:34:10 | -1.0 |
|
conda/conda | 3,685 | conda__conda-3685 | [
"3469",
"3469"
] | 94e75d401e415e17d20a0b789a03f1f0bc85b7dc | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -104,6 +104,9 @@ class Context(Configuration):
binstar_upload = PrimitiveParameter(None, aliases=('anaconda_upload',),
parameter_type=(bool, NoneType))
+ _envs_dirs = SequenceParameter(string_types, aliases=('envs_dirs', 'envs_path'),
+ string_delimiter=os.pathsep)
+
@property
def default_python(self):
ver = sys.version_info
@@ -156,8 +159,6 @@ def root_dir(self):
def root_writable(self):
return try_write(self.root_dir)
- _envs_dirs = SequenceParameter(string_types, aliases=('envs_dirs',))
-
@property
def envs_dirs(self):
return tuple(abspath(expanduser(p))
diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -289,6 +289,7 @@ def execute_config(args, parser):
'debug',
'default_channels',
'disallow',
+ 'envs_dirs',
'json',
'offline',
'proxy_servers',
| diff --git a/tests/base/test_context.py b/tests/base/test_context.py
--- a/tests/base/test_context.py
+++ b/tests/base/test_context.py
@@ -1,6 +1,8 @@
# -*- coding: utf-8 -*-
from __future__ import absolute_import, division, print_function, unicode_literals
+import os
+
import pytest
from conda._vendor.auxlib.ish import dals
from conda.base.context import context, reset_context
@@ -8,6 +10,7 @@
from conda.common.configuration import YamlRawParameter
from conda.common.yaml import yaml_load
from conda.models.channel import Channel
+from conda.utils import on_win
from unittest import TestCase
@@ -58,3 +61,23 @@ def test_old_channel_alias(self):
"ftp://new.url:8082/conda-forge/label/dev/linux-64",
"ftp://new.url:8082/conda-forge/label/dev/noarch",
]
+
+ def test_conda_envs_path(self):
+ saved_envs_path = os.environ.get('CONDA_ENVS_PATH')
+ beginning = "C:" + os.sep if on_win else os.sep
+ path1 = beginning + os.sep.join(['my', 'envs', 'dir', '1'])
+ path2 = beginning + os.sep.join(['my', 'envs', 'dir', '2'])
+ try:
+ os.environ['CONDA_ENVS_PATH'] = path1
+ reset_context()
+ assert context.envs_dirs[0] == path1
+
+ os.environ['CONDA_ENVS_PATH'] = os.pathsep.join([path1, path2])
+ reset_context()
+ assert context.envs_dirs[0] == path1
+ assert context.envs_dirs[1] == path2
+ finally:
+ if saved_envs_path:
+ os.environ['CONDA_ENVS_PATH'] = saved_envs_path
+ else:
+ del os.environ['CONDA_ENVS_PATH']
| Conda 4.2.x attempts to create lock files in readonly file-based channels and does not respect CONDA_ENVS_PATH
I am getting warnings and eventually an error after upgrading from 4.1.11 to 4.2.7 relating to lock files and packages attempting to write to shared read-only directories in our continuous build.
I modified `/shared/path/to/miniconda/lib/python2.7/site-packages/conda/lock.py` to find out where conda 4.2.x was putting lock files and found the following:
`WARNING conda.lock:touch(55): Failed to create lock, do not run conda in parallel processes [errno 13] /shared/path/to/vendor/conda/linux-64.pid24915.conda_lock`
`WARNING conda.lock:touch(55): Failed to create lock, do not run conda in parallel processes [errno 13] /shared/path/to/miniconda/pkgs/python-dateutil-2.4.2-py27_0.tar.bz2.pid24915.conda_lock`
`/shared/path/to` is read-only because it is used by multiple build-agents concurrently.
Additional I encountered the following error:
`CondaRuntimeError: Runtime error: RuntimeError: Runtime error: Could not open u'/shared/path/to/miniconda/pkgs/python-dateutil-2.4.2-py27_0.tar.bz2.part' for writing ([Errno 13] Permission denied: u'/shared/path/to/miniconda/pkgs/python-dateutil-2.4.2-py27_0.tar.bz2.part').`
In conda <= 4.1.11 we were able to set the `CONDA_ENVS_PATH` to have a shared installation of conda, but with separate package caches/lock files per build agent on our continuous build server, in order to avoid concurrent use of the package cache.
Two questions:
- How do we disable lock files being placed in read-only shared file-based channels.
- Does `CONDA_ENVS_PATH` no longer override the package cache/lock file directory from the root?
Conda 4.2.x attempts to create lock files in readonly file-based channels and does not respect CONDA_ENVS_PATH
I am getting warnings and eventually an error after upgrading from 4.1.11 to 4.2.7 relating to lock files and packages attempting to write to shared read-only directories in our continuous build.
I modified `/shared/path/to/miniconda/lib/python2.7/site-packages/conda/lock.py` to find out where conda 4.2.x was putting lock files and found the following:
`WARNING conda.lock:touch(55): Failed to create lock, do not run conda in parallel processes [errno 13] /shared/path/to/vendor/conda/linux-64.pid24915.conda_lock`
`WARNING conda.lock:touch(55): Failed to create lock, do not run conda in parallel processes [errno 13] /shared/path/to/miniconda/pkgs/python-dateutil-2.4.2-py27_0.tar.bz2.pid24915.conda_lock`
`/shared/path/to` is read-only because it is used by multiple build-agents concurrently.
Additional I encountered the following error:
`CondaRuntimeError: Runtime error: RuntimeError: Runtime error: Could not open u'/shared/path/to/miniconda/pkgs/python-dateutil-2.4.2-py27_0.tar.bz2.part' for writing ([Errno 13] Permission denied: u'/shared/path/to/miniconda/pkgs/python-dateutil-2.4.2-py27_0.tar.bz2.part').`
In conda <= 4.1.11 we were able to set the `CONDA_ENVS_PATH` to have a shared installation of conda, but with separate package caches/lock files per build agent on our continuous build server, in order to avoid concurrent use of the package cache.
Two questions:
- How do we disable lock files being placed in read-only shared file-based channels.
- Does `CONDA_ENVS_PATH` no longer override the package cache/lock file directory from the root?
| Re: lock files, ran into the same problem today -- conda tries to create lock files for readonly file-based channels which yields a ton of warnings:
``` sh
$ conda create -p ./foobar python=3.5
WARNING conda.lock:touch(53): Failed to create lock, do not run conda in parallel processes [errno 13]
WARNING conda.lock:touch(53): Failed to create lock, do not run conda in parallel processes [errno 13]
WARNING conda.lock:touch(53): Failed to create lock, do not run conda in parallel processes [errno 13]
```
This is a regression from 4.1.x, please fix.
@HugoTian @kalefranz ping
By the way, why does it try to lock at all when all we're doing is reading from an external channel? I get that package cache lock makes sense since it's inherently a mutable thing, but why this?
Anyone?..
We're bumping into this one as well. Has anyone got any info yet?
This is blocking our upgrade to 4.2 as well.
This issue with creating locks in read-only filesystem channels is a breaking our workflow as well
@kalefranz :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face:
@aldanor , I just check the history, previously, failing create lock will be handled with debug level logging . So there is no warning info.
I think there may be 2 solutions, first we change it back to debug, and there will be no warning. Second, we give another detailed warning based on exception types.
@gnarlie , @eyew , can you give us more info about the exception or error that you encountered during upgrade or workflow ?
Thanks in advance
@HugoTian why does Conda attempt to put a lockfile in a file-based channel in the first place? Are you saying all clients of a file-based channel need write access? How does that work with http channels?
@HugoTian The error message I get is: Runtime error: Could not open u/path_to_package/pkgs/packagename.tar.bz2.part' for writing ([Errno 13] Permission denied: ...)
The pkgs directory (and miniconda installation itself) is on a shared filesystem and read-only because that conda instance is shared among multiple continuous build agents. In conda 4.1.x each build agent can maintain its own (temporary) package cache and locks by setting `CONDA_ENVS_PATH`.
If we're going about this incorrectly, what is the proper way to share a conda installation among many agents? Having them each install miniconda to a temporary location before running a build is too slow to be workable in our case.
There's two separate issues discussed here:
1) locking `pkgs` directory which generally is a legit thing to do and which may require further discussion
2) locking remote file channels -- which is a very strange thing to do, anyone care to explain?
1) Locking the package directory makes sense. However, in versions < 4.2.x, one could specify where the package directory was located via CONDA_ENV_PATH. This no longer seems to work as of 4.2.x
2) Agreed.
I think we may bring back the CONDA_ENV_PATH setting, and I am not quite familiar with the cases when dealing with remote file-based channels.
When I design the filelock and directory lock, the primary goal is to lock local file and directory to avoid parallel execution. Sorry that I did not consider the remote file channel case.
This has affected me too. I install to a known location using `-p` so even having to set the `CONDA_ENV_PATH` to a writable location is counter-intuitive. However, it's the only workaround I have, and in 4.2 it's gone too.
Hi there,
we seem to run into the same issue. How is the CONDA_ENV_PATH variable supposed to work? I set "env_dirs" in .condarc to two values:
envs_dirs:
- ~/conda-envs
- /location/of/conda/installation/envs
This works so far as expected (so users installing something get this installed into their home directories (~/conda-envs)). But obviously some locking is still done in the installation dir of conda (which is not writeable for the users).
Would setting "CONDA_ENV_PATH" to some directory solve this issue (if it worked for 4.2.0)?
Cheers, ocarino
Re: lock files, ran into the same problem today -- conda tries to create lock files for readonly file-based channels which yields a ton of warnings:
``` sh
$ conda create -p ./foobar python=3.5
WARNING conda.lock:touch(53): Failed to create lock, do not run conda in parallel processes [errno 13]
WARNING conda.lock:touch(53): Failed to create lock, do not run conda in parallel processes [errno 13]
WARNING conda.lock:touch(53): Failed to create lock, do not run conda in parallel processes [errno 13]
```
This is a regression from 4.1.x, please fix.
@HugoTian @kalefranz ping
By the way, why does it try to lock at all when all we're doing is reading from an external channel? I get that package cache lock makes sense since it's inherently a mutable thing, but why this?
Anyone?..
We're bumping into this one as well. Has anyone got any info yet?
This is blocking our upgrade to 4.2 as well.
This issue with creating locks in read-only filesystem channels is a breaking our workflow as well
@kalefranz :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face: :panda_face:
@aldanor , I just check the history, previously, failing create lock will be handled with debug level logging . So there is no warning info.
I think there may be 2 solutions, first we change it back to debug, and there will be no warning. Second, we give another detailed warning based on exception types.
@gnarlie , @eyew , can you give us more info about the exception or error that you encountered during upgrade or workflow ?
Thanks in advance
@HugoTian why does Conda attempt to put a lockfile in a file-based channel in the first place? Are you saying all clients of a file-based channel need write access? How does that work with http channels?
@HugoTian The error message I get is: Runtime error: Could not open u/path_to_package/pkgs/packagename.tar.bz2.part' for writing ([Errno 13] Permission denied: ...)
The pkgs directory (and miniconda installation itself) is on a shared filesystem and read-only because that conda instance is shared among multiple continuous build agents. In conda 4.1.x each build agent can maintain its own (temporary) package cache and locks by setting `CONDA_ENVS_PATH`.
If we're going about this incorrectly, what is the proper way to share a conda installation among many agents? Having them each install miniconda to a temporary location before running a build is too slow to be workable in our case.
There's two separate issues discussed here:
1) locking `pkgs` directory which generally is a legit thing to do and which may require further discussion
2) locking remote file channels -- which is a very strange thing to do, anyone care to explain?
1) Locking the package directory makes sense. However, in versions < 4.2.x, one could specify where the package directory was located via CONDA_ENV_PATH. This no longer seems to work as of 4.2.x
2) Agreed.
I think we may bring back the CONDA_ENV_PATH setting, and I am not quite familiar with the cases when dealing with remote file-based channels.
When I design the filelock and directory lock, the primary goal is to lock local file and directory to avoid parallel execution. Sorry that I did not consider the remote file channel case.
This has affected me too. I install to a known location using `-p` so even having to set the `CONDA_ENV_PATH` to a writable location is counter-intuitive. However, it's the only workaround I have, and in 4.2 it's gone too.
Hi there,
we seem to run into the same issue. How is the CONDA_ENV_PATH variable supposed to work? I set "env_dirs" in .condarc to two values:
envs_dirs:
- ~/conda-envs
- /location/of/conda/installation/envs
This works so far as expected (so users installing something get this installed into their home directories (~/conda-envs)). But obviously some locking is still done in the installation dir of conda (which is not writeable for the users).
Would setting "CONDA_ENV_PATH" to some directory solve this issue (if it worked for 4.2.0)?
Cheers, ocarino
| 2016-10-20T20:55:54 | -1.0 |
conda/conda | 3,747 | conda__conda-3747 | [
"3732",
"3732"
] | 2604c2bd504996d1acf627ae9dcc1158a1d73fa5 | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -243,7 +243,6 @@ def local_build_root_channel(self):
location, name = url_parts.path.rsplit('/', 1)
if not location:
location = '/'
- assert name == 'conda-bld'
return Channel(scheme=url_parts.scheme, location=location, name=name)
@memoizedproperty
| diff --git a/tests/base/test_context.py b/tests/base/test_context.py
--- a/tests/base/test_context.py
+++ b/tests/base/test_context.py
@@ -1,6 +1,8 @@
# -*- coding: utf-8 -*-
from __future__ import absolute_import, division, print_function, unicode_literals
+from os.path import basename, dirname
+
import os
import pytest
@@ -8,6 +10,7 @@
from conda.base.context import context, reset_context
from conda.common.compat import odict
from conda.common.configuration import YamlRawParameter
+from conda.common.url import path_to_url, join_url
from conda.common.yaml import yaml_load
from conda.models.channel import Channel
from conda.utils import on_win
@@ -81,3 +84,96 @@ def test_conda_envs_path(self):
os.environ['CONDA_ENVS_PATH'] = saved_envs_path
else:
del os.environ['CONDA_ENVS_PATH']
+
+
+ def test_conda_bld_path_1(self):
+ saved_envs_path = os.environ.get('CONDA_BLD_PATH')
+ beginning = "C:" + os.sep if on_win else os.sep
+ path = beginning + os.sep.join(['tmp', 'conda-bld'])
+ url = path_to_url(path)
+ try:
+ os.environ['CONDA_BLD_PATH'] = path
+ reset_context()
+
+ channel = Channel('local')
+ assert channel.channel_name == "local"
+ assert channel.channel_location is None
+ assert channel.platform is None
+ assert channel.package_filename is None
+ assert channel.auth is None
+ assert channel.token is None
+ assert channel.scheme is None
+ assert channel.canonical_name == "local"
+ assert channel.url() is None
+ assert channel.urls() == [
+ join_url(url, context.subdir),
+ join_url(url, 'noarch'),
+ ]
+
+ channel = Channel(url)
+ assert channel.canonical_name == "local"
+ assert channel.platform is None
+ assert channel.package_filename is None
+ assert channel.auth is None
+ assert channel.token is None
+ assert channel.scheme == "file"
+ assert channel.urls() == [
+ join_url(url, context.subdir),
+ join_url(url, 'noarch'),
+ ]
+ assert channel.url() == join_url(url, context.subdir)
+ assert channel.channel_name == basename(path)
+ assert channel.channel_location == path_to_url(dirname(path)).replace('file://', '', 1)
+ assert channel.canonical_name == "local"
+
+ finally:
+ if saved_envs_path:
+ os.environ['CONDA_BLD_PATH'] = saved_envs_path
+ else:
+ del os.environ['CONDA_BLD_PATH']
+
+ def test_conda_bld_path_2(self):
+ saved_envs_path = os.environ.get('CONDA_BLD_PATH')
+ beginning = "C:" + os.sep if on_win else os.sep
+ path = beginning + os.sep.join(['random', 'directory'])
+ url = path_to_url(path)
+ try:
+ os.environ['CONDA_BLD_PATH'] = path
+ reset_context()
+
+ channel = Channel('local')
+ assert channel.channel_name == "local"
+ assert channel.channel_location is None
+ assert channel.platform is None
+ assert channel.package_filename is None
+ assert channel.auth is None
+ assert channel.token is None
+ assert channel.scheme is None
+ assert channel.canonical_name == "local"
+ assert channel.url() is None
+ assert channel.urls() == [
+ join_url(url, context.subdir),
+ join_url(url, 'noarch'),
+ ]
+
+ channel = Channel(url)
+ assert channel.canonical_name == "local"
+ assert channel.platform is None
+ assert channel.package_filename is None
+ assert channel.auth is None
+ assert channel.token is None
+ assert channel.scheme == "file"
+ assert channel.urls() == [
+ join_url(url, context.subdir),
+ join_url(url, 'noarch'),
+ ]
+ assert channel.url() == join_url(url, context.subdir)
+ assert channel.channel_name == basename(path)
+ assert channel.channel_location == path_to_url(dirname(path)).replace('file://', '', 1)
+ assert channel.canonical_name == "local"
+
+ finally:
+ if saved_envs_path:
+ os.environ['CONDA_BLD_PATH'] = saved_envs_path
+ else:
+ del os.environ['CONDA_BLD_PATH']
| Document that $CONDA_BLD_PATH must end with "/conda-bld" (and stop breaking stuff with minor releases)
On September 30, @kalefranz inserted a new assertion (`name == 'conda-bld'`) into `context.py` ([see here](https://github.com/conda/conda/blame/master/conda/base/context.py#L299)) causing `conda info` to fail when `CONDA_BLD_PATH` does not end with `/conda-bld`:
``` console
$ CONDA_BLD_PATH=/tmp conda info
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
`$ /home/user/conda/bin/conda info`
Traceback (most recent call last):
File "/home/user/conda/lib/python3.5/site-packages/conda/exceptions.py", line 479, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main.py", line 145, in _main
exit_code = args.func(args, p)
File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main_info.py", line 225, in execute
channels = list(prioritize_channels(channels).keys())
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 380, in prioritize_channels
channel = Channel(chn)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 161, in __call__
c = Channel.from_value(value)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 211, in from_value
return Channel.from_url(value)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 196, in from_url
return parse_conda_channel_url(url)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 132, in parse_conda_channel_url
configured_token) = _read_channel_configuration(scheme, host, port, path)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 97, in _read_channel_configuration
for name, channel in sorted(context.custom_channels.items(), reverse=True,
File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
cache[inner_attname] = func(self)
File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 265, in custom_channels
all_sources = self.default_channels, (self.local_build_root_channel,), custom_channels
File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
cache[inner_attname] = func(self)
File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 246, in local_build_root_channel
assert name == 'conda-bld'
AssertionError
```
This change was not documented and led to a hard to trace failure of our test suite (we set `$CONDA_BLD_PATH` in our test suite and `conda info` is, e.g., run by `conda build`).
Unfortunately, this was not the first time that conda/conda-build introduced subtle, but breaking changes in a patch release.
Document that $CONDA_BLD_PATH must end with "/conda-bld" (and stop breaking stuff with minor releases)
On September 30, @kalefranz inserted a new assertion (`name == 'conda-bld'`) into `context.py` ([see here](https://github.com/conda/conda/blame/master/conda/base/context.py#L299)) causing `conda info` to fail when `CONDA_BLD_PATH` does not end with `/conda-bld`:
``` console
$ CONDA_BLD_PATH=/tmp conda info
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
`$ /home/user/conda/bin/conda info`
Traceback (most recent call last):
File "/home/user/conda/lib/python3.5/site-packages/conda/exceptions.py", line 479, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main.py", line 145, in _main
exit_code = args.func(args, p)
File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main_info.py", line 225, in execute
channels = list(prioritize_channels(channels).keys())
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 380, in prioritize_channels
channel = Channel(chn)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 161, in __call__
c = Channel.from_value(value)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 211, in from_value
return Channel.from_url(value)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 196, in from_url
return parse_conda_channel_url(url)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 132, in parse_conda_channel_url
configured_token) = _read_channel_configuration(scheme, host, port, path)
File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 97, in _read_channel_configuration
for name, channel in sorted(context.custom_channels.items(), reverse=True,
File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
cache[inner_attname] = func(self)
File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 265, in custom_channels
all_sources = self.default_channels, (self.local_build_root_channel,), custom_channels
File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
cache[inner_attname] = func(self)
File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 246, in local_build_root_channel
assert name == 'conda-bld'
AssertionError
```
This change was not documented and led to a hard to trace failure of our test suite (we set `$CONDA_BLD_PATH` in our test suite and `conda info` is, e.g., run by `conda build`).
Unfortunately, this was not the first time that conda/conda-build introduced subtle, but breaking changes in a patch release.
| Noting the origin is related to https://github.com/conda/conda/issues/3717, and specifically https://github.com/conda/conda/issues/3717#issuecomment-255830103 and subsequent discussion.
I also thought I posted one reply to this already. I guess the comment didn't stick.
This is definitely a bug. Any assertion error is a bug, and we let assertion errors slip through the exception handler and provide an ugly stack trace to emphasize that point.
An assertion error is a bug. Full stop. That's why we let assertion errors have the big ugly stack trace.
I'll work on a fix today.
> On Oct 26, 2016, at 3:58 AM, Stefan Scherfke notifications@github.com wrote:
>
> On September 30, @kalefranz inserted a new assertion (name == 'conda-bld') into context.py (see here) causing conda info to fail when CONDA_BLD_PATH does not end with /conda-bld:
>
> $ CONDA_BLD_PATH=/tmp conda info
> An unexpected error has occurred.
> Please consider posting the following information to the
> conda GitHub issue tracker at:
>
> ```
> https://github.com/conda/conda/issues
> ```
>
> `$ /home/user/conda/bin/conda info`
>
> ```
> Traceback (most recent call last):
> File "/home/user/conda/lib/python3.5/site-packages/conda/exceptions.py", line 479, in conda_exception_handler
> return_value = func(*args, **kwargs)
> File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main.py", line 145, in _main
> exit_code = args.func(args, p)
> File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main_info.py", line 225, in execute
> channels = list(prioritize_channels(channels).keys())
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 380, in prioritize_channels
> channel = Channel(chn)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 161, in __call__
> c = Channel.from_value(value)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 211, in from_value
> return Channel.from_url(value)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 196, in from_url
> return parse_conda_channel_url(url)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 132, in parse_conda_channel_url
> configured_token) = _read_channel_configuration(scheme, host, port, path)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 97, in _read_channel_configuration
> for name, channel in sorted(context.custom_channels.items(), reverse=True,
> File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
> cache[inner_attname] = func(self)
> File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 265, in custom_channels
> all_sources = self.default_channels, (self.local_build_root_channel,), custom_channels
> File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
> cache[inner_attname] = func(self)
> File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 246, in local_build_root_channel
> assert name == 'conda-bld'
> AssertionError
> ```
>
> This change was not documented and led to a hard to trace failure of our test suite (we set $CONDA_BLD_PATH in our test suite and conda info is, e.g., run by conda build).
>
> Unfortunately, this was not the first time that conda/conda-build introduced subtle, but breaking changes in a patch release.
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub, or mute the thread.
Noting the origin is related to https://github.com/conda/conda/issues/3717, and specifically https://github.com/conda/conda/issues/3717#issuecomment-255830103 and subsequent discussion.
I also thought I posted one reply to this already. I guess the comment didn't stick.
This is definitely a bug. Any assertion error is a bug, and we let assertion errors slip through the exception handler and provide an ugly stack trace to emphasize that point.
An assertion error is a bug. Full stop. That's why we let assertion errors have the big ugly stack trace.
I'll work on a fix today.
> On Oct 26, 2016, at 3:58 AM, Stefan Scherfke notifications@github.com wrote:
>
> On September 30, @kalefranz inserted a new assertion (name == 'conda-bld') into context.py (see here) causing conda info to fail when CONDA_BLD_PATH does not end with /conda-bld:
>
> $ CONDA_BLD_PATH=/tmp conda info
> An unexpected error has occurred.
> Please consider posting the following information to the
> conda GitHub issue tracker at:
>
> ```
> https://github.com/conda/conda/issues
> ```
>
> `$ /home/user/conda/bin/conda info`
>
> ```
> Traceback (most recent call last):
> File "/home/user/conda/lib/python3.5/site-packages/conda/exceptions.py", line 479, in conda_exception_handler
> return_value = func(*args, **kwargs)
> File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main.py", line 145, in _main
> exit_code = args.func(args, p)
> File "/home/user/conda/lib/python3.5/site-packages/conda/cli/main_info.py", line 225, in execute
> channels = list(prioritize_channels(channels).keys())
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 380, in prioritize_channels
> channel = Channel(chn)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 161, in __call__
> c = Channel.from_value(value)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 211, in from_value
> return Channel.from_url(value)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 196, in from_url
> return parse_conda_channel_url(url)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 132, in parse_conda_channel_url
> configured_token) = _read_channel_configuration(scheme, host, port, path)
> File "/home/user/conda/lib/python3.5/site-packages/conda/models/channel.py", line 97, in _read_channel_configuration
> for name, channel in sorted(context.custom_channels.items(), reverse=True,
> File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
> cache[inner_attname] = func(self)
> File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 265, in custom_channels
> all_sources = self.default_channels, (self.local_build_root_channel,), custom_channels
> File "/home/user/conda/lib/python3.5/site-packages/conda/_vendor/auxlib/decorators.py", line 265, in new_fget
> cache[inner_attname] = func(self)
> File "/home/user/conda/lib/python3.5/site-packages/conda/base/context.py", line 246, in local_build_root_channel
> assert name == 'conda-bld'
> AssertionError
> ```
>
> This change was not documented and led to a hard to trace failure of our test suite (we set $CONDA_BLD_PATH in our test suite and conda info is, e.g., run by conda build).
>
> Unfortunately, this was not the first time that conda/conda-build introduced subtle, but breaking changes in a patch release.
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub, or mute the thread.
| 2016-10-27T18:45:20 | -1.0 |
conda/conda | 3,748 | conda__conda-3748 | [
"3717",
"3717"
] | 2604c2bd504996d1acf627ae9dcc1158a1d73fa5 | diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -73,9 +73,14 @@ def _get_channel_for_name_helper(name):
def _read_channel_configuration(scheme, host, port, path):
# return location, name, scheme, auth, token
- test_url = Url(host=host, port=port, path=path).url.rstrip('/')
+ path = path and path.rstrip('/')
+ test_url = Url(host=host, port=port, path=path).url
- # Step 1. migrated_custom_channels matches
+ # Step 1. No path given; channel name is None
+ if not path:
+ return Url(host=host, port=port).url.rstrip('/'), None, scheme or None, None, None
+
+ # Step 2. migrated_custom_channels matches
for name, location in sorted(context.migrated_custom_channels.items(), reverse=True,
key=lambda x: len(x[0])):
location, _scheme, _auth, _token = split_scheme_auth_token(location)
@@ -86,14 +91,14 @@ def _read_channel_configuration(scheme, host, port, path):
channel = _get_channel_for_name(channel_name)
return channel.location, channel_name, channel.scheme, channel.auth, channel.token
- # Step 2. migrated_channel_aliases matches
+ # Step 3. migrated_channel_aliases matches
for migrated_alias in context.migrated_channel_aliases:
if test_url.startswith(migrated_alias.location):
name = test_url.replace(migrated_alias.location, '', 1).strip('/')
ca = context.channel_alias
return ca.location, name, ca.scheme, ca.auth, ca.token
- # Step 3. custom_channels matches
+ # Step 4. custom_channels matches
for name, channel in sorted(context.custom_channels.items(), reverse=True,
key=lambda x: len(x[0])):
that_test_url = join_url(channel.location, channel.name)
@@ -102,13 +107,13 @@ def _read_channel_configuration(scheme, host, port, path):
return (channel.location, join_url(channel.name, subname), scheme,
channel.auth, channel.token)
- # Step 4. channel_alias match
+ # Step 5. channel_alias match
ca = context.channel_alias
if ca.location and test_url.startswith(ca.location):
name = test_url.replace(ca.location, '', 1).strip('/') or None
return ca.location, name, scheme, ca.auth, ca.token
- # Step 5. not-otherwise-specified file://-type urls
+ # Step 6. not-otherwise-specified file://-type urls
if host is None:
# this should probably only happen with a file:// type url
assert port is None
@@ -118,7 +123,7 @@ def _read_channel_configuration(scheme, host, port, path):
_scheme, _auth, _token = 'file', None, None
return location, name, _scheme, _auth, _token
- # Step 6. fall through to host:port as channel_location and path as channel_name
+ # Step 7. fall through to host:port as channel_location and path as channel_name
return (Url(host=host, port=port).url.rstrip('/'), path.strip('/') or None,
scheme or None, None, None)
@@ -250,7 +255,7 @@ def canonical_name(self):
return multiname
for that_name in context.custom_channels:
- if tokenized_startswith(self.name.split('/'), that_name.split('/')):
+ if self.name and tokenized_startswith(self.name.split('/'), that_name.split('/')):
return self.name
if any(c.location == self.location
@@ -259,7 +264,7 @@ def canonical_name(self):
# fall back to the equivalent of self.base_url
# re-defining here because base_url for MultiChannel is None
- return "%s://%s/%s" % (self.scheme, self.location, self.name)
+ return "%s://%s" % (self.scheme, join_url(self.location, self.name))
def urls(self, with_credentials=False, platform=None):
base = [self.location]
| diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -5,6 +5,7 @@
from conda.base.context import context, reset_context
from conda.common.compat import odict
from conda.common.configuration import YamlRawParameter
+from conda.common.url import join_url
from conda.common.yaml import yaml_load
from conda.models.channel import Channel
from conda.utils import on_win
@@ -85,6 +86,22 @@ def test_url_channel_w_platform(self):
'https://repo.continuum.io/pkgs/free/noarch',
]
+ def test_bare_channel(self):
+ url = "http://conda-01"
+ channel = Channel(url)
+ assert channel.scheme == "http"
+ assert channel.location == "conda-01"
+ assert channel.platform is None
+ assert channel.canonical_name == url
+ assert channel.name is None
+
+ assert channel.base_url == url
+ assert channel.url() == join_url(url, context.subdir)
+ assert channel.urls() == [
+ join_url(url, context.subdir),
+ join_url(url, 'noarch'),
+ ]
+
class AnacondaServerChannelTests(TestCase):
| regression bug: https://github.com/conda/conda/issues/3235 appears to have resurrected itself in another place
The bug was that conda handled the channel "http://conda-01" incorrectly. Here's the stack trace in conda 4.2.11:
```
Traceback (most recent call last):
File "C:\Miniconda3\lib\site-packages\conda\exceptions.py", line 479, in c
onda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Miniconda3\lib\site-packages\conda\cli\main.py", line 145, in _ma
in
exit_code = args.func(args, p)
File "C:\Miniconda3\lib\site-packages\conda\cli\main_update.py", line 65,
in execute
install(args, parser, 'update')
File "C:\Miniconda3\lib\site-packages\conda\cli\install.py", line 308, in
install
update_deps=context.update_dependencies)
File "C:\Miniconda3\lib\site-packages\conda\plan.py", line 526, in install
_actions
force=force, always_copy=always_copy)
File "C:\Miniconda3\lib\site-packages\conda\plan.py", line 308, in ensure_
linked_actions
fetched_in = is_fetched(dist)
File "C:\Miniconda3\lib\site-packages\conda\install.py", line 727, in is_f
etched
for fn in package_cache().get(dist, {}).get('files', ()):
File "C:\Miniconda3\lib\site-packages\conda\install.py", line 675, in pack
age_cache
add_cached_package(pdir, url)
File "C:\Miniconda3\lib\site-packages\conda\install.py", line 633, in add_
cached_package
schannel = Channel(url).canonical_name
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 161,
in __call__
c = Channel.from_value(value)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 211,
in from_value
return Channel.from_url(value)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 196,
in from_url
return parse_conda_channel_url(url)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 132,
in parse_conda_channel_url
configured_token) = _read_channel_configuration(scheme, host, port, path
)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 122,
in _read_channel_configuration
return (Url(host=host, port=port).url.rstrip('/'), path.strip('/') or No
ne,
AttributeError: 'NoneType' object has no attribute 'strip'
```
regression bug: https://github.com/conda/conda/issues/3235 appears to have resurrected itself in another place
The bug was that conda handled the channel "http://conda-01" incorrectly. Here's the stack trace in conda 4.2.11:
```
Traceback (most recent call last):
File "C:\Miniconda3\lib\site-packages\conda\exceptions.py", line 479, in c
onda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Miniconda3\lib\site-packages\conda\cli\main.py", line 145, in _ma
in
exit_code = args.func(args, p)
File "C:\Miniconda3\lib\site-packages\conda\cli\main_update.py", line 65,
in execute
install(args, parser, 'update')
File "C:\Miniconda3\lib\site-packages\conda\cli\install.py", line 308, in
install
update_deps=context.update_dependencies)
File "C:\Miniconda3\lib\site-packages\conda\plan.py", line 526, in install
_actions
force=force, always_copy=always_copy)
File "C:\Miniconda3\lib\site-packages\conda\plan.py", line 308, in ensure_
linked_actions
fetched_in = is_fetched(dist)
File "C:\Miniconda3\lib\site-packages\conda\install.py", line 727, in is_f
etched
for fn in package_cache().get(dist, {}).get('files', ()):
File "C:\Miniconda3\lib\site-packages\conda\install.py", line 675, in pack
age_cache
add_cached_package(pdir, url)
File "C:\Miniconda3\lib\site-packages\conda\install.py", line 633, in add_
cached_package
schannel = Channel(url).canonical_name
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 161,
in __call__
c = Channel.from_value(value)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 211,
in from_value
return Channel.from_url(value)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 196,
in from_url
return parse_conda_channel_url(url)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 132,
in parse_conda_channel_url
configured_token) = _read_channel_configuration(scheme, host, port, path
)
File "C:\Miniconda3\lib\site-packages\conda\models\channel.py", line 122,
in _read_channel_configuration
return (Url(host=host, port=port).url.rstrip('/'), path.strip('/') or No
ne,
AttributeError: 'NoneType' object has no attribute 'strip'
```
| Adding some print statements gives the following specific variable values from split_conda_url_easy_parts in the innermost calls:
```
url=http://conda-01/win-64/qt5-5.6.1.1-msvc2015rel_2.tar.bz2
host=conda-01
port=None
path=None
query=None
scheme=http
```
Yeah I know where the bug is. 4.2.11 introduced the requirement that every channel have a "name." At least one directory level beyond the host and port. I guess I didn't remember #3235, and was hoping everyone had at least one directory layer.
We need this to allow channels to have locations. That is, we need to uniquely identify channels, but they also need to be able to move locations. Each channel needs a deterministic name. 4.2.11 does that, but it assumes at least one directory level beyond the network location.
Cool, I guess the fix is to allow an empty string as the unique name, replacing None?
Maybe. I'm not sure just yet. Thinking...
Need to chat with @mcg1969 about this. But I'm out of the office today.
@mwiebe How invasive would it be to your infrastructure to add one directory level? Even if I hack a fix here, I think you're going to want to do this in the future.
We're progressively moving toward making "channel" a first-class-citizen in a package specification. You'll be able to specify channel along with package on the command line. Likely something similar to
```
conda install channel_name/package_name
```
Note there I have `channel_name`, because channels fundamentally need to move locations with proper configuration. The no-channel-name/empty-channel-name case just really doesn't work here.
I'm still thinking on solutions though...
Either way this is definitely a bug. Whether we ultimately find a way to handle no-name channels cleanly, or just need to alert that the specified channel is invalid.
It's definitely something we can do, just in a phased way. This bug basically trickled down to the "reinstall miniconda" fix.
In the system you're moving towards, how is a channel on anaconda.org distinguished from a channel on someone's local server? If we make http://conda-01/stable the location where we put packages once we're happy with them for development environments, how should that interact with the wholly separate anaconda/"defaults" set of channels?
There's very robust configuration for all of that. New in 4.2. The docs are behind; still working on those.
But, to answer your exact question, all you would do is add
```
custom_channels:
stable: http://conda-01
```
in your condarc file. (There are now multiple places for condarc configs, including in activated env directories.)
I'm seeing this also. I reference a channel of the form 'http://conda.ourserver.com' that I don't have control over right now. Any work around?
```
platform : linux-64
conda version : 4.2.11
conda is private : False
conda-env version : 4.2.11
conda-build version : 1.18.2
python version : 2.7.11.final.0
requests version : 2.11.1
```
Best workaround I've come up with is to wipe out all the contents of the `<anaconda>/pkgs` directory, then downgrade to 4.2.9.
Adding some print statements gives the following specific variable values from split_conda_url_easy_parts in the innermost calls:
```
url=http://conda-01/win-64/qt5-5.6.1.1-msvc2015rel_2.tar.bz2
host=conda-01
port=None
path=None
query=None
scheme=http
```
Yeah I know where the bug is. 4.2.11 introduced the requirement that every channel have a "name." At least one directory level beyond the host and port. I guess I didn't remember #3235, and was hoping everyone had at least one directory layer.
We need this to allow channels to have locations. That is, we need to uniquely identify channels, but they also need to be able to move locations. Each channel needs a deterministic name. 4.2.11 does that, but it assumes at least one directory level beyond the network location.
Cool, I guess the fix is to allow an empty string as the unique name, replacing None?
Maybe. I'm not sure just yet. Thinking...
Need to chat with @mcg1969 about this. But I'm out of the office today.
@mwiebe How invasive would it be to your infrastructure to add one directory level? Even if I hack a fix here, I think you're going to want to do this in the future.
We're progressively moving toward making "channel" a first-class-citizen in a package specification. You'll be able to specify channel along with package on the command line. Likely something similar to
```
conda install channel_name/package_name
```
Note there I have `channel_name`, because channels fundamentally need to move locations with proper configuration. The no-channel-name/empty-channel-name case just really doesn't work here.
I'm still thinking on solutions though...
Either way this is definitely a bug. Whether we ultimately find a way to handle no-name channels cleanly, or just need to alert that the specified channel is invalid.
It's definitely something we can do, just in a phased way. This bug basically trickled down to the "reinstall miniconda" fix.
In the system you're moving towards, how is a channel on anaconda.org distinguished from a channel on someone's local server? If we make http://conda-01/stable the location where we put packages once we're happy with them for development environments, how should that interact with the wholly separate anaconda/"defaults" set of channels?
There's very robust configuration for all of that. New in 4.2. The docs are behind; still working on those.
But, to answer your exact question, all you would do is add
```
custom_channels:
stable: http://conda-01
```
in your condarc file. (There are now multiple places for condarc configs, including in activated env directories.)
I'm seeing this also. I reference a channel of the form 'http://conda.ourserver.com' that I don't have control over right now. Any work around?
```
platform : linux-64
conda version : 4.2.11
conda is private : False
conda-env version : 4.2.11
conda-build version : 1.18.2
python version : 2.7.11.final.0
requests version : 2.11.1
```
Best workaround I've come up with is to wipe out all the contents of the `<anaconda>/pkgs` directory, then downgrade to 4.2.9.
| 2016-10-27T19:38:37 | -1.0 |
conda/conda | 3,969 | conda__conda-3969 | [
"4378"
] | ca57ef2a1ac70355d1aeb58ddc2da78f655c4fbc | diff --git a/conda/egg_info.py b/conda/egg_info.py
--- a/conda/egg_info.py
+++ b/conda/egg_info.py
@@ -30,6 +30,10 @@ def get_site_packages_dir(installed_pkgs):
def get_egg_info_files(sp_dir):
for fn in os.listdir(sp_dir):
+ if fn.endswith('.egg-link'):
+ with open(join(sp_dir, fn), 'r') as reader:
+ for egg in get_egg_info_files(reader.readline().strip()):
+ yield egg
if not fn.endswith(('.egg', '.egg-info', '.dist-info')):
continue
path = join(sp_dir, fn)
diff --git a/conda_env/installers/pip.py b/conda_env/installers/pip.py
--- a/conda_env/installers/pip.py
+++ b/conda_env/installers/pip.py
@@ -1,13 +1,56 @@
from __future__ import absolute_import
+
+import os
+import os.path as op
import subprocess
+import tempfile
from conda_env.pip_util import pip_args
from conda.exceptions import CondaValueError
-def install(prefix, specs, args, env, prune=False):
- pip_cmd = pip_args(prefix) + ['install', ] + specs
- process = subprocess.Popen(pip_cmd, universal_newlines=True)
- process.communicate()
+def _pip_install_via_requirements(prefix, specs, args, *_):
+ """
+ Installs the pip dependencies in specs using a temporary pip requirements file.
+
+ Args
+ ----
+ prefix: string
+ The path to the python and pip executables.
+
+ specs: iterable of strings
+ Each element should be a valid pip dependency.
+ See: https://pip.pypa.io/en/stable/user_guide/#requirements-files
+ https://pip.pypa.io/en/stable/reference/pip_install/#requirements-file-format
+ """
+ try:
+ pip_workdir = op.dirname(op.abspath(args.file))
+ except AttributeError:
+ pip_workdir = None
+ requirements = None
+ try:
+ # Generate the temporary requirements file
+ requirements = tempfile.NamedTemporaryFile(mode='w',
+ prefix='condaenv.',
+ suffix='.requirements.txt',
+ dir=pip_workdir,
+ delete=False)
+ requirements.write('\n'.join(specs))
+ requirements.close()
+ # pip command line...
+ pip_cmd = pip_args(prefix) + ['install', '-r', requirements.name]
+ # ...run it
+ process = subprocess.Popen(pip_cmd,
+ cwd=pip_workdir,
+ universal_newlines=True)
+ process.communicate()
+ if process.returncode != 0:
+ raise CondaValueError("pip returned an error")
+ finally:
+ # Win/Appveyor does not like it if we use context manager + delete=True.
+ # So we delete the temporary file in a finally block.
+ if requirements is not None and op.isfile(requirements.name):
+ os.remove(requirements.name)
+
- if process.returncode != 0:
- raise CondaValueError("pip returned an error.")
+# Conform to Installers API
+install = _pip_install_via_requirements
| diff --git a/tests/conda_env/installers/test_pip.py b/tests/conda_env/installers/test_pip.py
--- a/tests/conda_env/installers/test_pip.py
+++ b/tests/conda_env/installers/test_pip.py
@@ -4,21 +4,40 @@
except ImportError:
import mock
+import os
from conda_env.installers import pip
from conda.exceptions import CondaValueError
+
class PipInstallerTest(unittest.TestCase):
def test_straight_install(self):
- with mock.patch.object(pip.subprocess, 'Popen') as popen:
- popen.return_value.returncode = 0
- with mock.patch.object(pip, 'pip_args') as pip_args:
- pip_args.return_value = ['pip']
- pip.install('/some/prefix', ['foo'], '', '')
+ # To check that the correct file would be written
+ written_deps = []
+
+ def log_write(text):
+ written_deps.append(text)
+ return mock.DEFAULT
- popen.assert_called_with(['pip', 'install', 'foo'],
- universal_newlines=True)
- self.assertEqual(1, popen.return_value.communicate.call_count)
+ with mock.patch.object(pip.subprocess, 'Popen') as mock_popen, \
+ mock.patch.object(pip, 'pip_args') as mock_pip_args, \
+ mock.patch('tempfile.NamedTemporaryFile', mock.mock_open()) as mock_namedtemp:
+ # Mock
+ mock_popen.return_value.returncode = 0
+ mock_pip_args.return_value = ['pip']
+ mock_namedtemp.return_value.write.side_effect = log_write
+ mock_namedtemp.return_value.name = 'tmp-file'
+ args = mock.Mock()
+ root_dir = '/whatever' if os.name != 'nt' else 'C:\\whatever'
+ args.file = os.path.join(root_dir, 'environment.yml')
+ # Run
+ pip.install('/some/prefix', ['foo', '-e ./bar'], args)
+ # Check expectations
+ mock_popen.assert_called_with(['pip', 'install', '-r', 'tmp-file'],
+ cwd=root_dir,
+ universal_newlines=True)
+ self.assertEqual(1, mock_popen.return_value.communicate.call_count)
+ self.assertEqual(written_deps, ['foo\n-e ./bar'])
def test_stops_on_exception(self):
with mock.patch.object(pip.subprocess, 'Popen') as popen:
@@ -28,4 +47,4 @@ def test_stops_on_exception(self):
pip_args.return_value = ['pip']
self.assertRaises(CondaValueError, pip.install,
- '/some/prefix', ['foo'], '', '')
+ '/some/prefix', ['foo'], None)
diff --git a/tests/conda_env/support/advanced-pip/.gitignore b/tests/conda_env/support/advanced-pip/.gitignore
new file mode 100644
--- /dev/null
+++ b/tests/conda_env/support/advanced-pip/.gitignore
@@ -0,0 +1 @@
+src
diff --git a/tests/conda_env/support/advanced-pip/another-project-requirements.txt b/tests/conda_env/support/advanced-pip/another-project-requirements.txt
new file mode 100644
--- /dev/null
+++ b/tests/conda_env/support/advanced-pip/another-project-requirements.txt
@@ -0,0 +1 @@
+six
diff --git a/tests/conda_env/support/advanced-pip/environment.yml b/tests/conda_env/support/advanced-pip/environment.yml
new file mode 100644
--- /dev/null
+++ b/tests/conda_env/support/advanced-pip/environment.yml
@@ -0,0 +1,29 @@
+name: advanced-pip-example
+
+dependencies:
+ - pip
+ - pip:
+
+ # Global options can be tweaked.
+ # For example, if you want to use a pypi mirror first:
+ - --index-url https://pypi.doubanio.com/simple
+ - --extra-index-url https://pypi.python.org/simple
+ # (check https://www.pypi-mirrors.org/)
+
+ # Current syntax still works
+ - xmltodict==0.10.2
+
+ # Install in editable mode.
+ # More natural than - "--editable=git+https://github.com/neithere/argh.git#egg=argh
+ - -e git+https://github.com/neithere/argh.git#egg=argh
+
+ # You could also specify a package in a directory.
+ # The directory can be relative to this environment file.
+ - -e ./module_to_install_in_editable_mode
+
+ # Use another requirements file.
+ # Note that here also we can use relative paths.
+ # pip will be run from the environment file directory, if provided.
+ - -r another-project-requirements.txt
+
+ # Anything else that pip requirement files allows should work seamlessly...
diff --git a/tests/conda_env/support/advanced-pip/module_to_install_in_editable_mode/setup.py b/tests/conda_env/support/advanced-pip/module_to_install_in_editable_mode/setup.py
new file mode 100644
--- /dev/null
+++ b/tests/conda_env/support/advanced-pip/module_to_install_in_editable_mode/setup.py
@@ -0,0 +1,6 @@
+from setuptools import setup
+
+setup(
+ name='module_to_install_in_editable_mode',
+ packages=[],
+)
diff --git a/tests/conda_env/test_create.py b/tests/conda_env/test_create.py
--- a/tests/conda_env/test_create.py
+++ b/tests/conda_env/test_create.py
@@ -2,10 +2,12 @@
from __future__ import absolute_import, division, print_function
from argparse import ArgumentParser
+from unittest import TestCase
from conda.base.context import reset_context
from conda.common.io import env_var
+from conda.egg_info import get_egg_info
from conda.exports import text_type
from contextlib import contextmanager
from logging import getLogger, Handler
@@ -13,7 +15,6 @@
from shlex import split
from shutil import rmtree
from tempfile import mkdtemp
-from unittest import TestCase
from uuid import uuid4
import pytest
@@ -60,7 +61,7 @@ class Commands:
}
-def run_command(command, envs_dir, env_name, *arguments):
+def run_command(command, env_name, *arguments):
p = ArgumentParser()
sub_parsers = p.add_subparsers(metavar='command', dest='cmd')
parser_config[command](sub_parsers)
@@ -81,8 +82,8 @@ def make_temp_envs_dir():
rmtree(envs_dir, ignore_errors=True)
-def package_is_installed(prefix, dist, exact=False):
- packages = list(linked(prefix))
+def package_is_installed(prefix, dist, exact=False, pip=False):
+ packages = list(get_egg_info(prefix) if pip else linked(prefix))
if '::' not in text_type(dist):
packages = [p.dist_name for p in packages]
if exact:
@@ -90,8 +91,8 @@ def package_is_installed(prefix, dist, exact=False):
return any(p.startswith(dist) for p in packages)
-def assert_package_is_installed(prefix, package, exact=False):
- if not package_is_installed(prefix, package, exact):
+def assert_package_is_installed(prefix, package, exact=False, pip=False):
+ if not package_is_installed(prefix, package, exact, pip):
print(list(linked(prefix)))
raise AssertionError("package {0} is not in prefix".format(package))
@@ -112,10 +113,25 @@ def test_create_update(self):
prefix = join(envs_dir, env_name)
python_path = join(prefix, PYTHON_BINARY)
- run_command(Commands.CREATE, envs_dir, env_name, utils.support_file('example/environment_pinned.yml'))
+ run_command(Commands.CREATE, env_name, utils.support_file('example/environment_pinned.yml'))
assert exists(python_path)
assert_package_is_installed(prefix, 'flask-0.9')
- run_command(Commands.UPDATE, envs_dir, env_name, utils.support_file('example/environment_pinned_updated.yml'))
+ run_command(Commands.UPDATE, env_name, utils.support_file('example/environment_pinned_updated.yml'))
assert_package_is_installed(prefix, 'flask-0.10.1')
assert not package_is_installed(prefix, 'flask-0.9')
+
+ def test_create_advanced_pip(self):
+ with make_temp_envs_dir() as envs_dir:
+ with env_var('CONDA_ENVS_DIRS', envs_dir, reset_context):
+ env_name = str(uuid4())[:8]
+ prefix = join(envs_dir, env_name)
+ python_path = join(prefix, PYTHON_BINARY)
+
+ run_command(Commands.CREATE, env_name,
+ utils.support_file('advanced-pip/environment.yml'))
+ assert exists(python_path)
+ assert_package_is_installed(prefix, 'argh', exact=False, pip=True)
+ assert_package_is_installed(prefix, 'module-to-install-in-editable-mode', exact=False, pip=True)
+ assert_package_is_installed(prefix, 'six', exact=False, pip=True)
+ assert_package_is_installed(prefix, 'xmltodict-0.10.2-<pip>', exact=True, pip=True)
| Invalid requirement while trying to use pip options
Hi!
I have in my pip section inside envrionment.yaml file this line
```- rep --install-option='--no-deps'```
while I am trying to update my environment I am getting this error
```Invalid requirement: 'rep --install-option='--no-deps''```
if I do pip -r requirements.txt and I have that line as it is in requirements.txt it works.
| 2016-11-30T07:37:55 | -1.0 |
|
conda/conda | 4,100 | conda__conda-4100 | [
"4097",
"4097"
] | cdd0d5ab8ec583768875aab047de65040bead9cf | diff --git a/conda/base/constants.py b/conda/base/constants.py
--- a/conda/base/constants.py
+++ b/conda/base/constants.py
@@ -12,7 +12,10 @@
from os.path import join
on_win = bool(sys.platform == "win32")
-PREFIX_PLACEHOLDER = '/opt/anaconda1anaconda2anaconda3'
+PREFIX_PLACEHOLDER = ('/opt/anaconda1anaconda2'
+ # this is intentionally split into parts, such that running
+ # this program on itself will leave it unchanged
+ 'anaconda3')
machine_bits = 8 * tuple.__itemsize__
| diff --git a/tests/core/test_portability.py b/tests/core/test_portability.py
--- a/tests/core/test_portability.py
+++ b/tests/core/test_portability.py
@@ -51,11 +51,11 @@ def test_shebang_regex_matches(self):
def test_replace_long_shebang(self):
content_line = b"content line " * 5
- # # simple shebang no replacement
- # shebang = b"#!/simple/shebang/escaped\\ space --and --flags -x"
- # data = b'\n'.join((shebang, content_line, content_line, content_line))
- # new_data = replace_long_shebang(FileMode.text, data)
- # assert data == new_data
+ # simple shebang no replacement
+ shebang = b"#!/simple/shebang/escaped\\ space --and --flags -x"
+ data = b'\n'.join((shebang, content_line, content_line, content_line))
+ new_data = replace_long_shebang(FileMode.text, data)
+ assert data == new_data
# long shebang with truncation
# executable name is 'escaped space'
| On Windows, conda 4.0.5-py35_0 cannot be updated to 4.3.0-py35_1
On a fresh install of the latest Miniconda on Windows, the following fails:
`conda update -c conda-canary --all`
Giving:
```
Fetching package metadata: ......
Solving package specifications: .........
Package plan for installation in environment C:\Users\ray\m2-x64-3.5:
The following packages will be downloaded:
package | build
---------------------------|-----------------
conda-env-2.6.0 | 0 498 B
vs2015_runtime-14.0.25123 | 0 1.9 MB
python-3.5.2 | 0 30.3 MB
pycosat-0.6.1 | py35_1 80 KB
pycrypto-2.6.1 | py35_4 481 KB
pywin32-220 | py35_1 10.4 MB
pyyaml-3.12 | py35_0 118 KB
requests-2.12.4 | py35_0 791 KB
ruamel_yaml-0.11.14 | py35_0 217 KB
setuptools-27.2.0 | py35_1 761 KB
menuinst-1.4.2 | py35_1 108 KB
pip-9.0.1 | py35_1 1.7 MB
conda-4.3.0 | py35_1 510 KB
------------------------------------------------------------
Total: 47.3 MB
The following NEW packages will be INSTALLED:
pywin32: 220-py35_1
ruamel_yaml: 0.11.14-py35_0
The following packages will be UPDATED:
conda: 4.0.5-py35_0 --> 4.3.0-py35_1
conda-env: 2.4.5-py35_0 --> 2.6.0-0
menuinst: 1.3.2-py35_0 --> 1.4.2-py35_1
pip: 8.1.1-py35_1 --> 9.0.1-py35_1
pycosat: 0.6.1-py35_0 --> 0.6.1-py35_1
pycrypto: 2.6.1-py35_3 --> 2.6.1-py35_4
python: 3.5.1-4 --> 3.5.2-0
pyyaml: 3.11-py35_3 --> 3.12-py35_0
requests: 2.9.1-py35_0 --> 2.12.4-py35_0
setuptools: 20.3-py35_0 --> 27.2.0-py35_1
vs2015_runtime: 14.00.23026.0-0 --> 14.0.25123-0
Proceed ([y]/n)? y
menuinst-1.4.2 100% |###############################| Time: 0:00:00 2.35 MB/s
Fetching packages ...
conda-env-2.6. 100% |###############################| Time: 0:00:00 0.00 B/s
vs2015_runtime 100% |###############################| Time: 0:00:00 9.24 MB/s
python-3.5.2-0 100% |###############################| Time: 0:00:02 11.57 MB/s
pycosat-0.6.1- 100% |###############################| Time: 0:00:00 2.61 MB/s
pycrypto-2.6.1 100% |###############################| Time: 0:00:00 4.51 MB/s
pywin32-220-py 100% |###############################| Time: 0:00:00 10.85 MB/s
pyyaml-3.12-py 100% |###############################| Time: 0:00:00 2.57 MB/s
requests-2.12. 100% |###############################| Time: 0:00:00 5.76 MB/s
ruamel_yaml-0. 100% |###############################| Time: 0:00:00 2.84 MB/s
setuptools-27. 100% |###############################| Time: 0:00:00 4.53 MB/s
pip-9.0.1-py35 100% |###############################| Time: 0:00:00 5.70 MB/s
conda-4.3.0-py 100% |###############################| Time: 0:00:00 530.91 kB/s
Extracting packages ...
[ COMPLETE ]|##################################################| 100%
Unlinking packages ...
[ COMPLETE ]|##################################################| 100%
Linking packages ...
[ COMPLETE ]|##################################################| 100%
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 516, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 84, in _main
from ..base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\Scripts\conda-script.py", line 5, in <module>
sys.exit(conda.cli.main())
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 152, in main
return conda_exception_handler(_main)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 532, in conda_exception_handler
print_unexpected_error_message(e)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 463, in print_unexpected_error_message
from conda.base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 516, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 84, in _main
from ..base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\Scripts\conda-script.py", line 5, in <module>
sys.exit(conda.cli.main())
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 152, in main
return conda_exception_handler(_main)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 532, in conda_exception_handler
print_unexpected_error_message(e)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 463, in print_unexpected_error_message
from conda.base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
```
On Windows, conda 4.0.5-py35_0 cannot be updated to 4.3.0-py35_1
On a fresh install of the latest Miniconda on Windows, the following fails:
`conda update -c conda-canary --all`
Giving:
```
Fetching package metadata: ......
Solving package specifications: .........
Package plan for installation in environment C:\Users\ray\m2-x64-3.5:
The following packages will be downloaded:
package | build
---------------------------|-----------------
conda-env-2.6.0 | 0 498 B
vs2015_runtime-14.0.25123 | 0 1.9 MB
python-3.5.2 | 0 30.3 MB
pycosat-0.6.1 | py35_1 80 KB
pycrypto-2.6.1 | py35_4 481 KB
pywin32-220 | py35_1 10.4 MB
pyyaml-3.12 | py35_0 118 KB
requests-2.12.4 | py35_0 791 KB
ruamel_yaml-0.11.14 | py35_0 217 KB
setuptools-27.2.0 | py35_1 761 KB
menuinst-1.4.2 | py35_1 108 KB
pip-9.0.1 | py35_1 1.7 MB
conda-4.3.0 | py35_1 510 KB
------------------------------------------------------------
Total: 47.3 MB
The following NEW packages will be INSTALLED:
pywin32: 220-py35_1
ruamel_yaml: 0.11.14-py35_0
The following packages will be UPDATED:
conda: 4.0.5-py35_0 --> 4.3.0-py35_1
conda-env: 2.4.5-py35_0 --> 2.6.0-0
menuinst: 1.3.2-py35_0 --> 1.4.2-py35_1
pip: 8.1.1-py35_1 --> 9.0.1-py35_1
pycosat: 0.6.1-py35_0 --> 0.6.1-py35_1
pycrypto: 2.6.1-py35_3 --> 2.6.1-py35_4
python: 3.5.1-4 --> 3.5.2-0
pyyaml: 3.11-py35_3 --> 3.12-py35_0
requests: 2.9.1-py35_0 --> 2.12.4-py35_0
setuptools: 20.3-py35_0 --> 27.2.0-py35_1
vs2015_runtime: 14.00.23026.0-0 --> 14.0.25123-0
Proceed ([y]/n)? y
menuinst-1.4.2 100% |###############################| Time: 0:00:00 2.35 MB/s
Fetching packages ...
conda-env-2.6. 100% |###############################| Time: 0:00:00 0.00 B/s
vs2015_runtime 100% |###############################| Time: 0:00:00 9.24 MB/s
python-3.5.2-0 100% |###############################| Time: 0:00:02 11.57 MB/s
pycosat-0.6.1- 100% |###############################| Time: 0:00:00 2.61 MB/s
pycrypto-2.6.1 100% |###############################| Time: 0:00:00 4.51 MB/s
pywin32-220-py 100% |###############################| Time: 0:00:00 10.85 MB/s
pyyaml-3.12-py 100% |###############################| Time: 0:00:00 2.57 MB/s
requests-2.12. 100% |###############################| Time: 0:00:00 5.76 MB/s
ruamel_yaml-0. 100% |###############################| Time: 0:00:00 2.84 MB/s
setuptools-27. 100% |###############################| Time: 0:00:00 4.53 MB/s
pip-9.0.1-py35 100% |###############################| Time: 0:00:00 5.70 MB/s
conda-4.3.0-py 100% |###############################| Time: 0:00:00 530.91 kB/s
Extracting packages ...
[ COMPLETE ]|##################################################| 100%
Unlinking packages ...
[ COMPLETE ]|##################################################| 100%
Linking packages ...
[ COMPLETE ]|##################################################| 100%
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 516, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 84, in _main
from ..base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\Scripts\conda-script.py", line 5, in <module>
sys.exit(conda.cli.main())
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 152, in main
return conda_exception_handler(_main)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 532, in conda_exception_handler
print_unexpected_error_message(e)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 463, in print_unexpected_error_message
from conda.base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 516, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 84, in _main
from ..base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:\Users\ray\m2-x64-3.5\Scripts\conda-script.py", line 5, in <module>
sys.exit(conda.cli.main())
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\cli\main.py", line 152, in main
return conda_exception_handler(_main)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 532, in conda_exception_handler
print_unexpected_error_message(e)
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\exceptions.py", line 463, in print_unexpected_error_message
from conda.base.context import context
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\context.py", line 12, in <module>
from .constants import (APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS, ROOT_ENV_NAME,
File "C:\Users\ray\m2-x64-3.5\lib\site-packages\conda\base\constants.py", line 15
PREFIX_PLACEHOLDER = 'C:\Users\ray\m2-x64-3.5'
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
```
| 2016-12-19T01:03:44 | -1.0 |
|
conda/conda | 4,397 | conda__conda-4397 | [
"4393"
] | 500cbff008b37136ee66e7b213ee23d5f1ea7514 | diff --git a/conda/core/path_actions.py b/conda/core/path_actions.py
--- a/conda/core/path_actions.py
+++ b/conda/core/path_actions.py
@@ -601,12 +601,12 @@ def __init__(self, transaction_context, linked_package_data, target_prefix, targ
def execute(self):
if self.link_type != LinkType.directory:
log.trace("renaming %s => %s", self.target_short_path, self.holding_short_path)
- backoff_rename(self.target_full_path, self.holding_full_path)
+ backoff_rename(self.target_full_path, self.holding_full_path, force=True)
def reverse(self):
if self.link_type != LinkType.directory and lexists(self.holding_full_path):
log.trace("reversing rename %s => %s", self.holding_short_path, self.target_short_path)
- backoff_rename(self.holding_full_path, self.target_full_path)
+ backoff_rename(self.holding_full_path, self.target_full_path, force=True)
def cleanup(self):
if self.link_type == LinkType.directory:
@@ -718,7 +718,7 @@ def execute(self):
# the source and destination are the same file, so we're done
return
else:
- backoff_rename(self.target_full_path, self.hold_path)
+ backoff_rename(self.target_full_path, self.hold_path, force=True)
if self.url.startswith('file:/'):
source_path = url_to_path(self.url)
@@ -760,8 +760,7 @@ def execute(self):
def reverse(self):
if lexists(self.hold_path):
log.trace("moving %s => %s", self.hold_path, self.target_full_path)
- rm_rf(self.target_full_path)
- backoff_rename(self.hold_path, self.target_full_path)
+ backoff_rename(self.hold_path, self.target_full_path, force=True)
def cleanup(self):
rm_rf(self.hold_path)
diff --git a/conda/gateways/disk/update.py b/conda/gateways/disk/update.py
--- a/conda/gateways/disk/update.py
+++ b/conda/gateways/disk/update.py
@@ -2,19 +2,17 @@
from __future__ import absolute_import, division, print_function, unicode_literals
from logging import getLogger
-from os import rename, utime
+from os import rename as os_rename, utime
from os.path import lexists
import re
from conda._vendor.auxlib.path import expand
+from conda.gateways.disk.delete import rm_rf
from . import exp_backoff_fn
log = getLogger(__name__)
-# in the rest of conda's code, os.rename is preferably imported from here
-rename = rename
-
SHEBANG_REGEX = re.compile(br'^(#!((?:\\ |[^ \n\r])+)(.*))')
@@ -42,13 +40,18 @@ def update_file_in_place_as_binary(file_full_path, callback):
fh.close()
-def backoff_rename(source_path, destination_path):
+def rename(source_path, destination_path, force=False):
+ if lexists(destination_path) and force:
+ rm_rf(destination_path)
if lexists(source_path):
log.trace("renaming %s => %s", source_path, destination_path)
- exp_backoff_fn(rename, source_path, destination_path)
+ os_rename(source_path, destination_path)
else:
log.trace("cannot rename; source path does not exist '%s'", source_path)
- return
+
+
+def backoff_rename(source_path, destination_path, force=False):
+ exp_backoff_fn(rename, source_path, destination_path, force)
def touch(path):
| diff --git a/conda/gateways/disk/test.py b/conda/gateways/disk/test.py
--- a/conda/gateways/disk/test.py
+++ b/conda/gateways/disk/test.py
@@ -53,15 +53,20 @@ def hardlink_supported(source_file, dest_dir):
# file system configuration, a symbolic link may be created
# instead. If a symbolic link is created instead of a hard link,
# return False.
- log.trace("checking hard link capability for %s => %s", source_file, dest_dir)
test_file = join(dest_dir, '.tmp.' + basename(source_file))
assert isfile(source_file), source_file
assert isdir(dest_dir), dest_dir
assert not lexists(test_file), test_file
try:
create_link(source_file, test_file, LinkType.hardlink, force=True)
- return not islink(test_file)
+ is_supported = not islink(test_file)
+ if is_supported:
+ log.trace("hard link supported for %s => %s", source_file, dest_dir)
+ else:
+ log.trace("hard link IS NOT supported for %s => %s", source_file, dest_dir)
+ return is_supported
except (IOError, OSError):
+ log.trace("hard link IS NOT supported for %s => %s", source_file, dest_dir)
return False
finally:
rm_rf(test_file)
| conda 4.3.6 broken - Uncaught backoff with errno EEXIST 17
Trying to update all broke with a *file already exists* error when trying to install the packages:
```
C:\dev\code\sandbox> conda update --all
Fetching package metadata .................
Solving package specifications: .
Package plan for installation in environment C:\bin\Anaconda:
The following packages will be UPDATED:
<SNIP>
ipywidgets-5.2 100% |###############################| Time: 0:00:00 8.57 MB/s
pymc3-3.0-py35 100% |###############################| Time: 0:00:01 152.30 kB/s
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
ERROR conda.core.link:_execute_actions(318): An error occurred while uninstalling package 'conda-forge::jupyterlab-0.12.
1-py35_0'.
FileExistsError(17, 'Cannot create a file when that file already exists')
Attempting to roll back.
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
```
Trying again results in the same error (with a different package this time) leaving me unable to update my environment
```
C:\dev\code\sandbox> conda update --all
Fetching package metadata .................
Solving package specifications: .
Package plan for installation in environment C:\bin\Anaconda:
The following packages will be UPDATED:
<SNIP>
Proceed ([y]/n)? y
icu-58.1-vc14_ 100% |###############################| Time: 0:00:15 782.64 kB/s
libxml2-2.9.3- 100% |###############################| Time: 0:00:04 754.62 kB/s
qt-4.8.7-vc14_ 100% |###############################| Time: 0:00:34 1.51 MB/s
pyqt-4.11.4-py 100% |###############################| Time: 0:00:00 9.97 MB/s
matplotlib-2.0 100% |###############################| Time: 0:00:06 1.06 MB/s
anaconda-navig 100% |###############################| Time: 0:00:02 1.51 MB/s
qtconsole-4.2. 100% |###############################| Time: 0:00:00 14.07 MB/s
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
ERROR conda.core.link:_execute_actions(318): An error occurred while uninstalling package 'conda-forge::flask-cors-3.0.2-py35_0'.
FileExistsError(17, 'Cannot create a file when that file already exists')
Attempting to roll back.
WARNING conda.gateways.disk:exp_backoff_fn(47): Uncaught backoff with errno EEXIST 17
FileExistsError(17, 'Cannot create a file when that file already exists')
FileExistsError(17, 'Cannot create a file when that file already exists')
```
| Using conda 4.3.6 on py35 / win64.
Downgrading to conda 4.2.15 allowed me to complete the update.
I'd love to know where this error came from and fix it, but I need more information. Can you still reproduce it? If so, can you give me the output with the addition of `-v -v -v`?
@kalefranz - I reverted to the previous revision, reinstalled 4.3.6 and re-ran conda update with `-v -v -v` which appeared to fail with the same error.
Log available at - https://gist.github.com/dhirschfeld/2fa4c615e09c33d03f86f9d95deefcbf
NB: 4.3.7 also has the same problem for me | 2017-01-22T08:51:51 | -1.0 |
conda/conda | 4,406 | conda__conda-4406 | [
"5116"
] | 9591b0e7a49ba3ee99fc6fba5c470330f149ec14 | diff --git a/conda/cli/activate.py b/conda/cli/activate.py
--- a/conda/cli/activate.py
+++ b/conda/cli/activate.py
@@ -1,14 +1,15 @@
-from __future__ import print_function, division, absolute_import, unicode_literals
+from __future__ import absolute_import, division, print_function, unicode_literals
import errno
import os
-import re
+from os.path import abspath, isdir
+import re as regex
import sys
-from os.path import isdir, abspath
-from ..common.compat import text_type, on_win
-from ..exceptions import (CondaSystemExit, ArgumentError, CondaValueError, CondaEnvironmentError,
- TooManyArgumentsError, TooFewArgumentsError)
+from ..common.compat import on_win, text_type
+from ..exceptions import (ArgumentError, CondaEnvironmentError, CondaSystemExit, CondaValueError,
+ TooFewArgumentsError, TooManyArgumentsError)
+from ..utils import shells
def help(command, shell):
@@ -44,12 +45,13 @@ def help(command, shell):
raise CondaSystemExit("No help available for command %s" % sys.argv[1])
-def prefix_from_arg(arg, shelldict):
+def prefix_from_arg(arg, shell):
+ shelldict = shells[shell] if shell else {}
from ..base.context import context, locate_prefix_by_name
'Returns a platform-native path'
# MSYS2 converts Unix paths to Windows paths with unix seps
# so we must check for the drive identifier too.
- if shelldict['sep'] in arg and not re.match('[a-zA-Z]:', arg):
+ if shelldict['sep'] in arg and not regex.match('[a-zA-Z]:', arg):
# strip is removing " marks, not \ - look carefully
native_path = shelldict['path_from'](arg)
if isdir(abspath(native_path.strip("\""))):
@@ -61,23 +63,23 @@ def prefix_from_arg(arg, shelldict):
return prefix
-def binpath_from_arg(arg, shelldict):
- # prefix comes back as platform-native path
- prefix = prefix_from_arg(arg, shelldict=shelldict)
+def _get_prefix_paths(prefix):
if on_win:
- paths = [
- prefix.rstrip("\\"),
- os.path.join(prefix, 'Library', 'mingw-w64', 'bin'),
- os.path.join(prefix, 'Library', 'usr', 'bin'),
- os.path.join(prefix, 'Library', 'bin'),
- os.path.join(prefix, 'Scripts'),
- ]
+ yield prefix.rstrip("\\")
+ yield os.path.join(prefix, 'Library', 'mingw-w64', 'bin')
+ yield os.path.join(prefix, 'Library', 'usr', 'bin')
+ yield os.path.join(prefix, 'Library', 'bin')
+ yield os.path.join(prefix, 'Scripts')
else:
- paths = [
- os.path.join(prefix, 'bin'),
- ]
+ yield os.path.join(prefix, 'bin')
+
+
+def binpath_from_arg(arg, shell):
+ shelldict = shells[shell] if shell else {}
+ # prefix comes back as platform-native path
+ prefix = prefix_from_arg(arg, shell)
# convert paths to shell-native paths
- return [shelldict['path_to'](path) for path in paths]
+ return [shelldict['path_to'](path) for path in _get_prefix_paths(prefix)]
def pathlist_to_str(paths, escape_backslashes=True):
@@ -88,25 +90,15 @@ def pathlist_to_str(paths, escape_backslashes=True):
path = ' and '.join(paths)
if on_win and escape_backslashes:
# escape for printing to console - ends up as single \
- path = re.sub(r'(?<!\\)\\(?!\\)', r'\\\\', path)
+ path = regex.sub(r'(?<!\\)\\(?!\\)', r'\\\\', path)
else:
path = path.replace("\\\\", "\\")
return path
-def get_activate_path(shelldict):
- arg_num = len(sys.argv)
- if arg_num != 4:
- num_expected = 2
- if arg_num < 4:
- raise TooFewArgumentsError(num_expected, arg_num - num_expected,
- "..activate expected exactly two arguments:\
- shell and env name")
- if arg_num > 4:
- raise TooManyArgumentsError(num_expected, arg_num - num_expected, sys.argv[2:],
- "..activate expected exactly two arguments:\
- shell and env name")
- binpath = binpath_from_arg(sys.argv[3], shelldict=shelldict)
+def get_activate_path(prefix, shell):
+ shelldict = shells[shell] if shell else {}
+ binpath = binpath_from_arg(prefix, shell)
# prepend our new entries onto the existing path and make sure that the separator is native
path = shelldict['pathsep'].join(binpath)
@@ -115,32 +107,42 @@ def get_activate_path(shelldict):
def main():
from ..base.constants import ROOT_ENV_NAME
- from ..utils import shells
if '-h' in sys.argv or '--help' in sys.argv:
# all execution paths sys.exit at end.
help(sys.argv[1], sys.argv[2])
if len(sys.argv) > 2:
shell = sys.argv[2]
- shelldict = shells[shell]
else:
- shelldict = {}
+ shell = ''
+
+ if regex.match('^..(?:de|)activate$', sys.argv[1]):
+ arg_num = len(sys.argv)
+ if arg_num != 4:
+ num_expected = 2
+ if arg_num < 4:
+ raise TooFewArgumentsError(num_expected, arg_num - num_expected,
+ "{} expected exactly two arguments:\
+ shell and env name".format(sys.argv[1]))
+ if arg_num > 4:
+ raise TooManyArgumentsError(num_expected, arg_num - num_expected, sys.argv[2:],
+ "{} expected exactly two arguments:\
+ shell and env name".format(sys.argv[1]))
if sys.argv[1] == '..activate':
- print(get_activate_path(shelldict))
+ print(get_activate_path(sys.argv[3], shell))
sys.exit(0)
elif sys.argv[1] == '..deactivate.path':
- import re
- activation_path = get_activate_path(shelldict)
+ activation_path = get_activate_path(sys.argv[3], shell)
if os.getenv('_CONDA_HOLD'):
- new_path = re.sub(r'%s(:?)' % re.escape(activation_path),
- r'CONDA_PATH_PLACEHOLDER\1',
- os.environ[str('PATH')], 1)
+ new_path = regex.sub(r'%s(:?)' % regex.escape(activation_path),
+ r'CONDA_PATH_PLACEHOLDER\1',
+ os.environ[str('PATH')], 1)
else:
- new_path = re.sub(r'%s(:?)' % re.escape(activation_path), r'',
- os.environ[str('PATH')], 1)
+ new_path = regex.sub(r'%s(:?)' % regex.escape(activation_path), r'',
+ os.environ[str('PATH')], 1)
print(new_path)
sys.exit(0)
@@ -157,7 +159,7 @@ def main():
# this should throw an error and exit if the env or path can't be found.
try:
- prefix = prefix_from_arg(sys.argv[3], shelldict=shelldict)
+ prefix = prefix_from_arg(sys.argv[3], shell)
except ValueError as e:
raise CondaValueError(text_type(e))
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -1,26 +1,24 @@
# -*- coding: utf-8 -*-
-from __future__ import print_function, absolute_import, unicode_literals
-
-import subprocess
-import tempfile
+from __future__ import absolute_import, print_function, unicode_literals
+from datetime import datetime
import os
from os.path import dirname
import stat
+import subprocess
import sys
+import tempfile
-from datetime import datetime
import pytest
-from conda.compat import TemporaryDirectory, PY2
-from conda.config import root_dir, platform
+from conda.base.context import context
+from conda.cli.activate import _get_prefix_paths, binpath_from_arg
+from conda.compat import TemporaryDirectory
+from conda.config import platform, root_dir
from conda.install import symlink_conda
-from conda.utils import path_identity, shells, on_win, translate_stream
-from conda.cli.activate import binpath_from_arg
-
+from conda.utils import on_win, shells, translate_stream
from tests.helpers import assert_equals, assert_in, assert_not_in
-
# ENVS_PREFIX = "envs" if PY2 else "envsßôç"
ENVS_PREFIX = "envs"
@@ -38,22 +36,20 @@ def gen_test_env_paths(envs, shell, num_test_folders=5):
paths = [converter(path) for path in paths]
return paths
-def _envpaths(env_root, env_name="", shelldict={}):
+def _envpaths(env_root, env_name="", shell=None):
"""Supply the appropriate platform executable folders. rstrip on root removes
trailing slash if env_name is empty (the default)
Assumes that any prefix used here exists. Will not work on prefixes that don't.
"""
- sep = shelldict['sep']
- return binpath_from_arg(sep.join([env_root, env_name]), shelldict=shelldict)
+ sep = shells[shell]['sep']
+ return binpath_from_arg(sep.join([env_root, env_name]), shell)
PYTHONPATH = os.path.dirname(os.path.dirname(__file__))
# Make sure the subprocess activate calls this python
-syspath = os.pathsep.join(_envpaths(root_dir, shelldict={"path_to": path_identity,
- "path_from": path_identity,
- "sep": os.sep}))
+syspath = os.pathsep.join(_get_prefix_paths(context.root_prefix))
def print_ps1(env_dirs, raw_ps, number):
return (u"({}) ".format(env_dirs[number]) + raw_ps)
@@ -144,7 +140,7 @@ def test_activate_test1(shell):
""").format(envs=envs, env_dirs=gen_test_env_paths(envs, shell), **shell_vars)
stdout, stderr = run_in(commands, shell)
- assert_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 1', shelldict=shells[shell])),
+ assert_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 1', shell)),
stdout, shell)
@@ -159,7 +155,7 @@ def test_activate_env_from_env_with_root_activate(shell):
""").format(envs=envs, env_dirs=gen_test_env_paths(envs, shell), **shell_vars)
stdout, stderr = run_in(commands, shell)
- assert_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 2', shelldict=shells[shell])), stdout)
+ assert_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 2', shell)), stdout)
@pytest.mark.installed
@@ -190,7 +186,7 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
""").format(envs=envs, env_dirs=gen_test_env_paths(envs, shell), **shell_vars)
stdout, stderr = run_in(commands, shell)
- assert_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 1', shelldict=shells[shell])),stdout)
+ assert_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 1', shell)),stdout)
@pytest.mark.installed
@@ -222,7 +218,7 @@ def test_activate_root_simple(shell):
""").format(envs=envs, **shell_vars)
stdout, stderr = run_in(commands, shell)
- assert_in(shells[shell]['pathsep'].join(_envpaths(root_dir, shelldict=shells[shell])), stdout, stderr)
+ assert_in(shells[shell]['pathsep'].join(_envpaths(root_dir, shell=shell)), stdout, stderr)
commands = (shell_vars['command_setup'] + """
{source} "{syspath}{binpath}activate" root
@@ -246,9 +242,9 @@ def test_activate_root_env_from_other_env(shell):
""").format(envs=envs, env_dirs=gen_test_env_paths(envs, shell), **shell_vars)
stdout, stderr = run_in(commands, shell)
- assert_in(shells[shell]['pathsep'].join(_envpaths(root_dir, shelldict=shells[shell])),
+ assert_in(shells[shell]['pathsep'].join(_envpaths(root_dir, shell=shell)),
stdout)
- assert_not_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 1', shelldict=shells[shell])), stdout)
+ assert_not_in(shells[shell]['pathsep'].join(_envpaths(envs, 'test 1', shell)), stdout)
@pytest.mark.installed
| refactor conda/cli/activate.py to help menuinst
Let menuinst not have to work about shellsdict in conda 4.3.
| 2017-01-23T19:24:46 | -1.0 |
|
conda/conda | 4,518 | conda__conda-4518 | [
"4513"
] | f71c08a765ee5714980206b30115b74886813fec | diff --git a/conda/core/index.py b/conda/core/index.py
--- a/conda/core/index.py
+++ b/conda/core/index.py
@@ -9,7 +9,7 @@
from logging import DEBUG, getLogger
from mmap import ACCESS_READ, mmap
from os import makedirs
-from os.path import getmtime, isfile, join, split as path_split
+from os.path import getmtime, isfile, join, split as path_split, dirname
import pickle
import re
from textwrap import dedent
@@ -234,12 +234,13 @@ def maybe_decompress(filename, resp_content):
$ mkdir noarch
$ echo '{}' > noarch/repodata.json
$ bzip2 -k noarch/repodata.json
- """ % url)
+ """) % dirname(url)
stderrlog.warn(help_message)
return None
else:
help_message = dals("""
- The remote server could not find the channel you requested.
+ The remote server could not find the noarch directory for the
+ requested channel with url: %s
As of conda 4.3, a valid channel must contain a `noarch/repodata.json` and
associated `noarch/repodata.json.bz2` file, even if `noarch/repodata.json` is
@@ -252,7 +253,7 @@ def maybe_decompress(filename, resp_content):
You will need to adjust your conda configuration to proceed.
Use `conda config --show` to view your configuration's current state.
Further configuration help can be found at <%s>.
- """ % join_url(CONDA_HOMEPAGE_URL, 'docs/config.html'))
+ """) % (dirname(url), join_url(CONDA_HOMEPAGE_URL, 'docs/config.html'))
elif status_code == 403:
if not url.endswith('/noarch'):
@@ -272,12 +273,13 @@ def maybe_decompress(filename, resp_content):
$ mkdir noarch
$ echo '{}' > noarch/repodata.json
$ bzip2 -k noarch/repodata.json
- """ % url)
+ """) % dirname(url)
stderrlog.warn(help_message)
return None
else:
help_message = dals("""
- The channel you requested is not available on the remote server.
+ The remote server could not find the noarch directory for the
+ requested channel with url: %s
As of conda 4.3, a valid channel must contain a `noarch/repodata.json` and
associated `noarch/repodata.json.bz2` file, even if `noarch/repodata.json` is
@@ -290,7 +292,7 @@ def maybe_decompress(filename, resp_content):
You will need to adjust your conda configuration to proceed.
Use `conda config --show` to view your configuration's current state.
Further configuration help can be found at <%s>.
- """ % join_url(CONDA_HOMEPAGE_URL, 'docs/config.html'))
+ """) % (dirname(url), join_url(CONDA_HOMEPAGE_URL, 'docs/config.html'))
elif status_code == 401:
channel = Channel(url)
@@ -491,9 +493,12 @@ def fetch_repodata(url, schannel, priority,
touch(cache_path)
return read_local_repodata(cache_path, url, schannel, priority,
mod_etag_headers.get('_etag'), mod_etag_headers.get('_mod'))
+ if repodata is None:
+ return None
with open(cache_path, 'w') as fo:
json.dump(repodata, fo, indent=2, sort_keys=True, cls=EntityEncoder)
+
process_repodata(repodata, url, schannel, priority)
write_pickled_repodata(cache_path, repodata)
return repodata
@@ -548,7 +553,7 @@ def fetch_index(channel_urls, use_cache=False, index=None):
# this is sorta a lie; actually more primitve types
if index is None:
- index = dict()
+ index = {}
for _, repodata in repodatas:
if repodata:
index.update(repodata.get('packages', {}))
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -191,6 +191,12 @@ def test_install_python2(self):
assert exists(join(prefix, PYTHON_BINARY))
assert_package_is_installed(prefix, 'python-2')
+ # regression test for #4513
+ run_command(Commands.CONFIG, prefix, "--add channels https://repo.continuum.io/pkgs/not-a-channel")
+ stdout, stderr = run_command(Commands.SEARCH, prefix, "python --json")
+ packages = json.loads(stdout)
+ assert len(packages) > 1
+
@pytest.mark.timeout(900)
def test_create_install_update_remove(self):
with make_temp_env("python=3.5") as prefix:
| Conda breaks if it can't find the noarch channel
If the `noarch` URL of some custom channel doesn't exist, conda becomes completely broken. The error is:
```
Fetching package metadata ......
WARNING: The remote server could not find the noarch directory for the
requested channel with url: https://zigzah.com/static/conda-pkgs/noarch
It is possible you have given conda an invalid channel. Please double-check
your conda configuration using `conda config --show`.
If the requested url is in fact a valid conda channel, please request that the
channel administrator create `noarch/repodata.json` and associated
`noarch/repodata.json.bz2` files, even if `noarch/repodata.json` is empty.
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : linux-64
conda version : 4.3.9
conda is private : False
conda-env version : 4.3.9
conda-build version : 2.0.12
python version : 3.5.3.final.0
requests version : 2.13.0
root environment : /home/zah/anaconda3 (writable)
default environment : /home/zah/anaconda3
envs directories : /home/zah/anaconda3/envs
package cache : /home/zah/anaconda3/pkgs
channel URLs :
https://zigzah.com/static/conda-pkgs/linux-64
https://zigzah.com/static/conda-pkgs/noarch
https://conda.anaconda.org/conda-forge/linux-64
https://conda.anaconda.org/conda-forge/noarch
https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
https://conda.anaconda.org/r/linux-64
https://conda.anaconda.org/r/noarch
config file : /home/zah/.condarc
offline mode : False
user-agent : conda/4.3.9 requests/2.13.0 CPython/3.5.3 Linux/4.8.0-32-generic debian/stretch/sid glibc/2.23
UID:GID : 1000:1000
`$ /home/zah/anaconda3/bin/conda install validphys`
Traceback (most recent call last):
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/exceptions.py", line 616, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/cli/main.py", line 137, in _main
exit_code = args.func(args, p)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/cli/main_install.py", line 80, in execute
install(args, parser, 'install')
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/cli/install.py", line 222, in install
unknown=index_args['unknown'], prefix=prefix)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 124, in get_index
index = fetch_index(channel_priority_map, use_cache=use_cache)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 532, in fetch_index
repodatas = _collect_repodatas(use_cache, tasks)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 521, in _collect_repodatas
repodatas = _collect_repodatas_serial(use_cache, tasks)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 494, in _collect_repodatas_serial
for url, schan, pri in tasks]
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 494, in <listcomp>
for url, schan, pri in tasks]
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 143, in func
res = f(*args, **kwargs)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 483, in fetch_repodata
process_repodata(repodata, url, schannel, priority)
File "/home/zah/anaconda3/lib/python3.5/site-packages/conda/core/index.py", line 410, in process_repodata
opackages = repodata.setdefault('packages', {})
AttributeError: 'NoneType' object has no attribute 'setdefault'
```
| 2017-02-03T18:37:45 | -1.0 |
|
conda/conda | 4,548 | conda__conda-4548 | [
"4546"
] | 81d2f6e2d383bb056d19ead27819675a6d48a06e | diff --git a/conda/core/path_actions.py b/conda/core/path_actions.py
--- a/conda/core/path_actions.py
+++ b/conda/core/path_actions.py
@@ -385,14 +385,14 @@ def this_triplet(entry_point_def):
if noarch is not None and noarch.type == NoarchType.python:
actions = tuple(cls(transaction_context, package_info, target_prefix,
*this_triplet(ep_def))
- for ep_def in noarch.entry_points)
+ for ep_def in noarch.entry_points or ())
if on_win:
actions += tuple(
LinkPathAction.create_python_entry_point_windows_exe_action(
transaction_context, package_info, target_prefix,
requested_link_type, ep_def
- ) for ep_def in noarch.entry_points
+ ) for ep_def in noarch.entry_points or ()
)
return actions
diff --git a/conda/models/package_info.py b/conda/models/package_info.py
--- a/conda/models/package_info.py
+++ b/conda/models/package_info.py
@@ -20,12 +20,12 @@ def box(self, instance, val):
class Noarch(Entity):
type = NoarchField(NoarchType)
- entry_points = ListField(string_types, required=False)
+ entry_points = ListField(string_types, required=False, nullable=True)
class PreferredEnv(Entity):
name = StringField()
- executable_paths = ListField(string_types, required=False)
+ executable_paths = ListField(string_types, required=False, nullable=True)
class PackageMetadata(Entity):
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -231,7 +231,7 @@ def test_create_install_update_remove(self):
self.assertRaises(CondaError, run_command, Commands.INSTALL, prefix, 'constructor=1.0')
assert not package_is_installed(prefix, 'constructor')
- def test_noarch_python_package(self):
+ def test_noarch_python_package_with_entry_points(self):
with make_temp_env("-c conda-test flask") as prefix:
py_ver = get_python_version_for_prefix(prefix)
sp_dir = get_python_site_packages_short_path(py_ver)
@@ -250,6 +250,21 @@ def test_noarch_python_package(self):
assert not isfile(join(prefix, pyc_file))
assert not isfile(exe_path)
+ def test_noarch_python_package_without_entry_points(self):
+ # regression test for #4546
+ with make_temp_env("-c conda-test itsdangerous") as prefix:
+ py_ver = get_python_version_for_prefix(prefix)
+ sp_dir = get_python_site_packages_short_path(py_ver)
+ py_file = sp_dir + "/itsdangerous.py"
+ pyc_file = pyc_path(py_file, py_ver)
+ assert isfile(join(prefix, py_file))
+ assert isfile(join(prefix, pyc_file))
+
+ run_command(Commands.REMOVE, prefix, "itsdangerous")
+
+ assert not isfile(join(prefix, py_file))
+ assert not isfile(join(prefix, pyc_file))
+
def test_noarch_generic_package(self):
with make_temp_env("-c conda-test font-ttf-inconsolata") as prefix:
assert isfile(join(prefix, 'fonts', 'Inconsolata-Regular.ttf'))
| Unable to install noarch: python package without entry points
I've been playing with the `noarch: python` feature in conda 4.3 and enjoying it, really great addition!
One issue that I've found is that conda seems unable to install noarch python packages that do not have entry points. Building and installing `noarch: python` packages which have entry points works fine.
For example, trying to build a package for the following recipe:
``` yaml
package:
name: imagesize
version: 0.7.1
source:
fn: imagesize-0.7.1.tar.gz
url: https://pypi.io/packages/source/i/imagesize/imagesize-0.7.1.tar.gz
sha256: 0ab2c62b87987e3252f89d30b7cedbec12a01af9274af9ffa48108f2c13c6062
build:
number: 70
noarch: python
script: python setup.py install --single-version-externally-managed --record record.txt
requirements:
build:
- python
- setuptools
run:
- python
test:
imports:
- imagesize
```
The build fails when trying to install the package into the test environment:
```
$ conda build .
BUILD START: imagesize-0.7.1-py_70
...
TEST START: imagesize-0.7.1-py_70
Deleting work directory, /home/jhelmus/anaconda/conda-bld/imagesize_1486396885045/work/imagesize-0.7.1
updating index in: /home/jhelmus/anaconda/conda-bld/linux-64
updating index in: /home/jhelmus/anaconda/conda-bld/noarch
The following NEW packages will be INSTALLED:
imagesize: 0.7.1-py_70 local
openssl: 1.0.2k-0 defaults
python: 3.5.2-0 defaults
readline: 6.2-2 defaults
sqlite: 3.13.0-0 defaults
tk: 8.5.18-0 defaults
xz: 5.2.2-1 defaults
zlib: 1.2.8-3 defaults
Traceback (most recent call last):
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/_vendor/auxlib/entity.py", line 403, in __get__
val = instance.__dict__[self.name]
KeyError: 'entry_points'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/jhelmus/anaconda/bin/conda-build", line 6, in <module>
sys.exit(conda_build.cli.main_build.main())
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda_build/cli/main_build.py", line 322, in main
execute(sys.argv[1:])
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda_build/cli/main_build.py", line 313, in execute
noverify=args.no_verify)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda_build/api.py", line 97, in build
need_source_download=need_source_download, config=config)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda_build/build.py", line 1486, in build_tree
test(pkg, config=config)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda_build/build.py", line 1304, in test
create_env(config.test_prefix, specs, config=config)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda_build/build.py", line 684, in create_env
plan.execute_actions(actions, index, verbose=config.debug)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/plan.py", line 837, in execute_actions
execute_instructions(plan, index, verbose)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/instructions.py", line 258, in execute_instructions
cmd(state, arg)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/instructions.py", line 119, in UNLINKLINKTRANSACTION_CMD
txn.execute()
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/link.py", line 256, in execute
self.verify()
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/link.py", line 239, in verify
self.prepare()
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/link.py", line 170, in prepare
concatv(unlink_actions, link_actions))
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/link.py", line 169, in <genexpr>
self.all_actions = tuple(per_pkg_actions for per_pkg_actions in
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/link.py", line 166, in <genexpr>
for pkg_info, lt in zip(self.packages_info_to_link, link_types)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/link.py", line 395, in make_link_actions
python_entry_point_actions = CreatePythonEntryPointAction.create_actions(*required_quad)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/core/path_actions.py", line 388, in create_actions
for ep_def in noarch.entry_points)
File "/home/jhelmus/anaconda/lib/python3.5/site-packages/conda/_vendor/auxlib/entity.py", line 413, in __get__
raise AttributeError("A value for {0} has not been set".format(self.name))
AttributeError: A value for entry_points has not been set
```
The package can be build using the `--no-test` flag but the same error is given when attempting to install the package into a environment. I've uploaded this package to the [jjhelmus_testing channel](https://anaconda.org/jjhelmus_testing/imagesize/files) in case it is helpful for testing. The behavior can be replicated using the command: `conda create -n imagesize_27 -c jjhelmus_testing python=2.7 imagesize=0.7.1=py_70`.
---
conda info for the build/install environment if it helps:
```
$ conda info
Current conda install:
platform : linux-64
conda version : 4.3.9
conda is private : False
conda-env version : 4.3.9
conda-build version : 2.1.3
python version : 3.5.2.final.0
requests version : 2.12.4
root environment : /home/jhelmus/anaconda (writable)
default environment : /home/jhelmus/anaconda
envs directories : /home/jhelmus/anaconda/envs
package cache : /home/jhelmus/anaconda/pkgs
channel URLs : https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
config file : /home/jhelmus/.condarc
offline mode : False
user-agent : conda/4.3.9 requests/2.12.4 CPython/3.5.2 Linux/4.4.0-59-generic debian/stretch/sid glibc/2.23
UID:GID : 5001:1004
```
| Thanks for the bug report. I'll get a fix in the next patch release. | 2017-02-06T19:30:19 | -1.0 |
conda/conda | 4,774 | conda__conda-4774 | [
"4757"
] | 14dd0efb8e2116ed07d6cca01b8f63f3ac04cb66 | diff --git a/conda/common/configuration.py b/conda/common/configuration.py
--- a/conda/common/configuration.py
+++ b/conda/common/configuration.py
@@ -17,7 +17,7 @@
from __future__ import absolute_import, division, print_function, unicode_literals
from abc import ABCMeta, abstractmethod
-from collections import Mapping, defaultdict
+from collections import Mapping, Sequence, defaultdict
from glob import glob
from itertools import chain
from logging import getLogger
@@ -35,7 +35,7 @@
from .._vendor.auxlib.collection import AttrDict, first, frozendict, last, make_immutable
from .._vendor.auxlib.exceptions import ThisShouldNeverHappenError
from .._vendor.auxlib.path import expand
-from .._vendor.auxlib.type_coercion import TypeCoercionError, typify_data_structure
+from .._vendor.auxlib.type_coercion import TypeCoercionError, typify, typify_data_structure
try:
from cytoolz.dicttoolz import merge
@@ -561,7 +561,6 @@ def __init__(self, element_type, default=(), aliases=(), validation=None,
def collect_errors(self, instance, value, source="<<merged>>"):
errors = super(SequenceParameter, self).collect_errors(instance, value)
-
element_type = self._element_type
for idx, element in enumerate(value):
if not isinstance(element, element_type):
@@ -616,9 +615,10 @@ def repr_raw(self, raw_parameter):
def _get_all_matches(self, instance):
# this is necessary to handle argparse `action="append"`, which can't be set to a
# default value of NULL
- matches, multikey_exceptions = super(SequenceParameter, self)._get_all_matches(instance)
+ # it also config settings like `channels: ~`
+ matches, exceptions = super(SequenceParameter, self)._get_all_matches(instance)
matches = tuple(m for m in matches if m._raw_value is not None)
- return matches, multikey_exceptions
+ return matches, exceptions
class MapParameter(Parameter):
@@ -647,6 +647,7 @@ def collect_errors(self, instance, value, source="<<merged>>"):
errors.extend(InvalidElementTypeError(self.name, val, source, type(val),
element_type, key)
for key, val in iteritems(value) if not isinstance(val, element_type))
+
return errors
def _merge(self, matches):
@@ -676,6 +677,12 @@ def repr_raw(self, raw_parameter):
self._str_format_flag(valueflag)))
return '\n'.join(lines)
+ def _get_all_matches(self, instance):
+ # it also config settings like `proxy_servers: ~`
+ matches, exceptions = super(MapParameter, self)._get_all_matches(instance)
+ matches = tuple(m for m in matches if m._raw_value is not None)
+ return matches, exceptions
+
class ConfigurationType(type):
"""metaclass for Configuration"""
| diff --git a/tests/common/test_configuration.py b/tests/common/test_configuration.py
--- a/tests/common/test_configuration.py
+++ b/tests/common/test_configuration.py
@@ -7,7 +7,8 @@
from conda.common.compat import odict, string_types
from conda.common.configuration import (Configuration, MapParameter, ParameterFlag,
PrimitiveParameter, SequenceParameter, YamlRawParameter,
- load_file_configs, MultiValidationError, InvalidTypeError)
+ load_file_configs, MultiValidationError, InvalidTypeError,
+ CustomValidationError)
from conda.common.yaml import yaml_load
from conda.common.configuration import ValidationError
from os import environ, mkdir
@@ -125,6 +126,8 @@
an_int: 2
a_float: 1.2
a_complex: 1+2j
+ proxy_servers:
+ channels:
"""),
}
@@ -389,7 +392,15 @@ def test_validate_all(self):
config.validate_configuration()
config = SampleConfiguration()._set_raw_data(load_from_string_data('bad_boolean_map'))
- raises(ValidationError, config.validate_configuration)
+ try:
+ config.validate_configuration()
+ except ValidationError as e:
+ # the `proxy_servers: ~` part of 'bad_boolean_map' is a regression test for #4757
+ # in the future, the below should probably be a MultiValidationError
+ # with TypeValidationError for 'proxy_servers' and 'channels'
+ assert isinstance(e, CustomValidationError)
+ else:
+ assert False
def test_cross_parameter_validation(self):
pass
@@ -411,3 +422,9 @@ def test_config_resets(self):
with env_var("MYAPP_CHANGEPS1", "false"):
config.__init__(app_name=appname)
assert config.changeps1 is False
+
+ def test_empty_map_parameter(self):
+
+ config = SampleConfiguration()._set_raw_data(load_from_string_data('bad_boolean_map'))
+ config.check_source('bad_boolean_map')
+
| Error when installing r-essential on windows 7
When I try to install the r-essentials, I get the following error:
$ C:\ProgramData\Anaconda3\Scripts\conda-script.py install -c r r-essentials=1.5.2
Traceback (most recent call last):
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\exceptions.py", line 617, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\cli\main.py", line 137, in _main
exit_code = args.func(args, p)
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\cli\main_install.py", line 80, in execute
install(args, parser, 'install')
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\cli\install.py", line 118, in install
context.validate_configuration()
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\configuration.py", line 830, in validate_configuration
raise_errors(tuple(chain.from_iterable((errors, post_errors))))
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\configuration.py", line 828, in
for name in self.parameter_names)
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\configuration.py", line 821, in _collect_validation_error
func(*args, **kwargs)
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\configuration.py", line 446, in get
result = typify_data_structure(self._merge(matches) if matches else self.default,
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\configuration.py", line 662, in _merge
for match in relevant_matches)
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\configuration.py", line 662, in
for match in relevant_matches)
File "C:\ProgramData\Anaconda3\lib\site-packages\conda\common\compat.py", line 72, in iteritems
return iter(d.items(**kw))
AttributeError: 'NoneType' object has no attribute 'items'
Current conda install:
platform : win-64
conda version : 4.3.8
conda is private : False
conda-env version : 4.3.8
conda-build version : not installed
python version : 3.6.0.final.0
requests version : 2.12.4
root environment : C:\ProgramData\Anaconda3 (writable)
default environment : C:\ProgramData\Anaconda3
envs directories : C:\ProgramData\Anaconda3\envs
package cache : C:\ProgramData\Anaconda3\pkgs
channel URLs : https://conda.anaconda.org/r/win-64
https://conda.anaconda.org/r/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\xxx.condarc
offline mode : False
user-agent : conda/4.3.8 requests/2.12.4 CPython/3.6.0 Windows/7 Windows/6.1.7601
If somebody could help me with this, that would be great.
Thanks
| Latest conda version is 4.3.13. `conda update conda`
Thanks.
I had an error in the .condarc file.
It seems, that the above error occurs when another error message is going to be created.
Oh yeah. Syntax errors in yaml configuration files don't go through conda's normal error handler because it hasn't been initialized yet.
Can you give me what you actually had as contents for the bad condarc file? I'll write a regression test for it... | 2017-03-01T15:28:01 | -1.0 |
conda/conda | 4,963 | conda__conda-4963 | [
"4944",
"4944"
] | eb2687e7164437421058d592705f37d40bc86b45 | diff --git a/conda/cli/conda_argparse.py b/conda/cli/conda_argparse.py
--- a/conda/cli/conda_argparse.py
+++ b/conda/cli/conda_argparse.py
@@ -10,14 +10,11 @@
import os
import subprocess
import sys
-from difflib import get_close_matches
from .common import add_parser_help
from .find_commands import find_commands, find_executable
from ..exceptions import CommandNotFoundError
-build_commands = {'build', 'index', 'skeleton', 'package', 'metapackage',
- 'pipbuild', 'develop', 'convert'}
_ARGCOMPLETE_DEBUG = False
def debug_argcomplete(msg):
@@ -129,21 +126,7 @@ def error(self, message):
cmd = m.group(1)
executable = find_executable('conda-' + cmd)
if not executable:
- if cmd in build_commands:
- raise CommandNotFoundError(cmd, '''
-Error: You need to install conda-build in order to
-use the "conda %s" command.''' % cmd)
- else:
- message = "Error: Could not locate 'conda-%s'" % cmd
- possibilities = (set(argument.choices.keys()) |
- build_commands |
- set(find_commands()))
- close = get_close_matches(cmd, possibilities)
- if close:
- message += '\n\nDid you mean one of these?\n'
- for s in close:
- message += ' %s' % s
- raise CommandNotFoundError(cmd, message)
+ raise CommandNotFoundError(cmd)
args = [find_executable('conda-' + cmd)]
args.extend(sys.argv[2:])
diff --git a/conda/cli/main.py b/conda/cli/main.py
--- a/conda/cli/main.py
+++ b/conda/cli/main.py
@@ -152,14 +152,8 @@ def main(*args):
activate.main()
return
if argv1 in ('activate', 'deactivate'):
-
- message = "'%s' is not a conda command.\n" % argv1
- from ..common.compat import on_win
- if not on_win:
- message += ' Did you mean "source %s" ?\n' % ' '.join(args[1:])
-
from ..exceptions import CommandNotFoundError
- raise CommandNotFoundError(argv1, message)
+ raise CommandNotFoundError(argv1)
except Exception as e:
from ..exceptions import handle_exception
return handle_exception(e)
diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -11,7 +11,7 @@
from ._vendor.auxlib.entity import EntityEncoder
from ._vendor.auxlib.ish import dals
from .base.constants import PathConflict
-from .common.compat import iteritems, iterkeys, string_types
+from .common.compat import iteritems, iterkeys, on_win, string_types
from .common.signals import get_signal_name
from .common.url import maybe_unquote
@@ -159,7 +159,32 @@ def __init__(self, target_path, incompatible_package_dists, context):
class CommandNotFoundError(CondaError):
def __init__(self, command):
- message = "Conda could not find the command: '%(command)s'"
+ build_commands = {
+ 'build',
+ 'convert',
+ 'develop',
+ 'index',
+ 'inspect',
+ 'metapackage',
+ 'render',
+ 'skeleton',
+ }
+ needs_source = {
+ 'activate',
+ 'deactivate'
+ }
+ if command in build_commands:
+ message = dals("""
+ You need to install conda-build in order to
+ use the 'conda %(command)s' command.
+ """)
+ elif command in needs_source and not on_win:
+ message = dals("""
+ '%(command)s is not a conda command.
+ Did you mean 'source %(command)s'?
+ """)
+ else:
+ message = "Conda could not find the command: '%(command)s'"
super(CommandNotFoundError, self).__init__(message, command=command)
| diff --git a/tests/test_exceptions.py b/tests/test_exceptions.py
--- a/tests/test_exceptions.py
+++ b/tests/test_exceptions.py
@@ -1,6 +1,8 @@
import json
from unittest import TestCase
+from conda.common.compat import on_win
+
from conda import text_type
from conda._vendor.auxlib.ish import dals
from conda.base.context import reset_context, context
@@ -310,7 +312,7 @@ def test_CondaHTTPError(self):
Groot
""").strip()
- def test_CommandNotFoundError(self):
+ def test_CommandNotFoundError_simple(self):
cmd = "instate"
exc = CommandNotFoundError(cmd)
@@ -330,3 +332,52 @@ def test_CommandNotFoundError(self):
assert not c.stdout
assert c.stderr.strip() == "CommandNotFoundError: Conda could not find the command: 'instate'"
+
+ def test_CommandNotFoundError_conda_build(self):
+ cmd = "build"
+ exc = CommandNotFoundError(cmd)
+
+ with env_var("CONDA_JSON", "yes", reset_context):
+ with captured() as c, replace_log_streams():
+ conda_exception_handler(_raise_helper, exc)
+
+ json_obj = json.loads(c.stdout)
+ assert not c.stderr
+ assert json_obj['exception_type'] == "<class 'conda.exceptions.CommandNotFoundError'>"
+ assert json_obj['message'] == text_type(exc)
+ assert json_obj['error'] == repr(exc)
+
+ with env_var("CONDA_JSON", "no", reset_context):
+ with captured() as c, replace_log_streams():
+ conda_exception_handler(_raise_helper, exc)
+
+ assert not c.stdout
+ assert c.stderr.strip() == ("CommandNotFoundError: You need to install conda-build in order to\n" \
+ "use the 'conda build' command.")
+
+ def test_CommandNotFoundError_activate(self):
+ cmd = "activate"
+ exc = CommandNotFoundError(cmd)
+
+ with env_var("CONDA_JSON", "yes", reset_context):
+ with captured() as c, replace_log_streams():
+ conda_exception_handler(_raise_helper, exc)
+
+ json_obj = json.loads(c.stdout)
+ assert not c.stderr
+ assert json_obj['exception_type'] == "<class 'conda.exceptions.CommandNotFoundError'>"
+ assert json_obj['message'] == text_type(exc)
+ assert json_obj['error'] == repr(exc)
+
+ with env_var("CONDA_JSON", "no", reset_context):
+ with captured() as c, replace_log_streams():
+ conda_exception_handler(_raise_helper, exc)
+
+ assert not c.stdout
+
+ if on_win:
+ message = "CommandNotFoundError: Conda could not find the command: 'activate'"
+ else:
+ message = ("CommandNotFoundError: 'activate is not a conda command.\n"
+ "Did you mean 'source activate'?")
+ assert c.stderr.strip() == message
| Error in CommandNotFoundError
Commit 809532b7cc5ec1ea18f1aa565025d7c5599185b3 has broken the class CommandNotFoundError. Examples to trigger both code paths calling CommandNotFoundError():
```
(root) [root@d4cb01a1b5b6 ~]# conda activate
Traceback (most recent call last):
File "/conda/bin/conda", line 6, in <module>
sys.exit(conda.cli.main())
File "/conda/lib/python2.7/site-packages/conda/cli/main.py", line 161, in main
raise CommandNotFoundError(argv1, message)
TypeError: __init__() takes exactly 2 arguments (3 given)
```
```
(root) [root@d4cb01a1b5b6 ~]# conda -unknown unknown
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : linux-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : not installed
python version : 2.7.13.final.0
requests version : 2.12.4
root environment : /conda (writable)
default environment : /conda
envs directories : /conda/envs
/root/.conda/envs
package cache : /conda/pkgs
/root/.conda/pkgs
channel URLs : https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
config file : /root/.condarc
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/2.7.13 Linux/4.8.6-300.fc25.x86_64 CentOS/6.8 glibc/2.12
UID:GID : 0:0
`$ /conda/bin/conda -unknown unknown`
Traceback (most recent call last):
File "/conda/lib/python2.7/site-packages/conda/exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/conda/lib/python2.7/site-packages/conda/cli/main.py", line 119, in _main
args = p.parse_args(args)
File "/conda/lib/python2.7/site-packages/conda/cli/conda_argparse.py", line 173, in parse_args
return super(ArgumentParser, self).parse_args(*args, **kwargs)
File "/conda/lib/python2.7/argparse.py", line 1701, in parse_args
args, argv = self.parse_known_args(args, namespace)
File "/conda/lib/python2.7/argparse.py", line 1740, in parse_known_args
self.error(str(err))
File "/conda/lib/python2.7/site-packages/conda/cli/conda_argparse.py", line 146, in error
raise CommandNotFoundError(cmd, message)
TypeError: __init__() takes exactly 2 arguments (3 given)
```
Error in CommandNotFoundError
Commit 809532b7cc5ec1ea18f1aa565025d7c5599185b3 has broken the class CommandNotFoundError. Examples to trigger both code paths calling CommandNotFoundError():
```
(root) [root@d4cb01a1b5b6 ~]# conda activate
Traceback (most recent call last):
File "/conda/bin/conda", line 6, in <module>
sys.exit(conda.cli.main())
File "/conda/lib/python2.7/site-packages/conda/cli/main.py", line 161, in main
raise CommandNotFoundError(argv1, message)
TypeError: __init__() takes exactly 2 arguments (3 given)
```
```
(root) [root@d4cb01a1b5b6 ~]# conda -unknown unknown
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : linux-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : not installed
python version : 2.7.13.final.0
requests version : 2.12.4
root environment : /conda (writable)
default environment : /conda
envs directories : /conda/envs
/root/.conda/envs
package cache : /conda/pkgs
/root/.conda/pkgs
channel URLs : https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
config file : /root/.condarc
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/2.7.13 Linux/4.8.6-300.fc25.x86_64 CentOS/6.8 glibc/2.12
UID:GID : 0:0
`$ /conda/bin/conda -unknown unknown`
Traceback (most recent call last):
File "/conda/lib/python2.7/site-packages/conda/exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/conda/lib/python2.7/site-packages/conda/cli/main.py", line 119, in _main
args = p.parse_args(args)
File "/conda/lib/python2.7/site-packages/conda/cli/conda_argparse.py", line 173, in parse_args
return super(ArgumentParser, self).parse_args(*args, **kwargs)
File "/conda/lib/python2.7/argparse.py", line 1701, in parse_args
args, argv = self.parse_known_args(args, namespace)
File "/conda/lib/python2.7/argparse.py", line 1740, in parse_known_args
self.error(str(err))
File "/conda/lib/python2.7/site-packages/conda/cli/conda_argparse.py", line 146, in error
raise CommandNotFoundError(cmd, message)
TypeError: __init__() takes exactly 2 arguments (3 given)
```
| @nehaljwani thanks I will work on this one
I have the exact same problem.
----------------------------------------------------------------------------------------------------
In [24]: conda -unknown unknown
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : 2.0.2
python version : 2.7.13.final.0
requests version : 2.12.4
root environment : C:\Program Files\Anaconda2 (writable)
default environment : C:\Program Files\Anaconda2
envs directories : C:\Program Files\Anaconda2\envs
C:\Users\dparaskevopoulos\AppData\Local\conda\conda\envs
C:\Users\dparaskevopoulos\.conda\envs
package cache : C:\Program Files\Anaconda2\pkgs
C:\Users\dparaskevopoulos\AppData\Local\conda\conda\pkgs
channel URLs : https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : None
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/2.7.13 Windows/7 Windows/6.1.7601
`$ C:\Program Files\Anaconda2\Scripts\conda-script.py -unknown unknown`
Traceback (most recent call last):
File "C:\Program Files\Anaconda2\lib\site-packages\conda\exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Program Files\Anaconda2\lib\site-packages\conda\cli\main.py", line 119, in _main
args = p.parse_args(args)
File "C:\Program Files\Anaconda2\lib\site-packages\conda\cli\conda_argparse.py", line 173, in parse_args
return super(ArgumentParser, self).parse_args(*args, **kwargs)
File "C:\Program Files\Anaconda2\lib\argparse.py", line 1701, in parse_args
args, argv = self.parse_known_args(args, namespace)
File "C:\Program Files\Anaconda2\lib\argparse.py", line 1740, in parse_known_args
self.error(str(err))
File "C:\Program Files\Anaconda2\lib\site-packages\conda\cli\conda_argparse.py", line 146, in error
raise CommandNotFoundError(cmd, message)
TypeError: __init__() takes exactly 2 arguments (3 given)
@nehaljwani thanks I will work on this one
I have the exact same problem.
----------------------------------------------------------------------------------------------------
In [24]: conda -unknown unknown
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : 2.0.2
python version : 2.7.13.final.0
requests version : 2.12.4
root environment : C:\Program Files\Anaconda2 (writable)
default environment : C:\Program Files\Anaconda2
envs directories : C:\Program Files\Anaconda2\envs
C:\Users\dparaskevopoulos\AppData\Local\conda\conda\envs
C:\Users\dparaskevopoulos\.conda\envs
package cache : C:\Program Files\Anaconda2\pkgs
C:\Users\dparaskevopoulos\AppData\Local\conda\conda\pkgs
channel URLs : https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : None
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/2.7.13 Windows/7 Windows/6.1.7601
`$ C:\Program Files\Anaconda2\Scripts\conda-script.py -unknown unknown`
Traceback (most recent call last):
File "C:\Program Files\Anaconda2\lib\site-packages\conda\exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Program Files\Anaconda2\lib\site-packages\conda\cli\main.py", line 119, in _main
args = p.parse_args(args)
File "C:\Program Files\Anaconda2\lib\site-packages\conda\cli\conda_argparse.py", line 173, in parse_args
return super(ArgumentParser, self).parse_args(*args, **kwargs)
File "C:\Program Files\Anaconda2\lib\argparse.py", line 1701, in parse_args
args, argv = self.parse_known_args(args, namespace)
File "C:\Program Files\Anaconda2\lib\argparse.py", line 1740, in parse_known_args
self.error(str(err))
File "C:\Program Files\Anaconda2\lib\site-packages\conda\cli\conda_argparse.py", line 146, in error
raise CommandNotFoundError(cmd, message)
TypeError: __init__() takes exactly 2 arguments (3 given)
| 2017-03-29T14:00:49 | -1.0 |
conda/conda | 5,030 | conda__conda-5030 | [
"5034"
] | 7f96c658ab06a49d55ee7042d5d30e06fdcd3bc6 | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -93,6 +93,7 @@ class Context(Configuration):
string_delimiter=os.pathsep)
_pkgs_dirs = SequenceParameter(string_types, aliases=('pkgs_dirs',))
_subdir = PrimitiveParameter('', aliases=('subdir',))
+ _subdirs = SequenceParameter(string_types, aliases=('subdirs',))
local_repodata_ttl = PrimitiveParameter(1, element_type=(bool, int))
# number of seconds to cache repodata locally
@@ -241,6 +242,10 @@ def subdir(self):
else:
return '%s-%d' % (self.platform, self.bits)
+ @property
+ def subdirs(self):
+ return self._subdirs if self._subdirs else (self.subdir, 'noarch')
+
@property
def bits(self):
if self.force_32bit:
@@ -456,6 +461,7 @@ def list_parameters(self):
'root_dir',
'skip_safety_checks',
'subdir',
+ 'subdirs',
# https://conda.io/docs/config.html#disable-updating-of-dependencies-update-dependencies # NOQA
# I don't think this documentation is correct any longer. # NOQA
'update_dependencies',
diff --git a/conda/core/index.py b/conda/core/index.py
--- a/conda/core/index.py
+++ b/conda/core/index.py
@@ -88,7 +88,8 @@ def get_index(channel_urls=(), prepend=True, platform=None,
if context.offline and unknown is None:
unknown = True
- channel_priority_map = prioritize_channels(channel_urls, platform=platform)
+ subdirs = (platform, 'noarch') if platform is not None else context.subdirs
+ channel_priority_map = prioritize_channels(channel_urls, subdirs=subdirs)
index = fetch_index(channel_priority_map, use_cache=use_cache)
if prefix or unknown:
@@ -114,7 +115,7 @@ def fetch_index(channel_urls, use_cache=False, index=None):
if index is None:
index = {}
- for _, repodata in repodatas:
+ for _, repodata in reversed(repodatas):
if repodata:
index.update(repodata.get('packages', {}))
diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -7,7 +7,7 @@
from ..base.constants import (DEFAULTS_CHANNEL_NAME, DEFAULT_CHANNELS_UNIX, DEFAULT_CHANNELS_WIN,
MAX_CHANNEL_PRIORITY, UNKNOWN_CHANNEL)
from ..base.context import context
-from ..common.compat import ensure_text_type, iteritems, odict, with_metaclass
+from ..common.compat import ensure_text_type, isiterable, iteritems, odict, with_metaclass
from ..common.path import is_path, win_path_backout
from ..common.url import (Url, has_scheme, is_url, join_url, path_to_url,
split_conda_url_easy_parts, split_scheme_auth_token, urlparse)
@@ -268,9 +268,14 @@ def canonical_name(self):
else:
return join_url(self.location, self.name).lstrip('/')
- def urls(self, with_credentials=False, platform=None):
+ def urls(self, with_credentials=False, subdirs=None):
+ if subdirs is None:
+ subdirs = context.subdirs
+
+ assert isiterable(subdirs), subdirs # subdirs must be a non-string iterable
+
if self.canonical_name == UNKNOWN_CHANNEL:
- return Channel(DEFAULTS_CHANNEL_NAME).urls(with_credentials, platform)
+ return Channel(DEFAULTS_CHANNEL_NAME).urls(with_credentials, subdirs)
base = [self.location]
if with_credentials and self.token:
@@ -279,8 +284,14 @@ def urls(self, with_credentials=False, platform=None):
base = join_url(*base)
def _platforms():
- p = platform or self.platform or context.subdir
- return (p, 'noarch') if p != 'noarch' else ('noarch',)
+ if self.platform:
+ yield self.platform
+ if self.platform != 'noarch':
+ yield 'noarch'
+ else:
+ for subdir in subdirs:
+ yield subdir
+
bases = (join_url(base, p) for p in _platforms())
if with_credentials and self.auth:
@@ -301,7 +312,8 @@ def url(self, with_credentials=False):
if self.package_filename:
base.append(self.package_filename)
else:
- base.append(context.subdir)
+ first_non_noarch = next((s for s in context.subdirs if s != 'noarch'), 'noarch')
+ base.append(first_non_noarch)
base = join_url(*base)
@@ -373,15 +385,16 @@ def channel_location(self):
def canonical_name(self):
return self.name
- def urls(self, with_credentials=False, platform=None):
- if platform and platform != context.subdir and self.name == 'defaults':
- # necessary shenanigan because different platforms have different default channels
- urls = DEFAULT_CHANNELS_WIN if 'win' in platform else DEFAULT_CHANNELS_UNIX
- ca = context.channel_alias
- _channels = tuple(Channel.make_simple_channel(ca, v) for v in urls)
- else:
- _channels = self._channels
- return list(chain.from_iterable(c.urls(with_credentials, platform) for c in _channels))
+ def urls(self, with_credentials=False, subdirs=None):
+ _channels = self._channels
+ if self.name == 'defaults':
+ platform = next((s for s in reversed(subdirs or context.subdirs) if s != 'noarch'), '')
+ if platform != context.subdir:
+ # necessary shenanigan because different platforms have different default channels
+ urls = DEFAULT_CHANNELS_WIN if 'win' in platform else DEFAULT_CHANNELS_UNIX
+ ca = context.channel_alias
+ _channels = tuple(Channel.make_simple_channel(ca, v) for v in urls)
+ return list(chain.from_iterable(c.urls(with_credentials, subdirs) for c in _channels))
@property
def base_url(self):
@@ -391,17 +404,19 @@ def url(self, with_credentials=False):
return None
-def prioritize_channels(channels, with_credentials=True, platform=None):
+def prioritize_channels(channels, with_credentials=True, subdirs=None):
# prioritize_channels returns and OrderedDict with platform-specific channel
# urls as the key, and a tuple of canonical channel name and channel priority
# number as the value
# ('https://conda.anaconda.org/conda-forge/osx-64/', ('conda-forge', 1))
result = odict()
- for q, chn in enumerate(channels):
+ q = -1 # channel priority counter
+ for chn in channels:
channel = Channel(chn)
- for url in channel.urls(with_credentials, platform):
+ for url in channel.urls(with_credentials, subdirs):
if url in result:
continue
+ q += 1
result[url] = channel.canonical_name, min(q, MAX_CHANNEL_PRIORITY - 1)
return result
| diff --git a/tests/base/test_context.py b/tests/base/test_context.py
--- a/tests/base/test_context.py
+++ b/tests/base/test_context.py
@@ -24,7 +24,7 @@
from unittest import TestCase
-class ContextTests(TestCase):
+class ContextCustomRcTests(TestCase):
def setUp(self):
string = dals("""
@@ -171,3 +171,13 @@ def test_describe_all(self):
from pprint import pprint
for name in paramter_names:
pprint(context.describe_parameter(name))
+
+
+class ContextDefaultRcTests(TestCase):
+
+ def test_subdirs(self):
+ assert context.subdirs == (context.subdir, 'noarch')
+
+ subdirs = ('linux-highest', 'linux-64', 'noarch')
+ with env_var('CONDA_SUBDIRS', ','.join(subdirs), reset_context):
+ assert context.subdirs == subdirs
diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -5,7 +5,7 @@
import os
from tempfile import gettempdir
-from conda.base.constants import APP_NAME
+from conda.base.constants import APP_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNELS_UNIX
from conda.common.io import env_var
from conda._vendor.auxlib.ish import dals
@@ -789,11 +789,46 @@ def test_env_var_file_urls(self):
prioritized = prioritize_channels(new_context.channels)
assert prioritized == OrderedDict((
("file://network_share/shared_folder/path/conda/%s" % context.subdir, ("file://network_share/shared_folder/path/conda", 0)),
- ("file://network_share/shared_folder/path/conda/noarch", ("file://network_share/shared_folder/path/conda", 0)),
- ("https://some.url/ch_name/%s" % context.subdir, ("https://some.url/ch_name", 1)),
- ("https://some.url/ch_name/noarch", ("https://some.url/ch_name", 1)),
- ("file:///some/place/on/my/machine/%s" % context.subdir, ("file:///some/place/on/my/machine", 2)),
- ("file:///some/place/on/my/machine/noarch", ("file:///some/place/on/my/machine", 2)),
+ ("file://network_share/shared_folder/path/conda/noarch", ("file://network_share/shared_folder/path/conda", 1)),
+ ("https://some.url/ch_name/%s" % context.subdir, ("https://some.url/ch_name", 2)),
+ ("https://some.url/ch_name/noarch", ("https://some.url/ch_name", 3)),
+ ("file:///some/place/on/my/machine/%s" % context.subdir, ("file:///some/place/on/my/machine", 4)),
+ ("file:///some/place/on/my/machine/noarch", ("file:///some/place/on/my/machine", 5)),
+ ))
+
+ def test_subdirs(self):
+ subdirs = ('linux-highest', 'linux-64', 'noarch')
+
+ def _channel_urls(channels=None):
+ for channel in channels or DEFAULT_CHANNELS_UNIX:
+ channel = Channel(channel)
+ for subdir in subdirs:
+ yield join_url(channel.base_url, subdir)
+
+ with env_var('CONDA_SUBDIRS', ','.join(subdirs), reset_context):
+ c = Channel('defaults')
+ assert c.urls() == list(_channel_urls())
+
+ c = Channel('conda-forge')
+ assert c.urls() == list(_channel_urls(('conda-forge',)))
+
+ channels = ('bioconda', 'conda-forge')
+ prioritized = prioritize_channels(channels)
+ assert prioritized == OrderedDict((
+ ("https://conda.anaconda.org/bioconda/linux-highest", ("bioconda", 0)),
+ ("https://conda.anaconda.org/bioconda/linux-64", ("bioconda", 1)),
+ ("https://conda.anaconda.org/bioconda/noarch", ("bioconda", 2)),
+ ("https://conda.anaconda.org/conda-forge/linux-highest", ("conda-forge", 3)),
+ ("https://conda.anaconda.org/conda-forge/linux-64", ("conda-forge", 4)),
+ ("https://conda.anaconda.org/conda-forge/noarch", ("conda-forge", 5)),
+ ))
+
+ prioritized = prioritize_channels(channels, subdirs=('linux-again', 'noarch'))
+ assert prioritized == OrderedDict((
+ ("https://conda.anaconda.org/bioconda/linux-again", ("bioconda", 0)),
+ ("https://conda.anaconda.org/bioconda/noarch", ("bioconda", 1)),
+ ("https://conda.anaconda.org/conda-forge/linux-again", ("conda-forge", 2)),
+ ("https://conda.anaconda.org/conda-forge/noarch", ("conda-forge", 3)),
))
| add subdirs configuration parameter
As a beta and undocumented feature for future use, add a configurable subdirs parameter.
| 2017-04-10T17:06:10 | -1.0 |
|
conda/conda | 5,103 | conda__conda-5103 | [
"4849",
"4849"
] | 58b12f4949fb50c1890a810942660982153af440 | diff --git a/conda/plan.py b/conda/plan.py
--- a/conda/plan.py
+++ b/conda/plan.py
@@ -467,7 +467,6 @@ def install_actions_list(prefix, index, specs, force=False, only_names=None, alw
channel_priority_map=None, is_update=False):
# type: (str, Dict[Dist, Record], List[str], bool, Option[List[str]], bool, bool, bool,
# bool, bool, bool, Dict[str, Sequence[str, int]]) -> List[Dict[weird]]
- str_specs = specs
specs = [MatchSpec(spec) for spec in specs]
r = get_resolve_object(index.copy(), prefix)
@@ -483,11 +482,11 @@ def install_actions_list(prefix, index, specs, force=False, only_names=None, alw
# Replace SpecsForPrefix specs with specs that were passed in in order to retain
# version information
- required_solves = match_to_original_specs(str_specs, grouped_specs)
+ required_solves = match_to_original_specs(specs, grouped_specs)
- actions = [get_actions_for_dists(dists_by_prefix, only_names, index, force,
+ actions = [get_actions_for_dists(specs_by_prefix, only_names, index, force,
always_copy, prune, update_deps, pinned)
- for dists_by_prefix in required_solves]
+ for specs_by_prefix in required_solves]
# Need to add unlink actions if updating a private env from root
if is_update and prefix == context.root_prefix:
@@ -601,8 +600,8 @@ def get_r(preferred_env):
return prefix_with_dists_no_deps_has_resolve
-def match_to_original_specs(str_specs, specs_for_prefix):
- matches_any_spec = lambda dst: next(spc for spc in str_specs if spc.startswith(dst))
+def match_to_original_specs(specs, specs_for_prefix):
+ matches_any_spec = lambda dst: next(spc for spc in specs if spc.name == dst)
matched_specs_for_prefix = []
for prefix_with_dists in specs_for_prefix:
linked = linked_data(prefix_with_dists.prefix)
@@ -618,13 +617,12 @@ def match_to_original_specs(str_specs, specs_for_prefix):
return matched_specs_for_prefix
-def get_actions_for_dists(dists_for_prefix, only_names, index, force, always_copy, prune,
+def get_actions_for_dists(specs_by_prefix, only_names, index, force, always_copy, prune,
update_deps, pinned):
root_only = ('conda', 'conda-env')
- prefix = dists_for_prefix.prefix
- dists = dists_for_prefix.specs
- r = dists_for_prefix.r
- specs = [MatchSpec(dist) for dist in dists]
+ prefix = specs_by_prefix.prefix
+ r = specs_by_prefix.r
+ specs = [MatchSpec(s) for s in specs_by_prefix.specs]
specs = augment_specs(prefix, specs, pinned)
linked = linked_data(prefix)
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -925,13 +925,16 @@ def test_install_mkdir(self):
try:
prefix = make_temp_prefix()
assert isdir(prefix)
- run_command(Commands.INSTALL, prefix, "python=3.5", "--mkdir")
- assert_package_is_installed(prefix, "python-3.5")
+ run_command(Commands.INSTALL, prefix, "python=3.5.2", "--mkdir")
+ assert_package_is_installed(prefix, "python-3.5.2")
rm_rf(prefix)
assert not isdir(prefix)
- run_command(Commands.INSTALL, prefix, "python=3.5", "--mkdir")
- assert_package_is_installed(prefix, "python-3.5")
+
+ # this part also a regression test for #4849
+ run_command(Commands.INSTALL, prefix, "python-dateutil=2.6.0", "python=3.5.2", "--mkdir")
+ assert_package_is_installed(prefix, "python-3.5.2")
+ assert_package_is_installed(prefix, "python-dateutil-2.6.0")
finally:
rmtree(prefix, ignore_errors=True)
diff --git a/tests/test_plan.py b/tests/test_plan.py
--- a/tests/test_plan.py
+++ b/tests/test_plan.py
@@ -1062,13 +1062,14 @@ def test_match_to_original_specs(self):
specs=IndexedSet(("test",))),
plan.SpecsForPrefix(prefix="some/prefix", r=self.res,
specs=IndexedSet(("test-spec", "test-spec2")))]
- matched = plan.match_to_original_specs(str_specs, grouped_specs)
+ matched = plan.match_to_original_specs(tuple(MatchSpec(s) for s in str_specs),
+ grouped_specs)
expected_output = [
plan.SpecsForPrefix(prefix="some/prefix/envs/_ranenv_",
r=test_r,
- specs=["test 1.2.0"]),
+ specs=[MatchSpec("test 1.2.0")]),
plan.SpecsForPrefix(prefix="some/prefix", r=self.res,
- specs=["test-spec 1.1*", "test-spec2 <4.3"])]
+ specs=[MatchSpec("test-spec 1.1*"), MatchSpec("test-spec2 <4.3")])]
assert len(matched) == len(expected_output)
assert matched == expected_output
| Version constraint ignored depending on order of args to `conda create` (4.3.13)
All of these command lines should resolve `python=2.7.12` I think but some choose `python=2.7.13`.
To reproduce I have to use both a certain package (python-dateutil) along with python, and put the packages in a certain order (python-dateutil first breaks, python-dateutil second works). `six` instead of `python-dateutil` never seems to break.
If I actually create the environment and it chooses 2.7.13, I am able to subsequently `conda install python=2.7.12=0`, so there doesn't seem to be a hard version constraint preventing that.
```
$ echo n | conda create --prefix=/tmp/blahblahboo "python-dateutil=2.6.0=py27_0" "python=2.7.12=0" | grep 'python:'
Exiting
python: 2.7.13-0
$ echo n | conda create --prefix=/tmp/blahblahboo "python=2.7.12=0" "python-dateutil=2.6.0=py27_0" | grep 'python:'
Exiting
python: 2.7.12-0
$ echo n | conda create --prefix=/tmp/blahblahboo "python=2.7.12=0" "six=1.10.0=py27_0" | grep 'python:'
Exiting
python: 2.7.12-0
$ echo n | conda create --prefix=/tmp/blahblahboo "six=1.10.0=py27_0" "python=2.7.12=0" | grep 'python:'
Exiting
python: 2.7.12-0
$ echo n | conda create --prefix=/tmp/blahblahboo "six=1.10.0=py27_0" "python-dateutil=2.6.0=py27_0" "python=2.7.12=0" | grep 'python:'
Exiting
python: 2.7.13-0
$ echo n | conda create --prefix=/tmp/blahblahboo "six=1.10.0=py27_0" "python=2.7.12=0" "python-dateutil=2.6.0=py27_0" | grep 'python:'
Exiting
python: 2.7.12-0
```
conda info
```
$ conda info -a
Current conda install:
platform : linux-64
conda version : 4.3.13
conda is private : False
conda-env version : 4.3.13
conda-build version : 2.0.2
python version : 2.7.12.final.0
requests version : 2.13.0
root environment : /home/hp/bin/anaconda2 (writable)
default environment : /home/hp/bin/anaconda2/envs/kapsel
envs directories : /home/hp/bin/anaconda2/envs
/home/hp/.conda/envs
package cache : /home/hp/bin/anaconda2/pkgs
/home/hp/.conda/pkgs
channel URLs : https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
https://conda.anaconda.org/t/<TOKEN>/binstar/linux-64
https://conda.anaconda.org/t/<TOKEN>/binstar/noarch
config file : /home/hp/.condarc
offline mode : False
user-agent : conda/4.3.13 requests/2.13.0 CPython/2.7.12 Linux/4.9.12-100.fc24.x86_64 Fedora/24 glibc/2.23
UID:GID : 1000:1000
# conda environments:
#
anaconda-platform /home/hp/bin/anaconda2/envs/anaconda-platform
kapsel * /home/hp/bin/anaconda2/envs/kapsel
kapsel-canary /home/hp/bin/anaconda2/envs/kapsel-canary
py2 /home/hp/bin/anaconda2/envs/py2
testing /home/hp/bin/anaconda2/envs/testing
root /home/hp/bin/anaconda2
sys.version: 2.7.12 |Anaconda 4.2.0 (64-bit)| (defaul...
sys.prefix: /home/hp/bin/anaconda2
sys.executable: /home/hp/bin/anaconda2/bin/python
conda location: /home/hp/bin/anaconda2/lib/python2.7/site-packages/conda
conda-build: /home/hp/bin/anaconda2/bin/conda-build
conda-convert: /home/hp/bin/anaconda2/bin/conda-convert
conda-develop: /home/hp/bin/anaconda2/bin/conda-develop
conda-env: /home/hp/bin/anaconda2/bin/conda-env
conda-index: /home/hp/bin/anaconda2/bin/conda-index
conda-inspect: /home/hp/bin/anaconda2/bin/conda-inspect
conda-kapsel: /home/hp/bin/anaconda2/envs/kapsel/bin/conda-kapsel
conda-metapackage: /home/hp/bin/anaconda2/bin/conda-metapackage
conda-render: /home/hp/bin/anaconda2/bin/conda-render
conda-server: /home/hp/bin/anaconda2/envs/kapsel/bin/conda-server
conda-sign: /home/hp/bin/anaconda2/bin/conda-sign
conda-skeleton: /home/hp/bin/anaconda2/bin/conda-skeleton
user site dirs:
CIO_TEST: <not set>
CONDA_DEFAULT_ENV: kapsel
CONDA_ENVS_PATH: <not set>
LD_LIBRARY_PATH: <not set>
PATH: /home/hp/bin/anaconda2/envs/kapsel/bin:/home/hp/bin:/usr/lib64/qt-3.3/bin:/usr/lib64/ccache:/usr/local/bin:/usr/local/sbin:/usr/bin:/usr/sbin
PYTHONHOME: <not set>
PYTHONPATH: <not set>
License directories:
/home/hp/.continuum
/home/hp/bin/anaconda2/licenses
License files (license*.txt):
/home/hp/.continuum/license_anaconda_repo_20161003210957.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160804160500.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160727174434.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728092403.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160830123618.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160803091557.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160830104354.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo.txt
Reading license file : 6
Signature valid : 6
Vendor match : 3
product : u'mkl-optimizations'
packages : u'mkl'
end_date : u'2017-05-24'
type : u'Trial'
product : u'iopro'
packages : u'iopro'
end_date : u'2017-05-24'
type : u'Trial'
product : u'accelerate'
packages : u'numbapro mkl'
end_date : u'2017-05-24'
type : u'Trial'
/home/hp/.continuum/license_anaconda_repo_20160823133054.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160927143648.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160803113033.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728095857.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728094900.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160830104619.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728093131.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20161003210908.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728094321.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
Package/feature end dates:
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
`$ /home/hp/bin/anaconda2/envs/kapsel/bin/conda info -a`
Traceback (most recent call last):
File "/home/hp/bin/anaconda2/lib/python2.7/site-packages/conda/exceptions.py", line 591, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/home/hp/bin/anaconda2/lib/python2.7/site-packages/conda/cli/main.py", line 134, in _main
exit_code = args.func(args, p)
File "/home/hp/bin/anaconda2/lib/python2.7/site-packages/conda/cli/main_info.py", line 324, in execute
show_info()
File "_license.pyx", line 363, in _license.show_info (_license.c:10610)
File "_license.pyx", line 310, in _license.get_end_dates (_license.c:9015)
File "_license.pyx", line 166, in _license.filter_vendor (_license.c:6214)
KeyError: 'vendor'
$ conda --version
conda 4.3.13
```
Version constraint ignored depending on order of args to `conda create` (4.3.13)
All of these command lines should resolve `python=2.7.12` I think but some choose `python=2.7.13`.
To reproduce I have to use both a certain package (python-dateutil) along with python, and put the packages in a certain order (python-dateutil first breaks, python-dateutil second works). `six` instead of `python-dateutil` never seems to break.
If I actually create the environment and it chooses 2.7.13, I am able to subsequently `conda install python=2.7.12=0`, so there doesn't seem to be a hard version constraint preventing that.
```
$ echo n | conda create --prefix=/tmp/blahblahboo "python-dateutil=2.6.0=py27_0" "python=2.7.12=0" | grep 'python:'
Exiting
python: 2.7.13-0
$ echo n | conda create --prefix=/tmp/blahblahboo "python=2.7.12=0" "python-dateutil=2.6.0=py27_0" | grep 'python:'
Exiting
python: 2.7.12-0
$ echo n | conda create --prefix=/tmp/blahblahboo "python=2.7.12=0" "six=1.10.0=py27_0" | grep 'python:'
Exiting
python: 2.7.12-0
$ echo n | conda create --prefix=/tmp/blahblahboo "six=1.10.0=py27_0" "python=2.7.12=0" | grep 'python:'
Exiting
python: 2.7.12-0
$ echo n | conda create --prefix=/tmp/blahblahboo "six=1.10.0=py27_0" "python-dateutil=2.6.0=py27_0" "python=2.7.12=0" | grep 'python:'
Exiting
python: 2.7.13-0
$ echo n | conda create --prefix=/tmp/blahblahboo "six=1.10.0=py27_0" "python=2.7.12=0" "python-dateutil=2.6.0=py27_0" | grep 'python:'
Exiting
python: 2.7.12-0
```
conda info
```
$ conda info -a
Current conda install:
platform : linux-64
conda version : 4.3.13
conda is private : False
conda-env version : 4.3.13
conda-build version : 2.0.2
python version : 2.7.12.final.0
requests version : 2.13.0
root environment : /home/hp/bin/anaconda2 (writable)
default environment : /home/hp/bin/anaconda2/envs/kapsel
envs directories : /home/hp/bin/anaconda2/envs
/home/hp/.conda/envs
package cache : /home/hp/bin/anaconda2/pkgs
/home/hp/.conda/pkgs
channel URLs : https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
https://conda.anaconda.org/t/<TOKEN>/binstar/linux-64
https://conda.anaconda.org/t/<TOKEN>/binstar/noarch
config file : /home/hp/.condarc
offline mode : False
user-agent : conda/4.3.13 requests/2.13.0 CPython/2.7.12 Linux/4.9.12-100.fc24.x86_64 Fedora/24 glibc/2.23
UID:GID : 1000:1000
# conda environments:
#
anaconda-platform /home/hp/bin/anaconda2/envs/anaconda-platform
kapsel * /home/hp/bin/anaconda2/envs/kapsel
kapsel-canary /home/hp/bin/anaconda2/envs/kapsel-canary
py2 /home/hp/bin/anaconda2/envs/py2
testing /home/hp/bin/anaconda2/envs/testing
root /home/hp/bin/anaconda2
sys.version: 2.7.12 |Anaconda 4.2.0 (64-bit)| (defaul...
sys.prefix: /home/hp/bin/anaconda2
sys.executable: /home/hp/bin/anaconda2/bin/python
conda location: /home/hp/bin/anaconda2/lib/python2.7/site-packages/conda
conda-build: /home/hp/bin/anaconda2/bin/conda-build
conda-convert: /home/hp/bin/anaconda2/bin/conda-convert
conda-develop: /home/hp/bin/anaconda2/bin/conda-develop
conda-env: /home/hp/bin/anaconda2/bin/conda-env
conda-index: /home/hp/bin/anaconda2/bin/conda-index
conda-inspect: /home/hp/bin/anaconda2/bin/conda-inspect
conda-kapsel: /home/hp/bin/anaconda2/envs/kapsel/bin/conda-kapsel
conda-metapackage: /home/hp/bin/anaconda2/bin/conda-metapackage
conda-render: /home/hp/bin/anaconda2/bin/conda-render
conda-server: /home/hp/bin/anaconda2/envs/kapsel/bin/conda-server
conda-sign: /home/hp/bin/anaconda2/bin/conda-sign
conda-skeleton: /home/hp/bin/anaconda2/bin/conda-skeleton
user site dirs:
CIO_TEST: <not set>
CONDA_DEFAULT_ENV: kapsel
CONDA_ENVS_PATH: <not set>
LD_LIBRARY_PATH: <not set>
PATH: /home/hp/bin/anaconda2/envs/kapsel/bin:/home/hp/bin:/usr/lib64/qt-3.3/bin:/usr/lib64/ccache:/usr/local/bin:/usr/local/sbin:/usr/bin:/usr/sbin
PYTHONHOME: <not set>
PYTHONPATH: <not set>
License directories:
/home/hp/.continuum
/home/hp/bin/anaconda2/licenses
License files (license*.txt):
/home/hp/.continuum/license_anaconda_repo_20161003210957.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160804160500.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160727174434.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728092403.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160830123618.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160803091557.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160830104354.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo.txt
Reading license file : 6
Signature valid : 6
Vendor match : 3
product : u'mkl-optimizations'
packages : u'mkl'
end_date : u'2017-05-24'
type : u'Trial'
product : u'iopro'
packages : u'iopro'
end_date : u'2017-05-24'
type : u'Trial'
product : u'accelerate'
packages : u'numbapro mkl'
end_date : u'2017-05-24'
type : u'Trial'
/home/hp/.continuum/license_anaconda_repo_20160823133054.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160927143648.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160803113033.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728095857.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728094900.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160830104619.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728093131.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20161003210908.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
/home/hp/.continuum/license_anaconda_repo_20160728094321.txt
Reading license file : 1
Error: no signature in license: {u'license': u'test'}
Signature valid : 0
Vendor match : 0
Package/feature end dates:
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
`$ /home/hp/bin/anaconda2/envs/kapsel/bin/conda info -a`
Traceback (most recent call last):
File "/home/hp/bin/anaconda2/lib/python2.7/site-packages/conda/exceptions.py", line 591, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/home/hp/bin/anaconda2/lib/python2.7/site-packages/conda/cli/main.py", line 134, in _main
exit_code = args.func(args, p)
File "/home/hp/bin/anaconda2/lib/python2.7/site-packages/conda/cli/main_info.py", line 324, in execute
show_info()
File "_license.pyx", line 363, in _license.show_info (_license.c:10610)
File "_license.pyx", line 310, in _license.get_end_dates (_license.c:9015)
File "_license.pyx", line 166, in _license.filter_vendor (_license.c:6214)
KeyError: 'vendor'
$ conda --version
conda 4.3.13
```
| 2017-04-21T20:12:25 | -1.0 |
|
conda/conda | 5,107 | conda__conda-5107 | [
"5102",
"5102"
] | 1985160dac27c4c05d079015e65a8b63141b87aa | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -107,7 +107,7 @@ class Context(Configuration):
element_type=string_types + (NoneType,))
client_ssl_cert_key = PrimitiveParameter(None, aliases=('client_cert_key',),
element_type=string_types + (NoneType,))
- proxy_servers = MapParameter(string_types)
+ proxy_servers = MapParameter(string_types + (NoneType,))
remote_connect_timeout_secs = PrimitiveParameter(9.15)
remote_read_timeout_secs = PrimitiveParameter(60.)
remote_max_retries = PrimitiveParameter(3)
| diff --git a/tests/base/test_context.py b/tests/base/test_context.py
--- a/tests/base/test_context.py
+++ b/tests/base/test_context.py
@@ -45,6 +45,13 @@ def setUp(self):
channel_alias: ftp://new.url:8082
conda-build:
root-dir: /some/test/path
+ proxy_servers:
+ http: http://user:pass@corp.com:8080
+ https: none
+ ftp:
+ sftp: ''
+ ftps: false
+ rsync: 'false'
""")
reset_context()
rd = odict(testdata=YamlRawParameter.make_raw_parameters('testdata', yaml_load(string)))
@@ -157,6 +164,14 @@ def test_custom_multichannels(self):
Channel('learn_from_every_thing'),
)
+ def test_proxy_servers(self):
+ assert context.proxy_servers['http'] == 'http://user:pass@corp.com:8080'
+ assert context.proxy_servers['https'] is None
+ assert context.proxy_servers['ftp'] is None
+ assert context.proxy_servers['sftp'] == ''
+ assert context.proxy_servers['ftps'] == 'False'
+ assert context.proxy_servers['rsync'] == 'False'
+
def test_conda_build_root_dir(self):
assert context.conda_build['root-dir'] == "/some/test/path"
from conda.config import rc
| Bad interpreation of null in proxy_servers section in .condarc since ver. 4.2.13
Upon upgrade to conda to version 4.2.13 the following settings in .condarc, which were meant to unset any http_proxy or https_proxy variables no longer work; result in HTTP error.
```
proxy_servers:
# Unset proxies so we can define internal channels shouldn't go outside anyway
http:
https:
```
Error received upon conda install:
```
An HTTP error occurred when trying to retrieve this URL.
ConnectionError(MaxRetryError("HTTPConnectionPool(host=u'None', port=80): Max retries exceeded with url: http://sfp-inf.jpmchase.net:9000/conda/mrad/channel/dev/linux-64/repodata.json (Caused by ProxyError('Cannot connect to proxy.', NewConnectionError('<requests.packages.urllib3.connection.HTTPConnection object at 0x7f197d701610>: Failed to establish a new connection: [Errno -2] Name or service not known',)))",),)
```
The reason is wrongly reinterpreting null values as 'None' string and subsequently trying to connect to hostname 'None'.
For those of you need a workaround now, change your .condarc as follows:
```
proxy_servers:
# Unset proxies so we can define internal channels shouldn't go outside anyway
http: ""
https: ""
```
Bad interpreation of null in proxy_servers section in .condarc since ver. 4.2.13
Upon upgrade to conda to version 4.2.13 the following settings in .condarc, which were meant to unset any http_proxy or https_proxy variables no longer work; result in HTTP error.
```
proxy_servers:
# Unset proxies so we can define internal channels shouldn't go outside anyway
http:
https:
```
Error received upon conda install:
```
An HTTP error occurred when trying to retrieve this URL.
ConnectionError(MaxRetryError("HTTPConnectionPool(host=u'None', port=80): Max retries exceeded with url: http://sfp-inf.jpmchase.net:9000/conda/mrad/channel/dev/linux-64/repodata.json (Caused by ProxyError('Cannot connect to proxy.', NewConnectionError('<requests.packages.urllib3.connection.HTTPConnection object at 0x7f197d701610>: Failed to establish a new connection: [Errno -2] Name or service not known',)))",),)
```
The reason is wrongly reinterpreting null values as 'None' string and subsequently trying to connect to hostname 'None'.
For those of you need a workaround now, change your .condarc as follows:
```
proxy_servers:
# Unset proxies so we can define internal channels shouldn't go outside anyway
http: ""
https: ""
```
| Sounds similar to what @nehaljwani fixed in https://github.com/conda/conda/pull/5096
Sounds similar to what @nehaljwani fixed in https://github.com/conda/conda/pull/5096 | 2017-04-22T03:42:45 | -1.0 |
conda/conda | 5,112 | conda__conda-5112 | [
"5111",
"5111"
] | c6f06c91f984b6b0dea4839b93f613aa821d9488 | diff --git a/conda/_vendor/auxlib/type_coercion.py b/conda/_vendor/auxlib/type_coercion.py
--- a/conda/_vendor/auxlib/type_coercion.py
+++ b/conda/_vendor/auxlib/type_coercion.py
@@ -231,8 +231,8 @@ def typify(value, type_hint=None):
elif not (type_hint - (STRING_TYPES_SET | {bool})):
return boolify(value, return_string=True)
elif not (type_hint - (STRING_TYPES_SET | {NoneType})):
- value = typify_str_no_hint(text_type(value))
- return None if value is None else text_type(value)
+ value = text_type(value)
+ return None if value.lower() == 'none' else value
elif not (type_hint - {bool, int}):
return typify_str_no_hint(text_type(value))
else:
| diff --git a/tests/base/test_context.py b/tests/base/test_context.py
--- a/tests/base/test_context.py
+++ b/tests/base/test_context.py
@@ -170,7 +170,7 @@ def test_proxy_servers(self):
assert context.proxy_servers['ftp'] is None
assert context.proxy_servers['sftp'] == ''
assert context.proxy_servers['ftps'] == 'False'
- assert context.proxy_servers['rsync'] == 'False'
+ assert context.proxy_servers['rsync'] == 'false'
def test_conda_build_root_dir(self):
assert context.conda_build['root-dir'] == "/some/test/path"
| typify for str + NoneType is wrong
https://github.com/conda/conda/pull/5107#issuecomment-296356913
It still seems to me as if `typify()` is doing something wrong:
```python
>>> from conda._vendor.auxlib.configuration import typify
>>> typify('false', str)
'false'
>>> typify('false', (str, type(None)))
'False'
```
Why should addition of type `None` introduce such a change? The code flow is:
```python
elif not (type_hint - (STRING_TYPES_SET | {NoneType})):
value = typify_str_no_hint(text_type(value))
return None if value is None else text_type(value)
```
I wonder why not just return `text_type(value)`? Why call `typify_str_no_hint`?
typify for str + NoneType is wrong
https://github.com/conda/conda/pull/5107#issuecomment-296356913
It still seems to me as if `typify()` is doing something wrong:
```python
>>> from conda._vendor.auxlib.configuration import typify
>>> typify('false', str)
'false'
>>> typify('false', (str, type(None)))
'False'
```
Why should addition of type `None` introduce such a change? The code flow is:
```python
elif not (type_hint - (STRING_TYPES_SET | {NoneType})):
value = typify_str_no_hint(text_type(value))
return None if value is None else text_type(value)
```
I wonder why not just return `text_type(value)`? Why call `typify_str_no_hint`?
| 2017-04-22T09:13:49 | -1.0 |
|
conda/conda | 5,157 | conda__conda-5157 | [
"5160"
] | ca57ef2a1ac70355d1aeb58ddc2da78f655c4fbc | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -6,6 +6,7 @@
from os.path import abspath, basename, dirname, expanduser, expandvars, isdir, join
import re
import sys
+from tempfile import NamedTemporaryFile
try:
from cytoolz.itertoolz import concatv
@@ -38,11 +39,13 @@ class Activator(object):
def __init__(self, shell):
from .base.context import context
self.context = context
+ self.shell = shell
if shell == 'posix':
self.pathsep_join = ':'.join
self.path_conversion = native_path_to_unix
self.script_extension = '.sh'
+ self.finalizer_extension = None # don't write to file
self.unset_var_tmpl = 'unset %s'
self.set_var_tmpl = 'export %s="%s"'
@@ -52,15 +55,17 @@ def __init__(self, shell):
self.pathsep_join = ':'.join
self.path_conversion = native_path_to_unix
self.script_extension = '.csh'
+ self.finalizer_extension = None # don't write to file
self.unset_var_tmpl = 'unset %s'
self.set_var_tmpl = 'setenv %s "%s"'
self.run_script_tmpl = 'source "%s"'
elif shell == 'xonsh':
- self.pathsep_join = lambda x: "['%s']" % "', '".join(x)
- self.path_conversion = lambda x: x # not sure if you want unix paths on windows or not
+ self.pathsep_join = ':'.join
+ self.path_conversion = native_path_to_unix
self.script_extension = '.xsh'
+ self.finalizer_extension = '.xsh'
self.unset_var_tmpl = 'del $%s'
self.set_var_tmpl = '$%s = "%s"'
@@ -69,20 +74,34 @@ def __init__(self, shell):
else:
raise NotImplementedError()
+ def _finalize(self, commands, ext):
+ if ext is None:
+ return '\n'.join(commands)
+ elif ext:
+ with NamedTemporaryFile(suffix=ext, delete=False) as tf:
+ tf.write(ensure_binary('\n'.join(commands)))
+ tf.write(ensure_binary('\n'))
+ return tf.name
+ else:
+ raise NotImplementedError()
+
def activate(self, name_or_prefix):
- return '\n'.join(self._make_commands(self.build_activate(name_or_prefix)))
+ return self._finalize(self._yield_commands(self.build_activate(name_or_prefix)),
+ self.finalizer_extension)
def deactivate(self):
- return '\n'.join(self._make_commands(self.build_deactivate()))
+ return self._finalize(self._yield_commands(self.build_deactivate()),
+ self.finalizer_extension)
def reactivate(self):
- return '\n'.join(self._make_commands(self.build_reactivate()))
+ return self._finalize(self._yield_commands(self.build_reactivate()),
+ self.finalizer_extension)
- def _make_commands(self, cmds_dict):
- for key in cmds_dict.get('unset_vars', ()):
+ def _yield_commands(self, cmds_dict):
+ for key in sorted(cmds_dict.get('unset_vars', ())):
yield self.unset_var_tmpl % key
- for key, value in iteritems(cmds_dict.get('set_vars', {})):
+ for key, value in sorted(iteritems(cmds_dict.get('set_vars', {}))):
yield self.set_var_tmpl % (key, value)
for script in cmds_dict.get('deactivate_scripts', ()):
@@ -231,7 +250,7 @@ def _get_starting_path_list(self):
return path.split(os.pathsep)
def _get_path_dirs(self, prefix):
- if on_win:
+ if on_win: # pragma: unix no cover
yield prefix.rstrip("\\")
yield join(prefix, 'Library', 'mingw-w64', 'bin')
yield join(prefix, 'Library', 'usr', 'bin')
@@ -256,7 +275,7 @@ def _replace_prefix_in_path(self, old_prefix, new_prefix, starting_path_dirs=Non
path_list = self._get_starting_path_list()
else:
path_list = list(starting_path_dirs)
- if on_win:
+ if on_win: # pragma: unix no cover
# windows has a nasty habit of adding extra Library\bin directories
prefix_dirs = tuple(self._get_path_dirs(old_prefix))
try:
@@ -302,17 +321,24 @@ def expand(path):
return abspath(expanduser(expandvars(path)))
-def native_path_to_unix(*paths):
+def ensure_binary(value):
+ try:
+ return value.encode('utf-8')
+ except AttributeError: # pragma: no cover
+ # AttributeError: '<>' object has no attribute 'encode'
+ # In this case assume already binary type and do nothing
+ return value
+
+
+def native_path_to_unix(*paths): # pragma: unix no cover
# on windows, uses cygpath to convert windows native paths to posix paths
if not on_win:
return paths[0] if len(paths) == 1 else paths
from subprocess import PIPE, Popen
from shlex import split
- command = 'cygpath.exe --path -f -'
+ command = 'cygpath --path -f -'
p = Popen(split(command), stdin=PIPE, stdout=PIPE, stderr=PIPE)
- joined = ("%s" % os.pathsep).join(paths)
- if hasattr(joined, 'encode'):
- joined = joined.encode('utf-8')
+ joined = ensure_binary(("%s" % os.pathsep).join(paths))
stdout, stderr = p.communicate(input=joined)
rc = p.returncode
if rc != 0 or stderr:
@@ -366,4 +392,4 @@ def main():
if __name__ == '__main__':
- main()
+ sys.exit(main())
diff --git a/conda/cli/main.py b/conda/cli/main.py
--- a/conda/cli/main.py
+++ b/conda/cli/main.py
@@ -161,4 +161,4 @@ def main(*args):
if __name__ == '__main__':
- main()
+ sys.exit(main())
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -3,10 +3,13 @@
from logging import getLogger
import os
-from os.path import join
+from os.path import join, isdir
import sys
+from tempfile import gettempdir
from unittest import TestCase
+from uuid import uuid4
+from conda._vendor.auxlib.ish import dals
import pytest
from conda.activate import Activator
@@ -15,6 +18,7 @@
from conda.common.io import env_var
from conda.exceptions import EnvironmentLocationNotFound, EnvironmentNameNotFound
from conda.gateways.disk.create import mkdir_p
+from conda.gateways.disk.delete import rm_rf
from conda.gateways.disk.update import touch
from tests.helpers import tempdir
@@ -320,6 +324,93 @@ def test_build_deactivate_shlvl_1(self):
assert builder['deactivate_scripts'] == [deactivate_d_1]
+class ShellWrapperUnitTests(TestCase):
+
+ def setUp(self):
+ tempdirdir = gettempdir()
+
+ prefix_dirname = str(uuid4())[:4] + ' ' + str(uuid4())[:4]
+ self.prefix = join(tempdirdir, prefix_dirname)
+ mkdir_p(join(self.prefix, 'conda-meta'))
+ assert isdir(self.prefix)
+ touch(join(self.prefix, 'conda-meta', 'history'))
+
+ def tearDown(self):
+ rm_rf(self.prefix)
+
+ def make_dot_d_files(self, extension):
+ mkdir_p(join(self.prefix, 'etc', 'conda', 'activate.d'))
+ mkdir_p(join(self.prefix, 'etc', 'conda', 'deactivate.d'))
+
+ touch(join(self.prefix, 'etc', 'conda', 'activate.d', 'ignore.txt'))
+ touch(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'ignore.txt'))
+
+ touch(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1' + extension))
+ touch(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1' + extension))
+
+ @pytest.mark.xfail(on_win, strict=True, reason="native_path_to_unix is broken on appveyor; "
+ "will fix in future PR")
+ def test_xonsh_basic(self):
+ activator = Activator('xonsh')
+ self.make_dot_d_files(activator.script_extension)
+
+ activate_result = activator.activate(self.prefix)
+ with open(activate_result) as fh:
+ activate_data = fh.read()
+ rm_rf(activate_result)
+
+ new_path = activator.pathsep_join(activator._add_prefix_to_path(self.prefix))
+ assert activate_data == dals("""
+ $CONDA_DEFAULT_ENV = "%(prefix)s"
+ $CONDA_PREFIX = "%(prefix)s"
+ $CONDA_PROMPT_MODIFIER = "(%(prefix)s) "
+ $CONDA_PYTHON_EXE = "%(sys_executable)s"
+ $CONDA_SHLVL = "1"
+ $PATH = "%(new_path)s"
+ source "%(activate1)s"
+ """) % {
+ 'prefix': self.prefix,
+ 'new_path': new_path,
+ 'sys_executable': sys.executable,
+ 'activate1': join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.xsh')
+ }
+
+ with env_var('CONDA_PREFIX', self.prefix):
+ with env_var('CONDA_SHLVL', '1'):
+ with env_var('PATH', new_path):
+ reactivate_result = activator.reactivate()
+ with open(reactivate_result) as fh:
+ reactivate_data = fh.read()
+ rm_rf(reactivate_result)
+
+ assert reactivate_data == dals("""
+ source "%(deactivate1)s"
+ source "%(activate1)s"
+ """) % {
+ 'activate1': join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.xsh'),
+ 'deactivate1': join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.xsh'),
+ }
+
+ deactivate_result = activator.deactivate()
+ with open(deactivate_result) as fh:
+ deactivate_data = fh.read()
+ rm_rf(deactivate_result)
+
+ new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
+ assert deactivate_data == dals("""
+ del $CONDA_DEFAULT_ENV
+ del $CONDA_PREFIX
+ del $CONDA_PROMPT_MODIFIER
+ del $CONDA_PYTHON_EXE
+ $CONDA_SHLVL = "0"
+ $PATH = "%(new_path)s"
+ source "%(deactivate1)s"
+ """) % {
+ 'new_path': new_path,
+ 'deactivate1': join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.xsh'),
+ }
+
+
@pytest.mark.integration
class ActivatorIntegrationTests(TestCase):
| conda xontrib xonsh wrapper
Make a wrapper for xonsh.
| 2017-04-27T01:31:36 | -1.0 |
|
conda/conda | 5,186 | conda__conda-5186 | [
"5184",
"5184"
] | 2e97c19d63b0ec3c075ed5d706032b891222ec2d | diff --git a/conda/common/configuration.py b/conda/common/configuration.py
--- a/conda/common/configuration.py
+++ b/conda/common/configuration.py
@@ -105,7 +105,7 @@ def __init__(self, parameter_name, parameter_value, source, wrong_type, valid_ty
self.valid_types = valid_types
if msg is None:
msg = ("Parameter %s = %r declared in %s has type %s.\n"
- "Valid types: %s." % (parameter_name, parameter_value,
+ "Valid types:\n%s" % (parameter_name, parameter_value,
source, wrong_type, pretty_list(valid_types)))
super(InvalidTypeError, self).__init__(parameter_name, parameter_value, source, msg=msg)
@@ -654,18 +654,23 @@ def collect_errors(self, instance, value, source="<<merged>>"):
def _merge(self, matches):
# get matches up to and including first important_match
# but if no important_match, then all matches are important_matches
- relevant_matches = self._first_important_matches(matches)
+ relevant_matches_and_values = tuple((match, match.value(self)) for match in
+ self._first_important_matches(matches))
+ for match, value in relevant_matches_and_values:
+ if not isinstance(value, Mapping):
+ raise InvalidTypeError(self.name, value, match.source, value.__class__.__name__,
+ self._type.__name__)
# mapkeys with important matches
def key_is_important(match, key):
return match.valueflags(self).get(key) is ParameterFlag.final
important_maps = tuple(dict((k, v)
- for k, v in iteritems(match.value(self))
+ for k, v in iteritems(match_value)
if key_is_important(match, k))
- for match in relevant_matches)
+ for match, match_value in relevant_matches_and_values)
# dump all matches in a dict
# then overwrite with important matches
- return merge(concatv((m.value(self) for m in relevant_matches),
+ return merge(concatv((v for _, v in relevant_matches_and_values),
reversed(important_maps)))
def repr_raw(self, raw_parameter):
| diff --git a/tests/common/test_configuration.py b/tests/common/test_configuration.py
--- a/tests/common/test_configuration.py
+++ b/tests/common/test_configuration.py
@@ -424,7 +424,11 @@ def test_config_resets(self):
assert config.changeps1 is False
def test_empty_map_parameter(self):
-
config = SampleConfiguration()._set_raw_data(load_from_string_data('bad_boolean_map'))
config.check_source('bad_boolean_map')
+ def test_invalid_map_parameter(self):
+ data = odict(s1=YamlRawParameter.make_raw_parameters('s1', {'proxy_servers': 'blah'}))
+ config = SampleConfiguration()._set_raw_data(data)
+ with raises(InvalidTypeError):
+ config.proxy_servers
| "conda" install or update throwing AttributeError: 'str' object has no attribute items
I've installed the last version of Anaconda3 with Python 3.6, which has the "conda" command version 4.3.14 on a Windows 7 without administrator rights to my account, but I could successfully install Anaconda in my <user>\appdata\local Windows folder.
When I try to install or update any package, I've got the following error:
```
`$ C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-script.py updat
e`
Traceback (most recent call last):
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main.py", line 134, in _main
exit_code = args.func(args, p)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_update.py", line 65, in execute
install(args, parser, 'update')
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\install.py", line 130, in install
context.validate_configuration()
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 838, in validate_configuration
raise_errors(tuple(chain.from_iterable((errors, post_errors))))
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 836, in <genexpr>
for name in self.parameter_names)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 829, in _collect_validation_error
func(*args, **kwargs)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 447, in __get__
result = typify_data_structure(self._merge(matches) if matches else self
.default,
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in _merge
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in <genexpr>
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\compat.py", line 71, in iteritems
return iter(d.items(**kw))
AttributeError: 'str' object has no attribute 'items'
```
Any hints?
"conda" install or update throwing AttributeError: 'str' object has no attribute items
I've installed the last version of Anaconda3 with Python 3.6, which has the "conda" command version 4.3.14 on a Windows 7 without administrator rights to my account, but I could successfully install Anaconda in my <user>\appdata\local Windows folder.
When I try to install or update any package, I've got the following error:
```
`$ C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-script.py updat
e`
Traceback (most recent call last):
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main.py", line 134, in _main
exit_code = args.func(args, p)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_update.py", line 65, in execute
install(args, parser, 'update')
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\install.py", line 130, in install
context.validate_configuration()
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 838, in validate_configuration
raise_errors(tuple(chain.from_iterable((errors, post_errors))))
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 836, in <genexpr>
for name in self.parameter_names)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 829, in _collect_validation_error
func(*args, **kwargs)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 447, in __get__
result = typify_data_structure(self._merge(matches) if matches else self
.default,
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in _merge
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in <genexpr>
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\compat.py", line 71, in iteritems
return iter(d.items(**kw))
AttributeError: 'str' object has no attribute 'items'
```
Any hints?
| @srodriguex Could you please share the output for `conda info -a`?
@nehaljwani Here you go:
```
C:\Users\<username>>conda info -a
Current conda install:
platform : win-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : not installed
python version : 3.6.0.final.0
requests version : 2.12.4
root environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3 (wri
able)
default environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3
envs directories : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\envs
C:\Users\<username>\AppData\Local\conda\conda\envs
C:\Users\<username>\.conda\envs
package cache : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\pkgs
C:\Users\<username>\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/anaconda-fusion/win-64
https://conda.anaconda.org/anaconda-fusion/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\<username>\.condarc
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/3.6.0 Windows/7
indows/6.1.7601
# conda environments:
#
root * C:\Users\<username>\AppData\Local\Continuum\Anaconda3
sys.version: 3.6.0 |Anaconda 4.3.1 (64-bit)| (default...
sys.prefix: C:\Users\<username>\AppData\Local\Continuum\Anaconda3
sys.executable: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\python.exe
conda location: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packag
s\conda
conda-build: None
conda-env: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-env.ex
conda-server: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-ser
er.exe
user site dirs:
CIO_TEST: <not set>
CONDA_DEFAULT_ENV: <not set>
CONDA_ENVS_PATH: <not set>
PATH: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Library\bin;C:\oracle\pro
uct\11.2.0\client_1\bin;C:\ProgramData\Oracle\Java\javapath;C:\WINDOWS\system32
C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\
C:\Program Files (x86)\IXOS\bin;C:\Users\<username>\AppData\Local\Continuum\Anaconda3
C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts;C:\Users\<username>\AppData\L
cal\Continuum\Anaconda3\Library\bin;c:\users\<username>\bin
PYTHONHOME: <not set>
PYTHONPATH: <not set>
License directories:
C:\Users\<username>\.continuum
C:\Users\<username>\AppData\Local\Continuum\Anaconda3\licenses
C:\Users\<username>\AppData\Roaming\Continuum
License files (license*.txt):
Package/feature end dates:
```
@srodriguex Could you also share output for: `conda config --show`
@nehaljwani Right away sir:
```
C:\Users\<username>>conda config --show
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : not installed
python version : 3.6.0.final.0
requests version : 2.12.4
root environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3 (writ
able)
default environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3
envs directories : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\envs
C:\Users\<username>\AppData\Local\conda\conda\envs
C:\Users\<username>\.conda\envs
package cache : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\pkgs
C:\Users\<username>\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/anaconda-fusion/win-64
https://conda.anaconda.org/anaconda-fusion/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\<username>\.condarc
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/3.6.0 Windows/7 W
indows/6.1.7601
`$ C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-script.py confi
g --show`
Traceback (most recent call last):
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main.py", line 134, in _main
exit_code = args.func(args, p)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_config.py", line 234, in execute
execute_config(args, parser)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_config.py", line 308, in execute_config
'verbosity',
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_config.py", line 279, in <genexpr>
for key in sorted(('add_anaconda_token',
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 447, in __get__
result = typify_data_structure(self._merge(matches) if matches else self
.default,
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in _merge
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in <genexpr>
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\compat.py", line 71, in iteritems
return iter(d.items(**kw))
AttributeError: 'str' object has no attribute 'items'
```
Does `conda config --show-sources` also error?
And also `conda config --validate`?
I am able to reproduce the exact same error when, for any of of the config parameters , I pass a string instead of the expected list or key pair value. Example, if I have:
```
proxy_servers: http://blah.com:3128
```
Instead of:
```
proxy_servers:
http: http://blah.com:3128
```
In the above scenario, `conda config --show-sources` throws the exception (expected):
```
InvalidTypeError: Parameter proxy_servers = 'http://blah.com:3128' declared in <<merged>> has type <class 'str'>.
Valid types: - <class 'dict'>.
```
But `conda config --show` throws a traceback instead of exception.
@kalefranz Here are your answers:
````
C:\Users\<username>>conda config --show-sources
InvalidTypeError: Parameter proxy_servers = 'http:127.0.0.1:53128' declared in <
<merged>> has type <class 'str'>.
Valid types: - <class 'dict'>.
````
and
````
C:\Users\cmsi>conda --validate
usage: conda-script.py [-h] [-V] command ...
conda-script.py: error: the following arguments are required: command
````
Here you have my .condarc file, I'm behind a proxy so I'm using a CNTLM proxy wrapper:
````
proxy_servers: http:127.0.0.1:53128
channels:
- anaconda-fusion
- defaults
ssl_verify: true
````
@srodriguex Replace...
```
proxy_servers: http:127.0.0.1:53128
```
...with:
```
proxy_servers:
http: http://127.0.0.1:53128
https: http://127.0.0.1:53128
```
@nehaljwani The substitution works setting the "ssl_verify" to "false". So the problem was in the .condarc file... I was almost pretty sure I followed the [documentation for proxy configuration](https://conda.io/docs/config.html#configure-conda-for-use-behind-a-proxy-server-proxy-servers), but I have forgotten enclosing with the (') character.
Labelled this issue as a bug. PR coming for a better error message.
@srodriguex Could you please share the output for `conda info -a`?
@nehaljwani Here you go:
```
C:\Users\<username>>conda info -a
Current conda install:
platform : win-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : not installed
python version : 3.6.0.final.0
requests version : 2.12.4
root environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3 (wri
able)
default environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3
envs directories : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\envs
C:\Users\<username>\AppData\Local\conda\conda\envs
C:\Users\<username>\.conda\envs
package cache : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\pkgs
C:\Users\<username>\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/anaconda-fusion/win-64
https://conda.anaconda.org/anaconda-fusion/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\<username>\.condarc
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/3.6.0 Windows/7
indows/6.1.7601
# conda environments:
#
root * C:\Users\<username>\AppData\Local\Continuum\Anaconda3
sys.version: 3.6.0 |Anaconda 4.3.1 (64-bit)| (default...
sys.prefix: C:\Users\<username>\AppData\Local\Continuum\Anaconda3
sys.executable: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\python.exe
conda location: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packag
s\conda
conda-build: None
conda-env: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-env.ex
conda-server: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-ser
er.exe
user site dirs:
CIO_TEST: <not set>
CONDA_DEFAULT_ENV: <not set>
CONDA_ENVS_PATH: <not set>
PATH: C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Library\bin;C:\oracle\pro
uct\11.2.0\client_1\bin;C:\ProgramData\Oracle\Java\javapath;C:\WINDOWS\system32
C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\
C:\Program Files (x86)\IXOS\bin;C:\Users\<username>\AppData\Local\Continuum\Anaconda3
C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts;C:\Users\<username>\AppData\L
cal\Continuum\Anaconda3\Library\bin;c:\users\<username>\bin
PYTHONHOME: <not set>
PYTHONPATH: <not set>
License directories:
C:\Users\<username>\.continuum
C:\Users\<username>\AppData\Local\Continuum\Anaconda3\licenses
C:\Users\<username>\AppData\Roaming\Continuum
License files (license*.txt):
Package/feature end dates:
```
@srodriguex Could you also share output for: `conda config --show`
@nehaljwani Right away sir:
```
C:\Users\<username>>conda config --show
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.14
conda is private : False
conda-env version : 4.3.14
conda-build version : not installed
python version : 3.6.0.final.0
requests version : 2.12.4
root environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3 (writ
able)
default environment : C:\Users\<username>\AppData\Local\Continuum\Anaconda3
envs directories : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\envs
C:\Users\<username>\AppData\Local\conda\conda\envs
C:\Users\<username>\.conda\envs
package cache : C:\Users\<username>\AppData\Local\Continuum\Anaconda3\pkgs
C:\Users\<username>\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/anaconda-fusion/win-64
https://conda.anaconda.org/anaconda-fusion/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\<username>\.condarc
offline mode : False
user-agent : conda/4.3.14 requests/2.12.4 CPython/3.6.0 Windows/7 W
indows/6.1.7601
`$ C:\Users\<username>\AppData\Local\Continuum\Anaconda3\Scripts\conda-script.py confi
g --show`
Traceback (most recent call last):
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\exceptions.py", line 573, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main.py", line 134, in _main
exit_code = args.func(args, p)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_config.py", line 234, in execute
execute_config(args, parser)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_config.py", line 308, in execute_config
'verbosity',
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\cli\main_config.py", line 279, in <genexpr>
for key in sorted(('add_anaconda_token',
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 447, in __get__
result = typify_data_structure(self._merge(matches) if matches else self
.default,
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in _merge
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\configuration.py", line 664, in <genexpr>
for match in relevant_matches)
File "C:\Users\<username>\AppData\Local\Continuum\Anaconda3\lib\site-packages\co
nda\common\compat.py", line 71, in iteritems
return iter(d.items(**kw))
AttributeError: 'str' object has no attribute 'items'
```
Does `conda config --show-sources` also error?
And also `conda config --validate`?
I am able to reproduce the exact same error when, for any of of the config parameters , I pass a string instead of the expected list or key pair value. Example, if I have:
```
proxy_servers: http://blah.com:3128
```
Instead of:
```
proxy_servers:
http: http://blah.com:3128
```
In the above scenario, `conda config --show-sources` throws the exception (expected):
```
InvalidTypeError: Parameter proxy_servers = 'http://blah.com:3128' declared in <<merged>> has type <class 'str'>.
Valid types: - <class 'dict'>.
```
But `conda config --show` throws a traceback instead of exception.
@kalefranz Here are your answers:
````
C:\Users\<username>>conda config --show-sources
InvalidTypeError: Parameter proxy_servers = 'http:127.0.0.1:53128' declared in <
<merged>> has type <class 'str'>.
Valid types: - <class 'dict'>.
````
and
````
C:\Users\cmsi>conda --validate
usage: conda-script.py [-h] [-V] command ...
conda-script.py: error: the following arguments are required: command
````
Here you have my .condarc file, I'm behind a proxy so I'm using a CNTLM proxy wrapper:
````
proxy_servers: http:127.0.0.1:53128
channels:
- anaconda-fusion
- defaults
ssl_verify: true
````
@srodriguex Replace...
```
proxy_servers: http:127.0.0.1:53128
```
...with:
```
proxy_servers:
http: http://127.0.0.1:53128
https: http://127.0.0.1:53128
```
@nehaljwani The substitution works setting the "ssl_verify" to "false". So the problem was in the .condarc file... I was almost pretty sure I followed the [documentation for proxy configuration](https://conda.io/docs/config.html#configure-conda-for-use-behind-a-proxy-server-proxy-servers), but I have forgotten enclosing with the (') character.
Labelled this issue as a bug. PR coming for a better error message. | 2017-04-28T20:40:21 | -1.0 |
conda/conda | 5,192 | conda__conda-5192 | [
"5189",
"5189"
] | b4aae214af25845ac0f68da8e40f1d33c1dcd5ad | diff --git a/conda/common/configuration.py b/conda/common/configuration.py
--- a/conda/common/configuration.py
+++ b/conda/common/configuration.py
@@ -572,7 +572,12 @@ def collect_errors(self, instance, value, source="<<merged>>"):
def _merge(self, matches):
# get matches up to and including first important_match
# but if no important_match, then all matches are important_matches
- relevant_matches = self._first_important_matches(matches)
+ relevant_matches_and_values = tuple((match, match.value(self)) for match in
+ self._first_important_matches(matches))
+ for match, value in relevant_matches_and_values:
+ if not isinstance(value, tuple):
+ raise InvalidTypeError(self.name, value, match.source, value.__class__.__name__,
+ self._type.__name__)
# get individual lines from important_matches that were marked important
# these will be prepended to the final result
@@ -581,16 +586,17 @@ def get_marked_lines(match, marker, parameter_obj):
for line, flag in zip(match.value(parameter_obj),
match.valueflags(parameter_obj))
if flag is marker) if match else ()
- top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m in relevant_matches)
+ top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m, _ in
+ relevant_matches_and_values)
# also get lines that were marked as bottom, but reverse the match order so that lines
# coming earlier will ultimately be last
- bottom_lines = concat(get_marked_lines(m, ParameterFlag.bottom, self) for m in
- reversed(relevant_matches))
+ bottom_lines = concat(get_marked_lines(m, ParameterFlag.bottom, self) for m, _ in
+ reversed(relevant_matches_and_values))
# now, concat all lines, while reversing the matches
# reverse because elements closer to the end of search path take precedence
- all_lines = concat(m.value(self) for m in reversed(relevant_matches))
+ all_lines = concat(v for _, v in reversed(relevant_matches_and_values))
# stack top_lines + all_lines, then de-dupe
top_deduped = tuple(unique(concatv(top_lines, all_lines)))
| diff --git a/tests/common/test_configuration.py b/tests/common/test_configuration.py
--- a/tests/common/test_configuration.py
+++ b/tests/common/test_configuration.py
@@ -432,3 +432,9 @@ def test_invalid_map_parameter(self):
config = SampleConfiguration()._set_raw_data(data)
with raises(InvalidTypeError):
config.proxy_servers
+
+ def test_invalid_seq_parameter(self):
+ data = odict(s1=YamlRawParameter.make_raw_parameters('s1', {'channels': 'y_u_no_tuple'}))
+ config = SampleConfiguration()._set_raw_data(data)
+ with raises(InvalidTypeError):
+ config.channels
| Improve error message on invalid SequenceParameter
For a malformed configuration parameter of type sequence:
```
(test) [hulk@ranganork conda]# cat ~/.condarc
channels: defaults
```
The following shows the expected error:
```
(test) [hulk@ranganork conda]# conda config --validate
InvalidTypeError: Parameter _channels = 'defaults' declared in <<merged>> has type <class 'str'>.
Valid types:
- <class 'tuple'>
```
But the following throws an exception:
```
(test) [hulk@ranganork conda]# conda update conda
Traceback (most recent call last):
File "/root/conda/conda/exceptions.py", line 632, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/root/conda/conda/cli/main.py", line 134, in _main
exit_code = args.func(args, p)
File "/root/conda/conda/cli/main_update.py", line 65, in execute
install(args, parser, 'update')
File "/root/conda/conda/cli/install.py", line 128, in install
context.validate_configuration()
File "/root/conda/conda/common/configuration.py", line 844, in validate_configuration
raise_errors(tuple(chain.from_iterable((errors, post_errors))))
File "/root/conda/conda/common/configuration.py", line 842, in <genexpr>
for name in self.parameter_names)
File "/root/conda/conda/common/configuration.py", line 835, in _collect_validation_error
func(*args, **kwargs)
File "/root/conda/conda/common/configuration.py", line 448, in __get__
result = typify_data_structure(self._merge(matches) if matches else self.default,
File "/root/conda/conda/common/configuration.py", line 596, in _merge
top_deduped = tuple(unique(concatv(top_lines, all_lines)))
File "/root/conda/conda/_vendor/toolz/itertoolz.py", line 209, in unique
for item in seq:
File "/root/conda/conda/common/configuration.py", line 584, in <genexpr>
top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m in relevant_matches)
File "/root/conda/conda/common/configuration.py", line 583, in get_marked_lines
if flag is marker) if match else ()
TypeError: zip argument #2 must support iteration
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/conda/envs/test/bin/conda", line 11, in <module>
load_entry_point('conda', 'console_scripts', 'conda')()
File "/root/conda/conda/cli/main.py", line 162, in main
return conda_exception_handler(_main, *args)
File "/root/conda/conda/exceptions.py", line 636, in conda_exception_handler
return handle_exception(e)
File "/root/conda/conda/exceptions.py", line 626, in handle_exception
print_unexpected_error_message(e)
File "/root/conda/conda/exceptions.py", line 588, in print_unexpected_error_message
stderrlogger.info(get_main_info_str(get_info_dict()))
File "/root/conda/conda/cli/main_info.py", line 196, in get_info_dict
channels = list(prioritize_channels(context.channels).keys())
File "/root/conda/conda/base/context.py", line 448, in channels
return self._channels
File "/root/conda/conda/common/configuration.py", line 448, in __get__
result = typify_data_structure(self._merge(matches) if matches else self.default,
File "/root/conda/conda/common/configuration.py", line 596, in _merge
top_deduped = tuple(unique(concatv(top_lines, all_lines)))
File "/root/conda/conda/_vendor/toolz/itertoolz.py", line 209, in unique
for item in seq:
File "/root/conda/conda/common/configuration.py", line 584, in <genexpr>
top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m in relevant_matches)
File "/root/conda/conda/common/configuration.py", line 583, in get_marked_lines
if flag is marker) if match else ()
TypeError: zip argument #2 must support iteration
```
Improve error message on invalid SequenceParameter
For a malformed configuration parameter of type sequence:
```
(test) [hulk@ranganork conda]# cat ~/.condarc
channels: defaults
```
The following shows the expected error:
```
(test) [hulk@ranganork conda]# conda config --validate
InvalidTypeError: Parameter _channels = 'defaults' declared in <<merged>> has type <class 'str'>.
Valid types:
- <class 'tuple'>
```
But the following throws an exception:
```
(test) [hulk@ranganork conda]# conda update conda
Traceback (most recent call last):
File "/root/conda/conda/exceptions.py", line 632, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/root/conda/conda/cli/main.py", line 134, in _main
exit_code = args.func(args, p)
File "/root/conda/conda/cli/main_update.py", line 65, in execute
install(args, parser, 'update')
File "/root/conda/conda/cli/install.py", line 128, in install
context.validate_configuration()
File "/root/conda/conda/common/configuration.py", line 844, in validate_configuration
raise_errors(tuple(chain.from_iterable((errors, post_errors))))
File "/root/conda/conda/common/configuration.py", line 842, in <genexpr>
for name in self.parameter_names)
File "/root/conda/conda/common/configuration.py", line 835, in _collect_validation_error
func(*args, **kwargs)
File "/root/conda/conda/common/configuration.py", line 448, in __get__
result = typify_data_structure(self._merge(matches) if matches else self.default,
File "/root/conda/conda/common/configuration.py", line 596, in _merge
top_deduped = tuple(unique(concatv(top_lines, all_lines)))
File "/root/conda/conda/_vendor/toolz/itertoolz.py", line 209, in unique
for item in seq:
File "/root/conda/conda/common/configuration.py", line 584, in <genexpr>
top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m in relevant_matches)
File "/root/conda/conda/common/configuration.py", line 583, in get_marked_lines
if flag is marker) if match else ()
TypeError: zip argument #2 must support iteration
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/conda/envs/test/bin/conda", line 11, in <module>
load_entry_point('conda', 'console_scripts', 'conda')()
File "/root/conda/conda/cli/main.py", line 162, in main
return conda_exception_handler(_main, *args)
File "/root/conda/conda/exceptions.py", line 636, in conda_exception_handler
return handle_exception(e)
File "/root/conda/conda/exceptions.py", line 626, in handle_exception
print_unexpected_error_message(e)
File "/root/conda/conda/exceptions.py", line 588, in print_unexpected_error_message
stderrlogger.info(get_main_info_str(get_info_dict()))
File "/root/conda/conda/cli/main_info.py", line 196, in get_info_dict
channels = list(prioritize_channels(context.channels).keys())
File "/root/conda/conda/base/context.py", line 448, in channels
return self._channels
File "/root/conda/conda/common/configuration.py", line 448, in __get__
result = typify_data_structure(self._merge(matches) if matches else self.default,
File "/root/conda/conda/common/configuration.py", line 596, in _merge
top_deduped = tuple(unique(concatv(top_lines, all_lines)))
File "/root/conda/conda/_vendor/toolz/itertoolz.py", line 209, in unique
for item in seq:
File "/root/conda/conda/common/configuration.py", line 584, in <genexpr>
top_lines = concat(get_marked_lines(m, ParameterFlag.top, self) for m in relevant_matches)
File "/root/conda/conda/common/configuration.py", line 583, in get_marked_lines
if flag is marker) if match else ()
TypeError: zip argument #2 must support iteration
```
| Similar to #5184
Do you want to tackle this one @nehaljwani?
Similar to #5184
Do you want to tackle this one @nehaljwani? | 2017-04-29T05:42:46 | -1.0 |
conda/conda | 5,200 | conda__conda-5200 | [
"3470",
"3470"
] | 3beff3b3cb8c6ca3661a3364e77c614d1ec623e0 | diff --git a/conda/__init__.py b/conda/__init__.py
--- a/conda/__init__.py
+++ b/conda/__init__.py
@@ -41,7 +41,7 @@ def __init__(self, message, caused_by=None, **kwargs):
super(CondaError, self).__init__(message)
def __repr__(self):
- return '%s: %s\n' % (self.__class__.__name__, text_type(self))
+ return '%s: %s' % (self.__class__.__name__, text_type(self))
def __str__(self):
return text_type(self.message % self._kwargs)
diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -556,7 +556,7 @@ def print_conda_exception(exception):
stdoutlogger.info(json.dumps(exception.dump_map(), indent=2, sort_keys=True,
cls=EntityEncoder))
else:
- stderrlogger.info("\n\n%r", exception)
+ stderrlogger.info("\n%r", exception)
def print_unexpected_error_message(e):
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -1104,7 +1104,7 @@ def test_remove_spellcheck(self):
run_command(Commands.REMOVE, prefix, 'numpi')
exc_string = '%r' % exc.value
- assert exc_string == "PackageNotFoundError: No packages named 'numpi' found to remove from environment.\n"
+ assert exc_string == "PackageNotFoundError: No packages named 'numpi' found to remove from environment."
assert_package_is_installed(prefix, 'numpy')
| Whitespace in error messages
I started creating an environment then cancelled it. The error messages for the later commands have some extra vertical whitespace. Also, the "CondaValueError" part and "Value Error" parts seem like they shouldn't be included in the terminal output.
```
$conda create -n dot2tex python=2.7
CondaValueError: Value error: prefix already exists: /Users/aaronmeurer/anaconda/envs/dot2tex
$conda remove -n dot2tex --all
Remove all packages in environment /Users/aaronmeurer/anaconda/envs/dot2tex:
$conda create -n dot2tex python=2.7
...
```
Whitespace in error messages
I started creating an environment then cancelled it. The error messages for the later commands have some extra vertical whitespace. Also, the "CondaValueError" part and "Value Error" parts seem like they shouldn't be included in the terminal output.
```
$conda create -n dot2tex python=2.7
CondaValueError: Value error: prefix already exists: /Users/aaronmeurer/anaconda/envs/dot2tex
$conda remove -n dot2tex --all
Remove all packages in environment /Users/aaronmeurer/anaconda/envs/dot2tex:
$conda create -n dot2tex python=2.7
...
```
| Another error message issue (should be printed not as an exception):
```
ncurses-5.9-9. 100% |##############################################################################################################| Time: 0:00:10 94.92 kB/s
An unexpected error has occurred.########################################### | Time: 0:01:14 82.96 kB/s
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : osx-64
conda version : 4.2.7
conda is private : False
conda-env version : 4.2.7
conda-build version : 2.0.0
python version : 3.5.2.final.0
requests version : 2.10.0
root environment : /Users/aaronmeurer/anaconda (writable)
default environment : /Users/aaronmeurer/anaconda
envs directories : /Users/aaronmeurer/anaconda/envs
package cache : /Users/aaronmeurer/anaconda/pkgs
channel URLs : https://conda.anaconda.org/conda-forge/osx-64/
https://conda.anaconda.org/conda-forge/noarch/
https://repo.continuum.io/pkgs/free/osx-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/osx-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://conda.anaconda.org/asmeurer/osx-64/
https://conda.anaconda.org/asmeurer/noarch/
https://conda.anaconda.org/r/osx-64/
https://conda.anaconda.org/r/noarch/
https://conda.anaconda.org/pyne/osx-64/
https://conda.anaconda.org/pyne/noarch/
config file : /Users/aaronmeurer/.condarc
offline mode : False
`$ /Users/aaronmeurer/anaconda3/bin/conda create -n dot2tex python=2.7`
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 228, in _error_catcher
yield
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 310, in read
data = self._fp.read(amt)
File "/Users/aaronmeurer/anaconda/lib/python3.5/http/client.py", line 448, in read
n = self.readinto(b)
File "/Users/aaronmeurer/anaconda/lib/python3.5/http/client.py", line 488, in readinto
n = self.fp.readinto(b)
File "/Users/aaronmeurer/anaconda/lib/python3.5/socket.py", line 575, in readinto
return self._sock.recv_into(b)
File "/Users/aaronmeurer/anaconda/lib/python3.5/ssl.py", line 929, in recv_into
return self.read(nbytes, buffer)
File "/Users/aaronmeurer/anaconda/lib/python3.5/ssl.py", line 791, in read
return self._sslobj.read(len, buffer)
File "/Users/aaronmeurer/anaconda/lib/python3.5/ssl.py", line 575, in read
v = self._sslobj.read(len, buffer)
socket.timeout: The read operation timed out
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/models.py", line 664, in generate
for chunk in self.raw.stream(chunk_size, decode_content=True):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 353, in stream
data = self.read(amt=amt, decode_content=decode_content)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 320, in read
flush_decoder = True
File "/Users/aaronmeurer/anaconda/lib/python3.5/contextlib.py", line 77, in __exit__
self.gen.throw(type, value, traceback)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 233, in _error_catcher
raise ReadTimeoutError(self._pool, None, 'Read timed out.')
requests.packages.urllib3.exceptions.ReadTimeoutError: HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/fetch.py", line 419, in download
for chunk in resp.iter_content(2**14):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/models.py", line 671, in generate
raise ConnectionError(e)
requests.exceptions.ConnectionError: HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/install.py", line 405, in install
execute_actions(actions, index, verbose=not context.quiet)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/plan.py", line 643, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/instructions.py", line 134, in execute_instructions
cmd(state, arg)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/instructions.py", line 47, in FETCH_CMD
fetch_pkg(state['index'][arg + '.tar.bz2'])
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/fetch.py", line 336, in fetch_pkg
download(url, path, session=session, md5=info['md5'], urlstxt=True)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/fetch.py", line 438, in download
raise CondaRuntimeError("Could not open %r for writing (%s)." % (pp, e))
conda.exceptions.CondaRuntimeError: Runtime error: Could not open '/Users/aaronmeurer/anaconda/pkgs/python-2.7.12-1.tar.bz2.part' for writing (HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.).
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/exceptions.py", line 472, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/main_create.py", line 68, in execute
install(args, parser, 'create')
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/install.py", line 422, in install
raise CondaSystemExit('Exiting', e)
File "/Users/aaronmeurer/anaconda/lib/python3.5/contextlib.py", line 77, in __exit__
self.gen.throw(type, value, traceback)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/common.py", line 573, in json_progress_bars
yield
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/install.py", line 420, in install
raise CondaRuntimeError('RuntimeError: %s' % e)
conda.exceptions.CondaRuntimeError: Runtime error: RuntimeError: Runtime error: Could not open '/Users/aaronmeurer/anaconda/pkgs/python-2.7.12-1.tar.bz2.part' for writing (HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.).
```
So was this fixed?
I guess you can't ever *fix* "Read timed out." I've closed over 100 issues today reporting intermittent HTTP errors. There wasn't one report coming from conda 4.3, where we've dramatically improved the messaging for HTTP errors. See https://github.com/conda/conda/blob/4.3.17/conda/core/repodata.py#L168-L306 for example.
The bugs here are:
- Too much whitespace in the error messages
- An exception that was printed with a traceback, instead of just the error message
I agree the error itself isn't a bug in conda.
I'm mostly curious if the extra whitespace was fixed, as I find that quite annoying.
Another error message issue (should be printed not as an exception):
```
ncurses-5.9-9. 100% |##############################################################################################################| Time: 0:00:10 94.92 kB/s
An unexpected error has occurred.########################################### | Time: 0:01:14 82.96 kB/s
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : osx-64
conda version : 4.2.7
conda is private : False
conda-env version : 4.2.7
conda-build version : 2.0.0
python version : 3.5.2.final.0
requests version : 2.10.0
root environment : /Users/aaronmeurer/anaconda (writable)
default environment : /Users/aaronmeurer/anaconda
envs directories : /Users/aaronmeurer/anaconda/envs
package cache : /Users/aaronmeurer/anaconda/pkgs
channel URLs : https://conda.anaconda.org/conda-forge/osx-64/
https://conda.anaconda.org/conda-forge/noarch/
https://repo.continuum.io/pkgs/free/osx-64/
https://repo.continuum.io/pkgs/free/noarch/
https://repo.continuum.io/pkgs/pro/osx-64/
https://repo.continuum.io/pkgs/pro/noarch/
https://conda.anaconda.org/asmeurer/osx-64/
https://conda.anaconda.org/asmeurer/noarch/
https://conda.anaconda.org/r/osx-64/
https://conda.anaconda.org/r/noarch/
https://conda.anaconda.org/pyne/osx-64/
https://conda.anaconda.org/pyne/noarch/
config file : /Users/aaronmeurer/.condarc
offline mode : False
`$ /Users/aaronmeurer/anaconda3/bin/conda create -n dot2tex python=2.7`
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 228, in _error_catcher
yield
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 310, in read
data = self._fp.read(amt)
File "/Users/aaronmeurer/anaconda/lib/python3.5/http/client.py", line 448, in read
n = self.readinto(b)
File "/Users/aaronmeurer/anaconda/lib/python3.5/http/client.py", line 488, in readinto
n = self.fp.readinto(b)
File "/Users/aaronmeurer/anaconda/lib/python3.5/socket.py", line 575, in readinto
return self._sock.recv_into(b)
File "/Users/aaronmeurer/anaconda/lib/python3.5/ssl.py", line 929, in recv_into
return self.read(nbytes, buffer)
File "/Users/aaronmeurer/anaconda/lib/python3.5/ssl.py", line 791, in read
return self._sslobj.read(len, buffer)
File "/Users/aaronmeurer/anaconda/lib/python3.5/ssl.py", line 575, in read
v = self._sslobj.read(len, buffer)
socket.timeout: The read operation timed out
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/models.py", line 664, in generate
for chunk in self.raw.stream(chunk_size, decode_content=True):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 353, in stream
data = self.read(amt=amt, decode_content=decode_content)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 320, in read
flush_decoder = True
File "/Users/aaronmeurer/anaconda/lib/python3.5/contextlib.py", line 77, in __exit__
self.gen.throw(type, value, traceback)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/packages/urllib3/response.py", line 233, in _error_catcher
raise ReadTimeoutError(self._pool, None, 'Read timed out.')
requests.packages.urllib3.exceptions.ReadTimeoutError: HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/fetch.py", line 419, in download
for chunk in resp.iter_content(2**14):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/requests/models.py", line 671, in generate
raise ConnectionError(e)
requests.exceptions.ConnectionError: HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/install.py", line 405, in install
execute_actions(actions, index, verbose=not context.quiet)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/plan.py", line 643, in execute_actions
inst.execute_instructions(plan, index, verbose)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/instructions.py", line 134, in execute_instructions
cmd(state, arg)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/instructions.py", line 47, in FETCH_CMD
fetch_pkg(state['index'][arg + '.tar.bz2'])
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/fetch.py", line 336, in fetch_pkg
download(url, path, session=session, md5=info['md5'], urlstxt=True)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/fetch.py", line 438, in download
raise CondaRuntimeError("Could not open %r for writing (%s)." % (pp, e))
conda.exceptions.CondaRuntimeError: Runtime error: Could not open '/Users/aaronmeurer/anaconda/pkgs/python-2.7.12-1.tar.bz2.part' for writing (HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.).
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/exceptions.py", line 472, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/main.py", line 144, in _main
exit_code = args.func(args, p)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/main_create.py", line 68, in execute
install(args, parser, 'create')
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/install.py", line 422, in install
raise CondaSystemExit('Exiting', e)
File "/Users/aaronmeurer/anaconda/lib/python3.5/contextlib.py", line 77, in __exit__
self.gen.throw(type, value, traceback)
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/common.py", line 573, in json_progress_bars
yield
File "/Users/aaronmeurer/anaconda/lib/python3.5/site-packages/conda/cli/install.py", line 420, in install
raise CondaRuntimeError('RuntimeError: %s' % e)
conda.exceptions.CondaRuntimeError: Runtime error: RuntimeError: Runtime error: Could not open '/Users/aaronmeurer/anaconda/pkgs/python-2.7.12-1.tar.bz2.part' for writing (HTTPSConnectionPool(host='binstar-cio-packages-prod.s3.amazonaws.com', port=443): Read timed out.).
```
So was this fixed?
I guess you can't ever *fix* "Read timed out." I've closed over 100 issues today reporting intermittent HTTP errors. There wasn't one report coming from conda 4.3, where we've dramatically improved the messaging for HTTP errors. See https://github.com/conda/conda/blob/4.3.17/conda/core/repodata.py#L168-L306 for example.
The bugs here are:
- Too much whitespace in the error messages
- An exception that was printed with a traceback, instead of just the error message
I agree the error itself isn't a bug in conda.
I'm mostly curious if the extra whitespace was fixed, as I find that quite annoying. | 2017-05-01T22:15:36 | -1.0 |
conda/conda | 5,221 | conda__conda-5221 | [
"5207"
] | 052a5e7355297db55e49b39957b5f7e4114ce223 | diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -410,14 +410,12 @@ def prioritize_channels(channels, with_credentials=True, subdirs=None):
# number as the value
# ('https://conda.anaconda.org/conda-forge/osx-64/', ('conda-forge', 1))
result = odict()
- q = -1 # channel priority counter
- for chn in channels:
+ for channel_priority, chn in enumerate(channels):
channel = Channel(chn)
for url in channel.urls(with_credentials, subdirs):
if url in result:
continue
- q += 1
- result[url] = channel.canonical_name, min(q, MAX_CHANNEL_PRIORITY - 1)
+ result[url] = channel.canonical_name, min(channel_priority, MAX_CHANNEL_PRIORITY - 1)
return result
| diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -789,11 +789,11 @@ def test_env_var_file_urls(self):
prioritized = prioritize_channels(new_context.channels)
assert prioritized == OrderedDict((
("file://network_share/shared_folder/path/conda/%s" % context.subdir, ("file://network_share/shared_folder/path/conda", 0)),
- ("file://network_share/shared_folder/path/conda/noarch", ("file://network_share/shared_folder/path/conda", 1)),
- ("https://some.url/ch_name/%s" % context.subdir, ("https://some.url/ch_name", 2)),
- ("https://some.url/ch_name/noarch", ("https://some.url/ch_name", 3)),
- ("file:///some/place/on/my/machine/%s" % context.subdir, ("file:///some/place/on/my/machine", 4)),
- ("file:///some/place/on/my/machine/noarch", ("file:///some/place/on/my/machine", 5)),
+ ("file://network_share/shared_folder/path/conda/noarch", ("file://network_share/shared_folder/path/conda", 0)),
+ ("https://some.url/ch_name/%s" % context.subdir, ("https://some.url/ch_name", 1)),
+ ("https://some.url/ch_name/noarch", ("https://some.url/ch_name", 1)),
+ ("file:///some/place/on/my/machine/%s" % context.subdir, ("file:///some/place/on/my/machine", 2)),
+ ("file:///some/place/on/my/machine/noarch", ("file:///some/place/on/my/machine", 2)),
))
def test_subdirs(self):
@@ -816,19 +816,19 @@ def _channel_urls(channels=None):
prioritized = prioritize_channels(channels)
assert prioritized == OrderedDict((
("https://conda.anaconda.org/bioconda/linux-highest", ("bioconda", 0)),
- ("https://conda.anaconda.org/bioconda/linux-64", ("bioconda", 1)),
- ("https://conda.anaconda.org/bioconda/noarch", ("bioconda", 2)),
- ("https://conda.anaconda.org/conda-forge/linux-highest", ("conda-forge", 3)),
- ("https://conda.anaconda.org/conda-forge/linux-64", ("conda-forge", 4)),
- ("https://conda.anaconda.org/conda-forge/noarch", ("conda-forge", 5)),
+ ("https://conda.anaconda.org/bioconda/linux-64", ("bioconda", 0)),
+ ("https://conda.anaconda.org/bioconda/noarch", ("bioconda", 0)),
+ ("https://conda.anaconda.org/conda-forge/linux-highest", ("conda-forge", 1)),
+ ("https://conda.anaconda.org/conda-forge/linux-64", ("conda-forge", 1)),
+ ("https://conda.anaconda.org/conda-forge/noarch", ("conda-forge", 1)),
))
prioritized = prioritize_channels(channels, subdirs=('linux-again', 'noarch'))
assert prioritized == OrderedDict((
("https://conda.anaconda.org/bioconda/linux-again", ("bioconda", 0)),
- ("https://conda.anaconda.org/bioconda/noarch", ("bioconda", 1)),
- ("https://conda.anaconda.org/conda-forge/linux-again", ("conda-forge", 2)),
- ("https://conda.anaconda.org/conda-forge/noarch", ("conda-forge", 3)),
+ ("https://conda.anaconda.org/bioconda/noarch", ("bioconda", 0)),
+ ("https://conda.anaconda.org/conda-forge/linux-again", ("conda-forge", 1)),
+ ("https://conda.anaconda.org/conda-forge/noarch", ("conda-forge", 1)),
))
| Lower version platform pkgs preferred to newer noarch. 4.3.17 regression
With `conda 4.3.17` the solver prefers ~~Windows~~ platform-specific packages over `noarch` even if the `noarch` version has a higher version number. This ~~is not observable on a Linux and also~~ works fine ~~on Windows~~ for `conda 4.3.16`.
See the following:
```batch
(C:\home\maba\conda\miniconda-win64) C:\home\maba\conda\miniconda-win64\maba>conda create --dry-run -n tmp -c genomeinformatics amplikyzer2
Fetching package metadata ...............
Solving package specifications: .
Package plan for installation in environment C:\home\maba\conda\miniconda-win64\envs\tmp:
The following NEW packages will be INSTALLED:
amplikyzer2: 1.2.0-0 genomeinformatics
cycler: 0.10.0-py36_0
icu: 57.1-vc14_0 [vc14]
jpeg: 9b-vc14_0 [vc14]
libpng: 1.6.27-vc14_0 [vc14]
llvmlite: 0.17.0-py36_0
matplotlib: 2.0.0-np112py36_0
mkl: 2017.0.1-0
numba: 0.32.0-np112py36_0
numpy: 1.12.1-py36_0
openssl: 1.0.2k-vc14_0 [vc14]
pip: 9.0.1-py36_1
pyparsing: 2.1.4-py36_0
pyqt: 5.6.0-py36_2
python: 3.6.1-0
python-dateutil: 2.6.0-py36_0
pytz: 2017.2-py36_0
qt: 5.6.2-vc14_3 [vc14]
setuptools: 27.2.0-py36_1
sip: 4.18-py36_0
six: 1.10.0-py36_0
tk: 8.5.18-vc14_0 [vc14]
vs2015_runtime: 14.0.25123-0
wheel: 0.29.0-py36_0
zlib: 1.2.8-vc14_3 [vc14]
(C:\home\maba\conda\miniconda-win64) C:\home\maba\conda\miniconda-win64\maba>conda --version
conda 4.3.16
```
`amplikyzer2 1.2.0` is the most recent version and uses `noarch: python`.
After updating to `conda 4.3.17`, an outdated version is selected:
```batch
(C:\home\maba\conda\miniconda-win64) C:\home\maba\conda\miniconda-win64\maba>conda update conda
Fetching package metadata ...............
Solving package specifications: .
Package plan for installation in environment C:\home\maba\conda\miniconda-win64:
The following packages will be UPDATED:
conda: 4.3.16-py35_0 --> 4.3.17-py35_0
Proceed ([y]/n)?
conda-4.3.17-p 100% |###############################| Time: 0:00:00 2.42 MB/s
(C:\home\maba\conda\miniconda-win64) C:\home\maba\conda\miniconda-win64\maba>conda create --dry-run -n tmp -c genomeinformatics amplikyzer2
Fetching package metadata ...............
Solving package specifications: .
Package plan for installation in environment C:\home\maba\conda\miniconda-win64\envs\tmp:
The following NEW packages will be INSTALLED:
amplikyzer2: 1.1.2-0 genomeinformatics
cycler: 0.10.0-py36_0
icu: 57.1-vc14_0 [vc14]
jpeg: 9b-vc14_0 [vc14]
libpng: 1.6.27-vc14_0 [vc14]
llvmlite: 0.17.0-py36_0
matplotlib: 2.0.0-np112py36_0
mkl: 2017.0.1-0
numba: 0.32.0-np112py36_0
numpy: 1.12.1-py36_0
openssl: 1.0.2k-vc14_0 [vc14]
pip: 9.0.1-py36_1
pyparsing: 2.1.4-py36_0
pyqt: 5.6.0-py36_2
python: 3.6.1-0
python-dateutil: 2.6.0-py36_0
pytz: 2017.2-py36_0
qt: 5.6.2-vc14_3 [vc14]
setuptools: 27.2.0-py36_1
sip: 4.18-py36_0
six: 1.10.0-py36_0
tk: 8.5.18-vc14_0 [vc14]
vs2015_runtime: 14.0.25123-0
wheel: 0.29.0-py36_0
zlib: 1.2.8-vc14_3 [vc14]
```
`amplikyzer2 1.1.2-0` is the last platform-specific Windows build, but of course is superseded by `1.2.0`.
The same also happens for `conda install amplikyzer2` and `conda update amplikyzer2`. If the version is explicitly given, e.g., `conda install amplikyzer2=1.2.0`, the newest version is correctly installed. Running `conda update amplikyzer2` afterwards will try to downgrade to `1.1.2-0` again.
| Scratch that about being Windows-specific -- I accidentally didn't really update to `conda 4.3.17` on Linux.
Now with properly updated to `4.3.17` the same behavior is shown on Linux too.
I see #5030 introduced this. As I understand this, after #5030 the platform-specific and `noarch` directory in effect get treated as separate channels with `noarch` always having a lower priority.
Thus adding `--no-channel-priority` shows the expected result:
```bash
$ conda --version
conda 4.3.17
```
```bash
$ conda create --dry-run -n tmp -c genomeinformatics amplikyzer2
Fetching package metadata ...........
Solving package specifications: .
Package plan for installation in environment /home/maba/miniconda3/envs/tmp:
The following NEW packages will be INSTALLED:
amplikyzer2: 1.1.5-py36_8 genomeinformatics
...
```
```bash
$ conda create --dry-run -n tmp -c genomeinformatics amplikyzer2 --no-channel-priority
Fetching package metadata ...........
Solving package specifications: .
Package plan for installation in environment /home/maba/miniconda3/envs/tmp:
The following NEW packages will be INSTALLED:
amplikyzer2: 1.2.0-0 genomeinformatics
...
```
I strongly feel this is undesirable behavior and that *inside a single channel*, the package version must have the highest priority. Only on a version number tie should the platform-dependent build be preferred. | 2017-05-04T15:53:58 | -1.0 |
conda/conda | 5,231 | conda__conda-5231 | [
"3489"
] | 194d2ee2251dc07a7d4b71c5ea26f6a0e9215dc0 | diff --git a/conda/cli/main_remove.py b/conda/cli/main_remove.py
--- a/conda/cli/main_remove.py
+++ b/conda/cli/main_remove.py
@@ -9,7 +9,7 @@
from argparse import RawDescriptionHelpFormatter
from collections import defaultdict
import logging
-from os.path import abspath, join
+from os.path import abspath, join, isdir
import sys
from .conda_argparse import (add_parser_channels, add_parser_help, add_parser_json,
@@ -130,6 +130,10 @@ def execute(args, parser):
if args.all and prefix == context.default_prefix:
msg = "cannot remove current environment. deactivate and run conda remove again"
raise CondaEnvironmentError(msg)
+ if args.all and not isdir(prefix):
+ # full environment removal was requested, but environment doesn't exist anyway
+ return 0
+
ensure_use_local(args)
ensure_override_channels_requires_channel(args)
if not args.features and args.all:
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -1126,6 +1126,9 @@ def test_force_remove(self):
stdout, stderr = run_command(Commands.REMOVE, prefix, "flask")
assert not package_is_installed(prefix, "flask-")
+ # regression test for #3489
+ # don't raise for remove --all if environment doesn't exist
+ run_command(Commands.REMOVE, prefix, "--all")
def test_transactional_rollback_simple(self):
from conda.core.path_actions import CreateLinkedPackageRecordAction
| conda remove --all behavior changed in 4.2.7
`conda remove --all` used to remove an environment if it existed, and quietly succeed if it was already gone. Now this command fails when the environment does not exist. This makes something formerly easy to do in an automated way now difficult especially in a cross-platform way on Windows.
```
C:\>conda remove --all -n nonenv
CondaEnvironmentNotFoundError: Could not find environment: nonenv .
You can list all discoverable environments with `conda info --envs`.
C:\>echo %ERRORLEVEL%
1
```
Just suppressing the error is not appealing, because there are many error modes different from the environment not existing that should trigger a failure.
| Still exists in conda 4.3.x
```
kfranz@0283:~/continuum/conda *4.3.x ❯ python -m conda remove --all -n flub
CondaEnvironmentNotFoundError: Could not find environment: flub .
You can list all discoverable environments with `conda info --envs`.
```
I hate that we didn't get to this sooner. At this point, I don't know if we should change it in the 4.3.x branch. I'm happy to revert the behavior in 4.4 though. | 2017-05-05T01:05:19 | -1.0 |
conda/conda | 5,265 | conda__conda-5265 | [
"1543"
] | 94b1f760c82d178fa884f216b8aeaaf65714aa6d | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -155,7 +155,9 @@ class Context(Configuration):
dry_run = PrimitiveParameter(False)
force = PrimitiveParameter(False)
json = PrimitiveParameter(False)
+ no_dependencies = PrimitiveParameter(False, aliases=('no_deps',))
offline = PrimitiveParameter(False)
+ only_dependencies = PrimitiveParameter(False, aliases=('only_deps',))
quiet = PrimitiveParameter(False)
prune = PrimitiveParameter(False)
respect_pinned = PrimitiveParameter(True)
@@ -473,6 +475,8 @@ def list_parameters(self):
'force_32bit',
'max_shlvl',
'migrated_custom_channels',
+ 'no_dependencies',
+ 'only_dependencies',
'prune',
'respect_pinned',
'root_prefix',
diff --git a/conda/cli/conda_argparse.py b/conda/cli/conda_argparse.py
--- a/conda/cli/conda_argparse.py
+++ b/conda/cli/conda_argparse.py
@@ -125,6 +125,11 @@ def add_parser_create_install_update(p):
action="store_true",
help="Do not install dependencies.",
)
+ p.add_argument(
+ "--only-deps",
+ action="store_true",
+ help="Only install dependencies.",
+ )
p.add_argument(
'-m', "--mkdir",
action="store_true",
diff --git a/conda/core/solve.py b/conda/core/solve.py
--- a/conda/core/solve.py
+++ b/conda/core/solve.py
@@ -129,9 +129,43 @@ def solve_for_actions(prefix, r, specs_to_remove=(), specs_to_add=(), prune=Fals
# this is not for force-removing packages, which doesn't invoke the solver
solved_dists, _specs_to_add = solve_prefix(prefix, r, specs_to_remove, specs_to_add, prune)
+ # TODO: this _specs_to_add part should be refactored when we can better pin package channel origin # NOQA
dists_for_unlinking, dists_for_linking = sort_unlink_link_from_solve(prefix, solved_dists,
_specs_to_add)
- # TODO: this _specs_to_add part should be refactored when we can better pin package channel origin # NOQA
+
+ def remove_non_matching_dists(dists_set, specs_to_match):
+ _dists_set = IndexedSet(dists_set)
+ for dist in dists_set:
+ for spec in specs_to_match:
+ if spec.match(dist):
+ break
+ else: # executed if the loop ended normally (no break)
+ _dists_set.remove(dist)
+ return _dists_set
+
+ if context.no_dependencies:
+ # for `conda create --no-deps python=3 flask`, do we install python? yes
+ # the only dists we touch are the ones that match a specs_to_add
+ dists_for_linking = remove_non_matching_dists(dists_for_linking, specs_to_add)
+ dists_for_unlinking = remove_non_matching_dists(dists_for_unlinking, specs_to_add)
+ elif context.only_dependencies:
+ # for `conda create --only-deps python=3 flask`, do we install python? yes
+ # remove all dists that match a specs_to_add, as long as that dist isn't a dependency
+ # of other specs_to_add
+ _index = r.index
+ _match_any = lambda spec, dists: next((dist for dist in dists if spec.match(_index[dist])),
+ None)
+ _is_dependency = lambda spec, dist: any(r.depends_on(s, dist.name)
+ for s in specs_to_add if s != spec)
+ for spec in specs_to_add:
+ link_matching_dist = _match_any(spec, dists_for_linking)
+ if link_matching_dist:
+ if not _is_dependency(spec, link_matching_dist):
+ # as long as that dist isn't a dependency of other specs_to_add
+ dists_for_linking.remove(link_matching_dist)
+ unlink_matching_dist = _match_any(spec, dists_for_unlinking)
+ if unlink_matching_dist:
+ dists_for_unlinking.remove(unlink_matching_dist)
if context.force:
dists_for_unlinking, dists_for_linking = forced_reinstall_specs(prefix, solved_dists,
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -531,6 +531,21 @@ def test_install_prune(self):
assert package_is_installed(prefix, 'pytz')
assert package_is_installed(prefix, 'python-3')
+ def test_no_deps_flag(self):
+ with make_temp_env("python=2 flask --no-deps") as prefix:
+ assert package_is_installed(prefix, 'flask')
+ assert package_is_installed(prefix, 'python-2')
+ assert not package_is_installed(prefix, 'openssl')
+ assert not package_is_installed(prefix, 'itsdangerous')
+
+ def test_only_deps_flag(self):
+ with make_temp_env("python=2 flask --only-deps") as prefix:
+ assert not package_is_installed(prefix, 'flask')
+ assert package_is_installed(prefix, 'python')
+ if not on_win:
+ # python on windows doesn't actually have real dependencies
+ assert package_is_installed(prefix, 'openssl')
+ assert package_is_installed(prefix, 'itsdangerous')
@pytest.mark.skipif(on_win, reason="mkl package not available on Windows")
def test_install_features(self):
| --dependencies-only flag for development
It'd be nice to have a `conda install -dependencies-only` flag. This would install all of the dependencies of the required package, but not the package itself. This would be useful when developing a package - it would allow you to install all of the required packages in one fell swoop.
| I agree. It would be basically the opposite of `--no-deps`.
Even so, it's pretty easy to achieve the same thing with `conda install package; conda remove package`.
easy but feels like hacky....
+1 - this is a common scenario when wanting to automate unit tests prior to creating a package. Don't want to go through the whole conda build unnecessarily (and could fail, but I want my unit tests to fail).
@benbovy Is there progress on this? It seems that #3500 works already but no decision has been made whether this feature will become part of conda.
I would like to use it to setup the development environment easily and for testing as well.
I'm still waiting for some feedback or review on this.
It does work, although currently a (major) limitation is that we can't set specific versions for the dependencies, which is I actually need to easily create multiple dev/test envs for a given package.
> It does work, although currently a (major) limitation is that we can't set specific versions for the dependencies, which is I actually need to easily create multiple dev/test envs for a given package.
maybe then you need to change the option to be
```
--add-dependencies-from-package [packagename]
```
which will circumvent the whole installation process and only add the requirements to the install string. This way you can just add more constraints and be more flexible in building envs. Say your package `foo` requires `xyz>=1.0` and you want to test with later versions you run
```
conda install --add-dependencies-from-package foo xyz=1.1
```
which should act something like
```
conda install xyz>=1.0 xyz=1.1
```
(not that this syntax works but that is what I think would be nice)
This would also be useful if you want to update all dependencies when developing.
In my case -- just setting up an environment for testing -- it would be nice to have some option in conda-build instead that creates a suitable environment to execute, test or develop in. Conda-build used to not clean up its testing enviroment `_test` which I used to run nose tests a while ago. Having only this step as a single option with a name of the environment would be useful.
I imagine the case where the test branch needs additional packages then installing from an existing build is not enough. Probably the origin why it _feels_ hacky.
```
conda build --only-create-test-environment mytest path-to-recipe
```
I think the use case might fit better with conda-build's already-built capabilities too. @benbovy if we implemented something like what @jhprinz suggests in conda-build, would that cover your use case?
CC @msarahan
@kalefranz although I rarely use conda-build and I've not used it so far for setting up development environments, to me it feels a bit hacky to use a "tool for _building_ conda packages" to actually just _install_ packages in a new development environment. In my case -- create and submit PRs -- I don't need a recipe to build a conda package. Using `conda install package; conda remove package` would be easier than downloading or writing a recipe.
I actually like @jhprinz's suggestion of having a `--add-dependencies-from-package PACKAGE` option (maybe with a more succinct name like `--deps-from PACKAGE` ?). I can update #3500 to implement that option if needed.
If meta.yaml is involved, then conda build is the right way. If only run
time deps are involved, I agree with Benoit.
All this said, I do wonder if the conda develop command (part of conda
build) is what is needed here.
On Oct 24, 2016 6:32 AM, "Benoit Bovy" notifications@github.com wrote:
> @kalefranz https://github.com/kalefranz although I rarely use
> conda-build and I've not used it so far for setting up development
> environments, to me it feels a bit hacky to use a "tool for _building_
> conda packages" to actually just _install_ packages in a new development
> environment. In my case -- create and submit PRs -- I don't need a recipe
> to build a conda package. Using conda install package; conda remove
> package would be easier than downloading or writing a recipe.
>
> I actually like @jhprinz https://github.com/jhprinz's suggestion of
> having a --add-dependencies-from-package PACKAGE option (maybe with a
> more succinct name like --deps-from PACKAGE ?). I can update #3500
> https://github.com/conda/conda/pull/3500 to implement that option if
> needed.
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub
> https://github.com/conda/conda/issues/1543#issuecomment-255716085, or mute
> the thread
> https://github.com/notifications/unsubscribe-auth/AACV-axaiBKR0sXX_kWMYA8fqn1I7F1jks5q3JdkgaJpZM4Fvz9C
> .
@msarahan Yes, the following would be nice in my case:
```
$ conda create -n devenv --deps-from package
$ conda develop -n devenv ./package_repo
```
I guess what I meant was it would be neat if conda develop could (optionally) create new envs for you - and that the way to get development environments would be to use conda-build's conda develop command, and cut the conda create step entirely. I don't think that this is the way it is right now, but it seems like the right way to do things eventually.
```
conda develop -n devenv ./package_repo
```
would create the environment if it didn't already exist - otherwise, it would ensure that the dependencies were up to date, potentially adding new ones or even removing ones removed from the package. The removal is of course contentious, but might be the best way to ensure that you have a good, known-working recipe, since previously installed packages would allow things to run, even if they were no longer listed in the recipe.
Opinions?
Indeed, it would be nice to run conda develop only. However, I don't kown how we can get from `./package_repo` all the information needed to setup the environment (i.e., dependencies, channels) when no meta.yml is involved. A possible solution is to set it explicitly (as well as additional constraints on the versions of the packages to install) as new options of conda develop, but then it would probably end up adding a lot of conda create/install features to conda develop. Maybe I miss easier solutions (as my knowledge of conda and conda-build logic is quite limited)?
> @msarahan it would be neat if conda develop could (optionally) create new envs for you
I think I expected `conda develop` to work in the way you want it to: Install and/or update packages so that you can _develop_ on your package. If that is not the case it would definitely be a good option.
I will not cover my two usecases though:
1. I usually have a github repo I am working on that is also build into a conda package. What is (kind of) annoying is that once in a while a test on travis fails because some packages were updated (without me noticing) and I need to update my conda environment again.
So for this I would like some `conda build` option to update the current environment to the latest packages matching the specifications in `meta.yml`. I think it would be useful if `conda build` would check this when building or even disallow building when your current packages do not represent what would be installed from just using `meta.yml`. All of this should work in the current environment and I assume `conda build` would be the right place to put this functionality
2. I actually need a clean environment to run tests (on travis) that has all the dependencies and at best also all the dependencies in the `test` section of `meta.yml`. I realize that the cleaner way would be to run all the tests when building and put these into the `test` section but for travis CI we decided to run these separately after the build is done. Then use the freshly build package, install it and run the tests. For these we need to recreate the test environment a second time. I assume this would be very easy to implement since conda build can already do that.
The earlier proposed `--deps-from PACKAGE` would still be nice and make sense for `conda install` or `conda update` to provide similar functionality, but it is more a convenience for cases where you know a packages dependencies are complete in providing a certain range of packages. When developing this can be helpful but it is not really what you want. It still requires a package to already exists that needs the packages you want.
If I understand correctly then these cases are a little different from what @benbovy needs ?!
I guess that the main difference from @jhprinz's usecases is that the packages I'd like to contribute are available as conda packages but they don't include any conda recipe in their github repo (the recipes are maintained either in the Continuum's anaconda-recipes repo or as separate conda-forge feedstocks).
Moreover, when I'm working on a pull request I often prefer to run unit tests locally on multiple environments (e.g., py27, py35) before committing and pushing my commits, even if these tests will be run again automatically with CI. This way I avoid pushing too many commits like "fix tests with py27"...
It would indeed be neat if we could use `conda develop` to automatically create all these environments (at least one command for one environment), though it doesn't seem straightforward as the information needed to setup these environments is usually written in `meta.yml` (which is not directly available here) and/or in files like `.travis.yml`. A solution would be to explicitly provide that information as `conda develop` options, but IMO it feels a bit like copy/pasting the features of `conda create/install` to `conda develop`.
In the case explained here, I don't mind using the two commands `conda create; conda develop` to setup one environment, instead of just `conda develop`. The `--deps-from PACKAGE` option would avoid writing `conda create; conda remove; conda develop`.
It would also be useful if the command would work when multiple packages are specified.
That is, if `packageA` requires `packageB` and `packageC` and the line
`conda install --dependencies-only packageA packageB` is run, then only `packageC` would be installed.
This behavior would be useful in a CI environment where the tests are run on heads of `packageA` and `packageB`, and not the released package. | 2017-05-09T17:59:40 | -1.0 |
conda/conda | 5,310 | conda__conda-5310 | [
"4302"
] | 19c3c149801bbc4a9a0b834306e21cab6f7c81f0 | diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -383,18 +383,31 @@ def execute_config(args, parser):
json_warnings.append(message)
arglist.insert(0 if prepend else len(arglist), item)
+ primitive_parameters = frozenset(
+ p for p in context.list_parameters()
+ if context.describe_parameter(p)['parameter_type'] == 'primitive'
+ )
+ map_parameters = frozenset(
+ p for p in context.list_parameters()
+ if context.describe_parameter(p)['parameter_type'] == 'map'
+ )
+
# Set
for key, item in args.set:
- primitive_parameters = [p for p in context.list_parameters()
- if context.describe_parameter(p)['parameter_type'] == 'primitive']
- if key not in primitive_parameters:
+ key, subkey = key.split('.', 1) if '.' in key else (key, None)
+ if key in primitive_parameters:
+ value = context.typify_parameter(key, item)
+ rc_config[key] = value
+ elif key in map_parameters:
+ argmap = rc_config.setdefault(key, {})
+ argmap[subkey] = item
+ else:
from ..exceptions import CondaValueError
raise CondaValueError("Key '%s' is not a known primitive parameter." % key)
- value = context.typify_parameter(key, item)
- rc_config[key] = value
# Remove
for key, item in args.remove:
+ key, subkey = key.split('.', 1) if '.' in key else (key, None)
if key not in rc_config:
if key != 'channels':
from ..exceptions import CondaKeyError
@@ -408,6 +421,7 @@ def execute_config(args, parser):
# Remove Key
for key, in args.remove_key:
+ key, subkey = key.split('.', 1) if '.' in key else (key, None)
if key not in rc_config:
from ..exceptions import CondaKeyError
raise CondaKeyError(key, "key %r is not in the config file" %
| diff --git a/tests/test_config.py b/tests/test_config.py
--- a/tests/test_config.py
+++ b/tests/test_config.py
@@ -660,12 +660,31 @@ def test_config_set():
assert stdout == ''
assert stderr == ''
+ with open(rc) as fh:
+ content = yaml_load(fh.read())
+ assert content['always_yes'] is True
+
stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
'--set', 'always_yes', 'no')
assert stdout == ''
assert stderr == ''
+ with open(rc) as fh:
+ content = yaml_load(fh.read())
+ assert content['always_yes'] is False
+
+ stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
+ '--set', 'proxy_servers.http', '1.2.3.4:5678')
+
+ assert stdout == ''
+ assert stderr == ''
+
+ with open(rc) as fh:
+ content = yaml_load(fh.read())
+ assert content['always_yes'] is False
+ assert content['proxy_servers'] == {'http': '1.2.3.4:5678'}
+
def test_set_rc_string():
# Test setting string keys in .condarc
| Unable to set conda-build.root-dir with config
Not sure the right way to do this, but it looks like there might not be any right way ATM. I would like to be able to use `conda config` to get the following added to my `.condarc` file.
```yaml
conda-build:
root-dir: /tmp
```
If I try to set `conda-build.root-dir` with `conda config`, I get this error.
```bash
$ conda config --set "conda-build.root-dir" "/tmp"
CondaValueError: Value error: Error key must be one of add_anaconda_token, shortcuts, add_pip_as_python_dependency, ssl_verify, client_tls_cert, binstar_upload, use_pip, add_binstar_token, always_copy, always_yes, client_tls_cert_key, channel_alias, changeps1, channel_priority, show_channel_urls, update_dependencies, allow_softlinks, anaconda_upload, offline, allow_other_channels, auto_update_conda, not conda-build.root-dir
```
| cc @msarahan
`conda config --set` doesn't currently support map-type parameters.
It should be possible to do
`conda config --set proxy_servers.http 1.2.3.4:4004`
and
`conda config --get proxy_servers.http` | 2017-05-13T00:18:04 | -1.0 |
conda/conda | 5,312 | conda__conda-5312 | [
"5256",
"4302"
] | d0399db929580685ed0790f64d55b60d4b10ed64 | diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -21,8 +21,7 @@
from ..common.configuration import pretty_list, pretty_map
from ..common.constants import NULL
from ..common.yaml import yaml_dump, yaml_load
-from ..config import (rc_bool_keys, rc_list_keys, rc_other, rc_string_keys, sys_rc_path,
- user_rc_path)
+from ..config import rc_other, sys_rc_path, user_rc_path
descr = """
Modify configuration values in .condarc. This is modeled after the git
@@ -228,6 +227,10 @@ def format_dict(d):
def execute_config(args, parser):
+ try:
+ from cytoolz.itertoolz import groupby
+ except ImportError: # pragma: no cover
+ from .._vendor.toolz.itertoolz import groupby # NOQA
from .._vendor.auxlib.entity import EntityEncoder
json_warnings = []
json_get = {}
@@ -317,13 +320,19 @@ def execute_config(args, parser):
else:
rc_config = {}
+ grouped_paramaters = groupby(lambda p: context.describe_parameter(p)['parameter_type'],
+ context.list_parameters())
+ primitive_parameters = grouped_paramaters['primitive']
+ sequence_parameters = grouped_paramaters['sequence']
+ map_parameters = grouped_paramaters['map']
+
# Get
if args.get is not None:
context.validate_all()
if args.get == []:
args.get = sorted(rc_config.keys())
for key in args.get:
- if key not in rc_list_keys + rc_bool_keys + rc_string_keys:
+ if key not in primitive_parameters + sequence_parameters:
if key not in rc_other:
message = "unknown key %s" % key
if not context.json:
@@ -356,8 +365,6 @@ def execute_config(args, parser):
# prepend, append, add
for arg, prepend in zip((args.prepend, args.append), (True, False)):
- sequence_parameters = [p for p in context.list_parameters()
- if context.describe_parameter(p)['parameter_type'] == 'sequence']
for key, item in arg:
if key == 'channels' and key not in rc_config:
rc_config[key] = ['defaults']
@@ -385,16 +392,20 @@ def execute_config(args, parser):
# Set
for key, item in args.set:
- primitive_parameters = [p for p in context.list_parameters()
- if context.describe_parameter(p)['parameter_type'] == 'primitive']
- if key not in primitive_parameters:
+ key, subkey = key.split('.', 1) if '.' in key else (key, None)
+ if key in primitive_parameters:
+ value = context.typify_parameter(key, item)
+ rc_config[key] = value
+ elif key in map_parameters:
+ argmap = rc_config.setdefault(key, {})
+ argmap[subkey] = item
+ else:
from ..exceptions import CondaValueError
raise CondaValueError("Key '%s' is not a known primitive parameter." % key)
- value = context.typify_parameter(key, item)
- rc_config[key] = value
# Remove
for key, item in args.remove:
+ key, subkey = key.split('.', 1) if '.' in key else (key, None)
if key not in rc_config:
if key != 'channels':
from ..exceptions import CondaKeyError
@@ -408,6 +419,7 @@ def execute_config(args, parser):
# Remove Key
for key, in args.remove_key:
+ key, subkey = key.split('.', 1) if '.' in key else (key, None)
if key not in rc_config:
from ..exceptions import CondaKeyError
raise CondaKeyError(key, "key %r is not in the config file" %
| diff --git a/tests/test_config.py b/tests/test_config.py
--- a/tests/test_config.py
+++ b/tests/test_config.py
@@ -656,15 +656,38 @@ def test_config_set():
with make_temp_condarc() as rc:
stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
'--set', 'always_yes', 'yes')
-
assert stdout == ''
assert stderr == ''
+ with open(rc) as fh:
+ content = yaml_load(fh.read())
+ assert content['always_yes'] is True
stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
'--set', 'always_yes', 'no')
+ assert stdout == ''
+ assert stderr == ''
+ with open(rc) as fh:
+ content = yaml_load(fh.read())
+ assert content['always_yes'] is False
+ stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
+ '--set', 'proxy_servers.http', '1.2.3.4:5678')
assert stdout == ''
assert stderr == ''
+ with open(rc) as fh:
+ content = yaml_load(fh.read())
+ assert content['always_yes'] is False
+ assert content['proxy_servers'] == {'http': '1.2.3.4:5678'}
+
+ stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
+ '--set', 'ssl_verify', 'false')
+ assert stdout == ''
+ assert stderr == ''
+
+ stdout, stderr, return_code = run_command(Commands.CONFIG, '--file', rc,
+ '--get', 'ssl_verify')
+ assert stdout.strip() == '--set ssl_verify False'
+ assert stderr == ''
def test_set_rc_string():
| Enable ssl_verify with config --get
`config get ssl_verify`
Gives
```
usage: conda config [-h] [--json] [--debug] [--verbose]
[--system | --env | --file FILE]
(--show | --show-sources | --validate | --describe | --get [KEY [KEY ...]] | --append KEY VALUE | --prepend KEY VALUE | --set KEY VALUE | --remove KEY VALUE | --remove-key KEY)
conda config: error: argument --get: invalid choice: 'ssl_verify' (choose from 'channels', 'disallow', 'create_default_packages', 'track_features', 'envs_dirs', 'pkgs_dirs', 'default_channels', 'pinned_packages', 'add_binstar_token', 'add_anaconda_token', 'add_pip_as_python_dependency', 'always_yes', 'always_copy', 'allow_softlinks', 'always_softlink', 'auto_update_conda', 'changeps1', 'use_pip', 'offline', 'binstar_upload', 'anaconda_upload', 'show_channel_urls', 'allow_other_channels', 'update_dependencies', 'channel_priority', 'shortcuts')
```
It should be possible to set it, it is a valid config parameter
Unable to set conda-build.root-dir with config
Not sure the right way to do this, but it looks like there might not be any right way ATM. I would like to be able to use `conda config` to get the following added to my `.condarc` file.
```yaml
conda-build:
root-dir: /tmp
```
If I try to set `conda-build.root-dir` with `conda config`, I get this error.
```bash
$ conda config --set "conda-build.root-dir" "/tmp"
CondaValueError: Value error: Error key must be one of add_anaconda_token, shortcuts, add_pip_as_python_dependency, ssl_verify, client_tls_cert, binstar_upload, use_pip, add_binstar_token, always_copy, always_yes, client_tls_cert_key, channel_alias, changeps1, channel_priority, show_channel_urls, update_dependencies, allow_softlinks, anaconda_upload, offline, allow_other_channels, auto_update_conda, not conda-build.root-dir
```
|
cc @msarahan
`conda config --set` doesn't currently support map-type parameters.
It should be possible to do
`conda config --set proxy_servers.http 1.2.3.4:4004`
and
`conda config --get proxy_servers.http` | 2017-05-13T01:26:17 | -1.0 |
conda/conda | 5,342 | conda__conda-5342 | [
"5341"
] | 79f86d4cf31e6275cf3b201e76b43a95ecbb9888 | diff --git a/conda/cli/main.py b/conda/cli/main.py
--- a/conda/cli/main.py
+++ b/conda/cli/main.py
@@ -80,12 +80,24 @@ def generate_parser():
return p, sub_parsers
+def init_loggers(context):
+ from ..gateways.logging import set_all_logger_level, set_verbosity
+ if not context.json:
+ # Silence logging info to avoid interfering with JSON output
+ for logger in ('print', 'dotupdate', 'stdoutlog', 'stderrlog'):
+ getLogger(logger).setLevel(CRITICAL + 1)
+
+ if context.debug:
+ set_all_logger_level(DEBUG)
+ elif context.verbosity:
+ set_verbosity(context.verbosity)
+ log.debug("verbosity set to %s", context.verbosity)
+
+
def _main(*args):
from ..base.constants import SEARCH_PATH
from ..base.context import context
- from ..gateways.logging import set_all_logger_level, set_verbosity
-
if len(args) == 1:
args = args + ('-h',)
@@ -119,17 +131,7 @@ def completer(prefix, **kwargs):
args = p.parse_args(args)
context.__init__(SEARCH_PATH, 'conda', args)
-
- if getattr(args, 'json', False):
- # Silence logging info to avoid interfering with JSON output
- for logger in ('print', 'dotupdate', 'stdoutlog', 'stderrlog'):
- getLogger(logger).setLevel(CRITICAL + 1)
-
- if context.debug:
- set_all_logger_level(DEBUG)
- elif context.verbosity:
- set_verbosity(context.verbosity)
- log.debug("verbosity set to %s", context.verbosity)
+ init_loggers(context)
exit_code = args.func(args, p)
if isinstance(exit_code, int):
diff --git a/conda_env/cli/main.py b/conda_env/cli/main.py
--- a/conda_env/cli/main.py
+++ b/conda_env/cli/main.py
@@ -1,10 +1,13 @@
-from __future__ import print_function, division, absolute_import
-
-from logging import getLogger, CRITICAL
+from __future__ import absolute_import, division, print_function
import os
import sys
+from conda.base.constants import SEARCH_PATH
+from conda.base.context import context
+from conda.cli.conda_argparse import ArgumentParser
+from conda.cli.main import init_loggers
+
try:
from conda.exceptions import conda_exception_handler
except ImportError as e:
@@ -30,8 +33,6 @@
else:
raise e
-from conda.cli.conda_argparse import ArgumentParser
-
from . import main_attach
from . import main_create
from . import main_export
@@ -39,7 +40,7 @@
from . import main_remove
from . import main_upload
from . import main_update
-from conda.base.context import context
+
# TODO: This belongs in a helper library somewhere
# Note: This only works with `conda-env` as a sub-command. If this gets
@@ -68,15 +69,8 @@ def create_parser():
def main():
parser = create_parser()
args = parser.parse_args()
- context._set_argparse_args(args)
- if getattr(args, 'json', False):
- # # Silence logging info to avoid interfering with JSON output
- # for logger in Logger.manager.loggerDict:
- # if logger not in ('fetch', 'progress'):
- # getLogger(logger).setLevel(CRITICAL + 1)
- for logger in ('print', 'dotupdate', 'stdoutlog', 'stderrlog'):
- getLogger(logger).setLevel(CRITICAL + 1)
-
+ context.__init__(SEARCH_PATH, 'conda', args)
+ init_loggers(context)
return conda_exception_handler(args.func, args, parser)
diff --git a/conda_env/cli/main_export.py b/conda_env/cli/main_export.py
--- a/conda_env/cli/main_export.py
+++ b/conda_env/cli/main_export.py
@@ -4,7 +4,7 @@
import os
import textwrap
-from conda.cli.common import add_parser_prefix
+from conda.cli.common import add_parser_json, add_parser_prefix
# conda env import
from .common import get_prefix
from ..env import from_environment
@@ -63,7 +63,7 @@ def configure_parser(sub_parsers):
action='store_true',
required=False,
help='Do not include channel names with package names.')
-
+ add_parser_json(p)
p.set_defaults(func=execute)
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -65,6 +65,18 @@
except ImportError:
from mock import patch
+
+def get_win_locations():
+ try:
+ from menuinst.win32 import dirs_src as win_locations
+ except ImportError:
+ try:
+ from menuinst.win32 import dirs as win_locations
+ except ImportError:
+ win_locations = {}
+ return win_locations
+
+
log = getLogger(__name__)
TRACE, DEBUG = TRACE, DEBUG # these are so the imports aren't cleared, but it's easy to switch back and forth
TEST_LOG_LEVEL = DEBUG
@@ -623,7 +635,7 @@ def test_update_deps_flag_present(self):
@pytest.mark.skipif(not on_win, reason="shortcuts only relevant on Windows")
def test_shortcut_not_attempted_with_no_shortcuts_arg(self):
prefix = make_temp_prefix("_" + str(uuid4())[:7])
- from menuinst.win32 import dirs as win_locations
+ win_locations = get_win_locations()
user_mode = 'user' if exists(join(sys.prefix, u'.nonadmin')) else 'system'
shortcut_dir = win_locations[user_mode]["start"]
shortcut_file = join(shortcut_dir, "Anaconda Prompt ({0}).lnk".format(basename(prefix)))
@@ -636,7 +648,7 @@ def test_shortcut_not_attempted_with_no_shortcuts_arg(self):
@pytest.mark.skipif(not on_win, reason="shortcuts only relevant on Windows")
def test_shortcut_creation_installs_shortcut(self):
- from menuinst.win32 import dirs as win_locations
+ win_locations = get_win_locations()
user_mode = 'user' if exists(join(sys.prefix, u'.nonadmin')) else 'system'
shortcut_dir = win_locations[user_mode]["start"]
shortcut_dir = join(shortcut_dir, "Anaconda{0} ({1}-bit)"
@@ -662,7 +674,7 @@ def test_shortcut_creation_installs_shortcut(self):
@pytest.mark.skipif(not on_win, reason="shortcuts only relevant on Windows")
def test_shortcut_absent_does_not_barf_on_uninstall(self):
- from menuinst.win32 import dirs as win_locations
+ win_locations = get_win_locations()
user_mode = 'user' if exists(join(sys.prefix, u'.nonadmin')) else 'system'
shortcut_dir = win_locations[user_mode]["start"]
@@ -690,7 +702,7 @@ def test_shortcut_absent_does_not_barf_on_uninstall(self):
@pytest.mark.skipif(not on_win, reason="shortcuts only relevant on Windows")
def test_shortcut_absent_when_condarc_set(self):
- from menuinst.win32 import dirs as win_locations
+ win_locations = get_win_locations()
user_mode = 'user' if exists(join(sys.prefix, u'.nonadmin')) else 'system'
shortcut_dir = win_locations[user_mode]["start"]
shortcut_dir = join(shortcut_dir, "Anaconda{0} ({1}-bit)"
| Add --json flag on conda-env that works!
The json flag seems to be there, but it is not working.
With a file that looks like
```yaml
# environment.yaml
name: test_env_1
channels:
- defaults
dependencies:
- python
- pytest-cov
```
```
(root) $ conda-env create --json
Using Anaconda API: http s://api.anaconda.org
(root) $
```
So two problems here:
1.) No json output on the create process
2.) `Using Anaconda API: https://api.anaconda.org`
| 2017-05-16T18:47:06 | -1.0 |
|
conda/conda | 5,373 | conda__conda-5373 | [
"5248"
] | 4df735e8779af55b1452b6b3fc2d3d64d4ad06a7 | diff --git a/conda/plan.py b/conda/plan.py
--- a/conda/plan.py
+++ b/conda/plan.py
@@ -235,7 +235,7 @@ def format(s, pkg):
print(format(oldfmt[pkg] + arrow + newfmt[pkg], pkg))
if downgraded:
- print("\nThe following packages will be DOWNGRADED due to dependency conflicts:\n")
+ print("\nThe following packages will be DOWNGRADED:\n")
for pkg in sorted(downgraded):
print(format(oldfmt[pkg] + arrow + newfmt[pkg], pkg))
| diff --git a/tests/test_plan.py b/tests/test_plan.py
--- a/tests/test_plan.py
+++ b/tests/test_plan.py
@@ -215,7 +215,7 @@ def test_display_actions():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 --> 0.19-py33_0
@@ -241,7 +241,7 @@ def test_display_actions():
cython: 0.19-py33_0 --> 0.19.1-py33_0
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
dateutil: 2.1-py33_1 --> 1.5-py33_0 \n\
@@ -268,7 +268,7 @@ def test_display_actions():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 --> 0.19-py33_0
dateutil: 2.1-py33_1 --> 1.5-py33_0 \n\
@@ -356,7 +356,7 @@ def test_display_actions_show_channel_urls():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 <unknown> --> 0.19-py33_0 <unknown>
@@ -382,7 +382,7 @@ def test_display_actions_show_channel_urls():
cython: 0.19-py33_0 <unknown> --> 0.19.1-py33_0 <unknown>
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 <unknown>
@@ -409,7 +409,7 @@ def test_display_actions_show_channel_urls():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 <unknown> --> 0.19-py33_0 <unknown>
dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 <unknown>
@@ -440,7 +440,7 @@ def test_display_actions_show_channel_urls():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 my_channel --> 0.19-py33_0 <unknown> \n\
dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 my_channel
@@ -497,7 +497,7 @@ def test_display_actions_link_type():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 --> 0.19-py33_0 (softlink)
dateutil: 2.1-py33_1 --> 1.5-py33_0 (softlink)
@@ -547,7 +547,7 @@ def test_display_actions_link_type():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 --> 0.19-py33_0
dateutil: 2.1-py33_1 --> 1.5-py33_0 \n\
@@ -597,7 +597,7 @@ def test_display_actions_link_type():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 --> 0.19-py33_0 (copy)
dateutil: 2.1-py33_1 --> 1.5-py33_0 (copy)
@@ -655,7 +655,7 @@ def test_display_actions_link_type():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
cython: 0.19.1-py33_0 my_channel --> 0.19-py33_0 <unknown> (copy)
dateutil: 2.1-py33_1 <unknown> --> 1.5-py33_0 my_channel (copy)
@@ -699,7 +699,7 @@ def test_display_actions_features():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
numpy: 1.7.1-py33_p0 [mkl] --> 1.7.0-py33_p0 [mkl]
@@ -776,7 +776,7 @@ def test_display_actions_features():
display_actions(actions, index)
assert c.stdout == """
-The following packages will be DOWNGRADED due to dependency conflicts:
+The following packages will be DOWNGRADED:
numpy: 1.7.1-py33_p0 <unknown> [mkl] --> 1.7.0-py33_p0 <unknown> [mkl]
| confusing messages at downgrading
When I willfully downgrade a package, confusing dialogs are given related to dependency conflicts, possibly because the same dialog/logging is used when an automatic downgrade requirement was being determined:
```bash
(stable) └─❱❱❱ conda install pandas=0.19 +6295 8:05 ❰─┘
Fetching package metadata ...........
Solving package specifications: ..........
Package plan for installation in environment /Users/klay6683/miniconda3/envs/stable:
The following packages will be DOWNGRADED due to dependency conflicts:
pandas: 0.20.1-np112py36_0 conda-forge --> 0.19.2-np112py36_1 conda-forge
Proceed ([y]/n)?
```
I think it would be preferable, that in the deliberate downgrade the messages should not say `due to dependency conflicts` and that this part is only added to the dialog when a dependency conflict was automatically found.
version: 4.2.13 on macOS
| Seems to me the simplest thing for us to do here is to drop the "due to dependency conflicts." It's not clear to me we can reliably say that anyway. | 2017-05-19T01:51:04 | -1.0 |
conda/conda | 5,412 | conda__conda-5412 | [
"5411"
] | ce7e9d845ddd17f52967d9cc454f824617a78fdc | diff --git a/conda/cli/main_config.py b/conda/cli/main_config.py
--- a/conda/cli/main_config.py
+++ b/conda/cli/main_config.py
@@ -9,7 +9,7 @@
import collections
import json
import os
-from os.path import join
+from os.path import isfile, join
import sys
from textwrap import wrap
@@ -138,6 +138,13 @@ def configure_parser(sub_parsers):
action="store_true",
help="Describe available configuration parameters.",
)
+ action.add_argument(
+ "--write-default",
+ action="store_true",
+ help="Write the default configuration to a file. "
+ "Equivalent to `conda config --describe > ~/.condarc` "
+ "when no --env, --system, or --file flags are given.",
+ )
action.add_argument(
"--get",
nargs='*',
@@ -231,12 +238,45 @@ def format_dict(d):
return lines
+def parameter_description_builder(name):
+ from .._vendor.auxlib.entity import EntityEncoder
+ builder = []
+ details = context.describe_parameter(name)
+ aliases = details['aliases']
+ string_delimiter = details.get('string_delimiter')
+ element_types = details['element_types']
+ default_value_str = json.dumps(details['default_value'], cls=EntityEncoder)
+
+ if details['parameter_type'] == 'primitive':
+ builder.append("%s (%s)" % (name, ', '.join(sorted(set(et for et in element_types)))))
+ else:
+ builder.append("%s (%s: %s)" % (name, details['parameter_type'],
+ ', '.join(sorted(set(et for et in element_types)))))
+
+ if aliases:
+ builder.append(" aliases: %s" % ', '.join(aliases))
+ if string_delimiter:
+ builder.append(" string delimiter: '%s'" % string_delimiter)
+
+ builder.extend(' ' + line for line in wrap(details['description'], 70))
+
+ builder.append('')
+
+ builder.extend(yaml_dump({name: json.loads(default_value_str)}).strip().split('\n'))
+
+ builder = ['# ' + line for line in builder]
+ builder.append('')
+ builder.append('')
+ return builder
+
+
def execute_config(args, parser):
try:
- from cytoolz.itertoolz import groupby
+ from cytoolz.itertoolz import concat, groupby
except ImportError: # pragma: no cover
- from .._vendor.toolz.itertoolz import groupby # NOQA
+ from .._vendor.toolz.itertoolz import concat, groupby # NOQA
from .._vendor.auxlib.entity import EntityEncoder
+
json_warnings = []
json_get = {}
@@ -280,26 +320,8 @@ def execute_config(args, parser):
sort_keys=True, indent=2, separators=(',', ': '),
cls=EntityEncoder))
else:
- for name in paramater_names:
- details = context.describe_parameter(name)
- aliases = details['aliases']
- string_delimiter = details.get('string_delimiter')
- element_types = details['element_types']
- if details['parameter_type'] == 'primitive':
- print("%s (%s)" % (name, ', '.join(sorted(set(et for et in element_types)))))
- else:
- print("%s (%s: %s)" % (name, details['parameter_type'],
- ', '.join(sorted(set(et for et in element_types)))))
- def_str = ' default: %s' % json.dumps(details['default_value'], indent=2,
- separators=(',', ': '),
- cls=EntityEncoder)
- print('\n '.join(def_str.split('\n')))
- if aliases:
- print(" aliases: %s" % ', '.join(aliases))
- if string_delimiter:
- print(" string delimiter: '%s'" % string_delimiter)
- print('\n '.join(wrap(' ' + details['description'], 70)))
- print()
+ print('\n'.join(concat(parameter_description_builder(name)
+ for name in paramater_names)))
return
if args.validate:
@@ -318,6 +340,23 @@ def execute_config(args, parser):
else:
rc_path = user_rc_path
+ if args.write_default:
+ if isfile(rc_path):
+ with open(rc_path) as fh:
+ data = fh.read().strip()
+ if data:
+ raise CondaError("The file '%s' "
+ "already contains configuration information.\n"
+ "Remove the file to proceed.\n"
+ "Use `conda config --describe` to display default configuration."
+ % rc_path)
+
+ with open(rc_path, 'w') as fh:
+ paramater_names = context.list_parameters()
+ fh.write('\n'.join(concat(parameter_description_builder(name)
+ for name in paramater_names)))
+ return
+
# read existing condarc
if os.path.exists(rc_path):
with open(rc_path, 'r') as fh:
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -610,7 +610,7 @@ def test_conda_config_describe(self):
stdout, stderr = run_command(Commands.CONFIG, prefix, "--describe")
assert not stderr
for param_name in context.list_parameters():
- assert re.search(r'^%s \(' % param_name, stdout, re.MULTILINE)
+ assert re.search(r'^# %s \(' % param_name, stdout, re.MULTILINE)
stdout, stderr = run_command(Commands.CONFIG, prefix, "--describe --json")
assert not stderr
@@ -629,6 +629,19 @@ def test_conda_config_describe(self):
assert json_obj['envvars'] == {'quiet': True}
assert json_obj['cmd_line'] == {'json': True}
+ run_command(Commands.CONFIG, prefix, "--set changeps1 false")
+ with pytest.raises(CondaError):
+ run_command(Commands.CONFIG, prefix, "--write-default")
+
+ rm_rf(join(prefix, 'condarc'))
+ run_command(Commands.CONFIG, prefix, "--write-default")
+
+ with open(join(prefix, 'condarc')) as fh:
+ data = fh.read()
+
+ for param_name in context.list_parameters():
+ assert re.search(r'^# %s \(' % param_name, data, re.MULTILINE)
+
def test_conda_config_validate(self):
with make_temp_env() as prefix:
run_command(Commands.CONFIG, prefix, "--set ssl_verify no")
| Create a conda command to write default config values and info to condarc
It would be nice to have a way to materialize the whole spectrum of configuration options for conda in the user condarc (if empty).
`conda config --write-defaults`
I guess this could also be used for other locations
`conda config --write-defaults --sytem`
Something like:

| 2017-05-23T21:24:29 | -1.0 |
|
conda/conda | 5,414 | conda__conda-5414 | [
"5081",
"5081"
] | ce7e9d845ddd17f52967d9cc454f824617a78fdc | diff --git a/conda/resolve.py b/conda/resolve.py
--- a/conda/resolve.py
+++ b/conda/resolve.py
@@ -510,6 +510,9 @@ def generate_feature_metric(self, C):
def generate_removal_count(self, C, specs):
return {'!'+self.push_MatchSpec(C, ms.name): 1 for ms in specs}
+ def generate_install_count(self, C, specs):
+ return {self.push_MatchSpec(C, ms.name): 1 for ms in specs if ms.optional}
+
def generate_package_count(self, C, missing):
return {self.push_MatchSpec(C, nm): 1 for nm in missing}
@@ -687,7 +690,7 @@ def install_specs(self, specs, installed, update_deps=True):
def install(self, specs, installed=None, update_deps=True, returnall=False):
# type: (List[str], Option[?], bool, bool) -> List[Dist]
specs, preserve = self.install_specs(specs, installed or [], update_deps)
- pkgs = self.solve(specs, returnall=returnall)
+ pkgs = self.solve(specs, returnall=returnall, _remove=False)
self.restore_bad(pkgs, preserve)
return pkgs
@@ -725,11 +728,11 @@ def remove_specs(self, specs, installed):
def remove(self, specs, installed):
specs, preserve = self.remove_specs(specs, installed)
- pkgs = self.solve(specs)
+ pkgs = self.solve(specs, _remove=True)
self.restore_bad(pkgs, preserve)
return pkgs
- def solve(self, specs, returnall=False):
+ def solve(self, specs, returnall=False, _remove=False):
# type: (List[str], bool) -> List[Dist]
try:
stdoutlog.info("Solving package specifications: ")
@@ -771,9 +774,10 @@ def mysat(specs, add_if=False):
speca.extend(MatchSpec(s) for s in specm)
# Removed packages: minimize count
- eq_optional_c = r2.generate_removal_count(C, speco)
- solution, obj7 = C.minimize(eq_optional_c, solution)
- log.debug('Package removal metric: %d', obj7)
+ if _remove:
+ eq_optional_c = r2.generate_removal_count(C, speco)
+ solution, obj7 = C.minimize(eq_optional_c, solution)
+ log.debug('Package removal metric: %d', obj7)
# Requested packages: maximize versions
eq_req_v, eq_req_b = r2.generate_version_metrics(C, specr)
@@ -795,6 +799,12 @@ def mysat(specs, add_if=False):
solution, obj4 = C.minimize(eq_req_b, solution)
log.debug('Initial package build metric: %d', obj4)
+ # Optional installations: minimize count
+ if not _remove:
+ eq_optional_install = r2.generate_install_count(C, speco)
+ solution, obj49 = C.minimize(eq_optional_install, solution)
+ log.debug('Optional package install metric: %d', obj49)
+
# Dependencies: minimize the number of packages that need upgrading
eq_u = r2.generate_update_count(C, speca)
solution, obj50 = C.minimize(eq_u, solution)
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -714,6 +714,16 @@ def test_package_pinning(self):
assert not package_is_installed(prefix, "python-2.7")
assert not package_is_installed(prefix, "itsdangerous-0.23")
+ @pytest.mark.xfail(on_win, strict=True, reason="On Windows the Python package is pulled in as 'vc' feature-provider.")
+ def test_package_optional_pinning(self):
+ with make_temp_env("") as prefix:
+ run_command(Commands.CONFIG, prefix,
+ "--add pinned_packages", "python=3.6.1=2")
+ run_command(Commands.INSTALL, prefix, "openssl")
+ assert not package_is_installed(prefix, "python")
+ run_command(Commands.INSTALL, prefix, "flask")
+ assert package_is_installed(prefix, "python-3.6.1")
+
def test_update_deps_flag_absent(self):
with make_temp_env("python=2 itsdangerous=0.23") as prefix:
assert package_is_installed(prefix, 'python-2')
| Make pinned packages optional/constrained dependencies
In the conda 4.3 feature branch, make the `get_pinned_specs()` function in `conda/plan.py` return `MatchSpec` objects with the `optional=True` flag.
Make sure (by writing extra unit tests) that the `conda.cli.common.spec_from_line()` function is both working correctly and being used correctly.
Package pinning configuration was recently implemented within condarc files via https://github.com/conda/conda/pull/4921/files. Reviewing that PR would be a good place to start.
Make pinned packages optional/constrained dependencies
In the conda 4.3 feature branch, make the `get_pinned_specs()` function in `conda/plan.py` return `MatchSpec` objects with the `optional=True` flag.
Make sure (by writing extra unit tests) that the `conda.cli.common.spec_from_line()` function is both working correctly and being used correctly.
Package pinning configuration was recently implemented within condarc files via https://github.com/conda/conda/pull/4921/files. Reviewing that PR would be a good place to start.
| While working on the test I notice something isn't working quite right, I suspect the solver:
```
run_command(Commands.INSTALL, prefix, "openssl")
assert not package_is_installed(prefix, "python")
run_command(Commands.INSTALL, prefix, "flask")
assert package_is_installed(prefix, "python-3.6.1-2")
```
works fine, while
```
run_command(Commands.CONFIG, prefix,
"--add pinned_packages", "python=3.6.1-2")
run_command(Commands.INSTALL, prefix, "openssl")
assert not package_is_installed(prefix, "python")
run_command(Commands.INSTALL, prefix, "flask")
assert package_is_installed(prefix, "python-3.6.1-2")
```
yields the error
```
UnsatisfiableError: The following specifications were found to be in conflict:
- flask
- python 3.6.1-2 (optional)
```
@mcg1969 , do you have any idea why this may be happening, and where I should follow up (file a separate bug report, etc.) ?
Interesting. Normally I tell people _never doubt the solver_ but this is certainly sufficiently new functionality that we should check. I'll see what I can find.
ha ha! _never doubt the solver_.
`python=3.6.1-2` is not the correct spec. It's either `python=3.6.1=2` or `python 3.6.1 2`, depending on how you've implemented it. `python=3.6.1-2` means `Python version 3.6.1-2`, not `Python version 3.6.1 build 2`.
Thanks ! (Just a shot in the dark: the solver itself only treats a bunch of numbers (roughly speaking :-) ), which we generate from all the various inputs (match-spec, index content, etc.). I could imagine that our encoding of the request from those inputs into numbers might be wrong somehow, i.e. the query we submit to the solver proper may not be what we intended.)
Oh ! Now that isn't very intuitive, is it ? Is that documented somewhere ?
Anyhow, thanks for the quick find, I'll adjust my test logic and see whether it works.
Well, `python=3.6.1=2` is the proper command-line syntax, and that's documented. `python 3.6.1 2` is the proper MatchSpec syntax, which is also documented, but perhaps internally.
OK, fair enough. Thanks for your assistance !
```
run_command(Commands.CONFIG, prefix,
"--add pinned_packages", "python=3.6.1=2")
run_command(Commands.INSTALL, prefix, "openssl")
assert not package_is_installed(prefix, "python")
```
is failing, i.e. python is being installed even though openssl doesn't depend on it. The MatchSpec objects look good, though.
@stefanseefeld
> is failing, i.e. python is being installed even though openssl doesn't depend on it
Are you working in 4.3.x or 4.4.x?
Right, I understand.
This is on 4.4.x.
I guess that means then that the `optional=True` flag sent to the MatchSpec object isn't working correctly within resolve?
Is this on windows? If so, features are to blame, and we're discussing that in another issue.
This is GNU/Linux.
Ah, I'll bet I know why. You're passing an optional MatchSpec right to `Resolve.solve`, I'll bet.
That's not going to work, I don't think. The reason why is that I've been using the optional specification for a long time to effect package _removals_. When you do a `conda remove xxx`, the solver gets a set of specs that exclude `xxx`, and which make _all other packages optional_. That way, if it has to remove other packages because they have a dependency relationship with `xxx`, it is given permission to do so.
But of course, we don't _want_ to remove all the packages in the environment; indeed, we want to keep as many of them as possible. So the solver has a specific pass that _maximizes the number of optional specs it keeps around_.
I was frankly unaware that y'all were trying to do this. It makes sense, don't get me wrong, but optional specs fed directly to `Resolve.solve` have had a specific meaning for quite some time. It's not entirely clear to me how this is going to be fixed.
So it sounds like we need two kinds of optional dependencies: 1) optional, but try hard to include it; and 2) optional, but try hard _not_ to include it.
It's important to note that pinned dependencies are _not_ always optional. In Stefan's example above, for instance, `python=3.6.1=2` starts out as "optional but try hard not to include it". But as soon as it _does_ get included in the environment, it is _no longer optional at all_.
Yeah, from a user's perspective I find the terminology confusing. It's the "pinned" that is the operational term here. Optional merely means that the pinning should have no bearing on whether or not the package gets installed.
So perhaps we can just find a better term to express the concept, to be less confusing to ourselves.
Well, we're trying to _implement_ pinning using conda's internal optional dependency mechanism. We don't necessarily need to use the term "optional" in public facing documentation, that's for sure.
But I realized over lunch how we can get this to work. We can create a "virtual package" that is installed in each environment. That package would include as optional dependencies every pinned package. This would ensure that conda behaves properly no matter what the operations are.
That sounds good. (And yes, please let's make sure the user-facing information deploys a less ambiguous term to flag this). I'm getting confused each time I try to parse the title of this very issue. ;-)
@stefanseefeld I just found an error that will/should be affecting your tests here...
https://github.com/conda/conda/blob/4.4.x/conda/models/index_record.py#L126
Should be `optional=True`, not `option=True`.
Good catch ! Unfortunately, the test still fails with that fixed. I'll keep looking...
The tests _should_ fail unless the metapackage idea is implemented. If you supply an "optional=True" directly to the `conda.resolve.Resolve.solve` spec list, it's going to try to install it---it just won't complain if it can't.
OK, so it sounds like we need a plan for how to attack this. I'd like to help, but don't think I have enough knowledge about the solver internals to even understand what needs to be done.
While working on the test I notice something isn't working quite right, I suspect the solver:
```
run_command(Commands.INSTALL, prefix, "openssl")
assert not package_is_installed(prefix, "python")
run_command(Commands.INSTALL, prefix, "flask")
assert package_is_installed(prefix, "python-3.6.1-2")
```
works fine, while
```
run_command(Commands.CONFIG, prefix,
"--add pinned_packages", "python=3.6.1-2")
run_command(Commands.INSTALL, prefix, "openssl")
assert not package_is_installed(prefix, "python")
run_command(Commands.INSTALL, prefix, "flask")
assert package_is_installed(prefix, "python-3.6.1-2")
```
yields the error
```
UnsatisfiableError: The following specifications were found to be in conflict:
- flask
- python 3.6.1-2 (optional)
```
@mcg1969 , do you have any idea why this may be happening, and where I should follow up (file a separate bug report, etc.) ?
Interesting. Normally I tell people _never doubt the solver_ but this is certainly sufficiently new functionality that we should check. I'll see what I can find.
ha ha! _never doubt the solver_.
`python=3.6.1-2` is not the correct spec. It's either `python=3.6.1=2` or `python 3.6.1 2`, depending on how you've implemented it. `python=3.6.1-2` means `Python version 3.6.1-2`, not `Python version 3.6.1 build 2`.
Thanks ! (Just a shot in the dark: the solver itself only treats a bunch of numbers (roughly speaking :-) ), which we generate from all the various inputs (match-spec, index content, etc.). I could imagine that our encoding of the request from those inputs into numbers might be wrong somehow, i.e. the query we submit to the solver proper may not be what we intended.)
Oh ! Now that isn't very intuitive, is it ? Is that documented somewhere ?
Anyhow, thanks for the quick find, I'll adjust my test logic and see whether it works.
Well, `python=3.6.1=2` is the proper command-line syntax, and that's documented. `python 3.6.1 2` is the proper MatchSpec syntax, which is also documented, but perhaps internally.
OK, fair enough. Thanks for your assistance !
```
run_command(Commands.CONFIG, prefix,
"--add pinned_packages", "python=3.6.1=2")
run_command(Commands.INSTALL, prefix, "openssl")
assert not package_is_installed(prefix, "python")
```
is failing, i.e. python is being installed even though openssl doesn't depend on it. The MatchSpec objects look good, though.
@stefanseefeld
> is failing, i.e. python is being installed even though openssl doesn't depend on it
Are you working in 4.3.x or 4.4.x?
Right, I understand.
This is on 4.4.x.
I guess that means then that the `optional=True` flag sent to the MatchSpec object isn't working correctly within resolve?
Is this on windows? If so, features are to blame, and we're discussing that in another issue.
This is GNU/Linux.
Ah, I'll bet I know why. You're passing an optional MatchSpec right to `Resolve.solve`, I'll bet.
That's not going to work, I don't think. The reason why is that I've been using the optional specification for a long time to effect package _removals_. When you do a `conda remove xxx`, the solver gets a set of specs that exclude `xxx`, and which make _all other packages optional_. That way, if it has to remove other packages because they have a dependency relationship with `xxx`, it is given permission to do so.
But of course, we don't _want_ to remove all the packages in the environment; indeed, we want to keep as many of them as possible. So the solver has a specific pass that _maximizes the number of optional specs it keeps around_.
I was frankly unaware that y'all were trying to do this. It makes sense, don't get me wrong, but optional specs fed directly to `Resolve.solve` have had a specific meaning for quite some time. It's not entirely clear to me how this is going to be fixed.
So it sounds like we need two kinds of optional dependencies: 1) optional, but try hard to include it; and 2) optional, but try hard _not_ to include it.
It's important to note that pinned dependencies are _not_ always optional. In Stefan's example above, for instance, `python=3.6.1=2` starts out as "optional but try hard not to include it". But as soon as it _does_ get included in the environment, it is _no longer optional at all_.
Yeah, from a user's perspective I find the terminology confusing. It's the "pinned" that is the operational term here. Optional merely means that the pinning should have no bearing on whether or not the package gets installed.
So perhaps we can just find a better term to express the concept, to be less confusing to ourselves.
Well, we're trying to _implement_ pinning using conda's internal optional dependency mechanism. We don't necessarily need to use the term "optional" in public facing documentation, that's for sure.
But I realized over lunch how we can get this to work. We can create a "virtual package" that is installed in each environment. That package would include as optional dependencies every pinned package. This would ensure that conda behaves properly no matter what the operations are.
That sounds good. (And yes, please let's make sure the user-facing information deploys a less ambiguous term to flag this). I'm getting confused each time I try to parse the title of this very issue. ;-)
@stefanseefeld I just found an error that will/should be affecting your tests here...
https://github.com/conda/conda/blob/4.4.x/conda/models/index_record.py#L126
Should be `optional=True`, not `option=True`.
Good catch ! Unfortunately, the test still fails with that fixed. I'll keep looking...
The tests _should_ fail unless the metapackage idea is implemented. If you supply an "optional=True" directly to the `conda.resolve.Resolve.solve` spec list, it's going to try to install it---it just won't complain if it can't.
OK, so it sounds like we need a plan for how to attack this. I'd like to help, but don't think I have enough knowledge about the solver internals to even understand what needs to be done. | 2017-05-24T13:21:11 | -1.0 |
conda/conda | 5,426 | conda__conda-5426 | [
"5425"
] | c93159791bb4600a1f67a06c7108c5c73ffcfd98 | diff --git a/conda/common/platform.py b/conda/common/platform.py
--- a/conda/common/platform.py
+++ b/conda/common/platform.py
@@ -19,12 +19,12 @@ def is_admin_on_windows(): # pragma: unix no cover
return False
try:
from ctypes import windll
- return windll.shell32.IsUserAnAdmin()() != 0
+ return windll.shell32.IsUserAnAdmin() != 0
except ImportError as e:
log.debug('%r', e)
return 'unknown'
except Exception as e:
- log.warn('%r', e)
+ log.info('%r', e)
return 'unknown'
| diff --git a/tests/common/test_platform.py b/tests/common/test_platform.py
new file mode 100644
--- /dev/null
+++ b/tests/common/test_platform.py
@@ -0,0 +1,13 @@
+# -*- coding: utf-8 -*-
+from __future__ import absolute_import, division, print_function, unicode_literals
+
+from conda.common.compat import on_win
+from conda.common.platform import is_admin_on_windows
+
+
+def test_is_admin_on_windows():
+ result = is_admin_on_windows()
+ if not on_win:
+ assert result is False
+ else:
+ assert result is False or result is True
| Launching navigator via prompt warnings appear
_From @RidaZubair on May 24, 2017 9:47_
**OS:** Windows
**Anaconda: 4.4.0**
**Actual:**
On launching navigator via prompt following warning appears on prompt

_Copied from original issue: ContinuumIO/navigator#1189_
| _From @goanpeca on May 24, 2017 13:52_
@kalefranz whats with the conda.common.platform error there?
@RidaZubair warnings are just to inform that the conda commands that we called might have return something on the stderr, but by themselves they are not errors.
However I am currious about the first Warning on Conda. Is Navigator not working in any way?
In general WARNING messages are not a problem.
_From @goanpeca on May 25, 2017 12:56_
Remove any warnings for output on stderr when parsing conda commands.
Depending on the conda version, was probably https://github.com/conda/conda/pull/5368, fixed in 4.3.20.
Crud, that error is pretty obvious. https://github.com/conda/conda/pull/5368/files#diff-56d5b142f8053df84f4a4e13d99c3864R22
A second couple-character patch for 4.3.21. | 2017-05-25T13:14:13 | -1.0 |
conda/conda | 5,452 | conda__conda-5452 | [
"5451"
] | 2dd2233452e96adf3c2b2af232b3fb457ec6a51d | diff --git a/conda/cli/main_clean.py b/conda/cli/main_clean.py
--- a/conda/cli/main_clean.py
+++ b/conda/cli/main_clean.py
@@ -120,7 +120,7 @@ def rm_tarballs(args, pkgs_dirs, totalsize, verbose=True):
print(fmt % ('Total:', human_bytes(totalsize)))
print()
- if not context.json or not context.yes:
+ if not context.json or not context.always_yes:
confirm_yn(args)
if context.json and args.dry_run:
return
@@ -218,7 +218,7 @@ def rm_pkgs(args, pkgs_dirs, warnings, totalsize, pkgsizes,
print(fmt % ('Total:', human_bytes(totalsize)))
print()
- if not context.json or not context.yes:
+ if not context.json or not context.always_yes:
confirm_yn(args)
if context.json and args.dry_run:
return
@@ -279,7 +279,7 @@ def rm_source_cache(args, cache_dirs, warnings, cache_sizes, total_size):
print("%-40s %10s" % ("Total:", human_bytes(total_size)))
- if not context.json or not context.yes:
+ if not context.json or not context.always_yes:
confirm_yn(args)
if context.json and args.dry_run:
return
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -1072,8 +1072,11 @@ def test_clean_tarballs_and_packages(self):
assert any(basename(d).startswith('flask-') for d in pkgs_dir_dirs)
assert any(basename(f).startswith('flask-') for f in pkgs_dir_tarballs)
- run_command(Commands.CLEAN, prefix, "--packages --yes")
- run_command(Commands.CLEAN, prefix, "--tarballs --yes")
+ # --json flag is regression test for #5451
+ run_command(Commands.CLEAN, prefix, "--packages --yes --json")
+
+ # --json flag is regression test for #5451
+ run_command(Commands.CLEAN, prefix, "--tarballs --yes --json")
pkgs_dir_contents = [join(pkgs_dir, d) for d in os.listdir(pkgs_dir)]
pkgs_dir_dirs = [d for d in pkgs_dir_contents if isdir(d)]
@@ -1102,7 +1105,8 @@ def test_clean_source_cache(self):
assert all(isdir(d) for d in itervalues(cache_dirs))
- run_command(Commands.CLEAN, '', "--source-cache --yes")
+ # --json flag is regression test for #5451
+ run_command(Commands.CLEAN, '', "--source-cache --yes --json")
assert not all(isdir(d) for d in itervalues(cache_dirs))
| conda clean --json error
`conda clean -t --json --dry-run
{
"error": "Traceback (most recent call last):\n File \"C:\\Anaconda27\\lib\\site-packages\\conda\\exceptions.py\", line 632, in conda_exception_handler\n return_value = func(*args, **kwargs)\n File \"C:\\Anaconda27\\lib\\site-packages\\conda\\cli\\main.py\", line 137, in _main\n exit_code = args.func(args, p)\n File \"C:\\Anaconda27\\lib\\site-packages\\conda\\cli\\main_clean.py\", line 306, in execute\n rm_tarballs(args, pkgs_dirs, totalsize, verbose=not context.json)\n File \"C:\\Anaconda27\\lib\\site-packages\\conda\\cli\\main_clean.py\", line 123, in rm_tarballs\n if not context.json or not context.yes:\nAttributeError: 'Context' object has no attribute 'yes'\n"
}
conda info --all
Current conda install:
platform : win-64
conda version : 4.3.21
conda is private : False
conda-env version : 4.3.21
conda-build version : 2.1.15
python version : 2.7.13.final.0
requests version : 2.12.4
root environment : C:\Anaconda27 (writable)
default environment : C:\Anaconda27
envs directories : C:\Anaconda27\envs
C:\Users\Oammous\AppData\Local\conda\conda\envs
C:\Users\Oammous\.conda\envs
package cache : C:\Anaconda27\pkgs
C:\Users\Oammous\AppData\Local\conda\conda\pkgs
channel URLs : http://conda/prod/win-64
http://conda/prod/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
https://conda.anaconda.org/conda-forge/win-64
https://conda.anaconda.org/conda-forge/noarch
config file : C:\Users\Oammous\.condarc
netrc file : None
offline mode : False
user-agent : conda/4.3.21 requests/2.12.4 CPython/2.7.13 Windows/10 Windows/10.0.10586
administrator : False
# conda environments:
#
_test C:\Anaconda27\envs\_test
gulbis C:\Anaconda27\envs\gulbis
py3 C:\Anaconda27\envs\py3
root * C:\Anaconda27
sys.version: 2.7.13 |Anaconda custom (64-bit)| (defau...
sys.prefix: C:\Anaconda27
sys.executable: C:\Anaconda27\python.exe
conda location: C:\Anaconda27\lib\site-packages\conda
conda-build: C:\Anaconda27\Scripts\conda-build.exe
conda-convert: C:\Anaconda27\Scripts\conda-convert.exe
conda-develop: C:\Anaconda27\Scripts\conda-develop.exe
conda-env: C:\Anaconda27\Scripts\conda-env.exe
conda-index: C:\Anaconda27\Scripts\conda-index.exe
conda-inspect: C:\Anaconda27\Scripts\conda-inspect.exe
conda-metapackage: C:\Anaconda27\Scripts\conda-metapackage.exe
conda-render: C:\Anaconda27\Scripts\conda-render.exe
conda-server: C:\Anaconda27\Scripts\conda-server.exe
conda-sign: C:\Anaconda27\Scripts\conda-sign.exe
conda-skeleton: C:\Anaconda27\Scripts\conda-skeleton.exe
conda-verify: C:\Anaconda27\Scripts\conda-verify.exe
`
| 2017-05-30T16:03:00 | -1.0 |
|
conda/conda | 5,467 | conda__conda-5467 | [
"5466",
"5466"
] | 79e79bdbbf2e783eb6092e7e16493e4bfbc7a82e | diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -16,10 +16,10 @@
try:
from cytoolz.functoolz import excepts
- from cytoolz.itertoolz import concatv, topk
+ from cytoolz.itertoolz import concatv, drop
except ImportError: # pragma: no cover
from .._vendor.toolz.functoolz import excepts # NOQA
- from .._vendor.toolz.itertoolz import concatv, topk # NOQA
+ from .._vendor.toolz.itertoolz import concatv, drop # NOQA
log = getLogger(__name__)
@@ -420,7 +420,13 @@ def _read_channel_configuration(scheme, host, port, path):
return location, name, _scheme, _auth, _token
# Step 7. fall through to host:port as channel_location and path as channel_name
- return (Url(host=host, port=port).url.rstrip('/'), path.strip('/') or None,
+ # but bump the first token of paths starting with /conda for compatibility with
+ # Anaconda Enterprise Repository software.
+ bump = None
+ path_parts = path.strip('/').split('/')
+ if path_parts and path_parts[0] == 'conda':
+ bump, path = 'conda', '/'.join(drop(1, path_parts))
+ return (Url(host=host, port=port, path=bump).url.rstrip('/'), path.strip('/') or None,
scheme or None, None, None)
| diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -20,7 +20,6 @@
from conda.utils import on_win
from logging import getLogger
from unittest import TestCase
-import conda.models.channel
try:
from unittest.mock import patch
@@ -248,6 +247,31 @@ def test_canonicalized_url_gets_correct_token(self):
"https://10.2.3.4:8080/conda/t/x1029384756/bioconda/noarch",
]
+ def test_token_in_custom_channel(self):
+ channel = Channel("https://10.2.8.9:8080/conda/t/tk-987-321/bioconda/label/dev")
+ assert channel.name == "bioconda/label/dev"
+ assert channel.location == "10.2.8.9:8080/conda"
+ assert channel.urls() == [
+ "https://10.2.8.9:8080/conda/bioconda/label/dev/%s" % self.platform,
+ "https://10.2.8.9:8080/conda/bioconda/label/dev/noarch",
+ ]
+ assert channel.urls(with_credentials=True) == [
+ "https://10.2.8.9:8080/conda/t/tk-987-321/bioconda/label/dev/%s" % self.platform,
+ "https://10.2.8.9:8080/conda/t/tk-987-321/bioconda/label/dev/noarch",
+ ]
+
+ channel = Channel("https://10.2.8.9:8080/conda/t/tk-987-321/bioconda")
+ assert channel.name == "bioconda"
+ assert channel.location == "10.2.8.9:8080/conda"
+ assert channel.urls() == [
+ "https://10.2.8.9:8080/conda/bioconda/%s" % self.platform,
+ "https://10.2.8.9:8080/conda/bioconda/noarch",
+ ]
+ assert channel.urls(with_credentials=True) == [
+ "https://10.2.8.9:8080/conda/t/tk-987-321/bioconda/%s" % self.platform,
+ "https://10.2.8.9:8080/conda/t/tk-987-321/bioconda/noarch",
+ ]
+
class CustomConfigChannelTests(TestCase):
"""
| scrambled URL of non-alias channel with token
The URL parsing would swap around locations of URL parts if passed a `/t/<TOKEN>` URL that was not the `channel_alias`.
scrambled URL of non-alias channel with token
The URL parsing would swap around locations of URL parts if passed a `/t/<TOKEN>` URL that was not the `channel_alias`.
| 2017-06-01T23:48:30 | -1.0 |
|
conda/conda | 5,471 | conda__conda-5471 | [
"5470",
"5470"
] | 79e79bdbbf2e783eb6092e7e16493e4bfbc7a82e | diff --git a/conda/cli/python_api.py b/conda/cli/python_api.py
--- a/conda/cli/python_api.py
+++ b/conda/cli/python_api.py
@@ -8,7 +8,7 @@
from ..base.constants import APP_NAME, SEARCH_PATH
from ..base.context import context
from ..cli.main import generate_parser
-from ..common.io import captured, replace_log_streams, argv
+from ..common.io import captured, replace_log_streams, argv, CaptureTarget
from ..common.path import win_path_double_escape
from ..exceptions import conda_exception_handler
from ..gateways.logging import initialize_std_loggers
@@ -28,6 +28,10 @@ class Commands:
UPDATE = "update"
+STRING = CaptureTarget.STRING
+STDOUT = CaptureTarget.STDOUT
+
+
def get_configure_parser_function(command):
module = 'conda.cli.main_' + command
return import_module(module).configure_parser
@@ -49,8 +53,16 @@ def run_command(command, *arguments, **kwargs):
has occured, and instead give a non-zero return code
search_path: an optional non-standard search path for configuration information
that overrides the default SEARCH_PATH
+ stdout: Define capture behavior for stream sys.stdout. Defaults to STRING.
+ STRING captures as a string. None leaves stream untouched.
+ Otherwise redirect to file-like object stdout.
+ stderr: Define capture behavior for stream sys.stderr. Defaults to STRING.
+ STRING captures as a string. None leaves stream untouched.
+ STDOUT redirects to stdout target and returns None as stderr value.
+ Otherwise redirect to file-like object stderr.
- Returns: a tuple of stdout, stderr, and return_code
+ Returns: a tuple of stdout, stderr, and return_code.
+ stdout, stderr are either strings, None or the corresponding file-like function argument.
Examples:
>> run_command(Commands.CREATE, "-n newenv python=3 flask", use_exception_handler=True)
@@ -62,6 +74,8 @@ def run_command(command, *arguments, **kwargs):
initialize_std_loggers()
use_exception_handler = kwargs.get('use_exception_handler', False)
configuration_search_path = kwargs.get('search_path', SEARCH_PATH)
+ stdout = kwargs.get('stdout', STRING)
+ stderr = kwargs.get('stderr', STRING)
p, sub_parsers = generate_parser()
get_configure_parser_function(command)(sub_parsers)
@@ -78,7 +92,8 @@ def run_command(command, *arguments, **kwargs):
)
log.debug("executing command >>> conda %s", command_line)
try:
- with argv(['python_api'] + split_command_line), captured() as c, replace_log_streams():
+ python_api_command_line = ['python_api'] + split_command_line
+ with argv(python_api_command_line), captured(stdout, stderr) as c, replace_log_streams():
if use_exception_handler:
return_code = conda_exception_handler(args.func, args, p)
else:
diff --git a/conda/common/io.py b/conda/common/io.py
--- a/conda/common/io.py
+++ b/conda/common/io.py
@@ -2,6 +2,7 @@
from __future__ import absolute_import, division, print_function, unicode_literals
from contextlib import contextmanager
+from enum import Enum
import logging
from logging import CRITICAL, Formatter, NOTSET, StreamHandler, WARN, getLogger
import os
@@ -17,6 +18,15 @@
_FORMATTER = Formatter("%(levelname)s %(name)s:%(funcName)s(%(lineno)d): %(message)s")
+class CaptureTarget(Enum):
+ """Constants used for contextmanager captured.
+
+ Used similarily like the constants PIPE, STDOUT for stdlib's subprocess.Popen.
+ """
+ STRING = -1
+ STDOUT = -2
+
+
@contextmanager
def env_var(name, value, callback=None):
# NOTE: will likely want to call reset_context() when using this function, so pass
@@ -69,20 +79,58 @@ def cwd(directory):
@contextmanager
-def captured():
+def captured(stdout=CaptureTarget.STRING, stderr=CaptureTarget.STRING):
+ """Capture outputs of sys.stdout and sys.stderr.
+
+ If stdout is STRING, capture sys.stdout as a string,
+ if stdout is None, do not capture sys.stdout, leaving it untouched,
+ otherwise redirect sys.stdout to the file-like object given by stdout.
+
+ Behave correspondingly for stderr with the exception that if stderr is STDOUT,
+ redirect sys.stderr to stdout target and set stderr attribute of yielded object to None.
+
+ Args:
+ stdout: capture target for sys.stdout, one of STRING, None, or file-like object
+ stderr: capture target for sys.stderr, one of STRING, STDOUT, None, or file-like object
+
+ Yields:
+ CapturedText: has attributes stdout, stderr which are either strings, None or the
+ corresponding file-like function argument.
+ """
# NOTE: This function is not thread-safe. Using within multi-threading may cause spurious
# behavior of not returning sys.stdout and sys.stderr back to their 'proper' state
class CapturedText(object):
pass
saved_stdout, saved_stderr = sys.stdout, sys.stderr
- sys.stdout = outfile = StringIO()
- sys.stderr = errfile = StringIO()
+ if stdout == CaptureTarget.STRING:
+ sys.stdout = outfile = StringIO()
+ else:
+ outfile = stdout
+ if outfile is not None:
+ sys.stdout = outfile
+ if stderr == CaptureTarget.STRING:
+ sys.stderr = errfile = StringIO()
+ elif stderr == CaptureTarget.STDOUT:
+ sys.stderr = errfile = outfile
+ else:
+ errfile = stderr
+ if errfile is not None:
+ sys.stderr = errfile
c = CapturedText()
log.info("overtaking stderr and stdout")
try:
yield c
finally:
- c.stdout, c.stderr = outfile.getvalue(), errfile.getvalue()
+ if stdout == CaptureTarget.STRING:
+ c.stdout = outfile.getvalue()
+ else:
+ c.stdout = outfile
+ if stderr == CaptureTarget.STRING:
+ c.stderr = errfile.getvalue()
+ elif stderr == CaptureTarget.STDOUT:
+ c.stderr = None
+ else:
+ c.stderr = errfile
sys.stdout, sys.stderr = saved_stdout, saved_stderr
log.info("stderr and stdout yielding back")
| diff --git a/tests/common/test_io.py b/tests/common/test_io.py
--- a/tests/common/test_io.py
+++ b/tests/common/test_io.py
@@ -1,8 +1,48 @@
# -*- coding: utf-8 -*-
from __future__ import absolute_import, division, print_function, unicode_literals
-from conda.common.io import attach_stderr_handler, captured
+from conda.common.io import attach_stderr_handler, captured, CaptureTarget
+from io import StringIO
from logging import DEBUG, NOTSET, WARN, getLogger
+import sys
+
+
+def test_captured():
+ stdout_text = "stdout text"
+ stderr_text = "stderr text"
+
+ def print_captured(*args, **kwargs):
+ with captured(*args, **kwargs) as c:
+ print(stdout_text, end="")
+ print(stderr_text, end="", file=sys.stderr)
+ return c
+
+ c = print_captured()
+ assert c.stdout == stdout_text
+ assert c.stderr == stderr_text
+
+ c = print_captured(CaptureTarget.STRING, CaptureTarget.STRING)
+ assert c.stdout == stdout_text
+ assert c.stderr == stderr_text
+
+ c = print_captured(stderr=CaptureTarget.STDOUT)
+ assert c.stdout == stdout_text + stderr_text
+ assert c.stderr is None
+
+ caller_stdout = StringIO()
+ caller_stderr = StringIO()
+ c = print_captured(caller_stdout, caller_stderr)
+ assert c.stdout is caller_stdout
+ assert c.stderr is caller_stderr
+ assert caller_stdout.getvalue() == stdout_text
+ assert caller_stderr.getvalue() == stderr_text
+
+ with captured() as outer_c:
+ inner_c = print_captured(None, None)
+ assert inner_c.stdout is None
+ assert inner_c.stderr is None
+ assert outer_c.stdout == stdout_text
+ assert outer_c.stderr == stderr_text
def test_attach_stderr_handler():
| make stdout/stderr capture in python_api customizable
Let the user customize how and if the standard output/error streams should be captured.
make stdout/stderr capture in python_api customizable
Let the user customize how and if the standard output/error streams should be captured.
| 2017-06-02T00:04:22 | -1.0 |
|
conda/conda | 5,703 | conda__conda-5703 | [
"5649",
"5649"
] | 7cab18070a6397cbf2218144edd7fa64c17a5964 | diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -163,14 +163,13 @@ def install(args, parser, command='install'):
raise PackageNotInstalledError(prefix, name)
if newenv and not args.no_default_packages:
- default_packages = list(context.create_default_packages)
# Override defaults if they are specified at the command line
+ # TODO: rework in 4.4 branch using MatchSpec
+ args_packages_names = [pkg.replace(' ', '=').split('=', 1)[0] for pkg in args_packages]
for default_pkg in context.create_default_packages:
- if any(pkg.split('=')[0] == default_pkg for pkg in args_packages):
- default_packages.remove(default_pkg)
- args_packages.extend(default_packages)
- else:
- default_packages = []
+ default_pkg_name = default_pkg.replace(' ', '=').split('=', 1)[0]
+ if default_pkg_name not in args_packages_names:
+ args_packages.append(default_pkg)
common.ensure_use_local(args)
common.ensure_override_channels_requires_channel(args)
@@ -212,9 +211,8 @@ def install(args, parser, command='install'):
" filenames")
if newenv and args.clone:
- package_diff = set(args_packages) - set(default_packages)
- if package_diff:
- raise TooManyArgumentsError(0, len(package_diff), list(package_diff),
+ if args.packages:
+ raise TooManyArgumentsError(0, len(args.packages), list(args.packages),
'did not expect any arguments for --clone')
clone(args.clone, prefix, json=context.json, quiet=context.quiet, index_args=index_args)
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -191,7 +191,7 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
@pytest.mark.installed
def test_activate_deactivate(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 7, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2017, 8, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
@@ -208,7 +208,7 @@ def test_activate_deactivate(shell):
@pytest.mark.installed
def test_activate_root_simple(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 7, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2017, 8, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -674,7 +674,7 @@ def test_update_deps_flag_absent(self):
assert package_is_installed(prefix, 'itsdangerous-0.23')
assert package_is_installed(prefix, 'flask')
- @pytest.mark.xfail(datetime.now() < datetime(2017, 7, 1), reason="#5263", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2017, 8, 1), reason="#5263", strict=True)
def test_update_deps_flag_present(self):
with make_temp_env("python=2 itsdangerous=0.23") as prefix:
assert package_is_installed(prefix, 'python-2')
@@ -794,12 +794,11 @@ def test_create_default_packages(self):
prefix = make_temp_prefix(str(uuid4())[:7])
# set packages
- run_command(Commands.CONFIG, prefix, "--add create_default_packages python")
run_command(Commands.CONFIG, prefix, "--add create_default_packages pip")
run_command(Commands.CONFIG, prefix, "--add create_default_packages flask")
stdout, stderr = run_command(Commands.CONFIG, prefix, "--show")
yml_obj = yaml_load(stdout)
- assert yml_obj['create_default_packages'] == ['flask', 'pip', 'python']
+ assert yml_obj['create_default_packages'] == ['flask', 'pip']
assert not package_is_installed(prefix, 'python-2')
assert not package_is_installed(prefix, 'pytz')
@@ -818,12 +817,11 @@ def test_create_default_packages_no_default_packages(self):
prefix = make_temp_prefix(str(uuid4())[:7])
# set packages
- run_command(Commands.CONFIG, prefix, "--add create_default_packages python")
run_command(Commands.CONFIG, prefix, "--add create_default_packages pip")
run_command(Commands.CONFIG, prefix, "--add create_default_packages flask")
stdout, stderr = run_command(Commands.CONFIG, prefix, "--show")
yml_obj = yaml_load(stdout)
- assert yml_obj['create_default_packages'] == ['flask', 'pip', 'python']
+ assert yml_obj['create_default_packages'] == ['flask', 'pip']
assert not package_is_installed(prefix, 'python-2')
assert not package_is_installed(prefix, 'pytz')
diff --git a/tests/test_export.py b/tests/test_export.py
--- a/tests/test_export.py
+++ b/tests/test_export.py
@@ -31,7 +31,7 @@ def test_basic(self):
output2, error= run_command(Commands.LIST, prefix2, "-e")
self.assertEqual(output, output2)
- @pytest.mark.xfail(datetime.now() < datetime(2017, 7, 1), reason="Bring back `conda list --export` #3445", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2017, 8, 1), reason="Bring back `conda list --export` #3445", strict=True)
def test_multi_channel_export(self):
"""
When try to import from txt
| Conda 4.3 not installing default packages
I have the following `.condarc`:
```
channels:
- conda-forge
- defaults
create_default_packages:
- ipython
```
When creating a new environment with `conda 4.3.22` the `ipython` package is not included:
```
$ conda create -n testenv python=3
Fetching package metadata .............
Solving package specifications: .
Package plan for installation in environment /home/ubuntu/miniconda/envs/OCemptor5:
The following NEW packages will be INSTALLED:
ca-certificates: 2017.4.17-0 conda-forge
certifi: 2017.4.17-py36_0 conda-forge
ncurses: 5.9-10 conda-forge
openssl: 1.0.2k-0 conda-forge
pip: 9.0.1-py36_0 conda-forge
python: 3.6.1-3 conda-forge
readline: 6.2-0 conda-forge
setuptools: 33.1.1-py36_0 conda-forge
sqlite: 3.13.0-1 conda-forge
tk: 8.5.19-1 conda-forge
wheel: 0.29.0-py36_0 conda-forge
xz: 5.2.2-0 conda-forge
zlib: 1.2.11-0 conda-forge
Proceed ([y]/n)?
```
And when using latest 4.2.13 version the default packages are included:
```
$ conda create -n testenv python=3
Fetching package metadata ...........
Solving package specifications: ..........
Package plan for installation in environment /home/ubuntu/miniconda/envs/OCemptor5:
The following NEW packages will be INSTALLED:
ca-certificates: 2017.4.17-0 conda-forge
certifi: 2017.4.17-py36_0 conda-forge
decorator: 4.0.11-py36_0 conda-forge
ipython: 6.1.0-py36_0 conda-forge
ipython_genutils: 0.2.0-py36_0 conda-forge
jedi: 0.10.0-py36_0 conda-forge
ncurses: 5.9-10 conda-forge
openssl: 1.0.2k-0 conda-forge
pexpect: 4.2.1-py36_0 conda-forge
pickleshare: 0.7.3-py36_0 conda-forge
pip: 9.0.1-py36_0 conda-forge
prompt_toolkit: 1.0.14-py36_0 conda-forge
ptyprocess: 0.5.2-py36_0 conda-forge
pygments: 2.2.0-py36_0 conda-forge
python: 3.6.1-3 conda-forge
readline: 6.2-0 conda-forge
setuptools: 33.1.1-py36_0 conda-forge
simplegeneric: 0.8.1-py36_0 conda-forge
six: 1.10.0-py36_1 conda-forge
sqlite: 3.13.0-1 conda-forge
tk: 8.5.19-1 conda-forge
traitlets: 4.3.2-py36_0 conda-forge
wcwidth: 0.1.7-py36_0 conda-forge
wheel: 0.29.0-py36_0 conda-forge
xz: 5.2.2-0 conda-forge
zlib: 1.2.11-0 conda-forge
Proceed ([y]/n)?
```
Conda 4.3 not installing default packages
I have the following `.condarc`:
```
channels:
- conda-forge
- defaults
create_default_packages:
- ipython
```
When creating a new environment with `conda 4.3.22` the `ipython` package is not included:
```
$ conda create -n testenv python=3
Fetching package metadata .............
Solving package specifications: .
Package plan for installation in environment /home/ubuntu/miniconda/envs/OCemptor5:
The following NEW packages will be INSTALLED:
ca-certificates: 2017.4.17-0 conda-forge
certifi: 2017.4.17-py36_0 conda-forge
ncurses: 5.9-10 conda-forge
openssl: 1.0.2k-0 conda-forge
pip: 9.0.1-py36_0 conda-forge
python: 3.6.1-3 conda-forge
readline: 6.2-0 conda-forge
setuptools: 33.1.1-py36_0 conda-forge
sqlite: 3.13.0-1 conda-forge
tk: 8.5.19-1 conda-forge
wheel: 0.29.0-py36_0 conda-forge
xz: 5.2.2-0 conda-forge
zlib: 1.2.11-0 conda-forge
Proceed ([y]/n)?
```
And when using latest 4.2.13 version the default packages are included:
```
$ conda create -n testenv python=3
Fetching package metadata ...........
Solving package specifications: ..........
Package plan for installation in environment /home/ubuntu/miniconda/envs/OCemptor5:
The following NEW packages will be INSTALLED:
ca-certificates: 2017.4.17-0 conda-forge
certifi: 2017.4.17-py36_0 conda-forge
decorator: 4.0.11-py36_0 conda-forge
ipython: 6.1.0-py36_0 conda-forge
ipython_genutils: 0.2.0-py36_0 conda-forge
jedi: 0.10.0-py36_0 conda-forge
ncurses: 5.9-10 conda-forge
openssl: 1.0.2k-0 conda-forge
pexpect: 4.2.1-py36_0 conda-forge
pickleshare: 0.7.3-py36_0 conda-forge
pip: 9.0.1-py36_0 conda-forge
prompt_toolkit: 1.0.14-py36_0 conda-forge
ptyprocess: 0.5.2-py36_0 conda-forge
pygments: 2.2.0-py36_0 conda-forge
python: 3.6.1-3 conda-forge
readline: 6.2-0 conda-forge
setuptools: 33.1.1-py36_0 conda-forge
simplegeneric: 0.8.1-py36_0 conda-forge
six: 1.10.0-py36_1 conda-forge
sqlite: 3.13.0-1 conda-forge
tk: 8.5.19-1 conda-forge
traitlets: 4.3.2-py36_0 conda-forge
wcwidth: 0.1.7-py36_0 conda-forge
wheel: 0.29.0-py36_0 conda-forge
xz: 5.2.2-0 conda-forge
zlib: 1.2.11-0 conda-forge
Proceed ([y]/n)?
```
| Conda always prioritizes conda-forge over the default channel by design.
You can either temporarily remove conda-forge from your .condarc or add `-c defaults` in your command.
@tyler-thetyrant By default packages I mean the ones specified in the config `create_default_packages`.
When using conda 4.3 it doesn't honor the `create_default_packages` config.
@kalefranz I see this one too in the testing installer for the next AEN version. Can you take a look on this?
conda_info
```
(/projects/testuser11/test_public/envs/default) conda info
Current conda install:
platform : linux-64
conda version : 4.3.22
conda is private : False
conda-env version : 4.3.22
conda-build version : 3.0.6
python version : 2.7.13.final.0
requests version : 2.14.2
root environment : /opt/wakari/anaconda (read only)
default environment : /projects/testuser11/test_public/envs/default
envs directories : /projects/testuser11/test_public/envs
/projects/testuser11/test_public/DEFAULTS
/home/testuser11/.conda/envs
/opt/wakari/anaconda/envs
package cache : /opt/wakari/anaconda/pkgs
/home/testuser11/.conda/pkgs
channel URLs : https://conda.anaconda.org/r/linux-64
https://conda.anaconda.org/r/noarch
https://conda.anaconda.org/wakari/linux-64
https://conda.anaconda.org/wakari/noarch
https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
config file : /opt/wakari/anaconda/.condarc
netrc file : None
offline mode : False
user-agent : conda/4.3.22 requests/2.14.2 CPython/2.7.13 Linux/2.6.32-696.3.1.el6.x86_64 CentOS/6.9 glibc/2.12
UID:GID : 500:500
```
and `.condarc`
```
(projects/testuser11/test_public/envs/default) cat /opt/wakari/anaconda/.condarc
channels:
- r
- https://conda.anaconda.org/wakari
- defaults
create_default_packages:
- anaconda-client
- ipykernel
auto_update_conda: false
```
And when I try to create a env, it does not contain the default_packages as expected
```
(/projects/testuser11/test_public/envs/default) conda create -n bar
Fetching package metadata .............
Solving package specifications:
Package plan for installation in environment /projects/testuser11/test_public/envs/bar:
Proceed ([y]/n)? y
#
# To activate this environment, use:
# > source activate bar
#
# To deactivate an active environment, use:
# > source deactivate
#
(/projects/testuser11/test_public/envs/default) conda list -n bar
# packages in environment at /projects/testuser11/test_public/envs/bar:
#
(/projects/testuser11/test_public/envs/default) ^C
```
cc @csoja
Conda always prioritizes conda-forge over the default channel by design.
You can either temporarily remove conda-forge from your .condarc or add `-c defaults` in your command.
@tyler-thetyrant By default packages I mean the ones specified in the config `create_default_packages`.
When using conda 4.3 it doesn't honor the `create_default_packages` config.
@kalefranz I see this one too in the testing installer for the next AEN version. Can you take a look on this?
conda_info
```
(/projects/testuser11/test_public/envs/default) conda info
Current conda install:
platform : linux-64
conda version : 4.3.22
conda is private : False
conda-env version : 4.3.22
conda-build version : 3.0.6
python version : 2.7.13.final.0
requests version : 2.14.2
root environment : /opt/wakari/anaconda (read only)
default environment : /projects/testuser11/test_public/envs/default
envs directories : /projects/testuser11/test_public/envs
/projects/testuser11/test_public/DEFAULTS
/home/testuser11/.conda/envs
/opt/wakari/anaconda/envs
package cache : /opt/wakari/anaconda/pkgs
/home/testuser11/.conda/pkgs
channel URLs : https://conda.anaconda.org/r/linux-64
https://conda.anaconda.org/r/noarch
https://conda.anaconda.org/wakari/linux-64
https://conda.anaconda.org/wakari/noarch
https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
config file : /opt/wakari/anaconda/.condarc
netrc file : None
offline mode : False
user-agent : conda/4.3.22 requests/2.14.2 CPython/2.7.13 Linux/2.6.32-696.3.1.el6.x86_64 CentOS/6.9 glibc/2.12
UID:GID : 500:500
```
and `.condarc`
```
(projects/testuser11/test_public/envs/default) cat /opt/wakari/anaconda/.condarc
channels:
- r
- https://conda.anaconda.org/wakari
- defaults
create_default_packages:
- anaconda-client
- ipykernel
auto_update_conda: false
```
And when I try to create a env, it does not contain the default_packages as expected
```
(/projects/testuser11/test_public/envs/default) conda create -n bar
Fetching package metadata .............
Solving package specifications:
Package plan for installation in environment /projects/testuser11/test_public/envs/bar:
Proceed ([y]/n)? y
#
# To activate this environment, use:
# > source activate bar
#
# To deactivate an active environment, use:
# > source deactivate
#
(/projects/testuser11/test_public/envs/default) conda list -n bar
# packages in environment at /projects/testuser11/test_public/envs/bar:
#
(/projects/testuser11/test_public/envs/default) ^C
```
cc @csoja | 2017-07-20T21:28:07 | -1.0 |
conda/conda | 5,726 | conda__conda-5726 | [
"1741"
] | c1af02bb4fcfb8c962bb52d040ba705174345889 | diff --git a/conda/cli/main_list.py b/conda/cli/main_list.py
--- a/conda/cli/main_list.py
+++ b/conda/cli/main_list.py
@@ -39,6 +39,12 @@ def list_packages(prefix, installed, regex=None, format='human',
show_channel_urls=None):
res = 0
result = []
+
+ if format == 'human':
+ result.append('# packages in environment at %s:' % prefix)
+ result.append('#')
+ result.append('# %-23s %-15s %15s Channel' % ("Name", "Version", "Build"))
+
for dist in get_packages(installed, regex):
if format == 'canonical':
result.append(dist)
@@ -73,9 +79,6 @@ def print_packages(prefix, regex=None, format='human', piplist=False,
raise EnvironmentLocationNotFound(prefix)
if not json:
- if format == 'human':
- print('# packages in environment at %s:' % prefix)
- print('#')
if format == 'export':
print_export_header(context.subdir)
@@ -88,10 +91,12 @@ def print_packages(prefix, regex=None, format='human', piplist=False,
exitcode, output = list_packages(prefix, installed, regex, format=format,
show_channel_urls=show_channel_urls)
- if not json:
- print('\n'.join(map(text_type, output)))
- else:
+ if context.json:
stdout_json(output)
+
+ else:
+ print('\n'.join(map(text_type, output)))
+
return exitcode
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -546,7 +546,7 @@ def test_create_empty_env(self):
stderr = list_output[1]
expected_output = """# packages in environment at %s:
#
-
+# Name Version Build Channel
""" % prefix
self.assertEqual(stdout, expected_output)
self.assertEqual(stderr, '')
| `conda list` doesn't have column headers
The `conda list` command outputs 3 columns of data for which there are no column headers. The first appears to be `package name`, the second `version`, the third is possibly `feature+build number`? It would be great to have these headers added and could be done so in the already hash-prefixed lines so as not to upset filters/pipes relying on the package list format. Thanks.
| In addition, what would be the fourth column?
| 2017-07-25T23:02:50 | -1.0 |
conda/conda | 5,764 | conda__conda-5764 | [
"5763"
] | c60a7ab0540fa0ce3856c76c4f00a2fa15343c3a | diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -95,14 +95,14 @@ def _read_channel_configuration(scheme, host, port, path):
for name, channel in sorted(context.custom_channels.items(), reverse=True,
key=lambda x: len(x[0])):
that_test_url = join_url(channel.location, channel.name)
- if test_url.startswith(that_test_url):
+ if tokenized_startswith(test_url.split('/'), that_test_url.split('/')):
subname = test_url.replace(that_test_url, '', 1).strip('/')
return (channel.location, join_url(channel.name, subname), scheme,
channel.auth, channel.token)
# Step 5. channel_alias match
ca = context.channel_alias
- if ca.location and test_url.startswith(ca.location):
+ if ca.location and tokenized_startswith(test_url.split('/'), ca.location.split('/')):
name = test_url.replace(ca.location, '', 1).strip('/') or None
return ca.location, name, scheme, ca.auth, ca.token
| diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -887,3 +887,35 @@ def test_regression_against_unknown_none(self):
assert channel.base_url is None
assert channel.url() == defaults.url()
assert channel.urls() == defaults.urls()
+
+
+class OtherChannelParsingTests(TestCase):
+
+ @classmethod
+ def setUpClass(cls):
+ string = dals("""
+ default_channels:
+ - http://test/conda/anaconda
+ channels:
+ - http://test/conda/anaconda-cluster
+ """)
+ reset_context()
+ rd = odict(testdata=YamlRawParameter.make_raw_parameters('testdata', yaml_load(string)))
+ context._set_raw_data(rd)
+ Channel._reset_state()
+
+ cls.platform = context.subdir
+
+ @classmethod
+ def tearDownClass(cls):
+ reset_context()
+
+ def test_channels_with_dashes(self):
+ # regression test for #5763
+ assert context.channels == ('http://test/conda/anaconda-cluster',)
+ channel_urls = prioritize_channels(context.channels)
+ assert channel_urls == odict((
+ ('http://test/conda/anaconda-cluster/%s' % context.subdir, ('http://test/conda/anaconda-cluster', 0)),
+ ('http://test/conda/anaconda-cluster/noarch', ('http://test/conda/anaconda-cluster', 0)),
+ ))
+
diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -191,7 +191,7 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
@pytest.mark.installed
def test_activate_deactivate(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 8, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2017, 9, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
@@ -208,7 +208,7 @@ def test_activate_deactivate(shell):
@pytest.mark.installed
def test_activate_root_simple(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 8, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2017, 9, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -674,7 +674,7 @@ def test_update_deps_flag_absent(self):
assert package_is_installed(prefix, 'itsdangerous-0.23')
assert package_is_installed(prefix, 'flask')
- @pytest.mark.xfail(datetime.now() < datetime(2017, 8, 1), reason="#5263", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2017, 9, 1), reason="#5263", strict=True)
def test_update_deps_flag_present(self):
with make_temp_env("python=2 itsdangerous=0.23") as prefix:
assert package_is_installed(prefix, 'python-2')
diff --git a/tests/test_export.py b/tests/test_export.py
--- a/tests/test_export.py
+++ b/tests/test_export.py
@@ -31,7 +31,7 @@ def test_basic(self):
output2, error= run_command(Commands.LIST, prefix2, "-e")
self.assertEqual(output, output2)
- @pytest.mark.xfail(datetime.now() < datetime(2017, 8, 1), reason="Bring back `conda list --export` #3445", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2017, 9, 1), reason="Bring back `conda list --export` #3445", strict=True)
def test_multi_channel_export(self):
"""
When try to import from txt
| Conda mangles channels with hyphens
Update: This is not related to hyphens, only substrings.
Seen on versions: 4.3.21, 4.3.23 (maybe more versions)
Conda mangles channels that are a substring match when using default_channels and channels. The channel name "substring-channels" gets mangled into "substring/-channels". It looks like it happens when there is a substring that matches.
```
default_channels:
- http://test/conda/substring
- http://test/conda/default-channels
channels:
- http://test/conda/substring-channels
- http://test/conda/not_substring-channels
- defaults
```
Here is the output of conda info
```
Current conda install:
platform : linux-64
conda version : 4.3.23
conda is private : False
conda-env version : 4.3.23
conda-build version : 2.1.0
python version : 2.7.13.final.0
requests version : 2.12.4
root environment : /home/ec2-user/miniconda2 (writable)
default environment : /home/ec2-user/miniconda2
envs directories : /home/ec2-user/miniconda2/envs
/home/ec2-user/.conda/envs
package cache : /home/ec2-user/miniconda2/pkgs
/home/ec2-user/.conda/pkgs
channel URLs : http://test/conda/substring/-channels/linux-64 <<<<<<<<<<<<<<====
http://test/conda/substring/-channels/noarch <<<<<<<<<<<<<<====
http://test/conda/not_substring-channels/linux-64
http://test/conda/not_substring-channels/noarch
http://test/conda/substring/linux-64
http://test/conda/substring/noarch
http://test/conda/default-channels/linux-64
http://test/conda/default-channels/noarch
config file : /home/ec2-user/miniconda2/.condarc
netrc file : None
offline mode : False
user-agent : conda/4.3.23 requests/2.12.4 CPython/2.7.13 Linux/4.4.11-23.53.amzn1.x86_64 / glibc/2.17
UID:GID : 500:500
```
Also `conda info --unsafe-channels` seems to give weird output. It doesn't expand "defaults".
| I've updated this to say that it is substrings between channels and default_channels that causes this bugs, not hyphens. Here is a complete example. Substrings from default_channels cause problems with "channels".
```
default_channels:
- http://test/conda/substring <<<<< PROBLEM
- http://test/conda/default-channels
- http://test/conda/sub <<<<< PROBLEM
- http://test/conda/sub2 <<<<< Not a problem
- http://test/conda/sub2sub2 <<<<< not a problem
channels:
- http://test/conda/substring-channels
- http://test/conda/not_substring-channels
- http://test/conda/subsub
- defaults
```
It's this bit of logic: https://github.com/conda/conda/blob/master/conda/models/channel.py#L397-L404
I don't know why this does this, but that splits off anything that is a substring and joins it as a separate path component.
```
# Step 4. custom_channels matches
for name, channel in sorted(context.custom_channels.items(), reverse=True,
key=lambda x: len(x[0])):
that_test_url = join_url(channel.location, channel.name)
if test_url.startswith(that_test_url):
subname = test_url.replace(that_test_url, '', 1).strip('/')
return (channel.location, join_url(channel.name, subname), scheme,
channel.auth, channel.token)
``` | 2017-08-01T21:22:29 | -1.0 |
conda/conda | 5,831 | conda__conda-5831 | [
"5825"
] | 4f623168b74673105f866c35b1d33aa80f6a6bda | diff --git a/conda/cli/common.py b/conda/cli/common.py
--- a/conda/cli/common.py
+++ b/conda/cli/common.py
@@ -8,7 +8,6 @@
from .._vendor.auxlib.ish import dals
from ..base.constants import PREFIX_MAGIC_FILE, ROOT_ENV_NAME
from ..base.context import context
-from ..common.compat import itervalues
from ..models.match_spec import MatchSpec
@@ -165,19 +164,6 @@ def stdout_json(d):
def stdout_json_success(success=True, **kwargs):
result = {'success': success}
-
- # this code reverts json output for plan back to previous behavior
- # relied on by Anaconda Navigator and nb_conda
- unlink_link_transaction = kwargs.pop('unlink_link_transaction', None)
- if unlink_link_transaction:
- from .._vendor.toolz.itertoolz import concat
- actions = kwargs.setdefault('actions', {})
- actions['LINK'] = tuple(d.dist_str() for d in concat(
- stp.link_precs for stp in itervalues(unlink_link_transaction.prefix_setups)
- ))
- actions['UNLINK'] = tuple(d.dist_str() for d in concat(
- stp.unlink_precs for stp in itervalues(unlink_link_transaction.prefix_setups)
- ))
result.update(kwargs)
stdout_json(result)
diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -261,8 +261,8 @@ def handle_txn(progressive_fetch_extract, unlink_link_transaction, prefix, args,
common.confirm_yn()
elif context.dry_run:
- common.stdout_json_success(unlink_link_transaction=unlink_link_transaction, prefix=prefix,
- dry_run=True)
+ actions = unlink_link_transaction.make_legacy_action_groups(progressive_fetch_extract)[0]
+ common.stdout_json_success(prefix=prefix, actions=actions, dry_run=True)
raise DryRunExit()
try:
@@ -279,4 +279,4 @@ def handle_txn(progressive_fetch_extract, unlink_link_transaction, prefix, args,
if context.json:
actions = unlink_link_transaction.make_legacy_action_groups(progressive_fetch_extract)[0]
- common.stdout_json_success(actions=actions)
+ common.stdout_json_success(prefix=prefix, actions=actions)
diff --git a/conda/core/link.py b/conda/core/link.py
--- a/conda/core/link.py
+++ b/conda/core/link.py
@@ -672,6 +672,8 @@ def make_link_actions(transaction_context, package_info, target_prefix, requeste
))
def make_legacy_action_groups(self, pfe):
+ # this code reverts json output for plan back to previous behavior
+ # relied on by Anaconda Navigator and nb_conda
from ..models.dist import Dist
legacy_action_groups = []
@@ -684,9 +686,9 @@ def make_legacy_action_groups(self, pfe):
actions['PREFIX'] = setup.target_prefix
for prec in setup.unlink_precs:
- actions['UNLINK'].append(prec)
+ actions['UNLINK'].append(Dist(prec))
for prec in setup.link_precs:
- actions['LINK'].append(prec)
+ actions['LINK'].append(Dist(prec))
legacy_action_groups.append(actions)
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -374,9 +374,22 @@ def assert_json_parsable(content):
try:
prefix = make_temp_prefix(str(uuid4())[:7])
+ stdout, stderr = run_command(Commands.CREATE, prefix, "python=3.5 --json --dry-run", use_exception_handler=True)
+ assert_json_parsable(stdout)
+ assert not stderr
+
+ # regression test for #5825
+ # contents of LINK and UNLINK is expected to have Dist format
+ json_obj = json.loads(stdout)
+ dist_dump = json_obj['actions']['LINK'][0]
+ assert 'dist_name' in dist_dump
+
stdout, stderr = run_command(Commands.CREATE, prefix, "python=3.5 --json")
assert_json_parsable(stdout)
assert not stderr
+ json_obj = json.loads(stdout)
+ dist_dump = json_obj['actions']['LINK'][0]
+ assert 'dist_name' in dist_dump
stdout, stderr = run_command(Commands.INSTALL, prefix, 'flask=0.10 --json')
assert_json_parsable(stdout)
@@ -404,6 +417,12 @@ def assert_json_parsable(content):
assert not package_is_installed(prefix, 'flask-0.')
assert_package_is_installed(prefix, 'python-3')
+ # regression test for #5825
+ # contents of LINK and UNLINK is expected to have Dist format
+ json_obj = json.loads(stdout)
+ dist_dump = json_obj['actions']['UNLINK'][0]
+ assert 'dist_name' in dist_dump
+
stdout, stderr = run_command(Commands.LIST, prefix, '--revisions --json')
assert not stderr
json_obj = json.loads(stdout)
@@ -1037,8 +1056,9 @@ def test_create_dry_run_json(self):
stdout, stderr = run_command(Commands.CREATE, prefix, "flask", "--dry-run", "--json", use_exception_handler=True)
loaded = json.loads(stdout)
- assert "python" in "\n".join(loaded['actions']['LINK'])
- assert "flask" in "\n".join(loaded['actions']['LINK'])
+ names = set(d['name'] for d in loaded['actions']['LINK'])
+ assert "python" in names
+ assert "flask" in names
def test_packages_not_found(self):
with make_temp_env() as prefix:
| Conda 4.4 --json --dry-run returns a different format
Could be a feature... or a bug. These breaking changes should probably be reserved for major versions? So Bug it is :-p
## Conda 4.4
```
$ conda install rstudio --dry-run --json
{
"actions": {
"LINK": [
"defaults::font-ttf-dejavu-sans-mono-2.37-0",
"defaults::font-ttf-inconsolata-2.000-0",
"defaults::font-ttf-source-code-pro-2.030-0",
"defaults::font-ttf-ubuntu-0.83-0",
"defaults::krb5-1.13.2-0",
"defaults::libffi-3.2.1-1",
"defaults::libgcc-4.8.5-1",
"defaults::ncurses-5.9-10",
"defaults::pixman-0.34.0-0",
"defaults::zeromq-4.1.3-0",
"defaults::fonts-continuum-1-0",
"defaults::gettext-0.19.8-1",
"defaults::gsl-2.2.1-0",
"defaults::libssh2-1.8.0-0",
"defaults::pandoc-1.19.2.1-0",
"defaults::pcre-8.39-1",
"defaults::curl-7.54.1-0",
"defaults::glib-2.50.2-1",
"defaults::fontconfig-2.12.1-3",
"defaults::cairo-1.14.8-0",
"defaults::harfbuzz-0.9.39-2",
"defaults::pango-1.40.3-1",
"defaults::r-base-3.4.1-0",
"defaults::r-assertthat-0.2.0-r3.4.1_0",
"defaults::r-backports-1.1.0-r3.4.1_0",
"defaults::r-base64enc-0.1_3-r3.4.1_0",
"defaults::r-bh-1.62.0_1-r3.4.1_0",
"defaults::r-bindr-0.1-r3.4.1_0",
"defaults::r-bitops-1.0_6-r3.4.1_2",
"defaults::r-boot-1.3_19-r3.4.1_0",
"defaults::r-cluster-2.0.6-r3.4.1_0",
"defaults::r-codetools-0.2_15-r3.4.1_0",
"defaults::r-colorspace-1.3_2-r3.4.1_0",
"defaults::r-crayon-1.3.2-r3.4.1_0",
"defaults::r-curl-2.6-r3.4.1_0",
"defaults::r-data.table-1.10.4-r3.4.1_0",
"defaults::r-dbi-0.6_1-r3.4.1_0",
"defaults::r-dichromat-2.0_0-r3.4.1_2",
"defaults::r-digest-0.6.12-r3.4.1_0",
"defaults::r-foreign-0.8_68-r3.4.1_0",
"defaults::r-formatr-1.5-r3.4.1_0",
"defaults::r-glue-1.1.1-r3.4.1_0",
"defaults::r-gtable-0.2.0-r3.4.1_0",
"defaults::r-highr-0.6-r3.4.1_0",
"defaults::r-hms-0.3-r3.4.1_0",
"defaults::r-iterators-1.0.8-r3.4.1_0",
"defaults::r-jsonlite-1.5-r3.4.1_0",
"defaults::r-kernsmooth-2.23_15-r3.4.1_0",
"defaults::r-labeling-0.3-r3.4.1_2",
"defaults::r-lattice-0.20_35-r3.4.1_0",
"defaults::r-lazyeval-0.2.0-r3.4.1_0",
"defaults::r-magrittr-1.5-r3.4.1_2",
"defaults::r-maps-3.2.0-r3.4.1_0",
"defaults::r-mass-7.3_47-r3.4.1_0",
"defaults::r-mime-0.5-r3.4.1_0",
"defaults::r-mnormt-1.5_5-r3.4.1_0",
"defaults::r-nloptr-1.0.4-r3.4.1_2",
"defaults::r-nnet-7.3_12-r3.4.1_0",
"defaults::r-openssl-0.9.6-r3.4.1_0",
"defaults::r-packrat-0.4.8_1-r3.4.1_0",
"defaults::r-pkgconfig-2.0.1-r3.4.1_0",
"defaults::r-plogr-0.1_1-r3.4.1_0",
"defaults::r-r6-2.2.1-r3.4.1_0",
"defaults::r-randomforest-4.6_12-r3.4.1_0",
"defaults::r-rcolorbrewer-1.1_2-r3.4.1_3",
"defaults::r-rcpp-0.12.11-r3.4.1_0",
"defaults::r-rematch-1.0.1-r3.4.1_0",
"defaults::r-repr-0.10-r3.4.1_0",
"defaults::r-rjsonio-1.3_0-r3.4.1_2",
"defaults::r-rlang-0.1.1-r3.4.1_0",
"defaults::r-rpart-4.1_11-r3.4.1_0",
"defaults::r-rstudioapi-0.6-r3.4.1_0",
"defaults::r-sourcetools-0.1.6-r3.4.1_0",
"defaults::r-sparsem-1.77-r3.4.1_0",
"defaults::r-spatial-7.3_11-r3.4.1_0",
"defaults::r-stringi-1.1.5-r3.4.1_0",
"defaults::r-uuid-0.1_2-r3.4.1_0",
"defaults::r-xtable-1.8_2-r3.4.1_0",
"defaults::r-yaml-2.1.14-r3.4.1_0",
"defaults::r-bindrcpp-0.2-r3.4.1_0",
"defaults::r-catools-1.17.1-r3.4.1_2",
"defaults::r-class-7.3_14-r3.4.1_0",
"defaults::r-foreach-1.4.3-r3.4.1_0",
"defaults::r-hexbin-1.27.1-r3.4.1_0",
"defaults::r-htmltools-0.3.6-r3.4.1_0",
"defaults::r-httpuv-1.3.3-r3.4.1_0",
"defaults::r-httr-1.2.1-r3.4.1_0",
"defaults::r-irdisplay-0.4.4-r3.4.1_0",
"defaults::r-markdown-0.8-r3.4.1_0",
"defaults::r-matrix-1.2_10-r3.4.1_0",
"defaults::r-minqa-1.2.4-r3.4.1_2",
"defaults::r-modelmetrics-1.1.0-r3.4.1_0",
"defaults::r-munsell-0.4.3-r3.4.1_0",
"defaults::r-nlme-3.1_131-r3.4.1_0",
"defaults::r-pbdzmq-0.2_6-r3.4.1_0",
"defaults::r-pki-0.1_3-r3.4.1_0",
"defaults::r-plyr-1.8.4-r3.4.1_0",
"defaults::r-rcurl-1.95_4.8-r3.4.1_0",
"defaults::r-rprojroot-1.2-r3.4.1_0",
"defaults::r-stringr-1.2.0-r3.4.1_0",
"defaults::r-tibble-1.3.3-r3.4.1_0",
"defaults::r-xml2-1.1.1-r3.4.1_0",
"defaults::r-zoo-1.8_0-r3.4.1_0",
"defaults::r-cellranger-1.1.0-r3.4.1_0",
"defaults::r-dplyr-0.7.0-r3.4.1_0",
"defaults::r-evaluate-0.10-r3.4.1_0",
"defaults::r-forcats-0.2.0-r3.4.1_0",
"defaults::r-glmnet-2.0_10-r3.4.1_0",
"defaults::r-htmlwidgets-0.8-r3.4.1_1",
"defaults::r-lubridate-1.6.0-r3.4.1_0",
"defaults::r-matrixmodels-0.4_1-r3.4.1_0",
"defaults::r-mgcv-1.8_17-r3.4.1_0",
"defaults::r-pryr-0.1.2-r3.4.1_0",
"defaults::r-psych-1.7.5-r3.4.1_0",
"defaults::r-purrr-0.2.2.2-r3.4.1_0",
"defaults::r-rcppeigen-0.3.3.3.0-r3.4.1_0",
"defaults::r-readr-1.1.1-r3.4.1_0",
"defaults::r-reshape2-1.4.2-r3.4.1_0",
"defaults::r-rsconnect-0.8-r3.4.1_0",
"defaults::r-scales-0.4.1-r3.4.1_0",
"defaults::r-selectr-0.3_1-r3.4.1_0",
"defaults::r-shiny-1.0.3-r3.4.1_0",
"defaults::r-survival-2.41_3-r3.4.1_0",
"defaults::r-xts-0.9_7-r3.4.1_2",
"defaults::r-ggplot2-2.2.1-r3.4.1_0",
"defaults::r-haven-1.0.0-r3.4.1_0",
"defaults::r-irkernel-0.7.1-r3.4.1_0",
"defaults::r-knitr-1.16-r3.4.1_0",
"defaults::r-lme4-1.1_13-r3.4.1_0",
"defaults::r-quantreg-5.33-r3.4.1_0",
"defaults::r-readxl-1.0.0-r3.4.1_0",
"defaults::r-recommended-3.4.1-r3.4.1_0",
"defaults::r-rvest-0.3.2-r3.4.1_0",
"defaults::r-tidyr-0.6.3-r3.4.1_0",
"defaults::r-ttr-0.23_1-r3.4.1_0",
"defaults::r-broom-0.4.2-r3.4.1_0",
"defaults::r-pbkrtest-0.4_7-r3.4.1_0",
"defaults::r-quantmod-0.4_9-r3.4.1_0",
"defaults::r-rmarkdown-1.5-r3.4.1_0",
"defaults::r-car-2.1_4-r3.4.1_0",
"defaults::r-gistr-0.4.0-r3.4.1_0",
"defaults::r-modelr-0.1.0-r3.4.1_0",
"defaults::r-caret-6.0_76-r3.4.1_0",
"defaults::r-rbokeh-0.5.0-r3.4.1_0",
"defaults::r-tidyverse-1.1.1-r3.4.1_0",
"defaults::r-essentials-1.6.0-r3.4.1_0",
"defaults::rstudio-1.0.153-1"
],
"UNLINK": []
},
"dry_run": true,
"prefix": "/Users/gpena-castellanos/anaconda/envs/py36",
"success": true
}
```
## Conda 4.3
```
$ conda install rstudio --dry-run --json
{
"actions": [
{
"LINK": [
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "font-ttf-dejavu-sans-mono-2.37-0",
"name": "font-ttf-dejavu-sans-mono",
"platform": null,
"version": "2.37",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "font-ttf-inconsolata-2.000-0",
"name": "font-ttf-inconsolata",
"platform": null,
"version": "2.000",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "font-ttf-source-code-pro-2.030-0",
"name": "font-ttf-source-code-pro",
"platform": null,
"version": "2.030",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "font-ttf-ubuntu-0.83-0",
"name": "font-ttf-ubuntu",
"platform": null,
"version": "0.83",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "krb5-1.13.2-0",
"name": "krb5",
"platform": null,
"version": "1.13.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "libffi-3.2.1-1",
"name": "libffi",
"platform": null,
"version": "3.2.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "libgcc-4.8.5-1",
"name": "libgcc",
"platform": null,
"version": "4.8.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 10,
"build_string": "10",
"channel": "defaults",
"dist_name": "ncurses-5.9-10",
"name": "ncurses",
"platform": null,
"version": "5.9",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "pixman-0.34.0-0",
"name": "pixman",
"platform": null,
"version": "0.34.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "zeromq-4.1.3-0",
"name": "zeromq",
"platform": null,
"version": "4.1.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "fonts-continuum-1-0",
"name": "fonts-continuum",
"platform": null,
"version": "1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "gettext-0.19.8-1",
"name": "gettext",
"platform": null,
"version": "0.19.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "gsl-2.2.1-0",
"name": "gsl",
"platform": null,
"version": "2.2.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "libssh2-1.8.0-0",
"name": "libssh2",
"platform": null,
"version": "1.8.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "pandoc-1.19.2.1-0",
"name": "pandoc",
"platform": null,
"version": "1.19.2.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "pcre-8.39-1",
"name": "pcre",
"platform": null,
"version": "8.39",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "curl-7.54.1-0",
"name": "curl",
"platform": null,
"version": "7.54.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "glib-2.50.2-1",
"name": "glib",
"platform": null,
"version": "2.50.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 3,
"build_string": "3",
"channel": "defaults",
"dist_name": "fontconfig-2.12.1-3",
"name": "fontconfig",
"platform": null,
"version": "2.12.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "cairo-1.14.8-0",
"name": "cairo",
"platform": null,
"version": "1.14.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "2",
"channel": "defaults",
"dist_name": "harfbuzz-0.9.39-2",
"name": "harfbuzz",
"platform": null,
"version": "0.9.39",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "pango-1.40.3-1",
"name": "pango",
"platform": null,
"version": "1.40.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "0",
"channel": "defaults",
"dist_name": "r-base-3.4.1-0",
"name": "r-base",
"platform": null,
"version": "3.4.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-assertthat-0.2.0-r3.4.1_0",
"name": "r-assertthat",
"platform": null,
"version": "0.2.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-backports-1.1.0-r3.4.1_0",
"name": "r-backports",
"platform": null,
"version": "1.1.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-base64enc-0.1_3-r3.4.1_0",
"name": "r-base64enc",
"platform": null,
"version": "0.1_3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-bh-1.62.0_1-r3.4.1_0",
"name": "r-bh",
"platform": null,
"version": "1.62.0_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-bindr-0.1-r3.4.1_0",
"name": "r-bindr",
"platform": null,
"version": "0.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-bitops-1.0_6-r3.4.1_2",
"name": "r-bitops",
"platform": null,
"version": "1.0_6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-boot-1.3_19-r3.4.1_0",
"name": "r-boot",
"platform": null,
"version": "1.3_19",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-cluster-2.0.6-r3.4.1_0",
"name": "r-cluster",
"platform": null,
"version": "2.0.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-codetools-0.2_15-r3.4.1_0",
"name": "r-codetools",
"platform": null,
"version": "0.2_15",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-colorspace-1.3_2-r3.4.1_0",
"name": "r-colorspace",
"platform": null,
"version": "1.3_2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-crayon-1.3.2-r3.4.1_0",
"name": "r-crayon",
"platform": null,
"version": "1.3.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-curl-2.6-r3.4.1_0",
"name": "r-curl",
"platform": null,
"version": "2.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-data.table-1.10.4-r3.4.1_0",
"name": "r-data.table",
"platform": null,
"version": "1.10.4",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-dbi-0.6_1-r3.4.1_0",
"name": "r-dbi",
"platform": null,
"version": "0.6_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-dichromat-2.0_0-r3.4.1_2",
"name": "r-dichromat",
"platform": null,
"version": "2.0_0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-digest-0.6.12-r3.4.1_0",
"name": "r-digest",
"platform": null,
"version": "0.6.12",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-foreign-0.8_68-r3.4.1_0",
"name": "r-foreign",
"platform": null,
"version": "0.8_68",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-formatr-1.5-r3.4.1_0",
"name": "r-formatr",
"platform": null,
"version": "1.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-glue-1.1.1-r3.4.1_0",
"name": "r-glue",
"platform": null,
"version": "1.1.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-gtable-0.2.0-r3.4.1_0",
"name": "r-gtable",
"platform": null,
"version": "0.2.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-highr-0.6-r3.4.1_0",
"name": "r-highr",
"platform": null,
"version": "0.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-hms-0.3-r3.4.1_0",
"name": "r-hms",
"platform": null,
"version": "0.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-iterators-1.0.8-r3.4.1_0",
"name": "r-iterators",
"platform": null,
"version": "1.0.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-jsonlite-1.5-r3.4.1_0",
"name": "r-jsonlite",
"platform": null,
"version": "1.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-kernsmooth-2.23_15-r3.4.1_0",
"name": "r-kernsmooth",
"platform": null,
"version": "2.23_15",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-labeling-0.3-r3.4.1_2",
"name": "r-labeling",
"platform": null,
"version": "0.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-lattice-0.20_35-r3.4.1_0",
"name": "r-lattice",
"platform": null,
"version": "0.20_35",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-lazyeval-0.2.0-r3.4.1_0",
"name": "r-lazyeval",
"platform": null,
"version": "0.2.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-magrittr-1.5-r3.4.1_2",
"name": "r-magrittr",
"platform": null,
"version": "1.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-maps-3.2.0-r3.4.1_0",
"name": "r-maps",
"platform": null,
"version": "3.2.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-mass-7.3_47-r3.4.1_0",
"name": "r-mass",
"platform": null,
"version": "7.3_47",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-mime-0.5-r3.4.1_0",
"name": "r-mime",
"platform": null,
"version": "0.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-mnormt-1.5_5-r3.4.1_0",
"name": "r-mnormt",
"platform": null,
"version": "1.5_5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-nloptr-1.0.4-r3.4.1_2",
"name": "r-nloptr",
"platform": null,
"version": "1.0.4",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-nnet-7.3_12-r3.4.1_0",
"name": "r-nnet",
"platform": null,
"version": "7.3_12",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-openssl-0.9.6-r3.4.1_0",
"name": "r-openssl",
"platform": null,
"version": "0.9.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-packrat-0.4.8_1-r3.4.1_0",
"name": "r-packrat",
"platform": null,
"version": "0.4.8_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-pkgconfig-2.0.1-r3.4.1_0",
"name": "r-pkgconfig",
"platform": null,
"version": "2.0.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-plogr-0.1_1-r3.4.1_0",
"name": "r-plogr",
"platform": null,
"version": "0.1_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-r6-2.2.1-r3.4.1_0",
"name": "r-r6",
"platform": null,
"version": "2.2.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-randomforest-4.6_12-r3.4.1_0",
"name": "r-randomforest",
"platform": null,
"version": "4.6_12",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 3,
"build_string": "r3.4.1_3",
"channel": "defaults",
"dist_name": "r-rcolorbrewer-1.1_2-r3.4.1_3",
"name": "r-rcolorbrewer",
"platform": null,
"version": "1.1_2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rcpp-0.12.11-r3.4.1_0",
"name": "r-rcpp",
"platform": null,
"version": "0.12.11",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rematch-1.0.1-r3.4.1_0",
"name": "r-rematch",
"platform": null,
"version": "1.0.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-repr-0.10-r3.4.1_0",
"name": "r-repr",
"platform": null,
"version": "0.10",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-rjsonio-1.3_0-r3.4.1_2",
"name": "r-rjsonio",
"platform": null,
"version": "1.3_0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rlang-0.1.1-r3.4.1_0",
"name": "r-rlang",
"platform": null,
"version": "0.1.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rpart-4.1_11-r3.4.1_0",
"name": "r-rpart",
"platform": null,
"version": "4.1_11",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rstudioapi-0.6-r3.4.1_0",
"name": "r-rstudioapi",
"platform": null,
"version": "0.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-sourcetools-0.1.6-r3.4.1_0",
"name": "r-sourcetools",
"platform": null,
"version": "0.1.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-sparsem-1.77-r3.4.1_0",
"name": "r-sparsem",
"platform": null,
"version": "1.77",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-spatial-7.3_11-r3.4.1_0",
"name": "r-spatial",
"platform": null,
"version": "7.3_11",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-stringi-1.1.5-r3.4.1_0",
"name": "r-stringi",
"platform": null,
"version": "1.1.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-uuid-0.1_2-r3.4.1_0",
"name": "r-uuid",
"platform": null,
"version": "0.1_2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-xtable-1.8_2-r3.4.1_0",
"name": "r-xtable",
"platform": null,
"version": "1.8_2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-yaml-2.1.14-r3.4.1_0",
"name": "r-yaml",
"platform": null,
"version": "2.1.14",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-bindrcpp-0.2-r3.4.1_0",
"name": "r-bindrcpp",
"platform": null,
"version": "0.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-catools-1.17.1-r3.4.1_2",
"name": "r-catools",
"platform": null,
"version": "1.17.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-class-7.3_14-r3.4.1_0",
"name": "r-class",
"platform": null,
"version": "7.3_14",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-foreach-1.4.3-r3.4.1_0",
"name": "r-foreach",
"platform": null,
"version": "1.4.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-hexbin-1.27.1-r3.4.1_0",
"name": "r-hexbin",
"platform": null,
"version": "1.27.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-htmltools-0.3.6-r3.4.1_0",
"name": "r-htmltools",
"platform": null,
"version": "0.3.6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-httpuv-1.3.3-r3.4.1_0",
"name": "r-httpuv",
"platform": null,
"version": "1.3.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-httr-1.2.1-r3.4.1_0",
"name": "r-httr",
"platform": null,
"version": "1.2.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-irdisplay-0.4.4-r3.4.1_0",
"name": "r-irdisplay",
"platform": null,
"version": "0.4.4",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-markdown-0.8-r3.4.1_0",
"name": "r-markdown",
"platform": null,
"version": "0.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-matrix-1.2_10-r3.4.1_0",
"name": "r-matrix",
"platform": null,
"version": "1.2_10",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-minqa-1.2.4-r3.4.1_2",
"name": "r-minqa",
"platform": null,
"version": "1.2.4",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-modelmetrics-1.1.0-r3.4.1_0",
"name": "r-modelmetrics",
"platform": null,
"version": "1.1.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-munsell-0.4.3-r3.4.1_0",
"name": "r-munsell",
"platform": null,
"version": "0.4.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-nlme-3.1_131-r3.4.1_0",
"name": "r-nlme",
"platform": null,
"version": "3.1_131",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-pbdzmq-0.2_6-r3.4.1_0",
"name": "r-pbdzmq",
"platform": null,
"version": "0.2_6",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-pki-0.1_3-r3.4.1_0",
"name": "r-pki",
"platform": null,
"version": "0.1_3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-plyr-1.8.4-r3.4.1_0",
"name": "r-plyr",
"platform": null,
"version": "1.8.4",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rcurl-1.95_4.8-r3.4.1_0",
"name": "r-rcurl",
"platform": null,
"version": "1.95_4.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rprojroot-1.2-r3.4.1_0",
"name": "r-rprojroot",
"platform": null,
"version": "1.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-stringr-1.2.0-r3.4.1_0",
"name": "r-stringr",
"platform": null,
"version": "1.2.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-tibble-1.3.3-r3.4.1_0",
"name": "r-tibble",
"platform": null,
"version": "1.3.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-xml2-1.1.1-r3.4.1_0",
"name": "r-xml2",
"platform": null,
"version": "1.1.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-zoo-1.8_0-r3.4.1_0",
"name": "r-zoo",
"platform": null,
"version": "1.8_0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-cellranger-1.1.0-r3.4.1_0",
"name": "r-cellranger",
"platform": null,
"version": "1.1.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-dplyr-0.7.0-r3.4.1_0",
"name": "r-dplyr",
"platform": null,
"version": "0.7.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-evaluate-0.10-r3.4.1_0",
"name": "r-evaluate",
"platform": null,
"version": "0.10",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-forcats-0.2.0-r3.4.1_0",
"name": "r-forcats",
"platform": null,
"version": "0.2.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-glmnet-2.0_10-r3.4.1_0",
"name": "r-glmnet",
"platform": null,
"version": "2.0_10",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "r3.4.1_1",
"channel": "defaults",
"dist_name": "r-htmlwidgets-0.8-r3.4.1_1",
"name": "r-htmlwidgets",
"platform": null,
"version": "0.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-lubridate-1.6.0-r3.4.1_0",
"name": "r-lubridate",
"platform": null,
"version": "1.6.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-matrixmodels-0.4_1-r3.4.1_0",
"name": "r-matrixmodels",
"platform": null,
"version": "0.4_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-mgcv-1.8_17-r3.4.1_0",
"name": "r-mgcv",
"platform": null,
"version": "1.8_17",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-pryr-0.1.2-r3.4.1_0",
"name": "r-pryr",
"platform": null,
"version": "0.1.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-psych-1.7.5-r3.4.1_0",
"name": "r-psych",
"platform": null,
"version": "1.7.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-purrr-0.2.2.2-r3.4.1_0",
"name": "r-purrr",
"platform": null,
"version": "0.2.2.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rcppeigen-0.3.3.3.0-r3.4.1_0",
"name": "r-rcppeigen",
"platform": null,
"version": "0.3.3.3.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-readr-1.1.1-r3.4.1_0",
"name": "r-readr",
"platform": null,
"version": "1.1.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-reshape2-1.4.2-r3.4.1_0",
"name": "r-reshape2",
"platform": null,
"version": "1.4.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rsconnect-0.8-r3.4.1_0",
"name": "r-rsconnect",
"platform": null,
"version": "0.8",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-scales-0.4.1-r3.4.1_0",
"name": "r-scales",
"platform": null,
"version": "0.4.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-selectr-0.3_1-r3.4.1_0",
"name": "r-selectr",
"platform": null,
"version": "0.3_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-shiny-1.0.3-r3.4.1_0",
"name": "r-shiny",
"platform": null,
"version": "1.0.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-survival-2.41_3-r3.4.1_0",
"name": "r-survival",
"platform": null,
"version": "2.41_3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 2,
"build_string": "r3.4.1_2",
"channel": "defaults",
"dist_name": "r-xts-0.9_7-r3.4.1_2",
"name": "r-xts",
"platform": null,
"version": "0.9_7",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-ggplot2-2.2.1-r3.4.1_0",
"name": "r-ggplot2",
"platform": null,
"version": "2.2.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-haven-1.0.0-r3.4.1_0",
"name": "r-haven",
"platform": null,
"version": "1.0.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-irkernel-0.7.1-r3.4.1_0",
"name": "r-irkernel",
"platform": null,
"version": "0.7.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-knitr-1.16-r3.4.1_0",
"name": "r-knitr",
"platform": null,
"version": "1.16",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-lme4-1.1_13-r3.4.1_0",
"name": "r-lme4",
"platform": null,
"version": "1.1_13",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-quantreg-5.33-r3.4.1_0",
"name": "r-quantreg",
"platform": null,
"version": "5.33",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-readxl-1.0.0-r3.4.1_0",
"name": "r-readxl",
"platform": null,
"version": "1.0.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-recommended-3.4.1-r3.4.1_0",
"name": "r-recommended",
"platform": null,
"version": "3.4.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rvest-0.3.2-r3.4.1_0",
"name": "r-rvest",
"platform": null,
"version": "0.3.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-tidyr-0.6.3-r3.4.1_0",
"name": "r-tidyr",
"platform": null,
"version": "0.6.3",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-ttr-0.23_1-r3.4.1_0",
"name": "r-ttr",
"platform": null,
"version": "0.23_1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-broom-0.4.2-r3.4.1_0",
"name": "r-broom",
"platform": null,
"version": "0.4.2",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-pbkrtest-0.4_7-r3.4.1_0",
"name": "r-pbkrtest",
"platform": null,
"version": "0.4_7",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-quantmod-0.4_9-r3.4.1_0",
"name": "r-quantmod",
"platform": null,
"version": "0.4_9",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rmarkdown-1.5-r3.4.1_0",
"name": "r-rmarkdown",
"platform": null,
"version": "1.5",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-car-2.1_4-r3.4.1_0",
"name": "r-car",
"platform": null,
"version": "2.1_4",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-gistr-0.4.0-r3.4.1_0",
"name": "r-gistr",
"platform": null,
"version": "0.4.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-modelr-0.1.0-r3.4.1_0",
"name": "r-modelr",
"platform": null,
"version": "0.1.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-caret-6.0_76-r3.4.1_0",
"name": "r-caret",
"platform": null,
"version": "6.0_76",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-rbokeh-0.5.0-r3.4.1_0",
"name": "r-rbokeh",
"platform": null,
"version": "0.5.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-tidyverse-1.1.1-r3.4.1_0",
"name": "r-tidyverse",
"platform": null,
"version": "1.1.1",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 0,
"build_string": "r3.4.1_0",
"channel": "defaults",
"dist_name": "r-essentials-1.6.0-r3.4.1_0",
"name": "r-essentials",
"platform": null,
"version": "1.6.0",
"with_features_depends": null
},
{
"base_url": null,
"build_number": 1,
"build_string": "1",
"channel": "defaults",
"dist_name": "rstudio-1.0.153-1",
"name": "rstudio",
"platform": null,
"version": "1.0.153",
"with_features_depends": null
}
],
"PREFIX": "/Users/gpena-castellanos/anaconda/envs/py36",
"SYMLINK_CONDA": [
"/Users/gpena-castellanos/anaconda"
],
"op_order": [
"CHECK_FETCH",
"RM_FETCHED",
"FETCH",
"CHECK_EXTRACT",
"RM_EXTRACTED",
"EXTRACT",
"UNLINK",
"LINK",
"SYMLINK_CONDA"
]
}
],
"dry_run": true,
"success": true
}
```
| 2017-08-15T21:51:50 | -1.0 |
|
conda/conda | 5,846 | conda__conda-5846 | [
"5847"
] | d3b47d7b9ee483ba894a385e99361fa13ca9444b | diff --git a/conda/gateways/disk/delete.py b/conda/gateways/disk/delete.py
--- a/conda/gateways/disk/delete.py
+++ b/conda/gateways/disk/delete.py
@@ -30,16 +30,12 @@ def rm_rf(path, max_retries=5, trash=True):
path = abspath(path)
log.trace("rm_rf %s", path)
if isdir(path) and not islink(path):
- try:
- # On Windows, always move to trash first.
- if trash and on_win:
- move_result = move_path_to_trash(path, preclean=False)
- if move_result:
- return True
- backoff_rmdir(path)
- finally:
- from ...core.linked_data import delete_prefix_from_linked_data
- delete_prefix_from_linked_data(path)
+ # On Windows, always move to trash first.
+ if trash and on_win:
+ move_result = move_path_to_trash(path, preclean=False)
+ if move_result:
+ return True
+ backoff_rmdir(path)
elif lexists(path):
try:
backoff_unlink(path)
@@ -51,11 +47,12 @@ def rm_rf(path, max_retries=5, trash=True):
if move_result:
return True
log.info("Failed to remove %s.", path)
-
else:
log.trace("rm_rf failed. Not a link, file, or directory: %s", path)
return True
finally:
+ from ...core.linked_data import delete_prefix_from_linked_data
+ delete_prefix_from_linked_data(path)
if lexists(path):
log.info("rm_rf failed for %s", path)
return False
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -431,6 +431,12 @@ def test_list_with_pip_egg(self):
assert any(line.endswith("<pip>") for line in stdout_lines
if line.lower().startswith("flask"))
+ # regression test for #5847
+ # when using rm_rf on a directory
+ assert prefix in linked_data_
+ rm_rf(join(prefix, get_python_site_packages_short_path("3.5")))
+ assert prefix not in linked_data_
+
def test_list_with_pip_wheel(self):
with make_temp_env("python=3.5 pip") as prefix:
check_call(PYTHON_BINARY + " -m pip install flask==0.10.1",
@@ -444,6 +450,12 @@ def test_list_with_pip_wheel(self):
run_command(Commands.INSTALL, prefix, "python=3.4")
assert_package_is_installed(prefix, 'python-3.4.')
+ # regression test for #5847
+ # when using rm_rf on a file
+ assert prefix in linked_data_
+ rm_rf(join(prefix, get_python_site_packages_short_path("3.4")), "os.py")
+ assert prefix not in linked_data_
+
def test_install_tarball_from_local_channel(self):
# Regression test for #2812
# install from local channel
| Problem: conda-build doesn't clean up temporary files
@gabm commented on [Thu Aug 17 2017](https://github.com/conda/conda-build/issues/2289)
It seems conda-build doesn't clean up temporary files located under `/tmp/tmpxxxxxx` or `User/../AppData/Local/tmpxxxxxx` on windows. After the build these folders contain the decompressed packages that I suppose have been installed as dependencies during the build. Maybe its also a conda problem?!
Anyway, on our Windows build machine we accumulated 25Gb within some weeks ....
---
@gabm commented on [Thu Aug 17 2017](https://github.com/conda/conda-build/issues/2289#issuecomment-323134590)
.. the same files are located again in `miniconda3/pkgs`...
---
@msarahan commented on [Thu Aug 17 2017](https://github.com/conda/conda-build/issues/2289#issuecomment-323158750)
Conda-build uses this functionality in conda for temporary folders: https://github.com/conda/conda/blob/dbe99de1d45206c5d6f2e19017a9e2466f81dc6d/conda/compat.py#L23-L67
When we get files from packages, we do so with the package_has_file function: https://github.com/conda/conda-build/blob/master/conda_build/utils.py#L714-L730
package_has_file does not ever extract the whole package. This seems like a conda issue. Sorry, I don't currently have time to investigate this more. I can move this issue to the conda repo if you'd like.
---
@gabm commented on [Thu Aug 17 2017](https://github.com/conda/conda-build/issues/2289#issuecomment-323170508)
ok, then please move it there... If I find time, I'll have a further look. I realised some strange folders `/tmp/tmpxxxxxxx` some weeks ago, so could be easy to reproduce...
| 2017-08-17T16:44:03 | -1.0 |
|
conda/conda | 5,923 | conda__conda-5923 | [
"5922"
] | 40037889fba4b0afb4a9d60d04ed6014637632a6 | diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -4,8 +4,7 @@
from itertools import chain
from logging import getLogger
-from ..base.constants import (DEFAULTS_CHANNEL_NAME, DEFAULT_CHANNELS_UNIX, DEFAULT_CHANNELS_WIN,
- MAX_CHANNEL_PRIORITY, UNKNOWN_CHANNEL)
+from ..base.constants import (DEFAULTS_CHANNEL_NAME, MAX_CHANNEL_PRIORITY, UNKNOWN_CHANNEL)
from ..base.context import context
from ..common.compat import ensure_text_type, isiterable, iteritems, odict, with_metaclass
from ..common.path import is_path, win_path_backout
@@ -14,10 +13,10 @@
try:
from cytoolz.functoolz import excepts
- from cytoolz.itertoolz import concatv, topk
+ from cytoolz.itertoolz import concat, concatv, topk
except ImportError:
from .._vendor.toolz.functoolz import excepts # NOQA
- from .._vendor.toolz.itertoolz import concatv, topk # NOQA
+ from .._vendor.toolz.itertoolz import concat, concatv, topk # NOQA
log = getLogger(__name__)
@@ -387,13 +386,6 @@ def canonical_name(self):
def urls(self, with_credentials=False, subdirs=None):
_channels = self._channels
- if self.name == 'defaults':
- platform = next((s for s in reversed(subdirs or context.subdirs) if s != 'noarch'), '')
- if platform != context.subdir:
- # necessary shenanigan because different platforms have different default channels
- urls = DEFAULT_CHANNELS_WIN if 'win' in platform else DEFAULT_CHANNELS_UNIX
- ca = context.channel_alias
- _channels = tuple(Channel.make_simple_channel(ca, v) for v in urls)
return list(chain.from_iterable(c.urls(with_credentials, subdirs) for c in _channels))
@property
@@ -409,13 +401,15 @@ def prioritize_channels(channels, with_credentials=True, subdirs=None):
# urls as the key, and a tuple of canonical channel name and channel priority
# number as the value
# ('https://conda.anaconda.org/conda-forge/osx-64/', ('conda-forge', 1))
+ channels = concat((Channel(cc) for cc in c._channels) if isinstance(c, MultiChannel) else (c,)
+ for c in (Channel(c) for c in channels))
result = odict()
- for channel_priority, chn in enumerate(channels):
+ for priority_counter, chn in enumerate(channels):
channel = Channel(chn)
for url in channel.urls(with_credentials, subdirs):
if url in result:
continue
- result[url] = channel.canonical_name, min(channel_priority, MAX_CHANNEL_PRIORITY - 1)
+ result[url] = channel.canonical_name, min(priority_counter, MAX_CHANNEL_PRIORITY - 1)
return result
| diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -800,7 +800,7 @@ def test_subdirs_env_var(self):
subdirs = ('linux-highest', 'linux-64', 'noarch')
def _channel_urls(channels=None):
- for channel in channels or DEFAULT_CHANNELS_UNIX:
+ for channel in channels or DEFAULT_CHANNELS:
channel = Channel(channel)
for subdir in subdirs:
yield join_url(channel.base_url, subdir)
@@ -919,3 +919,47 @@ def test_channels_with_dashes(self):
('http://test/conda/anaconda-cluster/noarch', ('http://test/conda/anaconda-cluster', 0)),
))
+
+def test_multichannel_priority():
+ channels = ['conda-test', 'defaults', 'conda-forge']
+ subdirs = ['new-optimized-subdir', 'linux-32', 'noarch']
+ channel_priority_map = prioritize_channels(channels, with_credentials=True, subdirs=subdirs)
+ if on_win:
+ assert channel_priority_map == OrderedDict([
+ ('https://conda.anaconda.org/conda-test/new-optimized-subdir', ('conda-test', 0)),
+ ('https://conda.anaconda.org/conda-test/linux-32', ('conda-test', 0)),
+ ('https://conda.anaconda.org/conda-test/noarch', ('conda-test', 0)),
+ ('https://repo.continuum.io/pkgs/free/new-optimized-subdir', ('defaults', 1)),
+ ('https://repo.continuum.io/pkgs/free/linux-32', ('defaults', 1)),
+ ('https://repo.continuum.io/pkgs/free/noarch', ('defaults', 1)),
+ ('https://repo.continuum.io/pkgs/r/new-optimized-subdir', ('defaults', 2)),
+ ('https://repo.continuum.io/pkgs/r/linux-32', ('defaults', 2)),
+ ('https://repo.continuum.io/pkgs/r/noarch', ('defaults', 2)),
+ ('https://repo.continuum.io/pkgs/pro/new-optimized-subdir', ('defaults', 3)),
+ ('https://repo.continuum.io/pkgs/pro/linux-32', ('defaults', 3)),
+ ('https://repo.continuum.io/pkgs/pro/noarch', ('defaults', 3)),
+ ('https://repo.continuum.io/pkgs/msys2/new-optimized-subdir', ('defaults', 4)),
+ ('https://repo.continuum.io/pkgs/msys2/linux-32', ('defaults', 4)),
+ ('https://repo.continuum.io/pkgs/msys2/noarch', ('defaults', 4)),
+ ('https://conda.anaconda.org/conda-forge/new-optimized-subdir', ('conda-forge', 5)),
+ ('https://conda.anaconda.org/conda-forge/linux-32', ('conda-forge', 5)),
+ ('https://conda.anaconda.org/conda-forge/noarch', ('conda-forge', 5)),
+ ])
+ else:
+ assert channel_priority_map == OrderedDict([
+ ('https://conda.anaconda.org/conda-test/new-optimized-subdir', ('conda-test', 0)),
+ ('https://conda.anaconda.org/conda-test/linux-32', ('conda-test', 0)),
+ ('https://conda.anaconda.org/conda-test/noarch', ('conda-test', 0)),
+ ('https://repo.continuum.io/pkgs/free/new-optimized-subdir', ('defaults', 1)),
+ ('https://repo.continuum.io/pkgs/free/linux-32', ('defaults', 1)),
+ ('https://repo.continuum.io/pkgs/free/noarch', ('defaults', 1)),
+ ('https://repo.continuum.io/pkgs/r/new-optimized-subdir', ('defaults', 2)),
+ ('https://repo.continuum.io/pkgs/r/linux-32', ('defaults', 2)),
+ ('https://repo.continuum.io/pkgs/r/noarch', ('defaults', 2)),
+ ('https://repo.continuum.io/pkgs/pro/new-optimized-subdir', ('defaults', 3)),
+ ('https://repo.continuum.io/pkgs/pro/linux-32', ('defaults', 3)),
+ ('https://repo.continuum.io/pkgs/pro/noarch', ('defaults', 3)),
+ ('https://conda.anaconda.org/conda-forge/new-optimized-subdir', ('conda-forge', 4)),
+ ('https://conda.anaconda.org/conda-forge/linux-32', ('conda-forge', 4)),
+ ('https://conda.anaconda.org/conda-forge/noarch', ('conda-forge', 4)),
+ ])
diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -191,7 +191,7 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
@pytest.mark.installed
def test_activate_deactivate(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 9, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2017, 10, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
@@ -208,7 +208,7 @@ def test_activate_deactivate(shell):
@pytest.mark.installed
def test_activate_root_simple(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 9, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2017, 10, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -686,7 +686,7 @@ def test_update_deps_flag_absent(self):
assert package_is_installed(prefix, 'itsdangerous-0.23')
assert package_is_installed(prefix, 'flask')
- @pytest.mark.xfail(datetime.now() < datetime(2017, 9, 1), reason="#5263", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2017, 10, 1), reason="#5263", strict=True)
def test_update_deps_flag_present(self):
with make_temp_env("python=2 itsdangerous=0.23") as prefix:
assert package_is_installed(prefix, 'python-2')
@@ -887,7 +887,10 @@ def test_search_gawk_not_win(self):
def test_search_gawk_not_win_filter(self):
with make_temp_env() as prefix:
stdout, stderr = run_command(
- Commands.SEARCH, prefix, "gawk", "--platform", "win-64", "--json", use_exception_handler=True)
+ Commands.SEARCH, prefix, "gawk", "--platform", "win-64", "--json",
+ "-c", "https://repo.continuum.io/pkgs/msys2 --json",
+ use_exception_handler=True,
+ )
json_obj = json_loads(stdout.replace("Fetching package metadata ...", "").strip())
assert "gawk" in json_obj.keys()
assert "m2-gawk" in json_obj.keys()
diff --git a/tests/test_export.py b/tests/test_export.py
--- a/tests/test_export.py
+++ b/tests/test_export.py
@@ -31,7 +31,7 @@ def test_basic(self):
output2, error= run_command(Commands.LIST, prefix2, "-e")
self.assertEqual(output, output2)
- @pytest.mark.xfail(datetime.now() < datetime(2017, 9, 1), reason="Bring back `conda list --export` #3445", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2017, 10, 1), reason="Bring back `conda list --export` #3445", strict=True)
def test_multi_channel_export(self):
"""
When try to import from txt
diff --git a/tests/test_priority.py b/tests/test_priority.py
--- a/tests/test_priority.py
+++ b/tests/test_priority.py
@@ -1,5 +1,6 @@
from unittest import TestCase
+from conda.common.compat import on_win
import pytest
from conda.base.context import context
@@ -10,6 +11,7 @@
@pytest.mark.integration
class PriorityIntegrationTests(TestCase):
+ @pytest.mark.skipif(on_win, reason="xz packages are different on windows than unix")
def test_channel_order_channel_priority_true(self):
with make_temp_env("python=3.5 pycosat==0.6.1") as prefix:
assert_package_is_installed(prefix, 'python')
@@ -22,16 +24,16 @@ def test_channel_order_channel_priority_true(self):
# update --all
update_stdout, _ = run_command(Commands.UPDATE, prefix, '--all')
- # pycosat should be in the SUPERSEDED list
+ # xz should be in the SUPERSEDED list
superceded_split = update_stdout.split('SUPERSEDED')
assert len(superceded_split) == 2
- assert 'pycosat' in superceded_split[1]
+ assert 'xz' in superceded_split[1]
# python sys.version should show conda-forge python
python_tuple = get_conda_list_tuple(prefix, "python")
assert python_tuple[3] == 'conda-forge'
- # conda list should show pycosat coming from conda-forge
- pycosat_tuple = get_conda_list_tuple(prefix, "pycosat")
+ # conda list should show xz coming from conda-forge
+ pycosat_tuple = get_conda_list_tuple(prefix, "xz")
assert pycosat_tuple[3] == 'conda-forge'
def test_channel_priority_update(self):
| prioritize channels within multi-channels
| 2017-09-05T16:23:18 | -1.0 |
|
conda/conda | 5,982 | conda__conda-5982 | [
"5980",
"5980"
] | f0032e064a8122ebdb4a0d7885a0b61a053e58f5 | diff --git a/conda/gateways/disk/delete.py b/conda/gateways/disk/delete.py
--- a/conda/gateways/disk/delete.py
+++ b/conda/gateways/disk/delete.py
@@ -49,6 +49,8 @@ def rm_rf(path, max_retries=5, trash=True):
log.info("Failed to remove %s.", path)
else:
log.trace("rm_rf failed. Not a link, file, or directory: %s", path)
+ from ...core.linked_data import delete_prefix_from_linked_data
+ delete_prefix_from_linked_data(path)
return True
finally:
from ...core.linked_data import delete_prefix_from_linked_data
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -456,6 +456,19 @@ def test_list_with_pip_wheel(self):
rm_rf(join(prefix, get_python_site_packages_short_path("3.5")), "os.py")
assert prefix not in linked_data_
+ # regression test for #5980, related to #5847
+ with make_temp_env() as prefix:
+ assert isdir(prefix)
+ assert prefix in linked_data_
+
+ rmtree(prefix)
+ assert not isdir(prefix)
+ assert prefix in linked_data_
+
+ rm_rf(prefix)
+ assert not isdir(prefix)
+ assert prefix not in linked_data_
+
def test_install_tarball_from_local_channel(self):
# Regression test for #2812
# install from local channel
| Fix prefix data cache with rm_rf for conda-build on windows
As reported by @mingwandroid:
conda-build uses rd on Windows to remove the prefix before calling `rm_rf`, so we need conda to forget about the installed packages.
Fix prefix data cache with rm_rf for conda-build on windows
As reported by @mingwandroid:
conda-build uses rd on Windows to remove the prefix before calling `rm_rf`, so we need conda to forget about the installed packages.
| 2017-09-18T17:36:39 | -1.0 |
|
conda/conda | 5,986 | conda__conda-5986 | [
"5948",
"5980"
] | 40c6ae17729142fcd54478996417d171cc111ab9 | diff --git a/conda/cli/activate.py b/conda/cli/activate.py
--- a/conda/cli/activate.py
+++ b/conda/cli/activate.py
@@ -83,12 +83,15 @@ def _get_prefix_paths(prefix):
yield os.path.join(prefix, 'bin')
-def binpath_from_arg(arg, shell):
+def binpath_from_arg(arg, shell, going_to_shell=True):
shelldict = shells[shell] if shell else {}
# prefix comes back as platform-native path
prefix = prefix_from_arg(arg, shell)
# convert paths to shell-native paths
- return [shelldict['path_to'](path) for path in _get_prefix_paths(prefix)]
+ if going_to_shell:
+ return [shelldict['path_to'](path) for path in _get_prefix_paths(prefix)]
+ else:
+ return [path for path in _get_prefix_paths(prefix)]
def pathlist_to_str(paths, escape_backslashes=True):
@@ -105,12 +108,15 @@ def pathlist_to_str(paths, escape_backslashes=True):
return path
-def get_activate_path(prefix, shell):
+def get_activate_path(prefix, shell, going_to_shell=True):
shelldict = shells[shell] if shell else {}
- binpath = binpath_from_arg(prefix, shell)
+ binpath = binpath_from_arg(prefix, shell, going_to_shell)
# prepend our new entries onto the existing path and make sure that the separator is native
- path = shelldict['pathsep'].join(binpath)
+ if going_to_shell:
+ path = shelldict['pathsep'].join(binpath)
+ else:
+ path = os.pathsep.join(binpath)
return path
@@ -146,11 +152,11 @@ def main():
shell and env name".format(sys_argv[1]))
if sys_argv[1] == '..activate':
- print(get_activate_path(sys_argv[3], shell))
+ print(get_activate_path(sys_argv[3], shell, True))
sys.exit(0)
elif sys_argv[1] == '..deactivate.path':
- activation_path = get_activate_path(sys_argv[3], shell)
+ activation_path = get_activate_path(sys_argv[3], shell, False)
if os.getenv('_CONDA_HOLD'):
new_path = regex.sub(r'%s(:?)' % regex.escape(activation_path),
@@ -160,6 +166,7 @@ def main():
new_path = regex.sub(r'%s(:?)' % regex.escape(activation_path), r'',
os.environ[str('PATH')], 1)
+ new_path = shells[shell]['path_to'](new_path)
print(new_path)
sys.exit(0)
diff --git a/conda/common/path.py b/conda/common/path.py
--- a/conda/common/path.py
+++ b/conda/common/path.py
@@ -2,10 +2,12 @@
from __future__ import absolute_import, division, print_function, unicode_literals
from functools import reduce
+from logging import getLogger
import os
from os.path import (abspath, basename, dirname, expanduser, expandvars, join, normpath, split,
splitext)
import re
+import subprocess
from .compat import on_win, string_types
from .. import CondaError
@@ -25,6 +27,7 @@
except ImportError: # pragma: no cover
from .._vendor.toolz.itertoolz import accumulate, concat, take
+log = getLogger(__name__)
PATH_MATCH_REGEX = (
r"\./" # ./
@@ -253,14 +256,23 @@ def get_python_noarch_target_path(source_short_path, target_site_packages_short_
def win_path_to_unix(path, root_prefix=""):
- """Convert a path or ;-separated string of paths into a unix representation
-
- Does not add cygdrive. If you need that, set root_prefix to "/cygdrive"
- """
- path_re = '(?<![:/^a-zA-Z])([a-zA-Z]:[\/\\\\]+(?:[^:*?"<>|]+[\/\\\\]+)*[^:*?"<>|;\/\\\\]+?(?![a-zA-Z]:))' # noqa
-
- def _translation(found_path):
- found = found_path.group(1).replace("\\", "/").replace(":", "").replace("//", "/")
- return root_prefix + "/" + found
- path = re.sub(path_re, _translation, path).replace(";/", ":/")
+ # If the user wishes to drive conda from MSYS2 itself while also having
+ # msys2 packages in their environment this allows the path conversion to
+ # happen relative to the actual shell. The onus is on the user to set
+ # CYGPATH to e.g. /usr/bin/cygpath.exe (this will be translated to e.g.
+ # (C:\msys32\usr\bin\cygpath.exe by MSYS2) to ensure this one is used.
+ if not path:
+ return ''
+ cygpath = os.environ.get('CYGPATH', 'cygpath.exe')
+ try:
+ path = subprocess.check_output([cygpath, '-up', path]).decode('ascii').split('\n')[0]
+ except Exception as e:
+ log.debug('%r' % e, exc_info=True)
+ # Convert a path or ;-separated string of paths into a unix representation
+ # Does not add cygdrive. If you need that, set root_prefix to "/cygdrive"
+ def _translation(found_path): # NOQA
+ found = found_path.group(1).replace("\\", "/").replace(":", "").replace("//", "/")
+ return root_prefix + "/" + found
+ path_re = '(?<![:/^a-zA-Z])([a-zA-Z]:[\/\\\\]+(?:[^:*?"<>|]+[\/\\\\]+)*[^:*?"<>|;\/\\\\]+?(?![a-zA-Z]:))' # noqa
+ path = re.sub(path_re, _translation, path).replace(";/", ":/")
return path
diff --git a/conda/gateways/connection/adapters/s3.py b/conda/gateways/connection/adapters/s3.py
--- a/conda/gateways/connection/adapters/s3.py
+++ b/conda/gateways/connection/adapters/s3.py
@@ -1,11 +1,12 @@
# -*- coding: utf-8 -*-
from __future__ import absolute_import, division, print_function, unicode_literals
-from logging import getLogger, LoggerAdapter
-from tempfile import mkstemp
+import json
+from logging import LoggerAdapter, getLogger
+from tempfile import SpooledTemporaryFile
from .. import BaseAdapter, CaseInsensitiveDict, Response
-from ...disk.delete import rm_rf
+from ....common.compat import ensure_binary
from ....common.url import url_to_s3_info
log = getLogger(__name__)
@@ -16,24 +17,72 @@ class S3Adapter(BaseAdapter):
def __init__(self):
super(S3Adapter, self).__init__()
- self._temp_file = None
def send(self, request, stream=None, timeout=None, verify=None, cert=None, proxies=None):
-
resp = Response()
resp.status_code = 200
resp.url = request.url
try:
- import boto
+ import boto3
+ return self._send_boto3(boto3, resp, request)
except ImportError:
- stderrlog.info('\nError: boto is required for S3 channels. '
- 'Please install it with `conda install boto`\n'
- 'Make sure to run `source deactivate` if you '
- 'are in a conda environment.')
+ try:
+ import boto
+ return self._send_boto(boto, resp, request)
+ except ImportError:
+ stderrlog.info('\nError: boto3 is required for S3 channels. '
+ 'Please install with `conda install boto3`\n'
+ 'Make sure to run `source deactivate` if you '
+ 'are in a conda environment.\n')
+ resp.status_code = 404
+ return resp
+
+ def close(self):
+ pass
+
+ def _send_boto3(self, boto3, resp, request):
+ from botocore.exceptions import BotoCoreError, ClientError
+ bucket_name, key_string = url_to_s3_info(request.url)
+
+ # Get the key without validation that it exists and that we have
+ # permissions to list its contents.
+ key = boto3.resource('s3').Object(bucket_name, key_string[1:])
+
+ try:
+ response = key.get()
+ except (BotoCoreError, ClientError) as exc:
+ # This exception will occur if the bucket does not exist or if the
+ # user does not have permission to list its contents.
resp.status_code = 404
+ message = {
+ "error": "error downloading file from s3",
+ "path": request.url,
+ "exception": repr(exc),
+ }
+ fh = SpooledTemporaryFile()
+ fh.write(ensure_binary(json.dumps(message)))
+ fh.seek(0)
+ resp.raw = fh
+ resp.close = resp.raw.close
return resp
+ key_headers = response['ResponseMetadata']['HTTPHeaders']
+ resp.headers = CaseInsensitiveDict({
+ "Content-Type": key_headers.get('content-type', "text/plain"),
+ "Content-Length": key_headers['content-length'],
+ "Last-Modified": key_headers['last-modified'],
+ })
+
+ f = SpooledTemporaryFile()
+ key.download_fileobj(f)
+ f.seek(0)
+ resp.raw = f
+ resp.close = resp.raw.close
+
+ return resp
+
+ def _send_boto(self, boto, resp, request):
conn = boto.connect_s3()
bucket_name, key_string = url_to_s3_info(request.url)
@@ -60,16 +109,10 @@ def send(self, request, stream=None, timeout=None, verify=None, cert=None, proxi
"Last-Modified": modified,
})
- _, self._temp_file = mkstemp()
- key.get_contents_to_filename(self._temp_file)
- f = open(self._temp_file, 'rb')
- resp.raw = f
+ fh = SpooledTemporaryFile()
+ key.get_contents_to_file(fh)
+ fh.seek(0)
+ resp.raw = fh
resp.close = resp.raw.close
else:
resp.status_code = 404
-
- return resp
-
- def close(self):
- if self._temp_file:
- rm_rf(self._temp_file)
| diff --git a/tests/cli/test_activate.py b/tests/cli/test_activate.py
--- a/tests/cli/test_activate.py
+++ b/tests/cli/test_activate.py
@@ -181,6 +181,8 @@ def _format_vars(shell):
@pytest.mark.installed
def test_activate_test1(shell):
+ if shell == 'bash.exe':
+ pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
commands = (shell_vars['command_setup'] + """
@@ -195,6 +197,8 @@ def test_activate_test1(shell):
@pytest.mark.installed
def test_activate_env_from_env_with_root_activate(shell):
+ if shell == 'bash.exe':
+ pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
commands = (shell_vars['command_setup'] + """
@@ -227,6 +231,8 @@ def test_activate_bad_directory(shell):
@pytest.mark.installed
def test_activate_bad_env_keeps_existing_good_env(shell):
+ if shell == 'bash.exe':
+ pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
commands = (shell_vars['command_setup'] + """
@@ -241,6 +247,8 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
@pytest.mark.installed
def test_activate_deactivate(shell):
+ if shell == 'bash.exe':
+ pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
@@ -276,6 +284,8 @@ def test_activate_deactivate(shell):
@pytest.mark.installed
def test_activate_root_simple(shell):
+ if shell == 'bash.exe':
+ pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
commands = (shell_vars['command_setup'] + """
@@ -317,6 +327,8 @@ def test_activate_root_simple(shell):
@pytest.mark.installed
def test_wrong_args(shell):
+ if shell == 'bash.exe':
+ pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
commands = (shell_vars['command_setup'] + """
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -536,6 +536,7 @@ def test_create_empty_env(self):
self.assertIsInstance(stdout, str)
def test_list_with_pip_egg(self):
+ from conda.exports import rm_rf as _rm_rf
with make_temp_env("python=3.5 pip") as prefix:
check_call(PYTHON_BINARY + " -m pip install --egg --no-binary flask flask==0.10.1",
cwd=prefix, shell=True)
@@ -547,11 +548,11 @@ def test_list_with_pip_egg(self):
# regression test for #5847
# when using rm_rf on a directory
assert prefix in PrefixData._cache_
- from conda.exports import rm_rf as _rm_rf
_rm_rf(join(prefix, get_python_site_packages_short_path("3.5")))
assert prefix not in PrefixData._cache_
def test_list_with_pip_wheel(self):
+ from conda.exports import rm_rf as _rm_rf
with make_temp_env("python=3.6 pip") as prefix:
check_call(PYTHON_BINARY + " -m pip install flask==0.10.1",
cwd=prefix, shell=True)
@@ -567,10 +568,22 @@ def test_list_with_pip_wheel(self):
# regression test for #5847
# when using rm_rf on a file
assert prefix in PrefixData._cache_
- from conda.exports import rm_rf as _rm_rf
_rm_rf(join(prefix, get_python_site_packages_short_path("3.5")), "os.py")
assert prefix not in PrefixData._cache_
+ # regression test for #5980, related to #5847
+ with make_temp_env() as prefix:
+ assert isdir(prefix)
+ assert prefix in PrefixData._cache_
+
+ rmtree(prefix)
+ assert not isdir(prefix)
+ assert prefix in PrefixData._cache_
+
+ _rm_rf(prefix)
+ assert not isdir(prefix)
+ assert prefix not in PrefixData._cache_
+
def test_install_tarball_from_local_channel(self):
# Regression test for #2812
# install from local channel
diff --git a/tests/test_utils.py b/tests/test_utils.py
--- a/tests/test_utils.py
+++ b/tests/test_utils.py
@@ -8,12 +8,12 @@
def test_path_translations():
paths = [
- (r";z:\miniconda\Scripts\pip.exe",
- ":/z/miniconda/Scripts/pip.exe",
- ":/cygdrive/z/miniconda/Scripts/pip.exe"),
- (r";z:\miniconda;z:\Documents (x86)\pip.exe;C:\test",
- ":/z/miniconda:/z/Documents (x86)/pip.exe:/C/test",
- ":/cygdrive/z/miniconda:/cygdrive/z/Documents (x86)/pip.exe:/cygdrive/C/test"),
+ (r"z:\miniconda\Scripts\pip.exe",
+ "/z/miniconda/Scripts/pip.exe",
+ "/cygdrive/z/miniconda/Scripts/pip.exe"),
+ (r"z:\miniconda;z:\Documents (x86)\pip.exe;c:\test",
+ "/z/miniconda:/z/Documents (x86)/pip.exe:/c/test",
+ "/cygdrive/z/miniconda:/cygdrive/z/Documents (x86)/pip.exe:/cygdrive/c/test"),
# Failures:
# (r"z:\miniconda\Scripts\pip.exe",
# "/z/miniconda/Scripts/pip.exe",
@@ -30,12 +30,12 @@ def test_path_translations():
assert win_path_to_unix(windows_path) == unix_path
assert utils.unix_path_to_win(unix_path) == windows_path
- assert utils.win_path_to_cygwin(windows_path) == cygwin_path
- assert utils.cygwin_path_to_win(cygwin_path) == windows_path
+ # assert utils.win_path_to_cygwin(windows_path) == cygwin_path
+ # assert utils.cygwin_path_to_win(cygwin_path) == windows_path
def test_text_translations():
- test_win_text = "prepending z:\\msarahan\\code\\conda\\tests\\envsk5_b4i\\test 1 and z:\\msarahan\\code\\conda\\tests\\envsk5_b4i\\test 1\\scripts to path"
- test_unix_text = "prepending /z/msarahan/code/conda/tests/envsk5_b4i/test 1 and /z/msarahan/code/conda/tests/envsk5_b4i/test 1/scripts to path"
+ test_win_text = "z:\\msarahan\\code\\conda\\tests\\envsk5_b4i\\test 1"
+ test_unix_text = "/z/msarahan/code/conda/tests/envsk5_b4i/test 1"
assert_equals(test_win_text, utils.unix_path_to_win(test_unix_text))
assert_equals(test_unix_text, win_path_to_unix(test_win_text))
| add boto3 to conda's s3 adapter
Fix prefix data cache with rm_rf for conda-build on windows
As reported by @mingwandroid:
conda-build uses rd on Windows to remove the prefix before calling `rm_rf`, so we need conda to forget about the installed packages.
| 2017-09-19T18:45:41 | -1.0 |
|
conda/conda | 5,988 | conda__conda-5988 | [
"5983"
] | 91adf32066cf3ee10b5d10e3419bed4348bdcacd | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -97,6 +97,7 @@ class Context(Configuration):
element_type=string_types + (NoneType,),
validation=default_python_validation)
disallow = SequenceParameter(string_types)
+ download_only = PrimitiveParameter(False)
enable_private_envs = PrimitiveParameter(False)
force_32bit = PrimitiveParameter(False)
max_shlvl = PrimitiveParameter(2)
@@ -682,6 +683,10 @@ def get_help_dict():
Package specifications to disallow installing. The default is to allow
all packages.
"""),
+ 'download_only': dals("""
+ Solve an environment and ensure package caches are populated, but exit
+ prior to unlinking and linking packages into the prefix
+ """),
'envs_dirs': dals("""
The list of directories to search for named environments. When creating a new
named environment, the environment will be placed in the first writable
diff --git a/conda/cli/conda_argparse.py b/conda/cli/conda_argparse.py
--- a/conda/cli/conda_argparse.py
+++ b/conda/cli/conda_argparse.py
@@ -1079,6 +1079,13 @@ def add_parser_create_install_update(p):
"Can be used multiple times. "
"Equivalent to a MatchSpec specifying a single 'provides_features'.",
)
+ p.add_argument(
+ "--download-only",
+ action="store_true",
+ default=NULL,
+ help="Solve an environment and ensure package caches are populated, but exit "
+ "prior to unlinking and linking packages into the prefix.",
+ )
def add_parser_pscheck(p):
diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -10,21 +10,21 @@
import os
from os.path import abspath, basename, exists, isdir
-from conda.models.match_spec import MatchSpec
from . import common
from .common import check_non_admin
from .._vendor.auxlib.ish import dals
from ..base.constants import ROOT_ENV_NAME
from ..base.context import context
-from ..common.compat import text_type, on_win
+from ..common.compat import on_win, text_type
from ..core.envs_manager import EnvsDirectory
from ..core.index import calculate_channel_urls, get_index
from ..core.solve import Solver
-from ..exceptions import (CondaImportError, CondaOSError, CondaSystemExit, CondaValueError,
- DirectoryNotFoundError, DryRunExit, EnvironmentLocationNotFound,
- PackagesNotFoundError, TooManyArgumentsError,
- UnsatisfiableError)
+from ..exceptions import (CondaExitZero, CondaImportError, CondaOSError, CondaSystemExit,
+ CondaValueError, DirectoryNotFoundError, DryRunExit,
+ EnvironmentLocationNotFound, PackagesNotFoundError,
+ TooManyArgumentsError, UnsatisfiableError)
from ..misc import append_env, clone_env, explicit, touch_nonadmin
+from ..models.match_spec import MatchSpec
from ..plan import (revert_actions)
from ..resolve import ResolvePackageNotFound
@@ -267,6 +267,9 @@ def handle_txn(progressive_fetch_extract, unlink_link_transaction, prefix, args,
try:
progressive_fetch_extract.execute()
+ if context.download_only:
+ raise CondaExitZero('Package caches prepared. UnlinkLinkTransaction cancelled with '
+ '--download-only option.')
unlink_link_transaction.execute()
except SystemExit as e:
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -141,7 +141,7 @@ def make_temp_env(*packages, **kwargs):
try:
# try to clear any config that's been set by other tests
reset_context([os.path.join(prefix+os.sep, 'condarc')])
- run_command(Commands.CREATE, prefix, *packages)
+ run_command(Commands.CREATE, prefix, *packages, **kwargs)
yield prefix
finally:
rmtree(prefix, ignore_errors=True)
@@ -1455,6 +1455,14 @@ def test_force_remove(self):
rm_rf(prefix)
run_command(Commands.REMOVE, prefix, "--all")
+ def test_download_only_flag(self):
+ from conda.core.link import UnlinkLinkTransaction
+ with patch.object(UnlinkLinkTransaction, 'execute') as mock_method:
+ with make_temp_env('openssl --download-only', use_exception_handler=True) as prefix:
+ assert mock_method.call_count == 0
+ with make_temp_env('openssl', use_exception_handler=True) as prefix:
+ assert mock_method.call_count == 1
+
def test_transactional_rollback_simple(self):
from conda.core.path_actions import CreatePrefixRecordAction
with patch.object(CreatePrefixRecordAction, 'execute') as mock_method:
| conda install --download-only feature
Right now we have `--dry-run`, but is there a way to actually download the `.tar.bz2` files into `pkgs` without installing them? If not, can there be?
`--download-only` is one design with precedent, but also maybe as a separate subcommand, like `pip download`. The overarching goal is to get `pkgs` populated with all the dependencies necessary for an application and freezing it out into something akin to [a wheelhouse](https://pip.pypa.io/en/stable/reference/pip_wheel/#examples) for reproducible installs ([a la](https://www.paypal-engineering.com/2016/09/22/python-by-the-c-side/)).
Thanks in advance!
| Yeah we need this. It's been on the roadmap for the API for a while. Actually is trivial to implement at this point. I'll make sure it gets into 4.4.
Sent from my iPhone
> On Sep 18, 2017, at 7:34 PM, Mahmoud Hashemi <notifications@github.com> wrote:
>
> Right now we have --dry-run, but is there a way to actually download the .tar.bz2 files into pkgs without installing them? If not, can there be?
>
> --download-only is one design with precedent, but also maybe as a separate subcommand, like pip download. The overarching goal is to get pkgs populated with all the dependencies necessary for an application and freezing it out into something akin to a wheelhouse for reproducible installs (a la).
>
> Thanks in advance!
>
> —
> You are receiving this because you are subscribed to this thread.
> Reply to this email directly, view it on GitHub, or mute the thread.
>
| 2017-09-19T20:42:10 | -1.0 |
conda/conda | 6,044 | conda__conda-6044 | [
"5854"
] | 066fea062b6184a50acd6f0e3de16f52f4c84868 | diff --git a/conda/core/repodata.py b/conda/core/repodata.py
--- a/conda/core/repodata.py
+++ b/conda/core/repodata.py
@@ -51,7 +51,7 @@
log = getLogger(__name__)
stderrlog = getLogger('conda.stderrlog')
-REPODATA_PICKLE_VERSION = 6
+REPODATA_PICKLE_VERSION = 16
REPODATA_HEADER_RE = b'"(_etag|_mod|_cache_control)":[ ]?"(.*?[^\\\\])"[,\}\s]'
diff --git a/conda/models/index_record.py b/conda/models/index_record.py
--- a/conda/models/index_record.py
+++ b/conda/models/index_record.py
@@ -26,7 +26,7 @@
from .enums import FileMode, LinkType, NoarchType, PackageType, PathType, Platform
from .._vendor.auxlib.collection import frozendict
from .._vendor.auxlib.entity import (BooleanField, ComposableField, DictSafeMixin, Entity,
- EnumField, IntegerField, ListField, MapField,
+ EnumField, IntegerField, ListField, MapField, NumberField,
StringField)
from ..base.context import context
from ..common.compat import isiterable, iteritems, itervalues, string_types, text_type
@@ -48,6 +48,15 @@ def box(self, instance, instance_type, val):
return super(NoarchField, self).box(instance, instance_type, NoarchType.coerce(val))
+class TimestampField(NumberField):
+
+ def box(self, instance, instance_type, val):
+ val = super(TimestampField, self).box(instance, instance_type, val)
+ if val and val > 253402300799: # 9999-12-31
+ val /= 1000 # convert milliseconds to seconds; see conda/conda-build#1988
+ return val
+
+
class Link(DictSafeMixin, Entity):
source = StringField()
type = LinkTypeField(LinkType, required=False)
| diff --git a/tests/conda_env/test_create.py b/tests/conda_env/test_create.py
--- a/tests/conda_env/test_create.py
+++ b/tests/conda_env/test_create.py
@@ -133,5 +133,9 @@ def test_create_advanced_pip(self):
assert exists(python_path)
assert_package_is_installed(prefix, 'argh', exact=False, pip=True)
assert_package_is_installed(prefix, 'module-to-install-in-editable-mode', exact=False, pip=True)
- assert_package_is_installed(prefix, 'six', exact=False, pip=True)
+ try:
+ assert_package_is_installed(prefix, 'six', exact=False, pip=True)
+ except AssertionError:
+ # six may now be conda-installed because of packaging changes
+ assert_package_is_installed(prefix, 'six', exact=False, pip=False)
assert_package_is_installed(prefix, 'xmltodict-0.10.2-<pip>', exact=True, pip=True)
| Cannot open Anaconda Navigator
I have an issue when I try to open the Navigator. It throws an unexpected error occurred.
`An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.21
conda is private : False
conda-env version : 4.3.21
conda-build version : not installed
python version : 3.6.1.final.0
requests version : 2.14.2
root environment : C:\Anaconda3 (writable)
default environment : C:\Anaconda3
envs directories : C:\Anaconda3\envs
C:\Users\John\AppData\Local\conda\conda\envs
C:\Users\John\.conda\envs
package cache : C:\Anaconda3\pkgs
C:\Users\John\AppData\Local\conda\conda\pkgs
channel URLs : https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : None
netrc file : None
offline mode : False
user-agent : conda/4.3.21 requests/2.14.2 CPython/3.6.1 Windows/10 Windows/10.0.15063
administrator : False
`$ C:\Anaconda3\Scripts\conda-script.py ..checkenv cmd.exe C:\Anaconda3`
Traceback (most recent call last):
File "C:\Anaconda3\lib\site-packages\conda\cli\main.py", line 167, in main
import conda.cli.activate as activate
File "C:\Anaconda3\lib\site-packages\conda\cli\activate.py", line 12, in <module>
from ..utils import shells
File "C:\Anaconda3\lib\site-packages\conda\utils.py", line 13, in <module>
from .gateways.disk.read import compute_md5sum
File "C:\Anaconda3\lib\site-packages\conda\gateways\disk\read.py", line 22, in <module>
from ...models.channel import Channel
File "C:\Anaconda3\lib\site-packages\conda\models\channel.py", line 9, in <module>
from ..base.context import context
File "C:\Anaconda3\lib\site-packages\conda\base\context.py", line 18, in <module>
from .._vendor.auxlib.path import expand
File "C:\Anaconda3\lib\site-packages\conda\_vendor\auxlib\path.py", line 8, in <module>
import pkg_resources
File "<frozen importlib._bootstrap>", line 961, in _find_and_load
File "<frozen importlib._bootstrap>", line 950, in _find_and_load_unlocked
File "<frozen importlib._bootstrap>", line 646, in _load_unlocked
File "<frozen importlib._bootstrap>", line 616, in _load_backward_compatible
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 2985, in <module>
@_call_aside
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 2971, in _call_aside
f(*args, **kwargs)
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 3013, in _initialize_master_working_set
dist.activate(replace=False)
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 2544, in activate
declare_namespace(pkg)
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 2118, in declare_namespace
_handle_ns(packageName, path_item)
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 2058, in _handle_ns
_rebuild_mod_path(path, packageName, module)
File "C:\Anaconda3\lib\site-packages\setuptools-27.2.0-py3.6.egg\pkg_resources\__init__.py", line 2087, in _rebuild_mod_path
orig_path.sort(key=position_in_sys_path)
AttributeError: '_NamespacePath' object has no attribute 'sort'`
| Related to #5500. | 2017-09-29T21:59:21 | -1.0 |
conda/conda | 6,128 | conda__conda-6128 | [
"6096"
] | 8d5b26c24128ac88a725cd275250aa379f58eff0 | diff --git a/conda/models/index_record.py b/conda/models/index_record.py
--- a/conda/models/index_record.py
+++ b/conda/models/index_record.py
@@ -54,12 +54,31 @@ def box(self, instance, val):
class TimestampField(NumberField):
- def box(self, instance, val):
- val = super(TimestampField, self).box(instance, val)
- if val and val > 253402300799: # 9999-12-31
- val /= 1000 # convert milliseconds to seconds; see conda/conda-build#1988
+ @staticmethod
+ def _make_seconds(val):
+ if val:
+ val = int(val)
+ if val > 253402300799: # 9999-12-31
+ val //= 1000 # convert milliseconds to seconds; see conda/conda-build#1988
return val
+ # @staticmethod
+ # def _make_milliseconds(val):
+ # if val:
+ # val = int(val)
+ # if val < 253402300799: # 9999-12-31
+ # val *= 1000 # convert seconds to milliseconds
+ # return val
+
+ def box(self, instance, val):
+ return self._make_seconds(super(TimestampField, self).box(instance, val))
+
+ def unbox(self, instance, instance_type, val):
+ return self._make_seconds(super(TimestampField, self).unbox(instance, instance_type, val))
+
+ def dump(self, val):
+ return self._make_seconds(super(TimestampField, self).dump(val))
+
class Link(DictSafeMixin, Entity):
source = StringField()
| diff --git a/tests/models/test_index_record.py b/tests/models/test_index_record.py
new file mode 100644
--- /dev/null
+++ b/tests/models/test_index_record.py
@@ -0,0 +1,22 @@
+# -*- coding: utf-8 -*-
+from __future__ import absolute_import, division, print_function, unicode_literals
+from logging import getLogger
+from math import floor
+
+from conda.models.index_record import IndexRecord
+
+log = getLogger(__name__)
+
+
+def test_index_record_timestamp():
+ # regression test for #6096
+ ts = 1507565728.999
+ rec = IndexRecord(
+ name='test-package',
+ version='1.2.3',
+ build='2',
+ build_number=2,
+ timestamp=ts
+ )
+ assert rec.timestamp == floor(ts)
+ assert rec.dump()['timestamp'] == floor(ts)
| ValidationError: invalid value for timestamp
MODERATOR EDIT: This issue relates to an error that looks something like
ValidationError: Invalid value 1505856869.685 for timestamp
The issue relates to using conda 4.3.28, and then downgrading (presumably via conda-forge) to a previous version of conda, like 4.3.27.
# SOLUTION #
### To fix on macOS:
# in the command below, set PATH_TO_ENVIRONMENT yourself
sed -i '' -E 's|("timestamp": [0-9]+)\.|\1|' /PATH_TO_ENVIRONMENT/conda-meta/*.json
### To fix on Linux:
# in the command below, set PATH_TO_ENVIRONMENT yourself
sed -i -E 's|("timestamp": [0-9]+)\.|\1|' /PATH_TO_ENVIRONMENT/conda-meta/*.json
### To fix on Windows:
Open notepad, and copy the contents below to `c:\fix_timestamps.py`
```python
PATH_TO_ENVIRONMENT="c:\\ProgramData\\Anaconda3" # <-- fill this in yourself
# backslashes must be doubled
from glob import glob
import json
import os
for path in glob(os.path.join(PATH_TO_ENVIRONMENT, 'conda-meta', '*.json')):
with open(path) as fh:
content = json.load(fh)
if 'timestamp' in content:
old_timestamp = content['timestamp']
content['timestamp'] = int(old_timestamp)
if old_timestamp != content['timestamp']:
with open(path, 'w') as fh:
fh.write(json.dumps(content, indent=2, sort_keys=True, separators=(',', ': ')))
```
Also, change the path in the variable `PATH_TO_ENVIRONMENT` to point to the conda environment you want to fix. Then run the script with `python c:\fix_timestamps.py`.
----
EDITED: I realized this is not a pyqt issue, it's a conda issue
### steps to reproduce
1. Install 64-bit miniconda on windows 10 (version 4.3.27)
2. conda update to `4.3.28-py36h9daa44c_0`
3. conda install -c anaconda spyder
4. conda config --add channels conda-forge
Now any calls to conda results in the print-out below.
### conda info
This prints any time I try to use a conda command.
```conda install -c dsdale24 pyqt5
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.27
conda is private : False
conda-env version : 4.3.27
conda-build version : not installed
python version : 3.6.2.final.0
requests version : 2.18.4
root environment : C:\Users\jrinker\Miniconda3 (writable)
default environment : C:\Users\jrinker\Miniconda3
envs directories : C:\Users\jrinker\Miniconda3\envs
C:\Users\jrinker\AppData\Local\conda\conda\envs
C:\Users\jrinker\.conda\envs
package cache : C:\Users\jrinker\Miniconda3\pkgs
C:\Users\jrinker\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/dsdale24/win-64
https://conda.anaconda.org/dsdale24/noarch
https://conda.anaconda.org/conda-forge/win-64
https://conda.anaconda.org/conda-forge/noarch
https://repo.continuum.io/pkgs/main/win-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\jrinker\.condarc
netrc file : None
offline mode : False
user-agent : conda/4.3.27 requests/2.18.4 CPython/3.6.2 Windows/10 Windows/10.0.15063
administrator : False
`$ C:\Users\jrinker\Miniconda3\Scripts\conda install -c dsdale24 pyqt5`
Traceback (most recent call last):
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\exceptions.py", line 640, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\cli\main.py", line 140, in _main
exit_code = args.func(args, p)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\cli\main_install.py", line 80, in execute
install(args, parser, 'install')
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\cli\install.py", line 160, in install
linked_dists = install_linked(prefix)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\core\linked_data.py", line 123, in linked
return set(linked_data(prefix, ignore_channels=ignore_channels).keys())
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\core\linked_data.py", line 115, in linked_data
load_linked_data(prefix, dist_name, ignore_channels=ignore_channels)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\core\linked_data.py", line 68, in load_linked_data
linked_data_[prefix][dist] = rec = IndexRecord(**rec)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 702, in __call__
instance = super(EntityType, cls).__call__(*args, **kwargs)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 719, in __init__
setattr(self, key, kwargs[key])
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 424, in __set__
instance.__dict__[self.name] = self.validate(instance, self.box(instance, val))
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 465, in validate
raise ValidationError(getattr(self, 'name', 'undefined name'), val)
conda._vendor.auxlib.exceptions.ValidationError: Invalid value 1505856869.685 for timestamp
```
### things I've tried
- Removing conda forge from channels
- `conda update conda` (still prints out error)
| @kalefranz, @msarahan, is this something to do with the * 1000 thing? Is there some code that's dividing these numbers back down again? Was such code every released in the wild?
v4.3.28 has this: https://github.com/conda/conda/commit/527c0d3c6d988ae44280b324da58950005f2bbdd
Python2 will:
```
>>> 1505856869685/1000
1505856869
```
Python3 will:
```
>>> 1505856869685/1000
1505856869.685
```
sorry, i dont understand. How should I fix it?
If the issue is line 60 in `conda/models/index_record.py`, then a possible bug fix is just changing it to
`val //= 1000`
But I don't know enough about the code to know if this is what should be changed.
The problems seems mushroming
#6106
#6107
#6103
#6105
We are working to identify the proper fix. We are removing conda 4.3.28 from defaults in the meantime to prevent any further breakage.
For those who are already affected by this bug, we are working to come up with a simple series of steps to restore your functionality.
@msarahan Hopefully it can be smoothly fixed without the need to reinstall everything.
@Lemmaho yes, that is most certainly our goal. @nehaljwani will be posting the fix he's been testing shortly. | 2017-10-09T16:25:58 | -1.0 |
conda/conda | 6,131 | conda__conda-6131 | [
"6096"
] | 8d5b26c24128ac88a725cd275250aa379f58eff0 | diff --git a/conda/models/index_record.py b/conda/models/index_record.py
--- a/conda/models/index_record.py
+++ b/conda/models/index_record.py
@@ -54,12 +54,33 @@ def box(self, instance, val):
class TimestampField(NumberField):
- def box(self, instance, val):
- val = super(TimestampField, self).box(instance, val)
- if val and val > 253402300799: # 9999-12-31
- val /= 1000 # convert milliseconds to seconds; see conda/conda-build#1988
+ # @staticmethod
+ # def _make_seconds(val):
+ # if val:
+ # val = int(val)
+ # if val > 253402300799: # 9999-12-31
+ # val //= 1000 # convert milliseconds to seconds; see conda/conda-build#1988
+ # return val
+
+ @staticmethod
+ def _make_milliseconds(val):
+ if val:
+ if val < 253402300799: # 9999-12-31
+ val *= 1000 # convert seconds to milliseconds
+ val = int(val)
return val
+ def box(self, instance, val):
+ return self._make_milliseconds(super(TimestampField, self).box(instance, val))
+
+ def unbox(self, instance, instance_type, val):
+ return self._make_milliseconds(
+ super(TimestampField, self).unbox(instance, instance_type, val)
+ )
+
+ def dump(self, val):
+ return self._make_milliseconds(super(TimestampField, self).dump(val))
+
class Link(DictSafeMixin, Entity):
source = StringField()
| diff --git a/tests/models/test_index_record.py b/tests/models/test_index_record.py
new file mode 100644
--- /dev/null
+++ b/tests/models/test_index_record.py
@@ -0,0 +1,35 @@
+# -*- coding: utf-8 -*-
+from __future__ import absolute_import, division, print_function, unicode_literals
+from logging import getLogger
+
+from conda.models.index_record import IndexRecord
+
+log = getLogger(__name__)
+
+
+def test_index_record_timestamp():
+ # regression test for #6096
+ ts = 1507565728
+ new_ts = ts * 1000
+ rec = IndexRecord(
+ name='test-package',
+ version='1.2.3',
+ build='2',
+ build_number=2,
+ timestamp=ts
+ )
+ assert rec.timestamp == new_ts
+ assert rec.dump()['timestamp'] == new_ts
+
+ ts = 1507565728999
+ new_ts = ts
+ rec = IndexRecord(
+ name='test-package',
+ version='1.2.3',
+ build='2',
+ build_number=2,
+ timestamp=ts
+ )
+ assert rec.timestamp == new_ts
+ assert rec.dump()['timestamp'] == new_ts
+
| ValidationError: invalid value for timestamp
MODERATOR EDIT: This issue relates to an error that looks something like
ValidationError: Invalid value 1505856869.685 for timestamp
The issue relates to using conda 4.3.28, and then downgrading (presumably via conda-forge) to a previous version of conda, like 4.3.27.
# SOLUTION #
### To fix on macOS:
# in the command below, set PATH_TO_ENVIRONMENT yourself
sed -i '' -E 's|("timestamp": [0-9]+)\.|\1|' /PATH_TO_ENVIRONMENT/conda-meta/*.json
### To fix on Linux:
# in the command below, set PATH_TO_ENVIRONMENT yourself
sed -i -E 's|("timestamp": [0-9]+)\.|\1|' /PATH_TO_ENVIRONMENT/conda-meta/*.json
### To fix on Windows:
Open notepad, and copy the contents below to `c:\fix_timestamps.py`
```python
PATH_TO_ENVIRONMENT="c:\\ProgramData\\Anaconda3" # <-- fill this in yourself
# backslashes must be doubled
from glob import glob
import json
import os
for path in glob(os.path.join(PATH_TO_ENVIRONMENT, 'conda-meta', '*.json')):
with open(path) as fh:
content = json.load(fh)
if 'timestamp' in content:
old_timestamp = content['timestamp']
content['timestamp'] = int(old_timestamp)
if old_timestamp != content['timestamp']:
with open(path, 'w') as fh:
fh.write(json.dumps(content, indent=2, sort_keys=True, separators=(',', ': ')))
```
Also, change the path in the variable `PATH_TO_ENVIRONMENT` to point to the conda environment you want to fix. Then run the script with `python c:\fix_timestamps.py`.
----
EDITED: I realized this is not a pyqt issue, it's a conda issue
### steps to reproduce
1. Install 64-bit miniconda on windows 10 (version 4.3.27)
2. conda update to `4.3.28-py36h9daa44c_0`
3. conda install -c anaconda spyder
4. conda config --add channels conda-forge
Now any calls to conda results in the print-out below.
### conda info
This prints any time I try to use a conda command.
```conda install -c dsdale24 pyqt5
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : win-64
conda version : 4.3.27
conda is private : False
conda-env version : 4.3.27
conda-build version : not installed
python version : 3.6.2.final.0
requests version : 2.18.4
root environment : C:\Users\jrinker\Miniconda3 (writable)
default environment : C:\Users\jrinker\Miniconda3
envs directories : C:\Users\jrinker\Miniconda3\envs
C:\Users\jrinker\AppData\Local\conda\conda\envs
C:\Users\jrinker\.conda\envs
package cache : C:\Users\jrinker\Miniconda3\pkgs
C:\Users\jrinker\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/dsdale24/win-64
https://conda.anaconda.org/dsdale24/noarch
https://conda.anaconda.org/conda-forge/win-64
https://conda.anaconda.org/conda-forge/noarch
https://repo.continuum.io/pkgs/main/win-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : C:\Users\jrinker\.condarc
netrc file : None
offline mode : False
user-agent : conda/4.3.27 requests/2.18.4 CPython/3.6.2 Windows/10 Windows/10.0.15063
administrator : False
`$ C:\Users\jrinker\Miniconda3\Scripts\conda install -c dsdale24 pyqt5`
Traceback (most recent call last):
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\exceptions.py", line 640, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\cli\main.py", line 140, in _main
exit_code = args.func(args, p)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\cli\main_install.py", line 80, in execute
install(args, parser, 'install')
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\cli\install.py", line 160, in install
linked_dists = install_linked(prefix)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\core\linked_data.py", line 123, in linked
return set(linked_data(prefix, ignore_channels=ignore_channels).keys())
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\core\linked_data.py", line 115, in linked_data
load_linked_data(prefix, dist_name, ignore_channels=ignore_channels)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\core\linked_data.py", line 68, in load_linked_data
linked_data_[prefix][dist] = rec = IndexRecord(**rec)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 702, in __call__
instance = super(EntityType, cls).__call__(*args, **kwargs)
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 719, in __init__
setattr(self, key, kwargs[key])
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 424, in __set__
instance.__dict__[self.name] = self.validate(instance, self.box(instance, val))
File "C:\Users\jrinker\Miniconda3\lib\site-packages\conda\_vendor\auxlib\entity.py", line 465, in validate
raise ValidationError(getattr(self, 'name', 'undefined name'), val)
conda._vendor.auxlib.exceptions.ValidationError: Invalid value 1505856869.685 for timestamp
```
### things I've tried
- Removing conda forge from channels
- `conda update conda` (still prints out error)
| @kalefranz, @msarahan, is this something to do with the * 1000 thing? Is there some code that's dividing these numbers back down again? Was such code every released in the wild?
v4.3.28 has this: https://github.com/conda/conda/commit/527c0d3c6d988ae44280b324da58950005f2bbdd
Python2 will:
```
>>> 1505856869685/1000
1505856869
```
Python3 will:
```
>>> 1505856869685/1000
1505856869.685
```
sorry, i dont understand. How should I fix it?
If the issue is line 60 in `conda/models/index_record.py`, then a possible bug fix is just changing it to
`val //= 1000`
But I don't know enough about the code to know if this is what should be changed.
The problems seems mushroming
#6106
#6107
#6103
#6105
We are working to identify the proper fix. We are removing conda 4.3.28 from defaults in the meantime to prevent any further breakage.
For those who are already affected by this bug, we are working to come up with a simple series of steps to restore your functionality.
@msarahan Hopefully it can be smoothly fixed without the need to reinstall everything.
@Lemmaho yes, that is most certainly our goal. @nehaljwani will be posting the fix he's been testing shortly. | 2017-10-09T18:35:44 | -1.0 |
conda/conda | 6,154 | conda__conda-6154 | [
"6023"
] | 98c3549429009de3c51cffc5e25753dfe83a7417 | diff --git a/conda_env/cli/main_list.py b/conda_env/cli/main_list.py
--- a/conda_env/cli/main_list.py
+++ b/conda_env/cli/main_list.py
@@ -14,7 +14,7 @@
def configure_parser(sub_parsers):
- l = sub_parsers.add_parser(
+ list_parser = sub_parsers.add_parser(
'list',
formatter_class=RawDescriptionHelpFormatter,
description=description,
@@ -22,9 +22,9 @@ def configure_parser(sub_parsers):
epilog=example,
)
- common.add_parser_json(l)
+ common.add_parser_json(list_parser)
- l.set_defaults(func=execute)
+ list_parser.set_defaults(func=execute)
def execute(args, parser):
| diff --git a/conda/gateways/disk/test.py b/conda/gateways/disk/test.py
--- a/conda/gateways/disk/test.py
+++ b/conda/gateways/disk/test.py
@@ -4,6 +4,7 @@
from logging import getLogger
from os import W_OK, access
from os.path import basename, dirname, isdir, isfile, join, lexists
+from uuid import uuid4
from .create import create_link
from .delete import rm_rf
@@ -11,6 +12,7 @@
from .read import find_first_existing
from ... import CondaError
from ..._vendor.auxlib.decorators import memoize
+from ...common.compat import text_type
from ...common.path import expand, get_python_short_path
from ...models.enums import LinkType
@@ -77,7 +79,7 @@ def hardlink_supported(source_file, dest_dir):
# file system configuration, a symbolic link may be created
# instead. If a symbolic link is created instead of a hard link,
# return False.
- test_file = join(dest_dir, '.tmp.' + basename(source_file))
+ test_file = join(dest_dir, '.tmp.%s.%s' % (basename(source_file), text_type(uuid4())[:8]))
assert isfile(source_file), source_file
assert isdir(dest_dir), dest_dir
if lexists(test_file):
| Scarpy
Current conda install:
platform : win-64
conda version : 4.3.21
conda is private : False
conda-env version : 4.3.21
conda-build version : not installed
python version : 3.6.1.final.0
requests version : 2.14.2
root environment : C:\Users\dbsr7927\AppData\Local\Continuum\Anaconda3 (writable)
default environment : C:\Users\dbsr7927\AppData\Local\Continuum\Anaconda3
envs directories : C:\Users\dbsr7927\AppData\Local\Continuum\Anaconda3\envs
C:\Users\dbsr7927\AppData\Local\conda\conda\envs
C:\Users\dbsr7927\.conda\envs
package cache : C:\Users\dbsr7927\AppData\Local\Continuum\Anaconda3\pkgs
C:\Users\dbsr7927\AppData\Local\conda\conda\pkgs
channel URLs : https://conda.anaconda.org/conda-forge/win-64
https://conda.anaconda.org/conda-forge/noarch
https://repo.continuum.io/pkgs/free/win-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/win-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/win-64
https://repo.continuum.io/pkgs/pro/noarch
https://repo.continuum.io/pkgs/msys2/win-64
https://repo.continuum.io/pkgs/msys2/noarch
config file : None
netrc file : None
offline mode : False
user-agent : conda/4.3.21 requests/2.14.2 CPython/3.6.1 Windows/10 Windows/10.0.14393
administrator : False
`$ C:\Users\dbsr7927\AppData\Local\Continuum\Anaconda3\Scripts\conda-script.py install -c conda-forge scrapy`
Traceback (most recent call last):
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\exceptions.py", line 632, in conda_exception_handler
return_value = func(*args, **kwargs)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\cli\main.py", line 137, in _main
exit_code = args.func(args, p)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\cli\main_install.py", line 80, in execute
install(args, parser, 'install')
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\cli\install.py", line 357, in install
execute_actions(actions, index, verbose=not context.quiet)
File "C:\Users\dbsr7927\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\plan.py", line 830, in execute_actions
execute_instructions(plan, index, verbose)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\instructions.py", line 247, in execute_instructions
cmd(state, arg)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\instructions.py", line 108, in UNLINKLINKTRANSACTION_CMD
txn.execute()
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\core\link.py", line 263, in execute
self.verify()
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\core\link.py", line 242, in verify
self.prepare()
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\core\link.py", line 149, in prepare
for pkg_info in self.packages_info_to_link)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\core\link.py", line 149, in <genexpr>
for pkg_info in self.packages_info_to_link)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\core\link.py", line 54, in determine_link_type
if hardlink_supported(source_test_file, target_prefix):
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\_vendor\auxlib\decorators.py", line 56, in _memoized_func
result = func(*args, **kwargs)
File "C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\lib\site-packages\conda\gateways\disk\test.py", line 84, in hardlink_supported
assert not lexists(test_file), test_file
AssertionError: C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\.tmp.index.json
| The code being executed here is
```python
if lexists(test_file):
rm_rf(test_file)
assert not lexists(test_file), test_file
```
We definitely still have problems with `rm_rf` on Windows, perhaps related to https://github.com/conda/conda/issues/5154.
I'm submitting a PR that might fix future issues of this particular assertion error. It's not going to fix our more general problem with `rm_rf` on windows.
In the meantime, just manually deleting `C:\Users\xxxx\AppData\Local\Continuum\Anaconda3\.tmp.index.json` should get you on your way. | 2017-10-12T16:20:32 | -1.0 |
conda/conda | 6,221 | conda__conda-6221 | [
"6220"
] | ddf0446e73bcfc889f653d5d08b34bacfd9764ac | diff --git a/conda_env/env.py b/conda_env/env.py
--- a/conda_env/env.py
+++ b/conda_env/env.py
@@ -48,9 +48,9 @@ def from_environment(name, prefix, no_builds=False, ignore_channels=False):
pip_pkgs = sorted(installed - conda_pkgs)
if no_builds:
- dependencies = ['='.join(a.quad[0:3]) for a in sorted(conda_pkgs)]
+ dependencies = ['='.join((a.name, a.version)) for a in sorted(conda_pkgs)]
else:
- dependencies = ['='.join(a.quad[0:3]) for a in sorted(conda_pkgs)]
+ dependencies = ['='.join((a.name, a.version, a.build)) for a in sorted(conda_pkgs)]
if len(pip_pkgs) > 0:
dependencies.append({'pip': ['=='.join(a.rsplit('-', 2)[:2]) for a in pip_pkgs]})
# conda uses ruamel_yaml which returns a ruamel_yaml.comments.CommentedSeq
| diff --git a/tests/conda_env/test_cli.py b/tests/conda_env/test_cli.py
--- a/tests/conda_env/test_cli.py
+++ b/tests/conda_env/test_cli.py
@@ -8,6 +8,7 @@
from conda_env.exceptions import SpecNotFound
from conda_env.cli.main import create_parser
+from conda_env.yaml import load as yaml_load
from conda.base.context import context
from conda.base.constants import ROOT_ENV_NAME
@@ -243,7 +244,7 @@ def test_create_env(self):
run_conda_command(Commands.CREATE, test_env_name_2)
self.assertTrue(env_is_created(test_env_name_2))
- def test_export(self):
+ def test_env_export(self):
"""
Test conda env export
"""
@@ -257,11 +258,20 @@ def test_export(self):
env_yaml.write(snowflake)
env_yaml.flush()
env_yaml.close()
+
run_env_command(Commands.ENV_REMOVE, test_env_name_2)
self.assertFalse(env_is_created(test_env_name_2))
run_env_command(Commands.ENV_CREATE, env_yaml.name)
self.assertTrue(env_is_created(test_env_name_2))
+ # regression test for #6220
+ snowflake, e, = run_env_command(Commands.ENV_EXPORT, test_env_name_2, '--no-builds')
+ assert not e.strip()
+ env_description = yaml_load(snowflake)
+ assert len(env_description['dependencies'])
+ for spec_str in env_description['dependencies']:
+ assert spec_str.count('=') == 1
+
def test_list(self):
"""
Test conda list -e and conda create from txt
| Add flag to build environment.yml without build strings
https://gitter.im/conda/conda?at=59ef54ebe44c43700a70e9a4
https://twitter.com/drvinceknight/status/922837449092542464?ref_src=twsrc%5Etfw
> Due to hashes of packages being introduced in `envinronment.yml` I'm getting all sorts of issues with building envs from file. (Very new problem)
| Actually, looks like the flag might already be there as `--no-builds`
```
kfranz@0283:~/continuum/conda *4.3.x ❯ /conda/bin/conda env export --help
usage: conda-env export [-h] [-c CHANNEL] [--override-channels]
[-n ENVIRONMENT | -p PATH] [-f FILE] [--no-builds]
[--ignore-channels] [--json] [--debug] [--verbose]
Export a given environment
Options:
optional arguments:
-h, --help Show this help message and exit.
-c CHANNEL, --channel CHANNEL
Additional channel to include in the export
--override-channels Do not include .condarc channels
-n ENVIRONMENT, --name ENVIRONMENT
Name of environment (in
/conda/envs:/Users/kfranz/.conda/envs).
-p PATH, --prefix PATH
Full path to environment prefix (default: /conda).
-f FILE, --file FILE
--no-builds Remove build specification from dependencies
--ignore-channels Do not include channel names with package names.
--json Report all output as json. Suitable for using conda
programmatically.
--debug Show debug output.
--verbose, -v Use once for info, twice for debug, three times for
trace.
examples:
conda env export
conda env export --file SOME_FILE
```
But the `--no-builds` flag appears not to work, based on a spot test. | 2017-10-24T17:09:02 | -1.0 |
conda/conda | 6,274 | conda__conda-6274 | [
"6243"
] | 478137a45fcd12c80d93a441ecd4528535967c1a | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -282,7 +282,13 @@ def root_dir(self):
@property
def root_writable(self):
from ..gateways.disk.test import prefix_is_writable
- return prefix_is_writable(self.root_prefix)
+ try:
+ return prefix_is_writable(self.root_prefix)
+ except CondaError:
+ # With pyinstaller, conda code can sometimes be called even though sys.prefix isn't
+ # a conda environment with a conda-meta/ directory. In this case, it's safe to return
+ # False, because conda shouldn't itself be mutating that environment. See #6243
+ return False
@property
def envs_dirs(self):
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -191,7 +191,7 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
@pytest.mark.installed
def test_activate_deactivate(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 11, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2018, 1, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
@@ -208,7 +208,7 @@ def test_activate_deactivate(shell):
@pytest.mark.installed
def test_activate_root_simple(shell):
- if shell == "bash.exe" and datetime.now() < datetime(2017, 11, 1):
+ if shell == "bash.exe" and datetime.now() < datetime(2018, 1, 1):
pytest.xfail("fix this soon")
shell_vars = _format_vars(shell)
with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -552,7 +552,7 @@ def test_remove_all(self):
assert not exists(prefix)
@pytest.mark.skipif(on_win, reason="nomkl not present on windows")
- @pytest.mark.xfail(conda.__version__.startswith('4.3') and datetime.now() < datetime(2017, 11, 1), reason='currently broken in 4.3')
+ @pytest.mark.xfail(conda.__version__.startswith('4.3') and datetime.now() < datetime(2018, 1, 1), reason='currently broken in 4.3')
def test_remove_features(self):
with make_temp_env("python=2 numpy nomkl") as prefix:
assert exists(join(prefix, PYTHON_BINARY))
@@ -701,7 +701,7 @@ def test_update_deps_flag_absent(self):
assert package_is_installed(prefix, 'itsdangerous-0.23')
assert package_is_installed(prefix, 'flask')
- @pytest.mark.xfail(datetime.now() < datetime(2017, 11, 1), reason="#5263", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2018, 1, 1), reason="#5263", strict=True)
def test_update_deps_flag_present(self):
with make_temp_env("python=2 itsdangerous=0.23") as prefix:
assert package_is_installed(prefix, 'python-2')
diff --git a/tests/test_export.py b/tests/test_export.py
--- a/tests/test_export.py
+++ b/tests/test_export.py
@@ -31,7 +31,7 @@ def test_basic(self):
output2, error= run_command(Commands.LIST, prefix2, "-e")
self.assertEqual(output, output2)
- @pytest.mark.xfail(datetime.now() < datetime(2017, 11, 1), reason="Bring back `conda list --export` #3445", strict=True)
+ @pytest.mark.xfail(datetime.now() < datetime(2018, 1, 1), reason="Bring back `conda list --export` #3445", strict=True)
def test_multi_channel_export(self):
"""
When try to import from txt
diff --git a/tests/test_priority.py b/tests/test_priority.py
--- a/tests/test_priority.py
+++ b/tests/test_priority.py
@@ -15,7 +15,7 @@
class PriorityIntegrationTests(TestCase):
@pytest.mark.skipif(on_win, reason="xz packages are different on windows than unix")
- @pytest.mark.skipif(conda.__version__.startswith('4.3') and datetime.now() < datetime(2017, 11, 1),
+ @pytest.mark.skipif(conda.__version__.startswith('4.3') and datetime.now() < datetime(2018, 1, 1),
reason='currently broken in 4.3')
def test_channel_order_channel_priority_true(self):
with make_temp_env("python=3.5 pycosat==0.6.1") as prefix:
| test.py error "prefix is not writeable"
While attempting to use "pyinstaller" to create a standalone conda-index executable, the resulting binary failed in “conda/gateways/disk/test.py” prefix_is_writable because that method assumes the prefix is a python root directory, and in the case of the standalone, the “prefix” is the executable’s current directory. If I add append sys.executable to the end of the “find_first_existing” call parameter list, then it worked as expected.
Of course, this particular writability check seems unnecessary for conda-index, because the only writing is to the target, not the conda-meta directory.
Any change that would allow the creation of a standalone conda-index is appreciated.
| Adding @msarahan since we had an email conversation about this
Hey @tomashek, not opposed to adding `sys.executable` at the moment. It's possible it won't be completely future proof though, and it might be better to understand why conda-build's index needs to call that function. Can you attach the stack trace for the failure so we can see the code path?
Here is the stacktrace. Note that pyinstaller bundles the necessary files into a self-extracting executable that extracts to /tmp then executes the script from that location. That's why you see '/tmp/_MEI5zjz52' as the prefix. The first stack is only the relevant conda files. I also included the full stack trace that has the pyinstaller wrappers as well:
Traceback (most recent call last):
File "conda-index.py", line 7, in <module>
File "site-packages/conda_build/cli/main_index.py", line 7, in <module>
File "site-packages/conda_build/conda_interface.py", line 44, in <module>
File "site-packages/conda/exports.py", line 75, in <module>
File "site-packages/conda/config.py", line 70, in <module>
File "site-packages/conda/base/context.py", line 285, in root_writable
File "site-packages/conda/gateways/disk/test.py", line 70, in prefix_is_writable
conda.CondaError: Unable to determine if prefix '/tmp/_MEI5zjz52' is writable.
[19264] Failed to execute script conda-index
Traceback (most recent call last):
File "conda-index.py", line 7, in <module>
File "<frozen importlib._bootstrap>", line 969, in _find_and_load
File "<frozen importlib._bootstrap>", line 958, in _find_and_load_unlocked
File "<frozen importlib._bootstrap>", line 673, in _load_unlocked
File "/localdisk/work/miniconda3/lib/python3.5/site-packages/PyInstaller/loader/pyimod03_importers.py", line 631, in exec_module
exec(bytecode, module.__dict__)
File "site-packages/conda_build/cli/main_index.py", line 7, in <module>
File "<frozen importlib._bootstrap>", line 969, in _find_and_load
File "<frozen importlib._bootstrap>", line 958, in _find_and_load_unlocked
File "<frozen importlib._bootstrap>", line 673, in _load_unlocked
File "/localdisk/work/miniconda3/lib/python3.5/site-packages/PyInstaller/loader/pyimod03_importers.py", line 631, in exec_module
exec(bytecode, module.__dict__)
File "site-packages/conda_build/conda_interface.py", line 44, in <module>
File "<frozen importlib._bootstrap>", line 969, in _find_and_load
File "<frozen importlib._bootstrap>", line 958, in _find_and_load_unlocked
File "<frozen importlib._bootstrap>", line 673, in _load_unlocked
File "/localdisk/work/miniconda3/lib/python3.5/site-packages/PyInstaller/loader/pyimod03_importers.py", line 631, in exec_module
exec(bytecode, module.__dict__)
File "site-packages/conda/exports.py", line 75, in <module>
File "<frozen importlib._bootstrap>", line 969, in _find_and_load
File "<frozen importlib._bootstrap>", line 958, in _find_and_load_unlocked
File "<frozen importlib._bootstrap>", line 673, in _load_unlocked
File "/localdisk/work/miniconda3/lib/python3.5/site-packages/PyInstaller/loader/pyimod03_importers.py", line 631, in exec_module
exec(bytecode, module.__dict__)
File "site-packages/conda/config.py", line 70, in <module>
File "site-packages/conda/base/context.py", line 285, in root_writable
File "site-packages/conda/gateways/disk/test.py", line 70, in prefix_is_writable
conda.CondaError: Unable to determine if prefix '/tmp/_MEI5zjz52' is writable.
[19264] Failed to execute script conda-index
@kalefranz, any other ideas on a fix? Can the writability check be avoided for this operation? | 2017-11-07T19:37:26 | -1.0 |
conda/conda | 6,275 | conda__conda-6275 | [
"5680"
] | b934b58893b19c6d3a0b16322d4f7c020f5edcaf | diff --git a/conda_env/cli/main_create.py b/conda_env/cli/main_create.py
--- a/conda_env/cli/main_create.py
+++ b/conda_env/cli/main_create.py
@@ -110,7 +110,7 @@ def execute(args, parser):
sys.stderr.write(textwrap.dedent("""
Unable to install package for {0}.
- Please double check and ensure you dependencies file has
+ Please double check and ensure your dependencies file has
the correct spelling. You might also try installing the
conda-env-{0} package to see if provides the required
installer.
diff --git a/conda_env/env.py b/conda_env/env.py
--- a/conda_env/env.py
+++ b/conda_env/env.py
@@ -9,6 +9,7 @@
from conda.cli import common # TODO: this should never have to import form conda.cli
from conda.common.serialize import yaml_load
from conda.core.linked_data import linked
+from conda.models.match_spec import MatchSpec
from conda_env.yaml import dump
from . import compat, exceptions, yaml
from .pip_util import add_pip_installed
@@ -102,6 +103,12 @@ def parse(self):
else:
self['conda'].append(common.arg2spec(line))
+ if 'pip' in self:
+ if not self['pip']:
+ del self['pip']
+ if not any(MatchSpec(s).name == 'pip' for s in self['conda']):
+ self['conda'].append('pip')
+
# TODO only append when it's not already present
def add(self, package_name):
self.raw.append(package_name)
| diff --git a/tests/conda_env/test_env.py b/tests/conda_env/test_env.py
--- a/tests/conda_env/test_env.py
+++ b/tests/conda_env/test_env.py
@@ -90,7 +90,7 @@ def test_other_tips_of_dependencies_are_supported(self):
dependencies=['nltk', {'pip': ['foo', 'bar']}]
)
expected = OrderedDict([
- ('conda', ['nltk']),
+ ('conda', ['nltk', 'pip']),
('pip', ['foo', 'bar'])
])
self.assertEqual(e.dependencies, expected)
| Trivial pip subsection breaks conda env create
I observe that an empty-but-present pip subsection under dependencies breaks `conda env create`. I expect this command to run as if the `- pip:` subsection was not present.
```
$ cat environment.yml
dependencies:
- pip:
$ conda env create -n recreate --file=environment.yml
Fetching package metadata ...........
Solving package specifications: An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : linux-64
conda version : 4.3.13
conda is private : False
conda-env version : 4.3.13
conda-build version : 2.1.5
python version : 3.5.2.final.0
requests version : 2.13.0
root environment : /opt/ts/services/miniconda.ext_public_miniconda/dist (read only)
default environment : /opt/ts/services/miniconda.ext_public_miniconda/dist
envs directories : /nas/dft/ire/rhys/envs
/opt/ts/services/miniconda.ext_public_miniconda/dist/envs
/home/rhys/.conda/envs
package cache : /opt/ts/services/miniconda.ext_public_miniconda/dist/pkgs
/home/rhys/.conda/pkgs
channel URLs : http://python3.app.twosigma.com/conda/twosigma.com/ts/linux-64
http://python3.app.twosigma.com/conda/twosigma.com/ts/noarch
http://python3.app.twosigma.com/conda/twosigma.com/ext/linux-64
http://python3.app.twosigma.com/conda/twosigma.com/ext/noarch
http://python3.app.twosigma.com/conda/repo.continuum.io/pkgs/free/linux-64
http://python3.app.twosigma.com/conda/repo.continuum.io/pkgs/free/noarch
http://python3.app.twosigma.com/conda/repo.continuum.io/pkgs/pro/linux-64
http://python3.app.twosigma.com/conda/repo.continuum.io/pkgs/pro/noarch
config file : /home/rhys/.condarc
offline mode : False
user-agent : conda/4.3.13 requests/2.13.0 CPython/3.5.2 Linux/4.1.35-pv-ts2 debian/7.10 glibc/2.13
UID:GID : 11082:5000
`$ /opt/ts/services/miniconda.ext_public_miniconda/dist/bin/conda-env create -n recreate --file=environment.yml`
Traceback (most recent call last):
File "/opt/ts/services/miniconda.ext_public_miniconda/dist/lib/python3.5/site-packages/conda/exceptions.py", line 591, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/opt/ts/services/miniconda.ext_public_miniconda/dist/lib/python3.5/site-packages/conda_env/cli/main_create.py", line 108, in execute
installer.install(prefix, pkg_specs, args, env)
File "/opt/ts/services/miniconda.ext_public_miniconda/dist/lib/python3.5/site-packages/conda_env/installers/pip.py", line 8, in install
pip_cmd = pip_args(prefix) + ['install', ] + specs
TypeError: unsupported operand type(s) for +: 'NoneType' and 'list'
```
| Same here, that's because `pip_args` [returns `None`](https://github.com/conda/conda/blob/master/conda_env/pip_util.py#L38) when pip is not installed; While it's actually used find the [pip command and chains it with arguments](https://github.com/conda/conda/blob/master/conda_env/installers/pip.py#L40).
These seem to be used in [main_create](https://github.com/conda/conda/blob/master/conda_env/cli/main_update.py#L107) and [main_update](https://github.com/conda/conda/blob/master/conda_env/cli/main_update.py#L107) without proper checking (or requirement) for the installer to be available.
Can you verify that this is still a bug as of conda 4.3.30? 4.3.13 is very old at this point.
> Can you verify that this is still a bug as of conda 4.3.30? 4.3.13 is very old at this point.
Sorry , I tested on the latest without saying it;
```
$ conda env create -n whatever -f foo.yml
Using Anaconda API: https://api.anaconda.org
Fetching package metadata .............
Solving package specifications: .
An unexpected error has occurred.
Please consider posting the following information to the
conda GitHub issue tracker at:
https://github.com/conda/conda/issues
Current conda install:
platform : osx-64
conda version : 4.3.30
conda is private : False
conda-env version : 4.3.30
conda-build version : 2.1.17
python version : 3.6.3.final.0
requests version : 2.18.4
root environment : /Users/bussonniermatthias/anaconda (writable)
default environment : /Users/bussonniermatthias/anaconda
envs directories : /Users/bussonniermatthias/anaconda/envs
/Users/bussonniermatthias/.conda/envs
package cache : /Users/bussonniermatthias/anaconda/pkgs
/Users/bussonniermatthias/.conda/pkgs
channel URLs : https://conda.anaconda.org/conda-forge/osx-64
https://conda.anaconda.org/conda-forge/noarch
https://repo.continuum.io/pkgs/main/osx-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/osx-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/osx-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/osx-64
https://repo.continuum.io/pkgs/pro/noarch
config file : /Users/bussonniermatthias/.condarc
netrc file : /Users/bussonniermatthias/.netrc
offline mode : False
user-agent : conda/4.3.30 requests/2.18.4 CPython/3.6.3 Darwin/16.7.0 OSX/10.12.6
UID:GID : 501:20
`$ /Users/bussonniermatthias/anaconda/bin/conda-env create -n whatever -f foo.yml`
Traceback (most recent call last):
File "/Users/bussonniermatthias/anaconda/lib/python3.6/site-packages/conda/exceptions.py", line 640, in conda_exception_handler
return_value = func(*args, **kwargs)
File "/Users/bussonniermatthias/anaconda/lib/python3.6/site-packages/conda_env/cli/main_create.py", line 108, in execute
installer.install(prefix, pkg_specs, args, env)
File "/Users/bussonniermatthias/anaconda/lib/python3.6/site-packages/conda_env/installers/pip.py", line 8, in install
pip_cmd = pip_args(prefix) + ['install', ] + specs
TypeError: unsupported operand type(s) for +: 'NoneType' and 'list'
$ cat foo.yml
name: whatever
channels:
- conda-forge
dependencies:
- python=3.6
- ipython
#- pip # uncomment and it works
- pip:
- toml
```
The example I give for env.yml is the the trivial one (just to make sure install still works), but the reason is the same; the place I linked to in my previous comment don't check for None (hence the crash), and arguably `pip_args` should maybe also be type stable.
Same thing happen with updating an env.
Also, please consider making pip a default package. The number of users that
```
$ conda create env
$ source activate env
(env) $ pip install <whatever>
```
And don't understand why whatever is not working is large. Even I, who know what' going on get confused sometime; At least inject a fake `pip` executabe that just return a status of -1 and print "please conda install pip".
| 2017-11-07T21:36:36 | -1.0 |
conda/conda | 6,295 | conda__conda-6295 | [
"6220",
"6243"
] | 824ffae0ae9344a99343fe7aafbcefa89a74544c | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -11,7 +11,7 @@
from .constants import (APP_NAME, DEFAULTS_CHANNEL_NAME, DEFAULT_CHANNELS, DEFAULT_CHANNEL_ALIAS,
ERROR_UPLOAD_URL, PLATFORM_DIRECTORIES, PathConflict, ROOT_ENV_NAME,
SEARCH_PATH, SafetyChecks)
-from .. import __version__ as CONDA_VERSION
+from .. import __version__ as CONDA_VERSION, CondaError
from .._vendor.appdirs import user_data_dir
from .._vendor.auxlib.collection import frozendict
from .._vendor.auxlib.decorators import memoize, memoizedproperty
@@ -312,7 +312,13 @@ def root_dir(self):
@property
def root_writable(self):
from ..gateways.disk.test import prefix_is_writable
- return prefix_is_writable(self.root_prefix)
+ try:
+ return prefix_is_writable(self.root_prefix)
+ except CondaError: # pragma: no cover
+ # With pyinstaller, conda code can sometimes be called even though sys.prefix isn't
+ # a conda environment with a conda-meta/ directory. In this case, it's safe to return
+ # False, because conda shouldn't itself be mutating that environment. See #6243
+ return False
@property
def envs_dirs(self):
diff --git a/conda/resolve.py b/conda/resolve.py
--- a/conda/resolve.py
+++ b/conda/resolve.py
@@ -570,9 +570,13 @@ def generate_package_count(self, C, missing):
return {self.push_MatchSpec(C, nm): 1 for nm in missing}
def generate_version_metrics(self, C, specs, include0=False):
- eqc = {} # a C.minimize() objective: Dict[varname, coeff]
- eqv = {} # a C.minimize() objective: Dict[varname, coeff]
- eqb = {} # a C.minimize() objective: Dict[varname, coeff]
+ # each of these are weights saying how well packages match the specs
+ # format for each: a C.minimize() objective: Dict[varname, coeff]
+ eqc = {} # channel
+ eqv = {} # version
+ eqb = {} # build number
+ eqt = {} # timestamp
+
sdict = {} # Dict[package_name, Dist]
for s in specs:
@@ -594,18 +598,21 @@ def generate_version_metrics(self, C, specs, include0=False):
if targets and any(dist == t for t in targets):
continue
if pkey is None:
- ic = iv = ib = 0
+ ic = iv = ib = it = 0
# valid package, channel priority
elif pkey[0] != version_key[0] or pkey[1] != version_key[1]:
ic += 1
- iv = ib = 0
+ iv = ib = it = 0
# version
elif pkey[2] != version_key[2]:
iv += 1
- ib = 0
- # build number, timestamp
- elif pkey[3] != version_key[3] or pkey[4] != version_key[4]:
+ ib = it = 0
+ # build number
+ elif pkey[3] != version_key[3]:
ib += 1
+ it = 0
+ elif pkey[4] != version_key[4]:
+ it += 1
if ic or include0:
eqc[dist.full_name] = ic
@@ -613,9 +620,11 @@ def generate_version_metrics(self, C, specs, include0=False):
eqv[dist.full_name] = iv
if ib or include0:
eqb[dist.full_name] = ib
+ if it or include0:
+ eqt[dist.full_name] = it
pkey = version_key
- return eqc, eqv, eqb
+ return eqc, eqv, eqb, eqt
def dependency_sort(self, must_have):
# type: (Dict[package_name, Dist]) -> List[Dist]
@@ -865,10 +874,10 @@ def mysat(specs, add_if=False):
log.debug('Package removal metric: %d', obj7)
# Requested packages: maximize versions
- eq_req_c, eq_req_v, eq_req_b = r2.generate_version_metrics(C, specr)
+ eq_req_c, eq_req_v, eq_req_b, eq_req_t = r2.generate_version_metrics(C, specr)
solution, obj3a = C.minimize(eq_req_c, solution)
solution, obj3 = C.minimize(eq_req_v, solution)
- log.debug('Initial package version metric: %d/%d', obj3a, obj3)
+ log.debug('Initial package channel/version metric: %d/%d', obj3a, obj3)
# Track features: minimize feature count
eq_feature_count = r2.generate_feature_count(C)
@@ -897,11 +906,17 @@ def mysat(specs, add_if=False):
log.debug('Dependency update count: %d', obj50)
# Remaining packages: maximize versions, then builds
- eq_c, eq_v, eq_b = r2.generate_version_metrics(C, speca)
+ eq_c, eq_v, eq_b, eq_t = r2.generate_version_metrics(C, speca)
solution, obj5a = C.minimize(eq_c, solution)
solution, obj5 = C.minimize(eq_v, solution)
solution, obj6 = C.minimize(eq_b, solution)
- log.debug('Additional package version/build metrics: %d/%d/%d', obj5a, obj5, obj6)
+ log.debug('Additional package channel/version/build metrics: %d/%d/%d',
+ obj5a, obj5, obj6)
+
+ # Maximize timestamps
+ eq_t.update(eq_req_t)
+ solution, obj6t = C.minimize(eq_t, solution)
+ log.debug('Timestamp metric: %d', obj6t)
# Prune unnecessary packages
eq_c = r2.generate_package_count(C, specm)
diff --git a/conda_env/env.py b/conda_env/env.py
--- a/conda_env/env.py
+++ b/conda_env/env.py
@@ -51,9 +51,9 @@ def from_environment(name, prefix, no_builds=False, ignore_channels=False):
pip_pkgs = sorted(installed - conda_pkgs)
if no_builds:
- dependencies = ['='.join(a.quad[0:3]) for a in sorted(conda_pkgs)]
+ dependencies = ['='.join((a.name, a.version)) for a in sorted(conda_pkgs)]
else:
- dependencies = ['='.join(a.quad[0:3]) for a in sorted(conda_pkgs)]
+ dependencies = ['='.join((a.name, a.version, a.build)) for a in sorted(conda_pkgs)]
if len(pip_pkgs) > 0:
dependencies.append({'pip': ['=='.join(a.rsplit('-', 2)[:2]) for a in pip_pkgs]})
# conda uses ruamel_yaml which returns a ruamel_yaml.comments.CommentedSeq
| diff --git a/conda/gateways/disk/test.py b/conda/gateways/disk/test.py
--- a/conda/gateways/disk/test.py
+++ b/conda/gateways/disk/test.py
@@ -4,6 +4,7 @@
from logging import getLogger
from os import W_OK, access
from os.path import basename, dirname, isdir, isfile, join
+from uuid import uuid4
from .create import create_link
from .delete import rm_rf, try_rmdir_all_empty
@@ -12,6 +13,7 @@
from .update import touch
from ..._vendor.auxlib.decorators import memoize
from ...base.constants import PREFIX_MAGIC_FILE
+from ...common.compat import text_type
from ...common.path import expand, get_python_short_path
from ...models.enums import LinkType
@@ -91,7 +93,7 @@ def hardlink_supported(source_file, dest_dir):
# file system configuration, a symbolic link may be created
# instead. If a symbolic link is created instead of a hard link,
# return False.
- test_file = join(dest_dir, '.tmp.' + basename(source_file))
+ test_file = join(dest_dir, '.tmp.%s.%s' % (basename(source_file), text_type(uuid4())[:8]))
assert isfile(source_file), source_file
assert isdir(dest_dir), dest_dir
if lexists(test_file):
diff --git a/tests/conda_env/test_cli.py b/tests/conda_env/test_cli.py
--- a/tests/conda_env/test_cli.py
+++ b/tests/conda_env/test_cli.py
@@ -16,6 +16,7 @@
from conda.install import rm_rf
from conda_env.cli.main import create_parser
from conda_env.exceptions import SpecNotFound
+from conda_env.yaml import load as yaml_load
environment_1 = '''
name: env-1
@@ -240,7 +241,7 @@ def test_create_remove_env(self):
self.assertFalse(env_is_created(test_env_name_3))
- def test_export(self):
+ def test_env_export(self):
"""
Test conda env export
"""
@@ -254,11 +255,20 @@ def test_export(self):
env_yaml.write(snowflake)
env_yaml.flush()
env_yaml.close()
+
run_env_command(Commands.ENV_REMOVE, test_env_name_2)
self.assertFalse(env_is_created(test_env_name_2))
run_env_command(Commands.ENV_CREATE, env_yaml.name)
self.assertTrue(env_is_created(test_env_name_2))
+ # regression test for #6220
+ snowflake, e, = run_env_command(Commands.ENV_EXPORT, test_env_name_2, '--no-builds')
+ assert not e.strip()
+ env_description = yaml_load(snowflake)
+ assert len(env_description['dependencies'])
+ for spec_str in env_description['dependencies']:
+ assert spec_str.count('=') == 1
+
def test_list(self):
"""
Test conda list -e and conda create from txt
diff --git a/tests/test_resolve.py b/tests/test_resolve.py
--- a/tests/test_resolve.py
+++ b/tests/test_resolve.py
@@ -158,7 +158,7 @@ def test_generate_eq_1():
reduced_index = r.get_reduced_index(['anaconda'])
r2 = Resolve(reduced_index, True, True)
C = r2.gen_clauses()
- eqc, eqv, eqb = r2.generate_version_metrics(C, list(r2.groups.keys()))
+ eqc, eqv, eqb, eqt = r2.generate_version_metrics(C, list(r2.groups.keys()))
# Should satisfy the following criteria:
# - lower versions of the same package should should have higher
# coefficients.
@@ -170,6 +170,7 @@ def test_generate_eq_1():
eqc = {Dist(key).to_filename(): value for key, value in iteritems(eqc)}
eqv = {Dist(key).to_filename(): value for key, value in iteritems(eqv)}
eqb = {Dist(key).to_filename(): value for key, value in iteritems(eqb)}
+ eqt = {Dist(key).to_filename(): value for key, value in iteritems(eqt)}
assert eqc == {}
assert eqv == {
'accelerate-1.0.0-np15py26_p0.tar.bz2': 2,
@@ -483,6 +484,9 @@ def test_generate_eq_1():
'zeromq-2.2.0-0.tar.bz2': 1,
}
+ # No timestamps in the current data set
+ assert eqt == {}
+
def test_unsat():
# scipy 0.12.0b1 is not built for numpy 1.5, only 1.6 and 1.7
@@ -499,6 +503,48 @@ def test_nonexistent():
assert raises(ResolvePackageNotFound, lambda: r.install(['numpy 1.5']))
+def test_timestamps_and_deps():
+ # If timestamp maximization is performed too early in the solve optimization,
+ # it will force unnecessary changes to dependencies. Timestamp maximization needs
+ # to be done at low priority so that conda is free to consider packages with the
+ # same version and build that are most compatible with the installed environment.
+ index2 = {Dist(key): value for key, value in iteritems(index)}
+ index2[Dist('mypackage-1.0-hash12_0.tar.bz2')] = IndexRecord(**{
+ 'build': 'hash27_0',
+ 'build_number': 0,
+ 'depends': ['libpng 1.2.*'],
+ 'name': 'mypackage',
+ 'requires': ['libpng 1.2.*'],
+ 'version': '1.0',
+ 'timestamp': 1,
+ })
+ index2[Dist('mypackage-1.0-hash15_0.tar.bz2')] = IndexRecord(**{
+ 'build': 'hash15_0',
+ 'build_number': 0,
+ 'depends': ['libpng 1.5.*'],
+ 'name': 'mypackage',
+ 'requires': ['libpng 1.5.*'],
+ 'version': '1.0',
+ 'timestamp': 0,
+ })
+ r = Resolve(index2)
+ installed1 = r.install(['libpng 1.2.*', 'mypackage'])
+ print([k.dist_name for k in installed1])
+ assert any(k.name == 'libpng' and k.version.startswith('1.2') for k in installed1)
+ assert any(k.name == 'mypackage' and k.build == 'hash12_0' for k in installed1)
+ installed2 = r.install(['libpng 1.5.*', 'mypackage'])
+ assert any(k.name == 'libpng' and k.version.startswith('1.5') for k in installed2)
+ assert any(k.name == 'mypackage' and k.build == 'hash15_0' for k in installed2)
+ # this is testing that previously installed reqs are not disrupted by newer timestamps.
+ # regression test of sorts for https://github.com/conda/conda/issues/6271
+ installed3 = r.install(['mypackage'], r.install(['libpng 1.2.*']))
+ assert installed1 == installed3
+ installed4 = r.install(['mypackage'], r.install(['libpng 1.5.*']))
+ assert installed2 == installed4
+ # unspecified python version should maximize libpng (v1.5), even though it has a lower timestamp
+ installed5 = r.install(['mypackage'])
+ assert installed2 == installed5
+
def test_nonexistent_deps():
index2 = index.copy()
index2['mypackage-1.0-py33_0.tar.bz2'] = IndexRecord(**{
@@ -1169,7 +1215,7 @@ def test_channel_priority_2():
dists = this_r.get_reduced_index(spec)
r2 = Resolve(dists, True, True, channels=channels)
C = r2.gen_clauses()
- eqc, eqv, eqb = r2.generate_version_metrics(C, list(r2.groups.keys()))
+ eqc, eqv, eqb, eqt = r2.generate_version_metrics(C, list(r2.groups.keys()))
eqc = {str(Dist(key)): value for key, value in iteritems(eqc)}
assert eqc == {
'channel-3::openssl-1.0.1c-0': 1,
@@ -1266,7 +1312,7 @@ def test_channel_priority_2():
dists = this_r.get_reduced_index(spec)
r2 = Resolve(dists, True, True, channels=channels)
C = r2.gen_clauses()
- eqc, eqv, eqb = r2.generate_version_metrics(C, list(r2.groups.keys()))
+ eqc, eqv, eqb, eqt = r2.generate_version_metrics(C, list(r2.groups.keys()))
eqc = {str(Dist(key)): value for key, value in iteritems(eqc)}
assert eqc == {
'channel-1::accelerate-1.0.0-np16py27_p0': 2,
| Add flag to build environment.yml without build strings
https://gitter.im/conda/conda?at=59ef54ebe44c43700a70e9a4
https://twitter.com/drvinceknight/status/922837449092542464?ref_src=twsrc%5Etfw
> Due to hashes of packages being introduced in `envinronment.yml` I'm getting all sorts of issues with building envs from file. (Very new problem)
test.py error "prefix is not writeable"
While attempting to use "pyinstaller" to create a standalone conda-index executable, the resulting binary failed in “conda/gateways/disk/test.py” prefix_is_writable because that method assumes the prefix is a python root directory, and in the case of the standalone, the “prefix” is the executable’s current directory. If I add append sys.executable to the end of the “find_first_existing” call parameter list, then it worked as expected.
Of course, this particular writability check seems unnecessary for conda-index, because the only writing is to the target, not the conda-meta directory.
Any change that would allow the creation of a standalone conda-index is appreciated.
| 2017-11-13T21:43:58 | -1.0 |
|
conda/conda | 6,352 | conda__conda-6352 | [
"5827"
] | 58f340d45f87a3bebfcd6f2058e21aabb99f2021 | diff --git a/conda/cli/conda_argparse.py b/conda/cli/conda_argparse.py
--- a/conda/cli/conda_argparse.py
+++ b/conda/cli/conda_argparse.py
@@ -122,14 +122,13 @@ def error(self, message):
else:
argument = None
if argument and argument.dest == "cmd":
- m = re.compile(r"invalid choice: u?'([\w\-]+)'").match(exc.message)
+ m = re.match(r"invalid choice: u?'(\w+)'", exc.message)
if m:
cmd = m.group(1)
executable = find_executable('conda-' + cmd)
if not executable:
from ..exceptions import CommandNotFoundError
raise CommandNotFoundError(cmd)
-
args = [find_executable('conda-' + cmd)]
args.extend(sys.argv[2:])
os.execv(args[0], args)
@@ -140,10 +139,13 @@ def print_help(self):
super(ArgumentParser, self).print_help()
if sys.argv[1:] in ([], ['help'], ['-h'], ['--help']):
- print(dedent("""
- other commands, such as "conda build", are available when additional conda
- packages (e.g. conda-build) are installed
- """))
+ from .find_commands import find_commands
+ other_commands = find_commands()
+ if other_commands:
+ builder = ['']
+ builder.append("conda commands available from other packages:")
+ builder.extend(' %s' % cmd for cmd in sorted(other_commands))
+ print('\n'.join(builder))
class NullCountAction(_CountAction):
diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -170,6 +170,21 @@ def __init__(self, target_path, incompatible_package_dists, context):
class CommandNotFoundError(CondaError):
def __init__(self, command):
+ conda_commands = {
+ 'clean',
+ 'config',
+ 'create',
+ 'help',
+ 'info',
+ 'install',
+ 'list',
+ 'package',
+ 'remove',
+ 'search',
+ 'uninstall',
+ 'update',
+ 'upgrade',
+ }
build_commands = {
'build',
'convert',
@@ -180,22 +195,19 @@ def __init__(self, command):
'render',
'skeleton',
}
- needs_source = {
- 'activate',
- 'deactivate'
- }
if command in build_commands:
- message = dals("""
- You need to install conda-build in order to
- use the 'conda %(command)s' command.
- """)
- elif command in needs_source and not on_win:
- message = dals("""
- '%(command)s is not a conda command.
- Did you mean 'source %(command)s'?
- """)
+ message = "To use 'conda %(command)s', install conda-build."
else:
- message = "'%(command)s'"
+ from difflib import get_close_matches
+ from .cli.find_commands import find_commands
+ message = "Error: No command 'conda %(command)s'."
+ choices = conda_commands | build_commands | set(find_commands())
+ close = get_close_matches(command, choices)
+ if close:
+ message += "\nDid you mean 'conda %s'?" % close[0]
+ from .base.context import context
+ from .cli.main import init_loggers
+ init_loggers(context)
super(CommandNotFoundError, self).__init__(message, command=command)
| diff --git a/tests/test_exceptions.py b/tests/test_exceptions.py
--- a/tests/test_exceptions.py
+++ b/tests/test_exceptions.py
@@ -338,7 +338,8 @@ def test_CommandNotFoundError_simple(self):
conda_exception_handler(_raise_helper, exc)
assert not c.stdout
- assert c.stderr.strip() == "CommandNotFoundError: 'instate'"
+ assert c.stderr.strip() == ("CommandNotFoundError: Error: No command 'conda instate'.\n"
+ "Did you mean 'conda install'?")
def test_CommandNotFoundError_conda_build(self):
cmd = "build"
@@ -359,35 +360,7 @@ def test_CommandNotFoundError_conda_build(self):
conda_exception_handler(_raise_helper, exc)
assert not c.stdout
- assert c.stderr.strip() == ("CommandNotFoundError: You need to install conda-build in order to\n" \
- "use the 'conda build' command.")
-
- def test_CommandNotFoundError_activate(self):
- cmd = "activate"
- exc = CommandNotFoundError(cmd)
-
- with env_var("CONDA_JSON", "yes", reset_context):
- with captured() as c:
- conda_exception_handler(_raise_helper, exc)
-
- json_obj = json.loads(c.stdout)
- assert not c.stderr
- assert json_obj['exception_type'] == "<class 'conda.exceptions.CommandNotFoundError'>"
- assert json_obj['message'] == text_type(exc)
- assert json_obj['error'] == repr(exc)
-
- with env_var("CONDA_JSON", "no", reset_context):
- with captured() as c:
- conda_exception_handler(_raise_helper, exc)
-
- assert not c.stdout
-
- if on_win:
- message = "CommandNotFoundError: 'activate'"
- else:
- message = ("CommandNotFoundError: 'activate is not a conda command.\n"
- "Did you mean 'source activate'?")
- assert c.stderr.strip() == message
+ assert c.stderr.strip() == ("CommandNotFoundError: To use 'conda build', install conda-build.")
@patch('requests.post', side_effect=(
AttrDict(headers=AttrDict(Location='somewhere.else'), status_code=302,
| No error generated on non-existent sub-command
```
[root@70a337fa2e68 build_scripts]# conda info
active environment : None
user config file : /root/.condarc
populated config files : /opt/miniconda/.condarc
conda version : 4.4.0rc0
conda-build version : 3.0.9
python version : 3.6.1.final.0
base environment : /opt/miniconda (writable)
channel URLs : https://conda.anaconda.org/c3i_test/linux-64
https://conda.anaconda.org/c3i_test/noarch
https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
package cache : /opt/miniconda/pkgs
/root/.conda/pkgs
envs directories : /opt/miniconda/envs
/root/.conda/envs
platform : linux-64
user-agent : conda/4.4.0rc0 requests/2.14.2 CPython/3.6.1 Linux/3.10.0-514.el7.x86_64 centos/6.9 glibc/2.12
UID:GID : 0:0
netrc file : None
offline mode : False
[root@70a337fa2e68 build_scripts]# conda this-is-not-a-subcommand
[root@70a337fa2e68 build_scripts]# echo $?
1
```
| We just need to switch conda over to click already. | 2017-11-28T03:25:08 | -1.0 |
conda/conda | 6,363 | conda__conda-6363 | [
"6181"
] | a3254b18f1d7a379620f502ec6dd7e845cb03ae8 | diff --git a/conda/core/solve.py b/conda/core/solve.py
--- a/conda/core/solve.py
+++ b/conda/core/solve.py
@@ -1,12 +1,11 @@
# -*- coding: utf-8 -*-
from __future__ import absolute_import, division, print_function, unicode_literals
+from enum import Enum
from genericpath import exists
from logging import DEBUG, getLogger
from os.path import join
-from enum import Enum
-
from .index import get_reduced_index
from .link import PrefixSetup, UnlinkLinkTransaction
from .linked_data import PrefixData, linked_data
@@ -15,7 +14,7 @@
from ..common.compat import iteritems, itervalues, odict, string_types, text_type
from ..common.constants import NULL
from ..common.io import spinner
-from ..common.path import paths_equal
+from ..common.path import get_major_minor_version, paths_equal
from ..exceptions import PackagesNotFoundError
from ..gateways.logging import TRACE
from ..history import History
@@ -235,6 +234,16 @@ def solve_final_state(self, deps_modifier=NULL, prune=NULL, ignore_pinned=NULL,
specs_map = {pkg_name: MatchSpec(spec.name, optional=spec.optional)
for pkg_name, spec in iteritems(specs_map)}
+ # As a business rule, we never want to update python beyond the current minor version,
+ # unless that's requested explicitly by the user (which we actively discourage).
+ if 'python' in specs_map:
+ python_prefix_rec = prefix_data.get('python')
+ if python_prefix_rec:
+ python_spec = specs_map['python']
+ if not python_spec.get('version'):
+ pinned_version = get_major_minor_version(python_prefix_rec.version) + '.*'
+ specs_map['python'] = MatchSpec(python_spec, version=pinned_version)
+
# For the aggressive_update_packages configuration parameter, we strip any target
# that's been set.
if not context.offline:
| diff --git a/tests/core/test_solve.py b/tests/core/test_solve.py
--- a/tests/core/test_solve.py
+++ b/tests/core/test_solve.py
@@ -467,12 +467,11 @@ def test_update_all_1():
'channel-1::tk-8.5.13-0',
'channel-1::zlib-1.2.7-0',
'channel-1::llvm-3.2-0',
- 'channel-1::python-2.7.5-0',
- 'channel-1::llvmpy-0.10.2-py27_0',
- 'channel-1::meta-0.4.2.dev-py27_0',
- 'channel-1::nose-1.3.0-py27_0',
- 'channel-1::numpy-1.7.1-py27_0',
- 'channel-1::numba-0.6.0-np17py27_0'
+ 'channel-1::python-2.6.8-6', # stick with python=2.6 even though UPDATE_ALL
+ 'channel-1::llvmpy-0.10.2-py26_0',
+ 'channel-1::nose-1.3.0-py26_0',
+ 'channel-1::numpy-1.7.1-py26_0',
+ 'channel-1::numba-0.6.0-np17py26_0',
)
assert tuple(final_state_2) == tuple(solver._index[Dist(d)] for d in order)
@@ -1336,7 +1335,6 @@ def test_freeze_deps_1():
)
assert tuple(final_state_1) == tuple(solver._index[Dist(d)] for d in order)
- # to keep six=1.7 as a requested spec, we have to downgrade python to 2.7
specs_to_add = MatchSpec("bokeh"),
with get_solver_2(specs_to_add, prefix_records=final_state_1, history_specs=specs) as solver:
final_state_2 = solver.solve_final_state()
@@ -1351,23 +1349,18 @@ def test_freeze_deps_1():
'channel-2::xz-5.2.2-1',
'channel-2::yaml-0.1.6-0',
'channel-2::zlib-1.2.8-3',
- 'channel-2::python-2.7.13-0',
- 'channel-2::backports-1.0-py27_0',
- 'channel-2::backports_abc-0.5-py27_0',
- 'channel-2::futures-3.1.1-py27_0',
- 'channel-2::markupsafe-0.23-py27_2',
- 'channel-2::numpy-1.13.0-py27_0',
- 'channel-2::pyyaml-3.12-py27_0',
- 'channel-2::requests-2.14.2-py27_0',
- 'channel-2::setuptools-27.2.0-py27_0',
- 'channel-2::six-1.7.3-py27_0',
- 'channel-2::bkcharts-0.2-py27_0',
- 'channel-2::jinja2-2.9.6-py27_0',
- 'channel-2::python-dateutil-2.6.0-py27_0',
- 'channel-2::singledispatch-3.4.0.3-py27_0',
- 'channel-2::ssl_match_hostname-3.4.0.2-py27_1',
- 'channel-2::tornado-4.5.1-py27_0',
- 'channel-2::bokeh-0.12.6-py27_0',
+ 'channel-2::python-3.4.5-0',
+ 'channel-2::backports_abc-0.5-py34_0',
+ 'channel-2::markupsafe-0.23-py34_2',
+ 'channel-2::numpy-1.13.0-py34_0',
+ 'channel-2::pyyaml-3.12-py34_0',
+ 'channel-2::requests-2.14.2-py34_0',
+ 'channel-2::setuptools-27.2.0-py34_0',
+ 'channel-2::six-1.7.3-py34_0',
+ 'channel-2::jinja2-2.9.6-py34_0',
+ 'channel-2::python-dateutil-2.6.0-py34_0',
+ 'channel-2::tornado-4.4.2-py34_0',
+ 'channel-2::bokeh-0.12.4-py34_0',
)
assert tuple(final_state_2) == tuple(solver._index[Dist(d)] for d in order)
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -898,13 +898,13 @@ def test_package_pinning(self):
run_command(Commands.UPDATE, prefix, "--all")
assert package_is_installed(prefix, "itsdangerous-0.23")
# assert not package_is_installed(prefix, "python-3.5") # should be python-3.6, but it's not because of add_defaults_to_specs
- assert not package_is_installed(prefix, "python-2.7") # add_defaults_to_specs is right now removed for python pinning, TODO: discuss
+ assert package_is_installed(prefix, "python-2.7")
assert not package_is_installed(prefix, "pytz-2015.7")
assert package_is_installed(prefix, "pytz-")
run_command(Commands.UPDATE, prefix, "--all --no-pin")
- assert not package_is_installed(prefix, "python-2.7")
+ assert package_is_installed(prefix, "python-2.7")
assert not package_is_installed(prefix, "itsdangerous-0.23")
def test_package_optional_pinning(self):
| make sure conda 4.4 has soft-pinning for python
https://github.com/conda/conda/issues/6164#issuecomment-336733311
| 2017-11-29T15:27:57 | -1.0 |
|
conda/conda | 6,364 | conda__conda-6364 | [
"5417"
] | aba43f2015c8713a236d858aa91e7b3205d33845 | diff --git a/conda/core/solve.py b/conda/core/solve.py
--- a/conda/core/solve.py
+++ b/conda/core/solve.py
@@ -1,15 +1,14 @@
# -*- coding: utf-8 -*-
from __future__ import absolute_import, division, print_function, unicode_literals
+from enum import Enum
from genericpath import exists
from logging import DEBUG, getLogger
from os.path import join
-from enum import Enum
-
from .index import get_reduced_index
from .link import PrefixSetup, UnlinkLinkTransaction
-from .linked_data import PrefixData, linked_data
+from .linked_data import PrefixData
from .._vendor.boltons.setutils import IndexedSet
from ..base.context import context
from ..common.compat import iteritems, itervalues, odict, string_types, text_type
@@ -380,7 +379,11 @@ def solve_for_diff(self, deps_modifier=NULL, prune=NULL, ignore_pinned=NULL,
"""
final_precs = self.solve_final_state(deps_modifier, prune, ignore_pinned, force_remove)
- previous_records = IndexedSet(itervalues(linked_data(self.prefix)))
+ previous_records = IndexedSet(self._index[d] for d in self._r.dependency_sort(
+ {prefix_rec.name: Dist(prefix_rec)
+ for prefix_rec in PrefixData(self.prefix).iter_records()}
+ ))
+
unlink_precs = previous_records - final_precs
link_precs = final_precs - previous_records
@@ -397,6 +400,9 @@ def solve_for_diff(self, deps_modifier=NULL, prune=NULL, ignore_pinned=NULL,
# TODO: force_reinstall might not yet be fully implemented in :meth:`solve_final_state`,
# at least as described in the docstring.
+ unlink_precs = IndexedSet(reversed(sorted(unlink_precs,
+ key=lambda x: previous_records.index(x))))
+ link_precs = IndexedSet(sorted(link_precs, key=lambda x: final_precs.index(x)))
return unlink_precs, link_precs
def solve_for_transaction(self, deps_modifier=NULL, prune=NULL, ignore_pinned=NULL,
| diff --git a/tests/core/test_solve.py b/tests/core/test_solve.py
--- a/tests/core/test_solve.py
+++ b/tests/core/test_solve.py
@@ -170,49 +170,49 @@ def test_prune_1():
specs_to_remove = MatchSpec("numbapro"),
with get_solver(specs_to_remove=specs_to_remove, prefix_records=final_state_1,
history_specs=specs) as solver:
- final_state_2 = solver.solve_final_state(prune=False)
+ unlink_precs, link_precs = solver.solve_for_diff(prune=False)
# PrefixDag(final_state_2, specs).open_url()
- print([Dist(rec).full_name for rec in final_state_2])
- order = (
- 'channel-1::libnvvm-1.0-p0',
- 'channel-1::openssl-1.0.1c-0',
- 'channel-1::readline-6.2-0',
- 'channel-1::sqlite-3.7.13-0',
- 'channel-1::system-5.8-1',
- 'channel-1::tk-8.5.13-0',
- 'channel-1::zlib-1.2.7-0',
- 'channel-1::llvm-3.2-0',
- 'channel-1::mkl-rt-11.0-p0',
- 'channel-1::python-2.7.3-7',
- 'channel-1::bitarray-0.8.1-py27_0',
- 'channel-1::llvmpy-0.11.2-py27_0',
- 'channel-1::meta-0.4.2.dev-py27_0',
- 'channel-1::mkl-service-1.0.0-py27_p0',
- 'channel-1::numpy-1.6.2-py27_p4',
- 'channel-1::numba-0.8.1-np16py27_0',
- 'channel-1::numexpr-2.1-np16py27_p0',
- 'channel-1::scipy-0.12.0-np16py27_p0',
- 'channel-1::scikit-learn-0.13.1-np16py27_p0',
- 'channel-1::mkl-11.0-np16py27_p0',
+ print([Dist(rec).full_name for rec in unlink_precs])
+ unlink_order = (
+ 'channel-1::accelerate-1.1.0-np16py27_p0',
+ 'channel-1::numbapro-0.11.0-np16py27_p0',
)
- assert tuple(final_state_2) == tuple(solver._index[Dist(d)] for d in order)
+ assert tuple(unlink_precs) == tuple(solver._index[Dist(d)] for d in unlink_order)
+
+ print([Dist(rec).full_name for rec in link_precs])
+ link_order = ()
+ assert tuple(link_precs) == tuple(solver._index[Dist(d)] for d in link_order)
with get_solver(specs_to_remove=specs_to_remove, prefix_records=final_state_1,
history_specs=specs) as solver:
- final_state_2 = solver.solve_final_state(prune=True)
+ unlink_precs, link_precs = solver.solve_for_diff(prune=True)
# PrefixDag(final_state_2, specs).open_url()
- print([Dist(rec).full_name for rec in final_state_2])
- order = (
- 'channel-1::openssl-1.0.1c-0',
- 'channel-1::readline-6.2-0',
- 'channel-1::sqlite-3.7.13-0',
- 'channel-1::system-5.8-1',
- 'channel-1::tk-8.5.13-0',
- 'channel-1::zlib-1.2.7-0',
- 'channel-1::python-2.7.3-7',
+ print([Dist(rec).full_name for rec in unlink_precs])
+ unlink_order = (
+ 'channel-1::accelerate-1.1.0-np16py27_p0',
+ 'channel-1::mkl-11.0-np16py27_p0',
+ 'channel-1::scikit-learn-0.13.1-np16py27_p0',
+ 'channel-1::numbapro-0.11.0-np16py27_p0',
+ 'channel-1::scipy-0.12.0-np16py27_p0',
+ 'channel-1::numexpr-2.1-np16py27_p0',
+ 'channel-1::numba-0.8.1-np16py27_0',
+ 'channel-1::numpy-1.6.2-py27_p4',
+ 'channel-1::mkl-service-1.0.0-py27_p0',
+ 'channel-1::meta-0.4.2.dev-py27_0',
+ 'channel-1::llvmpy-0.11.2-py27_0',
+ 'channel-1::bitarray-0.8.1-py27_0',
+ 'channel-1::mkl-rt-11.0-p0',
+ 'channel-1::llvm-3.2-0',
+ 'channel-1::libnvvm-1.0-p0',
+ )
+ assert tuple(unlink_precs) == tuple(solver._index[Dist(d)] for d in unlink_order)
+
+ print([Dist(rec).full_name for rec in link_precs])
+ link_order = (
'channel-1::numpy-1.6.2-py27_4',
)
- assert tuple(final_state_2) == tuple(solver._index[Dist(d)] for d in order)
+ assert tuple(link_precs) == tuple(solver._index[Dist(d)] for d in link_order)
+
def test_force_remove_1():
| Respecting topological sorting for environment removal
Not sure if this is implemented in newer versions of `conda`, but it would be nice if environment removal performed a topological sort and uninstalled packages based on this sorting. Namely only removing packages that are not required for anything else first. For the most part this doesn't matter, but when `pre-unlink` scripts are involved it does.
| Also ran into a similar issue when doing `conda update --all` recently.
I *think* this should be done in 4.4. I'm adding the milestone so I can confirm later. If it's not done yet, it might get bumped past 4.4.0. Hopefully it's already done.
That's great news! Thanks @kalefranz. 😄 | 2017-11-29T17:33:27 | -1.0 |
conda/conda | 6,368 | conda__conda-6368 | [
"6045"
] | aba43f2015c8713a236d858aa91e7b3205d33845 | diff --git a/conda/cli/conda_argparse.py b/conda/cli/conda_argparse.py
--- a/conda/cli/conda_argparse.py
+++ b/conda/cli/conda_argparse.py
@@ -112,7 +112,6 @@ def _get_action_from_name(self, name):
def error(self, message):
import re
from .find_commands import find_executable
-
exc = sys.exc_info()[1]
if exc:
# this is incredibly lame, but argparse stupidly does not expose
@@ -122,23 +121,27 @@ def error(self, message):
else:
argument = None
if argument and argument.dest == "cmd":
- m = re.match(r"invalid choice: u?'(\w+)'", exc.message)
+ m = re.match(r"invalid choice: u?'(\w*?)'", exc.message)
if m:
cmd = m.group(1)
- executable = find_executable('conda-' + cmd)
- if not executable:
- from ..exceptions import CommandNotFoundError
- raise CommandNotFoundError(cmd)
- args = [find_executable('conda-' + cmd)]
- args.extend(sys.argv[2:])
- os.execv(args[0], args)
+ if not cmd:
+ self.print_help()
+ sys.exit(0)
+ else:
+ executable = find_executable('conda-' + cmd)
+ if not executable:
+ from ..exceptions import CommandNotFoundError
+ raise CommandNotFoundError(cmd)
+ args = [find_executable('conda-' + cmd)]
+ args.extend(sys.argv[2:])
+ os.execv(args[0], args)
super(ArgumentParser, self).error(message)
def print_help(self):
super(ArgumentParser, self).print_help()
- if sys.argv[1:] in ([], ['help'], ['-h'], ['--help']):
+ if sys.argv[1:] in ([], [''], ['help'], ['-h'], ['--help']):
from .find_commands import find_commands
other_commands = find_commands()
if other_commands:
diff --git a/conda/exceptions.py b/conda/exceptions.py
--- a/conda/exceptions.py
+++ b/conda/exceptions.py
@@ -200,7 +200,7 @@ def __init__(self, command):
else:
from difflib import get_close_matches
from .cli.find_commands import find_commands
- message = "Error: No command 'conda %(command)s'."
+ message = "No command 'conda %(command)s'."
choices = conda_commands | build_commands | set(find_commands())
close = get_close_matches(command, choices)
if close:
| diff --git a/tests/test_exceptions.py b/tests/test_exceptions.py
--- a/tests/test_exceptions.py
+++ b/tests/test_exceptions.py
@@ -338,7 +338,7 @@ def test_CommandNotFoundError_simple(self):
conda_exception_handler(_raise_helper, exc)
assert not c.stdout
- assert c.stderr.strip() == ("CommandNotFoundError: Error: No command 'conda instate'.\n"
+ assert c.stderr.strip() == ("CommandNotFoundError: No command 'conda instate'.\n"
"Did you mean 'conda install'?")
def test_CommandNotFoundError_conda_build(self):
| conda 4.4 + zsh: conda:shift:1: shift count must be <= $#
With conda 4.4.0rc1 and zsh, calling the new conda shell function with no arguments results in
```
$ conda
conda:shift:1: shift count must be <= $#
usage: conda [-h] [-V] command ...
conda: error: argument command: invalid choice: '' (choose from 'clean', 'config', 'create', 'help', 'info', 'install', 'list', 'package', 'remove', 'uninstall', 'search', 'update', 'upgrade')
```
It's mostly an esthetic point but it would be nice if the shell error message (shift count) was not shown.
| Thanks for the report!
Sent from my iPhone
> On Sep 30, 2017, at 12:06 AM, Antony Lee <notifications@github.com> wrote:
>
> With conda 4.4.0rc1 and zsh, calling the new conda shell function with no arguments results in
>
> $ conda
> conda:shift:1: shift count must be <= $#
> usage: conda [-h] [-V] command ...
> conda: error: argument command: invalid choice: '' (choose from 'clean', 'config', 'create', 'help', 'info', 'install', 'list', 'package', 'remove', 'uninstall', 'search', 'update', 'upgrade')
> It's mostly an esthetic point but it would be nice if the shell error message (shift count) was not shown.
>
> —
> You are receiving this because you are subscribed to this thread.
> Reply to this email directly, view it on GitHub, or mute the thread.
>
Some related issues:
1. `conda nonexistentcommand` exits with status 1 but does not print any error message.
2. The changelog recommends putting conda.sh in a profile folder but this usually won't work if the user's terminals are not login shells (as is the default on Linux): nonlogin shells do not read the profile folder and do not inherit *functions* (even though the *environment* is inherited. I just sourced conda.sh in my .zshrc (rather than my .zprofile). | 2017-11-29T22:58:35 | -1.0 |
conda/conda | 6,370 | conda__conda-6370 | [
"6194"
] | c06adf205b195329c7c13533cac2fd3e20302145 | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -89,6 +89,7 @@ class Context(Configuration):
add_pip_as_python_dependency = PrimitiveParameter(True)
allow_softlinks = PrimitiveParameter(False)
auto_update_conda = PrimitiveParameter(True, aliases=('self_update',))
+ notify_outdated_conda = PrimitiveParameter(True)
clobber = PrimitiveParameter(False)
changeps1 = PrimitiveParameter(True)
concurrent = PrimitiveParameter(True)
@@ -755,6 +756,10 @@ def get_help_dict():
Allows completion of conda's create, install, update, and remove operations, for
non-privileged (non-root or non-administrator) users.
"""),
+ 'notify_outdated_conda': dals("""
+ Notify if a newer version of conda is detected during a create, install, update,
+ or remove operation.
+ """),
'offline': dals("""
Restrict conda to cached download content and file:// based urls.
"""),
diff --git a/conda/core/solve.py b/conda/core/solve.py
--- a/conda/core/solve.py
+++ b/conda/core/solve.py
@@ -5,10 +5,14 @@
from genericpath import exists
from logging import DEBUG, getLogger
from os.path import join
+import sys
+from textwrap import dedent
from .index import get_reduced_index
from .link import PrefixSetup, UnlinkLinkTransaction
from .linked_data import PrefixData
+from .repodata import query_all
+from .. import __version__ as CONDA_VERSION
from .._vendor.boltons.setutils import IndexedSet
from ..base.context import context
from ..common.compat import iteritems, itervalues, odict, string_types, text_type
@@ -22,6 +26,7 @@
from ..models.dag import PrefixDag
from ..models.dist import Dist
from ..models.match_spec import MatchSpec
+from ..models.version import VersionOrder
from ..resolve import Resolve, dashlist
try:
@@ -450,7 +455,30 @@ def solve_for_transaction(self, deps_modifier=NULL, prune=NULL, ignore_pinned=NU
self.specs_to_remove, self.specs_to_add)
# TODO: Only explicitly requested remove and update specs are being included in
# History right now. Do we need to include other categories from the solve?
- return UnlinkLinkTransaction(stp)
+
+ if context.notify_outdated_conda:
+ conda_newer_spec = MatchSpec('conda >%s' % CONDA_VERSION)
+ if not any(conda_newer_spec.match(prec) for prec in link_precs):
+ conda_newer_records = sorted(
+ query_all(self.channels, self.subdirs, conda_newer_spec),
+ key=lambda x: VersionOrder(x.version)
+ )
+ if conda_newer_records:
+ latest_version = conda_newer_records[-1].version
+ sys.stderr.write(dedent("""
+
+ ==> WARNING: A newer version of conda exists. <==
+ current version: %s
+ latest version: %s
+
+ Please update conda by running
+
+ $ conda update -n base conda
+
+
+ """) % (CONDA_VERSION, latest_version))
+
+ return UnlinkLinkTransaction(stp)
def _prepare(self, prepared_specs):
# All of this _prepare() method is hidden away down here. Someday we may want to further
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -1277,6 +1277,7 @@ def side_effect(self, url, **kwargs):
del result.headers[header]
return result
+ SubdirData._cache_.clear()
mock_method.side_effect = side_effect
stdout, stderr = run_command(Commands.INFO, prefix, "flask --json")
assert mock_method.called
| conda only updates itself in the root environment
I was listening to a presentation about conda a couple of days ago where it was mentioned that conda aggressively auto-updates itself. Aggressive auto-updates can be problematic, because they can result in the quick spread of a buggy release that is no longer able to update itself, which has happened to me and co-workers in the past. But in general they are a good thing, because they can help make sure that the installed user base is (a) not using outdated versions where problems were fixed long ago, and (b) are using a similar set of versions so that we don't have to reason about the effects of all possible version combinations.
However, the main benefits of aggressively auto-updating only accrue if all or nearly all installations auto-update, which does not appear to be the case. Right now, it looks to me like auto-updates happen only when people regularly use the root environment. In my own workflows, I haven't installed anything in the root environment in many months; I always work on one of a number of more specialized environments instead. I didn't even realize this until recently, when I ran into a bug fixed several months ago in conda, and which I expected not to apply to me. I eventually fixed it by switching to the root environment and running ``conda update conda``, which was fine, but it was not at all clear that this would be needed. I imagine other users who don't use the root environment may encounter similar issues. I don't think conda is installed in any environment other than root, but maybe the other environments could warn on a ``conda install`` command if conda itself is more than a month or so out of date?
| This problem just reared its head in practice: as of conda 4.3.27, new packages are going into ``https://repo.continuum.io/pkgs/main/``, and so when I tried to install packages in any environment, I was getting out of date packages. After ``conda update conda`` to upgrade 4.3.25, it works fine, but I only knew to do that (given that my conda version wasn't even very old) because of an in-person suggestion from Michael Grant. This seems like something that will cause lots of confusion for any users who habitually use sub-environments.
Related issue: https://github.com/conda/conda/issues/6232 | 2017-11-30T00:23:30 | -1.0 |
conda/conda | 6,436 | conda__conda-6436 | [
"6431"
] | c06adf205b195329c7c13533cac2fd3e20302145 | diff --git a/conda/cli/main_info.py b/conda/cli/main_info.py
--- a/conda/cli/main_info.py
+++ b/conda/cli/main_info.py
@@ -195,33 +195,25 @@ def get_info_dict(system=False):
info_dict['UID'] = os.geteuid()
info_dict['GID'] = os.getegid()
- if system:
- evars = {
- 'CIO_TEST',
- 'CONDA_DEFAULT_ENV',
- 'CONDA_ENVS_PATH',
- 'DYLD_LIBRARY_PATH',
- 'FTP_PROXY',
- 'HTTP_PROXY',
- 'HTTPS_PROXY',
- 'LD_LIBRARY_PATH',
- 'PATH',
- 'PYTHONHOME',
- 'PYTHONPATH',
- 'REQUESTS_CA_BUNDLE',
- 'SSL_CERT_FILE',
- }
-
- evars.update(v for v in os.environ if v.startswith('CONDA_'))
- evars.update(v for v in os.environ if v.startswith('conda_'))
-
- info_dict.update({
- 'sys.version': sys.version,
- 'sys.prefix': sys.prefix,
- 'sys.executable': sys.executable,
- 'site_dirs': get_user_site(),
- 'env_vars': {ev: os.getenv(ev, os.getenv(ev.lower(), '<not set>')) for ev in evars},
- })
+ evars = {
+ 'CIO_TEST',
+ 'REQUESTS_CA_BUNDLE',
+ 'SSL_CERT_FILE',
+ }
+
+ # add all relevant env vars, e.g. startswith('CONDA') or endswith('PATH')
+ evars.update(v for v in os.environ if v.upper().startswith('CONDA'))
+ evars.update(v for v in os.environ if v.upper().startswith('PYTHON'))
+ evars.update(v for v in os.environ if v.upper().endswith('PROXY'))
+ evars.update(v for v in os.environ if v.upper().endswith('PATH'))
+
+ info_dict.update({
+ 'sys.version': sys.version,
+ 'sys.prefix': sys.prefix,
+ 'sys.executable': sys.executable,
+ 'site_dirs': get_user_site(),
+ 'env_vars': {ev: os.getenv(ev, os.getenv(ev.lower(), '<not set>')) for ev in evars},
+ })
return info_dict
| diff --git a/tests/test_info.py b/tests/test_info.py
--- a/tests/test_info.py
+++ b/tests/test_info.py
@@ -31,12 +31,8 @@ def test_info():
conda_info_s_out, conda_info_s_err, rc = run_command(Commands.INFO, '-s')
assert_equals(conda_info_s_err, '')
for name in ['sys.version', 'sys.prefix', 'sys.executable', 'conda location',
- 'conda-build', 'CONDA_DEFAULT_ENV', 'PATH', 'PYTHONPATH']:
+ 'conda-build', 'PATH']:
assert_in(name, conda_info_s_out)
- if context.platform == 'linux':
- assert_in('LD_LIBRARY_PATH', conda_info_s_out)
- if context.platform == 'osx':
- assert_in('DYLD_LIBRARY_PATH', conda_info_s_out)
conda_info_all_out, conda_info_all_err, rc = run_command(Commands.INFO, '--all')
assert_equals(conda_info_all_err, '')
| conda 4.4.0rc2 AssertionError on 'conda create'
"command": "/home/travis/miniconda/bin/conda create -y -p /home/travis/build/conda/conda-build/tests/conda_build_bootstrap_test git"
```
Traceback (most recent call last):
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 265, in _make_single_record
repodata_record = read_repodata_json(extracted_package_dir)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/gateways/disk/read.py\", line 123, in read_repodata_json
with open(join(extracted_package_directory, 'info', 'repodata_record.json')) as fi:
FileNotFoundError: [Errno 2] No such file or directory: '/home/travis/miniconda/pkgs/test_source_files-1-0/info/repodata_record.json'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 276, in _make_single_record
index_json_record = read_index_json(extracted_package_dir)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/gateways/disk/read.py\", line 111, in read_index_json
with open(join(extracted_package_directory, 'info', 'index.json')) as fi:
FileNotFoundError: [Errno 2] No such file or directory: '/home/travis/miniconda/pkgs/test_source_files-1-0/info/index.json'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/exceptions.py\", line 683, in __call__
return func(*args, **kwargs)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/cli/main.py\", line 78, in _main
exit_code = do_call(args, p)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/cli/conda_argparse.py\", line 75, in do_call
exit_code = getattr(module, func_name)(args, parser)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/cli/main_create.py\", line 11, in execute
install(args, parser, 'create')
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/cli/install.py\", line 241, in install
handle_txn(progressive_fetch_extract, unlink_link_transaction, prefix, args, newenv)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/cli/install.py\", line 258, in handle_txn
unlink_link_transaction.display_actions(progressive_fetch_extract)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/link.py\", line 704, in display_actions
legacy_action_groups = self.make_legacy_action_groups(pfe)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/link.py\", line 687, in make_legacy_action_groups
pfe.prepare()
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 504, in prepare
for prec in self.link_precs)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 504, in <genexpr>
for prec in self.link_precs)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 399, in make_actions_for_record
), None)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 396, in <genexpr>
pcrec for pcrec in concat(PackageCache(pkgs_dir).query(pref_or_spec)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 397, in <genexpr>
for pkgs_dir in context.pkgs_dirs)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 116, in query
return (pcrec for pcrec in itervalues(self._package_cache_records) if pcrec == param)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 208, in _package_cache_records
return self.__package_cache_records or self.load() or self.__package_cache_records
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 88, in load
package_cache_record = self._make_single_record(base_name)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/core/package_cache.py\", line 291, in _make_single_record
extract_tarball(package_tarball_full_path, extracted_package_dir)
File \"/home/travis/miniconda/lib/python3.6/site-packages/conda-4.4.0rc2.post15+0dc8573d-py3.6.egg/conda/gateways/disk/create.py\", line 133, in extract_tarball
assert not lexists(destination_directory), destination_directory
AssertionError: /home/travis/miniconda/pkgs/test_source_files-1-0
```
| This was in Travix CI, and a post 4.4.0rc2 version. | 2017-12-11T18:35:55 | -1.0 |
conda/conda | 6,446 | conda__conda-6446 | [
"6419"
] | 3d8374eba3a0335c3ac466c3000898d057187792 | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -51,7 +51,7 @@ def __init__(self, shell, arguments=None):
self.shift_args = 0
self.unset_var_tmpl = 'unset %s'
- self.set_var_tmpl = 'export %s="%s"'
+ self.set_var_tmpl = "export %s='%s'"
self.run_script_tmpl = '. "%s"'
elif shell == 'csh':
@@ -73,7 +73,7 @@ def __init__(self, shell, arguments=None):
self.shift_args = 0
self.unset_var_tmpl = 'del $%s'
- self.set_var_tmpl = '$%s = "%s"'
+ self.set_var_tmpl = "$%s = '%s'"
self.run_script_tmpl = 'source "%s"'
elif shell == 'cmd.exe':
@@ -257,6 +257,8 @@ def build_activate(self, env_name_or_prefix):
}
deactivate_scripts = ()
+ self._update_prompt(set_vars, conda_prompt_modifier)
+
return {
'unset_vars': (),
'set_vars': set_vars,
@@ -283,6 +285,7 @@ def build_deactivate(self):
assert old_conda_shlvl > 0
if old_conda_shlvl == 1:
# TODO: warn conda floor
+ conda_prompt_modifier = ''
unset_vars = (
'CONDA_PREFIX',
'CONDA_DEFAULT_ENV',
@@ -311,6 +314,8 @@ def build_deactivate(self):
}
activate_scripts = self._get_activate_scripts(new_prefix)
+ self._update_prompt(set_vars, conda_prompt_modifier)
+
return {
'unset_vars': unset_vars,
'set_vars': set_vars,
@@ -399,6 +404,19 @@ def _replace_prefix_in_path(self, old_prefix, new_prefix, starting_path_dirs=Non
path_list.insert(idx, join(new_prefix, 'bin'))
return self.path_conversion(path_list)
+ def _update_prompt(self, set_vars, conda_prompt_modifier):
+ if not context.changeps1:
+ return
+
+ if self.shell == 'posix':
+ ps1 = os.environ.get('PS1', '')
+ current_prompt_modifier = os.environ.get('CONDA_PROMPT_MODIFIER')
+ if current_prompt_modifier:
+ ps1 = re.sub(re.escape(current_prompt_modifier), r'', ps1)
+ set_vars.update({
+ 'PS1': conda_prompt_modifier + ps1,
+ })
+
def _default_env(self, prefix):
if prefix == context.root_prefix:
return 'base'
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -152,6 +152,8 @@ def test_build_activate_shlvl_0(self):
activator = Activator('posix')
builder = activator.build_activate(td)
new_path = activator.pathsep_join(activator._add_prefix_to_path(td))
+ conda_prompt_modifier = "(%s) " % td
+ ps1 = conda_prompt_modifier + os.environ.get('PS1', '')
assert builder['unset_vars'] == ()
@@ -161,7 +163,8 @@ def test_build_activate_shlvl_0(self):
'CONDA_PREFIX': td,
'CONDA_SHLVL': 1,
'CONDA_DEFAULT_ENV': td,
- 'CONDA_PROMPT_MODIFIER': "(%s) " % td,
+ 'CONDA_PROMPT_MODIFIER': conda_prompt_modifier,
+ 'PS1': ps1,
}
assert builder['set_vars'] == set_vars
assert builder['activate_scripts'] == (activator.path_conversion(activate_d_1),)
@@ -182,6 +185,8 @@ def test_build_activate_shlvl_1(self):
activator = Activator('posix')
builder = activator.build_activate(td)
new_path = activator.pathsep_join(activator._add_prefix_to_path(td))
+ conda_prompt_modifier = "(%s) " % td
+ ps1 = conda_prompt_modifier + os.environ.get('PS1', '')
assert builder['unset_vars'] == ()
@@ -191,7 +196,8 @@ def test_build_activate_shlvl_1(self):
'CONDA_PREFIX_1': old_prefix,
'CONDA_SHLVL': 2,
'CONDA_DEFAULT_ENV': td,
- 'CONDA_PROMPT_MODIFIER': "(%s) " % td,
+ 'CONDA_PROMPT_MODIFIER': conda_prompt_modifier,
+ 'PS1': ps1,
}
assert builder['set_vars'] == set_vars
assert builder['activate_scripts'] == (activator.path_conversion(activate_d_1),)
@@ -218,6 +224,8 @@ def test_build_activate_shlvl_2(self):
activator = Activator('posix')
builder = activator.build_activate(td)
new_path = activator.pathsep_join(activator._add_prefix_to_path(td))
+ conda_prompt_modifier = "(%s) " % td
+ ps1 = conda_prompt_modifier + os.environ.get('PS1', '')
assert builder['unset_vars'] == ()
@@ -225,7 +233,8 @@ def test_build_activate_shlvl_2(self):
'PATH': new_path,
'CONDA_PREFIX': td,
'CONDA_DEFAULT_ENV': td,
- 'CONDA_PROMPT_MODIFIER': "(%s) " % td,
+ 'CONDA_PROMPT_MODIFIER': conda_prompt_modifier,
+ 'PS1': ps1,
}
assert builder['set_vars'] == set_vars
assert builder['activate_scripts'] == (activator.path_conversion(activate_d_1),)
@@ -289,13 +298,16 @@ def test_build_deactivate_shlvl_2(self):
assert builder['unset_vars'] == ('CONDA_PREFIX_1',)
new_path = activator.pathsep_join(activator.path_conversion(original_path))
+ conda_prompt_modifier = "(%s) " % old_prefix
+ ps1 = conda_prompt_modifier + os.environ.get('PS1', '')
set_vars = {
'PATH': new_path,
'CONDA_SHLVL': 1,
'CONDA_PREFIX': old_prefix,
'CONDA_DEFAULT_ENV': old_prefix,
- 'CONDA_PROMPT_MODIFIER': "(%s) " % old_prefix,
+ 'CONDA_PROMPT_MODIFIER': conda_prompt_modifier,
+ 'PS1': ps1,
}
assert builder['set_vars'] == set_vars
assert builder['activate_scripts'] == (activator.path_conversion(activate_d_1),)
@@ -327,6 +339,7 @@ def test_build_deactivate_shlvl_1(self):
assert builder['set_vars'] == {
'PATH': new_path,
'CONDA_SHLVL': 0,
+ 'PS1': os.environ.get('PS1', ''),
}
assert builder['activate_scripts'] == ()
assert builder['deactivate_scripts'] == (activator.path_conversion(deactivate_d_1),)
@@ -389,12 +402,13 @@ def test_posix_basic(self):
new_path_parts = activator._add_prefix_to_path(self.prefix)
assert activate_data == dals("""
- export CONDA_DEFAULT_ENV="%(native_prefix)s"
- export CONDA_PREFIX="%(native_prefix)s"
- export CONDA_PROMPT_MODIFIER="(%(native_prefix)s) "
- export CONDA_PYTHON_EXE="%(sys_executable)s"
- export CONDA_SHLVL="1"
- export PATH="%(new_path)s"
+ export CONDA_DEFAULT_ENV='%(native_prefix)s'
+ export CONDA_PREFIX='%(native_prefix)s'
+ export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
+ export CONDA_PYTHON_EXE='%(sys_executable)s'
+ export CONDA_SHLVL='1'
+ export PATH='%(new_path)s'
+ export PS1='%(ps1)s'
. "%(activate1)s"
""") % {
'converted_prefix': activator.path_conversion(self.prefix),
@@ -402,6 +416,7 @@ def test_posix_basic(self):
'new_path': activator.pathsep_join(new_path_parts),
'sys_executable': activator.path_conversion(sys.executable),
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.sh')),
+ 'ps1': '(%s) ' % self.prefix + os.environ.get('PS1', '')
}
with env_vars({
@@ -416,8 +431,8 @@ def test_posix_basic(self):
reactivate_data = c.stdout
assert reactivate_data == dals("""
- export CONDA_PROMPT_MODIFIER="(%(native_prefix)s) "
- export CONDA_SHLVL="1"
+ export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
+ export CONDA_SHLVL='1'
. "%(deactivate1)s"
. "%(activate1)s"
""") % {
@@ -438,13 +453,14 @@ def test_posix_basic(self):
unset CONDA_PREFIX
unset CONDA_PROMPT_MODIFIER
unset CONDA_PYTHON_EXE
- export CONDA_SHLVL="0"
- export PATH="%(new_path)s"
+ export CONDA_SHLVL='0'
+ export PATH='%(new_path)s'
+ export PS1='%(ps1)s'
. "%(deactivate1)s"
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.sh')),
-
+ 'ps1': os.environ.get('PS1', ''),
}
def test_cmd_exe_basic(self):
@@ -612,12 +628,12 @@ def test_xonsh_basic(self):
new_path_parts = activator._add_prefix_to_path(self.prefix)
assert activate_data == dals("""
- $CONDA_DEFAULT_ENV = "%(native_prefix)s"
- $CONDA_PREFIX = "%(native_prefix)s"
- $CONDA_PROMPT_MODIFIER = "(%(native_prefix)s) "
- $CONDA_PYTHON_EXE = "%(sys_executable)s"
- $CONDA_SHLVL = "1"
- $PATH = "%(new_path)s"
+ $CONDA_DEFAULT_ENV = '%(native_prefix)s'
+ $CONDA_PREFIX = '%(native_prefix)s'
+ $CONDA_PROMPT_MODIFIER = '(%(native_prefix)s) '
+ $CONDA_PYTHON_EXE = '%(sys_executable)s'
+ $CONDA_SHLVL = '1'
+ $PATH = '%(new_path)s'
source "%(activate1)s"
""") % {
'converted_prefix': activator.path_conversion(self.prefix),
@@ -642,8 +658,8 @@ def test_xonsh_basic(self):
rm_rf(reactivate_result)
assert reactivate_data == dals("""
- $CONDA_PROMPT_MODIFIER = "(%(native_prefix)s) "
- $CONDA_SHLVL = "1"
+ $CONDA_PROMPT_MODIFIER = '(%(native_prefix)s) '
+ $CONDA_SHLVL = '1'
source "%(deactivate1)s"
source "%(activate1)s"
""") % {
@@ -667,8 +683,8 @@ def test_xonsh_basic(self):
del $CONDA_PREFIX
del $CONDA_PROMPT_MODIFIER
del $CONDA_PYTHON_EXE
- $CONDA_SHLVL = "0"
- $PATH = "%(new_path)s"
+ $CONDA_SHLVL = '0'
+ $PATH = '%(new_path)s'
source "%(deactivate1)s"
""") % {
'new_path': new_path,
@@ -931,7 +947,7 @@ def tearDown(self):
def basic_posix(self, shell):
shell.assert_env_var('CONDA_SHLVL', '0')
shell.sendline('conda activate root')
- shell.assert_env_var('PS1', '\$CONDA_PROMPT_MODIFIER.*')
+ shell.assert_env_var('PS1', '(base).*')
shell.assert_env_var('CONDA_SHLVL', '1')
shell.sendline('conda activate "%s"' % self.prefix)
shell.assert_env_var('CONDA_SHLVL', '2')
@@ -959,10 +975,10 @@ def test_dash_basic_integration(self):
shell.sendline('env | sort')
self.basic_posix(shell)
- # @pytest.mark.skipif(not which('zsh'), reason='zsh not installed')
- # def test_zsh_basic_integration(self):
- # with InteractiveShell('zsh') as shell:
- # self.basic_posix(shell)
+ @pytest.mark.skipif(not which('zsh'), reason='zsh not installed')
+ def test_zsh_basic_integration(self):
+ with InteractiveShell('zsh') as shell:
+ self.basic_posix(shell)
@pytest.mark.skipif(not which('cmd.exe'), reason='cmd.exe not installed')
def test_cmd_exe_basic_integration(self):
| conda 4.4 weird prompt addition in zsh
Before conda 4.4 my prompt with zsh would look like this, when I'm in my conda env `stable`:
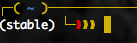
After the update to conda 4.4 (using the blog instructions), I have this:
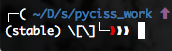
Note the addition of '\[\]' that was added after the environment ID.
How can I get rid of that?
Using zsh 5.4.2 on macOS 10.12.6
| 2017-12-13T16:31:58 | -1.0 |
|
conda/conda | 6,524 | conda__conda-6524 | [
"6523"
] | 09912b87b21b0a66d638e65561ed126677846a91 | diff --git a/conda/core/solve.py b/conda/core/solve.py
--- a/conda/core/solve.py
+++ b/conda/core/solve.py
@@ -144,7 +144,11 @@ def solve_final_state(self, deps_modifier=NULL, prune=NULL, ignore_pinned=NULL,
prefix_data = PrefixData(self.prefix)
solution = tuple(Dist(d) for d in prefix_data.iter_records())
specs_from_history_map = History(self.prefix).get_requested_specs_map()
- if prune or deps_modifier == DepsModifier.UPDATE_ALL:
+ if prune: # or deps_modifier == DepsModifier.UPDATE_ALL # pending conda/constructor#138
+ # Users are struggling with the prune functionality in --update-all, due to
+ # https://github.com/conda/constructor/issues/138. Until that issue is resolved,
+ # and for the foreseeable future, it's best to be more conservative with --update-all.
+
# Start with empty specs map for UPDATE_ALL because we're optimizing the update
# only for specs the user has requested; it's ok to remove dependencies.
specs_map = odict()
| diff --git a/tests/core/test_solve.py b/tests/core/test_solve.py
--- a/tests/core/test_solve.py
+++ b/tests/core/test_solve.py
@@ -1058,28 +1058,29 @@ def test_pinned_1():
)
assert tuple(final_state_5) == tuple(solver._index[Dist(d)] for d in order)
- # now update without pinning
- specs_to_add = MatchSpec("python"),
- history_specs = MatchSpec("python"), MatchSpec("system=5.8=0"), MatchSpec("numba"),
- with get_solver(specs_to_add=specs_to_add, prefix_records=final_state_4,
- history_specs=history_specs) as solver:
- final_state_5 = solver.solve_final_state(deps_modifier=DepsModifier.UPDATE_ALL)
- # PrefixDag(final_state_1, specs).open_url()
- print([Dist(rec).full_name for rec in final_state_5])
- order = (
- 'channel-1::openssl-1.0.1c-0',
- 'channel-1::readline-6.2-0',
- 'channel-1::sqlite-3.7.13-0',
- 'channel-1::system-5.8-1',
- 'channel-1::tk-8.5.13-0',
- 'channel-1::zlib-1.2.7-0',
- 'channel-1::llvm-3.2-0',
- 'channel-1::python-3.3.2-0',
- 'channel-1::llvmpy-0.11.2-py33_0',
- 'channel-1::numpy-1.7.1-py33_0',
- 'channel-1::numba-0.8.1-np17py33_0',
- )
- assert tuple(final_state_5) == tuple(solver._index[Dist(d)] for d in order)
+ # # TODO: re-enable when UPDATE_ALL gets prune behavior again, following completion of https://github.com/conda/constructor/issues/138
+ # # now update without pinning
+ # specs_to_add = MatchSpec("python"),
+ # history_specs = MatchSpec("python"), MatchSpec("system=5.8=0"), MatchSpec("numba"),
+ # with get_solver(specs_to_add=specs_to_add, prefix_records=final_state_4,
+ # history_specs=history_specs) as solver:
+ # final_state_5 = solver.solve_final_state(deps_modifier=DepsModifier.UPDATE_ALL)
+ # # PrefixDag(final_state_1, specs).open_url()
+ # print([Dist(rec).full_name for rec in final_state_5])
+ # order = (
+ # 'channel-1::openssl-1.0.1c-0',
+ # 'channel-1::readline-6.2-0',
+ # 'channel-1::sqlite-3.7.13-0',
+ # 'channel-1::system-5.8-1',
+ # 'channel-1::tk-8.5.13-0',
+ # 'channel-1::zlib-1.2.7-0',
+ # 'channel-1::llvm-3.2-0',
+ # 'channel-1::python-3.3.2-0',
+ # 'channel-1::llvmpy-0.11.2-py33_0',
+ # 'channel-1::numpy-1.7.1-py33_0',
+ # 'channel-1::numba-0.8.1-np17py33_0',
+ # )
+ # assert tuple(final_state_5) == tuple(solver._index[Dist(d)] for d in order)
def test_no_update_deps_1(): # i.e. FREEZE_DEPS
| 'conda update --all' causes "'python' is not recognized as an internal or external command"
repro steps:
1. fresh install of latest version of miniconda
2. `conda update --all` and yes to all questions
3. restart 'Anaconda Prompt'
and you will see
```
'python' is not recognized as an internal or external command,
operable program or batch file.
```
| And i ran 'conda update --all' again and yes to all questions, shortcut 'Anaconda Prompt' is then removed. I'm totally confused. | 2017-12-22T03:34:49 | -1.0 |
conda/conda | 6,550 | conda__conda-6550 | [
"6548"
] | 2060458e0c546a3c70fa041e738cb73b41c4b0d3 | diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -12,17 +12,21 @@
from . import common
from .common import check_non_admin
+from .. import CondaError
from .._vendor.auxlib.ish import dals
from ..base.constants import ROOT_ENV_NAME
from ..base.context import context, locate_prefix_by_name
from ..common.compat import on_win, text_type
from ..core.index import calculate_channel_urls, get_index
+from ..core.linked_data import PrefixData
from ..core.solve import Solver
from ..exceptions import (CondaExitZero, CondaImportError, CondaOSError, CondaSystemExit,
CondaValueError, DirectoryNotFoundError, DryRunExit,
- EnvironmentLocationNotFound, PackagesNotFoundError,
- TooManyArgumentsError, UnsatisfiableError)
+ EnvironmentLocationNotFound,
+ PackageNotInstalledError, PackagesNotFoundError, TooManyArgumentsError,
+ UnsatisfiableError)
from ..misc import clone_env, explicit, touch_nonadmin
+from ..models.match_spec import MatchSpec
from ..plan import (revert_actions)
from ..resolve import ResolvePackageNotFound
@@ -187,6 +191,18 @@ def install(args, parser, command='install'):
raise CondaValueError("too few arguments, "
"must supply command line package specs or --file")
+ # for 'conda update', make sure the requested specs actually exist in the prefix
+ # and that they are name-only specs
+ if isupdate and not args.all:
+ prefix_data = PrefixData(prefix)
+ for spec in specs:
+ spec = MatchSpec(spec)
+ if not spec.is_name_only_spec:
+ raise CondaError("Invalid spec for 'conda update': %s\n"
+ "Use 'conda install' instead." % spec)
+ if not prefix_data.get(spec.name, None):
+ raise PackageNotInstalledError(prefix, spec.name)
+
if newenv and args.clone:
if args.packages:
raise TooManyArgumentsError(0, len(args.packages), list(args.packages),
diff --git a/conda/models/match_spec.py b/conda/models/match_spec.py
--- a/conda/models/match_spec.py
+++ b/conda/models/match_spec.py
@@ -189,6 +189,12 @@ def get(self, field_name, default=None):
v = self.get_raw_value(field_name)
return default if v is None else v
+ @property
+ def is_name_only_spec(self):
+ return (len(self._match_components) == 1
+ and 'name' in self._match_components
+ and self.name != '*')
+
def dist_str(self):
return self.__str__()
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -42,7 +42,7 @@
from conda.core.package_cache import PackageCache
from conda.core.repodata import create_cache_dir
from conda.exceptions import CommandArgumentError, DryRunExit, OperationNotAllowed, \
- PackagesNotFoundError, RemoveError, conda_exception_handler
+ PackagesNotFoundError, RemoveError, conda_exception_handler, PackageNotInstalledError
from conda.gateways.anaconda_client import read_binstar_tokens
from conda.gateways.disk.create import mkdir_p
from conda.gateways.disk.delete import rm_rf
@@ -453,6 +453,15 @@ def assert_json_parsable(content):
finally:
rmtree(prefix, ignore_errors=True)
+ def test_conda_update_package_not_installed(self):
+ with make_temp_env() as prefix:
+ with pytest.raises(PackageNotInstalledError):
+ run_command(Commands.UPDATE, prefix, "sqlite openssl")
+
+ with pytest.raises(CondaError) as conda_error:
+ run_command(Commands.UPDATE, prefix, "conda-forge::*")
+ assert conda_error.value.message.startswith("Invalid spec for 'conda update'")
+
def test_noarch_python_package_with_entry_points(self):
with make_temp_env("-c conda-test flask") as prefix:
py_ver = get_python_version_for_prefix(prefix)
| conda 4.4 has no difference between 'conda install' and 'conda update'
That is, `conda update` installs packages, even if the package isn't already in the environment.
| 2017-12-23T04:11:05 | -1.0 |
|
conda/conda | 6,588 | conda__conda-6588 | [
"6579"
] | fbf3c0dac0667293d8926031119fb0798344d848 | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -266,6 +266,12 @@ def build_activate(self, env_name_or_prefix):
self._update_prompt(set_vars, conda_prompt_modifier)
+ if on_win and self.shell == 'cmd.exe':
+ import ctypes
+ export_vars.update({
+ "PYTHONIOENCODING": ctypes.cdll.kernel32.GetACP(),
+ })
+
return {
'unset_vars': (),
'set_vars': set_vars,
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -33,6 +33,13 @@
log = getLogger(__name__)
+if on_win:
+ import ctypes
+ PYTHONIOENCODING = ctypes.cdll.kernel32.GetACP()
+else:
+ PYTHONIOENCODING = None
+
+
class ActivatorUnitTests(TestCase):
def test_activate_environment_not_found(self):
@@ -274,7 +281,6 @@ def test_activate_same_environment(self):
'CONDA_PROMPT_MODIFIER': "(%s) " % td,
'CONDA_SHLVL': 1,
}
-
assert builder['unset_vars'] == ()
assert builder['set_vars'] == {}
assert builder['export_vars'] == export_vars
@@ -478,6 +484,7 @@ def test_posix_basic(self):
'ps1': os.environ.get('PS1', ''),
}
+ @pytest.mark.skipif(not on_win, reason="cmd.exe only on Windows")
def test_cmd_exe_basic(self):
activator = Activator('cmd.exe')
self.make_dot_d_files(activator.script_extension)
@@ -500,6 +507,7 @@ def test_cmd_exe_basic(self):
@SET "CONDA_PYTHON_EXE=%(sys_executable)s"
@SET "CONDA_SHLVL=1"
@SET "PATH=%(new_path)s"
+ @SET "PYTHONIOENCODING=%(PYTHONIOENCODING)s"
@CALL "%(activate1)s"
""") % {
'converted_prefix': activator.path_conversion(self.prefix),
@@ -507,6 +515,7 @@ def test_cmd_exe_basic(self):
'new_path': activator.pathsep_join(new_path_parts),
'sys_executable': activator.path_conversion(sys.executable),
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.bat')),
+ 'PYTHONIOENCODING': PYTHONIOENCODING,
}
with env_vars({
| Win 10: Update to conda 4.4.4 breaks python path
I updated to conda 4.4.4 recently, and now when I open a new "Anaconda Prompt" the first thing that is displayed is:
`'python' is not recognized as an internal or external command,
operable program or batch file.`
At this point, I can start Python just fine. However, if I activate and then deactivate another environment, I can't use Python from the command prompt anymore. I get the same error message as above. I've tried rolling back the conda update with revisions, but that broke my installation. (The package pycosat does not get uninstalled properly, which seemed to break my conda install.)
Not sure if this matters, but the text in parentheses before the path has changed. Before the update, It would read as the path to my Anaconda installation. In conda 4.4.4, it reads as `(base)`. This may be intended for this update, but I just thought I would share.
| Do you know what version of conda you had before you updated?
I started with a fresh Anacoda distribution. (Py3.6, Win64) | 2017-12-27T19:43:34 | -1.0 |
conda/conda | 6,602 | conda__conda-6602 | [
"6592"
] | fbf3c0dac0667293d8926031119fb0798344d848 | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -187,6 +187,9 @@ class Help(CondaError): # NOQA
self.command = command
def _yield_commands(self, cmds_dict):
+ for script in cmds_dict.get('deactivate_scripts', ()):
+ yield self.run_script_tmpl % script
+
for key in sorted(cmds_dict.get('unset_vars', ())):
yield self.unset_var_tmpl % key
@@ -196,9 +199,6 @@ def _yield_commands(self, cmds_dict):
for key, value in sorted(iteritems(cmds_dict.get('export_vars', {}))):
yield self.export_var_tmpl % (key, value)
- for script in cmds_dict.get('deactivate_scripts', ()):
- yield self.run_script_tmpl % script
-
for script in cmds_dict.get('activate_scripts', ()):
yield self.run_script_tmpl % script
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -446,9 +446,9 @@ def test_posix_basic(self):
reactivate_data = c.stdout
assert reactivate_data == dals("""
+ . "%(deactivate1)s"
export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
export CONDA_SHLVL='1'
- . "%(deactivate1)s"
. "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.sh')),
@@ -464,6 +464,7 @@ def test_posix_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
+ . "%(deactivate1)s"
unset CONDA_DEFAULT_ENV
unset CONDA_PREFIX
unset CONDA_PROMPT_MODIFIER
@@ -471,7 +472,6 @@ def test_posix_basic(self):
PS1='%(ps1)s'
export CONDA_SHLVL='0'
export PATH='%(new_path)s'
- . "%(deactivate1)s"
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.sh')),
@@ -524,9 +524,9 @@ def test_cmd_exe_basic(self):
rm_rf(reactivate_result)
assert reactivate_data == dals("""
+ @CALL "%(deactivate1)s"
@SET "CONDA_PROMPT_MODIFIER=(%(native_prefix)s) "
@SET "CONDA_SHLVL=1"
- @CALL "%(deactivate1)s"
@CALL "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.bat')),
@@ -545,13 +545,13 @@ def test_cmd_exe_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
+ @CALL "%(deactivate1)s"
@SET CONDA_DEFAULT_ENV=
@SET CONDA_PREFIX=
@SET CONDA_PROMPT_MODIFIER=
@SET CONDA_PYTHON_EXE=
@SET "CONDA_SHLVL=0"
@SET "PATH=%(new_path)s"
- @CALL "%(deactivate1)s"
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.bat')),
@@ -598,9 +598,9 @@ def test_csh_basic(self):
reactivate_data = c.stdout
assert reactivate_data == dals("""
+ source "%(deactivate1)s";
setenv CONDA_PROMPT_MODIFIER "(%(native_prefix)s) ";
setenv CONDA_SHLVL "1";
- source "%(deactivate1)s";
source "%(activate1)s";
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.csh')),
@@ -616,6 +616,7 @@ def test_csh_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
+ source "%(deactivate1)s";
unset CONDA_DEFAULT_ENV;
unset CONDA_PREFIX;
unset CONDA_PROMPT_MODIFIER;
@@ -623,7 +624,6 @@ def test_csh_basic(self):
set prompt='%(prompt)s';
setenv CONDA_SHLVL "0";
setenv PATH "%(new_path)s";
- source "%(deactivate1)s";
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.csh')),
@@ -676,9 +676,9 @@ def test_xonsh_basic(self):
rm_rf(reactivate_result)
assert reactivate_data == dals("""
+ source "%(deactivate1)s"
$CONDA_PROMPT_MODIFIER = '(%(native_prefix)s) '
$CONDA_SHLVL = '1'
- source "%(deactivate1)s"
source "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.xsh')),
@@ -697,13 +697,13 @@ def test_xonsh_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
+ source "%(deactivate1)s"
del $CONDA_DEFAULT_ENV
del $CONDA_PREFIX
del $CONDA_PROMPT_MODIFIER
del $CONDA_PYTHON_EXE
$CONDA_SHLVL = '0'
$PATH = '%(new_path)s'
- source "%(deactivate1)s"
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.xsh')),
@@ -748,9 +748,9 @@ def test_fish_basic(self):
reactivate_data = c.stdout
assert reactivate_data == dals("""
+ source "%(deactivate1)s";
set -gx CONDA_PROMPT_MODIFIER "(%(native_prefix)s) ";
set -gx CONDA_SHLVL "1";
- source "%(deactivate1)s";
source "%(activate1)s";
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.fish')),
@@ -766,13 +766,13 @@ def test_fish_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
+ source "%(deactivate1)s";
set -e CONDA_DEFAULT_ENV;
set -e CONDA_PREFIX;
set -e CONDA_PROMPT_MODIFIER;
set -e CONDA_PYTHON_EXE;
set -gx CONDA_SHLVL "0";
set -gx PATH "%(new_path)s";
- source "%(deactivate1)s";
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.fish')),
@@ -817,9 +817,9 @@ def test_powershell_basic(self):
reactivate_data = c.stdout
assert reactivate_data == dals("""
+ . "%(deactivate1)s"
$env:CONDA_PROMPT_MODIFIER = "(%(prefix)s) "
$env:CONDA_SHLVL = "1"
- . "%(deactivate1)s"
. "%(activate1)s"
""") % {
'activate1': join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.ps1'),
@@ -835,13 +835,13 @@ def test_powershell_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
+ . "%(deactivate1)s"
Remove-Variable CONDA_DEFAULT_ENV
Remove-Variable CONDA_PREFIX
Remove-Variable CONDA_PROMPT_MODIFIER
Remove-Variable CONDA_PYTHON_EXE
$env:CONDA_SHLVL = "0"
$env:PATH = "%(new_path)s"
- . "%(deactivate1)s"
""") % {
'new_path': new_path,
'deactivate1': join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.ps1'),
| deactivate sets `CONDA_PREFIX` to the wrong value.
Deactivating an env. into which the new compilers have been installed leads to:
```
ERROR: This cross-compiler package contains no program /opt/conda/bin/x86_64-conda_cos6-linux-gnu-cc
```
This comes from [here](https://github.com/AnacondaRecipes/aggregate/blob/master/ctng-compilers-activation-feedstock/recipe/deactivate-gcc.sh#L58)
It seems that `CONDA_PREFIX` has been set to the prefix of the new environment instead of that of the old environment.
It doesn't seem to matter whether the legacy activate scripts or the conda shell function are used here, both exhibit the same problem.
| > It seems that `CONDA_PREFIX` has been set to the prefix of the new environment instead of that of the old environment.
It's my understanding that `CONDA_PREFIX` has always been the path to the current active prefix. At least back to the #1727 days or so. Then again, I could be completely mis-remembering, and I've added pieces of code over time that made that assumption, because I can't find any reference to `CONDA_PREFIX` in the 4.3.x code concerning activate.
Oh, right. I was only looking in the code, not the shell wrappers.
https://github.com/conda/conda/blob/4.3.31/shell/activate#L88-L92 | 2017-12-28T17:57:46 | -1.0 |
conda/conda | 6,615 | conda__conda-6615 | [
"6612"
] | 0f3a83b1fd068c75e530ddece96e98cc5b5e6d8e | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -368,8 +368,20 @@ def build_reactivate(self):
def _get_starting_path_list(self):
path = os.environ['PATH']
if on_win:
- # on Windows, the python interpreter prepends sys.prefix\Library\bin on startup WTF
- return path.split(os.pathsep)[1:]
+ # On Windows, the Anaconda Python interpreter prepends sys.prefix\Library\bin on
+ # startup. It's a hack that allows users to avoid using the correct activation
+ # procedure; a hack that needs to go away because it doesn't add all the paths.
+ # See: https://github.com/AnacondaRecipes/python-feedstock/blob/master/recipe/0005-Win32-Ensure-Library-bin-is-in-os.environ-PATH.patch # NOQA
+ # But, we now detect if that has happened because:
+ # 1. In future we would like to remove this hack and require real activation.
+ # 2. We should not assume that the Anaconda Python interpreter is being used.
+ path_split = path.split(os.pathsep)
+ library_bin = r"%s\Library\bin" % (sys.prefix)
+ # ^^^ deliberately the same as: https://github.com/AnacondaRecipes/python-feedstock/blob/8e8aee4e2f4141ecfab082776a00b374c62bb6d6/recipe/0005-Win32-Ensure-Library-bin-is-in-os.environ-PATH.patch#L20 # NOQA
+ if normpath(path_split[0]) == normpath(library_bin):
+ return path_split[1:]
+ else:
+ return path_split
else:
return path.split(os.pathsep)
| diff --git a/tests/cli/test_activate.py b/tests/cli/test_activate.py
--- a/tests/cli/test_activate.py
+++ b/tests/cli/test_activate.py
@@ -246,7 +246,6 @@ def test_activate_bad_env_keeps_existing_good_env(shell):
@pytest.mark.installed
-@pytest.mark.xfail(on_win and datetime.now() < datetime(2018, 11, 21), reason="need to get 4.4.x merge-up finished", strict=True)
def test_activate_deactivate(shell):
if shell == 'bash.exe':
pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
@@ -284,7 +283,6 @@ def test_activate_deactivate(shell):
@pytest.mark.installed
-@pytest.mark.xfail(on_win and datetime.now() < datetime(2018, 11, 21), reason="need to get 4.4.x merge-up finished", strict=True)
def test_activate_root_simple(shell):
if shell == 'bash.exe':
pytest.skip("usage of cygpath in win_path_to_unix messes this test up")
| PATH manipulation problems with conda.bat activate on Windows
With conda 4.4.5:
```
C:\Users\builder>echo %PATH%
C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\;C:\Program Files (x86)\Windows Kits\8.1\Windows Performance Toolkit\;C:\Program Files (x86)\PuTTY\;C:\Program Files\MiKTeX 2.9\miktex\bin\x64\;C:\Program Files (x86)\Windows Kits\10\Windows Performance Toolkit\;C:\Program Files\dotnet\;C:\Users\builder\AppData\Local\Microsoft\WindowsApps;
c:/Users/builder/m64/Library/bin/conda.bat activate
'chcp' is not recognized as an internal or external command,
operable program or batch file.
(base) C:\Users\builder>(base) C:\Users\builder>echo %PATH%
c:\Users\builder\m64;c:\Users\builder\m64\Library\mingw-w64\bin;c:\Users\builder\m64\Library\usr\bin;c:\Users\builder\m64\Library\bin;c:\Users\builder\m64\Scripts;c:\Users\builder\m64\bin;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\;C:\Program Files (x86)\Windows Kits\8.1\Windows Performance Toolkit\;C:\Program Files (x86)\PuTTY\;C:\Program Files\MiKTeX 2.9\miktex\bin\x64\;C:\Program Files (x86)\Windows Kits\10\Windows Performance Toolkit\;C:\Program Files\dotnet\;C:\Users\builder\AppData\Local\Microsoft\WindowsApps;
```
Notice that `C:\WINDOWS\system32` got dropped which is why `chcp` isn't found (this folder contains lots of important programs too).
| Is this with 4.4.5 in canary?
Sent from my iPhone
> On Dec 29, 2017, at 8:19 PM, Ray Donnelly <notifications@github.com> wrote:
>
> C:\Users\builder>echo %PATH%
> C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\;C:\Program Files (x86)\Windows Kits\8.1\Windows Performance Toolkit\;C:\Program Files (x86)\PuTTY\;C:\Program Files\MiKTeX 2.9\miktex\bin\x64\;C:\Program Files (x86)\Windows Kits\10\Windows Performance Toolkit\;C:\Program Files\dotnet\;C:\Users\builder\AppData\Local\Microsoft\WindowsApps;
>
> c:/Users/builder/m64/Library/bin/conda.bat activate
> 'chcp' is not recognized as an internal or external command,
> operable program or batch file.
>
> c:\Users\builder\m64;c:\Users\builder\m64\Library\mingw-w64\bin;c:\Users\builder\m64\Library\usr\bin;c:\Users\builder\m64\Library\bin;c:\Users\builder\m64\Scripts;c:\Users\builder\m64\bin;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\;C:\Program Files (x86)\Windows Kits\8.1\Windows Performance Toolkit\;C:\Program Files (x86)\PuTTY\;C:\Program Files\MiKTeX 2.9\miktex\bin\x64\;C:\Program Files (x86)\Windows Kits\10\Windows Performance Toolkit\;C:\Program Files\dotnet\;C:\Users\builder\AppData\Local\Microsoft\WindowsApps;
> Notice that C:\WINDOWS\system32 got dropped which is why chcp isn't found (this folder contains lots of important programs too).
>
> —
> You are receiving this because you are subscribed to this thread.
> Reply to this email directly, view it on GitHub, or mute the thread.
>
Yes and 4.4.4 too
OK, I know what's going on and it's kind of my fault (but I want to make a PR to conda to avoid this anyway).
While investigating https://github.com/ContinuumIO/anaconda/issues/1158 to check whether [this horrible hack](https://github.com/AnacondaRecipes/python-feedstock/blob/master/recipe/0005-Win32-Ensure-Library-bin-is-in-os.environ-PATH.patch) was to blame I removed it.
That then tripped up [this code](https://github.com/conda/conda/blob/master/conda/activate.py#L368-L374):
```
def _get_starting_path_list(self):
path = os.environ['PATH']
if on_win:
# on Windows, the python interpreter prepends sys.prefix\Library\bin on startup WTF
return path.split(os.pathsep)[1:]
else:
return path.split(os.pathsep)
```
I will make a PR that will only elide the first element when the interpreter has actually done this (by testing it to see if the value of the first entry is indeed `sys.prefix\Library\bin`) and will add a link back to this unfortunate patch in our python recipe for reference (hopefully one day that link will point to nothing).
This will remove a dependency between future conda releasses and this hack in our Python interpreter and will also allow conda to work correctly on other builds of Python on Windows.
| 2017-12-30T23:17:42 | -1.0 |
conda/conda | 6,647 | conda__conda-6647 | [
"6643"
] | 114e4b3cfb0fae3d566fd08a0c0c1d7e24c19891 | diff --git a/conda/models/version.py b/conda/models/version.py
--- a/conda/models/version.py
+++ b/conda/models/version.py
@@ -463,12 +463,17 @@ def __new__(cls, spec):
self.op = VersionOrder.startswith
self.cmp = VersionOrder(spec.rstrip('*').rstrip('.'))
self.match = self.veval_match_
+ elif '@' not in spec:
+ self.op = opdict["=="]
+ self.cmp = VersionOrder(spec)
+ self.match = self.veval_match_
else:
self.match = self.exact_match_
return self
def is_exact(self):
- return self.match == self.exact_match_
+ return (self.match == self.exact_match_
+ or self.match == self.veval_match_ and self.op == op.__eq__)
def __eq__(self, other):
try:
| diff --git a/tests/models/test_match_spec.py b/tests/models/test_match_spec.py
--- a/tests/models/test_match_spec.py
+++ b/tests/models/test_match_spec.py
@@ -50,6 +50,7 @@ def test_match_1(self):
('numpy >1.8,<2|==1.7', False),('numpy >1.8,<2|>=1.7.1', True),
('numpy >=1.8|1.7*', True), ('numpy ==1.7', False),
('numpy >=1.5,>1.6', True), ('numpy ==1.7.1', True),
+ ('numpy ==1.7.1.0', True), ('numpy==1.7.1.0.0', True),
('numpy >=1,*.7.*', True), ('numpy *.7.*,>=1', True),
('numpy >=1,*.8.*', False), ('numpy >=2,*.7.*', False),
('numpy 1.6*|1.7*', True), ('numpy 1.6*|1.8*', False),
@@ -560,6 +561,14 @@ def test_parse_equal_equal(self):
"name": "numpy",
"version": "1.7",
}
+ assert _parse_spec_str("numpy=1.7") == {
+ "name": "numpy",
+ "version": "1.7*",
+ }
+ assert _parse_spec_str("numpy =1.7") == {
+ "name": "numpy",
+ "version": "1.7*",
+ }
def test_parse_hard(self):
assert _parse_spec_str("numpy>1.8,<2|==1.7") == {
| version '==' interpreted differently in 4.3 and 4.4
| 2018-01-04T18:03:41 | -1.0 |
|
conda/conda | 6,669 | conda__conda-6669 | [
"6667",
"6667"
] | 31a52a8a8771aafb0582822dd2e00b3274e1ffba | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -57,10 +57,10 @@ def __init__(self, shell, arguments=None):
self.shift_args = 0
self.command_join = '\n'
- self.unset_var_tmpl = 'unset %s'
- self.export_var_tmpl = "export %s='%s'"
+ self.unset_var_tmpl = '\\unset %s'
+ self.export_var_tmpl = "\\export %s='%s'"
self.set_var_tmpl = "%s='%s'"
- self.run_script_tmpl = '. "%s"'
+ self.run_script_tmpl = '\\. "%s"'
elif shell == 'csh':
self.pathsep_join = ':'.join
| diff --git a/tests/cli/test_activate.py b/tests/cli/test_activate.py
--- a/tests/cli/test_activate.py
+++ b/tests/cli/test_activate.py
@@ -137,12 +137,18 @@ def _format_vars(shell):
{set} CONDARC=
{set} CONDA_PATH_BACKUP=
{set} PATH="{new_path}"
+{set} _CONDA_ROOT="{shellpath}"
"""
if 'bash' in shell:
_command_setup += "set -u\n"
- command_setup = _command_setup.format(here=dirname(__file__), PYTHONPATH=shelldict['path_to'](PYTHONPATH),
- set=shelldict["set_var"], new_path=base_path)
+ command_setup = _command_setup.format(
+ here=dirname(__file__),
+ PYTHONPATH=shelldict['path_to'](PYTHONPATH),
+ set=shelldict["set_var"],
+ new_path=base_path,
+ shellpath=join(dirname(CONDA_PACKAGE_ROOT), 'conda', 'shell')
+ )
if shelldict["shell_suffix"] == '.bat':
command_setup = "@echo off\n" + command_setup
@@ -342,51 +348,6 @@ def test_wrong_args(shell):
assert_equals(stdout, shell_vars['base_path'], stderr)
-@pytest.mark.installed
-def test_activate_help(shell):
- shell_vars = _format_vars(shell)
- with TemporaryDirectory(prefix=ENVS_PREFIX, dir=dirname(__file__)) as envs:
- if shell not in ['powershell.exe', 'cmd.exe']:
- commands = (shell_vars['command_setup'] + """
- "{syspath}{binpath}activate" Zanzibar
- """).format(envs=envs, **shell_vars)
- stdout, stderr = run_in(commands, shell)
- assert_equals(stdout, '')
- assert_in("activate must be sourced", stderr)
- # assert_in("Usage: source activate ENV", stderr)
-
- commands = (shell_vars['command_setup'] + """
- {source} "{syspath}{binpath}activate" --help
- """).format(envs=envs, **shell_vars)
-
- stdout, stderr = run_in(commands, shell)
- assert_equals(stdout, '')
-
- if shell in ["cmd.exe", "powershell"]:
- # assert_in("Usage: activate ENV", stderr)
- pass
- else:
- # assert_in("Usage: source activate ENV", stderr)
-
- commands = (shell_vars['command_setup'] + """
- {syspath}{binpath}deactivate
- """).format(envs=envs, **shell_vars)
- stdout, stderr = run_in(commands, shell)
- assert_equals(stdout, '')
- assert_in("deactivate must be sourced", stderr)
- # assert_in("Usage: source deactivate", stderr)
-
- commands = (shell_vars['command_setup'] + """
- {source} {syspath}{binpath}deactivate --help
- """).format(envs=envs, **shell_vars)
- stdout, stderr = run_in(commands, shell)
- assert_equals(stdout, '')
- # if shell in ["cmd.exe", "powershell"]:
- # assert_in("Usage: deactivate", stderr)
- # else:
- # assert_in("Usage: source deactivate", stderr)
-
-
@pytest.mark.installed
def test_PS1_changeps1(shell): # , bash_profile
shell_vars = _format_vars(shell)
diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -459,13 +459,13 @@ def test_posix_basic(self):
new_path_parts = activator._add_prefix_to_path(self.prefix)
assert activate_data == dals("""
PS1='%(ps1)s'
- export CONDA_DEFAULT_ENV='%(native_prefix)s'
- export CONDA_PREFIX='%(native_prefix)s'
- export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
- export CONDA_PYTHON_EXE='%(sys_executable)s'
- export CONDA_SHLVL='1'
- export PATH='%(new_path)s'
- . "%(activate1)s"
+ \\export CONDA_DEFAULT_ENV='%(native_prefix)s'
+ \\export CONDA_PREFIX='%(native_prefix)s'
+ \\export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
+ \\export CONDA_PYTHON_EXE='%(sys_executable)s'
+ \\export CONDA_SHLVL='1'
+ \\export PATH='%(new_path)s'
+ \\. "%(activate1)s"
""") % {
'converted_prefix': activator.path_conversion(self.prefix),
'native_prefix': self.prefix,
@@ -489,12 +489,12 @@ def test_posix_basic(self):
new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
- . "%(deactivate1)s"
+ \\. "%(deactivate1)s"
PS1='%(ps1)s'
- export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
- export CONDA_SHLVL='1'
- export PATH='%(new_path)s'
- . "%(activate1)s"
+ \\export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
+ \\export CONDA_SHLVL='1'
+ \\export PATH='%(new_path)s'
+ \\. "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.sh')),
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.sh')),
@@ -511,14 +511,14 @@ def test_posix_basic(self):
new_path = activator.pathsep_join(activator._remove_prefix_from_path(self.prefix))
assert deactivate_data == dals("""
- . "%(deactivate1)s"
- unset CONDA_DEFAULT_ENV
- unset CONDA_PREFIX
- unset CONDA_PROMPT_MODIFIER
- unset CONDA_PYTHON_EXE
+ \\. "%(deactivate1)s"
+ \\unset CONDA_DEFAULT_ENV
+ \\unset CONDA_PREFIX
+ \\unset CONDA_PROMPT_MODIFIER
+ \\unset CONDA_PYTHON_EXE
PS1='%(ps1)s'
- export CONDA_SHLVL='0'
- export PATH='%(new_path)s'
+ \\export CONDA_SHLVL='0'
+ \\export PATH='%(new_path)s'
""") % {
'new_path': new_path,
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.sh')),
| Source activation for anaconda python environments no longer working (Mac)
The source activate command in conda is suddenly no longer working for me. When I type `source activate py3` in my Mac terminal, I get this error `-bash: _conda_activate: command not found`.
I recently created a new conda environment for TabPy (Tableau python server); I'm not sure if that changed anything. I have checked that the conda environments I'm trying to activate exist by using `conda info --envs`, here is that output:
```
# conda environments:
#
base * /Users/applemacbook/anaconda
Tableau-Python-Server /Users/applemacbook/anaconda/envs/Tableau-Python
Server
py3 /Users/applemacbook/anaconda/envs/py3
```
I already have the following in my bash profile from my initial installation of anaconda awhile ago `export PATH="/Users/applemacbook/anaconda/bin:$PATH"`.
Here is some additional information: The output of `which conda` is `/Users/applemacbook/anaconda/bin/conda`. The output of `type source` is `source is a shell builtin`. The output of `which activate` is `/Users/applemacbook/anaconda/bin/activate`. My conda version is conda 4.4.6
Source activation for anaconda python environments no longer working (Mac)
The source activate command in conda is suddenly no longer working for me. When I type `source activate py3` in my Mac terminal, I get this error `-bash: _conda_activate: command not found`.
I recently created a new conda environment for TabPy (Tableau python server); I'm not sure if that changed anything. I have checked that the conda environments I'm trying to activate exist by using `conda info --envs`, here is that output:
```
# conda environments:
#
base * /Users/applemacbook/anaconda
Tableau-Python-Server /Users/applemacbook/anaconda/envs/Tableau-Python
Server
py3 /Users/applemacbook/anaconda/envs/py3
```
I already have the following in my bash profile from my initial installation of anaconda awhile ago `export PATH="/Users/applemacbook/anaconda/bin:$PATH"`.
Here is some additional information: The output of `which conda` is `/Users/applemacbook/anaconda/bin/conda`. The output of `type source` is `source is a shell builtin`. The output of `which activate` is `/Users/applemacbook/anaconda/bin/activate`. My conda version is conda 4.4.6
| Guessing this is a duplicate of https://github.com/conda/conda/issues/6639?
Maybe not. Could you open a new terminal, and then give me the output of
env | sort
set -x
source activate py3
You'll want to close the terminal down after you do the above. Or
set +x
to get things back to normal.
Also, can you give me the output of
cat /Users/applemacbook/anaconda/bin/activate
Edited by moderator. Output given fixed-width below.
Alright, I think I've got all the outputs you asked for below:
```
$ env | sort
Apple_PubSub_Socket_Render=/private/tmp/com.apple.launchd.XLbN3qRfIV/Render
CDPATH=.:/Users/applemacbook:/Users/applemacbook/Documents/Galvanize:/Users/applemacbook/Documents/Galvanize/immersive:/Users/applemacbook/Documents:
COLORFGBG=7;0
COLORTERM=truecolor
EDITOR=emacs
HADOOP_HOME=/usr/local/hadoop
HOME=/Users/applemacbook
ITERM_PROFILE=Default
ITERM_SESSION_ID=w0t3p0:1061674E-3BF3-4203-A297-44BAC655F9A4
JAVA_HOME=/Library/Java/JavaVirtualMachines/jdk1.8.0_144.jdk/Contents/Home
LANG=en_US.UTF-8
LOGNAME=applemacbook
PATH=/usr/local/spark/bin:/Users/applemacbook/anaconda/bin:/Library/Frameworks/Python.framework/Versions/2.7/bin:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin
PS1=$
PWD=/Users/applemacbook
PYTHONPATH=/usr/local/spark/python:
SHELL=/bin/bash
SHLVL=1
SPARK_HOME=/usr/local/spark
SPARK_LOCAL_IP=localhost
SSH_AUTH_SOCK=/private/tmp/com.apple.launchd.FwpsT72RUz/Listeners
TERM=xterm-256color
TERM_PROGRAM=iTerm.app
TERM_PROGRAM_VERSION=3.1.5
TERM_SESSION_ID=w0t3p0:1061674E-3BF3-4203-A297-44BAC655F9A4
TMPDIR=/var/folders/yp/3f96txfd1rj8m8k28xxbvpkh0000gn/T/
USER=applemacbook
VISUAL=emacs
XPC_FLAGS=0x0
XPC_SERVICE_NAME=0
_=/usr/bin/env
__CF_USER_TEXT_ENCODING=0x0:0:0
$ set -x
+ set -x
$ source activate py3
+ source activate py3
++ _CONDA_ROOT=/Users/applemacbook/anaconda
++ _conda_script_is_sourced
++ sourced=0
++ '[' -n '' ']'
++ '[' -n '' ']'
++ '[' -n x ']'
++ '[' /Users/applemacbook/anaconda/bin/activate = -bash ']'
++ return 0
++ _conda_set_vars
++ '[' -n x ']'
++ _CONDA_SHELL_FLAVOR=bash
++ local script_dir
++ case "$_CONDA_SHELL_FLAVOR" in
+++ dirname /Users/applemacbook/anaconda/bin/activate
++ script_dir=/Users/applemacbook/anaconda/bin
++ '[' -z x ']'
++ cd .. /Users/applemacbook/anaconda/etc/profile.d/conda.sh
++ _conda_activate py3
-bash: _conda_activate: command not found
$ cat /Users/applemacbook/anaconda/bin/activate
+ cat /Users/applemacbook/anaconda/bin/activate
#!/bin/sh
_CONDA_ROOT="/Users/applemacbook/anaconda"
#!/bin/sh
_conda_set_vars() {
# set _CONDA_SHELL_FLAVOR
if [ -n "${BASH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=bash
elif [ -n "${ZSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=zsh
elif [ -n "${KSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=ksh
elif [ -n "${POSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=posh
else
# https://unix.stackexchange.com/a/120138/92065
local _q="$(ps -p$$ -o cmd="",comm="",fname="" 2>/dev/null | sed
's/^-//' | grep -oE '\w+' | head -n1)"
if [ "$_q" = dash ]; then
_CONDA_SHELL_FLAVOR=dash
else
(>&2 echo "Unrecognized shell.")
return 1
fi
fi
#
https://unix.stackexchange.com/questions/4650/determining-path-to-sourced-shell-script/
local script_dir
case "$_CONDA_SHELL_FLAVOR" in
bash) script_dir="$(dirname "${BASH_SOURCE[0]}")";;
zsh) script_dir="$(dirname "${(%):-%x}")";; #
http://stackoverflow.com/a/28336473/2127762
dash) x=$(lsof -p $$ -Fn0 | tail -1); script_dir="$(dirname
"${x#n}")";;
*) script_dir="$(cd "$(dirname "$_")" && echo "$PWD")";;
esac
if [ -z "${_CONDA_ROOT+x}" ]; then
_CONDA_ROOT="$(dirname "$script_dir")"
fi
}
_conda_script_is_sourced() {
# http://stackoverflow.com/a/28776166/2127762
sourced=0
if [ -n "${ZSH_EVAL_CONTEXT:+x}" ]; then
case $ZSH_EVAL_CONTEXT in *:file) sourced=1;; esac
elif [ -n "${KSH_VERSION:+x}" ]; then
[ "$(cd $(dirname -- $0) && pwd -P)/$(basename -- $0)" != "$(cd
$(dirname -- ${.sh.file}) && pwd -P)/$(basename -- ${.sh.file})" ] &&
sourced=1
elif [ -n "${BASH_VERSION:+x}" ]; then
[ "${BASH_SOURCE[0]}" = "$0" ] && sourced=1
else # All other shells: examine $0 for known shell binary filenames
# Detects `sh` and `dash`; add additional shell filenames as needed.
case ${0##*/} in sh|dash) sourced=0;; *) sourced=1;; esac
fi
return $sourced
}
if ! _conda_script_is_sourced; then
(
>&2 echo "Error: activate must be sourced. Run 'source activate
envname'"
>&2 echo "instead of 'activate envname'."
)
exit 1
fi
_conda_set_vars
. "$_CONDA_ROOT/etc/profile.d/conda.sh" || return $?
_conda_activate "$@"
```
Comparing from above
```
++ script_dir=/Users/applemacbook/anaconda/bin
++ '[' -z x ']'
++ cd .. /Users/applemacbook/anaconda/etc/profile.d/conda.sh
++ _conda_activate py3
-bash: _conda_activate: command not found
```
to what I get myself
```
++ script_dir=/Users/kfranz/miniconda/bin
++ '[' -z x ']'
++ . /Users/kfranz/miniconda/etc/profile.d/conda.sh
+++ _CONDA_EXE=/Users/kfranz/miniconda/bin/conda
+++ _CONDA_ROOT=/Users/kfranz/miniconda
```
Guessing this is a duplicate of https://github.com/conda/conda/issues/6639?
Maybe not. Could you open a new terminal, and then give me the output of
env | sort
set -x
source activate py3
You'll want to close the terminal down after you do the above. Or
set +x
to get things back to normal.
Also, can you give me the output of
cat /Users/applemacbook/anaconda/bin/activate
Edited by moderator. Output given fixed-width below.
Alright, I think I've got all the outputs you asked for below:
```
$ env | sort
Apple_PubSub_Socket_Render=/private/tmp/com.apple.launchd.XLbN3qRfIV/Render
CDPATH=.:/Users/applemacbook:/Users/applemacbook/Documents/Galvanize:/Users/applemacbook/Documents/Galvanize/immersive:/Users/applemacbook/Documents:
COLORFGBG=7;0
COLORTERM=truecolor
EDITOR=emacs
HADOOP_HOME=/usr/local/hadoop
HOME=/Users/applemacbook
ITERM_PROFILE=Default
ITERM_SESSION_ID=w0t3p0:1061674E-3BF3-4203-A297-44BAC655F9A4
JAVA_HOME=/Library/Java/JavaVirtualMachines/jdk1.8.0_144.jdk/Contents/Home
LANG=en_US.UTF-8
LOGNAME=applemacbook
PATH=/usr/local/spark/bin:/Users/applemacbook/anaconda/bin:/Library/Frameworks/Python.framework/Versions/2.7/bin:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin
PS1=$
PWD=/Users/applemacbook
PYTHONPATH=/usr/local/spark/python:
SHELL=/bin/bash
SHLVL=1
SPARK_HOME=/usr/local/spark
SPARK_LOCAL_IP=localhost
SSH_AUTH_SOCK=/private/tmp/com.apple.launchd.FwpsT72RUz/Listeners
TERM=xterm-256color
TERM_PROGRAM=iTerm.app
TERM_PROGRAM_VERSION=3.1.5
TERM_SESSION_ID=w0t3p0:1061674E-3BF3-4203-A297-44BAC655F9A4
TMPDIR=/var/folders/yp/3f96txfd1rj8m8k28xxbvpkh0000gn/T/
USER=applemacbook
VISUAL=emacs
XPC_FLAGS=0x0
XPC_SERVICE_NAME=0
_=/usr/bin/env
__CF_USER_TEXT_ENCODING=0x0:0:0
$ set -x
+ set -x
$ source activate py3
+ source activate py3
++ _CONDA_ROOT=/Users/applemacbook/anaconda
++ _conda_script_is_sourced
++ sourced=0
++ '[' -n '' ']'
++ '[' -n '' ']'
++ '[' -n x ']'
++ '[' /Users/applemacbook/anaconda/bin/activate = -bash ']'
++ return 0
++ _conda_set_vars
++ '[' -n x ']'
++ _CONDA_SHELL_FLAVOR=bash
++ local script_dir
++ case "$_CONDA_SHELL_FLAVOR" in
+++ dirname /Users/applemacbook/anaconda/bin/activate
++ script_dir=/Users/applemacbook/anaconda/bin
++ '[' -z x ']'
++ cd .. /Users/applemacbook/anaconda/etc/profile.d/conda.sh
++ _conda_activate py3
-bash: _conda_activate: command not found
$ cat /Users/applemacbook/anaconda/bin/activate
+ cat /Users/applemacbook/anaconda/bin/activate
#!/bin/sh
_CONDA_ROOT="/Users/applemacbook/anaconda"
#!/bin/sh
_conda_set_vars() {
# set _CONDA_SHELL_FLAVOR
if [ -n "${BASH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=bash
elif [ -n "${ZSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=zsh
elif [ -n "${KSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=ksh
elif [ -n "${POSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=posh
else
# https://unix.stackexchange.com/a/120138/92065
local _q="$(ps -p$$ -o cmd="",comm="",fname="" 2>/dev/null | sed
's/^-//' | grep -oE '\w+' | head -n1)"
if [ "$_q" = dash ]; then
_CONDA_SHELL_FLAVOR=dash
else
(>&2 echo "Unrecognized shell.")
return 1
fi
fi
#
https://unix.stackexchange.com/questions/4650/determining-path-to-sourced-shell-script/
local script_dir
case "$_CONDA_SHELL_FLAVOR" in
bash) script_dir="$(dirname "${BASH_SOURCE[0]}")";;
zsh) script_dir="$(dirname "${(%):-%x}")";; #
http://stackoverflow.com/a/28336473/2127762
dash) x=$(lsof -p $$ -Fn0 | tail -1); script_dir="$(dirname
"${x#n}")";;
*) script_dir="$(cd "$(dirname "$_")" && echo "$PWD")";;
esac
if [ -z "${_CONDA_ROOT+x}" ]; then
_CONDA_ROOT="$(dirname "$script_dir")"
fi
}
_conda_script_is_sourced() {
# http://stackoverflow.com/a/28776166/2127762
sourced=0
if [ -n "${ZSH_EVAL_CONTEXT:+x}" ]; then
case $ZSH_EVAL_CONTEXT in *:file) sourced=1;; esac
elif [ -n "${KSH_VERSION:+x}" ]; then
[ "$(cd $(dirname -- $0) && pwd -P)/$(basename -- $0)" != "$(cd
$(dirname -- ${.sh.file}) && pwd -P)/$(basename -- ${.sh.file})" ] &&
sourced=1
elif [ -n "${BASH_VERSION:+x}" ]; then
[ "${BASH_SOURCE[0]}" = "$0" ] && sourced=1
else # All other shells: examine $0 for known shell binary filenames
# Detects `sh` and `dash`; add additional shell filenames as needed.
case ${0##*/} in sh|dash) sourced=0;; *) sourced=1;; esac
fi
return $sourced
}
if ! _conda_script_is_sourced; then
(
>&2 echo "Error: activate must be sourced. Run 'source activate
envname'"
>&2 echo "instead of 'activate envname'."
)
exit 1
fi
_conda_set_vars
. "$_CONDA_ROOT/etc/profile.d/conda.sh" || return $?
_conda_activate "$@"
```
Comparing from above
```
++ script_dir=/Users/applemacbook/anaconda/bin
++ '[' -z x ']'
++ cd .. /Users/applemacbook/anaconda/etc/profile.d/conda.sh
++ _conda_activate py3
-bash: _conda_activate: command not found
```
to what I get myself
```
++ script_dir=/Users/kfranz/miniconda/bin
++ '[' -z x ']'
++ . /Users/kfranz/miniconda/etc/profile.d/conda.sh
+++ _CONDA_EXE=/Users/kfranz/miniconda/bin/conda
+++ _CONDA_ROOT=/Users/kfranz/miniconda
``` | 2018-01-05T20:59:02 | -1.0 |
conda/conda | 6,719 | conda__conda-6719 | [
"6718",
"6230"
] | ad07eba8949e998728c8041af4fc76c34f6bb9ee | diff --git a/conda/cli/install.py b/conda/cli/install.py
--- a/conda/cli/install.py
+++ b/conda/cli/install.py
@@ -287,7 +287,7 @@ def install(args, parser, command='install'):
spec_regex = r'^(%s)$' % '|'.join(re.escape(s.split()[0]) for s in ospecs)
print('\n# All requested packages already installed.')
for action in action_set:
- print_packages(action["PREFIX"], spec_regex)
+ print_packages(action.get("PREFIX", prefix), spec_regex)
else:
common.stdout_json_success(
message='All requested packages already installed.')
diff --git a/conda/plan.py b/conda/plan.py
--- a/conda/plan.py
+++ b/conda/plan.py
@@ -806,7 +806,7 @@ def revert_actions(prefix, revision=-1, index=None):
if state == curr:
return {}
- dists = (Dist(s) for s in state)
+ dists = tuple(Dist(s) for s in state)
actions = ensure_linked_actions(dists, prefix)
for dist in curr - state:
add_unlink(actions, Dist(dist))
@@ -819,7 +819,6 @@ def revert_actions(prefix, revision=-1, index=None):
if dist not in index:
msg = "Cannot revert to {}, since {} is not in repodata".format(revision, dist)
raise CondaRevisionError(msg)
-
return actions
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -28,7 +28,7 @@
import pytest
import requests
-from conda import CondaError, CondaMultiError, plan
+from conda import CondaError, CondaMultiError, plan, __version__ as CONDA_VERSION
from conda._vendor.auxlib.entity import EntityEncoder
from conda.base.context import context, reset_context
from conda.cli.common import get_index_trap
@@ -58,6 +58,7 @@
from conda.gateways.disk.delete import rm_rf
from conda.gateways.disk.update import touch
from conda.gateways.logging import TRACE
+from conda.gateways.subprocess import subprocess_call
from conda.models.index_record import IndexRecord
from conda.utils import on_win
@@ -157,7 +158,8 @@ def run_command(command, prefix, *arguments, **kwargs):
@contextmanager
def make_temp_env(*packages, **kwargs):
- prefix = kwargs.pop('prefix', None) or make_temp_prefix()
+ name = kwargs.pop('name', None)
+ prefix = kwargs.pop('prefix', None) or make_temp_prefix(name)
assert isdir(prefix), prefix
with disable_logger('fetch'), disable_logger('dotupdate'):
try:
@@ -1202,6 +1204,53 @@ def test_transactional_rollback_upgrade_downgrade(self):
run_command(Commands.INSTALL, prefix, 'flask=0.11.1')
assert_package_is_installed(prefix, 'flask-0.10.1')
+ def test_conda_downgrade(self):
+ # Create an environment with the current conda under test, but include an earlier
+ # version of conda and other packages in that environment.
+ # Make sure we can flip back and forth.
+ conda_exe = join('Scripts', 'conda.exe') if on_win else join('bin', 'conda')
+ with env_var("CONDA_AUTO_UPDATE_CONDA", "false", reset_context):
+ with make_temp_env("conda=4.3.27 python=%s" % sys.version_info[0],
+ name='_' + str(uuid4())[:8]) as prefix: # rev 0
+ assert package_is_installed(prefix, "conda")
+
+ run_command(Commands.INSTALL, prefix, "mccabe") # rev 1
+ assert package_is_installed(prefix, "mccabe")
+
+ subprocess_call("%s install -p %s -y itsdangerous" % (join(prefix, conda_exe), prefix)) # rev 2
+ linked_data_.clear()
+ assert package_is_installed(prefix, "itsdangerous")
+
+ run_command(Commands.INSTALL, prefix, "lockfile") # rev 3
+ assert package_is_installed(prefix, "lockfile")
+
+ subprocess_call("%s install -p %s -y conda=4.3" % (join(prefix, conda_exe), prefix)) # rev 4
+ linked_data_.clear()
+ assert not package_is_installed(prefix, "conda-4.3.27")
+
+ subprocess_call("%s install -p %s -y colorama" % (join(prefix, conda_exe), prefix)) # rev 5
+ linked_data_.clear()
+ assert package_is_installed(prefix, "colorama")
+
+ stdout, stderr = run_command(Commands.LIST, prefix, "--revisions")
+ print(stdout)
+
+ run_command(Commands.INSTALL, prefix, "--rev 3")
+ linked_data_.clear()
+ assert package_is_installed(prefix, "conda-4.3.27")
+ assert not package_is_installed(prefix, "colorama")
+
+ subprocess_call("%s install -y -p %s --rev 1" % (join(prefix, conda_exe), prefix))
+ linked_data_.clear()
+ assert not package_is_installed(prefix, "itsdangerous")
+ linked_data_.clear()
+ assert package_is_installed(prefix, "conda-4.3.27")
+ assert package_is_installed(prefix, "python-%s" % sys.version_info[0])
+
+ result = subprocess_call("%s info --json" % join(prefix, conda_exe))
+ conda_info = json.loads(result.stdout)
+ assert conda_info["conda_version"] == "4.3.27"
+
@pytest.mark.skipif(on_win, reason="openssl only has a postlink script on unix")
def test_run_script_called(self):
import conda.core.link
| conda install --rev is broken in 4.3
per https://github.com/ContinuumIO/anaconda-issues/issues/7855#issuecomment-357036226
Write downgrade integration tests
In an integration test:
1. Create an environment with the current conda under test, but include an earlier version of conda in that environment. Probably something like
conda create -n _test-env python=2.7 itsdangers conda=X.X
2. Subprocess to that conda, and make sure that conda can operate on that environment.
CONDA_ROOT/envs/_test-env/bin/conda install -p CONDA_ROOT/envs/_test-env/bin/conda flask
| 2018-01-11T23:28:25 | -1.0 |
|
conda/conda | 6,724 | conda__conda-6724 | [
"6628"
] | c23e57dfb935b5852eefb4e04187caf82b4e0b78 | diff --git a/conda/plan.py b/conda/plan.py
--- a/conda/plan.py
+++ b/conda/plan.py
@@ -351,7 +351,6 @@ def revert_actions(prefix, revision=-1, index=None):
# TODO: If revision raise a revision error, should always go back to a safe revision
# change
h = History(prefix)
- h.update()
user_requested_specs = itervalues(h.get_requested_specs_map())
try:
state = h.get_state(revision)
@@ -360,7 +359,7 @@ def revert_actions(prefix, revision=-1, index=None):
curr = h.get_state()
if state == curr:
- return {} # TODO: return txn with nothing_to_do
+ return UnlinkLinkTransaction()
_supplement_index_with_prefix(index, prefix)
r = Resolve(index)
@@ -371,11 +370,6 @@ def revert_actions(prefix, revision=-1, index=None):
link_dists = tuple(d for d in state if not is_linked(prefix, d))
unlink_dists = set(curr) - set(state)
- # dists = (Dist(s) for s in state)
- # actions = ensure_linked_actions(dists, prefix)
- # for dist in curr - state:
- # add_unlink(actions, Dist(dist))
-
# check whether it is a safe revision
for dist in concatv(link_dists, unlink_dists):
if dist not in index:
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -1568,6 +1568,7 @@ def test_conda_downgrade(self):
stdout, stderr = run_command(Commands.LIST, prefix, "--revisions")
print(stdout)
+ PrefixData._cache_.clear()
run_command(Commands.INSTALL, prefix, "--rev 3")
PrefixData._cache_.clear()
assert package_is_installed(prefix, "conda-4.3.27")
| Cannot roll back with 'conda install --revision'
```
error: AttributeError("'dict' object has no attribute 'get_pfe'",)
command: C:\Program Files\Anaconda3\Scripts\conda install --revision 4
user_agent: conda/4.4.4 requests/2.18.4 CPython/3.5.2 Windows/8.1 Windows/6.3.9600
_messageid: -9223372036843575560
_messagetime: 1514442489595 / 2017-12-28 00:28:09
Traceback (most recent call last):
File "C:\Program Files\Anaconda3\lib\site-packages\conda\exceptions.py", line 724, in __call__
return func(*args, **kwargs)
File "C:\Program Files\Anaconda3\lib\site-packages\conda\cli\main.py", line 78, in _main
exit_code = do_call(args, p)
File "C:\Program Files\Anaconda3\lib\site-packages\conda\cli\conda_argparse.py", line 75, in do_call
exit_code = getattr(module, func_name)(args, parser)
File "C:\Program Files\Anaconda3\lib\site-packages\conda\cli\main_install.py", line 11, in execute
install(args, parser, 'install')
File "C:\Program Files\Anaconda3\lib\site-packages\conda\cli\install.py", line 232, in install
progressive_fetch_extract = unlink_link_transaction.get_pfe()
AttributeError: 'dict' object has no attribute 'get_pfe'
```
| 2018-01-13T05:54:39 | -1.0 |
|
conda/conda | 6,741 | conda__conda-6741 | [
"6733"
] | d6d3ec3f8546ced1e803f5c232d39ec8da028057 | diff --git a/conda/common/configuration.py b/conda/common/configuration.py
--- a/conda/common/configuration.py
+++ b/conda/common/configuration.py
@@ -206,14 +206,12 @@ class EnvRawParameter(RawParameter):
def value(self, parameter_obj):
if hasattr(parameter_obj, 'string_delimiter'):
+ assert isinstance(self._raw_value, string_types)
string_delimiter = getattr(parameter_obj, 'string_delimiter')
# TODO: add stripping of !important, !top, and !bottom
- raw_value = self._raw_value
- if string_delimiter in raw_value:
- value = raw_value.split(string_delimiter)
- else:
- value = [raw_value]
- return tuple(v.strip() for v in value)
+ return tuple(v for v in (
+ vv.strip() for vv in self._raw_value.split(string_delimiter)
+ ) if v)
else:
return self.__important_split_value[0].strip()
| diff --git a/tests/common/test_configuration.py b/tests/common/test_configuration.py
--- a/tests/common/test_configuration.py
+++ b/tests/common/test_configuration.py
@@ -241,6 +241,21 @@ def make_key(appname, key):
finally:
[environ.pop(key) for key in test_dict]
+ def test_env_var_config_empty_sequence(self):
+ def make_key(appname, key):
+ return "{0}_{1}".format(appname.upper(), key.upper())
+ appname = "myapp"
+ test_dict = {}
+ test_dict[make_key(appname, 'channels')] = ''
+
+ try:
+ environ.update(test_dict)
+ assert 'MYAPP_CHANNELS' in environ
+ config = SampleConfiguration()._set_env_vars(appname)
+ assert config.channels == ()
+ finally:
+ [environ.pop(key) for key in test_dict]
+
def test_load_raw_configs(self):
try:
tempdir = mkdtemp()
| Empty sequences for configuration parameters cannot be expressed in environment variables
<!--
Hi!
This is an issue tracker for conda -- the package manager. File feature requests
for conda here, as well as bug reports about something conda has messed up.
If your issue is a bug report or feature request for:
* a specific conda package from Anaconda ('defaults' channel):
==> file at https://github.com/ContinuumIO/anaconda-issues
* a specific conda package from conda-forge:
==> file at the corresponding feedstock under https://github.com/conda-forge
* repo.continuum.io access and service:
==> file at https://github.com/ContinuumIO/anaconda-issues
* anaconda.org access and service:
==> file at https://anaconda.org/contact/report
* commands under 'conda build':
==> file at https://github.com/conda/conda-build
* commands under 'conda env':
==> please file it here!
* all other conda commands that start with 'conda':
==> please file it here!
-->
**I'm submitting a...**
- [ ] bug report
- [x] feature request
### Current Behavior
<!-- What actually happens? -->
For a configuration parameter that accepts sequences, an empty value in the corresponding environment variable gets interpreted as an one-element sequence containing an empty string, i.e., `[""]`. Thus there is no way to specify an empty sequence via an environment variable.
### Steps to Reproduce
<!-- If the current behavior is a bug, please provide specific, minimal steps to independently reproduce.
Include the exact conda commands that reproduce the issue. -->
```bash
$ export CONDA_CHANNELS=
$ conda config --show channels
channels:
-
$ export CONDA_CHANNELS=defaults,
$ conda config --show channels
channels:
- defaults
-
$ export CONDA_CHANNELS=defaults,,
$ conda config --show channels
channels:
- defaults
-
```
### Expected Behavior
<!-- What do you think should happen? -->
One possibility to allow empty sequences would be to allow and ignore trailing separator, i.e., interpret
* `,` as `[]`,
* `,,` as `[""]`,
* `,,,` as `["", ""]`,
* `defaults,` as `["defaults"]`,
* `defaults,,` as `["defaults", ""]`.
The commands given above would then yield:
```bash
$ export CONDA_CHANNELS=
$ conda config --show channels
channels: []
$ export CONDA_CHANNELS=defaults,
$ conda config --show channels
channels:
- defaults
$ export CONDA_CHANNELS=defaults,,
$ conda config --show channels
channels:
- defaults
-
```
##### `conda info`
<!-- between the ticks below, paste the output of 'conda info' -->
```
active environment : base
active env location : /home/maba/code/conda/conda-4.4
shell level : 1
user config file : /home/maba/.condarc
populated config files : /home/maba/code/conda/conda-4.4/.condarc
conda version : 4.4.7
conda-build version : not installed
python version : 3.6.4.final.0
base environment : /home/maba/code/conda/conda-4.4 (writable)
channel URLs : https://repo.continuum.io/pkgs/main/linux-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
package cache : /home/maba/code/conda/conda-4.4/pkgs
/home/maba/.conda/pkgs
envs directories : /home/maba/code/conda/conda-4.4/envs
/home/maba/.conda/envs
platform : linux-64
user-agent : conda/4.4.7 requests/2.18.4 CPython/3.6.4 Linux/4.14.12-1-MANJARO manjaro/17.1-dev glibc/2.26
UID:GID : 1000:1000
netrc file : None
offline mode : False
```
##### `conda config --show-sources`
<!-- between the ticks below, paste the output of 'conda config --show-sources' -->
(not needed)
##### `conda list --show-channel-urls`
<!-- between the ticks below, paste the output of 'conda list --show-channel-urls' -->
(not needed)
| I think this is the first template we’ve actually had filled out. Like, wow, this is awesome! 👍
Sent from my iPhone
> On Jan 14, 2018, at 5:00 PM, Marcel Bargull <notifications@github.com> wrote:
>
> I'm submitting a...
>
> bug report
> feature request
> Current Behavior
>
> For a configuration parameter that accepts sequences, an empty value in the corresponding environment variable gets interpreted as an one-element sequence containing an empty string, i.e., [""]. Thus there is no way to specify an empty sequence via an environment variable.
>
> Steps to Reproduce
>
> $ export CONDA_CHANNELS=
> $ conda config --show channels
> channels:
> -
> $ export CONDA_CHANNELS=defaults,
> $ conda config --show channels
> channels:
> - defaults
> -
> $ export CONDA_CHANNELS=defaults,,
> $ conda config --show channels
> channels:
> - defaults
> -
> Expected Behavior
>
> One possibility to allow empty sequences would be to allow and ignore trailing separator, i.e., interpret
>
> , as [],
> ,, as [""],
> ,,, as ["", ""],
> defaults, as ["defaults"],
> defaults,, as ["defaults", ""].
> The commands given above would then yield:
>
> $ export CONDA_CHANNELS=
> $ conda config --show channels
> channels: []
> $ export CONDA_CHANNELS=defaults,
> $ conda config --show channels
> channels:
> - defaults
> $ export CONDA_CHANNELS=defaults,,
> $ conda config --show channels
> channels:
> - defaults
> -
> conda info
>
> active environment : base
> active env location : /home/maba/code/conda/conda-4.4
> shell level : 1
> user config file : /home/maba/.condarc
> populated config files : /home/maba/code/conda/conda-4.4/.condarc
> conda version : 4.4.7
> conda-build version : not installed
> python version : 3.6.4.final.0
> base environment : /home/maba/code/conda/conda-4.4 (writable)
> channel URLs : https://repo.continuum.io/pkgs/main/linux-64
> https://repo.continuum.io/pkgs/main/noarch
> https://repo.continuum.io/pkgs/free/linux-64
> https://repo.continuum.io/pkgs/free/noarch
> https://repo.continuum.io/pkgs/r/linux-64
> https://repo.continuum.io/pkgs/r/noarch
> https://repo.continuum.io/pkgs/pro/linux-64
> https://repo.continuum.io/pkgs/pro/noarch
> package cache : /home/maba/code/conda/conda-4.4/pkgs
> /home/maba/.conda/pkgs
> envs directories : /home/maba/code/conda/conda-4.4/envs
> /home/maba/.conda/envs
> platform : linux-64
> user-agent : conda/4.4.7 requests/2.18.4 CPython/3.6.4 Linux/4.14.12-1-MANJARO manjaro/17.1-dev glibc/2.26
> UID:GID : 1000:1000
> netrc file : None
> offline mode : False
> conda config --show-sources
>
> (not needed)
>
> conda list --show-channel-urls
>
> (not needed)
>
> —
> You are receiving this because you are subscribed to this thread.
> Reply to this email directly, view it on GitHub, or mute the thread.
>
| 2018-01-17T19:29:33 | -1.0 |
conda/conda | 6,766 | conda__conda-6766 | [
"6765"
] | ad07eba8949e998728c8041af4fc76c34f6bb9ee | diff --git a/conda/resolve.py b/conda/resolve.py
--- a/conda/resolve.py
+++ b/conda/resolve.py
@@ -628,7 +628,14 @@ def generate_feature_metric(self, C):
for name, group in iteritems(self.groups):
nf = [len(self.features(dist)) for dist in group]
maxf = max(nf)
- eq.update({dist.full_name: maxf-fc for dist, fc in zip(group, nf) if fc < maxf})
+ if maxf > 0:
+ eq.update({dist.full_name: maxf-fc for dist, fc in zip(group, nf) if maxf > fc})
+ # This entry causes conda to weight the absence of a package the same
+ # as if a non-featured version were instaslled. If this were not here,
+ # conda will sometimes select an earlier build of a requested package
+ # if doing so can eliminate this package as a dependency.
+ # https://github.com/conda/conda/issues/6765
+ eq['!' + self.push_MatchSpec(C, name)] = maxf
total += maxf
return eq, total
| diff --git a/tests/test_resolve.py b/tests/test_resolve.py
--- a/tests/test_resolve.py
+++ b/tests/test_resolve.py
@@ -766,6 +766,28 @@ def test_install_package_with_feature():
r.install(['mypackage','feature 1.0'])
+def test_unintentional_feature_downgrade():
+ # See https://github.com/conda/conda/issues/6765
+ # With the bug in place, this bad build of scipy
+ # will be selected for install instead of a later
+ # build of scipy 0.11.0.
+ good_rec = index[Dist('scipy-0.11.0-np17py33_3.tar.bz2')]
+ bad_deps = tuple(d for d in good_rec.depends
+ if not d.startswith('numpy'))
+ bad_rec = IndexRecord.from_objects(good_rec,
+ build=good_rec.build.replace('_3','_x0'),
+ build_number=0, depends=bad_deps,
+ fn=good_rec.fn.replace('_3','_x0'),
+ url=good_rec.url.replace('_3','_x0'))
+ bad_dist = Dist(bad_rec)
+ index2 = index.copy()
+ index2[bad_dist] = bad_rec
+ r = Resolve(index2)
+ install = r.install(['scipy 0.11.0'])
+ assert bad_dist not in install
+ assert any(d.name == 'numpy' for d in install)
+
+
def test_circular_dependencies():
index2 = index.copy()
index2['package1-1.0-0.tar.bz2'] = IndexRecord(**{
| Unintentional build downgrades in mixed feature settings
Under certain limited circumstances involving features, `conda` unexpectedly downgrades to an earlier build of a package. The conditions are as follows:
- The current version of a package `foo` has multiple builds with distinct build numbers.
- Newer builds depend on a second package `bar`, while older builds do not.
- Some of the valid choices for the `bar` dependency have features, and some do not.
When those conditions are met, the command
```
conda create -n errtest foo
```
will select an older build of `foo`, without the `bar` dependency, instead of the latest build. (Contact me or @msarahan for a specific test case.)
Currently, this is not occurring with conda 4.4. The reasons for this are not fully clear, because the bug has been located and exists in 4.3 and 4.4. Bug fix coming.
| To help illustrate the issue, here is a preliminary version of the test that should fail with the current version of `Resolve`, and which will be fixed.
```
def test_unintentional_feature_downgrade():
# With the bug in place, this bad build of scipy
# will be selected for install instead of a later
# build of scipy 0.11.0.
index2 = index.copy()
bad_dist = Dist('scipy-0.11.0-np17py33_x0.tar.bz2')
index2[bad_dist] = IndexRecord(**{
'build': 'np17py33_x0',
'build_number': 0,
'depends': ['python 3.3*'],
'name': 'scipy',
'version': '0.11.0',
})
r = Resolve(index2)
install = r.install(['scipy 0.11.0'])
assert bad_dist not in install
assert any(d.name == 'numpy' for d in install)
``` | 2018-01-23T02:24:30 | -1.0 |
conda/conda | 6,777 | conda__conda-6777 | [
"6739"
] | d503c26e0a9165f21b758238cdf08c3f1b81d20f | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -43,6 +43,12 @@ def __init__(self, shell, arguments=None):
self.shell = shell
self._raw_arguments = arguments
+ if PY2:
+ self.environ = {ensure_fs_path_encoding(k): ensure_fs_path_encoding(v)
+ for k, v in iteritems(os.environ)}
+ else:
+ self.environ = os.environ.copy()
+
if shell == 'posix':
self.pathsep_join = ':'.join
self.path_conversion = native_path_to_unix
@@ -215,13 +221,13 @@ def build_activate(self, env_name_or_prefix):
prefix = normpath(prefix)
# query environment
- old_conda_shlvl = int(os.getenv('CONDA_SHLVL', 0))
- old_conda_prefix = os.getenv('CONDA_PREFIX')
+ old_conda_shlvl = int(self.environ.get('CONDA_SHLVL', 0))
+ old_conda_prefix = self.environ.get('CONDA_PREFIX')
max_shlvl = context.max_shlvl
if old_conda_prefix == prefix:
return self.build_reactivate()
- if os.getenv('CONDA_PREFIX_%s' % (old_conda_shlvl-1)) == prefix:
+ if self.environ.get('CONDA_PREFIX_%s' % (old_conda_shlvl-1)) == prefix:
# in this case, user is attempting to activate the previous environment,
# i.e. step back down
return self.build_deactivate()
@@ -282,8 +288,8 @@ def build_activate(self, env_name_or_prefix):
def build_deactivate(self):
# query environment
- old_conda_shlvl = int(os.getenv('CONDA_SHLVL', 0))
- old_conda_prefix = os.getenv('CONDA_PREFIX', None)
+ old_conda_shlvl = int(self.environ.get('CONDA_SHLVL', 0))
+ old_conda_prefix = self.environ.get('CONDA_PREFIX', None)
if old_conda_shlvl <= 0 or old_conda_prefix is None:
return {
'unset_vars': (),
@@ -314,7 +320,7 @@ def build_deactivate(self):
}
activate_scripts = ()
else:
- new_prefix = os.getenv('CONDA_PREFIX_%d' % new_conda_shlvl)
+ new_prefix = self.environ.get('CONDA_PREFIX_%d' % new_conda_shlvl)
conda_default_env = self._default_env(new_prefix)
conda_prompt_modifier = self._prompt_modifier(conda_default_env)
@@ -341,8 +347,8 @@ def build_deactivate(self):
}
def build_reactivate(self):
- conda_prefix = os.environ.get('CONDA_PREFIX')
- conda_shlvl = int(os.environ.get('CONDA_SHLVL', -1))
+ conda_prefix = self.environ.get('CONDA_PREFIX')
+ conda_shlvl = int(self.environ.get('CONDA_SHLVL', -1))
if not conda_prefix or conda_shlvl < 1:
# no active environment, so cannot reactivate; do nothing
return {
@@ -352,7 +358,7 @@ def build_reactivate(self):
'deactivate_scripts': (),
'activate_scripts': (),
}
- conda_default_env = os.environ.get('CONDA_DEFAULT_ENV', self._default_env(conda_prefix))
+ conda_default_env = self.environ.get('CONDA_DEFAULT_ENV', self._default_env(conda_prefix))
# environment variables are set only to aid transition from conda 4.3 to conda 4.4
return {
'unset_vars': (),
@@ -366,7 +372,7 @@ def build_reactivate(self):
}
def _get_starting_path_list(self):
- path = os.environ['PATH']
+ path = self.environ['PATH']
if on_win:
# On Windows, the Anaconda Python interpreter prepends sys.prefix\Library\bin on
# startup. It's a hack that allows users to avoid using the correct activation
@@ -446,8 +452,8 @@ def _update_prompt(self, set_vars, conda_prompt_modifier):
return
if self.shell == 'posix':
- ps1 = os.environ.get('PS1', '')
- current_prompt_modifier = os.environ.get('CONDA_PROMPT_MODIFIER')
+ ps1 = self.environ.get('PS1', '')
+ current_prompt_modifier = self.environ.get('CONDA_PROMPT_MODIFIER')
if current_prompt_modifier:
ps1 = re.sub(re.escape(current_prompt_modifier), r'', ps1)
# Because we're using single-quotes to set shell variables, we need to handle the
@@ -458,8 +464,8 @@ def _update_prompt(self, set_vars, conda_prompt_modifier):
'PS1': conda_prompt_modifier + ps1,
})
elif self.shell == 'csh':
- prompt = os.environ.get('prompt', '')
- current_prompt_modifier = os.environ.get('CONDA_PROMPT_MODIFIER')
+ prompt = self.environ.get('prompt', '')
+ current_prompt_modifier = self.environ.get('CONDA_PROMPT_MODIFIER')
if current_prompt_modifier:
prompt = re.sub(re.escape(current_prompt_modifier), r'', prompt)
set_vars.update({
@@ -498,6 +504,13 @@ def ensure_binary(value):
return value
+def ensure_fs_path_encoding(value):
+ try:
+ return value.decode(FILESYSTEM_ENCODING)
+ except AttributeError:
+ return value
+
+
def native_path_to_unix(paths): # pragma: unix no cover
# on windows, uses cygpath to convert windows native paths to posix paths
if not on_win:
@@ -532,6 +545,7 @@ def path_identity(paths):
on_win = bool(sys.platform == "win32")
PY2 = sys.version_info[0] == 2
+FILESYSTEM_ENCODING = sys.getfilesystemencoding()
if PY2: # pragma: py3 no cover
string_types = basestring, # NOQA
text_type = unicode # NOQA
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -471,6 +471,7 @@ def test_posix_basic(self):
'CONDA_SHLVL': '1',
'PATH': os.pathsep.join(concatv(new_path_parts, (os.environ['PATH'],))),
}):
+ activator = Activator('posix')
with captured() as c:
rc = activate_main(('', 'shell.posix', 'reactivate'))
assert not c.stderr
@@ -549,6 +550,7 @@ def test_cmd_exe_basic(self):
'CONDA_SHLVL': '1',
'PATH': os.pathsep.join(concatv(new_path_parts, (os.environ['PATH'],))),
}):
+ activator = Activator('cmd.exe')
with captured() as c:
assert activate_main(('', 'shell.cmd.exe', 'reactivate')) == 0
assert not c.stderr
@@ -626,6 +628,7 @@ def test_csh_basic(self):
'CONDA_SHLVL': '1',
'PATH': os.pathsep.join(concatv(new_path_parts, (os.environ['PATH'],))),
}):
+ activator = Activator('csh')
with captured() as c:
rc = activate_main(('', 'shell.csh', 'reactivate'))
assert not c.stderr
@@ -701,6 +704,7 @@ def test_xonsh_basic(self):
'CONDA_SHLVL': '1',
'PATH': os.pathsep.join(concatv(new_path_parts, (os.environ['PATH'],))),
}):
+ activator = Activator('xonsh')
with captured() as c:
assert activate_main(('', 'shell.xonsh', 'reactivate')) == 0
assert not c.stderr
@@ -776,6 +780,7 @@ def test_fish_basic(self):
'CONDA_SHLVL': '1',
'PATH': os.pathsep.join(concatv(new_path_parts, (os.environ['PATH'],))),
}):
+ activator = Activator('fish')
with captured() as c:
rc = activate_main(('', 'shell.fish', 'reactivate'))
assert not c.stderr
@@ -845,6 +850,7 @@ def test_powershell_basic(self):
'CONDA_SHLVL': '1',
'PATH': os.pathsep.join(concatv(new_path_parts, (os.environ['PATH'],))),
}):
+ activator = Activator('powershell')
with captured() as c:
rc = activate_main(('', 'shell.powershell', 'reactivate'))
assert not c.stderr
| source activate env error
**I'm submitting a...**
- [x] bug report
- [ ] feature request
### Current Behavior
when i try to activate a conda env,this doesn't work for me
the output is:
```
Traceback (most recent call last):
File "/anaconda/lib/python2.7/site-packages/conda/gateways/logging.py", line 64, in emit
msg = self.format(record)
File "/anaconda/lib/python2.7/logging/__init__.py", line 734, in format
return fmt.format(record)
File "/anaconda/lib/python2.7/logging/__init__.py", line 465, in format
record.message = record.getMessage()
File "/anaconda/lib/python2.7/logging/__init__.py", line 329, in getMessage
msg = msg % self.args
File "/anaconda/lib/python2.7/site-packages/conda/__init__.py", line 43, in __repr__
return '%s: %s' % (self.__class__.__name__, text_type(self))
File "/anaconda/lib/python2.7/site-packages/conda/__init__.py", line 47, in __str__
return text_type(self.message % self._kwargs)
ValueError: unsupported format character '{' (0x7b) at index 513
Logged from file exceptions.py, line 724
```
### Steps to Reproduce
i have regularly updated conda to the lateset version recently,
and i installed pipenv.i know that cannot work with a conda-created env,
but i suspect this may lead to this error
then this error came
### Expected Behavior
<!-- What do you think should happen? -->
##### `conda info`
<!-- between the ticks below, paste the output of 'conda info' -->
```
active environment : None
shell level : 0
user config file : /Users/bubu/.condarc
populated config files : /Users/bubu/.condarc
conda version : 4.4.7
conda-build version : 3.2.1
python version : 2.7.13.final.0
base environment : /anaconda (writable)
channel URLs : https://conda.anaconda.org/anaconda-fusion/osx-64
https://conda.anaconda.org/anaconda-fusion/noarch
https://repo.continuum.io/pkgs/main/osx-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/osx-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/osx-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/osx-64
https://repo.continuum.io/pkgs/pro/noarch
package cache : /anaconda/pkgs
/Users/bubu/.conda/pkgs
envs directories : /anaconda/envs
/Users/bubu/.conda/envs
platform : osx-64
user-agent : conda/4.4.7 requests/2.18.4 CPython/2.7.13 Darwin/17.3.0 OSX/10.13.2
UID:GID : 501:20
netrc file : None
offline mode : False
```
##### `conda config --show-sources`
<!-- between the ticks below, paste the output of 'conda config --show-sources' -->
```
==> /Users/bubu/.condarc <==
ssl_verify: True
channels:
- anaconda-fusion
- defaults
```
##### `conda list --show-channel-urls`
<!-- between the ticks below, paste the output of 'conda list --show-channel-urls' -->
```
# packages in environment at /anaconda:
#
_license 1.1 py27_1 defaults
alabaster 0.7.9 py27_0 defaults
amqp 1.4.9 <pip>
anaconda custom py27_0 defaults
anaconda-client 1.6.3 py27_0 defaults
anaconda-navigator 1.5.2 py27_0 defaults
anaconda-project 0.4.1 py27_0 defaults
ansible 2.4.1.0 <pip>
apipkg 1.4 <pip>
appdirs 1.4.3 <pip>
appnope 0.1.0 py27_0 defaults
appscript 1.0.1 py27_0 defaults
argcomplete 1.0.0 py27_1 defaults
argon2-cffi 16.3.0 <pip>
astroid 1.4.9 py27_0 defaults
astropy 1.3.3 np112py27_0 defaults
attrs 17.2.0 <pip>
autobahn 17.7.1 <pip>
Automat 0.6.0 <pip>
babel 2.3.4 py27_0 defaults
backports 1.0 py27_0 defaults
backports.weakref 1.0rc1 <pip>
backports_abc 0.5 py27_0 defaults
bcrypt 3.1.4 <pip>
beautifulsoup4 4.5.3 py27_0 defaults
billiard 3.3.0.23 <pip>
binaryornot 0.4.3 <pip>
bitarray 0.8.1 py27_0 defaults
blaze 0.10.1 py27_0 defaults
bleach 2.0.0 <pip>
bokeh 0.12.4 py27_0 defaults
boto 2.45.0 py27_0 defaults
bottle 0.12.13 <pip>
bottle-sqlite 0.1.3 <pip>
bottleneck 1.2.1 np112py27_0 defaults
ca-certificates 2017.08.26 ha1e5d58_0 defaults
cache-client 0.1.0 <pip>
cdecimal 2.3 py27_2 defaults
celery 3.1.23 <pip>
certifi 2017.11.5 py27hfa9a1c4_0 defaults
certifi 2017.4.17 <pip>
cffi 1.9.1 py27_0 defaults
Chameleon 3.1 <pip>
chardet 2.3.0 py27_0 defaults
chardet 3.0.3 <pip>
check-manifest 0.35 <pip>
chest 0.2.3 py27_0 defaults
click 6.7 py27_0 defaults
click-default-group 1.2 <pip>
cloudpickle 0.2.2 py27_0 defaults
clyent 1.2.2 py27_0 defaults
colorama 0.3.7 py27_0 defaults
conda 4.4.7 py27_0 defaults
conda-build 3.2.1 py27_0 defaults
conda-env 2.6.0 h36134e3_0 defaults
conda-verify 2.0.0 py27_0 defaults
configobj 5.0.6 py27_0 defaults
configparser 3.5.0 py27_0 defaults
constantly 15.1.0 <pip>
contextlib2 0.5.4 py27_0 defaults
cookiecutter 1.5.1 <pip>
crockford 0.0.2 <pip>
cryptography 1.7.1 py27_0 defaults
cssselect 1.0.1 <pip>
curl 7.52.1 0 defaults
cvxopt 1.1.9 <pip>
cycler 0.10.0 py27_0 defaults
cython 0.25.2 py27_0 defaults
cytoolz 0.8.2 py27_0 defaults
dask 0.13.0 py27_0 defaults
datashape 0.5.4 py27_0 defaults
decorator 4.0.11 py27_0 defaults
defusedxml 0.5.0 <pip>
devpi 2.2.0 <pip>
devpi-client 3.0.0 <pip>
devpi-common 3.1.0 <pip>
devpi-server 4.3.0 <pip>
devpi-web 3.2.0 <pip>
dill 0.2.5 py27_0 defaults
Django 1.10.5 <pip>
django-extensions 1.7.9 <pip>
django-filter 1.0.1 <pip>
django-rest-swagger 2.1.1 <pip>
django-silk 0.7.3 <pip>
djangorestframework 3.5.4 <pip>
djangorestframework-bulk 0.2.1 <pip>
dlib 19.4.0 <pip>
docopt 0.6.2 <pip>
docutils 0.13.1 py27_0 defaults
dpkt 1.9.1 <pip>
dry-rest-permissions 0.1.8 <pip>
entrypoints 0.2.2 py27_0 defaults
entrypoints 0.2.2 <pip>
enum34 1.1.6 py27_0 defaults
environment-kernels 1.1 <pip>
et_xmlfile 1.0.1 py27_0 defaults
etu-tools 0.3.0 <pip>
execnet 1.4.1 <pip>
factory-boy 2.8.1 <pip>
fake-useragent 0.1.7 <pip>
Faker 0.7.12 <pip>
falcon 1.2.0 <pip>
fastcache 1.0.2 py27_1 defaults
filelock 2.0.7 py27_0 defaults
fire 0.1.2 <pip>
first 2.0.1 <pip>
flake8 3.3.0 <pip>
Flask 0.3 <pip>
flask 0.12 py27_0 defaults
flask-cors 3.0.2 py27_0 defaults
Flask-Login 0.4.0 <pip>
freetype 2.5.5 2 defaults
funcsigs 1.0.2 py27_0 defaults
functools32 3.2.3.2 py27_0 defaults
future 0.16.0 <pip>
futures 3.0.5 py27_0 defaults
GB2260 0.4.1 <pip>
gdbn 0.1 <pip>
get_terminal_size 1.0.0 py27_0 defaults
gevent 1.2.2 <pip>
gevent 1.2.1 py27_0 defaults
Glances 2.9.1 <pip>
glob2 0.6 py27h55c9705_0 defaults
gnumpy 0.2 <pip>
greenlet 0.4.12 <pip>
greenlet 0.4.11 py27_0 defaults
grequests 0.3.0 <pip>
grin 1.2.1 py27_3 defaults
gunicorn 19.7.1 <pip>
gym 0.9.3 <pip>
h5py 2.7.0 np112py27_0 defaults
hdf5 1.8.17 1 defaults
heapdict 1.0.0 py27_1 defaults
hkdf 0.0.3 <pip>
holidays 0.8.1 <pip>
html5lib 0.999999999 <pip>
humanize 0.5.1 <pip>
hupper 1.0 <pip>
hyperlink 17.3.0 <pip>
icu 54.1 0 defaults
idna 2.6 <pip>
idna 2.2 py27_0 defaults
imagesize 0.7.1 py27_0 defaults
incremental 17.5.0 <pip>
ipaddress 1.0.18 py27_0 defaults
ipykernel 4.5.2 py27_0 defaults
ipykernel 4.6.1 <pip>
ipython 5.1.0 py27_1 defaults
ipython 5.4.1 <pip>
ipython_genutils 0.1.0 py27_0 defaults
ipywidgets 6.0.0 <pip>
ipywidgets 5.2.2 py27_1 defaults
isbnlib 3.7.2 <pip>
isort 4.2.5 py27_0 defaults
itchat 1.3.10 <pip>
itsdangerous 0.24 py27_0 defaults
jbig 2.1 0 defaults
jdcal 1.3 py27_0 defaults
jedi 0.10.2 <pip>
jedi 0.9.0 py27_1 defaults
jieba 0.39 <pip>
jinja2 2.9.4 py27_0 defaults
jinja2-time 0.2.0 <pip>
joblib 0.11 <pip>
jpeg 9b 0 defaults
js2xml 0.3.1 <pip>
json-lines 0.3.1 <pip>
jsonschema 2.5.1 py27_0 defaults
jsonschema 2.6.0 <pip>
jupyter 1.0.0 py27_3 defaults
jupyter-client 5.0.1 <pip>
jupyter-core 4.3.0 <pip>
jupyter_client 4.4.0 py27_0 defaults
jupyter_console 5.0.0 py27_0 defaults
jupyter_core 4.2.1 py27_0 defaults
kii-client 0.1.3 <pip>
kombu 3.0.37 <pip>
lazy-object-proxy 1.2.2 py27_0 defaults
libiconv 1.14 0 defaults
libpng 1.6.27 0 defaults
libtiff 4.0.6 3 defaults
libxml2 2.9.4 0 defaults
libxslt 1.1.29 0 defaults
line-profiler 2.0 <pip>
llvmlite 0.18.0 py27_0 defaults
locket 0.2.0 py27_1 defaults
lxml 3.7.2 py27_0 defaults
magic-wormhole 0.10.2 <pip>
Markdown 2.2.0 <pip>
markupsafe 0.23 py27_2 defaults
matplotlib 2.0.2 np112py27_0 defaults
mccabe 0.6.1 <pip>
meld3 1.0.2 <pip>
mistune 0.7.4 <pip>
mistune 0.7.3 py27_1 defaults
mkl 2017.0.1 0 defaults
mkl-service 1.1.2 py27_3 defaults
more-itertools 3.2.0 <pip>
mpmath 0.19 py27_1 defaults
multipledispatch 0.4.9 py27_0 defaults
mysql 0.0.1 <pip>
MySQL-python 1.2.5 <pip>
nbconvert 4.2.0 py27_0 defaults
nbconvert 5.2.1 <pip>
nbformat 4.2.0 py27_0 defaults
nbformat 4.3.0 <pip>
ncmbot 0.1.6 <pip>
networkx 1.11 py27_0 defaults
nlib 0.6 <pip>
nltk 3.2.2 py27_0 defaults
nolearn 0.5b1 <pip>
nose 1.3.7 py27_1 defaults
notebook 5.0.0 <pip>
notebook 4.3.1 py27_0 defaults
numba 0.33.0 np112py27_0 defaults
numexpr 2.6.2 np112py27_0 defaults
numpy 1.12.1 py27_0 defaults
numpydoc 0.6.0 py27_0 defaults
odo 0.5.0 py27_1 defaults
olefile 0.44 <pip>
openapi-codec 1.3.2 <pip>
opencv-python 3.2.0.7 <pip>
openface 0.2.1 <pip>
openpyxl 2.4.1 py27_0 defaults
openssl 1.0.2n hdbc3d79_0 defaults
org-client 0.4.12 <pip>
packaging 16.8 <pip>
pandas 0.17.1 <pip>
pandas 0.20.2 np112py27_0 defaults
pandocfilters 1.4.1 <pip>
paramiko 2.3.1 <pip>
parse-accept-language 0.1.2 <pip>
parsel 1.2.0 <pip>
partd 0.3.7 py27_0 defaults
passlib 1.7.1 <pip>
PasteDeploy 1.5.2 <pip>
path.py 10.0 py27_0 defaults
pathlib 1.0.1 <pip>
pathlib2 2.2.0 py27_0 defaults
patsy 0.4.1 py27_0 defaults
pendulum 1.0.2 <pip>
pep8 1.7.0 py27_0 defaults
petl 1.1.1 <pip>
pew 1.1.2 <pip>
pexpect 4.2.1 py27_0 defaults
pickleshare 0.7.4 py27_0 defaults
pigar 0.7.0 <pip>
pillow 4.0.0 py27_0 defaults
pip 9.0.1 py27h1567d89_4 defaults
pip-tools 1.9.0 <pip>
pipreqs 0.4.9 <pip>
pkginfo 1.4.1 <pip>
pkginfo 1.4.1 py27_0 defaults
pluggy 0.4.0 <pip>
ply 3.9 py27_0 defaults
poyo 0.4.1 <pip>
pprofile 1.11.0 <pip>
profiling 0.1.3 <pip>
progressbar 2.3 <pip>
prompt_toolkit 1.0.9 py27_0 defaults
protobuf 3.3.0 <pip>
psutil 5.0.1 py27_0 defaults
psycopg2 2.7.1 <pip>
ptpython 0.39 <pip>
ptyprocess 0.5.1 py27_0 defaults
py 1.4.32 py27_0 defaults
py-heat 0.0.2 <pip>
py-heat-magic 0.0.2 <pip>
pyasn1 0.1.9 py27_0 defaults
pyasn1-modules 0.0.10 <pip>
pyaudio 0.2.7 py27_0 defaults
pycodestyle 2.3.1 <pip>
pycosat 0.6.3 py27h6c51c7e_0 defaults
pycparser 2.17 py27_0 defaults
pycrypto 2.6.1 py27_4 defaults
pycurl 7.43.0 py27_2 defaults
PyDispatcher 2.0.5 <pip>
pyflakes 1.5.0 py27_0 defaults
pyglet 1.2.4 <pip>
pygments 2.1.3 py27_0 defaults
pylint 1.6.4 py27_1 defaults
pymongo 3.5.1 <pip>
PyMySQL 0.7.11 <pip>
PyNaCl 1.1.2 <pip>
pyopenssl 16.2.0 py27_0 defaults
pyparsing 2.1.4 py27_0 defaults
pyparsing 2.2.0 <pip>
pypng 0.0.18 <pip>
PyQRCode 1.2.1 <pip>
pyqt 5.6.0 py27_1 defaults
pyquery 1.3.0 <pip>
pyramid 1.8.3 <pip>
pyramid-chameleon 0.3 <pip>
pyresttest 1.7.2.dev0 <pip>
pyspider 0.3.9 <pip>
pytables 3.3.0 np112py27_0 defaults
pytest 3.0.5 py27_0 defaults
python 2.7.13 0 defaults
python-cjson 1.2.1 <pip>
python-dateutil 2.6.0 py27_0 defaults
python-mimeparse 1.6.0 <pip>
python.app 1.2 py27_4 defaults
pythonpy 0.4.11 <pip>
pytz 2016.10 py27_0 defaults
pywavelets 0.5.2 np112py27_0 defaults
pyyaml 3.12 py27_0 defaults
pyzmq 16.0.2 py27_0 defaults
qiniu 7.2.0 <pip>
qt 5.6.2 0 defaults
qtawesome 0.4.3 py27_0 defaults
qtconsole 4.2.1 py27_1 defaults
qtmodern 0.1.4 <pip>
qtpy 1.2.1 py27_0 defaults
QtPy 1.3.1 <pip>
queuelib 1.4.2 <pip>
raven 6.0.0 <pip>
readline 6.2 2 defaults
readme-renderer 17.2 <pip>
redis 3.2.0 0 defaults
redis-py 2.10.5 py27_0 defaults
repoze.lru 0.6 <pip>
requestium 0.1.9 <pip>
requests 2.12.4 py27_0 defaults
requests 2.18.4 <pip>
requests-file 1.4.2 <pip>
rope 0.9.4 py27_1 defaults
ruamel_yaml 0.11.14 py27_1 defaults
scandir 1.4 py27_0 defaults
scikit-image 0.13.0 np112py27_0 defaults
scikit-learn 0.18.2 np112py27_0 defaults
scikit-learn 0.17.1 <pip>
scipy 0.19.1 np112py27_0 defaults
scipy 0.16.1 <pip>
Scrapy 1.4.0 <pip>
seaborn 0.7.1 py27_0 defaults
selenium 3.8.0 <pip>
serpy 0.1.1 <pip>
service-identity 17.0.0 <pip>
setuptools 38.4.0 py27_0 defaults
setuptools 38.4.0 <pip>
shutilwhich 1.1.0 <pip>
simplegeneric 0.8.1 py27_1 defaults
simplejson 3.11.1 <pip>
singledispatch 3.4.0.3 py27_0 defaults
sip 4.18 py27_0 defaults
six 1.10.0 py27_0 defaults
sklearn 0.0 <pip>
slimit 0.8.1 <pip>
snowballstemmer 1.2.1 py27_0 defaults
sockjs-tornado 1.0.3 py27_0 defaults
spake2 0.7 <pip>
speechpy 1.1 <pip>
sphinx 1.5.1 py27_0 defaults
spyder 3.1.2 py27_0 defaults
sqlalchemy 1.1.5 py27_0 defaults
sqlite 3.13.0 0 defaults
ssl_match_hostname 3.4.0.2 py27_1 defaults
sso-auth-drf 1.4.2 <pip>
statsmodels 0.8.0 np112py27_0 defaults
subprocess32 3.2.7 py27_0 defaults
supervisor 3.3.2 <pip>
sympy 1.0 py27_0 defaults
tblib 1.3.2 <pip>
tensorflow 1.2.0 <pip>
terminado 0.6 py27_0 defaults
terminaltables 3.1.0 <pip>
testpath 0.3.1 <pip>
textrank4zh 0.3 <pip>
tflearn 0.3.2 <pip>
tk 8.5.18 0 defaults
tldextract 2.2.0 <pip>
toolz 0.8.2 py27_0 defaults
tornado 4.4.2 py27_0 defaults
tornado 4.5.1 <pip>
tox 2.7.0 <pip>
tqdm 4.15.0 <pip>
traitlets 4.3.1 py27_0 defaults
translationstring 1.3 <pip>
Twisted 17.5.0 <pip>
txaio 2.8.1 <pip>
txtorcon 0.19.3 <pip>
u-msgpack-python 2.4.1 <pip>
unicodecsv 0.14.1 py27_0 defaults
urllib3 1.22 <pip>
urwid 1.3.1 <pip>
valuedispatch 0.0.1 <pip>
venusian 1.1.0 <pip>
virtualenv 15.1.0 <pip>
virtualenv-clone 0.2.6 <pip>
w3lib 1.18.0 <pip>
waitress 1.0.2 <pip>
wcwidth 0.1.7 py27_0 defaults
web.py 0.38 <pip>
webencodings 0.5.1 <pip>
WebOb 1.7.2 <pip>
weibo 0.2.2 <pip>
Werkzeug 0.12.1 <pip>
werkzeug 0.11.15 py27_0 defaults
wheel 0.29.0 py27_0 defaults
whichcraft 0.4.1 <pip>
Whoosh 2.7.4 <pip>
widgetsnbextension 2.0.0 <pip>
widgetsnbextension 1.2.6 py27_0 defaults
wrapt 1.10.8 py27_0 defaults
WsgiDAV 2.2.4 <pip>
xlrd 1.0.0 py27_0 defaults
xlsxwriter 0.9.6 py27_0 defaults
xlwings 0.10.2 py27_0 defaults
xlwt 1.2.0 py27_0 defaults
xz 5.2.2 1 defaults
yaml 0.1.6 0 defaults
yarg 0.1.9 <pip>
zlib 1.2.8 3 defaults
zoo-client 0.7.8 <pip>
zope.deprecation 4.2.0 <pip>
zope.interface 4.4.1 <pip>
```
| Have the same issue after an update
The same issue +1
The same issue +1
Can someone who is having this problem please give me the output of
CONDA_VERBOSE=3 conda shell.posix activate base
For people having this problem, please give me step-by-step setup and commands I can use to reproduce this myself.
```
→ source activate py3
Traceback (most recent call last):
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/gateways/logging.py", line 64, in emit
msg = self.format(record)
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 734, in format
return fmt.format(record)
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 465, in format
record.message = record.getMessage()
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 329, in getMessage
msg = msg % self.args
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/__init__.py", line 43, in __repr__
return '%s: %s' % (self.__class__.__name__, text_type(self))
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/__init__.py", line 47, in __str__
return text_type(self.message % self._kwargs)
ValueError: unsupported format character '{' (0x7b) at index 447
Logged from file exceptions.py, line 724
```
```
→ CONDA_VERBOSE=3 conda shell.posix activate base
PS1='(base) '
export CONDA_DEFAULT_ENV='base'
export CONDA_PREFIX='/Users/sherbein/opt/miniconda2'
export CONDA_PROMPT_MODIFIER='(base) '
export CONDA_PYTHON_EXE='/Users/sherbein/opt/miniconda2/bin/python'
export CONDA_SHLVL='1'
export PATH='/Users/sherbein/opt/miniconda2/bin:/Users/sherbein/opt/miniconda2/bin:/Users/sherbein/.cargo/bin:/usr/local/bin:/usr/local/texlive/2016/bin/x86_64-darwin:/usr/local/sbin:/Users/sherbein/.dotfiles/bin:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/Applications/VMware Fusion.app/Contents/Public:/Library/TeX/texbin:/opt/X11/bin:/Applications/Wireshark.app/Contents/MacOS:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-zsh/lib:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-zsh/plugins/git:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-zsh/plugins/command-not-found:/Users/sherbein/.antigen/bundles/zsh-users/zsh-syntax-highlighting'
```
FWIW, I get the exact same error when I try and use `conda activate py3` (both before and after removing conda from my PATH and sourcing `conda.sh` in `etc/profile.d`)
@SteVwonder: Can you show the output of
```
/Users/sherbein/opt/miniconda2/bin/python -c 'import conda; conda.CondaError.__str__ = lambda self: "\n".join(map(conda.text_type, [self.message, self._kwargs])); from conda.cli.main import main; main("conda", "shell.posix", "activate", "py3")'
```
?
```
→ /Users/sherbein/opt/miniconda2/bin/python -c 'import conda; conda.CondaError.__str__ = lambda self: "\n".join(map(conda.text_type, [self.message, self._kwargs])); from conda.cli.main import main; main("conda", "shell.posix", "activate", "py3")'
# >>>>>>>>>>>>>>>>>>>>>> ERROR REPORT <<<<<<<<<<<<<<<<<<<<<<
Traceback (most recent call last):
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/cli/main.py", line 111, in main
return activator_main()
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/activate.py", line 551, in main
assert len(argv) >= 3
AssertionError
`$ -c`
environment variables:
CIO_TEST=<not set>
CONDA_ROOT=/Users/sherbein/opt/miniconda2
PATH=/Users/sherbein/opt/miniconda2/bin:/Users/sherbein/.cargo/bin:/usr/loc
al/bin:/usr/local/texlive/2016/bin/x86_64-darwin:/usr/local/sbin:/User
s/sherbein/.dotfiles/bin:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:
/Applications/VMware Fusion.app/Contents/Public:/Library/TeX/texbin:/o
pt/X11/bin:/Applications/Wireshark.app/Contents/MacOS:/Users/sherbein/
.antigen/bundles/robbyrussell/oh-my-
zsh/lib:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-
zsh/plugins/git:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-
zsh/plugins/command-not-found:/Users/sherbein/.antigen/bundles/zsh-
users/zsh-syntax-highlighting
PKG_CONFIG_PATH=/Users/sherbein/.local/munge/0.5.13/lib/pkgconfig
REQUESTS_CA_BUNDLE=<not set>
SSL_CERT_FILE=<not set>
SUDO_EDITOR=emacsclient -nw
active environment : None
user config file : /Users/sherbein/.condarc
populated config files :
conda version : 4.4.7
conda-build version : not installed
python version : 2.7.14.final.0
base environment : /Users/sherbein/opt/miniconda2 (writable)
channel URLs : https://repo.continuum.io/pkgs/main/osx-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/osx-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/osx-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/osx-64
https://repo.continuum.io/pkgs/pro/noarch
package cache : /Users/sherbein/opt/miniconda2/pkgs
/Users/sherbein/.conda/pkgs
envs directories : /Users/sherbein/opt/miniconda2/envs
/Users/sherbein/.conda/envs
platform : osx-64
user-agent : conda/4.4.7 requests/2.18.4 CPython/2.7.14 Darwin/17.3.0 OSX/10.13.2
UID:GID : 501:20
netrc file : None
offline mode : False
```
Ah, there was an error in the command I wrote down, sorry.
Can you try
```
/Users/sherbein/opt/miniconda2/bin/python -c 'import conda; conda.CondaError.__str__ = lambda self: "\n".join(map(conda.text_type, [self.message, self._kwargs])); from conda.cli.main import main; import sys; sys.argv[:]=["conda", "shell.posix", "activate", "py3"]; main()'
```
instead?
```
PS1='(py3) '
export CONDA_DEFAULT_ENV='py3'
export CONDA_PREFIX='/Users/sherbein/opt/miniconda2/envs/py3'
export CONDA_PROMPT_MODIFIER='(py3) '
export CONDA_PYTHON_EXE='/Users/sherbein/opt/miniconda2/bin/python'
export CONDA_SHLVL='1'
export PATH='/Users/sherbein/opt/miniconda2/envs/py3/bin:/Users/sherbein/opt/miniconda2/bin:/Users/sherbein/.cargo/bin:/usr/local/bin:/usr/local/texlive/2016/bin/x86_64-darwin:/usr/local/sbin:/Users/sherbein/.dotfiles/bin:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/Applications/VMware Fusion.app/Contents/Public:/Library/TeX/texbin:/opt/X11/bin:/Applications/Wireshark.app/Contents/MacOS:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-zsh/lib:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-zsh/plugins/git:/Users/sherbein/.antigen/bundles/robbyrussell/oh-my-zsh/plugins/command-not-found:/Users/sherbein/.antigen/bundles/zsh-users/zsh-syntax-highlighting'
```
Now that's strange.. I don't understand how your previous `source activate py3` was able to error out. The Python code that gets executed by `source activate` should be the same as with `conda shell.posix activate py3` which didn't fail somehow...
Can you show
```
head /Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh
```
?
```
→ head /Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh
_CONDA_EXE="/Users/sherbein/opt/miniconda2/bin/conda"
_CONDA_ROOT="/Users/sherbein/opt/miniconda2"
_conda_set_vars() {
# set _CONDA_SHELL_FLAVOR
if [ -n "${BASH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=bash
elif [ -n "${ZSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=zsh
elif [ -n "${KSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=ksh
```
And you can still reproduce the error with `source activate py3`?
```
→ source activate py3
Traceback (most recent call last):
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/gateways/logging.py", line 64, in emit
msg = self.format(record)
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 734, in format
return fmt.format(record)
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 465, in format
record.message = record.getMessage()
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 329, in getMessage
msg = msg % self.args
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/__init__.py", line 43, in __repr__
return '%s: %s' % (self.__class__.__name__, text_type(self))
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/__init__.py", line 47, in __str__
return text_type(self.message % self._kwargs)
ValueError: unsupported format character '{' (0x7b) at index 447
Logged from file exceptions.py, line 724
→ eval $(conda shell.posix activate py3)
→ which python
/Users/sherbein/opt/miniconda2/envs/py3/bin/python
```
What is
`which activate`
and
`cat $(which activate)`
?
```
→ which activate
/Users/sherbein/opt/miniconda2/bin/activate
→ cat $(which activate)
#!/bin/sh
_CONDA_ROOT="/Users/sherbein/opt/miniconda2"
#!/bin/sh
_conda_set_vars() {
# set _CONDA_SHELL_FLAVOR
if [ -n "${BASH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=bash
elif [ -n "${ZSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=zsh
elif [ -n "${KSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=ksh
elif [ -n "${POSH_VERSION:+x}" ]; then
_CONDA_SHELL_FLAVOR=posh
else
# https://unix.stackexchange.com/a/120138/92065
local _q="$(ps -p$$ -o cmd="",comm="",fname="" 2>/dev/null | sed 's/^-//' | grep -oE '\w+' | head -n1)"
if [ "$_q" = dash ]; then
_CONDA_SHELL_FLAVOR=dash
else
(>&2 echo "Unrecognized shell.")
return 1
fi
fi
# https://unix.stackexchange.com/questions/4650/determining-path-to-sourced-shell-script/
local script_dir
case "$_CONDA_SHELL_FLAVOR" in
bash) script_dir="$(dirname "${BASH_SOURCE[0]}")";;
zsh) script_dir="$(dirname "${(%):-%x}")";; # http://stackoverflow.com/a/28336473/2127762
dash) x=$(lsof -p $$ -Fn0 | tail -1); script_dir="$(dirname "${x#n}")";;
*) script_dir="$(cd "$(dirname "$_")" && echo "$PWD")";;
esac
if [ -z "${_CONDA_ROOT+x}" ]; then
_CONDA_ROOT="$(dirname "$script_dir")"
fi
}
_conda_script_is_sourced() {
# http://stackoverflow.com/a/28776166/2127762
sourced=0
if [ -n "${ZSH_EVAL_CONTEXT:+x}" ]; then
case $ZSH_EVAL_CONTEXT in *:file) sourced=1;; esac
elif [ -n "${KSH_VERSION:+x}" ]; then
[ "$(cd $(dirname -- $0) && pwd -P)/$(basename -- $0)" != "$(cd $(dirname -- ${.sh.file}) && pwd -P)/$(basename -- ${.sh.file})" ] && sourced=1
elif [ -n "${BASH_VERSION:+x}" ]; then
[ "${BASH_SOURCE[0]}" = "$0" ] && sourced=1
else # All other shells: examine $0 for known shell binary filenames
# Detects `sh` and `dash`; add additional shell filenames as needed.
case ${0##*/} in sh|dash) sourced=0;; *) sourced=1;; esac
fi
return $sourced
}
if ! _conda_script_is_sourced; then
(
>&2 echo "Error: activate must be sourced. Run 'source activate envname'"
>&2 echo "instead of 'activate envname'."
)
exit 1
fi
_conda_set_vars
. "$_CONDA_ROOT/etc/profile.d/conda.sh" || return $?
_conda_activate "$@"
```
I have still no clue..
What does
```
(set -x ; source activate py3)
```
output?
Oh. Good call. That might be it. I'm guessing this has to do with my zsh prompt (which I have been excluding from the above posts to hide my IP address/hostname, but is included here)
```
# sherbein at roaming-4-24-60.host.udel.edu in ~ [17:01:58]
→ (set -x ; source activate py3)
+-zsh:1> source activate py3
+/Users/sherbein/opt/miniconda2/bin/activate:2> _CONDA_ROOT=/Users/sherbein/opt/miniconda2
+/Users/sherbein/opt/miniconda2/bin/activate:57> _conda_script_is_sourced
+_conda_script_is_sourced:2> sourced=0
+_conda_script_is_sourced:3> [ -n x ']'
+_conda_script_is_sourced:4> case toplevel:file:shfunc (*:file)
+_conda_script_is_sourced:13> return 0
+/Users/sherbein/opt/miniconda2/bin/activate:65> _conda_set_vars
+_conda_set_vars:2> [ -n '' ']'
+_conda_set_vars:4> [ -n x ']'
+_conda_set_vars:5> _CONDA_SHELL_FLAVOR=zsh
+_conda_set_vars:22> local script_dir
+_conda_set_vars:23> case zsh (bash)
+_conda_set_vars:23> case zsh (zsh)
+_conda_set_vars:25> script_dir=+_conda_set_vars:25> dirname /Users/sherbein/opt/miniconda2/bin/activate
+_conda_set_vars:25> script_dir=/Users/sherbein/opt/miniconda2/bin
+_conda_set_vars:30> [ -z x ']'
+/Users/sherbein/opt/miniconda2/bin/activate:66> . /Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh
+/Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh:1> _CONDA_EXE=/Users/sherbein/opt/miniconda2/bin/conda
+/Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh:2> _CONDA_ROOT=/Users/sherbein/opt/miniconda2
+/Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh:105> _conda_set_vars
+_conda_set_vars:2> [ -n '' ']'
+_conda_set_vars:4> [ -n x ']'
+_conda_set_vars:5> _CONDA_SHELL_FLAVOR=zsh
+_conda_set_vars:15> [ -z x ']'
+_conda_set_vars:29> [ -z x ']'
+/Users/sherbein/opt/miniconda2/etc/profile.d/conda.sh:107> [ -z x ']'
+/Users/sherbein/opt/miniconda2/bin/activate:67> _conda_activate py3
+_conda_activate:1> [ -n '' ']'
+_conda_activate:8> local ask_conda
+_conda_activate:9> ask_conda=+_conda_activate:9> PS1=$'\n%{\C-[[1m\C-[[34m%}#%{\C-[[00m%} %{\C-[[36m%}%n %{\C-[[37m%}at %{\C-[[32m%}roaming-4-24-60.host.udel.edu %{\C-[[37m%}in %{\C-[[1m\C-[[33m%}${PWD/#$HOME/~}%{\C-[[00m%}$(ys_hg_prompt_info)$(git_prompt_info) %{\C-[[37m%}[%*]\n%{\C-[[1m\C-[[31m%}→ %{\C-[[00m%}' /Users/sherbein/opt/miniconda2/bin/conda shell.posix activate py3
Traceback (most recent call last):
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/gateways/logging.py", line 64, in emit
msg = self.format(record)
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 734, in format
return fmt.format(record)
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 465, in format
record.message = record.getMessage()
File "/Users/sherbein/opt/miniconda2/lib/python2.7/logging/__init__.py", line 329, in getMessage
msg = msg % self.args
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/__init__.py", line 43, in __repr__
return '%s: %s' % (self.__class__.__name__, text_type(self))
File "/Users/sherbein/opt/miniconda2/lib/python2.7/site-packages/conda/__init__.py", line 47, in __str__
return text_type(self.message % self._kwargs)
ValueError: unsupported format character '{' (0x7b) at index 447
Logged from file exceptions.py, line 724
+_conda_activate:9> ask_conda=''
+_conda_activate:9> return 1
```
```
# sherbein at roaming-4-24-60.host.udel.edu in ~ [17:04:06]
→ export PS1="#"
#
#source activate py3
(py3) #
```
Running `conda config --set changeps1 False` fixed this problem for me. Thanks @mbargull for helping me track this down! I know how frustrating remote troubleshooting can be. I appreciate your time and effort on this!
You're welcome. The funny thing is that the first things I tried was a `PS1='%}'` and `PS1='→'` 🙄 . The minimal working reproducer is the concatenation `PS1='%}→` 😆.
So the actual error is just
```
$ PS1=→
→source activate
EncodingError: A unicode encoding or decoding error has occurred.
Python 2 is the interpreter under which conda is running in your base environment.
Replacing your base environment with one having Python 3 may help resolve this issue.
If you still have a need for Python 2 environments, consider using 'conda create'
and 'conda activate'. For example:
$ conda create -n py2 python=2
$ conda activate py2
Error details: UnicodeDecodeError('ascii', '\xe2\x86\x92', 0, 1, 'ordinal not in range(128)')
```
but that gets masked by another bug that happens during error reporting: https://github.com/conda/conda/issues/6759.
My general advice would be to always use Miniconda3 and use Python 2 in a separate environment, i.e.,
```
conda create -n py2 python=2
```
or if you use `anaconda` then
```
conda create -n anaconda2 python=2 anaconda
```
. That way, `conda` itself will always use Python 3 and doesn't run into those Unicode decoding errors.
Many thx! @mbargull fixed!
From https://github.com/conda/conda/issues/6753#issuecomment-359110810, the root-cause stack trace here is
```
Traceback (most recent call last):
File "/Users/rthomas/bug/env/lib/python2.7/site-packages/conda/cli/main.py", line 111, in main
return activator_main()
File "/Users/rthomas/bug/env/lib/python2.7/site-packages/conda/activate.py", line 557, in main
print(activator.execute(), end='')
File "/Users/rthomas/bug/env/lib/python2.7/site-packages/conda/activate.py", line 149, in execute
return getattr(self, self.command)()
File "/Users/rthomas/bug/env/lib/python2.7/site-packages/conda/activate.py", line 135, in activate
return self._finalize(self._yield_commands(self.build_activate(self.env_name_or_prefix)),
File "/Users/rthomas/bug/env/lib/python2.7/site-packages/conda/activate.py", line 267, in build_activate
self._update_prompt(set_vars, conda_prompt_modifier)
File "/Users/rthomas/bug/env/lib/python2.7/site-packages/conda/activate.py", line 456, in _update_prompt
ps1 = ps1.replace("'", "'\"'\"'")
EncodingError: <unprintable EncodingError object>
``` | 2018-01-24T12:11:29 | -1.0 |
conda/conda | 6,782 | conda__conda-6782 | [
"5884",
"5884"
] | 33731c68637acb2b5cac46f122b50add46c10490 | diff --git a/conda/base/context.py b/conda/base/context.py
--- a/conda/base/context.py
+++ b/conda/base/context.py
@@ -95,9 +95,10 @@ def default_python_validation(value):
def ssl_verify_validation(value):
if isinstance(value, string_types):
- if not isfile(value):
- return ("ssl_verify value '%s' must be a boolean or a path to a "
- "certificate bundle." % value)
+ if not isfile(value) and not isdir(value):
+ return ("ssl_verify value '%s' must be a boolean, a path to a "
+ "certificate bundle file, or a path to a directory containing "
+ "certificates of trusted CAs." % value)
return True
@@ -147,7 +148,7 @@ class Context(Configuration):
# remote connection details
ssl_verify = PrimitiveParameter(True, element_type=string_types + (bool,),
- aliases=('insecure', 'verify_ssl',),
+ aliases=('verify_ssl',),
validation=ssl_verify_validation)
client_ssl_cert = PrimitiveParameter(None, aliases=('client_cert',),
element_type=string_types + (NoneType,))
diff --git a/conda/cli/conda_argparse.py b/conda/cli/conda_argparse.py
--- a/conda/cli/conda_argparse.py
+++ b/conda/cli/conda_argparse.py
@@ -1265,6 +1265,7 @@ def add_parser_insecure(p):
p.add_argument(
"-k", "--insecure",
action="store_false",
+ dest="ssl_verify",
default=NULL,
help="Allow conda to perform \"insecure\" SSL connections and transfers. "
"Equivalent to setting 'ssl_verify' to 'false'."
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -869,7 +869,7 @@ def test_conda_config_validate(self):
run_command(Commands.CONFIG, prefix, "--validate")
assert len(exc.value.errors) == 2
- assert "must be a boolean or a path to a certificate bundle" in str(exc.value)
+ assert "must be a boolean, a path to a certificate bundle file, or a path to a directory containing certificates of trusted CAs" in str(exc.value)
assert "default_python value 'anaconda' not of the form '[23].[0-9]'" in str(exc.value)
finally:
reset_context()
| ssl_verify functionality doesn't match description
Was experimenting to see if I could use a cert directory on Windows 7:
```
> conda config --set ssl_verify C:\Users\HarmerS\certs
```
However it seems like the description of `ssl_verify` doesn't agree with the implementation.
```
> conda config --describe
[...]
ssl_verify (basestring, bool)
default: true
aliases: insecure, verify_ssl
Conda verifies SSL certificates for HTTPS requests, just like a web
browser. By default, SSL verification is enabled, and conda operations
will fail if a required url's certificate cannot be verified. Setting
ssl_verify to False disables certification verificaiton. The value for
ssl_verify can also be (1) a path to a CA bundle file, or (2) a path
to a directory containing certificates of trusted CA.
[...]
> conda install -c conda-forge pydicom
CustomValidationError: Parameter ssl_verify = 'C:\\Users\\HarmerS\\certs' declared in <<merged>> is invalid.
ssl_verify value 'C:\Users\HarmerS\certs' must be a boolean or a path to a certificate bundle.
```
```
> conda --version
conda 4.3.25
```
Via Miniconda 4.3.21 [installer](https://repo.continuum.io/miniconda/Miniconda2-latest-Windows-x86.exe) Python 2.7 32-bit + `conda update conda` on Windows 7 64-bit.
I would prefer to have the feature of using a cert directory (much easier to manage) so I raised the issue here rather than under conda-docs.
ssl_verify functionality doesn't match description
Was experimenting to see if I could use a cert directory on Windows 7:
```
> conda config --set ssl_verify C:\Users\HarmerS\certs
```
However it seems like the description of `ssl_verify` doesn't agree with the implementation.
```
> conda config --describe
[...]
ssl_verify (basestring, bool)
default: true
aliases: insecure, verify_ssl
Conda verifies SSL certificates for HTTPS requests, just like a web
browser. By default, SSL verification is enabled, and conda operations
will fail if a required url's certificate cannot be verified. Setting
ssl_verify to False disables certification verificaiton. The value for
ssl_verify can also be (1) a path to a CA bundle file, or (2) a path
to a directory containing certificates of trusted CA.
[...]
> conda install -c conda-forge pydicom
CustomValidationError: Parameter ssl_verify = 'C:\\Users\\HarmerS\\certs' declared in <<merged>> is invalid.
ssl_verify value 'C:\Users\HarmerS\certs' must be a boolean or a path to a certificate bundle.
```
```
> conda --version
conda 4.3.25
```
Via Miniconda 4.3.21 [installer](https://repo.continuum.io/miniconda/Miniconda2-latest-Windows-x86.exe) Python 2.7 32-bit + `conda update conda` on Windows 7 64-bit.
I would prefer to have the feature of using a cert directory (much easier to manage) so I raised the issue here rather than under conda-docs.
| Conda just uses requests under the hood. Is what you're proposing compatible with http://docs.python-requests.org/en/master/user/advanced/#ssl-cert-verification ? If so, we can absolutely update all associated docs.
For reference, the documentation shown by `conda config --describe` is at https://github.com/conda/conda/blob/4.3.x/conda/base/context.py#L686
Conda just uses requests under the hood. Is what you're proposing compatible with http://docs.python-requests.org/en/master/user/advanced/#ssl-cert-verification ? If so, we can absolutely update all associated docs.
For reference, the documentation shown by `conda config --describe` is at https://github.com/conda/conda/blob/4.3.x/conda/base/context.py#L686 | 2018-01-24T18:33:16 | -1.0 |
conda/conda | 6,828 | conda__conda-6828 | [
"6796"
] | 158599161fcfcce024bf8257c7278a10fb3085e2 | diff --git a/conda/activate.py b/conda/activate.py
--- a/conda/activate.py
+++ b/conda/activate.py
@@ -361,11 +361,16 @@ def build_reactivate(self):
'activate_scripts': (),
}
conda_default_env = self.environ.get('CONDA_DEFAULT_ENV', self._default_env(conda_prefix))
+ new_path = self.pathsep_join(self._replace_prefix_in_path(conda_prefix, conda_prefix))
+ set_vars = {}
+ conda_prompt_modifier = self._prompt_modifier(conda_default_env)
+ self._update_prompt(set_vars, conda_prompt_modifier)
# environment variables are set only to aid transition from conda 4.3 to conda 4.4
return {
'unset_vars': (),
- 'set_vars': {},
+ 'set_vars': set_vars,
'export_vars': {
+ 'PATH': new_path,
'CONDA_SHLVL': conda_shlvl,
'CONDA_PROMPT_MODIFIER': self._prompt_modifier(conda_default_env),
},
| diff --git a/tests/test_activate.py b/tests/test_activate.py
--- a/tests/test_activate.py
+++ b/tests/test_activate.py
@@ -295,14 +295,23 @@ def test_activate_same_environment(self):
with env_var('CONDA_SHLVL', '1'):
with env_var('CONDA_PREFIX', old_prefix):
activator = Activator('posix')
+
builder = activator.build_activate(td)
+ new_path_parts = activator._replace_prefix_in_path(old_prefix, old_prefix)
+ conda_prompt_modifier = "(%s) " % old_prefix
+ ps1 = conda_prompt_modifier + os.environ.get('PS1', '')
+
+ set_vars = {
+ 'PS1': ps1,
+ }
export_vars = {
+ 'PATH': activator.pathsep_join(new_path_parts),
'CONDA_PROMPT_MODIFIER': "(%s) " % td,
'CONDA_SHLVL': 1,
}
assert builder['unset_vars'] == ()
- assert builder['set_vars'] == {}
+ assert builder['set_vars'] == set_vars
assert builder['export_vars'] == export_vars
assert builder['activate_scripts'] == (activator.path_conversion(activate_d_1),)
assert builder['deactivate_scripts'] == (activator.path_conversion(deactivate_d_1),)
@@ -478,15 +487,20 @@ def test_posix_basic(self):
assert rc == 0
reactivate_data = c.stdout
+ new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
. "%(deactivate1)s"
+ PS1='%(ps1)s'
export CONDA_PROMPT_MODIFIER='(%(native_prefix)s) '
export CONDA_SHLVL='1'
+ export PATH='%(new_path)s'
. "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.sh')),
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.sh')),
'native_prefix': self.prefix,
+ 'new_path': activator.pathsep_join(new_path_parts),
+ 'ps1': '(%s) ' % self.prefix + os.environ.get('PS1', ''),
}
with captured() as c:
@@ -560,15 +574,18 @@ def test_cmd_exe_basic(self):
reactivate_data = fh.read()
rm_rf(reactivate_result)
+ new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
@CALL "%(deactivate1)s"
@SET "CONDA_PROMPT_MODIFIER=(%(native_prefix)s) "
@SET "CONDA_SHLVL=1"
+ @SET "PATH=%(new_path)s"
@CALL "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.bat')),
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.bat')),
'native_prefix': self.prefix,
+ 'new_path': activator.pathsep_join(new_path_parts),
}
with captured() as c:
@@ -635,12 +652,17 @@ def test_csh_basic(self):
assert rc == 0
reactivate_data = c.stdout
+ new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
source "%(deactivate1)s";
+ set prompt='%(prompt)s';
setenv CONDA_PROMPT_MODIFIER "(%(native_prefix)s) ";
setenv CONDA_SHLVL "1";
+ setenv PATH "%(new_path)s";
source "%(activate1)s";
""") % {
+ 'prompt': '(%s) ' % self.prefix + os.environ.get('prompt', ''),
+ 'new_path': activator.pathsep_join(new_path_parts),
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.csh')),
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.csh')),
'native_prefix': self.prefix,
@@ -714,15 +736,18 @@ def test_xonsh_basic(self):
reactivate_data = fh.read()
rm_rf(reactivate_result)
+ new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
source "%(deactivate1)s"
$CONDA_PROMPT_MODIFIER = '(%(native_prefix)s) '
$CONDA_SHLVL = '1'
+ $PATH = '%(new_path)s'
source "%(activate1)s"
""") % {
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.xsh')),
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.xsh')),
'native_prefix': self.prefix,
+ 'new_path': activator.pathsep_join(new_path_parts),
}
with captured() as c:
@@ -787,12 +812,15 @@ def test_fish_basic(self):
assert rc == 0
reactivate_data = c.stdout
+ new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
source "%(deactivate1)s";
set -gx CONDA_PROMPT_MODIFIER "(%(native_prefix)s) ";
set -gx CONDA_SHLVL "1";
+ set -gx PATH "%(new_path)s";
source "%(activate1)s";
""") % {
+ 'new_path': activator.pathsep_join(new_path_parts),
'activate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.fish')),
'deactivate1': activator.path_conversion(join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.fish')),
'native_prefix': self.prefix,
@@ -857,15 +885,18 @@ def test_powershell_basic(self):
assert rc == 0
reactivate_data = c.stdout
+ new_path_parts = activator._replace_prefix_in_path(self.prefix, self.prefix)
assert reactivate_data == dals("""
. "%(deactivate1)s"
$env:CONDA_PROMPT_MODIFIER = "(%(prefix)s) "
$env:CONDA_SHLVL = "1"
+ $env:PATH = "%(new_path)s"
. "%(activate1)s"
""") % {
'activate1': join(self.prefix, 'etc', 'conda', 'activate.d', 'activate1.ps1'),
'deactivate1': join(self.prefix, 'etc', 'conda', 'deactivate.d', 'deactivate1.ps1'),
'prefix': self.prefix,
+ 'new_path': activator.pathsep_join(new_path_parts),
}
with captured() as c:
| Base environment PATH is not set when transitioning to tmux
<!--
Hi!
This is an issue tracker for conda -- the package manager. File feature requests
for conda here, as well as bug reports about something conda has messed up.
If your issue is a bug report or feature request for:
* a specific conda package from Anaconda ('defaults' channel):
==> file at https://github.com/ContinuumIO/anaconda-issues
* a specific conda package from conda-forge:
==> file at the corresponding feedstock under https://github.com/conda-forge
* repo.continuum.io access and service:
==> file at https://github.com/ContinuumIO/anaconda-issues
* anaconda.org access and service:
==> file at https://anaconda.org/contact/report
* commands under 'conda build':
==> file at https://github.com/conda/conda-build
* commands under 'conda env':
==> please file it here!
* all other conda commands that start with 'conda':
==> please file it here!
-->
**I'm submitting a...**
- [x] bug report
- [ ] feature request
## Current Behavior
<!-- What actually happens?
If you want to include console output, please use "Steps to Reproduce" below. -->
After a clean installation of anaconda and an update to conda 4.4.7 everything seemed to be working nicely. On restarting, I have found that the base environment is not activated correctly. The PATH is not being set, so only the system python installation is on the path. Activating any other environment sets the PATH correctly, but the base environment does not.
I'm running Ubuntu 16.04.
### Steps to Reproduce
<!-- If the current behavior is a bug, please provide specific, minimal steps to independently reproduce.
Include the exact conda commands that reproduce the issue and their output between the ticks below. -->
```
conda activate base
which python
> /usr/bin/python
conda activate other-environment
which python
> /home/me/anaconda3/envs/other-environment/bin/python
```
## Expected Behavior
<!-- What do you think should happen? -->
## Environment Information
<details open><summary><code>`conda info`</code></summary><p>
<!-- between the ticks below, paste the output of 'conda info' -->
'conda info':
```
active environment : service.thickener.pde
active env location : /home/me/anaconda3/envs/service.thickener.pde
shell level : 2
user config file : /home/me/.condarc
populated config files :
conda version : 4.4.7
conda-build version : 3.0.27
python version : 3.6.2.final.0
base environment : /home/me/anaconda3 (writable)
channel URLs : https://repo.continuum.io/pkgs/main/linux-64
https://repo.continuum.io/pkgs/main/noarch
https://repo.continuum.io/pkgs/free/linux-64
https://repo.continuum.io/pkgs/free/noarch
https://repo.continuum.io/pkgs/r/linux-64
https://repo.continuum.io/pkgs/r/noarch
https://repo.continuum.io/pkgs/pro/linux-64
https://repo.continuum.io/pkgs/pro/noarch
package cache : /home/me/anaconda3/pkgs
/home/me/.conda/pkgs
envs directories : /home/me/anaconda3/envs
/home/me/.conda/envs
platform : linux-64
user-agent : conda/4.4.7 requests/2.18.4 CPython/3.6.2 Linux/4.4.0-112-generic ubuntu/16.04 glibc/2.23
UID:GID : 1001:1001
netrc file : None
offline mode : False
```
</p></details>
| For now I've just cloned the base environment and put an `activate` command in my shell rc file
What shell are you using, and what version is it?
Can you give me the output of `echo $PATH` after `conda activate base` and `conda activate other-environment`?
zsh 5.1.1
I have just noticed that the issue only shows up within tmux — a "bare" terminal works ok but in tmux the base environment (only) doesn't activate properly.
this is `PATH` after `conda activate base` in tmux:
```
/home/me/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/home/me/.config/nvim/dein/repos/github.com/junegunn/fzf/bin:/home/me/texlive/2016/bin/x86_64-linux:/home/me/work/tools/bin:/usr/local/cuda/bin:/home/me/.hugh/vimfiles/dein/repos/github.com/junegunn/fzf/bin
```
and after `conda activate other-environment`:
```
/home/me/anaconda3/envs/other-environment/bin:/home/me/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/home/me/.config/nvim/dein/repos/github.com/junegunn/fzf/bin:/home/me/texlive/2016/bin/x86_64-linux:/home/me/work/tools/bin:/usr/local/cuda/bin:/home/me/.hugh/vimfiles/dein/repos/github.com/junegunn/fzf/bin
```
and after `conda activate base` outside tmux (which works as it should):
```
/home/me/anaconda3/bin:/home/me/anaconda3/envs/hugh/bin:/home/me/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/home/me/.config/nvim/dein/repos/github.com/junegunn/fzf/bin:/home/me/texlive/2016/bin/x86_64-linux:/home/me/work/tools/bin:/usr/local/cuda/bin:/home/me/.hugh/vimfiles/dein/repos/github.com/junegunn/fzf/bin
```
As part of `conda activate`, `source activate`, etc, we need to run `rehash` or `hash -r` to reset the shell's cache for stuff found on PATH. Is that perhaps what's not working correctly in tmux for some reason?
Scratch that. PATH is clearly not correct in your example above for tmux.
Can you do the following and see what we get...
1. open clean, new shell under tmux
2. `echo $PATH`
3. `type -a conda`
4. `conda shell.posix activate base` # won't actually activate; just show you what should be eval'd by the shell
5. `set -x`
6. `conda activate base`
This is from a new shell, with `base` activated
`echo $PATH`:
```
/home/me/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/home/me/.config/nvim/dein/repos/github.com/junegunn/fzf/bin:/home/me/texlive/2016/bin/x86_64-linux:/home/me/work/tools/bin:/usr/local/cuda/bin:/home/me/.hugh/vimfiles/dein/repos/github.com/junegunn/fzf/bin
```
`type -a conda`:
```
conda is a shell function from /home/me/anaconda3/etc/profile.d/conda.sh
```
`conda shell.posix activate base`:
```
export CONDA_PROMPT_MODIFIER='(base) '
export CONDA_SHLVL='1'
```
`set -x`
```
+omz_termsupport_preexec:1> emulate -L zsh
+omz_termsupport_preexec:2> setopt extended_glob
+omz_termsupport_preexec:4> [[ '' == true ]]
+omz_termsupport_preexec:9> local CMD=set
+omz_termsupport_preexec:10> local LINE='set -x'
+omz_termsupport_preexec:12> title '$CMD' '%100>...>$LINE%<<'
+title:1> emulate -L zsh
+title:2> setopt prompt_subst
+title:4> [[ '' == *term* ]]
+title:8> : '%100>...>$LINE%<<'
+title:10> case screen-256color (cygwin | xterm* | putty* | rxvt* | ansi)
+title:10> case screen-256color (screen*)
+title:16> print -Pn '\ek\$CMD\e\'
+prompt_geometry_set_cmd_title:1> prompt_geometry_print_title 'set -x @ %m'
+prompt_geometry_print_title:1> print -n '\e]0;'
+-zsh:15> set -x
+omz_termsupport_precmd:1> emulate -L zsh
+omz_termsupport_precmd:3> [[ '' == true ]]
+omz_termsupport_precmd:7> title '%15<..<%~%<<' '%n@%m: %~'
+title:1> emulate -L zsh
+title:2> setopt prompt_subst
+title:4> [[ '' == *term* ]]
+title:8> : '%n@%m: %~'
+title:10> case screen-256color (cygwin | xterm* | putty* | rxvt* | ansi)
+title:10> case screen-256color (screen*)
+title:16> print -Pn '\ek%15\<..\<%~%\<\<\e\'
+prompt_geometry_set_title:1> prompt_geometry_print_title '%~'
+prompt_geometry_print_title:1> print -n '\e]0;'
+prompt_geometry_render:1> [ 0 -eq 0 ']'
+prompt_geometry_render:2> PROMPT_SYMBOL=▲
+prompt_geometry_render:7> PROMPT='
%F{blue}%3~%f
%1{%(?.%F{white}▲%f.%F{magenta}△%f)%} '
+prompt_geometry_render:11> PROMPT2=' ◇ '
+prompt_geometry_render:12> RPROMPT=+prompt_geometry_render:12> echotc UP 1
+prompt_geometry_render:12> RPROMPT=+prompt_geometry_render:12> prompt_hugh_conda_env_info
+prompt_hugh_conda_env_info:1> env_name=base
+prompt_hugh_conda_env_info:2> [ base '!=' root ']'
+prompt_hugh_conda_env_info:2> [ base '!=' '' ']'
+prompt_hugh_conda_env_info:3> git rev-parse --git-dir
+prompt_hugh_conda_env_info:6> prompt_geometry_colorize 242 base
+prompt_geometry_colorize:1> echo '%F{242}base%f'
+prompt_hugh_conda_env_info:6> echo '%F{242}base%f'
+prompt_geometry_render:12> RPROMPT=+prompt_geometry_render:12>
prompt_geometry_git_info
+prompt_geometry_git_info:1> git rev-parse --git-dir
+prompt_geometry_render:12> RPROMPT=+pr%}%F{242}base%f%f%{r:12> echotc DO 1
+prompt_geometry_render:12> RPROMPT='%{ %}'
```
`conda activate base`:
```
+omz_termsupport_preexec:1> emulate -L zsh
+omz_termsupport_preexec:2> setopt extended_glob
+omz_termsupport_preexec:4> [[ '' == true ]]
+omz_termsupport_preexec:9> local CMD=conda
+omz_termsupport_preexec:10> local LINE='conda activate base'
+omz_termsupport_preexec:12> title '$CMD' '%100>...>$LINE%<<'
+title:1> emulate -L zsh
+title:2> setopt prompt_subst
+title:4> [[ '' == *term* ]]
+title:8> : '%100>...>$LINE%<<'
+title:10> case screen-256color (cygwin | xterm* | putty* | rxvt* | ansi)
+title:10> case screen-256color (screen*)
+title:16> print -Pn '\ek\$CMD\e\'
+prompt_geometry_set_cmd_title:1> prompt_geometry_print_title 'conda activate base @ %m'
+prompt_geometry_print_title:1> print -n '\e]0;'
+-zsh:16> conda activate base
+conda:1> [ 2 -ge 1 ']'
+conda:2> local cmd=activate
+conda:3> shift
+conda:7> case activate (activate)
+conda:9> _conda_activate base
+_conda_activate:1> [ -n '' ']'
+_conda_activate:8> local ask_conda
+_conda_activate:9> ask_conda=+_conda_activate:9> PS1='
%F{blue}%3~%f
%1{%(?.%F{white}▲%f.%F{magenta}△%f)%} ' /home/me/anaconda3/bin/conda
shell.posix activate base
+_conda_activate:9> ask_conda='export CONDA_PROMPT_MODIFIER='\''(base) '\''
export CONDA_SHLVL='\''1'\'
+_conda_activate:10> eval 'export CONDA_PROMPT_MODIFIER='\''(base) '\''
export CONDA_SHLVL='\''1'\'
+(eval):1> export CONDA_PROMPT_MODIFIER='(base) '
+(eval):2> export CONDA_SHLVL=1
+_conda_activate:12> _conda_hashr
+_conda_hashr:1> case zsh (zsh)
+_conda_hashr:2> rehash
+omz_termsupport_precmd:1> emulate -L zsh
+omz_termsupport_precmd:3> [[ '' == true ]]
+omz_termsupport_precmd:7> title '%15<..<%~%<<' '%n@%m: %~'
+title:1> emulate -L zsh
+title:2> setopt prompt_subst
+title:4> [[ '' == *term* ]]
+title:8> : '%n@%m: %~'
+title:10> case screen-256color (cygwin | xterm* | putty* | rxvt* | ansi)
+title:10> case screen-256color (screen*)
+title:16> print -Pn '\ek%15\<..\<%~%\<\<\e\'
+prompt_geometry_set_title:1> prompt_geometry_print_title '%~'
+prompt_geometry_print_title:1> print -n '\e]0;'
+prompt_geometry_render:1> [ 0 -eq 0 ']'
+prompt_geometry_render:2> PROMPT_SYMBOL=▲
+prompt_geometry_render:7> PROMPT='
%F{blue}%3~%f
%1{%(?.%F{white}▲%f.%F{magenta}△%f)%} '
+prompt_geometry_render:11> PROMPT2=' ◇ '
+prompt_geometry_render:12> RPROMPT=+prompt_geometry_render:12> echotc UP
1
+prompt_geometry_render:12> RPROMPT=+prompt_geometry_render:12>
prompt_hugh_conda_env_info
+prompt_hugh_conda_env_info:1> env_name=base
+prompt_hugh_conda_env_info:2> [ base '!=' root ']'
+prompt_hugh_conda_env_info:2> [ base '!=' '' ']'
+prompt_hugh_conda_env_info:3> git rev-parse --git-dir
+prompt_hugh_conda_env_info:6> prompt_geometry_colorize 242 base
+prompt_geometry_colorize:1> echo '%F{242}base%f'
+prompt_hugh_conda_env_info:6> echo '%F{242}base%f'
+prompt_geometry_render:12> RPROMPT=+prompt_geometry_render:12>
prompt_geometry_git_info
+prompt_geometry_git_info:1> git rev-parse --git-dir
+prompt_geometry_render:12> RPROMPT=+pr%}%F{242}base%f%f%{r:12> echotc DO
1
+prompt_geometry_render:12> RPROMPT='%{ %}'
```
there's lots of zsh prompt stuff in there, but it just looks like there's no path setting command at all for some reason...
Also, and this is a bit odd, tmux seems to activate the base environment even though I removed the `conda activate base` command from my zshrc
A bare shell doesn't activate anything, but as soon as I load tmux I get the `base` prompt showing up
That last comment seems to be part of the problem: if I load tmux, and _deactivate_ the `base` environment (so there's no environment active), then `conda shell.posix activate base` looks good, and `conda activate base` alters the path as expected
So for some reason tmux is improperly activating the base environment when it starts
Ok problem starts with
`conda shell.posix activate base`:
```
export CONDA_PROMPT_MODIFIER='(base) '
export CONDA_SHLVL='1'
```
So, one more request for information...
1. open clean, new shell under tmux
2. `env | sort`
3. `conda shell.posix activate base`
As I said, this is starting tmux without any `conda activate` anything in my zshrc.
`env | sort`:
```BROWSER=google-chrome
CLUTTER_IM_MODULE=xim
CONDA_DEFAULT_ENV=base
CONDA_PREFIX=/home/me/anaconda3
CONDA_PROMPT_MODIFIER=(base)
CONDA_PYTHON_EXE=/home/me/anaconda3/bin/python
CONDA_SHLVL=1
CPATH=/home/me/cuda/include:/home/me/cuda/include:
DBUS_SESSION_BUS_ADDRESS=unix:abstract=/tmp/dbus-hxf9qZRlyl
DEFAULTS_PATH=/usr/share/gconf/gnome.default.path
DESKTOP_MODE=1
DESKTOP_SESSION=gnome
DISPLAY=:0
EDITOR=nvim
EVENT_NOEPOLL=1
FZF_ALT_C_COMMAND=find -L . \( -path '*/\.*' -o -fstype 'dev' -o -fstype 'proc' \) -prune -o -type d -name -print 2> /dev/null | sed 1d | cut -b3-
FZF_CTRL_T_COMMAND=find -L . \( -fstype 'dev' -o -fstype 'proc' \) -prune -o -type f -print -o -type d -print -o -type l -print 2> /dev/null | sed 1d | cut -b3-
FZF_DEFAULT_COMMAND=ag -l --hidden -g ""
GDM_LANG=en_GB
GDMSESSION=gnome
GNOME_DESKTOP_SESSION_ID=this-is-deprecated
GNOME_KEYRING_CONTROL=
GNOME_KEYRING_PID=
GPG_AGENT_INFO=/home/me/.gnupg/S.gpg-agent:0:1
GTK2_MODULES=overlay-scrollbar
GTK_IM_MODULE=ibus
GTK_MODULES=gail:atk-bridge
HOME=/home/me
IM_CONFIG_PHASE=1
INSTANCE=GNOME
JOB=gnome-session
KEYTIMEOUT=1
LANG=en_GB.UTF-8
LANGUAGE=en_GB:en
LC_CTYPE=en_GB.UTF-8
LD_LIBRARY_PATH=/usr/local/cuda/lib64:/home/me/cuda/lib64:/usr/local/cuda/lib64:/home/me/cuda/lib64:
LESS=-R
LIBRARY_PATH=/usr/local/cuda/lib64:/home/me/cuda/lib64:/usr/local/cuda/lib64:/home/me/cuda/lib64:
LIBVIRT_DEFAULT_URI=qemu:///system
LOGNAME=me
LSCOLORS=Gxfxcxdxbxegedabagacad
LS_COLORS=rs=0:di=01;34:ln=01;36:mh=00:pi=40;33:so=01;35:do=01;35:bd=40;33;01:cd=40;33;01:or=40;31;01:mi=00:su=37;41:sg=30;43:ca=30;41:tw=30;42:ow=34;42:st=37;44:ex=01;32:*.tar=01;31:*.tgz=01;31:*.arc=01;31:*.arj=01;31:*.taz=01;31:*.lha=01;31:*.lz4=01;31:*.lzh=01;31:*.lzma=01;31:*.tlz=01;31:*.txz=01;31:*.tzo=01;31:*.t7z=01;31:*.zip=01;31:*.z=01;31:*.Z=01;31:*.dz=01;31:*.gz=01;31:*.lrz=01;31:*.lz=01;31:*.lzo=01;31:*.xz=01;31:*.bz2=01;31:*.bz=01;31:*.tbz=01;31:*.tbz2=01;31:*.tz=01;31:*.deb=01;31:*.rpm=01;31:*.jar=01;31:*.war=01;31:*.ear=01;31:*.sar=01;31:*.rar=01;31:*.alz=01;31:*.ace=01;31:*.zoo=01;31:*.cpio=01;31:*.7z=01;31:*.rz=01;31:*.cab=01;31:*.jpg=01;35:*.jpeg=01;35:*.gif=01;35:*.bmp=01;35:*.pbm=01;35:*.pgm=01;35:*.ppm=01;35:*.tga=01;35:*.xbm=01;35:*.xpm=01;35:*.tif=01;35:*.tiff=01;35:*.png=01;35:*.svg=01;35:*.svgz=01;35:*.mng=01;35:*.pcx=01;35:*.mov=01;35:*.mpg=01;35:*.mpeg=01;35:*.m2v=01;35:*.mkv=01;35:*.webm=01;35:*.ogm=01;35:*.mp4=01;35:*.m4v=01;35:*.mp4v=01;35:*.vob=01;35:*.qt=01;35:*.nuv=01;35:*.wmv=01;35:*.asf=01;35:*.rm=01;35:*.rmvb=01;35:*.flc=01;35:*.avi=01;35:*.fli=01;35:*.flv=01;35:*.gl=01;35:*.dl=01;35:*.xcf=01;35:*.xwd=01;35:*.yuv=01;35:*.cgm=01;35:*.emf=01;35:*.ogv=01;35:*.ogx=01;35:*.aac=00;36:*.au=00;36:*.flac=00;36:*.m4a=00;36:*.mid=00;36:*.midi=00;36:*.mka=00;36:*.mp3=00;36:*.mpc=00;36:*.ogg=00;36:*.ra=00;36:*.wav=00;36:*.oga=00;36:*.opus=00;36:*.spx=00;36:*.xspf=00;36:
MANDATORY_PATH=/usr/share/gconf/gnome.mandatory.path
MYZSH=/home/me/.hugh/general
NODE_PATH=/usr/lib/nodejs:/usr/lib/node_modules:/usr/share/javascript
OLDPWD=/home/me
PAGER=less
PAM_KWALLET5_LOGIN=/tmp/kwallet5_me.socket
PATH=/home/me/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/home/me/.config/nvim/dein/repos/github.com/junegunn/fzf/bin:/home/me/texlive/2016/bin/x86_64-linux:/home/me/work/tools/bin:/usr/local/cuda/bin:/home/me/.hugh/vimfiles/dein/repos/github.com/junegunn/fzf/bin
PWD=/home/me
QT4_IM_MODULE=xim
QT_ACCESSIBILITY=1
QT_IM_MODULE=ibus
QT_LINUX_ACCESSIBILITY_ALWAYS_ON=1
QT_QPA_PLATFORMTHEME=appmenu-qt5
SESSIONTYPE=gnome-session
SHELL=/usr/bin/zsh
SHLVL=2
SSH_AUTH_SOCK=/run/user/1001/keyring/ssh
TERM=screen-256color
TMUX_PANE=%30
TMUX=/tmp/tmux-1001/default,16045,7
UPDATE_ZSH_DAYS=7
UPSTART_EVENTS=started starting
UPSTART_INSTANCE=
UPSTART_JOB=gnome-settings-daemon
UPSTART_SESSION=unix:abstract=/com/ubuntu/upstart-session/1001/2610
USER=me
_=/usr/bin/env
VTE_VERSION=4205
WINDOWID=67170133
XAUTHORITY=/home/me/.Xauthority
XDG_CONFIG_DIRS=/etc/xdg/xdg-gnome:/usr/share/upstart/xdg:/etc/xdg
XDG_CURRENT_DESKTOP=GNOME
XDG_DATA_DIRS=/usr/share/gnome:/usr/local/share:/usr/share:/var/lib/snapd/desktop:/var/lib/snapd/desktop
XDG_GREETER_DATA_DIR=/var/lib/lightdm-data/me
XDG_RUNTIME_DIR=/run/user/1001
XDG_SEAT_PATH=/org/freedesktop/DisplayManager/Seat0
XDG_SEAT=seat0
XDG_SESSION_DESKTOP=gnome
XDG_SESSION_ID=c2
XDG_SESSION_PATH=/org/freedesktop/DisplayManager/Session0
XDG_SESSION_TYPE=x11
XDG_VTNR=7
XMODIFIERS=@im=ibus
ZSH=/home/me/.oh-my-zsh
```
`conda shell.posix activate base`:
```
export CONDA_PROMPT_MODIFIER='(base) '
export CONDA_SHLVL='1'
```
Ok so the `CONDA_` environment variables you have there... all of those are indicating that you have your base env activated. Which is the case before you start tmux. After you start tmux, you’re carrying over some of those environment variables, but also doing something to PATH. Probably resetting it to a hardcoded value that doesn’t respect previous state.
I thought that, so I tried starting tmux without any env activated and it stayed the same...
Haha well those `CONDA_` environment variables aren’t going to set themselves ¯\\\_(ツ)\_/¯
Ok, so that last comment was because I had a tmux session left running. I stopped every tmux session, started a fresh shell with base env active, and now when I run tmux I get the problem. It seems that tmux holds the environment variables over, so the new shell isn't "bare" when `conda activate base` is encountered in .zshrc
`env | sort`:
```
BROWSER=google-chrome
CLUTTER_IM_MODULE=xim
CONDA_DEFAULT_ENV=base
CONDA_PREFIX=/home/me/anaconda3
CONDA_PROMPT_MODIFIER=(base)
CONDA_PYTHON_EXE=/home/me/anaconda3/bin/python
CONDA_SHLVL=1
CPATH=/home/me/cuda/include:/home/me/cuda/include:
DBUS_SESSION_BUS_ADDRESS=unix:abstract=/tmp/dbus-zGJ7TsNLxt
DEFAULTS_PATH=/usr/share/gconf/gnome.default.path
DESKTOP_MODE=1
DESKTOP_SESSION=gnome
DISPLAY=:0
EDITOR=nvim
EVENT_NOEPOLL=1
FZF_ALT_C_COMMAND=find -L . \( -path '*/\.*' -o -fstype 'dev' -o -fstype 'proc' \) -prune -o -type d -name -print 2> /dev/null | sed 1d | cut -b3-
FZF_CTRL_T_COMMAND=find -L . \( -fstype 'dev' -o -fstype 'proc' \) -prune -o -type f -print -o -type d -print -o -type l -print 2> /dev/null | sed 1d | cut -b3-
FZF_DEFAULT_COMMAND=ag -l --hidden -g ""
GDM_LANG=en_GB
GDMSESSION=gnome
GNOME_DESKTOP_SESSION_ID=this-is-deprecated
GNOME_KEYRING_CONTROL=
GNOME_KEYRING_PID=
GPG_AGENT_INFO=/home/me/.gnupg/S.gpg-agent:0:1
GTK2_MODULES=overlay-scrollbar
GTK_IM_MODULE=ibus
GTK_MODULES=gail:atk-bridge
HOME=/home/me
IM_CONFIG_PHASE=1
INSTANCE=GNOME
JOB=gnome-session
KEYTIMEOUT=1
LANG=en_GB.UTF-8
LANGUAGE=en_GB:en
LC_CTYPE=en_GB.UTF-8
LD_LIBRARY_PATH=/usr/local/cuda/lib64:/home/me/cuda/lib64:/usr/local/cuda/lib64:/home/me/cuda/lib64:
LESS=-R
LIBRARY_PATH=/usr/local/cuda/lib64:/home/me/cuda/lib64:/usr/local/cuda/lib64:/home/me/cuda/lib64:
LIBVIRT_DEFAULT_URI=qemu:///system
LOGNAME=me
LSCOLORS=Gxfxcxdxbxegedabagacad
LS_COLORS=rs=0:di=01;34:ln=01;36:mh=00:pi=40;33:so=01;35:do=01;35:bd=40;33;01:cd=40;33;01:or=40;31;01:mi=00:su=37;41:sg=30;43:ca=30;41:tw=30;42:ow=34;42:st=37;44:ex=01;32:*.tar=01;31:*.tgz=01;31:*.arc=01;31:*.arj=01;31:*.taz=01;31:*.lha=01;31:*.lz4=01;31:*.lzh=01;31:*.lzma=01;31:*.tlz=01;31:*.txz=01;31:*.tzo=01;31:*.t7z=01;31:*.zip=01;31:*.z=01;31:*.Z=01;31:*.dz=01;31:*.gz=01;31:*.lrz=01;31:*.lz=01;31:*.lzo=01;31:*.xz=01;31:*.bz2=01;31:*.bz=01;31:*.tbz=01;31:*.tbz2=01;31:*.tz=01;31:*.deb=01;31:*.rpm=01;31:*.jar=01;31:*.war=01;31:*.ear=01;31:*.sar=01;31:*.rar=01;31:*.alz=01;31:*.ace=01;31:*.zoo=01;31:*.cpio=01;31:*.7z=01;31:*.rz=01;31:*.cab=01;31:*.jpg=01;35:*.jpeg=01;35:*.gif=01;35:*.bmp=01;35:*.pbm=01;35:*.pgm=01;35:*.ppm=01;35:*.tga=01;35:*.xbm=01;35:*.xpm=01;35:*.tif=01;35:*.tiff=01;35:*.png=01;35:*.svg=01;35:*.svgz=01;35:*.mng=01;35:*.pcx=01;35:*.mov=01;35:*.mpg=01;35:*.mpeg=01;35:*.m2v=01;35:*.mkv=01;35:*.webm=01;35:*.ogm=01;35:*.mp4=01;35:*.m4v=01;35:*.mp4v=01;35:*.vob=01;35:*.qt=01;35:*.nuv=01;35:*.wmv=01;35:*.asf=01;35:*.rm=01;35:*.rmvb=01;35:*.flc=01;35:*.avi=01;35:*.fli=01;35:*.flv=01;35:*.gl=01;35:*.dl=01;35:*.xcf=01;35:*.xwd=01;35:*.yuv=01;35:*.cgm=01;35:*.emf=01;35:*.ogv=01;35:*.ogx=01;35:*.aac=00;36:*.au=00;36:*.flac=00;36:*.m4a=00;36:*.mid=00;36:*.midi=00;36:*.mka=00;36:*.mp3=00;36:*.mpc=00;36:*.ogg=00;36:*.ra=00;36:*.wav=00;36:*.oga=00;36:*.opus=00;36:*.spx=00;36:*.xspf=00;36:
MANDATORY_PATH=/usr/share/gconf/gnome.mandatory.path
MYZSH=/home/me/.hugh/general
NODE_PATH=/usr/lib/nodejs:/usr/lib/node_modules:/usr/share/javascript
OLDPWD=/home/me
PAGER=less
PAM_KWALLET5_LOGIN=/tmp/kwallet5_me.socket
PATH=/home/me/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/home/me/.config/nvim/dein/repos/github.com/junegunn/fzf/bin:/home/me/texlive/2016/bin/x86_64-linux:/home/me/work/tools/bin:/usr/local/cuda/bin:/home/me/.hugh/vimfiles/dein/repos/github.com/junegunn/fzf/bin
PWD=/home/me
QT4_IM_MODULE=xim
QT_ACCESSIBILITY=1
QT_IM_MODULE=ibus
QT_LINUX_ACCESSIBILITY_ALWAYS_ON=1
QT_QPA_PLATFORMTHEME=appmenu-qt5
SESSIONTYPE=gnome-session
SHELL=/usr/bin/zsh
SHLVL=2
SSH_AUTH_SOCK=/run/user/1001/keyring/ssh
TERM=screen-256color
TMUX_PANE=%13
TMUX=/tmp/tmux-1001/default,5914,1
UPDATE_ZSH_DAYS=7
UPSTART_EVENTS=started starting
UPSTART_INSTANCE=
UPSTART_JOB=gnome-settings-daemon
UPSTART_SESSION=unix:abstract=/com/ubuntu/upstart-session/1001/2794
USER=me
_=/usr/bin/env
VTE_VERSION=4205
WINDOWID=54531185
XAUTHORITY=/home/me/.Xauthority
XDG_CONFIG_DIRS=/etc/xdg/xdg-gnome:/usr/share/upstart/xdg:/etc/xdg
XDG_CURRENT_DESKTOP=GNOME
XDG_DATA_DIRS=/usr/share/gnome:/usr/local/share:/usr/share:/var/lib/snapd/desktop:/var/lib/snapd/desktop
XDG_GREETER_DATA_DIR=/var/lib/lightdm-data/me
XDG_RUNTIME_DIR=/run/user/1001
XDG_SEAT_PATH=/org/freedesktop/DisplayManager/Seat0
XDG_SEAT=seat0
XDG_SESSION_DESKTOP=gnome
XDG_SESSION_ID=c2
XDG_SESSION_PATH=/org/freedesktop/DisplayManager/Session0
XDG_SESSION_TYPE=x11
XDG_VTNR=7
XMODIFIERS=@im=ibus
ZSH=/home/me/.oh-my-zsh
```
`conda shell.posix activate base`:
```
export CONDA_PROMPT_MODIFIER='(base) '
export CONDA_SHLVL='1'
```
For now I've added a check in `.zshrc`, if it detects that the shell is running in tmux then it does a `conda deactivate` before the `conda activate base` line. That works fine, I'm happy enough with the results | 2018-02-01T22:51:36 | -1.0 |
conda/conda | 6,853 | conda__conda-6853 | [
"6833"
] | 01cec212c8ec28274cf464c61380e72bc70dc3d0 | diff --git a/conda/resolve.py b/conda/resolve.py
--- a/conda/resolve.py
+++ b/conda/resolve.py
@@ -566,13 +566,21 @@ def get_dists_for_spec(self, ms, emptyok=False):
return sorted(dists, key=self.version_key)
@staticmethod
- def ms_to_v(ms):
+ def to_ms_clause_id(ms):
ms = MatchSpec(ms)
return '@s@' + ms.spec + ('?' if ms.optional else '')
+ @staticmethod
+ def to_notd_ms_clause_id(ms):
+ return '!@s@'+ms.name
+
+ @staticmethod
+ def to_feature_metric_id(dist, feat):
+ return '@fm@%s@%s' % (dist, feat)
+
def push_MatchSpec(self, C, ms):
ms = MatchSpec(ms)
- name = self.ms_to_v(ms)
+ name = self.to_ms_clause_id(ms)
m = C.from_name(name)
if m is not None:
return name
@@ -588,7 +596,7 @@ def push_MatchSpec(self, C, ms):
if m is None:
libs = [dist.full_name for dist in libs]
if ms.optional:
- libs.append('!@s@'+ms.name)
+ libs.append(self.to_notd_ms_clause_id(ms))
m = C.Any(libs)
C.name_var(m, name)
return name
@@ -601,7 +609,7 @@ def gen_clauses(self):
for fkey in group:
C.new_var(fkey)
# Create one variable for the group
- m = C.new_var(self.ms_to_v(name))
+ m = C.new_var(self.to_ms_clause_id(name))
# Exactly one of the package variables, OR
# the negation of the group variable, is true
C.Require(C.ExactlyOne, group + [C.Not(m)])
@@ -624,20 +632,22 @@ def generate_update_count(self, C, specs):
def generate_feature_metric(self, C):
eq = {} # a C.minimize() objective: Dict[varname, coeff]
- total = 0
+ # Given a pair (dist, feature), assign a "1" score IF:
+ # - The dist is installed
+ # - The dist does NOT require the feature
+ # - At least one package in the group DOES require the feature
+ # - A package that tracks the feature is installed
for name, group in iteritems(self.groups):
- nf = [len(self.features(dist)) for dist in group]
- maxf = max(nf)
- if maxf > 0:
- eq.update({dist.full_name: maxf-fc for dist, fc in zip(group, nf) if maxf > fc})
- # This entry causes conda to weight the absence of a package the same
- # as if a non-featured version were instaslled. If this were not here,
- # conda will sometimes select an earlier build of a requested package
- # if doing so can eliminate this package as a dependency.
- # https://github.com/conda/conda/issues/6765
- eq['!' + self.push_MatchSpec(C, name)] = maxf
- total += maxf
- return eq, total
+ dist_feats = {dist.full_name: self.features(dist) for dist in group}
+ active_feats = set.union(*dist_feats.values()).intersection(self.trackers)
+ for feat in active_feats:
+ clause_id_for_feature = self.push_MatchSpec(C, '@' + feat)
+ for dist, features in dist_feats.items():
+ if feat not in features:
+ feature_metric_id = self.to_feature_metric_id(dist, feat)
+ C.name_var(C.And(dist, clause_id_for_feature), feature_metric_id)
+ eq[feature_metric_id] = 1
+ return eq
def generate_removal_count(self, C, specs):
return {'!'+self.push_MatchSpec(C, ms.name): 1 for ms in specs}
@@ -935,11 +945,16 @@ def mysat(specs, add_if=False):
solution, obj1 = C.minimize(eq_feature_count, solution)
log.debug('Track feature count: %d', obj1)
- # Featured packages: maximize featured package count
- eq_feature_metric, ftotal = r2.generate_feature_metric(C)
+ # Featured packages: minimize number of featureless packages
+ # installed when a featured alternative is feasible.
+ # For example, package name foo exists with two built packages. One with
+ # 'track_features: 'feat1', and one with 'track_features': 'feat2'.
+ # The previous "Track features" minimization pass has chosen 'feat1' for the
+ # environment, but not 'feat2'. In this case, the 'feat2' version of foo is
+ # considered "featureless."
+ eq_feature_metric = r2.generate_feature_metric(C)
solution, obj2 = C.minimize(eq_feature_metric, solution)
- obj2 = ftotal - obj2
- log.debug('Package feature count: %d', obj2)
+ log.debug('Package misfeature count: %d', obj2)
# Requested packages: maximize builds
solution, obj4 = C.minimize(eq_req_b, solution)
| diff --git a/tests/test_resolve.py b/tests/test_resolve.py
--- a/tests/test_resolve.py
+++ b/tests/test_resolve.py
@@ -1395,3 +1395,77 @@ def test_update_deps():
'tk-8.5.13-0.tar.bz2',
'zlib-1.2.7-0.tar.bz2',
]]]
+
+
+def test_surplus_features_1():
+ index = {
+ 'feature-1.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'feature',
+ 'version': '1.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'track_features': 'feature',
+ }),
+ 'package1-1.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'package1',
+ 'version': '1.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'features': 'feature',
+ }),
+ 'package2-1.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'package2',
+ 'version': '1.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'depends': ['package1'],
+ 'features': 'feature',
+ }),
+ 'package2-2.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'package2',
+ 'version': '2.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'features': 'feature',
+ }),
+ }
+ r = Resolve({Dist(key): value for key, value in iteritems(index)})
+ install = r.install(['package2', 'feature'])
+ assert 'package1' not in set(d.name for d in install)
+
+
+def test_surplus_features_2():
+ index = {
+ 'feature-1.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'feature',
+ 'version': '1.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'track_features': 'feature',
+ }),
+ 'package1-1.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'package1',
+ 'version': '1.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'features': 'feature',
+ }),
+ 'package2-1.0-0.tar.bz2': IndexRecord(**{
+ 'name': 'package2',
+ 'version': '1.0',
+ 'build': '0',
+ 'build_number': 0,
+ 'depends': ['package1'],
+ 'features': 'feature',
+ }),
+ 'package2-1.0-1.tar.bz2': IndexRecord(**{
+ 'name': 'package2',
+ 'version': '1.0',
+ 'build': '1',
+ 'build_number': 1,
+ 'features': 'feature',
+ }),
+ }
+ r = Resolve({Dist(key): value for key, value in iteritems(index)})
+ install = r.install(['package2', 'feature'])
+ assert 'package1' not in set(d.name for d in install)
| Regression in conda 4.3.33 regarding build features
It seems that `conda 4.3.33` installs packages that have a build feature when installing any package that has the same build feature.
From a fresh miniconda, with conda pinned at `4.3.32`:
```sh
scorlay@mbp ~]$ conda install cryptopp -c QuantStack -c conda-forge
Fetching package metadata ...............
Solving package specifications: .
Package plan for installation in environment /Users/scorlay/miniconda3:
The following NEW packages will be INSTALLED:
cling-patches: 1-0 QuantStack
cryptopp: 5.6.5-cling_0 QuantStack [cling]
```
With `conda 4.3.33`
```sh
[scorlay@mbp ~]$ conda install cryptopp -c QuantStack -c conda-forge
Fetching package metadata ...............
Solving package specifications: .
Package plan for installation in environment /Users/scorlay/miniconda3:
The following NEW packages will be INSTALLED:
clangdev: 5.0.0-cling_1 QuantStack [cling]
cling-patches: 1-0 QuantStack
cryptopp: 5.6.5-cling_0 QuantStack [cling]
icu: 58.2-0 conda-forge
libiconv: 1.15-0 conda-forge
libxml2: 2.9.7-0 conda-forge
llvmdev: 5.0.0-cling_1 QuantStack [cling]
```
The only package tracking the `cling` build feature is `cling-patches`.
Notice in the second case that `clangdev` gets installed while it is not a dependency.
| As per @jjhelmus 's comment, this might be due to #6766. cc @mcg1969 .
Cannot reproduce thiswith a fresh `Miniconda3-4.3.31-Linux-x86_64.sh` installation updated to `conda 4.3.32`:
```bash
$ conda --version
conda 4.3.32
$ conda create -qnx --dry-run -c QuantStack -c conda-forge cryptopp cling@
Package plan for installation in environment /home/maba/code/conda/mc4332/envs/x:
The following NEW packages will be INSTALLED:
cling-patches: 1-0 QuantStack
cryptopp: 5.6.5-cling_0 QuantStack [cling]
libgcc-7: 7.2.0-0 QuantStack
$ conda install -qy conda=4.3.33
Package plan for installation in environment /home/maba/code/conda/mc4332:
The following packages will be UPDATED:
conda: 4.3.32-py36_0 --> 4.3.33-py36_0
$ conda create -qnx --dry-run -c QuantStack -c conda-forge cryptopp cling@
Package plan for installation in environment /home/maba/code/conda/mc4332/envs/x:
The following NEW packages will be INSTALLED:
cling-patches: 1-0 QuantStack
cryptopp: 5.6.5-cling_0 QuantStack [cling]
libgcc-7: 7.2.0-0 QuantStack
```
Can you give more information on your environment? (E.g., fill out the issue template.)
This is something I am seeing on OS X. Locally, and also on Travis. This is impacting our conda-forge style builds.
Features need to die a fiery death. They simply are not SAT representable as conceived :-(
I will see what I can do.
> Features need to die a fiery death. They simply are not SAT representable as conceived :-(
> I will see what I can do.
What should I use instead.
@SylvainCorlay can we get a `conda create` recipe from you that can reproduce the problem, so that it can be reliably reproduced?
> What should I used instead.
It depends exactly what you are trying to do.
> @SylvainCorlay can we get a conda create recipe from you that can reproduce the problem, so that it can be reliably reproduced?
I don't understand what you would like here, but I would be happy to help.
Your examples involve `conda install` commands --- I wonder if you can give us a `conda create` command instead that reveals the issue. With `conda install`, it assumes an existing envrionment with a set of packages that might be influencing the solution.
ok will do. FYI, I produced this from the root environment of a newly installed miniconda.
Note: I am not reproducing with `conda create`. Only `conda install`.
`conda create` reproducer:
```
|$ conda --version
conda 4.3.32
|$ CONDA_SUBDIR=osx-64 conda create -qnx --dry-run -c QuantStack -c conda-forge cryptopp icu
Package plan for installation in environment /home/maba/code/conda/conda-4.3/envs/x:
The following NEW packages will be INSTALLED:
cling-patches: 1-0 QuantStack
cryptopp: 5.6.5-cling_0 QuantStack [cling]
icu: 58.2-0 conda-forge
|$ conda install -qy conda=4.3.33
Package plan for installation in environment /home/maba/code/conda/conda-4.3:
The following packages will be UPDATED:
conda: 4.3.32-py36_0 --> 4.3.33-py36_0
|$ CONDA_SUBDIR=osx-64 conda create -qnx --dry-run -c QuantStack -c conda-forge cryptopp icu
Package plan for installation in environment /home/maba/code/conda/conda-4.3/envs/x:
The following NEW packages will be INSTALLED:
clangdev: 5.0.0-cling_1 QuantStack [cling]
cling-patches: 1-0 QuantStack
cryptopp: 5.6.5-cling_0 QuantStack [cling]
icu: 58.2-0 conda-forge
libiconv: 1.15-0 conda-forge
libxml2: 2.9.7-0 conda-forge
llvmdev: 5.0.0-cling_1 QuantStack [cling]
xz: 5.2.3-0 conda-forge
zlib: 1.2.11-0 conda-forge
```
(uses `CONDA_SUBDIR=osx-64` as I'm on Linux).
To reproduce it, all parts of `-c QuantStack -c conda-forge cryptopp icu` are needed.
@mcg1969 has told me that he probably supports reverting #6766 in a conda 4.3.34 release. Do you agree @mbargull?
That breaks my heart 😢. That PR improved the situation on previously failing cases a lot.
Reverting that #6766 would mean we are back to square one for https://github.com/conda/conda/issues/6269#issuecomment-344077631.
I still agree with
https://github.com/conda/conda/issues/6833#issuecomment-362604480:
> Features need to die a fiery death. They simply are not SAT representable as conceived :-(
https://github.com/conda/conda/pull/6296#issuecomment-347257443:
> We have discussed this at length internally. @mcg1969 is very hesitant to change anything about how features work. There's a lot of pain there, and his opinion is that it's very hard to anticipate any potential breakage from this PR.
But still, I had hoped those latest changes were to stay 😞.
Well, for a short time, people who experienced regressions can pin conda to an earlier version. But we need to figure something out soon.
We did just discover something useful that may help us form a solution. It turns out that just using a `track_feature` _with no corresponding `feature` packages_ has a useful interpretation for establishing preferences. I'm trying to see if we can reproduce that useful quality.
conda-forge relies a lot on build featutes for MSVC flavors. We do a lot at QuantStack for flavors of builds of llvm (for cling which requires patches).
In another case, we have used it to overcome problems with channel priorities not being respected, ehich appears to have been solved since but a lot of our builds still have the features.
Maybe a compromise would be to revert it in 4.3 but leave it in 4.4? Idk.
Sent from my iPhone
> On Feb 7, 2018, at 7:32 AM, Sylvain Corlay <notifications@github.com> wrote:
>
> conda-forge relies a lot on build featutes for MSVC flavors. We do a lot at QuantStack for flavors of builds of llvm (for cling which requires patches).
>
> In another case, we have used it to overcome problems with channel priorities not being respected, ehich appears to have been solved since but a lot of our builds still have the features.
>
> —
> You are receiving this because you commented.
> Reply to this email directly, view it on GitHub, or mute the thread.
>
May be @mwcraig @isuruf @pelson @jakirkham have an opinion on this. If it is a backward incompatible change, it would probably deserve a `5.0`.
Is there a good alternative to build features at the moment?
> Maybe a compromise would be to revert it in 4.3 but leave it in 4.4? Idk.
`conda-forge` and `bioconda` both benefit from `4.3.33` in some cases (and still need some time to update to 4.4)...
> If it is a backward incompatible change, it would probably deserve a `5.0`.
In regards to solver logic, there were already some changes in 4.x (channel priority, timestamps) -- so it's not the case that 4.x was stable in that regard anyway... (That's just mean as an objective and *not* suggestive comment.)
@SylvainCorlay, I haven't yet completely assessed the severity of the issue `4.3.33` introduced for you. Would that be a workflow-breaking change or could it be (in a broader sense) seen as an "inconvenience". I know that the introduction of additional packages (esp. during build) is undesirable, but the question is if that is workflow breaking or not.
This is not to downplay the issue, just to put it into perspective to the issues #6766 helped with (some of them were workflow-breaking), so we can decide, based on severity, what we should do.
| 2018-02-07T17:09:53 | -1.0 |
conda/conda | 6,881 | conda__conda-6881 | [
"6880"
] | 31a52a8a8771aafb0582822dd2e00b3274e1ffba | diff --git a/conda/models/version.py b/conda/models/version.py
--- a/conda/models/version.py
+++ b/conda/models/version.py
@@ -545,10 +545,13 @@ def __new__(cls, spec):
return self
def exact_match_(self, vspec):
- return self.spec == vspec
+ try:
+ return int(self.spec) == int(vspec)
+ except ValueError:
+ return False
def veval_match_(self, vspec):
- return self.op(VersionOrder(vspec), self.cmp)
+ return self.op(VersionOrder(text_type(vspec)), self.cmp)
def triv_match_(self, vspec):
return True
| diff --git a/tests/models/test_match_spec.py b/tests/models/test_match_spec.py
--- a/tests/models/test_match_spec.py
+++ b/tests/models/test_match_spec.py
@@ -395,6 +395,24 @@ def test_key_value_features_match(self):
assert c.match(DPkg(dst, track_features='nomkl'))
assert c.match(DPkg(dst, track_features='blas=nomkl debug'))
+ def test_bracket_matches(self):
+ record = {
+ 'name': 'numpy',
+ 'version': '1.11.0',
+ 'build': 'py34_7',
+ 'build_number': 7,
+ }
+
+ assert MatchSpec("numpy<2").match(record)
+ assert MatchSpec("numpy[version<2]").match(record)
+ assert not MatchSpec("numpy>2").match(record)
+ assert not MatchSpec("numpy[version='>2']").match(record)
+
+ assert MatchSpec("numpy[build_number='7']").match(record)
+ assert MatchSpec("numpy[build_number='<8']").match(record)
+ assert not MatchSpec("numpy[build_number='>7']").match(record)
+ assert MatchSpec("numpy[build_number='>=7']").match(record)
+
class TestArg2Spec(TestCase):
@@ -642,3 +660,47 @@ def test_parse_parens(self):
# "target": "blarg", # suppressing these for now
# "optional": True,
}
+
+ def test_parse_build_number_brackets(self):
+ assert _parse_spec_str("python[build_number=3]") == {
+ "name": "python",
+ "build_number": '3',
+ }
+ assert _parse_spec_str("python[build_number='>3']") == {
+ "name": "python",
+ "build_number": '>3',
+ }
+ assert _parse_spec_str("python[build_number='>=3']") == {
+ "name": "python",
+ "build_number": '>=3',
+ }
+
+ assert _parse_spec_str("python[build_number='<3']") == {
+ "name": "python",
+ "build_number": '<3',
+ }
+ assert _parse_spec_str("python[build_number='<=3']") == {
+ "name": "python",
+ "build_number": '<=3',
+ }
+
+ # # these don't work right now, should they?
+ # assert _parse_spec_str("python[build_number<3]") == {
+ # "name": "python",
+ # "build_number": '<3',
+ # }
+ # assert _parse_spec_str("python[build_number<=3]") == {
+ # "name": "python",
+ # "build_number": '<=3',
+ # }
+
+ # # these don't work right now, should they?
+ # assert _parse_spec_str("python[build_number>3]") == {
+ # "name": "python",
+ # "build_number": '>3',
+ # }
+ # assert _parse_spec_str("python[build_number>=3]") == {
+ # "name": "python",
+ # "build_number": '>=3',
+ # }
+
| build_number comparison not functional in match_spec
Version comparisons work.
conda search 'python[version="<3"]'
Seems that build_number comparisons do not.
conda search 'python[build_number="<5"]'
| 2018-02-14T14:56:27 | -1.0 |
|
conda/conda | 6,887 | conda__conda-6887 | [
"6886"
] | b5f1309be0b8aadefbe82c16a921a72d34695c93 | diff --git a/conda/base/constants.py b/conda/base/constants.py
--- a/conda/base/constants.py
+++ b/conda/base/constants.py
@@ -64,18 +64,18 @@
DEFAULT_CHANNELS_UNIX = (
- 'https://repo.continuum.io/pkgs/main',
- 'https://repo.continuum.io/pkgs/free',
- 'https://repo.continuum.io/pkgs/r',
- 'https://repo.continuum.io/pkgs/pro',
+ 'https://repo.anaconda.com/pkgs/main',
+ 'https://repo.anaconda.com/pkgs/free',
+ 'https://repo.anaconda.com/pkgs/r',
+ 'https://repo.anaconda.com/pkgs/pro',
)
DEFAULT_CHANNELS_WIN = (
- 'https://repo.continuum.io/pkgs/main',
- 'https://repo.continuum.io/pkgs/free',
- 'https://repo.continuum.io/pkgs/r',
- 'https://repo.continuum.io/pkgs/pro',
- 'https://repo.continuum.io/pkgs/msys2',
+ 'https://repo.anaconda.com/pkgs/main',
+ 'https://repo.anaconda.com/pkgs/free',
+ 'https://repo.anaconda.com/pkgs/r',
+ 'https://repo.anaconda.com/pkgs/pro',
+ 'https://repo.anaconda.com/pkgs/msys2',
)
# use the bool(sys.platform == "win32") definition here so we don't import .compat.on_win
diff --git a/conda/core/subdir_data.py b/conda/core/subdir_data.py
--- a/conda/core/subdir_data.py
+++ b/conda/core/subdir_data.py
@@ -390,7 +390,7 @@ def fetch_repodata_remote_request(url, etag, mod_stamp):
if mod_stamp:
headers["If-Modified-Since"] = mod_stamp
- if 'repo.continuum.io' in url:
+ if 'repo.anaconda.com' in url:
filename = 'repodata.json.bz2'
headers['Accept-Encoding'] = 'identity'
else:
diff --git a/conda/models/channel.py b/conda/models/channel.py
--- a/conda/models/channel.py
+++ b/conda/models/channel.py
@@ -114,7 +114,7 @@ def from_value(value):
return Channel.from_url(value)
else:
# at this point assume we don't have a bare (non-scheme) url
- # e.g. this would be bad: repo.continuum.io/pkgs/free
+ # e.g. this would be bad: repo.anaconda.com/pkgs/free
_stripped, platform = split_platform(value, context.known_subdirs)
if _stripped in context.custom_multichannels:
return MultiChannel(_stripped, context.custom_multichannels[_stripped], platform)
| diff --git a/tests/build-index2-json.py b/tests/build-index2-json.py
--- a/tests/build-index2-json.py
+++ b/tests/build-index2-json.py
@@ -3,7 +3,7 @@
def main():
- r1 = requests.get('https://repo.continuum.io/pkgs/free/linux-64/repodata.json')
+ r1 = requests.get('https://repo.anaconda.com/pkgs/free/linux-64/repodata.json')
r1.raise_for_status()
r2 = requests.get('https://conda.anaconda.org/conda-test/noarch/repodata.json')
r2.raise_for_status()
diff --git a/tests/build-index4-json.py b/tests/build-index4-json.py
--- a/tests/build-index4-json.py
+++ b/tests/build-index4-json.py
@@ -3,7 +3,7 @@
def main():
- r1 = requests.get('https://repo.continuum.io/pkgs/main/linux-64/repodata.json')
+ r1 = requests.get('https://repo.anaconda.com/pkgs/main/linux-64/repodata.json')
r1.raise_for_status()
r1json = r1.json()
diff --git a/tests/cli/test_config.py b/tests/cli/test_config.py
--- a/tests/cli/test_config.py
+++ b/tests/cli/test_config.py
@@ -22,7 +22,7 @@
# pass
# unset CIO_TEST. This is a Continuum-internal variable that draws packages from an internal server instead of
-# repo.continuum.io
+# repo.anaconda.com
@contextmanager
def make_temp_condarc(value=None):
diff --git a/tests/core/test_index.py b/tests/core/test_index.py
--- a/tests/core/test_index.py
+++ b/tests/core/test_index.py
@@ -36,7 +36,7 @@ def test_check_whitelist():
get_index(("conda-canary",))
with pytest.raises(OperationNotAllowed):
- get_index(("https://repo.continuum.io/pkgs/denied",))
+ get_index(("https://repo.anaconda.com/pkgs/denied",))
check_whitelist(("defaults",))
check_whitelist((DEFAULT_CHANNELS[0], DEFAULT_CHANNELS[1]))
diff --git a/tests/core/test_repodata.py b/tests/core/test_repodata.py
--- a/tests/core/test_repodata.py
+++ b/tests/core/test_repodata.py
@@ -35,7 +35,7 @@ def test_get_index_no_platform_with_offline_cache(self):
with env_var('CONDA_REPODATA_TIMEOUT_SECS', '0', reset_context):
with patch.object(conda.core.subdir_data, 'read_mod_and_etag') as read_mod_and_etag:
read_mod_and_etag.return_value = {}
- channel_urls = ('https://repo.continuum.io/pkgs/pro',)
+ channel_urls = ('https://repo.anaconda.com/pkgs/pro',)
with env_var('CONDA_REPODATA_TIMEOUT_SECS', '0', reset_context):
this_platform = context.subdir
index = get_index(channel_urls=channel_urls, prepend=False)
@@ -91,7 +91,7 @@ def test_read_mod_and_etag_mod_only(self):
def test_read_mod_and_etag_etag_only(self):
etag_only_str = """
{
- "_url": "https://repo.continuum.io/pkgs/r/noarch",
+ "_url": "https://repo.anaconda.com/pkgs/r/noarch",
"info": {},
"_etag": "\"569c0ecb-48\"",
"packages": {}
@@ -107,7 +107,7 @@ def test_read_mod_and_etag_etag_mod(self):
{
"_etag": "\"569c0ecb-48\"",
"_mod": "Sun, 17 Jan 2016 21:59:39 GMT",
- "_url": "https://repo.continuum.io/pkgs/r/noarch",
+ "_url": "https://repo.anaconda.com/pkgs/r/noarch",
"info": {},
"packages": {}
}
@@ -121,7 +121,7 @@ def test_read_mod_and_etag_mod_etag(self):
mod_etag_str = """
{
"_mod": "Sun, 17 Jan 2016 21:59:39 GMT",
- "_url": "https://repo.continuum.io/pkgs/r/noarch",
+ "_url": "https://repo.anaconda.com/pkgs/r/noarch",
"info": {},
"_etag": "\"569c0ecb-48\"",
"packages": {}
@@ -132,7 +132,7 @@ def test_read_mod_and_etag_mod_etag(self):
assert mod_etag_dict["_mod"] == "Sun, 17 Jan 2016 21:59:39 GMT"
assert mod_etag_dict["_etag"] == "\"569c0ecb-48\""
- def test_cache_fn_url(self):
+ def test_cache_fn_url_repo_continuum_io(self):
hash1 = cache_fn_url("http://repo.continuum.io/pkgs/free/osx-64/")
hash2 = cache_fn_url("http://repo.continuum.io/pkgs/free/osx-64")
assert "aa99d924.json" == hash1 == hash2
@@ -147,6 +147,21 @@ def test_cache_fn_url(self):
hash6 = cache_fn_url("https://repo.continuum.io/pkgs/r/osx-64")
assert hash4 != hash6
+ def test_cache_fn_url_repo_anaconda_com(self):
+ hash1 = cache_fn_url("http://repo.anaconda.com/pkgs/free/osx-64/")
+ hash2 = cache_fn_url("http://repo.anaconda.com/pkgs/free/osx-64")
+ assert "1e817819.json" == hash1 == hash2
+
+ hash3 = cache_fn_url("https://repo.anaconda.com/pkgs/free/osx-64/")
+ hash4 = cache_fn_url("https://repo.anaconda.com/pkgs/free/osx-64")
+ assert "3ce78580.json" == hash3 == hash4 != hash1
+
+ hash5 = cache_fn_url("https://repo.anaconda.com/pkgs/free/linux-64/")
+ assert hash4 != hash5
+
+ hash6 = cache_fn_url("https://repo.anaconda.com/pkgs/r/osx-64")
+ assert hash4 != hash6
+
# @pytest.mark.integration
# class SubdirDataTests(TestCase):
diff --git a/tests/models/test_channel.py b/tests/models/test_channel.py
--- a/tests/models/test_channel.py
+++ b/tests/models/test_channel.py
@@ -33,17 +33,17 @@ class DefaultConfigChannelTests(TestCase):
def setUpClass(cls):
reset_context()
cls.platform = context.subdir
- cls.DEFAULT_URLS = ['https://repo.continuum.io/pkgs/main/%s' % cls.platform,
- 'https://repo.continuum.io/pkgs/main/noarch',
- 'https://repo.continuum.io/pkgs/free/%s' % cls.platform,
- 'https://repo.continuum.io/pkgs/free/noarch',
- 'https://repo.continuum.io/pkgs/r/%s' % cls.platform,
- 'https://repo.continuum.io/pkgs/r/noarch',
- 'https://repo.continuum.io/pkgs/pro/%s' % cls.platform,
- 'https://repo.continuum.io/pkgs/pro/noarch']
+ cls.DEFAULT_URLS = ['https://repo.anaconda.com/pkgs/main/%s' % cls.platform,
+ 'https://repo.anaconda.com/pkgs/main/noarch',
+ 'https://repo.anaconda.com/pkgs/free/%s' % cls.platform,
+ 'https://repo.anaconda.com/pkgs/free/noarch',
+ 'https://repo.anaconda.com/pkgs/r/%s' % cls.platform,
+ 'https://repo.anaconda.com/pkgs/r/noarch',
+ 'https://repo.anaconda.com/pkgs/pro/%s' % cls.platform,
+ 'https://repo.anaconda.com/pkgs/pro/noarch']
if on_win:
- cls.DEFAULT_URLS.extend(['https://repo.continuum.io/pkgs/msys2/%s' % cls.platform,
- 'https://repo.continuum.io/pkgs/msys2/noarch'])
+ cls.DEFAULT_URLS.extend(['https://repo.anaconda.com/pkgs/msys2/%s' % cls.platform,
+ 'https://repo.anaconda.com/pkgs/msys2/noarch'])
def test_channel_alias_channels(self):
channel = Channel('binstar/label/dev')
@@ -103,24 +103,24 @@ def test_default_channel(self):
dc = Channel('defaults/win-32')
assert dc.canonical_name == 'defaults'
assert dc.subdir == 'win-32'
- assert dc.urls()[0] == 'https://repo.continuum.io/pkgs/main/win-32'
- assert dc.urls()[1] == 'https://repo.continuum.io/pkgs/main/noarch'
+ assert dc.urls()[0] == 'https://repo.anaconda.com/pkgs/main/win-32'
+ assert dc.urls()[1] == 'https://repo.anaconda.com/pkgs/main/noarch'
assert dc.urls()[2].endswith('/win-32')
def test_url_channel_w_platform(self):
- channel = Channel('https://repo.continuum.io/pkgs/free/osx-64')
+ channel = Channel('https://repo.anaconda.com/pkgs/free/osx-64')
assert channel.scheme == "https"
- assert channel.location == "repo.continuum.io"
+ assert channel.location == "repo.anaconda.com"
assert channel.platform == 'osx-64' == channel.subdir
assert channel.name == 'pkgs/free'
- assert channel.base_url == 'https://repo.continuum.io/pkgs/free'
+ assert channel.base_url == 'https://repo.anaconda.com/pkgs/free'
assert channel.canonical_name == 'defaults'
- assert channel.url() == 'https://repo.continuum.io/pkgs/free/osx-64'
+ assert channel.url() == 'https://repo.anaconda.com/pkgs/free/osx-64'
assert channel.urls() == [
- 'https://repo.continuum.io/pkgs/free/osx-64',
- 'https://repo.continuum.io/pkgs/free/noarch',
+ 'https://repo.anaconda.com/pkgs/free/osx-64',
+ 'https://repo.anaconda.com/pkgs/free/noarch',
]
def test_bare_channel(self):
@@ -293,7 +293,7 @@ def setUp(cls):
migrated_custom_channels:
darwin: s3://just/cant
chuck: file:///var/lib/repo/
- pkgs/anaconda: https://repo.continuum.io
+ pkgs/anaconda: https://repo.anaconda.com
migrated_channel_aliases:
- https://conda.anaconda.org
channel_alias: ftp://new.url:8082
@@ -331,7 +331,7 @@ def test_pkgs_free(self):
'http://192.168.0.15:8080/pkgs/anaconda/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/anaconda')
+ channel = Channel('https://repo.anaconda.com/pkgs/anaconda')
assert channel.channel_name == "pkgs/anaconda"
assert channel.channel_location == "192.168.0.15:8080"
assert channel.canonical_name == "defaults"
@@ -340,7 +340,7 @@ def test_pkgs_free(self):
'http://192.168.0.15:8080/pkgs/anaconda/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/anaconda/noarch')
+ channel = Channel('https://repo.anaconda.com/pkgs/anaconda/noarch')
assert channel.channel_name == "pkgs/anaconda"
assert channel.channel_location == "192.168.0.15:8080"
assert channel.canonical_name == "defaults"
@@ -348,7 +348,7 @@ def test_pkgs_free(self):
'http://192.168.0.15:8080/pkgs/anaconda/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/anaconda/label/dev')
+ channel = Channel('https://repo.anaconda.com/pkgs/anaconda/label/dev')
assert channel.channel_name == "pkgs/anaconda/label/dev"
assert channel.channel_location == "192.168.0.15:8080"
assert channel.canonical_name == "pkgs/anaconda/label/dev"
@@ -357,7 +357,7 @@ def test_pkgs_free(self):
'http://192.168.0.15:8080/pkgs/anaconda/label/dev/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/anaconda/noarch/flask-1.0.tar.bz2')
+ channel = Channel('https://repo.anaconda.com/pkgs/anaconda/noarch/flask-1.0.tar.bz2')
assert channel.channel_name == "pkgs/anaconda"
assert channel.channel_location == "192.168.0.15:8080"
assert channel.platform == "noarch"
@@ -377,40 +377,40 @@ def test_pkgs_pro(self):
'http://192.168.0.15:8080/pkgs/pro/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/pro')
+ channel = Channel('https://repo.anaconda.com/pkgs/pro')
assert channel.channel_name == "pkgs/pro"
- assert channel.channel_location == "repo.continuum.io"
+ assert channel.channel_location == "repo.anaconda.com"
assert channel.canonical_name == "defaults"
assert channel.urls() == [
- 'https://repo.continuum.io/pkgs/pro/%s' % self.platform,
- 'https://repo.continuum.io/pkgs/pro/noarch',
+ 'https://repo.anaconda.com/pkgs/pro/%s' % self.platform,
+ 'https://repo.anaconda.com/pkgs/pro/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/pro/noarch')
+ channel = Channel('https://repo.anaconda.com/pkgs/pro/noarch')
assert channel.channel_name == "pkgs/pro"
- assert channel.channel_location == "repo.continuum.io"
+ assert channel.channel_location == "repo.anaconda.com"
assert channel.canonical_name == "defaults"
assert channel.urls() == [
- 'https://repo.continuum.io/pkgs/pro/noarch',
+ 'https://repo.anaconda.com/pkgs/pro/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/pro/label/dev')
+ channel = Channel('https://repo.anaconda.com/pkgs/pro/label/dev')
assert channel.channel_name == "pkgs/pro/label/dev"
- assert channel.channel_location == "repo.continuum.io"
+ assert channel.channel_location == "repo.anaconda.com"
assert channel.canonical_name == "pkgs/pro/label/dev"
assert channel.urls() == [
- 'https://repo.continuum.io/pkgs/pro/label/dev/%s' % self.platform,
- 'https://repo.continuum.io/pkgs/pro/label/dev/noarch',
+ 'https://repo.anaconda.com/pkgs/pro/label/dev/%s' % self.platform,
+ 'https://repo.anaconda.com/pkgs/pro/label/dev/noarch',
]
- channel = Channel('https://repo.continuum.io/pkgs/pro/noarch/flask-1.0.tar.bz2')
+ channel = Channel('https://repo.anaconda.com/pkgs/pro/noarch/flask-1.0.tar.bz2')
assert channel.channel_name == "pkgs/pro"
- assert channel.channel_location == "repo.continuum.io"
+ assert channel.channel_location == "repo.anaconda.com"
assert channel.platform == "noarch"
assert channel.package_filename == "flask-1.0.tar.bz2"
assert channel.canonical_name == "defaults"
assert channel.urls() == [
- 'https://repo.continuum.io/pkgs/pro/noarch',
+ 'https://repo.anaconda.com/pkgs/pro/noarch',
]
def test_custom_channels(self):
@@ -976,21 +976,21 @@ def test_multichannel_priority():
('https://conda.anaconda.org/conda-test/new-optimized-subdir', ('conda-test', 0)),
('https://conda.anaconda.org/conda-test/linux-32', ('conda-test', 0)),
('https://conda.anaconda.org/conda-test/noarch', ('conda-test', 0)),
- ('https://repo.continuum.io/pkgs/main/new-optimized-subdir', ('defaults', 1)),
- ('https://repo.continuum.io/pkgs/main/linux-32', ('defaults', 1)),
- ('https://repo.continuum.io/pkgs/main/noarch', ('defaults', 1)),
- ('https://repo.continuum.io/pkgs/free/new-optimized-subdir', ('defaults', 2)),
- ('https://repo.continuum.io/pkgs/free/linux-32', ('defaults', 2)),
- ('https://repo.continuum.io/pkgs/free/noarch', ('defaults', 2)),
- ('https://repo.continuum.io/pkgs/r/new-optimized-subdir', ('defaults', 3)),
- ('https://repo.continuum.io/pkgs/r/linux-32', ('defaults', 3)),
- ('https://repo.continuum.io/pkgs/r/noarch', ('defaults', 3)),
- ('https://repo.continuum.io/pkgs/pro/new-optimized-subdir', ('defaults', 4)),
- ('https://repo.continuum.io/pkgs/pro/linux-32', ('defaults', 4)),
- ('https://repo.continuum.io/pkgs/pro/noarch', ('defaults', 4)),
- ('https://repo.continuum.io/pkgs/msys2/new-optimized-subdir', ('defaults', 5)),
- ('https://repo.continuum.io/pkgs/msys2/linux-32', ('defaults', 5)),
- ('https://repo.continuum.io/pkgs/msys2/noarch', ('defaults', 5)),
+ ('https://repo.anaconda.com/pkgs/main/new-optimized-subdir', ('defaults', 1)),
+ ('https://repo.anaconda.com/pkgs/main/linux-32', ('defaults', 1)),
+ ('https://repo.anaconda.com/pkgs/main/noarch', ('defaults', 1)),
+ ('https://repo.anaconda.com/pkgs/free/new-optimized-subdir', ('defaults', 2)),
+ ('https://repo.anaconda.com/pkgs/free/linux-32', ('defaults', 2)),
+ ('https://repo.anaconda.com/pkgs/free/noarch', ('defaults', 2)),
+ ('https://repo.anaconda.com/pkgs/r/new-optimized-subdir', ('defaults', 3)),
+ ('https://repo.anaconda.com/pkgs/r/linux-32', ('defaults', 3)),
+ ('https://repo.anaconda.com/pkgs/r/noarch', ('defaults', 3)),
+ ('https://repo.anaconda.com/pkgs/pro/new-optimized-subdir', ('defaults', 4)),
+ ('https://repo.anaconda.com/pkgs/pro/linux-32', ('defaults', 4)),
+ ('https://repo.anaconda.com/pkgs/pro/noarch', ('defaults', 4)),
+ ('https://repo.anaconda.com/pkgs/msys2/new-optimized-subdir', ('defaults', 5)),
+ ('https://repo.anaconda.com/pkgs/msys2/linux-32', ('defaults', 5)),
+ ('https://repo.anaconda.com/pkgs/msys2/noarch', ('defaults', 5)),
('https://conda.anaconda.org/conda-forge/new-optimized-subdir', ('conda-forge', 6)),
('https://conda.anaconda.org/conda-forge/linux-32', ('conda-forge', 6)),
('https://conda.anaconda.org/conda-forge/noarch', ('conda-forge', 6)),
@@ -1000,18 +1000,18 @@ def test_multichannel_priority():
('https://conda.anaconda.org/conda-test/new-optimized-subdir', ('conda-test', 0)),
('https://conda.anaconda.org/conda-test/linux-32', ('conda-test', 0)),
('https://conda.anaconda.org/conda-test/noarch', ('conda-test', 0)),
- ('https://repo.continuum.io/pkgs/main/new-optimized-subdir', ('defaults', 1)),
- ('https://repo.continuum.io/pkgs/main/linux-32', ('defaults', 1)),
- ('https://repo.continuum.io/pkgs/main/noarch', ('defaults', 1)),
- ('https://repo.continuum.io/pkgs/free/new-optimized-subdir', ('defaults', 2)),
- ('https://repo.continuum.io/pkgs/free/linux-32', ('defaults', 2)),
- ('https://repo.continuum.io/pkgs/free/noarch', ('defaults', 2)),
- ('https://repo.continuum.io/pkgs/r/new-optimized-subdir', ('defaults', 3)),
- ('https://repo.continuum.io/pkgs/r/linux-32', ('defaults', 3)),
- ('https://repo.continuum.io/pkgs/r/noarch', ('defaults', 3)),
- ('https://repo.continuum.io/pkgs/pro/new-optimized-subdir', ('defaults', 4)),
- ('https://repo.continuum.io/pkgs/pro/linux-32', ('defaults', 4)),
- ('https://repo.continuum.io/pkgs/pro/noarch', ('defaults', 4)),
+ ('https://repo.anaconda.com/pkgs/main/new-optimized-subdir', ('defaults', 1)),
+ ('https://repo.anaconda.com/pkgs/main/linux-32', ('defaults', 1)),
+ ('https://repo.anaconda.com/pkgs/main/noarch', ('defaults', 1)),
+ ('https://repo.anaconda.com/pkgs/free/new-optimized-subdir', ('defaults', 2)),
+ ('https://repo.anaconda.com/pkgs/free/linux-32', ('defaults', 2)),
+ ('https://repo.anaconda.com/pkgs/free/noarch', ('defaults', 2)),
+ ('https://repo.anaconda.com/pkgs/r/new-optimized-subdir', ('defaults', 3)),
+ ('https://repo.anaconda.com/pkgs/r/linux-32', ('defaults', 3)),
+ ('https://repo.anaconda.com/pkgs/r/noarch', ('defaults', 3)),
+ ('https://repo.anaconda.com/pkgs/pro/new-optimized-subdir', ('defaults', 4)),
+ ('https://repo.anaconda.com/pkgs/pro/linux-32', ('defaults', 4)),
+ ('https://repo.anaconda.com/pkgs/pro/noarch', ('defaults', 4)),
('https://conda.anaconda.org/conda-forge/new-optimized-subdir', ('conda-forge', 5)),
('https://conda.anaconda.org/conda-forge/linux-32', ('conda-forge', 5)),
('https://conda.anaconda.org/conda-forge/noarch', ('conda-forge', 5)),
diff --git a/tests/models/test_dist.py b/tests/models/test_dist.py
--- a/tests/models/test_dist.py
+++ b/tests/models/test_dist.py
@@ -56,7 +56,7 @@ class UrlDistTests(TestCase):
def test_dist_with_channel_url(self):
# standard named channel
- url = "https://repo.continuum.io/pkgs/free/win-64/spyder-app-2.3.8-py27_0.tar.bz2"
+ url = "https://repo.anaconda.com/pkgs/free/win-64/spyder-app-2.3.8-py27_0.tar.bz2"
d = Dist(url)
assert d.channel == 'defaults'
assert d.name == 'spyder-app'
@@ -120,7 +120,7 @@ def test_dist_with_channel_url(self):
def test_dist_with_non_channel_url(self):
# contrived url
- url = "https://repo.continuum.io/pkgs/anaconda/cffi-1.9.1-py34_0.tar.bz2"
+ url = "https://repo.anaconda.com/pkgs/anaconda/cffi-1.9.1-py34_0.tar.bz2"
d = Dist(url)
assert d.channel == '<unknown>'
assert d.name == 'cffi'
diff --git a/tests/models/test_index_record.py b/tests/models/test_index_record.py
--- a/tests/models/test_index_record.py
+++ b/tests/models/test_index_record.py
@@ -21,23 +21,23 @@ def test_prefix_record_no_channel(self):
version='1.2.3',
build_string='py34_2',
build_number=2,
- url="https://repo.continuum.io/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2",
+ url="https://repo.anaconda.com/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2",
subdir="win-32",
md5='0123456789',
files=(),
)
- assert pr.url == "https://repo.continuum.io/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2"
+ assert pr.url == "https://repo.anaconda.com/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2"
assert pr.channel.canonical_name == 'defaults'
assert pr.subdir == "win-32"
assert pr.fn == "austin-1.2.3-py34_2.tar.bz2"
- channel_str = text_type(Channel("https://repo.continuum.io/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2"))
- assert channel_str == "https://repo.continuum.io/pkgs/free/win-32"
+ channel_str = text_type(Channel("https://repo.anaconda.com/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2"))
+ assert channel_str == "https://repo.anaconda.com/pkgs/free/win-32"
assert dict(pr.dump()) == dict(
name='austin',
version='1.2.3',
build='py34_2',
build_number=2,
- url="https://repo.continuum.io/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2",
+ url="https://repo.anaconda.com/pkgs/free/win-32/austin-1.2.3-py34_2.tar.bz2",
md5='0123456789',
files=(),
channel=channel_str,
diff --git a/tests/models/test_match_spec.py b/tests/models/test_match_spec.py
--- a/tests/models/test_match_spec.py
+++ b/tests/models/test_match_spec.py
@@ -178,17 +178,17 @@ def test_canonical_string_forms(self):
assert m("numpy=1.7=py3*_2") == "numpy==1.7[build=py3*_2]"
assert m("numpy=1.7.*=py3*_2") == "numpy=1.7[build=py3*_2]"
- assert m("https://repo.continuum.io/pkgs/free::numpy") == "defaults::numpy"
- assert m("numpy[channel=https://repo.continuum.io/pkgs/free]") == "defaults::numpy"
+ assert m("https://repo.anaconda.com/pkgs/free::numpy") == "defaults::numpy"
+ assert m("numpy[channel=https://repo.anaconda.com/pkgs/free]") == "defaults::numpy"
assert m("defaults::numpy") == "defaults::numpy"
assert m("numpy[channel=defaults]") == "defaults::numpy"
assert m("conda-forge::numpy") == "conda-forge::numpy"
assert m("numpy[channel=conda-forge]") == "conda-forge::numpy"
assert m("numpy[channel=defaults,subdir=osx-64]") == "defaults/osx-64::numpy"
- assert m("numpy[channel=https://repo.continuum.io/pkgs/free/osx-64, subdir=linux-64]") == "defaults/linux-64::numpy"
- assert m("https://repo.continuum.io/pkgs/free/win-32::numpy") == "defaults/win-32::numpy"
- assert m("numpy[channel=https://repo.continuum.io/pkgs/free/osx-64]") == "defaults/osx-64::numpy"
+ assert m("numpy[channel=https://repo.anaconda.com/pkgs/free/osx-64, subdir=linux-64]") == "defaults/linux-64::numpy"
+ assert m("https://repo.anaconda.com/pkgs/free/win-32::numpy") == "defaults/win-32::numpy"
+ assert m("numpy[channel=https://repo.anaconda.com/pkgs/free/osx-64]") == "defaults/osx-64::numpy"
assert m("defaults/win-32::numpy") == "defaults/win-32::numpy"
assert m("conda-forge/linux-64::numpy") == "conda-forge/linux-64::numpy"
assert m("numpy[channel=conda-forge,subdir=noarch]") == "conda-forge/noarch::numpy"
@@ -198,8 +198,8 @@ def test_canonical_string_forms(self):
assert m("*/win-32::numpy[subdir=\"osx-64\"]") == 'numpy[subdir=osx-64]'
# TODO: should the result in these example pull out subdir?
- assert m("https://repo.continuum.io/pkgs/free/linux-32::numpy") == "defaults/linux-32::numpy"
- assert m("numpy[channel=https://repo.continuum.io/pkgs/free/linux-32]") == "defaults/linux-32::numpy"
+ assert m("https://repo.anaconda.com/pkgs/free/linux-32::numpy") == "defaults/linux-32::numpy"
+ assert m("numpy[channel=https://repo.anaconda.com/pkgs/free/linux-32]") == "defaults/linux-32::numpy"
assert m("numpy=1.10=py38_0") == "numpy==1.10=py38_0"
assert m("numpy==1.10=py38_0") == "numpy==1.10=py38_0"
@@ -280,13 +280,13 @@ def test_channel_matching(self):
# it might have to for backward compatibility
# but more ideally, the first would be true, and the second would be false
# (or maybe it's the other way around)
- assert ChannelMatch("https://repo.continuum.io/pkgs/free").match('defaults') is True
- assert ChannelMatch("defaults").match("https://repo.continuum.io/pkgs/free") is True
+ assert ChannelMatch("https://repo.anaconda.com/pkgs/free").match('defaults') is True
+ assert ChannelMatch("defaults").match("https://repo.anaconda.com/pkgs/free") is True
assert ChannelMatch("https://conda.anaconda.org/conda-forge").match('conda-forge') is True
assert ChannelMatch("conda-forge").match("https://conda.anaconda.org/conda-forge") is True
- assert ChannelMatch("https://repo.continuum.io/pkgs/free").match('conda-forge') is False
+ assert ChannelMatch("https://repo.anaconda.com/pkgs/free").match('conda-forge') is False
def test_matchspec_errors(self):
with pytest.raises(ValueError):
@@ -335,13 +335,13 @@ def test_dist(self):
depends=('openssl 1.0.2*', 'readline 6.2*', 'sqlite',
'tk 8.5*', 'xz 5.0.5', 'zlib 1.2*', 'pip'),
channel=Channel(scheme='https', auth=None,
- location='repo.continuum.io', token=None,
+ location='repo.anaconda.com', token=None,
name='pkgs/free', platform='osx-64',
package_filename=None),
subdir='osx-64', fn='python-3.5.1-0.tar.bz2',
md5='a813bc0a32691ab3331ac9f37125164c', size=14678857,
priority=0,
- url='https://repo.continuum.io/pkgs/free/osx-64/python-3.5.1-0.tar.bz2'))
+ url='https://repo.anaconda.com/pkgs/free/osx-64/python-3.5.1-0.tar.bz2'))
def test_index_record(self):
dst = Dist('defaults::foo-1.2.3-4.tar.bz2')
@@ -452,7 +452,7 @@ def test_pip_style(self):
class SpecStrParsingTests(TestCase):
def test_parse_spec_str_tarball_url(self):
- url = "https://repo.continuum.io/pkgs/free/linux-64/_license-1.1-py27_1.tar.bz2"
+ url = "https://repo.anaconda.com/pkgs/free/linux-64/_license-1.1-py27_1.tar.bz2"
assert _parse_spec_str(url) == {
"channel": "defaults",
"subdir": "linux-64",
@@ -502,7 +502,7 @@ def test_parse_spec_str_no_brackets(self):
"channel": "defaults",
"name": "numpy",
}
- assert _parse_spec_str("https://repo.continuum.io/pkgs/free::numpy") == {
+ assert _parse_spec_str("https://repo.anaconda.com/pkgs/free::numpy") == {
"channel": "defaults",
"name": "numpy",
}
diff --git a/tests/test_cli.py b/tests/test_cli.py
--- a/tests/test_cli.py
+++ b/tests/test_cli.py
@@ -118,7 +118,7 @@ def test_search_3(self):
result = stdout.replace("Loading channels: ...working... done", "")
assert "file name : nose-1.3.7-py36_1.tar.bz2" in result
assert "name : nose" in result
- assert "url : https://repo.continuum.io/pkgs/free/linux-64/nose-1.3.7-py36_1.tar.bz2" in result
+ assert "url : https://repo.anaconda.com/pkgs/free/linux-64/nose-1.3.7-py36_1.tar.bz2" in result
assert "md5 : f4f697f5ad4df9c8fe35357d269718a5" in result
@pytest.mark.integration
diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -280,7 +280,7 @@ def test_install_python2_and_search(self):
assert_package_is_installed(prefix, 'python-2')
# regression test for #4513
- run_command(Commands.CONFIG, prefix, "--add channels https://repo.continuum.io/pkgs/not-a-channel")
+ run_command(Commands.CONFIG, prefix, "--add channels https://repo.anaconda.com/pkgs/not-a-channel")
stdout, stderr = run_command(Commands.SEARCH, prefix, "python --json")
packages = json.loads(stdout)
assert len(packages) >= 1
@@ -883,7 +883,7 @@ def test_conda_config_validate(self):
def test_rpy_search(self):
with make_temp_env("python=3.5") as prefix:
- run_command(Commands.CONFIG, prefix, "--add channels https://repo.continuum.io/pkgs/free")
+ run_command(Commands.CONFIG, prefix, "--add channels https://repo.anaconda.com/pkgs/free")
run_command(Commands.CONFIG, prefix, "--remove channels defaults")
stdout, stderr = run_command(Commands.CONFIG, prefix, "--show", "--json")
json_obj = json_loads(stdout)
@@ -909,7 +909,7 @@ def test_rpy_search(self):
def test_clone_offline_multichannel_with_untracked(self):
with make_temp_env("python=3.5") as prefix:
- run_command(Commands.CONFIG, prefix, "--add channels https://repo.continuum.io/pkgs/free")
+ run_command(Commands.CONFIG, prefix, "--add channels https://repo.anaconda.com/pkgs/free")
run_command(Commands.CONFIG, prefix, "--remove channels defaults")
run_command(Commands.INSTALL, prefix, "-c conda-test flask")
@@ -1174,7 +1174,7 @@ def test_search_gawk_not_win_filter(self):
with make_temp_env() as prefix:
stdout, stderr = run_command(
Commands.SEARCH, prefix, "*gawk", "--platform", "win-64", "--json",
- "-c", "https://repo.continuum.io/pkgs/msys2 --json",
+ "-c", "https://repo.anaconda.com/pkgs/msys2 --json",
use_exception_handler=True,
)
json_obj = json_loads(stdout.replace("Fetching package metadata ...", "").strip())
diff --git a/tests/test_fetch.py b/tests/test_fetch.py
--- a/tests/test_fetch.py
+++ b/tests/test_fetch.py
@@ -42,7 +42,7 @@ def test_tmpDownload(self):
with env_var('CONDA_REMOTE_CONNECT_TIMEOUT_SECS', 1, reset_context):
with env_var('CONDA_REMOTE_READ_TIMEOUT_SECS', 1, reset_context):
with env_var('CONDA_REMOTE_MAX_RETRIES', 1, reset_context):
- url = "https://repo.continuum.io/pkgs/free/osx-64/appscript-1.0.1-py27_0.tar.bz2"
+ url = "https://repo.anaconda.com/pkgs/free/osx-64/appscript-1.0.1-py27_0.tar.bz2"
with TmpDownload(url) as dst:
assert exists(dst)
assert isfile(dst)
diff --git a/tests/test_misc.py b/tests/test_misc.py
--- a/tests/test_misc.py
+++ b/tests/test_misc.py
@@ -11,6 +11,8 @@ class TestMisc(unittest.TestCase):
def test_cache_fn_url(self):
url = "http://repo.continuum.io/pkgs/pro/osx-64/"
self.assertEqual(cache_fn_url(url), '7618c8b6.json')
+ url = "http://repo.anaconda.com/pkgs/pro/osx-64/"
+ self.assertEqual(cache_fn_url(url), 'e42afea8.json')
def test_url_pat_1(self):
m = url_pat.match('http://www.cont.io/pkgs/linux-64/foo.tar.bz2'
| repo.continuum.io is transitioning to repo.anaconda.com
There is currently no plan to stop service at repo.continuum.io. However, at some point in the distant future, repo.continuum.io might redirect to repo.anaconda.com.
This issue tracks the change for the `defaults` channel urls from repo.continuum.io to repo.anaconda.com.
| 2018-02-15T14:53:10 | -1.0 |
|
conda/conda | 6,921 | conda__conda-6921 | [
"6920"
] | 70ef29117690193a92181f2bd4e7e0ab845158ba | diff --git a/conda/core/index.py b/conda/core/index.py
--- a/conda/core/index.py
+++ b/conda/core/index.py
@@ -4,7 +4,7 @@
from itertools import chain
from logging import getLogger
-from .linked_data import linked_data
+from .linked_data import PrefixData, linked_data
from .package_cache import PackageCache
from .repodata import SubdirData, make_feature_record
from .._vendor.boltons.setutils import IndexedSet
@@ -196,12 +196,16 @@ def push_spec(spec):
pending_track_features.add(ftr_name)
def push_record(record):
+ push_spec(MatchSpec(record.name))
for _spec in record.combined_depends:
push_spec(_spec)
if record.track_features:
for ftr_name in record.track_features:
push_spec(MatchSpec(track_features=ftr_name))
+ if prefix:
+ for prefix_rec in PrefixData(prefix).iter_records():
+ push_record(prefix_rec)
for spec in specs:
push_spec(spec)
| diff --git a/tests/test_create.py b/tests/test_create.py
--- a/tests/test_create.py
+++ b/tests/test_create.py
@@ -683,10 +683,22 @@ def test_tarball_install_and_bad_metadata(self):
assert not package_is_installed(prefix, 'flask', exact=True)
assert_package_is_installed(prefix, 'flask-0.')
+ def test_update_with_pinned_packages(self):
+ # regression test for #6914
+ with make_temp_env("python=2.7.12") as prefix:
+ assert package_is_installed(prefix, "readline-6.2")
+ rm_rf(join(prefix, 'conda-meta', 'history'))
+ PrefixData._cache_.clear()
+ run_command(Commands.UPDATE, prefix, "readline")
+ assert package_is_installed(prefix, "readline")
+ assert not package_is_installed(prefix, "readline-6.2")
+ assert package_is_installed(prefix, "python-2.7")
+ assert not package_is_installed(prefix, "python-2.7.12")
+
def test_remove_all(self):
- with make_temp_env("python=2") as prefix:
+ with make_temp_env("python") as prefix:
assert exists(join(prefix, PYTHON_BINARY))
- assert_package_is_installed(prefix, 'python-2')
+ assert_package_is_installed(prefix, 'python')
run_command(Commands.REMOVE, prefix, '--all')
assert not exists(prefix)
| conda 4.4 can fail to update packages
For example, start with a fresh install of Anaconda3, and then `conda update anaconda-client` doesn't update, even though a new anaconda-client package exists.
| 2018-02-22T19:08:48 | -1.0 |
Subsets and Splits