diff --git a/.gitignore b/.gitignore
new file mode 100644
index 0000000000000000000000000000000000000000..502b3e5ce775e77efe477ecf57625169543b9393
--- /dev/null
+++ b/.gitignore
@@ -0,0 +1,2 @@
+flagged/
+*.pt
\ No newline at end of file
diff --git a/Readme.md b/Readme.md
new file mode 100644
index 0000000000000000000000000000000000000000..3f9f8e14649929f87461a023184f80d5cacce640
--- /dev/null
+++ b/Readme.md
@@ -0,0 +1,4 @@
+
+
+1. Pip install Ultralytics: Yolov8 package
+- pip install ultralytics
\ No newline at end of file
diff --git a/app.py b/app.py
new file mode 100644
index 0000000000000000000000000000000000000000..5adfd3511d9b020a16303f2cc911b3935b9a2af2
--- /dev/null
+++ b/app.py
@@ -0,0 +1,116 @@
+from ultralytics import YOLO
+import cv2
+import gradio as gr
+import numpy as np
+import os
+import torch
+
+from image_segmenter import ImageSegmenter
+from monocular_depth_estimator import MonocularDepthEstimator
+
+# params
+CANCEL_PROCESSING = False
+
+img_seg = ImageSegmenter(model_type='n')
+depth_estimator = MonocularDepthEstimator(side_by_side=False)
+
+def process_image(image):
+ return img_seg.predict(image), depth_estimator.make_prediction(image)
+
+def process_video(vid_path=None):
+ vid_cap = cv2.VideoCapture(vid_path)
+ while vid_cap.isOpened():
+ ret, frame = vid_cap.read()
+ if ret:
+ print("making predictions ....")
+ yield cv2.cvtColor(img_seg.predict(frame), cv2.COLOR_BGR2RGB), depth_estimator.make_prediction(frame)
+
+ return None
+
+def update_segmentation_options(options):
+ img_seg.is_show_bounding_boxes = True if 'Show Boundary Box' in options else False
+ img_seg.is_show_segmentation = True if 'Show Segmentation Region' in options else False
+ img_seg.is_show_segmentation_boundary = True if 'Show Segmentation Boundary' in options else False
+
+def update_confidence_threshold(thres_val):
+ img_seg.confidence_threshold = thres_val/100
+
+def cancel():
+ CANCEL_PROCESSING = True
+
+if __name__ == "__main__":
+ # img_1 = cv2.imread("assets/images/bus.jpg")
+ # pred_img = image_segmentation(img_1)
+ # cv2.imshow("output", pred_img)
+ # cv2.waitKey(0)
+ # cv2.destroyAllWindows()
+
+ # gradio gui app
+ with gr.Blocks() as my_app:
+
+ # title
+ gr.Markdown(
+ """
+ # Object segmentation and depth estimation
+ Input an image or Video
+ """)
+
+ # tabs
+ with gr.Tab("Image"):
+ with gr.Row():
+ with gr.Column(scale=1):
+ img_input = gr.Image()
+ options_checkbox_img = gr.CheckboxGroup(["Show Boundary Box", "Show Segmentation Region", "Show Segmentation Boundary"], label="Options")
+ conf_thres_img = gr.Slider(1, 100, value=60, label="Confidence Threshold", info="Choose the threshold above which objects should be detected")
+ submit_btn_img = gr.Button(value="Predict")
+
+ with gr.Column(scale=2):
+ with gr.Row():
+ segmentation_img_output = gr.Image(height=300, label="Segmentation")
+ depth_img_output = gr.Image(height=300, label="Depth Estimation")
+
+ gr.Markdown("## Sample Images")
+ gr.Examples(
+ examples=[os.path.join(os.path.dirname(__file__), "assets/images/bus.jpg")],
+ inputs=img_input,
+ outputs=[segmentation_img_output, depth_img_output],
+ fn=process_image,
+ cache_examples=True,
+ )
+
+ with gr.Tab("Video"):
+ with gr.Row():
+ with gr.Column(scale=1):
+ vid_input = gr.Video()
+ options_checkbox_vid = gr.CheckboxGroup(["Show Boundary Box", "Show Segmentation Region", "Show Segmentation Boundary"], label="Options")
+ conf_thres_vid = gr.Slider(1, 100, value=60, label="Confidence Threshold", info="Choose the threshold above which objects should be detected")
+ with gr.Row():
+ cancel_btn = gr.Button(value="Cancel")
+ submit_btn_vid = gr.Button(value="Predict")
+
+ with gr.Column(scale=2):
+ with gr.Row():
+ segmentation_vid_output = gr.Image(height=400, label="Segmentation")
+ depth_vid_output = gr.Image(height=400, label="Depth Estimation")
+
+ gr.Markdown("## Sample Videos")
+ gr.Examples(
+ examples=[os.path.join(os.path.dirname(__file__), "assets/videos/input_video.mp4")],
+ inputs=vid_input,
+ # outputs=vid_output,
+ # fn=vid_segmenation,
+ )
+
+ # image tab logic
+ submit_btn_img.click(process_image, inputs=img_input, outputs=[segmentation_img_output, depth_img_output])
+ options_checkbox_img.change(update_segmentation_options, options_checkbox_img, [])
+ conf_thres_img.change(update_confidence_threshold, conf_thres_img, [])
+
+ # video tab logic
+ submit_btn_vid.click(process_video, inputs=vid_input, outputs=[segmentation_vid_output, depth_vid_output])
+ cancel_btn.click(cancel, inputs=[], outputs=[])
+ options_checkbox_vid.change(update_segmentation_options, options_checkbox_vid, [])
+ conf_thres_vid.change(update_confidence_threshold, conf_thres_vid, [])
+
+
+ my_app.queue(concurrency_count=5, max_size=20).launch()
\ No newline at end of file
diff --git a/image_segmenter.py b/image_segmenter.py
new file mode 100644
index 0000000000000000000000000000000000000000..64759441da26defe5b040454cef2bdfa69daa8b3
--- /dev/null
+++ b/image_segmenter.py
@@ -0,0 +1,91 @@
+import cv2
+import numpy as np
+from ultralytics import YOLO
+from ultralytics.yolo.utils.ops import scale_image
+import random
+import torch
+
+class ImageSegmenter:
+ def __init__(self, model_type="n") -> None:
+
+ self.device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
+ self.model = YOLO('models/yolov8'+ model_type +'-seg.pt')
+ self.model.to(self.device)
+
+ self.is_show_bounding_boxes = False
+ self.is_show_segmentation_boundary = False
+ self.is_show_segmentation = True
+ self.confidence_threshold = 0.5
+ self.cls_clr = {}
+
+ # params
+ self.bb_thickness = 2
+ self.bb_clr = (255, 0, 0)
+
+
+ def get_cls_clr(self, cls_id):
+ if cls_id in self.cls_clr:
+ return self.cls_clr[cls_id]
+
+ # gen rand color
+ r = random.randint(50, 200)
+ g = random.randint(50, 200)
+ b = random.randint(50, 200)
+ self.cls_clr[cls_id] = (r, g, b)
+ return (r, g, b)
+
+ def predict(self, image):
+ # resizing the image for faster prediction
+ image = cv2.resize(image, (480, 640))
+ predictions = self.model.predict(image)
+
+ cls_ids = predictions[0].boxes.cls.cpu().numpy()
+ bounding_boxes = predictions[0].boxes.xyxy.int().cpu().numpy()
+ cls_conf = predictions[0].boxes.conf.cpu().numpy()
+ # segmentation
+ if predictions[0].masks:
+ seg_mask_boundary = predictions[0].masks.xy
+ seg_mask = predictions[0].masks.data.cpu().numpy()
+ else:
+ seg_mask_boundary, seg_mask = [], np.array([])
+
+ for id, cls in enumerate(cls_ids):
+ cls_clr = self.get_cls_clr(cls)
+
+ # draw bounding box with class name and score
+ if self.is_show_bounding_boxes and cls_conf[id] > self.confidence_threshold:
+ (x1, y1, x2, y2) = bounding_boxes[id]
+ cls_name = self.model.names[cls]
+ cls_confidence = cls_conf[id]
+ disp_str = cls_name +' '+ str(round(cls_confidence, 2))
+ cv2.rectangle(image, (x1, y1), (x2, y2), cls_clr, self.bb_thickness)
+ cv2.rectangle(image, (x1, y1), (x1+(len(disp_str)*18), y1+45), cls_clr, -1)
+ cv2.putText(image, disp_str, (x1+10, y1+30), cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 255, 255), 2)
+
+
+ # draw segmentation boundary
+ if len(seg_mask_boundary) and self.is_show_segmentation_boundary and cls_conf[id] > self.confidence_threshold:
+ cv2.polylines(image, [np.array(seg_mask_boundary[id], dtype=np.int32)], isClosed=True, color=cls_clr, thickness=2)
+
+ # draw filled segmentation region
+ if seg_mask.any() and self.is_show_segmentation and cls_conf[id] > self.confidence_threshold:
+ alpha = 0.8
+
+ # converting the mask from 1 channel to 3 channels
+ colored_mask = np.expand_dims(seg_mask[id], 0).repeat(3, axis=0)
+ colored_mask = np.moveaxis(colored_mask, 0, -1)
+
+ # Resize the mask to match the image size, if necessary
+ if image.shape[:2] != seg_mask[id].shape[:2]:
+ colored_mask = cv2.resize(colored_mask, (image.shape[1], image.shape[0]))
+
+ # filling the mased area with class color
+ masked = np.ma.MaskedArray(image, mask=colored_mask, fill_value=cls_clr)
+ image_overlay = masked.filled()
+ image = cv2.addWeighted(image, 1 - alpha, image_overlay, alpha, 0)
+
+ return image
+
+
+
+
\ No newline at end of file
diff --git a/midas/__init__.py b/midas/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/midas/__pycache__/__init__.cpython-38.pyc b/midas/__pycache__/__init__.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..95265f87e46ecdd3979ddc93da5bd2dd641fb752
Binary files /dev/null and b/midas/__pycache__/__init__.cpython-38.pyc differ
diff --git a/midas/__pycache__/base_model.cpython-37.pyc b/midas/__pycache__/base_model.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..95a6e86a07842c983a886e4fb7dc305df49e211c
Binary files /dev/null and b/midas/__pycache__/base_model.cpython-37.pyc differ
diff --git a/midas/__pycache__/base_model.cpython-38.pyc b/midas/__pycache__/base_model.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..4cbad7d5c77d8da80fc8e288d59015d578d91c9f
Binary files /dev/null and b/midas/__pycache__/base_model.cpython-38.pyc differ
diff --git a/midas/__pycache__/blocks.cpython-37.pyc b/midas/__pycache__/blocks.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..8d1c03785fbdf76eb1a820eed93b2caf3448ba4b
Binary files /dev/null and b/midas/__pycache__/blocks.cpython-37.pyc differ
diff --git a/midas/__pycache__/blocks.cpython-38.pyc b/midas/__pycache__/blocks.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..68f9ded98120be1562a7c2b726968cf112049697
Binary files /dev/null and b/midas/__pycache__/blocks.cpython-38.pyc differ
diff --git a/midas/__pycache__/dpt_depth.cpython-37.pyc b/midas/__pycache__/dpt_depth.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..0bd740418ca7413b616794f5481d29bc277f9418
Binary files /dev/null and b/midas/__pycache__/dpt_depth.cpython-37.pyc differ
diff --git a/midas/__pycache__/dpt_depth.cpython-38.pyc b/midas/__pycache__/dpt_depth.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..8effb96da94a03f720230b7100326134c79ac24c
Binary files /dev/null and b/midas/__pycache__/dpt_depth.cpython-38.pyc differ
diff --git a/midas/__pycache__/midas_net.cpython-37.pyc b/midas/__pycache__/midas_net.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..8f3f1259828fc31d45a8da9aa1f7aba9343133df
Binary files /dev/null and b/midas/__pycache__/midas_net.cpython-37.pyc differ
diff --git a/midas/__pycache__/midas_net.cpython-38.pyc b/midas/__pycache__/midas_net.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..5bc547b310a485c5651bd891f472aba70c014372
Binary files /dev/null and b/midas/__pycache__/midas_net.cpython-38.pyc differ
diff --git a/midas/__pycache__/midas_net_custom.cpython-37.pyc b/midas/__pycache__/midas_net_custom.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..1eae32c38e2ea8e808b55008c71b033936ef34e3
Binary files /dev/null and b/midas/__pycache__/midas_net_custom.cpython-37.pyc differ
diff --git a/midas/__pycache__/midas_net_custom.cpython-38.pyc b/midas/__pycache__/midas_net_custom.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..47d18249138eba5eba4cd17c4351778c5994eeb1
Binary files /dev/null and b/midas/__pycache__/midas_net_custom.cpython-38.pyc differ
diff --git a/midas/__pycache__/model_loader.cpython-37.pyc b/midas/__pycache__/model_loader.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..c85032683d04a69caccd91edef72855f2d4efe81
Binary files /dev/null and b/midas/__pycache__/model_loader.cpython-37.pyc differ
diff --git a/midas/__pycache__/model_loader.cpython-38.pyc b/midas/__pycache__/model_loader.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..15758e6bbccd17d6c33c5983e6919288f4925340
Binary files /dev/null and b/midas/__pycache__/model_loader.cpython-38.pyc differ
diff --git a/midas/__pycache__/transforms.cpython-37.pyc b/midas/__pycache__/transforms.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..195a43db7435d97219d771d67baac62d4f3c9ff7
Binary files /dev/null and b/midas/__pycache__/transforms.cpython-37.pyc differ
diff --git a/midas/__pycache__/transforms.cpython-38.pyc b/midas/__pycache__/transforms.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..2f41226a3513e0a8874bfcaf8d2f442115bc5e78
Binary files /dev/null and b/midas/__pycache__/transforms.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/beit.cpython-37.pyc b/midas/backbones/__pycache__/beit.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..ea234bac7680fb9b94491059f4805677cea8bb5d
Binary files /dev/null and b/midas/backbones/__pycache__/beit.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/beit.cpython-38.pyc b/midas/backbones/__pycache__/beit.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..00bd93d798f6fb2fcb342f9cedeb2faf1ae48c1a
Binary files /dev/null and b/midas/backbones/__pycache__/beit.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/levit.cpython-37.pyc b/midas/backbones/__pycache__/levit.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..84aeb88874b69eff83527b24d45b81e1f8803169
Binary files /dev/null and b/midas/backbones/__pycache__/levit.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/levit.cpython-38.pyc b/midas/backbones/__pycache__/levit.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..ef6f47d13d31bf54a5df6b259742aadf4af7a6c6
Binary files /dev/null and b/midas/backbones/__pycache__/levit.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/swin.cpython-37.pyc b/midas/backbones/__pycache__/swin.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..1be58fd4da96c093385c3af8db0bb375cba2938a
Binary files /dev/null and b/midas/backbones/__pycache__/swin.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/swin.cpython-38.pyc b/midas/backbones/__pycache__/swin.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..907351997b5ec68b872cb77662030ec241efa5d1
Binary files /dev/null and b/midas/backbones/__pycache__/swin.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/swin2.cpython-37.pyc b/midas/backbones/__pycache__/swin2.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..8f5e8f304d8da9b9eb597798b312bf87d4b2e3ea
Binary files /dev/null and b/midas/backbones/__pycache__/swin2.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/swin2.cpython-38.pyc b/midas/backbones/__pycache__/swin2.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..51e569ea9bcbd1d67284b53f561115928544646d
Binary files /dev/null and b/midas/backbones/__pycache__/swin2.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/swin_common.cpython-37.pyc b/midas/backbones/__pycache__/swin_common.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..a47f3ee58e5c1d99bffd709aa6a731122c215532
Binary files /dev/null and b/midas/backbones/__pycache__/swin_common.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/swin_common.cpython-38.pyc b/midas/backbones/__pycache__/swin_common.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..96b6fdde103e5f8c27df135d0ad5d0f34f120b83
Binary files /dev/null and b/midas/backbones/__pycache__/swin_common.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/utils.cpython-37.pyc b/midas/backbones/__pycache__/utils.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..af99252cd068ebdcc42fd8df46c3ea8d37816c01
Binary files /dev/null and b/midas/backbones/__pycache__/utils.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/utils.cpython-38.pyc b/midas/backbones/__pycache__/utils.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..e3cac5ac2dda18ff895660ca7f19b63a0feee23e
Binary files /dev/null and b/midas/backbones/__pycache__/utils.cpython-38.pyc differ
diff --git a/midas/backbones/__pycache__/vit.cpython-37.pyc b/midas/backbones/__pycache__/vit.cpython-37.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..07d82e4e93da98925863d9bc3732733c8a78e2a3
Binary files /dev/null and b/midas/backbones/__pycache__/vit.cpython-37.pyc differ
diff --git a/midas/backbones/__pycache__/vit.cpython-38.pyc b/midas/backbones/__pycache__/vit.cpython-38.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..fb1dff1dd4505d753171bcb786155b13dfd63aab
Binary files /dev/null and b/midas/backbones/__pycache__/vit.cpython-38.pyc differ
diff --git a/midas/backbones/beit.py b/midas/backbones/beit.py
new file mode 100644
index 0000000000000000000000000000000000000000..7a24e02cd2b979844bf638b46ac60949ee9ce691
--- /dev/null
+++ b/midas/backbones/beit.py
@@ -0,0 +1,196 @@
+import timm
+import torch
+import types
+
+import numpy as np
+import torch.nn.functional as F
+
+from .utils import forward_adapted_unflatten, make_backbone_default
+from timm.models.beit import gen_relative_position_index
+from torch.utils.checkpoint import checkpoint
+from typing import Optional
+
+
+def forward_beit(pretrained, x):
+ return forward_adapted_unflatten(pretrained, x, "forward_features")
+
+
+def patch_embed_forward(self, x):
+ """
+ Modification of timm.models.layers.patch_embed.py: PatchEmbed.forward to support arbitrary window sizes.
+ """
+ x = self.proj(x)
+ if self.flatten:
+ x = x.flatten(2).transpose(1, 2)
+ x = self.norm(x)
+ return x
+
+
+def _get_rel_pos_bias(self, window_size):
+ """
+ Modification of timm.models.beit.py: Attention._get_rel_pos_bias to support arbitrary window sizes.
+ """
+ old_height = 2 * self.window_size[0] - 1
+ old_width = 2 * self.window_size[1] - 1
+
+ new_height = 2 * window_size[0] - 1
+ new_width = 2 * window_size[1] - 1
+
+ old_relative_position_bias_table = self.relative_position_bias_table
+
+ old_num_relative_distance = self.num_relative_distance
+ new_num_relative_distance = new_height * new_width + 3
+
+ old_sub_table = old_relative_position_bias_table[:old_num_relative_distance - 3]
+
+ old_sub_table = old_sub_table.reshape(1, old_width, old_height, -1).permute(0, 3, 1, 2)
+ new_sub_table = F.interpolate(old_sub_table, size=(new_height, new_width), mode="bilinear")
+ new_sub_table = new_sub_table.permute(0, 2, 3, 1).reshape(new_num_relative_distance - 3, -1)
+
+ new_relative_position_bias_table = torch.cat(
+ [new_sub_table, old_relative_position_bias_table[old_num_relative_distance - 3:]])
+
+ key = str(window_size[1]) + "," + str(window_size[0])
+ if key not in self.relative_position_indices.keys():
+ self.relative_position_indices[key] = gen_relative_position_index(window_size)
+
+ relative_position_bias = new_relative_position_bias_table[
+ self.relative_position_indices[key].view(-1)].view(
+ window_size[0] * window_size[1] + 1,
+ window_size[0] * window_size[1] + 1, -1) # Wh*Ww,Wh*Ww,nH
+ relative_position_bias = relative_position_bias.permute(2, 0, 1).contiguous() # nH, Wh*Ww, Wh*Ww
+ return relative_position_bias.unsqueeze(0)
+
+
+def attention_forward(self, x, resolution, shared_rel_pos_bias: Optional[torch.Tensor] = None):
+ """
+ Modification of timm.models.beit.py: Attention.forward to support arbitrary window sizes.
+ """
+ B, N, C = x.shape
+
+ qkv_bias = torch.cat((self.q_bias, self.k_bias, self.v_bias)) if self.q_bias is not None else None
+ qkv = F.linear(input=x, weight=self.qkv.weight, bias=qkv_bias)
+ qkv = qkv.reshape(B, N, 3, self.num_heads, -1).permute(2, 0, 3, 1, 4)
+ q, k, v = qkv.unbind(0) # make torchscript happy (cannot use tensor as tuple)
+
+ q = q * self.scale
+ attn = (q @ k.transpose(-2, -1))
+
+ if self.relative_position_bias_table is not None:
+ window_size = tuple(np.array(resolution) // 16)
+ attn = attn + self._get_rel_pos_bias(window_size)
+ if shared_rel_pos_bias is not None:
+ attn = attn + shared_rel_pos_bias
+
+ attn = attn.softmax(dim=-1)
+ attn = self.attn_drop(attn)
+
+ x = (attn @ v).transpose(1, 2).reshape(B, N, -1)
+ x = self.proj(x)
+ x = self.proj_drop(x)
+ return x
+
+
+def block_forward(self, x, resolution, shared_rel_pos_bias: Optional[torch.Tensor] = None):
+ """
+ Modification of timm.models.beit.py: Block.forward to support arbitrary window sizes.
+ """
+ if self.gamma_1 is None:
+ x = x + self.drop_path(self.attn(self.norm1(x), resolution, shared_rel_pos_bias=shared_rel_pos_bias))
+ x = x + self.drop_path(self.mlp(self.norm2(x)))
+ else:
+ x = x + self.drop_path(self.gamma_1 * self.attn(self.norm1(x), resolution,
+ shared_rel_pos_bias=shared_rel_pos_bias))
+ x = x + self.drop_path(self.gamma_2 * self.mlp(self.norm2(x)))
+ return x
+
+
+def beit_forward_features(self, x):
+ """
+ Modification of timm.models.beit.py: Beit.forward_features to support arbitrary window sizes.
+ """
+ resolution = x.shape[2:]
+
+ x = self.patch_embed(x)
+ x = torch.cat((self.cls_token.expand(x.shape[0], -1, -1), x), dim=1)
+ if self.pos_embed is not None:
+ x = x + self.pos_embed
+ x = self.pos_drop(x)
+
+ rel_pos_bias = self.rel_pos_bias() if self.rel_pos_bias is not None else None
+ for blk in self.blocks:
+ if self.grad_checkpointing and not torch.jit.is_scripting():
+ x = checkpoint(blk, x, shared_rel_pos_bias=rel_pos_bias)
+ else:
+ x = blk(x, resolution, shared_rel_pos_bias=rel_pos_bias)
+ x = self.norm(x)
+ return x
+
+
+def _make_beit_backbone(
+ model,
+ features=[96, 192, 384, 768],
+ size=[384, 384],
+ hooks=[0, 4, 8, 11],
+ vit_features=768,
+ use_readout="ignore",
+ start_index=1,
+ start_index_readout=1,
+):
+ backbone = make_backbone_default(model, features, size, hooks, vit_features, use_readout, start_index,
+ start_index_readout)
+
+ backbone.model.patch_embed.forward = types.MethodType(patch_embed_forward, backbone.model.patch_embed)
+ backbone.model.forward_features = types.MethodType(beit_forward_features, backbone.model)
+
+ for block in backbone.model.blocks:
+ attn = block.attn
+ attn._get_rel_pos_bias = types.MethodType(_get_rel_pos_bias, attn)
+ attn.forward = types.MethodType(attention_forward, attn)
+ attn.relative_position_indices = {}
+
+ block.forward = types.MethodType(block_forward, block)
+
+ return backbone
+
+
+def _make_pretrained_beitl16_512(pretrained, use_readout="ignore", hooks=None):
+ model = timm.create_model("beit_large_patch16_512", pretrained=pretrained)
+
+ hooks = [5, 11, 17, 23] if hooks is None else hooks
+
+ features = [256, 512, 1024, 1024]
+
+ return _make_beit_backbone(
+ model,
+ features=features,
+ size=[512, 512],
+ hooks=hooks,
+ vit_features=1024,
+ use_readout=use_readout,
+ )
+
+
+def _make_pretrained_beitl16_384(pretrained, use_readout="ignore", hooks=None):
+ model = timm.create_model("beit_large_patch16_384", pretrained=pretrained)
+
+ hooks = [5, 11, 17, 23] if hooks is None else hooks
+ return _make_beit_backbone(
+ model,
+ features=[256, 512, 1024, 1024],
+ hooks=hooks,
+ vit_features=1024,
+ use_readout=use_readout,
+ )
+
+
+def _make_pretrained_beitb16_384(pretrained, use_readout="ignore", hooks=None):
+ model = timm.create_model("beit_base_patch16_384", pretrained=pretrained)
+
+ hooks = [2, 5, 8, 11] if hooks is None else hooks
+ return _make_beit_backbone(
+ model,
+ features=[96, 192, 384, 768],
+ hooks=hooks,
+ use_readout=use_readout,
+ )
diff --git a/midas/backbones/levit.py b/midas/backbones/levit.py
new file mode 100644
index 0000000000000000000000000000000000000000..6d023a98702a0451806d26f33f8bccf931814f10
--- /dev/null
+++ b/midas/backbones/levit.py
@@ -0,0 +1,106 @@
+import timm
+import torch
+import torch.nn as nn
+import numpy as np
+
+from .utils import activations, get_activation, Transpose
+
+
+def forward_levit(pretrained, x):
+ pretrained.model.forward_features(x)
+
+ layer_1 = pretrained.activations["1"]
+ layer_2 = pretrained.activations["2"]
+ layer_3 = pretrained.activations["3"]
+
+ layer_1 = pretrained.act_postprocess1(layer_1)
+ layer_2 = pretrained.act_postprocess2(layer_2)
+ layer_3 = pretrained.act_postprocess3(layer_3)
+
+ return layer_1, layer_2, layer_3
+
+
+def _make_levit_backbone(
+ model,
+ hooks=[3, 11, 21],
+ patch_grid=[14, 14]
+):
+ pretrained = nn.Module()
+
+ pretrained.model = model
+ pretrained.model.blocks[hooks[0]].register_forward_hook(get_activation("1"))
+ pretrained.model.blocks[hooks[1]].register_forward_hook(get_activation("2"))
+ pretrained.model.blocks[hooks[2]].register_forward_hook(get_activation("3"))
+
+ pretrained.activations = activations
+
+ patch_grid_size = np.array(patch_grid, dtype=int)
+
+ pretrained.act_postprocess1 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size(patch_grid_size.tolist()))
+ )
+ pretrained.act_postprocess2 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size((np.ceil(patch_grid_size / 2).astype(int)).tolist()))
+ )
+ pretrained.act_postprocess3 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size((np.ceil(patch_grid_size / 4).astype(int)).tolist()))
+ )
+
+ return pretrained
+
+
+class ConvTransposeNorm(nn.Sequential):
+ """
+ Modification of
+ https://github.com/rwightman/pytorch-image-models/blob/master/timm/models/levit.py: ConvNorm
+ such that ConvTranspose2d is used instead of Conv2d.
+ """
+
+ def __init__(
+ self, in_chs, out_chs, kernel_size=1, stride=1, pad=0, dilation=1,
+ groups=1, bn_weight_init=1):
+ super().__init__()
+ self.add_module('c',
+ nn.ConvTranspose2d(in_chs, out_chs, kernel_size, stride, pad, dilation, groups, bias=False))
+ self.add_module('bn', nn.BatchNorm2d(out_chs))
+
+ nn.init.constant_(self.bn.weight, bn_weight_init)
+
+ @torch.no_grad()
+ def fuse(self):
+ c, bn = self._modules.values()
+ w = bn.weight / (bn.running_var + bn.eps) ** 0.5
+ w = c.weight * w[:, None, None, None]
+ b = bn.bias - bn.running_mean * bn.weight / (bn.running_var + bn.eps) ** 0.5
+ m = nn.ConvTranspose2d(
+ w.size(1), w.size(0), w.shape[2:], stride=self.c.stride,
+ padding=self.c.padding, dilation=self.c.dilation, groups=self.c.groups)
+ m.weight.data.copy_(w)
+ m.bias.data.copy_(b)
+ return m
+
+
+def stem_b4_transpose(in_chs, out_chs, activation):
+ """
+ Modification of
+ https://github.com/rwightman/pytorch-image-models/blob/master/timm/models/levit.py: stem_b16
+ such that ConvTranspose2d is used instead of Conv2d and stem is also reduced to the half.
+ """
+ return nn.Sequential(
+ ConvTransposeNorm(in_chs, out_chs, 3, 2, 1),
+ activation(),
+ ConvTransposeNorm(out_chs, out_chs // 2, 3, 2, 1),
+ activation())
+
+
+def _make_pretrained_levit_384(pretrained, hooks=None):
+ model = timm.create_model("levit_384", pretrained=pretrained)
+
+ hooks = [3, 11, 21] if hooks == None else hooks
+ return _make_levit_backbone(
+ model,
+ hooks=hooks
+ )
diff --git a/midas/backbones/next_vit.py b/midas/backbones/next_vit.py
new file mode 100644
index 0000000000000000000000000000000000000000..8afdd8b743b5ab023a359dc3b721e601b1a40d11
--- /dev/null
+++ b/midas/backbones/next_vit.py
@@ -0,0 +1,39 @@
+import timm
+
+import torch.nn as nn
+
+from pathlib import Path
+from .utils import activations, forward_default, get_activation
+
+from ..external.next_vit.classification.nextvit import *
+
+
+def forward_next_vit(pretrained, x):
+ return forward_default(pretrained, x, "forward")
+
+
+def _make_next_vit_backbone(
+ model,
+ hooks=[2, 6, 36, 39],
+):
+ pretrained = nn.Module()
+
+ pretrained.model = model
+ pretrained.model.features[hooks[0]].register_forward_hook(get_activation("1"))
+ pretrained.model.features[hooks[1]].register_forward_hook(get_activation("2"))
+ pretrained.model.features[hooks[2]].register_forward_hook(get_activation("3"))
+ pretrained.model.features[hooks[3]].register_forward_hook(get_activation("4"))
+
+ pretrained.activations = activations
+
+ return pretrained
+
+
+def _make_pretrained_next_vit_large_6m(hooks=None):
+ model = timm.create_model("nextvit_large")
+
+ hooks = [2, 6, 36, 39] if hooks == None else hooks
+ return _make_next_vit_backbone(
+ model,
+ hooks=hooks,
+ )
diff --git a/midas/backbones/swin.py b/midas/backbones/swin.py
new file mode 100644
index 0000000000000000000000000000000000000000..f8c71367e3e78b087f80b2ab3e2f495a9c372f1a
--- /dev/null
+++ b/midas/backbones/swin.py
@@ -0,0 +1,13 @@
+import timm
+
+from .swin_common import _make_swin_backbone
+
+
+def _make_pretrained_swinl12_384(pretrained, hooks=None):
+ model = timm.create_model("swin_large_patch4_window12_384", pretrained=pretrained)
+
+ hooks = [1, 1, 17, 1] if hooks == None else hooks
+ return _make_swin_backbone(
+ model,
+ hooks=hooks
+ )
diff --git a/midas/backbones/swin2.py b/midas/backbones/swin2.py
new file mode 100644
index 0000000000000000000000000000000000000000..ce4c8f1d6fc1807a207dc6b9a261c6f7b14a87a3
--- /dev/null
+++ b/midas/backbones/swin2.py
@@ -0,0 +1,34 @@
+import timm
+
+from .swin_common import _make_swin_backbone
+
+
+def _make_pretrained_swin2l24_384(pretrained, hooks=None):
+ model = timm.create_model("swinv2_large_window12to24_192to384_22kft1k", pretrained=pretrained)
+
+ hooks = [1, 1, 17, 1] if hooks == None else hooks
+ return _make_swin_backbone(
+ model,
+ hooks=hooks
+ )
+
+
+def _make_pretrained_swin2b24_384(pretrained, hooks=None):
+ model = timm.create_model("swinv2_base_window12to24_192to384_22kft1k", pretrained=pretrained)
+
+ hooks = [1, 1, 17, 1] if hooks == None else hooks
+ return _make_swin_backbone(
+ model,
+ hooks=hooks
+ )
+
+
+def _make_pretrained_swin2t16_256(pretrained, hooks=None):
+ model = timm.create_model("swinv2_tiny_window16_256", pretrained=pretrained)
+
+ hooks = [1, 1, 5, 1] if hooks == None else hooks
+ return _make_swin_backbone(
+ model,
+ hooks=hooks,
+ patch_grid=[64, 64]
+ )
diff --git a/midas/backbones/swin_common.py b/midas/backbones/swin_common.py
new file mode 100644
index 0000000000000000000000000000000000000000..94d63d408f18511179d90b3ac6f697385d1e556d
--- /dev/null
+++ b/midas/backbones/swin_common.py
@@ -0,0 +1,52 @@
+import torch
+
+import torch.nn as nn
+import numpy as np
+
+from .utils import activations, forward_default, get_activation, Transpose
+
+
+def forward_swin(pretrained, x):
+ return forward_default(pretrained, x)
+
+
+def _make_swin_backbone(
+ model,
+ hooks=[1, 1, 17, 1],
+ patch_grid=[96, 96]
+):
+ pretrained = nn.Module()
+
+ pretrained.model = model
+ pretrained.model.layers[0].blocks[hooks[0]].register_forward_hook(get_activation("1"))
+ pretrained.model.layers[1].blocks[hooks[1]].register_forward_hook(get_activation("2"))
+ pretrained.model.layers[2].blocks[hooks[2]].register_forward_hook(get_activation("3"))
+ pretrained.model.layers[3].blocks[hooks[3]].register_forward_hook(get_activation("4"))
+
+ pretrained.activations = activations
+
+ if hasattr(model, "patch_grid"):
+ used_patch_grid = model.patch_grid
+ else:
+ used_patch_grid = patch_grid
+
+ patch_grid_size = np.array(used_patch_grid, dtype=int)
+
+ pretrained.act_postprocess1 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size(patch_grid_size.tolist()))
+ )
+ pretrained.act_postprocess2 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size((patch_grid_size // 2).tolist()))
+ )
+ pretrained.act_postprocess3 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size((patch_grid_size // 4).tolist()))
+ )
+ pretrained.act_postprocess4 = nn.Sequential(
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size((patch_grid_size // 8).tolist()))
+ )
+
+ return pretrained
diff --git a/midas/backbones/utils.py b/midas/backbones/utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..0558899dddcfccec5f01a764d4f21738eb612149
--- /dev/null
+++ b/midas/backbones/utils.py
@@ -0,0 +1,249 @@
+import torch
+
+import torch.nn as nn
+
+
+class Slice(nn.Module):
+ def __init__(self, start_index=1):
+ super(Slice, self).__init__()
+ self.start_index = start_index
+
+ def forward(self, x):
+ return x[:, self.start_index:]
+
+
+class AddReadout(nn.Module):
+ def __init__(self, start_index=1):
+ super(AddReadout, self).__init__()
+ self.start_index = start_index
+
+ def forward(self, x):
+ if self.start_index == 2:
+ readout = (x[:, 0] + x[:, 1]) / 2
+ else:
+ readout = x[:, 0]
+ return x[:, self.start_index:] + readout.unsqueeze(1)
+
+
+class ProjectReadout(nn.Module):
+ def __init__(self, in_features, start_index=1):
+ super(ProjectReadout, self).__init__()
+ self.start_index = start_index
+
+ self.project = nn.Sequential(nn.Linear(2 * in_features, in_features), nn.GELU())
+
+ def forward(self, x):
+ readout = x[:, 0].unsqueeze(1).expand_as(x[:, self.start_index:])
+ features = torch.cat((x[:, self.start_index:], readout), -1)
+
+ return self.project(features)
+
+
+class Transpose(nn.Module):
+ def __init__(self, dim0, dim1):
+ super(Transpose, self).__init__()
+ self.dim0 = dim0
+ self.dim1 = dim1
+
+ def forward(self, x):
+ x = x.transpose(self.dim0, self.dim1)
+ return x
+
+
+activations = {}
+
+
+def get_activation(name):
+ def hook(model, input, output):
+ activations[name] = output
+
+ return hook
+
+
+def forward_default(pretrained, x, function_name="forward_features"):
+ exec(f"pretrained.model.{function_name}(x)")
+
+ layer_1 = pretrained.activations["1"]
+ layer_2 = pretrained.activations["2"]
+ layer_3 = pretrained.activations["3"]
+ layer_4 = pretrained.activations["4"]
+
+ if hasattr(pretrained, "act_postprocess1"):
+ layer_1 = pretrained.act_postprocess1(layer_1)
+ if hasattr(pretrained, "act_postprocess2"):
+ layer_2 = pretrained.act_postprocess2(layer_2)
+ if hasattr(pretrained, "act_postprocess3"):
+ layer_3 = pretrained.act_postprocess3(layer_3)
+ if hasattr(pretrained, "act_postprocess4"):
+ layer_4 = pretrained.act_postprocess4(layer_4)
+
+ return layer_1, layer_2, layer_3, layer_4
+
+
+def forward_adapted_unflatten(pretrained, x, function_name="forward_features"):
+ b, c, h, w = x.shape
+
+ exec(f"glob = pretrained.model.{function_name}(x)")
+
+ layer_1 = pretrained.activations["1"]
+ layer_2 = pretrained.activations["2"]
+ layer_3 = pretrained.activations["3"]
+ layer_4 = pretrained.activations["4"]
+
+ layer_1 = pretrained.act_postprocess1[0:2](layer_1)
+ layer_2 = pretrained.act_postprocess2[0:2](layer_2)
+ layer_3 = pretrained.act_postprocess3[0:2](layer_3)
+ layer_4 = pretrained.act_postprocess4[0:2](layer_4)
+
+ unflatten = nn.Sequential(
+ nn.Unflatten(
+ 2,
+ torch.Size(
+ [
+ h // pretrained.model.patch_size[1],
+ w // pretrained.model.patch_size[0],
+ ]
+ ),
+ )
+ )
+
+ if layer_1.ndim == 3:
+ layer_1 = unflatten(layer_1)
+ if layer_2.ndim == 3:
+ layer_2 = unflatten(layer_2)
+ if layer_3.ndim == 3:
+ layer_3 = unflatten(layer_3)
+ if layer_4.ndim == 3:
+ layer_4 = unflatten(layer_4)
+
+ layer_1 = pretrained.act_postprocess1[3: len(pretrained.act_postprocess1)](layer_1)
+ layer_2 = pretrained.act_postprocess2[3: len(pretrained.act_postprocess2)](layer_2)
+ layer_3 = pretrained.act_postprocess3[3: len(pretrained.act_postprocess3)](layer_3)
+ layer_4 = pretrained.act_postprocess4[3: len(pretrained.act_postprocess4)](layer_4)
+
+ return layer_1, layer_2, layer_3, layer_4
+
+
+def get_readout_oper(vit_features, features, use_readout, start_index=1):
+ if use_readout == "ignore":
+ readout_oper = [Slice(start_index)] * len(features)
+ elif use_readout == "add":
+ readout_oper = [AddReadout(start_index)] * len(features)
+ elif use_readout == "project":
+ readout_oper = [
+ ProjectReadout(vit_features, start_index) for out_feat in features
+ ]
+ else:
+ assert (
+ False
+ ), "wrong operation for readout token, use_readout can be 'ignore', 'add', or 'project'"
+
+ return readout_oper
+
+
+def make_backbone_default(
+ model,
+ features=[96, 192, 384, 768],
+ size=[384, 384],
+ hooks=[2, 5, 8, 11],
+ vit_features=768,
+ use_readout="ignore",
+ start_index=1,
+ start_index_readout=1,
+):
+ pretrained = nn.Module()
+
+ pretrained.model = model
+ pretrained.model.blocks[hooks[0]].register_forward_hook(get_activation("1"))
+ pretrained.model.blocks[hooks[1]].register_forward_hook(get_activation("2"))
+ pretrained.model.blocks[hooks[2]].register_forward_hook(get_activation("3"))
+ pretrained.model.blocks[hooks[3]].register_forward_hook(get_activation("4"))
+
+ pretrained.activations = activations
+
+ readout_oper = get_readout_oper(vit_features, features, use_readout, start_index_readout)
+
+ # 32, 48, 136, 384
+ pretrained.act_postprocess1 = nn.Sequential(
+ readout_oper[0],
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size([size[0] // 16, size[1] // 16])),
+ nn.Conv2d(
+ in_channels=vit_features,
+ out_channels=features[0],
+ kernel_size=1,
+ stride=1,
+ padding=0,
+ ),
+ nn.ConvTranspose2d(
+ in_channels=features[0],
+ out_channels=features[0],
+ kernel_size=4,
+ stride=4,
+ padding=0,
+ bias=True,
+ dilation=1,
+ groups=1,
+ ),
+ )
+
+ pretrained.act_postprocess2 = nn.Sequential(
+ readout_oper[1],
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size([size[0] // 16, size[1] // 16])),
+ nn.Conv2d(
+ in_channels=vit_features,
+ out_channels=features[1],
+ kernel_size=1,
+ stride=1,
+ padding=0,
+ ),
+ nn.ConvTranspose2d(
+ in_channels=features[1],
+ out_channels=features[1],
+ kernel_size=2,
+ stride=2,
+ padding=0,
+ bias=True,
+ dilation=1,
+ groups=1,
+ ),
+ )
+
+ pretrained.act_postprocess3 = nn.Sequential(
+ readout_oper[2],
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size([size[0] // 16, size[1] // 16])),
+ nn.Conv2d(
+ in_channels=vit_features,
+ out_channels=features[2],
+ kernel_size=1,
+ stride=1,
+ padding=0,
+ ),
+ )
+
+ pretrained.act_postprocess4 = nn.Sequential(
+ readout_oper[3],
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size([size[0] // 16, size[1] // 16])),
+ nn.Conv2d(
+ in_channels=vit_features,
+ out_channels=features[3],
+ kernel_size=1,
+ stride=1,
+ padding=0,
+ ),
+ nn.Conv2d(
+ in_channels=features[3],
+ out_channels=features[3],
+ kernel_size=3,
+ stride=2,
+ padding=1,
+ ),
+ )
+
+ pretrained.model.start_index = start_index
+ pretrained.model.patch_size = [16, 16]
+
+ return pretrained
diff --git a/midas/backbones/vit.py b/midas/backbones/vit.py
new file mode 100644
index 0000000000000000000000000000000000000000..413f9693bd4548342280e329c9128c1a52cea920
--- /dev/null
+++ b/midas/backbones/vit.py
@@ -0,0 +1,221 @@
+import torch
+import torch.nn as nn
+import timm
+import types
+import math
+import torch.nn.functional as F
+
+from .utils import (activations, forward_adapted_unflatten, get_activation, get_readout_oper,
+ make_backbone_default, Transpose)
+
+
+def forward_vit(pretrained, x):
+ return forward_adapted_unflatten(pretrained, x, "forward_flex")
+
+
+def _resize_pos_embed(self, posemb, gs_h, gs_w):
+ posemb_tok, posemb_grid = (
+ posemb[:, : self.start_index],
+ posemb[0, self.start_index:],
+ )
+
+ gs_old = int(math.sqrt(len(posemb_grid)))
+
+ posemb_grid = posemb_grid.reshape(1, gs_old, gs_old, -1).permute(0, 3, 1, 2)
+ posemb_grid = F.interpolate(posemb_grid, size=(gs_h, gs_w), mode="bilinear")
+ posemb_grid = posemb_grid.permute(0, 2, 3, 1).reshape(1, gs_h * gs_w, -1)
+
+ posemb = torch.cat([posemb_tok, posemb_grid], dim=1)
+
+ return posemb
+
+
+def forward_flex(self, x):
+ b, c, h, w = x.shape
+
+ pos_embed = self._resize_pos_embed(
+ self.pos_embed, h // self.patch_size[1], w // self.patch_size[0]
+ )
+
+ B = x.shape[0]
+
+ if hasattr(self.patch_embed, "backbone"):
+ x = self.patch_embed.backbone(x)
+ if isinstance(x, (list, tuple)):
+ x = x[-1] # last feature if backbone outputs list/tuple of features
+
+ x = self.patch_embed.proj(x).flatten(2).transpose(1, 2)
+
+ if getattr(self, "dist_token", None) is not None:
+ cls_tokens = self.cls_token.expand(
+ B, -1, -1
+ ) # stole cls_tokens impl from Phil Wang, thanks
+ dist_token = self.dist_token.expand(B, -1, -1)
+ x = torch.cat((cls_tokens, dist_token, x), dim=1)
+ else:
+ if self.no_embed_class:
+ x = x + pos_embed
+ cls_tokens = self.cls_token.expand(
+ B, -1, -1
+ ) # stole cls_tokens impl from Phil Wang, thanks
+ x = torch.cat((cls_tokens, x), dim=1)
+
+ if not self.no_embed_class:
+ x = x + pos_embed
+ x = self.pos_drop(x)
+
+ for blk in self.blocks:
+ x = blk(x)
+
+ x = self.norm(x)
+
+ return x
+
+
+def _make_vit_b16_backbone(
+ model,
+ features=[96, 192, 384, 768],
+ size=[384, 384],
+ hooks=[2, 5, 8, 11],
+ vit_features=768,
+ use_readout="ignore",
+ start_index=1,
+ start_index_readout=1,
+):
+ pretrained = make_backbone_default(model, features, size, hooks, vit_features, use_readout, start_index,
+ start_index_readout)
+
+ # We inject this function into the VisionTransformer instances so that
+ # we can use it with interpolated position embeddings without modifying the library source.
+ pretrained.model.forward_flex = types.MethodType(forward_flex, pretrained.model)
+ pretrained.model._resize_pos_embed = types.MethodType(
+ _resize_pos_embed, pretrained.model
+ )
+
+ return pretrained
+
+
+def _make_pretrained_vitl16_384(pretrained, use_readout="ignore", hooks=None):
+ model = timm.create_model("vit_large_patch16_384", pretrained=pretrained)
+
+ hooks = [5, 11, 17, 23] if hooks == None else hooks
+ return _make_vit_b16_backbone(
+ model,
+ features=[256, 512, 1024, 1024],
+ hooks=hooks,
+ vit_features=1024,
+ use_readout=use_readout,
+ )
+
+
+def _make_pretrained_vitb16_384(pretrained, use_readout="ignore", hooks=None):
+ model = timm.create_model("vit_base_patch16_384", pretrained=pretrained)
+
+ hooks = [2, 5, 8, 11] if hooks == None else hooks
+ return _make_vit_b16_backbone(
+ model, features=[96, 192, 384, 768], hooks=hooks, use_readout=use_readout
+ )
+
+
+def _make_vit_b_rn50_backbone(
+ model,
+ features=[256, 512, 768, 768],
+ size=[384, 384],
+ hooks=[0, 1, 8, 11],
+ vit_features=768,
+ patch_size=[16, 16],
+ number_stages=2,
+ use_vit_only=False,
+ use_readout="ignore",
+ start_index=1,
+):
+ pretrained = nn.Module()
+
+ pretrained.model = model
+
+ used_number_stages = 0 if use_vit_only else number_stages
+ for s in range(used_number_stages):
+ pretrained.model.patch_embed.backbone.stages[s].register_forward_hook(
+ get_activation(str(s + 1))
+ )
+ for s in range(used_number_stages, 4):
+ pretrained.model.blocks[hooks[s]].register_forward_hook(get_activation(str(s + 1)))
+
+ pretrained.activations = activations
+
+ readout_oper = get_readout_oper(vit_features, features, use_readout, start_index)
+
+ for s in range(used_number_stages):
+ value = nn.Sequential(nn.Identity(), nn.Identity(), nn.Identity())
+ exec(f"pretrained.act_postprocess{s + 1}=value")
+ for s in range(used_number_stages, 4):
+ if s < number_stages:
+ final_layer = nn.ConvTranspose2d(
+ in_channels=features[s],
+ out_channels=features[s],
+ kernel_size=4 // (2 ** s),
+ stride=4 // (2 ** s),
+ padding=0,
+ bias=True,
+ dilation=1,
+ groups=1,
+ )
+ elif s > number_stages:
+ final_layer = nn.Conv2d(
+ in_channels=features[3],
+ out_channels=features[3],
+ kernel_size=3,
+ stride=2,
+ padding=1,
+ )
+ else:
+ final_layer = None
+
+ layers = [
+ readout_oper[s],
+ Transpose(1, 2),
+ nn.Unflatten(2, torch.Size([size[0] // 16, size[1] // 16])),
+ nn.Conv2d(
+ in_channels=vit_features,
+ out_channels=features[s],
+ kernel_size=1,
+ stride=1,
+ padding=0,
+ ),
+ ]
+ if final_layer is not None:
+ layers.append(final_layer)
+
+ value = nn.Sequential(*layers)
+ exec(f"pretrained.act_postprocess{s + 1}=value")
+
+ pretrained.model.start_index = start_index
+ pretrained.model.patch_size = patch_size
+
+ # We inject this function into the VisionTransformer instances so that
+ # we can use it with interpolated position embeddings without modifying the library source.
+ pretrained.model.forward_flex = types.MethodType(forward_flex, pretrained.model)
+
+ # We inject this function into the VisionTransformer instances so that
+ # we can use it with interpolated position embeddings without modifying the library source.
+ pretrained.model._resize_pos_embed = types.MethodType(
+ _resize_pos_embed, pretrained.model
+ )
+
+ return pretrained
+
+
+def _make_pretrained_vitb_rn50_384(
+ pretrained, use_readout="ignore", hooks=None, use_vit_only=False
+):
+ model = timm.create_model("vit_base_resnet50_384", pretrained=pretrained)
+
+ hooks = [0, 1, 8, 11] if hooks == None else hooks
+ return _make_vit_b_rn50_backbone(
+ model,
+ features=[256, 512, 768, 768],
+ size=[384, 384],
+ hooks=hooks,
+ use_vit_only=use_vit_only,
+ use_readout=use_readout,
+ )
diff --git a/midas/base_model.py b/midas/base_model.py
new file mode 100644
index 0000000000000000000000000000000000000000..5cf430239b47ec5ec07531263f26f5c24a2311cd
--- /dev/null
+++ b/midas/base_model.py
@@ -0,0 +1,16 @@
+import torch
+
+
+class BaseModel(torch.nn.Module):
+ def load(self, path):
+ """Load model from file.
+
+ Args:
+ path (str): file path
+ """
+ parameters = torch.load(path, map_location=torch.device('cpu'))
+
+ if "optimizer" in parameters:
+ parameters = parameters["model"]
+
+ self.load_state_dict(parameters)
diff --git a/midas/blocks.py b/midas/blocks.py
new file mode 100644
index 0000000000000000000000000000000000000000..6d87a00680bb6ed9a6d7c3043ea30a1e90361794
--- /dev/null
+++ b/midas/blocks.py
@@ -0,0 +1,439 @@
+import torch
+import torch.nn as nn
+
+from .backbones.beit import (
+ _make_pretrained_beitl16_512,
+ _make_pretrained_beitl16_384,
+ _make_pretrained_beitb16_384,
+ forward_beit,
+)
+from .backbones.swin_common import (
+ forward_swin,
+)
+from .backbones.swin2 import (
+ _make_pretrained_swin2l24_384,
+ _make_pretrained_swin2b24_384,
+ _make_pretrained_swin2t16_256,
+)
+from .backbones.swin import (
+ _make_pretrained_swinl12_384,
+)
+from .backbones.levit import (
+ _make_pretrained_levit_384,
+ forward_levit,
+)
+from .backbones.vit import (
+ _make_pretrained_vitb_rn50_384,
+ _make_pretrained_vitl16_384,
+ _make_pretrained_vitb16_384,
+ forward_vit,
+)
+
+def _make_encoder(backbone, features, use_pretrained, groups=1, expand=False, exportable=True, hooks=None,
+ use_vit_only=False, use_readout="ignore", in_features=[96, 256, 512, 1024]):
+ if backbone == "beitl16_512":
+ pretrained = _make_pretrained_beitl16_512(
+ use_pretrained, hooks=hooks, use_readout=use_readout
+ )
+ scratch = _make_scratch(
+ [256, 512, 1024, 1024], features, groups=groups, expand=expand
+ ) # BEiT_512-L (backbone)
+ elif backbone == "beitl16_384":
+ pretrained = _make_pretrained_beitl16_384(
+ use_pretrained, hooks=hooks, use_readout=use_readout
+ )
+ scratch = _make_scratch(
+ [256, 512, 1024, 1024], features, groups=groups, expand=expand
+ ) # BEiT_384-L (backbone)
+ elif backbone == "beitb16_384":
+ pretrained = _make_pretrained_beitb16_384(
+ use_pretrained, hooks=hooks, use_readout=use_readout
+ )
+ scratch = _make_scratch(
+ [96, 192, 384, 768], features, groups=groups, expand=expand
+ ) # BEiT_384-B (backbone)
+ elif backbone == "swin2l24_384":
+ pretrained = _make_pretrained_swin2l24_384(
+ use_pretrained, hooks=hooks
+ )
+ scratch = _make_scratch(
+ [192, 384, 768, 1536], features, groups=groups, expand=expand
+ ) # Swin2-L/12to24 (backbone)
+ elif backbone == "swin2b24_384":
+ pretrained = _make_pretrained_swin2b24_384(
+ use_pretrained, hooks=hooks
+ )
+ scratch = _make_scratch(
+ [128, 256, 512, 1024], features, groups=groups, expand=expand
+ ) # Swin2-B/12to24 (backbone)
+ elif backbone == "swin2t16_256":
+ pretrained = _make_pretrained_swin2t16_256(
+ use_pretrained, hooks=hooks
+ )
+ scratch = _make_scratch(
+ [96, 192, 384, 768], features, groups=groups, expand=expand
+ ) # Swin2-T/16 (backbone)
+ elif backbone == "swinl12_384":
+ pretrained = _make_pretrained_swinl12_384(
+ use_pretrained, hooks=hooks
+ )
+ scratch = _make_scratch(
+ [192, 384, 768, 1536], features, groups=groups, expand=expand
+ ) # Swin-L/12 (backbone)
+ elif backbone == "next_vit_large_6m":
+ from .backbones.next_vit import _make_pretrained_next_vit_large_6m
+ pretrained = _make_pretrained_next_vit_large_6m(hooks=hooks)
+ scratch = _make_scratch(
+ in_features, features, groups=groups, expand=expand
+ ) # Next-ViT-L on ImageNet-1K-6M (backbone)
+ elif backbone == "levit_384":
+ pretrained = _make_pretrained_levit_384(
+ use_pretrained, hooks=hooks
+ )
+ scratch = _make_scratch(
+ [384, 512, 768], features, groups=groups, expand=expand
+ ) # LeViT 384 (backbone)
+ elif backbone == "vitl16_384":
+ pretrained = _make_pretrained_vitl16_384(
+ use_pretrained, hooks=hooks, use_readout=use_readout
+ )
+ scratch = _make_scratch(
+ [256, 512, 1024, 1024], features, groups=groups, expand=expand
+ ) # ViT-L/16 - 85.0% Top1 (backbone)
+ elif backbone == "vitb_rn50_384":
+ pretrained = _make_pretrained_vitb_rn50_384(
+ use_pretrained,
+ hooks=hooks,
+ use_vit_only=use_vit_only,
+ use_readout=use_readout,
+ )
+ scratch = _make_scratch(
+ [256, 512, 768, 768], features, groups=groups, expand=expand
+ ) # ViT-H/16 - 85.0% Top1 (backbone)
+ elif backbone == "vitb16_384":
+ pretrained = _make_pretrained_vitb16_384(
+ use_pretrained, hooks=hooks, use_readout=use_readout
+ )
+ scratch = _make_scratch(
+ [96, 192, 384, 768], features, groups=groups, expand=expand
+ ) # ViT-B/16 - 84.6% Top1 (backbone)
+ elif backbone == "resnext101_wsl":
+ pretrained = _make_pretrained_resnext101_wsl(use_pretrained)
+ scratch = _make_scratch([256, 512, 1024, 2048], features, groups=groups, expand=expand) # efficientnet_lite3
+ elif backbone == "efficientnet_lite3":
+ pretrained = _make_pretrained_efficientnet_lite3(use_pretrained, exportable=exportable)
+ scratch = _make_scratch([32, 48, 136, 384], features, groups=groups, expand=expand) # efficientnet_lite3
+ else:
+ print(f"Backbone '{backbone}' not implemented")
+ assert False
+
+ return pretrained, scratch
+
+
+def _make_scratch(in_shape, out_shape, groups=1, expand=False):
+ scratch = nn.Module()
+
+ out_shape1 = out_shape
+ out_shape2 = out_shape
+ out_shape3 = out_shape
+ if len(in_shape) >= 4:
+ out_shape4 = out_shape
+
+ if expand:
+ out_shape1 = out_shape
+ out_shape2 = out_shape*2
+ out_shape3 = out_shape*4
+ if len(in_shape) >= 4:
+ out_shape4 = out_shape*8
+
+ scratch.layer1_rn = nn.Conv2d(
+ in_shape[0], out_shape1, kernel_size=3, stride=1, padding=1, bias=False, groups=groups
+ )
+ scratch.layer2_rn = nn.Conv2d(
+ in_shape[1], out_shape2, kernel_size=3, stride=1, padding=1, bias=False, groups=groups
+ )
+ scratch.layer3_rn = nn.Conv2d(
+ in_shape[2], out_shape3, kernel_size=3, stride=1, padding=1, bias=False, groups=groups
+ )
+ if len(in_shape) >= 4:
+ scratch.layer4_rn = nn.Conv2d(
+ in_shape[3], out_shape4, kernel_size=3, stride=1, padding=1, bias=False, groups=groups
+ )
+
+ return scratch
+
+
+def _make_pretrained_efficientnet_lite3(use_pretrained, exportable=False):
+ efficientnet = torch.hub.load(
+ "rwightman/gen-efficientnet-pytorch",
+ "tf_efficientnet_lite3",
+ pretrained=use_pretrained,
+ exportable=exportable
+ )
+ return _make_efficientnet_backbone(efficientnet)
+
+
+def _make_efficientnet_backbone(effnet):
+ pretrained = nn.Module()
+
+ pretrained.layer1 = nn.Sequential(
+ effnet.conv_stem, effnet.bn1, effnet.act1, *effnet.blocks[0:2]
+ )
+ pretrained.layer2 = nn.Sequential(*effnet.blocks[2:3])
+ pretrained.layer3 = nn.Sequential(*effnet.blocks[3:5])
+ pretrained.layer4 = nn.Sequential(*effnet.blocks[5:9])
+
+ return pretrained
+
+
+def _make_resnet_backbone(resnet):
+ pretrained = nn.Module()
+ pretrained.layer1 = nn.Sequential(
+ resnet.conv1, resnet.bn1, resnet.relu, resnet.maxpool, resnet.layer1
+ )
+
+ pretrained.layer2 = resnet.layer2
+ pretrained.layer3 = resnet.layer3
+ pretrained.layer4 = resnet.layer4
+
+ return pretrained
+
+
+def _make_pretrained_resnext101_wsl(use_pretrained):
+ resnet = torch.hub.load("facebookresearch/WSL-Images", "resnext101_32x8d_wsl")
+ return _make_resnet_backbone(resnet)
+
+
+
+class Interpolate(nn.Module):
+ """Interpolation module.
+ """
+
+ def __init__(self, scale_factor, mode, align_corners=False):
+ """Init.
+
+ Args:
+ scale_factor (float): scaling
+ mode (str): interpolation mode
+ """
+ super(Interpolate, self).__init__()
+
+ self.interp = nn.functional.interpolate
+ self.scale_factor = scale_factor
+ self.mode = mode
+ self.align_corners = align_corners
+
+ def forward(self, x):
+ """Forward pass.
+
+ Args:
+ x (tensor): input
+
+ Returns:
+ tensor: interpolated data
+ """
+
+ x = self.interp(
+ x, scale_factor=self.scale_factor, mode=self.mode, align_corners=self.align_corners
+ )
+
+ return x
+
+
+class ResidualConvUnit(nn.Module):
+ """Residual convolution module.
+ """
+
+ def __init__(self, features):
+ """Init.
+
+ Args:
+ features (int): number of features
+ """
+ super().__init__()
+
+ self.conv1 = nn.Conv2d(
+ features, features, kernel_size=3, stride=1, padding=1, bias=True
+ )
+
+ self.conv2 = nn.Conv2d(
+ features, features, kernel_size=3, stride=1, padding=1, bias=True
+ )
+
+ self.relu = nn.ReLU(inplace=True)
+
+ def forward(self, x):
+ """Forward pass.
+
+ Args:
+ x (tensor): input
+
+ Returns:
+ tensor: output
+ """
+ out = self.relu(x)
+ out = self.conv1(out)
+ out = self.relu(out)
+ out = self.conv2(out)
+
+ return out + x
+
+
+class FeatureFusionBlock(nn.Module):
+ """Feature fusion block.
+ """
+
+ def __init__(self, features):
+ """Init.
+
+ Args:
+ features (int): number of features
+ """
+ super(FeatureFusionBlock, self).__init__()
+
+ self.resConfUnit1 = ResidualConvUnit(features)
+ self.resConfUnit2 = ResidualConvUnit(features)
+
+ def forward(self, *xs):
+ """Forward pass.
+
+ Returns:
+ tensor: output
+ """
+ output = xs[0]
+
+ if len(xs) == 2:
+ output += self.resConfUnit1(xs[1])
+
+ output = self.resConfUnit2(output)
+
+ output = nn.functional.interpolate(
+ output, scale_factor=2, mode="bilinear", align_corners=True
+ )
+
+ return output
+
+
+
+
+class ResidualConvUnit_custom(nn.Module):
+ """Residual convolution module.
+ """
+
+ def __init__(self, features, activation, bn):
+ """Init.
+
+ Args:
+ features (int): number of features
+ """
+ super().__init__()
+
+ self.bn = bn
+
+ self.groups=1
+
+ self.conv1 = nn.Conv2d(
+ features, features, kernel_size=3, stride=1, padding=1, bias=True, groups=self.groups
+ )
+
+ self.conv2 = nn.Conv2d(
+ features, features, kernel_size=3, stride=1, padding=1, bias=True, groups=self.groups
+ )
+
+ if self.bn==True:
+ self.bn1 = nn.BatchNorm2d(features)
+ self.bn2 = nn.BatchNorm2d(features)
+
+ self.activation = activation
+
+ self.skip_add = nn.quantized.FloatFunctional()
+
+ def forward(self, x):
+ """Forward pass.
+
+ Args:
+ x (tensor): input
+
+ Returns:
+ tensor: output
+ """
+
+ out = self.activation(x)
+ out = self.conv1(out)
+ if self.bn==True:
+ out = self.bn1(out)
+
+ out = self.activation(out)
+ out = self.conv2(out)
+ if self.bn==True:
+ out = self.bn2(out)
+
+ if self.groups > 1:
+ out = self.conv_merge(out)
+
+ return self.skip_add.add(out, x)
+
+ # return out + x
+
+
+class FeatureFusionBlock_custom(nn.Module):
+ """Feature fusion block.
+ """
+
+ def __init__(self, features, activation, deconv=False, bn=False, expand=False, align_corners=True, size=None):
+ """Init.
+
+ Args:
+ features (int): number of features
+ """
+ super(FeatureFusionBlock_custom, self).__init__()
+
+ self.deconv = deconv
+ self.align_corners = align_corners
+
+ self.groups=1
+
+ self.expand = expand
+ out_features = features
+ if self.expand==True:
+ out_features = features//2
+
+ self.out_conv = nn.Conv2d(features, out_features, kernel_size=1, stride=1, padding=0, bias=True, groups=1)
+
+ self.resConfUnit1 = ResidualConvUnit_custom(features, activation, bn)
+ self.resConfUnit2 = ResidualConvUnit_custom(features, activation, bn)
+
+ self.skip_add = nn.quantized.FloatFunctional()
+
+ self.size=size
+
+ def forward(self, *xs, size=None):
+ """Forward pass.
+
+ Returns:
+ tensor: output
+ """
+ output = xs[0]
+
+ if len(xs) == 2:
+ res = self.resConfUnit1(xs[1])
+ output = self.skip_add.add(output, res)
+ # output += res
+
+ output = self.resConfUnit2(output)
+
+ if (size is None) and (self.size is None):
+ modifier = {"scale_factor": 2}
+ elif size is None:
+ modifier = {"size": self.size}
+ else:
+ modifier = {"size": size}
+
+ output = nn.functional.interpolate(
+ output, **modifier, mode="bilinear", align_corners=self.align_corners
+ )
+
+ output = self.out_conv(output)
+
+ return output
+
diff --git a/midas/dpt_depth.py b/midas/dpt_depth.py
new file mode 100644
index 0000000000000000000000000000000000000000..3129d09cb43a7c79b23916236991fabbedb78f55
--- /dev/null
+++ b/midas/dpt_depth.py
@@ -0,0 +1,166 @@
+import torch
+import torch.nn as nn
+
+from .base_model import BaseModel
+from .blocks import (
+ FeatureFusionBlock_custom,
+ Interpolate,
+ _make_encoder,
+ forward_beit,
+ forward_swin,
+ forward_levit,
+ forward_vit,
+)
+from .backbones.levit import stem_b4_transpose
+from timm.models.layers import get_act_layer
+
+
+def _make_fusion_block(features, use_bn, size = None):
+ return FeatureFusionBlock_custom(
+ features,
+ nn.ReLU(False),
+ deconv=False,
+ bn=use_bn,
+ expand=False,
+ align_corners=True,
+ size=size,
+ )
+
+
+class DPT(BaseModel):
+ def __init__(
+ self,
+ head,
+ features=256,
+ backbone="vitb_rn50_384",
+ readout="project",
+ channels_last=False,
+ use_bn=False,
+ **kwargs
+ ):
+
+ super(DPT, self).__init__()
+
+ self.channels_last = channels_last
+
+ # For the Swin, Swin 2, LeViT and Next-ViT Transformers, the hierarchical architectures prevent setting the
+ # hooks freely. Instead, the hooks have to be chosen according to the ranges specified in the comments.
+ hooks = {
+ "beitl16_512": [5, 11, 17, 23],
+ "beitl16_384": [5, 11, 17, 23],
+ "beitb16_384": [2, 5, 8, 11],
+ "swin2l24_384": [1, 1, 17, 1], # Allowed ranges: [0, 1], [0, 1], [ 0, 17], [ 0, 1]
+ "swin2b24_384": [1, 1, 17, 1], # [0, 1], [0, 1], [ 0, 17], [ 0, 1]
+ "swin2t16_256": [1, 1, 5, 1], # [0, 1], [0, 1], [ 0, 5], [ 0, 1]
+ "swinl12_384": [1, 1, 17, 1], # [0, 1], [0, 1], [ 0, 17], [ 0, 1]
+ "next_vit_large_6m": [2, 6, 36, 39], # [0, 2], [3, 6], [ 7, 36], [37, 39]
+ "levit_384": [3, 11, 21], # [0, 3], [6, 11], [14, 21]
+ "vitb_rn50_384": [0, 1, 8, 11],
+ "vitb16_384": [2, 5, 8, 11],
+ "vitl16_384": [5, 11, 17, 23],
+ }[backbone]
+
+ if "next_vit" in backbone:
+ in_features = {
+ "next_vit_large_6m": [96, 256, 512, 1024],
+ }[backbone]
+ else:
+ in_features = None
+
+ # Instantiate backbone and reassemble blocks
+ self.pretrained, self.scratch = _make_encoder(
+ backbone,
+ features,
+ False, # Set to true of you want to train from scratch, uses ImageNet weights
+ groups=1,
+ expand=False,
+ exportable=False,
+ hooks=hooks,
+ use_readout=readout,
+ in_features=in_features,
+ )
+
+ self.number_layers = len(hooks) if hooks is not None else 4
+ size_refinenet3 = None
+ self.scratch.stem_transpose = None
+
+ if "beit" in backbone:
+ self.forward_transformer = forward_beit
+ elif "swin" in backbone:
+ self.forward_transformer = forward_swin
+ elif "next_vit" in backbone:
+ from .backbones.next_vit import forward_next_vit
+ self.forward_transformer = forward_next_vit
+ elif "levit" in backbone:
+ self.forward_transformer = forward_levit
+ size_refinenet3 = 7
+ self.scratch.stem_transpose = stem_b4_transpose(256, 128, get_act_layer("hard_swish"))
+ else:
+ self.forward_transformer = forward_vit
+
+ self.scratch.refinenet1 = _make_fusion_block(features, use_bn)
+ self.scratch.refinenet2 = _make_fusion_block(features, use_bn)
+ self.scratch.refinenet3 = _make_fusion_block(features, use_bn, size_refinenet3)
+ if self.number_layers >= 4:
+ self.scratch.refinenet4 = _make_fusion_block(features, use_bn)
+
+ self.scratch.output_conv = head
+
+
+ def forward(self, x):
+ if self.channels_last == True:
+ x.contiguous(memory_format=torch.channels_last)
+
+ layers = self.forward_transformer(self.pretrained, x)
+ if self.number_layers == 3:
+ layer_1, layer_2, layer_3 = layers
+ else:
+ layer_1, layer_2, layer_3, layer_4 = layers
+
+ layer_1_rn = self.scratch.layer1_rn(layer_1)
+ layer_2_rn = self.scratch.layer2_rn(layer_2)
+ layer_3_rn = self.scratch.layer3_rn(layer_3)
+ if self.number_layers >= 4:
+ layer_4_rn = self.scratch.layer4_rn(layer_4)
+
+ if self.number_layers == 3:
+ path_3 = self.scratch.refinenet3(layer_3_rn, size=layer_2_rn.shape[2:])
+ else:
+ path_4 = self.scratch.refinenet4(layer_4_rn, size=layer_3_rn.shape[2:])
+ path_3 = self.scratch.refinenet3(path_4, layer_3_rn, size=layer_2_rn.shape[2:])
+ path_2 = self.scratch.refinenet2(path_3, layer_2_rn, size=layer_1_rn.shape[2:])
+ path_1 = self.scratch.refinenet1(path_2, layer_1_rn)
+
+ if self.scratch.stem_transpose is not None:
+ path_1 = self.scratch.stem_transpose(path_1)
+
+ out = self.scratch.output_conv(path_1)
+
+ return out
+
+
+class DPTDepthModel(DPT):
+ def __init__(self, path=None, non_negative=True, **kwargs):
+ features = kwargs["features"] if "features" in kwargs else 256
+ head_features_1 = kwargs["head_features_1"] if "head_features_1" in kwargs else features
+ head_features_2 = kwargs["head_features_2"] if "head_features_2" in kwargs else 32
+ kwargs.pop("head_features_1", None)
+ kwargs.pop("head_features_2", None)
+
+ head = nn.Sequential(
+ nn.Conv2d(head_features_1, head_features_1 // 2, kernel_size=3, stride=1, padding=1),
+ Interpolate(scale_factor=2, mode="bilinear", align_corners=True),
+ nn.Conv2d(head_features_1 // 2, head_features_2, kernel_size=3, stride=1, padding=1),
+ nn.ReLU(True),
+ nn.Conv2d(head_features_2, 1, kernel_size=1, stride=1, padding=0),
+ nn.ReLU(True) if non_negative else nn.Identity(),
+ nn.Identity(),
+ )
+
+ super().__init__(head, **kwargs)
+
+ if path is not None:
+ self.load(path)
+
+ def forward(self, x):
+ return super().forward(x).squeeze(dim=1)
diff --git a/midas/midas_net.py b/midas/midas_net.py
new file mode 100644
index 0000000000000000000000000000000000000000..8a954977800b0a0f48807e80fa63041910e33c1f
--- /dev/null
+++ b/midas/midas_net.py
@@ -0,0 +1,76 @@
+"""MidashNet: Network for monocular depth estimation trained by mixing several datasets.
+This file contains code that is adapted from
+https://github.com/thomasjpfan/pytorch_refinenet/blob/master/pytorch_refinenet/refinenet/refinenet_4cascade.py
+"""
+import torch
+import torch.nn as nn
+
+from .base_model import BaseModel
+from .blocks import FeatureFusionBlock, Interpolate, _make_encoder
+
+
+class MidasNet(BaseModel):
+ """Network for monocular depth estimation.
+ """
+
+ def __init__(self, path=None, features=256, non_negative=True):
+ """Init.
+
+ Args:
+ path (str, optional): Path to saved model. Defaults to None.
+ features (int, optional): Number of features. Defaults to 256.
+ backbone (str, optional): Backbone network for encoder. Defaults to resnet50
+ """
+ print("Loading weights: ", path)
+
+ super(MidasNet, self).__init__()
+
+ use_pretrained = False if path is None else True
+
+ self.pretrained, self.scratch = _make_encoder(backbone="resnext101_wsl", features=features, use_pretrained=use_pretrained)
+
+ self.scratch.refinenet4 = FeatureFusionBlock(features)
+ self.scratch.refinenet3 = FeatureFusionBlock(features)
+ self.scratch.refinenet2 = FeatureFusionBlock(features)
+ self.scratch.refinenet1 = FeatureFusionBlock(features)
+
+ self.scratch.output_conv = nn.Sequential(
+ nn.Conv2d(features, 128, kernel_size=3, stride=1, padding=1),
+ Interpolate(scale_factor=2, mode="bilinear"),
+ nn.Conv2d(128, 32, kernel_size=3, stride=1, padding=1),
+ nn.ReLU(True),
+ nn.Conv2d(32, 1, kernel_size=1, stride=1, padding=0),
+ nn.ReLU(True) if non_negative else nn.Identity(),
+ )
+
+ if path:
+ self.load(path)
+
+ def forward(self, x):
+ """Forward pass.
+
+ Args:
+ x (tensor): input data (image)
+
+ Returns:
+ tensor: depth
+ """
+
+ layer_1 = self.pretrained.layer1(x)
+ layer_2 = self.pretrained.layer2(layer_1)
+ layer_3 = self.pretrained.layer3(layer_2)
+ layer_4 = self.pretrained.layer4(layer_3)
+
+ layer_1_rn = self.scratch.layer1_rn(layer_1)
+ layer_2_rn = self.scratch.layer2_rn(layer_2)
+ layer_3_rn = self.scratch.layer3_rn(layer_3)
+ layer_4_rn = self.scratch.layer4_rn(layer_4)
+
+ path_4 = self.scratch.refinenet4(layer_4_rn)
+ path_3 = self.scratch.refinenet3(path_4, layer_3_rn)
+ path_2 = self.scratch.refinenet2(path_3, layer_2_rn)
+ path_1 = self.scratch.refinenet1(path_2, layer_1_rn)
+
+ out = self.scratch.output_conv(path_1)
+
+ return torch.squeeze(out, dim=1)
diff --git a/midas/midas_net_custom.py b/midas/midas_net_custom.py
new file mode 100644
index 0000000000000000000000000000000000000000..50e4acb5e53d5fabefe3dde16ab49c33c2b7797c
--- /dev/null
+++ b/midas/midas_net_custom.py
@@ -0,0 +1,128 @@
+"""MidashNet: Network for monocular depth estimation trained by mixing several datasets.
+This file contains code that is adapted from
+https://github.com/thomasjpfan/pytorch_refinenet/blob/master/pytorch_refinenet/refinenet/refinenet_4cascade.py
+"""
+import torch
+import torch.nn as nn
+
+from .base_model import BaseModel
+from .blocks import FeatureFusionBlock, FeatureFusionBlock_custom, Interpolate, _make_encoder
+
+
+class MidasNet_small(BaseModel):
+ """Network for monocular depth estimation.
+ """
+
+ def __init__(self, path=None, features=64, backbone="efficientnet_lite3", non_negative=True, exportable=True, channels_last=False, align_corners=True,
+ blocks={'expand': True}):
+ """Init.
+
+ Args:
+ path (str, optional): Path to saved model. Defaults to None.
+ features (int, optional): Number of features. Defaults to 256.
+ backbone (str, optional): Backbone network for encoder. Defaults to resnet50
+ """
+ print("Loading weights: ", path)
+
+ super(MidasNet_small, self).__init__()
+
+ use_pretrained = False if path else True
+
+ self.channels_last = channels_last
+ self.blocks = blocks
+ self.backbone = backbone
+
+ self.groups = 1
+
+ features1=features
+ features2=features
+ features3=features
+ features4=features
+ self.expand = False
+ if "expand" in self.blocks and self.blocks['expand'] == True:
+ self.expand = True
+ features1=features
+ features2=features*2
+ features3=features*4
+ features4=features*8
+
+ self.pretrained, self.scratch = _make_encoder(self.backbone, features, use_pretrained, groups=self.groups, expand=self.expand, exportable=exportable)
+
+ self.scratch.activation = nn.ReLU(False)
+
+ self.scratch.refinenet4 = FeatureFusionBlock_custom(features4, self.scratch.activation, deconv=False, bn=False, expand=self.expand, align_corners=align_corners)
+ self.scratch.refinenet3 = FeatureFusionBlock_custom(features3, self.scratch.activation, deconv=False, bn=False, expand=self.expand, align_corners=align_corners)
+ self.scratch.refinenet2 = FeatureFusionBlock_custom(features2, self.scratch.activation, deconv=False, bn=False, expand=self.expand, align_corners=align_corners)
+ self.scratch.refinenet1 = FeatureFusionBlock_custom(features1, self.scratch.activation, deconv=False, bn=False, align_corners=align_corners)
+
+
+ self.scratch.output_conv = nn.Sequential(
+ nn.Conv2d(features, features//2, kernel_size=3, stride=1, padding=1, groups=self.groups),
+ Interpolate(scale_factor=2, mode="bilinear"),
+ nn.Conv2d(features//2, 32, kernel_size=3, stride=1, padding=1),
+ self.scratch.activation,
+ nn.Conv2d(32, 1, kernel_size=1, stride=1, padding=0),
+ nn.ReLU(True) if non_negative else nn.Identity(),
+ nn.Identity(),
+ )
+
+ if path:
+ self.load(path)
+
+
+ def forward(self, x):
+ """Forward pass.
+
+ Args:
+ x (tensor): input data (image)
+
+ Returns:
+ tensor: depth
+ """
+ if self.channels_last==True:
+ print("self.channels_last = ", self.channels_last)
+ x.contiguous(memory_format=torch.channels_last)
+
+
+ layer_1 = self.pretrained.layer1(x)
+ layer_2 = self.pretrained.layer2(layer_1)
+ layer_3 = self.pretrained.layer3(layer_2)
+ layer_4 = self.pretrained.layer4(layer_3)
+
+ layer_1_rn = self.scratch.layer1_rn(layer_1)
+ layer_2_rn = self.scratch.layer2_rn(layer_2)
+ layer_3_rn = self.scratch.layer3_rn(layer_3)
+ layer_4_rn = self.scratch.layer4_rn(layer_4)
+
+
+ path_4 = self.scratch.refinenet4(layer_4_rn)
+ path_3 = self.scratch.refinenet3(path_4, layer_3_rn)
+ path_2 = self.scratch.refinenet2(path_3, layer_2_rn)
+ path_1 = self.scratch.refinenet1(path_2, layer_1_rn)
+
+ out = self.scratch.output_conv(path_1)
+
+ return torch.squeeze(out, dim=1)
+
+
+
+def fuse_model(m):
+ prev_previous_type = nn.Identity()
+ prev_previous_name = ''
+ previous_type = nn.Identity()
+ previous_name = ''
+ for name, module in m.named_modules():
+ if prev_previous_type == nn.Conv2d and previous_type == nn.BatchNorm2d and type(module) == nn.ReLU:
+ # print("FUSED ", prev_previous_name, previous_name, name)
+ torch.quantization.fuse_modules(m, [prev_previous_name, previous_name, name], inplace=True)
+ elif prev_previous_type == nn.Conv2d and previous_type == nn.BatchNorm2d:
+ # print("FUSED ", prev_previous_name, previous_name)
+ torch.quantization.fuse_modules(m, [prev_previous_name, previous_name], inplace=True)
+ # elif previous_type == nn.Conv2d and type(module) == nn.ReLU:
+ # print("FUSED ", previous_name, name)
+ # torch.quantization.fuse_modules(m, [previous_name, name], inplace=True)
+
+ prev_previous_type = previous_type
+ prev_previous_name = previous_name
+ previous_type = type(module)
+ previous_name = name
\ No newline at end of file
diff --git a/midas/model_loader.py b/midas/model_loader.py
new file mode 100644
index 0000000000000000000000000000000000000000..f1cd1f2d43054bfd3d650587c7b2ed35f1347c9e
--- /dev/null
+++ b/midas/model_loader.py
@@ -0,0 +1,242 @@
+import cv2
+import torch
+
+from midas.dpt_depth import DPTDepthModel
+from midas.midas_net import MidasNet
+from midas.midas_net_custom import MidasNet_small
+from midas.transforms import Resize, NormalizeImage, PrepareForNet
+
+from torchvision.transforms import Compose
+
+default_models = {
+ "dpt_beit_large_512": "weights/dpt_beit_large_512.pt",
+ "dpt_beit_large_384": "weights/dpt_beit_large_384.pt",
+ "dpt_beit_base_384": "weights/dpt_beit_base_384.pt",
+ "dpt_swin2_large_384": "weights/dpt_swin2_large_384.pt",
+ "dpt_swin2_base_384": "weights/dpt_swin2_base_384.pt",
+ "dpt_swin2_tiny_256": "weights/dpt_swin2_tiny_256.pt",
+ "dpt_swin_large_384": "weights/dpt_swin_large_384.pt",
+ "dpt_next_vit_large_384": "weights/dpt_next_vit_large_384.pt",
+ "dpt_levit_224": "weights/dpt_levit_224.pt",
+ "dpt_large_384": "weights/dpt_large_384.pt",
+ "dpt_hybrid_384": "weights/dpt_hybrid_384.pt",
+ "midas_v21_384": "weights/midas_v21_384.pt",
+ "midas_v21_small_256": "weights/midas_v21_small_256.pt",
+ "openvino_midas_v21_small_256": "weights/openvino_midas_v21_small_256.xml",
+}
+
+
+def load_model(device, model_path, model_type="dpt_large_384", optimize=True, height=None, square=False):
+ """Load the specified network.
+
+ Args:
+ device (device): the torch device used
+ model_path (str): path to saved model
+ model_type (str): the type of the model to be loaded
+ optimize (bool): optimize the model to half-integer on CUDA?
+ height (int): inference encoder image height
+ square (bool): resize to a square resolution?
+
+ Returns:
+ The loaded network, the transform which prepares images as input to the network and the dimensions of the
+ network input
+ """
+ if "openvino" in model_type:
+ from openvino.runtime import Core
+
+ keep_aspect_ratio = not square
+
+ if model_type == "dpt_beit_large_512":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="beitl16_512",
+ non_negative=True,
+ )
+ net_w, net_h = 512, 512
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_beit_large_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="beitl16_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_beit_base_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="beitb16_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_swin2_large_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="swin2l24_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ keep_aspect_ratio = False
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_swin2_base_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="swin2b24_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ keep_aspect_ratio = False
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_swin2_tiny_256":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="swin2t16_256",
+ non_negative=True,
+ )
+ net_w, net_h = 256, 256
+ keep_aspect_ratio = False
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_swin_large_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="swinl12_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ keep_aspect_ratio = False
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_next_vit_large_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="next_vit_large_6m",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ # We change the notation from dpt_levit_224 (MiDaS notation) to levit_384 (timm notation) here, where the 224 refers
+ # to the resolution 224x224 used by LeViT and 384 is the first entry of the embed_dim, see _cfg and model_cfgs of
+ # https://github.com/rwightman/pytorch-image-models/blob/main/timm/models/levit.py
+ # (commit id: 927f031293a30afb940fff0bee34b85d9c059b0e)
+ elif model_type == "dpt_levit_224":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="levit_384",
+ non_negative=True,
+ head_features_1=64,
+ head_features_2=8,
+ )
+ net_w, net_h = 224, 224
+ keep_aspect_ratio = False
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_large_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="vitl16_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "dpt_hybrid_384":
+ model = DPTDepthModel(
+ path=model_path,
+ backbone="vitb_rn50_384",
+ non_negative=True,
+ )
+ net_w, net_h = 384, 384
+ resize_mode = "minimal"
+ normalization = NormalizeImage(mean=[0.5, 0.5, 0.5], std=[0.5, 0.5, 0.5])
+
+ elif model_type == "midas_v21_384":
+ model = MidasNet(model_path, non_negative=True)
+ net_w, net_h = 384, 384
+ resize_mode = "upper_bound"
+ normalization = NormalizeImage(
+ mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]
+ )
+
+ elif model_type == "midas_v21_small_256":
+ model = MidasNet_small(model_path, features=64, backbone="efficientnet_lite3", exportable=True,
+ non_negative=True, blocks={'expand': True})
+ net_w, net_h = 256, 256
+ resize_mode = "upper_bound"
+ normalization = NormalizeImage(
+ mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]
+ )
+
+ elif model_type == "openvino_midas_v21_small_256":
+ ie = Core()
+ uncompiled_model = ie.read_model(model=model_path)
+ model = ie.compile_model(uncompiled_model, "CPU")
+ net_w, net_h = 256, 256
+ resize_mode = "upper_bound"
+ normalization = NormalizeImage(
+ mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]
+ )
+
+ else:
+ print(f"model_type '{model_type}' not implemented, use: --model_type large")
+ assert False
+
+ if not "openvino" in model_type:
+ print("Model loaded, number of parameters = {:.0f}M".format(sum(p.numel() for p in model.parameters()) / 1e6))
+ else:
+ print("Model loaded, optimized with OpenVINO")
+
+ if "openvino" in model_type:
+ keep_aspect_ratio = False
+
+ if height is not None:
+ net_w, net_h = height, height
+
+ transform = Compose(
+ [
+ Resize(
+ net_w,
+ net_h,
+ resize_target=None,
+ keep_aspect_ratio=keep_aspect_ratio,
+ ensure_multiple_of=32,
+ resize_method=resize_mode,
+ image_interpolation_method=cv2.INTER_CUBIC,
+ ),
+ normalization,
+ PrepareForNet(),
+ ]
+ )
+
+ if not "openvino" in model_type:
+ model.eval()
+
+ if optimize and (device == torch.device("cuda")):
+ if not "openvino" in model_type:
+ model = model.to(memory_format=torch.channels_last)
+ model = model.half()
+ else:
+ print("Error: OpenVINO models are already optimized. No optimization to half-float possible.")
+ exit()
+
+ if not "openvino" in model_type:
+ model.to(device)
+
+ return model, transform, net_w, net_h
diff --git a/midas/transforms.py b/midas/transforms.py
new file mode 100644
index 0000000000000000000000000000000000000000..350cbc11662633ad7f8968eb10be2e7de6e384e9
--- /dev/null
+++ b/midas/transforms.py
@@ -0,0 +1,234 @@
+import numpy as np
+import cv2
+import math
+
+
+def apply_min_size(sample, size, image_interpolation_method=cv2.INTER_AREA):
+ """Rezise the sample to ensure the given size. Keeps aspect ratio.
+
+ Args:
+ sample (dict): sample
+ size (tuple): image size
+
+ Returns:
+ tuple: new size
+ """
+ shape = list(sample["disparity"].shape)
+
+ if shape[0] >= size[0] and shape[1] >= size[1]:
+ return sample
+
+ scale = [0, 0]
+ scale[0] = size[0] / shape[0]
+ scale[1] = size[1] / shape[1]
+
+ scale = max(scale)
+
+ shape[0] = math.ceil(scale * shape[0])
+ shape[1] = math.ceil(scale * shape[1])
+
+ # resize
+ sample["image"] = cv2.resize(
+ sample["image"], tuple(shape[::-1]), interpolation=image_interpolation_method
+ )
+
+ sample["disparity"] = cv2.resize(
+ sample["disparity"], tuple(shape[::-1]), interpolation=cv2.INTER_NEAREST
+ )
+ sample["mask"] = cv2.resize(
+ sample["mask"].astype(np.float32),
+ tuple(shape[::-1]),
+ interpolation=cv2.INTER_NEAREST,
+ )
+ sample["mask"] = sample["mask"].astype(bool)
+
+ return tuple(shape)
+
+
+class Resize(object):
+ """Resize sample to given size (width, height).
+ """
+
+ def __init__(
+ self,
+ width,
+ height,
+ resize_target=True,
+ keep_aspect_ratio=False,
+ ensure_multiple_of=1,
+ resize_method="lower_bound",
+ image_interpolation_method=cv2.INTER_AREA,
+ ):
+ """Init.
+
+ Args:
+ width (int): desired output width
+ height (int): desired output height
+ resize_target (bool, optional):
+ True: Resize the full sample (image, mask, target).
+ False: Resize image only.
+ Defaults to True.
+ keep_aspect_ratio (bool, optional):
+ True: Keep the aspect ratio of the input sample.
+ Output sample might not have the given width and height, and
+ resize behaviour depends on the parameter 'resize_method'.
+ Defaults to False.
+ ensure_multiple_of (int, optional):
+ Output width and height is constrained to be multiple of this parameter.
+ Defaults to 1.
+ resize_method (str, optional):
+ "lower_bound": Output will be at least as large as the given size.
+ "upper_bound": Output will be at max as large as the given size. (Output size might be smaller than given size.)
+ "minimal": Scale as least as possible. (Output size might be smaller than given size.)
+ Defaults to "lower_bound".
+ """
+ self.__width = width
+ self.__height = height
+
+ self.__resize_target = resize_target
+ self.__keep_aspect_ratio = keep_aspect_ratio
+ self.__multiple_of = ensure_multiple_of
+ self.__resize_method = resize_method
+ self.__image_interpolation_method = image_interpolation_method
+
+ def constrain_to_multiple_of(self, x, min_val=0, max_val=None):
+ y = (np.round(x / self.__multiple_of) * self.__multiple_of).astype(int)
+
+ if max_val is not None and y > max_val:
+ y = (np.floor(x / self.__multiple_of) * self.__multiple_of).astype(int)
+
+ if y < min_val:
+ y = (np.ceil(x / self.__multiple_of) * self.__multiple_of).astype(int)
+
+ return y
+
+ def get_size(self, width, height):
+ # determine new height and width
+ scale_height = self.__height / height
+ scale_width = self.__width / width
+
+ if self.__keep_aspect_ratio:
+ if self.__resize_method == "lower_bound":
+ # scale such that output size is lower bound
+ if scale_width > scale_height:
+ # fit width
+ scale_height = scale_width
+ else:
+ # fit height
+ scale_width = scale_height
+ elif self.__resize_method == "upper_bound":
+ # scale such that output size is upper bound
+ if scale_width < scale_height:
+ # fit width
+ scale_height = scale_width
+ else:
+ # fit height
+ scale_width = scale_height
+ elif self.__resize_method == "minimal":
+ # scale as least as possbile
+ if abs(1 - scale_width) < abs(1 - scale_height):
+ # fit width
+ scale_height = scale_width
+ else:
+ # fit height
+ scale_width = scale_height
+ else:
+ raise ValueError(
+ f"resize_method {self.__resize_method} not implemented"
+ )
+
+ if self.__resize_method == "lower_bound":
+ new_height = self.constrain_to_multiple_of(
+ scale_height * height, min_val=self.__height
+ )
+ new_width = self.constrain_to_multiple_of(
+ scale_width * width, min_val=self.__width
+ )
+ elif self.__resize_method == "upper_bound":
+ new_height = self.constrain_to_multiple_of(
+ scale_height * height, max_val=self.__height
+ )
+ new_width = self.constrain_to_multiple_of(
+ scale_width * width, max_val=self.__width
+ )
+ elif self.__resize_method == "minimal":
+ new_height = self.constrain_to_multiple_of(scale_height * height)
+ new_width = self.constrain_to_multiple_of(scale_width * width)
+ else:
+ raise ValueError(f"resize_method {self.__resize_method} not implemented")
+
+ return (new_width, new_height)
+
+ def __call__(self, sample):
+ width, height = self.get_size(
+ sample["image"].shape[1], sample["image"].shape[0]
+ )
+
+ # resize sample
+ sample["image"] = cv2.resize(
+ sample["image"],
+ (width, height),
+ interpolation=self.__image_interpolation_method,
+ )
+
+ if self.__resize_target:
+ if "disparity" in sample:
+ sample["disparity"] = cv2.resize(
+ sample["disparity"],
+ (width, height),
+ interpolation=cv2.INTER_NEAREST,
+ )
+
+ if "depth" in sample:
+ sample["depth"] = cv2.resize(
+ sample["depth"], (width, height), interpolation=cv2.INTER_NEAREST
+ )
+
+ sample["mask"] = cv2.resize(
+ sample["mask"].astype(np.float32),
+ (width, height),
+ interpolation=cv2.INTER_NEAREST,
+ )
+ sample["mask"] = sample["mask"].astype(bool)
+
+ return sample
+
+
+class NormalizeImage(object):
+ """Normlize image by given mean and std.
+ """
+
+ def __init__(self, mean, std):
+ self.__mean = mean
+ self.__std = std
+
+ def __call__(self, sample):
+ sample["image"] = (sample["image"] - self.__mean) / self.__std
+
+ return sample
+
+
+class PrepareForNet(object):
+ """Prepare sample for usage as network input.
+ """
+
+ def __init__(self):
+ pass
+
+ def __call__(self, sample):
+ image = np.transpose(sample["image"], (2, 0, 1))
+ sample["image"] = np.ascontiguousarray(image).astype(np.float32)
+
+ if "mask" in sample:
+ sample["mask"] = sample["mask"].astype(np.float32)
+ sample["mask"] = np.ascontiguousarray(sample["mask"])
+
+ if "disparity" in sample:
+ disparity = sample["disparity"].astype(np.float32)
+ sample["disparity"] = np.ascontiguousarray(disparity)
+
+ if "depth" in sample:
+ depth = sample["depth"].astype(np.float32)
+ sample["depth"] = np.ascontiguousarray(depth)
+
+ return sample
diff --git a/monocular_depth_estimator.py b/monocular_depth_estimator.py
new file mode 100644
index 0000000000000000000000000000000000000000..3c1371a4b683dacab1866b4cdba03ed9774d144d
--- /dev/null
+++ b/monocular_depth_estimator.py
@@ -0,0 +1,175 @@
+import cv2
+import torch
+import numpy as np
+import time
+from midas.model_loader import default_models, load_model
+import os
+import urllib.request
+
+class MonocularDepthEstimator:
+ def __init__(self,
+ model_type="midas_v21_small_256",
+ model_weights_path="models/midas_v21_small_256.pt",
+ optimize=False,
+ side_by_side=True,
+ height=None,
+ square=False,
+ grayscale=False):
+
+ # model type
+ # MiDaS 3.1:
+ # For highest quality: dpt_beit_large_512
+ # For moderately less quality, but better speed-performance trade-off: dpt_swin2_large_384
+ # For embedded devices: dpt_swin2_tiny_256, dpt_levit_224
+ # For inference on Intel CPUs, OpenVINO may be used for the small legacy model: openvino_midas_v21_small .xml, .bin
+
+ # MiDaS 3.0:
+ # Legacy transformer models dpt_large_384 and dpt_hybrid_384
+
+ # MiDaS 2.1:
+ # Legacy convolutional models midas_v21_384 and midas_v21_small_256
+
+ # params
+ print("Initializing parameters and model...")
+ self.is_optimize = optimize
+ self.is_square = square
+ self.is_grayscale = grayscale
+ self.height = height
+ self.side_by_side = side_by_side
+
+ # select device
+ self.device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
+ print("Running inference on : %s" % self.device)
+ model_file_url = "https://github.com/isl-org/MiDaS/releases/download/v2_1/midas_v21_small_256.pt"
+
+ # loading model
+ if not os.path.exists(model_weights_path):
+ print("Model file not found. Downloading...")
+ # Download the model file
+ urllib.request.urlretrieve(model_file_url, model_weights_path)
+ print("Model file downloaded successfully.")
+
+ self.model, self.transform, self.net_w, self.net_h = load_model(self.device, model_weights_path,
+ model_type, optimize, height, square)
+ print("Net width and height: ", (self.net_w, self.net_h))
+
+
+ def predict(self, image, model, target_size):
+
+
+ # convert img to tensor and load to gpu
+ img_tensor = torch.from_numpy(image).to(self.device).unsqueeze(0)
+
+ if self.is_optimize and self.device == torch.device("cuda"):
+ img_tensor = img_tensor.to(memory_format=torch.channels_last)
+ img_tensor = img_tensor.half()
+
+ prediction = model.forward(img_tensor)
+ prediction = (
+ torch.nn.functional.interpolate(
+ prediction.unsqueeze(1),
+ size=target_size[::-1],
+ mode="bicubic",
+ align_corners=False,
+ )
+ .squeeze()
+ .cpu()
+ .numpy()
+ )
+
+ return prediction
+
+ def process_prediction(self, original_img, depth_img, is_grayscale=False, side_by_side=False):
+ """
+ Take an RGB image and depth map and place them side by side. This includes a proper normalization of the depth map
+ for better visibility.
+ Args:
+ original_img: the RGB image
+ depth_img: the depth map
+ is_grayscale: use a grayscale colormap?
+ Returns:
+ the image and depth map place side by side
+ """
+
+ # normalizing depth image
+ depth_min = depth_img.min()
+ depth_max = depth_img.max()
+ normalized_depth = 255 * (depth_img - depth_min) / (depth_max - depth_min)
+ normalized_depth *= 3
+
+ depth_side = np.repeat(np.expand_dims(normalized_depth, 2), 3, axis=2) / 3
+ if not is_grayscale:
+ depth_side = cv2.applyColorMap(np.uint8(depth_side), cv2.COLORMAP_INFERNO)
+
+ if side_by_side:
+ return np.concatenate((original_img, depth_side), axis=1)/255
+
+ return depth_side/255
+
+ def make_prediction(self, image):
+ with torch.no_grad():
+ original_image_rgb = np.flip(image, 2) # in [0, 255] (flip required to get RGB)
+ # resizing the image to feed to the model
+ image_tranformed = self.transform({"image": original_image_rgb/255})["image"]
+
+ # monocular depth prediction
+ prediction = self.predict(image_tranformed, self.model, target_size=original_image_rgb.shape[1::-1])
+ original_image_bgr = np.flip(original_image_rgb, 2) if self.side_by_side else None
+
+ # process the model predictions
+ output = self.process_prediction(original_image_bgr, prediction, is_grayscale=self.is_grayscale, side_by_side=self.side_by_side)
+ return output
+
+ def run(self, input_path):
+
+ # input video
+ cap = cv2.VideoCapture(input_path)
+
+ # Check if camera opened successfully
+ if not cap.isOpened():
+ print("Error opening video file")
+
+ with torch.no_grad():
+ while cap.isOpened():
+
+ # Capture frame-by-frame
+ inference_start_time = time.time()
+ ret, frame = cap.read()
+
+ if ret == True:
+ output = self.make_prediction(frame)
+ inference_end_time = time.time()
+ fps = round(1/(inference_end_time - inference_start_time))
+ cv2.putText(output, f'FPS: {fps}', (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (10, 255, 100), 2)
+ cv2.imshow('MiDaS Depth Estimation - Press Escape to close window ', output)
+
+ # Press ESC on keyboard to exit
+ if cv2.waitKey(1) == 27: # Escape key
+ break
+
+ else:
+ break
+
+
+ # When everything done, release
+ # the video capture object
+ cap.release()
+
+ # Closes all the frames
+ cv2.destroyAllWindows()
+
+
+
+if __name__ == "__main__":
+ # params
+ INPUT_PATH = "assets/videos/testvideo2.mp4"
+
+ os.environ['CUDA_VISIBLE_DEVICES'] = '0'
+
+ # set torch options
+ torch.backends.cudnn.enabled = True
+ torch.backends.cudnn.benchmark = True
+
+ depth_estimator = MonocularDepthEstimator(side_by_side=False)
+ depth_estimator.run(INPUT_PATH)
+
diff --git a/point_cloud_generator.py b/point_cloud_generator.py
new file mode 100644
index 0000000000000000000000000000000000000000..9804f2bd742449f126a58c3c68266589ef112aa9
--- /dev/null
+++ b/point_cloud_generator.py
@@ -0,0 +1,132 @@
+import cv2
+import numpy as np
+import matplotlib.pyplot as plt
+import open3d as o3d
+
+
+
+
+
+# print(pcd)
+# skip = 100 # Skip every n points
+
+# fig = plt.figure()
+# ax = fig.add_subplot(111, projection='3d')
+# point_range = range(0, pcd.shape[0], skip) # skip points to prevent crash
+# ax.scatter(pcd[point_range, 0], # x
+# pcd[point_range, 1], # y
+# pcd[point_range, 2], # z
+# c=pcd[point_range, 2], # height data for color
+# cmap='spectral',
+# marker="x")
+# ax.axis('scaled') # {equal, scaled}
+# plt.show()
+
+# pcd_o3d = o3d.geometry.PointCloud() # create point cloud object
+# pcd_o3d.points = o3d.utility.Vector3dVector(pcd) # set pcd_np as the point cloud points
+# # Visualize:
+# o3d.visualization.draw_geometries([pcd_o3d])
+
+
+class PointCloudGenerator:
+ def __init__(self):
+ # Depth camera parameters:
+ self.fx_depth = 5.8262448167737955e+02
+ self.fy_depth = 5.8269103270988637e+02
+ self.cx_depth = 3.1304475870804731e+02
+ self.cy_depth = 2.3844389626620386e+02
+
+ def conver_to_point_cloud_v1(self, depth_img):
+
+ pcd = []
+ height, width = depth_img.shape
+ for i in range(height):
+ for j in range(width):
+ z = depth_img[i][j]
+ x = (j - self.cx_depth) * z / self.fx_depth
+ y = (i - self.cy_depth) * z / self.fy_depth
+ pcd.append([x, y, z])
+
+ return pcd
+
+ def conver_to_point_cloud_v2(self, depth_img):
+
+ # get depth resolution:
+ height, width = depth_img.shape
+ length = height * width
+
+ # compute indices:
+ jj = np.tile(range(width), height)
+ ii = np.repeat(range(height), width)
+
+ # rechape depth image
+ z = depth_img.reshape(length)
+ # compute pcd:
+ pcd = np.dstack([(ii - self.cx_depth) * z / self.fx_depth,
+ (jj - self.cy_depth) * z / self.fy_depth,
+ z]).reshape((length, 3))
+
+ return pcd
+
+ def generate_point_cloud(self, image_path, vectorize=False):
+ depth_img = cv2.imread(image_path, 0)
+
+ print(f"Image resolution: {depth_img.shape}")
+ print(f"Data type: {depth_img.dtype}")
+ print(f"Min value: {np.min(depth_img)}")
+ print(f"Max value: {np.max(depth_img)}")
+
+
+ # normalizing depth image
+ depth_min = depth_img.min()
+ depth_max = depth_img.max()
+ normalized_depth = 255 * ((depth_img - depth_min) / (depth_max - depth_min))
+
+ depth_img = normalized_depth
+ print("After normalization: ")
+ print(f"Image resolution: {depth_img.shape}")
+ print(f"Data type: {depth_img.dtype}")
+ print(f"Min value: {np.min(depth_img)}")
+ print(f"Max value: {np.max(depth_img)}")
+
+
+ # convert depth to point cloud
+ if not vectorize:
+ self.pcd = self.conver_to_point_cloud_v1(depth_img)
+ if vectorize:
+ self.pcd = self.conver_to_point_cloud_v2(depth_img)
+
+
+ return self.pcd
+
+ def viz_point_cloud(self, use_matplotlib=False):
+
+ points = np.array(self.pcd)
+ skip = 200
+ point_range = range(0, points.shape[0], skip) # skip points to prevent crash
+
+ if use_matplotlib:
+ fig = plt.figure()
+ ax = fig.add_subplot(111, projection='3d')
+ ax.scatter(points[point_range, 0], points[point_range, 1], points[point_range, 2], c='r', marker='o')
+ ax.set_xlabel('X Label')
+ ax.set_ylabel('Y Label')
+ ax.set_zlabel('Z Label')
+ plt.show()
+
+ if not use_matplotlib:
+
+ pcd_o3d = o3d.geometry.PointCloud() # create point cloud object
+ pcd_o3d.points = o3d.utility.Vector3dVector(pcd) # set pcd_np as the point cloud points
+ # Visualize:
+ o3d.visualization.draw_geometries([pcd_o3d])
+
+
+if __name__ == "__main__":
+ input_image = "test/inputs/depth.png"
+ point_cloud_gen = PointCloudGenerator()
+ pcd = point_cloud_gen.generate_point_cloud(input_image)
+ point_cloud_gen.viz_point_cloud()
+
+
+
diff --git a/requirements.txt b/requirements.txt
new file mode 100644
index 0000000000000000000000000000000000000000..2534b56696410d0f815139f4a296cf75ef876994
--- /dev/null
+++ b/requirements.txt
@@ -0,0 +1,46 @@
+# Ultralytics requirements
+# Usage: pip install -r requirements.txt
+
+# Base ----------------------------------------
+matplotlib>=3.2.2
+numpy>=1.21.6
+opencv-python>=4.6.0
+Pillow>=7.1.2
+PyYAML>=5.3.1
+requests>=2.23.0
+scipy>=1.4.1
+torch>=1.7.0
+torchvision>=0.8.1
+tqdm>=4.64.0
+gradio>=3.36.1
+ultralytics>=8.0.132
+urllib3>=1.26.14
+
+# Logging -------------------------------------
+# tensorboard>=2.4.1
+# clearml
+# comet
+
+# Plotting ------------------------------------
+pandas>=1.1.4
+seaborn>=0.11.0
+
+# Export --------------------------------------
+# coremltools>=6.0 # CoreML export
+# onnx>=1.12.0 # ONNX export
+# onnxsim>=0.4.1 # ONNX simplifier
+# nvidia-pyindex # TensorRT export
+# nvidia-tensorrt # TensorRT export
+# scikit-learn==0.19.2 # CoreML quantization
+# tensorflow>=2.4.1 # TF exports (-cpu, -aarch64, -macos)
+# tflite-support
+# tensorflowjs>=3.9.0 # TF.js export
+# openvino-dev>=2022.3 # OpenVINO export
+
+# Extras --------------------------------------
+psutil # system utilization
+thop>=0.1.1 # FLOPs computation
+# ipython # interactive notebook
+# albumentations>=1.0.3
+# pycocotools>=2.0.6 # COCO mAP
+# roboflow
\ No newline at end of file
diff --git a/yolov8/.gitignore b/yolov8/.gitignore
new file mode 100644
index 0000000000000000000000000000000000000000..f8dcff9765990f250bf5c7a28044b13cf143e911
--- /dev/null
+++ b/yolov8/.gitignore
@@ -0,0 +1,156 @@
+# Byte-compiled / optimized / DLL files
+__pycache__/
+*.py[cod]
+*$py.class
+
+# C extensions
+*.so
+
+# Distribution / packaging
+.Python
+build/
+develop-eggs/
+dist/
+downloads/
+eggs/
+.eggs/
+lib/
+lib64/
+parts/
+sdist/
+var/
+wheels/
+pip-wheel-metadata/
+share/python-wheels/
+*.egg-info/
+.installed.cfg
+*.egg
+MANIFEST
+
+# PyInstaller
+# Usually these files are written by a python script from a template
+# before PyInstaller builds the exe, so as to inject date/other infos into it.
+*.manifest
+*.spec
+
+# Installer logs
+pip-log.txt
+pip-delete-this-directory.txt
+
+# Unit test / coverage reports
+htmlcov/
+.tox/
+.nox/
+.coverage
+.coverage.*
+.cache
+nosetests.xml
+coverage.xml
+*.cover
+*.py,cover
+.hypothesis/
+.pytest_cache/
+
+# Translations
+*.mo
+*.pot
+
+# Django stuff:
+*.log
+local_settings.py
+db.sqlite3
+db.sqlite3-journal
+
+# Flask stuff:
+instance/
+.webassets-cache
+
+# Scrapy stuff:
+.scrapy
+
+# Sphinx documentation
+docs/_build/
+
+# PyBuilder
+target/
+
+# Jupyter Notebook
+.ipynb_checkpoints
+
+# IPython
+profile_default/
+ipython_config.py
+
+# Profiling
+*.pclprof
+
+# pyenv
+.python-version
+
+# pipenv
+# According to pypa/pipenv#598, it is recommended to include Pipfile.lock in version control.
+# However, in case of collaboration, if having platform-specific dependencies or dependencies
+# having no cross-platform support, pipenv may install dependencies that don't work, or not
+# install all needed dependencies.
+#Pipfile.lock
+
+# PEP 582; used by e.g. github.com/David-OConnor/pyflow
+__pypackages__/
+
+# Celery stuff
+celerybeat-schedule
+celerybeat.pid
+
+# SageMath parsed files
+*.sage.py
+
+# Environments
+.env
+.venv
+.idea
+env/
+venv/
+ENV/
+env.bak/
+venv.bak/
+
+# Spyder project settings
+.spyderproject
+.spyproject
+
+# Rope project settings
+.ropeproject
+
+# mkdocs documentation
+/site
+
+# mypy
+.mypy_cache/
+.dmypy.json
+dmypy.json
+
+# Pyre type checker
+.pyre/
+
+# datasets and projects
+datasets/
+runs/
+wandb/
+
+.DS_Store
+
+# Neural Network weights -----------------------------------------------------------------------------------------------
+weights/
+*.weights
+*.pt
+*.pb
+*.onnx
+*.engine
+*.mlmodel
+*.torchscript
+*.tflite
+*.h5
+*_saved_model/
+*_web_model/
+*_openvino_model/
+*_paddle_model/
diff --git a/yolov8/.pre-commit-config.yaml b/yolov8/.pre-commit-config.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..192a0ff3cba277d171ef8323928f9eeec2c39c02
--- /dev/null
+++ b/yolov8/.pre-commit-config.yaml
@@ -0,0 +1,73 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# Pre-commit hooks. For more information see https://github.com/pre-commit/pre-commit-hooks/blob/main/README.md
+
+exclude: 'docs/'
+# Define bot property if installed via https://github.com/marketplace/pre-commit-ci
+ci:
+ autofix_prs: true
+ autoupdate_commit_msg: '[pre-commit.ci] pre-commit suggestions'
+ autoupdate_schedule: monthly
+ # submodules: true
+
+repos:
+ - repo: https://github.com/pre-commit/pre-commit-hooks
+ rev: v4.4.0
+ hooks:
+ - id: end-of-file-fixer
+ - id: trailing-whitespace
+ - id: check-case-conflict
+ # - id: check-yaml
+ - id: check-docstring-first
+ - id: double-quote-string-fixer
+ - id: detect-private-key
+
+ - repo: https://github.com/asottile/pyupgrade
+ rev: v3.8.0
+ hooks:
+ - id: pyupgrade
+ name: Upgrade code
+
+ - repo: https://github.com/PyCQA/isort
+ rev: 5.12.0
+ hooks:
+ - id: isort
+ name: Sort imports
+
+ - repo: https://github.com/google/yapf
+ rev: v0.40.0
+ hooks:
+ - id: yapf
+ name: YAPF formatting
+
+ - repo: https://github.com/executablebooks/mdformat
+ rev: 0.7.16
+ hooks:
+ - id: mdformat
+ name: MD formatting
+ additional_dependencies:
+ - mdformat-gfm
+ - mdformat-black
+ # exclude: "README.md|README.zh-CN.md|CONTRIBUTING.md"
+
+ - repo: https://github.com/PyCQA/flake8
+ rev: 6.0.0
+ hooks:
+ - id: flake8
+ name: PEP8
+
+ - repo: https://github.com/codespell-project/codespell
+ rev: v2.2.5
+ hooks:
+ - id: codespell
+ args:
+ - --ignore-words-list=crate,nd,strack,dota
+
+# - repo: https://github.com/asottile/yesqa
+# rev: v1.4.0
+# hooks:
+# - id: yesqa
+
+# - repo: https://github.com/asottile/dead
+# rev: v1.5.0
+# hooks:
+# - id: dead
diff --git a/yolov8/CITATION.cff b/yolov8/CITATION.cff
new file mode 100644
index 0000000000000000000000000000000000000000..8e85b7a7519db1054fe4d73805cea5d4ec5a2c3b
--- /dev/null
+++ b/yolov8/CITATION.cff
@@ -0,0 +1,20 @@
+cff-version: 1.2.0
+preferred-citation:
+ type: software
+ message: If you use this software, please cite it as below.
+ authors:
+ - family-names: Jocher
+ given-names: Glenn
+ orcid: "https://orcid.org/0000-0001-5950-6979"
+ - family-names: Chaurasia
+ given-names: Ayush
+ orcid: "https://orcid.org/0000-0002-7603-6750"
+ - family-names: Qiu
+ given-names: Jing
+ orcid: "https://orcid.org/0000-0003-3783-7069"
+ title: "YOLO by Ultralytics"
+ version: 8.0.0
+ # doi: 10.5281/zenodo.3908559 # TODO
+ date-released: 2023-1-10
+ license: AGPL-3.0
+ url: "https://github.com/ultralytics/ultralytics"
diff --git a/yolov8/CONTRIBUTING.md b/yolov8/CONTRIBUTING.md
new file mode 100644
index 0000000000000000000000000000000000000000..93269066416c297580e3ed96c9549cd4c7ac3c5f
--- /dev/null
+++ b/yolov8/CONTRIBUTING.md
@@ -0,0 +1,115 @@
+## Contributing to YOLOv8 🚀
+
+We love your input! We want to make contributing to YOLOv8 as easy and transparent as possible, whether it's:
+
+- Reporting a bug
+- Discussing the current state of the code
+- Submitting a fix
+- Proposing a new feature
+- Becoming a maintainer
+
+YOLOv8 works so well due to our combined community effort, and for every small improvement you contribute you will be
+helping push the frontiers of what's possible in AI 😃!
+
+## Submitting a Pull Request (PR) 🛠️
+
+Submitting a PR is easy! This example shows how to submit a PR for updating `requirements.txt` in 4 steps:
+
+### 1. Select File to Update
+
+Select `requirements.txt` to update by clicking on it in GitHub.
+
+
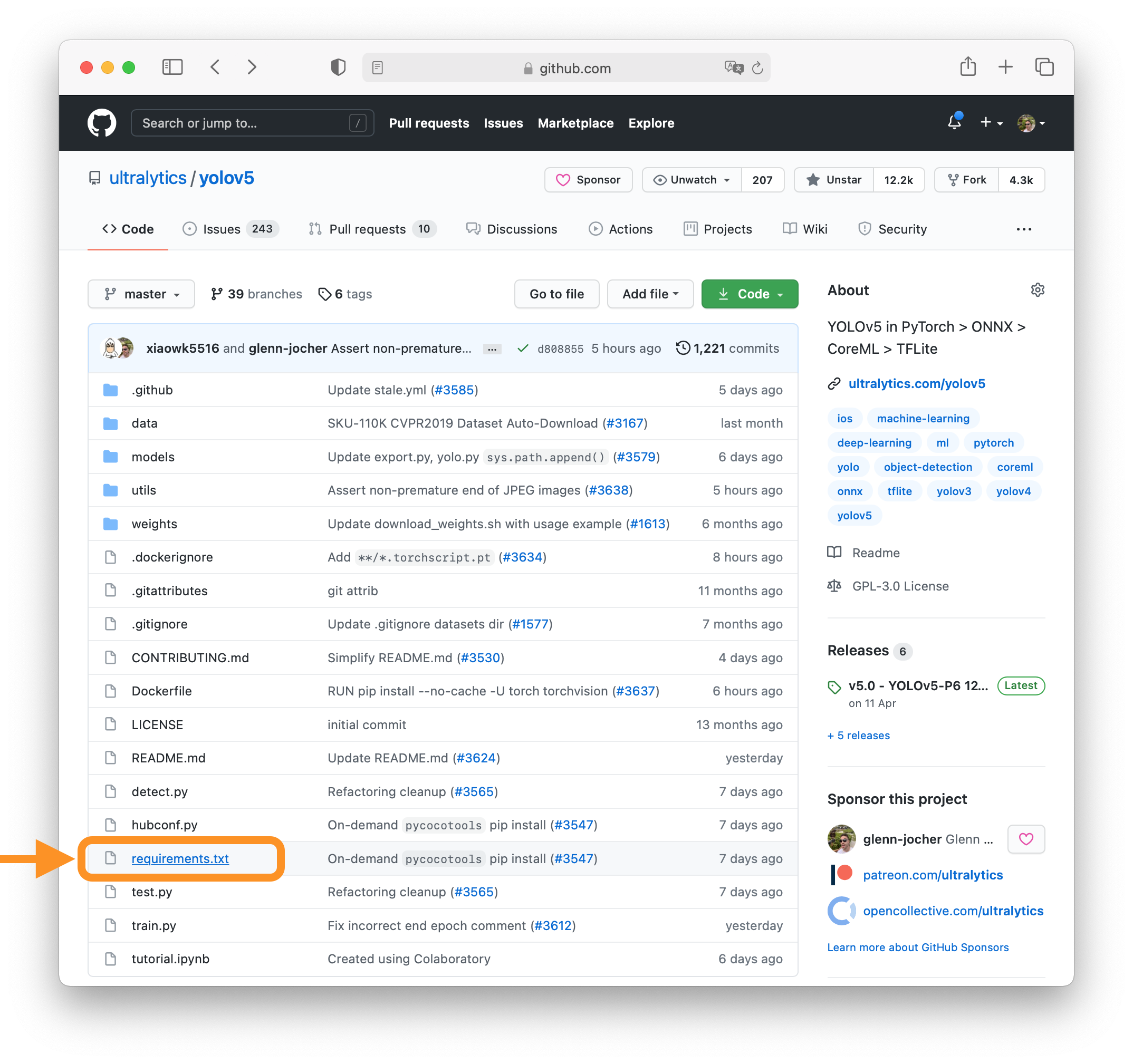
+
+### 2. Click 'Edit this file'
+
+Button is in top-right corner.
+
+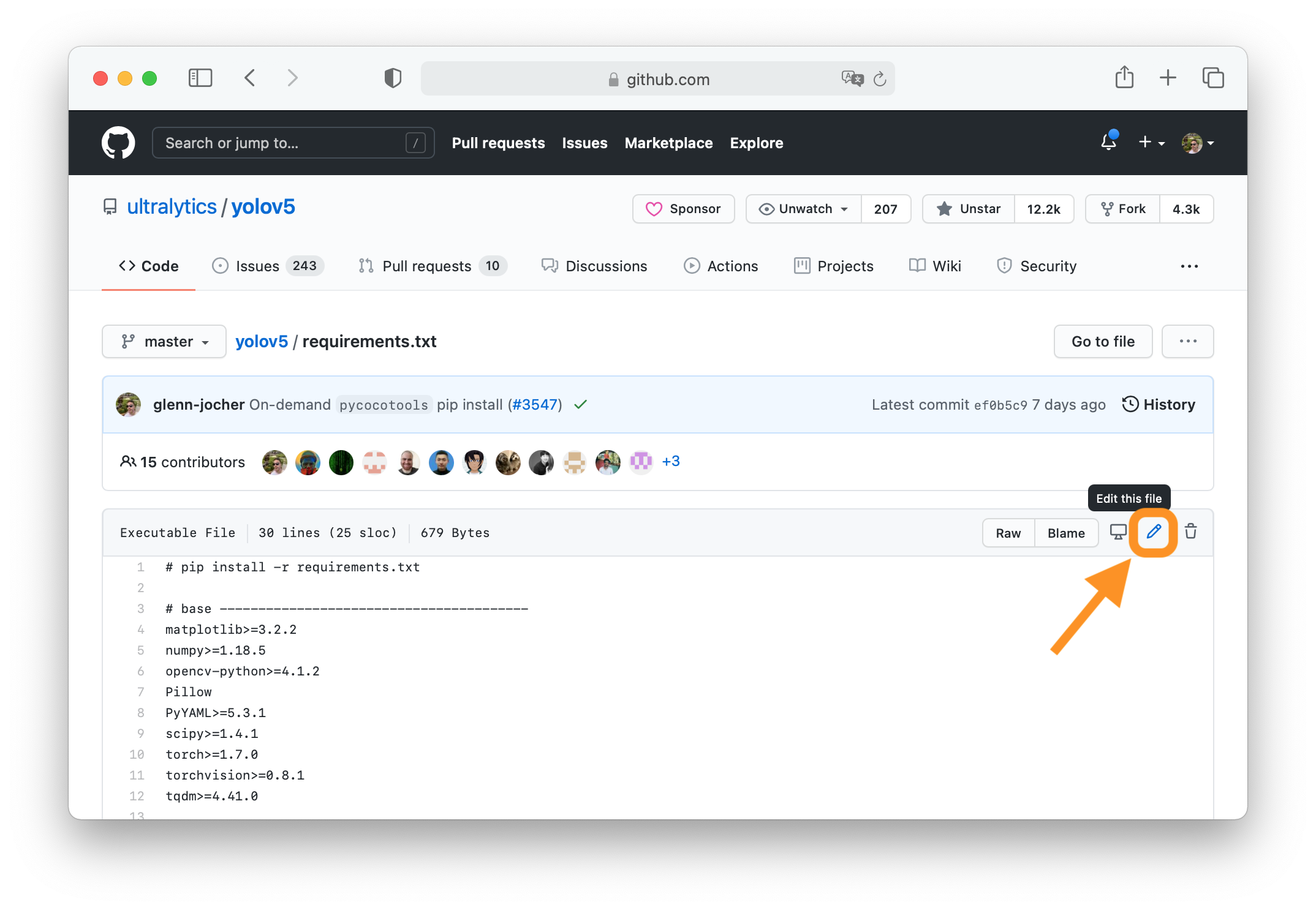
+
+### 3. Make Changes
+
+Change `matplotlib` version from `3.2.2` to `3.3`.
+
+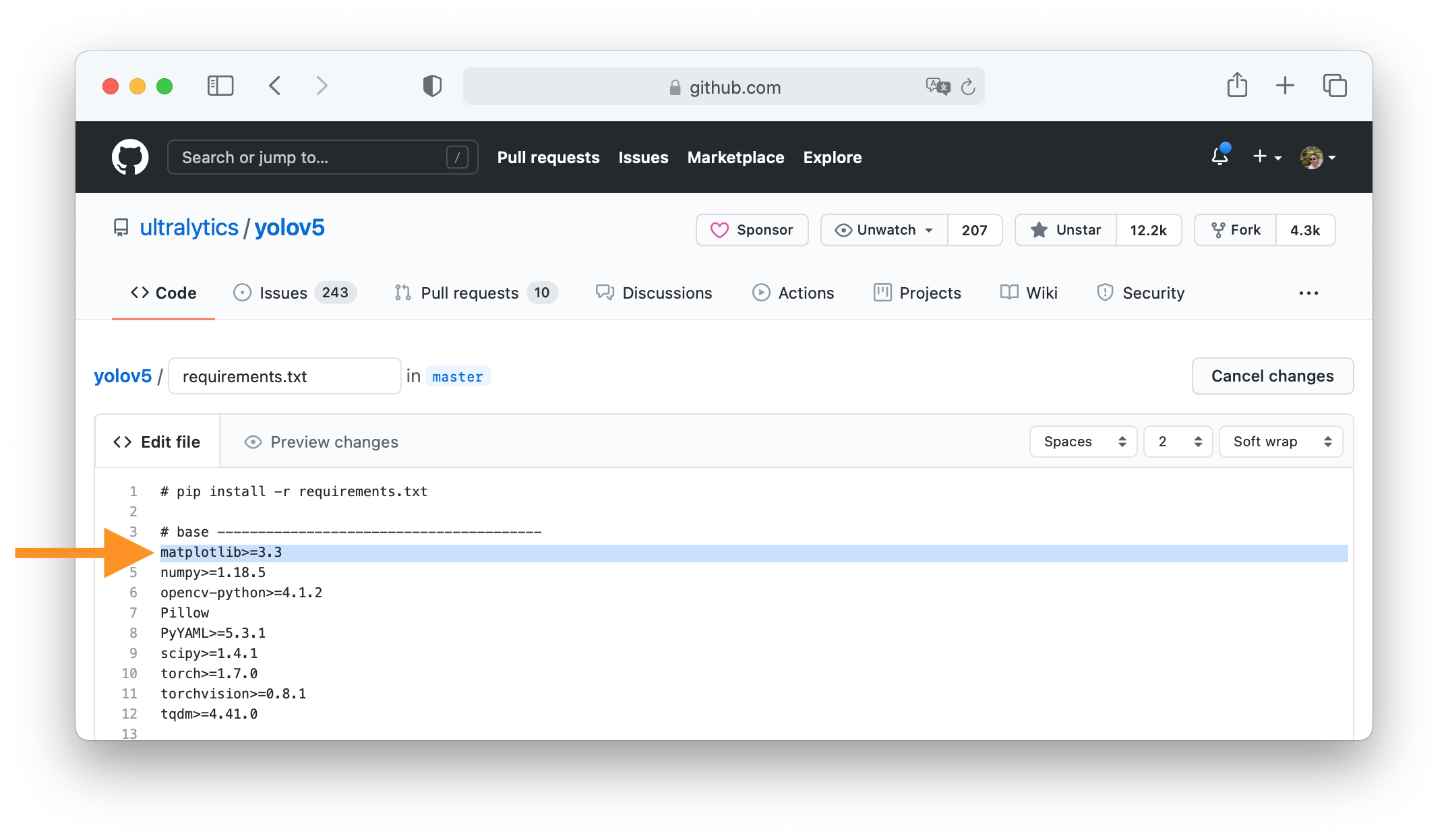
+
+### 4. Preview Changes and Submit PR
+
+Click on the **Preview changes** tab to verify your updates. At the bottom of the screen select 'Create a **new branch**
+for this commit', assign your branch a descriptive name such as `fix/matplotlib_version` and click the green **Propose
+changes** button. All done, your PR is now submitted to YOLOv8 for review and approval 😃!
+
+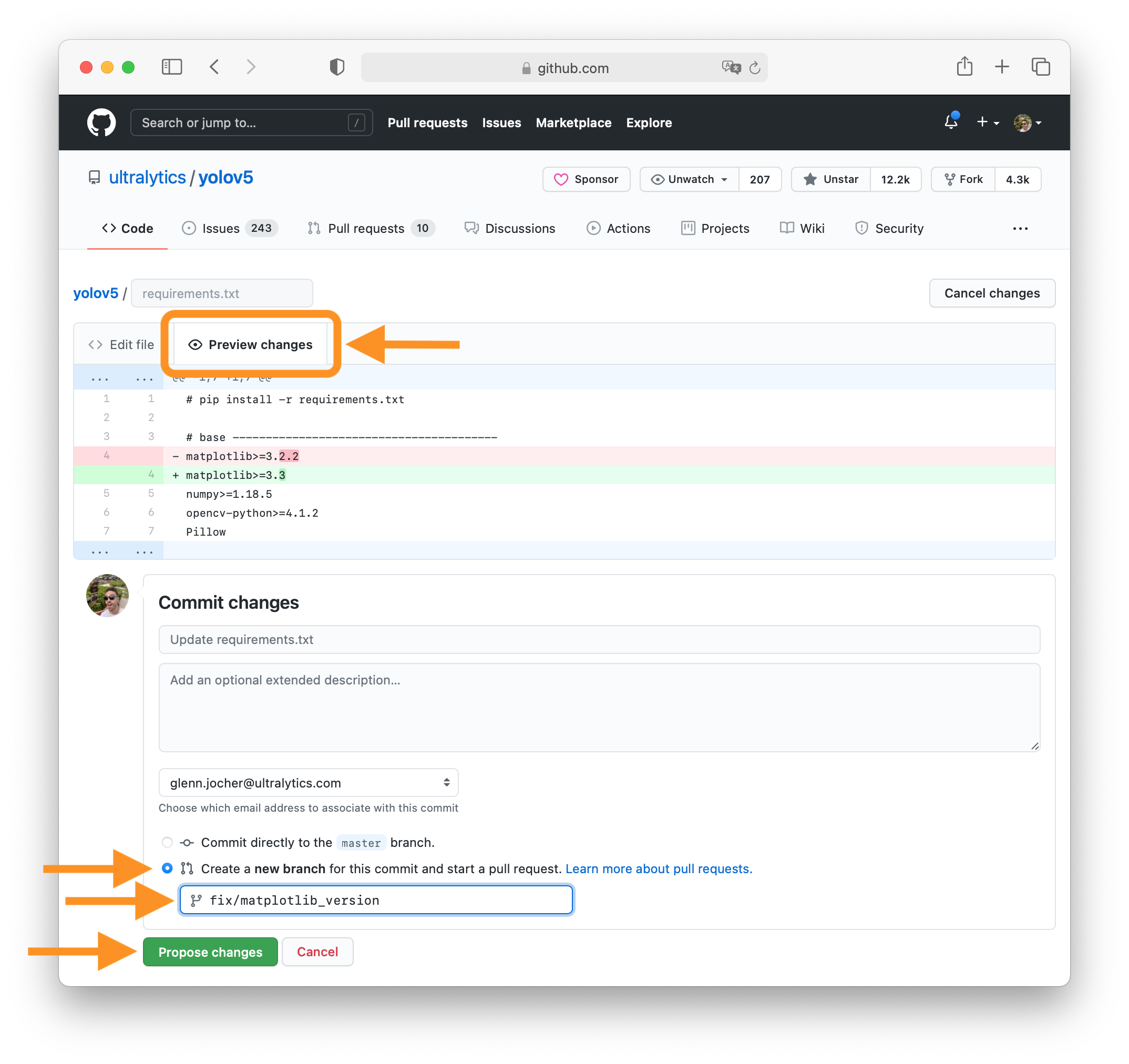
+
+### PR recommendations
+
+To allow your work to be integrated as seamlessly as possible, we advise you to:
+
+- ✅ Verify your PR is **up-to-date** with `ultralytics/ultralytics` `main` branch. If your PR is behind you can update
+ your code by clicking the 'Update branch' button or by running `git pull` and `git merge main` locally.
+
+
+
+- ✅ Verify all YOLOv8 Continuous Integration (CI) **checks are passing**.
+
+
+
+- ✅ Reduce changes to the absolute **minimum** required for your bug fix or feature addition. _"It is not daily increase
+ but daily decrease, hack away the unessential. The closer to the source, the less wastage there is."_ — Bruce Lee
+
+### Docstrings
+
+Not all functions or classes require docstrings but when they do, we
+follow [google-style docstrings format](https://google.github.io/styleguide/pyguide.html#38-comments-and-docstrings).
+Here is an example:
+
+```python
+"""
+ What the function does. Performs NMS on given detection predictions.
+
+ Args:
+ arg1: The description of the 1st argument
+ arg2: The description of the 2nd argument
+
+ Returns:
+ What the function returns. Empty if nothing is returned.
+
+ Raises:
+ Exception Class: When and why this exception can be raised by the function.
+"""
+```
+
+## Submitting a Bug Report 🐛
+
+If you spot a problem with YOLOv8 please submit a Bug Report!
+
+For us to start investigating a possible problem we need to be able to reproduce it ourselves first. We've created a few
+short guidelines below to help users provide what we need in order to get started.
+
+When asking a question, people will be better able to provide help if you provide **code** that they can easily
+understand and use to **reproduce** the problem. This is referred to by community members as creating
+a [minimum reproducible example](https://docs.ultralytics.com/help/minimum_reproducible_example/). Your code that reproduces
+the problem should be:
+
+- ✅ **Minimal** – Use as little code as possible that still produces the same problem
+- ✅ **Complete** – Provide **all** parts someone else needs to reproduce your problem in the question itself
+- ✅ **Reproducible** – Test the code you're about to provide to make sure it reproduces the problem
+
+In addition to the above requirements, for [Ultralytics](https://ultralytics.com/) to provide assistance your code
+should be:
+
+- ✅ **Current** – Verify that your code is up-to-date with current
+ GitHub [main](https://github.com/ultralytics/ultralytics/tree/main) branch, and if necessary `git pull` or `git clone`
+ a new copy to ensure your problem has not already been resolved by previous commits.
+- ✅ **Unmodified** – Your problem must be reproducible without any modifications to the codebase in this
+ repository. [Ultralytics](https://ultralytics.com/) does not provide support for custom code ⚠️.
+
+If you believe your problem meets all of the above criteria, please close this issue and raise a new one using the 🐛
+**Bug Report** [template](https://github.com/ultralytics/ultralytics/issues/new/choose) and providing
+a [minimum reproducible example](https://docs.ultralytics.com/help/minimum_reproducible_example/) to help us better
+understand and diagnose your problem.
+
+## License
+
+By contributing, you agree that your contributions will be licensed under
+the [AGPL-3.0 license](https://choosealicense.com/licenses/agpl-3.0/)
diff --git a/yolov8/LICENSE b/yolov8/LICENSE
new file mode 100644
index 0000000000000000000000000000000000000000..be3f7b28e564e7dd05eaf59d64adba1a4065ac0e
--- /dev/null
+++ b/yolov8/LICENSE
@@ -0,0 +1,661 @@
+ GNU AFFERO GENERAL PUBLIC LICENSE
+ Version 3, 19 November 2007
+
+ Copyright (C) 2007 Free Software Foundation, Inc.
+ Everyone is permitted to copy and distribute verbatim copies
+ of this license document, but changing it is not allowed.
+
+ Preamble
+
+ The GNU Affero General Public License is a free, copyleft license for
+software and other kinds of works, specifically designed to ensure
+cooperation with the community in the case of network server software.
+
+ The licenses for most software and other practical works are designed
+to take away your freedom to share and change the works. By contrast,
+our General Public Licenses are intended to guarantee your freedom to
+share and change all versions of a program--to make sure it remains free
+software for all its users.
+
+ When we speak of free software, we are referring to freedom, not
+price. Our General Public Licenses are designed to make sure that you
+have the freedom to distribute copies of free software (and charge for
+them if you wish), that you receive source code or can get it if you
+want it, that you can change the software or use pieces of it in new
+free programs, and that you know you can do these things.
+
+ Developers that use our General Public Licenses protect your rights
+with two steps: (1) assert copyright on the software, and (2) offer
+you this License which gives you legal permission to copy, distribute
+and/or modify the software.
+
+ A secondary benefit of defending all users' freedom is that
+improvements made in alternate versions of the program, if they
+receive widespread use, become available for other developers to
+incorporate. Many developers of free software are heartened and
+encouraged by the resulting cooperation. However, in the case of
+software used on network servers, this result may fail to come about.
+The GNU General Public License permits making a modified version and
+letting the public access it on a server without ever releasing its
+source code to the public.
+
+ The GNU Affero General Public License is designed specifically to
+ensure that, in such cases, the modified source code becomes available
+to the community. It requires the operator of a network server to
+provide the source code of the modified version running there to the
+users of that server. Therefore, public use of a modified version, on
+a publicly accessible server, gives the public access to the source
+code of the modified version.
+
+ An older license, called the Affero General Public License and
+published by Affero, was designed to accomplish similar goals. This is
+a different license, not a version of the Affero GPL, but Affero has
+released a new version of the Affero GPL which permits relicensing under
+this license.
+
+ The precise terms and conditions for copying, distribution and
+modification follow.
+
+ TERMS AND CONDITIONS
+
+ 0. Definitions.
+
+ "This License" refers to version 3 of the GNU Affero General Public License.
+
+ "Copyright" also means copyright-like laws that apply to other kinds of
+works, such as semiconductor masks.
+
+ "The Program" refers to any copyrightable work licensed under this
+License. Each licensee is addressed as "you". "Licensees" and
+"recipients" may be individuals or organizations.
+
+ To "modify" a work means to copy from or adapt all or part of the work
+in a fashion requiring copyright permission, other than the making of an
+exact copy. The resulting work is called a "modified version" of the
+earlier work or a work "based on" the earlier work.
+
+ A "covered work" means either the unmodified Program or a work based
+on the Program.
+
+ To "propagate" a work means to do anything with it that, without
+permission, would make you directly or secondarily liable for
+infringement under applicable copyright law, except executing it on a
+computer or modifying a private copy. Propagation includes copying,
+distribution (with or without modification), making available to the
+public, and in some countries other activities as well.
+
+ To "convey" a work means any kind of propagation that enables other
+parties to make or receive copies. Mere interaction with a user through
+a computer network, with no transfer of a copy, is not conveying.
+
+ An interactive user interface displays "Appropriate Legal Notices"
+to the extent that it includes a convenient and prominently visible
+feature that (1) displays an appropriate copyright notice, and (2)
+tells the user that there is no warranty for the work (except to the
+extent that warranties are provided), that licensees may convey the
+work under this License, and how to view a copy of this License. If
+the interface presents a list of user commands or options, such as a
+menu, a prominent item in the list meets this criterion.
+
+ 1. Source Code.
+
+ The "source code" for a work means the preferred form of the work
+for making modifications to it. "Object code" means any non-source
+form of a work.
+
+ A "Standard Interface" means an interface that either is an official
+standard defined by a recognized standards body, or, in the case of
+interfaces specified for a particular programming language, one that
+is widely used among developers working in that language.
+
+ The "System Libraries" of an executable work include anything, other
+than the work as a whole, that (a) is included in the normal form of
+packaging a Major Component, but which is not part of that Major
+Component, and (b) serves only to enable use of the work with that
+Major Component, or to implement a Standard Interface for which an
+implementation is available to the public in source code form. A
+"Major Component", in this context, means a major essential component
+(kernel, window system, and so on) of the specific operating system
+(if any) on which the executable work runs, or a compiler used to
+produce the work, or an object code interpreter used to run it.
+
+ The "Corresponding Source" for a work in object code form means all
+the source code needed to generate, install, and (for an executable
+work) run the object code and to modify the work, including scripts to
+control those activities. However, it does not include the work's
+System Libraries, or general-purpose tools or generally available free
+programs which are used unmodified in performing those activities but
+which are not part of the work. For example, Corresponding Source
+includes interface definition files associated with source files for
+the work, and the source code for shared libraries and dynamically
+linked subprograms that the work is specifically designed to require,
+such as by intimate data communication or control flow between those
+subprograms and other parts of the work.
+
+ The Corresponding Source need not include anything that users
+can regenerate automatically from other parts of the Corresponding
+Source.
+
+ The Corresponding Source for a work in source code form is that
+same work.
+
+ 2. Basic Permissions.
+
+ All rights granted under this License are granted for the term of
+copyright on the Program, and are irrevocable provided the stated
+conditions are met. This License explicitly affirms your unlimited
+permission to run the unmodified Program. The output from running a
+covered work is covered by this License only if the output, given its
+content, constitutes a covered work. This License acknowledges your
+rights of fair use or other equivalent, as provided by copyright law.
+
+ You may make, run and propagate covered works that you do not
+convey, without conditions so long as your license otherwise remains
+in force. You may convey covered works to others for the sole purpose
+of having them make modifications exclusively for you, or provide you
+with facilities for running those works, provided that you comply with
+the terms of this License in conveying all material for which you do
+not control copyright. Those thus making or running the covered works
+for you must do so exclusively on your behalf, under your direction
+and control, on terms that prohibit them from making any copies of
+your copyrighted material outside their relationship with you.
+
+ Conveying under any other circumstances is permitted solely under
+the conditions stated below. Sublicensing is not allowed; section 10
+makes it unnecessary.
+
+ 3. Protecting Users' Legal Rights From Anti-Circumvention Law.
+
+ No covered work shall be deemed part of an effective technological
+measure under any applicable law fulfilling obligations under article
+11 of the WIPO copyright treaty adopted on 20 December 1996, or
+similar laws prohibiting or restricting circumvention of such
+measures.
+
+ When you convey a covered work, you waive any legal power to forbid
+circumvention of technological measures to the extent such circumvention
+is effected by exercising rights under this License with respect to
+the covered work, and you disclaim any intention to limit operation or
+modification of the work as a means of enforcing, against the work's
+users, your or third parties' legal rights to forbid circumvention of
+technological measures.
+
+ 4. Conveying Verbatim Copies.
+
+ You may convey verbatim copies of the Program's source code as you
+receive it, in any medium, provided that you conspicuously and
+appropriately publish on each copy an appropriate copyright notice;
+keep intact all notices stating that this License and any
+non-permissive terms added in accord with section 7 apply to the code;
+keep intact all notices of the absence of any warranty; and give all
+recipients a copy of this License along with the Program.
+
+ You may charge any price or no price for each copy that you convey,
+and you may offer support or warranty protection for a fee.
+
+ 5. Conveying Modified Source Versions.
+
+ You may convey a work based on the Program, or the modifications to
+produce it from the Program, in the form of source code under the
+terms of section 4, provided that you also meet all of these conditions:
+
+ a) The work must carry prominent notices stating that you modified
+ it, and giving a relevant date.
+
+ b) The work must carry prominent notices stating that it is
+ released under this License and any conditions added under section
+ 7. This requirement modifies the requirement in section 4 to
+ "keep intact all notices".
+
+ c) You must license the entire work, as a whole, under this
+ License to anyone who comes into possession of a copy. This
+ License will therefore apply, along with any applicable section 7
+ additional terms, to the whole of the work, and all its parts,
+ regardless of how they are packaged. This License gives no
+ permission to license the work in any other way, but it does not
+ invalidate such permission if you have separately received it.
+
+ d) If the work has interactive user interfaces, each must display
+ Appropriate Legal Notices; however, if the Program has interactive
+ interfaces that do not display Appropriate Legal Notices, your
+ work need not make them do so.
+
+ A compilation of a covered work with other separate and independent
+works, which are not by their nature extensions of the covered work,
+and which are not combined with it such as to form a larger program,
+in or on a volume of a storage or distribution medium, is called an
+"aggregate" if the compilation and its resulting copyright are not
+used to limit the access or legal rights of the compilation's users
+beyond what the individual works permit. Inclusion of a covered work
+in an aggregate does not cause this License to apply to the other
+parts of the aggregate.
+
+ 6. Conveying Non-Source Forms.
+
+ You may convey a covered work in object code form under the terms
+of sections 4 and 5, provided that you also convey the
+machine-readable Corresponding Source under the terms of this License,
+in one of these ways:
+
+ a) Convey the object code in, or embodied in, a physical product
+ (including a physical distribution medium), accompanied by the
+ Corresponding Source fixed on a durable physical medium
+ customarily used for software interchange.
+
+ b) Convey the object code in, or embodied in, a physical product
+ (including a physical distribution medium), accompanied by a
+ written offer, valid for at least three years and valid for as
+ long as you offer spare parts or customer support for that product
+ model, to give anyone who possesses the object code either (1) a
+ copy of the Corresponding Source for all the software in the
+ product that is covered by this License, on a durable physical
+ medium customarily used for software interchange, for a price no
+ more than your reasonable cost of physically performing this
+ conveying of source, or (2) access to copy the
+ Corresponding Source from a network server at no charge.
+
+ c) Convey individual copies of the object code with a copy of the
+ written offer to provide the Corresponding Source. This
+ alternative is allowed only occasionally and noncommercially, and
+ only if you received the object code with such an offer, in accord
+ with subsection 6b.
+
+ d) Convey the object code by offering access from a designated
+ place (gratis or for a charge), and offer equivalent access to the
+ Corresponding Source in the same way through the same place at no
+ further charge. You need not require recipients to copy the
+ Corresponding Source along with the object code. If the place to
+ copy the object code is a network server, the Corresponding Source
+ may be on a different server (operated by you or a third party)
+ that supports equivalent copying facilities, provided you maintain
+ clear directions next to the object code saying where to find the
+ Corresponding Source. Regardless of what server hosts the
+ Corresponding Source, you remain obligated to ensure that it is
+ available for as long as needed to satisfy these requirements.
+
+ e) Convey the object code using peer-to-peer transmission, provided
+ you inform other peers where the object code and Corresponding
+ Source of the work are being offered to the general public at no
+ charge under subsection 6d.
+
+ A separable portion of the object code, whose source code is excluded
+from the Corresponding Source as a System Library, need not be
+included in conveying the object code work.
+
+ A "User Product" is either (1) a "consumer product", which means any
+tangible personal property which is normally used for personal, family,
+or household purposes, or (2) anything designed or sold for incorporation
+into a dwelling. In determining whether a product is a consumer product,
+doubtful cases shall be resolved in favor of coverage. For a particular
+product received by a particular user, "normally used" refers to a
+typical or common use of that class of product, regardless of the status
+of the particular user or of the way in which the particular user
+actually uses, or expects or is expected to use, the product. A product
+is a consumer product regardless of whether the product has substantial
+commercial, industrial or non-consumer uses, unless such uses represent
+the only significant mode of use of the product.
+
+ "Installation Information" for a User Product means any methods,
+procedures, authorization keys, or other information required to install
+and execute modified versions of a covered work in that User Product from
+a modified version of its Corresponding Source. The information must
+suffice to ensure that the continued functioning of the modified object
+code is in no case prevented or interfered with solely because
+modification has been made.
+
+ If you convey an object code work under this section in, or with, or
+specifically for use in, a User Product, and the conveying occurs as
+part of a transaction in which the right of possession and use of the
+User Product is transferred to the recipient in perpetuity or for a
+fixed term (regardless of how the transaction is characterized), the
+Corresponding Source conveyed under this section must be accompanied
+by the Installation Information. But this requirement does not apply
+if neither you nor any third party retains the ability to install
+modified object code on the User Product (for example, the work has
+been installed in ROM).
+
+ The requirement to provide Installation Information does not include a
+requirement to continue to provide support service, warranty, or updates
+for a work that has been modified or installed by the recipient, or for
+the User Product in which it has been modified or installed. Access to a
+network may be denied when the modification itself materially and
+adversely affects the operation of the network or violates the rules and
+protocols for communication across the network.
+
+ Corresponding Source conveyed, and Installation Information provided,
+in accord with this section must be in a format that is publicly
+documented (and with an implementation available to the public in
+source code form), and must require no special password or key for
+unpacking, reading or copying.
+
+ 7. Additional Terms.
+
+ "Additional permissions" are terms that supplement the terms of this
+License by making exceptions from one or more of its conditions.
+Additional permissions that are applicable to the entire Program shall
+be treated as though they were included in this License, to the extent
+that they are valid under applicable law. If additional permissions
+apply only to part of the Program, that part may be used separately
+under those permissions, but the entire Program remains governed by
+this License without regard to the additional permissions.
+
+ When you convey a copy of a covered work, you may at your option
+remove any additional permissions from that copy, or from any part of
+it. (Additional permissions may be written to require their own
+removal in certain cases when you modify the work.) You may place
+additional permissions on material, added by you to a covered work,
+for which you have or can give appropriate copyright permission.
+
+ Notwithstanding any other provision of this License, for material you
+add to a covered work, you may (if authorized by the copyright holders of
+that material) supplement the terms of this License with terms:
+
+ a) Disclaiming warranty or limiting liability differently from the
+ terms of sections 15 and 16 of this License; or
+
+ b) Requiring preservation of specified reasonable legal notices or
+ author attributions in that material or in the Appropriate Legal
+ Notices displayed by works containing it; or
+
+ c) Prohibiting misrepresentation of the origin of that material, or
+ requiring that modified versions of such material be marked in
+ reasonable ways as different from the original version; or
+
+ d) Limiting the use for publicity purposes of names of licensors or
+ authors of the material; or
+
+ e) Declining to grant rights under trademark law for use of some
+ trade names, trademarks, or service marks; or
+
+ f) Requiring indemnification of licensors and authors of that
+ material by anyone who conveys the material (or modified versions of
+ it) with contractual assumptions of liability to the recipient, for
+ any liability that these contractual assumptions directly impose on
+ those licensors and authors.
+
+ All other non-permissive additional terms are considered "further
+restrictions" within the meaning of section 10. If the Program as you
+received it, or any part of it, contains a notice stating that it is
+governed by this License along with a term that is a further
+restriction, you may remove that term. If a license document contains
+a further restriction but permits relicensing or conveying under this
+License, you may add to a covered work material governed by the terms
+of that license document, provided that the further restriction does
+not survive such relicensing or conveying.
+
+ If you add terms to a covered work in accord with this section, you
+must place, in the relevant source files, a statement of the
+additional terms that apply to those files, or a notice indicating
+where to find the applicable terms.
+
+ Additional terms, permissive or non-permissive, may be stated in the
+form of a separately written license, or stated as exceptions;
+the above requirements apply either way.
+
+ 8. Termination.
+
+ You may not propagate or modify a covered work except as expressly
+provided under this License. Any attempt otherwise to propagate or
+modify it is void, and will automatically terminate your rights under
+this License (including any patent licenses granted under the third
+paragraph of section 11).
+
+ However, if you cease all violation of this License, then your
+license from a particular copyright holder is reinstated (a)
+provisionally, unless and until the copyright holder explicitly and
+finally terminates your license, and (b) permanently, if the copyright
+holder fails to notify you of the violation by some reasonable means
+prior to 60 days after the cessation.
+
+ Moreover, your license from a particular copyright holder is
+reinstated permanently if the copyright holder notifies you of the
+violation by some reasonable means, this is the first time you have
+received notice of violation of this License (for any work) from that
+copyright holder, and you cure the violation prior to 30 days after
+your receipt of the notice.
+
+ Termination of your rights under this section does not terminate the
+licenses of parties who have received copies or rights from you under
+this License. If your rights have been terminated and not permanently
+reinstated, you do not qualify to receive new licenses for the same
+material under section 10.
+
+ 9. Acceptance Not Required for Having Copies.
+
+ You are not required to accept this License in order to receive or
+run a copy of the Program. Ancillary propagation of a covered work
+occurring solely as a consequence of using peer-to-peer transmission
+to receive a copy likewise does not require acceptance. However,
+nothing other than this License grants you permission to propagate or
+modify any covered work. These actions infringe copyright if you do
+not accept this License. Therefore, by modifying or propagating a
+covered work, you indicate your acceptance of this License to do so.
+
+ 10. Automatic Licensing of Downstream Recipients.
+
+ Each time you convey a covered work, the recipient automatically
+receives a license from the original licensors, to run, modify and
+propagate that work, subject to this License. You are not responsible
+for enforcing compliance by third parties with this License.
+
+ An "entity transaction" is a transaction transferring control of an
+organization, or substantially all assets of one, or subdividing an
+organization, or merging organizations. If propagation of a covered
+work results from an entity transaction, each party to that
+transaction who receives a copy of the work also receives whatever
+licenses to the work the party's predecessor in interest had or could
+give under the previous paragraph, plus a right to possession of the
+Corresponding Source of the work from the predecessor in interest, if
+the predecessor has it or can get it with reasonable efforts.
+
+ You may not impose any further restrictions on the exercise of the
+rights granted or affirmed under this License. For example, you may
+not impose a license fee, royalty, or other charge for exercise of
+rights granted under this License, and you may not initiate litigation
+(including a cross-claim or counterclaim in a lawsuit) alleging that
+any patent claim is infringed by making, using, selling, offering for
+sale, or importing the Program or any portion of it.
+
+ 11. Patents.
+
+ A "contributor" is a copyright holder who authorizes use under this
+License of the Program or a work on which the Program is based. The
+work thus licensed is called the contributor's "contributor version".
+
+ A contributor's "essential patent claims" are all patent claims
+owned or controlled by the contributor, whether already acquired or
+hereafter acquired, that would be infringed by some manner, permitted
+by this License, of making, using, or selling its contributor version,
+but do not include claims that would be infringed only as a
+consequence of further modification of the contributor version. For
+purposes of this definition, "control" includes the right to grant
+patent sublicenses in a manner consistent with the requirements of
+this License.
+
+ Each contributor grants you a non-exclusive, worldwide, royalty-free
+patent license under the contributor's essential patent claims, to
+make, use, sell, offer for sale, import and otherwise run, modify and
+propagate the contents of its contributor version.
+
+ In the following three paragraphs, a "patent license" is any express
+agreement or commitment, however denominated, not to enforce a patent
+(such as an express permission to practice a patent or covenant not to
+sue for patent infringement). To "grant" such a patent license to a
+party means to make such an agreement or commitment not to enforce a
+patent against the party.
+
+ If you convey a covered work, knowingly relying on a patent license,
+and the Corresponding Source of the work is not available for anyone
+to copy, free of charge and under the terms of this License, through a
+publicly available network server or other readily accessible means,
+then you must either (1) cause the Corresponding Source to be so
+available, or (2) arrange to deprive yourself of the benefit of the
+patent license for this particular work, or (3) arrange, in a manner
+consistent with the requirements of this License, to extend the patent
+license to downstream recipients. "Knowingly relying" means you have
+actual knowledge that, but for the patent license, your conveying the
+covered work in a country, or your recipient's use of the covered work
+in a country, would infringe one or more identifiable patents in that
+country that you have reason to believe are valid.
+
+ If, pursuant to or in connection with a single transaction or
+arrangement, you convey, or propagate by procuring conveyance of, a
+covered work, and grant a patent license to some of the parties
+receiving the covered work authorizing them to use, propagate, modify
+or convey a specific copy of the covered work, then the patent license
+you grant is automatically extended to all recipients of the covered
+work and works based on it.
+
+ A patent license is "discriminatory" if it does not include within
+the scope of its coverage, prohibits the exercise of, or is
+conditioned on the non-exercise of one or more of the rights that are
+specifically granted under this License. You may not convey a covered
+work if you are a party to an arrangement with a third party that is
+in the business of distributing software, under which you make payment
+to the third party based on the extent of your activity of conveying
+the work, and under which the third party grants, to any of the
+parties who would receive the covered work from you, a discriminatory
+patent license (a) in connection with copies of the covered work
+conveyed by you (or copies made from those copies), or (b) primarily
+for and in connection with specific products or compilations that
+contain the covered work, unless you entered into that arrangement,
+or that patent license was granted, prior to 28 March 2007.
+
+ Nothing in this License shall be construed as excluding or limiting
+any implied license or other defenses to infringement that may
+otherwise be available to you under applicable patent law.
+
+ 12. No Surrender of Others' Freedom.
+
+ If conditions are imposed on you (whether by court order, agreement or
+otherwise) that contradict the conditions of this License, they do not
+excuse you from the conditions of this License. If you cannot convey a
+covered work so as to satisfy simultaneously your obligations under this
+License and any other pertinent obligations, then as a consequence you may
+not convey it at all. For example, if you agree to terms that obligate you
+to collect a royalty for further conveying from those to whom you convey
+the Program, the only way you could satisfy both those terms and this
+License would be to refrain entirely from conveying the Program.
+
+ 13. Remote Network Interaction; Use with the GNU General Public License.
+
+ Notwithstanding any other provision of this License, if you modify the
+Program, your modified version must prominently offer all users
+interacting with it remotely through a computer network (if your version
+supports such interaction) an opportunity to receive the Corresponding
+Source of your version by providing access to the Corresponding Source
+from a network server at no charge, through some standard or customary
+means of facilitating copying of software. This Corresponding Source
+shall include the Corresponding Source for any work covered by version 3
+of the GNU General Public License that is incorporated pursuant to the
+following paragraph.
+
+ Notwithstanding any other provision of this License, you have
+permission to link or combine any covered work with a work licensed
+under version 3 of the GNU General Public License into a single
+combined work, and to convey the resulting work. The terms of this
+License will continue to apply to the part which is the covered work,
+but the work with which it is combined will remain governed by version
+3 of the GNU General Public License.
+
+ 14. Revised Versions of this License.
+
+ The Free Software Foundation may publish revised and/or new versions of
+the GNU Affero General Public License from time to time. Such new versions
+will be similar in spirit to the present version, but may differ in detail to
+address new problems or concerns.
+
+ Each version is given a distinguishing version number. If the
+Program specifies that a certain numbered version of the GNU Affero General
+Public License "or any later version" applies to it, you have the
+option of following the terms and conditions either of that numbered
+version or of any later version published by the Free Software
+Foundation. If the Program does not specify a version number of the
+GNU Affero General Public License, you may choose any version ever published
+by the Free Software Foundation.
+
+ If the Program specifies that a proxy can decide which future
+versions of the GNU Affero General Public License can be used, that proxy's
+public statement of acceptance of a version permanently authorizes you
+to choose that version for the Program.
+
+ Later license versions may give you additional or different
+permissions. However, no additional obligations are imposed on any
+author or copyright holder as a result of your choosing to follow a
+later version.
+
+ 15. Disclaimer of Warranty.
+
+ THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY
+APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT
+HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY
+OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO,
+THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
+PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM
+IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF
+ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
+
+ 16. Limitation of Liability.
+
+ IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
+WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS
+THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY
+GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE
+USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF
+DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD
+PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS),
+EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF
+SUCH DAMAGES.
+
+ 17. Interpretation of Sections 15 and 16.
+
+ If the disclaimer of warranty and limitation of liability provided
+above cannot be given local legal effect according to their terms,
+reviewing courts shall apply local law that most closely approximates
+an absolute waiver of all civil liability in connection with the
+Program, unless a warranty or assumption of liability accompanies a
+copy of the Program in return for a fee.
+
+ END OF TERMS AND CONDITIONS
+
+ How to Apply These Terms to Your New Programs
+
+ If you develop a new program, and you want it to be of the greatest
+possible use to the public, the best way to achieve this is to make it
+free software which everyone can redistribute and change under these terms.
+
+ To do so, attach the following notices to the program. It is safest
+to attach them to the start of each source file to most effectively
+state the exclusion of warranty; and each file should have at least
+the "copyright" line and a pointer to where the full notice is found.
+
+
+ Copyright (C)
+
+ This program is free software: you can redistribute it and/or modify
+ it under the terms of the GNU Affero General Public License as published by
+ the Free Software Foundation, either version 3 of the License, or
+ (at your option) any later version.
+
+ This program is distributed in the hope that it will be useful,
+ but WITHOUT ANY WARRANTY; without even the implied warranty of
+ MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ GNU Affero General Public License for more details.
+
+ You should have received a copy of the GNU Affero General Public License
+ along with this program. If not, see .
+
+Also add information on how to contact you by electronic and paper mail.
+
+ If your software can interact with users remotely through a computer
+network, you should also make sure that it provides a way for users to
+get its source. For example, if your program is a web application, its
+interface could display a "Source" link that leads users to an archive
+of the code. There are many ways you could offer source, and different
+solutions will be better for different programs; see section 13 for the
+specific requirements.
+
+ You should also get your employer (if you work as a programmer) or school,
+if any, to sign a "copyright disclaimer" for the program, if necessary.
+For more information on this, and how to apply and follow the GNU AGPL, see
+.
diff --git a/yolov8/MANIFEST.in b/yolov8/MANIFEST.in
new file mode 100644
index 0000000000000000000000000000000000000000..def1ad3c3a3950d81bf8e7aeb83d37953c1ed74f
--- /dev/null
+++ b/yolov8/MANIFEST.in
@@ -0,0 +1,8 @@
+include *.md
+include requirements.txt
+include LICENSE
+include setup.py
+include ultralytics/assets/bus.jpg
+include ultralytics/assets/zidane.jpg
+recursive-include ultralytics *.yaml
+recursive-exclude __pycache__ *
diff --git a/yolov8/README.md b/yolov8/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..bf7947f2aa807864bd1afbd927eb9125cb1dde29
--- /dev/null
+++ b/yolov8/README.md
@@ -0,0 +1,268 @@
+
+
+
+
+
+
+[English](README.md) | [简体中文](README.zh-CN.md)
+
+
+
+
+
+[Ultralytics](https://ultralytics.com) [YOLOv8](https://github.com/ultralytics/ultralytics) is a cutting-edge, state-of-the-art (SOTA) model that builds upon the success of previous YOLO versions and introduces new features and improvements to further boost performance and flexibility. YOLOv8 is designed to be fast, accurate, and easy to use, making it an excellent choice for a wide range of object detection and tracking, instance segmentation, image classification and pose estimation tasks.
+
+We hope that the resources here will help you get the most out of YOLOv8. Please browse the YOLOv8
Docs for details, raise an issue on
GitHub for support, and join our
Discord community for questions and discussions!
+
+To request an Enterprise License please complete the form at [Ultralytics Licensing](https://ultralytics.com/license).
+
+
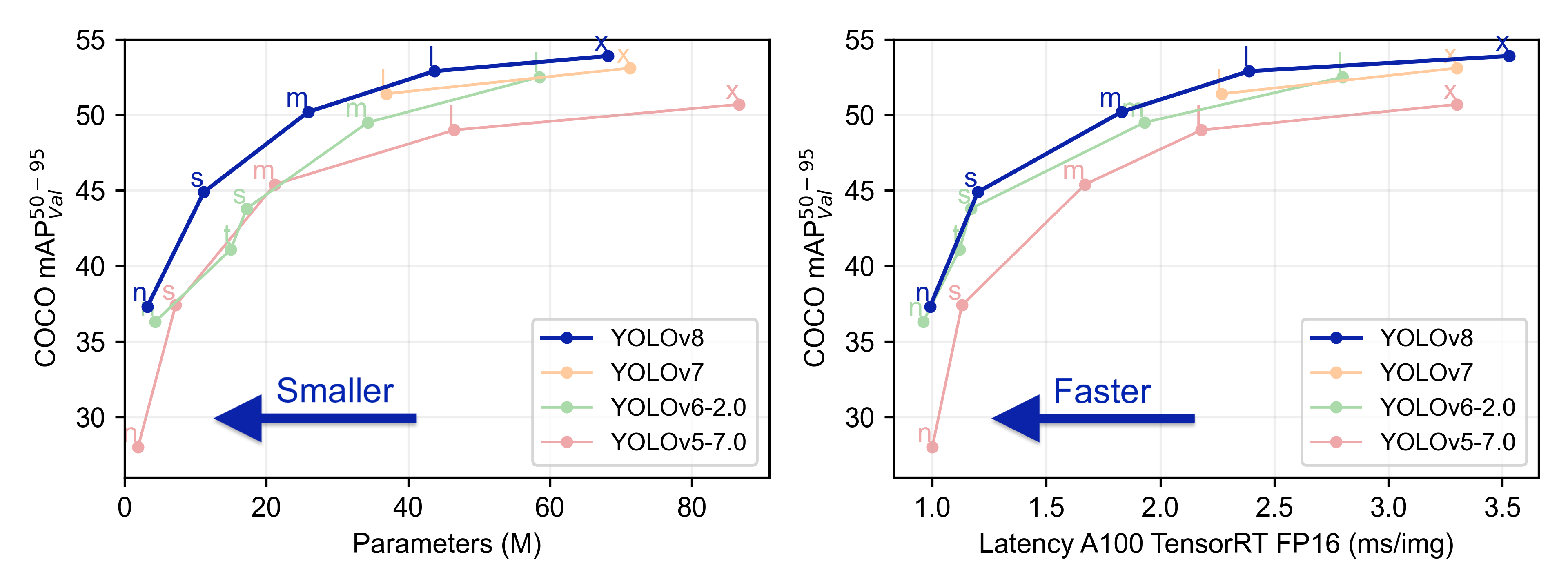
+
+
+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+
+
+
+## Documentation
+
+See below for a quickstart installation and usage example, and see the [YOLOv8 Docs](https://docs.ultralytics.com) for full documentation on training, validation, prediction and deployment.
+
+
+Install
+
+Pip install the ultralytics package including all [requirements](https://github.com/ultralytics/ultralytics/blob/main/requirements.txt) in a [**Python>=3.8**](https://www.python.org/) environment with [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/).
+
+[](https://badge.fury.io/py/ultralytics) [](https://pepy.tech/project/ultralytics)
+
+```bash
+pip install ultralytics
+```
+
+For alternative installation methods including Conda, Docker, and Git, please refer to the [Quickstart Guide](https://docs.ultralytics.com/quickstart).
+
+
+
+
+Usage
+
+#### CLI
+
+YOLOv8 may be used directly in the Command Line Interface (CLI) with a `yolo` command:
+
+```bash
+yolo predict model=yolov8n.pt source='https://ultralytics.com/images/bus.jpg'
+```
+
+`yolo` can be used for a variety of tasks and modes and accepts additional arguments, i.e. `imgsz=640`. See the YOLOv8 [CLI Docs](https://docs.ultralytics.com/usage/cli) for examples.
+
+#### Python
+
+YOLOv8 may also be used directly in a Python environment, and accepts the same [arguments](https://docs.ultralytics.com/usage/cfg/) as in the CLI example above:
+
+```python
+from ultralytics import YOLO
+
+# Load a model
+model = YOLO("yolov8n.yaml") # build a new model from scratch
+model = YOLO("yolov8n.pt") # load a pretrained model (recommended for training)
+
+# Use the model
+model.train(data="coco128.yaml", epochs=3) # train the model
+metrics = model.val() # evaluate model performance on the validation set
+results = model("https://ultralytics.com/images/bus.jpg") # predict on an image
+path = model.export(format="onnx") # export the model to ONNX format
+```
+
+[Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) download automatically from the latest Ultralytics [release](https://github.com/ultralytics/assets/releases). See YOLOv8 [Python Docs](https://docs.ultralytics.com/usage/python) for more examples.
+
+
+
+## Models
+
+YOLOv8 [Detect](https://docs.ultralytics.com/tasks/detect), [Segment](https://docs.ultralytics.com/tasks/segment) and [Pose](https://docs.ultralytics.com/tasks/pose) models pretrained on the [COCO](https://docs.ultralytics.com/datasets/detect/coco) dataset are available here, as well as YOLOv8 [Classify](https://docs.ultralytics.com/tasks/classify) models pretrained on the [ImageNet](https://docs.ultralytics.com/datasets/classify/imagenet) dataset. [Track](https://docs.ultralytics.com/modes/track) mode is available for all Detect, Segment and Pose models.
+
+
+
+All [Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) download automatically from the latest Ultralytics [release](https://github.com/ultralytics/assets/releases) on first use.
+
+Detection
+
+See [Detection Docs](https://docs.ultralytics.com/tasks/detect/) for usage examples with these models.
+
+| Model | size
(pixels) | mAPval
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+| ------------------------------------------------------------------------------------ | --------------------- | -------------------- | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+| [YOLOv8n](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n.pt) | 640 | 37.3 | 80.4 | 0.99 | 3.2 | 8.7 |
+| [YOLOv8s](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s.pt) | 640 | 44.9 | 128.4 | 1.20 | 11.2 | 28.6 |
+| [YOLOv8m](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m.pt) | 640 | 50.2 | 234.7 | 1.83 | 25.9 | 78.9 |
+| [YOLOv8l](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l.pt) | 640 | 52.9 | 375.2 | 2.39 | 43.7 | 165.2 |
+| [YOLOv8x](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x.pt) | 640 | 53.9 | 479.1 | 3.53 | 68.2 | 257.8 |
+
+- **mAPval** values are for single-model single-scale on [COCO val2017](http://cocodataset.org) dataset.
+
Reproduce by `yolo val detect data=coco.yaml device=0`
+- **Speed** averaged over COCO val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) instance.
+
Reproduce by `yolo val detect data=coco128.yaml batch=1 device=0|cpu`
+
+
+
+Segmentation
+
+See [Segmentation Docs](https://docs.ultralytics.com/tasks/segment/) for usage examples with these models.
+
+| Model | size
(pixels) | mAPbox
50-95 | mAPmask
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+| -------------------------------------------------------------------------------------------- | --------------------- | -------------------- | --------------------- | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+| [YOLOv8n-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-seg.pt) | 640 | 36.7 | 30.5 | 96.1 | 1.21 | 3.4 | 12.6 |
+| [YOLOv8s-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-seg.pt) | 640 | 44.6 | 36.8 | 155.7 | 1.47 | 11.8 | 42.6 |
+| [YOLOv8m-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-seg.pt) | 640 | 49.9 | 40.8 | 317.0 | 2.18 | 27.3 | 110.2 |
+| [YOLOv8l-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-seg.pt) | 640 | 52.3 | 42.6 | 572.4 | 2.79 | 46.0 | 220.5 |
+| [YOLOv8x-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-seg.pt) | 640 | 53.4 | 43.4 | 712.1 | 4.02 | 71.8 | 344.1 |
+
+- **mAPval** values are for single-model single-scale on [COCO val2017](http://cocodataset.org) dataset.
+
Reproduce by `yolo val segment data=coco.yaml device=0`
+- **Speed** averaged over COCO val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) instance.
+
Reproduce by `yolo val segment data=coco128-seg.yaml batch=1 device=0|cpu`
+
+
+
+Classification
+
+See [Classification Docs](https://docs.ultralytics.com/tasks/classify/) for usage examples with these models.
+
+| Model | size
(pixels) | acc
top1 | acc
top5 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) at 640 |
+| -------------------------------------------------------------------------------------------- | --------------------- | ---------------- | ---------------- | ------------------------------ | ----------------------------------- | ------------------ | ------------------------ |
+| [YOLOv8n-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-cls.pt) | 224 | 66.6 | 87.0 | 12.9 | 0.31 | 2.7 | 4.3 |
+| [YOLOv8s-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-cls.pt) | 224 | 72.3 | 91.1 | 23.4 | 0.35 | 6.4 | 13.5 |
+| [YOLOv8m-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-cls.pt) | 224 | 76.4 | 93.2 | 85.4 | 0.62 | 17.0 | 42.7 |
+| [YOLOv8l-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-cls.pt) | 224 | 78.0 | 94.1 | 163.0 | 0.87 | 37.5 | 99.7 |
+| [YOLOv8x-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-cls.pt) | 224 | 78.4 | 94.3 | 232.0 | 1.01 | 57.4 | 154.8 |
+
+- **acc** values are model accuracies on the [ImageNet](https://www.image-net.org/) dataset validation set.
+
Reproduce by `yolo val classify data=path/to/ImageNet device=0`
+- **Speed** averaged over ImageNet val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) instance.
+
Reproduce by `yolo val classify data=path/to/ImageNet batch=1 device=0|cpu`
+
+
+
+Pose
+
+See [Pose Docs](https://docs.ultralytics.com/tasks/pose) for usage examples with these models.
+
+| Model | size
(pixels) | mAPpose
50-95 | mAPpose
50 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+| ---------------------------------------------------------------------------------------------------- | --------------------- | --------------------- | ------------------ | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+| [YOLOv8n-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-pose.pt) | 640 | 50.4 | 80.1 | 131.8 | 1.18 | 3.3 | 9.2 |
+| [YOLOv8s-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-pose.pt) | 640 | 60.0 | 86.2 | 233.2 | 1.42 | 11.6 | 30.2 |
+| [YOLOv8m-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-pose.pt) | 640 | 65.0 | 88.8 | 456.3 | 2.00 | 26.4 | 81.0 |
+| [YOLOv8l-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-pose.pt) | 640 | 67.6 | 90.0 | 784.5 | 2.59 | 44.4 | 168.6 |
+| [YOLOv8x-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose.pt) | 640 | 69.2 | 90.2 | 1607.1 | 3.73 | 69.4 | 263.2 |
+| [YOLOv8x-pose-p6](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose-p6.pt) | 1280 | 71.6 | 91.2 | 4088.7 | 10.04 | 99.1 | 1066.4 |
+
+- **mAPval** values are for single-model single-scale on [COCO Keypoints val2017](http://cocodataset.org)
+ dataset.
+
Reproduce by `yolo val pose data=coco-pose.yaml device=0`
+- **Speed** averaged over COCO val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) instance.
+
Reproduce by `yolo val pose data=coco8-pose.yaml batch=1 device=0|cpu`
+
+
+
+## Integrations
+
+
+
+
+
+
+
+
+
+| Roboflow | ClearML ⭐ NEW | Comet ⭐ NEW | Neural Magic ⭐ NEW |
+| :--------------------------------------------------------------------------------------------------------------------------: | :---------------------------------------------------------------------------------------------------------------------------------: | :-------------------------------------------------------------------------------------------------------------------------------------------------------: | :----------------------------------------------------------------------------------------------------: |
+| Label and export your custom datasets directly to YOLOv8 for training with [Roboflow](https://roboflow.com/?ref=ultralytics) | Automatically track, visualize and even remotely train YOLOv8 using [ClearML](https://cutt.ly/yolov5-readme-clearml) (open-source!) | Free forever, [Comet](https://bit.ly/yolov8-readme-comet) lets you save YOLOv8 models, resume training, and interactively visualize and debug predictions | Run YOLOv8 inference up to 6x faster with [Neural Magic DeepSparse](https://bit.ly/yolov5-neuralmagic) |
+
+## Ultralytics HUB
+
+Experience seamless AI with [Ultralytics HUB](https://bit.ly/ultralytics_hub) ⭐, the all-in-one solution for data visualization, YOLOv5 and YOLOv8 🚀 model training and deployment, without any coding. Transform images into actionable insights and bring your AI visions to life with ease using our cutting-edge platform and user-friendly [Ultralytics App](https://ultralytics.com/app_install). Start your journey for **Free** now!
+
+
+
+
+## Contribute
+
+We love your input! YOLOv5 and YOLOv8 would not be possible without help from our community. Please see our [Contributing Guide](https://docs.ultralytics.com/help/contributing) to get started, and fill out our [Survey](https://ultralytics.com/survey?utm_source=github&utm_medium=social&utm_campaign=Survey) to send us feedback on your experience. Thank you 🙏 to all our contributors!
+
+
+
+
+
+
+## License
+
+YOLOv8 is available under two different licenses:
+
+- **AGPL-3.0 License**: See [LICENSE](https://github.com/ultralytics/ultralytics/blob/main/LICENSE) file for details.
+- **Enterprise License**: Provides greater flexibility for commercial product development without the open-source requirements of AGPL-3.0. Typical use cases are embedding Ultralytics software and AI models in commercial products and applications. Request an Enterprise License at [Ultralytics Licensing](https://ultralytics.com/license).
+
+## Contact
+
+For YOLOv8 bug reports and feature requests please visit [GitHub Issues](https://github.com/ultralytics/ultralytics/issues), and join our [Discord](https://discord.gg/2wNGbc6g9X) community for questions and discussions!
+
+
+
+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+
diff --git a/yolov8/README.zh-CN.md b/yolov8/README.zh-CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..872624907d021c3131fe6cb377189a50b504216f
--- /dev/null
+++ b/yolov8/README.zh-CN.md
@@ -0,0 +1,266 @@
+
+
+
+
+
+
+[English](README.md) | [简体中文](README.zh-CN.md)
+
+
+
+
+
+[Ultralytics](https://ultralytics.com) [YOLOv8](https://github.com/ultralytics/ultralytics) 是一款前沿、最先进(SOTA)的模型,基于先前 YOLO 版本的成功,引入了新功能和改进,进一步提升性能和灵活性。YOLOv8 设计快速、准确且易于使用,使其成为各种物体检测与跟踪、实例分割、图像分类和姿态估计任务的绝佳选择。
+
+我们希望这里的资源能帮助您充分利用 YOLOv8。请浏览 YOLOv8
文档 了解详细信息,在
GitHub 上提交问题以获得支持,并加入我们的
Discord 社区进行问题和讨论!
+
+如需申请企业许可,请在 [Ultralytics Licensing](https://ultralytics.com/license) 处填写表格
+
+
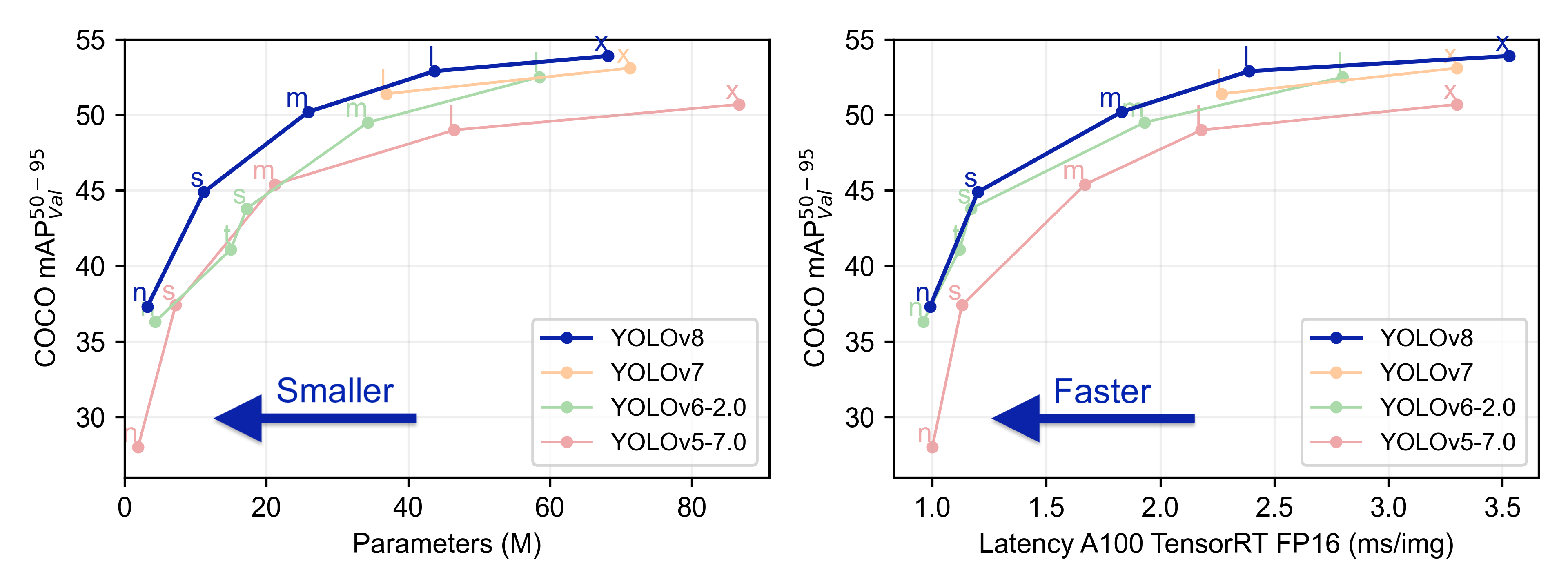
+
+
+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+
+
+
+## 文档
+
+请参阅下面的快速安装和使用示例,以及 [YOLOv8 文档](https://docs.ultralytics.com) 上有关培训、验证、预测和部署的完整文档。
+
+
+安装
+
+使用Pip在一个[**Python>=3.8**](https://www.python.org/)环境中安装`ultralytics`包,此环境还需包含[**PyTorch>=1.7**](https://pytorch.org/get-started/locally/)。这也会安装所有必要的[依赖项](https://github.com/ultralytics/ultralytics/blob/main/requirements.txt)。
+
+[](https://badge.fury.io/py/ultralytics) [](https://pepy.tech/project/ultralytics)
+
+```bash
+pip install ultralytics
+```
+
+如需使用包括Conda、Docker和Git在内的其他安装方法,请参考[快速入门指南](https://docs.ultralytics.com/quickstart)。
+
+
+
+
+Usage
+
+#### CLI
+
+YOLOv8 可以在命令行界面(CLI)中直接使用,只需输入 `yolo` 命令:
+
+```bash
+yolo predict model=yolov8n.pt source='https://ultralytics.com/images/bus.jpg'
+```
+
+`yolo` 可用于各种任务和模式,并接受其他参数,例如 `imgsz=640`。查看 YOLOv8 [CLI 文档](https://docs.ultralytics.com/usage/cli)以获取示例。
+
+#### Python
+
+YOLOv8 也可以在 Python 环境中直接使用,并接受与上述 CLI 示例中相同的[参数](https://docs.ultralytics.com/usage/cfg/):
+
+```python
+from ultralytics import YOLO
+
+# 加载模型
+model = YOLO("yolov8n.yaml") # 从头开始构建新模型
+model = YOLO("yolov8n.pt") # 加载预训练模型(建议用于训练)
+
+# 使用模型
+model.train(data="coco128.yaml", epochs=3) # 训练模型
+metrics = model.val() # 在验证集上评估模型性能
+results = model("https://ultralytics.com/images/bus.jpg") # 对图像进行预测
+success = model.export(format="onnx") # 将模型导出为 ONNX 格式
+```
+
+[模型](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) 会自动从最新的 Ultralytics [发布版本](https://github.com/ultralytics/assets/releases)中下载。查看 YOLOv8 [Python 文档](https://docs.ultralytics.com/usage/python)以获取更多示例。
+
+
+
+## 模型
+
+在[COCO](https://docs.ultralytics.com/datasets/detect/coco)数据集上预训练的YOLOv8 [检测](https://docs.ultralytics.com/tasks/detect),[分割](https://docs.ultralytics.com/tasks/segment)和[姿态](https://docs.ultralytics.com/tasks/pose)模型可以在这里找到,以及在[ImageNet](https://docs.ultralytics.com/datasets/classify/imagenet)数据集上预训练的YOLOv8 [分类](https://docs.ultralytics.com/tasks/classify)模型。所有的检测,分割和姿态模型都支持[追踪](https://docs.ultralytics.com/modes/track)模式。
+
+
+
+所有[模型](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models)在首次使用时会自动从最新的Ultralytics [发布版本](https://github.com/ultralytics/assets/releases)下载。
+
+检测
+
+查看 [检测文档](https://docs.ultralytics.com/tasks/detect/) 以获取使用这些模型的示例。
+
+| 模型 | 尺寸
(像素) | mAPval
50-95 | 速度
CPU ONNX
(ms) | 速度
A100 TensorRT
(ms) | 参数
(M) | FLOPs
(B) |
+| ------------------------------------------------------------------------------------ | --------------- | -------------------- | --------------------------- | -------------------------------- | -------------- | ----------------- |
+| [YOLOv8n](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n.pt) | 640 | 37.3 | 80.4 | 0.99 | 3.2 | 8.7 |
+| [YOLOv8s](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s.pt) | 640 | 44.9 | 128.4 | 1.20 | 11.2 | 28.6 |
+| [YOLOv8m](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m.pt) | 640 | 50.2 | 234.7 | 1.83 | 25.9 | 78.9 |
+| [YOLOv8l](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l.pt) | 640 | 52.9 | 375.2 | 2.39 | 43.7 | 165.2 |
+| [YOLOv8x](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x.pt) | 640 | 53.9 | 479.1 | 3.53 | 68.2 | 257.8 |
+
+- **mAPval** 值是基于单模型单尺度在 [COCO val2017](http://cocodataset.org) 数据集上的结果。
+
通过 `yolo val detect data=coco.yaml device=0` 复现
+- **速度** 是使用 [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) 实例对 COCO val 图像进行平均计算的。
+
通过 `yolo val detect data=coco128.yaml batch=1 device=0|cpu` 复现
+
+
+
+分割
+
+查看 [分割文档](https://docs.ultralytics.com/tasks/segment/) 以获取使用这些模型的示例。
+
+| 模型 | 尺寸
(像素) | mAPbox
50-95 | mAPmask
50-95 | 速度
CPU ONNX
(ms) | 速度
A100 TensorRT
(ms) | 参数
(M) | FLOPs
(B) |
+| -------------------------------------------------------------------------------------------- | --------------- | -------------------- | --------------------- | --------------------------- | -------------------------------- | -------------- | ----------------- |
+| [YOLOv8n-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-seg.pt) | 640 | 36.7 | 30.5 | 96.1 | 1.21 | 3.4 | 12.6 |
+| [YOLOv8s-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-seg.pt) | 640 | 44.6 | 36.8 | 155.7 | 1.47 | 11.8 | 42.6 |
+| [YOLOv8m-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-seg.pt) | 640 | 49.9 | 40.8 | 317.0 | 2.18 | 27.3 | 110.2 |
+| [YOLOv8l-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-seg.pt) | 640 | 52.3 | 42.6 | 572.4 | 2.79 | 46.0 | 220.5 |
+| [YOLOv8x-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-seg.pt) | 640 | 53.4 | 43.4 | 712.1 | 4.02 | 71.8 | 344.1 |
+
+- **mAPval** 值是基于单模型单尺度在 [COCO val2017](http://cocodataset.org) 数据集上的结果。
+
通过 `yolo val segment data=coco.yaml device=0` 复现
+- **速度** 是使用 [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) 实例对 COCO val 图像进行平均计算的。
+
通过 `yolo val segment data=coco128-seg.yaml batch=1 device=0|cpu` 复现
+
+
+
+分类
+
+查看 [分类文档](https://docs.ultralytics.com/tasks/classify/) 以获取使用这些模型的示例。
+
+| 模型 | 尺寸
(像素) | acc
top1 | acc
top5 | 速度
CPU ONNX
(ms) | 速度
A100 TensorRT
(ms) | 参数
(M) | FLOPs
(B) at 640 |
+| -------------------------------------------------------------------------------------------- | --------------- | ---------------- | ---------------- | --------------------------- | -------------------------------- | -------------- | ------------------------ |
+| [YOLOv8n-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-cls.pt) | 224 | 66.6 | 87.0 | 12.9 | 0.31 | 2.7 | 4.3 |
+| [YOLOv8s-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-cls.pt) | 224 | 72.3 | 91.1 | 23.4 | 0.35 | 6.4 | 13.5 |
+| [YOLOv8m-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-cls.pt) | 224 | 76.4 | 93.2 | 85.4 | 0.62 | 17.0 | 42.7 |
+| [YOLOv8l-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-cls.pt) | 224 | 78.0 | 94.1 | 163.0 | 0.87 | 37.5 | 99.7 |
+| [YOLOv8x-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-cls.pt) | 224 | 78.4 | 94.3 | 232.0 | 1.01 | 57.4 | 154.8 |
+
+- **acc** 值是模型在 [ImageNet](https://www.image-net.org/) 数据集验证集上的准确率。
+
通过 `yolo val classify data=path/to/ImageNet device=0` 复现
+- **速度** 是使用 [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) 实例对 ImageNet val 图像进行平均计算的。
+
通过 `yolo val classify data=path/to/ImageNet batch=1 device=0|cpu` 复现
+
+
+
+姿态
+
+查看 [姿态文档](https://docs.ultralytics.com/tasks/) 以获取使用这些模型的示例。
+
+| 模型 | 尺寸
(像素) | mAPpose
50-95 | mAPpose
50 | 速度
CPU ONNX
(ms) | 速度
A100 TensorRT
(ms) | 参数
(M) | FLOPs
(B) |
+| ---------------------------------------------------------------------------------------------------- | --------------- | --------------------- | ------------------ | --------------------------- | -------------------------------- | -------------- | ----------------- |
+| [YOLOv8n-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-pose.pt) | 640 | 50.4 | 80.1 | 131.8 | 1.18 | 3.3 | 9.2 |
+| [YOLOv8s-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-pose.pt) | 640 | 60.0 | 86.2 | 233.2 | 1.42 | 11.6 | 30.2 |
+| [YOLOv8m-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-pose.pt) | 640 | 65.0 | 88.8 | 456.3 | 2.00 | 26.4 | 81.0 |
+| [YOLOv8l-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-pose.pt) | 640 | 67.6 | 90.0 | 784.5 | 2.59 | 44.4 | 168.6 |
+| [YOLOv8x-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose.pt) | 640 | 69.2 | 90.2 | 1607.1 | 3.73 | 69.4 | 263.2 |
+| [YOLOv8x-pose-p6](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose-p6.pt) | 1280 | 71.6 | 91.2 | 4088.7 | 10.04 | 99.1 | 1066.4 |
+
+- **mAPval** 值是基于单模型单尺度在 [COCO Keypoints val2017](http://cocodataset.org) 数据集上的结果。
+
通过 `yolo val pose data=coco-pose.yaml device=0` 复现
+- **速度** 是使用 [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/) 实例对 COCO val 图像进行平均计算的。
+
通过 `yolo val pose data=coco8-pose.yaml batch=1 device=0|cpu` 复现
+
+
+
+## 集成
+
+
+
+
+
+
+
+
+
+| Roboflow | ClearML ⭐ NEW | Comet ⭐ NEW | Neural Magic ⭐ NEW |
+| :--------------------------------------------------------------------------------: | :----------------------------------------------------------------------------: | :----------------------------------------------------------------------------------: | :-----------------------------------------------------------------------------------: |
+| 使用 [Roboflow](https://roboflow.com/?ref=ultralytics) 将您的自定义数据集直接标记并导出至 YOLOv8 进行训练 | 使用 [ClearML](https://cutt.ly/yolov5-readme-clearml)(开源!)自动跟踪、可视化,甚至远程训练 YOLOv8 | 免费且永久,[Comet](https://bit.ly/yolov8-readme-comet) 让您保存 YOLOv8 模型、恢复训练,并以交互式方式查看和调试预测 | 使用 [Neural Magic DeepSparse](https://bit.ly/yolov5-neuralmagic) 使 YOLOv8 推理速度提高多达 6 倍 |
+
+## Ultralytics HUB
+
+体验 [Ultralytics HUB](https://bit.ly/ultralytics_hub) ⭐ 带来的无缝 AI,这是一个一体化解决方案,用于数据可视化、YOLOv5 和即将推出的 YOLOv8 🚀 模型训练和部署,无需任何编码。通过我们先进的平台和用户友好的 [Ultralytics 应用程序](https://ultralytics.com/app_install),轻松将图像转化为可操作的见解,并实现您的 AI 愿景。现在就开始您的**免费**之旅!
+
+
+
+
+## 贡献
+
+我们喜欢您的参与!没有社区的帮助,YOLOv5 和 YOLOv8 将无法实现。请参阅我们的[贡献指南](https://docs.ultralytics.com/help/contributing)以开始使用,并填写我们的[调查问卷](https://ultralytics.com/survey?utm_source=github&utm_medium=social&utm_campaign=Survey)向我们提供您的使用体验反馈。感谢所有贡献者的支持!🙏
+
+
+
+
+
+
+## 许可证
+
+YOLOv8 提供两种不同的许可证:
+
+- **AGPL-3.0 许可证**:详细信息请参阅 [LICENSE](https://github.com/ultralytics/ultralytics/blob/main/LICENSE) 文件。
+- **企业许可证**:为商业产品开发提供更大的灵活性,无需遵循 AGPL-3.0 的开源要求。典型的用例是将 Ultralytics 软件和 AI 模型嵌入商业产品和应用中。在 [Ultralytics 授权](https://ultralytics.com/license) 处申请企业许可证。
+
+## 联系方式
+
+对于 YOLOv8 的错误报告和功能请求,请访问 [GitHub Issues](https://github.com/ultralytics/ultralytics/issues),并加入我们的 [Discord](https://discord.gg/2wNGbc6g9X) 社区进行问题和讨论!
+
+
+
+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+
+ 
+
diff --git a/yolov8/docs/CNAME b/yolov8/docs/CNAME
new file mode 100644
index 0000000000000000000000000000000000000000..773aac86c4a69ad9574e6148c3c4cf9b041814a2
--- /dev/null
+++ b/yolov8/docs/CNAME
@@ -0,0 +1 @@
+docs.ultralytics.com
\ No newline at end of file
diff --git a/yolov8/docs/README.md b/yolov8/docs/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..9b6df255d92515fe2bf38a9acffd1ae4ae992b0a
--- /dev/null
+++ b/yolov8/docs/README.md
@@ -0,0 +1,90 @@
+---
+description: Learn how to install the Ultralytics package in developer mode and build/serve locally using MkDocs. Deploy your project to your host easily.
+keywords: install Ultralytics package, deploy documentation, building locally, deploy site, GitHub Pages, GitLab Pages, Amazon S3, MkDocs documentation
+---
+
+# Ultralytics Docs
+
+Ultralytics Docs are deployed to [https://docs.ultralytics.com](https://docs.ultralytics.com).
+
+### Install Ultralytics package
+
+To install the ultralytics package in developer mode, you will need to have Git and Python 3 installed on your system.
+Then, follow these steps:
+
+1. Clone the ultralytics repository to your local machine using Git:
+
+```bash
+git clone https://github.com/ultralytics/ultralytics.git
+```
+
+2. Navigate to the root directory of the repository:
+
+```bash
+cd ultralytics
+```
+
+3. Install the package in developer mode using pip:
+
+```bash
+pip install -e '.[dev]'
+```
+
+This will install the ultralytics package and its dependencies in developer mode, allowing you to make changes to the
+package code and have them reflected immediately in your Python environment.
+
+Note that you may need to use the pip3 command instead of pip if you have multiple versions of Python installed on your
+system.
+
+### Building and Serving Locally
+
+The `mkdocs serve` command is used to build and serve a local version of the MkDocs documentation site. It is typically
+used during the development and testing phase of a documentation project.
+
+```bash
+mkdocs serve
+```
+
+Here is a breakdown of what this command does:
+
+- `mkdocs`: This is the command-line interface (CLI) for the MkDocs static site generator. It is used to build and serve
+ MkDocs sites.
+- `serve`: This is a subcommand of the `mkdocs` CLI that tells it to build and serve the documentation site locally.
+- `-a`: This flag specifies the hostname and port number to bind the server to. The default value is `localhost:8000`.
+- `-t`: This flag specifies the theme to use for the documentation site. The default value is `mkdocs`.
+- `-s`: This flag tells the `serve` command to serve the site in silent mode, which means it will not display any log
+ messages or progress updates.
+ When you run the `mkdocs serve` command, it will build the documentation site using the files in the `docs/` directory
+ and serve it at the specified hostname and port number. You can then view the site by going to the URL in your web
+ browser.
+
+While the site is being served, you can make changes to the documentation files and see them reflected in the live site
+immediately. This is useful for testing and debugging your documentation before deploying it to a live server.
+
+To stop the serve command and terminate the local server, you can use the `CTRL+C` keyboard shortcut.
+
+### Deploying Your Documentation Site
+
+To deploy your MkDocs documentation site, you will need to choose a hosting provider and a deployment method. Some
+popular options include GitHub Pages, GitLab Pages, and Amazon S3.
+
+Before you can deploy your site, you will need to configure your `mkdocs.yml` file to specify the remote host and any
+other necessary deployment settings.
+
+Once you have configured your `mkdocs.yml` file, you can use the `mkdocs deploy` command to build and deploy your site.
+This command will build the documentation site using the files in the `docs/` directory and the specified configuration
+file and theme, and then deploy the site to the specified remote host.
+
+For example, to deploy your site to GitHub Pages using the gh-deploy plugin, you can use the following command:
+
+```bash
+mkdocs gh-deploy
+```
+
+If you are using GitHub Pages, you can set a custom domain for your documentation site by going to the "Settings" page
+for your repository and updating the "Custom domain" field in the "GitHub Pages" section.
+
+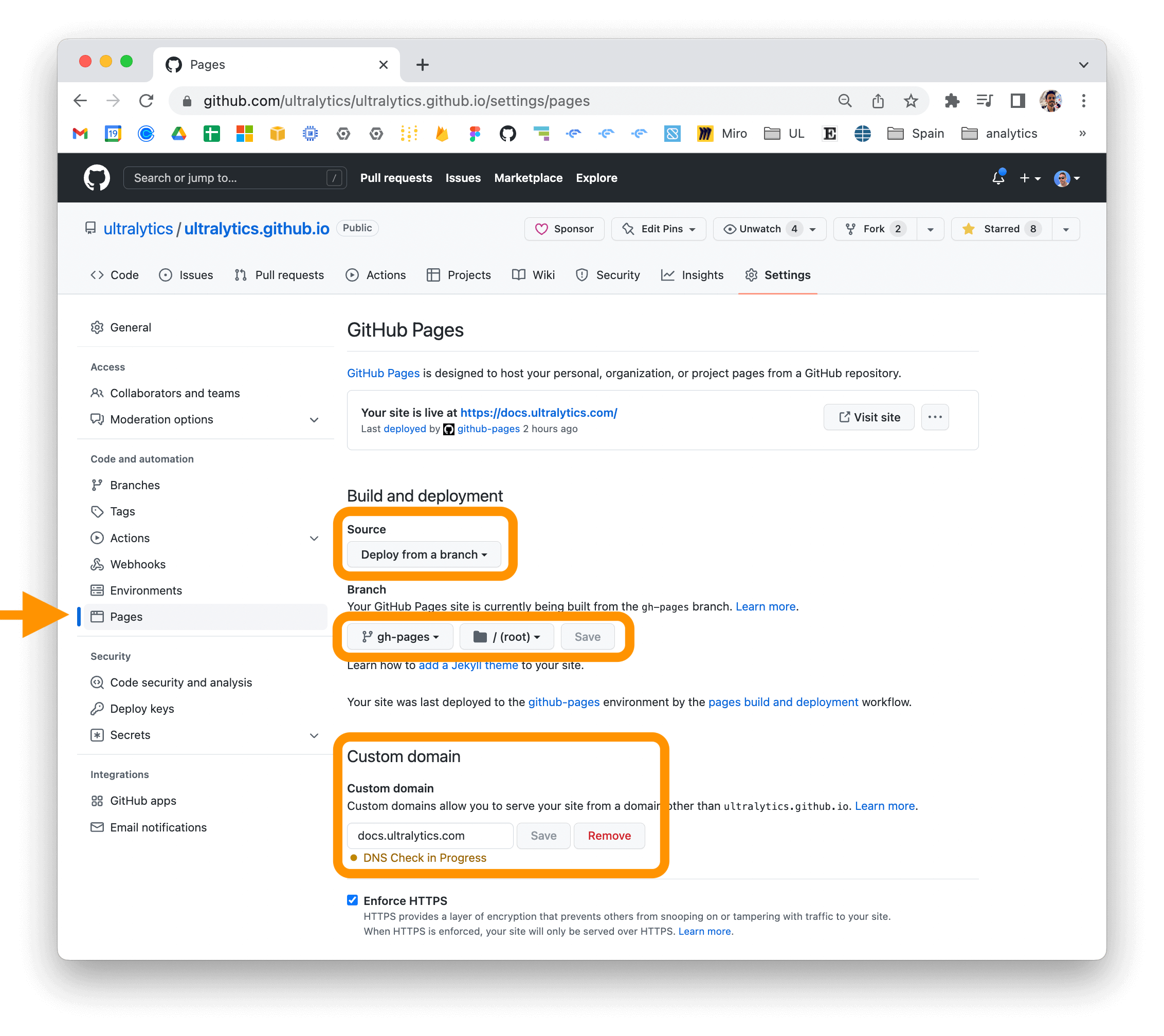
+
+For more information on deploying your MkDocs documentation site, see
+the [MkDocs documentation](https://www.mkdocs.org/user-guide/deploying-your-docs/).
\ No newline at end of file
diff --git a/yolov8/docs/SECURITY.md b/yolov8/docs/SECURITY.md
new file mode 100644
index 0000000000000000000000000000000000000000..a32fb4f78526d79b482d6ae6902fb1019474399a
--- /dev/null
+++ b/yolov8/docs/SECURITY.md
@@ -0,0 +1,26 @@
+---
+description: Ensure robust security with Ultralytics' open-source projects. We use advanced vulnerability scans and actively address potential risks. Your safety is our priority.
+keywords: Ultralytics, security policy, Snyk, CodeQL scanning, security vulnerability, security issues, report security issue
+---
+
+# Security Policy
+
+At [Ultralytics](https://ultralytics.com), the security of our users' data and systems is of utmost importance. To ensure the safety and security of our [open-source projects](https://github.com/ultralytics), we have implemented several measures to detect and prevent security vulnerabilities.
+
+## Snyk Scanning
+
+We use [Snyk](https://snyk.io/advisor/python/ultralytics) to regularly scan all Ultralytics repositories for vulnerabilities and security issues. Our goal is to identify and remediate any potential threats as soon as possible, to minimize any risks to our users.
+
+[](https://snyk.io/advisor/python/ultralytics)
+
+## GitHub CodeQL Scanning
+
+In addition to our Snyk scans, we also use GitHub's [CodeQL](https://docs.github.com/en/code-security/code-scanning/automatically-scanning-your-code-for-vulnerabilities-and-errors/about-code-scanning-with-codeql) scans to proactively identify and address security vulnerabilities across all Ultralytics repositories.
+
+[](https://github.com/ultralytics/ultralytics/actions/workflows/codeql.yaml)
+
+## Reporting Security Issues
+
+If you suspect or discover a security vulnerability in any of our repositories, please let us know immediately. You can reach out to us directly via our [contact form](https://ultralytics.com/contact) or via [security@ultralytics.com](mailto:security@ultralytics.com). Our security team will investigate and respond as soon as possible.
+
+We appreciate your help in keeping all Ultralytics open-source projects secure and safe for everyone.
\ No newline at end of file
diff --git a/yolov8/docs/assets/favicon.ico b/yolov8/docs/assets/favicon.ico
new file mode 100644
index 0000000000000000000000000000000000000000..7aa5066187ae0bb3179a5bc13c282e481871404d
Binary files /dev/null and b/yolov8/docs/assets/favicon.ico differ
diff --git a/yolov8/docs/build_reference.py b/yolov8/docs/build_reference.py
new file mode 100644
index 0000000000000000000000000000000000000000..65dcc6c0d623978b426a192022478bfccac6c7aa
--- /dev/null
+++ b/yolov8/docs/build_reference.py
@@ -0,0 +1,117 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Helper file to build Ultralytics Docs reference section. Recursively walks through ultralytics dir and builds an MkDocs
+reference section of *.md files composed of classes and functions, and also creates a nav menu for use in mkdocs.yaml.
+
+Note: Must be run from repository root directory. Do not run from docs directory.
+"""
+
+import os
+import re
+from collections import defaultdict
+from pathlib import Path
+from ultralytics.yolo.utils import ROOT
+
+NEW_YAML_DIR = ROOT.parent
+CODE_DIR = ROOT
+REFERENCE_DIR = ROOT.parent / 'docs/reference'
+
+
+def extract_classes_and_functions(filepath):
+ with open(filepath, 'r') as file:
+ content = file.read()
+
+ class_pattern = r"(?:^|\n)class\s(\w+)(?:\(|:)"
+ func_pattern = r"(?:^|\n)def\s(\w+)\("
+
+ classes = re.findall(class_pattern, content)
+ functions = re.findall(func_pattern, content)
+
+ return classes, functions
+
+
+def create_markdown(py_filepath, module_path, classes, functions):
+ md_filepath = py_filepath.with_suffix('.md')
+
+ # Read existing content and keep header content between first two ---
+ header_content = ""
+ if md_filepath.exists():
+ with open(md_filepath, 'r') as file:
+ existing_content = file.read()
+ header_parts = existing_content.split('---', 2)
+ if len(header_parts) >= 3:
+ header_content = f"{header_parts[0]}---{header_parts[1]}---\n\n"
+
+ module_path = module_path.replace('.__init__', '')
+ md_content = [f"## {class_name}\n---\n### ::: {module_path}.{class_name}\n
\n" for class_name in classes]
+ md_content.extend(f"## {func_name}\n---\n### ::: {module_path}.{func_name}\n
\n" for func_name in functions)
+ md_content = header_content + "\n".join(md_content)
+
+ os.makedirs(os.path.dirname(md_filepath), exist_ok=True)
+ with open(md_filepath, 'w') as file:
+ file.write(md_content)
+
+ return md_filepath.relative_to(NEW_YAML_DIR)
+
+
+def nested_dict():
+ return defaultdict(nested_dict)
+
+
+def sort_nested_dict(d):
+ return {
+ key: sort_nested_dict(value) if isinstance(value, dict) else value
+ for key, value in sorted(d.items())
+ }
+
+
+def create_nav_menu_yaml(nav_items):
+ nav_tree = nested_dict()
+
+ for item_str in nav_items:
+ item = Path(item_str)
+ parts = item.parts
+ current_level = nav_tree['reference']
+ for part in parts[2:-1]: # skip the first two parts (docs and reference) and the last part (filename)
+ current_level = current_level[part]
+
+ md_file_name = parts[-1].replace('.md', '')
+ current_level[md_file_name] = item
+
+ nav_tree_sorted = sort_nested_dict(nav_tree)
+
+ def _dict_to_yaml(d, level=0):
+ yaml_str = ""
+ indent = " " * level
+ for k, v in d.items():
+ if isinstance(v, dict):
+ yaml_str += f"{indent}- {k}:\n{_dict_to_yaml(v, level + 1)}"
+ else:
+ yaml_str += f"{indent}- {k}: {str(v).replace('docs/', '')}\n"
+ return yaml_str
+
+ with open(NEW_YAML_DIR / 'nav_menu_updated.yml', 'w') as file:
+ yaml_str = _dict_to_yaml(nav_tree_sorted)
+ file.write(yaml_str)
+
+
+def main():
+ nav_items = []
+ for root, _, files in os.walk(CODE_DIR):
+ for file in files:
+ if file.endswith(".py"):
+ py_filepath = Path(root) / file
+ classes, functions = extract_classes_and_functions(py_filepath)
+
+ if classes or functions:
+ py_filepath_rel = py_filepath.relative_to(CODE_DIR)
+ md_filepath = REFERENCE_DIR / py_filepath_rel
+ module_path = f"ultralytics.{py_filepath_rel.with_suffix('').as_posix().replace('/', '.')}"
+ md_rel_filepath = create_markdown(md_filepath, module_path, classes, functions)
+ nav_items.append(str(md_rel_filepath))
+
+ create_nav_menu_yaml(nav_items)
+
+
+if __name__ == "__main__":
+ main()
diff --git a/yolov8/docs/help/CI.md b/yolov8/docs/help/CI.md
new file mode 100644
index 0000000000000000000000000000000000000000..2fdad908cc2e7123aa71f74a2f2769f6d37c7c6b
--- /dev/null
+++ b/yolov8/docs/help/CI.md
@@ -0,0 +1,35 @@
+---
+comments: true
+description: Understand all the Continuous Integration (CI) tests for Ultralytics repositories and see their statuses in a clear, concise table.
+keywords: Ultralytics, CI Tests, Continuous Integration, Docker Deployment, Broken Links, CodeQL, PyPI Publishing
+---
+
+# Continuous Integration (CI)
+
+Continuous Integration (CI) is an essential aspect of software development which involves integrating changes and testing them automatically. CI allows us to maintain high-quality code by catching issues early and often in the development process. At Ultralytics, we use various CI tests to ensure the quality and integrity of our codebase.
+
+Here's a brief description of our CI tests:
+
+- **CI:** This is our primary CI test that involves running unit tests, linting checks, and sometimes more comprehensive tests depending on the repository.
+- **Docker Deployment:** This test checks the deployment of the project using Docker to ensure the Dockerfile and related scripts are working correctly.
+- **Broken Links:** This test scans the codebase for any broken or dead links in our markdown or HTML files.
+- **CodeQL:** CodeQL is a tool from GitHub that performs semantic analysis on our code, helping to find potential security vulnerabilities and maintain high-quality code.
+- **PyPi Publishing:** This test checks if the project can be packaged and published to PyPi without any errors.
+
+Below is the table showing the status of these CI tests for our main repositories:
+
+| Repository | CI | Docker Deployment | Broken Links | CodeQL | PyPi and Docs Publishing |
+|-----------------------------------------------------------|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------|------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
+| [yolov3](https://github.com/ultralytics/yolov3) | [](https://github.com/ultralytics/yolov3/actions/workflows/ci-testing.yml) | [](https://github.com/ultralytics/yolov3/actions/workflows/docker.yml) | [](https://github.com/ultralytics/yolov3/actions/workflows/links.yml) | [](https://github.com/ultralytics/yolov3/actions/workflows/codeql-analysis.yml) | |
+| [yolov5](https://github.com/ultralytics/yolov5) | [](https://github.com/ultralytics/yolov5/actions/workflows/ci-testing.yml) | [](https://github.com/ultralytics/yolov5/actions/workflows/docker.yml) | [](https://github.com/ultralytics/yolov5/actions/workflows/links.yml) | [](https://github.com/ultralytics/yolov5/actions/workflows/codeql-analysis.yml) | |
+| [ultralytics](https://github.com/ultralytics/ultralytics) | [](https://github.com/ultralytics/ultralytics/actions/workflows/ci.yaml) | [](https://github.com/ultralytics/ultralytics/actions/workflows/docker.yaml) | [](https://github.com/ultralytics/ultralytics/actions/workflows/links.yml) | [](https://github.com/ultralytics/ultralytics/actions/workflows/codeql.yaml) | [](https://github.com/ultralytics/ultralytics/actions/workflows/publish.yml) |
+| [hub](https://github.com/ultralytics/hub) | [](https://github.com/ultralytics/hub/actions/workflows/ci.yaml) | | [](https://github.com/ultralytics/hub/actions/workflows/links.yml) | | |
+| [docs](https://github.com/ultralytics/docs) | | | | | [](https://github.com/ultralytics/docs/actions/workflows/pages/pages-build-deployment) |
+
+Each badge shows the status of the last run of the corresponding CI test on the `main` branch of the respective repository. If a test fails, the badge will display a "failing" status, and if it passes, it will display a "passing" status.
+
+If you notice a test failing, it would be a great help if you could report it through a GitHub issue in the respective repository.
+
+Remember, a successful CI test does not mean that everything is perfect. It is always recommended to manually review the code before deployment or merging changes.
+
+Happy coding!
\ No newline at end of file
diff --git a/yolov8/docs/help/CLA.md b/yolov8/docs/help/CLA.md
new file mode 100644
index 0000000000000000000000000000000000000000..ffc9371880f2f94b3c0f5038f00f974380bc7726
--- /dev/null
+++ b/yolov8/docs/help/CLA.md
@@ -0,0 +1,70 @@
+---
+description: Individual Contributor License Agreement. Settle Intellectual Property issues for Contributions made to anything open source released by Ultralytics.
+keywords: Ultralytics, Individual, Contributor, License, Agreement, open source, software, projects, contributions
+---
+
+# Ultralytics Individual Contributor License Agreement
+
+Thank you for your interest in contributing to open source software projects (“Projects”) made available by Ultralytics
+SE or its affiliates (“Ultralytics”). This Individual Contributor License Agreement (“Agreement”) sets out the terms
+governing any source code, object code, bug fixes, configuration changes, tools, specifications, documentation, data,
+materials, feedback, information or other works of authorship that you submit or have submitted, in any form and in any
+manner, to Ultralytics in respect of any of the Projects (collectively “Contributions”). If you have any questions
+respecting this Agreement, please contact hello@ultralytics.com.
+
+You agree that the following terms apply to all of your past, present and future Contributions. Except for the licenses
+granted in this Agreement, you retain all of your right, title and interest in and to your Contributions.
+
+**Copyright License.** You hereby grant, and agree to grant, to Ultralytics a non-exclusive, perpetual, irrevocable,
+worldwide, fully-paid, royalty-free, transferable copyright license to reproduce, prepare derivative works of, publicly
+display, publicly perform, and distribute your Contributions and such derivative works, with the right to sublicense the
+foregoing rights through multiple tiers of sublicensees.
+
+**Patent License.** You hereby grant, and agree to grant, to Ultralytics a non-exclusive, perpetual, irrevocable,
+worldwide, fully-paid, royalty-free, transferable patent license to make, have made, use, offer to sell, sell,
+import, and otherwise transfer your Contributions, where such license applies only to those patent claims
+licensable by you that are necessarily infringed by your Contributions alone or by combination of your
+Contributions with the Project to which such Contributions were submitted, with the right to sublicense the
+foregoing rights through multiple tiers of sublicensees.
+
+**Moral Rights.** To the fullest extent permitted under applicable law, you hereby waive, and agree not to
+assert, all of your “moral rights” in or relating to your Contributions for the benefit of Ultralytics, its assigns, and
+their respective direct and indirect sublicensees.
+
+**Third Party Content/Rights.** If your Contribution includes or is based on any source code, object code, bug
+fixes, configuration changes, tools, specifications, documentation, data, materials, feedback, information or
+other works of authorship that were not authored by you (“Third Party Content”) or if you are aware of any
+third party intellectual property or proprietary rights associated with your Contribution (“Third Party Rights”),
+then you agree to include with the submission of your Contribution full details respecting such Third Party
+Content and Third Party Rights, including, without limitation, identification of which aspects of your
+Contribution contain Third Party Content or are associated with Third Party Rights, the owner/author of the
+Third Party Content and Third Party Rights, where you obtained the Third Party Content, and any applicable
+third party license terms or restrictions respecting the Third Party Content and Third Party Rights. For greater
+certainty, the foregoing obligations respecting the identification of Third Party Content and Third Party Rights
+do not apply to any portion of a Project that is incorporated into your Contribution to that same Project.
+
+**Representations.** You represent that, other than the Third Party Content and Third Party Rights identified by
+you in accordance with this Agreement, you are the sole author of your Contributions and are legally entitled
+to grant the foregoing licenses and waivers in respect of your Contributions. If your Contributions were
+created in the course of your employment with your past or present employer(s), you represent that such
+employer(s) has authorized you to make your Contributions on behalf of such employer(s) or such employer
+(s) has waived all of their right, title or interest in or to your Contributions.
+
+**Disclaimer.** To the fullest extent permitted under applicable law, your Contributions are provided on an "asis"
+basis, without any warranties or conditions, express or implied, including, without limitation, any implied
+warranties or conditions of non-infringement, merchantability or fitness for a particular purpose. You are not
+required to provide support for your Contributions, except to the extent you desire to provide support.
+
+**No Obligation.** You acknowledge that Ultralytics is under no obligation to use or incorporate your Contributions
+into any of the Projects. The decision to use or incorporate your Contributions into any of the Projects will be
+made at the sole discretion of Ultralytics or its authorized delegates ..
+
+**Disputes.** This Agreement shall be governed by and construed in accordance with the laws of the State of
+New York, United States of America, without giving effect to its principles or rules regarding conflicts of laws,
+other than such principles directing application of New York law. The parties hereby submit to venue in, and
+jurisdiction of the courts located in New York, New York for purposes relating to this Agreement. In the event
+that any of the provisions of this Agreement shall be held by a court or other tribunal of competent jurisdiction
+to be unenforceable, the remaining portions hereof shall remain in full force and effect.
+
+**Assignment.** You agree that Ultralytics may assign this Agreement, and all of its rights, obligations and licenses
+hereunder.
\ No newline at end of file
diff --git a/yolov8/docs/help/FAQ.md b/yolov8/docs/help/FAQ.md
new file mode 100644
index 0000000000000000000000000000000000000000..13692024be082fb3b79af5925d2ae4182d7711f6
--- /dev/null
+++ b/yolov8/docs/help/FAQ.md
@@ -0,0 +1,39 @@
+---
+comments: true
+description: 'Get quick answers to common Ultralytics YOLO questions: Hardware requirements, fine-tuning, conversion, real-time detection, and accuracy tips.'
+keywords: Ultralytics YOLO, Frequently Asked Questions, hardware requirements, model fine-tuning, converting to ONNX, TensorFlow, real-time detection, improving model accuracy
+---
+
+# Ultralytics YOLO Frequently Asked Questions (FAQ)
+
+This FAQ section addresses some common questions and issues users might encounter while working with Ultralytics YOLO repositories.
+
+## 1. What are the hardware requirements for running Ultralytics YOLO?
+
+Ultralytics YOLO can be run on a variety of hardware configurations, including CPUs, GPUs, and even some edge devices. However, for optimal performance and faster training and inference, we recommend using a GPU with a minimum of 8GB of memory. NVIDIA GPUs with CUDA support are ideal for this purpose.
+
+## 2. How do I fine-tune a pre-trained YOLO model on my custom dataset?
+
+To fine-tune a pre-trained YOLO model on your custom dataset, you'll need to create a dataset configuration file (YAML) that defines the dataset's properties, such as the path to the images, the number of classes, and class names. Next, you'll need to modify the model configuration file to match the number of classes in your dataset. Finally, use the `train.py` script to start the training process with your custom dataset and the pre-trained model. You can find a detailed guide on fine-tuning YOLO in the Ultralytics documentation.
+
+## 3. How do I convert a YOLO model to ONNX or TensorFlow format?
+
+Ultralytics provides built-in support for converting YOLO models to ONNX format. You can use the `export.py` script to convert a saved model to ONNX format. If you need to convert the model to TensorFlow format, you can use the ONNX model as an intermediary and then use the ONNX-TensorFlow converter to convert the ONNX model to TensorFlow format.
+
+## 4. Can I use Ultralytics YOLO for real-time object detection?
+
+Yes, Ultralytics YOLO is designed to be efficient and fast, making it suitable for real-time object detection tasks. The actual performance will depend on your hardware configuration and the complexity of the model. Using a GPU and optimizing the model for your specific use case can help achieve real-time performance.
+
+## 5. How can I improve the accuracy of my YOLO model?
+
+Improving the accuracy of a YOLO model may involve several strategies, such as:
+
+- Fine-tuning the model on more annotated data
+- Data augmentation to increase the variety of training samples
+- Using a larger or more complex model architecture
+- Adjusting the learning rate, batch size, and other hyperparameters
+- Using techniques like transfer learning or knowledge distillation
+
+Remember that there's often a trade-off between accuracy and inference speed, so finding the right balance is crucial for your specific application.
+
+If you have any more questions or need assistance, don't hesitate to consult the Ultralytics documentation or reach out to the community through GitHub Issues or the official discussion forum.
\ No newline at end of file
diff --git a/yolov8/docs/help/code_of_conduct.md b/yolov8/docs/help/code_of_conduct.md
new file mode 100644
index 0000000000000000000000000000000000000000..1cc27e16c8f87bfc367bebba5d654222233afb91
--- /dev/null
+++ b/yolov8/docs/help/code_of_conduct.md
@@ -0,0 +1,134 @@
+---
+comments: true
+description: Read the Ultralytics Contributor Covenant Code of Conduct. Learn ways to create a welcoming community & consequences for inappropriate conduct.
+keywords: Ultralytics, contributor, covenant, code, conduct, pledge, standards, enforcement, harassment-free, community, guidelines
+---
+
+# Ultralytics Contributor Covenant Code of Conduct
+
+## Our Pledge
+
+We as members, contributors, and leaders pledge to make participation in our
+community a harassment-free experience for everyone, regardless of age, body
+size, visible or invisible disability, ethnicity, sex characteristics, gender
+identity and expression, level of experience, education, socio-economic status,
+nationality, personal appearance, race, religion, or sexual identity
+and orientation.
+
+We pledge to act and interact in ways that contribute to an open, welcoming,
+diverse, inclusive, and healthy community.
+
+## Our Standards
+
+Examples of behavior that contributes to a positive environment for our
+community include:
+
+- Demonstrating empathy and kindness toward other people
+- Being respectful of differing opinions, viewpoints, and experiences
+- Giving and gracefully accepting constructive feedback
+- Accepting responsibility and apologizing to those affected by our mistakes,
+ and learning from the experience
+- Focusing on what is best not just for us as individuals, but for the
+ overall community
+
+Examples of unacceptable behavior include:
+
+- The use of sexualized language or imagery, and sexual attention or
+ advances of any kind
+- Trolling, insulting or derogatory comments, and personal or political attacks
+- Public or private harassment
+- Publishing others' private information, such as a physical or email
+ address, without their explicit permission
+- Other conduct which could reasonably be considered inappropriate in a
+ professional setting
+
+## Enforcement Responsibilities
+
+Community leaders are responsible for clarifying and enforcing our standards of
+acceptable behavior and will take appropriate and fair corrective action in
+response to any behavior that they deem inappropriate, threatening, offensive,
+or harmful.
+
+Community leaders have the right and responsibility to remove, edit, or reject
+comments, commits, code, wiki edits, issues, and other contributions that are
+not aligned to this Code of Conduct, and will communicate reasons for moderation
+decisions when appropriate.
+
+## Scope
+
+This Code of Conduct applies within all community spaces, and also applies when
+an individual is officially representing the community in public spaces.
+Examples of representing our community include using an official e-mail address,
+posting via an official social media account, or acting as an appointed
+representative at an online or offline event.
+
+## Enforcement
+
+Instances of abusive, harassing, or otherwise unacceptable behavior may be
+reported to the community leaders responsible for enforcement at
+hello@ultralytics.com.
+All complaints will be reviewed and investigated promptly and fairly.
+
+All community leaders are obligated to respect the privacy and security of the
+reporter of any incident.
+
+## Enforcement Guidelines
+
+Community leaders will follow these Community Impact Guidelines in determining
+the consequences for any action they deem in violation of this Code of Conduct:
+
+### 1. Correction
+
+**Community Impact**: Use of inappropriate language or other behavior deemed
+unprofessional or unwelcome in the community.
+
+**Consequence**: A private, written warning from community leaders, providing
+clarity around the nature of the violation and an explanation of why the
+behavior was inappropriate. A public apology may be requested.
+
+### 2. Warning
+
+**Community Impact**: A violation through a single incident or series
+of actions.
+
+**Consequence**: A warning with consequences for continued behavior. No
+interaction with the people involved, including unsolicited interaction with
+those enforcing the Code of Conduct, for a specified period of time. This
+includes avoiding interactions in community spaces as well as external channels
+like social media. Violating these terms may lead to a temporary or
+permanent ban.
+
+### 3. Temporary Ban
+
+**Community Impact**: A serious violation of community standards, including
+sustained inappropriate behavior.
+
+**Consequence**: A temporary ban from any sort of interaction or public
+communication with the community for a specified period of time. No public or
+private interaction with the people involved, including unsolicited interaction
+with those enforcing the Code of Conduct, is allowed during this period.
+Violating these terms may lead to a permanent ban.
+
+### 4. Permanent Ban
+
+**Community Impact**: Demonstrating a pattern of violation of community
+standards, including sustained inappropriate behavior, harassment of an
+individual, or aggression toward or disparagement of classes of individuals.
+
+**Consequence**: A permanent ban from any sort of public interaction within
+the community.
+
+## Attribution
+
+This Code of Conduct is adapted from the [Contributor Covenant][homepage],
+version 2.0, available at
+https://www.contributor-covenant.org/version/2/0/code_of_conduct.html.
+
+Community Impact Guidelines were inspired by [Mozilla's code of conduct
+enforcement ladder](https://github.com/mozilla/diversity).
+
+For answers to common questions about this code of conduct, see the FAQ at
+https://www.contributor-covenant.org/faq. Translations are available at
+https://www.contributor-covenant.org/translations.
+
+[homepage]: https://www.contributor-covenant.org
\ No newline at end of file
diff --git a/yolov8/docs/help/contributing.md b/yolov8/docs/help/contributing.md
new file mode 100644
index 0000000000000000000000000000000000000000..4fa8f633832bc48e21a77aabbd51292db471cd2b
--- /dev/null
+++ b/yolov8/docs/help/contributing.md
@@ -0,0 +1,75 @@
+---
+comments: true
+description: Learn how to contribute to Ultralytics Open-Source YOLO Repositories with contributions guidelines, pull requests requirements, and GitHub CI tests.
+keywords: Ultralytics YOLO, Open source, Contribution guidelines, Pull requests, CLA, GitHub Actions CI Tests, Google-style docstrings
+---
+
+# Contributing to Ultralytics Open-Source YOLO Repositories
+
+First of all, thank you for your interest in contributing to Ultralytics open-source YOLO repositories! Your contributions will help improve the project and benefit the community. This document provides guidelines and best practices for contributing to Ultralytics YOLO repositories.
+
+## Table of Contents
+
+- [Code of Conduct](#code-of-conduct)
+- [Pull Requests](#pull-requests)
+ - [CLA Signing](#cla-signing)
+ - [Google-Style Docstrings](#google-style-docstrings)
+ - [GitHub Actions CI Tests](#github-actions-ci-tests)
+- [Bug Reports](#bug-reports)
+ - [Minimum Reproducible Example](#minimum-reproducible-example)
+- [License and Copyright](#license-and-copyright)
+
+## Code of Conduct
+
+All contributors are expected to adhere to the [Code of Conduct](code_of_conduct.md) to ensure a welcoming and inclusive environment for everyone.
+
+## Pull Requests
+
+We welcome contributions in the form of pull requests. To make the review process smoother, please follow these guidelines:
+
+1. **Fork the repository**: Fork the Ultralytics YOLO repository to your own GitHub account.
+
+2. **Create a branch**: Create a new branch in your forked repository with a descriptive name for your changes.
+
+3. **Make your changes**: Make the changes you want to contribute. Ensure that your changes follow the coding style of the project and do not introduce new errors or warnings.
+
+4. **Test your changes**: Test your changes locally to ensure that they work as expected and do not introduce new issues.
+
+5. **Commit your changes**: Commit your changes with a descriptive commit message. Make sure to include any relevant issue numbers in your commit message.
+
+6. **Create a pull request**: Create a pull request from your forked repository to the main Ultralytics YOLO repository. In the pull request description, provide a clear explanation of your changes and how they improve the project.
+
+### CLA Signing
+
+Before we can accept your pull request, you need to sign a [Contributor License Agreement (CLA)](CLA.md). This is a legal document stating that you agree to the terms of contributing to the Ultralytics YOLO repositories. The CLA ensures that your contributions are properly licensed and that the project can continue to be distributed under the AGPL-3.0 license.
+
+To sign the CLA, follow the instructions provided by the CLA bot after you submit your PR.
+
+### Google-Style Docstrings
+
+When adding new functions or classes, please include a [Google-style docstring](https://google.github.io/styleguide/pyguide.html) to provide clear and concise documentation for other developers. This will help ensure that your contributions are easy to understand and maintain.
+
+Example Google-style docstring:
+
+```python
+def example_function(arg1: int, arg2: str) -> bool:
+ """Example function that demonstrates Google-style docstrings.
+
+ Args:
+ arg1 (int): The first argument.
+ arg2 (str): The second argument.
+
+ Returns:
+ bool: True if successful, False otherwise.
+
+ Raises:
+ ValueError: If `arg1` is negative or `arg2` is empty.
+ """
+ if arg1 < 0 or not arg2:
+ raise ValueError("Invalid input values")
+ return True
+```
+
+### GitHub Actions CI Tests
+
+Before your pull request can be merged, all GitHub Actions Continuous Integration (CI) tests must pass. These tests include linting, unit tests, and other checks to ensure that your changes meet the quality standards of the project. Make sure to review the output of the GitHub Actions and fix any issues
\ No newline at end of file
diff --git a/yolov8/docs/help/index.md b/yolov8/docs/help/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..9647552b3fcee8049816f735b4e85d2f4fffdde5
--- /dev/null
+++ b/yolov8/docs/help/index.md
@@ -0,0 +1,17 @@
+---
+comments: true
+description: Get comprehensive resources for Ultralytics YOLO repositories. Find guides, FAQs, MRE creation, CLA & more. Join the supportive community now!
+keywords: ultralytics, yolo, help, guide, resources, faq, contributing, continuous integration, contributor license agreement, minimum reproducible example, code of conduct, security policy
+---
+
+Welcome to the Ultralytics Help page! We are committed to providing you with comprehensive resources to make your experience with Ultralytics YOLO repositories as smooth and enjoyable as possible. On this page, you'll find essential links to guides and documents that will help you navigate through common tasks and address any questions you might have while using our repositories.
+
+- [Frequently Asked Questions (FAQ)](FAQ.md): Find answers to common questions and issues faced by users and contributors of Ultralytics YOLO repositories.
+- [Contributing Guide](contributing.md): Learn the best practices for submitting pull requests, reporting bugs, and contributing to the development of our repositories.
+- [Continuous Integration (CI) Guide](CI.md): Understand the CI tests we perform for each Ultralytics repository and see their current statuses.
+- [Contributor License Agreement (CLA)](CLA.md): Familiarize yourself with our CLA to understand the terms and conditions for contributing to Ultralytics projects.
+- [Minimum Reproducible Example (MRE) Guide](minimum_reproducible_example.md): Understand how to create an MRE when submitting bug reports to ensure that our team can quickly and efficiently address the issue.
+- [Code of Conduct](code_of_conduct.md): Learn about our community guidelines and expectations to ensure a welcoming and inclusive environment for all participants.
+- [Security Policy](../SECURITY.md): Understand our security practices and how to report security vulnerabilities responsibly.
+
+We highly recommend going through these guides to make the most of your collaboration with the Ultralytics community. Our goal is to maintain a welcoming and supportive environment for all users and contributors. If you need further assistance, don't hesitate to reach out to us through GitHub Issues or the official discussion forum. Happy coding!
\ No newline at end of file
diff --git a/yolov8/docs/help/minimum_reproducible_example.md b/yolov8/docs/help/minimum_reproducible_example.md
new file mode 100644
index 0000000000000000000000000000000000000000..1a8acd27b9e14438d10c093baa9e3b86c5eee6e9
--- /dev/null
+++ b/yolov8/docs/help/minimum_reproducible_example.md
@@ -0,0 +1,78 @@
+---
+comments: true
+description: Learn how to create a Minimum Reproducible Example (MRE) for Ultralytics YOLO bug reports to help maintainers and contributors understand your issue better.
+keywords: Ultralytics, YOLO, bug report, minimum reproducible example, MRE, isolate problem, public models, public datasets, necessary dependencies, clear description, format code properly, test code, GitHub code block, error message
+---
+
+# Creating a Minimum Reproducible Example for Bug Reports in Ultralytics YOLO Repositories
+
+When submitting a bug report for Ultralytics YOLO repositories, it's essential to provide a [minimum reproducible example](https://docs.ultralytics.com/help/minimum_reproducible_example/) (MRE). An MRE is a small, self-contained piece of code that demonstrates the problem you're experiencing. Providing an MRE helps maintainers and contributors understand the issue and work on a fix more efficiently. This guide explains how to create an MRE when submitting bug reports to Ultralytics YOLO repositories.
+
+## 1. Isolate the Problem
+
+The first step in creating an MRE is to isolate the problem. This means removing any unnecessary code or dependencies that are not directly related to the issue. Focus on the specific part of the code that is causing the problem and remove any irrelevant code.
+
+## 2. Use Public Models and Datasets
+
+When creating an MRE, use publicly available models and datasets to reproduce the issue. For example, use the 'yolov8n.pt' model and the 'coco8.yaml' dataset. This ensures that the maintainers and contributors can easily run your example and investigate the problem without needing access to proprietary data or custom models.
+
+## 3. Include All Necessary Dependencies
+
+Make sure to include all the necessary dependencies in your MRE. If your code relies on external libraries, specify the required packages and their versions. Ideally, provide a `requirements.txt` file or list the dependencies in your bug report.
+
+## 4. Write a Clear Description of the Issue
+
+Provide a clear and concise description of the issue you're experiencing. Explain the expected behavior and the actual behavior you're encountering. If applicable, include any relevant error messages or logs.
+
+## 5. Format Your Code Properly
+
+When submitting an MRE, format your code properly using code blocks in the issue description. This makes it easier for others to read and understand your code. In GitHub, you can create a code block by wrapping your code with triple backticks (\```) and specifying the language:
+
+
+```python
+# Your Python code goes here
+```
+
+
+## 6. Test Your MRE
+
+Before submitting your MRE, test it to ensure that it accurately reproduces the issue. Make sure that others can run your example without any issues or modifications.
+
+## Example of an MRE
+
+Here's an example of an MRE for a hypothetical bug report:
+
+**Bug description:**
+
+When running the `detect.py` script on the sample image from the 'coco8.yaml' dataset, I get an error related to the dimensions of the input tensor.
+
+**MRE:**
+
+```python
+import torch
+from ultralytics import YOLO
+
+# Load the model
+model = YOLO("yolov8n.pt")
+
+# Load a 0-channel image
+image = torch.rand(1, 0, 640, 640)
+
+# Run the model
+results = model(image)
+```
+
+**Error message:**
+
+```
+RuntimeError: Expected input[1, 0, 640, 640] to have 3 channels, but got 0 channels instead
+```
+
+**Dependencies:**
+
+- torch==2.0.0
+- ultralytics==8.0.90
+
+In this example, the MRE demonstrates the issue with a minimal amount of code, uses a public model ('yolov8n.pt'), includes all necessary dependencies, and provides a clear description of the problem along with the error message.
+
+By following these guidelines, you'll help the maintainers and contributors of Ultralytics YOLO repositories to understand and resolve your issue more efficiently.
\ No newline at end of file
diff --git a/yolov8/docs/hub/app/android.md b/yolov8/docs/hub/app/android.md
new file mode 100644
index 0000000000000000000000000000000000000000..2f2ea9b4f7e47e353e3243d66f8bc9e1803aa99a
--- /dev/null
+++ b/yolov8/docs/hub/app/android.md
@@ -0,0 +1,66 @@
+---
+comments: true
+description: Run YOLO models on your Android device for real-time object detection with Ultralytics Android App. Utilizes TensorFlow Lite and hardware delegates.
+keywords: Ultralytics, Android, app, YOLO models, real-time object detection, TensorFlow Lite, quantization, acceleration, delegates, performance variability
+---
+
+# Ultralytics Android App: Real-time Object Detection with YOLO Models
+
+The Ultralytics Android App is a powerful tool that allows you to run YOLO models directly on your Android device for real-time object detection. This app utilizes TensorFlow Lite for model optimization and various hardware delegates for acceleration, enabling fast and efficient object detection.
+
+## Quantization and Acceleration
+
+To achieve real-time performance on your Android device, YOLO models are quantized to either FP16 or INT8 precision. Quantization is a process that reduces the numerical precision of the model's weights and biases, thus reducing the model's size and the amount of computation required. This results in faster inference times without significantly affecting the model's accuracy.
+
+### FP16 Quantization
+
+FP16 (or half-precision) quantization converts the model's 32-bit floating-point numbers to 16-bit floating-point numbers. This reduces the model's size by half and speeds up the inference process, while maintaining a good balance between accuracy and performance.
+
+### INT8 Quantization
+
+INT8 (or 8-bit integer) quantization further reduces the model's size and computation requirements by converting its 32-bit floating-point numbers to 8-bit integers. This quantization method can result in a significant speedup, but it may lead to a slight reduction in mean average precision (mAP) due to the lower numerical precision.
+
+!!! tip "mAP Reduction in INT8 Models"
+
+ The reduced numerical precision in INT8 models can lead to some loss of information during the quantization process, which may result in a slight decrease in mAP. However, this trade-off is often acceptable considering the substantial performance gains offered by INT8 quantization.
+
+## Delegates and Performance Variability
+
+Different delegates are available on Android devices to accelerate model inference. These delegates include CPU, [GPU](https://www.tensorflow.org/lite/android/delegates/gpu), [Hexagon](https://www.tensorflow.org/lite/android/delegates/hexagon) and [NNAPI](https://www.tensorflow.org/lite/android/delegates/nnapi). The performance of these delegates varies depending on the device's hardware vendor, product line, and specific chipsets used in the device.
+
+1. **CPU**: The default option, with reasonable performance on most devices.
+2. **GPU**: Utilizes the device's GPU for faster inference. It can provide a significant performance boost on devices with powerful GPUs.
+3. **Hexagon**: Leverages Qualcomm's Hexagon DSP for faster and more efficient processing. This option is available on devices with Qualcomm Snapdragon processors.
+4. **NNAPI**: The Android Neural Networks API (NNAPI) serves as an abstraction layer for running ML models on Android devices. NNAPI can utilize various hardware accelerators, such as CPU, GPU, and dedicated AI chips (e.g., Google's Edge TPU, or the Pixel Neural Core).
+
+Here's a table showing the primary vendors, their product lines, popular devices, and supported delegates:
+
+| Vendor | Product Lines | Popular Devices | Delegates Supported |
+|-----------------------------------------|---------------------------------------------------------------------------------------------------------------|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|--------------------------|
+| [Qualcomm](https://www.qualcomm.com/) | [Snapdragon (e.g., 800 series)](https://www.qualcomm.com/snapdragon) | [Samsung Galaxy S21](https://www.samsung.com/global/galaxy/galaxy-s21-5g/), [OnePlus 9](https://www.oneplus.com/9), [Google Pixel 6](https://store.google.com/product/pixel_6) | CPU, GPU, Hexagon, NNAPI |
+| [Samsung](https://www.samsung.com/) | [Exynos (e.g., Exynos 2100)](https://www.samsung.com/semiconductor/minisite/exynos/) | [Samsung Galaxy S21 (Global version)](https://www.samsung.com/global/galaxy/galaxy-s21-5g/) | CPU, GPU, NNAPI |
+| [MediaTek](https://www.mediatek.com/) | [Dimensity (e.g., Dimensity 1200)](https://www.mediatek.com/products/smartphones) | [Realme GT](https://www.realme.com/global/realme-gt), [Xiaomi Redmi Note](https://www.mi.com/en/phone/redmi/note-list) | CPU, GPU, NNAPI |
+| [HiSilicon](https://www.hisilicon.com/) | [Kirin (e.g., Kirin 990)](https://www.hisilicon.com/en/products/Kirin) | [Huawei P40 Pro](https://consumer.huawei.com/en/phones/p40-pro/), [Huawei Mate 30 Pro](https://consumer.huawei.com/en/phones/mate30-pro/) | CPU, GPU, NNAPI |
+| [NVIDIA](https://www.nvidia.com/) | [Tegra (e.g., Tegra X1)](https://www.nvidia.com/en-us/autonomous-machines/embedded-systems-dev-kits-modules/) | [NVIDIA Shield TV](https://www.nvidia.com/en-us/shield/shield-tv/), [Nintendo Switch](https://www.nintendo.com/switch/) | CPU, GPU, NNAPI |
+
+Please note that the list of devices mentioned is not exhaustive and may vary depending on the specific chipsets and device models. Always test your models on your target devices to ensure compatibility and optimal performance.
+
+Keep in mind that the choice of delegate can affect performance and model compatibility. For example, some models may not work with certain delegates, or a delegate may not be available on a specific device. As such, it's essential to test your model and the chosen delegate on your target devices for the best results.
+
+## Getting Started with the Ultralytics Android App
+
+To get started with the Ultralytics Android App, follow these steps:
+
+1. Download the Ultralytics App from the [Google Play Store](https://play.google.com/store/apps/details?id=com.ultralytics.ultralytics_app).
+
+2. Launch the app on your Android device and sign in with your Ultralytics account. If you don't have an account yet, create one [here](https://hub.ultralytics.com/).
+
+3. Once signed in, you will see a list of your trained YOLO models. Select a model to use for object detection.
+
+4. Grant the app permission to access your device's camera.
+
+5. Point your device's camera at objects you want to detect. The app will display bounding boxes and class labels in real-time as it detects objects.
+
+6. Explore the app's settings to adjust the detection threshold, enable or disable specific object classes, and more.
+
+With the Ultralytics Android App, you now have the power of real-time object detection using YOLO models right at your fingertips. Enjoy exploring the app's features and optimizing its settings to suit your specific use cases.
\ No newline at end of file
diff --git a/yolov8/docs/hub/app/index.md b/yolov8/docs/hub/app/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..76b8ce3ebbb6758f387006704157e8f67bfec0ff
--- /dev/null
+++ b/yolov8/docs/hub/app/index.md
@@ -0,0 +1,52 @@
+---
+comments: true
+description: Experience the power of YOLOv5 and YOLOv8 models with Ultralytics HUB app. Download from Google Play and App Store now.
+keywords: Ultralytics, HUB, App, Mobile, Object Detection, Image Recognition, YOLOv5, YOLOv8, Hardware Acceleration, Custom Model Training, iOS, Android
+---
+
+# Ultralytics HUB App
+
+
+
+
+
+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+
+
+
+ 
+
+ 
+
+
+Welcome to the Ultralytics HUB App! We are excited to introduce this powerful mobile app that allows you to run YOLOv5 and YOLOv8 models directly on your [iOS](https://apps.apple.com/xk/app/ultralytics/id1583935240) and [Android](https://play.google.com/store/apps/details?id=com.ultralytics.ultralytics_app) devices. With the HUB App, you can utilize hardware acceleration features like Apple's Neural Engine (ANE) or Android GPU and Neural Network API (NNAPI) delegates to achieve impressive performance on your mobile device.
+
+## Features
+
+- **Run YOLOv5 and YOLOv8 models**: Experience the power of YOLO models on your mobile device for real-time object detection and image recognition tasks.
+- **Hardware Acceleration**: Benefit from Apple ANE on iOS devices or Android GPU and NNAPI delegates for optimized performance.
+- **Custom Model Training**: Train custom models with the Ultralytics HUB platform and preview them live using the HUB App.
+- **Mobile Compatibility**: The HUB App supports both iOS and Android devices, bringing the power of YOLO models to a wide range of users.
+
+## App Documentation
+
+- [**iOS**](./ios.md): Learn about YOLO CoreML models accelerated on Apple's Neural Engine for iPhones and iPads.
+- [**Android**](./android.md): Explore TFLite acceleration on Android mobile devices.
+
+Get started today by downloading the Ultralytics HUB App on your mobile device and unlock the potential of YOLOv5 and YOLOv8 models on-the-go. Don't forget to check out our comprehensive [HUB Docs](../) for more information on training, deploying, and using your custom models with the Ultralytics HUB platform.
\ No newline at end of file
diff --git a/yolov8/docs/hub/app/ios.md b/yolov8/docs/hub/app/ios.md
new file mode 100644
index 0000000000000000000000000000000000000000..ed49f45b0f60ac84dbda742dc592dd2d9748008f
--- /dev/null
+++ b/yolov8/docs/hub/app/ios.md
@@ -0,0 +1,56 @@
+---
+comments: true
+description: Get started with the Ultralytics iOS app and run YOLO models in real-time for object detection on your iPhone or iPad with the Apple Neural Engine.
+keywords: YOLO, object detection, iOS app, Ultralytics, Apple Neural Engine, quantization, FP16, INT8, Core ML, machine learning
+---
+
+# Ultralytics iOS App: Real-time Object Detection with YOLO Models
+
+The Ultralytics iOS App is a powerful tool that allows you to run YOLO models directly on your iPhone or iPad for real-time object detection. This app utilizes the Apple Neural Engine and Core ML for model optimization and acceleration, enabling fast and efficient object detection.
+
+## Quantization and Acceleration
+
+To achieve real-time performance on your iOS device, YOLO models are quantized to either FP16 or INT8 precision. Quantization is a process that reduces the numerical precision of the model's weights and biases, thus reducing the model's size and the amount of computation required. This results in faster inference times without significantly affecting the model's accuracy.
+
+### FP16 Quantization
+
+FP16 (or half-precision) quantization converts the model's 32-bit floating-point numbers to 16-bit floating-point numbers. This reduces the model's size by half and speeds up the inference process, while maintaining a good balance between accuracy and performance.
+
+### INT8 Quantization
+
+INT8 (or 8-bit integer) quantization further reduces the model's size and computation requirements by converting its 32-bit floating-point numbers to 8-bit integers. This quantization method can result in a significant speedup, but it may lead to a slight reduction in accuracy.
+
+## Apple Neural Engine
+
+The Apple Neural Engine (ANE) is a dedicated hardware component integrated into Apple's A-series and M-series chips. It's designed to accelerate machine learning tasks, particularly for neural networks, allowing for faster and more efficient execution of your YOLO models.
+
+By combining quantized YOLO models with the Apple Neural Engine, the Ultralytics iOS App achieves real-time object detection on your iOS device without compromising on accuracy or performance.
+
+| Release Year | iPhone Name | Chipset Name | Node Size | ANE TOPs |
+|--------------|------------------------------------------------------|-------------------------------------------------------|-----------|----------|
+| 2017 | [iPhone X](https://en.wikipedia.org/wiki/IPhone_X) | [A11 Bionic](https://en.wikipedia.org/wiki/Apple_A11) | 10 nm | 0.6 |
+| 2018 | [iPhone XS](https://en.wikipedia.org/wiki/IPhone_XS) | [A12 Bionic](https://en.wikipedia.org/wiki/Apple_A12) | 7 nm | 5 |
+| 2019 | [iPhone 11](https://en.wikipedia.org/wiki/IPhone_11) | [A13 Bionic](https://en.wikipedia.org/wiki/Apple_A13) | 7 nm | 6 |
+| 2020 | [iPhone 12](https://en.wikipedia.org/wiki/IPhone_12) | [A14 Bionic](https://en.wikipedia.org/wiki/Apple_A14) | 5 nm | 11 |
+| 2021 | [iPhone 13](https://en.wikipedia.org/wiki/IPhone_13) | [A15 Bionic](https://en.wikipedia.org/wiki/Apple_A15) | 5 nm | 15.8 |
+| 2022 | [iPhone 14](https://en.wikipedia.org/wiki/IPhone_14) | [A16 Bionic](https://en.wikipedia.org/wiki/Apple_A16) | 4 nm | 17.0 |
+
+Please note that this list only includes iPhone models from 2017 onwards, and the ANE TOPs values are approximate.
+
+## Getting Started with the Ultralytics iOS App
+
+To get started with the Ultralytics iOS App, follow these steps:
+
+1. Download the Ultralytics App from the [App Store](https://apps.apple.com/xk/app/ultralytics/id1583935240).
+
+2. Launch the app on your iOS device and sign in with your Ultralytics account. If you don't have an account yet, create one [here](https://hub.ultralytics.com/).
+
+3. Once signed in, you will see a list of your trained YOLO models. Select a model to use for object detection.
+
+4. Grant the app permission to access your device's camera.
+
+5. Point your device's camera at objects you want to detect. The app will display bounding boxes and class labels in real-time as it detects objects.
+
+6. Explore the app's settings to adjust the detection threshold, enable or disable specific object classes, and more.
+
+With the Ultralytics iOS App, you can now leverage the power of YOLO models for real-time object detection on your iPhone or iPad, powered by the Apple Neural Engine and optimized with FP16 or INT8 quantization.
\ No newline at end of file
diff --git a/yolov8/docs/hub/datasets.md b/yolov8/docs/hub/datasets.md
new file mode 100644
index 0000000000000000000000000000000000000000..c1bdc38efc59465c9913d5e2266d2d2afc06729b
--- /dev/null
+++ b/yolov8/docs/hub/datasets.md
@@ -0,0 +1,159 @@
+---
+comments: true
+description: Efficiently manage and use custom datasets on Ultralytics HUB for streamlined training with YOLOv5 and YOLOv8 models.
+keywords: Ultralytics, HUB, Datasets, Upload, Visualize, Train, Custom Data, YAML, YOLOv5, YOLOv8
+---
+
+# HUB Datasets
+
+Ultralytics HUB datasets are a practical solution for managing and leveraging your custom datasets.
+
+Once uploaded, datasets can be immediately utilized for model training. This integrated approach facilitates a seamless transition from dataset management to model training, significantly simplifying the entire process.
+
+## Upload Dataset
+
+Ultralytics HUB datasets are just like YOLOv5 and YOLOv8 🚀 datasets. They use the same structure and the same label formats to keep
+everything simple.
+
+Before you upload a dataset to Ultralytics HUB, make sure to **place your dataset YAML file inside the dataset root directory** and that **your dataset YAML, directory and ZIP have the same name**, as shown in the example below, and then zip the dataset directory.
+
+For example, if your dataset is called "coco8", as our [COCO8](https://docs.ultralytics.com/datasets/detect/coco8) example dataset, then you should have a `coco8.yaml` inside your `coco8/` directory, which will create a `coco8.zip` when zipped:
+
+```bash
+zip -r coco8.zip coco8
+```
+
+You can download our [COCO8](https://github.com/ultralytics/hub/blob/master/example_datasets/coco8.zip) example dataset and unzip it to see exactly how to structure your dataset.
+
+
+
+
+
+The dataset YAML is the same standard YOLOv5 and YOLOv8 YAML format.
+
+!!! example "coco8.yaml"
+
+ ```yaml
+ --8<-- "ultralytics/datasets/coco8.yaml"
+ ```
+
+After zipping your dataset, you should validate it before uploading it to Ultralytics HUB. Ultralytics HUB conducts the dataset validation check post-upload, so by ensuring your dataset is correctly formatted and error-free ahead of time, you can forestall any setbacks due to dataset rejection.
+
+```py
+from ultralytics.hub import check_dataset
+check_dataset('path/to/coco8.zip')
+```
+
+Once your dataset ZIP is ready, navigate to the [Datasets](https://hub.ultralytics.com/datasets) page by clicking on the **Datasets** button in the sidebar.
+
+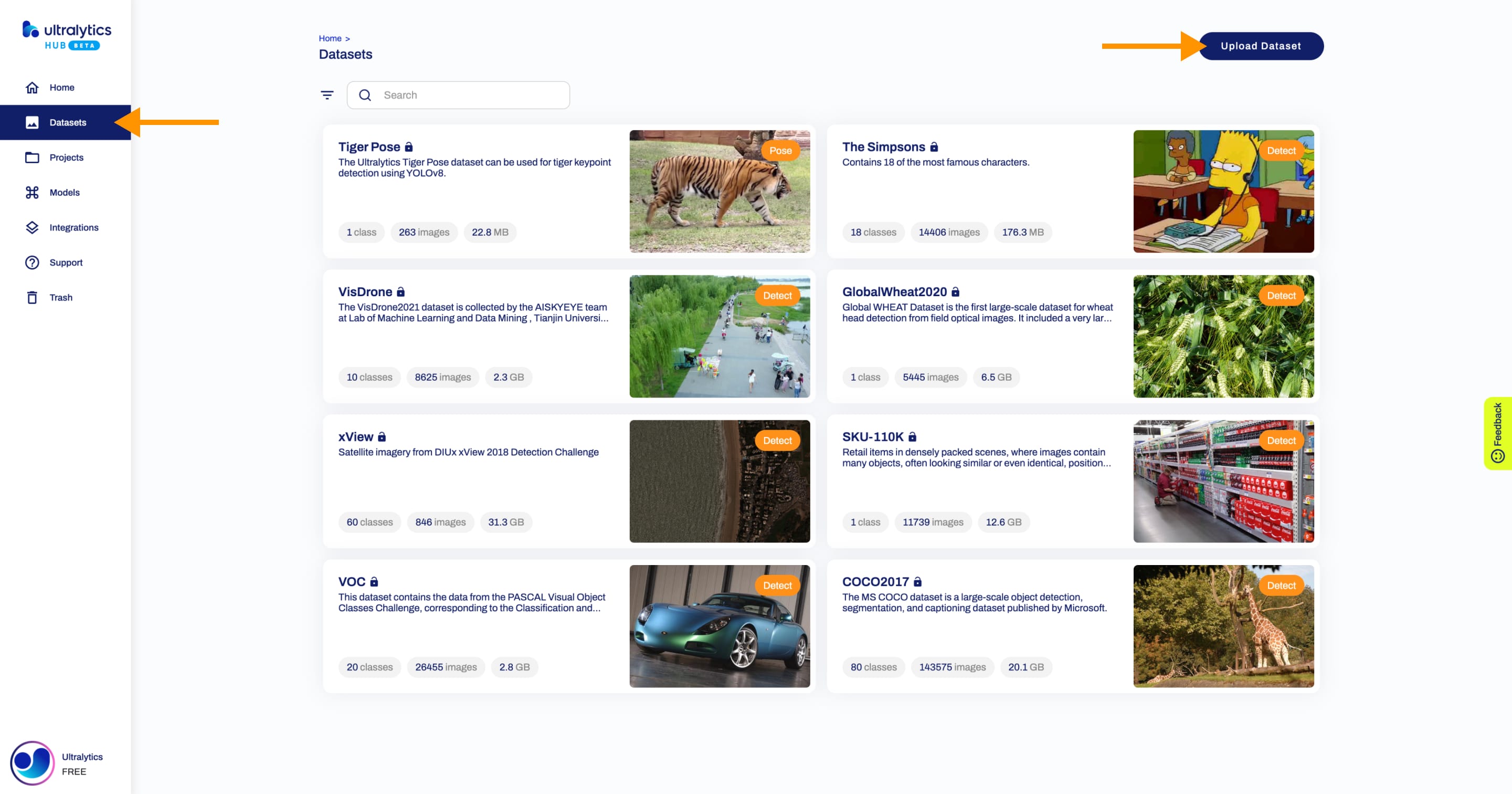
+
+??? tip "Tip"
+
+ You can also upload a dataset directly from the [Home](https://hub.ultralytics.com/home) page.
+
+ 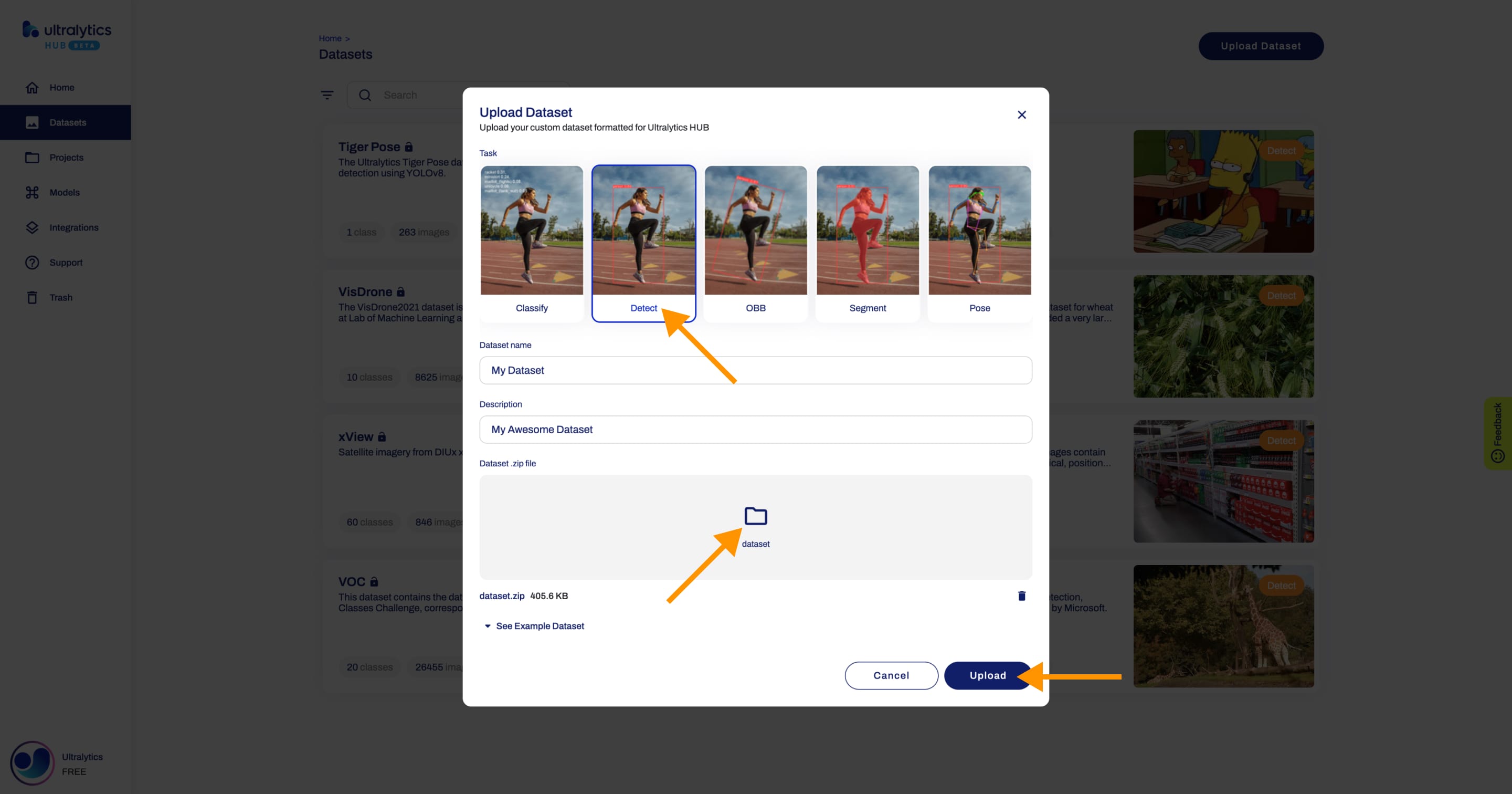
+
+Click on the **Upload Dataset** button on the top right of the page. This action will trigger the **Upload Dataset** dialog.
+
+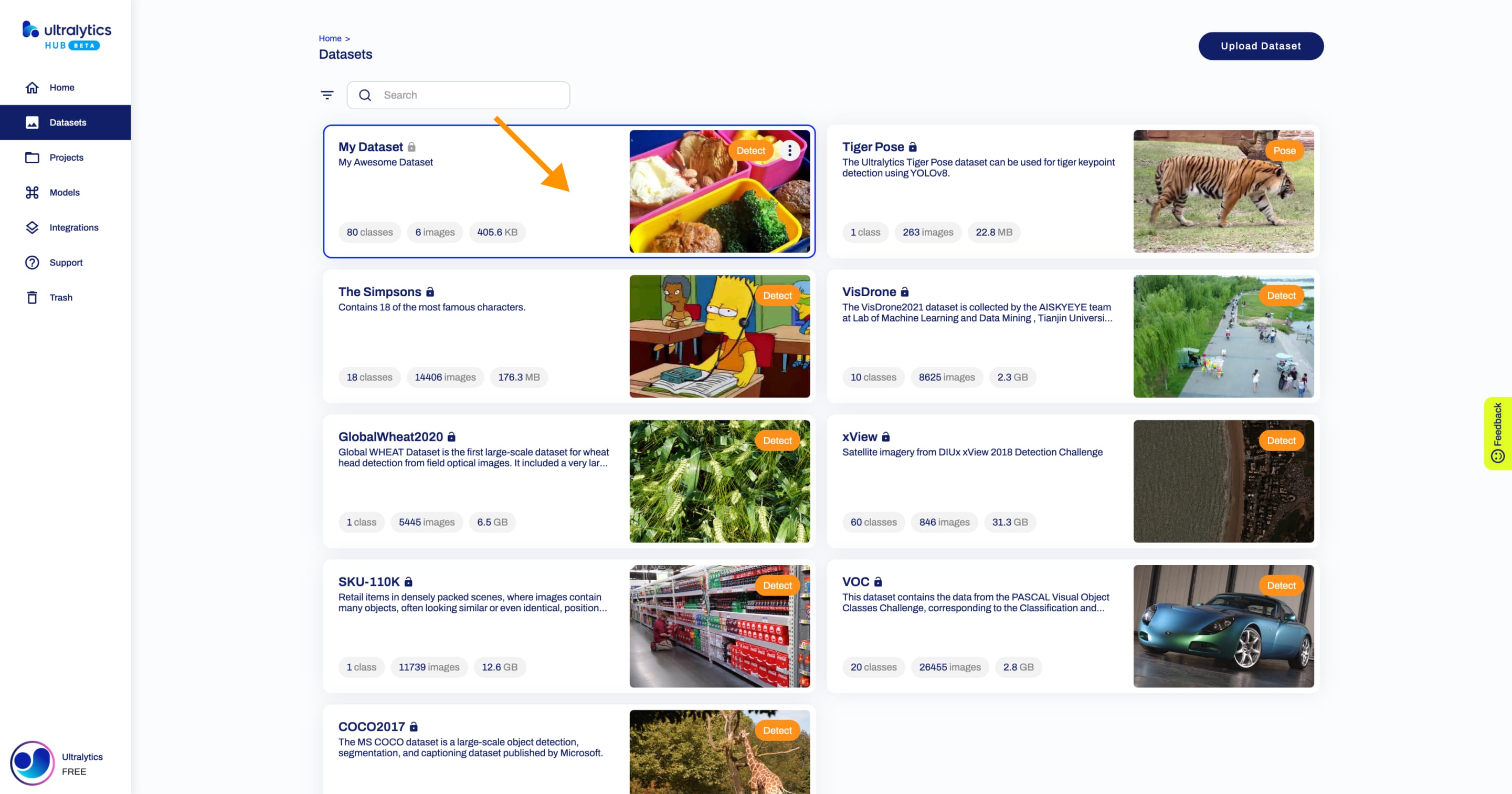
+
+Upload your dataset in the _Dataset .zip file_ field.
+
+You have the additional option to set a custom name and description for your Ultralytics HUB dataset.
+
+When you're happy with your dataset configuration, click **Upload**.
+
+
+
+After your dataset is uploaded and processed, you will be able to access it from the Datasets page.
+
+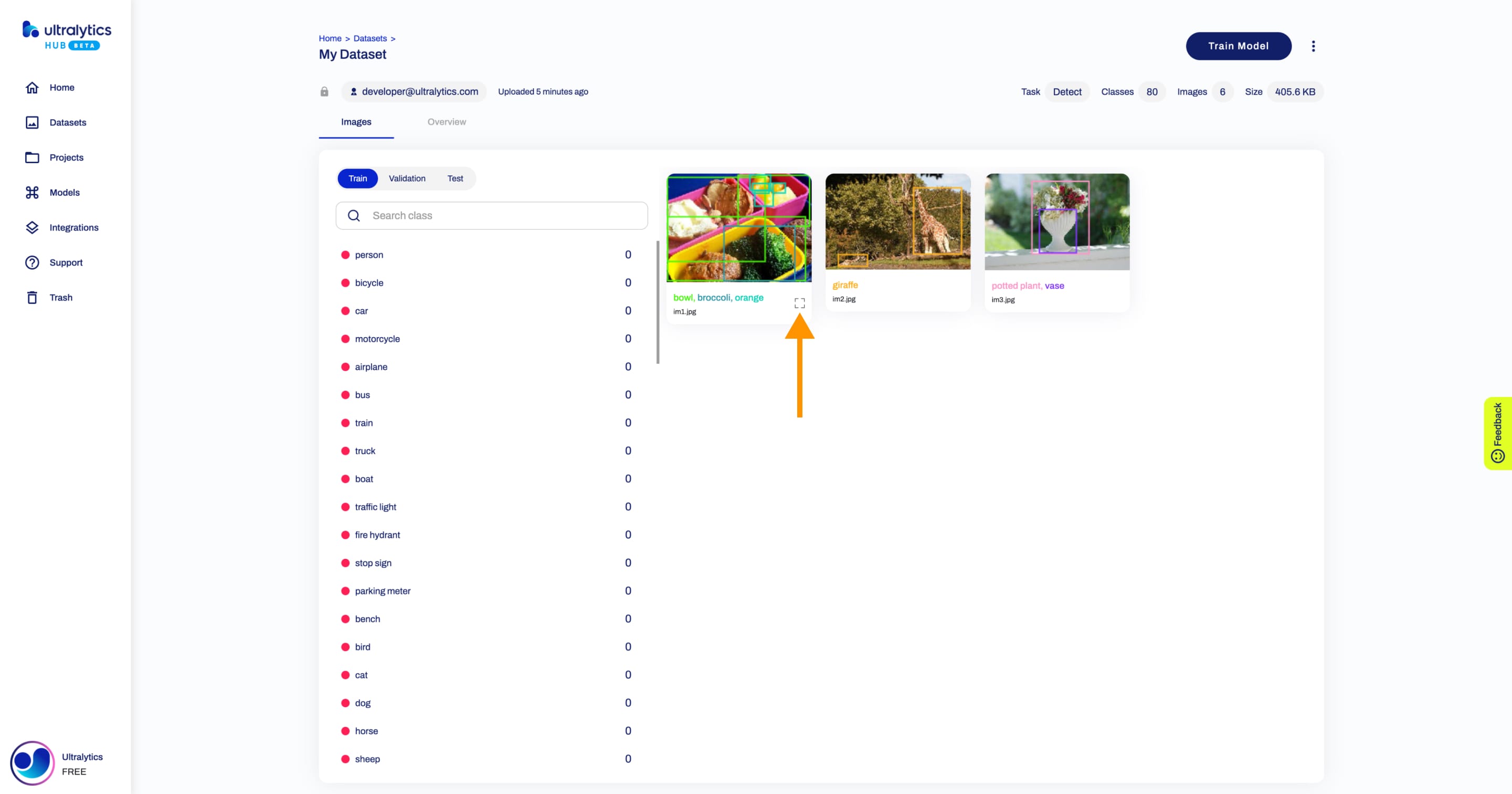
+
+You can view the images in your dataset grouped by splits (Train, Validation, Test).
+
+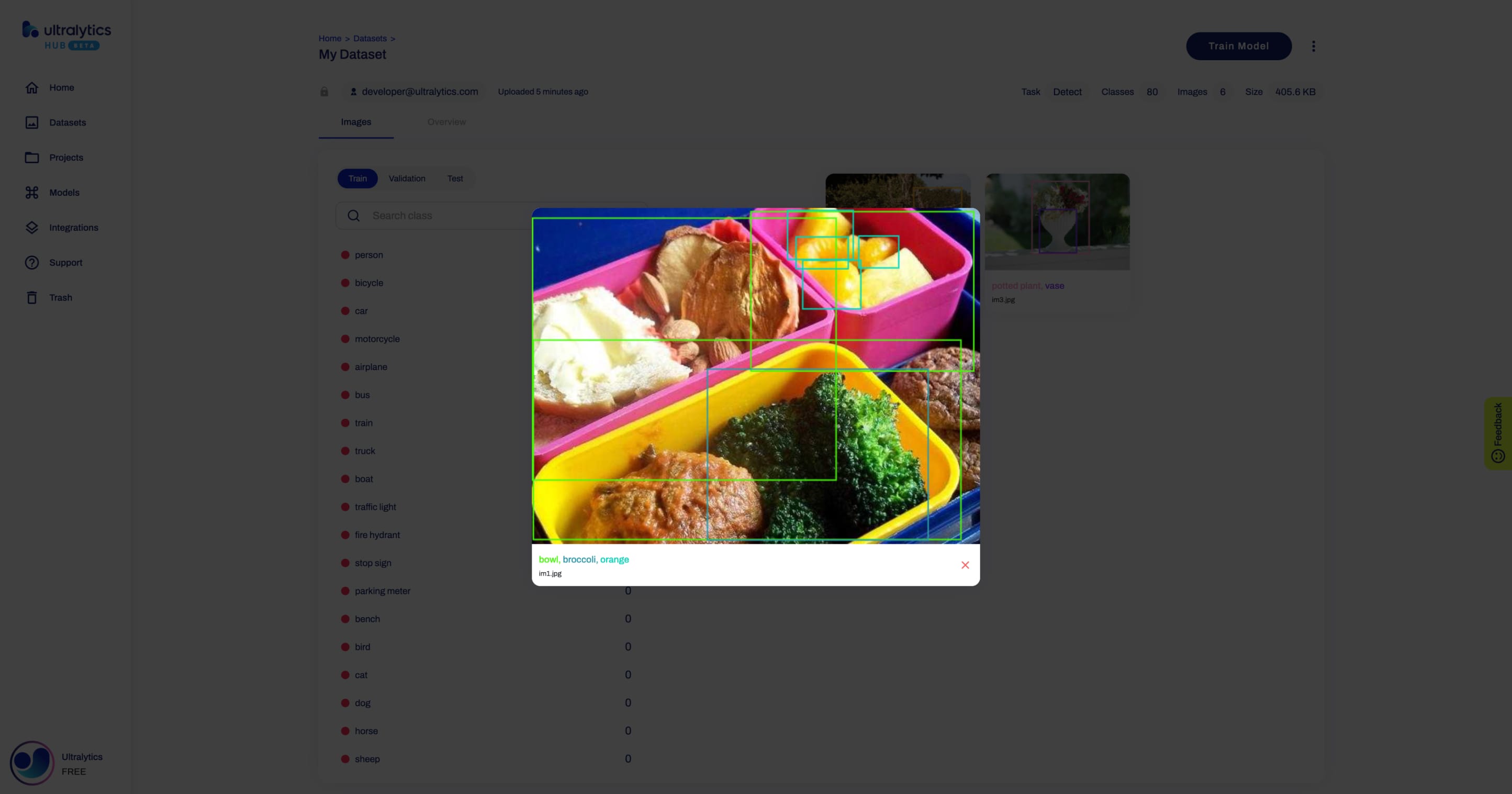
+
+??? tip "Tip"
+
+ Each image can be enlarged for better visualization.
+
+ 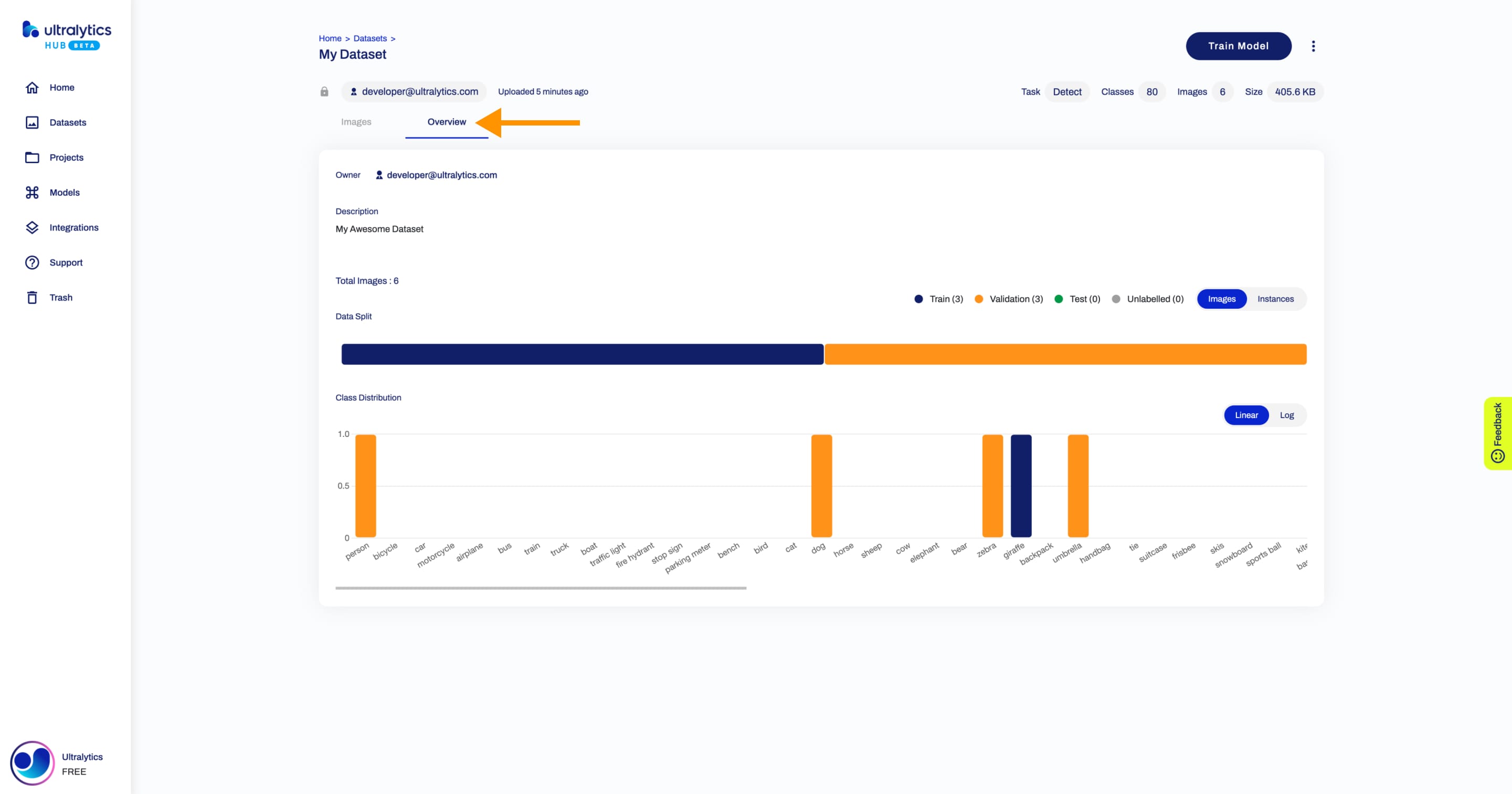
+
+ 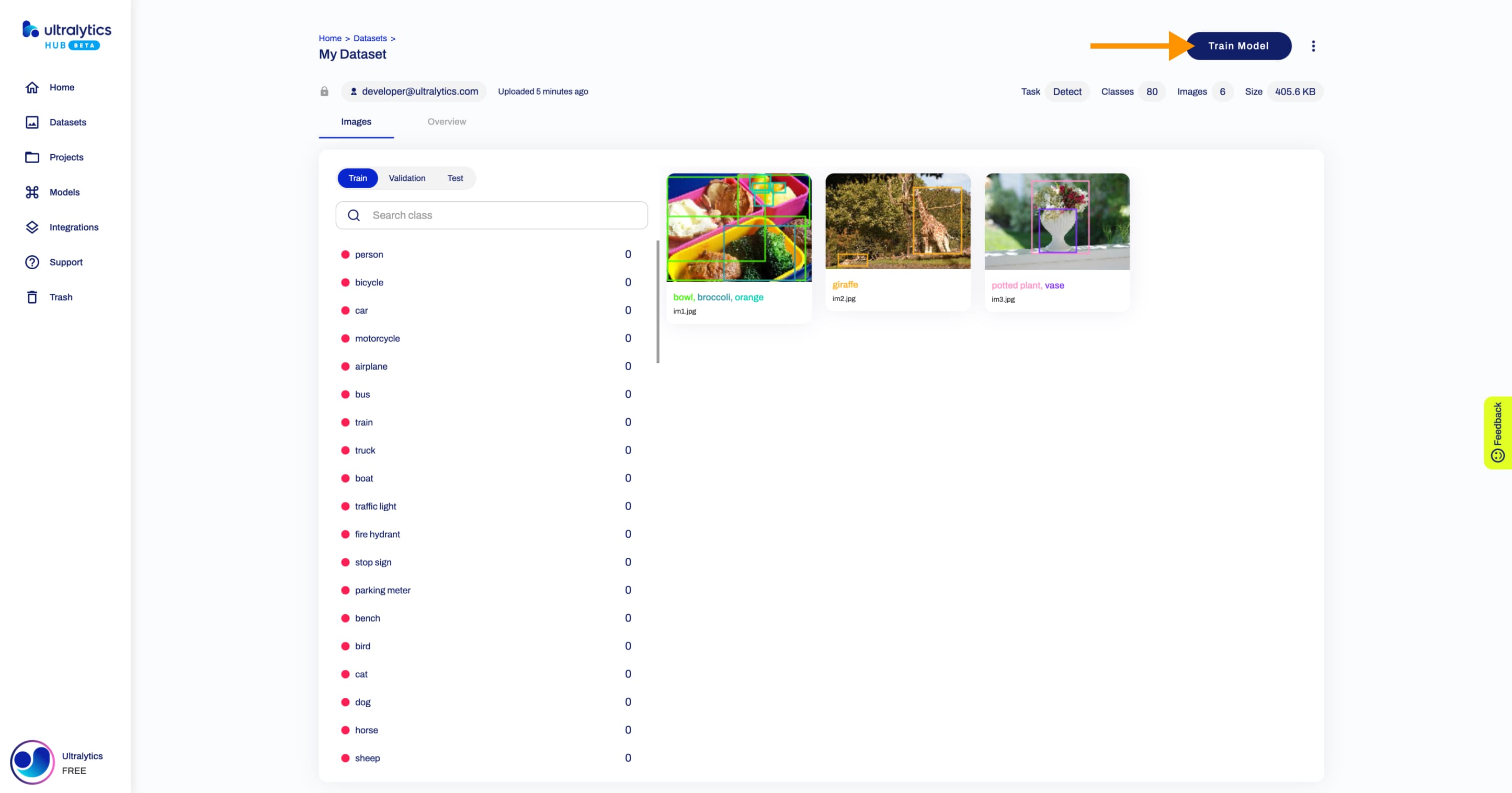
+
+Also, you can analyze your dataset by click on the **Overview** tab.
+
+
+
+Next, [train a model](https://docs.ultralytics.com/hub/models/#train-model) on your dataset.
+
+
+
+## Share Dataset
+
+!!! info "Info"
+
+ Ultralytics HUB's sharing functionality provides a convenient way to share datasets with others. This feature is designed to accommodate both existing Ultralytics HUB users and those who have yet to create an account.
+
+??? note "Note"
+
+ You have control over the general access of your datasets.
+
+ You can choose to set the general access to "Private", in which case, only you will have access to it. Alternatively, you can set the general access to "Unlisted" which grants viewing access to anyone who has the direct link to the dataset, regardless of whether they have an Ultralytics HUB account or not.
+
+Navigate to the Dataset page of the dataset you want to share, open the dataset actions dropdown and click on the **Share** option. This action will trigger the **Share Dataset** dialog.
+
+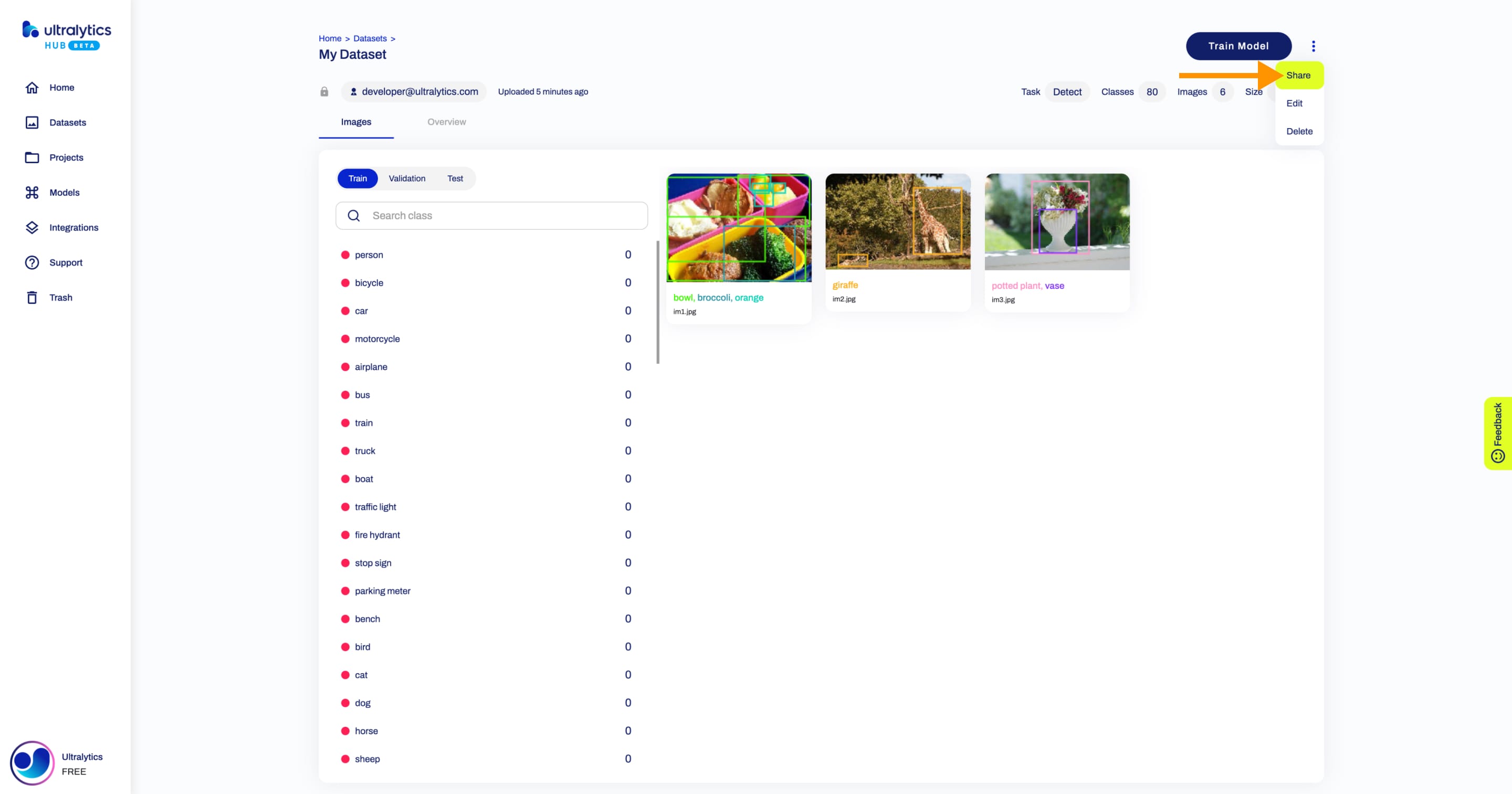
+
+??? tip "Tip"
+
+ You can also share a dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
+
+ 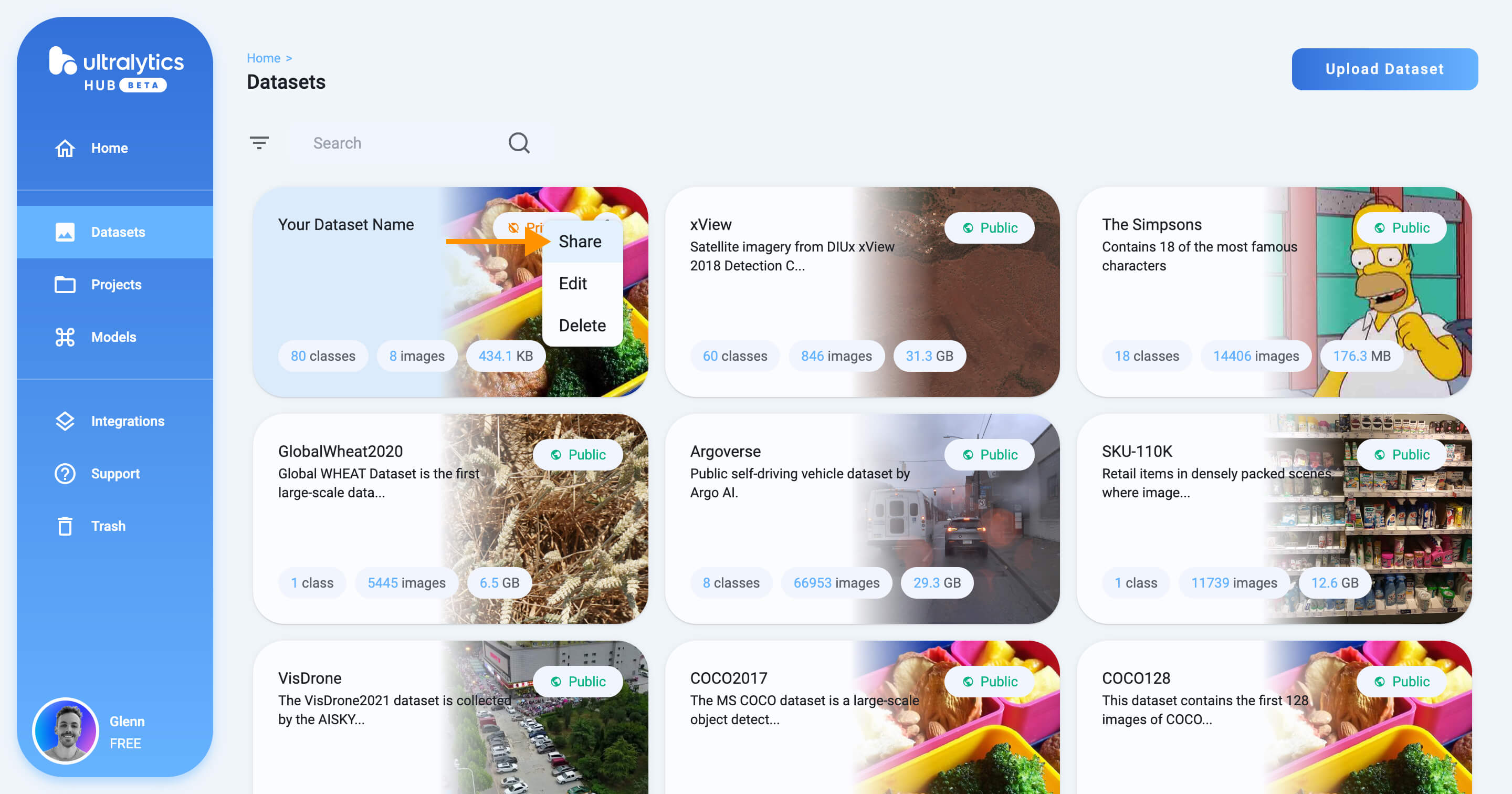
+
+Set the general access to "Unlisted" and click **Save**.
+
+
+
+Now, anyone who has the direct link to your dataset can view it.
+
+??? tip "Tip"
+
+ You can easily click on the dataset's link shown in the **Share Dataset** dialog to copy it.
+
+ 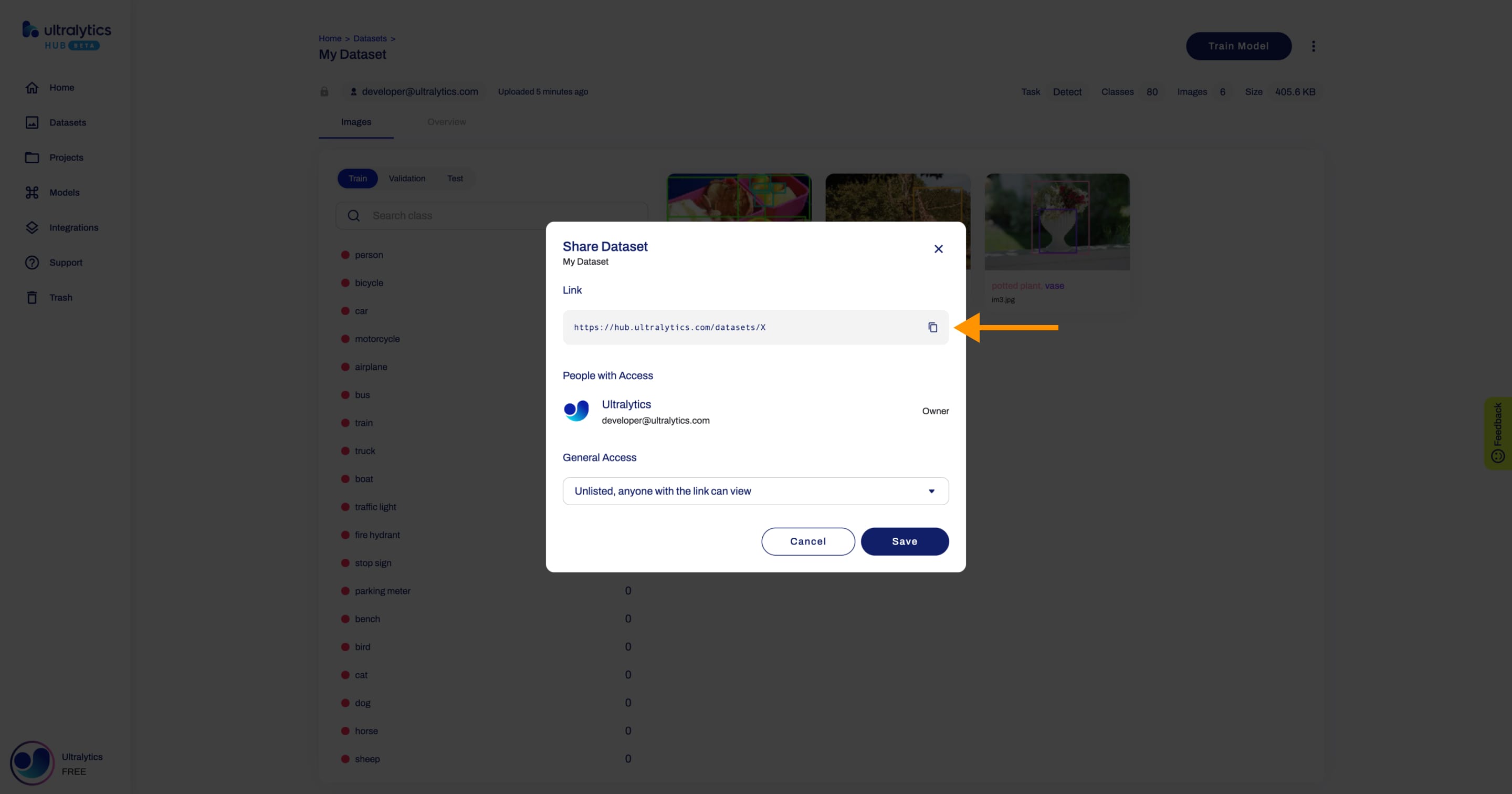
+
+## Edit Dataset
+
+Navigate to the Dataset page of the dataset you want to edit, open the dataset actions dropdown and click on the **Edit** option. This action will trigger the **Update Dataset** dialog.
+
+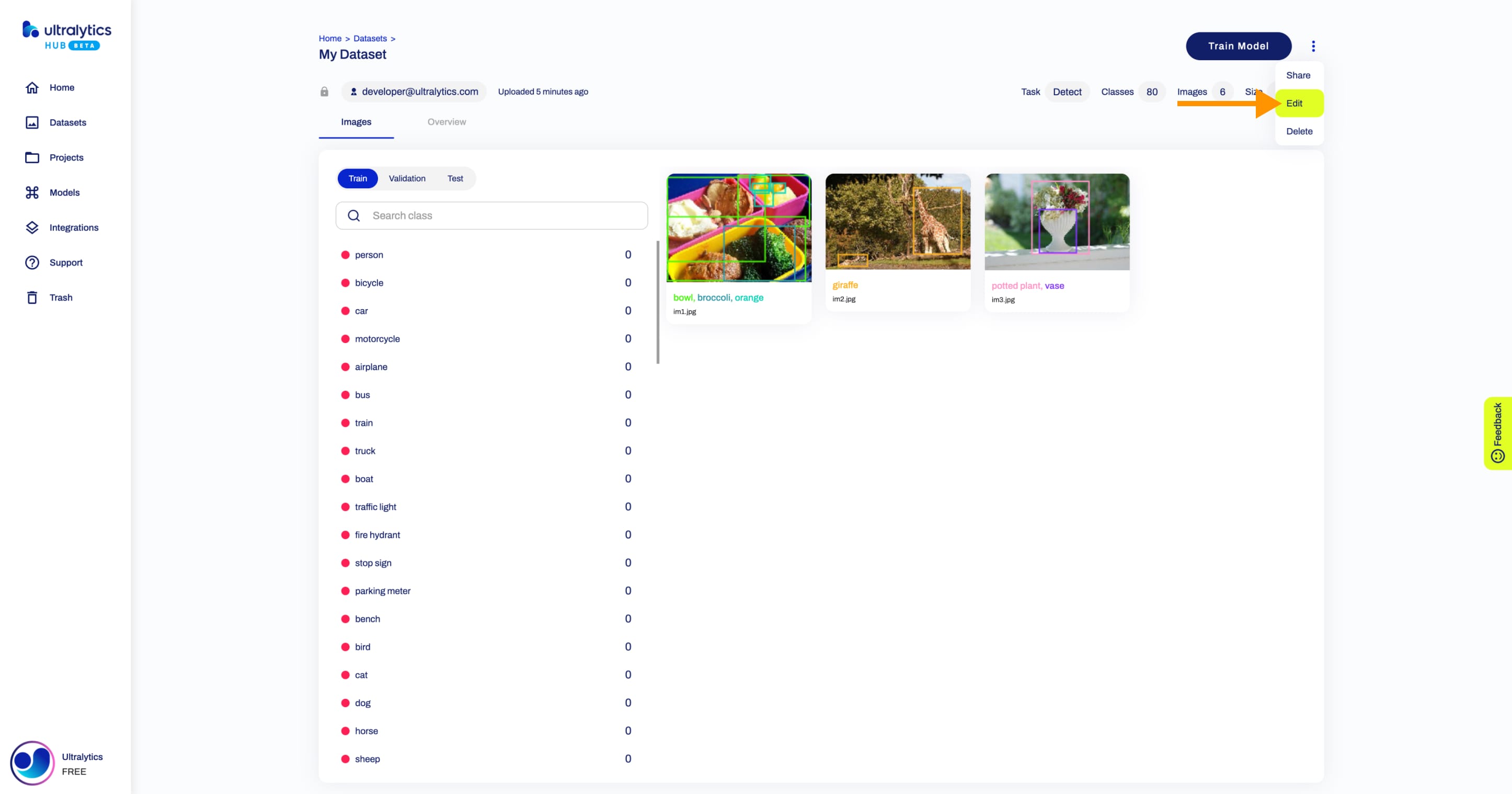
+
+??? tip "Tip"
+
+ You can also edit a dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
+
+ 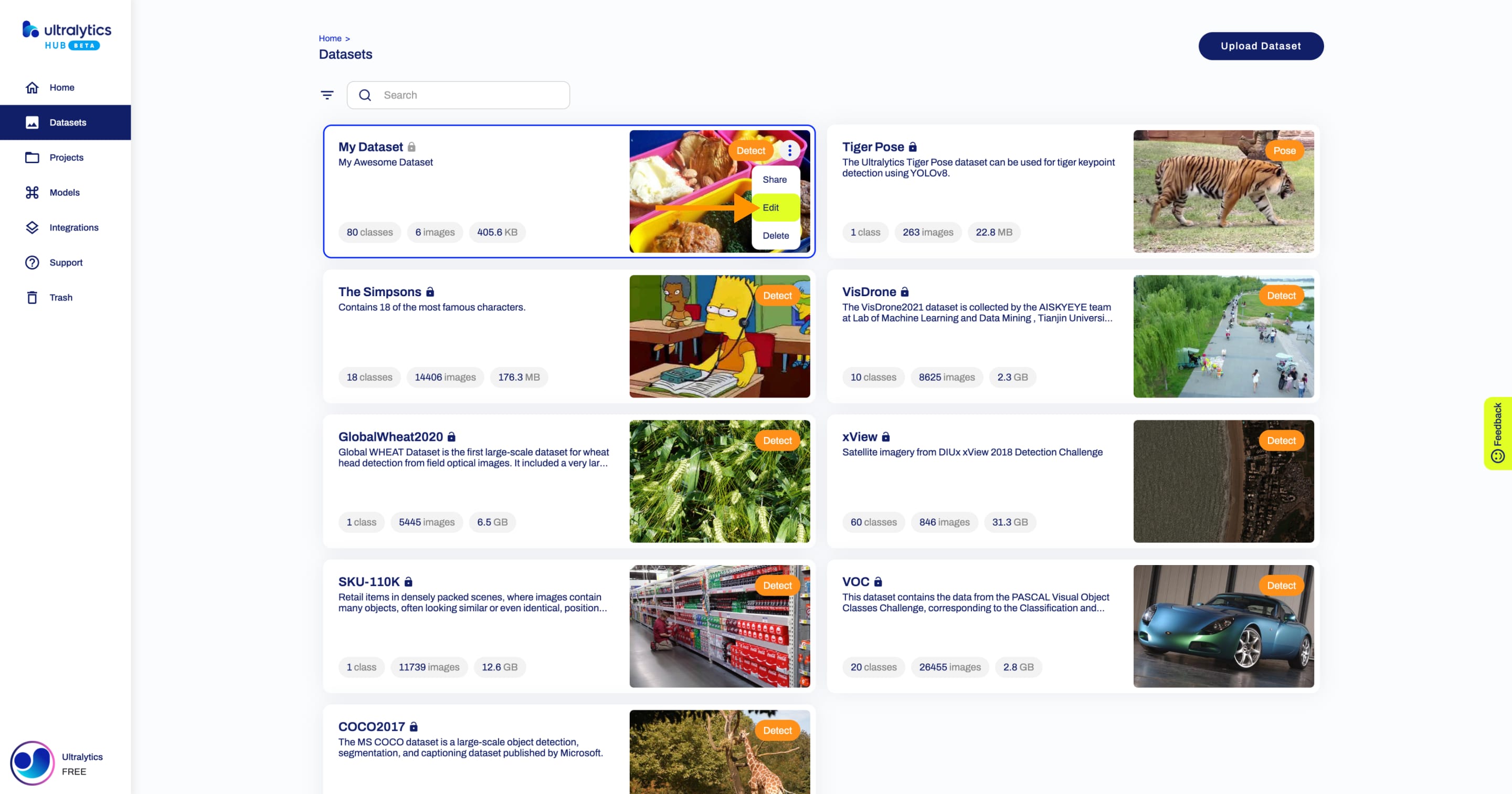
+
+Apply the desired modifications to your dataset and then confirm the changes by clicking **Save**.
+
+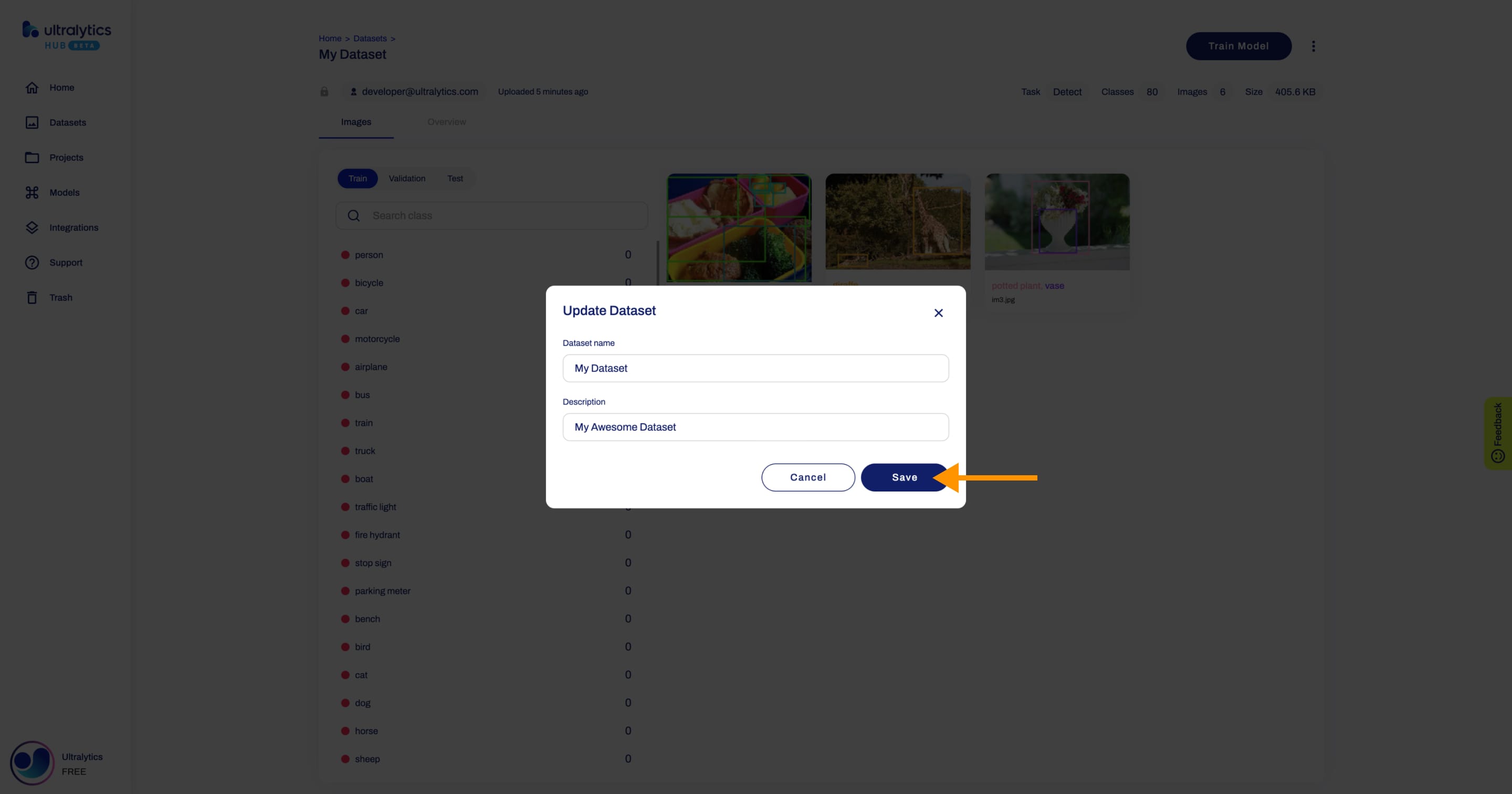
+
+## Delete Dataset
+
+Navigate to the Dataset page of the dataset you want to delete, open the dataset actions dropdown and click on the **Delete** option. This action will delete the dataset.
+
+
+
+??? tip "Tip"
+
+ You can also delete a dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
+
+ 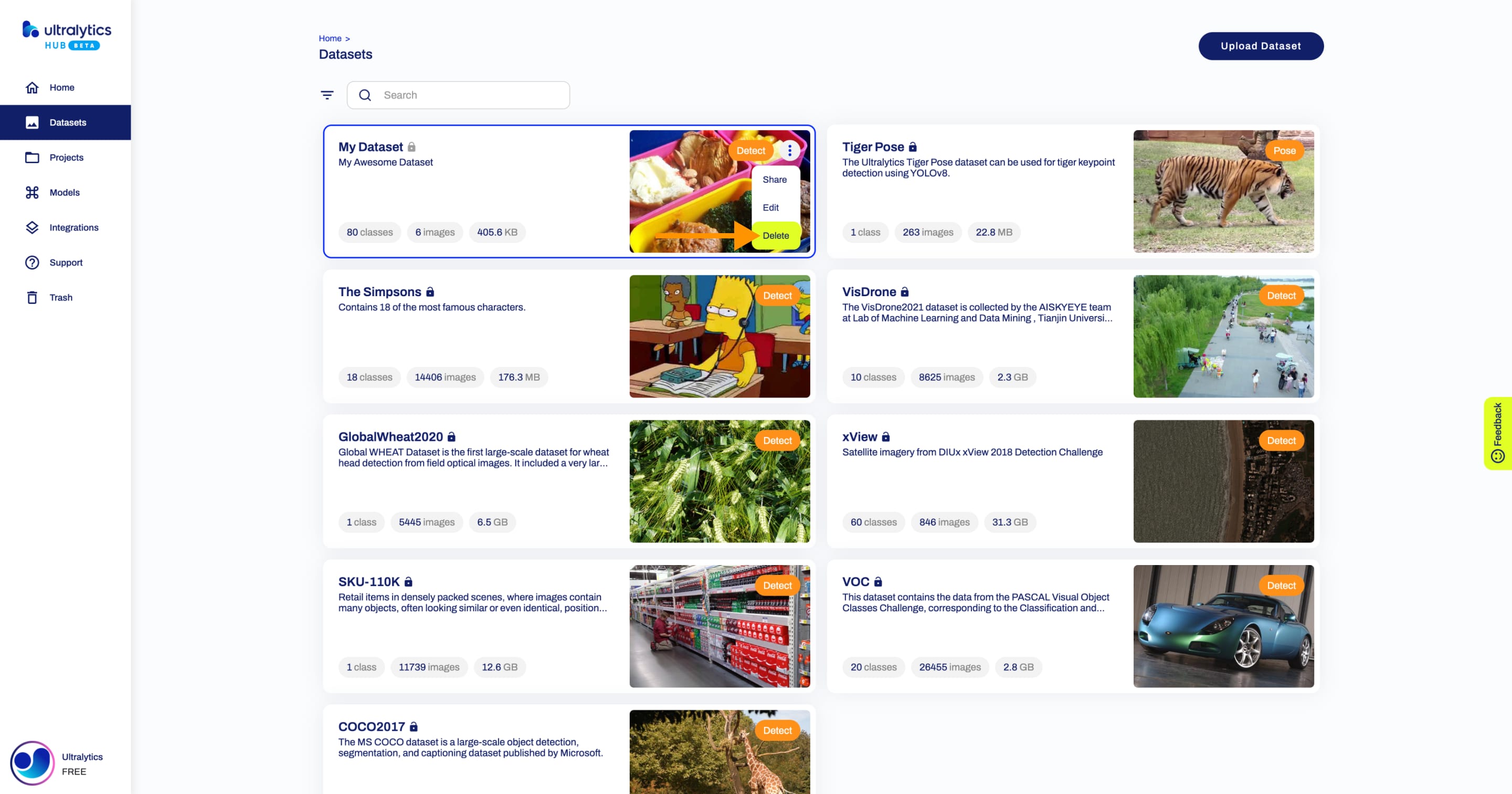
+
+??? note "Note"
+
+ If you change your mind, you can restore the dataset from the [Trash](https://hub.ultralytics.com/trash) page.
+
+ 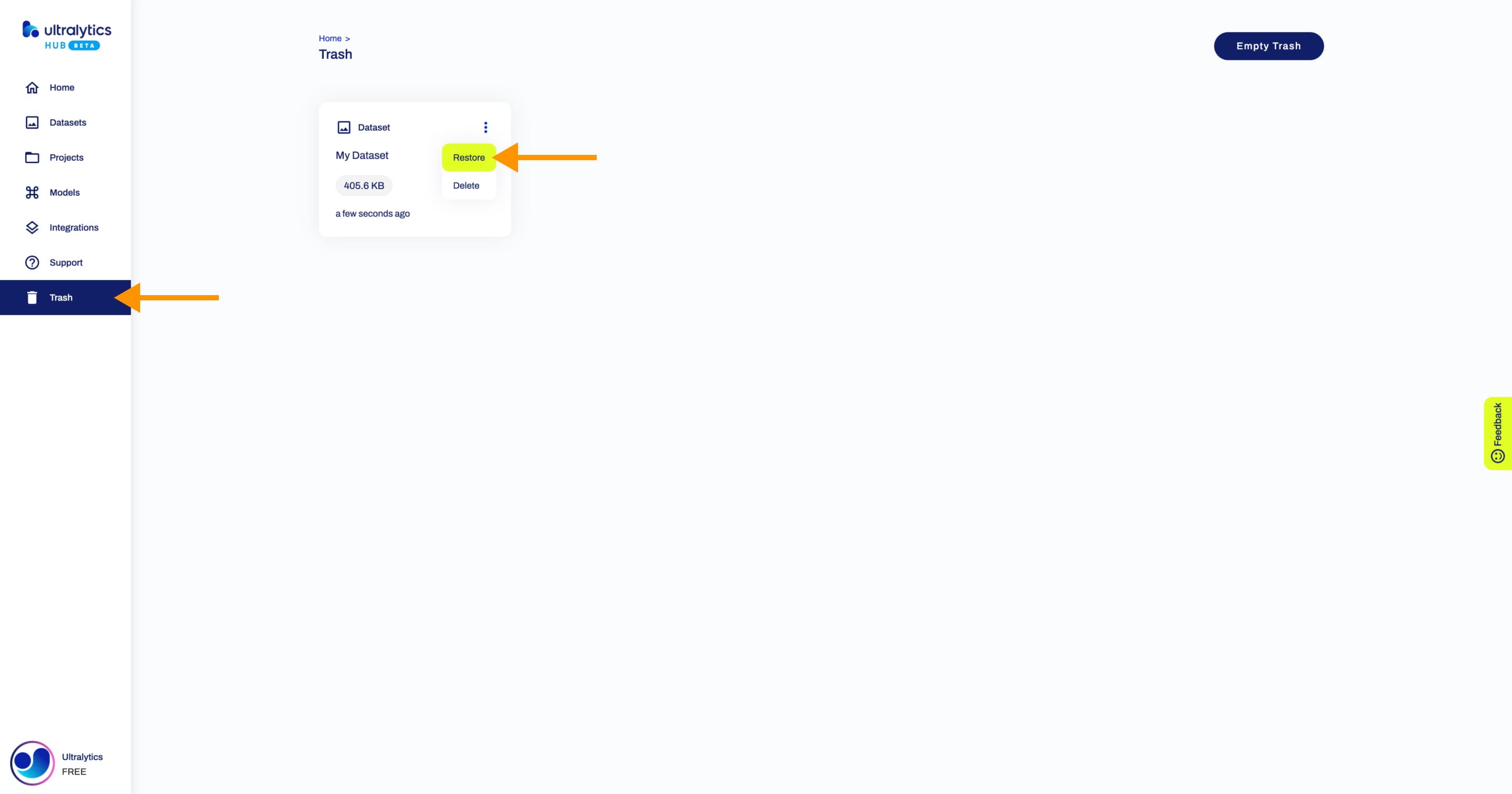
diff --git a/yolov8/docs/hub/index.md b/yolov8/docs/hub/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..30f1652b6f30207904be603d8638a9da6779cb3a
--- /dev/null
+++ b/yolov8/docs/hub/index.md
@@ -0,0 +1,42 @@
+---
+comments: true
+description: 'Ultralytics HUB: Train & deploy YOLO models from one spot! Use drag-and-drop interface with templates & pre-training models. Check quickstart, datasets, and more.'
+keywords: Ultralytics HUB, YOLOv5, YOLOv8, object detection, instance segmentation, classification, drag-and-drop interface, pre-trained models, integrations, mobile app, Inference API, datasets, projects, models
+---
+
+# Ultralytics HUB
+
+
+
+
+
+
+
+
+👋 Hello from the [Ultralytics](https://ultralytics.com/) Team! We've been working hard these last few months to
+launch [Ultralytics HUB](https://bit.ly/ultralytics_hub), a new web tool for training and deploying all your YOLOv5 and YOLOv8 🚀
+models from one spot!
+
+## Introduction
+
+HUB is designed to be user-friendly and intuitive, with a drag-and-drop interface that allows users to
+easily upload their data and train new models quickly. It offers a range of pre-trained models and
+templates to choose from, making it easy for users to get started with training their own models. Once a model is
+trained, it can be easily deployed and used for real-time object detection, instance segmentation and classification tasks.
+
+We hope that the resources here will help you get the most out of HUB. Please browse the HUB Docs for details, raise an issue on GitHub for support, and join our Discord community for questions and discussions!
+
+- [**Quickstart**](./quickstart.md). Start training and deploying YOLO models with HUB in seconds.
+- [**Datasets: Preparing and Uploading**](./datasets.md). Learn how to prepare and upload your datasets to HUB in YOLO format.
+- [**Projects: Creating and Managing**](./projects.md). Group your models into projects for improved organization.
+- [**Models: Training and Exporting**](./models.md). Train YOLOv5 and YOLOv8 models on your custom datasets and export them to various formats for deployment.
+- [**Integrations: Options**](./integrations.md). Explore different integration options for your trained models, such as TensorFlow, ONNX, OpenVINO, CoreML, and PaddlePaddle.
+- [**Ultralytics HUB App**](./app/index.md). Learn about the Ultralytics App for iOS and Android, which allows you to run models directly on your mobile device.
+ * [**iOS**](./app/ios.md). Learn about YOLO CoreML models accelerated on Apple's Neural Engine on iPhones and iPads.
+ * [**Android**](./app/android.md). Explore TFLite acceleration on mobile devices.
+- [**Inference API**](./inference_api.md). Understand how to use the Inference API for running your trained models in the cloud to generate predictions.
\ No newline at end of file
diff --git a/yolov8/docs/hub/inference_api.md b/yolov8/docs/hub/inference_api.md
new file mode 100644
index 0000000000000000000000000000000000000000..22851abade99b73be038c86b63caabd2d49cb4e4
--- /dev/null
+++ b/yolov8/docs/hub/inference_api.md
@@ -0,0 +1,458 @@
+---
+comments: true
+description: Explore Ultralytics YOLOv8 Inference API for efficient object detection. Check out our Python and CLI examples to streamline your image analysis.
+keywords: YOLO, object detection, Ultralytics, inference API, RESTful API
+---
+
+# YOLO Inference API
+
+The YOLO Inference API allows you to access the YOLOv8 object detection capabilities via a RESTful API. This enables you to run object detection on images without the need to install and set up the YOLOv8 environment locally.
+
+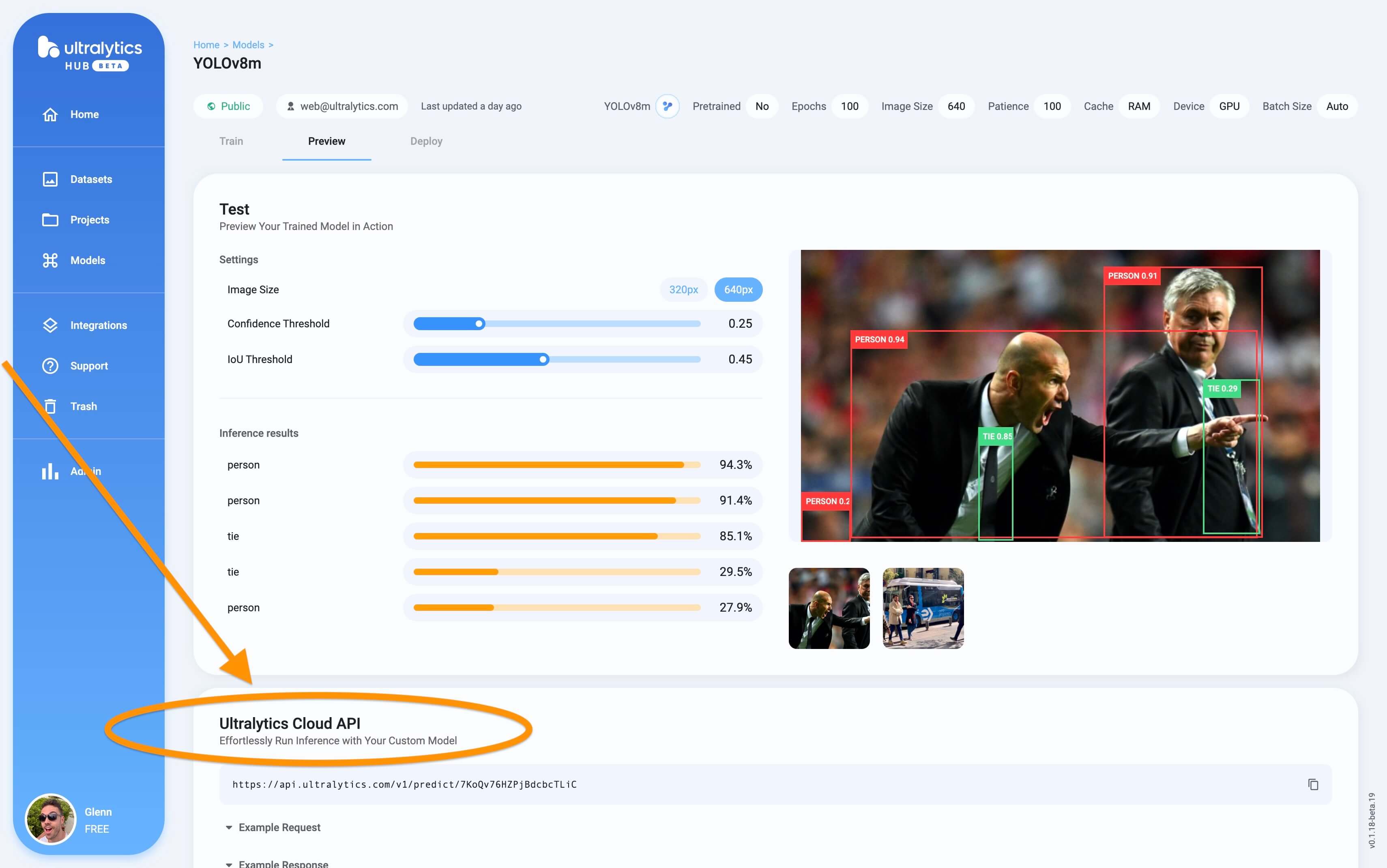
+Screenshot of the Inference API section in the trained model Preview tab.
+
+## API URL
+
+The API URL is the address used to access the YOLO Inference API. In this case, the base URL is:
+
+```
+https://api.ultralytics.com/v1/predict
+```
+
+## Example Usage in Python
+
+To access the YOLO Inference API with the specified model and API key using Python, you can use the following code:
+
+```python
+import requests
+
+# API URL, use actual MODEL_ID
+url = f"https://api.ultralytics.com/v1/predict/MODEL_ID"
+
+# Headers, use actual API_KEY
+headers = {"x-api-key": "API_KEY"}
+
+# Inference arguments (optional)
+data = {"size": 640, "confidence": 0.25, "iou": 0.45}
+
+# Load image and send request
+with open("path/to/image.jpg", "rb") as image_file:
+ files = {"image": image_file}
+ response = requests.post(url, headers=headers, files=files, data=data)
+
+print(response.json())
+```
+
+In this example, replace `API_KEY` with your actual API key, `MODEL_ID` with the desired model ID, and `path/to/image.jpg` with the path to the image you want to analyze.
+
+## Example Usage with CLI
+
+You can use the YOLO Inference API with the command-line interface (CLI) by utilizing the `curl` command. Replace `API_KEY` with your actual API key, `MODEL_ID` with the desired model ID, and `image.jpg` with the path to the image you want to analyze:
+
+```bash
+curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
+ -H "x-api-key: API_KEY" \
+ -F "image=@/path/to/image.jpg" \
+ -F "size=640" \
+ -F "confidence=0.25" \
+ -F "iou=0.45"
+```
+
+## Passing Arguments
+
+This command sends a POST request to the YOLO Inference API with the specified `MODEL_ID` in the URL and the `API_KEY` in the request `headers`, along with the image file specified by `@path/to/image.jpg`.
+
+Here's an example of passing the `size`, `confidence`, and `iou` arguments via the API URL using the `requests` library in Python:
+
+```python
+import requests
+
+# API URL, use actual MODEL_ID
+url = f"https://api.ultralytics.com/v1/predict/MODEL_ID"
+
+# Headers, use actual API_KEY
+headers = {"x-api-key": "API_KEY"}
+
+# Inference arguments (optional)
+data = {"size": 640, "confidence": 0.25, "iou": 0.45}
+
+# Load image and send request
+with open("path/to/image.jpg", "rb") as image_file:
+ files = {"image": image_file}
+ response = requests.post(url, headers=headers, files=files, data=data)
+
+print(response.json())
+```
+
+In this example, the `data` dictionary contains the query arguments `size`, `confidence`, and `iou`, which tells the API to run inference at image size 640 with confidence and IoU thresholds of 0.25 and 0.45.
+
+This will send the query parameters along with the file in the POST request. See the table below for a full list of available inference arguments.
+
+| Inference Argument | Default | Type | Notes |
+|--------------------|---------|---------|------------------------------------------------|
+| `size` | `640` | `int` | valid range is `32` - `1280` pixels |
+| `confidence` | `0.25` | `float` | valid range is `0.01` - `1.0` |
+| `iou` | `0.45` | `float` | valid range is `0.0` - `0.95` |
+| `url` | `''` | `str` | optional image URL if not image file is passed |
+| `normalize` | `False` | `bool` | |
+
+## Return JSON format
+
+The YOLO Inference API returns a JSON list with the detection results. The format of the JSON list will be the same as the one produced locally by the `results[0].tojson()` command.
+
+The JSON list contains information about the detected objects, their coordinates, classes, and confidence scores.
+
+### Detect Model Format
+
+YOLO detection models, such as `yolov8n.pt`, can return JSON responses from local inference, CLI API inference, and Python API inference. All of these methods produce the same JSON response format.
+
+!!! example "Detect Model JSON Response"
+
+ === "Local"
+ ```python
+ from ultralytics import YOLO
+
+ # Load model
+ model = YOLO('yolov8n.pt')
+
+ # Run inference
+ results = model('image.jpg')
+
+ # Print image.jpg results in JSON format
+ print(results[0].tojson())
+ ```
+
+ === "CLI API"
+ ```bash
+ curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
+ -H "x-api-key: API_KEY" \
+ -F "image=@/path/to/image.jpg" \
+ -F "size=640" \
+ -F "confidence=0.25" \
+ -F "iou=0.45"
+ ```
+
+ === "Python API"
+ ```python
+ import requests
+
+ # API URL, use actual MODEL_ID
+ url = f"https://api.ultralytics.com/v1/predict/MODEL_ID"
+
+ # Headers, use actual API_KEY
+ headers = {"x-api-key": "API_KEY"}
+
+ # Inference arguments (optional)
+ data = {"size": 640, "confidence": 0.25, "iou": 0.45}
+
+ # Load image and send request
+ with open("path/to/image.jpg", "rb") as image_file:
+ files = {"image": image_file}
+ response = requests.post(url, headers=headers, files=files, data=data)
+
+ print(response.json())
+ ```
+
+ === "JSON Response"
+ ```json
+ {
+ "success": True,
+ "message": "Inference complete.",
+ "data": [
+ {
+ "name": "person",
+ "class": 0,
+ "confidence": 0.8359682559967041,
+ "box": {
+ "x1": 0.08974208831787109,
+ "y1": 0.27418340047200523,
+ "x2": 0.8706787109375,
+ "y2": 0.9887352837456598
+ }
+ },
+ {
+ "name": "person",
+ "class": 0,
+ "confidence": 0.8189555406570435,
+ "box": {
+ "x1": 0.5847355842590332,
+ "y1": 0.05813225640190972,
+ "x2": 0.8930277824401855,
+ "y2": 0.9903111775716146
+ }
+ },
+ {
+ "name": "tie",
+ "class": 27,
+ "confidence": 0.2909725308418274,
+ "box": {
+ "x1": 0.3433395862579346,
+ "y1": 0.6070465511745877,
+ "x2": 0.40964522361755373,
+ "y2": 0.9849439832899306
+ }
+ }
+ ]
+ }
+ ```
+
+### Segment Model Format
+
+YOLO segmentation models, such as `yolov8n-seg.pt`, can return JSON responses from local inference, CLI API inference, and Python API inference. All of these methods produce the same JSON response format.
+
+!!! example "Segment Model JSON Response"
+
+ === "Local"
+ ```python
+ from ultralytics import YOLO
+
+ # Load model
+ model = YOLO('yolov8n-seg.pt')
+
+ # Run inference
+ results = model('image.jpg')
+
+ # Print image.jpg results in JSON format
+ print(results[0].tojson())
+ ```
+
+ === "CLI API"
+ ```bash
+ curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
+ -H "x-api-key: API_KEY" \
+ -F "image=@/path/to/image.jpg" \
+ -F "size=640" \
+ -F "confidence=0.25" \
+ -F "iou=0.45"
+ ```
+
+ === "Python API"
+ ```python
+ import requests
+
+ # API URL, use actual MODEL_ID
+ url = f"https://api.ultralytics.com/v1/predict/MODEL_ID"
+
+ # Headers, use actual API_KEY
+ headers = {"x-api-key": "API_KEY"}
+
+ # Inference arguments (optional)
+ data = {"size": 640, "confidence": 0.25, "iou": 0.45}
+
+ # Load image and send request
+ with open("path/to/image.jpg", "rb") as image_file:
+ files = {"image": image_file}
+ response = requests.post(url, headers=headers, files=files, data=data)
+
+ print(response.json())
+ ```
+
+ === "JSON Response"
+ Note `segments` `x` and `y` lengths may vary from one object to another. Larger or more complex objects may have more segment points.
+ ```json
+ {
+ "success": True,
+ "message": "Inference complete.",
+ "data": [
+ {
+ "name": "person",
+ "class": 0,
+ "confidence": 0.856913149356842,
+ "box": {
+ "x1": 0.1064866065979004,
+ "y1": 0.2798851860894097,
+ "x2": 0.8738358497619629,
+ "y2": 0.9894873725043403
+ },
+ "segments": {
+ "x": [
+ 0.421875,
+ 0.4203124940395355,
+ 0.41718751192092896
+ ...
+ ],
+ "y": [
+ 0.2888889014720917,
+ 0.2916666567325592,
+ 0.2916666567325592
+ ...
+ ]
+ }
+ },
+ {
+ "name": "person",
+ "class": 0,
+ "confidence": 0.8512625694274902,
+ "box": {
+ "x1": 0.5757311820983887,
+ "y1": 0.053943040635850696,
+ "x2": 0.8960096359252929,
+ "y2": 0.985154045952691
+ },
+ "segments": {
+ "x": [
+ 0.7515624761581421,
+ 0.75,
+ 0.7437499761581421
+ ...
+ ],
+ "y": [
+ 0.0555555559694767,
+ 0.05833333358168602,
+ 0.05833333358168602
+ ...
+ ]
+ }
+ },
+ {
+ "name": "tie",
+ "class": 27,
+ "confidence": 0.6485961675643921,
+ "box": {
+ "x1": 0.33911995887756347,
+ "y1": 0.6057066175672743,
+ "x2": 0.4081430912017822,
+ "y2": 0.9916408962673611
+ },
+ "segments": {
+ "x": [
+ 0.37187498807907104,
+ 0.37031251192092896,
+ 0.3687500059604645
+ ...
+ ],
+ "y": [
+ 0.6111111044883728,
+ 0.6138888597488403,
+ 0.6138888597488403
+ ...
+ ]
+ }
+ }
+ ]
+ }
+ ```
+
+### Pose Model Format
+
+YOLO pose models, such as `yolov8n-pose.pt`, can return JSON responses from local inference, CLI API inference, and Python API inference. All of these methods produce the same JSON response format.
+
+!!! example "Pose Model JSON Response"
+
+ === "Local"
+ ```python
+ from ultralytics import YOLO
+
+ # Load model
+ model = YOLO('yolov8n-seg.pt')
+
+ # Run inference
+ results = model('image.jpg')
+
+ # Print image.jpg results in JSON format
+ print(results[0].tojson())
+ ```
+
+ === "CLI API"
+ ```bash
+ curl -X POST "https://api.ultralytics.com/v1/predict/MODEL_ID" \
+ -H "x-api-key: API_KEY" \
+ -F "image=@/path/to/image.jpg" \
+ -F "size=640" \
+ -F "confidence=0.25" \
+ -F "iou=0.45"
+ ```
+
+ === "Python API"
+ ```python
+ import requests
+
+ # API URL, use actual MODEL_ID
+ url = f"https://api.ultralytics.com/v1/predict/MODEL_ID"
+
+ # Headers, use actual API_KEY
+ headers = {"x-api-key": "API_KEY"}
+
+ # Inference arguments (optional)
+ data = {"size": 640, "confidence": 0.25, "iou": 0.45}
+
+ # Load image and send request
+ with open("path/to/image.jpg", "rb") as image_file:
+ files = {"image": image_file}
+ response = requests.post(url, headers=headers, files=files, data=data)
+
+ print(response.json())
+ ```
+
+ === "JSON Response"
+ Note COCO-keypoints pretrained models will have 17 human keypoints. The `visible` part of the keypoints indicates whether a keypoint is visible or obscured. Obscured keypoints may be outside the image or may not be visible, i.e. a person's eyes facing away from the camera.
+ ```json
+ {
+ "success": True,
+ "message": "Inference complete.",
+ "data": [
+ {
+ "name": "person",
+ "class": 0,
+ "confidence": 0.8439509868621826,
+ "box": {
+ "x1": 0.1125,
+ "y1": 0.28194444444444444,
+ "x2": 0.7953125,
+ "y2": 0.9902777777777778
+ },
+ "keypoints": {
+ "x": [
+ 0.5058594942092896,
+ 0.5103894472122192,
+ 0.4920862317085266
+ ...
+ ],
+ "y": [
+ 0.48964157700538635,
+ 0.4643048942089081,
+ 0.4465252459049225
+ ...
+ ],
+ "visible": [
+ 0.8726999163627625,
+ 0.653947651386261,
+ 0.9130823612213135
+ ...
+ ]
+ }
+ },
+ {
+ "name": "person",
+ "class": 0,
+ "confidence": 0.7474289536476135,
+ "box": {
+ "x1": 0.58125,
+ "y1": 0.0625,
+ "x2": 0.8859375,
+ "y2": 0.9888888888888889
+ },
+ "keypoints": {
+ "x": [
+ 0.778544008731842,
+ 0.7976160049438477,
+ 0.7530890107154846
+ ...
+ ],
+ "y": [
+ 0.27595141530036926,
+ 0.2378823608160019,
+ 0.23644638061523438
+ ...
+ ],
+ "visible": [
+ 0.8900790810585022,
+ 0.789978563785553,
+ 0.8974530100822449
+ ...
+ ]
+ }
+ }
+ ]
+ }
+ ```
\ No newline at end of file
diff --git a/yolov8/docs/hub/integrations.md b/yolov8/docs/hub/integrations.md
new file mode 100644
index 0000000000000000000000000000000000000000..19480d27d8234ce677ded54d5e134bc8862bc0bf
--- /dev/null
+++ b/yolov8/docs/hub/integrations.md
@@ -0,0 +1,7 @@
+---
+comments: true
+---
+
+# 🚧 Page Under Construction ⚒
+
+This page is currently under construction!️ 👷Please check back later for updates. 😃🔜
diff --git a/yolov8/docs/hub/models.md b/yolov8/docs/hub/models.md
new file mode 100644
index 0000000000000000000000000000000000000000..31cba2df9fe47e697bd2575b72fca4a9e2c703fd
--- /dev/null
+++ b/yolov8/docs/hub/models.md
@@ -0,0 +1,213 @@
+---
+comments: true
+description: Train and Deploy your Model to 13 different formats, including TensorFlow, ONNX, OpenVINO, CoreML, Paddle or directly on Mobile.
+keywords: Ultralytics, HUB, models, artificial intelligence, APIs, export models, TensorFlow, ONNX, Paddle, OpenVINO, CoreML, iOS, Android
+---
+
+# Ultralytics HUB Models
+
+Ultralytics HUB models provide a streamlined solution for training vision AI models on your custom datasets.
+
+The process is user-friendly and efficient, involving a simple three-step creation and accelerated training powered by Utralytics YOLOv8. During training, real-time updates on model metrics are available so that you can monitor each step of the progress. Once training is completed, you can preview your model and easily deploy it to real-world applications. Therefore, Ultralytics HUB offers a comprehensive yet straightforward system for model creation, training, evaluation, and deployment.
+
+## Train Model
+
+Navigate to the [Models](https://hub.ultralytics.com/models) page by clicking on the **Models** button in the sidebar.
+
+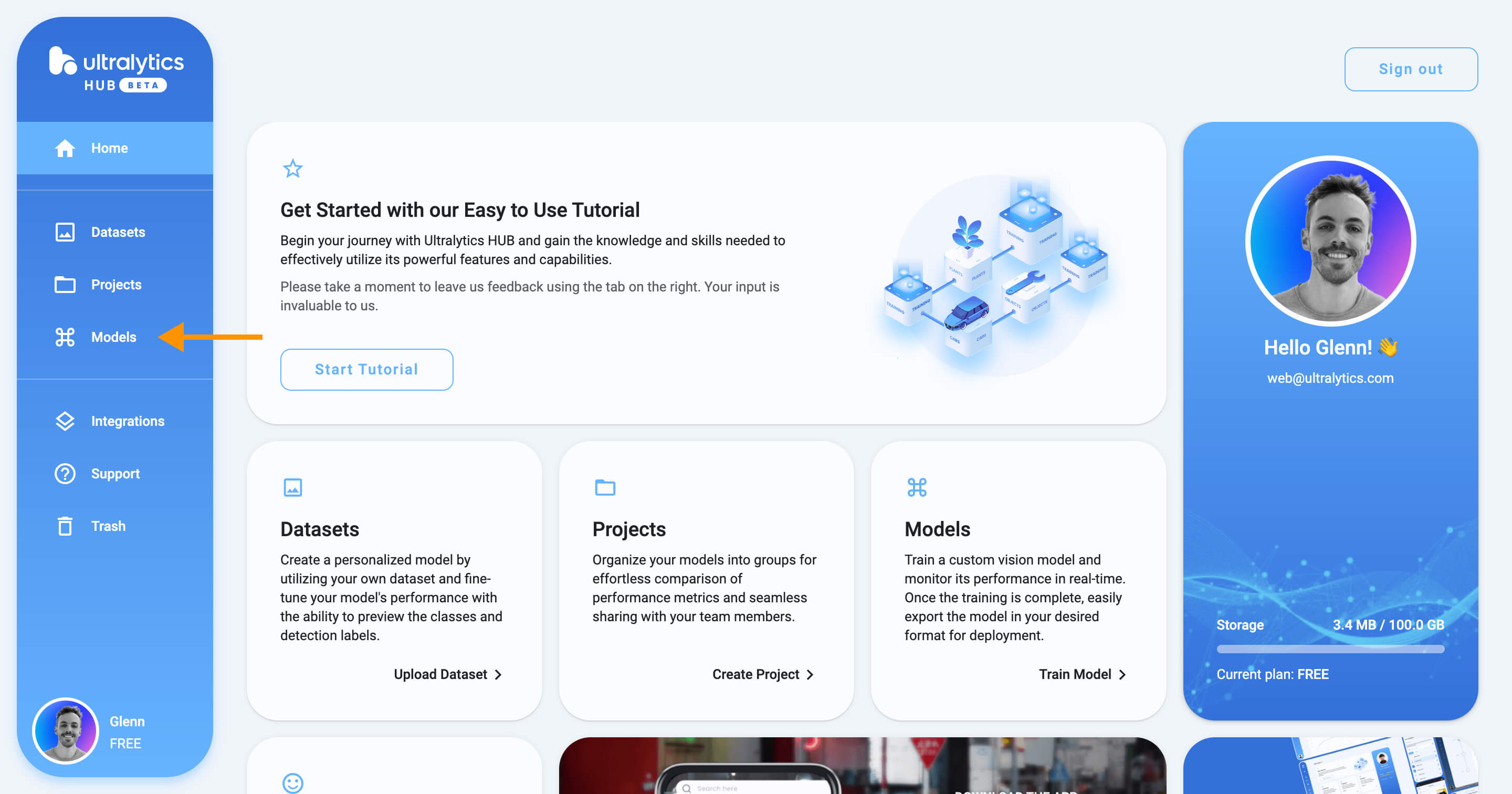
+
+??? tip "Tip"
+
+ You can also train a model directly from the [Home](https://hub.ultralytics.com/home) page.
+
+ 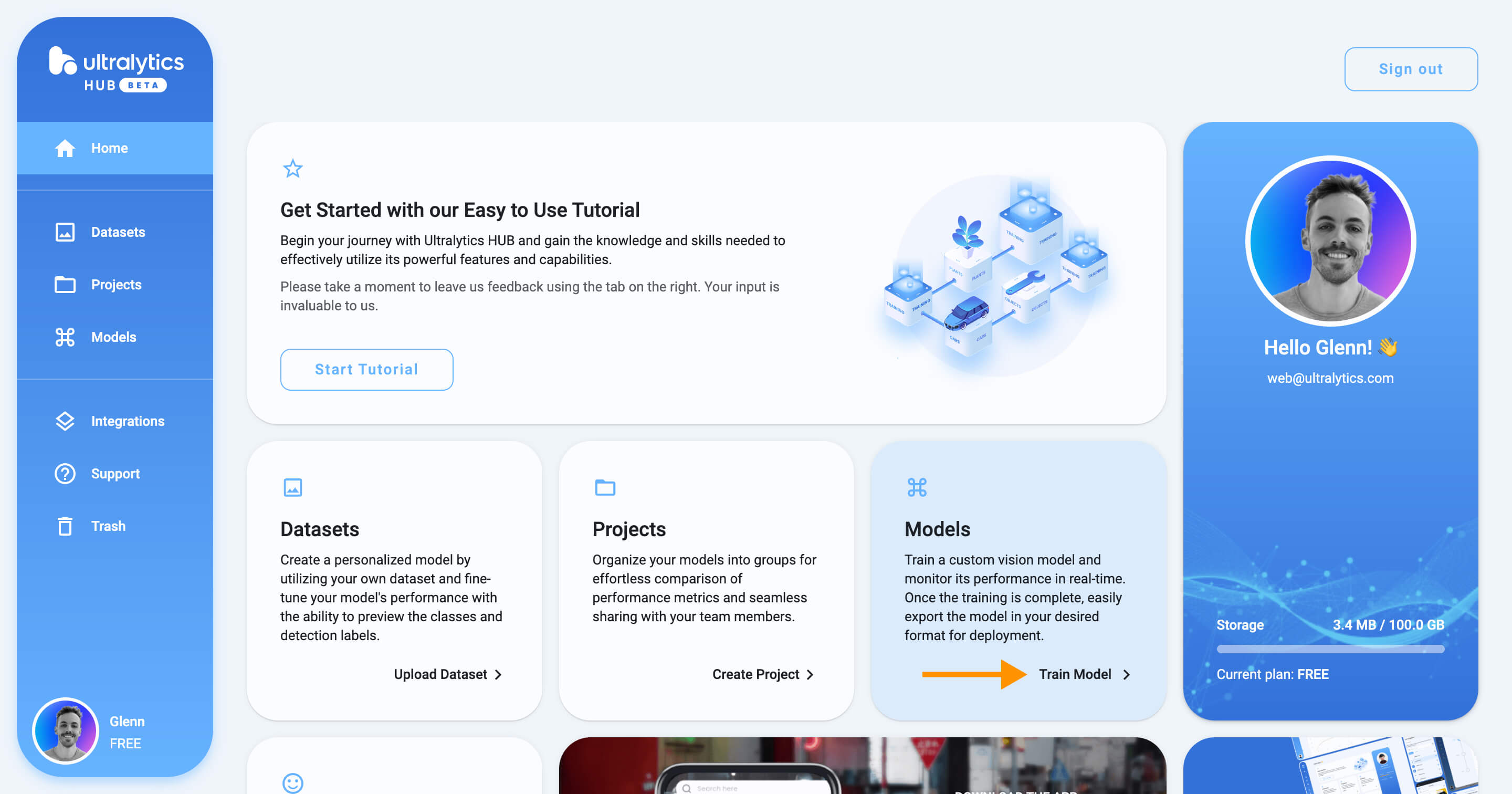
+
+Click on the **Train Model** button on the top right of the page. This action will trigger the **Train Model** dialog.
+
+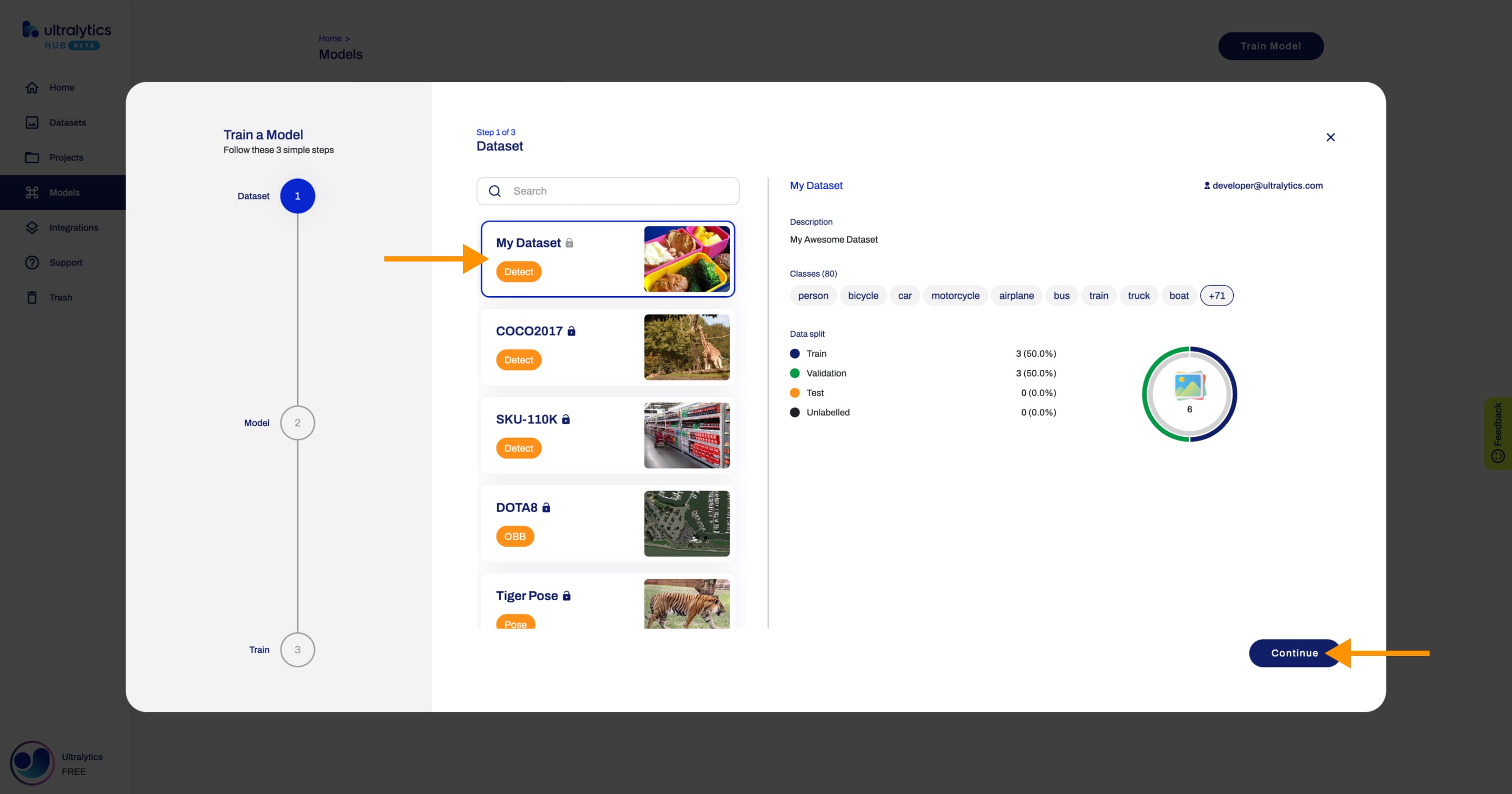
+
+The **Train Model** dialog has three simple steps, explained below.
+
+### 1. Dataset
+
+In this step, you have to select the dataset you want to train your model on. After you selected a dataset, click **Continue**.
+
+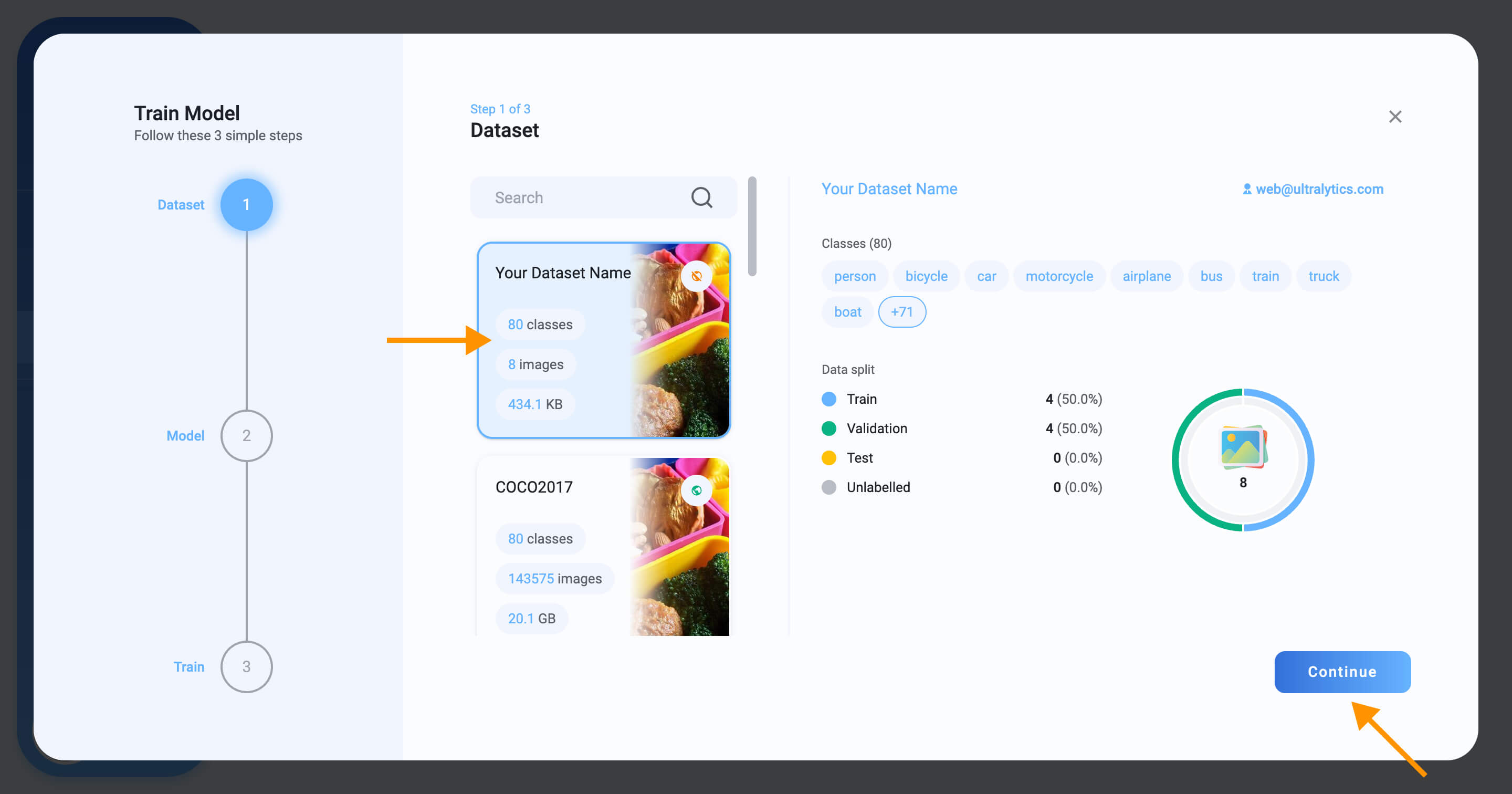
+
+??? tip "Tip"
+
+ You can skip this step if you train a model directly from the Dataset page.
+
+ 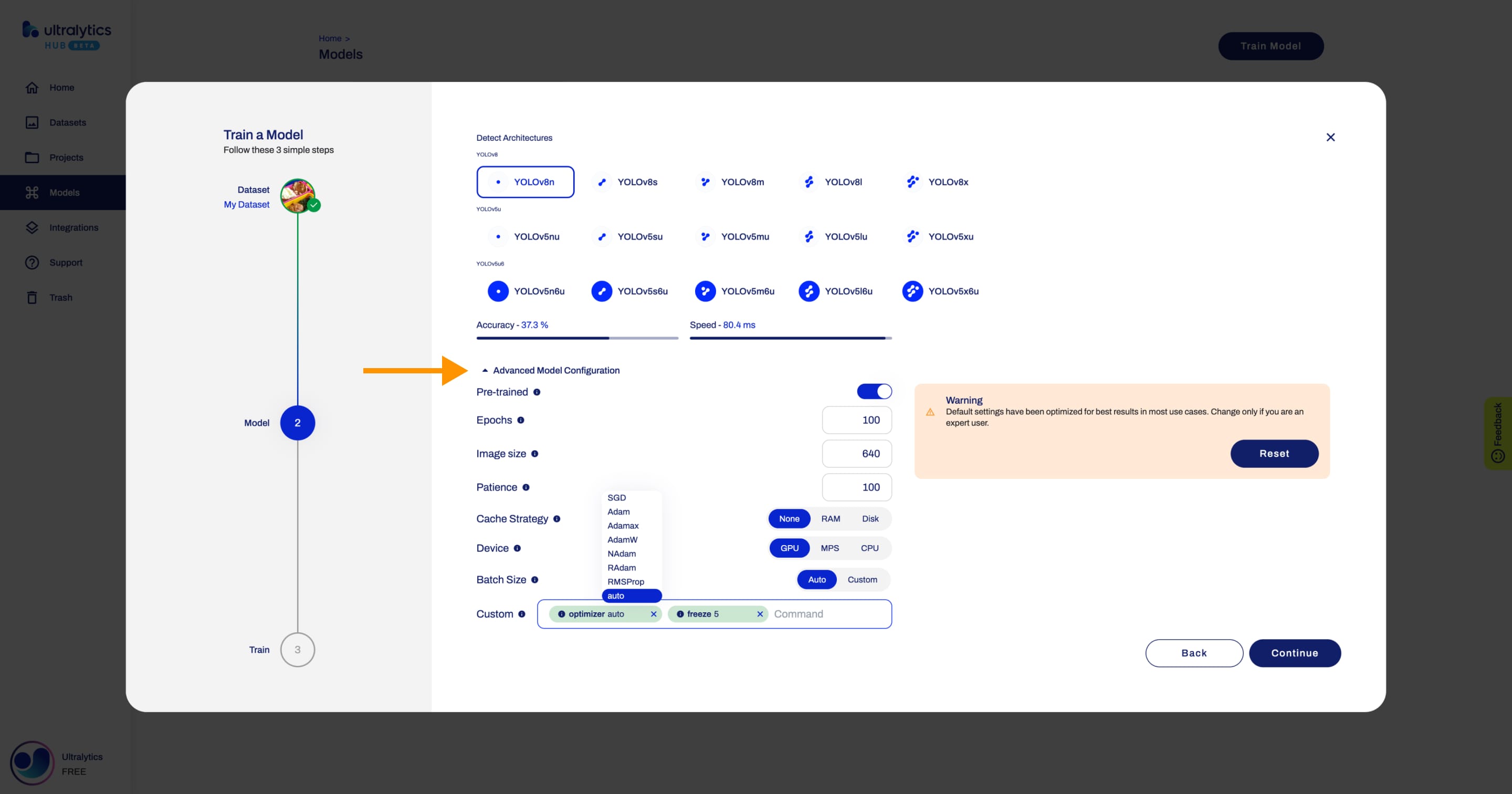
+
+### 2. Model
+
+In this step, you have to choose the project in which you want to create your model, the name of your model and your model's architecture.
+
+??? note "Note"
+
+ Ultralytics HUB will try to pre-select the project.
+
+ If you opened the **Train Model** dialog as described above, Ultralytics HUB will pre-select the last project you used.
+
+ If you opened the **Train Model** dialog from the Project page, Ultralytics HUB will pre-select the project you were inside of.
+
+ 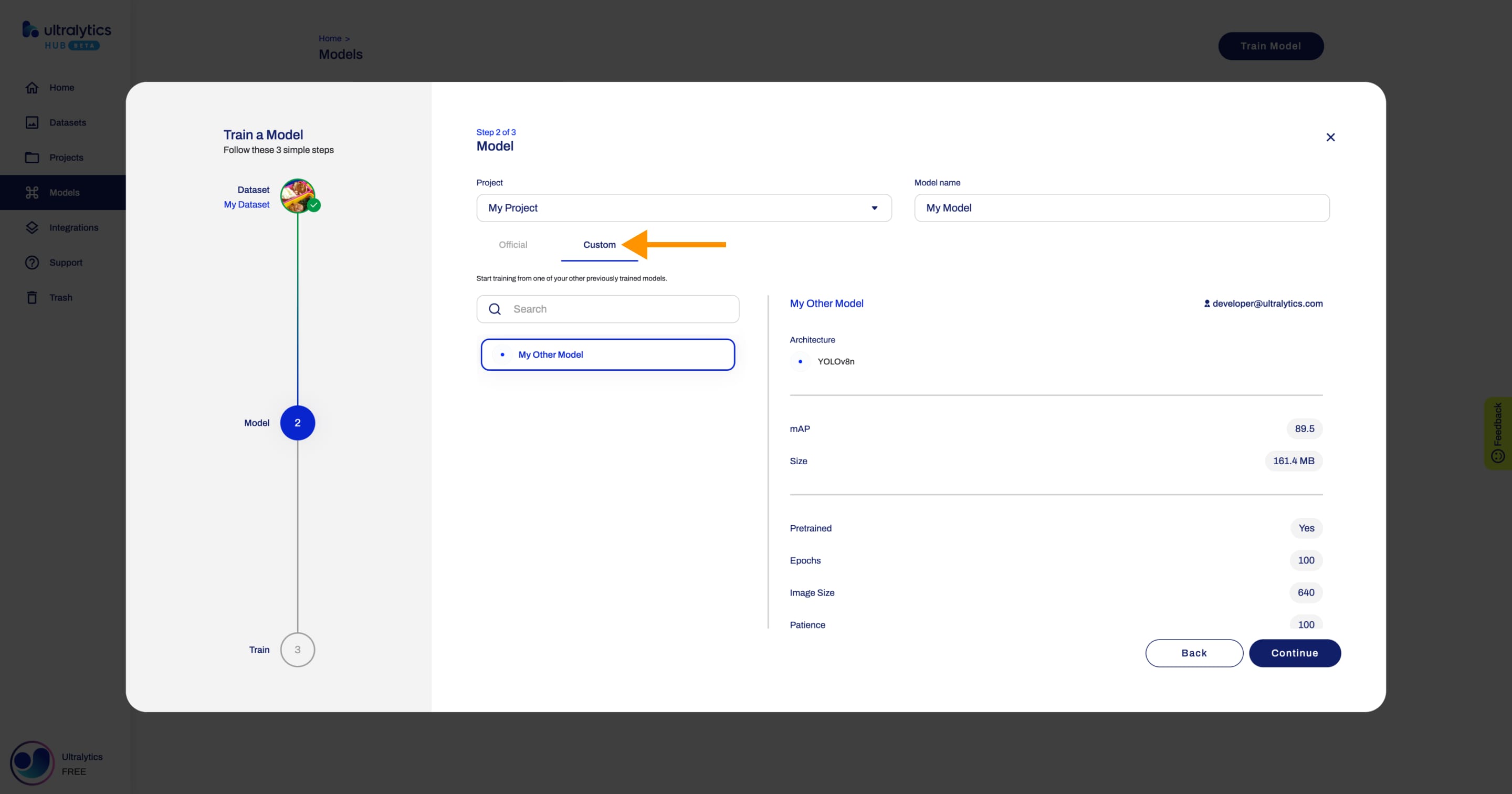
+
+ In case you don't have a project created yet, you can set the name of your project in this step and it will be created together with your model.
+
+ 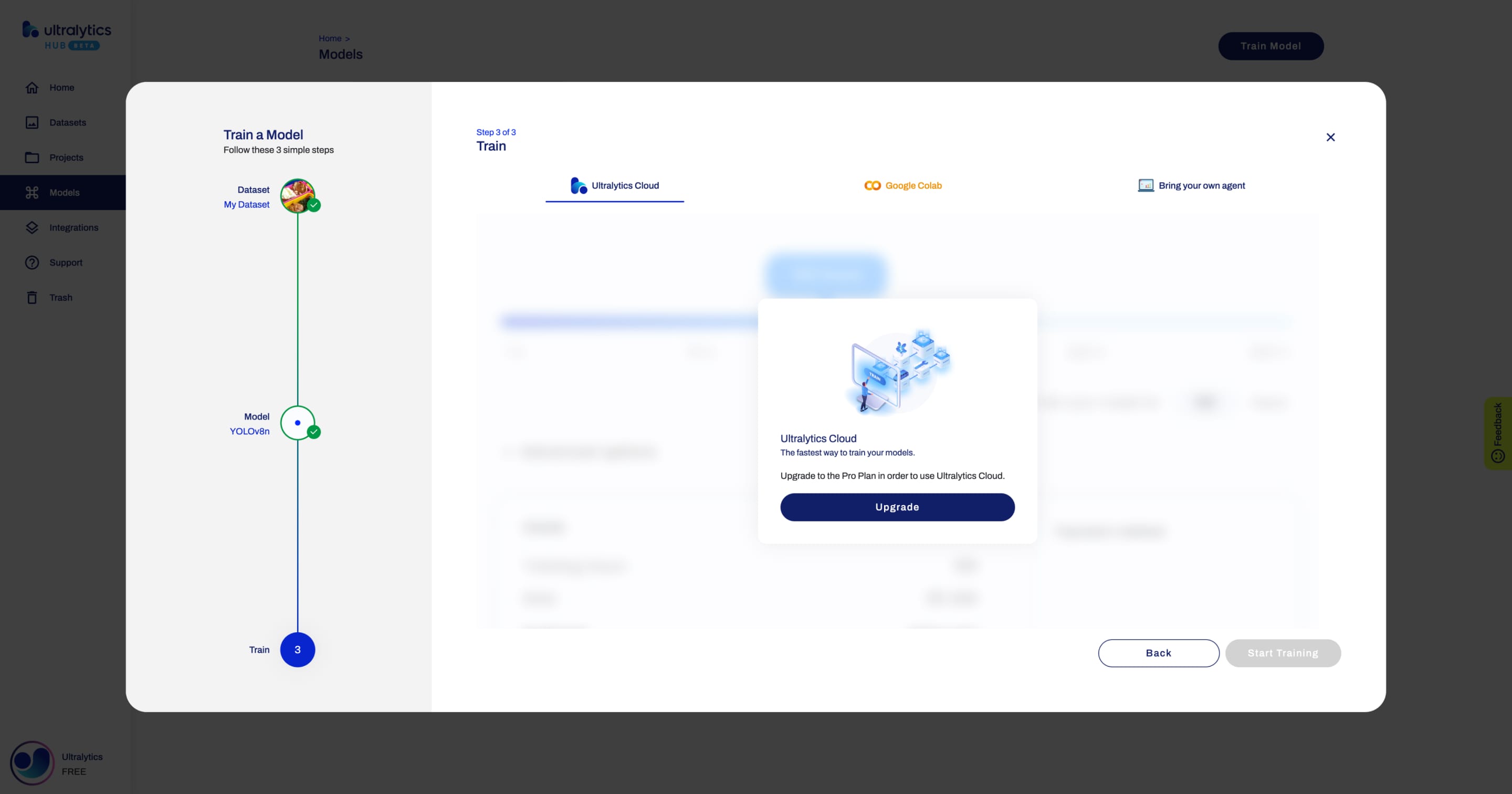
+
+!!! info "Info"
+
+ You can read more about the available [YOLOv8](https://docs.ultralytics.com/models/yolov8) (and [YOLOv5](https://docs.ultralytics.com/models/yolov5)) architectures in our documentation.
+
+When you're happy with your model configuration, click **Continue**.
+
+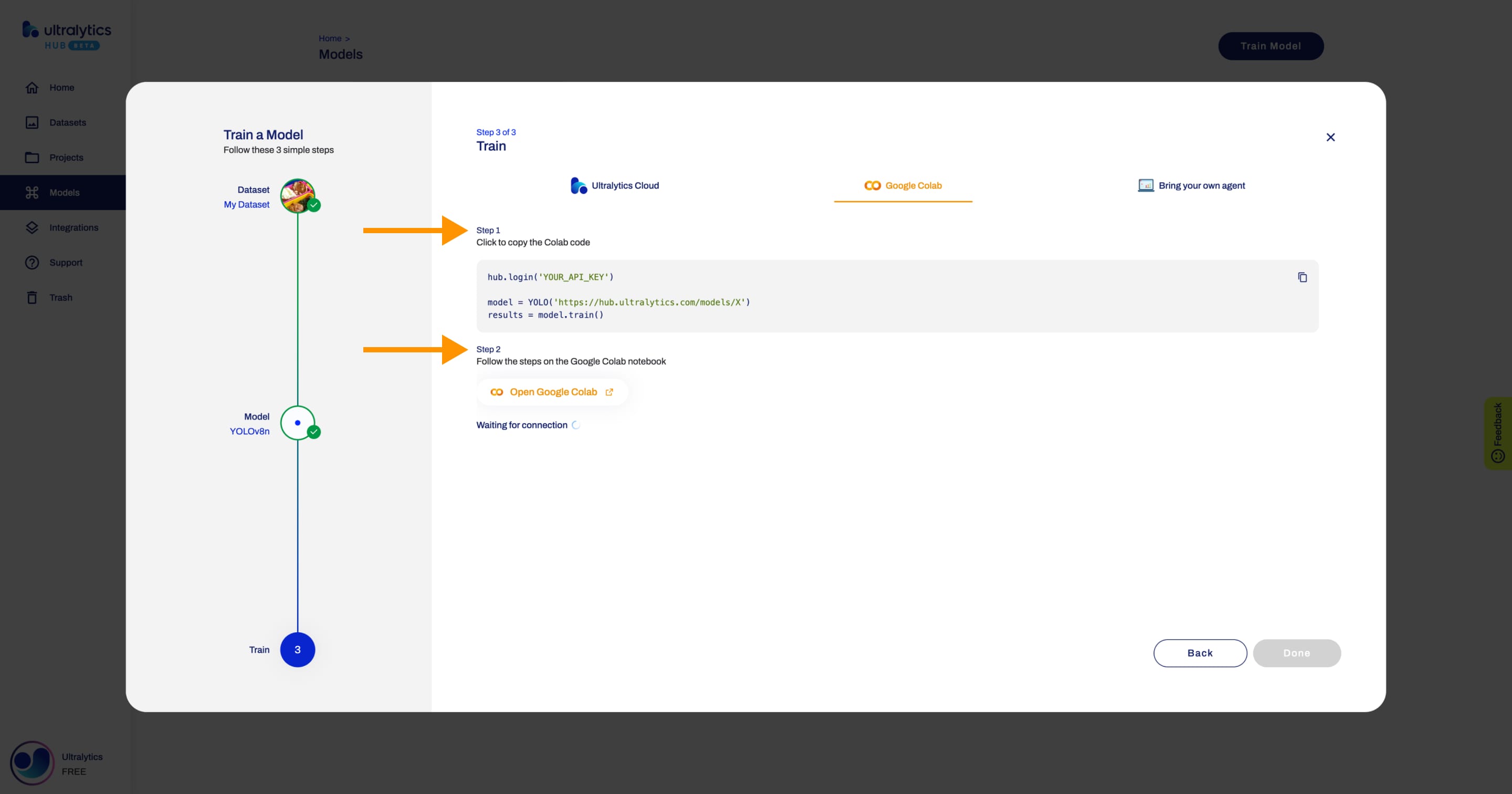
+
+??? note "Note"
+
+ By default, your model will use a pre-trained model (trained on the [COCO](https://docs.ultralytics.com/datasets/detect/coco) dataset) to reduce training time.
+
+ You can change this behaviour by opening the **Advanced Options** accordion.
+
+### 3. Train
+
+In this step, you will start training you model.
+
+Ultralytics HUB offers three training options:
+
+- Ultralytics Cloud **(COMING SOON)**
+- Google Colab
+- Bring your own agent
+
+In order to start training your model, follow the instructions presented in this step.
+
+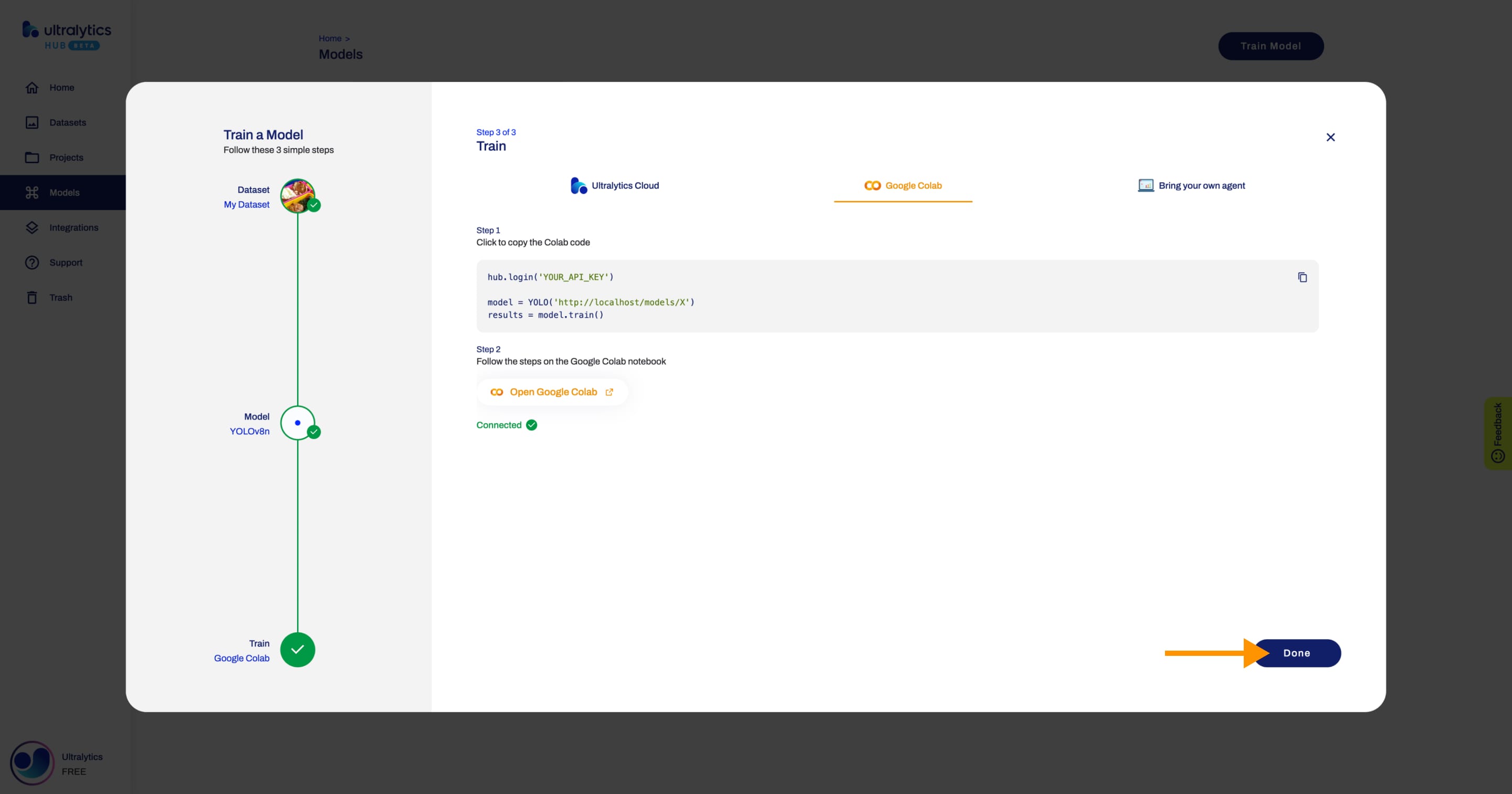
+
+??? note "Note"
+
+ When you are on this step, before the training starts, you can change the default training configuration by opening the **Advanced Options** accordion.
+
+ 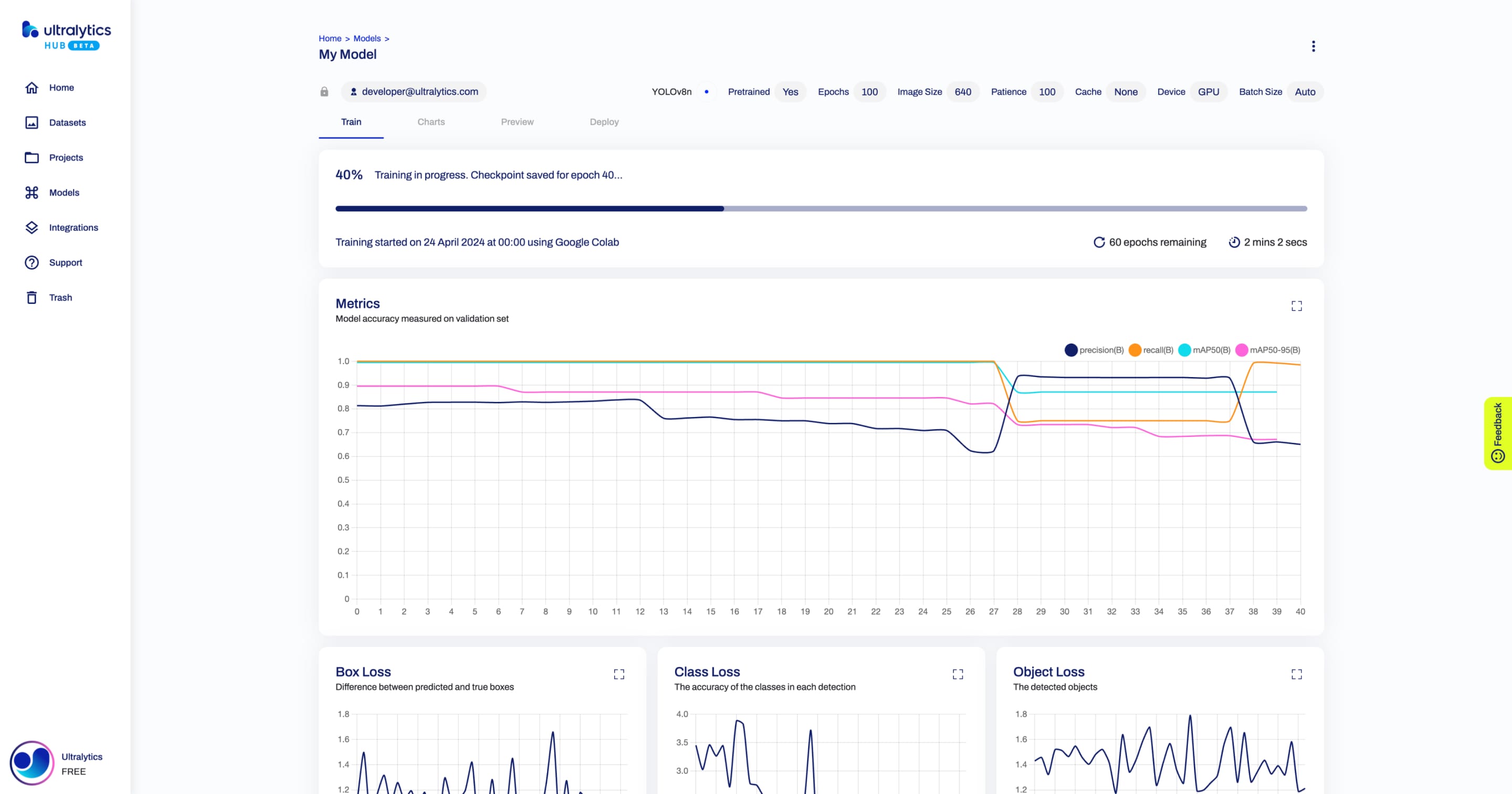
+
+??? note "Note"
+
+ When you are on this step, you have the option to close the **Train Model** dialog and start training your model from the Model page later.
+
+ 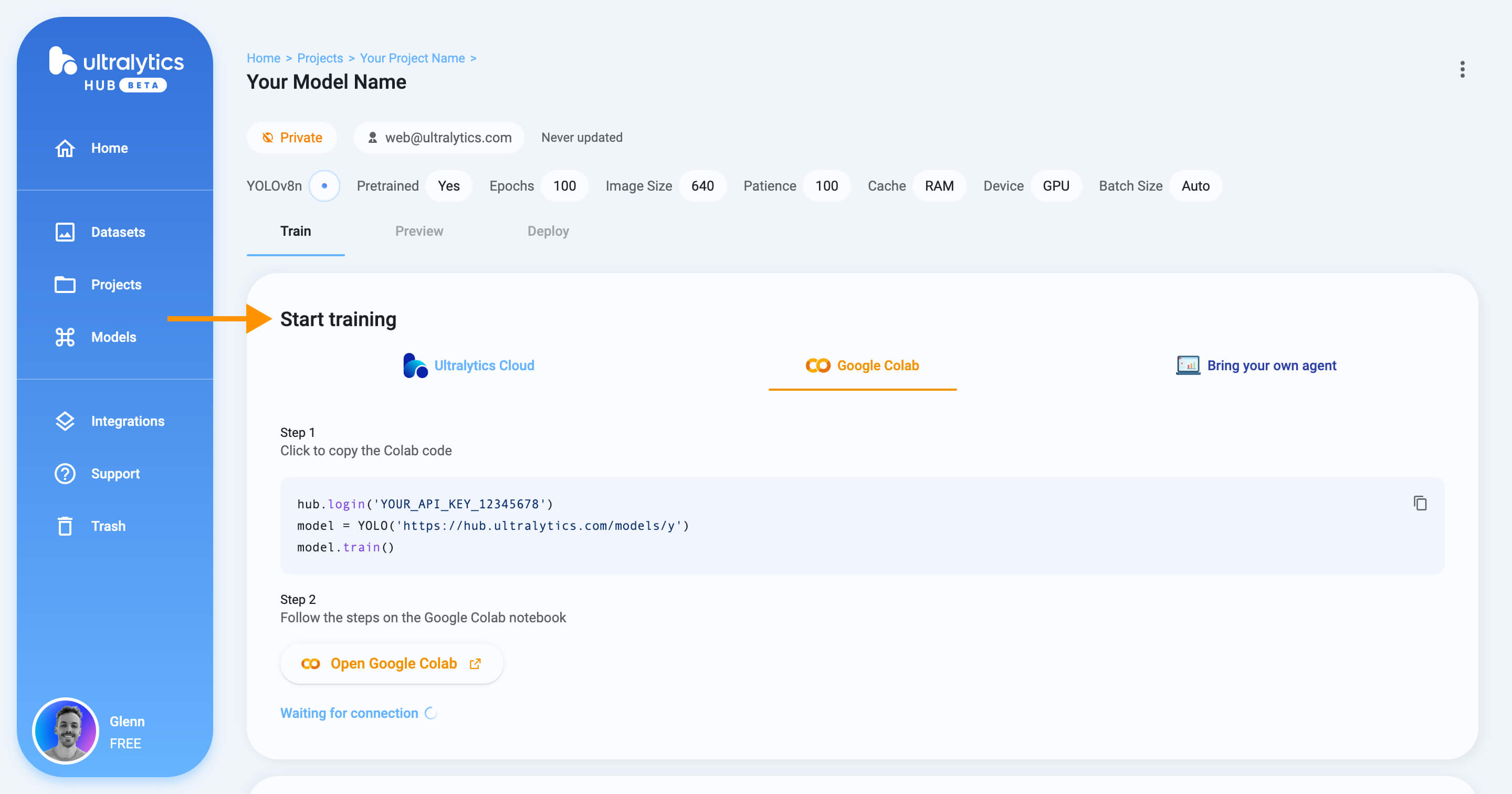
+
+To start training your model using Google Colab, simply follow the instructions shown above or on the Google Colab notebook.
+
+
+
+
+
+When the training starts, you can click **Done** and monitor the training progress on the Model page.
+
+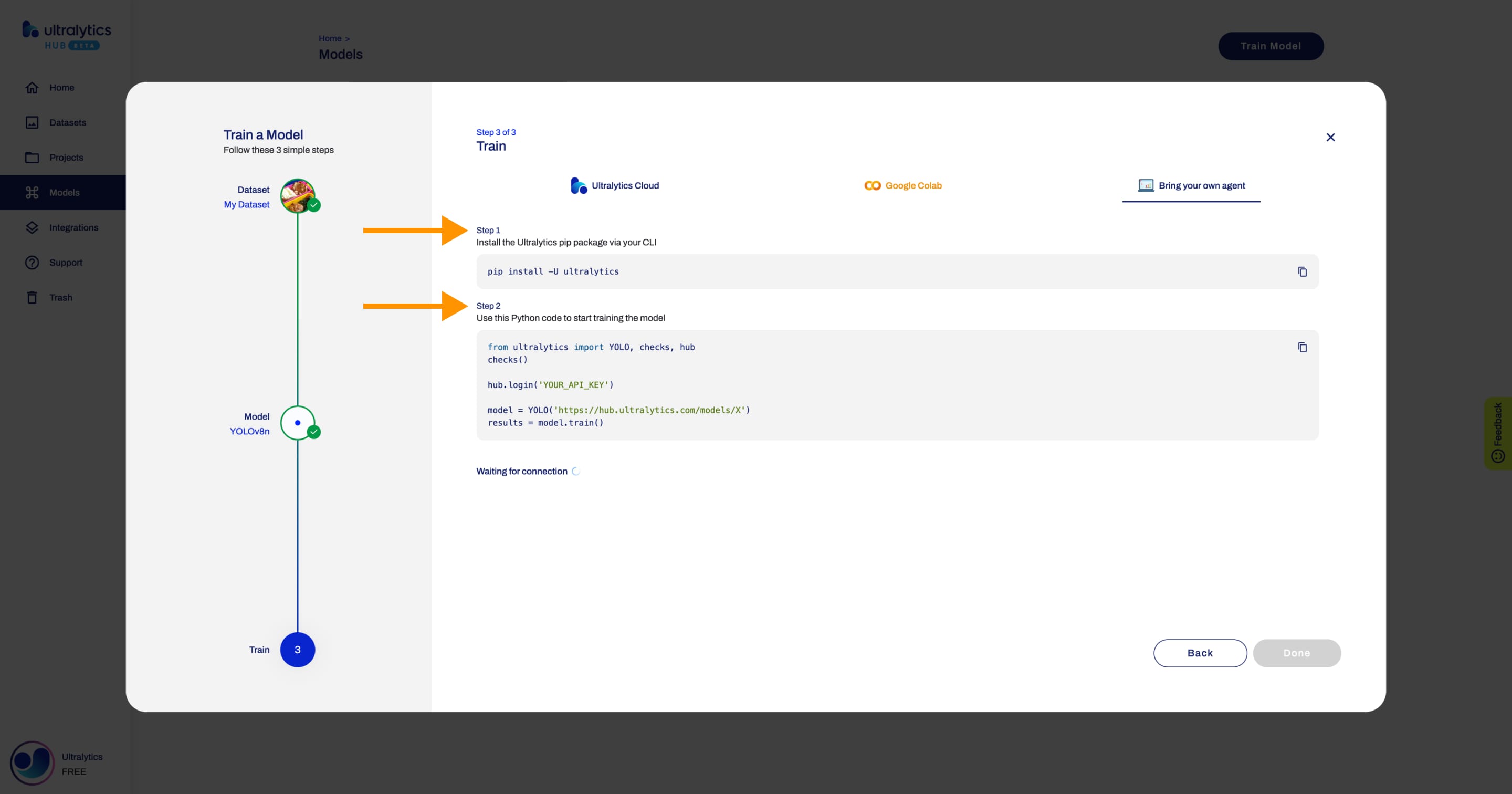
+
+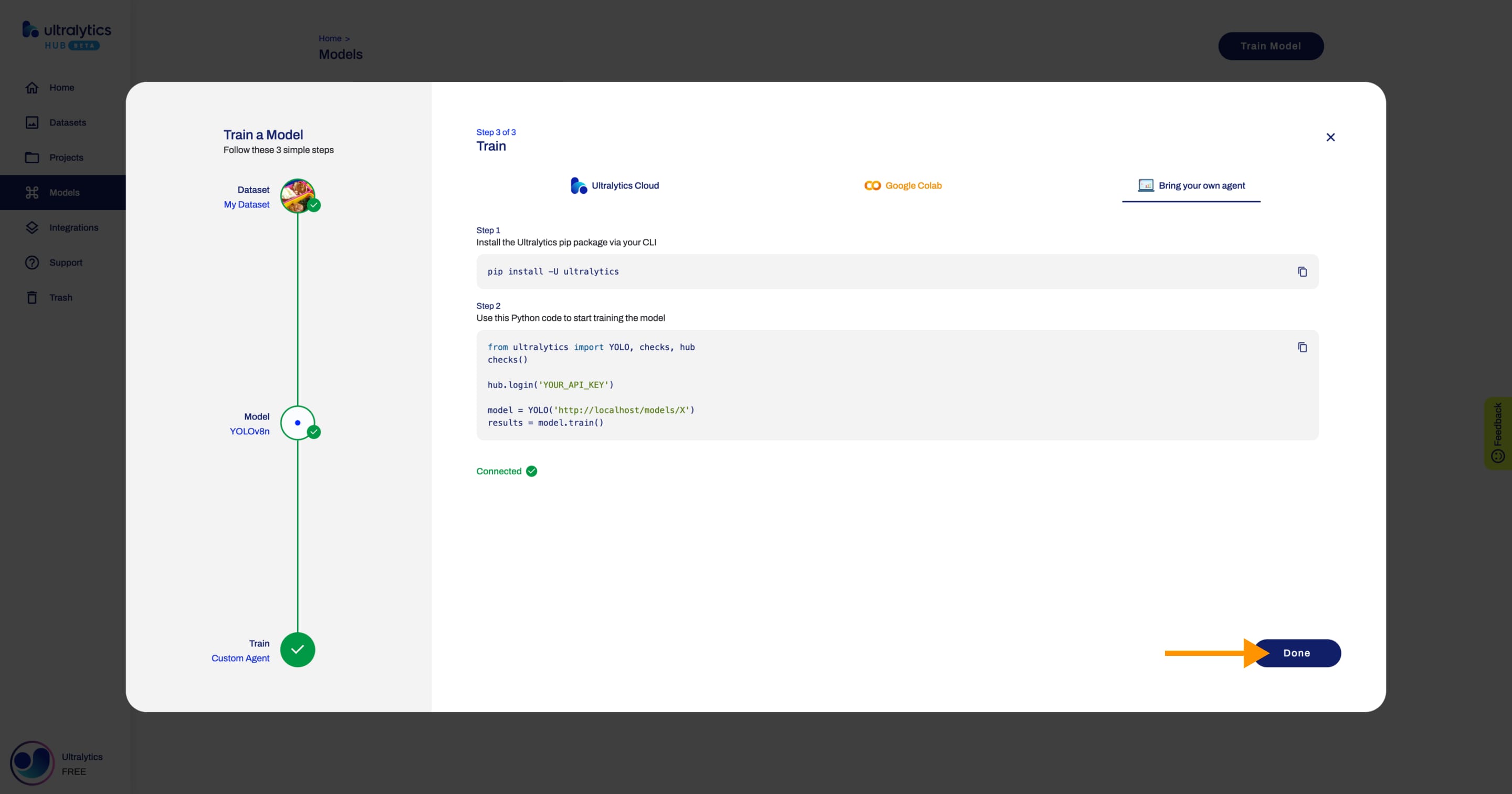
+
+??? note "Note"
+
+ In case the training stops and a checkpoint was saved, you can resume training your model from the Model page.
+
+ 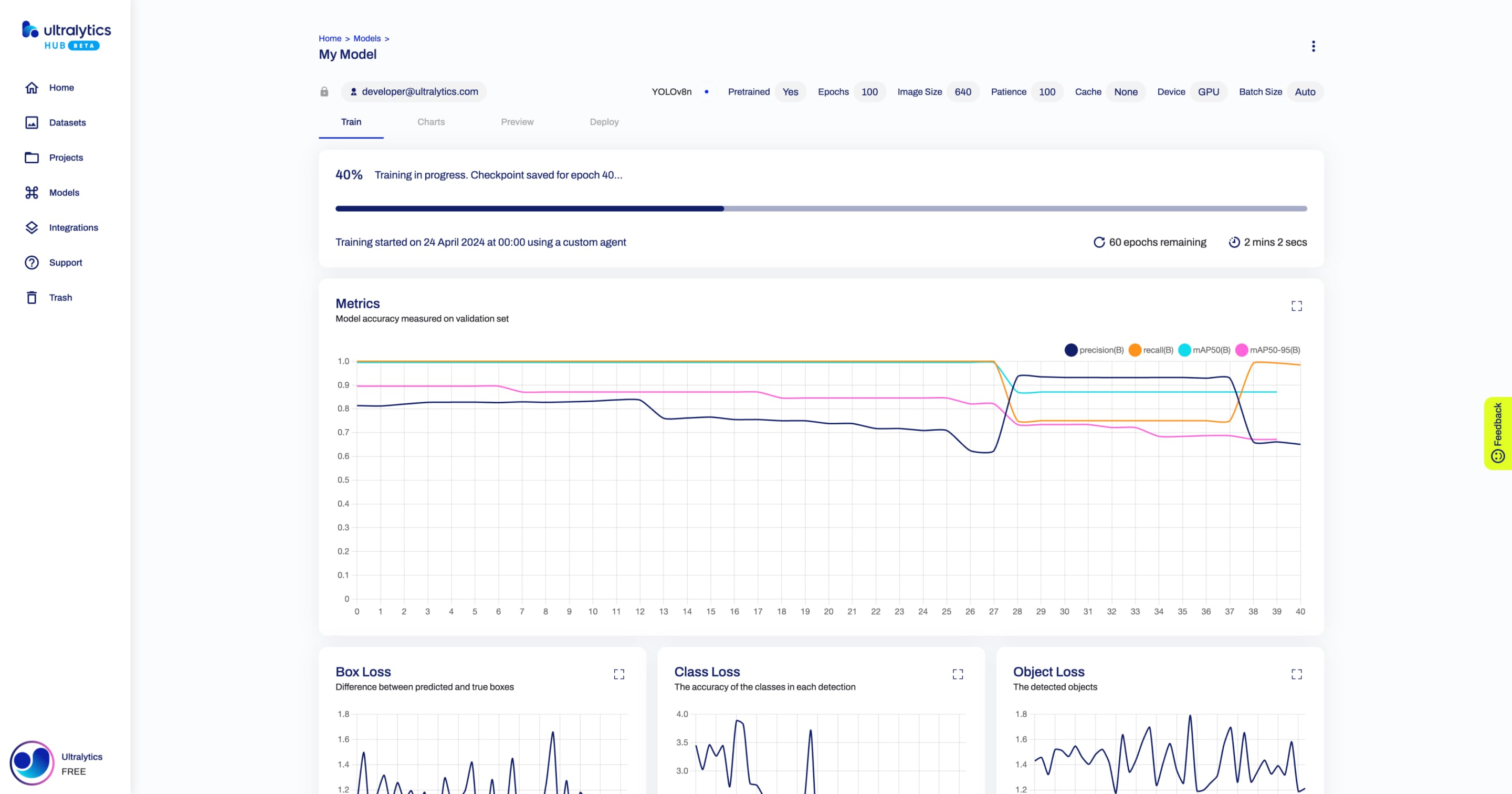
+
+## Preview Model
+
+Ultralytics HUB offers a variety of ways to preview your trained model.
+
+You can preview your model if you click on the **Preview** tab and upload an image in the **Test** card.
+
+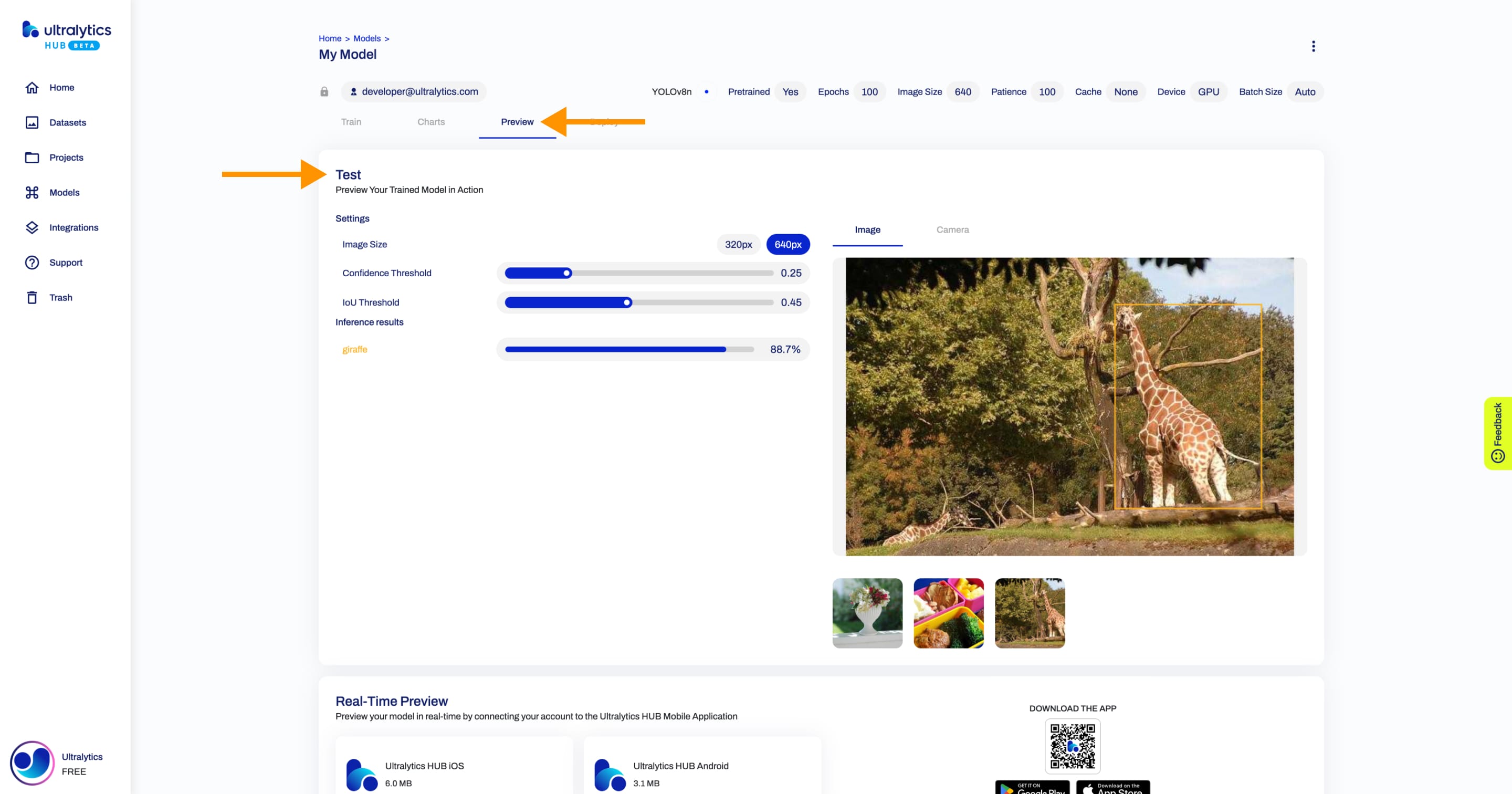
+
+You can also use our Ultralytics Cloud API to effortlessly [run inference](https://docs.ultralytics.com/hub/inference_api) with your custom model.
+
+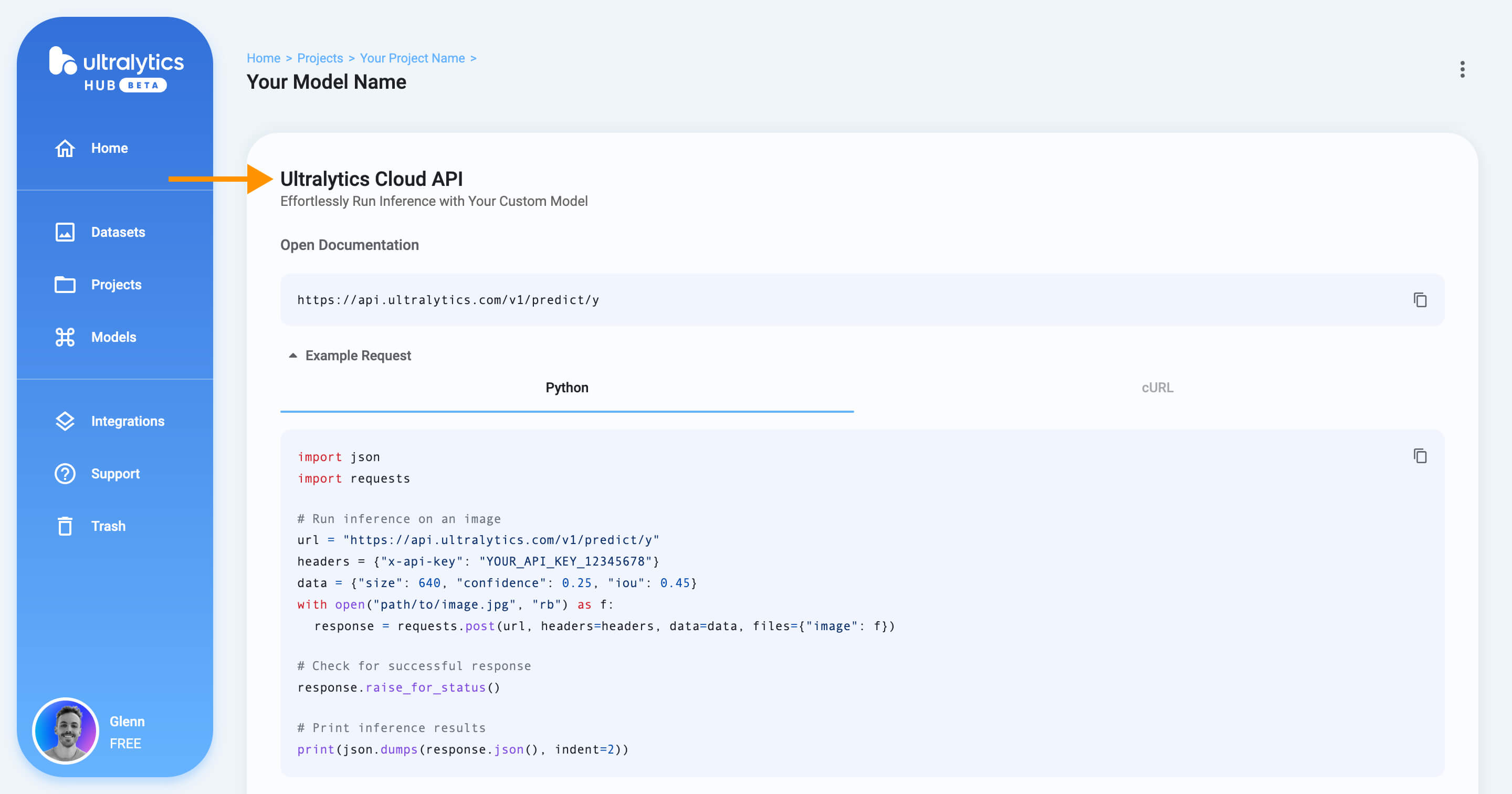
+
+Furthermore, you can preview your model in real-time directly on your [iOS](https://apps.apple.com/xk/app/ultralytics/id1583935240) or [Android](https://play.google.com/store/apps/details?id=com.ultralytics.ultralytics_app) mobile device by [downloading](https://ultralytics.com/app_install) our [Ultralytics HUB Mobile Application](./app/index.md).
+
+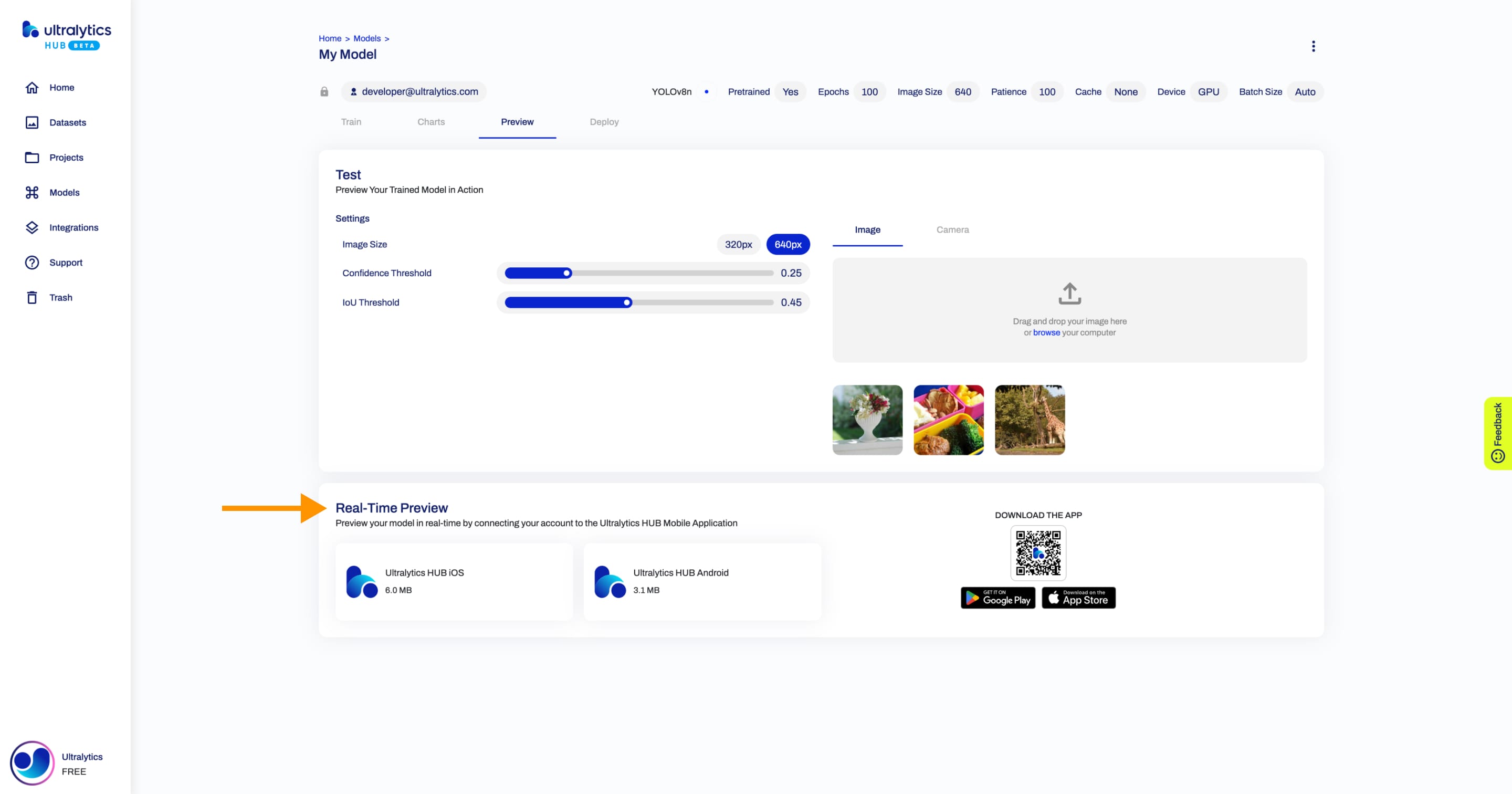
+
+## Deploy Model
+
+You can export your model to 13 different formats, including ONNX, OpenVINO, CoreML, TensorFlow, Paddle and many others.
+
+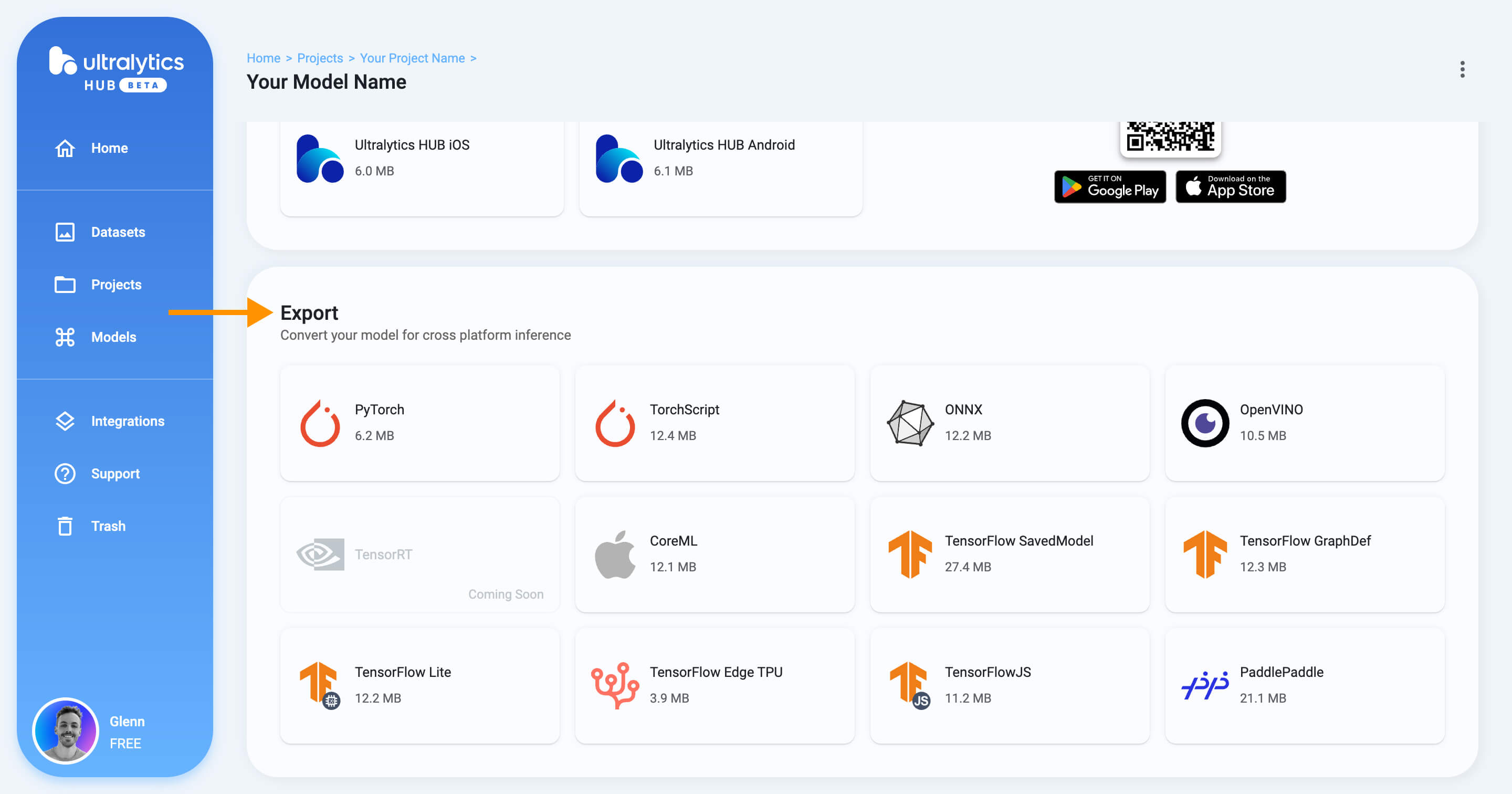
+
+??? tip "Tip"
+
+ You can customize the export options of each format if you open the export actions dropdown and click on the **Advanced** option.
+
+ 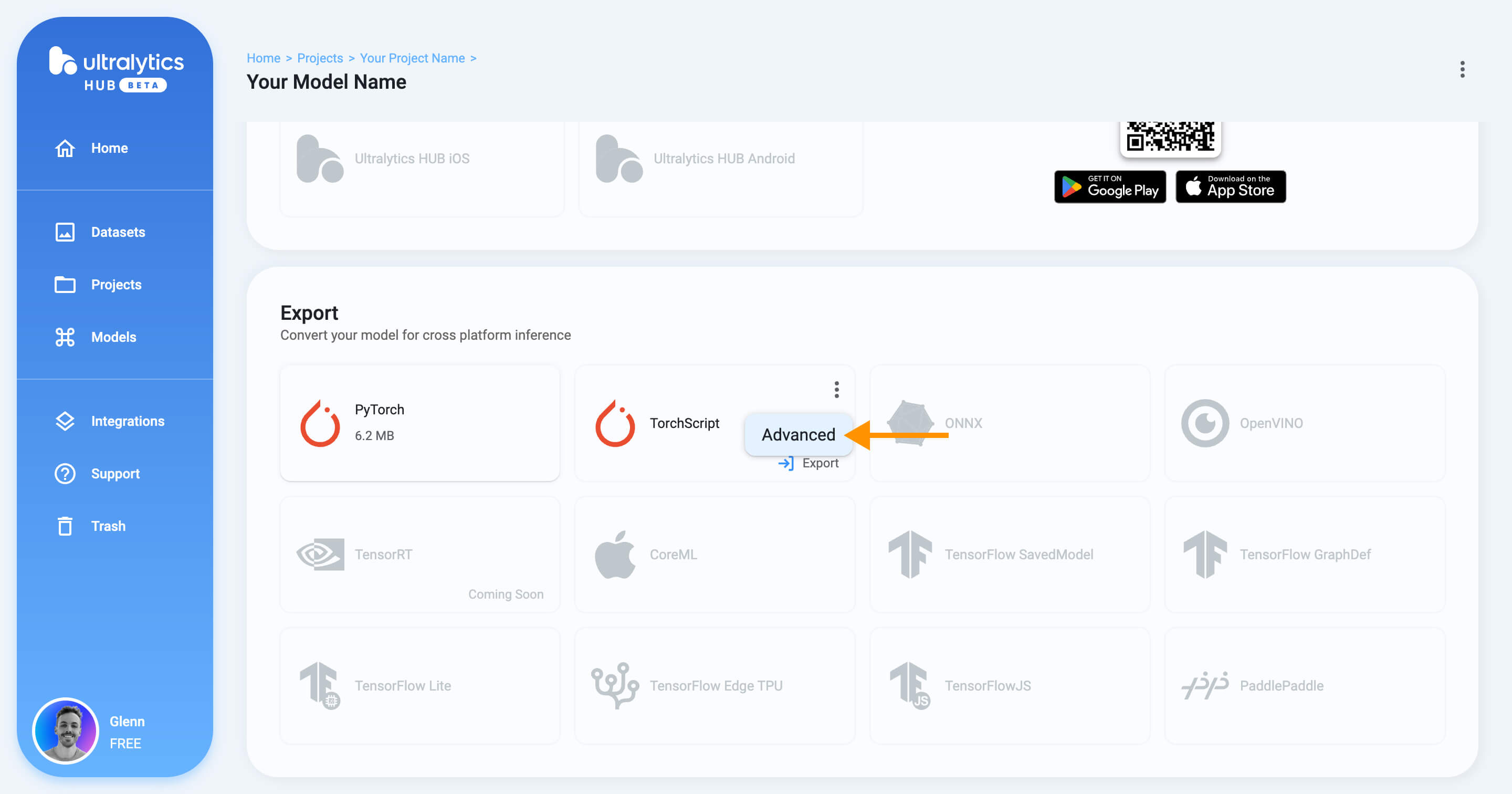
+
+## Share Model
+
+!!! info "Info"
+
+ Ultralytics HUB's sharing functionality provides a convenient way to share models with others. This feature is designed to accommodate both existing Ultralytics HUB users and those who have yet to create an account.
+
+??? note "Note"
+
+ You have control over the general access of your models.
+
+ You can choose to set the general access to "Private", in which case, only you will have access to it. Alternatively, you can set the general access to "Unlisted" which grants viewing access to anyone who has the direct link to the model, regardless of whether they have an Ultralytics HUB account or not.
+
+Navigate to the Model page of the model you want to share, open the model actions dropdown and click on the **Share** option. This action will trigger the **Share Model** dialog.
+
+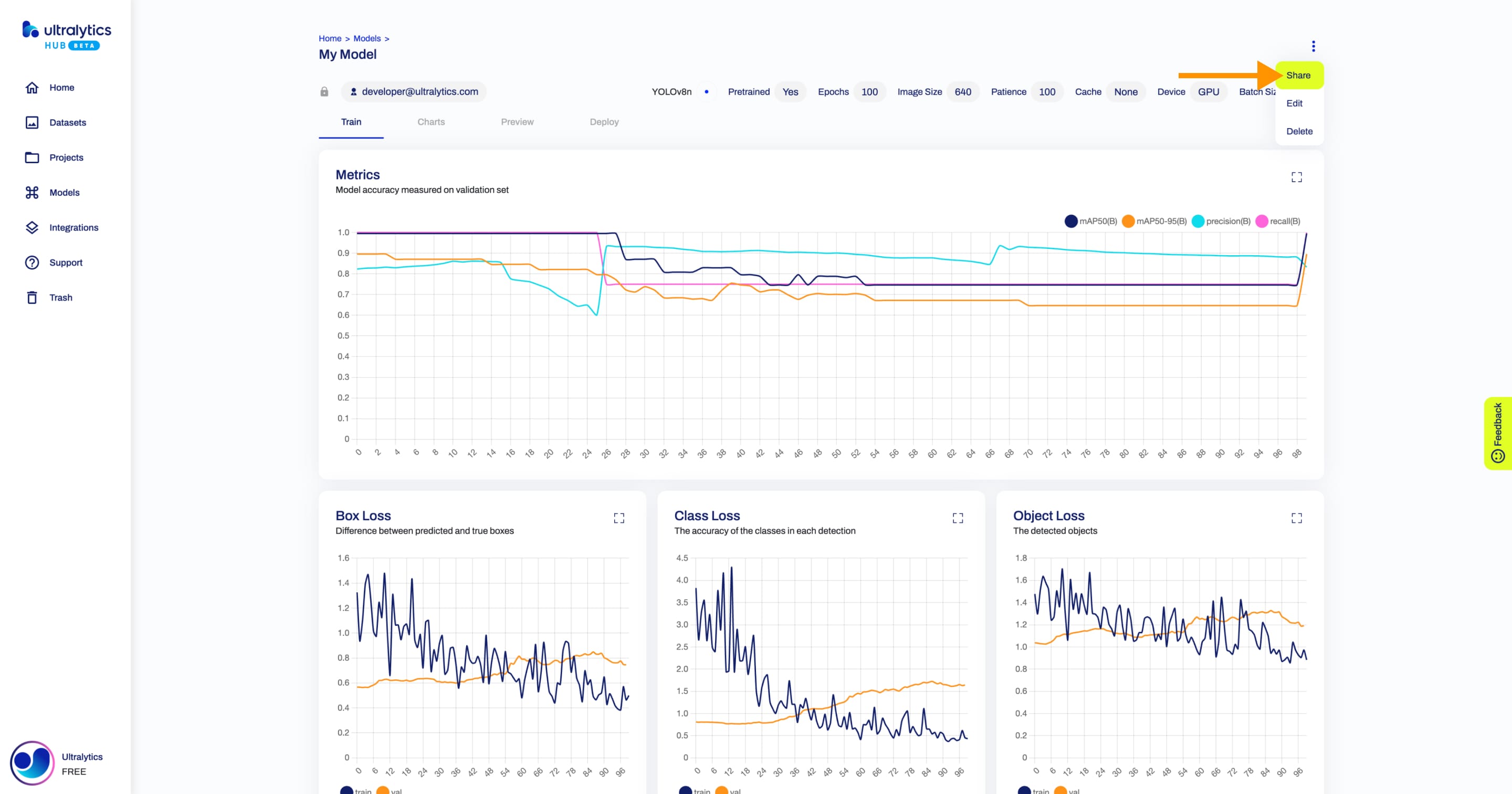
+
+??? tip "Tip"
+
+ You can also share a model directly from the [Models](https://hub.ultralytics.com/models) page or from the Project page of the project where your model is located.
+
+ 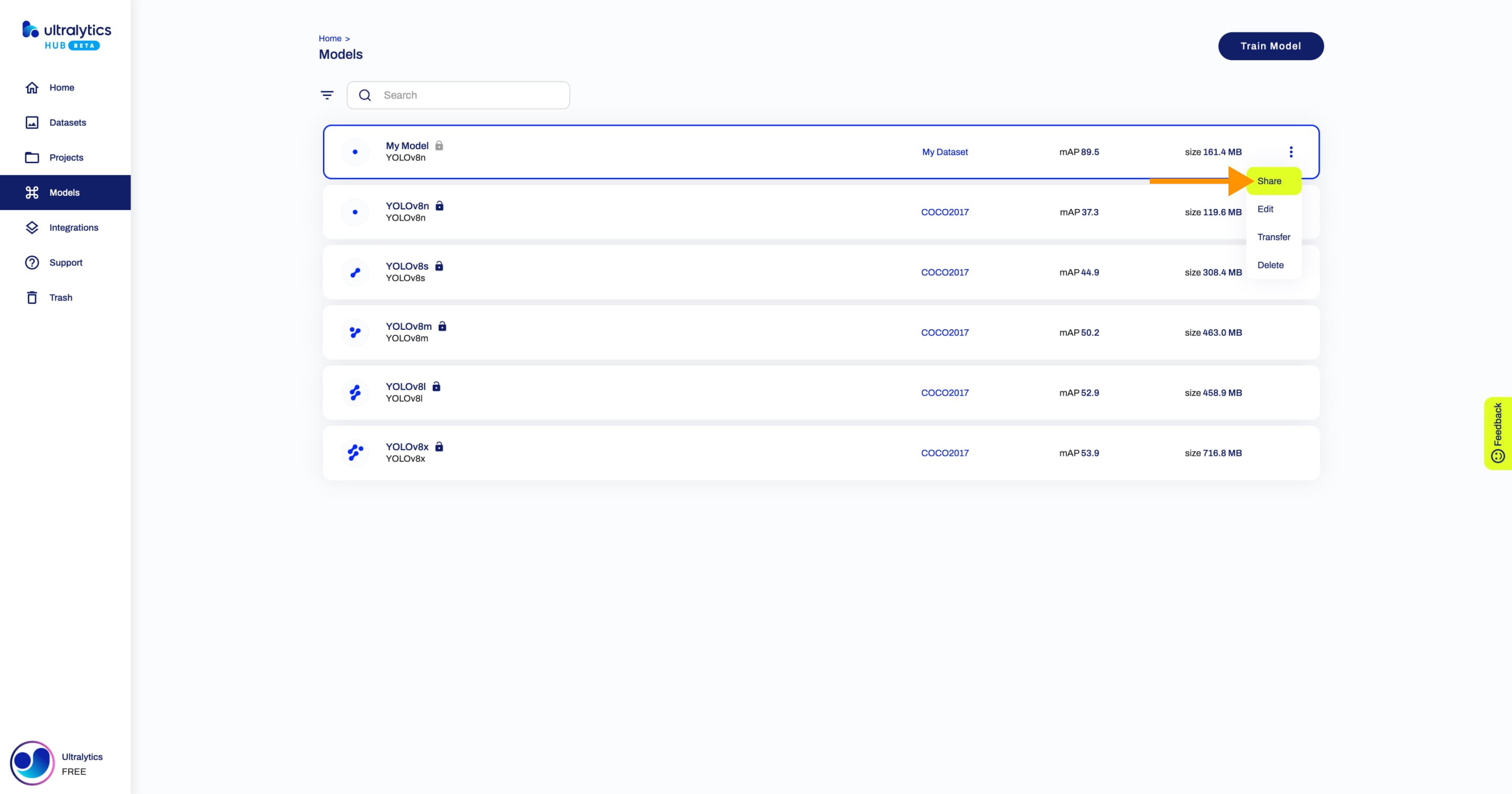
+
+Set the general access to "Unlisted" and click **Save**.
+
+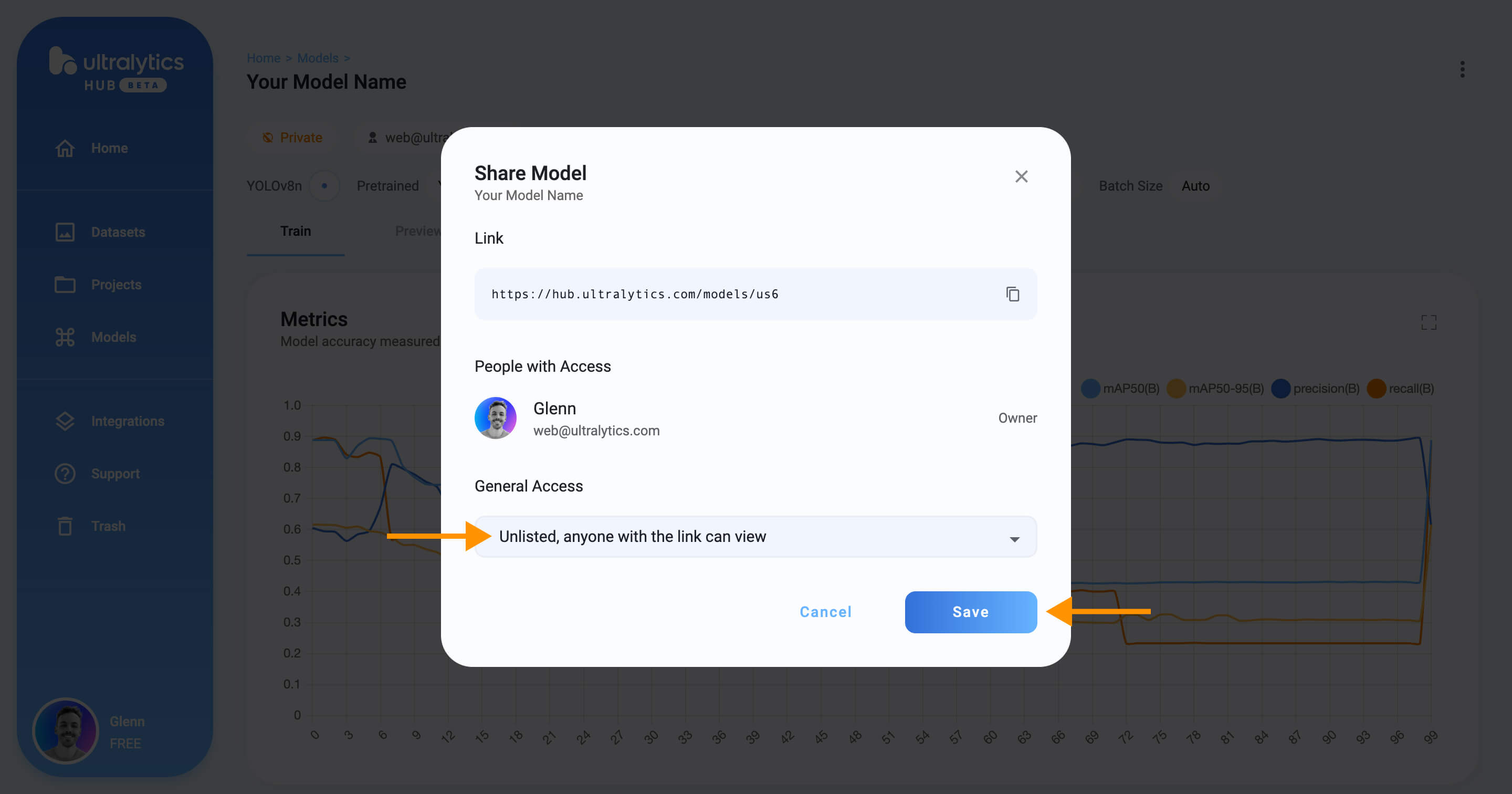
+
+Now, anyone who has the direct link to your model can view it.
+
+??? tip "Tip"
+
+ You can easily click on the models's link shown in the **Share Model** dialog to copy it.
+
+ 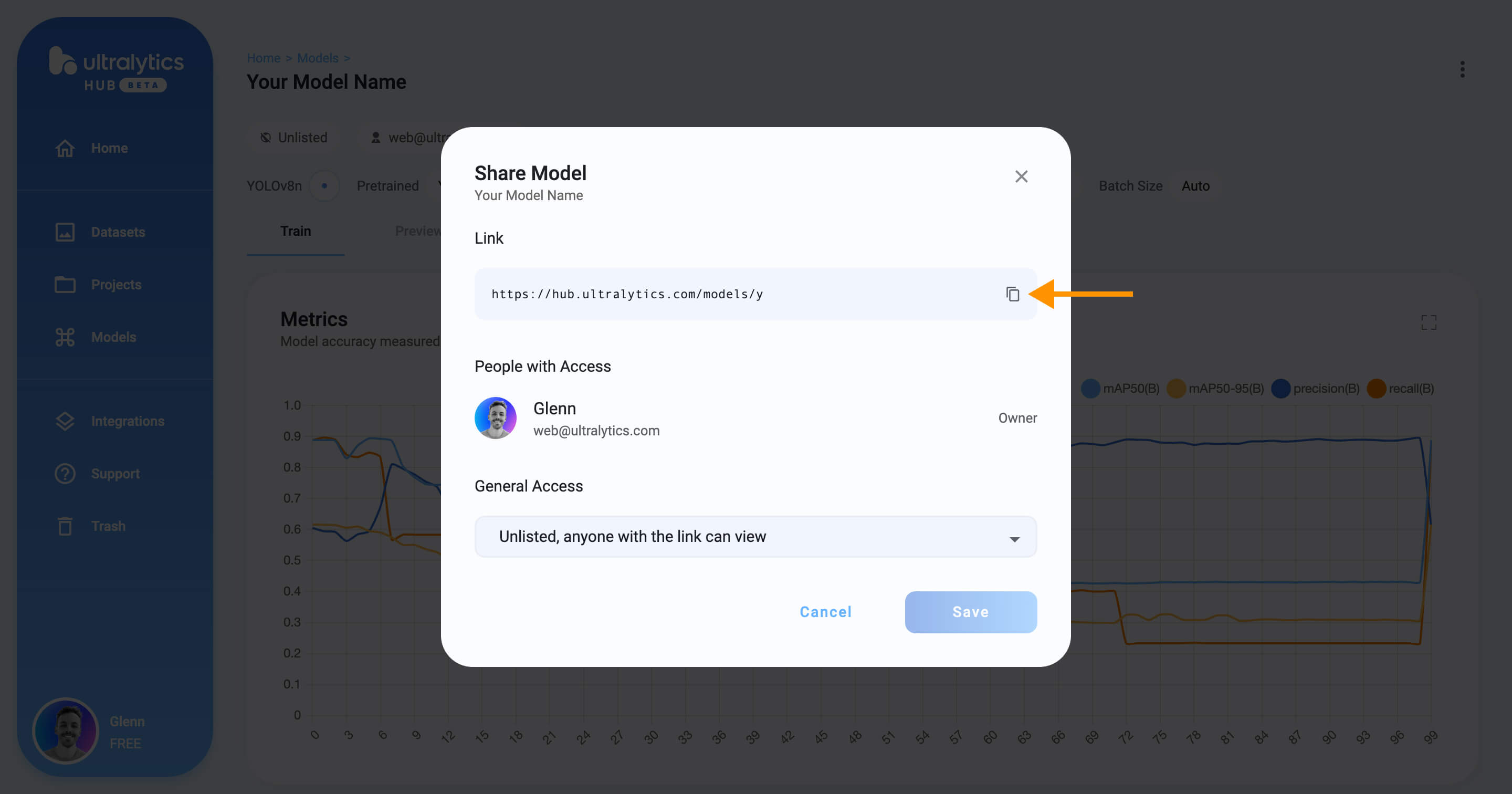
+
+## Edit Model
+
+Navigate to the Model page of the model you want to edit, open the model actions dropdown and click on the **Edit** option. This action will trigger the **Update Model** dialog.
+
+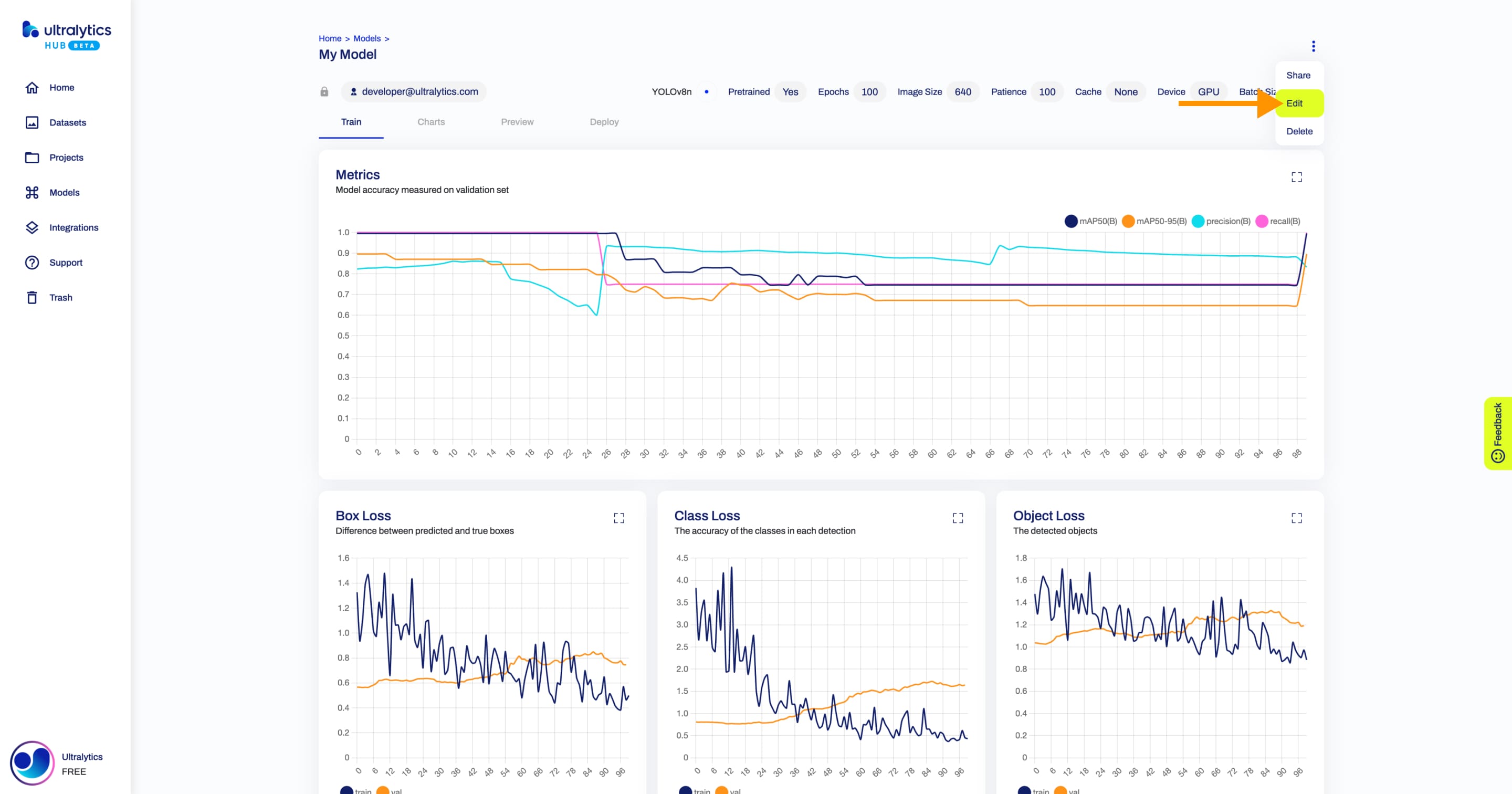
+
+??? tip "Tip"
+
+ You can also edit a model directly from the [Models](https://hub.ultralytics.com/models) page or from the Project page of the project where your model is located.
+
+ 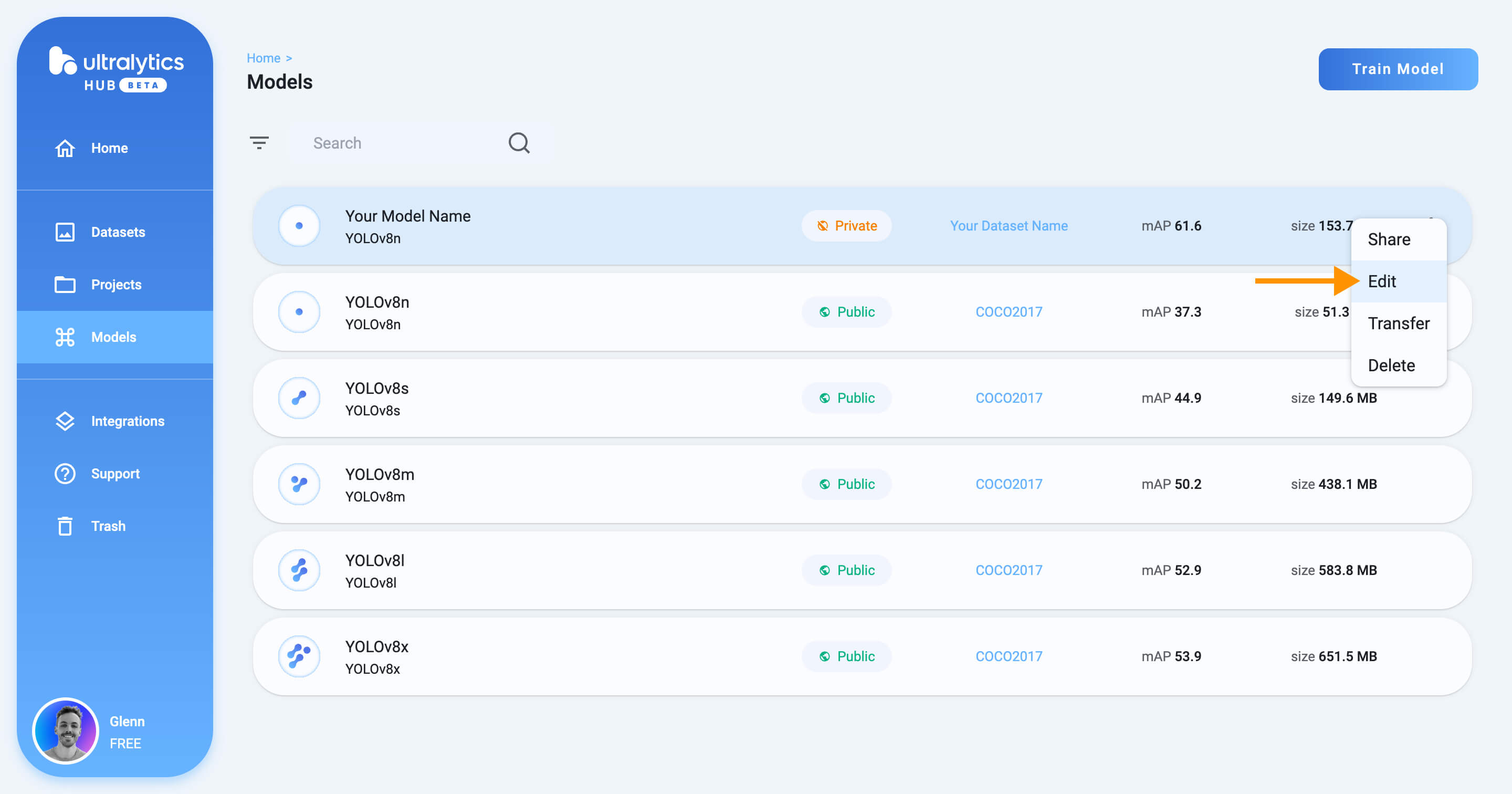
+
+Apply the desired modifications to your model and then confirm the changes by clicking **Save**.
+
+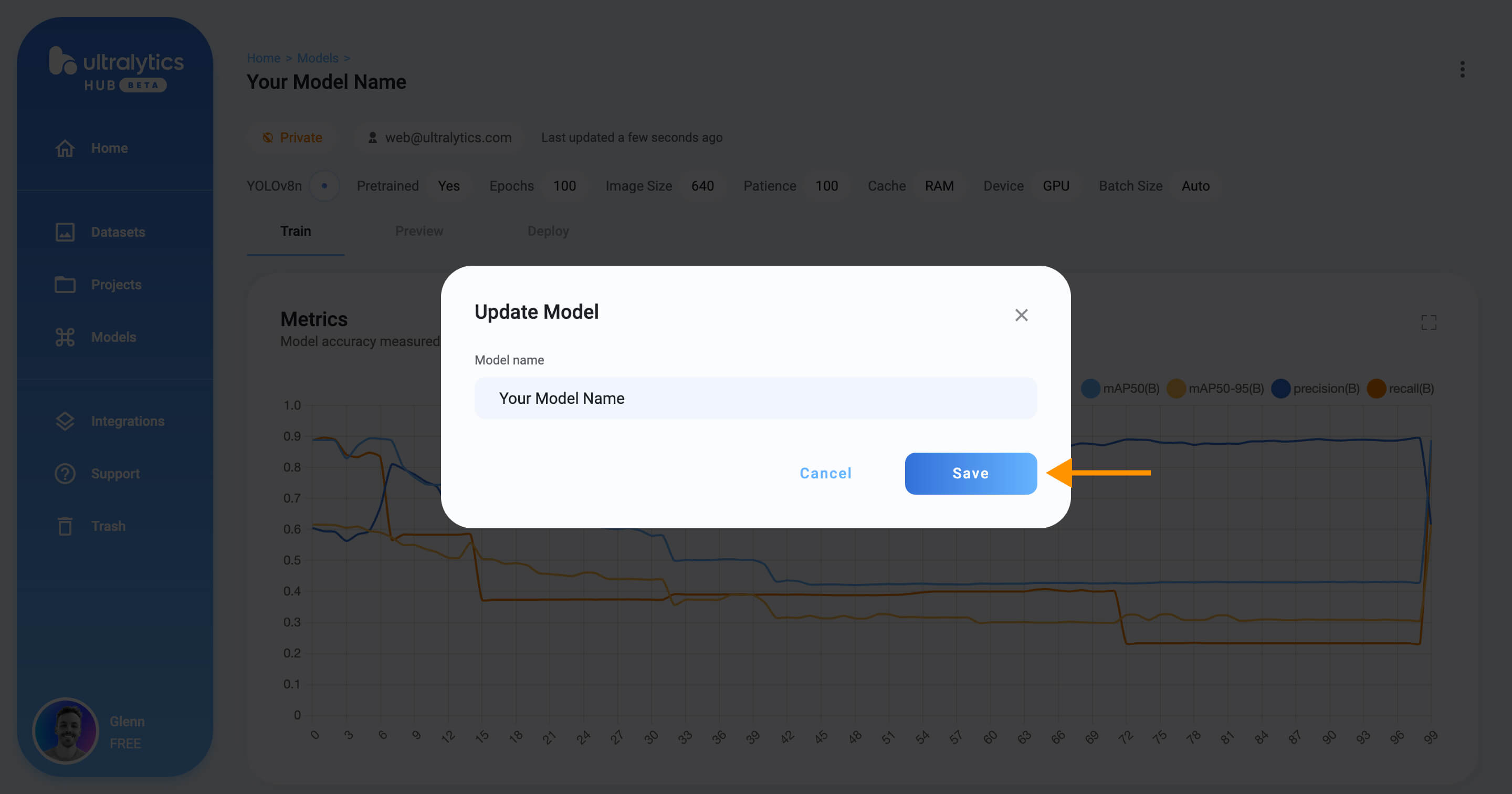
+
+## Delete Model
+
+Navigate to the Model page of the model you want to delete, open the model actions dropdown and click on the **Delete** option. This action will delete the model.
+
+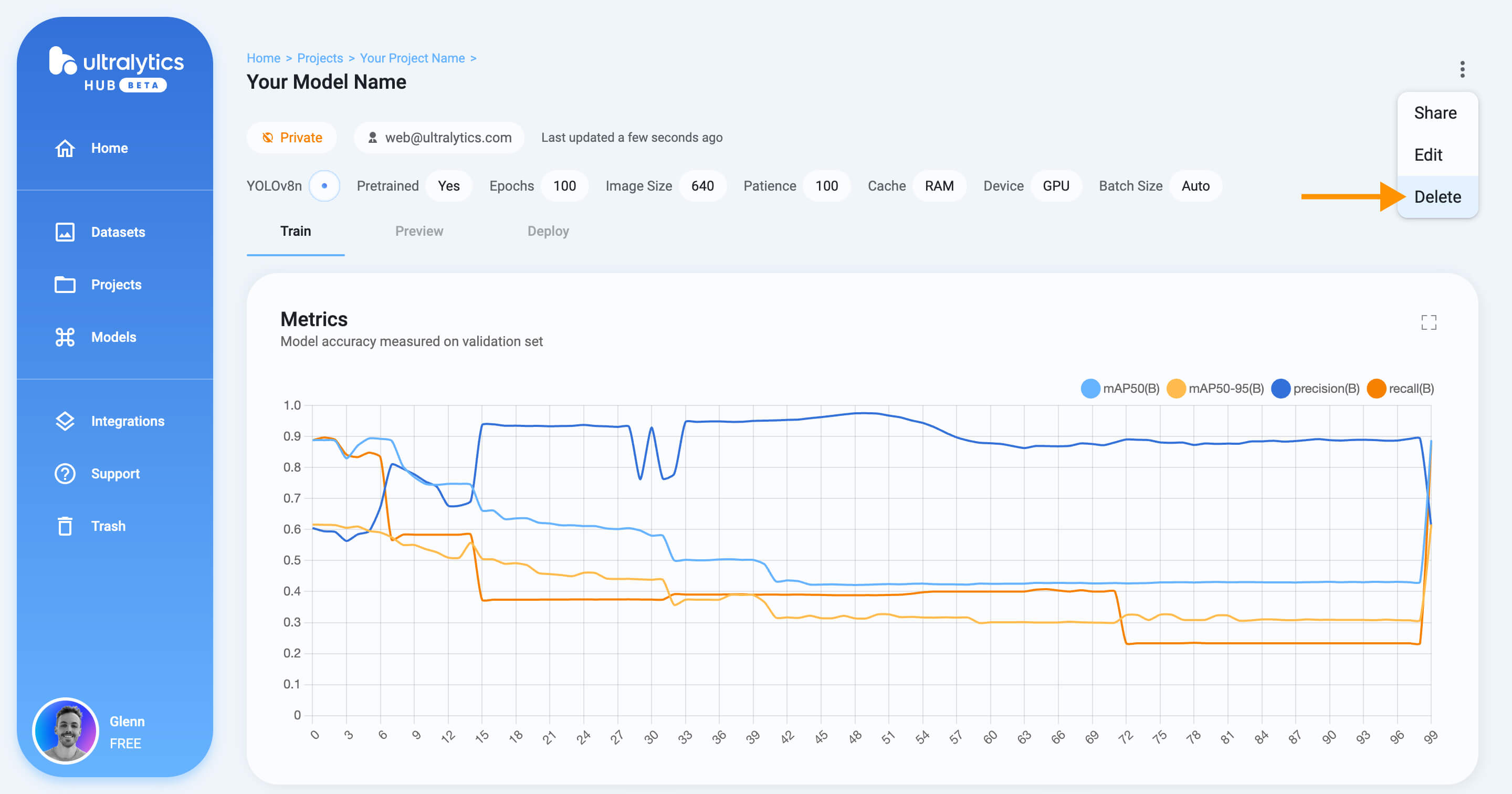
+
+??? tip "Tip"
+
+ You can also delete a model directly from the [Models](https://hub.ultralytics.com/models) page or from the Project page of the project where your model is located.
+
+ 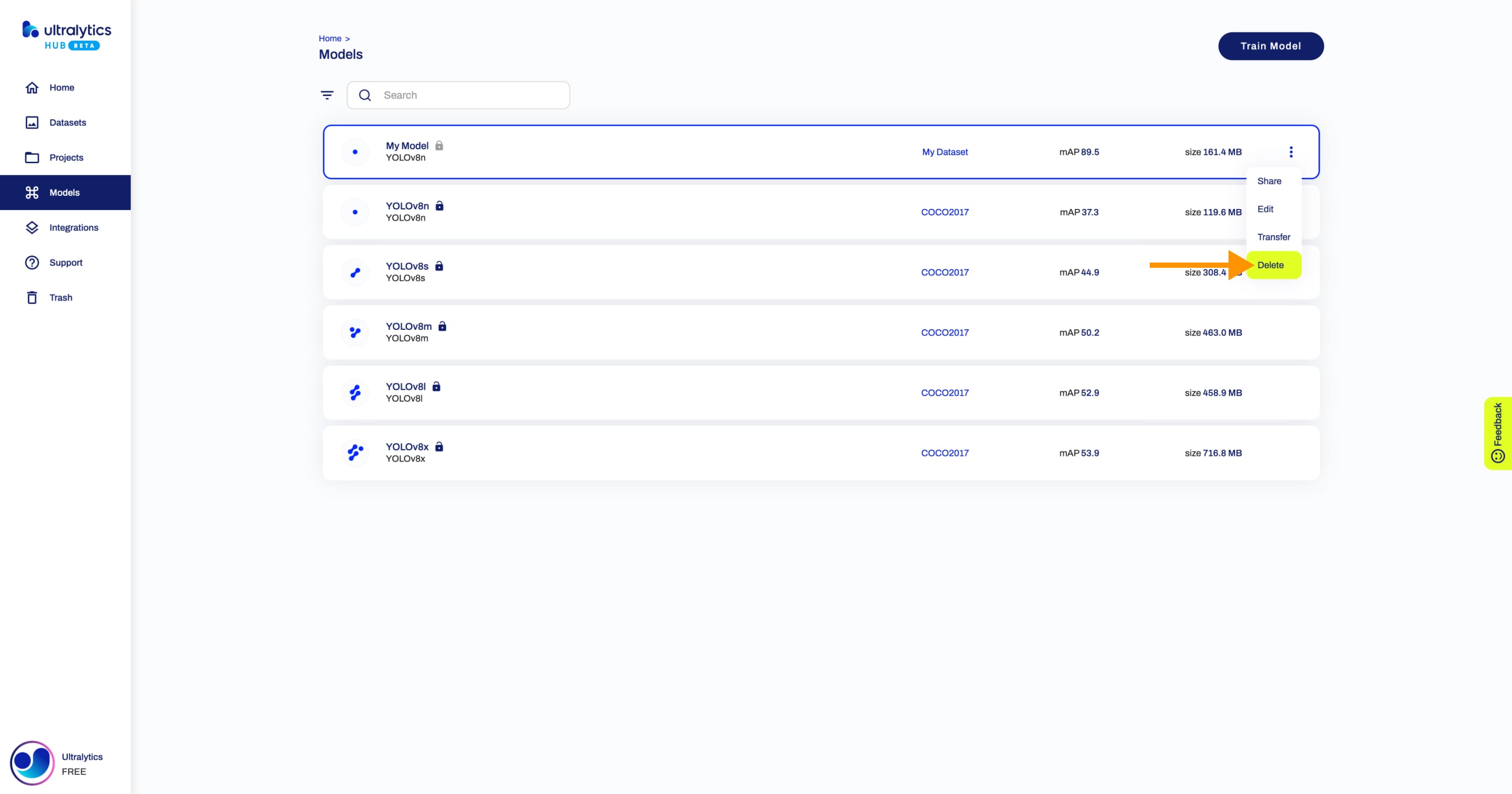
+
+??? note "Note"
+
+ If you change your mind, you can restore the model from the [Trash](https://hub.ultralytics.com/trash) page.
+
+ 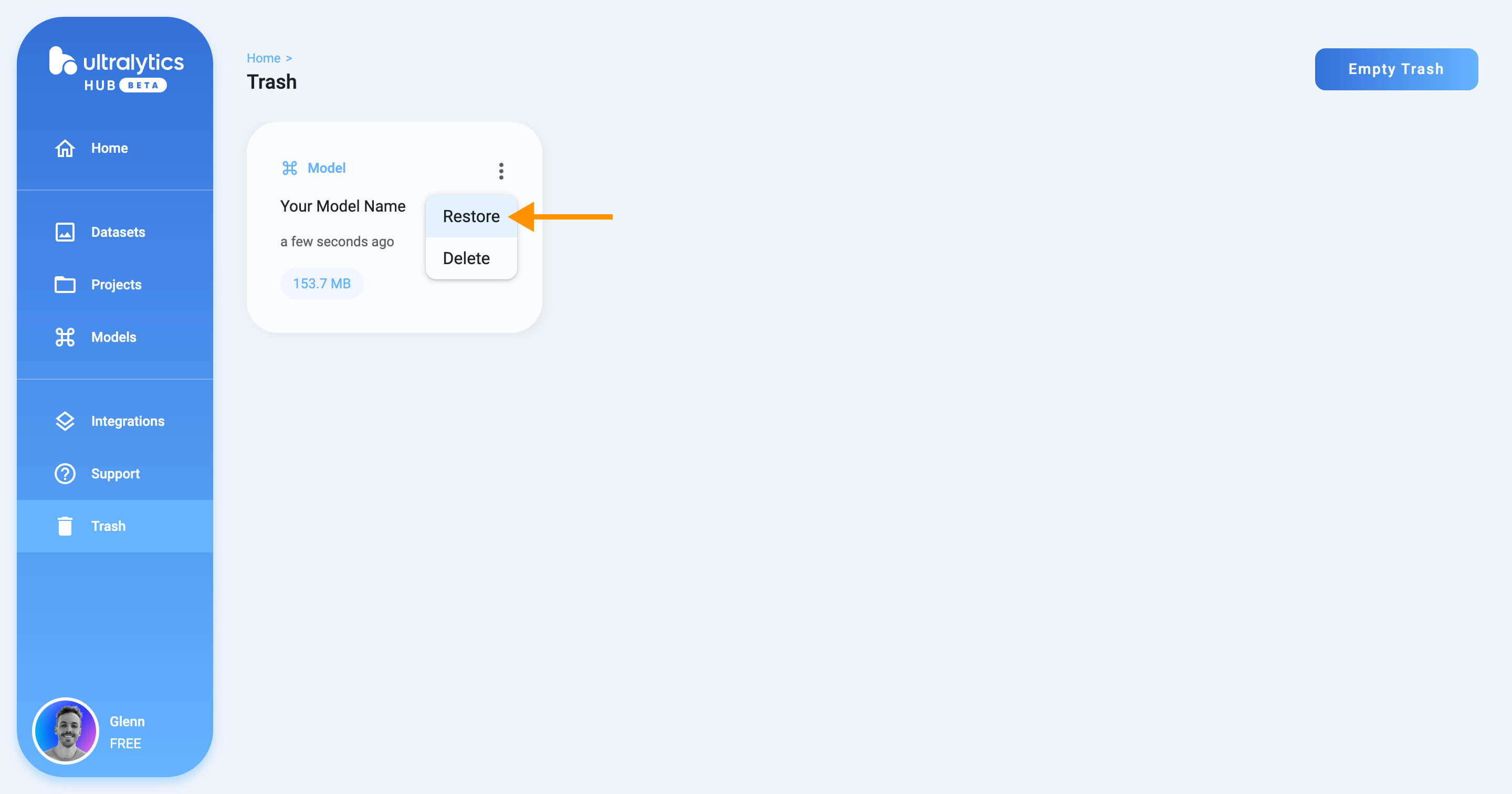
diff --git a/yolov8/docs/hub/projects.md b/yolov8/docs/hub/projects.md
new file mode 100644
index 0000000000000000000000000000000000000000..d8e0f864900308d1afa96bc19ae8a8f2003feb82
--- /dev/null
+++ b/yolov8/docs/hub/projects.md
@@ -0,0 +1,169 @@
+---
+comments: true
+description: Efficiently manage and compare AI models with Ultralytics HUB Projects. Create, share, and edit projects for streamlined model development.
+keywords: Ultralytics HUB projects, model management, model comparison, create project, share project, edit project, delete project, compare models
+---
+
+# Ultralytics HUB Projects
+
+Ultralytics HUB projects provide an effective solution for consolidating and managing your models. If you are working with several models that perform similar tasks or have related purposes, Ultralytics HUB projects allow you to group these models together.
+
+This creates a unified and organized workspace that facilitates easier model management, comparison and development. Having similar models or various iterations together can facilitate rapid benchmarking, as you can compare their effectiveness. This can lead to faster, more insightful iterative development and refinement of your models.
+
+## Create Project
+
+Navigate to the [Projects](https://hub.ultralytics.com/projects) page by clicking on the **Projects** button in the sidebar.
+
+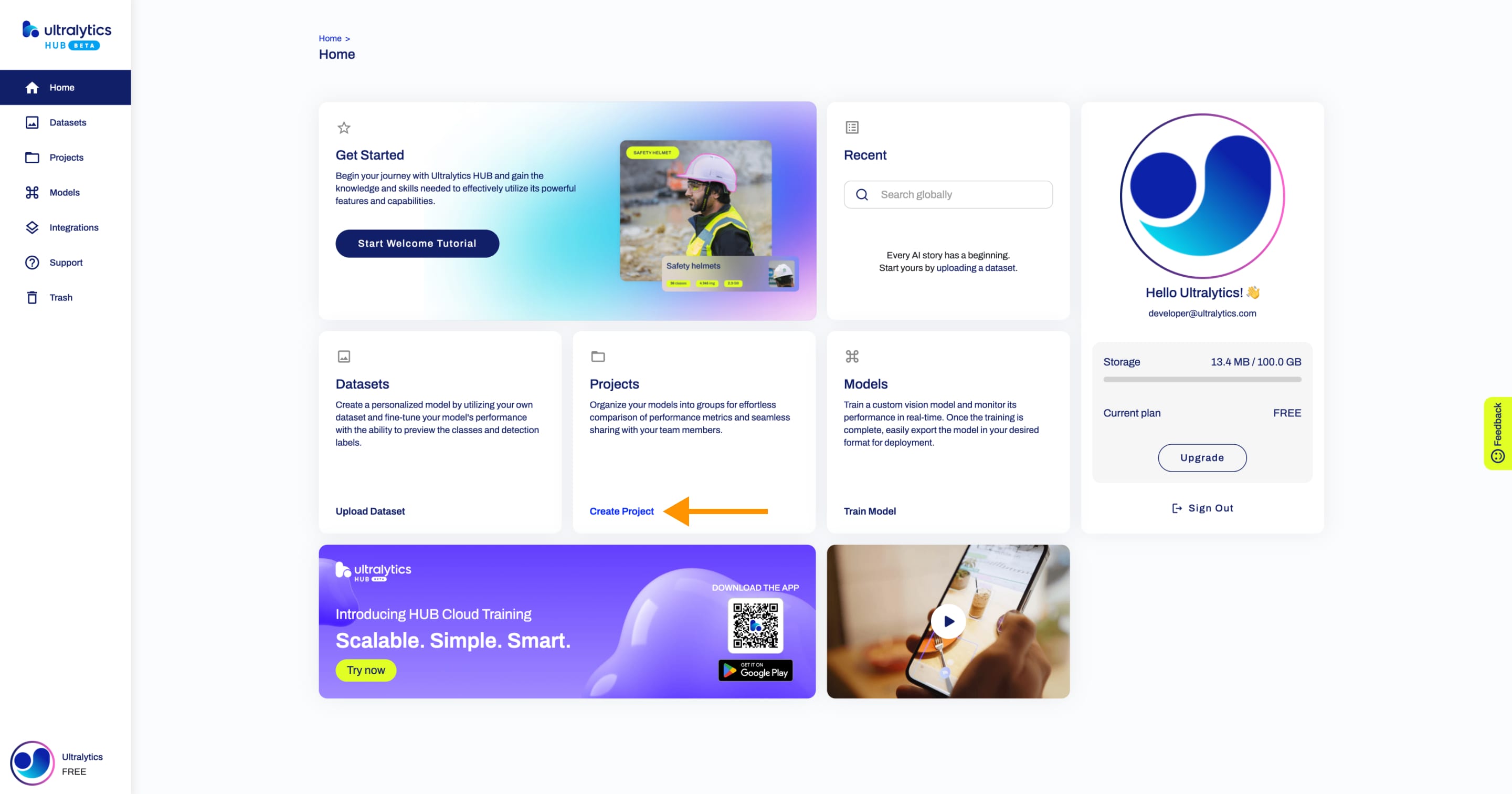
+
+??? tip "Tip"
+
+ You can also create a project directly from the [Home](https://hub.ultralytics.com/home) page.
+
+ 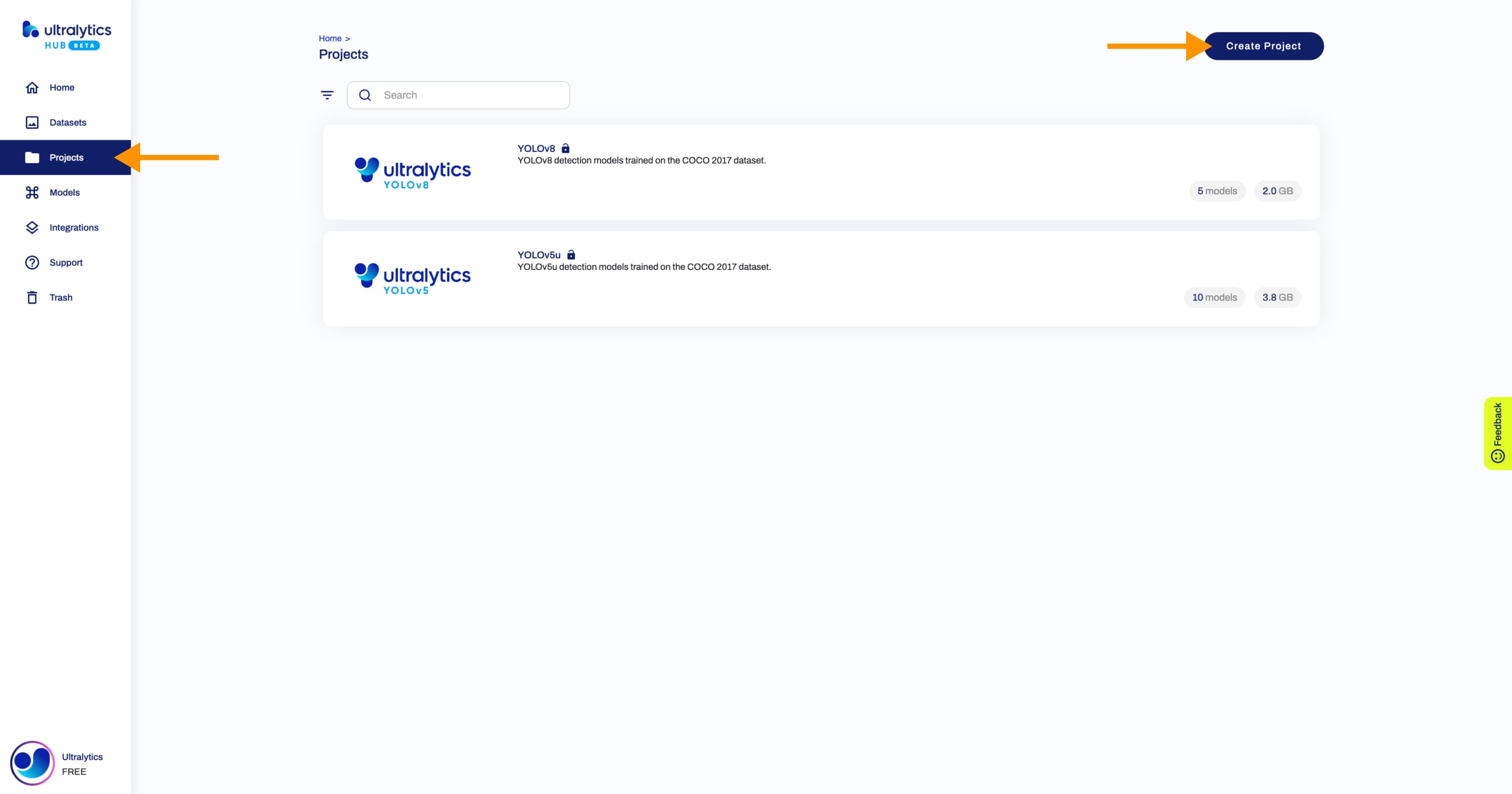
+
+Click on the **Create Project** button on the top right of the page. This action will trigger the **Create Project** dialog, opening up a suite of options for tailoring your project to your needs.
+
+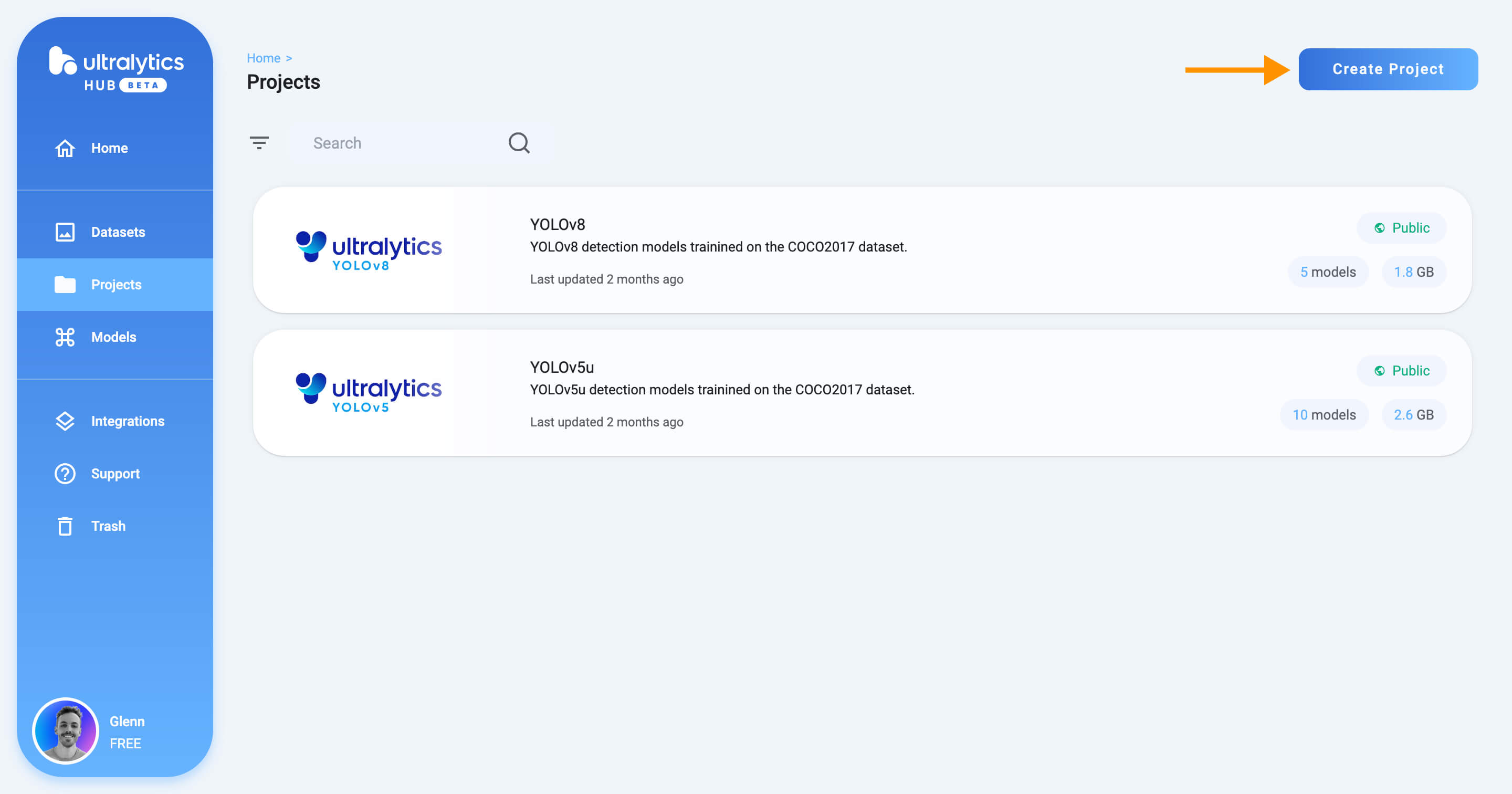
+
+Type the name of your project in the _Project name_ field or keep the default name and finalize the project creation with a single click.
+
+You have the additional option to enrich your project with a description and a unique image, enhancing its recognizability on the Projects page.
+
+When you're happy with your project configuration, click **Create**.
+
+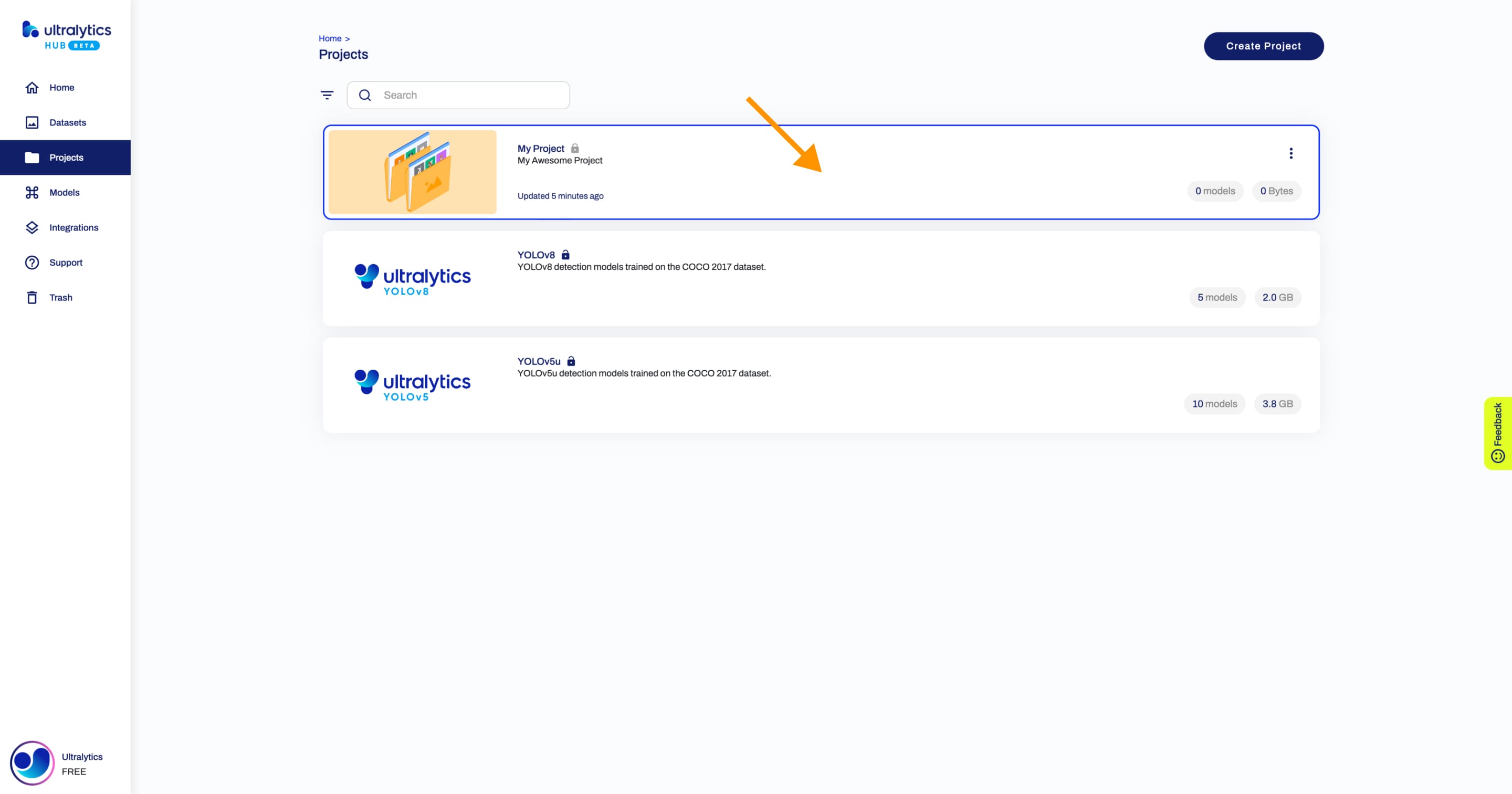
+
+After your project is created, you will be able to access it from the Projects page.
+
+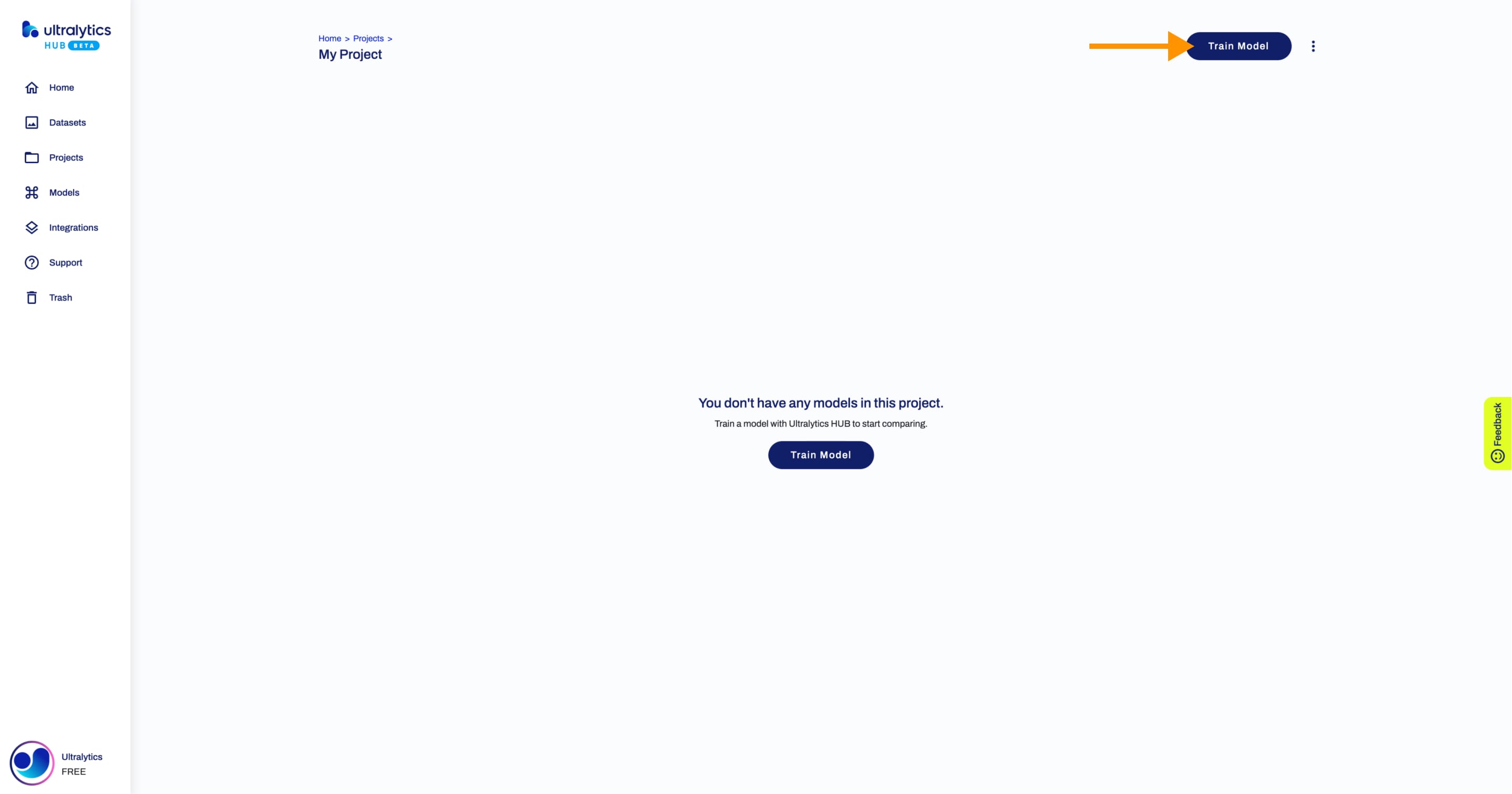
+
+Next, [train a model](https://docs.ultralytics.com/hub/models/#train-model) inside your project.
+
+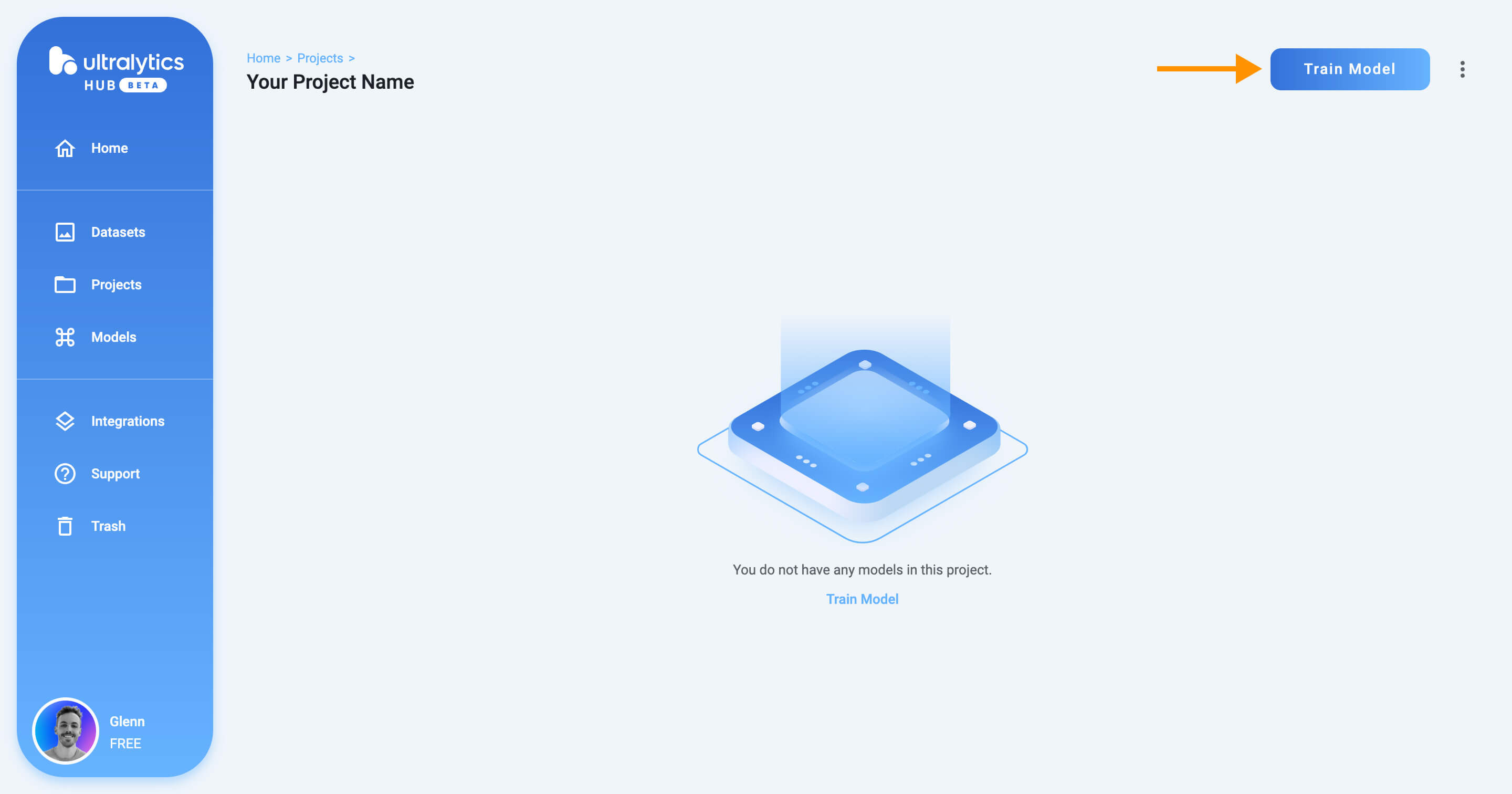
+
+## Share Project
+
+!!! info "Info"
+
+ Ultralytics HUB's sharing functionality provides a convenient way to share projects with others. This feature is designed to accommodate both existing Ultralytics HUB users and those who have yet to create an account.
+
+??? note "Note"
+
+ You have control over the general access of your projects.
+
+ You can choose to set the general access to "Private", in which case, only you will have access to it. Alternatively, you can set the general access to "Unlisted" which grants viewing access to anyone who has the direct link to the project, regardless of whether they have an Ultralytics HUB account or not.
+
+Navigate to the Project page of the project you want to share, open the project actions dropdown and click on the **Share** option. This action will trigger the **Share Project** dialog.
+
+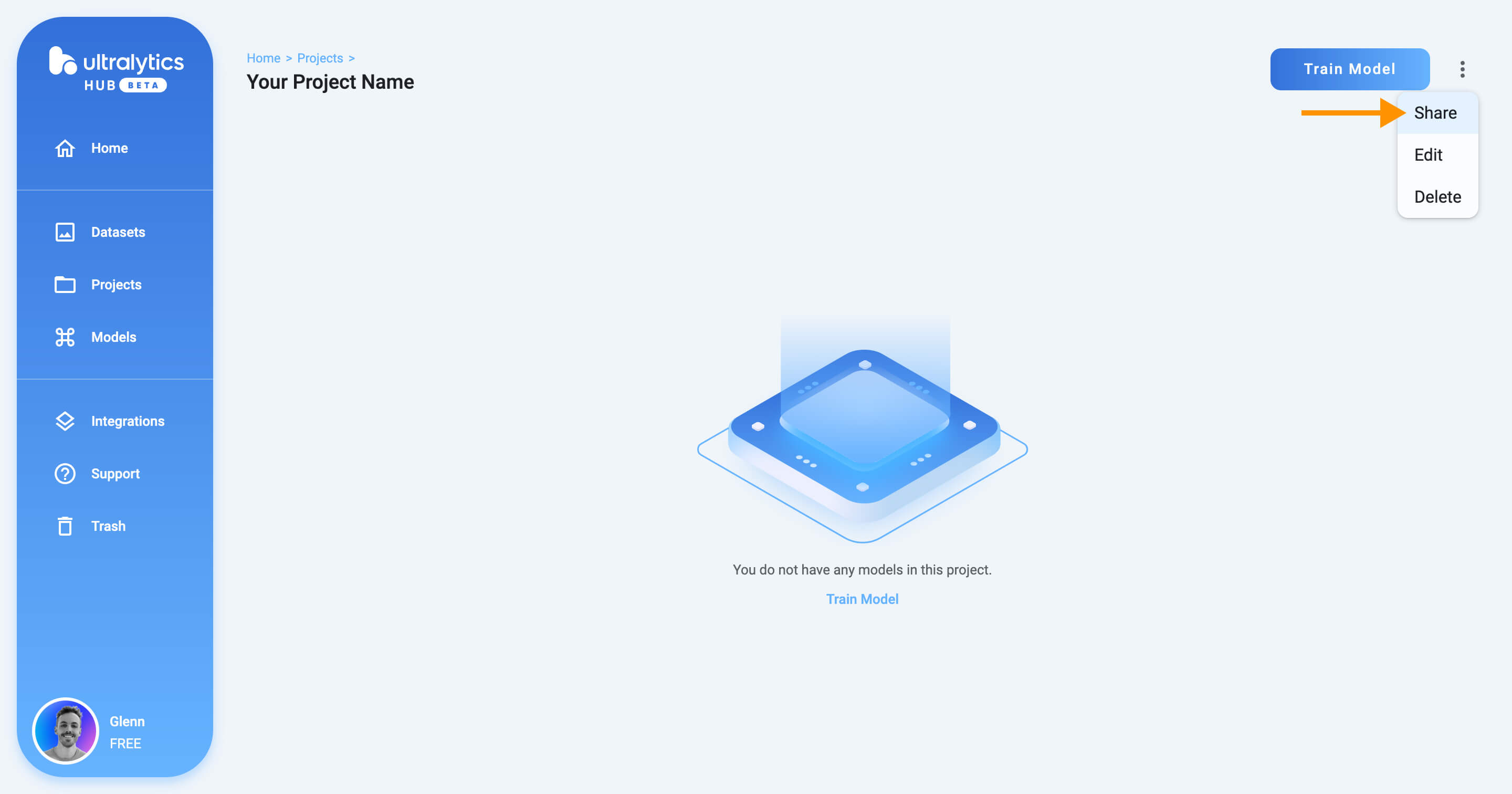
+
+??? tip "Tip"
+
+ You can also share a project directly from the [Projects](https://hub.ultralytics.com/projects) page.
+
+ 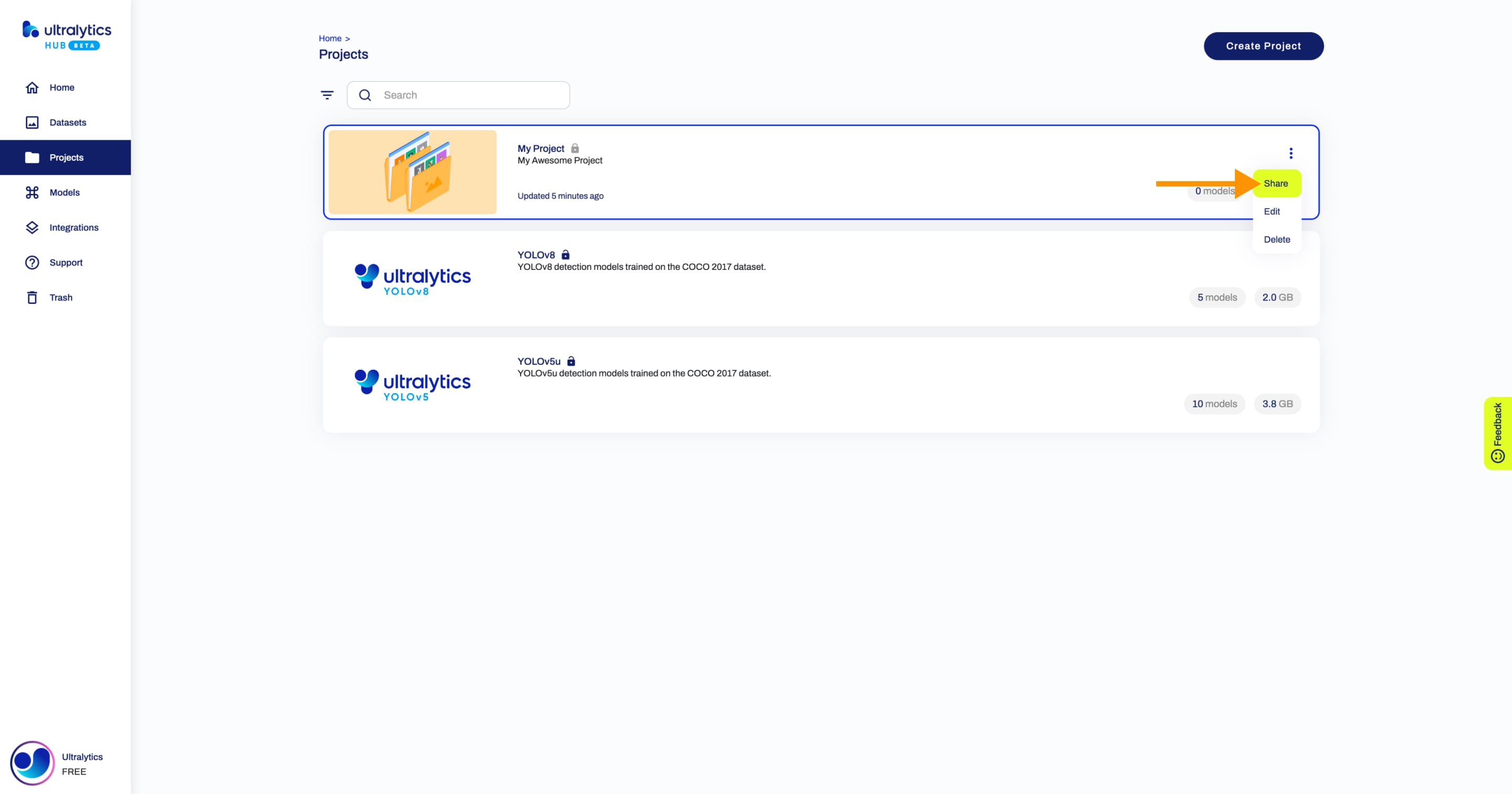
+
+Set the general access to "Unlisted" and click **Save**.
+
+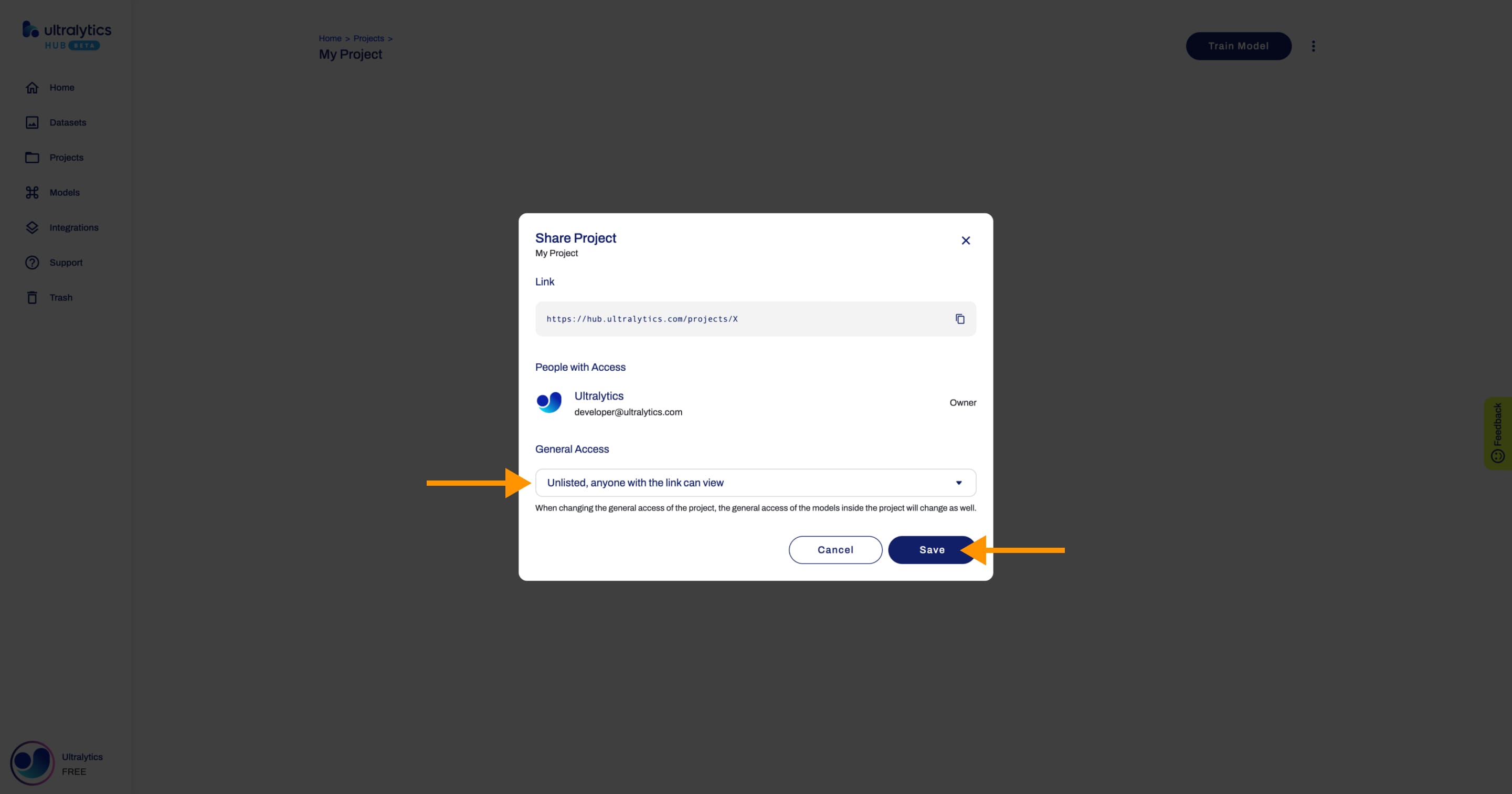
+
+!!! warning "Warning"
+
+ When changing the general access of a project, the general access of the models inside the project will be changed as well.
+
+Now, anyone who has the direct link to your project can view it.
+
+??? tip "Tip"
+
+ You can easily click on the project's link shown in the **Share Project** dialog to copy it.
+
+ 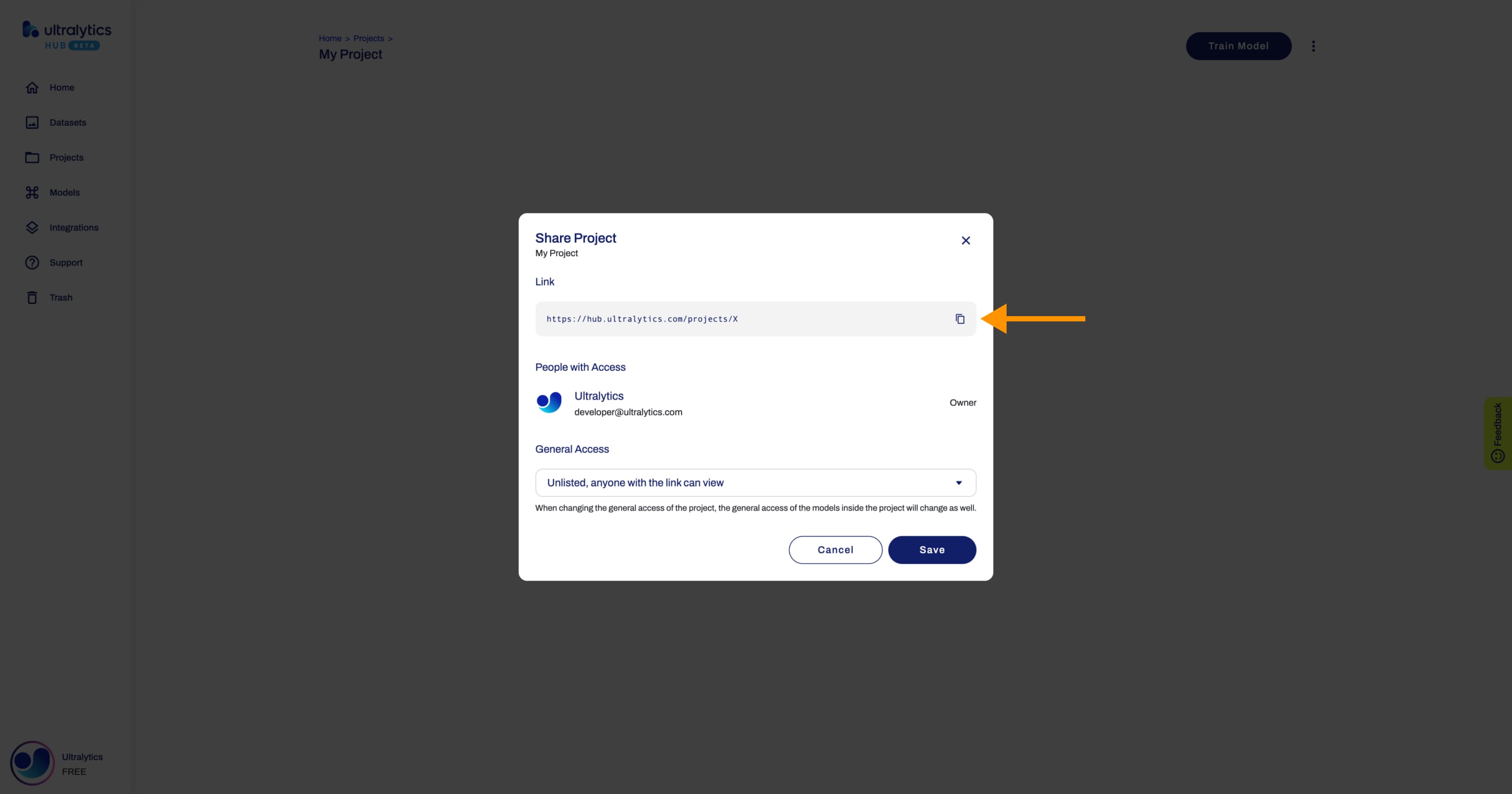
+
+## Edit Project
+
+Navigate to the Project page of the project you want to edit, open the project actions dropdown and click on the **Edit** option. This action will trigger the **Update Project** dialog.
+
+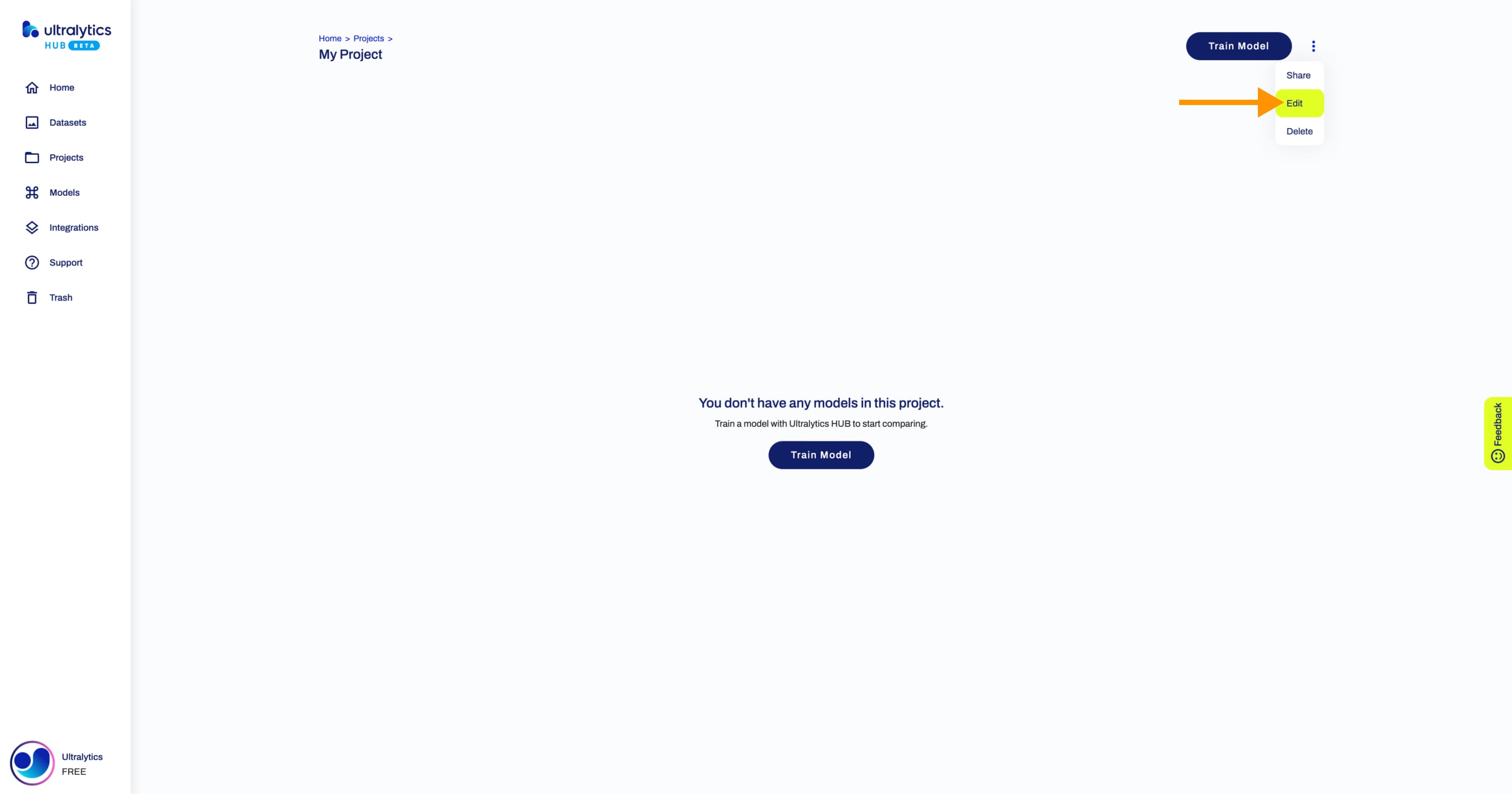
+
+??? tip "Tip"
+
+ You can also edit a project directly from the [Projects](https://hub.ultralytics.com/projects) page.
+
+ 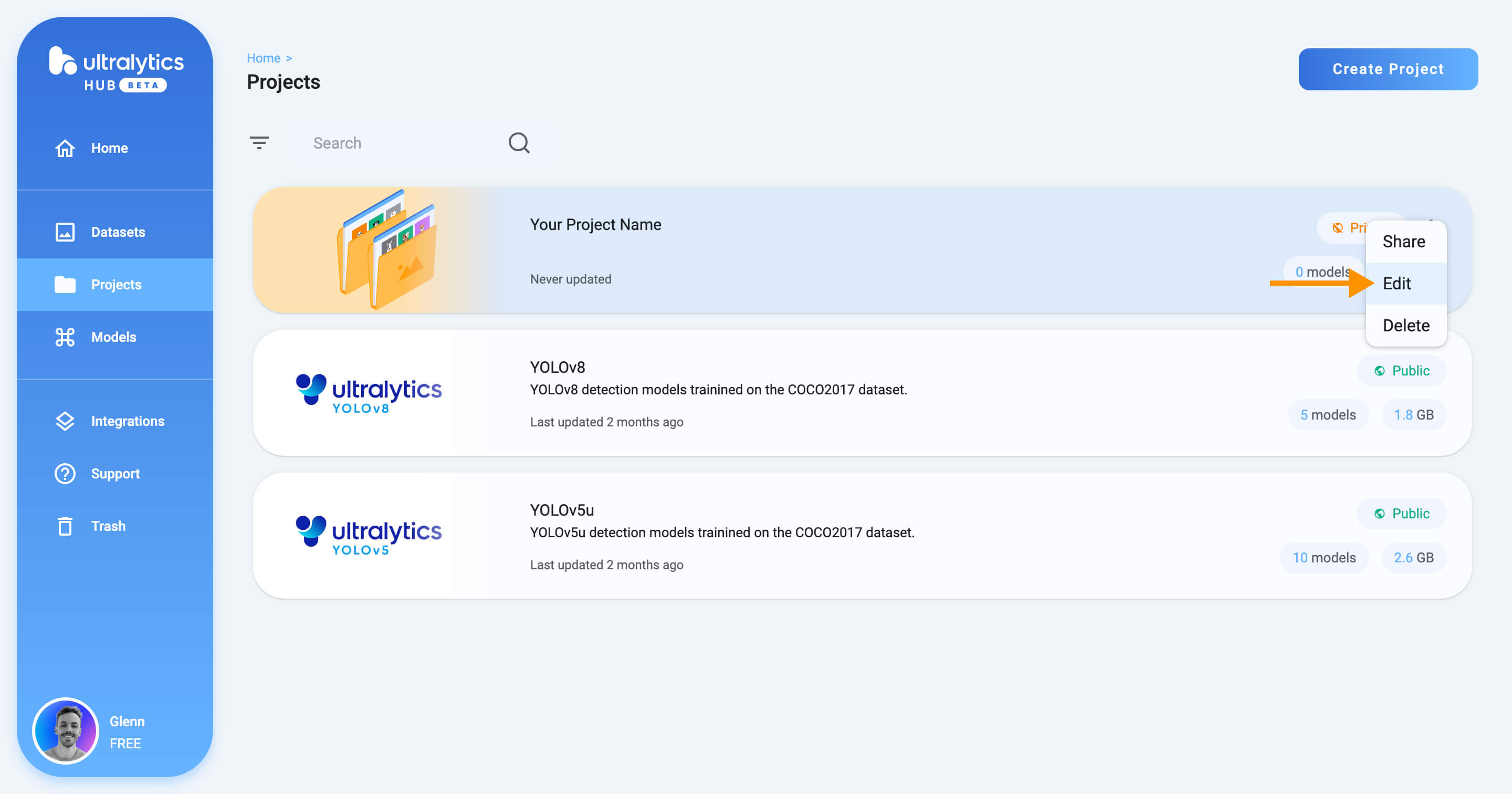
+
+Apply the desired modifications to your project and then confirm the changes by clicking **Save**.
+
+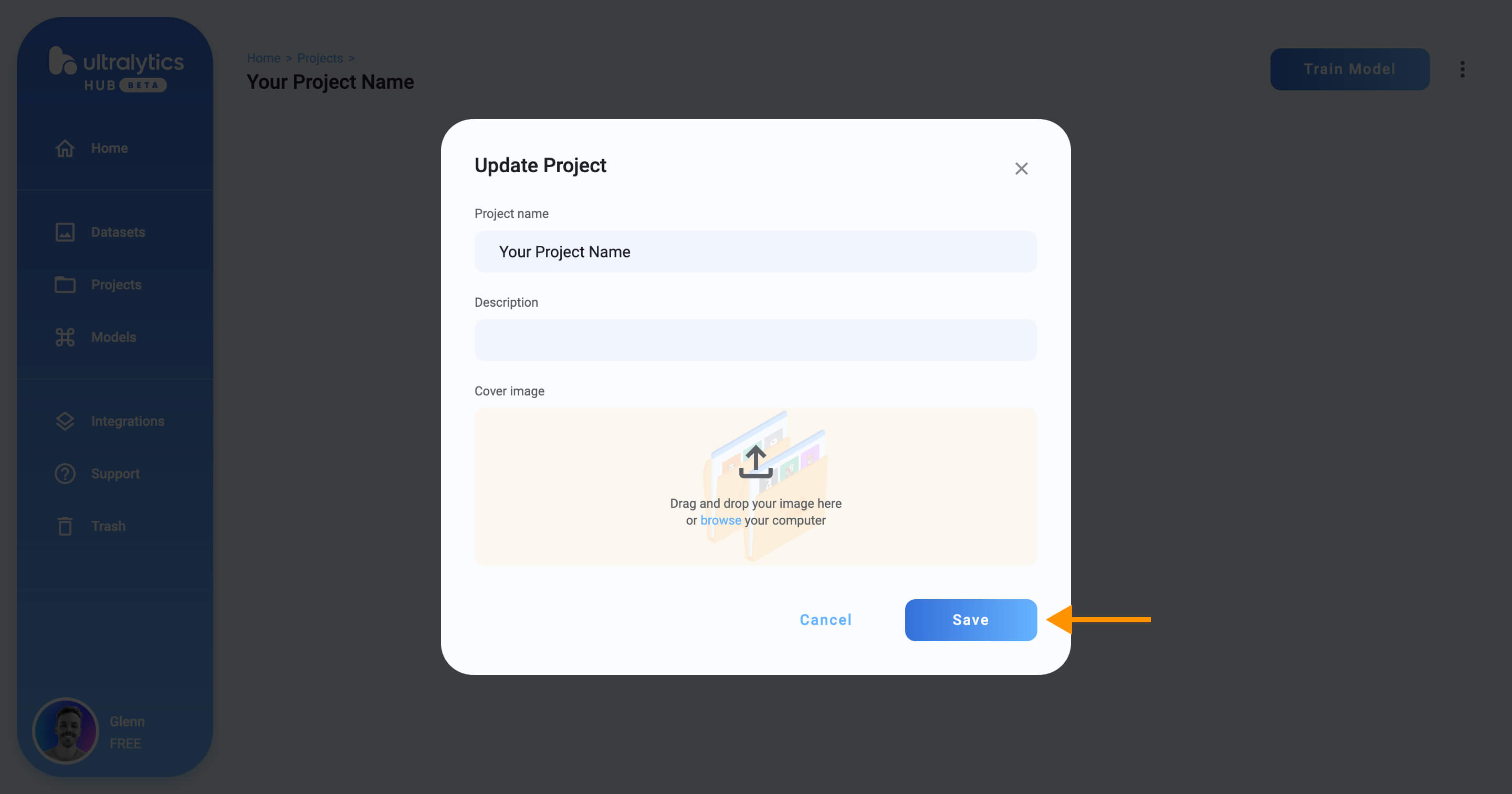
+
+## Delete Project
+
+Navigate to the Project page of the project you want to delete, open the project actions dropdown and click on the **Delete** option. This action will delete the project.
+
+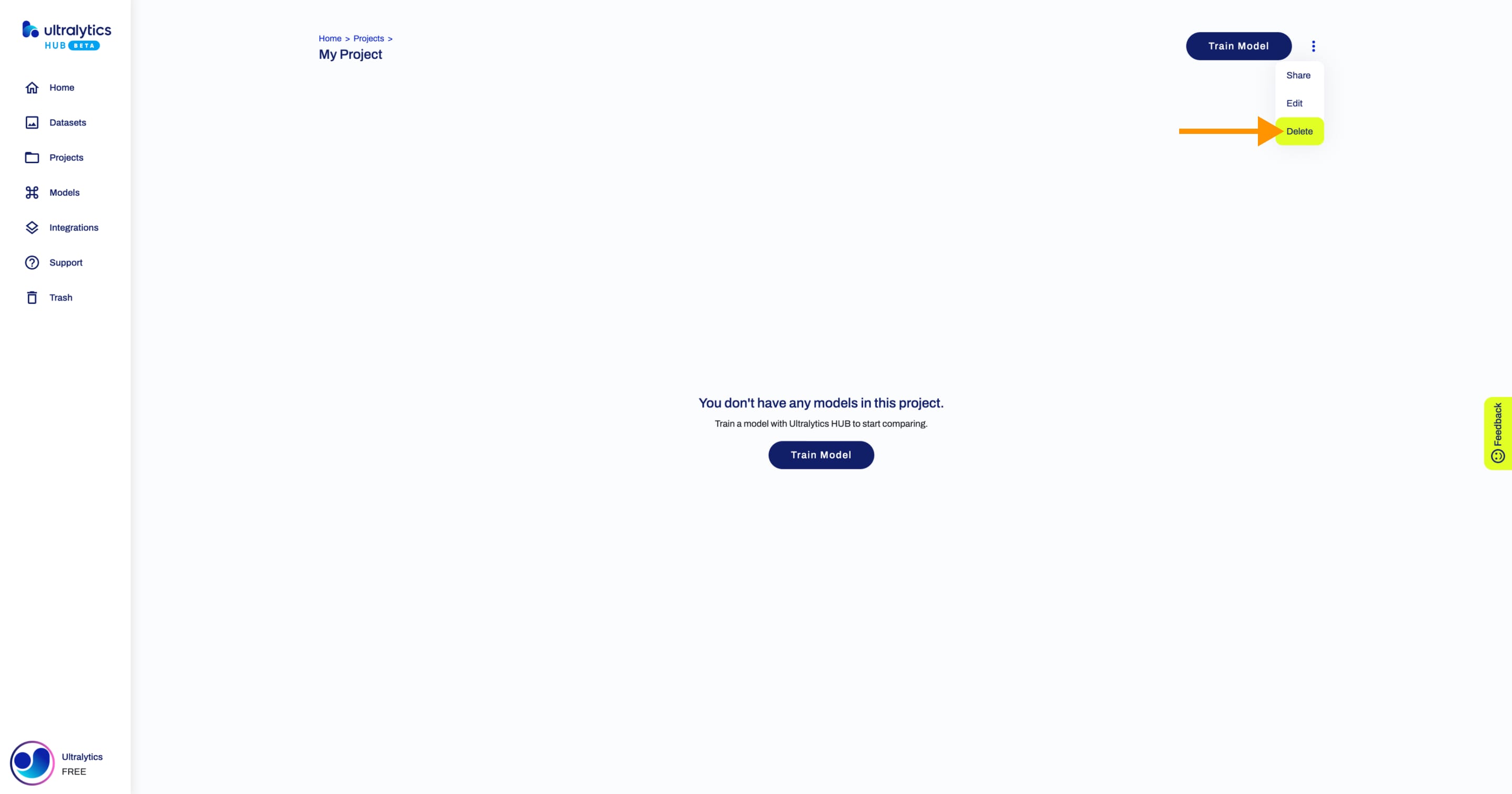
+
+??? tip "Tip"
+
+ You can also delete a project directly from the [Projects](https://hub.ultralytics.com/projects) page.
+
+ 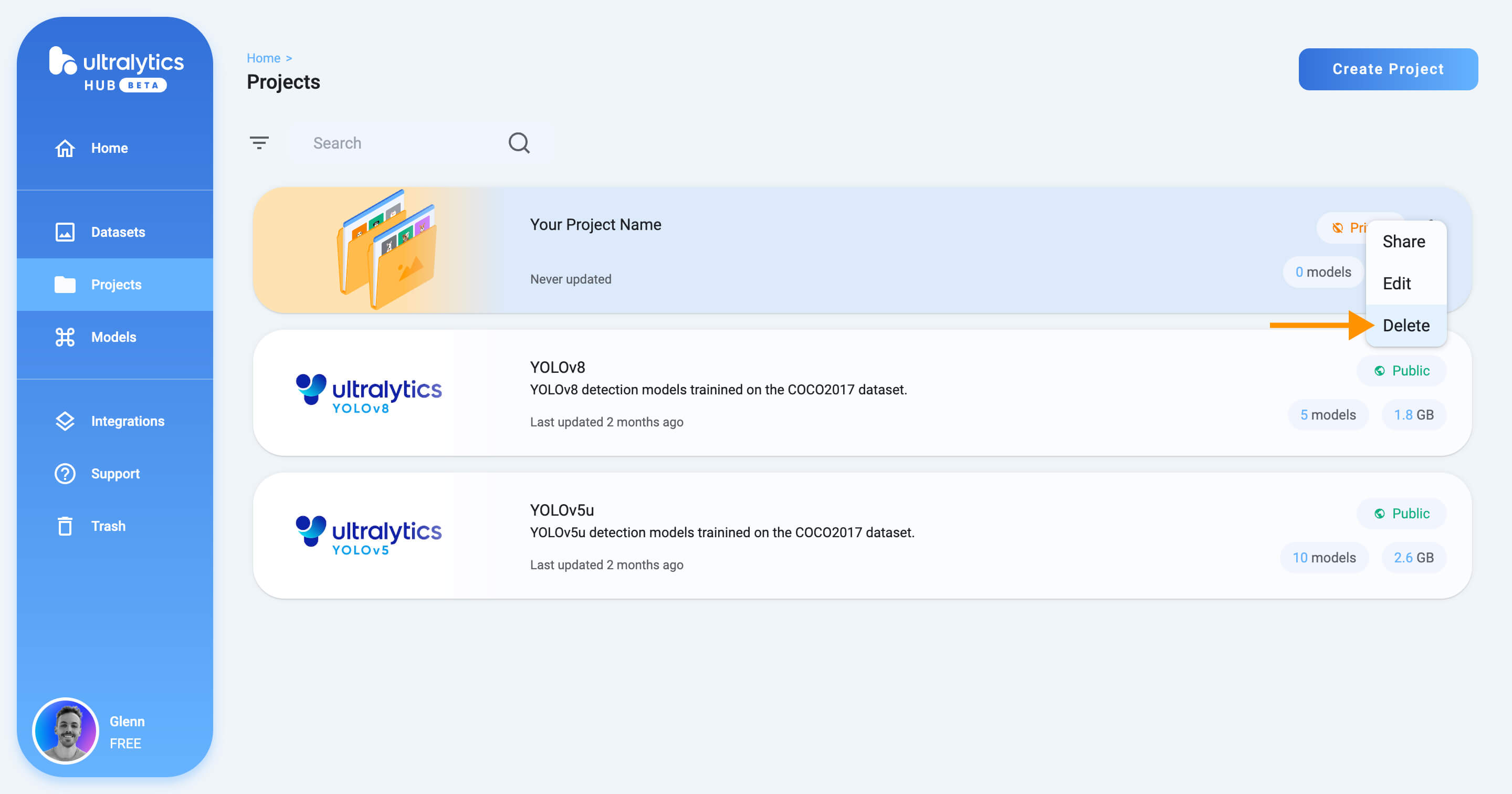
+
+!!! warning "Warning"
+
+ When deleting a project, the the models inside the project will be deleted as well.
+
+??? note "Note"
+
+ If you change your mind, you can restore the project from the [Trash](https://hub.ultralytics.com/trash) page.
+
+ 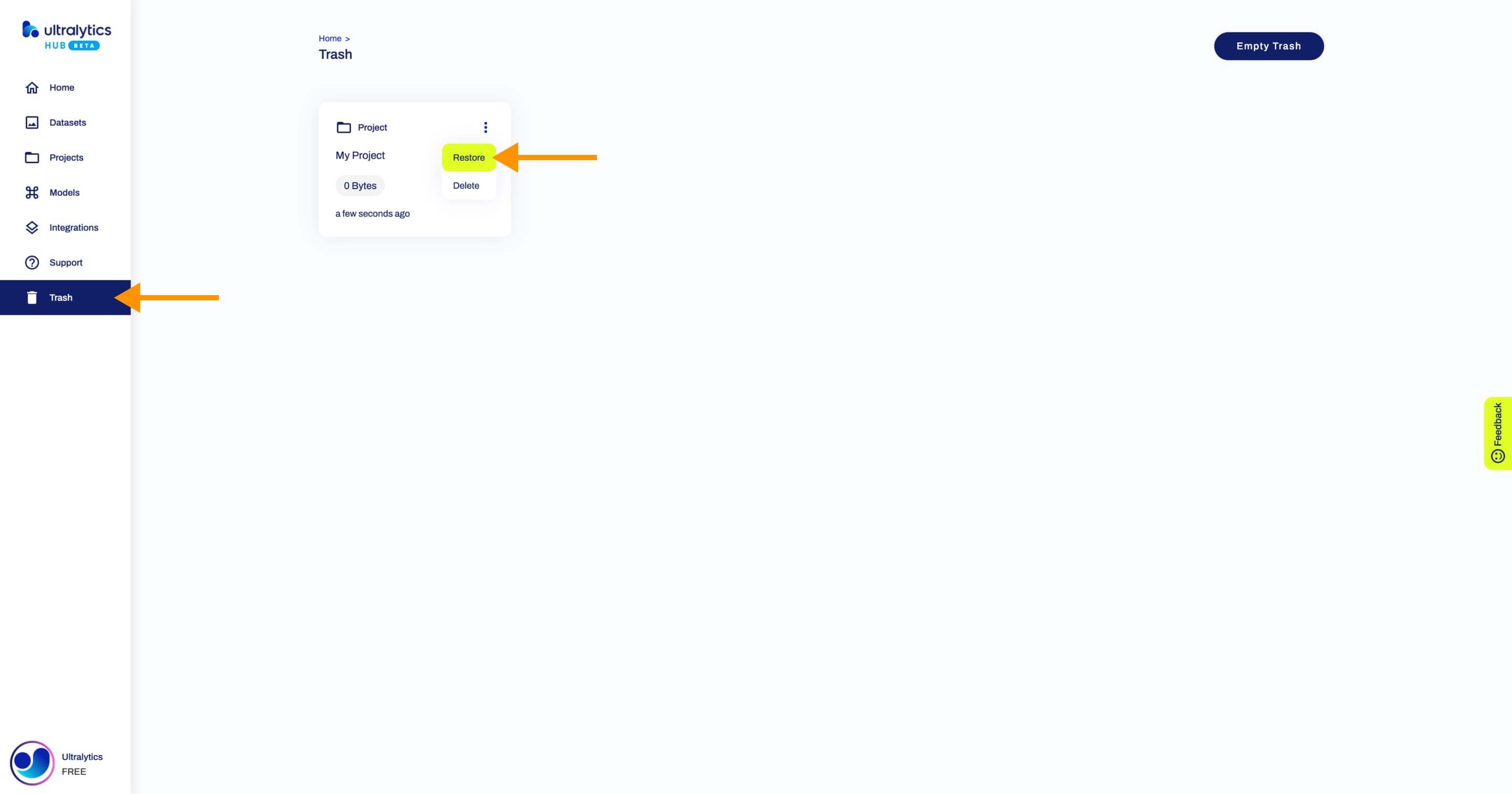
+
+## Compare Models
+
+Navigate to the Project page of the project where the models you want to compare are located. To use the model comparison feature, click on the **Charts** tab.
+
+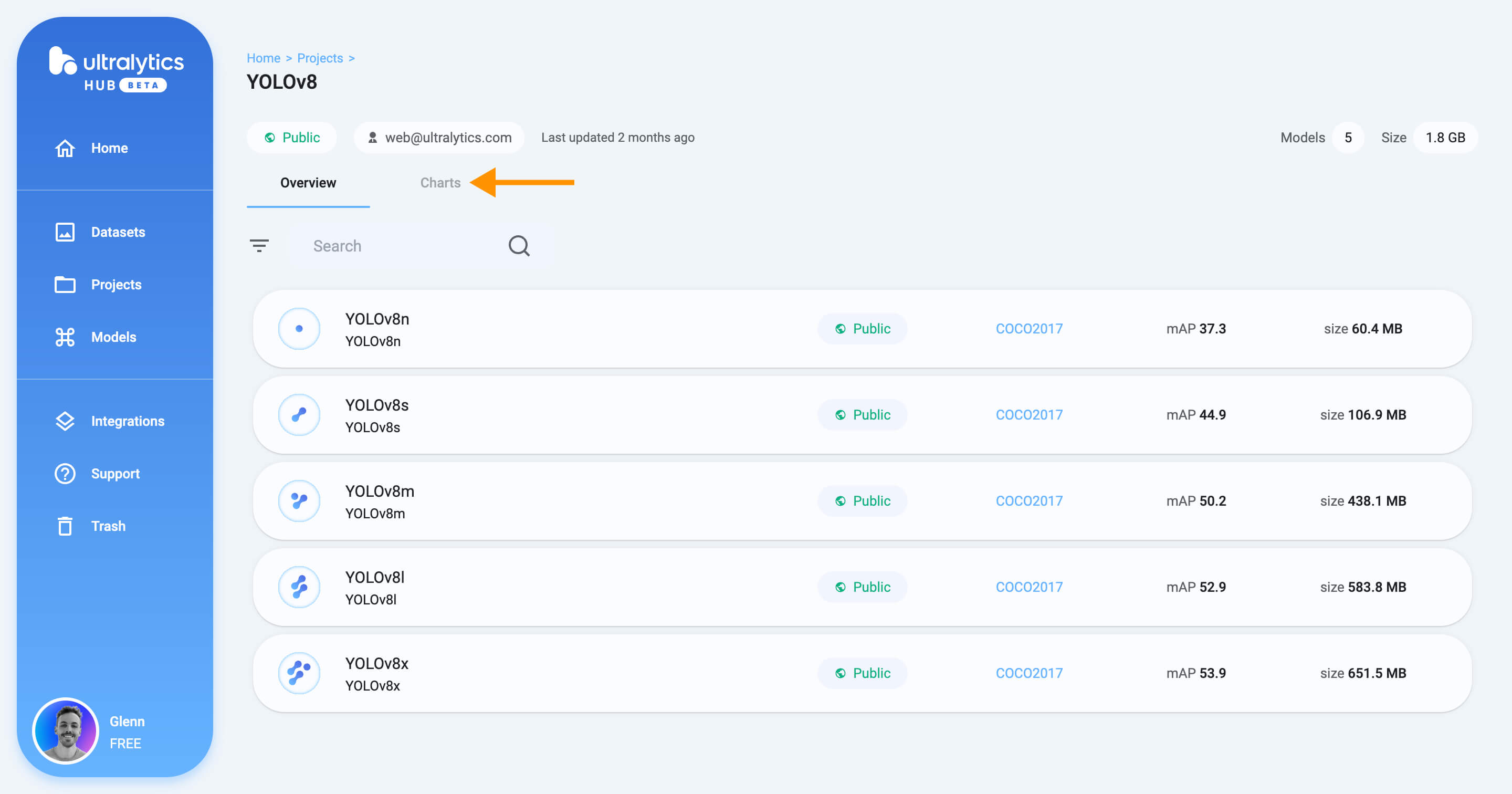
+
+This will display all the relevant charts. Each chart corresponds to a different metric and contains the performance of each model for that metric. The models are represented by different colors and you can hover over each data point to get more information.
+
+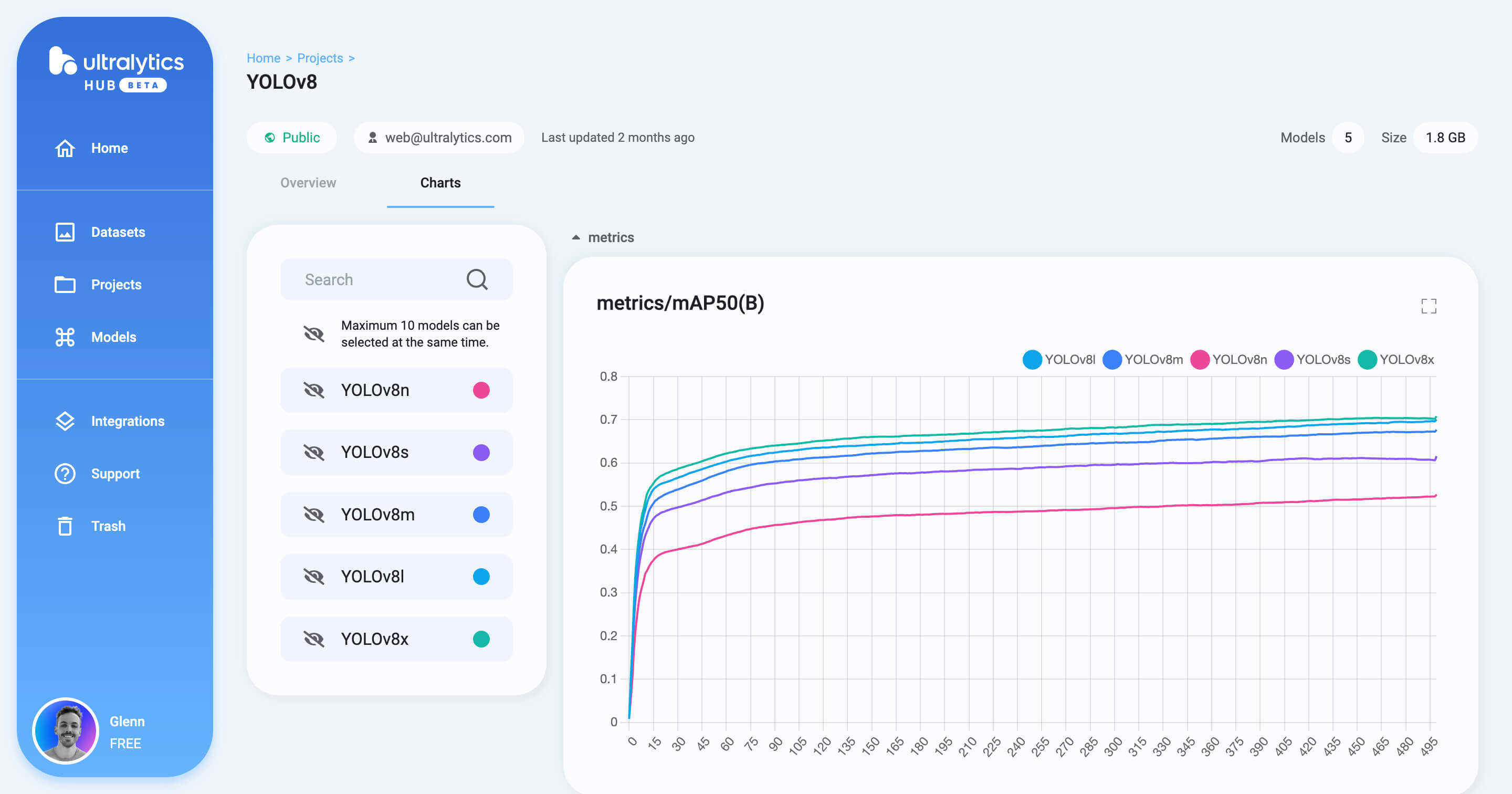
+
+??? tip "Tip"
+
+ Each chart can be enlarged for better visualization.
+
+ 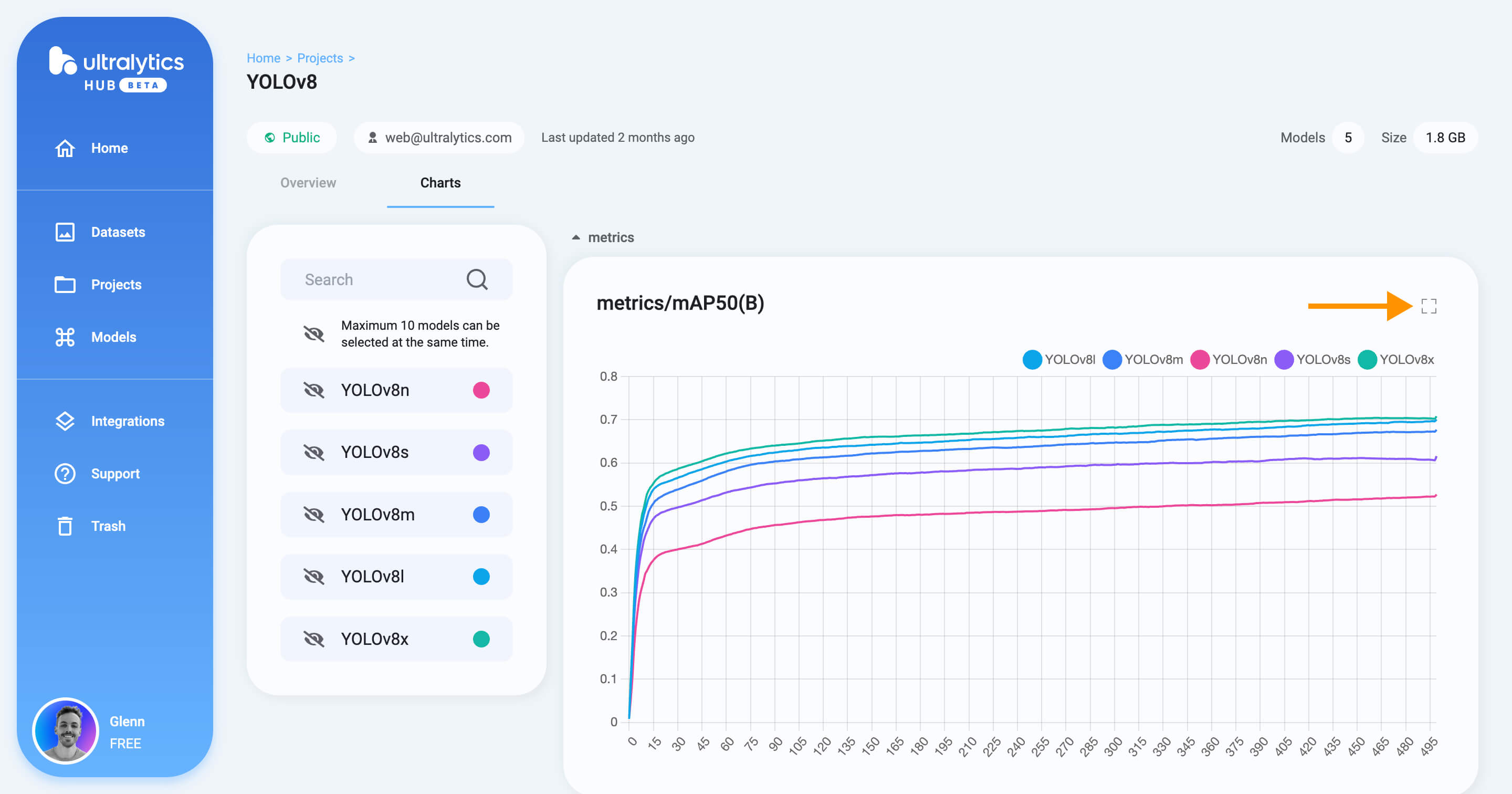
+
+ 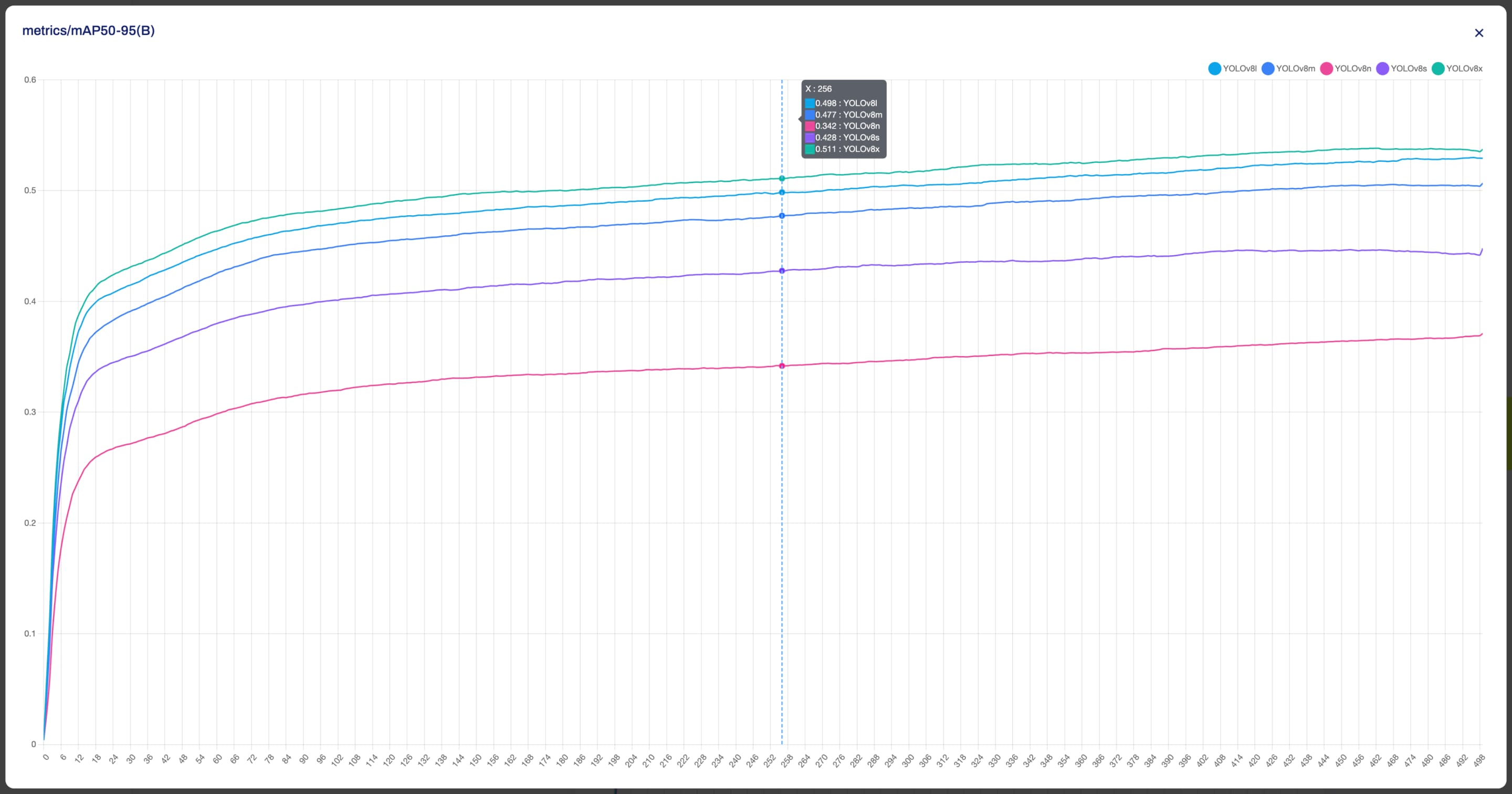
+
+??? tip "Tip"
+
+ You have the flexibility to customize your view by selectively hiding certain models. This feature allows you to concentrate on the models of interest.
+
+ 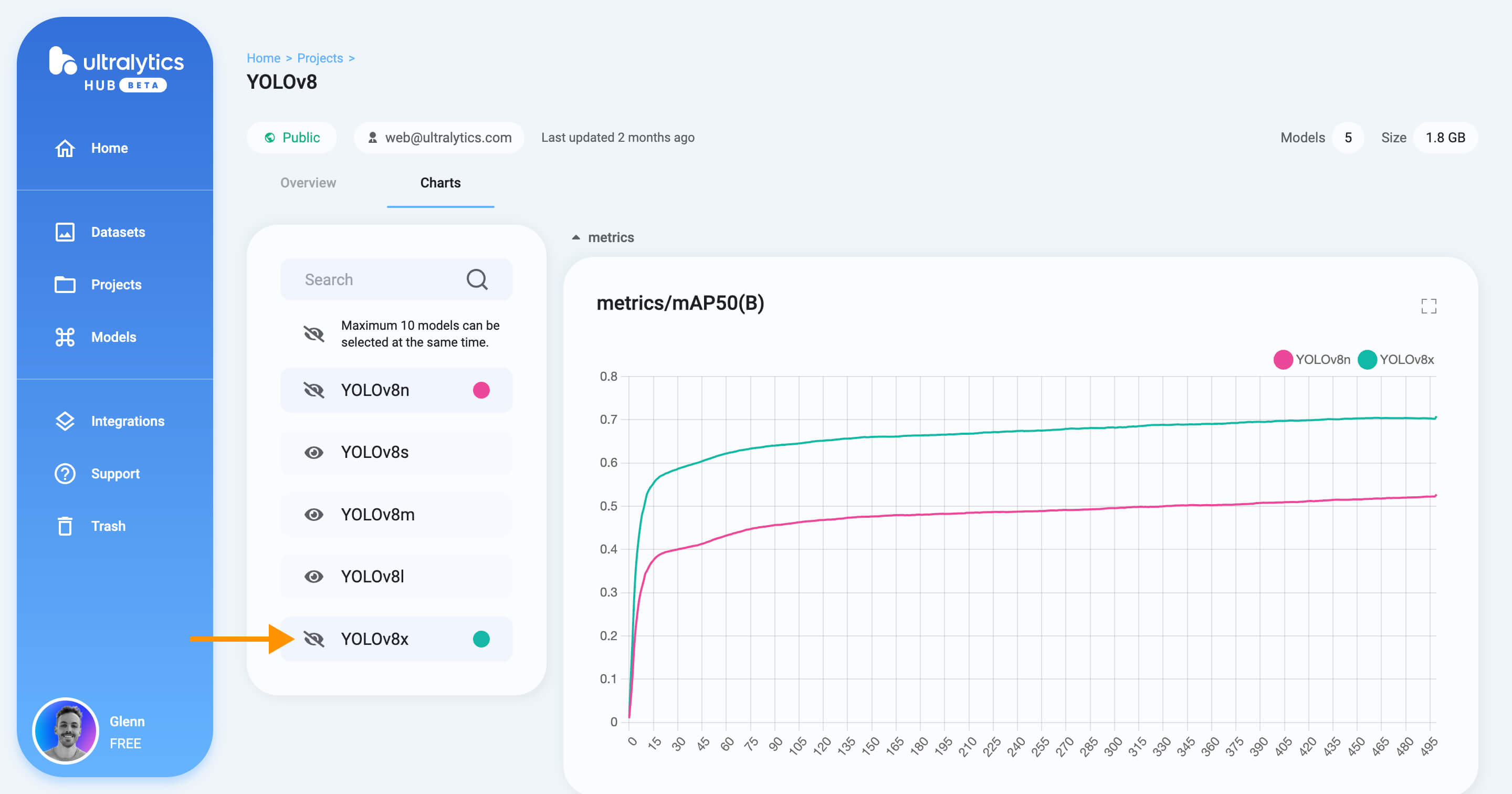
+
+## Reorder Models
+
+??? note "Note"
+
+ Ultralytics HUB's reordering functionality works only inside projects you own.
+
+Navigate to the Project page of the project where the models you want to reorder are located. Click on the designated reorder icon of the model you want to move and drag it to the desired location.
+
+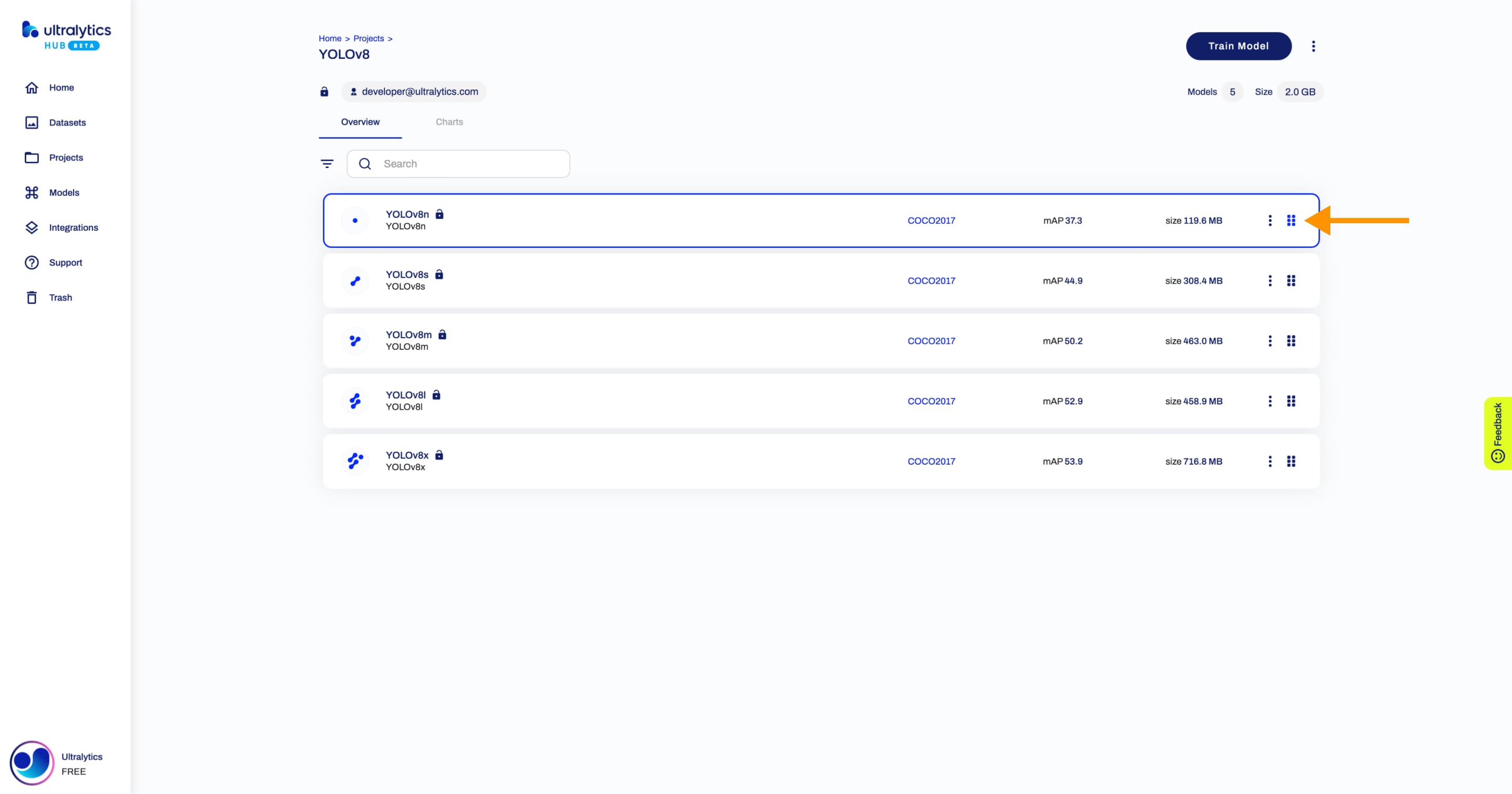
+
+## Transfer Models
+
+Navigate to the Project page of the project where the model you want to mode is located, open the project actions dropdown and click on the **Transfer** option. This action will trigger the **Transfer Model** dialog.
+
+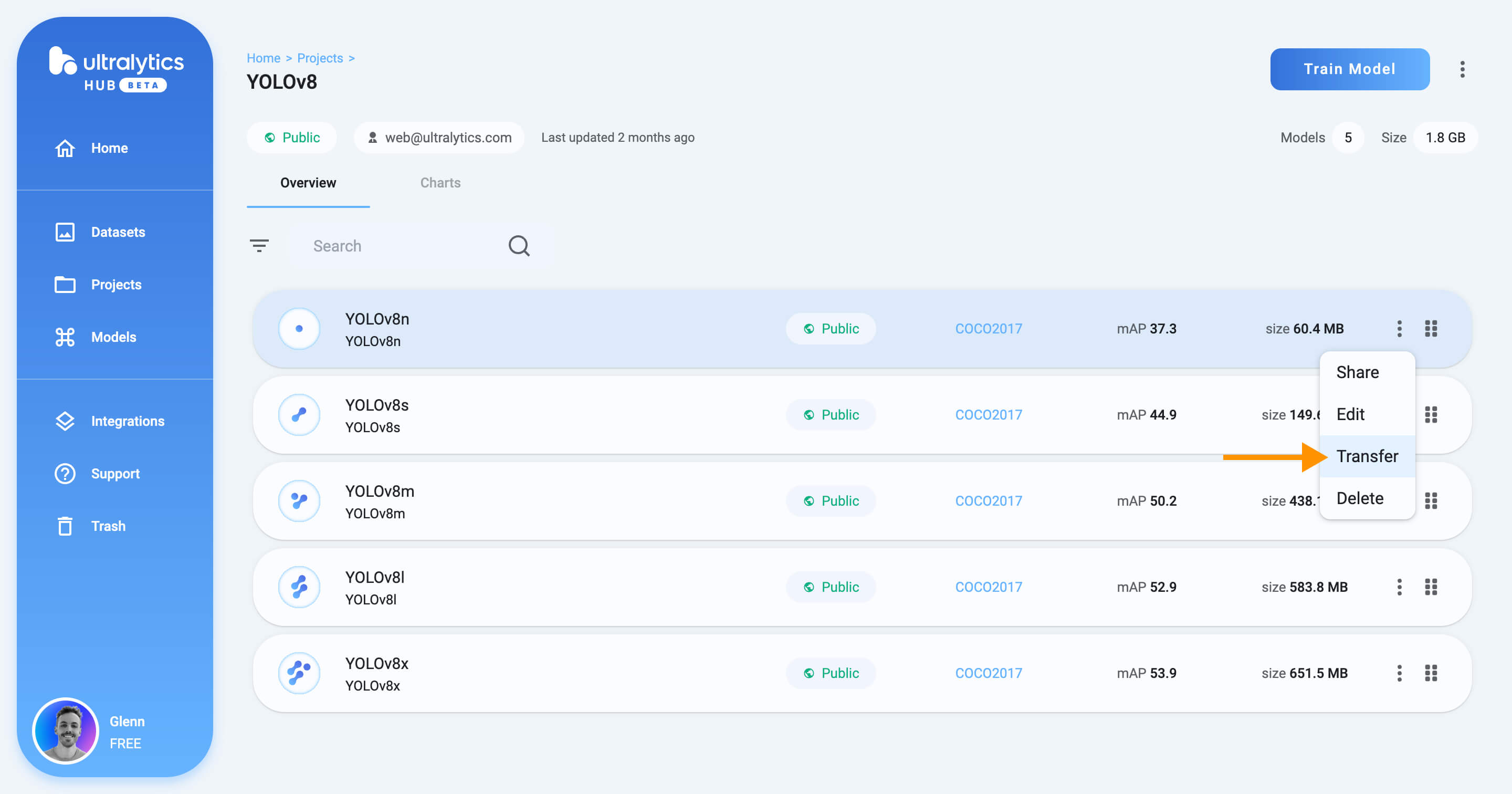
+
+??? tip "Tip"
+
+ You can also transfer a model directly from the [Models](https://hub.ultralytics.com/models) page.
+
+ 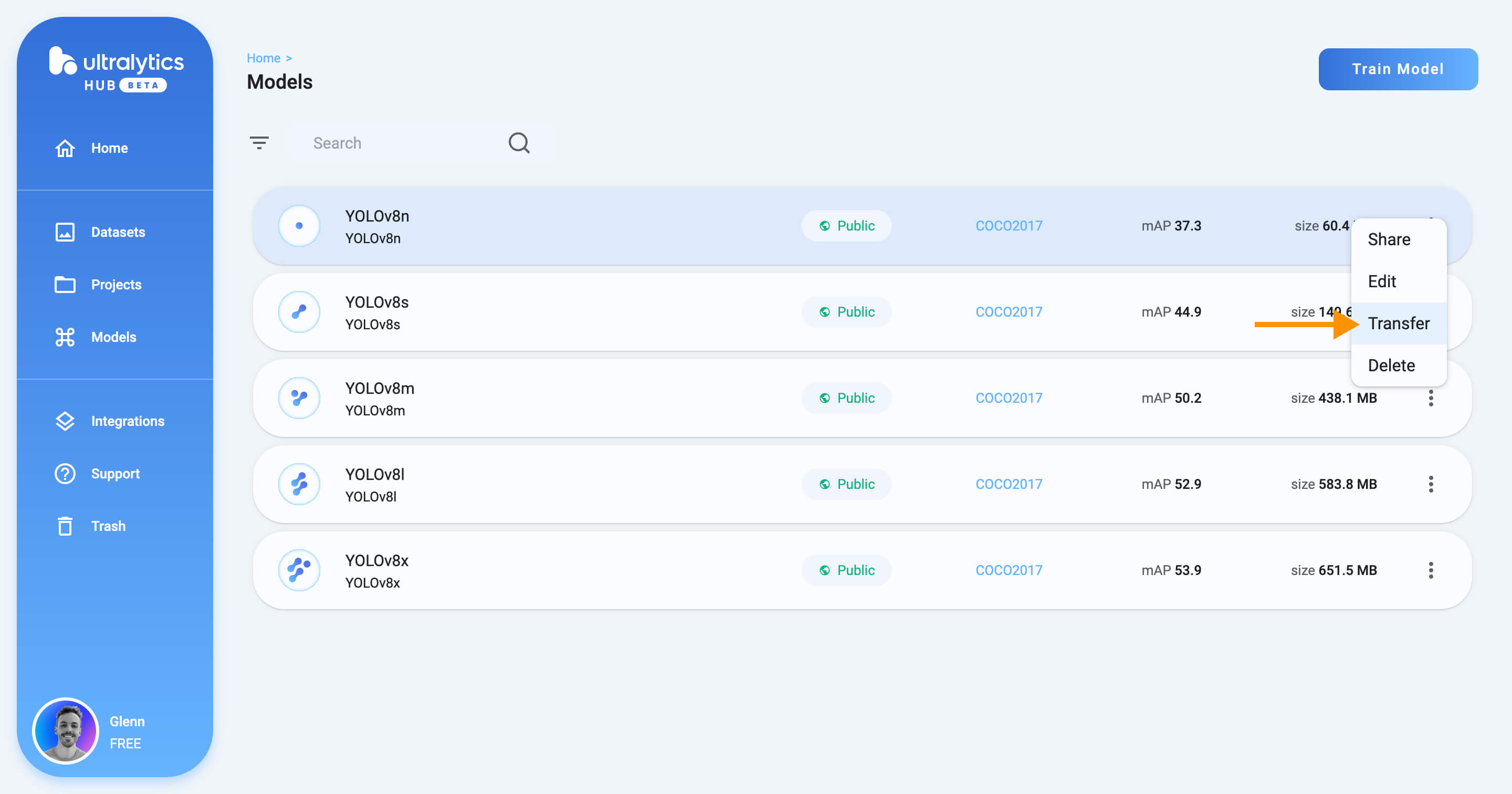
+
+Select the project you want to transfer the model to and click **Save**.
+
+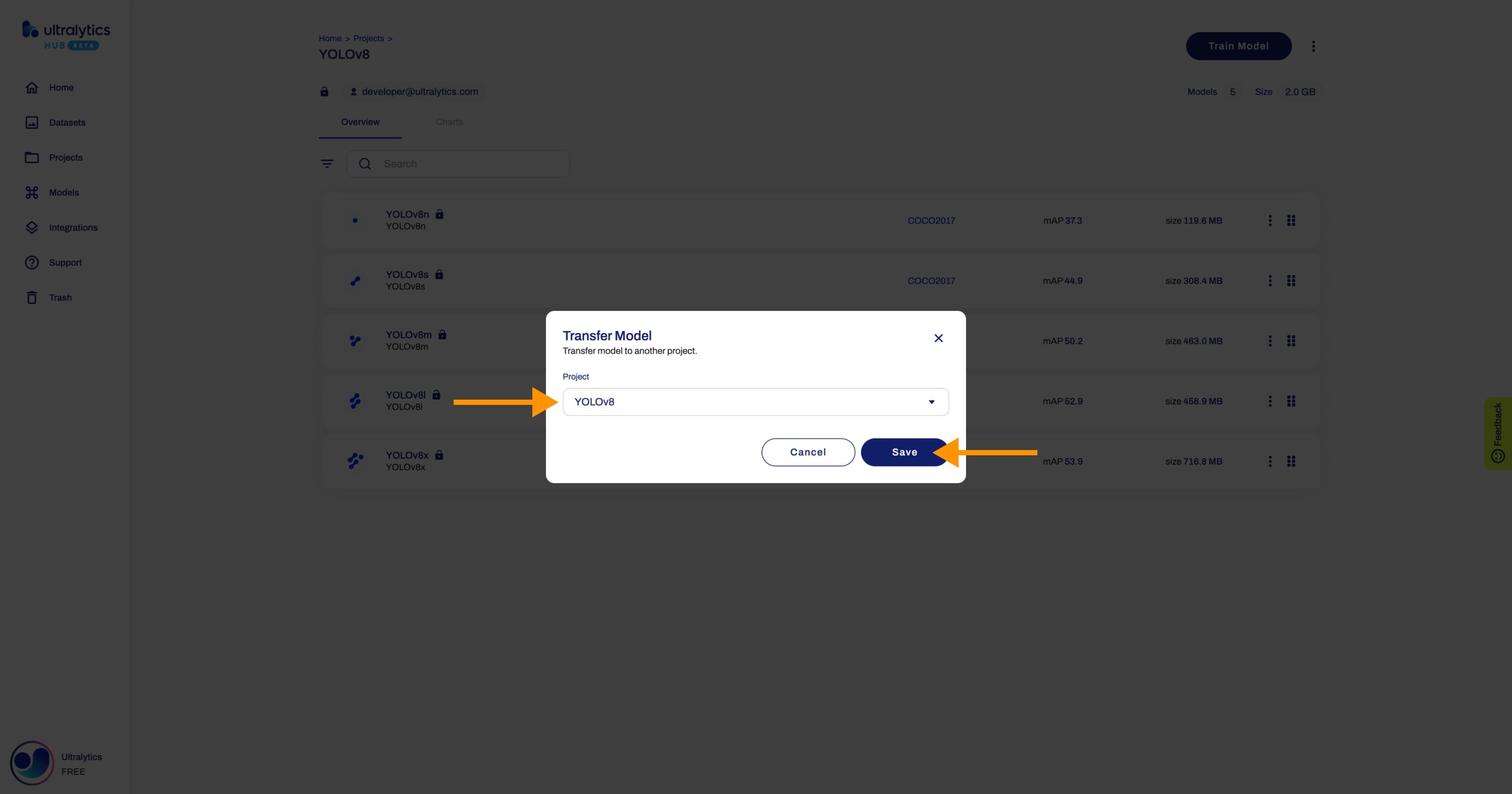
diff --git a/yolov8/docs/hub/quickstart.md b/yolov8/docs/hub/quickstart.md
new file mode 100644
index 0000000000000000000000000000000000000000..19480d27d8234ce677ded54d5e134bc8862bc0bf
--- /dev/null
+++ b/yolov8/docs/hub/quickstart.md
@@ -0,0 +1,7 @@
+---
+comments: true
+---
+
+# 🚧 Page Under Construction ⚒
+
+This page is currently under construction!️ 👷Please check back later for updates. 😃🔜
diff --git a/yolov8/docs/index.md b/yolov8/docs/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..79768a1b0025eff1e19367abfdafb7ced34d224f
--- /dev/null
+++ b/yolov8/docs/index.md
@@ -0,0 +1,51 @@
+---
+comments: true
+description: Explore Ultralytics YOLOv8, a cutting-edge real-time object detection and image segmentation model for various applications and hardware platforms.
+keywords: YOLOv8, object detection, image segmentation, computer vision, machine learning, deep learning, AGPL-3.0 License, Enterprise License
+---
+
+
+
+Introducing [Ultralytics](https://ultralytics.com) [YOLOv8](https://github.com/ultralytics/ultralytics), the latest version of the acclaimed real-time object detection and image segmentation model. YOLOv8 is built on cutting-edge advancements in deep learning and computer vision, offering unparalleled performance in terms of speed and accuracy. Its streamlined design makes it suitable for various applications and easily adaptable to different hardware platforms, from edge devices to cloud APIs.
+
+Explore the YOLOv8 Docs, a comprehensive resource designed to help you understand and utilize its features and capabilities. Whether you are a seasoned machine learning practitioner or new to the field, this hub aims to maximize YOLOv8's potential in your projects
+
+## Where to Start
+
+- **Install** `ultralytics` with pip and get up and running in minutes [:material-clock-fast: Get Started](quickstart.md){ .md-button }
+- **Predict** new images and videos with YOLOv8 [:octicons-image-16: Predict on Images](modes/predict.md){ .md-button }
+- **Train** a new YOLOv8 model on your own custom dataset [:fontawesome-solid-brain: Train a Model](modes/train.md){ .md-button }
+- **Explore** YOLOv8 tasks like segment, classify, pose and track [:material-magnify-expand: Explore Tasks](tasks/index.md){ .md-button }
+
+## YOLO: A Brief History
+
+[YOLO](https://arxiv.org/abs/1506.02640) (You Only Look Once), a popular object detection and image segmentation model, was developed by Joseph Redmon and Ali Farhadi at the University of Washington. Launched in 2015, YOLO quickly gained popularity for its high speed and accuracy.
+
+- [YOLOv2](https://arxiv.org/abs/1612.08242), released in 2016, improved the original model by incorporating batch normalization, anchor boxes, and dimension clusters.
+- [YOLOv3](https://pjreddie.com/media/files/papers/YOLOv3.pdf), launched in 2018, further enhanced the model's performance using a more efficient backbone network, multiple anchors and spatial pyramid pooling.
+- [YOLOv4](https://arxiv.org/abs/2004.10934) was released in 2020, introducing innovations like Mosaic data augmentation, a new anchor-free detection head, and a new loss function.
+- [YOLOv5](https://github.com/ultralytics/yolov5) further improved the model's performance and added new features such as hyperparameter optimization, integrated experiment tracking and automatic export to popular export formats.
+- [YOLOv6](https://github.com/meituan/YOLOv6) was open-sourced by [Meituan](https://about.meituan.com/) in 2022 and is in use in many of the company's autonomous delivery robots.
+- [YOLOv7](https://github.com/WongKinYiu/yolov7) added additional tasks such as pose estimation on the COCO keypoints dataset.
+- [YOLOv8](https://github.com/ultralytics/ultralytics) is the latest version of YOLO by Ultralytics. As a cutting-edge, state-of-the-art (SOTA) model, YOLOv8 builds on the success of previous versions, introducing new features and improvements for enhanced performance, flexibility, and efficiency. YOLOv8 supports a full range of vision AI tasks, including [detection](tasks/detect.md), [segmentation](tasks/segment.md), [pose estimation](tasks/pose.md), [tracking](modes/track.md), and [classification](tasks/classify.md). This versatility allows users to leverage YOLOv8's capabilities across diverse applications and domains.
+
+## YOLO Licenses: How is Ultralytics YOLO licensed?
+
+Ultralytics YOLO repositories like YOLOv3, YOLOv5, or YOLOv8 are available under two different licenses:
+
+- **AGPL-3.0 License**: See [LICENSE](https://github.com/ultralytics/ultralytics/blob/main/LICENSE) file for details.
+- **Enterprise License**: Provides greater flexibility for commercial product development without the open-source requirements of AGPL-3.0. Typical use cases are embedding Ultralytics software and AI models in commercial products and applications. Request an Enterprise License at [Ultralytics Licensing](https://ultralytics.com/license).
+
+Please note our licensing approach ensures that any enhancements made to our open-source projects are shared back to the community. We firmly believe in the principles of open source, and we are committed to ensuring that our work can be used and improved upon in a manner that benefits everyone.
\ No newline at end of file
diff --git a/yolov8/docs/models/fast-sam.md b/yolov8/docs/models/fast-sam.md
new file mode 100644
index 0000000000000000000000000000000000000000..eefb5809361cba2b077236d47c402837e492849e
--- /dev/null
+++ b/yolov8/docs/models/fast-sam.md
@@ -0,0 +1,169 @@
+---
+comments: true
+description: Explore the Fast Segment Anything Model (FastSAM), a real-time solution for the segment anything task that leverages a Convolutional Neural Network (CNN) for segmenting any object within an image, guided by user interaction prompts.
+keywords: FastSAM, Segment Anything Model, SAM, Convolutional Neural Network, CNN, image segmentation, real-time image processing
+---
+
+# Fast Segment Anything Model (FastSAM)
+
+The Fast Segment Anything Model (FastSAM) is a novel, real-time CNN-based solution for the Segment Anything task. This task is designed to segment any object within an image based on various possible user interaction prompts. FastSAM significantly reduces computational demands while maintaining competitive performance, making it a practical choice for a variety of vision tasks.
+
+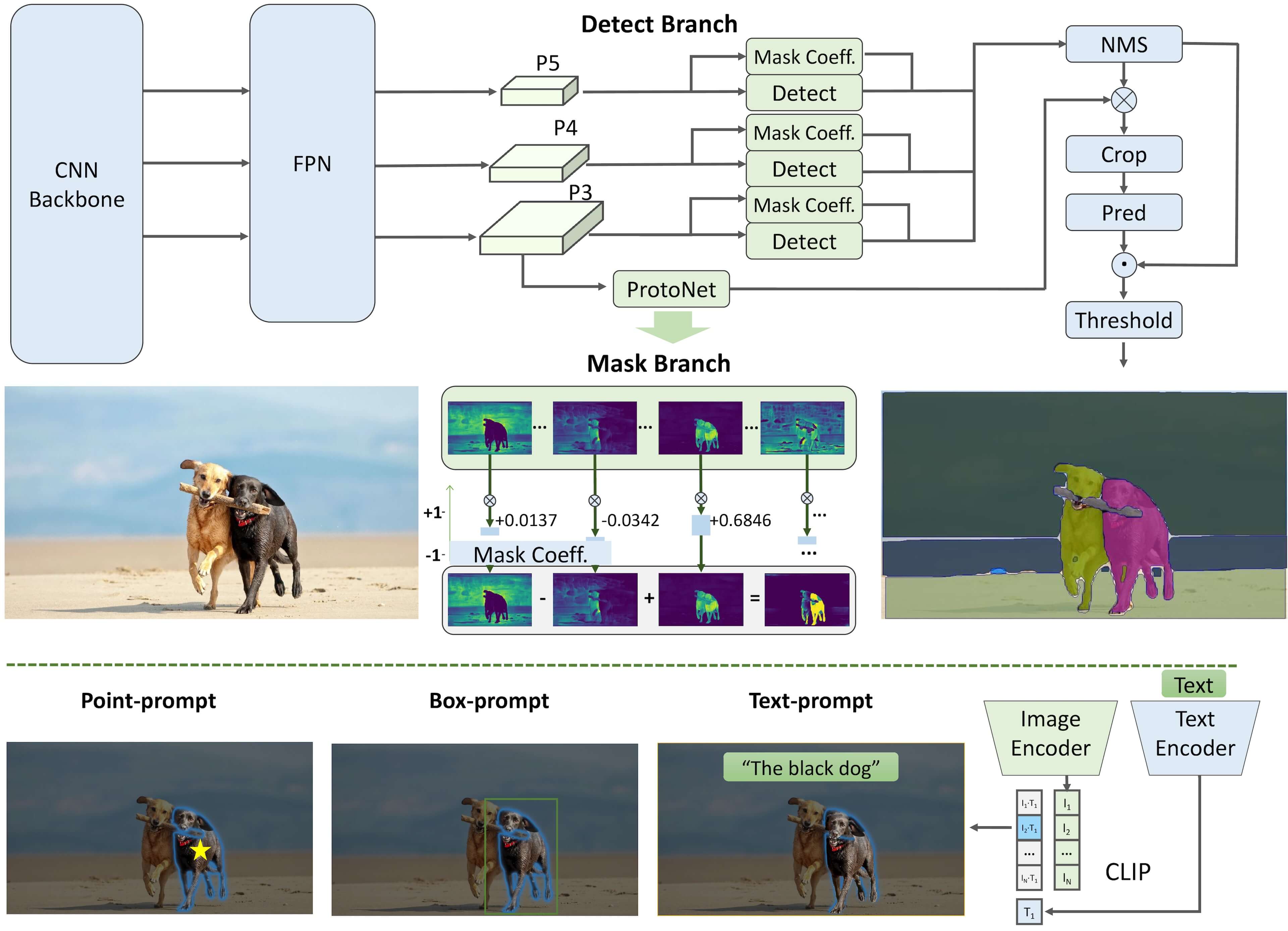
+
+## Overview
+
+FastSAM is designed to address the limitations of the [Segment Anything Model (SAM)](sam.md), a heavy Transformer model with substantial computational resource requirements. The FastSAM decouples the segment anything task into two sequential stages: all-instance segmentation and prompt-guided selection. The first stage uses [YOLOv8-seg](../tasks/segment.md) to produce the segmentation masks of all instances in the image. In the second stage, it outputs the region-of-interest corresponding to the prompt.
+
+## Key Features
+
+1. **Real-time Solution:** By leveraging the computational efficiency of CNNs, FastSAM provides a real-time solution for the segment anything task, making it valuable for industrial applications that require quick results.
+
+2. **Efficiency and Performance:** FastSAM offers a significant reduction in computational and resource demands without compromising on performance quality. It achieves comparable performance to SAM but with drastically reduced computational resources, enabling real-time application.
+
+3. **Prompt-guided Segmentation:** FastSAM can segment any object within an image guided by various possible user interaction prompts, providing flexibility and adaptability in different scenarios.
+
+4. **Based on YOLOv8-seg:** FastSAM is based on [YOLOv8-seg](../tasks/segment.md), an object detector equipped with an instance segmentation branch. This allows it to effectively produce the segmentation masks of all instances in an image.
+
+5. **Competitive Results on Benchmarks:** On the object proposal task on MS COCO, FastSAM achieves high scores at a significantly faster speed than [SAM](sam.md) on a single NVIDIA RTX 3090, demonstrating its efficiency and capability.
+
+6. **Practical Applications:** The proposed approach provides a new, practical solution for a large number of vision tasks at a really high speed, tens or hundreds of times faster than current methods.
+
+7. **Model Compression Feasibility:** FastSAM demonstrates the feasibility of a path that can significantly reduce the computational effort by introducing an artificial prior to the structure, thus opening new possibilities for large model architecture for general vision tasks.
+
+## Usage
+
+### Python API
+
+The FastSAM models are easy to integrate into your Python applications. Ultralytics provides a user-friendly Python API to streamline the process.
+
+#### Predict Usage
+
+To perform object detection on an image, use the `predict` method as shown below:
+
+```python
+from ultralytics import FastSAM
+from ultralytics.yolo.fastsam import FastSAMPrompt
+
+# Define image path and inference device
+IMAGE_PATH = 'ultralytics/assets/bus.jpg'
+DEVICE = 'cpu'
+
+# Create a FastSAM model
+model = FastSAM('FastSAM-s.pt') # or FastSAM-x.pt
+
+# Run inference on an image
+everything_results = model(IMAGE_PATH,
+ device=DEVICE,
+ retina_masks=True,
+ imgsz=1024,
+ conf=0.4,
+ iou=0.9)
+
+prompt_process = FastSAMPrompt(IMAGE_PATH, everything_results, device=DEVICE)
+
+# Everything prompt
+ann = prompt_process.everything_prompt()
+
+# Bbox default shape [0,0,0,0] -> [x1,y1,x2,y2]
+ann = prompt_process.box_prompt(bbox=[200, 200, 300, 300])
+
+# Text prompt
+ann = prompt_process.text_prompt(text='a photo of a dog')
+
+# Point prompt
+# points default [[0,0]] [[x1,y1],[x2,y2]]
+# point_label default [0] [1,0] 0:background, 1:foreground
+ann = prompt_process.point_prompt(points=[[200, 200]], pointlabel=[1])
+prompt_process.plot(annotations=ann, output='./')
+```
+
+This snippet demonstrates the simplicity of loading a pre-trained model and running a prediction on an image.
+
+#### Val Usage
+
+Validation of the model on a dataset can be done as follows:
+
+```python
+from ultralytics import FastSAM
+
+# Create a FastSAM model
+model = FastSAM('FastSAM-s.pt') # or FastSAM-x.pt
+
+# Validate the model
+results = model.val(data='coco8-seg.yaml')
+```
+
+Please note that FastSAM only supports detection and segmentation of a single class of object. This means it will recognize and segment all objects as the same class. Therefore, when preparing the dataset, you need to convert all object category IDs to 0.
+
+### FastSAM official Usage
+
+FastSAM is also available directly from the [https://github.com/CASIA-IVA-Lab/FastSAM](https://github.com/CASIA-IVA-Lab/FastSAM) repository. Here is a brief overview of the typical steps you might take to use FastSAM:
+
+#### Installation
+
+1. Clone the FastSAM repository:
+ ```shell
+ git clone https://github.com/CASIA-IVA-Lab/FastSAM.git
+ ```
+
+2. Create and activate a Conda environment with Python 3.9:
+ ```shell
+ conda create -n FastSAM python=3.9
+ conda activate FastSAM
+ ```
+
+3. Navigate to the cloned repository and install the required packages:
+ ```shell
+ cd FastSAM
+ pip install -r requirements.txt
+ ```
+
+4. Install the CLIP model:
+ ```shell
+ pip install git+https://github.com/openai/CLIP.git
+ ```
+
+#### Example Usage
+
+1. Download a [model checkpoint](https://drive.google.com/file/d/1m1sjY4ihXBU1fZXdQ-Xdj-mDltW-2Rqv/view?usp=sharing).
+
+2. Use FastSAM for inference. Example commands:
+
+ - Segment everything in an image:
+ ```shell
+ python Inference.py --model_path ./weights/FastSAM.pt --img_path ./images/dogs.jpg
+ ```
+
+ - Segment specific objects using text prompt:
+ ```shell
+ python Inference.py --model_path ./weights/FastSAM.pt --img_path ./images/dogs.jpg --text_prompt "the yellow dog"
+ ```
+
+ - Segment objects within a bounding box (provide box coordinates in xywh format):
+ ```shell
+ python Inference.py --model_path ./weights/FastSAM.pt --img_path ./images/dogs.jpg --box_prompt "[570,200,230,400]"
+ ```
+
+ - Segment objects near specific points:
+ ```shell
+ python Inference.py --model_path ./weights/FastSAM.pt --img_path ./images/dogs.jpg --point_prompt "[[520,360],[620,300]]" --point_label "[1,0]"
+ ```
+
+Additionally, you can try FastSAM through a [Colab demo](https://colab.research.google.com/drive/1oX14f6IneGGw612WgVlAiy91UHwFAvr9?usp=sharing) or on the [HuggingFace web demo](https://huggingface.co/spaces/An-619/FastSAM) for a visual experience.
+
+## Citations and Acknowledgements
+
+We would like to acknowledge the FastSAM authors for their significant contributions in the field of real-time instance segmentation:
+
+```bibtex
+@misc{zhao2023fast,
+ title={Fast Segment Anything},
+ author={Xu Zhao and Wenchao Ding and Yongqi An and Yinglong Du and Tao Yu and Min Li and Ming Tang and Jinqiao Wang},
+ year={2023},
+ eprint={2306.12156},
+ archivePrefix={arXiv},
+ primaryClass={cs.CV}
+}
+```
+
+The original FastSAM paper can be found on [arXiv](https://arxiv.org/abs/2306.12156). The authors have made their work publicly available, and the codebase can be accessed on [GitHub](https://github.com/CASIA-IVA-Lab/FastSAM). We appreciate their efforts in advancing the field and making their work accessible to the broader community.
diff --git a/yolov8/docs/models/index.md b/yolov8/docs/models/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..611cad7fba350d6e3b9f42bb4b0aaf0723c262c2
--- /dev/null
+++ b/yolov8/docs/models/index.md
@@ -0,0 +1,47 @@
+---
+comments: true
+description: Learn about the supported models and architectures, such as YOLOv3, YOLOv5, and YOLOv8, and how to contribute your own model to Ultralytics.
+keywords: Ultralytics YOLO, YOLOv3, YOLOv4, YOLOv5, YOLOv6, YOLOv7, YOLOv8, SAM, YOLO-NAS, RT-DETR, object detection, instance segmentation, detection transformers, real-time detection, computer vision, CLI, Python
+---
+
+# Models
+
+Ultralytics supports many models and architectures with more to come in the future. Want to add your model architecture? [Here's](../help/contributing.md) how you can contribute.
+
+In this documentation, we provide information on four major models:
+
+1. [YOLOv3](./yolov3.md): The third iteration of the YOLO model family originally by Joseph Redmon, known for its efficient real-time object detection capabilities.
+2. [YOLOv4](./yolov3.md): A darknet-native update to YOLOv3 released by Alexey Bochkovskiy in 2020.
+3. [YOLOv5](./yolov5.md): An improved version of the YOLO architecture by Ultralytics, offering better performance and speed tradeoffs compared to previous versions.
+4. [YOLOv6](./yolov6.md): Released by [Meituan](https://about.meituan.com/) in 2022 and is in use in many of the company's autonomous delivery robots.
+5. [YOLOv7](./yolov7.md): Updated YOLO models released in 2022 by the authors of YOLOv4.
+6. [YOLOv8](./yolov8.md): The latest version of the YOLO family, featuring enhanced capabilities such as instance segmentation, pose/keypoints estimation, and classification.
+7. [Segment Anything Model (SAM)](./sam.md): Meta's Segment Anything Model (SAM).
+8. [Fast Segment Anything Model (FastSAM)](./fast-sam.md): FastSAM by Image & Video Analysis Group, Institute of Automation, Chinese Academy of Sciences.
+9. [YOLO-NAS](./yolo-nas.md): YOLO Neural Architecture Search (NAS) Models.
+10. [Realtime Detection Transformers (RT-DETR)](./rtdetr.md): Baidu's PaddlePaddle Realtime Detection Transformer (RT-DETR) models.
+
+You can use many of these models directly in the Command Line Interface (CLI) or in a Python environment. Below are examples of how to use the models with CLI and Python:
+
+## CLI Example
+
+Use the `model` argument to pass a model YAML such as `model=yolov8n.yaml` or a pretrained *.pt file such as `model=yolov8n.pt`
+
+```bash
+yolo task=detect mode=train model=yolov8n.pt data=coco128.yaml epochs=100
+```
+
+## Python Example
+
+PyTorch pretrained models as well as model YAML files can also be passed to the `YOLO()`, `SAM()`, `NAS()` and `RTDETR()` classes to create a model instance in python:
+
+```python
+from ultralytics import YOLO
+
+model = YOLO("yolov8n.pt") # load a pretrained YOLOv8n model
+
+model.info() # display model information
+model.train(data="coco128.yaml", epochs=100) # train the model
+```
+
+For more details on each model, their supported tasks, modes, and performance, please visit their respective documentation pages linked above.
\ No newline at end of file
diff --git a/yolov8/docs/models/rtdetr.md b/yolov8/docs/models/rtdetr.md
new file mode 100644
index 0000000000000000000000000000000000000000..f2c65168c0b7f1672354f893eed3a1b6011049cd
--- /dev/null
+++ b/yolov8/docs/models/rtdetr.md
@@ -0,0 +1,74 @@
+---
+comments: true
+description: Dive into Baidu's RT-DETR, a revolutionary real-time object detection model built on the foundation of Vision Transformers (ViT). Learn how to use pre-trained PaddlePaddle RT-DETR models with the Ultralytics Python API for various tasks.
+keywords: RT-DETR, Transformer, ViT, Vision Transformers, Baidu RT-DETR, PaddlePaddle, Paddle Paddle RT-DETR, real-time object detection, Vision Transformers-based object detection, pre-trained PaddlePaddle RT-DETR models, Baidu's RT-DETR usage, Ultralytics Python API, object detector
+---
+
+# Baidu's RT-DETR: A Vision Transformer-Based Real-Time Object Detector
+
+## Overview
+
+Real-Time Detection Transformer (RT-DETR), developed by Baidu, is a cutting-edge end-to-end object detector that provides real-time performance while maintaining high accuracy. It leverages the power of Vision Transformers (ViT) to efficiently process multiscale features by decoupling intra-scale interaction and cross-scale fusion. RT-DETR is highly adaptable, supporting flexible adjustment of inference speed using different decoder layers without retraining. The model excels on accelerated backends like CUDA with TensorRT, outperforming many other real-time object detectors.
+
+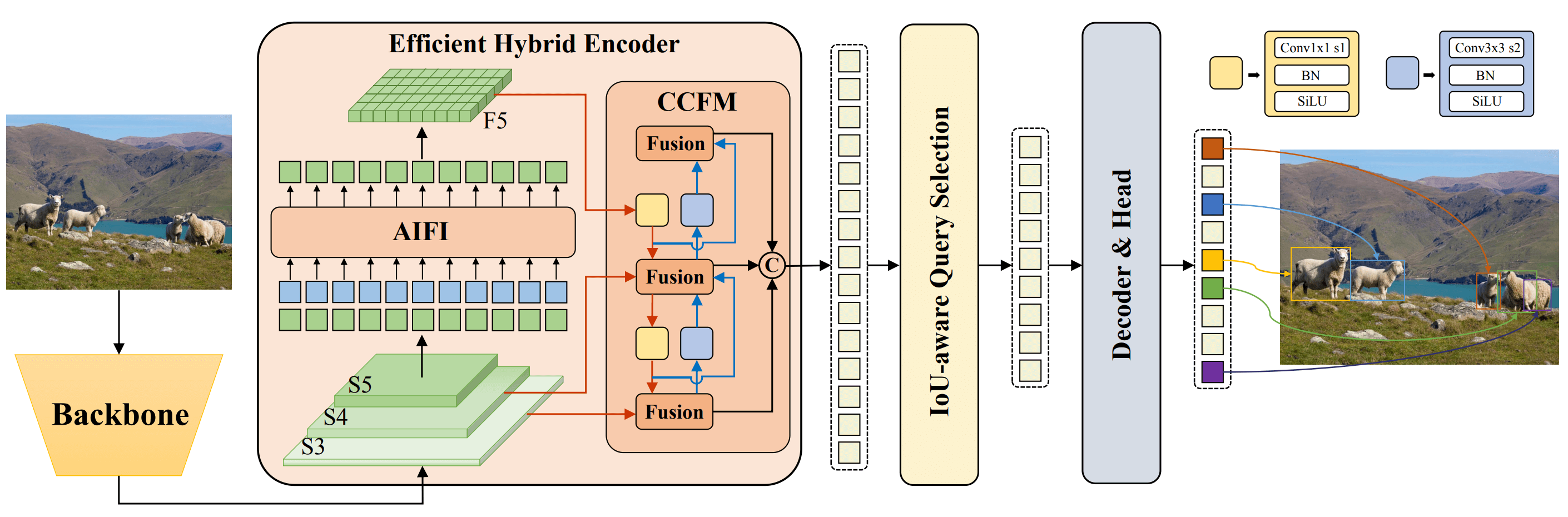
+**Overview of Baidu's RT-DETR.** The RT-DETR model architecture diagram shows the last three stages of the backbone {S3, S4, S5} as the input to the encoder. The efficient hybrid encoder transforms multiscale features into a sequence of image features through intrascale feature interaction (AIFI) and cross-scale feature-fusion module (CCFM). The IoU-aware query selection is employed to select a fixed number of image features to serve as initial object queries for the decoder. Finally, the decoder with auxiliary prediction heads iteratively optimizes object queries to generate boxes and confidence scores ([source](https://arxiv.org/pdf/2304.08069.pdf)).
+
+### Key Features
+
+- **Efficient Hybrid Encoder:** Baidu's RT-DETR uses an efficient hybrid encoder that processes multi-scale features by decoupling intra-scale interaction and cross-scale fusion. This unique Vision Transformers-based design reduces computational costs and allows for real-time object detection.
+- **IoU-aware Query Selection:** Baidu's RT-DETR improves object query initialization by utilizing IoU-aware query selection. This allows the model to focus on the most relevant objects in the scene, enhancing the detection accuracy.
+- **Adaptable Inference Speed:** Baidu's RT-DETR supports flexible adjustments of inference speed by using different decoder layers without the need for retraining. This adaptability facilitates practical application in various real-time object detection scenarios.
+
+## Pre-trained Models
+
+The Ultralytics Python API provides pre-trained PaddlePaddle RT-DETR models with different scales:
+
+- RT-DETR-L: 53.0% AP on COCO val2017, 114 FPS on T4 GPU
+- RT-DETR-X: 54.8% AP on COCO val2017, 74 FPS on T4 GPU
+
+## Usage
+
+### Python API
+
+```python
+from ultralytics import RTDETR
+
+model = RTDETR("rtdetr-l.pt")
+model.info() # display model information
+model.train(data="coco8.yaml") # train
+model.predict("path/to/image.jpg") # predict
+```
+
+### Supported Tasks
+
+| Model Type | Pre-trained Weights | Tasks Supported |
+|---------------------|---------------------|------------------|
+| RT-DETR Large | `rtdetr-l.pt` | Object Detection |
+| RT-DETR Extra-Large | `rtdetr-x.pt` | Object Detection |
+
+### Supported Modes
+
+| Mode | Supported |
+|------------|--------------------|
+| Inference | :heavy_check_mark: |
+| Validation | :heavy_check_mark: |
+| Training | :heavy_check_mark: |
+
+# Citations and Acknowledgements
+
+If you use Baidu's RT-DETR in your research or development work, please cite the [original paper](https://arxiv.org/abs/2304.08069):
+
+```bibtex
+@misc{lv2023detrs,
+ title={DETRs Beat YOLOs on Real-time Object Detection},
+ author={Wenyu Lv and Shangliang Xu and Yian Zhao and Guanzhong Wang and Jinman Wei and Cheng Cui and Yuning Du and Qingqing Dang and Yi Liu},
+ year={2023},
+ eprint={2304.08069},
+ archivePrefix={arXiv},
+ primaryClass={cs.CV}
+}
+```
+
+We would like to acknowledge Baidu and the [PaddlePaddle](https://github.com/PaddlePaddle/PaddleDetection) team for creating and maintaining this valuable resource for the computer vision community. Their contribution to the field with the development of the Vision Transformers-based real-time object detector, RT-DETR, is greatly appreciated.
+
+*Keywords: RT-DETR, Transformer, ViT, Vision Transformers, Baidu RT-DETR, PaddlePaddle, Paddle Paddle RT-DETR, real-time object detection, Vision Transformers-based object detection, pre-trained PaddlePaddle RT-DETR models, Baidu's RT-DETR usage, Ultralytics Python API*
diff --git a/yolov8/docs/models/sam.md b/yolov8/docs/models/sam.md
new file mode 100644
index 0000000000000000000000000000000000000000..8dd1e35c24b19ccc21ece951b8de4bf85957b240
--- /dev/null
+++ b/yolov8/docs/models/sam.md
@@ -0,0 +1,99 @@
+---
+comments: true
+description: Discover the Segment Anything Model (SAM), a revolutionary promptable image segmentation model, and delve into the details of its advanced architecture and the large-scale SA-1B dataset.
+keywords: Segment Anything, Segment Anything Model, SAM, Meta SAM, image segmentation, promptable segmentation, zero-shot performance, SA-1B dataset, advanced architecture, auto-annotation, Ultralytics, pre-trained models, SAM base, SAM large, instance segmentation, computer vision, AI, artificial intelligence, machine learning, data annotation, segmentation masks, detection model, YOLO detection model, bibtex, Meta AI
+---
+
+# Segment Anything Model (SAM)
+
+Welcome to the frontier of image segmentation with the Segment Anything Model, or SAM. This revolutionary model has changed the game by introducing promptable image segmentation with real-time performance, setting new standards in the field.
+
+## Introduction to SAM: The Segment Anything Model
+
+The Segment Anything Model, or SAM, is a cutting-edge image segmentation model that allows for promptable segmentation, providing unparalleled versatility in image analysis tasks. SAM forms the heart of the Segment Anything initiative, a groundbreaking project that introduces a novel model, task, and dataset for image segmentation.
+
+SAM's advanced design allows it to adapt to new image distributions and tasks without prior knowledge, a feature known as zero-shot transfer. Trained on the expansive [SA-1B dataset](https://ai.facebook.com/datasets/segment-anything/), which contains more than 1 billion masks spread over 11 million carefully curated images, SAM has displayed impressive zero-shot performance, surpassing previous fully supervised results in many cases.
+
+
+Example images with overlaid masks from our newly introduced dataset, SA-1B. SA-1B contains 11M diverse, high-resolution, licensed, and privacy protecting images and 1.1B high-quality segmentation masks. These masks were annotated fully automatically by SAM, and as verified by human ratings and numerous experiments, are of high quality and diversity. Images are grouped by number of masks per image for visualization (there are ∼100 masks per image on average).
+
+## Key Features of the Segment Anything Model (SAM)
+
+- **Promptable Segmentation Task:** SAM was designed with a promptable segmentation task in mind, allowing it to generate valid segmentation masks from any given prompt, such as spatial or text clues identifying an object.
+- **Advanced Architecture:** The Segment Anything Model employs a powerful image encoder, a prompt encoder, and a lightweight mask decoder. This unique architecture enables flexible prompting, real-time mask computation, and ambiguity awareness in segmentation tasks.
+- **The SA-1B Dataset:** Introduced by the Segment Anything project, the SA-1B dataset features over 1 billion masks on 11 million images. As the largest segmentation dataset to date, it provides SAM with a diverse and large-scale training data source.
+- **Zero-Shot Performance:** SAM displays outstanding zero-shot performance across various segmentation tasks, making it a ready-to-use tool for diverse applications with minimal need for prompt engineering.
+
+For an in-depth look at the Segment Anything Model and the SA-1B dataset, please visit the [Segment Anything website](https://segment-anything.com) and check out the research paper [Segment Anything](https://arxiv.org/abs/2304.02643).
+
+## How to Use SAM: Versatility and Power in Image Segmentation
+
+The Segment Anything Model can be employed for a multitude of downstream tasks that go beyond its training data. This includes edge detection, object proposal generation, instance segmentation, and preliminary text-to-mask prediction. With prompt engineering, SAM can swiftly adapt to new tasks and data distributions in a zero-shot manner, establishing it as a versatile and potent tool for all your image segmentation needs.
+
+```python
+from ultralytics import SAM
+
+model = SAM('sam_b.pt')
+model.info() # display model information
+model.predict('path/to/image.jpg') # predict
+```
+
+## Available Models and Supported Tasks
+
+| Model Type | Pre-trained Weights | Tasks Supported |
+|------------|---------------------|-----------------------|
+| SAM base | `sam_b.pt` | Instance Segmentation |
+| SAM large | `sam_l.pt` | Instance Segmentation |
+
+## Operating Modes
+
+| Mode | Supported |
+|------------|--------------------|
+| Inference | :heavy_check_mark: |
+| Validation | :x: |
+| Training | :x: |
+
+## Auto-Annotation: A Quick Path to Segmentation Datasets
+
+Auto-annotation is a key feature of SAM, allowing users to generate a [segmentation dataset](https://docs.ultralytics.com/datasets/segment) using a pre-trained detection model. This feature enables rapid and accurate annotation of a large number of images, bypassing the need for time-consuming manual labeling.
+
+### Generate Your Segmentation Dataset Using a Detection Model
+
+To auto-annotate your dataset with the Ultralytics framework, use the `auto_annotate` function as shown below:
+
+```python
+from ultralytics.yolo.data.annotator import auto_annotate
+
+auto_annotate(data="path/to/images", det_model="yolov8x.pt", sam_model='sam_b.pt')
+```
+
+| Argument | Type | Description | Default |
+|------------|---------------------|---------------------------------------------------------------------------------------------------------|--------------|
+| data | str | Path to a folder containing images to be annotated. | |
+| det_model | str, optional | Pre-trained YOLO detection model. Defaults to 'yolov8x.pt'. | 'yolov8x.pt' |
+| sam_model | str, optional | Pre-trained SAM segmentation model. Defaults to 'sam_b.pt'. | 'sam_b.pt' |
+| device | str, optional | Device to run the models on. Defaults to an empty string (CPU or GPU, if available). | |
+| output_dir | str, None, optional | Directory to save the annotated results. Defaults to a 'labels' folder in the same directory as 'data'. | None |
+
+The `auto_annotate` function takes the path to your images, with optional arguments for specifying the pre-trained detection and SAM segmentation models, the device to run the models on, and the output directory for saving the annotated results.
+
+Auto-annotation with pre-trained models can dramatically cut down the time and effort required for creating high-quality segmentation datasets. This feature is especially beneficial for researchers and developers dealing with large image collections, as it allows them to focus on model development and evaluation rather than manual annotation.
+
+## Citations and Acknowledgements
+
+If you find SAM useful in your research or development work, please consider citing our paper:
+
+```bibtex
+@misc{kirillov2023segment,
+ title={Segment Anything},
+ author={Alexander Kirillov and Eric Mintun and Nikhila Ravi and Hanzi Mao and Chloe Rolland and Laura Gustafson and Tete Xiao and Spencer Whitehead and Alexander C. Berg and Wan-Yen Lo and Piotr Dollár and Ross Girshick},
+ year={2023},
+ eprint={2304.02643},
+ archivePrefix={arXiv},
+ primaryClass={cs.CV}
+}
+```
+
+We would like to express our gratitude to Meta AI for creating and maintaining this valuable resource for the computer vision community.
+
+*keywords: Segment Anything, Segment Anything Model, SAM, Meta SAM, image segmentation, promptable segmentation, zero-shot performance, SA-1B dataset, advanced architecture, auto-annotation, Ultralytics, pre-trained models, SAM base, SAM large, instance segmentation, computer vision, AI, artificial intelligence, machine learning, data annotation, segmentation masks, detection model, YOLO detection model, bibtex, Meta AI.*
\ No newline at end of file
diff --git a/yolov8/docs/models/yolo-nas.md b/yolov8/docs/models/yolo-nas.md
new file mode 100644
index 0000000000000000000000000000000000000000..4ce38e85a048e6b9896ea497aec26880d04ed4f2
--- /dev/null
+++ b/yolov8/docs/models/yolo-nas.md
@@ -0,0 +1,109 @@
+---
+comments: true
+description: Dive into YOLO-NAS, Deci's next-generation object detection model, offering breakthroughs in speed and accuracy. Learn how to utilize pre-trained models using the Ultralytics Python API for various tasks.
+keywords: YOLO-NAS, Deci AI, Ultralytics, object detection, deep learning, neural architecture search, Python API, pre-trained models, quantization
+---
+
+# YOLO-NAS
+
+## Overview
+
+Developed by Deci AI, YOLO-NAS is a groundbreaking object detection foundational model. It is the product of advanced Neural Architecture Search technology, meticulously designed to address the limitations of previous YOLO models. With significant improvements in quantization support and accuracy-latency trade-offs, YOLO-NAS represents a major leap in object detection.
+
+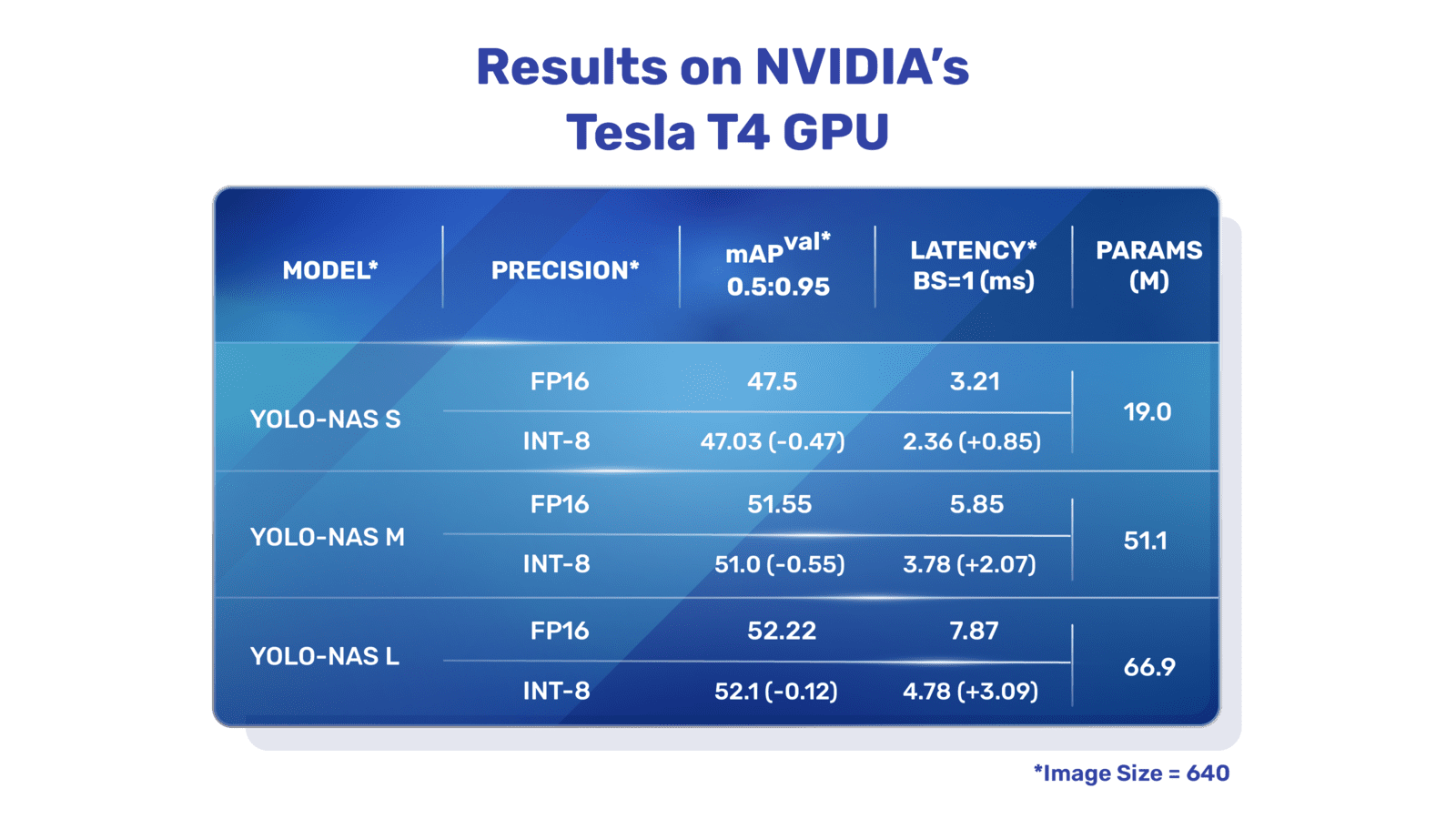
+**Overview of YOLO-NAS.** YOLO-NAS employs quantization-aware blocks and selective quantization for optimal performance. The model, when converted to its INT8 quantized version, experiences a minimal precision drop, a significant improvement over other models. These advancements culminate in a superior architecture with unprecedented object detection capabilities and outstanding performance.
+
+### Key Features
+
+- **Quantization-Friendly Basic Block:** YOLO-NAS introduces a new basic block that is friendly to quantization, addressing one of the significant limitations of previous YOLO models.
+- **Sophisticated Training and Quantization:** YOLO-NAS leverages advanced training schemes and post-training quantization to enhance performance.
+- **AutoNAC Optimization and Pre-training:** YOLO-NAS utilizes AutoNAC optimization and is pre-trained on prominent datasets such as COCO, Objects365, and Roboflow 100. This pre-training makes it extremely suitable for downstream object detection tasks in production environments.
+
+## Pre-trained Models
+
+Experience the power of next-generation object detection with the pre-trained YOLO-NAS models provided by Ultralytics. These models are designed to deliver top-notch performance in terms of both speed and accuracy. Choose from a variety of options tailored to your specific needs:
+
+| Model | mAP | Latency (ms) |
+|------------------|-------|--------------|
+| YOLO-NAS S | 47.5 | 3.21 |
+| YOLO-NAS M | 51.55 | 5.85 |
+| YOLO-NAS L | 52.22 | 7.87 |
+| YOLO-NAS S INT-8 | 47.03 | 2.36 |
+| YOLO-NAS M INT-8 | 51.0 | 3.78 |
+| YOLO-NAS L INT-8 | 52.1 | 4.78 |
+
+Each model variant is designed to offer a balance between Mean Average Precision (mAP) and latency, helping you optimize your object detection tasks for both performance and speed.
+
+## Usage
+
+### Python API
+
+The YOLO-NAS models are easy to integrate into your Python applications. Ultralytics provides a user-friendly Python API to streamline the process.
+
+#### Predict Usage
+
+To perform object detection on an image, use the `predict` method as shown below:
+
+```python
+from ultralytics import NAS
+
+model = NAS('yolo_nas_s')
+results = model.predict('ultralytics/assets/bus.jpg')
+```
+
+This snippet demonstrates the simplicity of loading a pre-trained model and running a prediction on an image.
+
+#### Val Usage
+
+Validation of the model on a dataset can be done as follows:
+
+```python
+from ultralytics import NAS
+
+model = NAS('yolo_nas_s')
+results = model.val(data='coco8.yaml)
+```
+
+In this example, the model is validated against the dataset specified in the 'coco8.yaml' file.
+
+### Supported Tasks
+
+The YOLO-NAS models are primarily designed for object detection tasks. You can download the pre-trained weights for each variant of the model as follows:
+
+| Model Type | Pre-trained Weights | Tasks Supported |
+|------------|-----------------------------------------------------------------------------------------------|------------------|
+| YOLO-NAS-s | [yolo_nas_s.pt](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolo_nas_s.pt) | Object Detection |
+| YOLO-NAS-m | [yolo_nas_m.pt](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolo_nas_m.pt) | Object Detection |
+| YOLO-NAS-l | [yolo_nas_l.pt](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolo_nas_l.pt) | Object Detection |
+
+### Supported Modes
+
+The YOLO-NAS models support both inference and validation modes, allowing you to predict and validate results with ease. Training mode, however, is currently not supported.
+
+| Mode | Supported |
+|------------|--------------------|
+| Inference | :heavy_check_mark: |
+| Validation | :heavy_check_mark: |
+| Training | :x: |
+
+Harness the power of the YOLO-NAS models to drive your object detection tasks to new heights of performance and speed.
+
+## Acknowledgements and Citations
+
+If you employ YOLO-NAS in your research or development work, please cite SuperGradients:
+
+```bibtex
+@misc{supergradients,
+ doi = {10.5281/ZENODO.7789328},
+ url = {https://zenodo.org/record/7789328},
+ author = {Aharon, Shay and {Louis-Dupont} and {Ofri Masad} and Yurkova, Kate and {Lotem Fridman} and {Lkdci} and Khvedchenya, Eugene and Rubin, Ran and Bagrov, Natan and Tymchenko, Borys and Keren, Tomer and Zhilko, Alexander and {Eran-Deci}},
+ title = {Super-Gradients},
+ publisher = {GitHub},
+ journal = {GitHub repository},
+ year = {2021},
+}
+```
+
+We express our gratitude to Deci AI's [SuperGradients](https://github.com/Deci-AI/super-gradients/) team for their efforts in creating and maintaining this valuable resource for the computer vision community. We believe YOLO-NAS, with its innovative architecture and superior object detection capabilities, will become a critical tool for developers and researchers alike.
+
+*Keywords: YOLO-NAS, Deci AI, object detection, deep learning, neural architecture search, Ultralytics Python API, YOLO model, SuperGradients, pre-trained models, quantization-friendly basic block, advanced training schemes, post-training quantization, AutoNAC optimization, COCO, Objects365, Roboflow 100*
\ No newline at end of file
diff --git a/yolov8/docs/models/yolov3.md b/yolov8/docs/models/yolov3.md
new file mode 100644
index 0000000000000000000000000000000000000000..da1415e06064546fa13aad1af5c937d6bf8124f7
--- /dev/null
+++ b/yolov8/docs/models/yolov3.md
@@ -0,0 +1,80 @@
+---
+comments: true
+description: YOLOv3, YOLOv3-Ultralytics and YOLOv3u by Ultralytics explained. Learn the evolution of these models and their specifications.
+keywords: YOLOv3, Ultralytics YOLOv3, YOLO v3, YOLOv3 models, object detection, models, machine learning, AI, image recognition, object recognition
+---
+
+# YOLOv3, YOLOv3-Ultralytics, and YOLOv3u
+
+## Overview
+
+This document presents an overview of three closely related object detection models, namely [YOLOv3](https://pjreddie.com/darknet/yolo/), [YOLOv3-Ultralytics](https://github.com/ultralytics/yolov3), and [YOLOv3u](https://github.com/ultralytics/ultralytics).
+
+1. **YOLOv3:** This is the third version of the You Only Look Once (YOLO) object detection algorithm. Originally developed by Joseph Redmon, YOLOv3 improved on its predecessors by introducing features such as multiscale predictions and three different sizes of detection kernels.
+
+2. **YOLOv3-Ultralytics:** This is Ultralytics' implementation of the YOLOv3 model. It reproduces the original YOLOv3 architecture and offers additional functionalities, such as support for more pre-trained models and easier customization options.
+
+3. **YOLOv3u:** This is an updated version of YOLOv3-Ultralytics that incorporates the anchor-free, objectness-free split head used in YOLOv8 models. YOLOv3u maintains the same backbone and neck architecture as YOLOv3 but with the updated detection head from YOLOv8.
+
+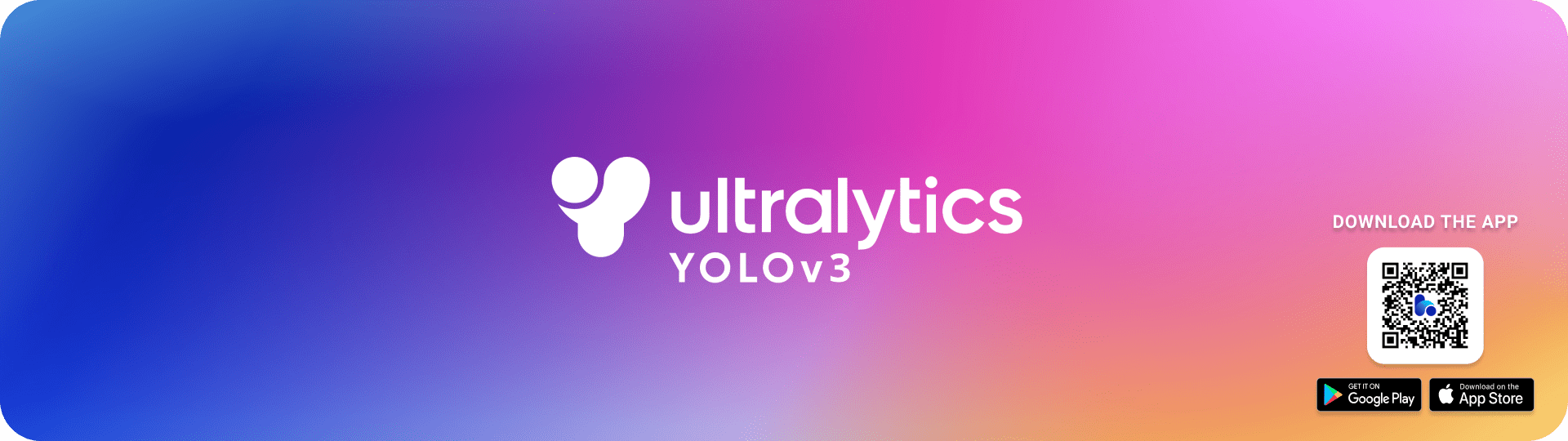
+
+## Key Features
+
+- **YOLOv3:** Introduced the use of three different scales for detection, leveraging three different sizes of detection kernels: 13x13, 26x26, and 52x52. This significantly improved detection accuracy for objects of different sizes. Additionally, YOLOv3 added features such as multi-label predictions for each bounding box and a better feature extractor network.
+
+- **YOLOv3-Ultralytics:** Ultralytics' implementation of YOLOv3 provides the same performance as the original model but comes with added support for more pre-trained models, additional training methods, and easier customization options. This makes it more versatile and user-friendly for practical applications.
+
+- **YOLOv3u:** This updated model incorporates the anchor-free, objectness-free split head from YOLOv8. By eliminating the need for pre-defined anchor boxes and objectness scores, this detection head design can improve the model's ability to detect objects of varying sizes and shapes. This makes YOLOv3u more robust and accurate for object detection tasks.
+
+## Supported Tasks
+
+YOLOv3, YOLOv3-Ultralytics, and YOLOv3u all support the following tasks:
+
+- Object Detection
+
+## Supported Modes
+
+All three models support the following modes:
+
+- Inference
+- Validation
+- Training
+- Export
+
+## Performance
+
+Below is a comparison of the performance of the three models. The performance is measured in terms of the Mean Average Precision (mAP) on the COCO dataset:
+
+TODO
+
+## Usage
+
+You can use these models for object detection tasks using the Ultralytics YOLOv3 repository. The following is a sample code snippet showing how to use the YOLOv3u model for inference:
+
+```python
+from ultralytics import YOLO
+
+# Load the model
+model = YOLO('yolov3.pt') # load a pretrained model
+
+# Perform inference
+results = model('image.jpg')
+
+# Print the results
+results.print()
+```
+
+## Citations and Acknowledgments
+
+If you use YOLOv3 in your research, please cite the original YOLO papers and the Ultralytics YOLOv3 repository:
+
+```bibtex
+@article{redmon2018yolov3,
+ title={YOLOv3: An Incremental Improvement},
+ author={Redmon, Joseph and Farhadi, Ali},
+ journal={arXiv preprint arXiv:1804.02767},
+ year={2018}
+}
+```
+
+Thank you to Joseph Redmon and Ali Farhadi for developing the original YOLOv3.
\ No newline at end of file
diff --git a/yolov8/docs/models/yolov4.md b/yolov8/docs/models/yolov4.md
new file mode 100644
index 0000000000000000000000000000000000000000..36a09ccba9077da5538063bd6bfe78dbdd0f95ac
--- /dev/null
+++ b/yolov8/docs/models/yolov4.md
@@ -0,0 +1,67 @@
+---
+comments: true
+description: Explore YOLOv4, a state-of-the-art, real-time object detector. Learn about its architecture, features, and performance.
+keywords: YOLOv4, object detection, real-time, CNN, GPU, Ultralytics, documentation, YOLOv4 architecture, YOLOv4 features, YOLOv4 performance
+---
+
+# YOLOv4: High-Speed and Precise Object Detection
+
+Welcome to the Ultralytics documentation page for YOLOv4, a state-of-the-art, real-time object detector launched in 2020 by Alexey Bochkovskiy at [https://github.com/AlexeyAB/darknet](https://github.com/AlexeyAB/darknet). YOLOv4 is designed to provide the optimal balance between speed and accuracy, making it an excellent choice for many applications.
+
+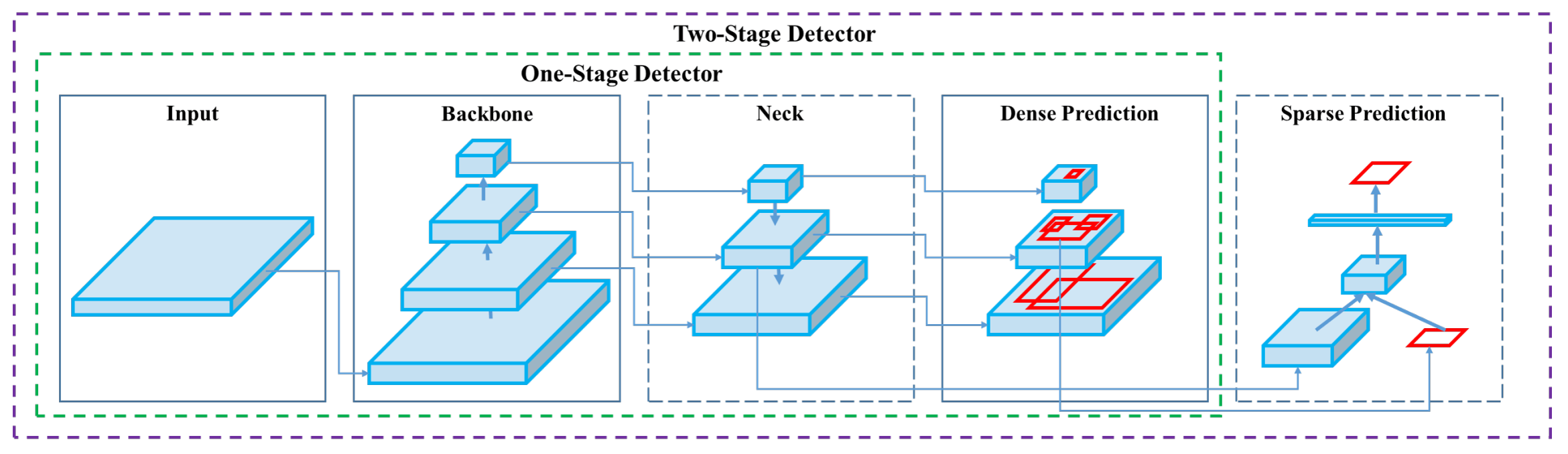
+**YOLOv4 architecture diagram**. Showcasing the intricate network design of YOLOv4, including the backbone, neck, and head components, and their interconnected layers for optimal real-time object detection.
+
+## Introduction
+
+YOLOv4 stands for You Only Look Once version 4. It is a real-time object detection model developed to address the limitations of previous YOLO versions like [YOLOv3](./yolov3.md) and other object detection models. Unlike other convolutional neural network (CNN) based object detectors, YOLOv4 is not only applicable for recommendation systems but also for standalone process management and human input reduction. Its operation on conventional graphics processing units (GPUs) allows for mass usage at an affordable price, and it is designed to work in real-time on a conventional GPU while requiring only one such GPU for training.
+
+## Architecture
+
+YOLOv4 makes use of several innovative features that work together to optimize its performance. These include Weighted-Residual-Connections (WRC), Cross-Stage-Partial-connections (CSP), Cross mini-Batch Normalization (CmBN), Self-adversarial-training (SAT), Mish-activation, Mosaic data augmentation, DropBlock regularization, and CIoU loss. These features are combined to achieve state-of-the-art results.
+
+A typical object detector is composed of several parts including the input, the backbone, the neck, and the head. The backbone of YOLOv4 is pre-trained on ImageNet and is used to predict classes and bounding boxes of objects. The backbone could be from several models including VGG, ResNet, ResNeXt, or DenseNet. The neck part of the detector is used to collect feature maps from different stages and usually includes several bottom-up paths and several top-down paths. The head part is what is used to make the final object detections and classifications.
+
+## Bag of Freebies
+
+YOLOv4 also makes use of methods known as "bag of freebies," which are techniques that improve the accuracy of the model during training without increasing the cost of inference. Data augmentation is a common bag of freebies technique used in object detection, which increases the variability of the input images to improve the robustness of the model. Some examples of data augmentation include photometric distortions (adjusting the brightness, contrast, hue, saturation, and noise of an image) and geometric distortions (adding random scaling, cropping, flipping, and rotating). These techniques help the model to generalize better to different types of images.
+
+## Features and Performance
+
+YOLOv4 is designed for optimal speed and accuracy in object detection. The architecture of YOLOv4 includes CSPDarknet53 as the backbone, PANet as the neck, and YOLOv3 as the detection head. This design allows YOLOv4 to perform object detection at an impressive speed, making it suitable for real-time applications. YOLOv4 also excels in accuracy, achieving state-of-the-art results in object detection benchmarks.
+
+## Usage Examples
+
+As of the time of writing, Ultralytics does not currently support YOLOv4 models. Therefore, any users interested in using YOLOv4 will need to refer directly to the YOLOv4 GitHub repository for installation and usage instructions.
+
+Here is a brief overview of the typical steps you might take to use YOLOv4:
+
+1. Visit the YOLOv4 GitHub repository: [https://github.com/AlexeyAB/darknet](https://github.com/AlexeyAB/darknet).
+
+2. Follow the instructions provided in the README file for installation. This typically involves cloning the repository, installing necessary dependencies, and setting up any necessary environment variables.
+
+3. Once installation is complete, you can train and use the model as per the usage instructions provided in the repository. This usually involves preparing your dataset, configuring the model parameters, training the model, and then using the trained model to perform object detection.
+
+Please note that the specific steps may vary depending on your specific use case and the current state of the YOLOv4 repository. Therefore, it is strongly recommended to refer directly to the instructions provided in the YOLOv4 GitHub repository.
+
+We regret any inconvenience this may cause and will strive to update this document with usage examples for Ultralytics once support for YOLOv4 is implemented.
+
+## Conclusion
+
+YOLOv4 is a powerful and efficient object detection model that strikes a balance between speed and accuracy. Its use of unique features and bag of freebies techniques during training allows it to perform excellently in real-time object detection tasks. YOLOv4 can be trained and used by anyone with a conventional GPU, making it accessible and practical for a wide range of applications.
+
+## Citations and Acknowledgements
+
+We would like to acknowledge the YOLOv4 authors for their significant contributions in the field of real-time object detection:
+
+```bibtex
+@misc{bochkovskiy2020yolov4,
+ title={YOLOv4: Optimal Speed and Accuracy of Object Detection},
+ author={Alexey Bochkovskiy and Chien-Yao Wang and Hong-Yuan Mark Liao},
+ year={2020},
+ eprint={2004.10934},
+ archivePrefix={arXiv},
+ primaryClass={cs.CV}
+}
+```
+
+The original YOLOv4 paper can be found on [arXiv](https://arxiv.org/pdf/2004.10934.pdf). The authors have made their work publicly available, and the codebase can be accessed on [GitHub](https://github.com/AlexeyAB/darknet). We appreciate their efforts in advancing the field and making their work accessible to the broader community.
\ No newline at end of file
diff --git a/yolov8/docs/models/yolov5.md b/yolov8/docs/models/yolov5.md
new file mode 100644
index 0000000000000000000000000000000000000000..884fea6bc7a118aa20357f24b16dbacd6e9e12b0
--- /dev/null
+++ b/yolov8/docs/models/yolov5.md
@@ -0,0 +1,89 @@
+---
+comments: true
+description: YOLOv5 by Ultralytics explained. Discover the evolution of this model and its key specifications. Experience faster and more accurate object detection.
+keywords: YOLOv5, Ultralytics YOLOv5, YOLO v5, YOLOv5 models, YOLO, object detection, model, neural network, accuracy, speed, pre-trained weights, inference, validation, training
+---
+
+# YOLOv5
+
+## Overview
+
+YOLOv5u is an enhanced version of the [YOLOv5](https://github.com/ultralytics/yolov5) object detection model from Ultralytics. This iteration incorporates the anchor-free, objectness-free split head that is featured in the [YOLOv8](./yolov8.md) models. Although it maintains the same backbone and neck architecture as YOLOv5, YOLOv5u provides an improved accuracy-speed tradeoff for object detection tasks, making it a robust choice for numerous applications.
+
+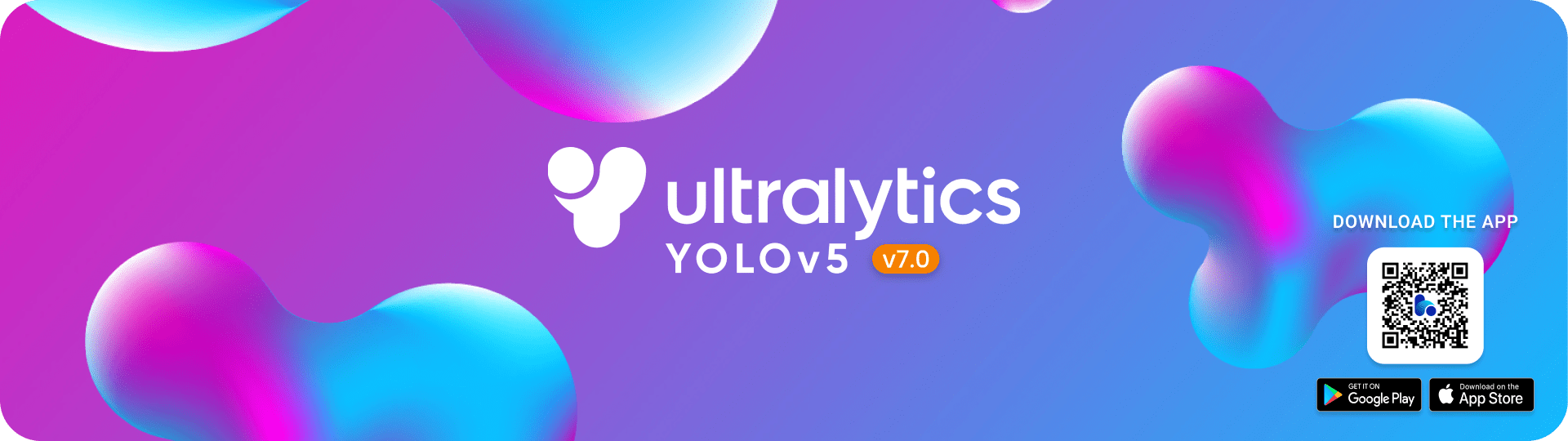
+
+## Key Features
+
+- **Anchor-free Split Ultralytics Head:** YOLOv5u replaces the conventional anchor-based detection head with an anchor-free split Ultralytics head, boosting performance in object detection tasks.
+
+- **Optimized Accuracy-Speed Tradeoff:** By delivering a better balance between accuracy and speed, YOLOv5u is suitable for a diverse range of real-time applications, from autonomous driving to video surveillance.
+
+- **Variety of Pre-trained Models:** YOLOv5u includes numerous pre-trained models for tasks like Inference, Validation, and Training, providing the flexibility to tackle various object detection challenges.
+
+## Supported Tasks
+
+| Model Type | Pre-trained Weights | Task |
+|------------|-----------------------------------------------------------------------------------------------------------------------------|-----------|
+| YOLOv5u | `yolov5nu`, `yolov5su`, `yolov5mu`, `yolov5lu`, `yolov5xu`, `yolov5n6u`, `yolov5s6u`, `yolov5m6u`, `yolov5l6u`, `yolov5x6u` | Detection |
+
+## Supported Modes
+
+| Mode | Supported |
+|------------|--------------------|
+| Inference | :heavy_check_mark: |
+| Validation | :heavy_check_mark: |
+| Training | :heavy_check_mark: |
+
+!!! Performance
+
+ === "Detection"
+
+ | Model | size
(pixels) | mAPval
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+ | ---------------------------------------------------------------------------------------- | --------------------- | -------------------- | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+ | [YOLOv5nu](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5nu.pt) | 640 | 34.3 | 73.6 | 1.06 | 2.6 | 7.7 |
+ | [YOLOv5su](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5su.pt) | 640 | 43.0 | 120.7 | 1.27 | 9.1 | 24.0 |
+ | [YOLOv5mu](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5mu.pt) | 640 | 49.0 | 233.9 | 1.86 | 25.1 | 64.2 |
+ | [YOLOv5lu](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5lu.pt) | 640 | 52.2 | 408.4 | 2.50 | 53.2 | 135.0 |
+ | [YOLOv5xu](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5xu.pt) | 640 | 53.2 | 763.2 | 3.81 | 97.2 | 246.4 |
+ | | | | | | | |
+ | [YOLOv5n6u](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5n6u.pt) | 1280 | 42.1 | 211.0 | 1.83 | 4.3 | 7.8 |
+ | [YOLOv5s6u](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5s6u.pt) | 1280 | 48.6 | 422.6 | 2.34 | 15.3 | 24.6 |
+ | [YOLOv5m6u](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5m6u.pt) | 1280 | 53.6 | 810.9 | 4.36 | 41.2 | 65.7 |
+ | [YOLOv5l6u](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5l6u.pt) | 1280 | 55.7 | 1470.9 | 5.47 | 86.1 | 137.4 |
+ | [YOLOv5x6u](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov5x6u.pt) | 1280 | 56.8 | 2436.5 | 8.98 | 155.4 | 250.7 |
+
+## Usage
+
+You can use YOLOv5u for object detection tasks using the Ultralytics repository. The following is a sample code snippet showing how to use YOLOv5u model for inference:
+
+```python
+from ultralytics import YOLO
+
+# Load the model
+model = YOLO('yolov5n.pt') # load a pretrained model
+
+# Perform inference
+results = model('image.jpg')
+
+# Print the results
+results.print()
+```
+
+## Citations and Acknowledgments
+
+If you use YOLOv5 or YOLOv5u in your research, please cite the Ultralytics YOLOv5 repository as follows:
+
+```bibtex
+@software{yolov5,
+ title = {Ultralytics YOLOv5},
+ author = {Glenn Jocher},
+ year = {2020},
+ version = {7.0},
+ license = {AGPL-3.0},
+ url = {https://github.com/ultralytics/yolov5},
+ doi = {10.5281/zenodo.3908559},
+ orcid = {0000-0001-5950-6979}
+}
+```
+
+Special thanks to Glenn Jocher and the Ultralytics team for their work on developing and maintaining the YOLOv5 and YOLOv5u models.
\ No newline at end of file
diff --git a/yolov8/docs/models/yolov6.md b/yolov8/docs/models/yolov6.md
new file mode 100644
index 0000000000000000000000000000000000000000..a8a2449f25c4e17f59a01f00dc097fb1bba89d98
--- /dev/null
+++ b/yolov8/docs/models/yolov6.md
@@ -0,0 +1,82 @@
+---
+comments: true
+description: Discover Meituan YOLOv6, a robust real-time object detector. Learn how to utilize pre-trained models with Ultralytics Python API for a variety of tasks.
+keywords: Meituan, YOLOv6, object detection, Bi-directional Concatenation (BiC), anchor-aided training (AAT), pre-trained models, high-resolution input, real-time, ultra-fast computations
+---
+
+# Meituan YOLOv6
+
+## Overview
+
+[Meituan](https://about.meituan.com/) YOLOv6 is a cutting-edge object detector that offers remarkable balance between speed and accuracy, making it a popular choice for real-time applications. This model introduces several notable enhancements on its architecture and training scheme, including the implementation of a Bi-directional Concatenation (BiC) module, an anchor-aided training (AAT) strategy, and an improved backbone and neck design for state-of-the-art accuracy on the COCO dataset.
+
+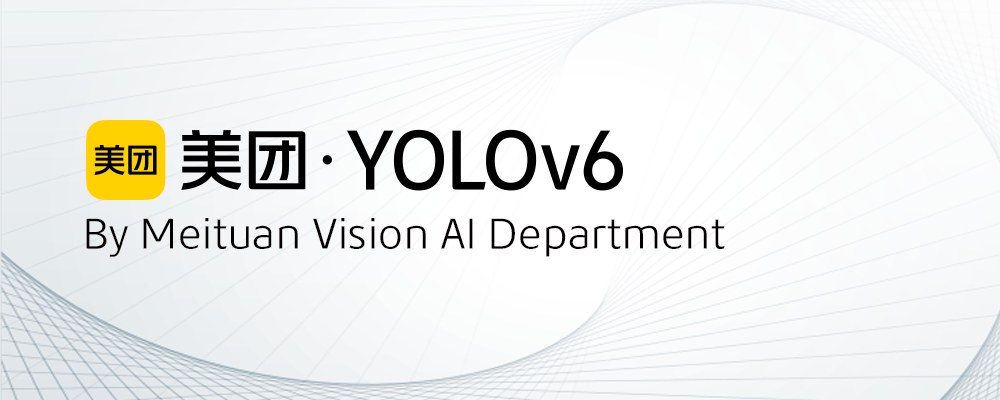
+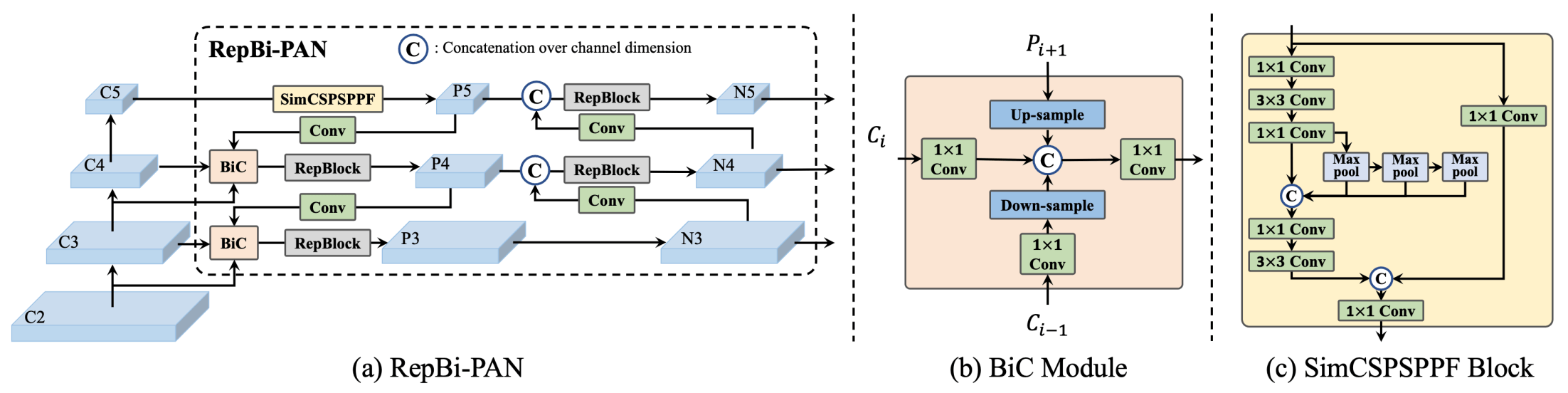
+**Overview of YOLOv6.** Model architecture diagram showing the redesigned network components and training strategies that have led to significant performance improvements. (a) The neck of YOLOv6 (N and S are shown). Note for M/L, RepBlocks is replaced with CSPStackRep. (b) The
+structure of a BiC module. (c) A SimCSPSPPF block. ([source](https://arxiv.org/pdf/2301.05586.pdf)).
+
+### Key Features
+
+- **Bi-directional Concatenation (BiC) Module:** YOLOv6 introduces a BiC module in the neck of the detector, enhancing localization signals and delivering performance gains with negligible speed degradation.
+- **Anchor-Aided Training (AAT) Strategy:** This model proposes AAT to enjoy the benefits of both anchor-based and anchor-free paradigms without compromising inference efficiency.
+- **Enhanced Backbone and Neck Design:** By deepening YOLOv6 to include another stage in the backbone and neck, this model achieves state-of-the-art performance on the COCO dataset at high-resolution input.
+- **Self-Distillation Strategy:** A new self-distillation strategy is implemented to boost the performance of smaller models of YOLOv6, enhancing the auxiliary regression branch during training and removing it at inference to avoid a marked speed decline.
+
+## Pre-trained Models
+
+YOLOv6 provides various pre-trained models with different scales:
+
+- YOLOv6-N: 37.5% AP on COCO val2017 at 1187 FPS with NVIDIA Tesla T4 GPU.
+- YOLOv6-S: 45.0% AP at 484 FPS.
+- YOLOv6-M: 50.0% AP at 226 FPS.
+- YOLOv6-L: 52.8% AP at 116 FPS.
+- YOLOv6-L6: State-of-the-art accuracy in real-time.
+
+YOLOv6 also provides quantized models for different precisions and models optimized for mobile platforms.
+
+## Usage
+
+### Python API
+
+```python
+from ultralytics import YOLO
+
+model = YOLO("yolov6n.yaml") # build new model from scratch
+model.info() # display model information
+model.predict("path/to/image.jpg") # predict
+```
+
+### Supported Tasks
+
+| Model Type | Pre-trained Weights | Tasks Supported |
+|------------|---------------------|------------------|
+| YOLOv6-N | `yolov6-n.pt` | Object Detection |
+| YOLOv6-S | `yolov6-s.pt` | Object Detection |
+| YOLOv6-M | `yolov6-m.pt` | Object Detection |
+| YOLOv6-L | `yolov6-l.pt` | Object Detection |
+| YOLOv6-L6 | `yolov6-l6.pt` | Object Detection |
+
+## Supported Modes
+
+| Mode | Supported |
+|------------|--------------------|
+| Inference | :heavy_check_mark: |
+| Validation | :heavy_check_mark: |
+| Training | :heavy_check_mark: |
+
+## Citations and Acknowledgements
+
+We would like to acknowledge the authors for their significant contributions in the field of real-time object detection:
+
+```bibtex
+@misc{li2023yolov6,
+ title={YOLOv6 v3.0: A Full-Scale Reloading},
+ author={Chuyi Li and Lulu Li and Yifei Geng and Hongliang Jiang and Meng Cheng and Bo Zhang and Zaidan Ke and Xiaoming Xu and Xiangxiang Chu},
+ year={2023},
+ eprint={2301.05586},
+ archivePrefix={arXiv},
+ primaryClass={cs.CV}
+}
+```
+
+The original YOLOv6 paper can be found on [arXiv](https://arxiv.org/abs/2301.05586). The authors have made their work publicly available, and the codebase can be accessed on [GitHub](https://github.com/meituan/YOLOv6). We appreciate their efforts in advancing the field and making their work accessible to the broader community.
\ No newline at end of file
diff --git a/yolov8/docs/models/yolov7.md b/yolov8/docs/models/yolov7.md
new file mode 100644
index 0000000000000000000000000000000000000000..d8b1ea62745e0deba5da628fa08503f40d4a15c0
--- /dev/null
+++ b/yolov8/docs/models/yolov7.md
@@ -0,0 +1,61 @@
+---
+comments: true
+description: Discover YOLOv7, a cutting-edge real-time object detector that surpasses competitors in speed and accuracy. Explore its unique trainable bag-of-freebies.
+keywords: object detection, real-time object detector, YOLOv7, MS COCO, computer vision, neural networks, AI, deep learning, deep neural networks, real-time, GPU, GitHub, arXiv
+---
+
+# YOLOv7: Trainable Bag-of-Freebies
+
+YOLOv7 is a state-of-the-art real-time object detector that surpasses all known object detectors in both speed and accuracy in the range from 5 FPS to 160 FPS. It has the highest accuracy (56.8% AP) among all known real-time object detectors with 30 FPS or higher on GPU V100. Moreover, YOLOv7 outperforms other object detectors such as YOLOR, YOLOX, Scaled-YOLOv4, YOLOv5, and many others in speed and accuracy. The model is trained on the MS COCO dataset from scratch without using any other datasets or pre-trained weights. Source code for YOLOv7 is available on GitHub.
+
+
+**Comparison of state-of-the-art object detectors.** From the results in Table 2 we know that the proposed method has the best speed-accuracy trade-off comprehensively. If we compare YOLOv7-tiny-SiLU with YOLOv5-N (r6.1), our method is 127 fps faster and 10.7% more accurate on AP. In addition, YOLOv7 has 51.4% AP at frame rate of 161 fps, while PPYOLOE-L with the same AP has only 78 fps frame rate. In terms of parameter usage, YOLOv7 is 41% less than PPYOLOE-L. If we compare YOLOv7-X with 114 fps inference speed to YOLOv5-L (r6.1) with 99 fps inference speed, YOLOv7-X can improve AP by 3.9%. If YOLOv7-X is compared with YOLOv5-X (r6.1) of similar scale, the inference speed of YOLOv7-X is 31 fps faster. In addition, in terms the amount of parameters and computation, YOLOv7-X reduces 22% of parameters and 8% of computation compared to YOLOv5-X (r6.1), but improves AP by 2.2% ([Source](https://arxiv.org/pdf/2207.02696.pdf)).
+
+## Overview
+
+Real-time object detection is an important component in many computer vision systems, including multi-object tracking, autonomous driving, robotics, and medical image analysis. In recent years, real-time object detection development has focused on designing efficient architectures and improving the inference speed of various CPUs, GPUs, and neural processing units (NPUs). YOLOv7 supports both mobile GPU and GPU devices, from the edge to the cloud.
+
+Unlike traditional real-time object detectors that focus on architecture optimization, YOLOv7 introduces a focus on the optimization of the training process. This includes modules and optimization methods designed to improve the accuracy of object detection without increasing the inference cost, a concept known as the "trainable bag-of-freebies".
+
+## Key Features
+
+YOLOv7 introduces several key features:
+
+1. **Model Re-parameterization**: YOLOv7 proposes a planned re-parameterized model, which is a strategy applicable to layers in different networks with the concept of gradient propagation path.
+
+2. **Dynamic Label Assignment**: The training of the model with multiple output layers presents a new issue: "How to assign dynamic targets for the outputs of different branches?" To solve this problem, YOLOv7 introduces a new label assignment method called coarse-to-fine lead guided label assignment.
+
+3. **Extended and Compound Scaling**: YOLOv7 proposes "extend" and "compound scaling" methods for the real-time object detector that can effectively utilize parameters and computation.
+
+4. **Efficiency**: The method proposed by YOLOv7 can effectively reduce about 40% parameters and 50% computation of state-of-the-art real-time object detector, and has faster inference speed and higher detection accuracy.
+
+## Usage Examples
+
+As of the time of writing, Ultralytics does not currently support YOLOv7 models. Therefore, any users interested in using YOLOv7 will need to refer directly to the YOLOv7 GitHub repository for installation and usage instructions.
+
+Here is a brief overview of the typical steps you might take to use YOLOv7:
+
+1. Visit the YOLOv7 GitHub repository: [https://github.com/WongKinYiu/yolov7](https://github.com/WongKinYiu/yolov7).
+
+2. Follow the instructions provided in the README file for installation. This typically involves cloning the repository, installing necessary dependencies, and setting up any necessary environment variables.
+
+3. Once installation is complete, you can train and use the model as per the usage instructions provided in the repository. This usually involves preparing your dataset, configuring the model parameters, training the model, and then using the trained model to perform object detection.
+
+Please note that the specific steps may vary depending on your specific use case and the current state of the YOLOv7 repository. Therefore, it is strongly recommended to refer directly to the instructions provided in the YOLOv7 GitHub repository.
+
+We regret any inconvenience this may cause and will strive to update this document with usage examples for Ultralytics once support for YOLOv7 is implemented.
+
+## Citations and Acknowledgements
+
+We would like to acknowledge the YOLOv7 authors for their significant contributions in the field of real-time object detection:
+
+```bibtex
+@article{wang2022yolov7,
+ title={{YOLOv7}: Trainable bag-of-freebies sets new state-of-the-art for real-time object detectors},
+ author={Wang, Chien-Yao and Bochkovskiy, Alexey and Liao, Hong-Yuan Mark},
+ journal={arXiv preprint arXiv:2207.02696},
+ year={2022}
+}
+```
+
+The original YOLOv7 paper can be found on [arXiv](https://arxiv.org/pdf/2207.02696.pdf). The authors have made their work publicly available, and the codebase can be accessed on [GitHub](https://github.com/WongKinYiu/yolov7). We appreciate their efforts in advancing the field and making their work accessible to the broader community.
\ No newline at end of file
diff --git a/yolov8/docs/models/yolov8.md b/yolov8/docs/models/yolov8.md
new file mode 100644
index 0000000000000000000000000000000000000000..8907248cdf453c83ed33b23b76a73b65aa632c6b
--- /dev/null
+++ b/yolov8/docs/models/yolov8.md
@@ -0,0 +1,115 @@
+---
+comments: true
+description: Learn about YOLOv8's pre-trained weights supporting detection, instance segmentation, pose, and classification tasks. Get performance details.
+keywords: YOLOv8, real-time object detection, object detection, deep learning, machine learning
+---
+
+# YOLOv8
+
+## Overview
+
+YOLOv8 is the latest iteration in the YOLO series of real-time object detectors, offering cutting-edge performance in terms of accuracy and speed. Building upon the advancements of previous YOLO versions, YOLOv8 introduces new features and optimizations that make it an ideal choice for various object detection tasks in a wide range of applications.
+
+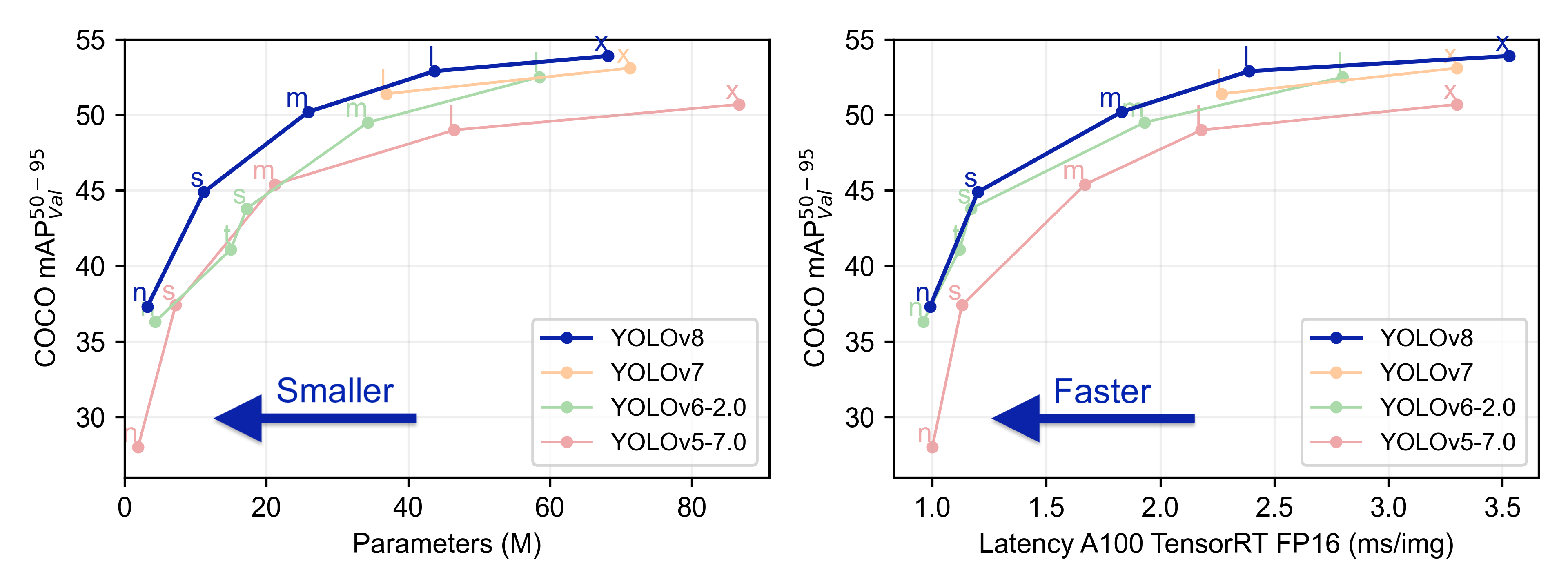
+
+## Key Features
+
+- **Advanced Backbone and Neck Architectures:** YOLOv8 employs state-of-the-art backbone and neck architectures, resulting in improved feature extraction and object detection performance.
+- **Anchor-free Split Ultralytics Head:** YOLOv8 adopts an anchor-free split Ultralytics head, which contributes to better accuracy and a more efficient detection process compared to anchor-based approaches.
+- **Optimized Accuracy-Speed Tradeoff:** With a focus on maintaining an optimal balance between accuracy and speed, YOLOv8 is suitable for real-time object detection tasks in diverse application areas.
+- **Variety of Pre-trained Models:** YOLOv8 offers a range of pre-trained models to cater to various tasks and performance requirements, making it easier to find the right model for your specific use case.
+
+## Supported Tasks
+
+| Model Type | Pre-trained Weights | Task |
+|-------------|------------------------------------------------------------------------------------------------------------------|-----------------------|
+| YOLOv8 | `yolov8n.pt`, `yolov8s.pt`, `yolov8m.pt`, `yolov8l.pt`, `yolov8x.pt` | Detection |
+| YOLOv8-seg | `yolov8n-seg.pt`, `yolov8s-seg.pt`, `yolov8m-seg.pt`, `yolov8l-seg.pt`, `yolov8x-seg.pt` | Instance Segmentation |
+| YOLOv8-pose | `yolov8n-pose.pt`, `yolov8s-pose.pt`, `yolov8m-pose.pt`, `yolov8l-pose.pt`, `yolov8x-pose.pt` ,`yolov8x-pose-p6` | Pose/Keypoints |
+| YOLOv8-cls | `yolov8n-cls.pt`, `yolov8s-cls.pt`, `yolov8m-cls.pt`, `yolov8l-cls.pt`, `yolov8x-cls.pt` | Classification |
+
+## Supported Modes
+
+| Mode | Supported |
+|------------|--------------------|
+| Inference | :heavy_check_mark: |
+| Validation | :heavy_check_mark: |
+| Training | :heavy_check_mark: |
+
+!!! Performance
+
+ === "Detection"
+
+ | Model | size
(pixels) | mAPval
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+ | ------------------------------------------------------------------------------------ | --------------------- | -------------------- | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+ | [YOLOv8n](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n.pt) | 640 | 37.3 | 80.4 | 0.99 | 3.2 | 8.7 |
+ | [YOLOv8s](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s.pt) | 640 | 44.9 | 128.4 | 1.20 | 11.2 | 28.6 |
+ | [YOLOv8m](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m.pt) | 640 | 50.2 | 234.7 | 1.83 | 25.9 | 78.9 |
+ | [YOLOv8l](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l.pt) | 640 | 52.9 | 375.2 | 2.39 | 43.7 | 165.2 |
+ | [YOLOv8x](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x.pt) | 640 | 53.9 | 479.1 | 3.53 | 68.2 | 257.8 |
+
+ === "Segmentation"
+
+ | Model | size
(pixels) | mAPbox
50-95 | mAPmask
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+ | -------------------------------------------------------------------------------------------- | --------------------- | -------------------- | --------------------- | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+ | [YOLOv8n-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-seg.pt) | 640 | 36.7 | 30.5 | 96.1 | 1.21 | 3.4 | 12.6 |
+ | [YOLOv8s-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-seg.pt) | 640 | 44.6 | 36.8 | 155.7 | 1.47 | 11.8 | 42.6 |
+ | [YOLOv8m-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-seg.pt) | 640 | 49.9 | 40.8 | 317.0 | 2.18 | 27.3 | 110.2 |
+ | [YOLOv8l-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-seg.pt) | 640 | 52.3 | 42.6 | 572.4 | 2.79 | 46.0 | 220.5 |
+ | [YOLOv8x-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-seg.pt) | 640 | 53.4 | 43.4 | 712.1 | 4.02 | 71.8 | 344.1 |
+
+ === "Classification"
+
+ | Model | size
(pixels) | acc
top1 | acc
top5 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) at 640 |
+ | -------------------------------------------------------------------------------------------- | --------------------- | ---------------- | ---------------- | ------------------------------ | ----------------------------------- | ------------------ | ------------------------ |
+ | [YOLOv8n-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-cls.pt) | 224 | 66.6 | 87.0 | 12.9 | 0.31 | 2.7 | 4.3 |
+ | [YOLOv8s-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-cls.pt) | 224 | 72.3 | 91.1 | 23.4 | 0.35 | 6.4 | 13.5 |
+ | [YOLOv8m-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-cls.pt) | 224 | 76.4 | 93.2 | 85.4 | 0.62 | 17.0 | 42.7 |
+ | [YOLOv8l-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-cls.pt) | 224 | 78.0 | 94.1 | 163.0 | 0.87 | 37.5 | 99.7 |
+ | [YOLOv8x-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-cls.pt) | 224 | 78.4 | 94.3 | 232.0 | 1.01 | 57.4 | 154.8 |
+
+ === "Pose"
+
+ | Model | size
(pixels) | mAPpose
50-95 | mAPpose
50 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+ | ---------------------------------------------------------------------------------------------------- | --------------------- | --------------------- | ------------------ | ------------------------------ | ----------------------------------- | ------------------ | ----------------- |
+ | [YOLOv8n-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-pose.pt) | 640 | 50.4 | 80.1 | 131.8 | 1.18 | 3.3 | 9.2 |
+ | [YOLOv8s-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-pose.pt) | 640 | 60.0 | 86.2 | 233.2 | 1.42 | 11.6 | 30.2 |
+ | [YOLOv8m-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-pose.pt) | 640 | 65.0 | 88.8 | 456.3 | 2.00 | 26.4 | 81.0 |
+ | [YOLOv8l-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-pose.pt) | 640 | 67.6 | 90.0 | 784.5 | 2.59 | 44.4 | 168.6 |
+ | [YOLOv8x-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose.pt) | 640 | 69.2 | 90.2 | 1607.1 | 3.73 | 69.4 | 263.2 |
+ | [YOLOv8x-pose-p6](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose-p6.pt) | 1280 | 71.6 | 91.2 | 4088.7 | 10.04 | 99.1 | 1066.4 |
+
+## Usage
+
+You can use YOLOv8 for object detection tasks using the Ultralytics pip package. The following is a sample code snippet showing how to use YOLOv8 models for inference:
+
+```python
+from ultralytics import YOLO
+
+# Load the model
+model = YOLO('yolov8n.pt') # load a pretrained model
+
+# Perform inference
+results = model('image.jpg')
+
+# Print the results
+results.print()
+```
+
+## Citation
+
+If you use the YOLOv8 model or any other software from this repository in your work, please cite it using the following format:
+
+```bibtex
+@software{yolov8_ultralytics,
+ author = {Glenn Jocher and Ayush Chaurasia and Jing Qiu},
+ title = {Ultralytics YOLOv8},
+ version = {8.0.0},
+ year = {2023},
+ url = {https://github.com/ultralytics/ultralytics},
+ orcid = {0000-0001-5950-6979, 0000-0002-7603-6750, 0000-0003-3783-7069},
+ license = {AGPL-3.0}
+}
+```
+
+Please note that the DOI is pending and will be added to the citation once it is available. The usage of the software is in accordance with the AGPL-3.0 license.
\ No newline at end of file
diff --git a/yolov8/docs/modes/benchmark.md b/yolov8/docs/modes/benchmark.md
new file mode 100644
index 0000000000000000000000000000000000000000..c1c159e6bed97519b2a830ba70068068d3875da5
--- /dev/null
+++ b/yolov8/docs/modes/benchmark.md
@@ -0,0 +1,74 @@
+---
+comments: true
+description: Benchmark mode compares speed and accuracy of various YOLOv8 export formats like ONNX or OpenVINO. Optimize formats for speed or accuracy.
+keywords: YOLOv8, Benchmark Mode, Export Formats, ONNX, OpenVINO, TensorRT, Ultralytics Docs
+---
+
+
+
+**Benchmark mode** is used to profile the speed and accuracy of various export formats for YOLOv8. The benchmarks
+provide information on the size of the exported format, its `mAP50-95` metrics (for object detection, segmentation and pose)
+or `accuracy_top5` metrics (for classification), and the inference time in milliseconds per image across various export
+formats like ONNX, OpenVINO, TensorRT and others. This information can help users choose the optimal export format for
+their specific use case based on their requirements for speed and accuracy.
+
+!!! tip "Tip"
+
+ * Export to ONNX or OpenVINO for up to 3x CPU speedup.
+ * Export to TensorRT for up to 5x GPU speedup.
+
+## Usage Examples
+
+Run YOLOv8n benchmarks on all supported export formats including ONNX, TensorRT etc. See Arguments section below for a
+full list of export arguments.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics.yolo.utils.benchmarks import benchmark
+
+ # Benchmark on GPU
+ benchmark(model='yolov8n.pt', imgsz=640, half=False, device=0)
+ ```
+ === "CLI"
+
+ ```bash
+ yolo benchmark model=yolov8n.pt imgsz=640 half=False device=0
+ ```
+
+## Arguments
+
+Arguments such as `model`, `imgsz`, `half`, `device`, and `hard_fail` provide users with the flexibility to fine-tune
+the benchmarks to their specific needs and compare the performance of different export formats with ease.
+
+| Key | Value | Description |
+|-------------|---------|----------------------------------------------------------------------|
+| `model` | `None` | path to model file, i.e. yolov8n.pt, yolov8n.yaml |
+| `imgsz` | `640` | image size as scalar or (h, w) list, i.e. (640, 480) |
+| `half` | `False` | FP16 quantization |
+| `int8` | `False` | INT8 quantization |
+| `device` | `None` | device to run on, i.e. cuda device=0 or device=0,1,2,3 or device=cpu |
+| `hard_fail` | `False` | do not continue on error (bool), or val floor threshold (float) |
+
+## Export Formats
+
+Benchmarks will attempt to run automatically on all possible export formats below.
+
+| Format | `format` Argument | Model | Metadata |
+|--------------------------------------------------------------------|-------------------|---------------------------|----------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n.pt` | ✅ |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n.torchscript` | ✅ |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n.onnx` | ✅ |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n_openvino_model/` | ✅ |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n.engine` | ✅ |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n.mlmodel` | ✅ |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n_saved_model/` | ✅ |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n.pb` | ❌ |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n.tflite` | ✅ |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n_edgetpu.tflite` | ✅ |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n_web_model/` | ✅ |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n_paddle_model/` | ✅ |
+
+See full `export` details in the [Export](https://docs.ultralytics.com/modes/export/) page.
\ No newline at end of file
diff --git a/yolov8/docs/modes/export.md b/yolov8/docs/modes/export.md
new file mode 100644
index 0000000000000000000000000000000000000000..54518b2961086b716ab06074336404691026cd67
--- /dev/null
+++ b/yolov8/docs/modes/export.md
@@ -0,0 +1,88 @@
+---
+comments: true
+description: 'Export mode: Create a deployment-ready YOLOv8 model by converting it to various formats. Export to ONNX or OpenVINO for up to 3x CPU speedup.'
+keywords: ultralytics docs, YOLOv8, export YOLOv8, YOLOv8 model deployment, exporting YOLOv8, ONNX, OpenVINO, TensorRT, CoreML, TF SavedModel, PaddlePaddle, TorchScript, ONNX format, OpenVINO format, TensorRT format, CoreML format, TF SavedModel format, PaddlePaddle format, Tencent NCNN, NCNN
+---
+
+
+
+**Export mode** is used for exporting a YOLOv8 model to a format that can be used for deployment. In this mode, the
+model is converted to a format that can be used by other software applications or hardware devices. This mode is useful
+when deploying the model to production environments.
+
+!!! tip "Tip"
+
+ * Export to ONNX or OpenVINO for up to 3x CPU speedup.
+ * Export to TensorRT for up to 5x GPU speedup.
+
+## Usage Examples
+
+Export a YOLOv8n model to a different format like ONNX or TensorRT. See Arguments section below for a full list of
+export arguments.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom trained
+
+ # Export the model
+ model.export(format='onnx')
+ ```
+ === "CLI"
+
+ ```bash
+ yolo export model=yolov8n.pt format=onnx # export official model
+ yolo export model=path/to/best.pt format=onnx # export custom trained model
+ ```
+
+## Arguments
+
+Export settings for YOLO models refer to the various configurations and options used to save or
+export the model for use in other environments or platforms. These settings can affect the model's performance, size,
+and compatibility with different systems. Some common YOLO export settings include the format of the exported model
+file (e.g. ONNX, TensorFlow SavedModel), the device on which the model will be run (e.g. CPU, GPU), and the presence of
+additional features such as masks or multiple labels per box. Other factors that may affect the export process include
+the specific task the model is being used for and the requirements or constraints of the target environment or platform.
+It is important to carefully consider and configure these settings to ensure that the exported model is optimized for
+the intended use case and can be used effectively in the target environment.
+
+| Key | Value | Description |
+|-------------|-----------------|------------------------------------------------------|
+| `format` | `'torchscript'` | format to export to |
+| `imgsz` | `640` | image size as scalar or (h, w) list, i.e. (640, 480) |
+| `keras` | `False` | use Keras for TF SavedModel export |
+| `optimize` | `False` | TorchScript: optimize for mobile |
+| `half` | `False` | FP16 quantization |
+| `int8` | `False` | INT8 quantization |
+| `dynamic` | `False` | ONNX/TensorRT: dynamic axes |
+| `simplify` | `False` | ONNX/TensorRT: simplify model |
+| `opset` | `None` | ONNX: opset version (optional, defaults to latest) |
+| `workspace` | `4` | TensorRT: workspace size (GB) |
+| `nms` | `False` | CoreML: add NMS |
+
+## Export Formats
+
+Available YOLOv8 export formats are in the table below. You can export to any format using the `format` argument,
+i.e. `format='onnx'` or `format='engine'`.
+
+| Format | `format` Argument | Model | Metadata | Arguments |
+|--------------------------------------------------------------------|-------------------|---------------------------|----------|-----------------------------------------------------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n.pt` | ✅ | - |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n.torchscript` | ✅ | `imgsz`, `optimize` |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n.onnx` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `opset` |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n_openvino_model/` | ✅ | `imgsz`, `half` |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n.engine` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `workspace` |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n.mlmodel` | ✅ | `imgsz`, `half`, `int8`, `nms` |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n_saved_model/` | ✅ | `imgsz`, `keras` |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n.pb` | ❌ | `imgsz` |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n.tflite` | ✅ | `imgsz`, `half`, `int8` |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n_edgetpu.tflite` | ✅ | `imgsz` |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n_web_model/` | ✅ | `imgsz` |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n_paddle_model/` | ✅ | `imgsz` |
+| [NCNN](https://github.com/Tencent/ncnn) | `ncnn` | `yolov8n_ncnn_model/` | ✅ | `imgsz`, `half` |
\ No newline at end of file
diff --git a/yolov8/docs/modes/index.md b/yolov8/docs/modes/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..5a00afa5d39590995cb5c516243b3b7f65e41a4f
--- /dev/null
+++ b/yolov8/docs/modes/index.md
@@ -0,0 +1,68 @@
+---
+comments: true
+description: Use Ultralytics YOLOv8 Modes (Train, Val, Predict, Export, Track, Benchmark) to train, validate, predict, track, export or benchmark.
+keywords: yolov8, yolo, ultralytics, training, validation, prediction, export, tracking, benchmarking, real-time object detection, object tracking
+---
+
+# Ultralytics YOLOv8 Modes
+
+
+
+Ultralytics YOLOv8 supports several **modes** that can be used to perform different tasks. These modes are:
+
+- **Train**: For training a YOLOv8 model on a custom dataset.
+- **Val**: For validating a YOLOv8 model after it has been trained.
+- **Predict**: For making predictions using a trained YOLOv8 model on new images or videos.
+- **Export**: For exporting a YOLOv8 model to a format that can be used for deployment.
+- **Track**: For tracking objects in real-time using a YOLOv8 model.
+- **Benchmark**: For benchmarking YOLOv8 exports (ONNX, TensorRT, etc.) speed and accuracy.
+
+## [Train](train.md)
+
+Train mode is used for training a YOLOv8 model on a custom dataset. In this mode, the model is trained using the
+specified dataset and hyperparameters. The training process involves optimizing the model's parameters so that it can
+accurately predict the classes and locations of objects in an image.
+
+[Train Examples](train.md){ .md-button .md-button--primary}
+
+## [Val](val.md)
+
+Val mode is used for validating a YOLOv8 model after it has been trained. In this mode, the model is evaluated on a
+validation set to measure its accuracy and generalization performance. This mode can be used to tune the hyperparameters
+of the model to improve its performance.
+
+[Val Examples](val.md){ .md-button .md-button--primary}
+
+## [Predict](predict.md)
+
+Predict mode is used for making predictions using a trained YOLOv8 model on new images or videos. In this mode, the
+model is loaded from a checkpoint file, and the user can provide images or videos to perform inference. The model
+predicts the classes and locations of objects in the input images or videos.
+
+[Predict Examples](predict.md){ .md-button .md-button--primary}
+
+## [Export](export.md)
+
+Export mode is used for exporting a YOLOv8 model to a format that can be used for deployment. In this mode, the model is
+converted to a format that can be used by other software applications or hardware devices. This mode is useful when
+deploying the model to production environments.
+
+[Export Examples](export.md){ .md-button .md-button--primary}
+
+## [Track](track.md)
+
+Track mode is used for tracking objects in real-time using a YOLOv8 model. In this mode, the model is loaded from a
+checkpoint file, and the user can provide a live video stream to perform real-time object tracking. This mode is useful
+for applications such as surveillance systems or self-driving cars.
+
+[Track Examples](track.md){ .md-button .md-button--primary}
+
+## [Benchmark](benchmark.md)
+
+Benchmark mode is used to profile the speed and accuracy of various export formats for YOLOv8. The benchmarks provide
+information on the size of the exported format, its `mAP50-95` metrics (for object detection, segmentation and pose)
+or `accuracy_top5` metrics (for classification), and the inference time in milliseconds per image across various export
+formats like ONNX, OpenVINO, TensorRT and others. This information can help users choose the optimal export format for
+their specific use case based on their requirements for speed and accuracy.
+
+[Benchmark Examples](benchmark.md){ .md-button .md-button--primary}
\ No newline at end of file
diff --git a/yolov8/docs/modes/predict.md b/yolov8/docs/modes/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..8708933e618499c628c59dda2fc9eee78a9c23d2
--- /dev/null
+++ b/yolov8/docs/modes/predict.md
@@ -0,0 +1,525 @@
+---
+comments: true
+description: Get started with YOLOv8 Predict mode and input sources. Accepts various input sources such as images, videos, and directories.
+keywords: YOLOv8, predict mode, generator, streaming mode, input sources, video formats, arguments customization
+---
+
+
+
+YOLOv8 **predict mode** can generate predictions for various tasks, returning either a list of `Results` objects or a
+memory-efficient generator of `Results` objects when using the streaming mode. Enable streaming mode by
+passing `stream=True` in the predictor's call method.
+
+!!! example "Predict"
+
+ === "Return a list with `stream=False`"
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # pretrained YOLOv8n model
+
+ # Run batched inference on a list of images
+ results = model(['im1.jpg', 'im2.jpg']) # return a list of Results objects
+
+ # Process results list
+ for result in results:
+ boxes = result.boxes # Boxes object for bbox outputs
+ masks = result.masks # Masks object for segmentation masks outputs
+ keypoints = result.keypoints # Keypoints object for pose outputs
+ probs = result.probs # Class probabilities for classification outputs
+ ```
+
+ === "Return a generator with `stream=True`"
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # pretrained YOLOv8n model
+
+ # Run batched inference on a list of images
+ results = model(['im1.jpg', 'im2.jpg'], stream=True) # return a generator of Results objects
+
+ # Process results generator
+ for result in results:
+ boxes = result.boxes # Boxes object for bbox outputs
+ masks = result.masks # Masks object for segmentation masks outputs
+ keypoints = result.keypoints # Keypoints object for pose outputs
+ probs = result.probs # Class probabilities for classification outputs
+ ```
+
+## Inference Sources
+
+YOLOv8 can process different types of input sources for inference, as shown in the table below. The sources include static images, video streams, and various data formats. The table also indicates whether each source can be used in streaming mode with the argument `stream=True` ✅. Streaming mode is beneficial for processing videos or live streams as it creates a generator of results instead of loading all frames into memory.
+
+!!! tip "Tip"
+
+ Use `stream=True` for processing long videos or large datasets to efficiently manage memory. When `stream=False`, the results for all frames or data points are stored in memory, which can quickly add up and cause out-of-memory errors for large inputs. In contrast, `stream=True` utilizes a generator, which only keeps the results of the current frame or data point in memory, significantly reducing memory consumption and preventing out-of-memory issues.
+
+| Source | Argument | Type | Notes |
+|-------------|--------------------------------------------|---------------------------------------|----------------------------------------------------------------------------|
+| image | `'image.jpg'` | `str` or `Path` | Single image file. |
+| URL | `'https://ultralytics.com/images/bus.jpg'` | `str` | URL to an image. |
+| screenshot | `'screen'` | `str` | Capture a screenshot. |
+| PIL | `Image.open('im.jpg')` | `PIL.Image` | HWC format with RGB channels. |
+| OpenCV | `cv2.imread('im.jpg')` | `np.ndarray` of `uint8 (0-255)` | HWC format with BGR channels. |
+| numpy | `np.zeros((640,1280,3))` | `np.ndarray` of `uint8 (0-255)` | HWC format with BGR channels. |
+| torch | `torch.zeros(16,3,320,640)` | `torch.Tensor` of `float32 (0.0-1.0)` | BCHW format with RGB channels. |
+| CSV | `'sources.csv'` | `str` or `Path` | CSV file containing paths to images, videos, or directories. |
+| video ✅ | `'video.mp4'` | `str` or `Path` | Video file in formats like MP4, AVI, etc. |
+| directory ✅ | `'path/'` | `str` or `Path` | Path to a directory containing images or videos. |
+| glob ✅ | `'path/*.jpg'` | `str` | Glob pattern to match multiple files. Use the `*` character as a wildcard. |
+| YouTube ✅ | `'https://youtu.be/Zgi9g1ksQHc'` | `str` | URL to a YouTube video. |
+| stream ✅ | `'rtsp://example.com/media.mp4'` | `str` | URL for streaming protocols such as RTSP, RTMP, or an IP address. |
+
+Below are code examples for using each source type:
+
+!!! example "Prediction sources"
+
+ === "image"
+ Run inference on an image file.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define path to the image file
+ source = 'path/to/image.jpg'
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "screenshot"
+ Run inference on the current screen content as a screenshot.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define current screenshot as source
+ source = 'screen'
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "URL"
+ Run inference on an image or video hosted remotely via URL.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define remote image or video URL
+ source = 'https://ultralytics.com/images/bus.jpg'
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "PIL"
+ Run inference on an image opened with Python Imaging Library (PIL).
+ ```python
+ from PIL import Image
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Open an image using PIL
+ source = Image.open('path/to/image.jpg')
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "OpenCV"
+ Run inference on an image read with OpenCV.
+ ```python
+ import cv2
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Read an image using OpenCV
+ source = cv2.imread('path/to/image.jpg')
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "numpy"
+ Run inference on an image represented as a numpy array.
+ ```python
+ import numpy as np
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Create a random numpy array of HWC shape (640, 640, 3) with values in range [0, 255] and type uint8
+ source = np.random.randint(low=0, high=255, size=(640, 640, 3), dtype='uint8')
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "torch"
+ Run inference on an image represented as a PyTorch tensor.
+ ```python
+ import torch
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Create a random torch tensor of BCHW shape (1, 3, 640, 640) with values in range [0, 1] and type float32
+ source = torch.rand(1, 3, 640, 640, dtype=torch.float32)
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "CSV"
+ Run inference on a collection of images, URLs, videos and directories listed in a CSV file.
+ ```python
+ import torch
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define a path to a CSV file with images, URLs, videos and directories
+ source = 'path/to/file.csv'
+
+ # Run inference on the source
+ results = model(source) # list of Results objects
+ ```
+
+ === "video"
+ Run inference on a video file. By using `stream=True`, you can create a generator of Results objects to reduce memory usage.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define path to video file
+ source = 'path/to/video.mp4'
+
+ # Run inference on the source
+ results = model(source, stream=True) # generator of Results objects
+ ```
+
+ === "directory"
+ Run inference on all images and videos in a directory. To also capture images and videos in subdirectories use a glob pattern, i.e. `path/to/dir/**/*`.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define path to directory containing images and videos for inference
+ source = 'path/to/dir'
+
+ # Run inference on the source
+ results = model(source, stream=True) # generator of Results objects
+ ```
+
+ === "glob"
+ Run inference on all images and videos that match a glob expression with `*` characters.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define a glob search for all JPG files in a directory
+ source = 'path/to/dir/*.jpg'
+
+ # OR define a recursive glob search for all JPG files including subdirectories
+ source = 'path/to/dir/**/*.jpg'
+
+ # Run inference on the source
+ results = model(source, stream=True) # generator of Results objects
+ ```
+
+ === "YouTube"
+ Run inference on a YouTube video. By using `stream=True`, you can create a generator of Results objects to reduce memory usage for long videos.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define source as YouTube video URL
+ source = 'https://youtu.be/Zgi9g1ksQHc'
+
+ # Run inference on the source
+ results = model(source, stream=True) # generator of Results objects
+ ```
+
+ === "Stream"
+ Run inference on remote streaming sources using RTSP, RTMP, and IP address protocols.
+ ```python
+ from ultralytics import YOLO
+
+ # Load a pretrained YOLOv8n model
+ model = YOLO('yolov8n.pt')
+
+ # Define source as RTSP, RTMP or IP streaming address
+ source = 'rtsp://example.com/media.mp4'
+
+ # Run inference on the source
+ results = model(source, stream=True) # generator of Results objects
+ ```
+
+## Inference Arguments
+
+`model.predict` accepts multiple arguments that control the prediction operation. These arguments can be passed directly to `model.predict`:
+!!! example
+
+ ```python
+ model.predict(source, save=True, imgsz=320, conf=0.5)
+ ```
+
+All supported arguments:
+
+| Key | Value | Description |
+|----------------|------------------------|--------------------------------------------------------------------------------|
+| `source` | `'ultralytics/assets'` | source directory for images or videos |
+| `conf` | `0.25` | object confidence threshold for detection |
+| `iou` | `0.7` | intersection over union (IoU) threshold for NMS |
+| `half` | `False` | use half precision (FP16) |
+| `device` | `None` | device to run on, i.e. cuda device=0/1/2/3 or device=cpu |
+| `show` | `False` | show results if possible |
+| `save` | `False` | save images with results |
+| `save_txt` | `False` | save results as .txt file |
+| `save_conf` | `False` | save results with confidence scores |
+| `save_crop` | `False` | save cropped images with results |
+| `hide_labels` | `False` | hide labels |
+| `hide_conf` | `False` | hide confidence scores |
+| `max_det` | `300` | maximum number of detections per image |
+| `vid_stride` | `False` | video frame-rate stride |
+| `line_width` | `None` | The line width of the bounding boxes. If None, it is scaled to the image size. |
+| `visualize` | `False` | visualize model features |
+| `augment` | `False` | apply image augmentation to prediction sources |
+| `agnostic_nms` | `False` | class-agnostic NMS |
+| `retina_masks` | `False` | use high-resolution segmentation masks |
+| `classes` | `None` | filter results by class, i.e. class=0, or class=[0,2,3] |
+| `boxes` | `True` | Show boxes in segmentation predictions |
+
+## Image and Video Formats
+
+YOLOv8 supports various image and video formats, as specified in [yolo/data/utils.py](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/yolo/data/utils.py). See the tables below for the valid suffixes and example predict commands.
+
+### Image Suffixes
+
+The below table contains valid Ultralytics image formats.
+
+| Image Suffixes | Example Predict Command | Reference |
+|----------------|----------------------------------|-------------------------------------------------------------------------------|
+| .bmp | `yolo predict source=image.bmp` | [Microsoft BMP File Format](https://en.wikipedia.org/wiki/BMP_file_format) |
+| .dng | `yolo predict source=image.dng` | [Adobe DNG](https://www.adobe.com/products/photoshop/extend.displayTab2.html) |
+| .jpeg | `yolo predict source=image.jpeg` | [JPEG](https://en.wikipedia.org/wiki/JPEG) |
+| .jpg | `yolo predict source=image.jpg` | [JPEG](https://en.wikipedia.org/wiki/JPEG) |
+| .mpo | `yolo predict source=image.mpo` | [Multi Picture Object](https://fileinfo.com/extension/mpo) |
+| .png | `yolo predict source=image.png` | [Portable Network Graphics](https://en.wikipedia.org/wiki/PNG) |
+| .tif | `yolo predict source=image.tif` | [Tag Image File Format](https://en.wikipedia.org/wiki/TIFF) |
+| .tiff | `yolo predict source=image.tiff` | [Tag Image File Format](https://en.wikipedia.org/wiki/TIFF) |
+| .webp | `yolo predict source=image.webp` | [WebP](https://en.wikipedia.org/wiki/WebP) |
+| .pfm | `yolo predict source=image.pfm` | [Portable FloatMap](https://en.wikipedia.org/wiki/Netpbm#File_formats) |
+
+### Video Suffixes
+
+The below table contains valid Ultralytics video formats.
+
+| Video Suffixes | Example Predict Command | Reference |
+|----------------|----------------------------------|----------------------------------------------------------------------------------|
+| .asf | `yolo predict source=video.asf` | [Advanced Systems Format](https://en.wikipedia.org/wiki/Advanced_Systems_Format) |
+| .avi | `yolo predict source=video.avi` | [Audio Video Interleave](https://en.wikipedia.org/wiki/Audio_Video_Interleave) |
+| .gif | `yolo predict source=video.gif` | [Graphics Interchange Format](https://en.wikipedia.org/wiki/GIF) |
+| .m4v | `yolo predict source=video.m4v` | [MPEG-4 Part 14](https://en.wikipedia.org/wiki/M4V) |
+| .mkv | `yolo predict source=video.mkv` | [Matroska](https://en.wikipedia.org/wiki/Matroska) |
+| .mov | `yolo predict source=video.mov` | [QuickTime File Format](https://en.wikipedia.org/wiki/QuickTime_File_Format) |
+| .mp4 | `yolo predict source=video.mp4` | [MPEG-4 Part 14 - Wikipedia](https://en.wikipedia.org/wiki/MPEG-4_Part_14) |
+| .mpeg | `yolo predict source=video.mpeg` | [MPEG-1 Part 2](https://en.wikipedia.org/wiki/MPEG-1) |
+| .mpg | `yolo predict source=video.mpg` | [MPEG-1 Part 2](https://en.wikipedia.org/wiki/MPEG-1) |
+| .ts | `yolo predict source=video.ts` | [MPEG Transport Stream](https://en.wikipedia.org/wiki/MPEG_transport_stream) |
+| .wmv | `yolo predict source=video.wmv` | [Windows Media Video](https://en.wikipedia.org/wiki/Windows_Media_Video) |
+| .webm | `yolo predict source=video.webm` | [WebM Project](https://en.wikipedia.org/wiki/WebM) |
+
+## Working with Results
+
+The `Results` object contains the following components:
+
+- `Results.boxes`: `Boxes` object with properties and methods for manipulating bounding boxes
+- `Results.masks`: `Masks` object for indexing masks or getting segment coordinates
+- `Results.keypoints`: `Keypoints` object for with properties and methods for manipulating predicted keypoints.
+- `Results.probs`: `Probs` object for containing class probabilities.
+- `Results.orig_img`: Original image loaded in memory
+- `Results.path`: `Path` containing the path to the input image
+
+Each result is composed of a `torch.Tensor` by default, which allows for easy manipulation:
+
+!!! example "Results"
+
+ ```python
+ results = results.cuda()
+ results = results.cpu()
+ results = results.to('cpu')
+ results = results.numpy()
+ ```
+
+### Boxes
+
+`Boxes` object can be used to index, manipulate, and convert bounding boxes to different formats. Box format conversion
+operations are cached, meaning they're only calculated once per object, and those values are reused for future calls.
+
+- Indexing a `Boxes` object returns a `Boxes` object:
+
+!!! example "Boxes"
+
+ ```python
+ results = model(img)
+ boxes = results[0].boxes
+ box = boxes[0] # returns one box
+ box.xyxy
+ ```
+
+- Properties and conversions
+
+!!! example "Boxes Properties"
+
+ ```python
+ boxes.xyxy # box with xyxy format, (N, 4)
+ boxes.xywh # box with xywh format, (N, 4)
+ boxes.xyxyn # box with xyxy format but normalized, (N, 4)
+ boxes.xywhn # box with xywh format but normalized, (N, 4)
+ boxes.conf # confidence score, (N, )
+ boxes.cls # cls, (N, )
+ boxes.data # raw bboxes tensor, (N, 6) or boxes.boxes
+ ```
+
+### Masks
+
+`Masks` object can be used index, manipulate and convert masks to segments. The segment conversion operation is cached.
+
+!!! example "Masks"
+
+ ```python
+ results = model(inputs)
+ masks = results[0].masks # Masks object
+ masks.xy # x, y segments (pixels), List[segment] * N
+ masks.xyn # x, y segments (normalized), List[segment] * N
+ masks.data # raw masks tensor, (N, H, W) or masks.masks
+ ```
+
+### Keypoints
+
+`Keypoints` object can be used index, manipulate and normalize coordinates. The keypoint conversion operation is cached.
+
+!!! example "Keypoints"
+
+ ```python
+ results = model(inputs)
+ keypoints = results[0].keypoints # Masks object
+ keypoints.xy # x, y keypoints (pixels), (num_dets, num_kpts, 2/3), the last dimension can be 2 or 3, depends the model.
+ keypoints.xyn # x, y keypoints (normalized), (num_dets, num_kpts, 2/3)
+ keypoints.conf # confidence score(num_dets, num_kpts) of each keypoint if the last dimension is 3.
+ keypoints.data # raw keypoints tensor, (num_dets, num_kpts, 2/3)
+ ```
+
+### probs
+
+`Probs` object can be used index, get top1&top5 indices and scores of classification.
+
+!!! example "Probs"
+
+ ```python
+ results = model(inputs)
+ probs = results[0].probs # cls prob, (num_class, )
+ probs.top5 # The top5 indices of classification, List[Int] * 5.
+ probs.top1 # The top1 indices of classification, a value with Int type.
+ probs.top5conf # The top5 scores of classification, a tensor with shape (5, ).
+ probs.top1conf # The top1 scores of classification. a value with torch.tensor type.
+ keypoints.data # raw probs tensor, (num_class, )
+ ```
+
+Class reference documentation for `Results` module and its components can be found [here](../reference/yolo/engine/results.md)
+
+## Plotting results
+
+You can use `plot()` function of `Result` object to plot results on in image object. It plots all components(boxes,
+masks, classification probabilities, etc.) found in the results object
+
+!!! example "Plotting"
+
+ ```python
+ res = model(img)
+ res_plotted = res[0].plot()
+ cv2.imshow("result", res_plotted)
+ ```
+
+| Argument | Description |
+|-------------------------------|----------------------------------------------------------------------------------------|
+| `conf (bool)` | Whether to plot the detection confidence score. |
+| `line_width (int, optional)` | The line width of the bounding boxes. If None, it is scaled to the image size. |
+| `font_size (float, optional)` | The font size of the text. If None, it is scaled to the image size. |
+| `font (str)` | The font to use for the text. |
+| `pil (bool)` | Whether to use PIL for image plotting. |
+| `example (str)` | An example string to display. Useful for indicating the expected format of the output. |
+| `img (numpy.ndarray)` | Plot to another image. if not, plot to original image. |
+| `labels (bool)` | Whether to plot the label of bounding boxes. |
+| `boxes (bool)` | Whether to plot the bounding boxes. |
+| `masks (bool)` | Whether to plot the masks. |
+| `probs (bool)` | Whether to plot classification probability. |
+
+## Streaming Source `for`-loop
+
+Here's a Python script using OpenCV (cv2) and YOLOv8 to run inference on video frames. This script assumes you have already installed the necessary packages (opencv-python and ultralytics).
+
+!!! example "Streaming for-loop"
+
+ ```python
+ import cv2
+ from ultralytics import YOLO
+
+ # Load the YOLOv8 model
+ model = YOLO('yolov8n.pt')
+
+ # Open the video file
+ video_path = "path/to/your/video/file.mp4"
+ cap = cv2.VideoCapture(video_path)
+
+ # Loop through the video frames
+ while cap.isOpened():
+ # Read a frame from the video
+ success, frame = cap.read()
+
+ if success:
+ # Run YOLOv8 inference on the frame
+ results = model(frame)
+
+ # Visualize the results on the frame
+ annotated_frame = results[0].plot()
+
+ # Display the annotated frame
+ cv2.imshow("YOLOv8 Inference", annotated_frame)
+
+ # Break the loop if 'q' is pressed
+ if cv2.waitKey(1) & 0xFF == ord("q"):
+ break
+ else:
+ # Break the loop if the end of the video is reached
+ break
+
+ # Release the video capture object and close the display window
+ cap.release()
+ cv2.destroyAllWindows()
+ ```
\ No newline at end of file
diff --git a/yolov8/docs/modes/track.md b/yolov8/docs/modes/track.md
new file mode 100644
index 0000000000000000000000000000000000000000..7b9a83a714bcb26500fd914f272f3788fa799eaf
--- /dev/null
+++ b/yolov8/docs/modes/track.md
@@ -0,0 +1,101 @@
+---
+comments: true
+description: Explore YOLOv8n-based object tracking with Ultralytics' BoT-SORT and ByteTrack. Learn configuration, usage, and customization tips.
+keywords: object tracking, YOLO, trackers, BoT-SORT, ByteTrack
+---
+
+
+
+Object tracking is a task that involves identifying the location and class of objects, then assigning a unique ID to
+that detection in video streams.
+
+The output of tracker is the same as detection with an added object ID.
+
+## Available Trackers
+
+The following tracking algorithms have been implemented and can be enabled by passing `tracker=tracker_type.yaml`
+
+* [BoT-SORT](https://github.com/NirAharon/BoT-SORT) - `botsort.yaml`
+* [ByteTrack](https://github.com/ifzhang/ByteTrack) - `bytetrack.yaml`
+
+The default tracker is BoT-SORT.
+
+## Tracking
+
+Use a trained YOLOv8n/YOLOv8n-seg model to run tracker on video streams.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official detection model
+ model = YOLO('yolov8n-seg.pt') # load an official segmentation model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Track with the model
+ results = model.track(source="https://youtu.be/Zgi9g1ksQHc", show=True)
+ results = model.track(source="https://youtu.be/Zgi9g1ksQHc", show=True, tracker="bytetrack.yaml")
+ ```
+ === "CLI"
+
+ ```bash
+ yolo track model=yolov8n.pt source="https://youtu.be/Zgi9g1ksQHc" # official detection model
+ yolo track model=yolov8n-seg.pt source=... # official segmentation model
+ yolo track model=path/to/best.pt source=... # custom model
+ yolo track model=path/to/best.pt tracker="bytetrack.yaml" # bytetrack tracker
+
+ ```
+
+As in the above usage, we support both the detection and segmentation models for tracking and the only thing you need to
+do is loading the corresponding (detection or segmentation) model.
+
+## Configuration
+
+### Tracking
+
+Tracking shares the configuration with predict, i.e `conf`, `iou`, `show`. More configurations please refer
+to [predict page](https://docs.ultralytics.com/modes/predict/).
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.pt')
+ results = model.track(source="https://youtu.be/Zgi9g1ksQHc", conf=0.3, iou=0.5, show=True)
+ ```
+ === "CLI"
+
+ ```bash
+ yolo track model=yolov8n.pt source="https://youtu.be/Zgi9g1ksQHc" conf=0.3, iou=0.5 show
+
+ ```
+
+### Tracker
+
+We also support using a modified tracker config file, just copy a config file i.e `custom_tracker.yaml`
+from [ultralytics/tracker/cfg](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/tracker/cfg) and modify
+any configurations(expect the `tracker_type`) you need to.
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.pt')
+ results = model.track(source="https://youtu.be/Zgi9g1ksQHc", tracker='custom_tracker.yaml')
+ ```
+ === "CLI"
+
+ ```bash
+ yolo track model=yolov8n.pt source="https://youtu.be/Zgi9g1ksQHc" tracker='custom_tracker.yaml'
+ ```
+
+Please refer to [ultralytics/tracker/cfg](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/tracker/cfg)
+page
\ No newline at end of file
diff --git a/yolov8/docs/modes/train.md b/yolov8/docs/modes/train.md
new file mode 100644
index 0000000000000000000000000000000000000000..16c32ef84e35d0d0e1945599ed3d2581cb8388ad
--- /dev/null
+++ b/yolov8/docs/modes/train.md
@@ -0,0 +1,242 @@
+---
+comments: true
+description: Learn how to train custom YOLOv8 models on various datasets, configure hyperparameters, and use Ultralytics' YOLO for seamless training.
+keywords: YOLOv8, train mode, train a custom YOLOv8 model, hyperparameters, train a model, Comet, ClearML, TensorBoard, logging, loggers
+---
+
+
+
+**Train mode** is used for training a YOLOv8 model on a custom dataset. In this mode, the model is trained using the specified dataset and hyperparameters. The training process involves optimizing the model's parameters so that it can accurately predict the classes and locations of objects in an image.
+
+!!! tip "Tip"
+
+ * YOLOv8 datasets like COCO, VOC, ImageNet and many others automatically download on first use, i.e. `yolo train data=coco.yaml`
+
+## Usage Examples
+
+Train YOLOv8n on the COCO128 dataset for 100 epochs at image size 640. See Arguments section below for a full list of training arguments.
+
+!!! example "Single-GPU and CPU Training Example"
+
+ Device is determined automatically. If a GPU is available then it will be used, otherwise training will start on CPU.
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.yaml') # build a new model from YAML
+ model = YOLO('yolov8n.pt') # load a pretrained model (recommended for training)
+ model = YOLO('yolov8n.yaml').load('yolov8n.pt') # build from YAML and transfer weights
+
+ # Train the model
+ model.train(data='coco128.yaml', epochs=100, imgsz=640)
+ ```
+ === "CLI"
+
+ ```bash
+ # Build a new model from YAML and start training from scratch
+ yolo detect train data=coco128.yaml model=yolov8n.yaml epochs=100 imgsz=640
+
+ # Start training from a pretrained *.pt model
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=100 imgsz=640
+
+ # Build a new model from YAML, transfer pretrained weights to it and start training
+ yolo detect train data=coco128.yaml model=yolov8n.yaml pretrained=yolov8n.pt epochs=100 imgsz=640
+ ```
+
+### Multi-GPU Training
+
+The training device can be specified using the `device` argument. If no argument is passed GPU `device=0` will be used if available, otherwise `device=cpu` will be used.
+
+!!! example "Multi-GPU Training Example"
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load a pretrained model (recommended for training)
+
+ # Train the model with 2 GPUs
+ model.train(data='coco128.yaml', epochs=100, imgsz=640, device=[0, 1])
+ ```
+ === "CLI"
+
+ ```bash
+ # Start training from a pretrained *.pt model using GPUs 0 and 1
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=100 imgsz=640 device=0,1
+ ```
+
+### Apple M1 and M2 MPS Training
+
+With the support for Apple M1 and M2 chips integrated in the Ultralytics YOLO models, it's now possible to train your models on devices utilizing the powerful Metal Performance Shaders (MPS) framework. The MPS offers a high-performance way of executing computation and image processing tasks on Apple's custom silicon.
+
+To enable training on Apple M1 and M2 chips, you should specify 'mps' as your device when initiating the training process. Below is an example of how you could do this in Python and via the command line:
+
+!!! example "MPS Training Example"
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load a pretrained model (recommended for training)
+
+ # Train the model with 2 GPUs
+ model.train(data='coco128.yaml', epochs=100, imgsz=640, device='mps')
+ ```
+ === "CLI"
+
+ ```bash
+ # Start training from a pretrained *.pt model using GPUs 0 and 1
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=100 imgsz=640 device=mps
+ ```
+
+While leveraging the computational power of the M1/M2 chips, this enables more efficient processing of the training tasks. For more detailed guidance and advanced configuration options, please refer to the [PyTorch MPS documentation](https://pytorch.org/docs/stable/notes/mps.html).
+
+### Resuming Interrupted Trainings
+
+Resuming training from a previously saved state is a crucial feature when working with deep learning models. This can come in handy in various scenarios, like when the training process has been unexpectedly interrupted, or when you wish to continue training a model with new data or for more epochs.
+
+When training is resumed, Ultralytics YOLO loads the weights from the last saved model and also restores the optimizer state, learning rate scheduler, and the epoch number. This allows you to continue the training process seamlessly from where it was left off.
+
+You can easily resume training in Ultralytics YOLO by setting the `resume` argument to `True` when calling the `train` method, and specifying the path to the `.pt` file containing the partially trained model weights.
+
+Below is an example of how to resume an interrupted training using Python and via the command line:
+
+!!! example "Resume Training Example"
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('path/to/last.pt') # load a partially trained model
+
+ # Resume training
+ model.train(resume=True)
+ ```
+ === "CLI"
+
+ ```bash
+ # Resume an interrupted training
+ yolo train resume model=path/to/last.pt
+ ```
+
+By setting `resume=True`, the `train` function will continue training from where it left off, using the state stored in the 'path/to/last.pt' file. If the `resume` argument is omitted or set to `False`, the `train` function will start a new training session.
+
+Remember that checkpoints are saved at the end of every epoch by default, or at fixed interval using the `save_period` argument, so you must complete at least 1 epoch to resume a training run.
+
+## Arguments
+
+Training settings for YOLO models refer to the various hyperparameters and configurations used to train the model on a dataset. These settings can affect the model's performance, speed, and accuracy. Some common YOLO training settings include the batch size, learning rate, momentum, and weight decay. Other factors that may affect the training process include the choice of optimizer, the choice of loss function, and the size and composition of the training dataset. It is important to carefully tune and experiment with these settings to achieve the best possible performance for a given task.
+
+| Key | Value | Description |
+|-------------------|----------|-----------------------------------------------------------------------------------|
+| `model` | `None` | path to model file, i.e. yolov8n.pt, yolov8n.yaml |
+| `data` | `None` | path to data file, i.e. coco128.yaml |
+| `epochs` | `100` | number of epochs to train for |
+| `patience` | `50` | epochs to wait for no observable improvement for early stopping of training |
+| `batch` | `16` | number of images per batch (-1 for AutoBatch) |
+| `imgsz` | `640` | size of input images as integer or w,h |
+| `save` | `True` | save train checkpoints and predict results |
+| `save_period` | `-1` | Save checkpoint every x epochs (disabled if < 1) |
+| `cache` | `False` | True/ram, disk or False. Use cache for data loading |
+| `device` | `None` | device to run on, i.e. cuda device=0 or device=0,1,2,3 or device=cpu |
+| `workers` | `8` | number of worker threads for data loading (per RANK if DDP) |
+| `project` | `None` | project name |
+| `name` | `None` | experiment name |
+| `exist_ok` | `False` | whether to overwrite existing experiment |
+| `pretrained` | `False` | whether to use a pretrained model |
+| `optimizer` | `'auto'` | optimizer to use, choices=[SGD, Adam, Adamax, AdamW, NAdam, RAdam, RMSProp, auto] |
+| `verbose` | `False` | whether to print verbose output |
+| `seed` | `0` | random seed for reproducibility |
+| `deterministic` | `True` | whether to enable deterministic mode |
+| `single_cls` | `False` | train multi-class data as single-class |
+| `rect` | `False` | rectangular training with each batch collated for minimum padding |
+| `cos_lr` | `False` | use cosine learning rate scheduler |
+| `close_mosaic` | `0` | (int) disable mosaic augmentation for final epochs |
+| `resume` | `False` | resume training from last checkpoint |
+| `amp` | `True` | Automatic Mixed Precision (AMP) training, choices=[True, False] |
+| `fraction` | `1.0` | dataset fraction to train on (default is 1.0, all images in train set) |
+| `profile` | `False` | profile ONNX and TensorRT speeds during training for loggers |
+| `lr0` | `0.01` | initial learning rate (i.e. SGD=1E-2, Adam=1E-3) |
+| `lrf` | `0.01` | final learning rate (lr0 * lrf) |
+| `momentum` | `0.937` | SGD momentum/Adam beta1 |
+| `weight_decay` | `0.0005` | optimizer weight decay 5e-4 |
+| `warmup_epochs` | `3.0` | warmup epochs (fractions ok) |
+| `warmup_momentum` | `0.8` | warmup initial momentum |
+| `warmup_bias_lr` | `0.1` | warmup initial bias lr |
+| `box` | `7.5` | box loss gain |
+| `cls` | `0.5` | cls loss gain (scale with pixels) |
+| `dfl` | `1.5` | dfl loss gain |
+| `pose` | `12.0` | pose loss gain (pose-only) |
+| `kobj` | `2.0` | keypoint obj loss gain (pose-only) |
+| `label_smoothing` | `0.0` | label smoothing (fraction) |
+| `nbs` | `64` | nominal batch size |
+| `overlap_mask` | `True` | masks should overlap during training (segment train only) |
+| `mask_ratio` | `4` | mask downsample ratio (segment train only) |
+| `dropout` | `0.0` | use dropout regularization (classify train only) |
+| `val` | `True` | validate/test during training |
+
+## Logging
+
+In training a YOLOv8 model, you might find it valuable to keep track of the model's performance over time. This is where logging comes into play. Ultralytics' YOLO provides support for three types of loggers - Comet, ClearML, and TensorBoard.
+
+To use a logger, select it from the dropdown menu in the code snippet above and run it. The chosen logger will be installed and initialized.
+
+### Comet
+
+[Comet](https://www.comet.ml/site/) is a platform that allows data scientists and developers to track, compare, explain and optimize experiments and models. It provides functionalities such as real-time metrics, code diffs, and hyperparameters tracking.
+
+To use Comet:
+
+```python
+# pip install comet_ml
+import comet_ml
+
+comet_ml.init()
+```
+
+Remember to sign in to your Comet account on their website and get your API key. You will need to add this to your environment variables or your script to log your experiments.
+
+### ClearML
+
+[ClearML](https://www.clear.ml/) is an open-source platform that automates tracking of experiments and helps with efficient sharing of resources. It is designed to help teams manage, execute, and reproduce their ML work more efficiently.
+
+To use ClearML:
+
+```python
+# pip install clearml
+import clearml
+
+clearml.browser_login()
+```
+
+After running this script, you will need to sign in to your ClearML account on the browser and authenticate your session.
+
+### TensorBoard
+
+[TensorBoard](https://www.tensorflow.org/tensorboard) is a visualization toolkit for TensorFlow. It allows you to visualize your TensorFlow graph, plot quantitative metrics about the execution of your graph, and show additional data like images that pass through it.
+
+To use TensorBoard in [Google Colab](https://colab.research.google.com/github/ultralytics/ultralytics/blob/main/examples/tutorial.ipynb):
+
+```bash
+load_ext tensorboard
+tensorboard --logdir ultralytics/runs # replace with 'runs' directory
+```
+
+To use TensorBoard locally run the below command and view results at http://localhost:6006/.
+
+```bash
+tensorboard --logdir ultralytics/runs # replace with 'runs' directory
+```
+
+This will load TensorBoard and direct it to the directory where your training logs are saved.
+
+After setting up your logger, you can then proceed with your model training. All training metrics will be automatically logged in your chosen platform, and you can access these logs to monitor your model's performance over time, compare different models, and identify areas for improvement.
\ No newline at end of file
diff --git a/yolov8/docs/modes/val.md b/yolov8/docs/modes/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..4ffff738dbe818043e2ed37e62ee4580b6ba5788
--- /dev/null
+++ b/yolov8/docs/modes/val.md
@@ -0,0 +1,64 @@
+---
+comments: true
+description: Validate and improve YOLOv8n model accuracy on COCO128 and other datasets using hyperparameter & configuration tuning, in Val mode.
+keywords: Ultralytics, YOLO, YOLOv8, Val, Validation, Hyperparameters, Performance, Accuracy, Generalization, COCO, Export Formats, PyTorch
+---
+
+
+
+**Val mode** is used for validating a YOLOv8 model after it has been trained. In this mode, the model is evaluated on a validation set to measure its accuracy and generalization performance. This mode can be used to tune the hyperparameters of the model to improve its performance.
+
+!!! tip "Tip"
+
+ * YOLOv8 models automatically remember their training settings, so you can validate a model at the same image size and on the original dataset easily with just `yolo val model=yolov8n.pt` or `model('yolov8n.pt').val()`
+
+## Usage Examples
+
+Validate trained YOLOv8n model accuracy on the COCO128 dataset. No argument need to passed as the `model` retains it's training `data` and arguments as model attributes. See Arguments section below for a full list of export arguments.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Validate the model
+ metrics = model.val() # no arguments needed, dataset and settings remembered
+ metrics.box.map # map50-95
+ metrics.box.map50 # map50
+ metrics.box.map75 # map75
+ metrics.box.maps # a list contains map50-95 of each category
+ ```
+ === "CLI"
+
+ ```bash
+ yolo detect val model=yolov8n.pt # val official model
+ yolo detect val model=path/to/best.pt # val custom model
+ ```
+
+## Arguments
+
+Validation settings for YOLO models refer to the various hyperparameters and configurations used to evaluate the model's performance on a validation dataset. These settings can affect the model's performance, speed, and accuracy. Some common YOLO validation settings include the batch size, the frequency with which validation is performed during training, and the metrics used to evaluate the model's performance. Other factors that may affect the validation process include the size and composition of the validation dataset and the specific task the model is being used for. It is important to carefully tune and experiment with these settings to ensure that the model is performing well on the validation dataset and to detect and prevent overfitting.
+
+| Key | Value | Description |
+|---------------|---------|--------------------------------------------------------------------|
+| `data` | `None` | path to data file, i.e. coco128.yaml |
+| `imgsz` | `640` | image size as scalar or (h, w) list, i.e. (640, 480) |
+| `batch` | `16` | number of images per batch (-1 for AutoBatch) |
+| `save_json` | `False` | save results to JSON file |
+| `save_hybrid` | `False` | save hybrid version of labels (labels + additional predictions) |
+| `conf` | `0.001` | object confidence threshold for detection |
+| `iou` | `0.6` | intersection over union (IoU) threshold for NMS |
+| `max_det` | `300` | maximum number of detections per image |
+| `half` | `True` | use half precision (FP16) |
+| `device` | `None` | device to run on, i.e. cuda device=0/1/2/3 or device=cpu |
+| `dnn` | `False` | use OpenCV DNN for ONNX inference |
+| `plots` | `False` | show plots during training |
+| `rect` | `False` | rectangular val with each batch collated for minimum padding |
+| `split` | `val` | dataset split to use for validation, i.e. 'val', 'test' or 'train' |
+|
\ No newline at end of file
diff --git a/yolov8/docs/overrides/partials/comments.html b/yolov8/docs/overrides/partials/comments.html
new file mode 100644
index 0000000000000000000000000000000000000000..ff1455b25eac59b84f260561ce177cf33dde8d33
--- /dev/null
+++ b/yolov8/docs/overrides/partials/comments.html
@@ -0,0 +1,50 @@
+{% if page.meta.comments %}
+
+
+
+
+
+
+
+{% endif %}
diff --git a/yolov8/docs/overrides/partials/source-file.html b/yolov8/docs/overrides/partials/source-file.html
new file mode 100644
index 0000000000000000000000000000000000000000..84e2ab1f7dad99a3c8971dd6cb931b8ad0bb6d5b
--- /dev/null
+++ b/yolov8/docs/overrides/partials/source-file.html
@@ -0,0 +1,26 @@
+{% import "partials/language.html" as lang with context %}
+
+
+
+
+
+
+
+
+ {% if page.meta.git_revision_date_localized %}
+ 📅 {{ lang.t("source.file.date.updated") }}:
+ {{ page.meta.git_revision_date_localized }}
+ {% if page.meta.git_creation_date_localized %}
+
+ 🎂 {{ lang.t("source.file.date.created") }}:
+ {{ page.meta.git_creation_date_localized }}
+ {% endif %}
+
+
+ {% elif page.meta.revision_date %}
+ 📅 {{ lang.t("source.file.date.updated") }}:
+ {{ page.meta.revision_date }}
+ {% endif %}
+
+
diff --git a/yolov8/docs/quickstart.md b/yolov8/docs/quickstart.md
new file mode 100644
index 0000000000000000000000000000000000000000..cd8d63054f2721eb349070a6e86890eecac54efc
--- /dev/null
+++ b/yolov8/docs/quickstart.md
@@ -0,0 +1,183 @@
+---
+comments: true
+description: Install and use YOLOv8 via CLI or Python. Run single-line commands or integrate with Python projects for object detection, segmentation, and classification.
+keywords: YOLOv8, object detection, segmentation, classification, pip, git, CLI, Python
+---
+
+## Install Ultralytics
+
+Ultralytics provides various installation methods including pip, conda, and Docker. Install YOLOv8 via the `ultralytics` pip package for the latest stable release or by cloning the [Ultralytics GitHub repository](https://github.com/ultralytics/ultralytics) for the most up-to-date version. Docker can be used to execute the package in an isolated container, avoiding local installation.
+
+!!! example "Install"
+
+ === "Pip install (recommended)"
+ Install the `ultralytics` package using pip, or update an existing installation by running `pip install -U ultralytics`. Visit the Python Package Index (PyPI) for more details on the `ultralytics` package: [https://pypi.org/project/ultralytics/](https://pypi.org/project/ultralytics/).
+
+ [](https://badge.fury.io/py/ultralytics) [](https://pepy.tech/project/ultralytics)
+
+ ```bash
+ # Install the ultralytics package using pip
+ pip install ultralytics
+ ```
+
+ === "Conda install"
+ Conda is an alternative package manager to pip which may also be used for installation. Visit Anaconda for more details at [https://anaconda.org/conda-forge/ultralytics](https://anaconda.org/conda-forge/ultralytics). Ultralytics feedstock repository for updating the conda package is at [https://github.com/conda-forge/ultralytics-feedstock/](https://github.com/conda-forge/ultralytics-feedstock/).
+
+
+ [](https://anaconda.org/conda-forge/ultralytics) [](https://anaconda.org/conda-forge/ultralytics) [](https://anaconda.org/conda-forge/ultralytics) [](https://anaconda.org/conda-forge/ultralytics)
+
+ ```bash
+ # Install the ultralytics package using conda
+ conda install ultralytics
+ ```
+
+ === "Git clone"
+ Clone the `ultralytics` repository if you are interested in contributing to the development or wish to experiment with the latest source code. After cloning, navigate into the directory and install the package in editable mode `-e` using pip.
+ ```bash
+ # Clone the ultralytics repository
+ git clone https://github.com/ultralytics/ultralytics
+
+ # Navigate to the cloned directory
+ cd ultralytics
+
+ # Install the package in editable mode for development
+ pip install -e .
+ ```
+
+ === "Docker"
+ Utilize Docker to execute the `ultralytics` package in an isolated container. By employing the official `ultralytics` image from [Docker Hub](https://hub.docker.com/r/ultralytics/ultralytics), you can avoid local installation. Below are the commands to get the latest image and execute it:
+
+
+
+ ```bash
+ # Set image name as a variable
+ t=ultralytics/ultralytics:latest
+
+ # Pull the latest ultralytics image from Docker Hub
+ sudo docker pull $t
+
+ # Run the ultralytics image in a container with GPU support
+ sudo docker run -it --ipc=host --gpus all $t
+ ```
+
+ The above command initializes a Docker container with the latest `ultralytics` image. The `-it` flag assigns a pseudo-TTY and maintains stdin open, enabling you to interact with the container. The `--ipc=host` flag sets the IPC (Inter-Process Communication) namespace to the host, which is essential for sharing memory between processes. The `--gpus all` flag enables access to all available GPUs inside the container, which is crucial for tasks that require GPU computation.
+
+ Note: To work with files on your local machine within the container, use Docker volumes for mounting a local directory into the container:
+
+ ```bash
+ # Mount local directory to a directory inside the container
+ sudo docker run -it --ipc=host --gpus all -v /path/on/host:/path/in/container $t
+ ```
+
+ Alter `/path/on/host` with the directory path on your local machine, and `/path/in/container` with the desired path inside the Docker container for accessibility.
+
+See the `ultralytics` [requirements.txt](https://github.com/ultralytics/ultralytics/blob/main/requirements.txt) file for a list of dependencies. Note that all examples above install all required dependencies.
+
+!!! tip "Tip"
+
+ PyTorch requirements vary by operating system and CUDA requirements, so it's recommended to install PyTorch first following instructions at [https://pytorch.org/get-started/locally](https://pytorch.org/get-started/locally).
+
+
+
+
+
+## Use Ultralytics with CLI
+
+The Ultralytics command line interface (CLI) allows for simple single-line commands without the need for a Python environment.
+CLI requires no customization or Python code. You can simply run all tasks from the terminal with the `yolo` command. Check out the [CLI Guide](usage/cli.md) to learn more about using YOLOv8 from the command line.
+
+!!! example
+
+ === "Syntax"
+
+ Ultralytics `yolo` commands use the following syntax:
+ ```bash
+ yolo TASK MODE ARGS
+
+ Where TASK (optional) is one of [detect, segment, classify]
+ MODE (required) is one of [train, val, predict, export, track]
+ ARGS (optional) are any number of custom 'arg=value' pairs like 'imgsz=320' that override defaults.
+ ```
+ See all ARGS in the full [Configuration Guide](usage/cfg.md) or with `yolo cfg`
+
+ === "Train"
+
+ Train a detection model for 10 epochs with an initial learning_rate of 0.01
+ ```bash
+ yolo train data=coco128.yaml model=yolov8n.pt epochs=10 lr0=0.01
+ ```
+
+ === "Predict"
+
+ Predict a YouTube video using a pretrained segmentation model at image size 320:
+ ```bash
+ yolo predict model=yolov8n-seg.pt source='https://youtu.be/Zgi9g1ksQHc' imgsz=320
+ ```
+
+ === "Val"
+
+ Val a pretrained detection model at batch-size 1 and image size 640:
+ ```bash
+ yolo val model=yolov8n.pt data=coco128.yaml batch=1 imgsz=640
+ ```
+
+ === "Export"
+
+ Export a YOLOv8n classification model to ONNX format at image size 224 by 128 (no TASK required)
+ ```bash
+ yolo export model=yolov8n-cls.pt format=onnx imgsz=224,128
+ ```
+
+ === "Special"
+
+ Run special commands to see version, view settings, run checks and more:
+ ```bash
+ yolo help
+ yolo checks
+ yolo version
+ yolo settings
+ yolo copy-cfg
+ yolo cfg
+ ```
+
+!!! warning "Warning"
+
+ Arguments must be passed as `arg=val` pairs, split by an equals `=` sign and delimited by spaces ` ` between pairs. Do not use `--` argument prefixes or commas `,` between arguments.
+
+ - `yolo predict model=yolov8n.pt imgsz=640 conf=0.25` ✅
+ - `yolo predict model yolov8n.pt imgsz 640 conf 0.25` ❌
+ - `yolo predict --model yolov8n.pt --imgsz 640 --conf 0.25` ❌
+
+[CLI Guide](usage/cli.md){ .md-button .md-button--primary}
+
+## Use Ultralytics with Python
+
+YOLOv8's Python interface allows for seamless integration into your Python projects, making it easy to load, run, and process the model's output. Designed with simplicity and ease of use in mind, the Python interface enables users to quickly implement object detection, segmentation, and classification in their projects. This makes YOLOv8's Python interface an invaluable tool for anyone looking to incorporate these functionalities into their Python projects.
+
+For example, users can load a model, train it, evaluate its performance on a validation set, and even export it to ONNX format with just a few lines of code. Check out the [Python Guide](usage/python.md) to learn more about using YOLOv8 within your Python projects.
+
+!!! example
+
+ ```python
+ from ultralytics import YOLO
+
+ # Create a new YOLO model from scratch
+ model = YOLO('yolov8n.yaml')
+
+ # Load a pretrained YOLO model (recommended for training)
+ model = YOLO('yolov8n.pt')
+
+ # Train the model using the 'coco128.yaml' dataset for 3 epochs
+ results = model.train(data='coco128.yaml', epochs=3)
+
+ # Evaluate the model's performance on the validation set
+ results = model.val()
+
+ # Perform object detection on an image using the model
+ results = model('https://ultralytics.com/images/bus.jpg')
+
+ # Export the model to ONNX format
+ success = model.export(format='onnx')
+ ```
+
+[Python Guide](usage/python.md){.md-button .md-button--primary}
\ No newline at end of file
diff --git a/yolov8/docs/reference/hub/__init__.md b/yolov8/docs/reference/hub/__init__.md
new file mode 100644
index 0000000000000000000000000000000000000000..767ec1485ab82d49262fed742302243d2313dea4
--- /dev/null
+++ b/yolov8/docs/reference/hub/__init__.md
@@ -0,0 +1,44 @@
+---
+description: Access Ultralytics HUB, manage API keys, train models, and export in various formats with ease using the HUB API.
+keywords: Ultralytics, YOLO, Docs HUB, API, login, logout, reset model, export model, check dataset, HUBDatasetStats, YOLO training, YOLO model
+---
+
+## login
+---
+### ::: ultralytics.hub.login
+
+
+## logout
+---
+### ::: ultralytics.hub.logout
+
+
+## start
+---
+### ::: ultralytics.hub.start
+
+
+## reset_model
+---
+### ::: ultralytics.hub.reset_model
+
+
+## export_fmts_hub
+---
+### ::: ultralytics.hub.export_fmts_hub
+
+
+## export_model
+---
+### ::: ultralytics.hub.export_model
+
+
+## get_export
+---
+### ::: ultralytics.hub.get_export
+
+
+## check_dataset
+---
+### ::: ultralytics.hub.check_dataset
+
diff --git a/yolov8/docs/reference/hub/auth.md b/yolov8/docs/reference/hub/auth.md
new file mode 100644
index 0000000000000000000000000000000000000000..2098e8eb5dcfeba776339c65a595c82fd47782dd
--- /dev/null
+++ b/yolov8/docs/reference/hub/auth.md
@@ -0,0 +1,9 @@
+---
+description: Learn how to use Ultralytics hub authentication in your projects with examples and guidelines from the Auth page on Ultralytics Docs.
+keywords: Ultralytics, ultralytics hub, api keys, authentication, collab accounts, requests, hub management, monitoring
+---
+
+## Auth
+---
+### ::: ultralytics.hub.auth.Auth
+
diff --git a/yolov8/docs/reference/hub/session.md b/yolov8/docs/reference/hub/session.md
new file mode 100644
index 0000000000000000000000000000000000000000..8fe82c4f20cc86a45dd2fa1e672779616ab428f7
--- /dev/null
+++ b/yolov8/docs/reference/hub/session.md
@@ -0,0 +1,9 @@
+---
+description: Accelerate your AI development with the Ultralytics HUB Training Session. High-performance training of object detection models.
+keywords: YOLOv5, object detection, HUBTrainingSession, custom models, Ultralytics Docs
+---
+
+## HUBTrainingSession
+---
+### ::: ultralytics.hub.session.HUBTrainingSession
+
diff --git a/yolov8/docs/reference/hub/utils.md b/yolov8/docs/reference/hub/utils.md
new file mode 100644
index 0000000000000000000000000000000000000000..9b896b048b2889f015fb800bbf775a51320f20f2
--- /dev/null
+++ b/yolov8/docs/reference/hub/utils.md
@@ -0,0 +1,24 @@
+---
+description: Explore Ultralytics events, including 'request_with_credentials' and 'smart_request', to improve your project's performance and efficiency.
+keywords: Ultralytics, Hub Utils, API Documentation, Python, requests_with_progress, Events, classes, usage, examples
+---
+
+## Events
+---
+### ::: ultralytics.hub.utils.Events
+
+
+## request_with_credentials
+---
+### ::: ultralytics.hub.utils.request_with_credentials
+
+
+## requests_with_progress
+---
+### ::: ultralytics.hub.utils.requests_with_progress
+
+
+## smart_request
+---
+### ::: ultralytics.hub.utils.smart_request
+
diff --git a/yolov8/docs/reference/nn/autobackend.md b/yolov8/docs/reference/nn/autobackend.md
new file mode 100644
index 0000000000000000000000000000000000000000..ccd10773e80630b2a68e63a740eb075f054b3f64
--- /dev/null
+++ b/yolov8/docs/reference/nn/autobackend.md
@@ -0,0 +1,14 @@
+---
+description: Ensure class names match filenames for easy imports. Use AutoBackend to automatically rename and refactor model files.
+keywords: AutoBackend, ultralytics, nn, autobackend, check class names, neural network
+---
+
+## AutoBackend
+---
+### ::: ultralytics.nn.autobackend.AutoBackend
+
+
+## check_class_names
+---
+### ::: ultralytics.nn.autobackend.check_class_names
+
diff --git a/yolov8/docs/reference/nn/autoshape.md b/yolov8/docs/reference/nn/autoshape.md
new file mode 100644
index 0000000000000000000000000000000000000000..b009e090a2a2ee3ca0ca9314537bb9a7e32b71b7
--- /dev/null
+++ b/yolov8/docs/reference/nn/autoshape.md
@@ -0,0 +1,14 @@
+---
+description: Detect 80+ object categories with bounding box coordinates and class probabilities using AutoShape in Ultralytics YOLO. Explore Detections now.
+keywords: Ultralytics, YOLO, docs, autoshape, detections, object detection, customized shapes, bounding boxes, computer vision
+---
+
+## AutoShape
+---
+### ::: ultralytics.nn.autoshape.AutoShape
+
+
+## Detections
+---
+### ::: ultralytics.nn.autoshape.Detections
+
diff --git a/yolov8/docs/reference/nn/modules/block.md b/yolov8/docs/reference/nn/modules/block.md
new file mode 100644
index 0000000000000000000000000000000000000000..3f1a548253175c41b986b6198ab9b5dbeef24445
--- /dev/null
+++ b/yolov8/docs/reference/nn/modules/block.md
@@ -0,0 +1,89 @@
+---
+description: Explore ultralytics.nn.modules.block to build powerful YOLO object detection models. Master DFL, HGStem, SPP, CSP components and more.
+keywords: Ultralytics, NN Modules, Blocks, DFL, HGStem, SPP, C1, C2f, C3x, C3TR, GhostBottleneck, BottleneckCSP, Computer Vision
+---
+
+## DFL
+---
+### ::: ultralytics.nn.modules.block.DFL
+
+
+## Proto
+---
+### ::: ultralytics.nn.modules.block.Proto
+
+
+## HGStem
+---
+### ::: ultralytics.nn.modules.block.HGStem
+
+
+## HGBlock
+---
+### ::: ultralytics.nn.modules.block.HGBlock
+
+
+## SPP
+---
+### ::: ultralytics.nn.modules.block.SPP
+
+
+## SPPF
+---
+### ::: ultralytics.nn.modules.block.SPPF
+
+
+## C1
+---
+### ::: ultralytics.nn.modules.block.C1
+
+
+## C2
+---
+### ::: ultralytics.nn.modules.block.C2
+
+
+## C2f
+---
+### ::: ultralytics.nn.modules.block.C2f
+
+
+## C3
+---
+### ::: ultralytics.nn.modules.block.C3
+
+
+## C3x
+---
+### ::: ultralytics.nn.modules.block.C3x
+
+
+## RepC3
+---
+### ::: ultralytics.nn.modules.block.RepC3
+
+
+## C3TR
+---
+### ::: ultralytics.nn.modules.block.C3TR
+
+
+## C3Ghost
+---
+### ::: ultralytics.nn.modules.block.C3Ghost
+
+
+## GhostBottleneck
+---
+### ::: ultralytics.nn.modules.block.GhostBottleneck
+
+
+## Bottleneck
+---
+### ::: ultralytics.nn.modules.block.Bottleneck
+
+
+## BottleneckCSP
+---
+### ::: ultralytics.nn.modules.block.BottleneckCSP
+
diff --git a/yolov8/docs/reference/nn/modules/conv.md b/yolov8/docs/reference/nn/modules/conv.md
new file mode 100644
index 0000000000000000000000000000000000000000..6a5df52c3949d96678dab3d252a6228470c84a59
--- /dev/null
+++ b/yolov8/docs/reference/nn/modules/conv.md
@@ -0,0 +1,74 @@
+---
+description: Explore convolutional neural network modules & techniques such as LightConv, DWConv, ConvTranspose, GhostConv, CBAM & autopad with Ultralytics Docs.
+keywords: Ultralytics, Convolutional Neural Network, Conv2, DWConv, ConvTranspose, GhostConv, ChannelAttention, CBAM, autopad
+---
+
+## Conv
+---
+### ::: ultralytics.nn.modules.conv.Conv
+
+
+## Conv2
+---
+### ::: ultralytics.nn.modules.conv.Conv2
+
+
+## LightConv
+---
+### ::: ultralytics.nn.modules.conv.LightConv
+
+
+## DWConv
+---
+### ::: ultralytics.nn.modules.conv.DWConv
+
+
+## DWConvTranspose2d
+---
+### ::: ultralytics.nn.modules.conv.DWConvTranspose2d
+
+
+## ConvTranspose
+---
+### ::: ultralytics.nn.modules.conv.ConvTranspose
+
+
+## Focus
+---
+### ::: ultralytics.nn.modules.conv.Focus
+
+
+## GhostConv
+---
+### ::: ultralytics.nn.modules.conv.GhostConv
+
+
+## RepConv
+---
+### ::: ultralytics.nn.modules.conv.RepConv
+
+
+## ChannelAttention
+---
+### ::: ultralytics.nn.modules.conv.ChannelAttention
+
+
+## SpatialAttention
+---
+### ::: ultralytics.nn.modules.conv.SpatialAttention
+
+
+## CBAM
+---
+### ::: ultralytics.nn.modules.conv.CBAM
+
+
+## Concat
+---
+### ::: ultralytics.nn.modules.conv.Concat
+
+
+## autopad
+---
+### ::: ultralytics.nn.modules.conv.autopad
+
diff --git a/yolov8/docs/reference/nn/modules/head.md b/yolov8/docs/reference/nn/modules/head.md
new file mode 100644
index 0000000000000000000000000000000000000000..7a460b521f69955da05050b16109e081c9be680c
--- /dev/null
+++ b/yolov8/docs/reference/nn/modules/head.md
@@ -0,0 +1,29 @@
+---
+description: 'Learn about Ultralytics YOLO modules: Segment, Classify, and RTDETRDecoder. Optimize object detection and classification in your project.'
+keywords: Ultralytics, YOLO, object detection, pose estimation, RTDETRDecoder, modules, classes, documentation
+---
+
+## Detect
+---
+### ::: ultralytics.nn.modules.head.Detect
+
+
+## Segment
+---
+### ::: ultralytics.nn.modules.head.Segment
+
+
+## Pose
+---
+### ::: ultralytics.nn.modules.head.Pose
+
+
+## Classify
+---
+### ::: ultralytics.nn.modules.head.Classify
+
+
+## RTDETRDecoder
+---
+### ::: ultralytics.nn.modules.head.RTDETRDecoder
+
diff --git a/yolov8/docs/reference/nn/modules/transformer.md b/yolov8/docs/reference/nn/modules/transformer.md
new file mode 100644
index 0000000000000000000000000000000000000000..a927f9b806efc5b10201f98055dca4eaf61cf2c0
--- /dev/null
+++ b/yolov8/docs/reference/nn/modules/transformer.md
@@ -0,0 +1,54 @@
+---
+description: Explore the Ultralytics nn modules pages on Transformer and MLP blocks, LayerNorm2d, and Deformable Transformer Decoder Layer.
+keywords: Ultralytics, NN Modules, TransformerEncoderLayer, TransformerLayer, MLPBlock, LayerNorm2d, DeformableTransformerDecoderLayer, examples, code snippets, tutorials
+---
+
+## TransformerEncoderLayer
+---
+### ::: ultralytics.nn.modules.transformer.TransformerEncoderLayer
+
+
+## AIFI
+---
+### ::: ultralytics.nn.modules.transformer.AIFI
+
+
+## TransformerLayer
+---
+### ::: ultralytics.nn.modules.transformer.TransformerLayer
+
+
+## TransformerBlock
+---
+### ::: ultralytics.nn.modules.transformer.TransformerBlock
+
+
+## MLPBlock
+---
+### ::: ultralytics.nn.modules.transformer.MLPBlock
+
+
+## MLP
+---
+### ::: ultralytics.nn.modules.transformer.MLP
+
+
+## LayerNorm2d
+---
+### ::: ultralytics.nn.modules.transformer.LayerNorm2d
+
+
+## MSDeformAttn
+---
+### ::: ultralytics.nn.modules.transformer.MSDeformAttn
+
+
+## DeformableTransformerDecoderLayer
+---
+### ::: ultralytics.nn.modules.transformer.DeformableTransformerDecoderLayer
+
+
+## DeformableTransformerDecoder
+---
+### ::: ultralytics.nn.modules.transformer.DeformableTransformerDecoder
+
diff --git a/yolov8/docs/reference/nn/modules/utils.md b/yolov8/docs/reference/nn/modules/utils.md
new file mode 100644
index 0000000000000000000000000000000000000000..94c4f3e51389b46c1efed13f1db05947314102b1
--- /dev/null
+++ b/yolov8/docs/reference/nn/modules/utils.md
@@ -0,0 +1,29 @@
+---
+description: 'Learn about Ultralytics NN modules: get_clones, linear_init_, and multi_scale_deformable_attn_pytorch. Code examples and usage tips.'
+keywords: Ultralytics, NN Utils, Docs, PyTorch, bias initialization, linear initialization, multi-scale deformable attention
+---
+
+## _get_clones
+---
+### ::: ultralytics.nn.modules.utils._get_clones
+
+
+## bias_init_with_prob
+---
+### ::: ultralytics.nn.modules.utils.bias_init_with_prob
+
+
+## linear_init_
+---
+### ::: ultralytics.nn.modules.utils.linear_init_
+
+
+## inverse_sigmoid
+---
+### ::: ultralytics.nn.modules.utils.inverse_sigmoid
+
+
+## multi_scale_deformable_attn_pytorch
+---
+### ::: ultralytics.nn.modules.utils.multi_scale_deformable_attn_pytorch
+
diff --git a/yolov8/docs/reference/nn/tasks.md b/yolov8/docs/reference/nn/tasks.md
new file mode 100644
index 0000000000000000000000000000000000000000..010cea15e5351669948fc1aacaaa33b59cc66943
--- /dev/null
+++ b/yolov8/docs/reference/nn/tasks.md
@@ -0,0 +1,74 @@
+---
+description: Learn how to work with Ultralytics YOLO Detection, Segmentation & Classification Models, load weights and parse models in PyTorch.
+keywords: neural network, deep learning, computer vision, object detection, image segmentation, image classification, model ensemble, PyTorch
+---
+
+## BaseModel
+---
+### ::: ultralytics.nn.tasks.BaseModel
+
+
+## DetectionModel
+---
+### ::: ultralytics.nn.tasks.DetectionModel
+
+
+## SegmentationModel
+---
+### ::: ultralytics.nn.tasks.SegmentationModel
+
+
+## PoseModel
+---
+### ::: ultralytics.nn.tasks.PoseModel
+
+
+## ClassificationModel
+---
+### ::: ultralytics.nn.tasks.ClassificationModel
+
+
+## RTDETRDetectionModel
+---
+### ::: ultralytics.nn.tasks.RTDETRDetectionModel
+
+
+## Ensemble
+---
+### ::: ultralytics.nn.tasks.Ensemble
+
+
+## torch_safe_load
+---
+### ::: ultralytics.nn.tasks.torch_safe_load
+
+
+## attempt_load_weights
+---
+### ::: ultralytics.nn.tasks.attempt_load_weights
+
+
+## attempt_load_one_weight
+---
+### ::: ultralytics.nn.tasks.attempt_load_one_weight
+
+
+## parse_model
+---
+### ::: ultralytics.nn.tasks.parse_model
+
+
+## yaml_model_load
+---
+### ::: ultralytics.nn.tasks.yaml_model_load
+
+
+## guess_model_scale
+---
+### ::: ultralytics.nn.tasks.guess_model_scale
+
+
+## guess_model_task
+---
+### ::: ultralytics.nn.tasks.guess_model_task
+
diff --git a/yolov8/docs/reference/tracker/track.md b/yolov8/docs/reference/tracker/track.md
new file mode 100644
index 0000000000000000000000000000000000000000..88db7f264fe2ceba31ec703fb53e8a4dd3dbe46c
--- /dev/null
+++ b/yolov8/docs/reference/tracker/track.md
@@ -0,0 +1,19 @@
+---
+description: Learn how to register custom event-tracking and track predictions with Ultralytics YOLO via on_predict_start and register_tracker methods.
+keywords: Ultralytics YOLO, tracker registration, on_predict_start, object detection
+---
+
+## on_predict_start
+---
+### ::: ultralytics.tracker.track.on_predict_start
+
+
+## on_predict_postprocess_end
+---
+### ::: ultralytics.tracker.track.on_predict_postprocess_end
+
+
+## register_tracker
+---
+### ::: ultralytics.tracker.track.register_tracker
+
diff --git a/yolov8/docs/reference/tracker/trackers/basetrack.md b/yolov8/docs/reference/tracker/trackers/basetrack.md
new file mode 100644
index 0000000000000000000000000000000000000000..ab5cf58cd9b0d2e1dfb5213b0c20644fa3bebad6
--- /dev/null
+++ b/yolov8/docs/reference/tracker/trackers/basetrack.md
@@ -0,0 +1,14 @@
+---
+description: 'TrackState: A comprehensive guide to Ultralytics tracker''s BaseTrack for monitoring model performance. Improve your tracking capabilities now!'
+keywords: object detection, object tracking, Ultralytics YOLO, TrackState, workflow improvement
+---
+
+## TrackState
+---
+### ::: ultralytics.tracker.trackers.basetrack.TrackState
+
+
+## BaseTrack
+---
+### ::: ultralytics.tracker.trackers.basetrack.BaseTrack
+
diff --git a/yolov8/docs/reference/tracker/trackers/bot_sort.md b/yolov8/docs/reference/tracker/trackers/bot_sort.md
new file mode 100644
index 0000000000000000000000000000000000000000..b53e9b14318dfd3e615c9ef0f7e55fa1ef4f1940
--- /dev/null
+++ b/yolov8/docs/reference/tracker/trackers/bot_sort.md
@@ -0,0 +1,14 @@
+---
+description: '"Optimize tracking with Ultralytics BOTrack. Easily sort and track bots with BOTSORT. Streamline data collection for improved performance."'
+keywords: BOTrack, Ultralytics YOLO Docs, features, usage
+---
+
+## BOTrack
+---
+### ::: ultralytics.tracker.trackers.bot_sort.BOTrack
+
+
+## BOTSORT
+---
+### ::: ultralytics.tracker.trackers.bot_sort.BOTSORT
+
diff --git a/yolov8/docs/reference/tracker/trackers/byte_tracker.md b/yolov8/docs/reference/tracker/trackers/byte_tracker.md
new file mode 100644
index 0000000000000000000000000000000000000000..797be1db66284e90904cd520f149a42a11dc1ed0
--- /dev/null
+++ b/yolov8/docs/reference/tracker/trackers/byte_tracker.md
@@ -0,0 +1,14 @@
+---
+description: Learn how to track ByteAI model sizes and tips for model optimization with STrack, a byte tracking tool from Ultralytics.
+keywords: Byte Tracker, Ultralytics STrack, application monitoring, bytes sent, bytes received, code examples, setup instructions
+---
+
+## STrack
+---
+### ::: ultralytics.tracker.trackers.byte_tracker.STrack
+
+
+## BYTETracker
+---
+### ::: ultralytics.tracker.trackers.byte_tracker.BYTETracker
+
diff --git a/yolov8/docs/reference/tracker/utils/gmc.md b/yolov8/docs/reference/tracker/utils/gmc.md
new file mode 100644
index 0000000000000000000000000000000000000000..6441f071d15c824c69c766391a4b9c1bae29bbfd
--- /dev/null
+++ b/yolov8/docs/reference/tracker/utils/gmc.md
@@ -0,0 +1,9 @@
+---
+description: '"Track Google Marketing Campaigns in GMC with Ultralytics Tracker. Learn to set up and use GMC for detailed analytics. Get started now."'
+keywords: Ultralytics, YOLO, object detection, tracker, optimization, models, documentation
+---
+
+## GMC
+---
+### ::: ultralytics.tracker.utils.gmc.GMC
+
diff --git a/yolov8/docs/reference/tracker/utils/kalman_filter.md b/yolov8/docs/reference/tracker/utils/kalman_filter.md
new file mode 100644
index 0000000000000000000000000000000000000000..fbe547305ae1f1c58133f1153cf7c26f85630ff6
--- /dev/null
+++ b/yolov8/docs/reference/tracker/utils/kalman_filter.md
@@ -0,0 +1,14 @@
+---
+description: Improve object tracking with KalmanFilterXYAH in Ultralytics YOLO - an efficient and accurate algorithm for state estimation.
+keywords: KalmanFilterXYAH, Ultralytics Docs, Kalman filter algorithm, object tracking, computer vision, YOLO
+---
+
+## KalmanFilterXYAH
+---
+### ::: ultralytics.tracker.utils.kalman_filter.KalmanFilterXYAH
+
+
+## KalmanFilterXYWH
+---
+### ::: ultralytics.tracker.utils.kalman_filter.KalmanFilterXYWH
+
diff --git a/yolov8/docs/reference/tracker/utils/matching.md b/yolov8/docs/reference/tracker/utils/matching.md
new file mode 100644
index 0000000000000000000000000000000000000000..385b39ce860bf8b34fb9a4699f011cea04085198
--- /dev/null
+++ b/yolov8/docs/reference/tracker/utils/matching.md
@@ -0,0 +1,64 @@
+---
+description: Learn how to match and fuse object detections for accurate target tracking using Ultralytics' YOLO merge_matches, iou_distance, and embedding_distance.
+keywords: Ultralytics, multi-object tracking, object tracking, detection, recognition, matching, indices, iou distance, gate cost matrix, fuse iou, bbox ious
+---
+
+## merge_matches
+---
+### ::: ultralytics.tracker.utils.matching.merge_matches
+
+
+## _indices_to_matches
+---
+### ::: ultralytics.tracker.utils.matching._indices_to_matches
+
+
+## linear_assignment
+---
+### ::: ultralytics.tracker.utils.matching.linear_assignment
+
+
+## ious
+---
+### ::: ultralytics.tracker.utils.matching.ious
+
+
+## iou_distance
+---
+### ::: ultralytics.tracker.utils.matching.iou_distance
+
+
+## v_iou_distance
+---
+### ::: ultralytics.tracker.utils.matching.v_iou_distance
+
+
+## embedding_distance
+---
+### ::: ultralytics.tracker.utils.matching.embedding_distance
+
+
+## gate_cost_matrix
+---
+### ::: ultralytics.tracker.utils.matching.gate_cost_matrix
+
+
+## fuse_motion
+---
+### ::: ultralytics.tracker.utils.matching.fuse_motion
+
+
+## fuse_iou
+---
+### ::: ultralytics.tracker.utils.matching.fuse_iou
+
+
+## fuse_score
+---
+### ::: ultralytics.tracker.utils.matching.fuse_score
+
+
+## bbox_ious
+---
+### ::: ultralytics.tracker.utils.matching.bbox_ious
+
diff --git a/yolov8/docs/reference/vit/rtdetr/model.md b/yolov8/docs/reference/vit/rtdetr/model.md
new file mode 100644
index 0000000000000000000000000000000000000000..c979186eaec1a6ae2acfd7991c74994f382d381a
--- /dev/null
+++ b/yolov8/docs/reference/vit/rtdetr/model.md
@@ -0,0 +1,9 @@
+---
+description: Learn about the RTDETR model in Ultralytics YOLO Docs and how it can be used for object detection with improved speed and accuracy. Find implementation details and more.
+keywords: RTDETR, Ultralytics, YOLO, object detection, speed, accuracy, implementation details
+---
+
+## RTDETR
+---
+### ::: ultralytics.vit.rtdetr.model.RTDETR
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/rtdetr/predict.md b/yolov8/docs/reference/vit/rtdetr/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..c5b5420a4068d480e45b1583dbbf91c8dce80971
--- /dev/null
+++ b/yolov8/docs/reference/vit/rtdetr/predict.md
@@ -0,0 +1,9 @@
+---
+description: Learn about the RTDETRPredictor class and how to use it for vision transformer object detection with Ultralytics YOLO.
+keywords: RTDETRPredictor, object detection, vision transformer, Ultralytics YOLO
+---
+
+## RTDETRPredictor
+---
+### ::: ultralytics.vit.rtdetr.predict.RTDETRPredictor
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/rtdetr/train.md b/yolov8/docs/reference/vit/rtdetr/train.md
new file mode 100644
index 0000000000000000000000000000000000000000..b7bb384eb5506d685d875ad09ff8f28484ffc7dc
--- /dev/null
+++ b/yolov8/docs/reference/vit/rtdetr/train.md
@@ -0,0 +1,14 @@
+---
+description: Learn how to use RTDETRTrainer from Ultralytics YOLO Docs. Train object detection models with the latest VIT-based RTDETR system.
+keywords: RTDETRTrainer, Ultralytics YOLO Docs, object detection, VIT-based RTDETR system, train
+---
+
+## RTDETRTrainer
+---
+### ::: ultralytics.vit.rtdetr.train.RTDETRTrainer
+
+
+## train
+---
+### ::: ultralytics.vit.rtdetr.train.train
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/rtdetr/val.md b/yolov8/docs/reference/vit/rtdetr/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..43c1898b35326853693bafd54bb7760ae8a47979
--- /dev/null
+++ b/yolov8/docs/reference/vit/rtdetr/val.md
@@ -0,0 +1,14 @@
+---
+description: Documentation for RTDETRValidator data validation tool in Ultralytics RTDETRDataset.
+keywords: RTDETRDataset, RTDETRValidator, data validation, documentation
+---
+
+## RTDETRDataset
+---
+### ::: ultralytics.vit.rtdetr.val.RTDETRDataset
+
+
+## RTDETRValidator
+---
+### ::: ultralytics.vit.rtdetr.val.RTDETRValidator
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/amg.md b/yolov8/docs/reference/vit/sam/amg.md
new file mode 100644
index 0000000000000000000000000000000000000000..82c66e8b19e721a69e1644845391026368233732
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/amg.md
@@ -0,0 +1,89 @@
+---
+description: Explore and learn about functions in Ultralytics MaskData library such as mask_to_rle_pytorch, area_from_rle, generate_crop_boxes, and more.
+keywords: Ultralytics, SAM, MaskData, mask_to_rle_pytorch, area_from_rle, generate_crop_boxes, batched_mask_to_box, documentation
+---
+
+## MaskData
+---
+### ::: ultralytics.vit.sam.amg.MaskData
+
+
+## is_box_near_crop_edge
+---
+### ::: ultralytics.vit.sam.amg.is_box_near_crop_edge
+
+
+## box_xyxy_to_xywh
+---
+### ::: ultralytics.vit.sam.amg.box_xyxy_to_xywh
+
+
+## batch_iterator
+---
+### ::: ultralytics.vit.sam.amg.batch_iterator
+
+
+## mask_to_rle_pytorch
+---
+### ::: ultralytics.vit.sam.amg.mask_to_rle_pytorch
+
+
+## rle_to_mask
+---
+### ::: ultralytics.vit.sam.amg.rle_to_mask
+
+
+## area_from_rle
+---
+### ::: ultralytics.vit.sam.amg.area_from_rle
+
+
+## calculate_stability_score
+---
+### ::: ultralytics.vit.sam.amg.calculate_stability_score
+
+
+## build_point_grid
+---
+### ::: ultralytics.vit.sam.amg.build_point_grid
+
+
+## build_all_layer_point_grids
+---
+### ::: ultralytics.vit.sam.amg.build_all_layer_point_grids
+
+
+## generate_crop_boxes
+---
+### ::: ultralytics.vit.sam.amg.generate_crop_boxes
+
+
+## uncrop_boxes_xyxy
+---
+### ::: ultralytics.vit.sam.amg.uncrop_boxes_xyxy
+
+
+## uncrop_points
+---
+### ::: ultralytics.vit.sam.amg.uncrop_points
+
+
+## uncrop_masks
+---
+### ::: ultralytics.vit.sam.amg.uncrop_masks
+
+
+## remove_small_regions
+---
+### ::: ultralytics.vit.sam.amg.remove_small_regions
+
+
+## coco_encode_rle
+---
+### ::: ultralytics.vit.sam.amg.coco_encode_rle
+
+
+## batched_mask_to_box
+---
+### ::: ultralytics.vit.sam.amg.batched_mask_to_box
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/autosize.md b/yolov8/docs/reference/vit/sam/autosize.md
new file mode 100644
index 0000000000000000000000000000000000000000..cbb0ca7c055fa680ffc9080a0c4d4db650134327
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/autosize.md
@@ -0,0 +1,9 @@
+---
+description: Learn how to use the ResizeLongestSide module in Ultralytics YOLO for automatic image resizing. Resize your images with ease.
+keywords: ResizeLongestSide, Ultralytics YOLO, automatic image resizing, image resizing
+---
+
+## ResizeLongestSide
+---
+### ::: ultralytics.vit.sam.autosize.ResizeLongestSide
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/build.md b/yolov8/docs/reference/vit/sam/build.md
new file mode 100644
index 0000000000000000000000000000000000000000..faa26eeadd019c7acf46c8ae8d2a158bf503fddf
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/build.md
@@ -0,0 +1,29 @@
+---
+description: Learn how to build SAM and VIT models with Ultralytics YOLO Docs. Enhance your understanding of computer vision models today!.
+keywords: SAM, VIT, computer vision models, build SAM models, build VIT models, Ultralytics YOLO Docs
+---
+
+## build_sam_vit_h
+---
+### ::: ultralytics.vit.sam.build.build_sam_vit_h
+
+
+## build_sam_vit_l
+---
+### ::: ultralytics.vit.sam.build.build_sam_vit_l
+
+
+## build_sam_vit_b
+---
+### ::: ultralytics.vit.sam.build.build_sam_vit_b
+
+
+## _build_sam
+---
+### ::: ultralytics.vit.sam.build._build_sam
+
+
+## build_sam
+---
+### ::: ultralytics.vit.sam.build.build_sam
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/model.md b/yolov8/docs/reference/vit/sam/model.md
new file mode 100644
index 0000000000000000000000000000000000000000..7d924d4a93d8c50f2536174f46ef236091915bda
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/model.md
@@ -0,0 +1,9 @@
+---
+description: Learn about the Ultralytics VIT SAM model for object detection and how it can help streamline your computer vision workflow. Check out the documentation for implementation details and examples.
+keywords: Ultralytics, VIT, SAM, object detection, computer vision, deep learning, implementation, examples
+---
+
+## SAM
+---
+### ::: ultralytics.vit.sam.model.SAM
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/modules/decoders.md b/yolov8/docs/reference/vit/sam/modules/decoders.md
new file mode 100644
index 0000000000000000000000000000000000000000..e89ca9d68b46e25d57eb2f3294e89990b6d0ae38
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/modules/decoders.md
@@ -0,0 +1,9 @@
+## MaskDecoder
+---
+### ::: ultralytics.vit.sam.modules.decoders.MaskDecoder
+
+
+## MLP
+---
+### ::: ultralytics.vit.sam.modules.decoders.MLP
+
diff --git a/yolov8/docs/reference/vit/sam/modules/encoders.md b/yolov8/docs/reference/vit/sam/modules/encoders.md
new file mode 100644
index 0000000000000000000000000000000000000000..8c338bc6de4a03419d435d29fcc23026b6338e78
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/modules/encoders.md
@@ -0,0 +1,54 @@
+---
+description: Learn about Ultralytics ViT encoder, position embeddings, attention, window partition, and more in our comprehensive documentation.
+keywords: Ultralytics YOLO, ViT Encoder, Position Embeddings, Attention, Window Partition, Rel Pos Encoding
+---
+
+## ImageEncoderViT
+---
+### ::: ultralytics.vit.sam.modules.encoders.ImageEncoderViT
+
+
+## PromptEncoder
+---
+### ::: ultralytics.vit.sam.modules.encoders.PromptEncoder
+
+
+## PositionEmbeddingRandom
+---
+### ::: ultralytics.vit.sam.modules.encoders.PositionEmbeddingRandom
+
+
+## Block
+---
+### ::: ultralytics.vit.sam.modules.encoders.Block
+
+
+## Attention
+---
+### ::: ultralytics.vit.sam.modules.encoders.Attention
+
+
+## PatchEmbed
+---
+### ::: ultralytics.vit.sam.modules.encoders.PatchEmbed
+
+
+## window_partition
+---
+### ::: ultralytics.vit.sam.modules.encoders.window_partition
+
+
+## window_unpartition
+---
+### ::: ultralytics.vit.sam.modules.encoders.window_unpartition
+
+
+## get_rel_pos
+---
+### ::: ultralytics.vit.sam.modules.encoders.get_rel_pos
+
+
+## add_decomposed_rel_pos
+---
+### ::: ultralytics.vit.sam.modules.encoders.add_decomposed_rel_pos
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/modules/mask_generator.md b/yolov8/docs/reference/vit/sam/modules/mask_generator.md
new file mode 100644
index 0000000000000000000000000000000000000000..e2e1251c1169f162df822737f34d0c83cf8fe0cb
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/modules/mask_generator.md
@@ -0,0 +1,9 @@
+---
+description: Learn about the SamAutomaticMaskGenerator module in Ultralytics YOLO, an automatic mask generator for image segmentation.
+keywords: SamAutomaticMaskGenerator, Ultralytics YOLO, automatic mask generator, image segmentation
+---
+
+## SamAutomaticMaskGenerator
+---
+### ::: ultralytics.vit.sam.modules.mask_generator.SamAutomaticMaskGenerator
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/modules/prompt_predictor.md b/yolov8/docs/reference/vit/sam/modules/prompt_predictor.md
new file mode 100644
index 0000000000000000000000000000000000000000..f7e3b3713b0c189bd0d8819651b9d8880b1f5e4b
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/modules/prompt_predictor.md
@@ -0,0 +1,9 @@
+---
+description: Learn about PromptPredictor - a module in Ultralytics VIT SAM that predicts image captions based on prompts. Get started today!.
+keywords: PromptPredictor, Ultralytics, YOLO, VIT SAM, image captioning, deep learning, computer vision
+---
+
+## PromptPredictor
+---
+### ::: ultralytics.vit.sam.modules.prompt_predictor.PromptPredictor
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/modules/sam.md b/yolov8/docs/reference/vit/sam/modules/sam.md
new file mode 100644
index 0000000000000000000000000000000000000000..acd467b1946988f52f70b977caec9c60f43fddd5
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/modules/sam.md
@@ -0,0 +1,9 @@
+---
+description: Explore the Sam module in Ultralytics VIT, a PyTorch-based vision library, and learn how to improve your image classification and segmentation tasks.
+keywords: Ultralytics VIT, Sam module, PyTorch vision library, image classification, segmentation tasks
+---
+
+## Sam
+---
+### ::: ultralytics.vit.sam.modules.sam.Sam
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/modules/transformer.md b/yolov8/docs/reference/vit/sam/modules/transformer.md
new file mode 100644
index 0000000000000000000000000000000000000000..994b984555391c0131caff65714956b82d5a673e
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/modules/transformer.md
@@ -0,0 +1,19 @@
+---
+description: Explore the Attention and TwoWayTransformer modules in Ultralytics YOLO documentation. Learn how to integrate them in your project efficiently.
+keywords: Ultralytics YOLO, Attention module, TwoWayTransformer module, Object Detection, Deep Learning
+---
+
+## TwoWayTransformer
+---
+### ::: ultralytics.vit.sam.modules.transformer.TwoWayTransformer
+
+
+## TwoWayAttentionBlock
+---
+### ::: ultralytics.vit.sam.modules.transformer.TwoWayAttentionBlock
+
+
+## Attention
+---
+### ::: ultralytics.vit.sam.modules.transformer.Attention
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/sam/predict.md b/yolov8/docs/reference/vit/sam/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..836d91e3c66df0b2f27e54acad0f1cf80cd287ee
--- /dev/null
+++ b/yolov8/docs/reference/vit/sam/predict.md
@@ -0,0 +1,9 @@
+---
+description: The VIT SAM Predictor from Ultralytics provides object detection capabilities for YOLO. Learn how to use it and speed up your object detection models.
+keywords: Ultralytics, VIT SAM Predictor, object detection, YOLO
+---
+
+## Predictor
+---
+### ::: ultralytics.vit.sam.predict.Predictor
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/utils/loss.md b/yolov8/docs/reference/vit/utils/loss.md
new file mode 100644
index 0000000000000000000000000000000000000000..3eb366ed31603c2295577b4ab486d9b621649676
--- /dev/null
+++ b/yolov8/docs/reference/vit/utils/loss.md
@@ -0,0 +1,14 @@
+---
+description: DETRLoss is a method for optimizing detection of objects in images. Learn how to use it in RTDETRDetectionLoss at Ultralytics Docs.
+keywords: DETRLoss, RTDETRDetectionLoss, Ultralytics, object detection, image classification, computer vision
+---
+
+## DETRLoss
+---
+### ::: ultralytics.vit.utils.loss.DETRLoss
+
+
+## RTDETRDetectionLoss
+---
+### ::: ultralytics.vit.utils.loss.RTDETRDetectionLoss
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/vit/utils/ops.md b/yolov8/docs/reference/vit/utils/ops.md
new file mode 100644
index 0000000000000000000000000000000000000000..f4b7c81764cc41519372cfad3d4e60b613983c3d
--- /dev/null
+++ b/yolov8/docs/reference/vit/utils/ops.md
@@ -0,0 +1,19 @@
+---
+description: Learn about HungarianMatcher and inverse_sigmoid functions in the Ultralytics YOLO Docs. Improve your object detection skills today!.
+keywords: Ultralytics, YOLO, object detection, HungarianMatcher, inverse_sigmoid
+---
+
+## HungarianMatcher
+---
+### ::: ultralytics.vit.utils.ops.HungarianMatcher
+
+
+## get_cdn_group
+---
+### ::: ultralytics.vit.utils.ops.get_cdn_group
+
+
+## inverse_sigmoid
+---
+### ::: ultralytics.vit.utils.ops.inverse_sigmoid
+
\ No newline at end of file
diff --git a/yolov8/docs/reference/yolo/cfg/__init__.md b/yolov8/docs/reference/yolo/cfg/__init__.md
new file mode 100644
index 0000000000000000000000000000000000000000..26f4e54adde43223ae4aab2b96f42774e318b10c
--- /dev/null
+++ b/yolov8/docs/reference/yolo/cfg/__init__.md
@@ -0,0 +1,49 @@
+---
+description: Explore Ultralytics YOLO's configuration functions and tools. Handle settings, manage defaults, and deal with deprecations in your YOLO configuration.
+keywords: Ultralytics, YOLO, configuration, cfg2dict, get_cfg, handle_deprecation, check_cfg_mismatch, merge_equals_args, handle_yolo_hub, handle_yolo_settings, entrypoint, copy_default_cfg
+---
+
+## cfg2dict
+---
+### ::: ultralytics.yolo.cfg.cfg2dict
+
+
+## get_cfg
+---
+### ::: ultralytics.yolo.cfg.get_cfg
+
+
+## _handle_deprecation
+---
+### ::: ultralytics.yolo.cfg._handle_deprecation
+
+
+## check_cfg_mismatch
+---
+### ::: ultralytics.yolo.cfg.check_cfg_mismatch
+
+
+## merge_equals_args
+---
+### ::: ultralytics.yolo.cfg.merge_equals_args
+
+
+## handle_yolo_hub
+---
+### ::: ultralytics.yolo.cfg.handle_yolo_hub
+
+
+## handle_yolo_settings
+---
+### ::: ultralytics.yolo.cfg.handle_yolo_settings
+
+
+## entrypoint
+---
+### ::: ultralytics.yolo.cfg.entrypoint
+
+
+## copy_default_cfg
+---
+### ::: ultralytics.yolo.cfg.copy_default_cfg
+
diff --git a/yolov8/docs/reference/yolo/data/annotator.md b/yolov8/docs/reference/yolo/data/annotator.md
new file mode 100644
index 0000000000000000000000000000000000000000..0999d58666d79c762d34a7cf832346bd00e88c1a
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/annotator.md
@@ -0,0 +1,9 @@
+---
+description: Learn how to use auto_annotate in Ultralytics YOLO to generate annotations automatically for your dataset. Simplify object detection workflows.
+keywords: Ultralytics YOLO, Auto Annotator, AI, image annotation, object detection, labelling, tool
+---
+
+## auto_annotate
+---
+### ::: ultralytics.yolo.data.annotator.auto_annotate
+
diff --git a/yolov8/docs/reference/yolo/data/augment.md b/yolov8/docs/reference/yolo/data/augment.md
new file mode 100644
index 0000000000000000000000000000000000000000..634f183c0d3559e03d146db4c6f362e55766780f
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/augment.md
@@ -0,0 +1,99 @@
+---
+description: Use Ultralytics YOLO Data Augmentation transforms with Base, MixUp, and Albumentations for object detection and classification.
+keywords: YOLO, data augmentation, transforms, BaseTransform, MixUp, RandomHSV, Albumentations, ToTensor, classify_transforms, classify_albumentations
+---
+
+## BaseTransform
+---
+### ::: ultralytics.yolo.data.augment.BaseTransform
+
+
+## Compose
+---
+### ::: ultralytics.yolo.data.augment.Compose
+
+
+## BaseMixTransform
+---
+### ::: ultralytics.yolo.data.augment.BaseMixTransform
+
+
+## Mosaic
+---
+### ::: ultralytics.yolo.data.augment.Mosaic
+
+
+## MixUp
+---
+### ::: ultralytics.yolo.data.augment.MixUp
+
+
+## RandomPerspective
+---
+### ::: ultralytics.yolo.data.augment.RandomPerspective
+
+
+## RandomHSV
+---
+### ::: ultralytics.yolo.data.augment.RandomHSV
+
+
+## RandomFlip
+---
+### ::: ultralytics.yolo.data.augment.RandomFlip
+
+
+## LetterBox
+---
+### ::: ultralytics.yolo.data.augment.LetterBox
+
+
+## CopyPaste
+---
+### ::: ultralytics.yolo.data.augment.CopyPaste
+
+
+## Albumentations
+---
+### ::: ultralytics.yolo.data.augment.Albumentations
+
+
+## Format
+---
+### ::: ultralytics.yolo.data.augment.Format
+
+
+## ClassifyLetterBox
+---
+### ::: ultralytics.yolo.data.augment.ClassifyLetterBox
+
+
+## CenterCrop
+---
+### ::: ultralytics.yolo.data.augment.CenterCrop
+
+
+## ToTensor
+---
+### ::: ultralytics.yolo.data.augment.ToTensor
+
+
+## v8_transforms
+---
+### ::: ultralytics.yolo.data.augment.v8_transforms
+
+
+## classify_transforms
+---
+### ::: ultralytics.yolo.data.augment.classify_transforms
+
+
+## hsv2colorjitter
+---
+### ::: ultralytics.yolo.data.augment.hsv2colorjitter
+
+
+## classify_albumentations
+---
+### ::: ultralytics.yolo.data.augment.classify_albumentations
+
diff --git a/yolov8/docs/reference/yolo/data/base.md b/yolov8/docs/reference/yolo/data/base.md
new file mode 100644
index 0000000000000000000000000000000000000000..3742e672edd4c6ef6986f522e5a4bb0de616132d
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/base.md
@@ -0,0 +1,9 @@
+---
+description: Learn about BaseDataset in Ultralytics YOLO, a flexible dataset class for object detection. Maximize your YOLO performance with custom datasets.
+keywords: BaseDataset, Ultralytics YOLO, object detection, real-world applications, documentation
+---
+
+## BaseDataset
+---
+### ::: ultralytics.yolo.data.base.BaseDataset
+
diff --git a/yolov8/docs/reference/yolo/data/build.md b/yolov8/docs/reference/yolo/data/build.md
new file mode 100644
index 0000000000000000000000000000000000000000..cfee450b58e110deffc172feacf76470fadcff27
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/build.md
@@ -0,0 +1,39 @@
+---
+description: Maximize YOLO performance with Ultralytics' InfiniteDataLoader, seed_worker, build_dataloader, and load_inference_source functions.
+keywords: Ultralytics, YOLO, object detection, data loading, build dataloader, load inference source
+---
+
+## InfiniteDataLoader
+---
+### ::: ultralytics.yolo.data.build.InfiniteDataLoader
+
+
+## _RepeatSampler
+---
+### ::: ultralytics.yolo.data.build._RepeatSampler
+
+
+## seed_worker
+---
+### ::: ultralytics.yolo.data.build.seed_worker
+
+
+## build_yolo_dataset
+---
+### ::: ultralytics.yolo.data.build.build_yolo_dataset
+
+
+## build_dataloader
+---
+### ::: ultralytics.yolo.data.build.build_dataloader
+
+
+## check_source
+---
+### ::: ultralytics.yolo.data.build.check_source
+
+
+## load_inference_source
+---
+### ::: ultralytics.yolo.data.build.load_inference_source
+
diff --git a/yolov8/docs/reference/yolo/data/converter.md b/yolov8/docs/reference/yolo/data/converter.md
new file mode 100644
index 0000000000000000000000000000000000000000..6fd5d7e29207f392aa9af24827afdfc4a00bebaa
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/converter.md
@@ -0,0 +1,34 @@
+---
+description: Convert COCO-91 to COCO-80 class, RLE to polygon, and merge multi-segment images with Ultralytics YOLO data converter. Improve your object detection.
+keywords: Ultralytics, YOLO, converter, COCO91, COCO80, rle2polygon, merge_multi_segment, annotations
+---
+
+## coco91_to_coco80_class
+---
+### ::: ultralytics.yolo.data.converter.coco91_to_coco80_class
+
+
+## convert_coco
+---
+### ::: ultralytics.yolo.data.converter.convert_coco
+
+
+## rle2polygon
+---
+### ::: ultralytics.yolo.data.converter.rle2polygon
+
+
+## min_index
+---
+### ::: ultralytics.yolo.data.converter.min_index
+
+
+## merge_multi_segment
+---
+### ::: ultralytics.yolo.data.converter.merge_multi_segment
+
+
+## delete_dsstore
+---
+### ::: ultralytics.yolo.data.converter.delete_dsstore
+
diff --git a/yolov8/docs/reference/yolo/data/dataloaders/stream_loaders.md b/yolov8/docs/reference/yolo/data/dataloaders/stream_loaders.md
new file mode 100644
index 0000000000000000000000000000000000000000..40afd3d64957e14972197131e2068c9d7eb5cfb4
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/dataloaders/stream_loaders.md
@@ -0,0 +1,44 @@
+---
+description: 'Ultralytics YOLO Docs: Learn about stream loaders for image and tensor data, as well as autocasting techniques. Check out SourceTypes and more.'
+keywords: Ultralytics YOLO, data loaders, stream load images, screenshots, tensor data, autocast list, youtube URL retriever
+---
+
+## SourceTypes
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.SourceTypes
+
+
+## LoadStreams
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.LoadStreams
+
+
+## LoadScreenshots
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.LoadScreenshots
+
+
+## LoadImages
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.LoadImages
+
+
+## LoadPilAndNumpy
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.LoadPilAndNumpy
+
+
+## LoadTensor
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.LoadTensor
+
+
+## autocast_list
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.autocast_list
+
+
+## get_best_youtube_url
+---
+### ::: ultralytics.yolo.data.dataloaders.stream_loaders.get_best_youtube_url
+
diff --git a/yolov8/docs/reference/yolo/data/dataloaders/v5augmentations.md b/yolov8/docs/reference/yolo/data/dataloaders/v5augmentations.md
new file mode 100644
index 0000000000000000000000000000000000000000..63df3692cb755d8cd835318a95d7a3cf4c95c139
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/dataloaders/v5augmentations.md
@@ -0,0 +1,89 @@
+---
+description: Enhance image data with Albumentations CenterCrop, normalize, augment_hsv, replicate, random_perspective, cutout, & box_candidates.
+keywords: YOLO, object detection, data loaders, V5 augmentations, CenterCrop, normalize, random_perspective
+---
+
+## Albumentations
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.Albumentations
+
+
+## LetterBox
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.LetterBox
+
+
+## CenterCrop
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.CenterCrop
+
+
+## ToTensor
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.ToTensor
+
+
+## normalize
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.normalize
+
+
+## denormalize
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.denormalize
+
+
+## augment_hsv
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.augment_hsv
+
+
+## hist_equalize
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.hist_equalize
+
+
+## replicate
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.replicate
+
+
+## letterbox
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.letterbox
+
+
+## random_perspective
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.random_perspective
+
+
+## copy_paste
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.copy_paste
+
+
+## cutout
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.cutout
+
+
+## mixup
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.mixup
+
+
+## box_candidates
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.box_candidates
+
+
+## classify_albumentations
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.classify_albumentations
+
+
+## classify_transforms
+---
+### ::: ultralytics.yolo.data.dataloaders.v5augmentations.classify_transforms
+
diff --git a/yolov8/docs/reference/yolo/data/dataloaders/v5loader.md b/yolov8/docs/reference/yolo/data/dataloaders/v5loader.md
new file mode 100644
index 0000000000000000000000000000000000000000..55986951904b870c34e3d0343109e0ac56c1d880
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/dataloaders/v5loader.md
@@ -0,0 +1,94 @@
+---
+description: Efficiently load images and labels to models using Ultralytics YOLO's InfiniteDataLoader, LoadScreenshots, and LoadStreams.
+keywords: YOLO, data loader, image classification, object detection, Ultralytics
+---
+
+## InfiniteDataLoader
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.InfiniteDataLoader
+
+
+## _RepeatSampler
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader._RepeatSampler
+
+
+## LoadScreenshots
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.LoadScreenshots
+
+
+## LoadImages
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.LoadImages
+
+
+## LoadStreams
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.LoadStreams
+
+
+## LoadImagesAndLabels
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.LoadImagesAndLabels
+
+
+## ClassificationDataset
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.ClassificationDataset
+
+
+## get_hash
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.get_hash
+
+
+## exif_size
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.exif_size
+
+
+## exif_transpose
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.exif_transpose
+
+
+## seed_worker
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.seed_worker
+
+
+## create_dataloader
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.create_dataloader
+
+
+## img2label_paths
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.img2label_paths
+
+
+## flatten_recursive
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.flatten_recursive
+
+
+## extract_boxes
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.extract_boxes
+
+
+## autosplit
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.autosplit
+
+
+## verify_image_label
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.verify_image_label
+
+
+## create_classification_dataloader
+---
+### ::: ultralytics.yolo.data.dataloaders.v5loader.create_classification_dataloader
+
diff --git a/yolov8/docs/reference/yolo/data/dataset.md b/yolov8/docs/reference/yolo/data/dataset.md
new file mode 100644
index 0000000000000000000000000000000000000000..f42a0a11ff869d2ddef7d2be1500f328f1251a3e
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/dataset.md
@@ -0,0 +1,19 @@
+---
+description: Create custom YOLOv5 datasets with Ultralytics YOLODataset and SemanticDataset. Streamline your object detection and segmentation projects.
+keywords: YOLODataset, SemanticDataset, Ultralytics YOLO Docs, Object Detection, Segmentation
+---
+
+## YOLODataset
+---
+### ::: ultralytics.yolo.data.dataset.YOLODataset
+
+
+## ClassificationDataset
+---
+### ::: ultralytics.yolo.data.dataset.ClassificationDataset
+
+
+## SemanticDataset
+---
+### ::: ultralytics.yolo.data.dataset.SemanticDataset
+
diff --git a/yolov8/docs/reference/yolo/data/dataset_wrappers.md b/yolov8/docs/reference/yolo/data/dataset_wrappers.md
new file mode 100644
index 0000000000000000000000000000000000000000..c56806df30047e2971e818518af530bb3fe8ed1f
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/dataset_wrappers.md
@@ -0,0 +1,9 @@
+---
+description: Create a custom dataset of mixed and oriented rectangular objects with Ultralytics YOLO's MixAndRectDataset.
+keywords: Ultralytics YOLO, MixAndRectDataset, dataset wrapper, image-level annotations, object-level annotations, rectangular object detection
+---
+
+## MixAndRectDataset
+---
+### ::: ultralytics.yolo.data.dataset_wrappers.MixAndRectDataset
+
diff --git a/yolov8/docs/reference/yolo/data/utils.md b/yolov8/docs/reference/yolo/data/utils.md
new file mode 100644
index 0000000000000000000000000000000000000000..f0f2e2fad4e478da499cf0920e7727dd8f0751c6
--- /dev/null
+++ b/yolov8/docs/reference/yolo/data/utils.md
@@ -0,0 +1,69 @@
+---
+description: Efficiently handle data in YOLO with Ultralytics. Utilize HUBDatasetStats and customize dataset with these data utility functions.
+keywords: YOLOv4, Object Detection, Computer Vision, Deep Learning, Convolutional Neural Network, CNN, Ultralytics Docs
+---
+
+## HUBDatasetStats
+---
+### ::: ultralytics.yolo.data.utils.HUBDatasetStats
+
+
+## img2label_paths
+---
+### ::: ultralytics.yolo.data.utils.img2label_paths
+
+
+## get_hash
+---
+### ::: ultralytics.yolo.data.utils.get_hash
+
+
+## exif_size
+---
+### ::: ultralytics.yolo.data.utils.exif_size
+
+
+## verify_image_label
+---
+### ::: ultralytics.yolo.data.utils.verify_image_label
+
+
+## polygon2mask
+---
+### ::: ultralytics.yolo.data.utils.polygon2mask
+
+
+## polygons2masks
+---
+### ::: ultralytics.yolo.data.utils.polygons2masks
+
+
+## polygons2masks_overlap
+---
+### ::: ultralytics.yolo.data.utils.polygons2masks_overlap
+
+
+## check_det_dataset
+---
+### ::: ultralytics.yolo.data.utils.check_det_dataset
+
+
+## check_cls_dataset
+---
+### ::: ultralytics.yolo.data.utils.check_cls_dataset
+
+
+## compress_one_image
+---
+### ::: ultralytics.yolo.data.utils.compress_one_image
+
+
+## delete_dsstore
+---
+### ::: ultralytics.yolo.data.utils.delete_dsstore
+
+
+## zip_directory
+---
+### ::: ultralytics.yolo.data.utils.zip_directory
+
diff --git a/yolov8/docs/reference/yolo/engine/exporter.md b/yolov8/docs/reference/yolo/engine/exporter.md
new file mode 100644
index 0000000000000000000000000000000000000000..37189d46fadbd0cc6fc85f3db042fdf2531c13de
--- /dev/null
+++ b/yolov8/docs/reference/yolo/engine/exporter.md
@@ -0,0 +1,34 @@
+---
+description: Learn how to export your YOLO model in various formats using Ultralytics' exporter package - iOS, GDC, and more.
+keywords: Ultralytics, YOLO, exporter, iOS detect model, gd_outputs, export
+---
+
+## Exporter
+---
+### ::: ultralytics.yolo.engine.exporter.Exporter
+
+
+## iOSDetectModel
+---
+### ::: ultralytics.yolo.engine.exporter.iOSDetectModel
+
+
+## export_formats
+---
+### ::: ultralytics.yolo.engine.exporter.export_formats
+
+
+## gd_outputs
+---
+### ::: ultralytics.yolo.engine.exporter.gd_outputs
+
+
+## try_export
+---
+### ::: ultralytics.yolo.engine.exporter.try_export
+
+
+## export
+---
+### ::: ultralytics.yolo.engine.exporter.export
+
diff --git a/yolov8/docs/reference/yolo/engine/model.md b/yolov8/docs/reference/yolo/engine/model.md
new file mode 100644
index 0000000000000000000000000000000000000000..9ba79328085fa2c8c97e06ded51fc7eb82d70283
--- /dev/null
+++ b/yolov8/docs/reference/yolo/engine/model.md
@@ -0,0 +1,9 @@
+---
+description: Discover the YOLO model of Ultralytics engine to simplify your object detection tasks with state-of-the-art models.
+keywords: YOLO, object detection, model, architecture, usage, customization, Ultralytics Docs
+---
+
+## YOLO
+---
+### ::: ultralytics.yolo.engine.model.YOLO
+
diff --git a/yolov8/docs/reference/yolo/engine/predictor.md b/yolov8/docs/reference/yolo/engine/predictor.md
new file mode 100644
index 0000000000000000000000000000000000000000..f4aed3c06653fa3188a5222357fe36c76fbde1f2
--- /dev/null
+++ b/yolov8/docs/reference/yolo/engine/predictor.md
@@ -0,0 +1,9 @@
+---
+description: '"The BasePredictor class in Ultralytics YOLO Engine predicts object detection in images and videos. Learn to implement YOLO with ease."'
+keywords: Ultralytics, YOLO, BasePredictor, Object Detection, Computer Vision, Fast Model, Insights
+---
+
+## BasePredictor
+---
+### ::: ultralytics.yolo.engine.predictor.BasePredictor
+
diff --git a/yolov8/docs/reference/yolo/engine/results.md b/yolov8/docs/reference/yolo/engine/results.md
new file mode 100644
index 0000000000000000000000000000000000000000..38e568fa61024b35a511c37474869eaf0c336967
--- /dev/null
+++ b/yolov8/docs/reference/yolo/engine/results.md
@@ -0,0 +1,34 @@
+---
+description: Learn about BaseTensor & Boxes in Ultralytics YOLO Engine. Check out Ultralytics Docs for quality tutorials and resources on object detection.
+keywords: YOLO, Engine, Results, Masks, Probs, Ultralytics
+---
+
+## BaseTensor
+---
+### ::: ultralytics.yolo.engine.results.BaseTensor
+
+
+## Results
+---
+### ::: ultralytics.yolo.engine.results.Results
+
+
+## Boxes
+---
+### ::: ultralytics.yolo.engine.results.Boxes
+
+
+## Masks
+---
+### ::: ultralytics.yolo.engine.results.Masks
+
+
+## Keypoints
+---
+### ::: ultralytics.yolo.engine.results.Keypoints
+
+
+## Probs
+---
+### ::: ultralytics.yolo.engine.results.Probs
+
diff --git a/yolov8/docs/reference/yolo/engine/trainer.md b/yolov8/docs/reference/yolo/engine/trainer.md
new file mode 100644
index 0000000000000000000000000000000000000000..e9a06e45b817616d9d1298606bef3143f7682b4f
--- /dev/null
+++ b/yolov8/docs/reference/yolo/engine/trainer.md
@@ -0,0 +1,9 @@
+---
+description: Train faster with mixed precision. Learn how to use BaseTrainer with Advanced Mixed Precision to optimize YOLOv3 and YOLOv4 models.
+keywords: Ultralytics YOLO, BaseTrainer, object detection models, training guide
+---
+
+## BaseTrainer
+---
+### ::: ultralytics.yolo.engine.trainer.BaseTrainer
+
diff --git a/yolov8/docs/reference/yolo/engine/validator.md b/yolov8/docs/reference/yolo/engine/validator.md
new file mode 100644
index 0000000000000000000000000000000000000000..c9355ea18a08370eb1870f9ff69fa92b975e4ade
--- /dev/null
+++ b/yolov8/docs/reference/yolo/engine/validator.md
@@ -0,0 +1,9 @@
+---
+description: Ensure YOLOv5 models meet constraints and standards with the BaseValidator class. Learn how to use it here.
+keywords: Ultralytics, YOLO, BaseValidator, models, validation, object detection
+---
+
+## BaseValidator
+---
+### ::: ultralytics.yolo.engine.validator.BaseValidator
+
diff --git a/yolov8/docs/reference/yolo/nas/model.md b/yolov8/docs/reference/yolo/nas/model.md
new file mode 100644
index 0000000000000000000000000000000000000000..9c34659690a0ce0c439f48ac3bb48e97db1c1c04
--- /dev/null
+++ b/yolov8/docs/reference/yolo/nas/model.md
@@ -0,0 +1,9 @@
+---
+description: Learn about the Neural Architecture Search (NAS) feature available in Ultralytics YOLO. Find out how NAS can improve object detection models and increase accuracy. Get started today!.
+keywords: Ultralytics YOLO, object detection, NAS, Neural Architecture Search, model optimization, accuracy improvement
+---
+
+## NAS
+---
+### ::: ultralytics.yolo.nas.model.NAS
+
diff --git a/yolov8/docs/reference/yolo/nas/predict.md b/yolov8/docs/reference/yolo/nas/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..fb2be3ffb4ca1e342f83624a2d08e9eeb465fddc
--- /dev/null
+++ b/yolov8/docs/reference/yolo/nas/predict.md
@@ -0,0 +1,9 @@
+---
+description: Learn how to use NASPredictor in Ultralytics YOLO for deploying efficient CNN models with search algorithms in neural architecture search.
+keywords: Ultralytics YOLO, NASPredictor, neural architecture search, efficient CNN models, search algorithms
+---
+
+## NASPredictor
+---
+### ::: ultralytics.yolo.nas.predict.NASPredictor
+
diff --git a/yolov8/docs/reference/yolo/nas/val.md b/yolov8/docs/reference/yolo/nas/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..804ced3cf0a36b460198db341783b6c2d4f128a5
--- /dev/null
+++ b/yolov8/docs/reference/yolo/nas/val.md
@@ -0,0 +1,9 @@
+---
+description: Learn about NASValidator in the Ultralytics YOLO Docs. Properly validate YOLO neural architecture search results for optimal performance.
+keywords: NASValidator, YOLO, neural architecture search, validation, performance, Ultralytics
+---
+
+## NASValidator
+---
+### ::: ultralytics.yolo.nas.val.NASValidator
+
diff --git a/yolov8/docs/reference/yolo/utils/__init__.md b/yolov8/docs/reference/yolo/utils/__init__.md
new file mode 100644
index 0000000000000000000000000000000000000000..77b658429d856eec625c0b43cae77d8f95cb9b7d
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/__init__.md
@@ -0,0 +1,169 @@
+---
+description: Uncover utility functions in Ultralytics YOLO. Handle YAML, threading, logging, error-checking, and platform identification. Enhance your YOLO development process.
+keywords: Ultralytics, YOLO, utils, SimpleClass, IterableSimpleNamespace, EmojiFilter, TryExcept, plt_settings, set_logging, emojis, yaml_save, yaml_load, yaml_print, is_colab, is_kaggle, is_jupyter, is_docker, is_online, is_pip_package, is_dir_writeable, is_pytest_running, is_github_actions_ci, is_git_dir, get_git_dir, get_git_origin_url, get_git_branch, get_default_args, get_user_config_dir, colorstr, threaded, set_sentry, get_settings, set_settings, deprecation_warn, clean_url, url2file
+---
+
+## SimpleClass
+---
+### ::: ultralytics.yolo.utils.SimpleClass
+
+
+## IterableSimpleNamespace
+---
+### ::: ultralytics.yolo.utils.IterableSimpleNamespace
+
+
+## EmojiFilter
+---
+### ::: ultralytics.yolo.utils.EmojiFilter
+
+
+## TryExcept
+---
+### ::: ultralytics.yolo.utils.TryExcept
+
+
+## plt_settings
+---
+### ::: ultralytics.yolo.utils.plt_settings
+
+
+## set_logging
+---
+### ::: ultralytics.yolo.utils.set_logging
+
+
+## emojis
+---
+### ::: ultralytics.yolo.utils.emojis
+
+
+## yaml_save
+---
+### ::: ultralytics.yolo.utils.yaml_save
+
+
+## yaml_load
+---
+### ::: ultralytics.yolo.utils.yaml_load
+
+
+## yaml_print
+---
+### ::: ultralytics.yolo.utils.yaml_print
+
+
+## is_colab
+---
+### ::: ultralytics.yolo.utils.is_colab
+
+
+## is_kaggle
+---
+### ::: ultralytics.yolo.utils.is_kaggle
+
+
+## is_jupyter
+---
+### ::: ultralytics.yolo.utils.is_jupyter
+
+
+## is_docker
+---
+### ::: ultralytics.yolo.utils.is_docker
+
+
+## is_online
+---
+### ::: ultralytics.yolo.utils.is_online
+
+
+## is_pip_package
+---
+### ::: ultralytics.yolo.utils.is_pip_package
+
+
+## is_dir_writeable
+---
+### ::: ultralytics.yolo.utils.is_dir_writeable
+
+
+## is_pytest_running
+---
+### ::: ultralytics.yolo.utils.is_pytest_running
+
+
+## is_github_actions_ci
+---
+### ::: ultralytics.yolo.utils.is_github_actions_ci
+
+
+## is_git_dir
+---
+### ::: ultralytics.yolo.utils.is_git_dir
+
+
+## get_git_dir
+---
+### ::: ultralytics.yolo.utils.get_git_dir
+
+
+## get_git_origin_url
+---
+### ::: ultralytics.yolo.utils.get_git_origin_url
+
+
+## get_git_branch
+---
+### ::: ultralytics.yolo.utils.get_git_branch
+
+
+## get_default_args
+---
+### ::: ultralytics.yolo.utils.get_default_args
+
+
+## get_user_config_dir
+---
+### ::: ultralytics.yolo.utils.get_user_config_dir
+
+
+## colorstr
+---
+### ::: ultralytics.yolo.utils.colorstr
+
+
+## threaded
+---
+### ::: ultralytics.yolo.utils.threaded
+
+
+## set_sentry
+---
+### ::: ultralytics.yolo.utils.set_sentry
+
+
+## get_settings
+---
+### ::: ultralytics.yolo.utils.get_settings
+
+
+## set_settings
+---
+### ::: ultralytics.yolo.utils.set_settings
+
+
+## deprecation_warn
+---
+### ::: ultralytics.yolo.utils.deprecation_warn
+
+
+## clean_url
+---
+### ::: ultralytics.yolo.utils.clean_url
+
+
+## url2file
+---
+### ::: ultralytics.yolo.utils.url2file
+
diff --git a/yolov8/docs/reference/yolo/utils/autobatch.md b/yolov8/docs/reference/yolo/utils/autobatch.md
new file mode 100644
index 0000000000000000000000000000000000000000..fe9ba660fd423d10dcf8d36f4eae711ebe571402
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/autobatch.md
@@ -0,0 +1,14 @@
+---
+description: Dynamically adjusts input size to optimize GPU memory usage during training. Learn how to use check_train_batch_size with Ultralytics YOLO.
+keywords: YOLOv5, batch size, training, Ultralytics Autobatch, object detection, model performance
+---
+
+## check_train_batch_size
+---
+### ::: ultralytics.yolo.utils.autobatch.check_train_batch_size
+
+
+## autobatch
+---
+### ::: ultralytics.yolo.utils.autobatch.autobatch
+
diff --git a/yolov8/docs/reference/yolo/utils/benchmarks.md b/yolov8/docs/reference/yolo/utils/benchmarks.md
new file mode 100644
index 0000000000000000000000000000000000000000..7c3750209bed420a33b2fa2f488915a29b51db31
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/benchmarks.md
@@ -0,0 +1,14 @@
+---
+description: Improve your YOLO's performance and measure its speed. Benchmark utility for YOLOv5.
+keywords: Ultralytics YOLO, ProfileModels, benchmark, model inference, detection
+---
+
+## ProfileModels
+---
+### ::: ultralytics.yolo.utils.benchmarks.ProfileModels
+
+
+## benchmark
+---
+### ::: ultralytics.yolo.utils.benchmarks.benchmark
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/base.md b/yolov8/docs/reference/yolo/utils/callbacks/base.md
new file mode 100644
index 0000000000000000000000000000000000000000..bdb3ae154617fb31bf18213d317aeff94e6396fd
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/base.md
@@ -0,0 +1,139 @@
+---
+description: Learn about YOLO's callback functions from on_train_start to add_integration_callbacks. See how these callbacks modify and save models.
+keywords: YOLO, Ultralytics, callbacks, object detection, training, inference
+---
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_pretrain_routine_start
+
+
+## on_pretrain_routine_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_pretrain_routine_end
+
+
+## on_train_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_train_start
+
+
+## on_train_epoch_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_train_epoch_start
+
+
+## on_train_batch_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_train_batch_start
+
+
+## optimizer_step
+---
+### ::: ultralytics.yolo.utils.callbacks.base.optimizer_step
+
+
+## on_before_zero_grad
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_before_zero_grad
+
+
+## on_train_batch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_train_batch_end
+
+
+## on_train_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_train_epoch_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_fit_epoch_end
+
+
+## on_model_save
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_model_save
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_train_end
+
+
+## on_params_update
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_params_update
+
+
+## teardown
+---
+### ::: ultralytics.yolo.utils.callbacks.base.teardown
+
+
+## on_val_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_val_start
+
+
+## on_val_batch_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_val_batch_start
+
+
+## on_val_batch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_val_batch_end
+
+
+## on_val_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_val_end
+
+
+## on_predict_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_predict_start
+
+
+## on_predict_batch_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_predict_batch_start
+
+
+## on_predict_batch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_predict_batch_end
+
+
+## on_predict_postprocess_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_predict_postprocess_end
+
+
+## on_predict_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_predict_end
+
+
+## on_export_start
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_export_start
+
+
+## on_export_end
+---
+### ::: ultralytics.yolo.utils.callbacks.base.on_export_end
+
+
+## get_default_callbacks
+---
+### ::: ultralytics.yolo.utils.callbacks.base.get_default_callbacks
+
+
+## add_integration_callbacks
+---
+### ::: ultralytics.yolo.utils.callbacks.base.add_integration_callbacks
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/clearml.md b/yolov8/docs/reference/yolo/utils/callbacks/clearml.md
new file mode 100644
index 0000000000000000000000000000000000000000..54b8bc6afd997b69a082e6084d9c21e87b88af58
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/clearml.md
@@ -0,0 +1,39 @@
+---
+description: Improve your YOLOv5 model training with callbacks from ClearML. Learn about log debug samples, pre-training routines, validation and more.
+keywords: Ultralytics YOLO, callbacks, log plots, epoch monitoring, training end events
+---
+
+## _log_debug_samples
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml._log_debug_samples
+
+
+## _log_plot
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml._log_plot
+
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml.on_pretrain_routine_start
+
+
+## on_train_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml.on_train_epoch_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml.on_fit_epoch_end
+
+
+## on_val_end
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml.on_val_end
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.clearml.on_train_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/comet.md b/yolov8/docs/reference/yolo/utils/callbacks/comet.md
new file mode 100644
index 0000000000000000000000000000000000000000..1d45191954c1c330ac5b3a219ba0a879917a967d
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/comet.md
@@ -0,0 +1,124 @@
+---
+description: Learn about YOLO callbacks using the Comet.ml platform, enhancing object detection training and testing with custom logging and visualizations.
+keywords: Ultralytics, YOLO, callbacks, Comet ML, log images, log predictions, log plots, fetch metadata, fetch annotations, create experiment data, format experiment data
+---
+
+## _get_comet_mode
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._get_comet_mode
+
+
+## _get_comet_model_name
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._get_comet_model_name
+
+
+## _get_eval_batch_logging_interval
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._get_eval_batch_logging_interval
+
+
+## _get_max_image_predictions_to_log
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._get_max_image_predictions_to_log
+
+
+## _scale_confidence_score
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._scale_confidence_score
+
+
+## _should_log_confusion_matrix
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._should_log_confusion_matrix
+
+
+## _should_log_image_predictions
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._should_log_image_predictions
+
+
+## _get_experiment_type
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._get_experiment_type
+
+
+## _create_experiment
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._create_experiment
+
+
+## _fetch_trainer_metadata
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._fetch_trainer_metadata
+
+
+## _scale_bounding_box_to_original_image_shape
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._scale_bounding_box_to_original_image_shape
+
+
+## _format_ground_truth_annotations_for_detection
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._format_ground_truth_annotations_for_detection
+
+
+## _format_prediction_annotations_for_detection
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._format_prediction_annotations_for_detection
+
+
+## _fetch_annotations
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._fetch_annotations
+
+
+## _create_prediction_metadata_map
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._create_prediction_metadata_map
+
+
+## _log_confusion_matrix
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._log_confusion_matrix
+
+
+## _log_images
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._log_images
+
+
+## _log_image_predictions
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._log_image_predictions
+
+
+## _log_plots
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._log_plots
+
+
+## _log_model
+---
+### ::: ultralytics.yolo.utils.callbacks.comet._log_model
+
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.comet.on_pretrain_routine_start
+
+
+## on_train_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.comet.on_train_epoch_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.comet.on_fit_epoch_end
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.comet.on_train_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/dvc.md b/yolov8/docs/reference/yolo/utils/callbacks/dvc.md
new file mode 100644
index 0000000000000000000000000000000000000000..b32fc7a47e8b3acaaeca39b4eaf3fb62362db45d
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/dvc.md
@@ -0,0 +1,54 @@
+---
+description: Explore Ultralytics YOLO Utils DVC Callbacks such as logging images, plots, confusion matrices, and training progress.
+keywords: Ultralytics, YOLO, Utils, DVC, Callbacks, images, plots, confusion matrices, training progress
+---
+
+## _logger_disabled
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc._logger_disabled
+
+
+## _log_images
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc._log_images
+
+
+## _log_plots
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc._log_plots
+
+
+## _log_confusion_matrix
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc._log_confusion_matrix
+
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc.on_pretrain_routine_start
+
+
+## on_pretrain_routine_end
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc.on_pretrain_routine_end
+
+
+## on_train_start
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc.on_train_start
+
+
+## on_train_epoch_start
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc.on_train_epoch_start
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc.on_fit_epoch_end
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.dvc.on_train_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/hub.md b/yolov8/docs/reference/yolo/utils/callbacks/hub.md
new file mode 100644
index 0000000000000000000000000000000000000000..7b2419982a165f8877ba6ea836ae5d56805ced30
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/hub.md
@@ -0,0 +1,44 @@
+---
+description: Improve YOLOv5 model training with Ultralytics' on-train callbacks. Boost performance on-pretrain-routine-end, model-save, train/predict start.
+keywords: Ultralytics, YOLO, callbacks, on_pretrain_routine_end, on_fit_epoch_end, on_train_start, on_val_start, on_predict_start, on_export_start
+---
+
+## on_pretrain_routine_end
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_pretrain_routine_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_fit_epoch_end
+
+
+## on_model_save
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_model_save
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_train_end
+
+
+## on_train_start
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_train_start
+
+
+## on_val_start
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_val_start
+
+
+## on_predict_start
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_predict_start
+
+
+## on_export_start
+---
+### ::: ultralytics.yolo.utils.callbacks.hub.on_export_start
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/mlflow.md b/yolov8/docs/reference/yolo/utils/callbacks/mlflow.md
new file mode 100644
index 0000000000000000000000000000000000000000..9d69d0fd7a200ab1fb8f50121b6d1a3f05e26324
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/mlflow.md
@@ -0,0 +1,19 @@
+---
+description: Track model performance and metrics with MLflow in YOLOv5. Use callbacks like on_pretrain_routine_end or on_train_end to log information.
+keywords: Ultralytics, YOLO, Utils, MLflow, callbacks, on_pretrain_routine_end, on_train_end, Tracking, Model Management, training
+---
+
+## on_pretrain_routine_end
+---
+### ::: ultralytics.yolo.utils.callbacks.mlflow.on_pretrain_routine_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.mlflow.on_fit_epoch_end
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.mlflow.on_train_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/neptune.md b/yolov8/docs/reference/yolo/utils/callbacks/neptune.md
new file mode 100644
index 0000000000000000000000000000000000000000..cadbfb515e2dd0aed903d526e5936f66f26402ff
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/neptune.md
@@ -0,0 +1,44 @@
+---
+description: Improve YOLOv5 training with Neptune, a powerful logging tool. Track metrics like images, plots, and epochs for better model performance.
+keywords: Ultralytics, YOLO, Neptune, Callbacks, log scalars, log images, log plots, training, validation
+---
+
+## _log_scalars
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune._log_scalars
+
+
+## _log_images
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune._log_images
+
+
+## _log_plot
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune._log_plot
+
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune.on_pretrain_routine_start
+
+
+## on_train_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune.on_train_epoch_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune.on_fit_epoch_end
+
+
+## on_val_end
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune.on_val_end
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.neptune.on_train_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/raytune.md b/yolov8/docs/reference/yolo/utils/callbacks/raytune.md
new file mode 100644
index 0000000000000000000000000000000000000000..2771c2e22592d92cbd8a200ce7c461566b59f5e5
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/raytune.md
@@ -0,0 +1,9 @@
+---
+description: '"Improve YOLO model performance with on_fit_epoch_end callback. Learn to integrate with Ray Tune for hyperparameter tuning. Ultralytics YOLO docs."'
+keywords: on_fit_epoch_end, Ultralytics YOLO, callback function, training, model tuning
+---
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.raytune.on_fit_epoch_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/tensorboard.md b/yolov8/docs/reference/yolo/utils/callbacks/tensorboard.md
new file mode 100644
index 0000000000000000000000000000000000000000..b5eea1be36312e2ea52128256916a528ceb62b33
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/tensorboard.md
@@ -0,0 +1,24 @@
+---
+description: Learn how to monitor the training process with Tensorboard using Ultralytics YOLO's "_log_scalars" and "on_batch_end" methods.
+keywords: TensorBoard callbacks, YOLO training, ultralytics YOLO
+---
+
+## _log_scalars
+---
+### ::: ultralytics.yolo.utils.callbacks.tensorboard._log_scalars
+
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.tensorboard.on_pretrain_routine_start
+
+
+## on_batch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.tensorboard.on_batch_end
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.tensorboard.on_fit_epoch_end
+
diff --git a/yolov8/docs/reference/yolo/utils/callbacks/wb.md b/yolov8/docs/reference/yolo/utils/callbacks/wb.md
new file mode 100644
index 0000000000000000000000000000000000000000..1116adc8958d700c369632d9b7df52ce0d4b2d51
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/callbacks/wb.md
@@ -0,0 +1,29 @@
+---
+description: Learn how to use Ultralytics YOLO's built-in callbacks `on_pretrain_routine_start` and `on_train_epoch_end` for improved training performance.
+keywords: Ultralytics, YOLO, callbacks, weights, biases, training
+---
+
+## _log_plots
+---
+### ::: ultralytics.yolo.utils.callbacks.wb._log_plots
+
+
+## on_pretrain_routine_start
+---
+### ::: ultralytics.yolo.utils.callbacks.wb.on_pretrain_routine_start
+
+
+## on_fit_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.wb.on_fit_epoch_end
+
+
+## on_train_epoch_end
+---
+### ::: ultralytics.yolo.utils.callbacks.wb.on_train_epoch_end
+
+
+## on_train_end
+---
+### ::: ultralytics.yolo.utils.callbacks.wb.on_train_end
+
diff --git a/yolov8/docs/reference/yolo/utils/checks.md b/yolov8/docs/reference/yolo/utils/checks.md
new file mode 100644
index 0000000000000000000000000000000000000000..14bc9bd36b80123f86077a4aa159ef37f72ddec6
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/checks.md
@@ -0,0 +1,89 @@
+---
+description: 'Check functions for YOLO utils: image size, version, font, requirements, filename suffix, YAML file, YOLO, and Git version.'
+keywords: YOLO, Ultralytics, Utils, Checks, image sizing, version updates, font compatibility, Python requirements, file suffixes, YAML syntax, image showing, AMP
+---
+
+## is_ascii
+---
+### ::: ultralytics.yolo.utils.checks.is_ascii
+
+
+## check_imgsz
+---
+### ::: ultralytics.yolo.utils.checks.check_imgsz
+
+
+## check_version
+---
+### ::: ultralytics.yolo.utils.checks.check_version
+
+
+## check_latest_pypi_version
+---
+### ::: ultralytics.yolo.utils.checks.check_latest_pypi_version
+
+
+## check_pip_update_available
+---
+### ::: ultralytics.yolo.utils.checks.check_pip_update_available
+
+
+## check_font
+---
+### ::: ultralytics.yolo.utils.checks.check_font
+
+
+## check_python
+---
+### ::: ultralytics.yolo.utils.checks.check_python
+
+
+## check_requirements
+---
+### ::: ultralytics.yolo.utils.checks.check_requirements
+
+
+## check_suffix
+---
+### ::: ultralytics.yolo.utils.checks.check_suffix
+
+
+## check_yolov5u_filename
+---
+### ::: ultralytics.yolo.utils.checks.check_yolov5u_filename
+
+
+## check_file
+---
+### ::: ultralytics.yolo.utils.checks.check_file
+
+
+## check_yaml
+---
+### ::: ultralytics.yolo.utils.checks.check_yaml
+
+
+## check_imshow
+---
+### ::: ultralytics.yolo.utils.checks.check_imshow
+
+
+## check_yolo
+---
+### ::: ultralytics.yolo.utils.checks.check_yolo
+
+
+## check_amp
+---
+### ::: ultralytics.yolo.utils.checks.check_amp
+
+
+## git_describe
+---
+### ::: ultralytics.yolo.utils.checks.git_describe
+
+
+## print_args
+---
+### ::: ultralytics.yolo.utils.checks.print_args
+
diff --git a/yolov8/docs/reference/yolo/utils/dist.md b/yolov8/docs/reference/yolo/utils/dist.md
new file mode 100644
index 0000000000000000000000000000000000000000..3e5033ebaa11a05979a08232c7808ebdb7f387e5
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/dist.md
@@ -0,0 +1,24 @@
+---
+description: Learn how to find free network port and generate DDP (Distributed Data Parallel) command in Ultralytics YOLO with easy examples.
+keywords: ultralytics, YOLO, utils, dist, distributed deep learning, DDP file, DDP cleanup
+---
+
+## find_free_network_port
+---
+### ::: ultralytics.yolo.utils.dist.find_free_network_port
+
+
+## generate_ddp_file
+---
+### ::: ultralytics.yolo.utils.dist.generate_ddp_file
+
+
+## generate_ddp_command
+---
+### ::: ultralytics.yolo.utils.dist.generate_ddp_command
+
+
+## ddp_cleanup
+---
+### ::: ultralytics.yolo.utils.dist.ddp_cleanup
+
diff --git a/yolov8/docs/reference/yolo/utils/downloads.md b/yolov8/docs/reference/yolo/utils/downloads.md
new file mode 100644
index 0000000000000000000000000000000000000000..dd07646c17f7124191e0040532d6d4b55be0b5e8
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/downloads.md
@@ -0,0 +1,34 @@
+---
+description: Download and unzip YOLO pretrained models. Ultralytics YOLO docs utils.downloads.unzip_file, checks disk space, downloads and attempts assets.
+keywords: Ultralytics YOLO, downloads, trained models, datasets, weights, deep learning, computer vision
+---
+
+## is_url
+---
+### ::: ultralytics.yolo.utils.downloads.is_url
+
+
+## unzip_file
+---
+### ::: ultralytics.yolo.utils.downloads.unzip_file
+
+
+## check_disk_space
+---
+### ::: ultralytics.yolo.utils.downloads.check_disk_space
+
+
+## safe_download
+---
+### ::: ultralytics.yolo.utils.downloads.safe_download
+
+
+## attempt_download_asset
+---
+### ::: ultralytics.yolo.utils.downloads.attempt_download_asset
+
+
+## download
+---
+### ::: ultralytics.yolo.utils.downloads.download
+
diff --git a/yolov8/docs/reference/yolo/utils/errors.md b/yolov8/docs/reference/yolo/utils/errors.md
new file mode 100644
index 0000000000000000000000000000000000000000..f3ddfc41f72c438826d54ff4f241e88e3751ec74
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/errors.md
@@ -0,0 +1,9 @@
+---
+description: Learn about HUBModelError in Ultralytics YOLO Docs. Resolve the error and get the most out of your YOLO model.
+keywords: HUBModelError, Ultralytics YOLO, YOLO Documentation, Object detection errors, YOLO Errors, HUBModelError Solutions
+---
+
+## HUBModelError
+---
+### ::: ultralytics.yolo.utils.errors.HUBModelError
+
diff --git a/yolov8/docs/reference/yolo/utils/files.md b/yolov8/docs/reference/yolo/utils/files.md
new file mode 100644
index 0000000000000000000000000000000000000000..bad47b43a265b07719793c952235433fd5fb9f85
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/files.md
@@ -0,0 +1,39 @@
+---
+description: 'Learn about Ultralytics YOLO files and directory utilities: WorkingDirectory, file_age, file_size, and make_dirs.'
+keywords: YOLO, object detection, file utils, file age, file size, working directory, make directories, Ultralytics Docs
+---
+
+## WorkingDirectory
+---
+### ::: ultralytics.yolo.utils.files.WorkingDirectory
+
+
+## increment_path
+---
+### ::: ultralytics.yolo.utils.files.increment_path
+
+
+## file_age
+---
+### ::: ultralytics.yolo.utils.files.file_age
+
+
+## file_date
+---
+### ::: ultralytics.yolo.utils.files.file_date
+
+
+## file_size
+---
+### ::: ultralytics.yolo.utils.files.file_size
+
+
+## get_latest_run
+---
+### ::: ultralytics.yolo.utils.files.get_latest_run
+
+
+## make_dirs
+---
+### ::: ultralytics.yolo.utils.files.make_dirs
+
diff --git a/yolov8/docs/reference/yolo/utils/instance.md b/yolov8/docs/reference/yolo/utils/instance.md
new file mode 100644
index 0000000000000000000000000000000000000000..953fe222e88f558cec6a58755b28e8dfeb17750f
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/instance.md
@@ -0,0 +1,19 @@
+---
+description: Learn about Bounding Boxes (Bboxes) and _ntuple in Ultralytics YOLO for object detection. Improve accuracy and speed with these powerful tools.
+keywords: Ultralytics, YOLO, Bboxes, _ntuple, object detection, instance segmentation
+---
+
+## Bboxes
+---
+### ::: ultralytics.yolo.utils.instance.Bboxes
+
+
+## Instances
+---
+### ::: ultralytics.yolo.utils.instance.Instances
+
+
+## _ntuple
+---
+### ::: ultralytics.yolo.utils.instance._ntuple
+
diff --git a/yolov8/docs/reference/yolo/utils/loss.md b/yolov8/docs/reference/yolo/utils/loss.md
new file mode 100644
index 0000000000000000000000000000000000000000..7d89d6e5cdffe471485d4930723aee411425105a
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/loss.md
@@ -0,0 +1,44 @@
+---
+description: Learn about Varifocal Loss and Keypoint Loss in Ultralytics YOLO for advanced bounding box and pose estimation. Visit our docs for more.
+keywords: Ultralytics, YOLO, loss functions, object detection, keypoint detection, segmentation, classification
+---
+
+## VarifocalLoss
+---
+### ::: ultralytics.yolo.utils.loss.VarifocalLoss
+
+
+## FocalLoss
+---
+### ::: ultralytics.yolo.utils.loss.FocalLoss
+
+
+## BboxLoss
+---
+### ::: ultralytics.yolo.utils.loss.BboxLoss
+
+
+## KeypointLoss
+---
+### ::: ultralytics.yolo.utils.loss.KeypointLoss
+
+
+## v8DetectionLoss
+---
+### ::: ultralytics.yolo.utils.loss.v8DetectionLoss
+
+
+## v8SegmentationLoss
+---
+### ::: ultralytics.yolo.utils.loss.v8SegmentationLoss
+
+
+## v8PoseLoss
+---
+### ::: ultralytics.yolo.utils.loss.v8PoseLoss
+
+
+## v8ClassificationLoss
+---
+### ::: ultralytics.yolo.utils.loss.v8ClassificationLoss
+
diff --git a/yolov8/docs/reference/yolo/utils/metrics.md b/yolov8/docs/reference/yolo/utils/metrics.md
new file mode 100644
index 0000000000000000000000000000000000000000..204096dca28db48120f1364a321b318c3e3ecb85
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/metrics.md
@@ -0,0 +1,94 @@
+---
+description: Explore Ultralytics YOLO's FocalLoss, DetMetrics, PoseMetrics, ClassifyMetrics, and more with Ultralytics Metrics documentation.
+keywords: YOLOv5, metrics, losses, confusion matrix, detection metrics, pose metrics, classification metrics, intersection over area, intersection over union, keypoint intersection over union, average precision, per class average precision, Ultralytics Docs
+---
+
+## ConfusionMatrix
+---
+### ::: ultralytics.yolo.utils.metrics.ConfusionMatrix
+
+
+## Metric
+---
+### ::: ultralytics.yolo.utils.metrics.Metric
+
+
+## DetMetrics
+---
+### ::: ultralytics.yolo.utils.metrics.DetMetrics
+
+
+## SegmentMetrics
+---
+### ::: ultralytics.yolo.utils.metrics.SegmentMetrics
+
+
+## PoseMetrics
+---
+### ::: ultralytics.yolo.utils.metrics.PoseMetrics
+
+
+## ClassifyMetrics
+---
+### ::: ultralytics.yolo.utils.metrics.ClassifyMetrics
+
+
+## box_area
+---
+### ::: ultralytics.yolo.utils.metrics.box_area
+
+
+## bbox_ioa
+---
+### ::: ultralytics.yolo.utils.metrics.bbox_ioa
+
+
+## box_iou
+---
+### ::: ultralytics.yolo.utils.metrics.box_iou
+
+
+## bbox_iou
+---
+### ::: ultralytics.yolo.utils.metrics.bbox_iou
+
+
+## mask_iou
+---
+### ::: ultralytics.yolo.utils.metrics.mask_iou
+
+
+## kpt_iou
+---
+### ::: ultralytics.yolo.utils.metrics.kpt_iou
+
+
+## smooth_BCE
+---
+### ::: ultralytics.yolo.utils.metrics.smooth_BCE
+
+
+## smooth
+---
+### ::: ultralytics.yolo.utils.metrics.smooth
+
+
+## plot_pr_curve
+---
+### ::: ultralytics.yolo.utils.metrics.plot_pr_curve
+
+
+## plot_mc_curve
+---
+### ::: ultralytics.yolo.utils.metrics.plot_mc_curve
+
+
+## compute_ap
+---
+### ::: ultralytics.yolo.utils.metrics.compute_ap
+
+
+## ap_per_class
+---
+### ::: ultralytics.yolo.utils.metrics.ap_per_class
+
diff --git a/yolov8/docs/reference/yolo/utils/ops.md b/yolov8/docs/reference/yolo/utils/ops.md
new file mode 100644
index 0000000000000000000000000000000000000000..f35584a07ec4b17e1f5244c4f8f369a504543741
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/ops.md
@@ -0,0 +1,139 @@
+---
+description: Learn about various utility functions in Ultralytics YOLO, including x, y, width, height conversions, non-max suppression, and more.
+keywords: Ultralytics, YOLO, Utils Ops, Functions, coco80_to_coco91_class, scale_boxes, non_max_suppression, clip_coords, xyxy2xywh, xywhn2xyxy, xyn2xy, xyxy2ltwh, ltwh2xyxy, resample_segments, process_mask_upsample, process_mask_native, masks2segments, clean_str
+---
+
+## Profile
+---
+### ::: ultralytics.yolo.utils.ops.Profile
+
+
+## coco80_to_coco91_class
+---
+### ::: ultralytics.yolo.utils.ops.coco80_to_coco91_class
+
+
+## segment2box
+---
+### ::: ultralytics.yolo.utils.ops.segment2box
+
+
+## scale_boxes
+---
+### ::: ultralytics.yolo.utils.ops.scale_boxes
+
+
+## make_divisible
+---
+### ::: ultralytics.yolo.utils.ops.make_divisible
+
+
+## non_max_suppression
+---
+### ::: ultralytics.yolo.utils.ops.non_max_suppression
+
+
+## clip_boxes
+---
+### ::: ultralytics.yolo.utils.ops.clip_boxes
+
+
+## clip_coords
+---
+### ::: ultralytics.yolo.utils.ops.clip_coords
+
+
+## scale_image
+---
+### ::: ultralytics.yolo.utils.ops.scale_image
+
+
+## xyxy2xywh
+---
+### ::: ultralytics.yolo.utils.ops.xyxy2xywh
+
+
+## xywh2xyxy
+---
+### ::: ultralytics.yolo.utils.ops.xywh2xyxy
+
+
+## xywhn2xyxy
+---
+### ::: ultralytics.yolo.utils.ops.xywhn2xyxy
+
+
+## xyxy2xywhn
+---
+### ::: ultralytics.yolo.utils.ops.xyxy2xywhn
+
+
+## xyn2xy
+---
+### ::: ultralytics.yolo.utils.ops.xyn2xy
+
+
+## xywh2ltwh
+---
+### ::: ultralytics.yolo.utils.ops.xywh2ltwh
+
+
+## xyxy2ltwh
+---
+### ::: ultralytics.yolo.utils.ops.xyxy2ltwh
+
+
+## ltwh2xywh
+---
+### ::: ultralytics.yolo.utils.ops.ltwh2xywh
+
+
+## ltwh2xyxy
+---
+### ::: ultralytics.yolo.utils.ops.ltwh2xyxy
+
+
+## segments2boxes
+---
+### ::: ultralytics.yolo.utils.ops.segments2boxes
+
+
+## resample_segments
+---
+### ::: ultralytics.yolo.utils.ops.resample_segments
+
+
+## crop_mask
+---
+### ::: ultralytics.yolo.utils.ops.crop_mask
+
+
+## process_mask_upsample
+---
+### ::: ultralytics.yolo.utils.ops.process_mask_upsample
+
+
+## process_mask
+---
+### ::: ultralytics.yolo.utils.ops.process_mask
+
+
+## process_mask_native
+---
+### ::: ultralytics.yolo.utils.ops.process_mask_native
+
+
+## scale_coords
+---
+### ::: ultralytics.yolo.utils.ops.scale_coords
+
+
+## masks2segments
+---
+### ::: ultralytics.yolo.utils.ops.masks2segments
+
+
+## clean_str
+---
+### ::: ultralytics.yolo.utils.ops.clean_str
+
diff --git a/yolov8/docs/reference/yolo/utils/patches.md b/yolov8/docs/reference/yolo/utils/patches.md
new file mode 100644
index 0000000000000000000000000000000000000000..85ceefa323e7e5b8fd16816becc71fb3f5c8ace7
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/patches.md
@@ -0,0 +1,24 @@
+---
+description: Learn how to use the Ultralytics YOLO Utils package's imread and imshow functions. These functions are used for reading and writing image files. Try out our TorchSave feature today.
+keywords: imread, imshow, ultralytics, YOLO, image files, torchsave
+---
+
+## imread
+---
+### ::: ultralytics.yolo.utils.patches.imread
+
+
+## imwrite
+---
+### ::: ultralytics.yolo.utils.patches.imwrite
+
+
+## imshow
+---
+### ::: ultralytics.yolo.utils.patches.imshow
+
+
+## torch_save
+---
+### ::: ultralytics.yolo.utils.patches.torch_save
+
diff --git a/yolov8/docs/reference/yolo/utils/plotting.md b/yolov8/docs/reference/yolo/utils/plotting.md
new file mode 100644
index 0000000000000000000000000000000000000000..0fd0babd091b8c7d8f9e46d183fe412b5eb326dd
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/plotting.md
@@ -0,0 +1,44 @@
+---
+description: 'Discover the power of YOLO''s plotting functions: Colors, Labels and Images. Code examples to output targets and visualize features. Check it now.'
+keywords: YOLO, object detection, plotting, visualization, annotator, save one box, plot results, feature visualization, Ultralytics
+---
+
+## Colors
+---
+### ::: ultralytics.yolo.utils.plotting.Colors
+
+
+## Annotator
+---
+### ::: ultralytics.yolo.utils.plotting.Annotator
+
+
+## plot_labels
+---
+### ::: ultralytics.yolo.utils.plotting.plot_labels
+
+
+## save_one_box
+---
+### ::: ultralytics.yolo.utils.plotting.save_one_box
+
+
+## plot_images
+---
+### ::: ultralytics.yolo.utils.plotting.plot_images
+
+
+## plot_results
+---
+### ::: ultralytics.yolo.utils.plotting.plot_results
+
+
+## output_to_target
+---
+### ::: ultralytics.yolo.utils.plotting.output_to_target
+
+
+## feature_visualization
+---
+### ::: ultralytics.yolo.utils.plotting.feature_visualization
+
diff --git a/yolov8/docs/reference/yolo/utils/tal.md b/yolov8/docs/reference/yolo/utils/tal.md
new file mode 100644
index 0000000000000000000000000000000000000000..f5a665289402135a096a4b2157e0aed7b6b8aa83
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/tal.md
@@ -0,0 +1,34 @@
+---
+description: Improve your YOLO models with Ultralytics' TaskAlignedAssigner, select_highest_overlaps, and dist2bbox utilities. Streamline your workflow today.
+keywords: Ultrayltics, YOLO, select_candidates_in_gts, make_anchor, bbox2dist, object detection, tracking
+---
+
+## TaskAlignedAssigner
+---
+### ::: ultralytics.yolo.utils.tal.TaskAlignedAssigner
+
+
+## select_candidates_in_gts
+---
+### ::: ultralytics.yolo.utils.tal.select_candidates_in_gts
+
+
+## select_highest_overlaps
+---
+### ::: ultralytics.yolo.utils.tal.select_highest_overlaps
+
+
+## make_anchors
+---
+### ::: ultralytics.yolo.utils.tal.make_anchors
+
+
+## dist2bbox
+---
+### ::: ultralytics.yolo.utils.tal.dist2bbox
+
+
+## bbox2dist
+---
+### ::: ultralytics.yolo.utils.tal.bbox2dist
+
diff --git a/yolov8/docs/reference/yolo/utils/torch_utils.md b/yolov8/docs/reference/yolo/utils/torch_utils.md
new file mode 100644
index 0000000000000000000000000000000000000000..6d24cac45bc9d9cf6020f52073c2083f5b0b58d5
--- /dev/null
+++ b/yolov8/docs/reference/yolo/utils/torch_utils.md
@@ -0,0 +1,134 @@
+---
+description: Optimize your PyTorch models with Ultralytics YOLO's torch_utils functions such as ModelEMA, select_device, and is_parallel.
+keywords: Ultralytics YOLO, Torch, Utils, Pytorch, Object Detection
+---
+
+## ModelEMA
+---
+### ::: ultralytics.yolo.utils.torch_utils.ModelEMA
+
+
+## EarlyStopping
+---
+### ::: ultralytics.yolo.utils.torch_utils.EarlyStopping
+
+
+## torch_distributed_zero_first
+---
+### ::: ultralytics.yolo.utils.torch_utils.torch_distributed_zero_first
+
+
+## smart_inference_mode
+---
+### ::: ultralytics.yolo.utils.torch_utils.smart_inference_mode
+
+
+## select_device
+---
+### ::: ultralytics.yolo.utils.torch_utils.select_device
+
+
+## time_sync
+---
+### ::: ultralytics.yolo.utils.torch_utils.time_sync
+
+
+## fuse_conv_and_bn
+---
+### ::: ultralytics.yolo.utils.torch_utils.fuse_conv_and_bn
+
+
+## fuse_deconv_and_bn
+---
+### ::: ultralytics.yolo.utils.torch_utils.fuse_deconv_and_bn
+
+
+## model_info
+---
+### ::: ultralytics.yolo.utils.torch_utils.model_info
+
+
+## get_num_params
+---
+### ::: ultralytics.yolo.utils.torch_utils.get_num_params
+
+
+## get_num_gradients
+---
+### ::: ultralytics.yolo.utils.torch_utils.get_num_gradients
+
+
+## model_info_for_loggers
+---
+### ::: ultralytics.yolo.utils.torch_utils.model_info_for_loggers
+
+
+## get_flops
+---
+### ::: ultralytics.yolo.utils.torch_utils.get_flops
+
+
+## get_flops_with_torch_profiler
+---
+### ::: ultralytics.yolo.utils.torch_utils.get_flops_with_torch_profiler
+
+
+## initialize_weights
+---
+### ::: ultralytics.yolo.utils.torch_utils.initialize_weights
+
+
+## scale_img
+---
+### ::: ultralytics.yolo.utils.torch_utils.scale_img
+
+
+## make_divisible
+---
+### ::: ultralytics.yolo.utils.torch_utils.make_divisible
+
+
+## copy_attr
+---
+### ::: ultralytics.yolo.utils.torch_utils.copy_attr
+
+
+## get_latest_opset
+---
+### ::: ultralytics.yolo.utils.torch_utils.get_latest_opset
+
+
+## intersect_dicts
+---
+### ::: ultralytics.yolo.utils.torch_utils.intersect_dicts
+
+
+## is_parallel
+---
+### ::: ultralytics.yolo.utils.torch_utils.is_parallel
+
+
+## de_parallel
+---
+### ::: ultralytics.yolo.utils.torch_utils.de_parallel
+
+
+## one_cycle
+---
+### ::: ultralytics.yolo.utils.torch_utils.one_cycle
+
+
+## init_seeds
+---
+### ::: ultralytics.yolo.utils.torch_utils.init_seeds
+
+
+## strip_optimizer
+---
+### ::: ultralytics.yolo.utils.torch_utils.strip_optimizer
+
+
+## profile
+---
+### ::: ultralytics.yolo.utils.torch_utils.profile
+
diff --git a/yolov8/docs/reference/yolo/v8/classify/predict.md b/yolov8/docs/reference/yolo/v8/classify/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..83bd5d6bf672e887b92fa2bd6315fe6b4567641b
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/classify/predict.md
@@ -0,0 +1,14 @@
+---
+description: Learn how to use ClassificationPredictor in Ultralytics YOLOv8 for object classification tasks in a simple and efficient way.
+keywords: Ultralytics, YOLO, v8, Classify Predictor, object detection, classification, computer vision
+---
+
+## ClassificationPredictor
+---
+### ::: ultralytics.yolo.v8.classify.predict.ClassificationPredictor
+
+
+## predict
+---
+### ::: ultralytics.yolo.v8.classify.predict.predict
+
diff --git a/yolov8/docs/reference/yolo/v8/classify/train.md b/yolov8/docs/reference/yolo/v8/classify/train.md
new file mode 100644
index 0000000000000000000000000000000000000000..f488eac15f6e97db191d7debc909b28f1be4e886
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/classify/train.md
@@ -0,0 +1,14 @@
+---
+description: Train a custom image classification model using Ultralytics YOLOv8 with ClassificationTrainer. Boost accuracy and efficiency today.
+keywords: Ultralytics, YOLOv8, object detection, classification, training, API
+---
+
+## ClassificationTrainer
+---
+### ::: ultralytics.yolo.v8.classify.train.ClassificationTrainer
+
+
+## train
+---
+### ::: ultralytics.yolo.v8.classify.train.train
+
diff --git a/yolov8/docs/reference/yolo/v8/classify/val.md b/yolov8/docs/reference/yolo/v8/classify/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..76fe5305ac4e5150f7ca912e130a8899edde79c2
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/classify/val.md
@@ -0,0 +1,14 @@
+---
+description: Ensure model classification accuracy with Ultralytics YOLO's ClassificationValidator. Validate and improve your model with ease.
+keywords: ClassificationValidator, Ultralytics YOLO, Validation, Data Science, Deep Learning
+---
+
+## ClassificationValidator
+---
+### ::: ultralytics.yolo.v8.classify.val.ClassificationValidator
+
+
+## val
+---
+### ::: ultralytics.yolo.v8.classify.val.val
+
diff --git a/yolov8/docs/reference/yolo/v8/detect/predict.md b/yolov8/docs/reference/yolo/v8/detect/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..63499d3c7e9298149a1a9c4d6ee002a114d7033e
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/detect/predict.md
@@ -0,0 +1,14 @@
+---
+description: Detect and predict objects in images and videos using the Ultralytics YOLO v8 model with DetectionPredictor.
+keywords: detectionpredictor, ultralytics yolo, object detection, neural network, machine learning
+---
+
+## DetectionPredictor
+---
+### ::: ultralytics.yolo.v8.detect.predict.DetectionPredictor
+
+
+## predict
+---
+### ::: ultralytics.yolo.v8.detect.predict.predict
+
diff --git a/yolov8/docs/reference/yolo/v8/detect/train.md b/yolov8/docs/reference/yolo/v8/detect/train.md
new file mode 100644
index 0000000000000000000000000000000000000000..8cf2809b1a9eadb90140767fd68fe1b43f20cb7c
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/detect/train.md
@@ -0,0 +1,14 @@
+---
+description: Train and optimize custom object detection models with Ultralytics DetectionTrainer and train functions. Get started with YOLO v8 today.
+keywords: DetectionTrainer, Ultralytics YOLO, custom object detection, train models, AI applications
+---
+
+## DetectionTrainer
+---
+### ::: ultralytics.yolo.v8.detect.train.DetectionTrainer
+
+
+## train
+---
+### ::: ultralytics.yolo.v8.detect.train.train
+
diff --git a/yolov8/docs/reference/yolo/v8/detect/val.md b/yolov8/docs/reference/yolo/v8/detect/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..06ef65f4ff62848c53510bd2823087edd839a824
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/detect/val.md
@@ -0,0 +1,14 @@
+---
+description: Validate YOLOv5 detections using this PyTorch module. Ensure model accuracy with NMS IOU threshold tuning and label mapping.
+keywords: detection, validator, YOLOv5, object detection, model improvement, Ultralytics Docs
+---
+
+## DetectionValidator
+---
+### ::: ultralytics.yolo.v8.detect.val.DetectionValidator
+
+
+## val
+---
+### ::: ultralytics.yolo.v8.detect.val.val
+
diff --git a/yolov8/docs/reference/yolo/v8/pose/predict.md b/yolov8/docs/reference/yolo/v8/pose/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..f8ac26b30a3548967216f1363c2c719176105ce0
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/pose/predict.md
@@ -0,0 +1,14 @@
+---
+description: Predict human pose coordinates and confidence scores using YOLOv5. Use on real-time video streams or static images.
+keywords: Ultralytics, YOLO, v8, documentation, PosePredictor, pose prediction, pose estimation, predict method
+---
+
+## PosePredictor
+---
+### ::: ultralytics.yolo.v8.pose.predict.PosePredictor
+
+
+## predict
+---
+### ::: ultralytics.yolo.v8.pose.predict.predict
+
diff --git a/yolov8/docs/reference/yolo/v8/pose/train.md b/yolov8/docs/reference/yolo/v8/pose/train.md
new file mode 100644
index 0000000000000000000000000000000000000000..8c988fe7bb339d5c2ff0303e5eea9dfc1c029213
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/pose/train.md
@@ -0,0 +1,14 @@
+---
+description: Boost posture detection using PoseTrainer and train models using train() API. Learn PoseLoss for ultra-fast and accurate pose detection with Ultralytics YOLO.
+keywords: PoseTrainer, human pose models, deep learning, computer vision, Ultralytics YOLO, v8
+---
+
+## PoseTrainer
+---
+### ::: ultralytics.yolo.v8.pose.train.PoseTrainer
+
+
+## train
+---
+### ::: ultralytics.yolo.v8.pose.train.train
+
diff --git a/yolov8/docs/reference/yolo/v8/pose/val.md b/yolov8/docs/reference/yolo/v8/pose/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..c26c255e3010b579ee0734431fdf22897b458bdb
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/pose/val.md
@@ -0,0 +1,14 @@
+---
+description: Ensure proper human poses in images with YOLOv8 Pose Validation, part of the Ultralytics YOLO v8 suite.
+keywords: PoseValidator, Ultralytics YOLO, object detection, pose analysis, validation
+---
+
+## PoseValidator
+---
+### ::: ultralytics.yolo.v8.pose.val.PoseValidator
+
+
+## val
+---
+### ::: ultralytics.yolo.v8.pose.val.val
+
diff --git a/yolov8/docs/reference/yolo/v8/segment/predict.md b/yolov8/docs/reference/yolo/v8/segment/predict.md
new file mode 100644
index 0000000000000000000000000000000000000000..eadf463f65a560a285fdc02c849d6601e1b43a98
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/segment/predict.md
@@ -0,0 +1,14 @@
+---
+description: '"Use SegmentationPredictor in YOLOv8 for efficient object detection and segmentation. Explore Ultralytics YOLO Docs for more information."'
+keywords: Ultralytics YOLO, SegmentationPredictor, object detection, segmentation masks, predict
+---
+
+## SegmentationPredictor
+---
+### ::: ultralytics.yolo.v8.segment.predict.SegmentationPredictor
+
+
+## predict
+---
+### ::: ultralytics.yolo.v8.segment.predict.predict
+
diff --git a/yolov8/docs/reference/yolo/v8/segment/train.md b/yolov8/docs/reference/yolo/v8/segment/train.md
new file mode 100644
index 0000000000000000000000000000000000000000..20bcc91f8a3b30779bd3eaab3ad85a1c2f3f86b2
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/segment/train.md
@@ -0,0 +1,14 @@
+---
+description: Learn about SegmentationTrainer and Train in Ultralytics YOLO v8 for efficient object detection models. Improve your training with Ultralytics Docs.
+keywords: SegmentationTrainer, Ultralytics YOLO, object detection, segmentation, train, tutorial, guide, code examples
+---
+
+## SegmentationTrainer
+---
+### ::: ultralytics.yolo.v8.segment.train.SegmentationTrainer
+
+
+## train
+---
+### ::: ultralytics.yolo.v8.segment.train.train
+
diff --git a/yolov8/docs/reference/yolo/v8/segment/val.md b/yolov8/docs/reference/yolo/v8/segment/val.md
new file mode 100644
index 0000000000000000000000000000000000000000..137302f88b7d0d8e2e9dcc28dab2fe496bb7a5a1
--- /dev/null
+++ b/yolov8/docs/reference/yolo/v8/segment/val.md
@@ -0,0 +1,14 @@
+---
+description: Ensure segmentation quality on large datasets with SegmentationValidator. Review and visualize results with ease. Learn more at Ultralytics Docs.
+keywords: SegmentationValidator, YOLOv8, Ultralytics Docs, segmentation model, validation
+---
+
+## SegmentationValidator
+---
+### ::: ultralytics.yolo.v8.segment.val.SegmentationValidator
+
+
+## val
+---
+### ::: ultralytics.yolo.v8.segment.val.val
+
diff --git a/yolov8/docs/robots.txt b/yolov8/docs/robots.txt
new file mode 100644
index 0000000000000000000000000000000000000000..7d329b1db3f0b9383f111d23fc0c945b7e957017
--- /dev/null
+++ b/yolov8/docs/robots.txt
@@ -0,0 +1 @@
+User-agent: *
diff --git a/yolov8/docs/stylesheets/style.css b/yolov8/docs/stylesheets/style.css
new file mode 100644
index 0000000000000000000000000000000000000000..e5259c2be0f84df5688eda65d54c00c00e7c0987
--- /dev/null
+++ b/yolov8/docs/stylesheets/style.css
@@ -0,0 +1,39 @@
+/* Table format like GitHub ----------------------------------------------------------------------------------------- */
+th, td {
+ border: 1px solid var(--md-typeset-table-color);
+ border-spacing: 0;
+ border-bottom: none;
+ border-left: none;
+ border-top: none;
+}
+
+.md-typeset__table {
+ line-height: 1;
+}
+
+.md-typeset__table table:not([class]) {
+ font-size: .74rem;
+ border-right: none;
+}
+
+.md-typeset__table table:not([class]) td,
+.md-typeset__table table:not([class]) th {
+ padding: 9px;
+}
+
+/* light mode alternating table bg colors */
+.md-typeset__table tr:nth-child(2n) {
+ background-color: #f8f8f8;
+}
+
+/* dark mode alternating table bg colors */
+[data-md-color-scheme="slate"] .md-typeset__table tr:nth-child(2n) {
+ background-color: hsla(var(--md-hue),25%,25%,1)
+}
+/* Table format like GitHub ----------------------------------------------------------------------------------------- */
+
+/* Code block vertical scroll */
+div.highlight {
+ max-height: 20rem;
+ overflow-y: auto; /* for adding a scrollbar when needed */
+}
\ No newline at end of file
diff --git a/yolov8/docs/tasks/classify.md b/yolov8/docs/tasks/classify.md
new file mode 100644
index 0000000000000000000000000000000000000000..cfcf3872b72d73bd71f0c20b63294cf5842b8225
--- /dev/null
+++ b/yolov8/docs/tasks/classify.md
@@ -0,0 +1,181 @@
+---
+comments: true
+description: Check YOLO class label with only one class for the whole image, using image classification. Get strategies for training and validation models.
+keywords: YOLOv8n-cls, image classification, pretrained models
+---
+
+Image classification is the simplest of the three tasks and involves classifying an entire image into one of a set of
+predefined classes.
+
+
+
+The output of an image classifier is a single class label and a confidence score. Image
+classification is useful when you need to know only what class an image belongs to and don't need to know where objects
+of that class are located or what their exact shape is.
+
+!!! tip "Tip"
+
+ YOLOv8 Classify models use the `-cls` suffix, i.e. `yolov8n-cls.pt` and are pretrained on [ImageNet](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/ImageNet.yaml).
+
+## [Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models/v8)
+
+YOLOv8 pretrained Classify models are shown here. Detect, Segment and Pose models are pretrained on
+the [COCO](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco.yaml) dataset, while Classify
+models are pretrained on
+the [ImageNet](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/ImageNet.yaml) dataset.
+
+[Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) download automatically from the latest
+Ultralytics [release](https://github.com/ultralytics/assets/releases) on first use.
+
+| Model | size
(pixels) | acc
top1 | acc
top5 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) at 640 |
+|----------------------------------------------------------------------------------------------|-----------------------|------------------|------------------|--------------------------------|-------------------------------------|--------------------|--------------------------|
+| [YOLOv8n-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-cls.pt) | 224 | 66.6 | 87.0 | 12.9 | 0.31 | 2.7 | 4.3 |
+| [YOLOv8s-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-cls.pt) | 224 | 72.3 | 91.1 | 23.4 | 0.35 | 6.4 | 13.5 |
+| [YOLOv8m-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-cls.pt) | 224 | 76.4 | 93.2 | 85.4 | 0.62 | 17.0 | 42.7 |
+| [YOLOv8l-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-cls.pt) | 224 | 78.0 | 94.1 | 163.0 | 0.87 | 37.5 | 99.7 |
+| [YOLOv8x-cls](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-cls.pt) | 224 | 78.4 | 94.3 | 232.0 | 1.01 | 57.4 | 154.8 |
+
+- **acc** values are model accuracies on the [ImageNet](https://www.image-net.org/) dataset validation set.
+
Reproduce by `yolo val classify data=path/to/ImageNet device=0`
+- **Speed** averaged over ImageNet val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/)
+ instance.
+
Reproduce by `yolo val classify data=path/to/ImageNet batch=1 device=0|cpu`
+
+## Train
+
+Train YOLOv8n-cls on the MNIST160 dataset for 100 epochs at image size 64. For a full list of available arguments
+see the [Configuration](../usage/cfg.md) page.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-cls.yaml') # build a new model from YAML
+ model = YOLO('yolov8n-cls.pt') # load a pretrained model (recommended for training)
+ model = YOLO('yolov8n-cls.yaml').load('yolov8n-cls.pt') # build from YAML and transfer weights
+
+ # Train the model
+ model.train(data='mnist160', epochs=100, imgsz=64)
+ ```
+
+ === "CLI"
+
+ ```bash
+ # Build a new model from YAML and start training from scratch
+ yolo classify train data=mnist160 model=yolov8n-cls.yaml epochs=100 imgsz=64
+
+ # Start training from a pretrained *.pt model
+ yolo classify train data=mnist160 model=yolov8n-cls.pt epochs=100 imgsz=64
+
+ # Build a new model from YAML, transfer pretrained weights to it and start training
+ yolo classify train data=mnist160 model=yolov8n-cls.yaml pretrained=yolov8n-cls.pt epochs=100 imgsz=64
+ ```
+
+### Dataset format
+
+YOLO classification dataset format can be found in detail in the [Dataset Guide](../datasets/classify/index.md).
+
+## Val
+
+Validate trained YOLOv8n-cls model accuracy on the MNIST160 dataset. No argument need to passed as the `model` retains
+it's training `data` and arguments as model attributes.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-cls.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Validate the model
+ metrics = model.val() # no arguments needed, dataset and settings remembered
+ metrics.top1 # top1 accuracy
+ metrics.top5 # top5 accuracy
+ ```
+ === "CLI"
+
+ ```bash
+ yolo classify val model=yolov8n-cls.pt # val official model
+ yolo classify val model=path/to/best.pt # val custom model
+ ```
+
+## Predict
+
+Use a trained YOLOv8n-cls model to run predictions on images.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-cls.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Predict with the model
+ results = model('https://ultralytics.com/images/bus.jpg') # predict on an image
+ ```
+ === "CLI"
+
+ ```bash
+ yolo classify predict model=yolov8n-cls.pt source='https://ultralytics.com/images/bus.jpg' # predict with official model
+ yolo classify predict model=path/to/best.pt source='https://ultralytics.com/images/bus.jpg' # predict with custom model
+ ```
+
+See full `predict` mode details in the [Predict](https://docs.ultralytics.com/modes/predict/) page.
+
+## Export
+
+Export a YOLOv8n-cls model to a different format like ONNX, CoreML, etc.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-cls.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom trained
+
+ # Export the model
+ model.export(format='onnx')
+ ```
+ === "CLI"
+
+ ```bash
+ yolo export model=yolov8n-cls.pt format=onnx # export official model
+ yolo export model=path/to/best.pt format=onnx # export custom trained model
+ ```
+
+Available YOLOv8-cls export formats are in the table below. You can predict or validate directly on exported models,
+i.e. `yolo predict model=yolov8n-cls.onnx`. Usage examples are shown for your model after export completes.
+
+| Format | `format` Argument | Model | Metadata | Arguments |
+|--------------------------------------------------------------------|-------------------|-------------------------------|----------|-----------------------------------------------------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n-cls.pt` | ✅ | - |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n-cls.torchscript` | ✅ | `imgsz`, `optimize` |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n-cls.onnx` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `opset` |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n-cls_openvino_model/` | ✅ | `imgsz`, `half` |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n-cls.engine` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `workspace` |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n-cls.mlmodel` | ✅ | `imgsz`, `half`, `int8`, `nms` |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n-cls_saved_model/` | ✅ | `imgsz`, `keras` |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n-cls.pb` | ❌ | `imgsz` |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n-cls.tflite` | ✅ | `imgsz`, `half`, `int8` |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n-cls_edgetpu.tflite` | ✅ | `imgsz` |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n-cls_web_model/` | ✅ | `imgsz` |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n-cls_paddle_model/` | ✅ | `imgsz` |
+| [NCNN](https://github.com/Tencent/ncnn) | `ncnn` | `yolov8n-cls_ncnn_model/` | ✅ | `imgsz`, `half` |
+
+See full `export` details in the [Export](https://docs.ultralytics.com/modes/export/) page.
\ No newline at end of file
diff --git a/yolov8/docs/tasks/detect.md b/yolov8/docs/tasks/detect.md
new file mode 100644
index 0000000000000000000000000000000000000000..18d2a6f3923865a2f236481b293db7c080ddeb8a
--- /dev/null
+++ b/yolov8/docs/tasks/detect.md
@@ -0,0 +1,172 @@
+---
+comments: true
+description: Learn how to use YOLOv8, an object detection model pre-trained with COCO and about the different YOLOv8 models and how to train and export them.
+keywords: object detection, YOLOv8 Detect models, COCO dataset, models, train, predict, export
+---
+
+Object detection is a task that involves identifying the location and class of objects in an image or video stream.
+
+
+
+The output of an object detector is a set of bounding boxes that enclose the objects in the image, along with class labels and confidence scores for each box. Object detection is a good choice when you need to identify objects of interest in a scene, but don't need to know exactly where the object is or its exact shape.
+
+!!! tip "Tip"
+
+ YOLOv8 Detect models are the default YOLOv8 models, i.e. `yolov8n.pt` and are pretrained on [COCO](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco.yaml).
+
+## [Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models/v8)
+
+YOLOv8 pretrained Detect models are shown here. Detect, Segment and Pose models are pretrained on the [COCO](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco.yaml) dataset, while Classify models are pretrained on the [ImageNet](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/ImageNet.yaml) dataset.
+
+[Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) download automatically from the latest Ultralytics [release](https://github.com/ultralytics/assets/releases) on first use.
+
+| Model | size
(pixels) | mAPval
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+|--------------------------------------------------------------------------------------|-----------------------|----------------------|--------------------------------|-------------------------------------|--------------------|-------------------|
+| [YOLOv8n](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n.pt) | 640 | 37.3 | 80.4 | 0.99 | 3.2 | 8.7 |
+| [YOLOv8s](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s.pt) | 640 | 44.9 | 128.4 | 1.20 | 11.2 | 28.6 |
+| [YOLOv8m](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m.pt) | 640 | 50.2 | 234.7 | 1.83 | 25.9 | 78.9 |
+| [YOLOv8l](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l.pt) | 640 | 52.9 | 375.2 | 2.39 | 43.7 | 165.2 |
+| [YOLOv8x](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x.pt) | 640 | 53.9 | 479.1 | 3.53 | 68.2 | 257.8 |
+
+- **mAPval** values are for single-model single-scale on [COCO val2017](http://cocodataset.org) dataset.
+
Reproduce by `yolo val detect data=coco.yaml device=0`
+- **Speed** averaged over COCO val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/)
+ instance.
+
Reproduce by `yolo val detect data=coco128.yaml batch=1 device=0|cpu`
+
+## Train
+
+Train YOLOv8n on the COCO128 dataset for 100 epochs at image size 640. For a full list of available arguments see the [Configuration](../usage/cfg.md) page.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.yaml') # build a new model from YAML
+ model = YOLO('yolov8n.pt') # load a pretrained model (recommended for training)
+ model = YOLO('yolov8n.yaml').load('yolov8n.pt') # build from YAML and transfer weights
+
+ # Train the model
+ model.train(data='coco128.yaml', epochs=100, imgsz=640)
+ ```
+ === "CLI"
+
+ ```bash
+ # Build a new model from YAML and start training from scratch
+ yolo detect train data=coco128.yaml model=yolov8n.yaml epochs=100 imgsz=640
+
+ # Start training from a pretrained *.pt model
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=100 imgsz=640
+
+ # Build a new model from YAML, transfer pretrained weights to it and start training
+ yolo detect train data=coco128.yaml model=yolov8n.yaml pretrained=yolov8n.pt epochs=100 imgsz=640
+ ```
+
+### Dataset format
+
+YOLO detection dataset format can be found in detail in the [Dataset Guide](../datasets/detect/index.md). To convert your existing dataset from other formats( like COCO etc.) to YOLO format, please use [json2yolo tool](https://github.com/ultralytics/JSON2YOLO) by Ultralytics.
+
+## Val
+
+Validate trained YOLOv8n model accuracy on the COCO128 dataset. No argument need to passed as the `model` retains it's training `data` and arguments as model attributes.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Validate the model
+ metrics = model.val() # no arguments needed, dataset and settings remembered
+ metrics.box.map # map50-95
+ metrics.box.map50 # map50
+ metrics.box.map75 # map75
+ metrics.box.maps # a list contains map50-95 of each category
+ ```
+ === "CLI"
+
+ ```bash
+ yolo detect val model=yolov8n.pt # val official model
+ yolo detect val model=path/to/best.pt # val custom model
+ ```
+
+## Predict
+
+Use a trained YOLOv8n model to run predictions on images.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Predict with the model
+ results = model('https://ultralytics.com/images/bus.jpg') # predict on an image
+ ```
+ === "CLI"
+
+ ```bash
+ yolo detect predict model=yolov8n.pt source='https://ultralytics.com/images/bus.jpg' # predict with official model
+ yolo detect predict model=path/to/best.pt source='https://ultralytics.com/images/bus.jpg' # predict with custom model
+ ```
+
+See full `predict` mode details in the [Predict](https://docs.ultralytics.com/modes/predict/) page.
+
+## Export
+
+Export a YOLOv8n model to a different format like ONNX, CoreML, etc.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom trained
+
+ # Export the model
+ model.export(format='onnx')
+ ```
+ === "CLI"
+
+ ```bash
+ yolo export model=yolov8n.pt format=onnx # export official model
+ yolo export model=path/to/best.pt format=onnx # export custom trained model
+ ```
+
+Available YOLOv8 export formats are in the table below. You can predict or validate directly on exported models, i.e. `yolo predict model=yolov8n.onnx`. Usage examples are shown for your model after export completes.
+
+| Format | `format` Argument | Model | Metadata | Arguments |
+|--------------------------------------------------------------------|-------------------|---------------------------|----------|-----------------------------------------------------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n.pt` | ✅ | - |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n.torchscript` | ✅ | `imgsz`, `optimize` |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n.onnx` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `opset` |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n_openvino_model/` | ✅ | `imgsz`, `half` |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n.engine` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `workspace` |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n.mlmodel` | ✅ | `imgsz`, `half`, `int8`, `nms` |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n_saved_model/` | ✅ | `imgsz`, `keras` |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n.pb` | ❌ | `imgsz` |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n.tflite` | ✅ | `imgsz`, `half`, `int8` |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n_edgetpu.tflite` | ✅ | `imgsz` |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n_web_model/` | ✅ | `imgsz` |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n_paddle_model/` | ✅ | `imgsz` |
+| [NCNN](https://github.com/Tencent/ncnn) | `ncnn` | `yolov8n_ncnn_model/` | ✅ | `imgsz`, `half` |
+
+See full `export` details in the [Export](https://docs.ultralytics.com/modes/export/) page.
\ No newline at end of file
diff --git a/yolov8/docs/tasks/index.md b/yolov8/docs/tasks/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..23e384bd347bc3ac6387da9162c989f9447a19bf
--- /dev/null
+++ b/yolov8/docs/tasks/index.md
@@ -0,0 +1,51 @@
+---
+comments: true
+description: Learn how Ultralytics YOLOv8 AI framework supports detection, segmentation, classification, and pose/keypoint estimation tasks.
+keywords: YOLOv8, computer vision, detection, segmentation, classification, pose, keypoint detection, image segmentation, medical imaging
+---
+
+# Ultralytics YOLOv8 Tasks
+
+YOLOv8 is an AI framework that supports multiple computer vision **tasks**. The framework can be used to
+perform [detection](detect.md), [segmentation](segment.md), [classification](classify.md),
+and [pose](pose.md) estimation. Each of these tasks has a different objective and use case.
+
+
+
+
+## [Detection](detect.md)
+
+Detection is the primary task supported by YOLOv8. It involves detecting objects in an image or video frame and drawing
+bounding boxes around them. The detected objects are classified into different categories based on their features.
+YOLOv8 can detect multiple objects in a single image or video frame with high accuracy and speed.
+
+[Detection Examples](detect.md){ .md-button .md-button--primary}
+
+## [Segmentation](segment.md)
+
+Segmentation is a task that involves segmenting an image into different regions based on the content of the image. Each
+region is assigned a label based on its content. This task is useful in applications such as image segmentation and
+medical imaging. YOLOv8 uses a variant of the U-Net architecture to perform segmentation.
+
+[Segmentation Examples](segment.md){ .md-button .md-button--primary}
+
+## [Classification](classify.md)
+
+Classification is a task that involves classifying an image into different categories. YOLOv8 can be used to classify
+images based on their content. It uses a variant of the EfficientNet architecture to perform classification.
+
+[Classification Examples](classify.md){ .md-button .md-button--primary}
+
+## [Pose](pose.md)
+
+Pose/keypoint detection is a task that involves detecting specific points in an image or video frame. These points are
+referred to as keypoints and are used to track movement or pose estimation. YOLOv8 can detect keypoints in an image or
+video frame with high accuracy and speed.
+
+[Pose Examples](pose.md){ .md-button .md-button--primary}
+
+## Conclusion
+
+YOLOv8 supports multiple tasks, including detection, segmentation, classification, and keypoints detection. Each of
+these tasks has different objectives and use cases. By understanding the differences between these tasks, you can choose
+the appropriate task for your computer vision application.
\ No newline at end of file
diff --git a/yolov8/docs/tasks/pose.md b/yolov8/docs/tasks/pose.md
new file mode 100644
index 0000000000000000000000000000000000000000..6ed68aee50da18acb93d8781e277d16a8dbaa7b8
--- /dev/null
+++ b/yolov8/docs/tasks/pose.md
@@ -0,0 +1,186 @@
+---
+comments: true
+description: Learn how to use YOLOv8 pose estimation models to identify the position of keypoints on objects in an image, and how to train, validate, predict, and export these models for use with various formats such as ONNX or CoreML.
+keywords: YOLOv8, Pose Models, Keypoint Detection, COCO dataset, COCO val2017, Amazon EC2 P4d, PyTorch
+---
+
+Pose estimation is a task that involves identifying the location of specific points in an image, usually referred
+to as keypoints. The keypoints can represent various parts of the object such as joints, landmarks, or other distinctive
+features. The locations of the keypoints are usually represented as a set of 2D `[x, y]` or 3D `[x, y, visible]`
+coordinates.
+
+
+
+The output of a pose estimation model is a set of points that represent the keypoints on an object in the image, usually
+along with the confidence scores for each point. Pose estimation is a good choice when you need to identify specific
+parts of an object in a scene, and their location in relation to each other.
+
+!!! tip "Tip"
+
+ YOLOv8 _pose_ models use the `-pose` suffix, i.e. `yolov8n-pose.pt`. These models are trained on the [COCO keypoints](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco-pose.yaml) dataset and are suitable for a variety of pose estimation tasks.
+
+## [Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models/v8)
+
+YOLOv8 pretrained Pose models are shown here. Detect, Segment and Pose models are pretrained on
+the [COCO](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco.yaml) dataset, while Classify
+models are pretrained on
+the [ImageNet](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/ImageNet.yaml) dataset.
+
+[Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) download automatically from the latest
+Ultralytics [release](https://github.com/ultralytics/assets/releases) on first use.
+
+| Model | size
(pixels) | mAPpose
50-95 | mAPpose
50 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+|------------------------------------------------------------------------------------------------------|-----------------------|-----------------------|--------------------|--------------------------------|-------------------------------------|--------------------|-------------------|
+| [YOLOv8n-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-pose.pt) | 640 | 50.4 | 80.1 | 131.8 | 1.18 | 3.3 | 9.2 |
+| [YOLOv8s-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-pose.pt) | 640 | 60.0 | 86.2 | 233.2 | 1.42 | 11.6 | 30.2 |
+| [YOLOv8m-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-pose.pt) | 640 | 65.0 | 88.8 | 456.3 | 2.00 | 26.4 | 81.0 |
+| [YOLOv8l-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-pose.pt) | 640 | 67.6 | 90.0 | 784.5 | 2.59 | 44.4 | 168.6 |
+| [YOLOv8x-pose](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose.pt) | 640 | 69.2 | 90.2 | 1607.1 | 3.73 | 69.4 | 263.2 |
+| [YOLOv8x-pose-p6](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-pose-p6.pt) | 1280 | 71.6 | 91.2 | 4088.7 | 10.04 | 99.1 | 1066.4 |
+
+- **mAPval** values are for single-model single-scale on [COCO Keypoints val2017](http://cocodataset.org)
+ dataset.
+
Reproduce by `yolo val pose data=coco-pose.yaml device=0`
+- **Speed** averaged over COCO val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/)
+ instance.
+
Reproduce by `yolo val pose data=coco8-pose.yaml batch=1 device=0|cpu`
+
+## Train
+
+Train a YOLOv8-pose model on the COCO128-pose dataset.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-pose.yaml') # build a new model from YAML
+ model = YOLO('yolov8n-pose.pt') # load a pretrained model (recommended for training)
+ model = YOLO('yolov8n-pose.yaml').load('yolov8n-pose.pt') # build from YAML and transfer weights
+
+ # Train the model
+ model.train(data='coco8-pose.yaml', epochs=100, imgsz=640)
+ ```
+ === "CLI"
+
+ ```bash
+ # Build a new model from YAML and start training from scratch
+ yolo pose train data=coco8-pose.yaml model=yolov8n-pose.yaml epochs=100 imgsz=640
+
+ # Start training from a pretrained *.pt model
+ yolo pose train data=coco8-pose.yaml model=yolov8n-pose.pt epochs=100 imgsz=640
+
+ # Build a new model from YAML, transfer pretrained weights to it and start training
+ yolo pose train data=coco8-pose.yaml model=yolov8n-pose.yaml pretrained=yolov8n-pose.pt epochs=100 imgsz=640
+ ```
+
+### Dataset format
+
+YOLO pose dataset format can be found in detail in the [Dataset Guide](../datasets/pose/index.md). To convert your existing dataset from other formats( like COCO etc.) to YOLO format, please use [json2yolo tool](https://github.com/ultralytics/JSON2YOLO) by Ultralytics.
+
+## Val
+
+Validate trained YOLOv8n-pose model accuracy on the COCO128-pose dataset. No argument need to passed as the `model`
+retains it's
+training `data` and arguments as model attributes.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-pose.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Validate the model
+ metrics = model.val() # no arguments needed, dataset and settings remembered
+ metrics.box.map # map50-95
+ metrics.box.map50 # map50
+ metrics.box.map75 # map75
+ metrics.box.maps # a list contains map50-95 of each category
+ ```
+ === "CLI"
+
+ ```bash
+ yolo pose val model=yolov8n-pose.pt # val official model
+ yolo pose val model=path/to/best.pt # val custom model
+ ```
+
+## Predict
+
+Use a trained YOLOv8n-pose model to run predictions on images.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-pose.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Predict with the model
+ results = model('https://ultralytics.com/images/bus.jpg') # predict on an image
+ ```
+ === "CLI"
+
+ ```bash
+ yolo pose predict model=yolov8n-pose.pt source='https://ultralytics.com/images/bus.jpg' # predict with official model
+ yolo pose predict model=path/to/best.pt source='https://ultralytics.com/images/bus.jpg' # predict with custom model
+ ```
+
+See full `predict` mode details in the [Predict](https://docs.ultralytics.com/modes/predict/) page.
+
+## Export
+
+Export a YOLOv8n Pose model to a different format like ONNX, CoreML, etc.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-pose.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom trained
+
+ # Export the model
+ model.export(format='onnx')
+ ```
+ === "CLI"
+
+ ```bash
+ yolo export model=yolov8n-pose.pt format=onnx # export official model
+ yolo export model=path/to/best.pt format=onnx # export custom trained model
+ ```
+
+Available YOLOv8-pose export formats are in the table below. You can predict or validate directly on exported models,
+i.e. `yolo predict model=yolov8n-pose.onnx`. Usage examples are shown for your model after export completes.
+
+| Format | `format` Argument | Model | Metadata | Arguments |
+|--------------------------------------------------------------------|-------------------|--------------------------------|----------|-----------------------------------------------------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n-pose.pt` | ✅ | - |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n-pose.torchscript` | ✅ | `imgsz`, `optimize` |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n-pose.onnx` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `opset` |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n-pose_openvino_model/` | ✅ | `imgsz`, `half` |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n-pose.engine` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `workspace` |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n-pose.mlmodel` | ✅ | `imgsz`, `half`, `int8`, `nms` |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n-pose_saved_model/` | ✅ | `imgsz`, `keras` |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n-pose.pb` | ❌ | `imgsz` |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n-pose.tflite` | ✅ | `imgsz`, `half`, `int8` |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n-pose_edgetpu.tflite` | ✅ | `imgsz` |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n-pose_web_model/` | ✅ | `imgsz` |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n-pose_paddle_model/` | ✅ | `imgsz` |
+| [NCNN](https://github.com/Tencent/ncnn) | `ncnn` | `yolov8n-pose_ncnn_model/` | ✅ | `imgsz`, `half` |
+
+See full `export` details in the [Export](https://docs.ultralytics.com/modes/export/) page.
\ No newline at end of file
diff --git a/yolov8/docs/tasks/segment.md b/yolov8/docs/tasks/segment.md
new file mode 100644
index 0000000000000000000000000000000000000000..586bcfcd412756705301fdd14ab9f6f5efa360e0
--- /dev/null
+++ b/yolov8/docs/tasks/segment.md
@@ -0,0 +1,186 @@
+---
+comments: true
+description: Learn what Instance segmentation is. Get pretrained YOLOv8 segment models, and how to train and export them to segments masks. Check the preformance metrics!
+keywords: instance segmentation, YOLOv8, Ultralytics, pretrained models, train, predict, export, datasets
+---
+
+Instance segmentation goes a step further than object detection and involves identifying individual objects in an image
+and segmenting them from the rest of the image.
+
+
+
+The output of an instance segmentation model is a set of masks or
+contours that outline each object in the image, along with class labels and confidence scores for each object. Instance
+segmentation is useful when you need to know not only where objects are in an image, but also what their exact shape is.
+
+!!! tip "Tip"
+
+ YOLOv8 Segment models use the `-seg` suffix, i.e. `yolov8n-seg.pt` and are pretrained on [COCO](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco.yaml).
+
+## [Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models/v8)
+
+YOLOv8 pretrained Segment models are shown here. Detect, Segment and Pose models are pretrained on
+the [COCO](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/coco.yaml) dataset, while Classify
+models are pretrained on
+the [ImageNet](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/datasets/ImageNet.yaml) dataset.
+
+[Models](https://github.com/ultralytics/ultralytics/tree/main/ultralytics/models) download automatically from the latest
+Ultralytics [release](https://github.com/ultralytics/assets/releases) on first use.
+
+| Model | size
(pixels) | mAPbox
50-95 | mAPmask
50-95 | Speed
CPU ONNX
(ms) | Speed
A100 TensorRT
(ms) | params
(M) | FLOPs
(B) |
+|----------------------------------------------------------------------------------------------|-----------------------|----------------------|-----------------------|--------------------------------|-------------------------------------|--------------------|-------------------|
+| [YOLOv8n-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n-seg.pt) | 640 | 36.7 | 30.5 | 96.1 | 1.21 | 3.4 | 12.6 |
+| [YOLOv8s-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8s-seg.pt) | 640 | 44.6 | 36.8 | 155.7 | 1.47 | 11.8 | 42.6 |
+| [YOLOv8m-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8m-seg.pt) | 640 | 49.9 | 40.8 | 317.0 | 2.18 | 27.3 | 110.2 |
+| [YOLOv8l-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8l-seg.pt) | 640 | 52.3 | 42.6 | 572.4 | 2.79 | 46.0 | 220.5 |
+| [YOLOv8x-seg](https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8x-seg.pt) | 640 | 53.4 | 43.4 | 712.1 | 4.02 | 71.8 | 344.1 |
+
+- **mAPval** values are for single-model single-scale on [COCO val2017](http://cocodataset.org) dataset.
+
Reproduce by `yolo val segment data=coco.yaml device=0`
+- **Speed** averaged over COCO val images using an [Amazon EC2 P4d](https://aws.amazon.com/ec2/instance-types/p4/)
+ instance.
+
Reproduce by `yolo val segment data=coco128-seg.yaml batch=1 device=0|cpu`
+
+## Train
+
+Train YOLOv8n-seg on the COCO128-seg dataset for 100 epochs at image size 640. For a full list of available
+arguments see the [Configuration](../usage/cfg.md) page.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-seg.yaml') # build a new model from YAML
+ model = YOLO('yolov8n-seg.pt') # load a pretrained model (recommended for training)
+ model = YOLO('yolov8n-seg.yaml').load('yolov8n.pt') # build from YAML and transfer weights
+
+ # Train the model
+ model.train(data='coco128-seg.yaml', epochs=100, imgsz=640)
+ ```
+ === "CLI"
+
+ ```bash
+ # Build a new model from YAML and start training from scratch
+ yolo segment train data=coco128-seg.yaml model=yolov8n-seg.yaml epochs=100 imgsz=640
+
+ # Start training from a pretrained *.pt model
+ yolo segment train data=coco128-seg.yaml model=yolov8n-seg.pt epochs=100 imgsz=640
+
+ # Build a new model from YAML, transfer pretrained weights to it and start training
+ yolo segment train data=coco128-seg.yaml model=yolov8n-seg.yaml pretrained=yolov8n-seg.pt epochs=100 imgsz=640
+ ```
+
+### Dataset format
+
+YOLO segmentation dataset format can be found in detail in the [Dataset Guide](../datasets/segment/index.md). To convert your existing dataset from other formats( like COCO etc.) to YOLO format, please use [json2yolo tool](https://github.com/ultralytics/JSON2YOLO) by Ultralytics.
+
+## Val
+
+Validate trained YOLOv8n-seg model accuracy on the COCO128-seg dataset. No argument need to passed as the `model`
+retains it's training `data` and arguments as model attributes.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-seg.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Validate the model
+ metrics = model.val() # no arguments needed, dataset and settings remembered
+ metrics.box.map # map50-95(B)
+ metrics.box.map50 # map50(B)
+ metrics.box.map75 # map75(B)
+ metrics.box.maps # a list contains map50-95(B) of each category
+ metrics.seg.map # map50-95(M)
+ metrics.seg.map50 # map50(M)
+ metrics.seg.map75 # map75(M)
+ metrics.seg.maps # a list contains map50-95(M) of each category
+ ```
+ === "CLI"
+
+ ```bash
+ yolo segment val model=yolov8n-seg.pt # val official model
+ yolo segment val model=path/to/best.pt # val custom model
+ ```
+
+## Predict
+
+Use a trained YOLOv8n-seg model to run predictions on images.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-seg.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Predict with the model
+ results = model('https://ultralytics.com/images/bus.jpg') # predict on an image
+ ```
+ === "CLI"
+
+ ```bash
+ yolo segment predict model=yolov8n-seg.pt source='https://ultralytics.com/images/bus.jpg' # predict with official model
+ yolo segment predict model=path/to/best.pt source='https://ultralytics.com/images/bus.jpg' # predict with custom model
+ ```
+
+See full `predict` mode details in the [Predict](https://docs.ultralytics.com/modes/predict/) page.
+
+## Export
+
+Export a YOLOv8n-seg model to a different format like ONNX, CoreML, etc.
+
+!!! example ""
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n-seg.pt') # load an official model
+ model = YOLO('path/to/best.pt') # load a custom trained
+
+ # Export the model
+ model.export(format='onnx')
+ ```
+ === "CLI"
+
+ ```bash
+ yolo export model=yolov8n-seg.pt format=onnx # export official model
+ yolo export model=path/to/best.pt format=onnx # export custom trained model
+ ```
+
+Available YOLOv8-seg export formats are in the table below. You can predict or validate directly on exported models,
+i.e. `yolo predict model=yolov8n-seg.onnx`. Usage examples are shown for your model after export completes.
+
+| Format | `format` Argument | Model | Metadata | Arguments |
+|--------------------------------------------------------------------|-------------------|-------------------------------|----------|-----------------------------------------------------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n-seg.pt` | ✅ | - |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n-seg.torchscript` | ✅ | `imgsz`, `optimize` |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n-seg.onnx` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `opset` |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n-seg_openvino_model/` | ✅ | `imgsz`, `half` |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n-seg.engine` | ✅ | `imgsz`, `half`, `dynamic`, `simplify`, `workspace` |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n-seg.mlmodel` | ✅ | `imgsz`, `half`, `int8`, `nms` |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n-seg_saved_model/` | ✅ | `imgsz`, `keras` |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n-seg.pb` | ❌ | `imgsz` |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n-seg.tflite` | ✅ | `imgsz`, `half`, `int8` |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n-seg_edgetpu.tflite` | ✅ | `imgsz` |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n-seg_web_model/` | ✅ | `imgsz` |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n-seg_paddle_model/` | ✅ | `imgsz` |
+| [NCNN](https://github.com/Tencent/ncnn) | `ncnn` | `yolov8n-seg_ncnn_model/` | ✅ | `imgsz`, `half` |
+
+See full `export` details in the [Export](https://docs.ultralytics.com/modes/export/) page.
\ No newline at end of file
diff --git a/yolov8/docs/usage/callbacks.md b/yolov8/docs/usage/callbacks.md
new file mode 100644
index 0000000000000000000000000000000000000000..7968fafdd04b28117c4e850a9d536f84a13c6842
--- /dev/null
+++ b/yolov8/docs/usage/callbacks.md
@@ -0,0 +1,88 @@
+---
+comments: true
+description: Learn how to leverage callbacks in Ultralytics YOLO framework to perform custom tasks in trainer, validator, predictor and exporter modes.
+keywords: callbacks, Ultralytics framework, Trainer, Validator, Predictor, Exporter, train, val, export, predict, YOLO, Object Detection
+---
+
+## Callbacks
+
+Ultralytics framework supports callbacks as entry points in strategic stages of train, val, export, and predict modes.
+Each callback accepts a `Trainer`, `Validator`, or `Predictor` object depending on the operation type. All properties of
+these objects can be found in Reference section of the docs.
+
+## Examples
+
+### Returning additional information with Prediction
+
+In this example, we want to return the original frame with each result object. Here's how we can do that
+
+```python
+def on_predict_batch_end(predictor):
+ # Retrieve the batch data
+ _, im0s, _, _ = predictor.batch
+
+ # Ensure that im0s is a list
+ im0s = im0s if isinstance(im0s, list) else [im0s]
+
+ # Combine the prediction results with the corresponding frames
+ predictor.results = zip(predictor.results, im0s)
+
+# Create a YOLO model instance
+model = YOLO(f'yolov8n.pt')
+
+# Add the custom callback to the model
+model.add_callback("on_predict_batch_end", on_predict_batch_end)
+
+# Iterate through the results and frames
+for (result, frame) in model.track/predict():
+ pass
+```
+
+## All callbacks
+
+Here are all supported callbacks. See callbacks [source code](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/yolo/utils/callbacks/base.py) for additional details.
+
+### Trainer Callbacks
+
+| Callback | Description |
+|-----------------------------|---------------------------------------------------------|
+| `on_pretrain_routine_start` | Triggered at the beginning of pre-training routine |
+| `on_pretrain_routine_end` | Triggered at the end of pre-training routine |
+| `on_train_start` | Triggered when the training starts |
+| `on_train_epoch_start` | Triggered at the start of each training epoch |
+| `on_train_batch_start` | Triggered at the start of each training batch |
+| `optimizer_step` | Triggered during the optimizer step |
+| `on_before_zero_grad` | Triggered before gradients are zeroed |
+| `on_train_batch_end` | Triggered at the end of each training batch |
+| `on_train_epoch_end` | Triggered at the end of each training epoch |
+| `on_fit_epoch_end` | Triggered at the end of each fit epoch |
+| `on_model_save` | Triggered when the model is saved |
+| `on_train_end` | Triggered when the training process ends |
+| `on_params_update` | Triggered when model parameters are updated |
+| `teardown` | Triggered when the training process is being cleaned up |
+
+### Validator Callbacks
+
+| Callback | Description |
+|----------------------|-------------------------------------------------|
+| `on_val_start` | Triggered when the validation starts |
+| `on_val_batch_start` | Triggered at the start of each validation batch |
+| `on_val_batch_end` | Triggered at the end of each validation batch |
+| `on_val_end` | Triggered when the validation ends |
+
+### Predictor Callbacks
+
+| Callback | Description |
+|------------------------------|---------------------------------------------------|
+| `on_predict_start` | Triggered when the prediction process starts |
+| `on_predict_batch_start` | Triggered at the start of each prediction batch |
+| `on_predict_postprocess_end` | Triggered at the end of prediction postprocessing |
+| `on_predict_batch_end` | Triggered at the end of each prediction batch |
+| `on_predict_end` | Triggered when the prediction process ends |
+
+### Exporter Callbacks
+
+| Callback | Description |
+|-------------------|------------------------------------------|
+| `on_export_start` | Triggered when the export process starts |
+| `on_export_end` | Triggered when the export process ends |
\ No newline at end of file
diff --git a/yolov8/docs/usage/cfg.md b/yolov8/docs/usage/cfg.md
new file mode 100644
index 0000000000000000000000000000000000000000..b027da310e66bf9680bde4c6a45d41a836fb5b73
--- /dev/null
+++ b/yolov8/docs/usage/cfg.md
@@ -0,0 +1,254 @@
+---
+comments: true
+description: Learn about YOLO settings and modes for different tasks like detection, segmentation etc. Train and predict with custom argparse commands.
+keywords: YOLO settings, hyperparameters, YOLOv8, Ultralytics, YOLO guide, YOLO commands, YOLO tasks, YOLO modes, YOLO training, YOLO detect, YOLO segment, YOLO classify, YOLO pose, YOLO train, YOLO val, YOLO predict, YOLO export, YOLO track, YOLO benchmark
+---
+
+YOLO settings and hyperparameters play a critical role in the model's performance, speed, and accuracy. These settings
+and hyperparameters can affect the model's behavior at various stages of the model development process, including
+training, validation, and prediction.
+
+YOLOv8 'yolo' CLI commands use the following syntax:
+
+!!! example ""
+
+ === "CLI"
+
+ ```bash
+ yolo TASK MODE ARGS
+ ```
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a YOLOv8 model from a pre-trained weights file
+ model = YOLO('yolov8n.pt')
+
+ # Run MODE mode using the custom arguments ARGS (guess TASK)
+ model.MODE(ARGS)
+ ```
+
+Where:
+
+- `TASK` (optional) is one of `[detect, segment, classify, pose]`. If it is not passed explicitly YOLOv8 will try to
+ guess
+ the `TASK` from the model type.
+- `MODE` (required) is one of `[train, val, predict, export, track, benchmark]`
+- `ARGS` (optional) are any number of custom `arg=value` pairs like `imgsz=320` that override defaults.
+ For a full list of available `ARGS` see the [Configuration](cfg.md) page and `defaults.yaml`
+ GitHub [source](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/yolo/cfg/default.yaml).
+
+#### Tasks
+
+YOLO models can be used for a variety of tasks, including detection, segmentation, classification and pose. These tasks
+differ in the type of output they produce and the specific problem they are designed to solve.
+
+**Detect**: For identifying and localizing objects or regions of interest in an image or video.
+**Segment**: For dividing an image or video into regions or pixels that correspond to different objects or classes.
+**Classify**: For predicting the class label of an input image.
+**Pose**: For identifying objects and estimating their keypoints in an image or video.
+
+| Key | Value | Description |
+|--------|------------|-------------------------------------------------|
+| `task` | `'detect'` | YOLO task, i.e. detect, segment, classify, pose |
+
+[Tasks Guide](../tasks/index.md){ .md-button .md-button--primary}
+
+#### Modes
+
+YOLO models can be used in different modes depending on the specific problem you are trying to solve. These modes
+include:
+
+**Train**: For training a YOLOv8 model on a custom dataset.
+**Val**: For validating a YOLOv8 model after it has been trained.
+**Predict**: For making predictions using a trained YOLOv8 model on new images or videos.
+**Export**: For exporting a YOLOv8 model to a format that can be used for deployment.
+**Track**: For tracking objects in real-time using a YOLOv8 model.
+**Benchmark**: For benchmarking YOLOv8 exports (ONNX, TensorRT, etc.) speed and accuracy.
+
+| Key | Value | Description |
+|--------|-----------|---------------------------------------------------------------|
+| `mode` | `'train'` | YOLO mode, i.e. train, val, predict, export, track, benchmark |
+
+[Modes Guide](../modes/index.md){ .md-button .md-button--primary}
+
+## Train
+
+The training settings for YOLO models encompass various hyperparameters and configurations used during the training process. These settings influence the model's performance, speed, and accuracy. Key training settings include batch size, learning rate, momentum, and weight decay. Additionally, the choice of optimizer, loss function, and training dataset composition can impact the training process. Careful tuning and experimentation with these settings are crucial for optimizing performance.
+
+| Key | Value | Description |
+|-------------------|----------|-----------------------------------------------------------------------------------|
+| `model` | `None` | path to model file, i.e. yolov8n.pt, yolov8n.yaml |
+| `data` | `None` | path to data file, i.e. coco128.yaml |
+| `epochs` | `100` | number of epochs to train for |
+| `patience` | `50` | epochs to wait for no observable improvement for early stopping of training |
+| `batch` | `16` | number of images per batch (-1 for AutoBatch) |
+| `imgsz` | `640` | size of input images as integer or w,h |
+| `save` | `True` | save train checkpoints and predict results |
+| `save_period` | `-1` | Save checkpoint every x epochs (disabled if < 1) |
+| `cache` | `False` | True/ram, disk or False. Use cache for data loading |
+| `device` | `None` | device to run on, i.e. cuda device=0 or device=0,1,2,3 or device=cpu |
+| `workers` | `8` | number of worker threads for data loading (per RANK if DDP) |
+| `project` | `None` | project name |
+| `name` | `None` | experiment name |
+| `exist_ok` | `False` | whether to overwrite existing experiment |
+| `pretrained` | `False` | whether to use a pretrained model |
+| `optimizer` | `'auto'` | optimizer to use, choices=[SGD, Adam, Adamax, AdamW, NAdam, RAdam, RMSProp, auto] |
+| `verbose` | `False` | whether to print verbose output |
+| `seed` | `0` | random seed for reproducibility |
+| `deterministic` | `True` | whether to enable deterministic mode |
+| `single_cls` | `False` | train multi-class data as single-class |
+| `rect` | `False` | rectangular training with each batch collated for minimum padding |
+| `cos_lr` | `False` | use cosine learning rate scheduler |
+| `close_mosaic` | `0` | (int) disable mosaic augmentation for final epochs |
+| `resume` | `False` | resume training from last checkpoint |
+| `amp` | `True` | Automatic Mixed Precision (AMP) training, choices=[True, False] |
+| `fraction` | `1.0` | dataset fraction to train on (default is 1.0, all images in train set) |
+| `profile` | `False` | profile ONNX and TensorRT speeds during training for loggers |
+| `lr0` | `0.01` | initial learning rate (i.e. SGD=1E-2, Adam=1E-3) |
+| `lrf` | `0.01` | final learning rate (lr0 * lrf) |
+| `momentum` | `0.937` | SGD momentum/Adam beta1 |
+| `weight_decay` | `0.0005` | optimizer weight decay 5e-4 |
+| `warmup_epochs` | `3.0` | warmup epochs (fractions ok) |
+| `warmup_momentum` | `0.8` | warmup initial momentum |
+| `warmup_bias_lr` | `0.1` | warmup initial bias lr |
+| `box` | `7.5` | box loss gain |
+| `cls` | `0.5` | cls loss gain (scale with pixels) |
+| `dfl` | `1.5` | dfl loss gain |
+| `pose` | `12.0` | pose loss gain (pose-only) |
+| `kobj` | `2.0` | keypoint obj loss gain (pose-only) |
+| `label_smoothing` | `0.0` | label smoothing (fraction) |
+| `nbs` | `64` | nominal batch size |
+| `overlap_mask` | `True` | masks should overlap during training (segment train only) |
+| `mask_ratio` | `4` | mask downsample ratio (segment train only) |
+| `dropout` | `0.0` | use dropout regularization (classify train only) |
+| `val` | `True` | validate/test during training |
+
+[Train Guide](../modes/train.md){ .md-button .md-button--primary}
+
+## Predict
+
+The prediction settings for YOLO models encompass a range of hyperparameters and configurations that influence the model's performance, speed, and accuracy during inference on new data. Careful tuning and experimentation with these settings are essential to achieve optimal performance for a specific task. Key settings include the confidence threshold, Non-Maximum Suppression (NMS) threshold, and the number of classes considered. Additional factors affecting the prediction process are input data size and format, the presence of supplementary features such as masks or multiple labels per box, and the particular task the model is employed for.
+
+| Key | Value | Description |
+|----------------|------------------------|--------------------------------------------------------------------------------|
+| `source` | `'ultralytics/assets'` | source directory for images or videos |
+| `conf` | `0.25` | object confidence threshold for detection |
+| `iou` | `0.7` | intersection over union (IoU) threshold for NMS |
+| `half` | `False` | use half precision (FP16) |
+| `device` | `None` | device to run on, i.e. cuda device=0/1/2/3 or device=cpu |
+| `show` | `False` | show results if possible |
+| `save` | `False` | save images with results |
+| `save_txt` | `False` | save results as .txt file |
+| `save_conf` | `False` | save results with confidence scores |
+| `save_crop` | `False` | save cropped images with results |
+| `show_labels` | `True` | show object labels in plots |
+| `show_conf` | `True` | show object confidence scores in plots |
+| `max_det` | `300` | maximum number of detections per image |
+| `vid_stride` | `False` | video frame-rate stride |
+| `line_width` | `None` | The line width of the bounding boxes. If None, it is scaled to the image size. |
+| `visualize` | `False` | visualize model features |
+| `augment` | `False` | apply image augmentation to prediction sources |
+| `agnostic_nms` | `False` | class-agnostic NMS |
+| `retina_masks` | `False` | use high-resolution segmentation masks |
+| `classes` | `None` | filter results by class, i.e. class=0, or class=[0,2,3] |
+| `boxes` | `True` | Show boxes in segmentation predictions |
+
+[Predict Guide](../modes/predict.md){ .md-button .md-button--primary}
+
+## Val
+
+The val (validation) settings for YOLO models involve various hyperparameters and configurations used to evaluate the model's performance on a validation dataset. These settings influence the model's performance, speed, and accuracy. Common YOLO validation settings include batch size, validation frequency during training, and performance evaluation metrics. Other factors affecting the validation process include the validation dataset's size and composition, as well as the specific task the model is employed for. Careful tuning and experimentation with these settings are crucial to ensure optimal performance on the validation dataset and detect and prevent overfitting.
+
+| Key | Value | Description |
+|---------------|---------|--------------------------------------------------------------------|
+| `save_json` | `False` | save results to JSON file |
+| `save_hybrid` | `False` | save hybrid version of labels (labels + additional predictions) |
+| `conf` | `0.001` | object confidence threshold for detection |
+| `iou` | `0.6` | intersection over union (IoU) threshold for NMS |
+| `max_det` | `300` | maximum number of detections per image |
+| `half` | `True` | use half precision (FP16) |
+| `device` | `None` | device to run on, i.e. cuda device=0/1/2/3 or device=cpu |
+| `dnn` | `False` | use OpenCV DNN for ONNX inference |
+| `plots` | `False` | show plots during training |
+| `rect` | `False` | rectangular val with each batch collated for minimum padding |
+| `split` | `val` | dataset split to use for validation, i.e. 'val', 'test' or 'train' |
+
+[Val Guide](../modes/val.md){ .md-button .md-button--primary}
+
+## Export
+
+Export settings for YOLO models encompass configurations and options related to saving or exporting the model for use in different environments or platforms. These settings can impact the model's performance, size, and compatibility with various systems. Key export settings include the exported model file format (e.g., ONNX, TensorFlow SavedModel), the target device (e.g., CPU, GPU), and additional features such as masks or multiple labels per box. The export process may also be affected by the model's specific task and the requirements or constraints of the destination environment or platform. It is crucial to thoughtfully configure these settings to ensure the exported model is optimized for the intended use case and functions effectively in the target environment.
+
+| Key | Value | Description |
+|-------------|-----------------|------------------------------------------------------|
+| `format` | `'torchscript'` | format to export to |
+| `imgsz` | `640` | image size as scalar or (h, w) list, i.e. (640, 480) |
+| `keras` | `False` | use Keras for TF SavedModel export |
+| `optimize` | `False` | TorchScript: optimize for mobile |
+| `half` | `False` | FP16 quantization |
+| `int8` | `False` | INT8 quantization |
+| `dynamic` | `False` | ONNX/TF/TensorRT: dynamic axes |
+| `simplify` | `False` | ONNX: simplify model |
+| `opset` | `None` | ONNX: opset version (optional, defaults to latest) |
+| `workspace` | `4` | TensorRT: workspace size (GB) |
+| `nms` | `False` | CoreML: add NMS |
+
+[Export Guide](../modes/export.md){ .md-button .md-button--primary}
+
+## Augmentation
+
+Augmentation settings for YOLO models refer to the various transformations and modifications
+applied to the training data to increase the diversity and size of the dataset. These settings can affect the model's
+performance, speed, and accuracy. Some common YOLO augmentation settings include the type and intensity of the
+transformations applied (e.g. random flips, rotations, cropping, color changes), the probability with which each
+transformation is applied, and the presence of additional features such as masks or multiple labels per box. Other
+factors that may affect the augmentation process include the size and composition of the original dataset and the
+specific task the model is being used for. It is important to carefully tune and experiment with these settings to
+ensure that the augmented dataset is diverse and representative enough to train a high-performing model.
+
+| Key | Value | Description |
+|---------------|-------|-------------------------------------------------|
+| `hsv_h` | 0.015 | image HSV-Hue augmentation (fraction) |
+| `hsv_s` | 0.7 | image HSV-Saturation augmentation (fraction) |
+| `hsv_v` | 0.4 | image HSV-Value augmentation (fraction) |
+| `degrees` | 0.0 | image rotation (+/- deg) |
+| `translate` | 0.1 | image translation (+/- fraction) |
+| `scale` | 0.5 | image scale (+/- gain) |
+| `shear` | 0.0 | image shear (+/- deg) |
+| `perspective` | 0.0 | image perspective (+/- fraction), range 0-0.001 |
+| `flipud` | 0.0 | image flip up-down (probability) |
+| `fliplr` | 0.5 | image flip left-right (probability) |
+| `mosaic` | 1.0 | image mosaic (probability) |
+| `mixup` | 0.0 | image mixup (probability) |
+| `copy_paste` | 0.0 | segment copy-paste (probability) |
+
+## Logging, checkpoints, plotting and file management
+
+Logging, checkpoints, plotting, and file management are important considerations when training a YOLO model.
+
+- Logging: It is often helpful to log various metrics and statistics during training to track the model's progress and
+ diagnose any issues that may arise. This can be done using a logging library such as TensorBoard or by writing log
+ messages to a file.
+- Checkpoints: It is a good practice to save checkpoints of the model at regular intervals during training. This allows
+ you to resume training from a previous point if the training process is interrupted or if you want to experiment with
+ different training configurations.
+- Plotting: Visualizing the model's performance and training progress can be helpful for understanding how the model is
+ behaving and identifying potential issues. This can be done using a plotting library such as matplotlib or by
+ generating plots using a logging library such as TensorBoard.
+- File management: Managing the various files generated during the training process, such as model checkpoints, log
+ files, and plots, can be challenging. It is important to have a clear and organized file structure to keep track of
+ these files and make it easy to access and analyze them as needed.
+
+Effective logging, checkpointing, plotting, and file management can help you keep track of the model's progress and make
+it easier to debug and optimize the training process.
+
+| Key | Value | Description |
+|------------|----------|------------------------------------------------------------------------------------------------|
+| `project` | `'runs'` | project name |
+| `name` | `'exp'` | experiment name. `exp` gets automatically incremented if not specified, i.e, `exp`, `exp2` ... |
+| `exist_ok` | `False` | whether to overwrite existing experiment |
+| `plots` | `False` | save plots during train/val |
+| `save` | `False` | save train checkpoints and predict results |
\ No newline at end of file
diff --git a/yolov8/docs/usage/cli.md b/yolov8/docs/usage/cli.md
new file mode 100644
index 0000000000000000000000000000000000000000..21879d7e8510ff66054851c18ad1af44376bc3f2
--- /dev/null
+++ b/yolov8/docs/usage/cli.md
@@ -0,0 +1,227 @@
+---
+comments: true
+description: Learn how to use YOLOv8 from the Command Line Interface (CLI) through simple, single-line commands with `yolo` without Python code.
+keywords: YOLO, CLI, command line interface, detect, segment, classify, train, validate, predict, export, Ultralytics Docs
+---
+
+# Command Line Interface Usage
+
+The YOLO command line interface (CLI) allows for simple single-line commands without the need for a Python environment.
+CLI requires no customization or Python code. You can simply run all tasks from the terminal with the `yolo` command.
+
+!!! example
+
+ === "Syntax"
+
+ Ultralytics `yolo` commands use the following syntax:
+ ```bash
+ yolo TASK MODE ARGS
+
+ Where TASK (optional) is one of [detect, segment, classify]
+ MODE (required) is one of [train, val, predict, export, track]
+ ARGS (optional) are any number of custom 'arg=value' pairs like 'imgsz=320' that override defaults.
+ ```
+ See all ARGS in the full [Configuration Guide](./cfg.md) or with `yolo cfg`
+
+ === "Train"
+
+ Train a detection model for 10 epochs with an initial learning_rate of 0.01
+ ```bash
+ yolo train data=coco128.yaml model=yolov8n.pt epochs=10 lr0=0.01
+ ```
+
+ === "Predict"
+
+ Predict a YouTube video using a pretrained segmentation model at image size 320:
+ ```bash
+ yolo predict model=yolov8n-seg.pt source='https://youtu.be/Zgi9g1ksQHc' imgsz=320
+ ```
+
+ === "Val"
+
+ Val a pretrained detection model at batch-size 1 and image size 640:
+ ```bash
+ yolo val model=yolov8n.pt data=coco128.yaml batch=1 imgsz=640
+ ```
+
+ === "Export"
+
+ Export a YOLOv8n classification model to ONNX format at image size 224 by 128 (no TASK required)
+ ```bash
+ yolo export model=yolov8n-cls.pt format=onnx imgsz=224,128
+ ```
+
+ === "Special"
+
+ Run special commands to see version, view settings, run checks and more:
+ ```bash
+ yolo help
+ yolo checks
+ yolo version
+ yolo settings
+ yolo copy-cfg
+ yolo cfg
+ ```
+
+Where:
+
+- `TASK` (optional) is one of `[detect, segment, classify]`. If it is not passed explicitly YOLOv8 will try to guess
+ the `TASK` from the model type.
+- `MODE` (required) is one of `[train, val, predict, export, track]`
+- `ARGS` (optional) are any number of custom `arg=value` pairs like `imgsz=320` that override defaults.
+ For a full list of available `ARGS` see the [Configuration](cfg.md) page and `defaults.yaml`
+ GitHub [source](https://github.com/ultralytics/ultralytics/blob/main/ultralytics/yolo/cfg/default.yaml).
+
+!!! warning "Warning"
+
+ Arguments must be passed as `arg=val` pairs, split by an equals `=` sign and delimited by spaces ` ` between pairs. Do not use `--` argument prefixes or commas `,` beteen arguments.
+
+ - `yolo predict model=yolov8n.pt imgsz=640 conf=0.25` ✅
+ - `yolo predict model yolov8n.pt imgsz 640 conf 0.25` ❌
+ - `yolo predict --model yolov8n.pt --imgsz 640 --conf 0.25` ❌
+
+## Train
+
+Train YOLOv8n on the COCO128 dataset for 100 epochs at image size 640. For a full list of available arguments see
+the [Configuration](cfg.md) page.
+
+!!! example "Example"
+
+ === "Train"
+
+ Start training YOLOv8n on COCO128 for 100 epochs at image-size 640.
+ ```bash
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=100 imgsz=640
+ ```
+
+ === "Resume"
+
+ Resume an interrupted training.
+ ```bash
+ yolo detect train resume model=last.pt
+ ```
+
+## Val
+
+Validate trained YOLOv8n model accuracy on the COCO128 dataset. No argument need to passed as the `model` retains it's
+training `data` and arguments as model attributes.
+
+!!! example "Example"
+
+ === "Official"
+
+ Validate an official YOLOv8n model.
+ ```bash
+ yolo detect val model=yolov8n.pt
+ ```
+
+ === "Custom"
+
+ Validate a custom-trained model.
+ ```bash
+ yolo detect val model=path/to/best.pt
+ ```
+
+## Predict
+
+Use a trained YOLOv8n model to run predictions on images.
+
+!!! example "Example"
+
+ === "Official"
+
+ Predict with an official YOLOv8n model.
+ ```bash
+ yolo detect predict model=yolov8n.pt source='https://ultralytics.com/images/bus.jpg'
+ ```
+
+ === "Custom"
+
+ Predict with a custom model.
+ ```bash
+ yolo detect predict model=path/to/best.pt source='https://ultralytics.com/images/bus.jpg'
+ ```
+
+## Export
+
+Export a YOLOv8n model to a different format like ONNX, CoreML, etc.
+
+!!! example "Example"
+
+ === "Official"
+
+ Export an official YOLOv8n model to ONNX format.
+ ```bash
+ yolo export model=yolov8n.pt format=onnx
+ ```
+
+ === "Custom"
+
+ Export a custom-trained model to ONNX format.
+ ```bash
+ yolo export model=path/to/best.pt format=onnx
+ ```
+
+Available YOLOv8 export formats are in the table below. You can export to any format using the `format` argument,
+i.e. `format='onnx'` or `format='engine'`.
+
+| Format | `format` Argument | Model | Metadata |
+|--------------------------------------------------------------------|-------------------|---------------------------|----------|
+| [PyTorch](https://pytorch.org/) | - | `yolov8n.pt` | ✅ |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n.torchscript` | ✅ |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n.onnx` | ✅ |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n_openvino_model/` | ✅ |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n.engine` | ✅ |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n.mlmodel` | ✅ |
+| [TF SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n_saved_model/` | ✅ |
+| [TF GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n.pb` | ❌ |
+| [TF Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n.tflite` | ✅ |
+| [TF Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n_edgetpu.tflite` | ✅ |
+| [TF.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n_web_model/` | ✅ |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n_paddle_model/` | ✅ |
+
+---
+
+## Overriding default arguments
+
+Default arguments can be overridden by simply passing them as arguments in the CLI in `arg=value` pairs.
+
+!!! tip ""
+
+ === "Train"
+ Train a detection model for `10 epochs` with `learning_rate` of `0.01`
+ ```bash
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=10 lr0=0.01
+ ```
+
+ === "Predict"
+ Predict a YouTube video using a pretrained segmentation model at image size 320:
+ ```bash
+ yolo segment predict model=yolov8n-seg.pt source='https://youtu.be/Zgi9g1ksQHc' imgsz=320
+ ```
+
+ === "Val"
+ Validate a pretrained detection model at batch-size 1 and image size 640:
+ ```bash
+ yolo detect val model=yolov8n.pt data=coco128.yaml batch=1 imgsz=640
+ ```
+
+---
+
+## Overriding default config file
+
+You can override the `default.yaml` config file entirely by passing a new file with the `cfg` arguments,
+i.e. `cfg=custom.yaml`.
+
+To do this first create a copy of `default.yaml` in your current working dir with the `yolo copy-cfg` command.
+
+This will create `default_copy.yaml`, which you can then pass as `cfg=default_copy.yaml` along with any additional args,
+like `imgsz=320` in this example:
+
+!!! example ""
+
+ === "CLI"
+ ```bash
+ yolo copy-cfg
+ yolo cfg=default_copy.yaml imgsz=320
+ ```
\ No newline at end of file
diff --git a/yolov8/docs/usage/engine.md b/yolov8/docs/usage/engine.md
new file mode 100644
index 0000000000000000000000000000000000000000..8f6444390c5dbd25e576d3603d5544c1e8217a4f
--- /dev/null
+++ b/yolov8/docs/usage/engine.md
@@ -0,0 +1,87 @@
+---
+comments: true
+description: Learn how to train and customize your models fast with the Ultralytics YOLO 'DetectionTrainer' and 'CustomTrainer'. Read more here!
+keywords: Ultralytics, YOLO, DetectionTrainer, BaseTrainer, engine components, trainers, customizing, callbacks, validators, predictors
+---
+
+Both the Ultralytics YOLO command-line and python interfaces are simply a high-level abstraction on the base engine
+executors. Let's take a look at the Trainer engine.
+
+## BaseTrainer
+
+BaseTrainer contains the generic boilerplate training routine. It can be customized for any task based over overriding
+the required functions or operations as long the as correct formats are followed. For example, you can support your own
+custom model and dataloader by just overriding these functions:
+
+* `get_model(cfg, weights)` - The function that builds the model to be trained
+* `get_dataloder()` - The function that builds the dataloader
+ More details and source code can be found in [`BaseTrainer` Reference](../reference/yolo/engine/trainer.md)
+
+## DetectionTrainer
+
+Here's how you can use the YOLOv8 `DetectionTrainer` and customize it.
+
+```python
+from ultralytics.yolo.v8.detect import DetectionTrainer
+
+trainer = DetectionTrainer(overrides={...})
+trainer.train()
+trained_model = trainer.best # get best model
+```
+
+### Customizing the DetectionTrainer
+
+Let's customize the trainer **to train a custom detection model** that is not supported directly. You can do this by
+simply overloading the existing the `get_model` functionality:
+
+```python
+from ultralytics.yolo.v8.detect import DetectionTrainer
+
+
+class CustomTrainer(DetectionTrainer):
+ def get_model(self, cfg, weights):
+ ...
+
+
+trainer = CustomTrainer(overrides={...})
+trainer.train()
+```
+
+You now realize that you need to customize the trainer further to:
+
+* Customize the `loss function`.
+* Add `callback` that uploads model to your Google Drive after every 10 `epochs`
+ Here's how you can do it:
+
+```python
+from ultralytics.yolo.v8.detect import DetectionTrainer
+from ultralytics.nn.tasks import DetectionModel
+
+
+class MyCustomModel(DetectionModel):
+ def init_criterion(self):
+ ...
+
+
+class CustomTrainer(DetectionTrainer):
+ def get_model(self, cfg, weights):
+ return MyCustomModel(...)
+
+
+# callback to upload model weights
+def log_model(trainer):
+ last_weight_path = trainer.last
+ ...
+
+
+trainer = CustomTrainer(overrides={...})
+trainer.add_callback("on_train_epoch_end", log_model) # Adds to existing callback
+trainer.train()
+```
+
+To know more about Callback triggering events and entry point, checkout our [Callbacks Guide](callbacks.md)
+
+## Other engine components
+
+There are other components that can be customized similarly like `Validators` and `Predictors`
+See Reference section for more information on these.
\ No newline at end of file
diff --git a/yolov8/docs/usage/hyperparameter_tuning.md b/yolov8/docs/usage/hyperparameter_tuning.md
new file mode 100644
index 0000000000000000000000000000000000000000..1b25ade163cfe3fae335cef01c64332ccb14c672
--- /dev/null
+++ b/yolov8/docs/usage/hyperparameter_tuning.md
@@ -0,0 +1,169 @@
+---
+comments: true
+description: Learn to integrate hyperparameter tuning using Ray Tune with Ultralytics YOLOv8, and optimize your model's performance efficiently.
+keywords: yolov8, ray tune, hyperparameter tuning, hyperparameter optimization, machine learning, computer vision, deep learning, image recognition
+---
+
+# Efficient Hyperparameter Tuning with Ray Tune and YOLOv8
+
+Hyperparameter tuning is vital in achieving peak model performance by discovering the optimal set of hyperparameters. This involves running trials with different hyperparameters and evaluating each trial’s performance.
+
+## Accelerate Tuning with Ultralytics YOLOv8 and Ray Tune
+
+[Ultralytics YOLOv8](https://ultralytics.com) incorporates Ray Tune for hyperparameter tuning, streamlining the optimization of YOLOv8 model hyperparameters. With Ray Tune, you can utilize advanced search strategies, parallelism, and early stopping to expedite the tuning process.
+
+### Ray Tune
+
+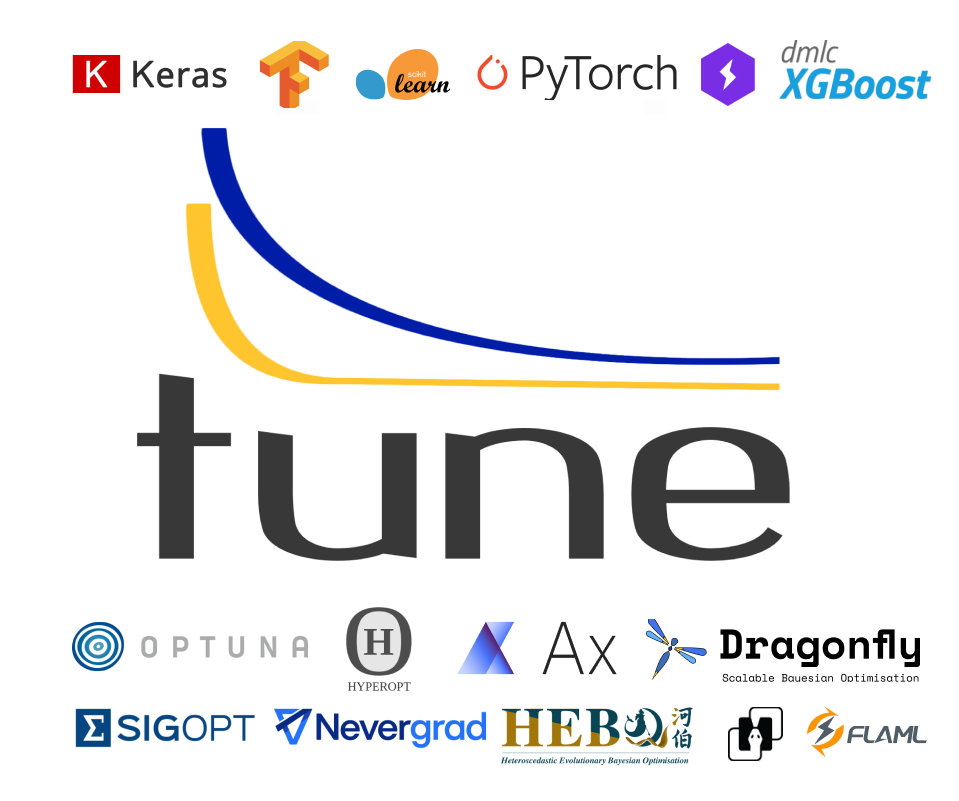
+
+[Ray Tune](https://docs.ray.io/en/latest/tune/index.html) is a hyperparameter tuning library designed for efficiency and flexibility. It supports various search strategies, parallelism, and early stopping strategies, and seamlessly integrates with popular machine learning frameworks, including Ultralytics YOLOv8.
+
+### Integration with Weights & Biases
+
+YOLOv8 also allows optional integration with [Weights & Biases](https://wandb.ai/site) for monitoring the tuning process.
+
+## Installation
+
+To install the required packages, run:
+
+!!! tip "Installation"
+
+ ```bash
+ # Install and update Ultralytics and Ray Tune pacakges
+ pip install -U ultralytics 'ray[tune]'
+
+ # Optionally install W&B for logging
+ pip install wandb
+ ```
+
+## Usage
+
+!!! example "Usage"
+
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO("yolov8n.pt")
+ result_grid = model.tune(data="coco128.yaml")
+ ```
+
+## `tune()` Method Parameters
+
+The `tune()` method in YOLOv8 provides an easy-to-use interface for hyperparameter tuning with Ray Tune. It accepts several arguments that allow you to customize the tuning process. Below is a detailed explanation of each parameter:
+
+| Parameter | Type | Description | Default Value |
+|-----------------|----------------|------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|---------------|
+| `data` | str | The dataset configuration file (in YAML format) to run the tuner on. This file should specify the training and validation data paths, as well as other dataset-specific settings. | |
+| `space` | dict, optional | A dictionary defining the hyperparameter search space for Ray Tune. Each key corresponds to a hyperparameter name, and the value specifies the range of values to explore during tuning. If not provided, YOLOv8 uses a default search space with various hyperparameters. | |
+| `grace_period` | int, optional | The grace period in epochs for the [ASHA scheduler](https://docs.ray.io/en/latest/tune/api/schedulers.html) in Ray Tune. The scheduler will not terminate any trial before this number of epochs, allowing the model to have some minimum training before making a decision on early stopping. | 10 |
+| `gpu_per_trial` | int, optional | The number of GPUs to allocate per trial during tuning. This helps manage GPU usage, particularly in multi-GPU environments. If not provided, the tuner will use all available GPUs. | None |
+| `max_samples` | int, optional | The maximum number of trials to run during tuning. This parameter helps control the total number of hyperparameter combinations tested, ensuring the tuning process does not run indefinitely. | 10 |
+| `**train_args` | dict, optional | Additional arguments to pass to the `train()` method during tuning. These arguments can include settings like the number of training epochs, batch size, and other training-specific configurations. | {} |
+
+By customizing these parameters, you can fine-tune the hyperparameter optimization process to suit your specific needs and available computational resources.
+
+## Default Search Space Description
+
+The following table lists the default search space parameters for hyperparameter tuning in YOLOv8 with Ray Tune. Each parameter has a specific value range defined by `tune.uniform()`.
+
+| Parameter | Value Range | Description |
+|-----------------|----------------------------|------------------------------------------|
+| lr0 | `tune.uniform(1e-5, 1e-1)` | Initial learning rate |
+| lrf | `tune.uniform(0.01, 1.0)` | Final learning rate factor |
+| momentum | `tune.uniform(0.6, 0.98)` | Momentum |
+| weight_decay | `tune.uniform(0.0, 0.001)` | Weight decay |
+| warmup_epochs | `tune.uniform(0.0, 5.0)` | Warmup epochs |
+| warmup_momentum | `tune.uniform(0.0, 0.95)` | Warmup momentum |
+| box | `tune.uniform(0.02, 0.2)` | Box loss weight |
+| cls | `tune.uniform(0.2, 4.0)` | Class loss weight |
+| hsv_h | `tune.uniform(0.0, 0.1)` | Hue augmentation range |
+| hsv_s | `tune.uniform(0.0, 0.9)` | Saturation augmentation range |
+| hsv_v | `tune.uniform(0.0, 0.9)` | Value (brightness) augmentation range |
+| degrees | `tune.uniform(0.0, 45.0)` | Rotation augmentation range (degrees) |
+| translate | `tune.uniform(0.0, 0.9)` | Translation augmentation range |
+| scale | `tune.uniform(0.0, 0.9)` | Scaling augmentation range |
+| shear | `tune.uniform(0.0, 10.0)` | Shear augmentation range (degrees) |
+| perspective | `tune.uniform(0.0, 0.001)` | Perspective augmentation range |
+| flipud | `tune.uniform(0.0, 1.0)` | Vertical flip augmentation probability |
+| fliplr | `tune.uniform(0.0, 1.0)` | Horizontal flip augmentation probability |
+| mosaic | `tune.uniform(0.0, 1.0)` | Mosaic augmentation probability |
+| mixup | `tune.uniform(0.0, 1.0)` | Mixup augmentation probability |
+| copy_paste | `tune.uniform(0.0, 1.0)` | Copy-paste augmentation probability |
+
+## Custom Search Space Example
+
+In this example, we demonstrate how to use a custom search space for hyperparameter tuning with Ray Tune and YOLOv8. By providing a custom search space, you can focus the tuning process on specific hyperparameters of interest.
+
+!!! example "Usage"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Define a YOLO model
+ model = YOLO("yolov8n.pt")
+
+ # Run Ray Tune on the model
+ result_grid = model.tune(data="coco128.yaml",
+ space={"lr0": tune.uniform(1e-5, 1e-1)},
+ epochs=50)
+ ```
+
+In the code snippet above, we create a YOLO model with the "yolov8n.pt" pretrained weights. Then, we call the `tune()` method, specifying the dataset configuration with "coco128.yaml". We provide a custom search space for the initial learning rate `lr0` using a dictionary with the key "lr0" and the value `tune.uniform(1e-5, 1e-1)`. Finally, we pass additional training arguments, such as the number of epochs directly to the tune method as `epochs=50`.
+
+# Processing Ray Tune Results
+
+After running a hyperparameter tuning experiment with Ray Tune, you might want to perform various analyses on the obtained results. This guide will take you through common workflows for processing and analyzing these results.
+
+## Loading Tune Experiment Results from a Directory
+
+After running the tuning experiment with `tuner.fit()`, you can load the results from a directory. This is useful, especially if you're performing the analysis after the initial training script has exited.
+
+```python
+experiment_path = f"{storage_path}/{exp_name}"
+print(f"Loading results from {experiment_path}...")
+
+restored_tuner = tune.Tuner.restore(experiment_path, trainable=train_mnist)
+result_grid = restored_tuner.get_results()
+```
+
+## Basic Experiment-Level Analysis
+
+Get an overview of how trials performed. You can quickly check if there were any errors during the trials.
+
+```python
+if result_grid.errors:
+ print("One or more trials failed!")
+else:
+ print("No errors!")
+```
+
+## Basic Trial-Level Analysis
+
+Access individual trial hyperparameter configurations and the last reported metrics.
+
+```python
+for i, result in enumerate(result_grid):
+ print(f"Trial #{i}: Configuration: {result.config}, Last Reported Metrics: {result.metrics}")
+```
+
+## Plotting the Entire History of Reported Metrics for a Trial
+
+You can plot the history of reported metrics for each trial to see how the metrics evolved over time.
+
+```python
+import matplotlib.pyplot as plt
+
+for result in result_grid:
+ plt.plot(result.metrics_dataframe["training_iteration"], result.metrics_dataframe["mean_accuracy"], label=f"Trial {i}")
+
+plt.xlabel('Training Iterations')
+plt.ylabel('Mean Accuracy')
+plt.legend()
+plt.show()
+```
+
+## Summary
+
+In this documentation, we covered common workflows to analyze the results of experiments run with Ray Tune using Ultralytics. The key steps include loading the experiment results from a directory, performing basic experiment-level and trial-level analysis and plotting metrics.
+
+Explore further by looking into Ray Tune’s [Analyze Results](https://docs.ray.io/en/latest/tune/examples/tune_analyze_results.html) docs page to get the most out of your hyperparameter tuning experiments.
diff --git a/yolov8/docs/usage/python.md b/yolov8/docs/usage/python.md
new file mode 100644
index 0000000000000000000000000000000000000000..2d8bb4c4e6f9a8bebdde0d1a7e6aa26266d148f0
--- /dev/null
+++ b/yolov8/docs/usage/python.md
@@ -0,0 +1,283 @@
+---
+comments: true
+description: Integrate YOLOv8 in Python. Load, use pretrained models, train, and infer images. Export to ONNX. Track objects in videos.
+keywords: yolov8, python usage, object detection, segmentation, classification, pretrained models, train models, image predictions
+---
+
+# Python Usage
+
+Welcome to the YOLOv8 Python Usage documentation! This guide is designed to help you seamlessly integrate YOLOv8 into
+your Python projects for object detection, segmentation, and classification. Here, you'll learn how to load and use
+pretrained models, train new models, and perform predictions on images. The easy-to-use Python interface is a valuable
+resource for anyone looking to incorporate YOLOv8 into their Python projects, allowing you to quickly implement advanced
+object detection capabilities. Let's get started!
+
+For example, users can load a model, train it, evaluate its performance on a validation set, and even export it to ONNX
+format with just a few lines of code.
+
+!!! example "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Create a new YOLO model from scratch
+ model = YOLO('yolov8n.yaml')
+
+ # Load a pretrained YOLO model (recommended for training)
+ model = YOLO('yolov8n.pt')
+
+ # Train the model using the 'coco128.yaml' dataset for 3 epochs
+ results = model.train(data='coco128.yaml', epochs=3)
+
+ # Evaluate the model's performance on the validation set
+ results = model.val()
+
+ # Perform object detection on an image using the model
+ results = model('https://ultralytics.com/images/bus.jpg')
+
+ # Export the model to ONNX format
+ success = model.export(format='onnx')
+ ```
+
+## [Train](../modes/train.md)
+
+Train mode is used for training a YOLOv8 model on a custom dataset. In this mode, the model is trained using the
+specified dataset and hyperparameters. The training process involves optimizing the model's parameters so that it can
+accurately predict the classes and locations of objects in an image.
+
+!!! example "Train"
+
+ === "From pretrained(recommended)"
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.pt') # pass any model type
+ model.train(epochs=5)
+ ```
+
+ === "From scratch"
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.yaml')
+ model.train(data='coco128.yaml', epochs=5)
+ ```
+
+ === "Resume"
+ ```python
+ model = YOLO("last.pt")
+ model.train(resume=True)
+ ```
+
+[Train Examples](../modes/train.md){ .md-button .md-button--primary}
+
+## [Val](../modes/val.md)
+
+Val mode is used for validating a YOLOv8 model after it has been trained. In this mode, the model is evaluated on a
+validation set to measure its accuracy and generalization performance. This mode can be used to tune the hyperparameters
+of the model to improve its performance.
+
+!!! example "Val"
+
+ === "Val after training"
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.yaml')
+ model.train(data='coco128.yaml', epochs=5)
+ model.val() # It'll automatically evaluate the data you trained.
+ ```
+
+ === "Val independently"
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO("model.pt")
+ # It'll use the data yaml file in model.pt if you don't set data.
+ model.val()
+ # or you can set the data you want to val
+ model.val(data='coco128.yaml')
+ ```
+
+[Val Examples](../modes/val.md){ .md-button .md-button--primary}
+
+## [Predict](../modes/predict.md)
+
+Predict mode is used for making predictions using a trained YOLOv8 model on new images or videos. In this mode, the
+model is loaded from a checkpoint file, and the user can provide images or videos to perform inference. The model
+predicts the classes and locations of objects in the input images or videos.
+
+!!! example "Predict"
+
+ === "From source"
+ ```python
+ from ultralytics import YOLO
+ from PIL import Image
+ import cv2
+
+ model = YOLO("model.pt")
+ # accepts all formats - image/dir/Path/URL/video/PIL/ndarray. 0 for webcam
+ results = model.predict(source="0")
+ results = model.predict(source="folder", show=True) # Display preds. Accepts all YOLO predict arguments
+
+ # from PIL
+ im1 = Image.open("bus.jpg")
+ results = model.predict(source=im1, save=True) # save plotted images
+
+ # from ndarray
+ im2 = cv2.imread("bus.jpg")
+ results = model.predict(source=im2, save=True, save_txt=True) # save predictions as labels
+
+ # from list of PIL/ndarray
+ results = model.predict(source=[im1, im2])
+ ```
+
+ === "Results usage"
+ ```python
+ # results would be a list of Results object including all the predictions by default
+ # but be careful as it could occupy a lot memory when there're many images,
+ # especially the task is segmentation.
+ # 1. return as a list
+ results = model.predict(source="folder")
+
+ # results would be a generator which is more friendly to memory by setting stream=True
+ # 2. return as a generator
+ results = model.predict(source=0, stream=True)
+
+ for result in results:
+ # Detection
+ result.boxes.xyxy # box with xyxy format, (N, 4)
+ result.boxes.xywh # box with xywh format, (N, 4)
+ result.boxes.xyxyn # box with xyxy format but normalized, (N, 4)
+ result.boxes.xywhn # box with xywh format but normalized, (N, 4)
+ result.boxes.conf # confidence score, (N, 1)
+ result.boxes.cls # cls, (N, 1)
+
+ # Segmentation
+ result.masks.data # masks, (N, H, W)
+ result.masks.xy # x,y segments (pixels), List[segment] * N
+ result.masks.xyn # x,y segments (normalized), List[segment] * N
+
+ # Classification
+ result.probs # cls prob, (num_class, )
+
+ # Each result is composed of torch.Tensor by default,
+ # in which you can easily use following functionality:
+ result = result.cuda()
+ result = result.cpu()
+ result = result.to("cpu")
+ result = result.numpy()
+ ```
+
+[Predict Examples](../modes/predict.md){ .md-button .md-button--primary}
+
+## [Export](../modes/export.md)
+
+Export mode is used for exporting a YOLOv8 model to a format that can be used for deployment. In this mode, the model is
+converted to a format that can be used by other software applications or hardware devices. This mode is useful when
+deploying the model to production environments.
+
+!!! example "Export"
+
+ === "Export to ONNX"
+
+ Export an official YOLOv8n model to ONNX with dynamic batch-size and image-size.
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.pt')
+ model.export(format='onnx', dynamic=True)
+ ```
+
+ === "Export to TensorRT"
+
+ Export an official YOLOv8n model to TensorRT on `device=0` for acceleration on CUDA devices.
+ ```python
+ from ultralytics import YOLO
+
+ model = YOLO('yolov8n.pt')
+ model.export(format='onnx', device=0)
+ ```
+
+[Export Examples](../modes/export.md){ .md-button .md-button--primary}
+
+## [Track](../modes/track.md)
+
+Track mode is used for tracking objects in real-time using a YOLOv8 model. In this mode, the model is loaded from a
+checkpoint file, and the user can provide a live video stream to perform real-time object tracking. This mode is useful
+for applications such as surveillance systems or self-driving cars.
+
+!!! example "Track"
+
+ === "Python"
+
+ ```python
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.pt') # load an official detection model
+ model = YOLO('yolov8n-seg.pt') # load an official segmentation model
+ model = YOLO('path/to/best.pt') # load a custom model
+
+ # Track with the model
+ results = model.track(source="https://youtu.be/Zgi9g1ksQHc", show=True)
+ results = model.track(source="https://youtu.be/Zgi9g1ksQHc", show=True, tracker="bytetrack.yaml")
+ ```
+
+[Track Examples](../modes/track.md){ .md-button .md-button--primary}
+
+## [Benchmark](../modes/benchmark.md)
+
+Benchmark mode is used to profile the speed and accuracy of various export formats for YOLOv8. The benchmarks provide
+information on the size of the exported format, its `mAP50-95` metrics (for object detection and segmentation)
+or `accuracy_top5` metrics (for classification), and the inference time in milliseconds per image across various export
+formats like ONNX, OpenVINO, TensorRT and others. This information can help users choose the optimal export format for
+their specific use case based on their requirements for speed and accuracy.
+
+!!! example "Benchmark"
+
+ === "Python"
+
+ Benchmark an official YOLOv8n model across all export formats.
+ ```python
+ from ultralytics.yolo.utils.benchmarks import benchmark
+
+ # Benchmark
+ benchmark(model='yolov8n.pt', imgsz=640, half=False, device=0)
+ ```
+
+[Benchmark Examples](../modes/benchmark.md){ .md-button .md-button--primary}
+
+## Using Trainers
+
+`YOLO` model class is a high-level wrapper on the Trainer classes. Each YOLO task has its own trainer that inherits
+from `BaseTrainer`.
+
+!!! tip "Detection Trainer Example"
+
+ ```python
+ from ultralytics.yolo import v8 import DetectionTrainer, DetectionValidator, DetectionPredictor
+
+ # trainer
+ trainer = DetectionTrainer(overrides={})
+ trainer.train()
+ trained_model = trainer.best
+
+ # Validator
+ val = DetectionValidator(args=...)
+ val(model=trained_model)
+
+ # predictor
+ pred = DetectionPredictor(overrides={})
+ pred(source=SOURCE, model=trained_model)
+
+ # resume from last weight
+ overrides["resume"] = trainer.last
+ trainer = detect.DetectionTrainer(overrides=overrides)
+ ```
+
+You can easily customize Trainers to support custom tasks or explore R&D ideas.
+Learn more about Customizing `Trainers`, `Validators` and `Predictors` to suit your project needs in the Customization
+Section.
+
+[Customization tutorials](engine.md){ .md-button .md-button--primary}
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/environments/aws_quickstart_tutorial.md b/yolov8/docs/yolov5/environments/aws_quickstart_tutorial.md
new file mode 100644
index 0000000000000000000000000000000000000000..3e3ea83cc59e7a287c970228dddba3655614c889
--- /dev/null
+++ b/yolov8/docs/yolov5/environments/aws_quickstart_tutorial.md
@@ -0,0 +1,88 @@
+---
+comments: true
+description: Get started with YOLOv5 on AWS. Our comprehensive guide provides everything you need to know to run YOLOv5 on an Amazon Deep Learning instance.
+keywords: YOLOv5, AWS, Deep Learning, Instance, Guide, Quickstart
+---
+
+# YOLOv5 🚀 on AWS Deep Learning Instance: A Comprehensive Guide
+
+This guide will help new users run YOLOv5 on an Amazon Web Services (AWS) Deep Learning instance. AWS offers a [Free Tier](https://aws.amazon.com/free/) and a [credit program](https://aws.amazon.com/activate/) for a quick and affordable start.
+
+Other quickstart options for YOLOv5 include our [Colab Notebook](https://colab.research.google.com/github/ultralytics/yolov5/blob/master/tutorial.ipynb)
, [GCP Deep Learning VM](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial), and our Docker image at [Docker Hub](https://hub.docker.com/r/ultralytics/yolov5)
. *Updated: 21 April 2023*.
+
+## 1. AWS Console Sign-in
+
+Create an account or sign in to the AWS console at [https://aws.amazon.com/console/](https://aws.amazon.com/console/) and select the **EC2** service.
+
+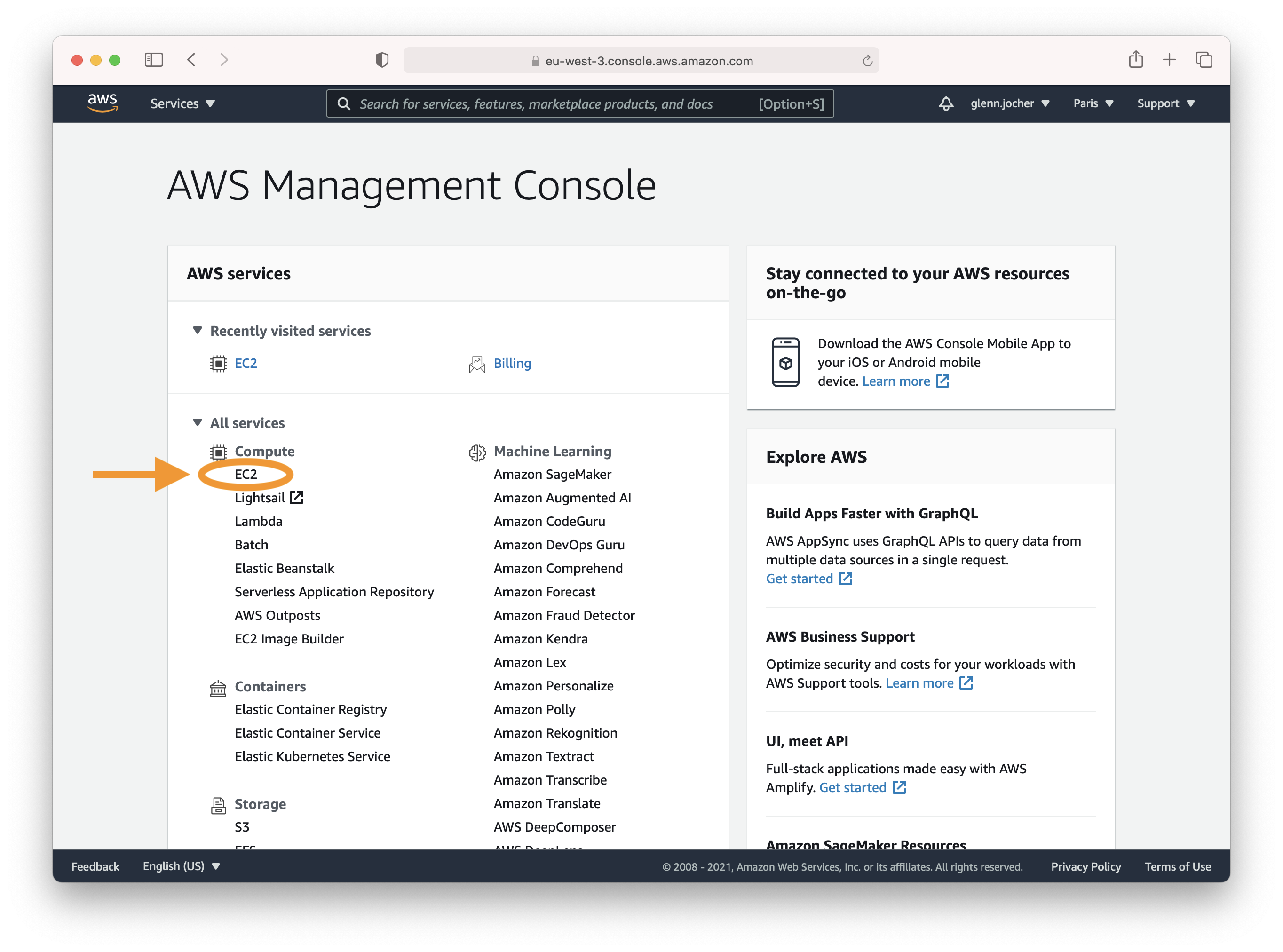
+
+## 2. Launch Instance
+
+In the EC2 section of the AWS console, click the **Launch instance** button.
+
+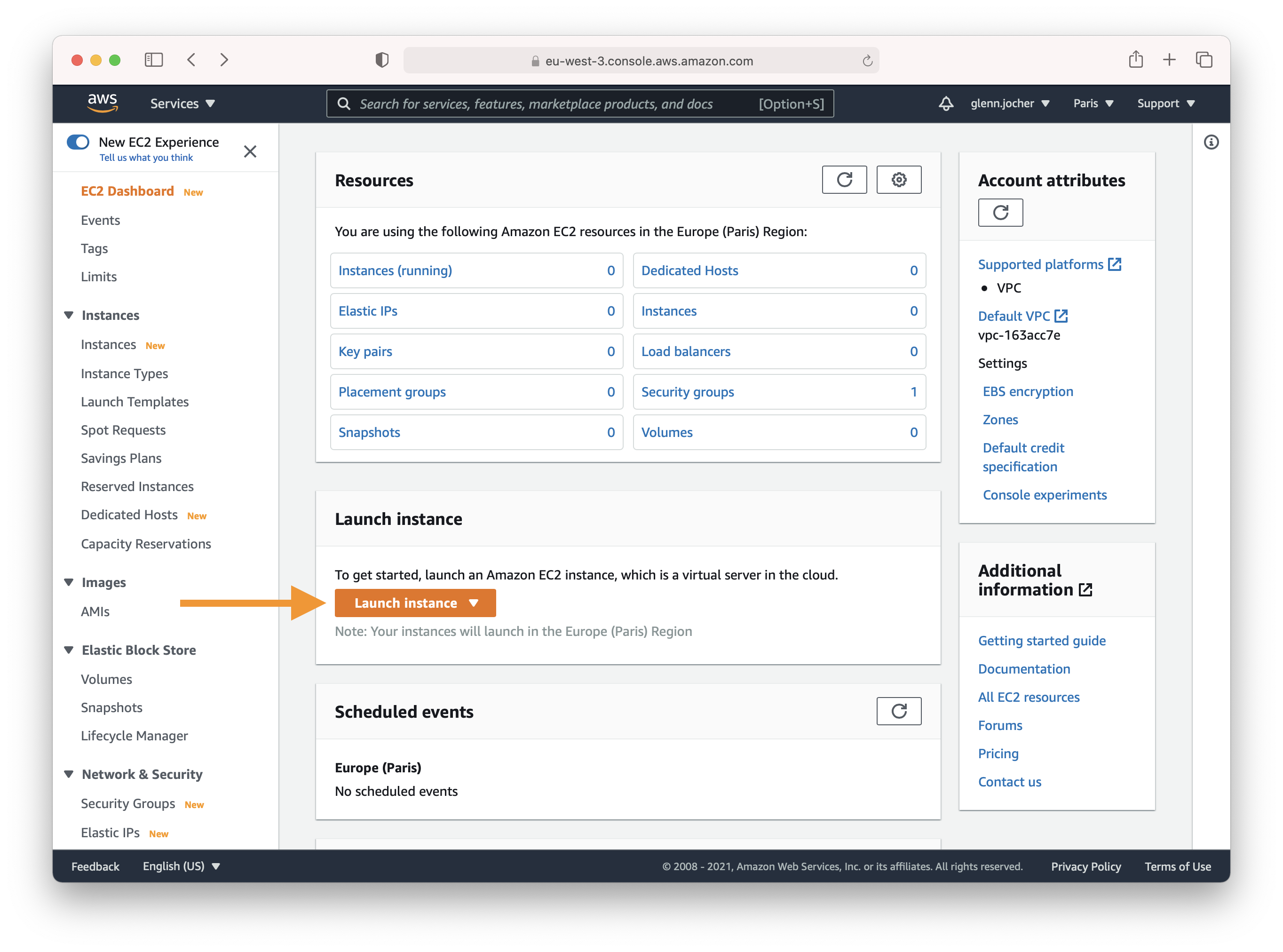
+
+### Choose an Amazon Machine Image (AMI)
+
+Enter 'Deep Learning' in the search field and select the most recent Ubuntu Deep Learning AMI (recommended), or an alternative Deep Learning AMI. For more information on selecting an AMI, see [Choosing Your DLAMI](https://docs.aws.amazon.com/dlami/latest/devguide/options.html).
+
+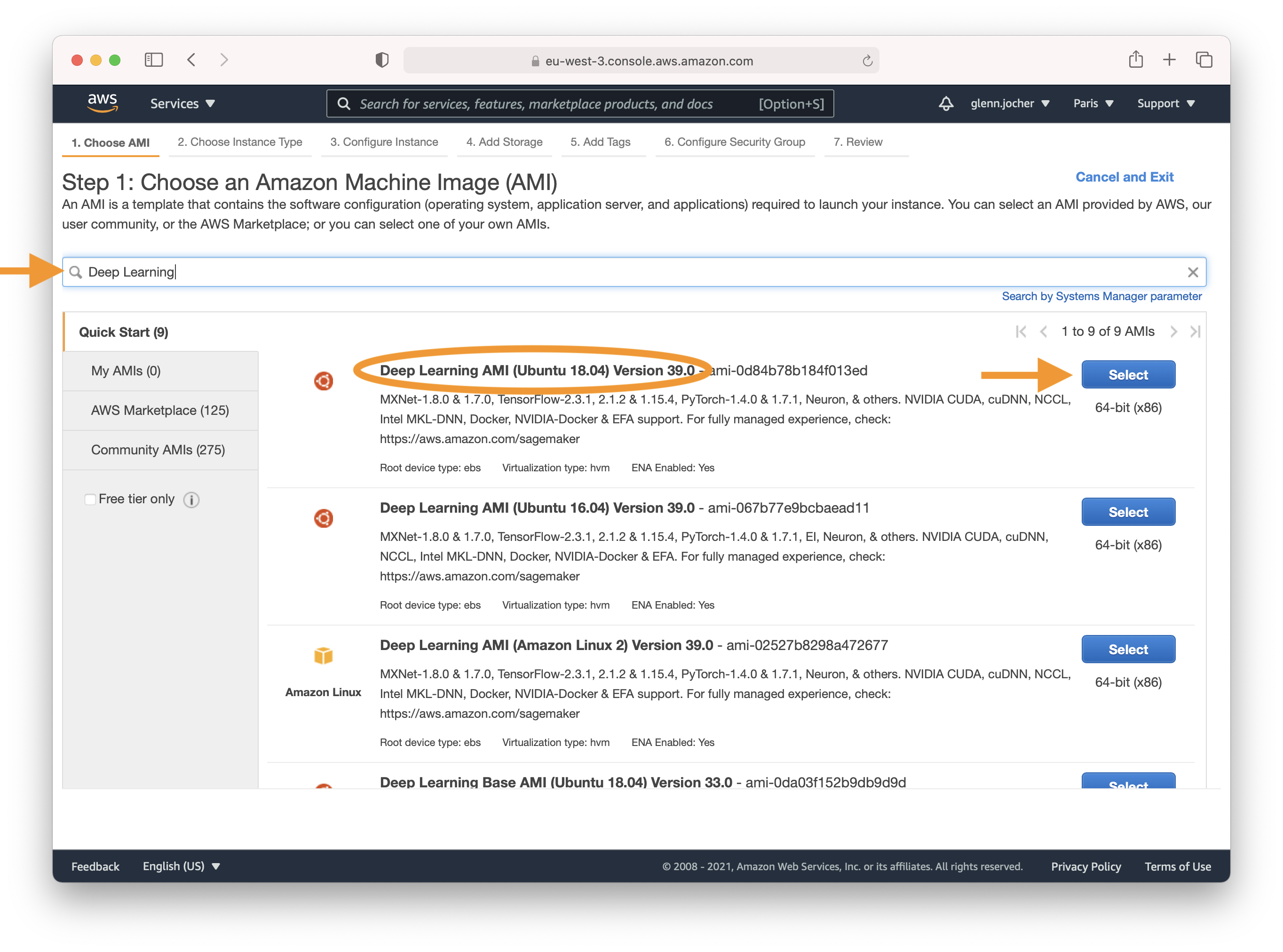
+
+### Select an Instance Type
+
+A GPU instance is recommended for most deep learning purposes. Training new models will be faster on a GPU instance than a CPU instance. Multi-GPU instances or distributed training across multiple instances with GPUs can offer sub-linear scaling. To set up distributed training, see [Distributed Training](https://docs.aws.amazon.com/dlami/latest/devguide/distributed-training.html).
+
+**Note:** The size of your model should be a factor in selecting an instance. If your model exceeds an instance's available RAM, select a different instance type with enough memory for your application.
+
+Refer to [EC2 Instance Types](https://aws.amazon.com/ec2/instance-types/) and choose Accelerated Computing to see the different GPU instance options.
+
+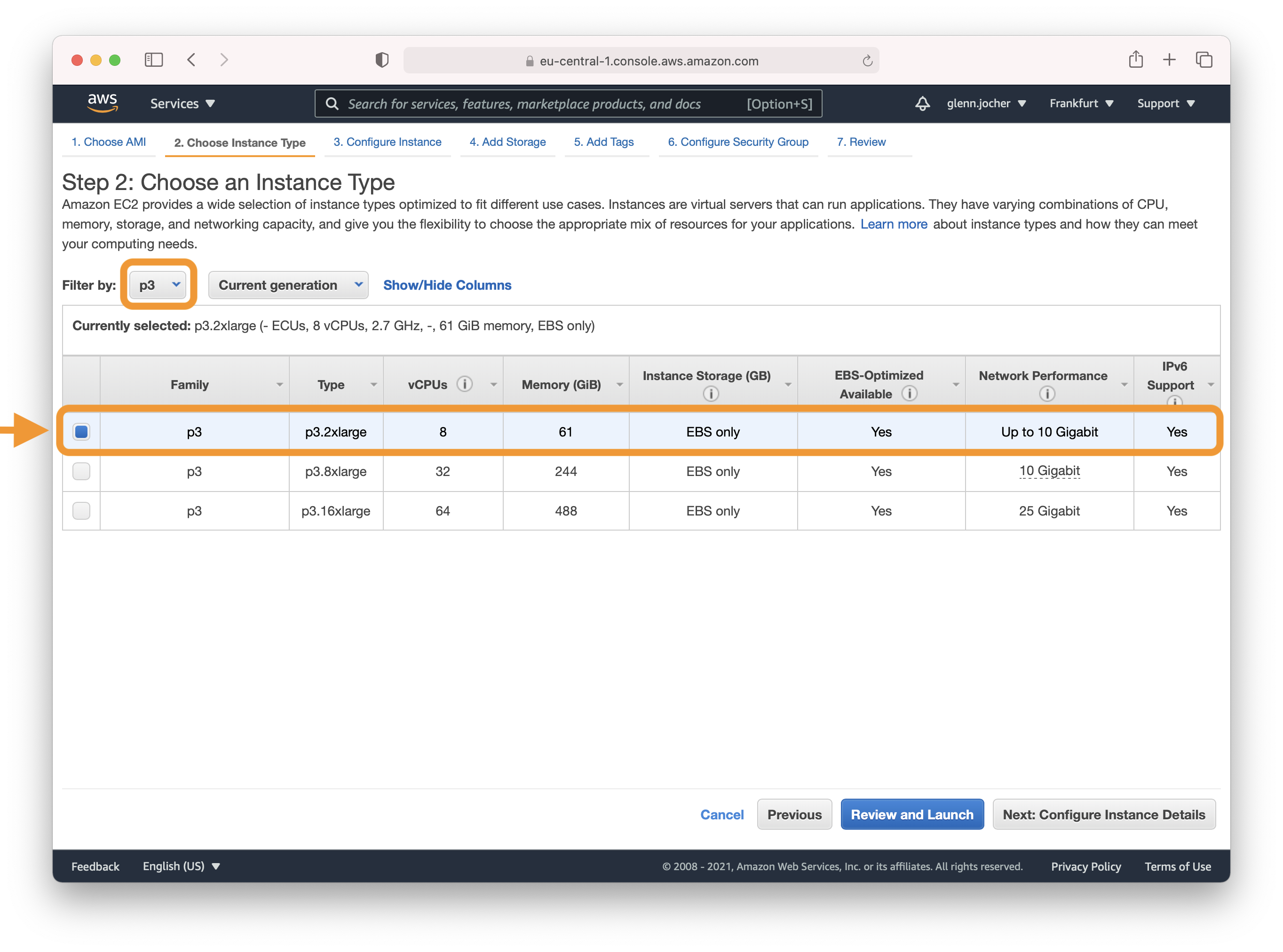
+
+For more information on GPU monitoring and optimization, see [GPU Monitoring and Optimization](https://docs.aws.amazon.com/dlami/latest/devguide/tutorial-gpu.html). For pricing, see [On-Demand Pricing](https://aws.amazon.com/ec2/pricing/on-demand/) and [Spot Pricing](https://aws.amazon.com/ec2/spot/pricing/).
+
+### Configure Instance Details
+
+Amazon EC2 Spot Instances let you take advantage of unused EC2 capacity in the AWS cloud. Spot Instances are available at up to a 70% discount compared to On-Demand prices. We recommend a persistent spot instance, which will save your data and restart automatically when spot instance availability returns after spot instance termination. For full-price On-Demand instances, leave these settings at their default values.
+
+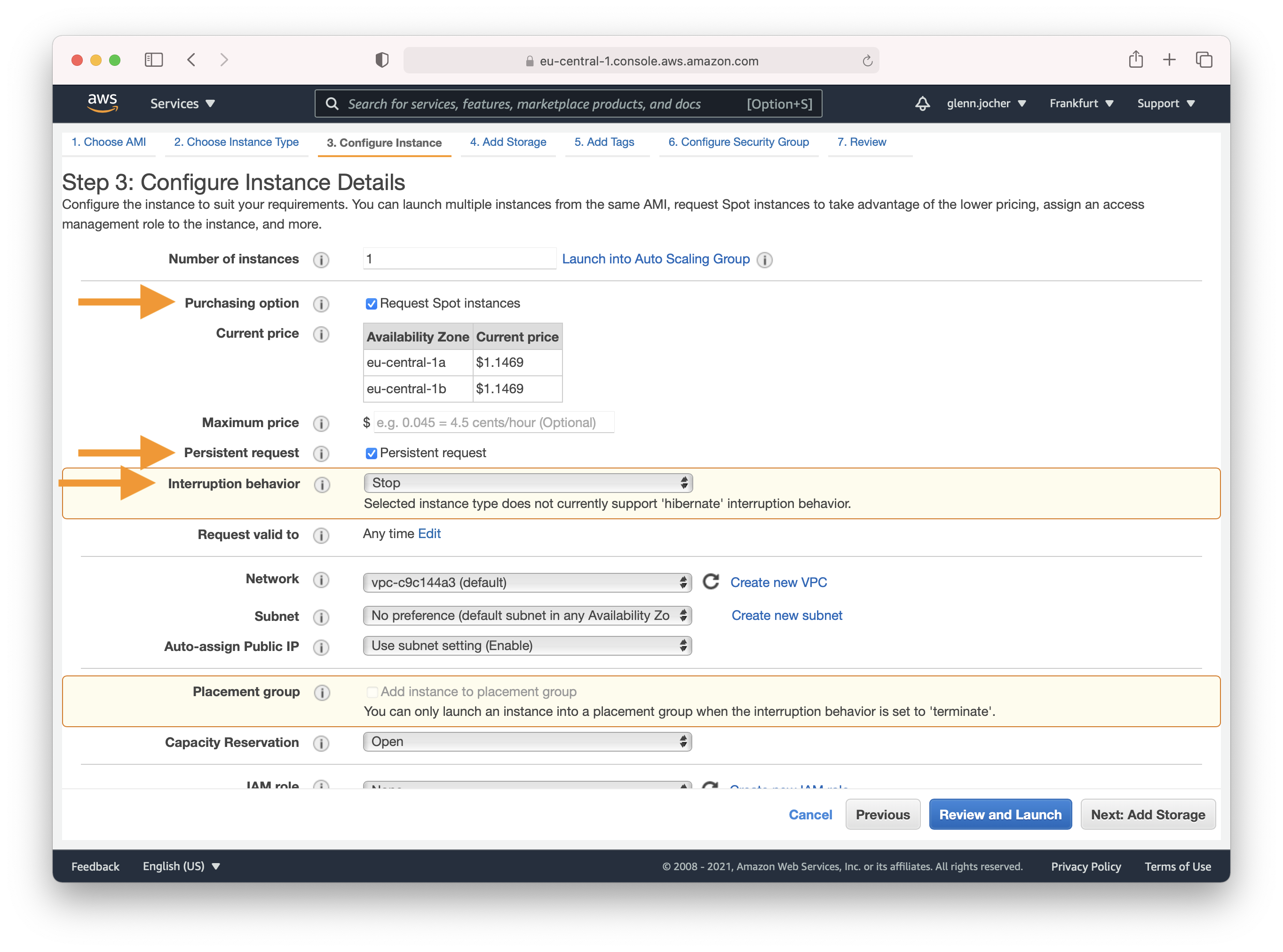
+
+Complete Steps 4-7 to finalize your instance hardware and security settings, and then launch the instance.
+
+## 3. Connect to Instance
+
+Select the checkbox next to your running instance, and then click Connect. Copy and paste the SSH terminal command into a terminal of your choice to connect to your instance.
+
+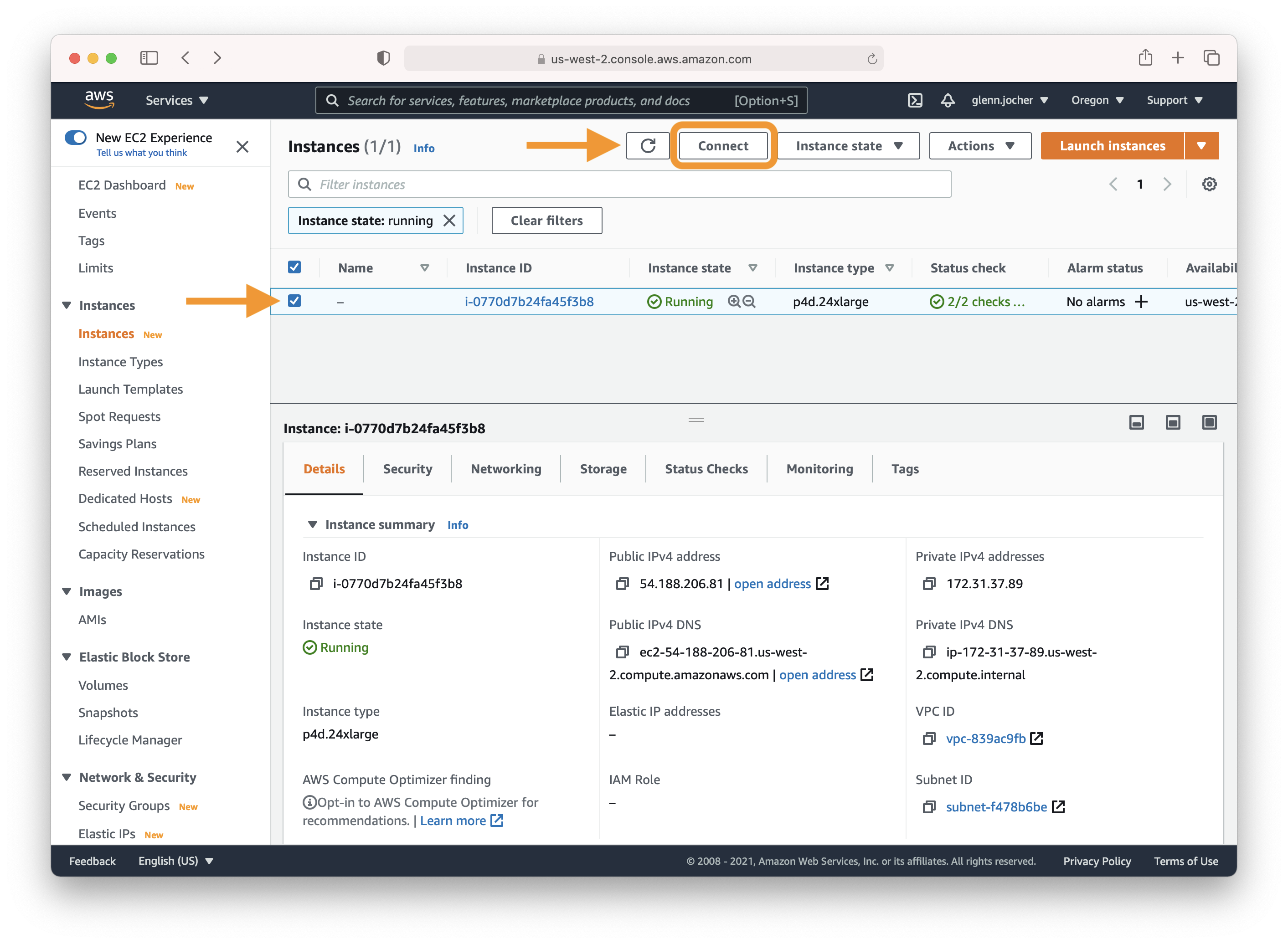
+
+## 4. Run YOLOv5
+
+Once you have logged in to your instance, clone the repository and install the dependencies in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+Then, start training, testing, detecting, and exporting YOLOv5 models:
+
+```bash
+python train.py # train a model
+python val.py --weights yolov5s.pt # validate a model for Precision, Recall, and mAP
+python detect.py --weights yolov5s.pt --source path/to/images # run inference on images and videos
+python export.py --weights yolov5s.pt --include onnx coreml tflite # export models to other formats
+```
+
+## Optional Extras
+
+Add 64GB of swap memory (to `--cache` large datasets):
+
+```bash
+sudo fallocate -l 64G /swapfile
+sudo chmod 600 /swapfile
+sudo mkswap /swapfile
+sudo swapon /swapfile
+free -h # check memory
+```
+
+Now you have successfully set up and run YOLOv5 on an AWS Deep Learning instance. Enjoy training, testing, and deploying your object detection models!
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/environments/docker_image_quickstart_tutorial.md b/yolov8/docs/yolov5/environments/docker_image_quickstart_tutorial.md
new file mode 100644
index 0000000000000000000000000000000000000000..b2b90c9b49d61203b65e324f176427109901709e
--- /dev/null
+++ b/yolov8/docs/yolov5/environments/docker_image_quickstart_tutorial.md
@@ -0,0 +1,64 @@
+---
+comments: true
+description: Get started with YOLOv5 in a Docker container. Learn to set up and run YOLOv5 models and explore other quickstart options. 🚀
+keywords: YOLOv5, Docker, tutorial, setup, training, testing, detection
+---
+
+# Get Started with YOLOv5 🚀 in Docker
+
+This tutorial will guide you through the process of setting up and running YOLOv5 in a Docker container.
+
+You can also explore other quickstart options for YOLOv5, such as our [Colab Notebook](https://colab.research.google.com/github/ultralytics/yolov5/blob/master/tutorial.ipynb)
, [GCP Deep Learning VM](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial), and [Amazon AWS](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial). *Updated: 21 April 2023*.
+
+## Prerequisites
+
+1. **Nvidia Driver**: Version 455.23 or higher. Download from [Nvidia's website](https://www.nvidia.com/Download/index.aspx).
+2. **Nvidia-Docker**: Allows Docker to interact with your local GPU. Installation instructions are available on the [Nvidia-Docker GitHub repository](https://github.com/NVIDIA/nvidia-docker).
+3. **Docker Engine - CE**: Version 19.03 or higher. Download and installation instructions can be found on the [Docker website](https://docs.docker.com/install/).
+
+## Step 1: Pull the YOLOv5 Docker Image
+
+The Ultralytics YOLOv5 DockerHub repository is available at [https://hub.docker.com/r/ultralytics/yolov5](https://hub.docker.com/r/ultralytics/yolov5). Docker Autobuild ensures that the `ultralytics/yolov5:latest` image is always in sync with the most recent repository commit. To pull the latest image, run the following command:
+
+```bash
+sudo docker pull ultralytics/yolov5:latest
+```
+
+## Step 2: Run the Docker Container
+
+### Basic container:
+
+Run an interactive instance of the YOLOv5 Docker image (called a "container") using the `-it` flag:
+
+```bash
+sudo docker run --ipc=host -it ultralytics/yolov5:latest
+```
+
+### Container with local file access:
+
+To run a container with access to local files (e.g., COCO training data in `/datasets`), use the `-v` flag:
+
+```bash
+sudo docker run --ipc=host -it -v "$(pwd)"/datasets:/usr/src/datasets ultralytics/yolov5:latest
+```
+
+### Container with GPU access:
+
+To run a container with GPU access, use the `--gpus all` flag:
+
+```bash
+sudo docker run --ipc=host -it --gpus all ultralytics/yolov5:latest
+```
+
+## Step 3: Use YOLOv5 🚀 within the Docker Container
+
+Now you can train, test, detect, and export YOLOv5 models within the running Docker container:
+
+```bash
+python train.py # train a model
+python val.py --weights yolov5s.pt # validate a model for Precision, Recall, and mAP
+python detect.py --weights yolov5s.pt --source path/to/images # run inference on images and videos
+python export.py --weights yolov5s.pt --include onnx coreml tflite # export models to other formats
+```
+
+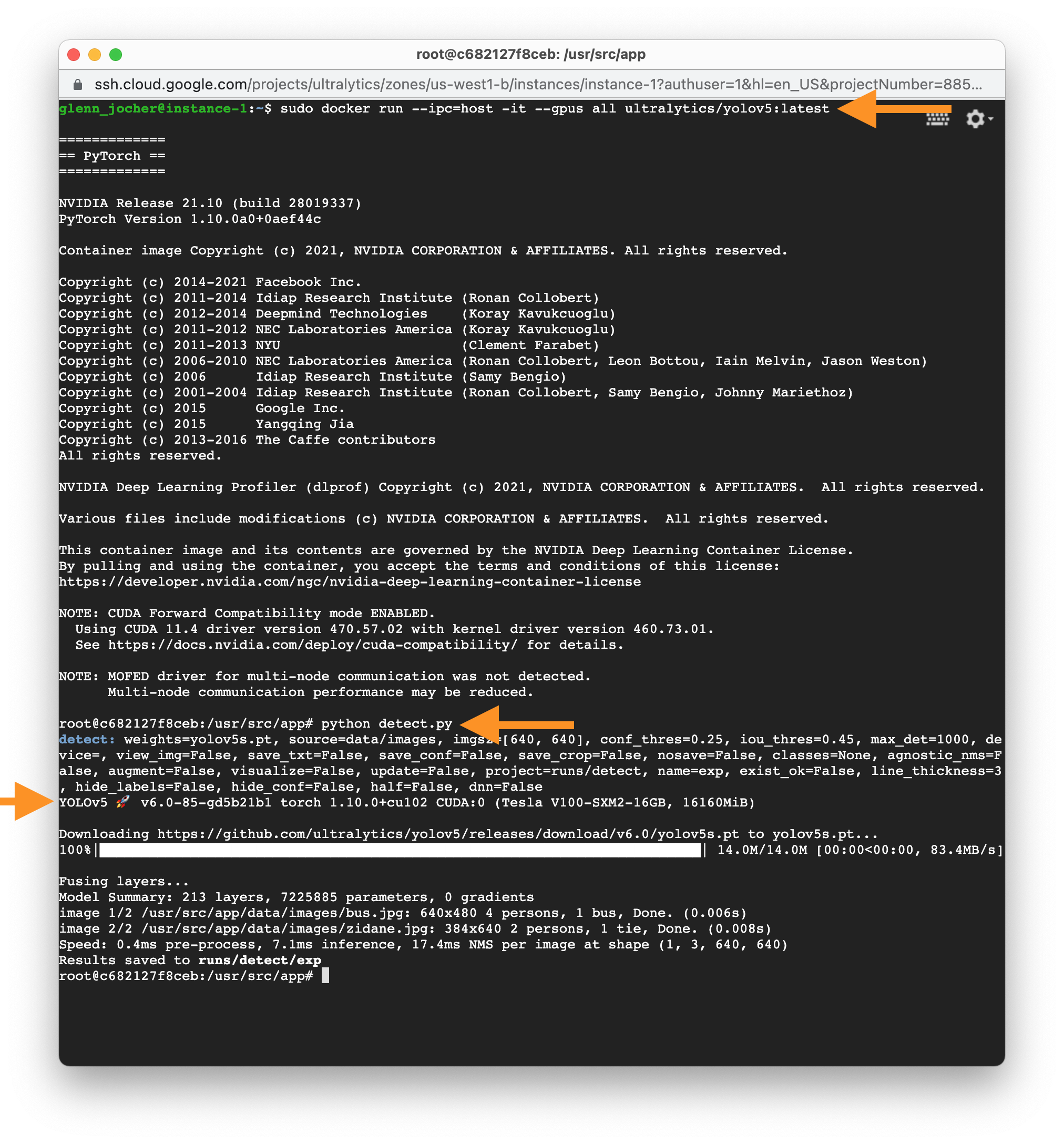
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/environments/google_cloud_quickstart_tutorial.md b/yolov8/docs/yolov5/environments/google_cloud_quickstart_tutorial.md
new file mode 100644
index 0000000000000000000000000000000000000000..c834a18a35afaf76b0328d70109df6fe277bd3db
--- /dev/null
+++ b/yolov8/docs/yolov5/environments/google_cloud_quickstart_tutorial.md
@@ -0,0 +1,49 @@
+---
+comments: true
+description: Set up YOLOv5 on a Google Cloud Platform (GCP) Deep Learning VM. Train, test, detect, and export YOLOv5 models. Tutorial updated April 2023.
+keywords: YOLOv5, GCP, deep learning, tutorial, Google Cloud Platform, virtual machine, VM, setup, free credit, Colab Notebook, AWS, Docker
+---
+
+# Run YOLOv5 🚀 on Google Cloud Platform (GCP) Deep Learning Virtual Machine (VM) ⭐
+
+This tutorial will guide you through the process of setting up and running YOLOv5 on a GCP Deep Learning VM. New GCP users are eligible for a [$300 free credit offer](https://cloud.google.com/free/docs/gcp-free-tier#free-trial).
+
+You can also explore other quickstart options for YOLOv5, such as our [Colab Notebook](https://colab.research.google.com/github/ultralytics/yolov5/blob/master/tutorial.ipynb)
, [Amazon AWS](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial) and our Docker image at [Docker Hub](https://hub.docker.com/r/ultralytics/yolov5)
. *Updated: 21 April 2023*.
+
+**Last Updated**: 6 May 2022
+
+## Step 1: Create a Deep Learning VM
+
+1. Go to the [GCP marketplace](https://console.cloud.google.com/marketplace/details/click-to-deploy-images/deeplearning) and select a **Deep Learning VM**.
+2. Choose an **n1-standard-8** instance (with 8 vCPUs and 30 GB memory).
+3. Add a GPU of your choice.
+4. Check 'Install NVIDIA GPU driver automatically on first startup?'
+5. Select a 300 GB SSD Persistent Disk for sufficient I/O speed.
+6. Click 'Deploy'.
+
+The preinstalled [Anaconda](https://docs.anaconda.com/anaconda/packages/pkg-docs/) Python environment includes all dependencies.
+
+
+
+## Step 2: Set Up the VM
+
+Clone the YOLOv5 repository and install the [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) will be downloaded automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Step 3: Run YOLOv5 🚀 on the VM
+
+You can now train, test, detect, and export YOLOv5 models on your VM:
+
+```bash
+python train.py # train a model
+python val.py --weights yolov5s.pt # validate a model for Precision, Recall, and mAP
+python detect.py --weights yolov5s.pt --source path/to/images # run inference on images and videos
+python export.py --weights yolov5s.pt --include onnx coreml tflite # export models to other formats
+```
+
+
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/index.md b/yolov8/docs/yolov5/index.md
new file mode 100644
index 0000000000000000000000000000000000000000..74f8feee4004003cfbd01e7f40455f586e994fbf
--- /dev/null
+++ b/yolov8/docs/yolov5/index.md
@@ -0,0 +1,90 @@
+---
+comments: true
+description: Explore the extensive functionalities of the YOLOv5 object detection model, renowned for its speed and precision. Dive into our comprehensive guide for installation, architectural insights, use-cases, and more to unlock the full potential of YOLOv5 for your computer vision applications.
+keywords: ultralytics, yolov5, object detection, deep learning, pytorch, computer vision, tutorial, architecture, documentation, frameworks, real-time, model training, multicore, multithreading
+---
+
+# Comprehensive Guide to Ultralytics YOLOv5
+
+
+
+
+
+
+
+

+

+

+
+

+

+

+
+
+
+Welcome to the Ultralytics' YOLOv5 🚀 Documentation! YOLOv5, the fifth iteration of the revolutionary "You Only Look Once" object detection model, is designed to deliver high-speed, high-accuracy results in real-time.
+
+Built on PyTorch, this powerful deep learning framework has garnered immense popularity for its versatility, ease of use, and high performance. Our documentation guides you through the installation process, explains the architectural nuances of the model, showcases various use-cases, and provides a series of detailed tutorials. These resources will help you harness the full potential of YOLOv5 for your computer vision projects. Let's get started!
+
+
+
+## Tutorials
+
+Here's a compilation of comprehensive tutorials that will guide you through different aspects of YOLOv5.
+
+* [Train Custom Data](tutorials/train_custom_data.md) 🚀 RECOMMENDED: Learn how to train the YOLOv5 model on your custom dataset.
+* [Tips for Best Training Results](tutorials/tips_for_best_training_results.md) ☘️: Uncover practical tips to optimize your model training process.
+* [Multi-GPU Training](tutorials/multi_gpu_training.md): Understand how to leverage multiple GPUs to expedite your training.
+* [PyTorch Hub](tutorials/pytorch_hub_model_loading.md) 🌟 NEW: Learn to load pre-trained models via PyTorch Hub.
+* [TFLite, ONNX, CoreML, TensorRT Export](tutorials/model_export.md) 🚀: Understand how to export your model to different formats.
+* [NVIDIA Jetson platform Deployment](tutorials/running_on_jetson_nano.md) 🌟 NEW: Learn how to deploy your YOLOv5 model on NVIDIA Jetson platform.
+* [Test-Time Augmentation (TTA)](tutorials/test_time_augmentation.md): Explore how to use TTA to improve your model's prediction accuracy.
+* [Model Ensembling](tutorials/model_ensembling.md): Learn the strategy of combining multiple models for improved performance.
+* [Model Pruning/Sparsity](tutorials/model_pruning_and_sparsity.md): Understand pruning and sparsity concepts, and how to create a more efficient model.
+* [Hyperparameter Evolution](tutorials/hyperparameter_evolution.md): Discover the process of automated hyperparameter tuning for better model performance.
+* [Transfer Learning with Frozen Layers](tutorials/transfer_learning_with_frozen_layers.md): Learn how to implement transfer learning by freezing layers in YOLOv5.
+* [Architecture Summary](tutorials/architecture_description.md) 🌟 Delve into the structural details of the YOLOv5 model.
+* [Roboflow for Datasets](tutorials/roboflow_datasets_integration.md): Understand how to utilize Roboflow for dataset management, labeling, and active learning.
+* [ClearML Logging](tutorials/clearml_logging_integration.md) 🌟 Learn how to integrate ClearML for efficient logging during your model training.
+* [YOLOv5 with Neural Magic](tutorials/neural_magic_pruning_quantization.md) Discover how to use Neural Magic's Deepsparse to prune and quantize your YOLOv5 model.
+* [Comet Logging](tutorials/comet_logging_integration.md) 🌟 NEW: Explore how to utilize Comet for improved model training logging.
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date, verified environments, with all dependencies (including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/), and [PyTorch](https://pytorch.org/)) pre-installed:
+
+- **Notebooks** with free
+ GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](environments/google_cloud_quickstart_tutorial.md)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](environments/aws_quickstart_tutorial.md)
+- **Docker Image**. See [Docker Quickstart Guide](environments/docker_image_quickstart_tutorial.md)
+
+## Status
+
+
+
+This badge signifies that all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify the correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and with every new commit.
+
+
+
+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+

+
+ 
+
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/quickstart_tutorial.md b/yolov8/docs/yolov5/quickstart_tutorial.md
new file mode 100644
index 0000000000000000000000000000000000000000..e46091f83309de8f905ce8b6e5dac9a1d5d9018b
--- /dev/null
+++ b/yolov8/docs/yolov5/quickstart_tutorial.md
@@ -0,0 +1,80 @@
+---
+comments: true
+description: Learn how to quickly start using YOLOv5 including installation, inference, and training on this Ultralytics Docs page.
+keywords: YOLOv5, object detection, PyTorch, quickstart, detect.py, training, Ultralytics Docs
+---
+
+# YOLOv5 Quickstart
+
+See below for quickstart examples.
+
+## Install
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a
+[**Python>=3.7.0**](https://www.python.org/) environment, including
+[**PyTorch>=1.7**](https://pytorch.org/get-started/locally/).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Inference
+
+YOLOv5 [PyTorch Hub](https://docs.ultralytics.com/yolov5/tutorials/pytorch_hub_model_loading) inference. [Models](https://github.com/ultralytics/yolov5/tree/master/models) download automatically from the latest
+YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```python
+import torch
+
+# Model
+model = torch.hub.load("ultralytics/yolov5", "yolov5s") # or yolov5n - yolov5x6, custom
+
+# Images
+img = "https://ultralytics.com/images/zidane.jpg" # or file, Path, PIL, OpenCV, numpy, list
+
+# Inference
+results = model(img)
+
+# Results
+results.print() # or .show(), .save(), .crop(), .pandas(), etc.
+```
+
+## Inference with detect.py
+
+`detect.py` runs inference on a variety of sources, downloading [models](https://github.com/ultralytics/yolov5/tree/master/models) automatically from
+the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases) and saving results to `runs/detect`.
+
+```bash
+python detect.py --weights yolov5s.pt --source 0 # webcam
+ img.jpg # image
+ vid.mp4 # video
+ screen # screenshot
+ path/ # directory
+ list.txt # list of images
+ list.streams # list of streams
+ 'path/*.jpg' # glob
+ 'https://youtu.be/Zgi9g1ksQHc' # YouTube
+ 'rtsp://example.com/media.mp4' # RTSP, RTMP, HTTP stream
+```
+
+## Training
+
+The commands below reproduce YOLOv5 [COCO](https://github.com/ultralytics/yolov5/blob/master/data/scripts/get_coco.sh)
+results. [Models](https://github.com/ultralytics/yolov5/tree/master/models)
+and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest
+YOLOv5 [release](https://github.com/ultralytics/yolov5/releases). Training times for YOLOv5n/s/m/l/x are
+1/2/4/6/8 days on a V100 GPU ([Multi-GPU](https://docs.ultralytics.com/yolov5/tutorials/multi_gpu_training) times faster). Use the
+largest `--batch-size` possible, or pass `--batch-size -1` for
+YOLOv5 [AutoBatch](https://github.com/ultralytics/yolov5/pull/5092). Batch sizes shown for V100-16GB.
+
+```bash
+python train.py --data coco.yaml --epochs 300 --weights '' --cfg yolov5n.yaml --batch-size 128
+ yolov5s 64
+ yolov5m 40
+ yolov5l 24
+ yolov5x 16
+```
+
+
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/architecture_description.md b/yolov8/docs/yolov5/tutorials/architecture_description.md
new file mode 100644
index 0000000000000000000000000000000000000000..26a2d91166185580f5a44e8b50a9cdba49866963
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/architecture_description.md
@@ -0,0 +1,224 @@
+---
+comments: true
+description: Explore the details of Ultralytics YOLOv5 architecture, a comprehensive guide to its model structure, data augmentation techniques, training strategies, and various features. Understand the intricacies of object detection algorithms and improve your skills in the machine learning field.
+keywords: yolov5 architecture, data augmentation, training strategies, object detection, yolo docs, ultralytics
+---
+
+# Ultralytics YOLOv5 Architecture
+
+YOLOv5 (v6.0/6.1) is a powerful object detection algorithm developed by Ultralytics. This article dives deep into the YOLOv5 architecture, data augmentation strategies, training methodologies, and loss computation techniques. This comprehensive understanding will help improve your practical application of object detection in various fields, including surveillance, autonomous vehicles, and image recognition.
+
+## 1. Model Structure
+
+YOLOv5's architecture consists of three main parts:
+
+- **Backbone**: This is the main body of the network. For YOLOv5, the backbone is designed using the `New CSP-Darknet53` structure, a modification of the Darknet architecture used in previous versions.
+- **Neck**: This part connects the backbone and the head. In YOLOv5, `SPPF` and `New CSP-PAN` structures are utilized.
+- **Head**: This part is responsible for generating the final output. YOLOv5 uses the `YOLOv3 Head` for this purpose.
+
+The structure of the model is depicted in the image below. The model structure details can be found in `yolov5l.yaml`.
+
+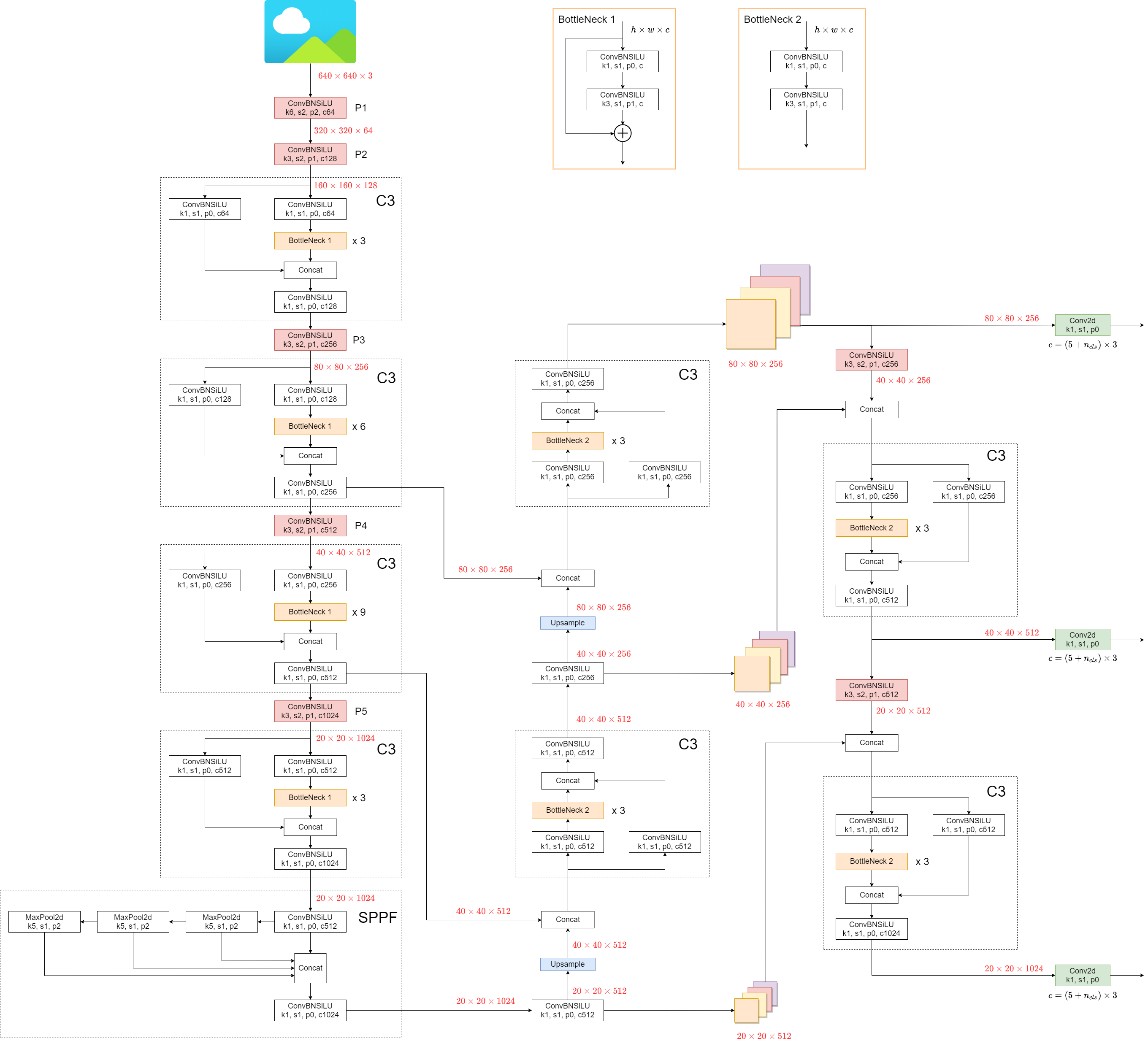
+
+YOLOv5 introduces some minor changes compared to its predecessors:
+
+1. The `Focus` structure, found in earlier versions, is replaced with a `6x6 Conv2d` structure. This change boosts efficiency [#4825](https://github.com/ultralytics/yolov5/issues/4825).
+2. The `SPP` structure is replaced with `SPPF`. This alteration more than doubles the speed of processing.
+
+To test the speed of `SPP` and `SPPF`, the following code can be used:
+
+
+SPP vs SPPF speed profiling example (click to open)
+
+```python
+import time
+import torch
+import torch.nn as nn
+
+
+class SPP(nn.Module):
+ def __init__(self):
+ super().__init__()
+ self.maxpool1 = nn.MaxPool2d(5, 1, padding=2)
+ self.maxpool2 = nn.MaxPool2d(9, 1, padding=4)
+ self.maxpool3 = nn.MaxPool2d(13, 1, padding=6)
+
+ def forward(self, x):
+ o1 = self.maxpool1(x)
+ o2 = self.maxpool2(x)
+ o3 = self.maxpool3(x)
+ return torch.cat([x, o1, o2, o3], dim=1)
+
+
+class SPPF(nn.Module):
+ def __init__(self):
+ super().__init__()
+ self.maxpool = nn.MaxPool2d(5, 1, padding=2)
+
+ def forward(self, x):
+ o1 = self.maxpool(x)
+ o2 = self.maxpool(o1)
+ o3 = self.maxpool(o2)
+ return torch.cat([x, o1, o2, o3], dim=1)
+
+
+def main():
+ input_tensor = torch.rand(8, 32, 16, 16)
+ spp = SPP()
+ sppf = SPPF()
+ output1 = spp(input_tensor)
+ output2 = sppf(input_tensor)
+
+ print(torch.equal(output1, output2))
+
+ t_start = time.time()
+ for _ in range(100):
+ spp(input_tensor)
+ print(f"SPP time: {time.time() - t_start}")
+
+ t_start = time.time()
+ for _ in range(100):
+ sppf(input_tensor)
+ print(f"SPPF time: {time.time() - t_start}")
+
+
+if __name__ == '__main__':
+ main()
+```
+
+result:
+
+```
+True
+SPP time: 0.5373051166534424
+SPPF time: 0.20780706405639648
+```
+
+
+
+## 2. Data Augmentation Techniques
+
+YOLOv5 employs various data augmentation techniques to improve the model's ability to generalize and reduce overfitting. These techniques include:
+
+- **Mosaic Augmentation**: An image processing technique that combines four training images into one in ways that encourage object detection models to better handle various object scales and translations.
+
+ 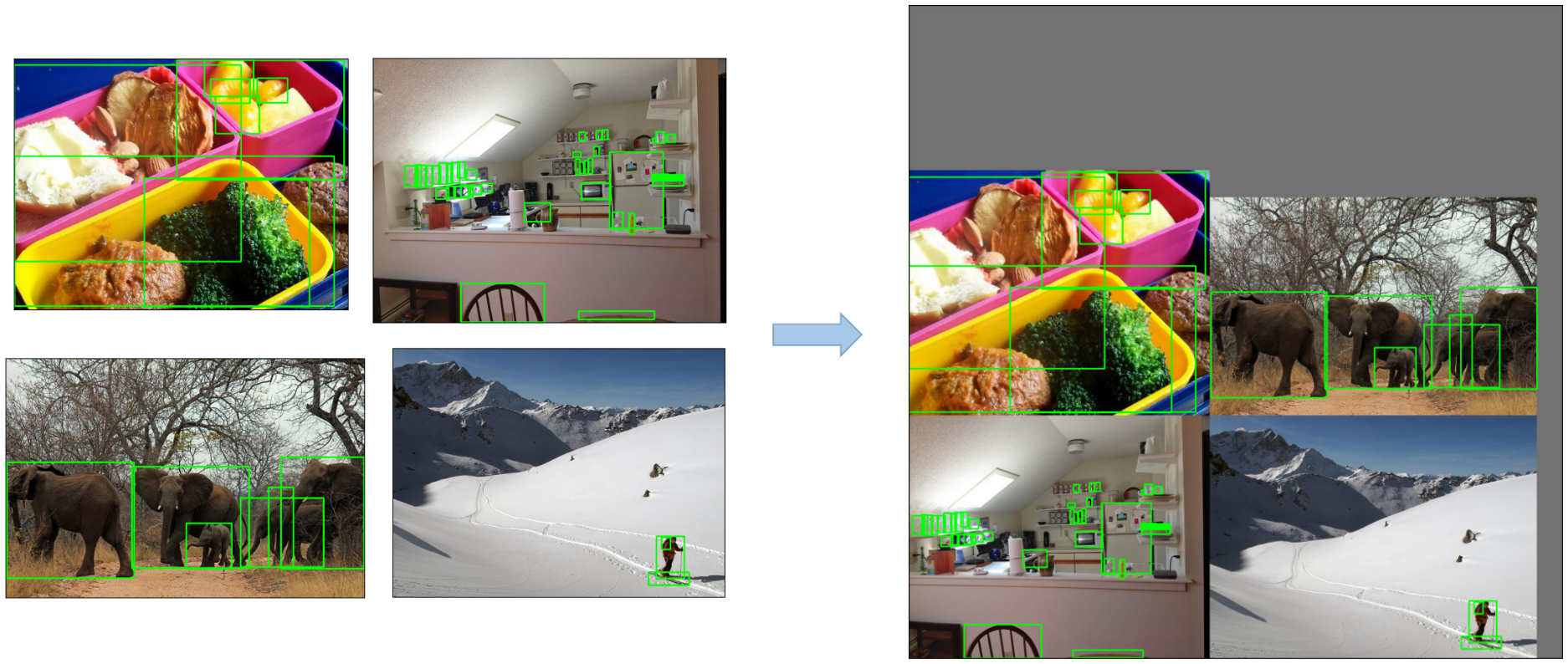
+
+- **Copy-Paste Augmentation**: An innovative data augmentation method that copies random patches from an image and pastes them onto another randomly chosen image, effectively generating a new training sample.
+
+ 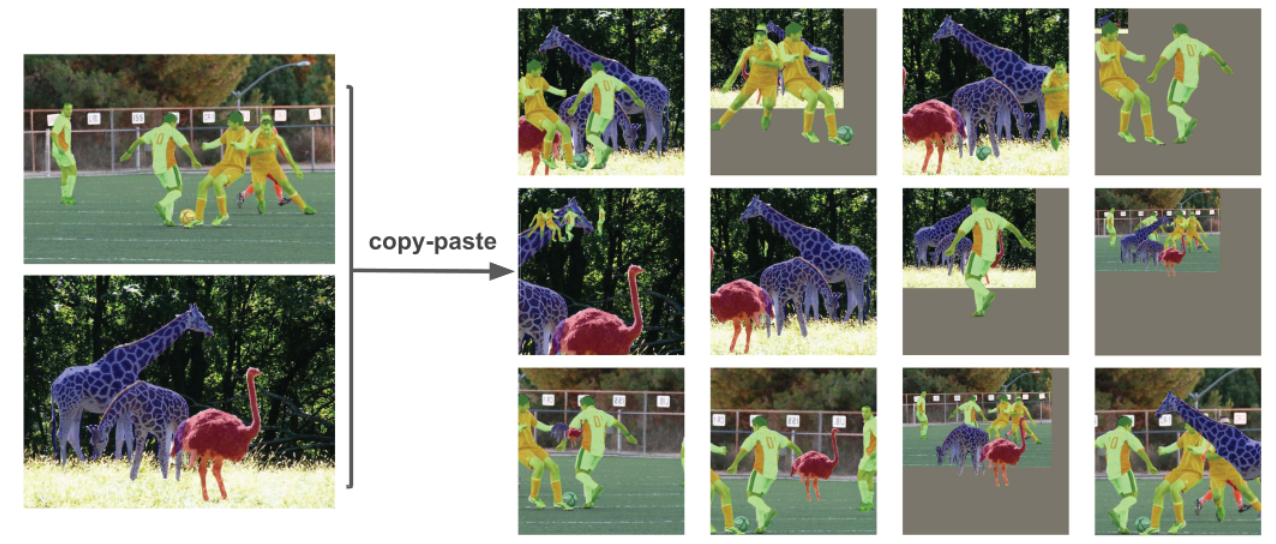
+
+- **Random Affine Transformations**: This includes random rotation, scaling, translation, and shearing of the images.
+
+ 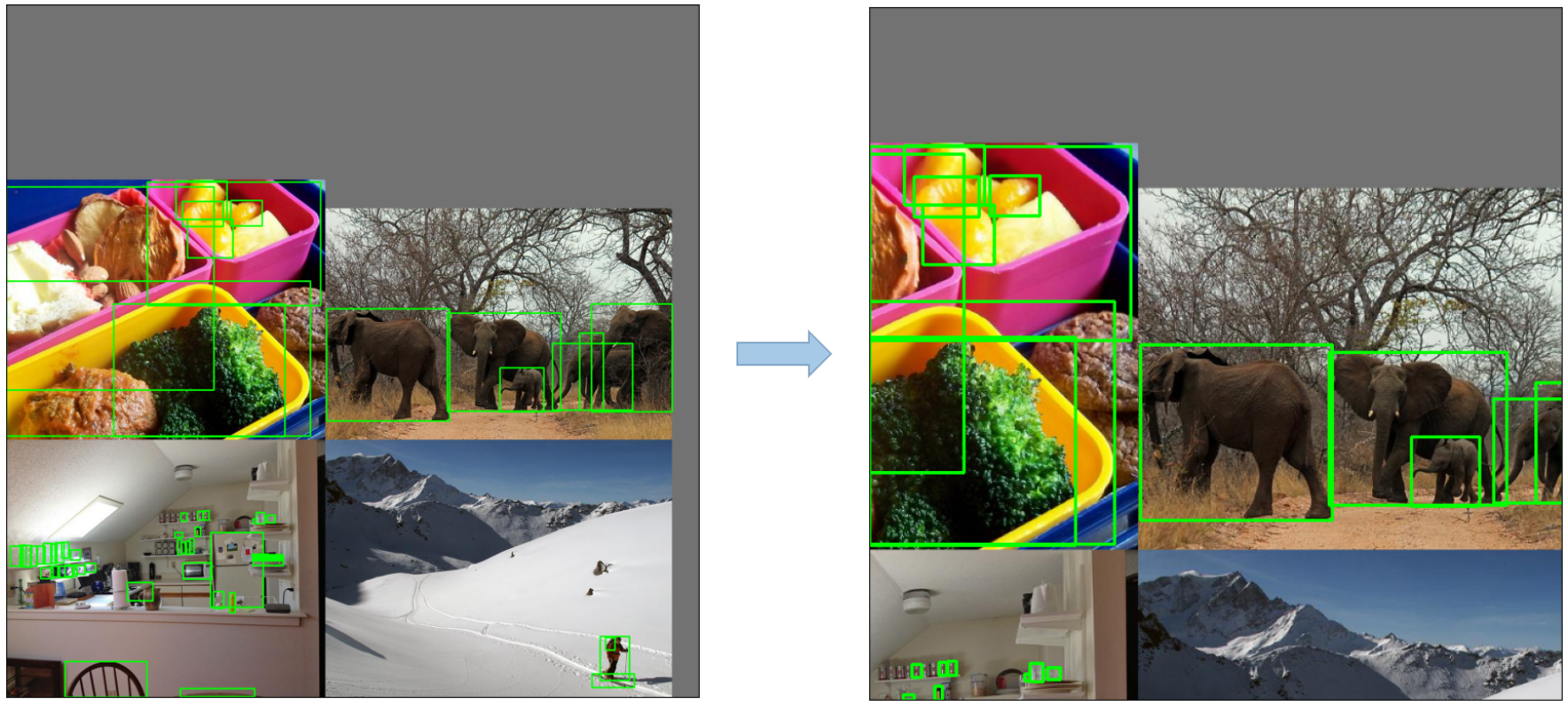
+
+- **MixUp Augmentation**: A method that creates composite images by taking a linear combination of two images and their associated labels.
+
+ 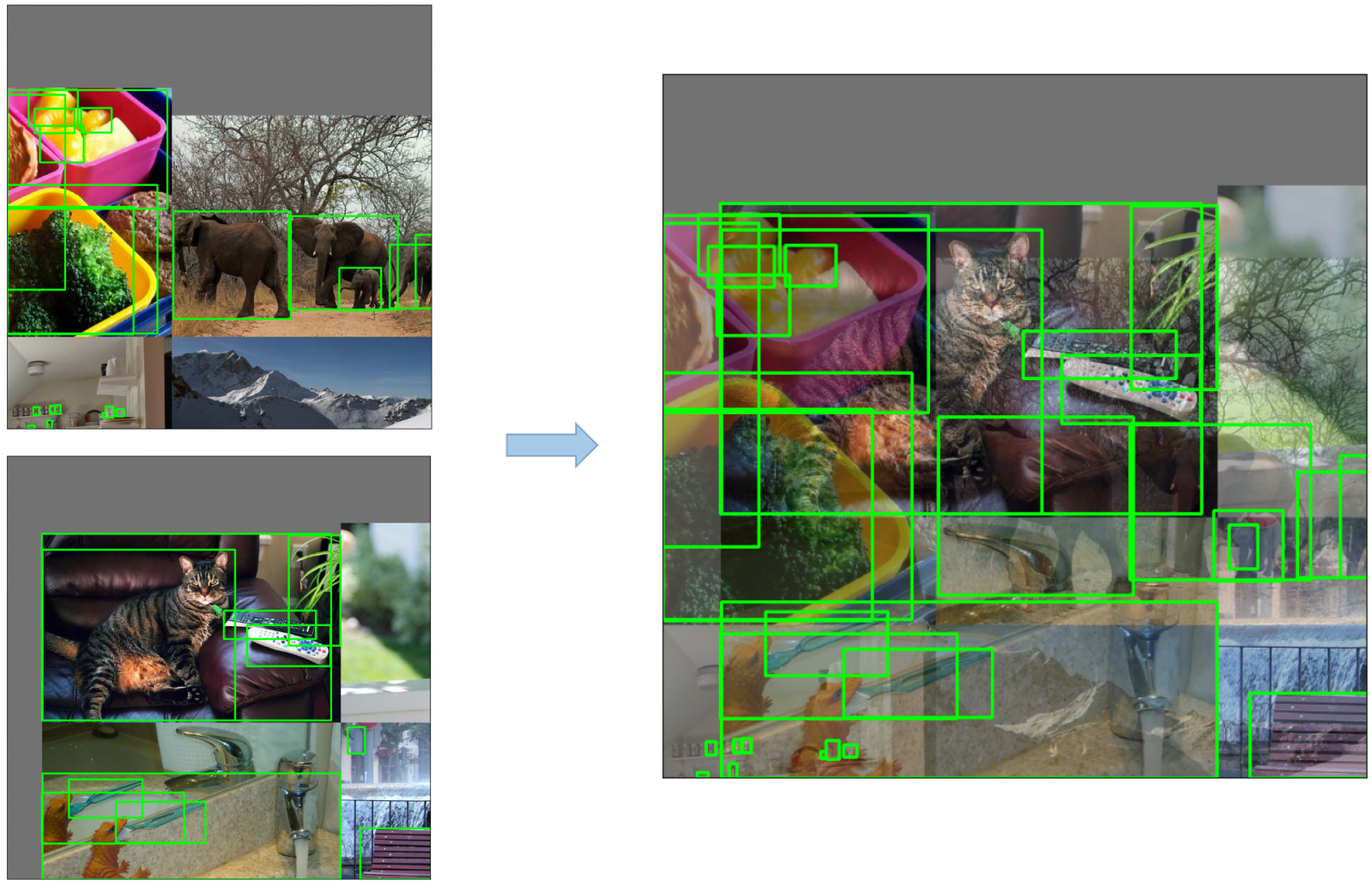
+
+- **Albumentations**: A powerful library for image augmenting that supports a wide variety of augmentation techniques.
+- **HSV Augmentation**: Random changes to the Hue, Saturation, and Value of the images.
+
+ 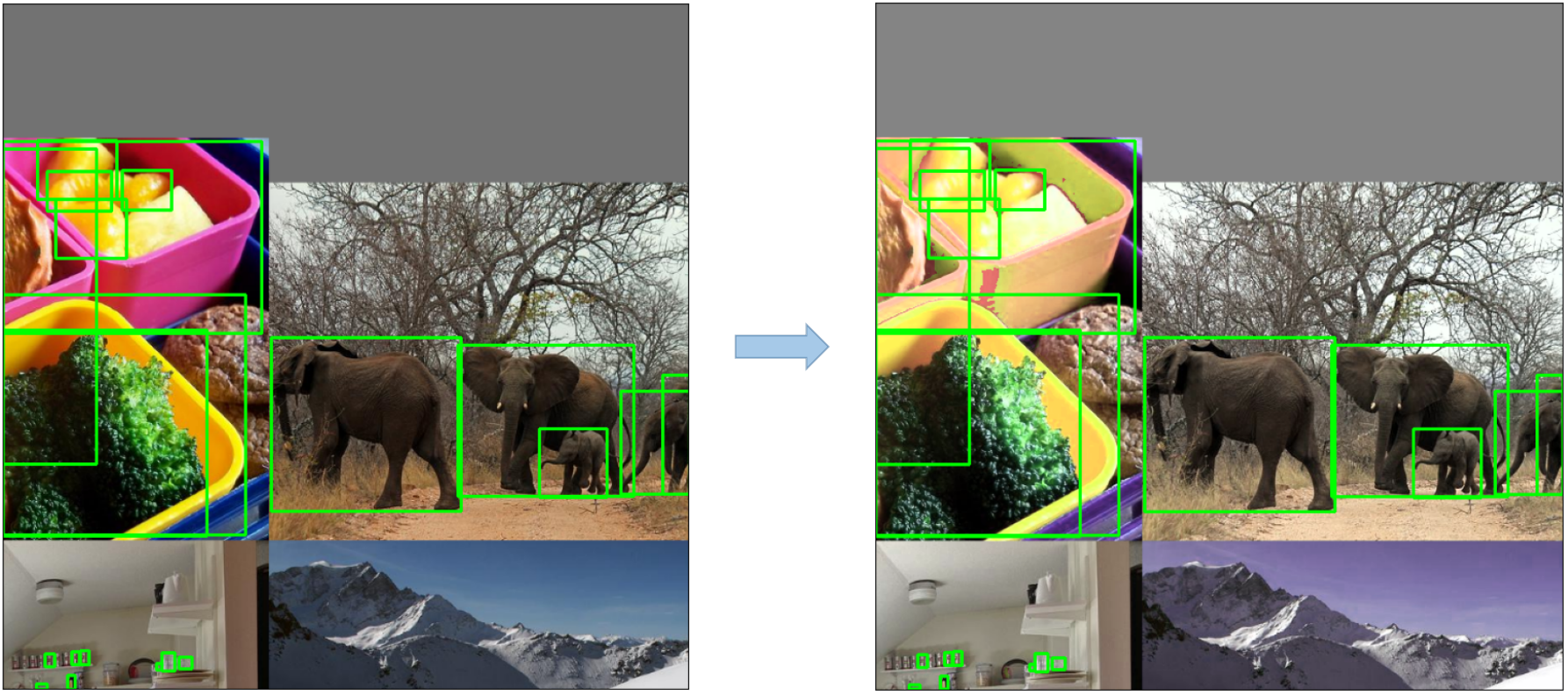
+
+- **Random Horizontal Flip**: An augmentation method that randomly flips images horizontally.
+
+ 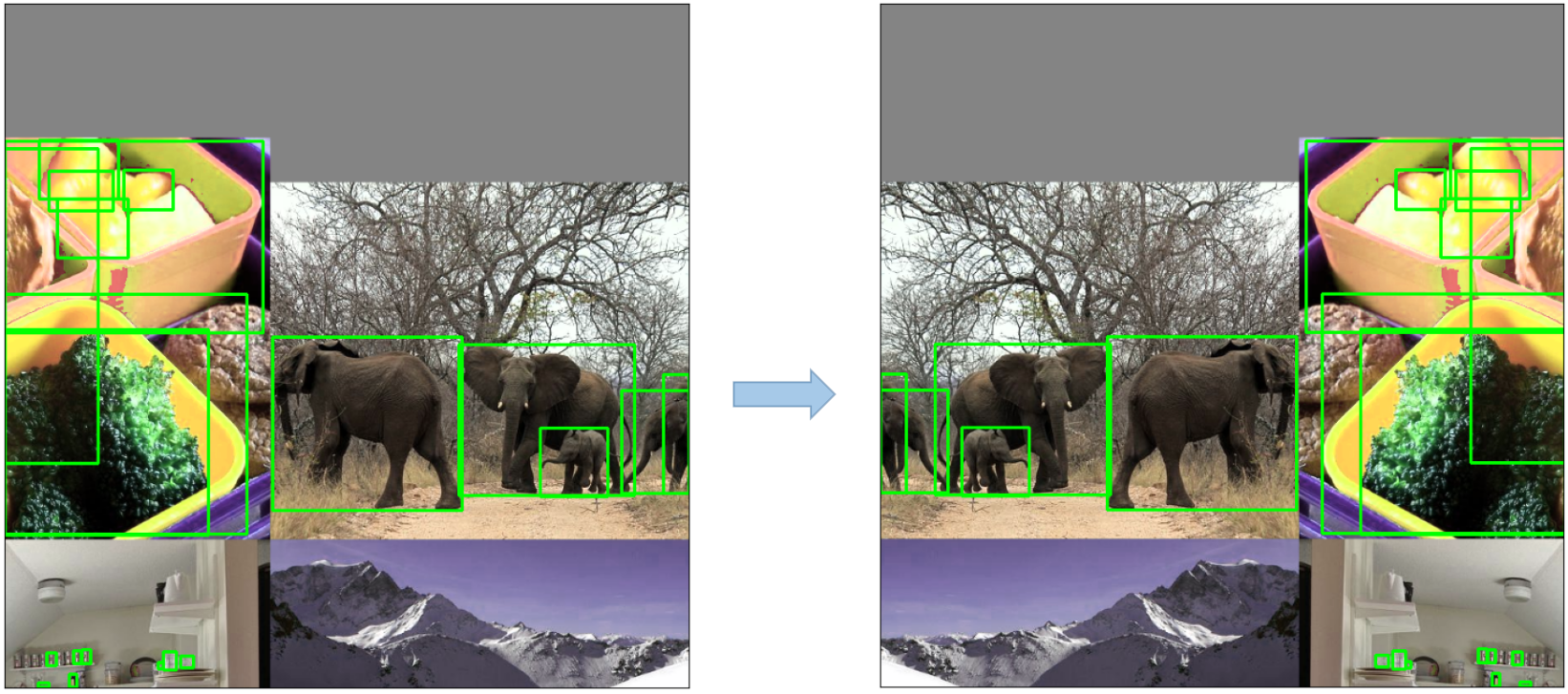
+
+## 3. Training Strategies
+
+YOLOv5 applies several sophisticated training strategies to enhance the model's performance. They include:
+
+- **Multiscale Training**: The input images are randomly rescaled within a range of 0.5 to 1.5 times their original size during the training process.
+- **AutoAnchor**: This strategy optimizes the prior anchor boxes to match the statistical characteristics of the ground truth boxes in your custom data.
+- **Warmup and Cosine LR Scheduler**: A method to adjust the learning rate to enhance model performance.
+- **Exponential Moving Average (EMA)**: A strategy that uses the average of parameters over past steps to stabilize the training process and reduce generalization error.
+- **Mixed Precision Training**: A method to perform operations in half-precision format, reducing memory usage and enhancing computational speed.
+- **Hyperparameter Evolution**: A strategy to automatically tune hyperparameters to achieve optimal performance.
+
+## 4. Additional Features
+
+### 4.1 Compute Losses
+
+The loss in YOLOv5 is computed as a combination of three individual loss components:
+
+- **Classes Loss (BCE Loss)**: Binary Cross-Entropy loss, measures the error for the classification task.
+- **Objectness Loss (BCE Loss)**: Another Binary Cross-Entropy loss, calculates the error in detecting whether an object is present in a particular grid cell or not.
+- **Location Loss (CIoU Loss)**: Complete IoU loss, measures the error in localizing the object within the grid cell.
+
+The overall loss function is depicted by:
+
+
+
+### 4.2 Balance Losses
+
+The objectness losses of the three prediction layers (`P3`, `P4`, `P5`) are weighted differently. The balance weights are `[4.0, 1.0, 0.4]` respectively. This approach ensures that the predictions at different scales contribute appropriately to the total loss.
+
+
+
+### 4.3 Eliminate Grid Sensitivity
+
+The YOLOv5 architecture makes some important changes to the box prediction strategy compared to earlier versions of YOLO. In YOLOv2 and YOLOv3, the box coordinates were directly predicted using the activation of the last layer.
+
++c_x)
++c_y)
+
+
+
+
+
+However, in YOLOv5, the formula for predicting the box coordinates has been updated to reduce grid sensitivity and prevent the model from predicting unbounded box dimensions.
+
+The revised formulas for calculating the predicted bounding box are as follows:
+
+-0.5)+c_x)
+-0.5)+c_y)
+)^2)
+)^2)
+
+Compare the center point offset before and after scaling. The center point offset range is adjusted from (0, 1) to (-0.5, 1.5).
+Therefore, offset can easily get 0 or 1.
+
+
+
+Compare the height and width scaling ratio(relative to anchor) before and after adjustment. The original yolo/darknet box equations have a serious flaw. Width and Height are completely unbounded as they are simply out=exp(in), which is dangerous, as it can lead to runaway gradients, instabilities, NaN losses and ultimately a complete loss of training. [refer this issue](https://github.com/ultralytics/yolov5/issues/471#issuecomment-662009779)
+
+
+
+### 4.4 Build Targets
+
+The build target process in YOLOv5 is critical for training efficiency and model accuracy. It involves assigning ground truth boxes to the appropriate grid cells in the output map and matching them with the appropriate anchor boxes.
+
+This process follows these steps:
+
+- Calculate the ratio of the ground truth box dimensions and the dimensions of each anchor template.
+
+
+
+
+
+)
+
+)
+
+)
+
+
+
+
+
+- If the calculated ratio is within the threshold, match the ground truth box with the corresponding anchor.
+
+
+
+- Assign the matched anchor to the appropriate cells, keeping in mind that due to the revised center point offset, a ground truth box can be assigned to more than one anchor. Because the center point offset range is adjusted from (0, 1) to (-0.5, 1.5). GT Box can be assigned to more anchors.
+
+
+
+This way, the build targets process ensures that each ground truth object is properly assigned and matched during the training process, allowing YOLOv5 to learn the task of object detection more effectively.
+
+## Conclusion
+
+In conclusion, YOLOv5 represents a significant step forward in the development of real-time object detection models. By incorporating various new features, enhancements, and training strategies, it surpasses previous versions of the YOLO family in performance and efficiency.
+
+The primary enhancements in YOLOv5 include the use of a dynamic architecture, an extensive range of data augmentation techniques, innovative training strategies, as well as important adjustments in computing losses and the process of building targets. All these innovations significantly improve the accuracy and efficiency of object detection while retaining a high degree of speed, which is the trademark of YOLO models.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/clearml_logging_integration.md b/yolov8/docs/yolov5/tutorials/clearml_logging_integration.md
new file mode 100644
index 0000000000000000000000000000000000000000..3d8672d09cc46e42a9e2f8ebe69323876cb388e0
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/clearml_logging_integration.md
@@ -0,0 +1,243 @@
+---
+comments: true
+description: Integrate ClearML with YOLOv5 to track experiments and manage data versions. Optimize hyperparameters and remotely monitor your runs.
+keywords: YOLOv5, ClearML, experiment manager, remotely train, monitor, hyperparameter optimization, data versioning tool, HPO, data version management, optimization locally, agent, training progress, custom YOLOv5, AI development, model building
+---
+
+# ClearML Integration
+
+
+
+## About ClearML
+
+[ClearML](https://cutt.ly/yolov5-tutorial-clearml) is an [open-source](https://github.com/allegroai/clearml) toolbox designed to save you time ⏱️.
+
+🔨 Track every YOLOv5 training run in the experiment manager
+
+🔧 Version and easily access your custom training data with the integrated ClearML Data Versioning Tool
+
+🔦 Remotely train and monitor your YOLOv5 training runs using ClearML Agent
+
+🔬 Get the very best mAP using ClearML Hyperparameter Optimization
+
+🔭 Turn your newly trained YOLOv5 model into an API with just a few commands using ClearML Serving
+
+
+And so much more. It's up to you how many of these tools you want to use, you can stick to the experiment manager, or chain them all together into an impressive pipeline!
+
+
+
+
+
+
+
+
+## 🦾 Setting Things Up
+
+To keep track of your experiments and/or data, ClearML needs to communicate to a server. You have 2 options to get one:
+
+Either sign up for free to the [ClearML Hosted Service](https://cutt.ly/yolov5-tutorial-clearml) or you can set up your own server, see [here](https://clear.ml/docs/latest/docs/deploying_clearml/clearml_server). Even the server is open-source, so even if you're dealing with sensitive data, you should be good to go!
+
+1. Install the `clearml` python package:
+
+ ```bash
+ pip install clearml
+ ```
+
+2. Connect the ClearML SDK to the server by [creating credentials](https://app.clear.ml/settings/workspace-configuration) (go right top to Settings -> Workspace -> Create new credentials), then execute the command below and follow the instructions:
+
+ ```bash
+ clearml-init
+ ```
+
+That's it! You're done 😎
+
+
+
+## 🚀 Training YOLOv5 With ClearML
+
+To enable ClearML experiment tracking, simply install the ClearML pip package.
+
+```bash
+pip install clearml>=1.2.0
+```
+
+This will enable integration with the YOLOv5 training script. Every training run from now on, will be captured and stored by the ClearML experiment manager.
+
+If you want to change the `project_name` or `task_name`, use the `--project` and `--name` arguments of the `train.py` script, by default the project will be called `YOLOv5` and the task `Training`.
+PLEASE NOTE: ClearML uses `/` as a delimiter for subprojects, so be careful when using `/` in your project name!
+
+```bash
+python train.py --img 640 --batch 16 --epochs 3 --data coco128.yaml --weights yolov5s.pt --cache
+```
+
+or with custom project and task name:
+
+```bash
+python train.py --project my_project --name my_training --img 640 --batch 16 --epochs 3 --data coco128.yaml --weights yolov5s.pt --cache
+```
+
+This will capture:
+
+- Source code + uncommitted changes
+- Installed packages
+- (Hyper)parameters
+- Model files (use `--save-period n` to save a checkpoint every n epochs)
+- Console output
+- Scalars (mAP_0.5, mAP_0.5:0.95, precision, recall, losses, learning rates, ...)
+- General info such as machine details, runtime, creation date etc.
+- All produced plots such as label correlogram and confusion matrix
+- Images with bounding boxes per epoch
+- Mosaic per epoch
+- Validation images per epoch
+- ...
+
+That's a lot right? 🤯
+Now, we can visualize all of this information in the ClearML UI to get an overview of our training progress. Add custom columns to the table view (such as e.g. mAP_0.5) so you can easily sort on the best performing model. Or select multiple experiments and directly compare them!
+
+There even more we can do with all of this information, like hyperparameter optimization and remote execution, so keep reading if you want to see how that works!
+
+
+
+## 🔗 Dataset Version Management
+
+Versioning your data separately from your code is generally a good idea and makes it easy to acquire the latest version too. This repository supports supplying a dataset version ID, and it will make sure to get the data if it's not there yet. Next to that, this workflow also saves the used dataset ID as part of the task parameters, so you will always know for sure which data was used in which experiment!
+
+
+
+### Prepare Your Dataset
+
+The YOLOv5 repository supports a number of different datasets by using yaml files containing their information. By default datasets are downloaded to the `../datasets` folder in relation to the repository root folder. So if you downloaded the `coco128` dataset using the link in the yaml or with the scripts provided by yolov5, you get this folder structure:
+
+```
+..
+|_ yolov5
+|_ datasets
+ |_ coco128
+ |_ images
+ |_ labels
+ |_ LICENSE
+ |_ README.txt
+```
+
+But this can be any dataset you wish. Feel free to use your own, as long as you keep to this folder structure.
+
+Next, ⚠️**copy the corresponding yaml file to the root of the dataset folder**⚠️. This yaml files contains the information ClearML will need to properly use the dataset. You can make this yourself too, of course, just follow the structure of the example yamls.
+
+Basically we need the following keys: `path`, `train`, `test`, `val`, `nc`, `names`.
+
+```
+..
+|_ yolov5
+|_ datasets
+ |_ coco128
+ |_ images
+ |_ labels
+ |_ coco128.yaml # <---- HERE!
+ |_ LICENSE
+ |_ README.txt
+```
+
+### Upload Your Dataset
+
+To get this dataset into ClearML as a versioned dataset, go to the dataset root folder and run the following command:
+
+```bash
+cd coco128
+clearml-data sync --project YOLOv5 --name coco128 --folder .
+```
+
+The command `clearml-data sync` is actually a shorthand command. You could also run these commands one after the other:
+
+```bash
+# Optionally add --parent if you want to base
+# this version on another dataset version, so no duplicate files are uploaded!
+clearml-data create --name coco128 --project YOLOv5
+clearml-data add --files .
+clearml-data close
+```
+
+### Run Training Using A ClearML Dataset
+
+Now that you have a ClearML dataset, you can very simply use it to train custom YOLOv5 🚀 models!
+
+```bash
+python train.py --img 640 --batch 16 --epochs 3 --data clearml:// --weights yolov5s.pt --cache
+```
+
+
+
+## 👀 Hyperparameter Optimization
+
+Now that we have our experiments and data versioned, it's time to take a look at what we can build on top!
+
+Using the code information, installed packages and environment details, the experiment itself is now **completely reproducible**. In fact, ClearML allows you to clone an experiment and even change its parameters. We can then just rerun it with these new parameters automatically, this is basically what HPO does!
+
+To **run hyperparameter optimization locally**, we've included a pre-made script for you. Just make sure a training task has been run at least once, so it is in the ClearML experiment manager, we will essentially clone it and change its hyperparameters.
+
+You'll need to fill in the ID of this `template task` in the script found at `utils/loggers/clearml/hpo.py` and then just run it :) You can change `task.execute_locally()` to `task.execute()` to put it in a ClearML queue and have a remote agent work on it instead.
+
+```bash
+# To use optuna, install it first, otherwise you can change the optimizer to just be RandomSearch
+pip install optuna
+python utils/loggers/clearml/hpo.py
+```
+
+
+
+## 🤯 Remote Execution (advanced)
+
+Running HPO locally is really handy, but what if we want to run our experiments on a remote machine instead? Maybe you have access to a very powerful GPU machine on-site, or you have some budget to use cloud GPUs.
+This is where the ClearML Agent comes into play. Check out what the agent can do here:
+
+- [YouTube video](https://youtu.be/MX3BrXnaULs)
+- [Documentation](https://clear.ml/docs/latest/docs/clearml_agent)
+
+In short: every experiment tracked by the experiment manager contains enough information to reproduce it on a different machine (installed packages, uncommitted changes etc.). So a ClearML agent does just that: it listens to a queue for incoming tasks and when it finds one, it recreates the environment and runs it while still reporting scalars, plots etc. to the experiment manager.
+
+You can turn any machine (a cloud VM, a local GPU machine, your own laptop ... ) into a ClearML agent by simply running:
+
+```bash
+clearml-agent daemon --queue [--docker]
+```
+
+### Cloning, Editing And Enqueuing
+
+With our agent running, we can give it some work. Remember from the HPO section that we can clone a task and edit the hyperparameters? We can do that from the interface too!
+
+🪄 Clone the experiment by right-clicking it
+
+🎯 Edit the hyperparameters to what you wish them to be
+
+⏳ Enqueue the task to any of the queues by right-clicking it
+
+
+
+### Executing A Task Remotely
+
+Now you can clone a task like we explained above, or simply mark your current script by adding `task.execute_remotely()` and on execution it will be put into a queue, for the agent to start working on!
+
+To run the YOLOv5 training script remotely, all you have to do is add this line to the training.py script after the clearml logger has been instantiated:
+
+```python
+# ...
+# Loggers
+data_dict = None
+if RANK in {-1, 0}:
+ loggers = Loggers(save_dir, weights, opt, hyp, LOGGER) # loggers instance
+ if loggers.clearml:
+ loggers.clearml.task.execute_remotely(queue="my_queue") # <------ ADD THIS LINE
+ # Data_dict is either None is user did not choose for ClearML dataset or is filled in by ClearML
+ data_dict = loggers.clearml.data_dict
+# ...
+```
+
+When running the training script after this change, python will run the script up until that line, after which it will package the code and send it to the queue instead!
+
+### Autoscaling workers
+
+ClearML comes with autoscalers too! This tool will automatically spin up new remote machines in the cloud of your choice (AWS, GCP, Azure) and turn them into ClearML agents for you whenever there are experiments detected in the queue. Once the tasks are processed, the autoscaler will automatically shut down the remote machines, and you stop paying!
+
+Check out the autoscalers getting started video below.
+
+[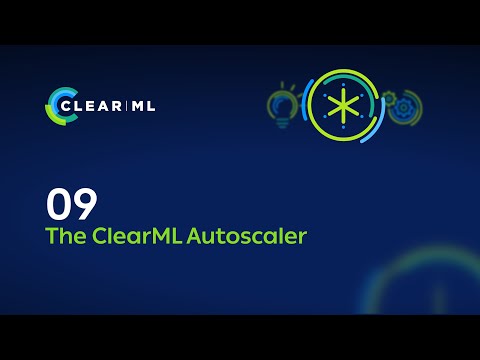](https://youtu.be/j4XVMAaUt3E)
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/comet_logging_integration.md b/yolov8/docs/yolov5/tutorials/comet_logging_integration.md
new file mode 100644
index 0000000000000000000000000000000000000000..263f14689891830e1c8ac682875c9b8f9ec71ecf
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/comet_logging_integration.md
@@ -0,0 +1,264 @@
+---
+comments: true
+description: Learn how to use YOLOv5 with Comet, a tool for logging and visualizing machine learning model metrics in real-time. Install, log and analyze seamlessly.
+keywords: object detection, YOLOv5, Comet, model metrics, deep learning, image classification, Colab notebook, machine learning, datasets, hyperparameters tracking, training script, checkpoint
+---
+
+
+
+# YOLOv5 with Comet
+
+This guide will cover how to use YOLOv5 with [Comet](https://bit.ly/yolov5-readme-comet2)
+
+# About Comet
+
+Comet builds tools that help data scientists, engineers, and team leaders accelerate and optimize machine learning and deep learning models.
+
+Track and visualize model metrics in real time, save your hyperparameters, datasets, and model checkpoints, and visualize your model predictions with [Comet Custom Panels](https://www.comet.com/docs/v2/guides/comet-dashboard/code-panels/about-panels/?utm_source=yolov5&utm_medium=partner&utm_campaign=partner_yolov5_2022&utm_content=github)!
+Comet makes sure you never lose track of your work and makes it easy to share results and collaborate across teams of all sizes!
+
+# Getting Started
+
+## Install Comet
+
+```shell
+pip install comet_ml
+```
+
+## Configure Comet Credentials
+
+There are two ways to configure Comet with YOLOv5.
+
+You can either set your credentials through environment variables
+
+**Environment Variables**
+
+```shell
+export COMET_API_KEY=
+export COMET_PROJECT_NAME= # This will default to 'yolov5'
+```
+
+Or create a `.comet.config` file in your working directory and set your credentials there.
+
+**Comet Configuration File**
+
+```
+[comet]
+api_key=
+project_name= # This will default to 'yolov5'
+```
+
+## Run the Training Script
+
+```shell
+# Train YOLOv5s on COCO128 for 5 epochs
+python train.py --img 640 --batch 16 --epochs 5 --data coco128.yaml --weights yolov5s.pt
+```
+
+That's it! Comet will automatically log your hyperparameters, command line arguments, training and validation metrics. You can visualize and analyze your runs in the Comet UI
+
+
+
+# Try out an Example!
+
+Check out an example of a [completed run here](https://www.comet.com/examples/comet-example-yolov5/a0e29e0e9b984e4a822db2a62d0cb357?experiment-tab=chart&showOutliers=true&smoothing=0&transformY=smoothing&xAxis=step&utm_source=yolov5&utm_medium=partner&utm_campaign=partner_yolov5_2022&utm_content=github)
+
+Or better yet, try it out yourself in this Colab Notebook
+
+[](https://colab.research.google.com/drive/1RG0WOQyxlDlo5Km8GogJpIEJlg_5lyYO?usp=sharing)
+
+# Log automatically
+
+By default, Comet will log the following items
+
+## Metrics
+
+- Box Loss, Object Loss, Classification Loss for the training and validation data
+- mAP_0.5, mAP_0.5:0.95 metrics for the validation data.
+- Precision and Recall for the validation data
+
+## Parameters
+
+- Model Hyperparameters
+- All parameters passed through the command line options
+
+## Visualizations
+
+- Confusion Matrix of the model predictions on the validation data
+- Plots for the PR and F1 curves across all classes
+- Correlogram of the Class Labels
+
+# Configure Comet Logging
+
+Comet can be configured to log additional data either through command line flags passed to the training script
+or through environment variables.
+
+```shell
+export COMET_MODE=online # Set whether to run Comet in 'online' or 'offline' mode. Defaults to online
+export COMET_MODEL_NAME= #Set the name for the saved model. Defaults to yolov5
+export COMET_LOG_CONFUSION_MATRIX=false # Set to disable logging a Comet Confusion Matrix. Defaults to true
+export COMET_MAX_IMAGE_UPLOADS= # Controls how many total image predictions to log to Comet. Defaults to 100.
+export COMET_LOG_PER_CLASS_METRICS=true # Set to log evaluation metrics for each detected class at the end of training. Defaults to false
+export COMET_DEFAULT_CHECKPOINT_FILENAME= # Set this if you would like to resume training from a different checkpoint. Defaults to 'last.pt'
+export COMET_LOG_BATCH_LEVEL_METRICS=true # Set this if you would like to log training metrics at the batch level. Defaults to false.
+export COMET_LOG_PREDICTIONS=true # Set this to false to disable logging model predictions
+```
+
+## Logging Checkpoints with Comet
+
+Logging Models to Comet is disabled by default. To enable it, pass the `save-period` argument to the training script. This will save the
+logged checkpoints to Comet based on the interval value provided by `save-period`
+
+```shell
+python train.py \
+--img 640 \
+--batch 16 \
+--epochs 5 \
+--data coco128.yaml \
+--weights yolov5s.pt \
+--save-period 1
+```
+
+## Logging Model Predictions
+
+By default, model predictions (images, ground truth labels and bounding boxes) will be logged to Comet.
+
+You can control the frequency of logged predictions and the associated images by passing the `bbox_interval` command line argument. Predictions can be visualized using Comet's Object Detection Custom Panel. This frequency corresponds to every Nth batch of data per epoch. In the example below, we are logging every 2nd batch of data for each epoch.
+
+**Note:** The YOLOv5 validation dataloader will default to a batch size of 32, so you will have to set the logging frequency accordingly.
+
+Here is an [example project using the Panel](https://www.comet.com/examples/comet-example-yolov5?shareable=YcwMiJaZSXfcEXpGOHDD12vA1&utm_source=yolov5&utm_medium=partner&utm_campaign=partner_yolov5_2022&utm_content=github)
+
+```shell
+python train.py \
+--img 640 \
+--batch 16 \
+--epochs 5 \
+--data coco128.yaml \
+--weights yolov5s.pt \
+--bbox_interval 2
+```
+
+### Controlling the number of Prediction Images logged to Comet
+
+When logging predictions from YOLOv5, Comet will log the images associated with each set of predictions. By default a maximum of 100 validation images are logged. You can increase or decrease this number using the `COMET_MAX_IMAGE_UPLOADS` environment variable.
+
+```shell
+env COMET_MAX_IMAGE_UPLOADS=200 python train.py \
+--img 640 \
+--batch 16 \
+--epochs 5 \
+--data coco128.yaml \
+--weights yolov5s.pt \
+--bbox_interval 1
+```
+
+### Logging Class Level Metrics
+
+Use the `COMET_LOG_PER_CLASS_METRICS` environment variable to log mAP, precision, recall, f1 for each class.
+
+```shell
+env COMET_LOG_PER_CLASS_METRICS=true python train.py \
+--img 640 \
+--batch 16 \
+--epochs 5 \
+--data coco128.yaml \
+--weights yolov5s.pt
+```
+
+## Uploading a Dataset to Comet Artifacts
+
+If you would like to store your data using [Comet Artifacts](https://www.comet.com/docs/v2/guides/data-management/using-artifacts/#learn-more?utm_source=yolov5&utm_medium=partner&utm_campaign=partner_yolov5_2022&utm_content=github), you can do so using the `upload_dataset` flag.
+
+The dataset be organized in the way described in the [YOLOv5 documentation](train_custom_data.md). The dataset config `yaml` file must follow the same format as that of the `coco128.yaml` file.
+
+```shell
+python train.py \
+--img 640 \
+--batch 16 \
+--epochs 5 \
+--data coco128.yaml \
+--weights yolov5s.pt \
+--upload_dataset
+```
+
+You can find the uploaded dataset in the Artifacts tab in your Comet Workspace
+
+
+You can preview the data directly in the Comet UI.
+
+
+Artifacts are versioned and also support adding metadata about the dataset. Comet will automatically log the metadata from your dataset `yaml` file
+
+
+### Using a saved Artifact
+
+If you would like to use a dataset from Comet Artifacts, set the `path` variable in your dataset `yaml` file to point to the following Artifact resource URL.
+
+```
+# contents of artifact.yaml file
+path: "comet:///:"
+```
+
+Then pass this file to your training script in the following way
+
+```shell
+python train.py \
+--img 640 \
+--batch 16 \
+--epochs 5 \
+--data artifact.yaml \
+--weights yolov5s.pt
+```
+
+Artifacts also allow you to track the lineage of data as it flows through your Experimentation workflow. Here you can see a graph that shows you all the experiments that have used your uploaded dataset.
+
+
+## Resuming a Training Run
+
+If your training run is interrupted for any reason, e.g. disrupted internet connection, you can resume the run using the `resume` flag and the Comet Run Path.
+
+The Run Path has the following format `comet:////`.
+
+This will restore the run to its state before the interruption, which includes restoring the model from a checkpoint, restoring all hyperparameters and training arguments and downloading Comet dataset Artifacts if they were used in the original run. The resumed run will continue logging to the existing Experiment in the Comet UI
+
+```shell
+python train.py \
+--resume "comet://"
+```
+
+## Hyperparameter Search with the Comet Optimizer
+
+YOLOv5 is also integrated with Comet's Optimizer, making is simple to visualize hyperparameter sweeps in the Comet UI.
+
+### Configuring an Optimizer Sweep
+
+To configure the Comet Optimizer, you will have to create a JSON file with the information about the sweep. An example file has been provided in `utils/loggers/comet/optimizer_config.json`
+
+```shell
+python utils/loggers/comet/hpo.py \
+ --comet_optimizer_config "utils/loggers/comet/optimizer_config.json"
+```
+
+The `hpo.py` script accepts the same arguments as `train.py`. If you wish to pass additional arguments to your sweep simply add them after
+the script.
+
+```shell
+python utils/loggers/comet/hpo.py \
+ --comet_optimizer_config "utils/loggers/comet/optimizer_config.json" \
+ --save-period 1 \
+ --bbox_interval 1
+```
+
+### Running a Sweep in Parallel
+
+```shell
+comet optimizer -j utils/loggers/comet/hpo.py \
+ utils/loggers/comet/optimizer_config.json"
+```
+
+### Visualizing Results
+
+Comet provides a number of ways to visualize the results of your sweep. Take a look at a [project with a completed sweep here](https://www.comet.com/examples/comet-example-yolov5/view/PrlArHGuuhDTKC1UuBmTtOSXD/panels?utm_source=yolov5&utm_medium=partner&utm_campaign=partner_yolov5_2022&utm_content=github)
+
+
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/hyperparameter_evolution.md b/yolov8/docs/yolov5/tutorials/hyperparameter_evolution.md
new file mode 100644
index 0000000000000000000000000000000000000000..8134b36e476e7462b0d1fda1819dd84a2829e495
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/hyperparameter_evolution.md
@@ -0,0 +1,166 @@
+---
+comments: true
+description: Learn to find optimum YOLOv5 hyperparameters via **evolution**. A guide to learn hyperparameter tuning with Genetic Algorithms.
+keywords: YOLOv5, Hyperparameter Evolution, Genetic Algorithm, Hyperparameter Optimization, Fitness, Evolve, Visualize
+---
+
+📚 This guide explains **hyperparameter evolution** for YOLOv5 🚀. Hyperparameter evolution is a method of [Hyperparameter Optimization](https://en.wikipedia.org/wiki/Hyperparameter_optimization) using a [Genetic Algorithm](https://en.wikipedia.org/wiki/Genetic_algorithm) (GA) for optimization. UPDATED 25 September 2022.
+
+Hyperparameters in ML control various aspects of training, and finding optimal values for them can be a challenge. Traditional methods like grid searches can quickly become intractable due to 1) the high dimensional search space 2) unknown correlations among the dimensions, and 3) expensive nature of evaluating the fitness at each point, making GA a suitable candidate for hyperparameter searches.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## 1. Initialize Hyperparameters
+
+YOLOv5 has about 30 hyperparameters used for various training settings. These are defined in `*.yaml` files in the `/data/hyps` directory. Better initial guesses will produce better final results, so it is important to initialize these values properly before evolving. If in doubt, simply use the default values, which are optimized for YOLOv5 COCO training from scratch.
+
+```yaml
+# YOLOv5 🚀 by Ultralytics, AGPL-3.0 license
+# Hyperparameters for low-augmentation COCO training from scratch
+# python train.py --batch 64 --cfg yolov5n6.yaml --weights '' --data coco.yaml --img 640 --epochs 300 --linear
+# See tutorials for hyperparameter evolution https://github.com/ultralytics/yolov5#tutorials
+
+lr0: 0.01 # initial learning rate (SGD=1E-2, Adam=1E-3)
+lrf: 0.01 # final OneCycleLR learning rate (lr0 * lrf)
+momentum: 0.937 # SGD momentum/Adam beta1
+weight_decay: 0.0005 # optimizer weight decay 5e-4
+warmup_epochs: 3.0 # warmup epochs (fractions ok)
+warmup_momentum: 0.8 # warmup initial momentum
+warmup_bias_lr: 0.1 # warmup initial bias lr
+box: 0.05 # box loss gain
+cls: 0.5 # cls loss gain
+cls_pw: 1.0 # cls BCELoss positive_weight
+obj: 1.0 # obj loss gain (scale with pixels)
+obj_pw: 1.0 # obj BCELoss positive_weight
+iou_t: 0.20 # IoU training threshold
+anchor_t: 4.0 # anchor-multiple threshold
+# anchors: 3 # anchors per output layer (0 to ignore)
+fl_gamma: 0.0 # focal loss gamma (efficientDet default gamma=1.5)
+hsv_h: 0.015 # image HSV-Hue augmentation (fraction)
+hsv_s: 0.7 # image HSV-Saturation augmentation (fraction)
+hsv_v: 0.4 # image HSV-Value augmentation (fraction)
+degrees: 0.0 # image rotation (+/- deg)
+translate: 0.1 # image translation (+/- fraction)
+scale: 0.5 # image scale (+/- gain)
+shear: 0.0 # image shear (+/- deg)
+perspective: 0.0 # image perspective (+/- fraction), range 0-0.001
+flipud: 0.0 # image flip up-down (probability)
+fliplr: 0.5 # image flip left-right (probability)
+mosaic: 1.0 # image mosaic (probability)
+mixup: 0.0 # image mixup (probability)
+copy_paste: 0.0 # segment copy-paste (probability)
+```
+
+## 2. Define Fitness
+
+Fitness is the value we seek to maximize. In YOLOv5 we define a default fitness function as a weighted combination of metrics: `mAP@0.5` contributes 10% of the weight and `mAP@0.5:0.95` contributes the remaining 90%, with [Precision `P` and Recall `R`](https://en.wikipedia.org/wiki/Precision_and_recall) absent. You may adjust these as you see fit or use the default fitness definition in utils/metrics.py (recommended).
+
+```python
+def fitness(x):
+ # Model fitness as a weighted combination of metrics
+ w = [0.0, 0.0, 0.1, 0.9] # weights for [P, R, mAP@0.5, mAP@0.5:0.95]
+ return (x[:, :4] * w).sum(1)
+```
+
+## 3. Evolve
+
+Evolution is performed about a base scenario which we seek to improve upon. The base scenario in this example is finetuning COCO128 for 10 epochs using pretrained YOLOv5s. The base scenario training command is:
+
+```bash
+python train.py --epochs 10 --data coco128.yaml --weights yolov5s.pt --cache
+```
+
+To evolve hyperparameters **specific to this scenario**, starting from our initial values defined in **Section 1.**, and maximizing the fitness defined in **Section 2.**, append `--evolve`:
+
+```bash
+# Single-GPU
+python train.py --epochs 10 --data coco128.yaml --weights yolov5s.pt --cache --evolve
+
+# Multi-GPU
+for i in 0 1 2 3 4 5 6 7; do
+ sleep $(expr 30 \* $i) && # 30-second delay (optional)
+ echo 'Starting GPU '$i'...' &&
+ nohup python train.py --epochs 10 --data coco128.yaml --weights yolov5s.pt --cache --device $i --evolve > evolve_gpu_$i.log &
+done
+
+# Multi-GPU bash-while (not recommended)
+for i in 0 1 2 3 4 5 6 7; do
+ sleep $(expr 30 \* $i) && # 30-second delay (optional)
+ echo 'Starting GPU '$i'...' &&
+ "$(while true; do nohup python train.py... --device $i --evolve 1 > evolve_gpu_$i.log; done)" &
+done
+```
+
+The default evolution settings will run the base scenario 300 times, i.e. for 300 generations. You can modify generations via the `--evolve` argument, i.e. `python train.py --evolve 1000`.
+https://github.com/ultralytics/yolov5/blob/6a3ee7cf03efb17fbffde0e68b1a854e80fe3213/train.py#L608
+
+The main genetic operators are **crossover** and **mutation**. In this work mutation is used, with an 80% probability and a 0.04 variance to create new offspring based on a combination of the best parents from all previous generations. Results are logged to `runs/evolve/exp/evolve.csv`, and the highest fitness offspring is saved every generation as `runs/evolve/hyp_evolved.yaml`:
+
+```yaml
+# YOLOv5 Hyperparameter Evolution Results
+# Best generation: 287
+# Last generation: 300
+# metrics/precision, metrics/recall, metrics/mAP_0.5, metrics/mAP_0.5:0.95, val/box_loss, val/obj_loss, val/cls_loss
+# 0.54634, 0.55625, 0.58201, 0.33665, 0.056451, 0.042892, 0.013441
+
+lr0: 0.01 # initial learning rate (SGD=1E-2, Adam=1E-3)
+lrf: 0.2 # final OneCycleLR learning rate (lr0 * lrf)
+momentum: 0.937 # SGD momentum/Adam beta1
+weight_decay: 0.0005 # optimizer weight decay 5e-4
+warmup_epochs: 3.0 # warmup epochs (fractions ok)
+warmup_momentum: 0.8 # warmup initial momentum
+warmup_bias_lr: 0.1 # warmup initial bias lr
+box: 0.05 # box loss gain
+cls: 0.5 # cls loss gain
+cls_pw: 1.0 # cls BCELoss positive_weight
+obj: 1.0 # obj loss gain (scale with pixels)
+obj_pw: 1.0 # obj BCELoss positive_weight
+iou_t: 0.20 # IoU training threshold
+anchor_t: 4.0 # anchor-multiple threshold
+# anchors: 3 # anchors per output layer (0 to ignore)
+fl_gamma: 0.0 # focal loss gamma (efficientDet default gamma=1.5)
+hsv_h: 0.015 # image HSV-Hue augmentation (fraction)
+hsv_s: 0.7 # image HSV-Saturation augmentation (fraction)
+hsv_v: 0.4 # image HSV-Value augmentation (fraction)
+degrees: 0.0 # image rotation (+/- deg)
+translate: 0.1 # image translation (+/- fraction)
+scale: 0.5 # image scale (+/- gain)
+shear: 0.0 # image shear (+/- deg)
+perspective: 0.0 # image perspective (+/- fraction), range 0-0.001
+flipud: 0.0 # image flip up-down (probability)
+fliplr: 0.5 # image flip left-right (probability)
+mosaic: 1.0 # image mosaic (probability)
+mixup: 0.0 # image mixup (probability)
+copy_paste: 0.0 # segment copy-paste (probability)
+```
+
+We recommend a minimum of 300 generations of evolution for best results. Note that **evolution is generally expensive and time-consuming**, as the base scenario is trained hundreds of times, possibly requiring hundreds or thousands of GPU hours.
+
+## 4. Visualize
+
+`evolve.csv` is plotted as `evolve.png` by `utils.plots.plot_evolve()` after evolution finishes with one subplot per hyperparameter showing fitness (y-axis) vs hyperparameter values (x-axis). Yellow indicates higher concentrations. Vertical distributions indicate that a parameter has been disabled and does not mutate. This is user selectable in the `meta` dictionary in train.py, and is useful for fixing parameters and preventing them from evolving.
+
+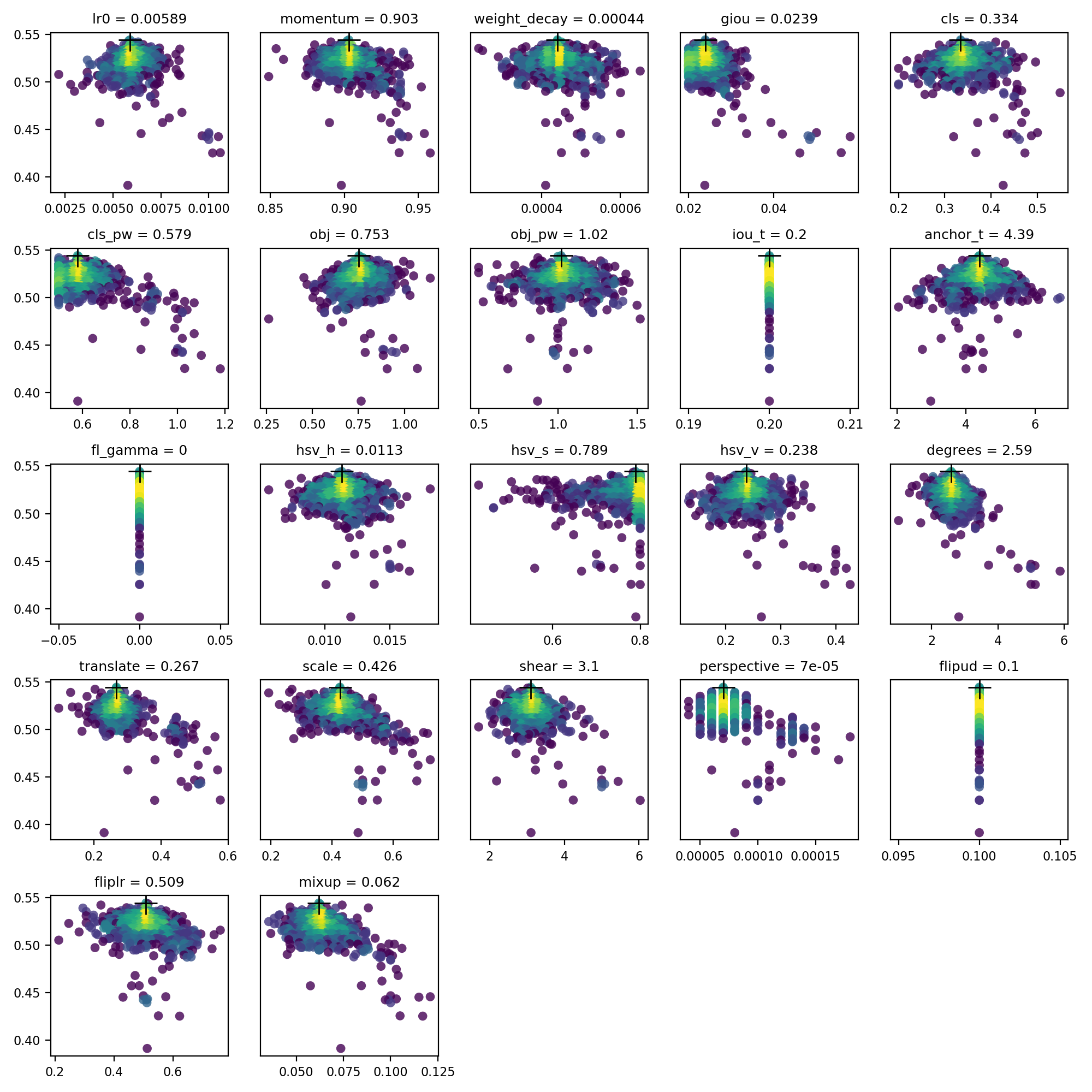
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/model_ensembling.md b/yolov8/docs/yolov5/tutorials/model_ensembling.md
new file mode 100644
index 0000000000000000000000000000000000000000..3e13435048ecf8b133cca0dad677432b8c1a5012
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/model_ensembling.md
@@ -0,0 +1,147 @@
+---
+comments: true
+description: Learn how to ensemble YOLOv5 models for improved mAP and Recall! Clone the repo, install requirements, and start testing and inference.
+keywords: YOLOv5, object detection, ensemble learning, mAP, Recall
+---
+
+📚 This guide explains how to use YOLOv5 🚀 **model ensembling** during testing and inference for improved mAP and Recall.
+UPDATED 25 September 2022.
+
+From [https://en.wikipedia.org/wiki/Ensemble_learning](https://en.wikipedia.org/wiki/Ensemble_learning):
+> Ensemble modeling is a process where multiple diverse models are created to predict an outcome, either by using many different modeling algorithms or using different training data sets. The ensemble model then aggregates the prediction of each base model and results in once final prediction for the unseen data. The motivation for using ensemble models is to reduce the generalization error of the prediction. As long as the base models are diverse and independent, the prediction error of the model decreases when the ensemble approach is used. The approach seeks the wisdom of crowds in making a prediction. Even though the ensemble model has multiple base models within the model, it acts and performs as a single model.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Test Normally
+
+Before ensembling we want to establish the baseline performance of a single model. This command tests YOLOv5x on COCO val2017 at image size 640 pixels. `yolov5x.pt` is the largest and most accurate model available. Other options are `yolov5s.pt`, `yolov5m.pt` and `yolov5l.pt`, or you own checkpoint from training a custom dataset `./weights/best.pt`. For details on all available models please see our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints).
+
+```bash
+python val.py --weights yolov5x.pt --data coco.yaml --img 640 --half
+```
+
+Output:
+
+```shell
+val: data=./data/coco.yaml, weights=['yolov5x.pt'], batch_size=32, imgsz=640, conf_thres=0.001, iou_thres=0.65, task=val, device=, single_cls=False, augment=False, verbose=False, save_txt=False, save_hybrid=False, save_conf=False, save_json=True, project=runs/val, name=exp, exist_ok=False, half=True
+YOLOv5 🚀 v5.0-267-g6a3ee7c torch 1.9.0+cu102 CUDA:0 (Tesla P100-PCIE-16GB, 16280.875MB)
+
+Fusing layers...
+Model Summary: 476 layers, 87730285 parameters, 0 gradients
+
+val: Scanning '../datasets/coco/val2017' images and labels...4952 found, 48 missing, 0 empty, 0 corrupted: 100% 5000/5000 [00:01<00:00, 2846.03it/s]
+val: New cache created: ../datasets/coco/val2017.cache
+ Class Images Labels P R mAP@.5 mAP@.5:.95: 100% 157/157 [02:30<00:00, 1.05it/s]
+ all 5000 36335 0.746 0.626 0.68 0.49
+Speed: 0.1ms pre-process, 22.4ms inference, 1.4ms NMS per image at shape (32, 3, 640, 640) # <--- baseline speed
+
+Evaluating pycocotools mAP... saving runs/val/exp/yolov5x_predictions.json...
+...
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.504 # <--- baseline mAP
+ Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.688
+ Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.546
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.351
+ Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.551
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.644
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.382
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.628
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.681 # <--- baseline mAR
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.524
+ Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.735
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.826
+```
+
+## Ensemble Test
+
+Multiple pretrained models may be ensembled together at test and inference time by simply appending extra models to the `--weights` argument in any existing val.py or detect.py command. This example tests an ensemble of 2 models together:
+
+- YOLOv5x
+- YOLOv5l6
+
+```bash
+python val.py --weights yolov5x.pt yolov5l6.pt --data coco.yaml --img 640 --half
+```
+
+Output:
+
+```shell
+val: data=./data/coco.yaml, weights=['yolov5x.pt', 'yolov5l6.pt'], batch_size=32, imgsz=640, conf_thres=0.001, iou_thres=0.6, task=val, device=, single_cls=False, augment=False, verbose=False, save_txt=False, save_hybrid=False, save_conf=False, save_json=True, project=runs/val, name=exp, exist_ok=False, half=True
+YOLOv5 🚀 v5.0-267-g6a3ee7c torch 1.9.0+cu102 CUDA:0 (Tesla P100-PCIE-16GB, 16280.875MB)
+
+Fusing layers...
+Model Summary: 476 layers, 87730285 parameters, 0 gradients # Model 1
+Fusing layers...
+Model Summary: 501 layers, 77218620 parameters, 0 gradients # Model 2
+Ensemble created with ['yolov5x.pt', 'yolov5l6.pt'] # Ensemble notice
+
+val: Scanning '../datasets/coco/val2017.cache' images and labels... 4952 found, 48 missing, 0 empty, 0 corrupted: 100% 5000/5000 [00:00<00:00, 49695545.02it/s]
+ Class Images Labels P R mAP@.5 mAP@.5:.95: 100% 157/157 [03:58<00:00, 1.52s/it]
+ all 5000 36335 0.747 0.637 0.692 0.502
+Speed: 0.1ms pre-process, 39.5ms inference, 2.0ms NMS per image at shape (32, 3, 640, 640) # <--- ensemble speed
+
+Evaluating pycocotools mAP... saving runs/val/exp3/yolov5x_predictions.json...
+...
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.515 # <--- ensemble mAP
+ Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.699
+ Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.557
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.356
+ Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.563
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.668
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.387
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.638
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.689 # <--- ensemble mAR
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.526
+ Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.743
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.844
+```
+
+## Ensemble Inference
+
+Append extra models to the `--weights` argument to run ensemble inference:
+
+```bash
+python detect.py --weights yolov5x.pt yolov5l6.pt --img 640 --source data/images
+```
+
+Output:
+
+```bash
+detect: weights=['yolov5x.pt', 'yolov5l6.pt'], source=data/images, imgsz=640, conf_thres=0.25, iou_thres=0.45, max_det=1000, device=, view_img=False, save_txt=False, save_conf=False, save_crop=False, nosave=False, classes=None, agnostic_nms=False, augment=False, update=False, project=runs/detect, name=exp, exist_ok=False, line_width=3, hide_labels=False, hide_conf=False, half=False
+YOLOv5 🚀 v5.0-267-g6a3ee7c torch 1.9.0+cu102 CUDA:0 (Tesla P100-PCIE-16GB, 16280.875MB)
+
+Fusing layers...
+Model Summary: 476 layers, 87730285 parameters, 0 gradients
+Fusing layers...
+Model Summary: 501 layers, 77218620 parameters, 0 gradients
+Ensemble created with ['yolov5x.pt', 'yolov5l6.pt']
+
+image 1/2 /content/yolov5/data/images/bus.jpg: 640x512 4 persons, 1 bus, 1 tie, Done. (0.063s)
+image 2/2 /content/yolov5/data/images/zidane.jpg: 384x640 3 persons, 2 ties, Done. (0.056s)
+Results saved to runs/detect/exp2
+Done. (0.223s)
+```
+
+
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/model_export.md b/yolov8/docs/yolov5/tutorials/model_export.md
new file mode 100644
index 0000000000000000000000000000000000000000..53afd0152039e157efb6a1dc235a69ef3a423490
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/model_export.md
@@ -0,0 +1,246 @@
+---
+comments: true
+description: Export YOLOv5 models to TFLite, ONNX, CoreML, and TensorRT formats. Achieve up to 5x GPU speedup using TensorRT. Benchmarks included.
+keywords: YOLOv5, object detection, export, ONNX, CoreML, TensorFlow, TensorRT, OpenVINO
+---
+
+# TFLite, ONNX, CoreML, TensorRT Export
+
+📚 This guide explains how to export a trained YOLOv5 🚀 model from PyTorch to ONNX and TorchScript formats.
+UPDATED 8 December 2022.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+For [TensorRT](https://developer.nvidia.com/tensorrt) export example (requires GPU) see our Colab [notebook](https://colab.research.google.com/github/ultralytics/yolov5/blob/master/tutorial.ipynb#scrollTo=VTRwsvA9u7ln&line=2&uniqifier=1) appendix section.
+
+## Formats
+
+YOLOv5 inference is officially supported in 11 formats:
+
+💡 ProTip: Export to ONNX or OpenVINO for up to 3x CPU speedup. See [CPU Benchmarks](https://github.com/ultralytics/yolov5/pull/6613).
+💡 ProTip: Export to TensorRT for up to 5x GPU speedup. See [GPU Benchmarks](https://github.com/ultralytics/yolov5/pull/6963).
+
+| Format | `export.py --include` | Model |
+|:---------------------------------------------------------------------------|:----------------------|:--------------------------|
+| [PyTorch](https://pytorch.org/) | - | `yolov5s.pt` |
+| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov5s.torchscript` |
+| [ONNX](https://onnx.ai/) | `onnx` | `yolov5s.onnx` |
+| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov5s_openvino_model/` |
+| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov5s.engine` |
+| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov5s.mlmodel` |
+| [TensorFlow SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov5s_saved_model/` |
+| [TensorFlow GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov5s.pb` |
+| [TensorFlow Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov5s.tflite` |
+| [TensorFlow Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov5s_edgetpu.tflite` |
+| [TensorFlow.js](https://www.tensorflow.org/js) | `tfjs` | `yolov5s_web_model/` |
+| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov5s_paddle_model/` |
+
+## Benchmarks
+
+Benchmarks below run on a Colab Pro with the YOLOv5 tutorial notebook
. To reproduce:
+
+```bash
+python benchmarks.py --weights yolov5s.pt --imgsz 640 --device 0
+```
+
+### Colab Pro V100 GPU
+
+```
+benchmarks: weights=/content/yolov5/yolov5s.pt, imgsz=640, batch_size=1, data=/content/yolov5/data/coco128.yaml, device=0, half=False, test=False
+Checking setup...
+YOLOv5 🚀 v6.1-135-g7926afc torch 1.10.0+cu111 CUDA:0 (Tesla V100-SXM2-16GB, 16160MiB)
+Setup complete ✅ (8 CPUs, 51.0 GB RAM, 46.7/166.8 GB disk)
+
+Benchmarks complete (458.07s)
+ Format mAP@0.5:0.95 Inference time (ms)
+0 PyTorch 0.4623 10.19
+1 TorchScript 0.4623 6.85
+2 ONNX 0.4623 14.63
+3 OpenVINO NaN NaN
+4 TensorRT 0.4617 1.89
+5 CoreML NaN NaN
+6 TensorFlow SavedModel 0.4623 21.28
+7 TensorFlow GraphDef 0.4623 21.22
+8 TensorFlow Lite NaN NaN
+9 TensorFlow Edge TPU NaN NaN
+10 TensorFlow.js NaN NaN
+```
+
+### Colab Pro CPU
+
+```
+benchmarks: weights=/content/yolov5/yolov5s.pt, imgsz=640, batch_size=1, data=/content/yolov5/data/coco128.yaml, device=cpu, half=False, test=False
+Checking setup...
+YOLOv5 🚀 v6.1-135-g7926afc torch 1.10.0+cu111 CPU
+Setup complete ✅ (8 CPUs, 51.0 GB RAM, 41.5/166.8 GB disk)
+
+Benchmarks complete (241.20s)
+ Format mAP@0.5:0.95 Inference time (ms)
+0 PyTorch 0.4623 127.61
+1 TorchScript 0.4623 131.23
+2 ONNX 0.4623 69.34
+3 OpenVINO 0.4623 66.52
+4 TensorRT NaN NaN
+5 CoreML NaN NaN
+6 TensorFlow SavedModel 0.4623 123.79
+7 TensorFlow GraphDef 0.4623 121.57
+8 TensorFlow Lite 0.4623 316.61
+9 TensorFlow Edge TPU NaN NaN
+10 TensorFlow.js NaN NaN
+```
+
+## Export a Trained YOLOv5 Model
+
+This command exports a pretrained YOLOv5s model to TorchScript and ONNX formats. `yolov5s.pt` is the 'small' model, the second-smallest model available. Other options are `yolov5n.pt`, `yolov5m.pt`, `yolov5l.pt` and `yolov5x.pt`, along with their P6 counterparts i.e. `yolov5s6.pt` or you own custom training checkpoint i.e. `runs/exp/weights/best.pt`. For details on all available models please see our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints).
+
+```bash
+python export.py --weights yolov5s.pt --include torchscript onnx
+```
+
+💡 ProTip: Add `--half` to export models at FP16 half precision for smaller file sizes
+
+Output:
+
+```bash
+export: data=data/coco128.yaml, weights=['yolov5s.pt'], imgsz=[640, 640], batch_size=1, device=cpu, half=False, inplace=False, train=False, keras=False, optimize=False, int8=False, dynamic=False, simplify=False, opset=12, verbose=False, workspace=4, nms=False, agnostic_nms=False, topk_per_class=100, topk_all=100, iou_thres=0.45, conf_thres=0.25, include=['torchscript', 'onnx']
+YOLOv5 🚀 v6.2-104-ge3e5122 Python-3.7.13 torch-1.12.1+cu113 CPU
+
+Downloading https://github.com/ultralytics/yolov5/releases/download/v6.2/yolov5s.pt to yolov5s.pt...
+100% 14.1M/14.1M [00:00<00:00, 274MB/s]
+
+Fusing layers...
+YOLOv5s summary: 213 layers, 7225885 parameters, 0 gradients
+
+PyTorch: starting from yolov5s.pt with output shape (1, 25200, 85) (14.1 MB)
+
+TorchScript: starting export with torch 1.12.1+cu113...
+TorchScript: export success ✅ 1.7s, saved as yolov5s.torchscript (28.1 MB)
+
+ONNX: starting export with onnx 1.12.0...
+ONNX: export success ✅ 2.3s, saved as yolov5s.onnx (28.0 MB)
+
+Export complete (5.5s)
+Results saved to /content/yolov5
+Detect: python detect.py --weights yolov5s.onnx
+Validate: python val.py --weights yolov5s.onnx
+PyTorch Hub: model = torch.hub.load('ultralytics/yolov5', 'custom', 'yolov5s.onnx')
+Visualize: https://netron.app/
+```
+
+The 3 exported models will be saved alongside the original PyTorch model:
+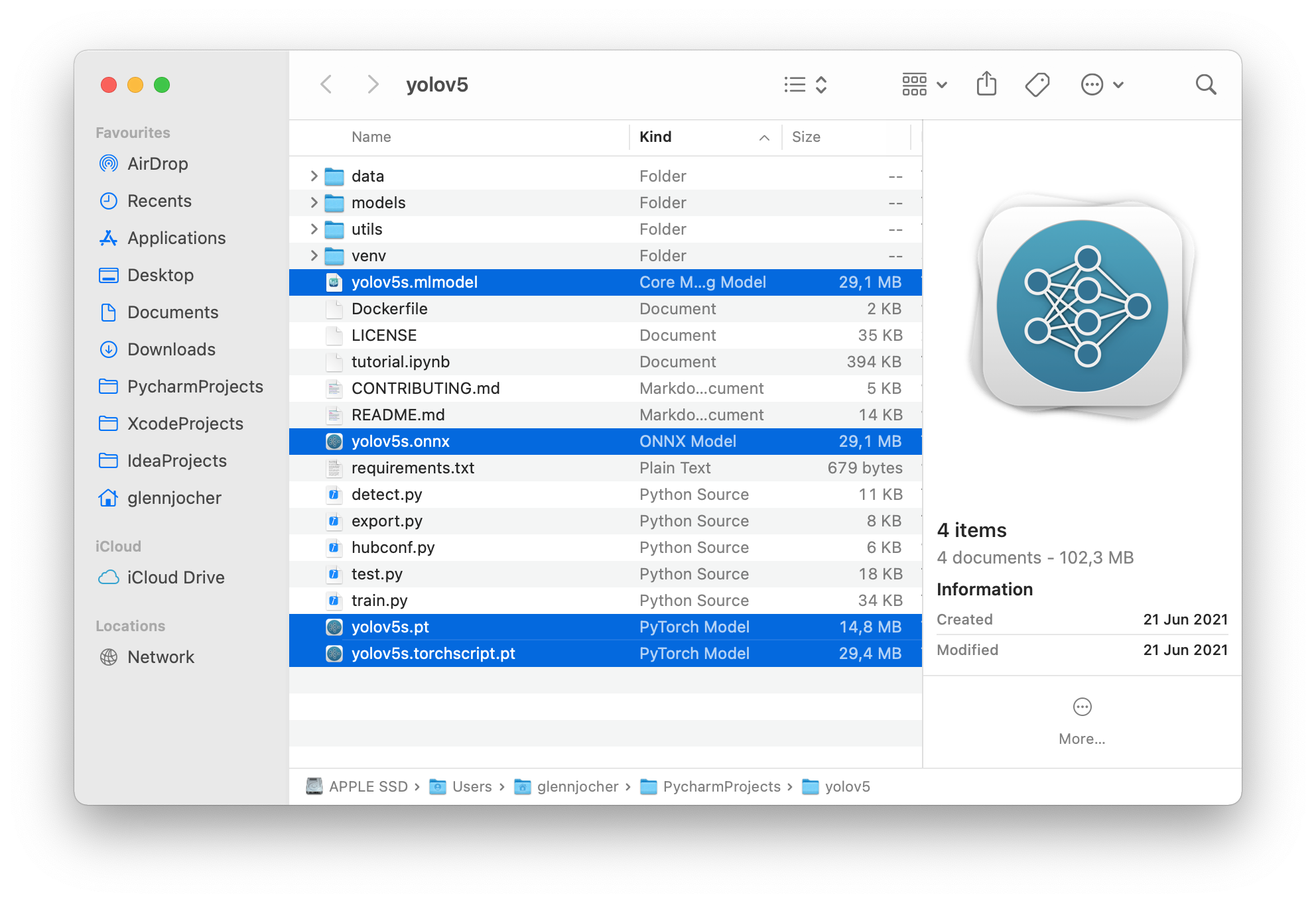
+
+[Netron Viewer](https://github.com/lutzroeder/netron) is recommended for visualizing exported models:
+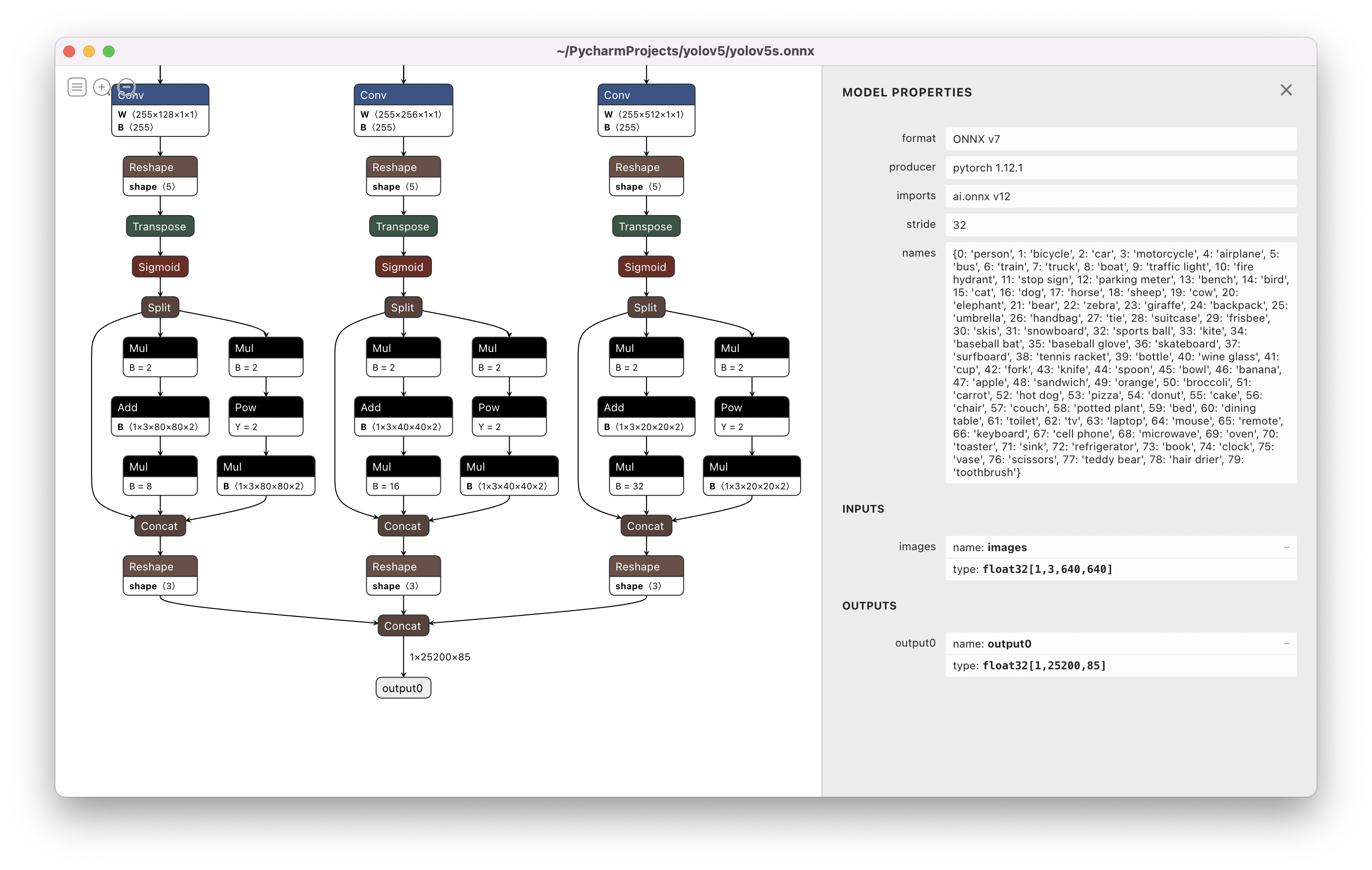
+
+## Exported Model Usage Examples
+
+`detect.py` runs inference on exported models:
+
+```bash
+python detect.py --weights yolov5s.pt # PyTorch
+ yolov5s.torchscript # TorchScript
+ yolov5s.onnx # ONNX Runtime or OpenCV DNN with --dnn
+ yolov5s_openvino_model # OpenVINO
+ yolov5s.engine # TensorRT
+ yolov5s.mlmodel # CoreML (macOS only)
+ yolov5s_saved_model # TensorFlow SavedModel
+ yolov5s.pb # TensorFlow GraphDef
+ yolov5s.tflite # TensorFlow Lite
+ yolov5s_edgetpu.tflite # TensorFlow Edge TPU
+ yolov5s_paddle_model # PaddlePaddle
+```
+
+`val.py` runs validation on exported models:
+
+```bash
+python val.py --weights yolov5s.pt # PyTorch
+ yolov5s.torchscript # TorchScript
+ yolov5s.onnx # ONNX Runtime or OpenCV DNN with --dnn
+ yolov5s_openvino_model # OpenVINO
+ yolov5s.engine # TensorRT
+ yolov5s.mlmodel # CoreML (macOS Only)
+ yolov5s_saved_model # TensorFlow SavedModel
+ yolov5s.pb # TensorFlow GraphDef
+ yolov5s.tflite # TensorFlow Lite
+ yolov5s_edgetpu.tflite # TensorFlow Edge TPU
+ yolov5s_paddle_model # PaddlePaddle
+```
+
+Use PyTorch Hub with exported YOLOv5 models:
+
+``` python
+import torch
+
+# Model
+model = torch.hub.load('ultralytics/yolov5', 'custom', 'yolov5s.pt')
+ 'yolov5s.torchscript ') # TorchScript
+ 'yolov5s.onnx') # ONNX Runtime
+ 'yolov5s_openvino_model') # OpenVINO
+ 'yolov5s.engine') # TensorRT
+ 'yolov5s.mlmodel') # CoreML (macOS Only)
+ 'yolov5s_saved_model') # TensorFlow SavedModel
+ 'yolov5s.pb') # TensorFlow GraphDef
+ 'yolov5s.tflite') # TensorFlow Lite
+ 'yolov5s_edgetpu.tflite') # TensorFlow Edge TPU
+ 'yolov5s_paddle_model') # PaddlePaddle
+
+# Images
+img = 'https://ultralytics.com/images/zidane.jpg' # or file, Path, PIL, OpenCV, numpy, list
+
+# Inference
+results = model(img)
+
+# Results
+results.print() # or .show(), .save(), .crop(), .pandas(), etc.
+```
+
+## OpenCV DNN inference
+
+OpenCV inference with ONNX models:
+
+```bash
+python export.py --weights yolov5s.pt --include onnx
+
+python detect.py --weights yolov5s.onnx --dnn # detect
+python val.py --weights yolov5s.onnx --dnn # validate
+```
+
+## C++ Inference
+
+YOLOv5 OpenCV DNN C++ inference on exported ONNX model examples:
+
+- [https://github.com/Hexmagic/ONNX-yolov5/blob/master/src/test.cpp](https://github.com/Hexmagic/ONNX-yolov5/blob/master/src/test.cpp)
+- [https://github.com/doleron/yolov5-opencv-cpp-python](https://github.com/doleron/yolov5-opencv-cpp-python)
+
+YOLOv5 OpenVINO C++ inference examples:
+
+- [https://github.com/dacquaviva/yolov5-openvino-cpp-python](https://github.com/dacquaviva/yolov5-openvino-cpp-python)
+- [https://github.com/UNeedCryDear/yolov5-seg-opencv-dnn-cpp](https://github.com/UNeedCryDear/yolov5-seg-opencv-dnn-cpp)
+
+## TensorFlow.js Web Browser Inference
+
+- [https://aukerul-shuvo.github.io/YOLOv5_TensorFlow-JS/](https://aukerul-shuvo.github.io/YOLOv5_TensorFlow-JS/)
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/model_pruning_and_sparsity.md b/yolov8/docs/yolov5/tutorials/model_pruning_and_sparsity.md
new file mode 100644
index 0000000000000000000000000000000000000000..25e4f8c300a364ad008007628799cfc738752829
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/model_pruning_and_sparsity.md
@@ -0,0 +1,110 @@
+---
+comments: true
+description: Learn how to apply pruning to your YOLOv5 models. See the before and after performance with an explanation of sparsity and more.
+keywords: YOLOv5, ultralytics, pruning, deep learning, computer vision, object detection, AI, tutorial
+---
+
+📚 This guide explains how to apply **pruning** to YOLOv5 🚀 models.
+UPDATED 25 September 2022.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Test Normally
+
+Before pruning we want to establish a baseline performance to compare to. This command tests YOLOv5x on COCO val2017 at image size 640 pixels. `yolov5x.pt` is the largest and most accurate model available. Other options are `yolov5s.pt`, `yolov5m.pt` and `yolov5l.pt`, or you own checkpoint from training a custom dataset `./weights/best.pt`. For details on all available models please see our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints).
+
+```bash
+python val.py --weights yolov5x.pt --data coco.yaml --img 640 --half
+```
+
+Output:
+
+```shell
+val: data=/content/yolov5/data/coco.yaml, weights=['yolov5x.pt'], batch_size=32, imgsz=640, conf_thres=0.001, iou_thres=0.65, task=val, device=, workers=8, single_cls=False, augment=False, verbose=False, save_txt=False, save_hybrid=False, save_conf=False, save_json=True, project=runs/val, name=exp, exist_ok=False, half=True, dnn=False
+YOLOv5 🚀 v6.0-224-g4c40933 torch 1.10.0+cu111 CUDA:0 (Tesla V100-SXM2-16GB, 16160MiB)
+
+Fusing layers...
+Model Summary: 444 layers, 86705005 parameters, 0 gradients
+val: Scanning '/content/datasets/coco/val2017.cache' images and labels... 4952 found, 48 missing, 0 empty, 0 corrupt: 100% 5000/5000 [00:00, ?it/s]
+ Class Images Labels P R mAP@.5 mAP@.5:.95: 100% 157/157 [01:12<00:00, 2.16it/s]
+ all 5000 36335 0.732 0.628 0.683 0.496
+Speed: 0.1ms pre-process, 5.2ms inference, 1.7ms NMS per image at shape (32, 3, 640, 640) # <--- base speed
+
+Evaluating pycocotools mAP... saving runs/val/exp2/yolov5x_predictions.json...
+...
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.507 # <--- base mAP
+ Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.689
+ Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.552
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.345
+ Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.559
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.652
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.381
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.630
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.682
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.526
+ Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.731
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.829
+Results saved to runs/val/exp
+```
+
+## Test YOLOv5x on COCO (0.30 sparsity)
+
+We repeat the above test with a pruned model by using the `torch_utils.prune()` command. We update `val.py` to prune YOLOv5x to 0.3 sparsity:
+
+
+
+30% pruned output:
+
+```bash
+val: data=/content/yolov5/data/coco.yaml, weights=['yolov5x.pt'], batch_size=32, imgsz=640, conf_thres=0.001, iou_thres=0.65, task=val, device=, workers=8, single_cls=False, augment=False, verbose=False, save_txt=False, save_hybrid=False, save_conf=False, save_json=True, project=runs/val, name=exp, exist_ok=False, half=True, dnn=False
+YOLOv5 🚀 v6.0-224-g4c40933 torch 1.10.0+cu111 CUDA:0 (Tesla V100-SXM2-16GB, 16160MiB)
+
+Fusing layers...
+Model Summary: 444 layers, 86705005 parameters, 0 gradients
+Pruning model... 0.3 global sparsity
+val: Scanning '/content/datasets/coco/val2017.cache' images and labels... 4952 found, 48 missing, 0 empty, 0 corrupt: 100% 5000/5000 [00:00, ?it/s]
+ Class Images Labels P R mAP@.5 mAP@.5:.95: 100% 157/157 [01:11<00:00, 2.19it/s]
+ all 5000 36335 0.724 0.614 0.671 0.478
+Speed: 0.1ms pre-process, 5.2ms inference, 1.7ms NMS per image at shape (32, 3, 640, 640) # <--- prune mAP
+
+Evaluating pycocotools mAP... saving runs/val/exp3/yolov5x_predictions.json...
+...
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.489 # <--- prune mAP
+ Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.677
+ Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.537
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.334
+ Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.542
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.635
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.370
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.612
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.664
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.496
+ Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.722
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.803
+Results saved to runs/val/exp3
+```
+
+In the results we can observe that we have achieved a **sparsity of 30%** in our model after pruning, which means that 30% of the model's weight parameters in `nn.Conv2d` layers are equal to 0. **Inference time is essentially unchanged**, while the model's **AP and AR scores a slightly reduced**.
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/multi_gpu_training.md b/yolov8/docs/yolov5/tutorials/multi_gpu_training.md
new file mode 100644
index 0000000000000000000000000000000000000000..24221db783ab90b0d28b555f57b35bb61804ec66
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/multi_gpu_training.md
@@ -0,0 +1,191 @@
+---
+comments: true
+description: Learn how to train your dataset on single or multiple machines using YOLOv5 on multiple GPUs. Use simple commands with DDP mode for faster performance.
+keywords: ultralytics, yolo, yolov5, multi-gpu, training, dataset, dataloader, data parallel, distributed data parallel, docker, pytorch
+---
+
+📚 This guide explains how to properly use **multiple** GPUs to train a dataset with YOLOv5 🚀 on single or multiple machine(s).
+UPDATED 25 December 2022.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+💡 ProTip! **Docker Image** is recommended for all Multi-GPU trainings. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+💡 ProTip! `torch.distributed.run` replaces `torch.distributed.launch` in **PyTorch>=1.9**. See [docs](https://pytorch.org/docs/stable/distributed.html) for details.
+
+## Training
+
+Select a pretrained model to start training from. Here we select [YOLOv5s](https://github.com/ultralytics/yolov5/blob/master/models/yolov5s.yaml), the smallest and fastest model available. See our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints) for a full comparison of all models. We will train this model with Multi-GPU on the [COCO](https://github.com/ultralytics/yolov5/blob/master/data/scripts/get_coco.sh) dataset.
+
+
+
+### Single GPU
+
+```bash
+python train.py --batch 64 --data coco.yaml --weights yolov5s.pt --device 0
+```
+
+### Multi-GPU [DataParallel](https://pytorch.org/docs/stable/nn.html#torch.nn.DataParallel) Mode (⚠️ not recommended)
+
+You can increase the `device` to use Multiple GPUs in DataParallel mode.
+
+```bash
+python train.py --batch 64 --data coco.yaml --weights yolov5s.pt --device 0,1
+```
+
+This method is slow and barely speeds up training compared to using just 1 GPU.
+
+### Multi-GPU [DistributedDataParallel](https://pytorch.org/docs/stable/nn.html#torch.nn.parallel.DistributedDataParallel) Mode (✅ recommended)
+
+You will have to pass `python -m torch.distributed.run --nproc_per_node`, followed by the usual arguments.
+
+```bash
+python -m torch.distributed.run --nproc_per_node 2 train.py --batch 64 --data coco.yaml --weights yolov5s.pt --device 0,1
+```
+
+`--nproc_per_node` specifies how many GPUs you would like to use. In the example above, it is 2.
+`--batch ` is the total batch-size. It will be divided evenly to each GPU. In the example above, it is 64/2=32 per GPU.
+
+The code above will use GPUs `0... (N-1)`.
+
+
+ Use specific GPUs (click to expand)
+
+You can do so by simply passing `--device` followed by your specific GPUs. For example, in the code below, we will use GPUs `2,3`.
+
+```bash
+python -m torch.distributed.run --nproc_per_node 2 train.py --batch 64 --data coco.yaml --cfg yolov5s.yaml --weights '' --device 2,3
+```
+
+
+
+
+ Use SyncBatchNorm (click to expand)
+
+[SyncBatchNorm](https://pytorch.org/docs/master/generated/torch.nn.SyncBatchNorm.html) could increase accuracy for multiple gpu training, however, it will slow down training by a significant factor. It is **only** available for Multiple GPU DistributedDataParallel training.
+
+It is best used when the batch-size on **each** GPU is small (<= 8).
+
+To use SyncBatchNorm, simple pass `--sync-bn` to the command like below,
+
+```bash
+python -m torch.distributed.run --nproc_per_node 2 train.py --batch 64 --data coco.yaml --cfg yolov5s.yaml --weights '' --sync-bn
+```
+
+
+
+
+ Use Multiple machines (click to expand)
+
+This is **only** available for Multiple GPU DistributedDataParallel training.
+
+Before we continue, make sure the files on all machines are the same, dataset, codebase, etc. Afterwards, make sure the machines can communicate to each other.
+
+You will have to choose a master machine(the machine that the others will talk to). Note down its address(`master_addr`) and choose a port(`master_port`). I will use `master_addr = 192.168.1.1` and `master_port = 1234` for the example below.
+
+To use it, you can do as the following,
+
+```bash
+# On master machine 0
+python -m torch.distributed.run --nproc_per_node G --nnodes N --node_rank 0 --master_addr "192.168.1.1" --master_port 1234 train.py --batch 64 --data coco.yaml --cfg yolov5s.yaml --weights ''
+```
+
+```bash
+# On machine R
+python -m torch.distributed.run --nproc_per_node G --nnodes N --node_rank R --master_addr "192.168.1.1" --master_port 1234 train.py --batch 64 --data coco.yaml --cfg yolov5s.yaml --weights ''
+```
+
+where `G` is number of GPU per machine, `N` is the number of machines, and `R` is the machine number from `0...(N-1)`.
+Let's say I have two machines with two GPUs each, it would be `G = 2` , `N = 2`, and `R = 1` for the above.
+
+Training will not start until all `N` machines are connected. Output will only be shown on master machine!
+
+
+
+### Notes
+
+- Windows support is untested, Linux is recommended.
+- `--batch ` must be a multiple of the number of GPUs.
+- GPU 0 will take slightly more memory than the other GPUs as it maintains EMA and is responsible for checkpointing etc.
+- If you get `RuntimeError: Address already in use`, it could be because you are running multiple trainings at a time. To fix this, simply use a different port number by adding `--master_port` like below,
+
+```bash
+python -m torch.distributed.run --master_port 1234 --nproc_per_node 2 ...
+```
+
+## Results
+
+DDP profiling results on an [AWS EC2 P4d instance](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/) with 8x A100 SXM4-40GB for YOLOv5l for 1 COCO epoch.
+
+
+ Profiling code
+
+```bash
+# prepare
+t=ultralytics/yolov5:latest && sudo docker pull $t && sudo docker run -it --ipc=host --gpus all -v "$(pwd)"/coco:/usr/src/coco $t
+pip3 install torch==1.9.0+cu111 torchvision==0.10.0+cu111 -f https://download.pytorch.org/whl/torch_stable.html
+cd .. && rm -rf app && git clone https://github.com/ultralytics/yolov5 -b master app && cd app
+cp data/coco.yaml data/coco_profile.yaml
+
+# profile
+python train.py --batch-size 16 --data coco_profile.yaml --weights yolov5l.pt --epochs 1 --device 0
+python -m torch.distributed.run --nproc_per_node 2 train.py --batch-size 32 --data coco_profile.yaml --weights yolov5l.pt --epochs 1 --device 0,1
+python -m torch.distributed.run --nproc_per_node 4 train.py --batch-size 64 --data coco_profile.yaml --weights yolov5l.pt --epochs 1 --device 0,1,2,3
+python -m torch.distributed.run --nproc_per_node 8 train.py --batch-size 128 --data coco_profile.yaml --weights yolov5l.pt --epochs 1 --device 0,1,2,3,4,5,6,7
+```
+
+
+
+| GPUs
A100 | batch-size | CUDA_mem
device0 (G) | COCO
train | COCO
val |
+|--------------|------------|------------------------------|--------------------|------------------|
+| 1x | 16 | 26GB | 20:39 | 0:55 |
+| 2x | 32 | 26GB | 11:43 | 0:57 |
+| 4x | 64 | 26GB | 5:57 | 0:55 |
+| 8x | 128 | 26GB | 3:09 | 0:57 |
+
+## FAQ
+
+If an error occurs, please read the checklist below first! (It could save your time)
+
+
+ Checklist (click to expand)
+
+
+ - Have you properly read this post?
+ - Have you tried to reclone the codebase? The code changes daily.
+ - Have you tried to search for your error? Someone may have already encountered it in this repo or in another and have the solution.
+ - Have you installed all the requirements listed on top (including the correct Python and Pytorch versions)?
+ - Have you tried in other environments listed in the "Environments" section below?
+ - Have you tried with another dataset like coco128 or coco2017? It will make it easier to find the root cause.
+
+
+If you went through all the above, feel free to raise an Issue by giving as much detail as possible following the template.
+
+
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
+
+## Credits
+
+I would like to thank @MagicFrogSJTU, who did all the heavy lifting, and @glenn-jocher for guiding us along the way.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/neural_magic_pruning_quantization.md b/yolov8/docs/yolov5/tutorials/neural_magic_pruning_quantization.md
new file mode 100644
index 0000000000000000000000000000000000000000..839f7ef9b7ee458dc693a54c7c7cbbcb9c2e9e1a
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/neural_magic_pruning_quantization.md
@@ -0,0 +1,271 @@
+---
+comments: true
+description: Learn how to deploy YOLOv5 with DeepSparse to achieve exceptional CPU performance close to GPUs, using pruning, and quantization.
+keywords: YOLOv5, DeepSparse, Neural Magic, CPU, Production, Performance, Deployments, APIs, SparseZoo, Ultralytics, Model Sparsity, Inference, Open-source, ONNX, Server
+---
+
+
+
+Welcome to software-delivered AI.
+
+This guide explains how to deploy YOLOv5 with Neural Magic's DeepSparse.
+
+DeepSparse is an inference runtime with exceptional performance on CPUs. For instance, compared to the ONNX Runtime baseline, DeepSparse offers a 5.8x speed-up for YOLOv5s, running on the same machine!
+
+
+
+
+
+For the first time, your deep learning workloads can meet the performance demands of production without the complexity and costs of hardware accelerators.
+Put simply, DeepSparse gives you the performance of GPUs and the simplicity of software:
+
+- **Flexible Deployments**: Run consistently across cloud, data center, and edge with any hardware provider from Intel to AMD to ARM
+- **Infinite Scalability**: Scale vertically to 100s of cores, out with standard Kubernetes, or fully-abstracted with Serverless
+- **Easy Integration**: Clean APIs for integrating your model into an application and monitoring it in production
+
+### How Does DeepSparse Achieve GPU-Class Performance?
+
+DeepSparse takes advantage of model sparsity to gain its performance speedup.
+
+Sparsification through pruning and quantization is a broadly studied technique, allowing order-of-magnitude reductions in the size and compute needed to
+execute a network, while maintaining high accuracy. DeepSparse is sparsity-aware, meaning it skips the zeroed out parameters, shrinking amount of compute
+in a forward pass. Since the sparse computation is now memory bound, DeepSparse executes the network depth-wise, breaking the problem into Tensor Columns,
+vertical stripes of computation that fit in cache.
+
+
+
+
+
+Sparse networks with compressed computation, executed depth-wise in cache, allows DeepSparse to deliver GPU-class performance on CPUs!
+
+### How Do I Create A Sparse Version of YOLOv5 Trained on My Data?
+
+Neural Magic's open-source model repository, SparseZoo, contains pre-sparsified checkpoints of each YOLOv5 model. Using SparseML, which is integrated with Ultralytics, you can fine-tune a sparse checkpoint onto your data with a single CLI command.
+
+[Checkout Neural Magic's YOLOv5 documentation for more details](https://docs.neuralmagic.com/use-cases/object-detection/sparsifying).
+
+## DeepSparse Usage
+
+We will walk through an example benchmarking and deploying a sparse version of YOLOv5s with DeepSparse.
+
+### Install DeepSparse
+
+Run the following to install DeepSparse. We recommend you use a virtual environment with Python.
+
+```bash
+pip install deepsparse[server,yolo,onnxruntime]
+```
+
+### Collect an ONNX File
+
+DeepSparse accepts a model in the ONNX format, passed either as:
+
+- A SparseZoo stub which identifies an ONNX file in the SparseZoo
+- A local path to an ONNX model in a filesystem
+
+The examples below use the standard dense and pruned-quantized YOLOv5s checkpoints, identified by the following SparseZoo stubs:
+
+```bash
+zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none
+zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none
+```
+
+### Deploy a Model
+
+DeepSparse offers convenient APIs for integrating your model into an application.
+
+To try the deployment examples below, pull down a sample image and save it as `basilica.jpg` with the following:
+
+```bash
+wget -O basilica.jpg https://raw.githubusercontent.com/neuralmagic/deepsparse/main/src/deepsparse/yolo/sample_images/basilica.jpg
+```
+
+#### Python API
+
+`Pipelines` wrap pre-processing and output post-processing around the runtime, providing a clean interface for adding DeepSparse to an application.
+The DeepSparse-Ultralytics integration includes an out-of-the-box `Pipeline` that accepts raw images and outputs the bounding boxes.
+
+Create a `Pipeline` and run inference:
+
+```python
+from deepsparse import Pipeline
+
+# list of images in local filesystem
+images = ["basilica.jpg"]
+
+# create Pipeline
+model_stub = "zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none"
+yolo_pipeline = Pipeline.create(
+ task="yolo",
+ model_path=model_stub,
+)
+
+# run inference on images, receive bounding boxes + classes
+pipeline_outputs = yolo_pipeline(images=images, iou_thres=0.6, conf_thres=0.001)
+print(pipeline_outputs)
+```
+
+If you are running in the cloud, you may get an error that open-cv cannot find `libGL.so.1`. Running the following on Ubuntu installs it:
+
+```
+apt-get install libgl1-mesa-glx
+```
+
+#### HTTP Server
+
+DeepSparse Server runs on top of the popular FastAPI web framework and Uvicorn web server. With just a single CLI command, you can easily setup a model
+service endpoint with DeepSparse. The Server supports any Pipeline from DeepSparse, including object detection with YOLOv5, enabling you to send raw
+images to the endpoint and receive the bounding boxes.
+
+Spin up the Server with the pruned-quantized YOLOv5s:
+
+```bash
+deepsparse.server \
+ --task yolo \
+ --model_path zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none
+```
+
+An example request, using Python's `requests` package:
+
+```python
+import requests, json
+
+# list of images for inference (local files on client side)
+path = ['basilica.jpg']
+files = [('request', open(img, 'rb')) for img in path]
+
+# send request over HTTP to /predict/from_files endpoint
+url = 'http://0.0.0.0:5543/predict/from_files'
+resp = requests.post(url=url, files=files)
+
+# response is returned in JSON
+annotations = json.loads(resp.text) # dictionary of annotation results
+bounding_boxes = annotations["boxes"]
+labels = annotations["labels"]
+```
+
+#### Annotate CLI
+
+You can also use the annotate command to have the engine save an annotated photo on disk. Try --source 0 to annotate your live webcam feed!
+
+```bash
+deepsparse.object_detection.annotate --model_filepath zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none --source basilica.jpg
+```
+
+Running the above command will create an `annotation-results` folder and save the annotated image inside.
+
+
+
+
+
+## Benchmarking Performance
+
+We will compare DeepSparse's throughput to ONNX Runtime's throughput on YOLOv5s, using DeepSparse's benchmarking script.
+
+The benchmarks were run on an AWS `c6i.8xlarge` instance (16 cores).
+
+### Batch 32 Performance Comparison
+
+#### ONNX Runtime Baseline
+
+At batch 32, ONNX Runtime achieves 42 images/sec with the standard dense YOLOv5s:
+
+```bash
+deepsparse.benchmark zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none -s sync -b 32 -nstreams 1 -e onnxruntime
+
+> Original Model Path: zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none
+> Batch Size: 32
+> Scenario: sync
+> Throughput (items/sec): 41.9025
+```
+
+#### DeepSparse Dense Performance
+
+While DeepSparse offers its best performance with optimized sparse models, it also performs well with the standard dense YOLOv5s.
+
+At batch 32, DeepSparse achieves 70 images/sec with the standard dense YOLOv5s, a **1.7x performance improvement over ORT**!
+
+```bash
+deepsparse.benchmark zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none -s sync -b 32 -nstreams 1
+
+> Original Model Path: zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none
+> Batch Size: 32
+> Scenario: sync
+> Throughput (items/sec): 69.5546
+```
+
+#### DeepSparse Sparse Performance
+
+When sparsity is applied to the model, DeepSparse's performance gains over ONNX Runtime is even stronger.
+
+At batch 32, DeepSparse achieves 241 images/sec with the pruned-quantized YOLOv5s, a **5.8x performance improvement over ORT**!
+
+```bash
+deepsparse.benchmark zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none -s sync -b 32 -nstreams 1
+
+> Original Model Path: zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none
+> Batch Size: 32
+> Scenario: sync
+> Throughput (items/sec): 241.2452
+```
+
+### Batch 1 Performance Comparison
+
+DeepSparse is also able to gain a speed-up over ONNX Runtime for the latency-sensitive, batch 1 scenario.
+
+#### ONNX Runtime Baseline
+
+At batch 1, ONNX Runtime achieves 48 images/sec with the standard, dense YOLOv5s.
+
+```bash
+deepsparse.benchmark zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none -s sync -b 1 -nstreams 1 -e onnxruntime
+
+> Original Model Path: zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/base-none
+> Batch Size: 1
+> Scenario: sync
+> Throughput (items/sec): 48.0921
+```
+
+#### DeepSparse Sparse Performance
+
+At batch 1, DeepSparse achieves 135 items/sec with a pruned-quantized YOLOv5s, **a 2.8x performance gain over ONNX Runtime!**
+
+```bash
+deepsparse.benchmark zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none -s sync -b 1 -nstreams 1
+
+> Original Model Path: zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned65_quant-none
+> Batch Size: 1
+> Scenario: sync
+> Throughput (items/sec): 134.9468
+```
+
+Since `c6i.8xlarge` instances have VNNI instructions, DeepSparse's throughput can be pushed further if weights are pruned in blocks of 4.
+
+At batch 1, DeepSparse achieves 180 items/sec with a 4-block pruned-quantized YOLOv5s, a **3.7x performance gain over ONNX Runtime!**
+
+```bash
+deepsparse.benchmark zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned35_quant-none-vnni -s sync -b 1 -nstreams 1
+
+> Original Model Path: zoo:cv/detection/yolov5-s/pytorch/ultralytics/coco/pruned35_quant-none-vnni
+> Batch Size: 1
+> Scenario: sync
+> Throughput (items/sec): 179.7375
+```
+
+## Get Started With DeepSparse
+
+**Research or Testing?** DeepSparse Community is free for research and testing. Get started with our [Documentation](https://docs.neuralmagic.com/).
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/pytorch_hub_model_loading.md b/yolov8/docs/yolov5/tutorials/pytorch_hub_model_loading.md
new file mode 100644
index 0000000000000000000000000000000000000000..576c3e6c9010cb9000fdc0e121c9ec590ae87d62
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/pytorch_hub_model_loading.md
@@ -0,0 +1,331 @@
+---
+comments: true
+description: Learn how to load YOLOv5🚀 from PyTorch Hub at https://pytorch.org/hub/ultralytics_yolov5 and perform image inference. UPDATED 26 March 2023.
+keywords: YOLOv5, PyTorch Hub, object detection, computer vision, machine learning, artificial intelligence
+---
+
+📚 This guide explains how to load YOLOv5 🚀 from PyTorch Hub at [https://pytorch.org/hub/ultralytics_yolov5](https://pytorch.org/hub/ultralytics_yolov5).
+UPDATED 26 March 2023.
+
+## Before You Start
+
+Install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+pip install -r https://raw.githubusercontent.com/ultralytics/yolov5/master/requirements.txt
+```
+
+💡 ProTip: Cloning [https://github.com/ultralytics/yolov5](https://github.com/ultralytics/yolov5) is **not** required 😃
+
+## Load YOLOv5 with PyTorch Hub
+
+### Simple Example
+
+This example loads a pretrained YOLOv5s model from PyTorch Hub as `model` and passes an image for inference. `'yolov5s'` is the lightest and fastest YOLOv5 model. For details on all available models please see the [README](https://github.com/ultralytics/yolov5#pretrained-checkpoints).
+
+```python
+import torch
+
+# Model
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s')
+
+# Image
+im = 'https://ultralytics.com/images/zidane.jpg'
+
+# Inference
+results = model(im)
+
+results.pandas().xyxy[0]
+# xmin ymin xmax ymax confidence class name
+# 0 749.50 43.50 1148.0 704.5 0.874023 0 person
+# 1 433.50 433.50 517.5 714.5 0.687988 27 tie
+# 2 114.75 195.75 1095.0 708.0 0.624512 0 person
+# 3 986.00 304.00 1028.0 420.0 0.286865 27 tie
+```
+
+### Detailed Example
+
+This example shows **batched inference** with **PIL** and **OpenCV** image sources. `results` can be **printed** to console, **saved** to `runs/hub`, **showed** to screen on supported environments, and returned as **tensors** or **pandas** dataframes.
+
+```python
+import cv2
+import torch
+from PIL import Image
+
+# Model
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s')
+
+# Images
+for f in 'zidane.jpg', 'bus.jpg':
+ torch.hub.download_url_to_file('https://ultralytics.com/images/' + f, f) # download 2 images
+im1 = Image.open('zidane.jpg') # PIL image
+im2 = cv2.imread('bus.jpg')[..., ::-1] # OpenCV image (BGR to RGB)
+
+# Inference
+results = model([im1, im2], size=640) # batch of images
+
+# Results
+results.print()
+results.save() # or .show()
+
+results.xyxy[0] # im1 predictions (tensor)
+results.pandas().xyxy[0] # im1 predictions (pandas)
+# xmin ymin xmax ymax confidence class name
+# 0 749.50 43.50 1148.0 704.5 0.874023 0 person
+# 1 433.50 433.50 517.5 714.5 0.687988 27 tie
+# 2 114.75 195.75 1095.0 708.0 0.624512 0 person
+# 3 986.00 304.00 1028.0 420.0 0.286865 27 tie
+```
+
+
+
+For all inference options see YOLOv5 `AutoShape()` forward [method](https://github.com/ultralytics/yolov5/blob/30e4c4f09297b67afedf8b2bcd851833ddc9dead/models/common.py#L243-L252).
+
+### Inference Settings
+
+YOLOv5 models contain various inference attributes such as **confidence threshold**, **IoU threshold**, etc. which can be set by:
+
+```python
+model.conf = 0.25 # NMS confidence threshold
+ iou = 0.45 # NMS IoU threshold
+ agnostic = False # NMS class-agnostic
+ multi_label = False # NMS multiple labels per box
+ classes = None # (optional list) filter by class, i.e. = [0, 15, 16] for COCO persons, cats and dogs
+ max_det = 1000 # maximum number of detections per image
+ amp = False # Automatic Mixed Precision (AMP) inference
+
+results = model(im, size=320) # custom inference size
+```
+
+### Device
+
+Models can be transferred to any device after creation:
+
+```python
+model.cpu() # CPU
+model.cuda() # GPU
+model.to(device) # i.e. device=torch.device(0)
+```
+
+Models can also be created directly on any `device`:
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', device='cpu') # load on CPU
+```
+
+💡 ProTip: Input images are automatically transferred to the correct model device before inference.
+
+### Silence Outputs
+
+Models can be loaded silently with `_verbose=False`:
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', _verbose=False) # load silently
+```
+
+### Input Channels
+
+To load a pretrained YOLOv5s model with 4 input channels rather than the default 3:
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', channels=4)
+```
+
+In this case the model will be composed of pretrained weights **except for** the very first input layer, which is no longer the same shape as the pretrained input layer. The input layer will remain initialized by random weights.
+
+### Number of Classes
+
+To load a pretrained YOLOv5s model with 10 output classes rather than the default 80:
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', classes=10)
+```
+
+In this case the model will be composed of pretrained weights **except for** the output layers, which are no longer the same shape as the pretrained output layers. The output layers will remain initialized by random weights.
+
+### Force Reload
+
+If you run into problems with the above steps, setting `force_reload=True` may help by discarding the existing cache and force a fresh download of the latest YOLOv5 version from PyTorch Hub.
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', force_reload=True) # force reload
+```
+
+### Screenshot Inference
+
+To run inference on your desktop screen:
+
+```python
+import torch
+from PIL import ImageGrab
+
+# Model
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s')
+
+# Image
+im = ImageGrab.grab() # take a screenshot
+
+# Inference
+results = model(im)
+```
+
+### Multi-GPU Inference
+
+YOLOv5 models can be loaded to multiple GPUs in parallel with threaded inference:
+
+```python
+import torch
+import threading
+
+def run(model, im):
+ results = model(im)
+ results.save()
+
+# Models
+model0 = torch.hub.load('ultralytics/yolov5', 'yolov5s', device=0)
+model1 = torch.hub.load('ultralytics/yolov5', 'yolov5s', device=1)
+
+# Inference
+threading.Thread(target=run, args=[model0, 'https://ultralytics.com/images/zidane.jpg'], daemon=True).start()
+threading.Thread(target=run, args=[model1, 'https://ultralytics.com/images/bus.jpg'], daemon=True).start()
+```
+
+### Training
+
+To load a YOLOv5 model for training rather than inference, set `autoshape=False`. To load a model with randomly initialized weights (to train from scratch) use `pretrained=False`. You must provide your own training script in this case. Alternatively see our YOLOv5 [Train Custom Data Tutorial](https://docs.ultralytics.com/yolov5/tutorials/train_custom_data) for model training.
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', autoshape=False) # load pretrained
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s', autoshape=False, pretrained=False) # load scratch
+```
+
+### Base64 Results
+
+For use with API services. See https://github.com/ultralytics/yolov5/pull/2291 and [Flask REST API](https://github.com/ultralytics/yolov5/tree/master/utils/flask_rest_api) example for details.
+
+```python
+results = model(im) # inference
+
+results.ims # array of original images (as np array) passed to model for inference
+results.render() # updates results.ims with boxes and labels
+for im in results.ims:
+ buffered = BytesIO()
+ im_base64 = Image.fromarray(im)
+ im_base64.save(buffered, format="JPEG")
+ print(base64.b64encode(buffered.getvalue()).decode('utf-8')) # base64 encoded image with results
+```
+
+### Cropped Results
+
+Results can be returned and saved as detection crops:
+
+```python
+results = model(im) # inference
+crops = results.crop(save=True) # cropped detections dictionary
+```
+
+### Pandas Results
+
+Results can be returned as [Pandas DataFrames](https://pandas.pydata.org/):
+
+```python
+results = model(im) # inference
+results.pandas().xyxy[0] # Pandas DataFrame
+```
+
+
+ Pandas Output (click to expand)
+
+```python
+print(results.pandas().xyxy[0])
+# xmin ymin xmax ymax confidence class name
+# 0 749.50 43.50 1148.0 704.5 0.874023 0 person
+# 1 433.50 433.50 517.5 714.5 0.687988 27 tie
+# 2 114.75 195.75 1095.0 708.0 0.624512 0 person
+# 3 986.00 304.00 1028.0 420.0 0.286865 27 tie
+```
+
+
+
+### Sorted Results
+
+Results can be sorted by column, i.e. to sort license plate digit detection left-to-right (x-axis):
+
+```python
+results = model(im) # inference
+results.pandas().xyxy[0].sort_values('xmin') # sorted left-right
+```
+
+### Box-Cropped Results
+
+Results can be returned and saved as detection crops:
+
+```python
+results = model(im) # inference
+crops = results.crop(save=True) # cropped detections dictionary
+```
+
+### JSON Results
+
+Results can be returned in JSON format once converted to `.pandas()` dataframes using the `.to_json()` method. The JSON format can be modified using the `orient` argument. See pandas `.to_json()` [documentation](https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.to_json.html) for details.
+
+```python
+results = model(ims) # inference
+results.pandas().xyxy[0].to_json(orient="records") # JSON img1 predictions
+```
+
+
+ JSON Output (click to expand)
+
+```json
+[
+{"xmin":749.5,"ymin":43.5,"xmax":1148.0,"ymax":704.5,"confidence":0.8740234375,"class":0,"name":"person"},
+{"xmin":433.5,"ymin":433.5,"xmax":517.5,"ymax":714.5,"confidence":0.6879882812,"class":27,"name":"tie"},
+{"xmin":115.25,"ymin":195.75,"xmax":1096.0,"ymax":708.0,"confidence":0.6254882812,"class":0,"name":"person"},
+{"xmin":986.0,"ymin":304.0,"xmax":1028.0,"ymax":420.0,"confidence":0.2873535156,"class":27,"name":"tie"}
+]
+```
+
+
+
+## Custom Models
+
+This example loads a custom 20-class [VOC](https://github.com/ultralytics/yolov5/blob/master/data/VOC.yaml)-trained YOLOv5s model `'best.pt'` with PyTorch Hub.
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'custom', path='path/to/best.pt') # local model
+model = torch.hub.load('path/to/yolov5', 'custom', path='path/to/best.pt', source='local') # local repo
+```
+
+## TensorRT, ONNX and OpenVINO Models
+
+PyTorch Hub supports inference on most YOLOv5 export formats, including custom trained models. See [TFLite, ONNX, CoreML, TensorRT Export tutorial](https://docs.ultralytics.com/yolov5/tutorials/model_export) for details on exporting models.
+
+💡 ProTip: **TensorRT** may be up to 2-5X faster than PyTorch on [**GPU benchmarks**](https://github.com/ultralytics/yolov5/pull/6963)
+💡 ProTip: **ONNX** and **OpenVINO** may be up to 2-3X faster than PyTorch on [**CPU benchmarks**](https://github.com/ultralytics/yolov5/pull/6613)
+
+```python
+model = torch.hub.load('ultralytics/yolov5', 'custom', path='yolov5s.pt') # PyTorch
+ 'yolov5s.torchscript') # TorchScript
+ 'yolov5s.onnx') # ONNX
+ 'yolov5s_openvino_model/') # OpenVINO
+ 'yolov5s.engine') # TensorRT
+ 'yolov5s.mlmodel') # CoreML (macOS-only)
+ 'yolov5s.tflite') # TFLite
+ 'yolov5s_paddle_model/') # PaddlePaddle
+```
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/roboflow_datasets_integration.md b/yolov8/docs/yolov5/tutorials/roboflow_datasets_integration.md
new file mode 100644
index 0000000000000000000000000000000000000000..5288188484c5d5b8d99a2696c380f36f8b82012f
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/roboflow_datasets_integration.md
@@ -0,0 +1,53 @@
+---
+comments: true
+description: Use Roboflow to organize, label, prepare, version & host datasets for training YOLOv5 models. Upload via UI, API, or Python, making versions with custom preprocessing and offline augmentation. Export in YOLOv5 format and access custom training tutorials. Use active learning to improve model deployments.
+keywords: YOLOv5, Roboflow, Dataset, Labeling, Versioning, Darknet, Export, Python, Upload, Active Learning, Preprocessing
+---
+
+# Roboflow Datasets
+
+You can now use Roboflow to organize, label, prepare, version, and host your datasets for training YOLOv5 🚀 models. Roboflow is free to use with YOLOv5 if you make your workspace public.
+UPDATED 7 June 2023.
+
+!!! warning
+
+ Roboflow users can use Ultralytics under the [AGPL license](https://github.com/ultralytics/ultralytics/blob/main/LICENSE) or procure an [Enterprise license](https://ultralytics.com/license) directly from Ultralytics. Be aware that Roboflow does **not** provide Ultralytics licenses, and it is the responsibility of the user to ensure appropriate licensing.
+
+## Upload
+
+You can upload your data to Roboflow via [web UI](https://docs.roboflow.com/adding-data), [rest API](https://docs.roboflow.com/adding-data/upload-api), or [python](https://docs.roboflow.com/python).
+
+## Labeling
+
+After uploading data to Roboflow, you can label your data and review previous labels.
+
+[](https://roboflow.com/annotate)
+
+## Versioning
+
+You can make versions of your dataset with different preprocessing and offline augmentation options. YOLOv5 does online augmentations natively, so be intentional when layering Roboflow's offline augs on top.
+
+
+
+## Exporting Data
+
+You can download your data in YOLOv5 format to quickly begin training.
+
+```
+from roboflow import Roboflow
+rf = Roboflow(api_key="YOUR API KEY HERE")
+project = rf.workspace().project("YOUR PROJECT")
+dataset = project.version("YOUR VERSION").download("yolov5")
+```
+
+## Custom Training
+
+We have released a custom training tutorial demonstrating all of the above capabilities. You can access the code here:
+
+[](https://colab.research.google.com/github/roboflow-ai/yolov5-custom-training-tutorial/blob/main/yolov5-custom-training.ipynb)
+
+## Active Learning
+
+The real world is messy and your model will invariably encounter situations your dataset didn't anticipate. Using [active learning](https://blog.roboflow.com/what-is-active-learning/) is an important strategy to iteratively improve your dataset and model. With the Roboflow and YOLOv5 integration, you can quickly make improvements on your model deployments by using a battle tested machine learning pipeline.
+
+
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/running_on_jetson_nano.md b/yolov8/docs/yolov5/tutorials/running_on_jetson_nano.md
new file mode 100644
index 0000000000000000000000000000000000000000..f41d3e3b93e4620ae64d50b0b5050b49bdaf0f13
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/running_on_jetson_nano.md
@@ -0,0 +1,320 @@
+---
+comments: true
+description: Deploy YOLOv5 on NVIDIA Jetson using TensorRT and DeepStream SDK for high performance inference. Step-by-step guide with code snippets.
+keywords: YOLOv5, NVIDIA Jetson, TensorRT, DeepStream SDK, deployment, AI at edge, PyTorch, computer vision, object detection, CUDA
+---
+
+# Deploy on NVIDIA Jetson using TensorRT and DeepStream SDK
+
+📚 This guide explains how to deploy a trained model into NVIDIA Jetson Platform and perform inference using TensorRT and DeepStream SDK. Here we use TensorRT to maximize the inference performance on the Jetson platform.
+UPDATED 18 November 2022.
+
+## Hardware Verification
+
+We have tested and verified this guide on the following Jetson devices
+
+- [Seeed reComputer J1010 built with Jetson Nano module](https://www.seeedstudio.com/Jetson-10-1-A0-p-5336.html)
+- [Seeed reComputer J2021 built with Jetson Xavier NX module](https://www.seeedstudio.com/reComputer-J2021-p-5438.html)
+
+## Before You Start
+
+Make sure you have properly installed **JetPack SDK** with all the **SDK Components** and **DeepStream SDK** on the Jetson device as this includes CUDA, TensorRT and DeepStream SDK which are needed for this guide.
+
+JetPack SDK provides a full development environment for hardware-accelerated AI-at-the-edge development. All Jetson modules and developer kits are supported by JetPack SDK.
+
+There are two major installation methods including,
+
+1. SD Card Image Method
+2. NVIDIA SDK Manager Method
+
+You can find a very detailed installation guide from NVIDIA [official website](https://developer.nvidia.com/jetpack-sdk-461). You can also find guides corresponding to the above-mentioned [reComputer J1010](https://wiki.seeedstudio.com/reComputer_J1010_J101_Flash_Jetpack) and [reComputer J2021](https://wiki.seeedstudio.com/reComputer_J2021_J202_Flash_Jetpack).
+
+## Install Necessary Packages
+
+- **Step 1.** Access the terminal of Jetson device, install pip and upgrade it
+
+```sh
+sudo apt update
+sudo apt install -y python3-pip
+pip3 install --upgrade pip
+```
+
+- **Step 2.** Clone the following repo
+
+```sh
+git clone https://github.com/ultralytics/yolov5
+```
+
+- **Step 3.** Open **requirements.txt**
+
+```sh
+cd yolov5
+vi requirements.txt
+```
+
+- **Step 5.** Edit the following lines. Here you need to press **i** first to enter editing mode. Press **ESC**, then type **:wq** to save and quit
+
+```sh
+# torch>=1.7.0
+# torchvision>=0.8.1
+```
+
+**Note:** torch and torchvision are excluded for now because they will be installed later.
+
+- **Step 6.** install the below dependency
+
+```sh
+sudo apt install -y libfreetype6-dev
+```
+
+- **Step 7.** Install the necessary packages
+
+```sh
+pip3 install -r requirements.txt
+```
+
+## Install PyTorch and Torchvision
+
+We cannot install PyTorch and Torchvision from pip because they are not compatible to run on Jetson platform which is based on **ARM aarch64 architecture**. Therefore, we need to manually install pre-built PyTorch pip wheel and compile/ install Torchvision from source.
+
+Visit [this page](https://forums.developer.nvidia.com/t/pytorch-for-jetson) to access all the PyTorch and Torchvision links.
+
+Here are some of the versions supported by JetPack 4.6 and above.
+
+**PyTorch v1.10.0**
+
+Supported by JetPack 4.4 (L4T R32.4.3) / JetPack 4.4.1 (L4T R32.4.4) / JetPack 4.5 (L4T R32.5.0) / JetPack 4.5.1 (L4T R32.5.1) / JetPack 4.6 (L4T R32.6.1) with Python 3.6
+
+**file_name:** torch-1.10.0-cp36-cp36m-linux_aarch64.whl
+**URL:** [https://nvidia.box.com/shared/static/fjtbno0vpo676a25cgvuqc1wty0fkkg6.whl](https://nvidia.box.com/shared/static/fjtbno0vpo676a25cgvuqc1wty0fkkg6.whl)
+
+**PyTorch v1.12.0**
+
+Supported by JetPack 5.0 (L4T R34.1.0) / JetPack 5.0.1 (L4T R34.1.1) / JetPack 5.0.2 (L4T R35.1.0) with Python 3.8
+
+**file_name:** torch-1.12.0a0+2c916ef.nv22.3-cp38-cp38-linux_aarch64.whl
+**URL:** [https://developer.download.nvidia.com/compute/redist/jp/v50/pytorch/torch-1.12.0a0+2c916ef.nv22.3-cp38-cp38-linux_aarch64.whl](https://developer.download.nvidia.com/compute/redist/jp/v50/pytorch/torch-1.12.0a0+2c916ef.nv22.3-cp38-cp38-linux_aarch64.whl)
+
+- **Step 1.** Install torch according to your JetPack version in the following format
+
+```sh
+wget -O
+pip3 install
+```
+
+For example, here we are running **JP4.6.1**, and therefore we choose **PyTorch v1.10.0**
+
+```sh
+cd ~
+sudo apt-get install -y libopenblas-base libopenmpi-dev
+wget https://nvidia.box.com/shared/static/fjtbno0vpo676a25cgvuqc1wty0fkkg6.whl -O torch-1.10.0-cp36-cp36m-linux_aarch64.whl
+pip3 install torch-1.10.0-cp36-cp36m-linux_aarch64.whl
+```
+
+- **Step 2.** Install torchvision depending on the version of PyTorch that you have installed. For example, we chose **PyTorch v1.10.0**, which means, we need to choose **Torchvision v0.11.1**
+
+```sh
+sudo apt install -y libjpeg-dev zlib1g-dev
+git clone --branch v0.11.1 https://github.com/pytorch/vision torchvision
+cd torchvision
+sudo python3 setup.py install
+```
+
+Here a list of the corresponding torchvision version that you need to install according to the PyTorch version:
+
+- PyTorch v1.10 - torchvision v0.11.1
+- PyTorch v1.12 - torchvision v0.13.0
+
+## DeepStream Configuration for YOLOv5
+
+- **Step 1.** Clone the following repo
+
+```sh
+cd ~
+git clone https://github.com/marcoslucianops/DeepStream-Yolo
+```
+
+- **Step 2.** Copy **gen_wts_yoloV5.py** from **DeepStream-Yolo/utils** into **yolov5** directory
+
+```sh
+cp DeepStream-Yolo/utils/gen_wts_yoloV5.py yolov5
+```
+
+- **Step 3.** Inside the yolov5 repo, download **pt file** from YOLOv5 releases (example for YOLOv5s 6.1)
+
+```sh
+cd yolov5
+wget https://github.com/ultralytics/yolov5/releases/download/v6.1/yolov5s.pt
+```
+
+- **Step 4.** Generate the **cfg** and **wts** files
+
+```sh
+python3 gen_wts_yoloV5.py -w yolov5s.pt
+```
+
+**Note**: To change the inference size (default: 640)
+
+```sh
+-s SIZE
+--size SIZE
+-s HEIGHT WIDTH
+--size HEIGHT WIDTH
+
+Example for 1280:
+
+-s 1280
+or
+-s 1280 1280
+```
+
+- **Step 5.** Copy the generated **cfg** and **wts** files into the **DeepStream-Yolo** folder
+
+```sh
+cp yolov5s.cfg ~/DeepStream-Yolo
+cp yolov5s.wts ~/DeepStream-Yolo
+```
+
+- **Step 6.** Open the **DeepStream-Yolo** folder and compile the library
+
+```sh
+cd ~/DeepStream-Yolo
+CUDA_VER=11.4 make -C nvdsinfer_custom_impl_Yolo # for DeepStream 6.1
+CUDA_VER=10.2 make -C nvdsinfer_custom_impl_Yolo # for DeepStream 6.0.1 / 6.0
+```
+
+- **Step 7.** Edit the **config_infer_primary_yoloV5.txt** file according to your model
+
+```sh
+[property]
+...
+custom-network-config=yolov5s.cfg
+model-file=yolov5s.wts
+...
+```
+
+- **Step 8.** Edit the **deepstream_app_config** file
+
+```sh
+...
+[primary-gie]
+...
+config-file=config_infer_primary_yoloV5.txt
+```
+
+- **Step 9.** Change the video source in **deepstream_app_config** file. Here a default video file is loaded as you can see below
+
+```sh
+...
+[source0]
+...
+uri=file:///opt/nvidia/deepstream/deepstream/samples/streams/sample_1080p_h264.mp4
+```
+
+## Run the Inference
+
+```sh
+deepstream-app -c deepstream_app_config.txt
+```
+
+
+
+The above result is running on **Jetson Xavier NX** with **FP32** and **YOLOv5s 640x640**. We can see that the **FPS** is around **30**.
+
+## INT8 Calibration
+
+If you want to use INT8 precision for inference, you need to follow the steps below
+
+- **Step 1.** Install OpenCV
+
+```sh
+sudo apt-get install libopencv-dev
+```
+
+- **Step 2.** Compile/recompile the **nvdsinfer_custom_impl_Yolo** library with OpenCV support
+
+```sh
+cd ~/DeepStream-Yolo
+CUDA_VER=11.4 OPENCV=1 make -C nvdsinfer_custom_impl_Yolo # for DeepStream 6.1
+CUDA_VER=10.2 OPENCV=1 make -C nvdsinfer_custom_impl_Yolo # for DeepStream 6.0.1 / 6.0
+```
+
+- **Step 3.** For COCO dataset, download the [val2017](https://drive.google.com/file/d/1gbvfn7mcsGDRZ_luJwtITL-ru2kK99aK/view?usp=sharing), extract, and move to **DeepStream-Yolo** folder
+
+- **Step 4.** Make a new directory for calibration images
+
+```sh
+mkdir calibration
+```
+
+- **Step 5.** Run the following to select 1000 random images from COCO dataset to run calibration
+
+```sh
+for jpg in $(ls -1 val2017/*.jpg | sort -R | head -1000); do \
+ cp ${jpg} calibration/; \
+done
+```
+
+**Note:** NVIDIA recommends at least 500 images to get a good accuracy. On this example, 1000 images are chosen to get better accuracy (more images = more accuracy). Higher INT8_CALIB_BATCH_SIZE values will result in more accuracy and faster calibration speed. Set it according to you GPU memory. You can set it from **head -1000**. For example, for 2000 images, **head -2000**. This process can take a long time.
+
+- **Step 6.** Create the **calibration.txt** file with all selected images
+
+```sh
+realpath calibration/*jpg > calibration.txt
+```
+
+- **Step 7.** Set environment variables
+
+```sh
+export INT8_CALIB_IMG_PATH=calibration.txt
+export INT8_CALIB_BATCH_SIZE=1
+```
+
+- **Step 8.** Update the **config_infer_primary_yoloV5.txt** file
+
+From
+
+```sh
+...
+model-engine-file=model_b1_gpu0_fp32.engine
+#int8-calib-file=calib.table
+...
+network-mode=0
+...
+```
+
+To
+
+```sh
+...
+model-engine-file=model_b1_gpu0_int8.engine
+int8-calib-file=calib.table
+...
+network-mode=1
+...
+```
+
+- **Step 9.** Run the inference
+
+```sh
+deepstream-app -c deepstream_app_config.txt
+```
+
+
+
+The above result is running on **Jetson Xavier NX** with **INT8** and **YOLOv5s 640x640**. We can see that the **FPS** is around **60**.
+
+## Benchmark results
+
+The following table summarizes how different models perform on **Jetson Xavier NX**.
+
+| Model Name | Precision | Inference Size | Inference Time (ms) | FPS |
+|------------|-----------|----------------|---------------------|-----|
+| YOLOv5s | FP32 | 320x320 | 16.66 | 60 |
+| | FP32 | 640x640 | 33.33 | 30 |
+| | INT8 | 640x640 | 16.66 | 60 |
+| YOLOv5n | FP32 | 640x640 | 16.66 | 60 |
+
+### Additional
+
+This tutorial is written by our friends at seeed @lakshanthad and Elaine
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/test_time_augmentation.md b/yolov8/docs/yolov5/tutorials/test_time_augmentation.md
new file mode 100644
index 0000000000000000000000000000000000000000..577c5ddc1f85cda2eb3851dabae8b2158f235c72
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/test_time_augmentation.md
@@ -0,0 +1,165 @@
+---
+comments: true
+description: Learn how to use Test Time Augmentation (TTA) with YOLOv5 to improve mAP and Recall during testing and inference. Code examples included.
+keywords: YOLOv5, test time augmentation, TTA, mAP, recall, object detection, deep learning, computer vision, PyTorch
+---
+
+# Test-Time Augmentation (TTA)
+
+📚 This guide explains how to use Test Time Augmentation (TTA) during testing and inference for improved mAP and Recall with YOLOv5 🚀.
+UPDATED 25 September 2022.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Test Normally
+
+Before trying TTA we want to establish a baseline performance to compare to. This command tests YOLOv5x on COCO val2017 at image size 640 pixels. `yolov5x.pt` is the largest and most accurate model available. Other options are `yolov5s.pt`, `yolov5m.pt` and `yolov5l.pt`, or you own checkpoint from training a custom dataset `./weights/best.pt`. For details on all available models please see our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints).
+
+```bash
+python val.py --weights yolov5x.pt --data coco.yaml --img 640 --half
+```
+
+Output:
+
+```shell
+val: data=./data/coco.yaml, weights=['yolov5x.pt'], batch_size=32, imgsz=640, conf_thres=0.001, iou_thres=0.65, task=val, device=, single_cls=False, augment=False, verbose=False, save_txt=False, save_hybrid=False, save_conf=False, save_json=True, project=runs/val, name=exp, exist_ok=False, half=True
+YOLOv5 🚀 v5.0-267-g6a3ee7c torch 1.9.0+cu102 CUDA:0 (Tesla P100-PCIE-16GB, 16280.875MB)
+
+Fusing layers...
+Model Summary: 476 layers, 87730285 parameters, 0 gradients
+
+val: Scanning '../datasets/coco/val2017' images and labels...4952 found, 48 missing, 0 empty, 0 corrupted: 100% 5000/5000 [00:01<00:00, 2846.03it/s]
+val: New cache created: ../datasets/coco/val2017.cache
+ Class Images Labels P R mAP@.5 mAP@.5:.95: 100% 157/157 [02:30<00:00, 1.05it/s]
+ all 5000 36335 0.746 0.626 0.68 0.49
+Speed: 0.1ms pre-process, 22.4ms inference, 1.4ms NMS per image at shape (32, 3, 640, 640) # <--- baseline speed
+
+Evaluating pycocotools mAP... saving runs/val/exp/yolov5x_predictions.json...
+...
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.504 # <--- baseline mAP
+ Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.688
+ Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.546
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.351
+ Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.551
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.644
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.382
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.628
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.681 # <--- baseline mAR
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.524
+ Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.735
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.826
+```
+
+## Test with TTA
+
+Append `--augment` to any existing `val.py` command to enable TTA, and increase the image size by about 30% for improved results. Note that inference with TTA enabled will typically take about 2-3X the time of normal inference as the images are being left-right flipped and processed at 3 different resolutions, with the outputs merged before NMS. Part of the speed decrease is simply due to larger image sizes (832 vs 640), while part is due to the actual TTA operations.
+
+```bash
+python val.py --weights yolov5x.pt --data coco.yaml --img 832 --augment --half
+```
+
+Output:
+
+```shell
+val: data=./data/coco.yaml, weights=['yolov5x.pt'], batch_size=32, imgsz=832, conf_thres=0.001, iou_thres=0.6, task=val, device=, single_cls=False, augment=True, verbose=False, save_txt=False, save_hybrid=False, save_conf=False, save_json=True, project=runs/val, name=exp, exist_ok=False, half=True
+YOLOv5 🚀 v5.0-267-g6a3ee7c torch 1.9.0+cu102 CUDA:0 (Tesla P100-PCIE-16GB, 16280.875MB)
+
+Fusing layers...
+/usr/local/lib/python3.7/dist-packages/torch/nn/functional.py:718: UserWarning: Named tensors and all their associated APIs are an experimental feature and subject to change. Please do not use them for anything important until they are released as stable. (Triggered internally at /pytorch/c10/core/TensorImpl.h:1156.)
+ return torch.max_pool2d(input, kernel_size, stride, padding, dilation, ceil_mode)
+Model Summary: 476 layers, 87730285 parameters, 0 gradients
+val: Scanning '../datasets/coco/val2017' images and labels...4952 found, 48 missing, 0 empty, 0 corrupted: 100% 5000/5000 [00:01<00:00, 2885.61it/s]
+val: New cache created: ../datasets/coco/val2017.cache
+ Class Images Labels P R mAP@.5 mAP@.5:.95: 100% 157/157 [07:29<00:00, 2.86s/it]
+ all 5000 36335 0.718 0.656 0.695 0.503
+Speed: 0.2ms pre-process, 80.6ms inference, 2.7ms NMS per image at shape (32, 3, 832, 832) # <--- TTA speed
+
+Evaluating pycocotools mAP... saving runs/val/exp2/yolov5x_predictions.json...
+...
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.516 # <--- TTA mAP
+ Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.701
+ Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.562
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.361
+ Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.564
+ Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.656
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.388
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.640
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.696 # <--- TTA mAR
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.553
+ Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.744
+ Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = 0.833
+```
+
+## Inference with TTA
+
+`detect.py` TTA inference operates identically to `val.py` TTA: simply append `--augment` to any existing `detect.py` command:
+
+```bash
+python detect.py --weights yolov5s.pt --img 832 --source data/images --augment
+```
+
+Output:
+
+```bash
+detect: weights=['yolov5s.pt'], source=data/images, imgsz=832, conf_thres=0.25, iou_thres=0.45, max_det=1000, device=, view_img=False, save_txt=False, save_conf=False, save_crop=False, nosave=False, classes=None, agnostic_nms=False, augment=True, update=False, project=runs/detect, name=exp, exist_ok=False, line_width=3, hide_labels=False, hide_conf=False, half=False
+YOLOv5 🚀 v5.0-267-g6a3ee7c torch 1.9.0+cu102 CUDA:0 (Tesla P100-PCIE-16GB, 16280.875MB)
+
+Downloading https://github.com/ultralytics/yolov5/releases/download/v5.0/yolov5s.pt to yolov5s.pt...
+100% 14.1M/14.1M [00:00<00:00, 81.9MB/s]
+
+Fusing layers...
+Model Summary: 224 layers, 7266973 parameters, 0 gradients
+image 1/2 /content/yolov5/data/images/bus.jpg: 832x640 4 persons, 1 bus, 1 fire hydrant, Done. (0.029s)
+image 2/2 /content/yolov5/data/images/zidane.jpg: 480x832 3 persons, 3 ties, Done. (0.024s)
+Results saved to runs/detect/exp
+Done. (0.156s)
+```
+
+
+
+### PyTorch Hub TTA
+
+TTA is automatically integrated into all [YOLOv5 PyTorch Hub](https://pytorch.org/hub/ultralytics_yolov5) models, and can be accessed by passing `augment=True` at inference time.
+
+```python
+import torch
+
+# Model
+model = torch.hub.load('ultralytics/yolov5', 'yolov5s') # or yolov5m, yolov5x, custom
+
+# Images
+img = 'https://ultralytics.com/images/zidane.jpg' # or file, PIL, OpenCV, numpy, multiple
+
+# Inference
+results = model(img, augment=True) # <--- TTA inference
+
+# Results
+results.print() # or .show(), .save(), .crop(), .pandas(), etc.
+```
+
+### Customize
+
+You can customize the TTA ops applied in the YOLOv5 `forward_augment()` method [here](https://github.com/ultralytics/yolov5/blob/8c6f9e15bfc0000d18b976a95b9d7c17d407ec91/models/yolo.py#L125-L137).
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/tips_for_best_training_results.md b/yolov8/docs/yolov5/tutorials/tips_for_best_training_results.md
new file mode 100644
index 0000000000000000000000000000000000000000..815ac1666e404d8cd25aebcd8e9732da86cac4bf
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/tips_for_best_training_results.md
@@ -0,0 +1,66 @@
+---
+comments: true
+description: Get the most out of YOLOv5 with this guide; producing best results, checking dataset, hypertuning & more. Updated May 2022.
+keywords: YOLOv5 training guide, mAP, best results, dataset, model selection, training settings, hyperparameters, Ultralytics Docs
+---
+
+📚 This guide explains how to produce the best mAP and training results with YOLOv5 🚀.
+UPDATED 25 May 2022.
+
+Most of the time good results can be obtained with no changes to the models or training settings, **provided your dataset is sufficiently large and well labelled**. If at first you don't get good results, there are steps you might be able to take to improve, but we always recommend users **first train with all default settings** before considering any changes. This helps establish a performance baseline and spot areas for improvement.
+
+If you have questions about your training results **we recommend you provide the maximum amount of information possible** if you expect a helpful response, including results plots (train losses, val losses, P, R, mAP), PR curve, confusion matrix, training mosaics, test results and dataset statistics images such as labels.png. All of these are located in your `project/name` directory, typically `yolov5/runs/train/exp`.
+
+We've put together a full guide for users looking to get the best results on their YOLOv5 trainings below.
+
+## Dataset
+
+- **Images per class.** ≥ 1500 images per class recommended
+- **Instances per class.** ≥ 10000 instances (labeled objects) per class recommended
+- **Image variety.** Must be representative of deployed environment. For real-world use cases we recommend images from different times of day, different seasons, different weather, different lighting, different angles, different sources (scraped online, collected locally, different cameras) etc.
+- **Label consistency.** All instances of all classes in all images must be labelled. Partial labelling will not work.
+- **Label accuracy.** Labels must closely enclose each object. No space should exist between an object and it's bounding box. No objects should be missing a label.
+- **Label verification.** View `train_batch*.jpg` on train start to verify your labels appear correct, i.e. see [example](https://docs.ultralytics.com/yolov5/tutorials/train_custom_data#local-logging) mosaic.
+- **Background images.** Background images are images with no objects that are added to a dataset to reduce False Positives (FP). We recommend about 0-10% background images to help reduce FPs (COCO has 1000 background images for reference, 1% of the total). No labels are required for background images.
+
+
+
+## Model Selection
+
+Larger models like YOLOv5x and [YOLOv5x6](https://github.com/ultralytics/yolov5/releases/tag/v5.0) will produce better results in nearly all cases, but have more parameters, require more CUDA memory to train, and are slower to run. For **mobile** deployments we recommend YOLOv5s/m, for **cloud** deployments we recommend YOLOv5l/x. See our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints) for a full comparison of all models.
+
+
+
+- **Start from Pretrained weights.** Recommended for small to medium-sized datasets (i.e. [VOC](https://github.com/ultralytics/yolov5/blob/master/data/VOC.yaml), [VisDrone](https://github.com/ultralytics/yolov5/blob/master/data/VisDrone.yaml), [GlobalWheat](https://github.com/ultralytics/yolov5/blob/master/data/GlobalWheat2020.yaml)). Pass the name of the model to the `--weights` argument. Models download automatically from the [latest YOLOv5 release](https://github.com/ultralytics/yolov5/releases).
+
+```shell
+python train.py --data custom.yaml --weights yolov5s.pt
+ yolov5m.pt
+ yolov5l.pt
+ yolov5x.pt
+ custom_pretrained.pt
+```
+
+- **Start from Scratch.** Recommended for large datasets (i.e. [COCO](https://github.com/ultralytics/yolov5/blob/master/data/coco.yaml), [Objects365](https://github.com/ultralytics/yolov5/blob/master/data/Objects365.yaml), [OIv6](https://storage.googleapis.com/openimages/web/index.html)). Pass the model architecture yaml you are interested in, along with an empty `--weights ''` argument:
+
+```bash
+python train.py --data custom.yaml --weights '' --cfg yolov5s.yaml
+ yolov5m.yaml
+ yolov5l.yaml
+ yolov5x.yaml
+```
+
+## Training Settings
+
+Before modifying anything, **first train with default settings to establish a performance baseline**. A full list of train.py settings can be found in the [train.py](https://github.com/ultralytics/yolov5/blob/master/train.py) argparser.
+
+- **Epochs.** Start with 300 epochs. If this overfits early then you can reduce epochs. If overfitting does not occur after 300 epochs, train longer, i.e. 600, 1200 etc epochs.
+- **Image size.** COCO trains at native resolution of `--img 640`, though due to the high amount of small objects in the dataset it can benefit from training at higher resolutions such as `--img 1280`. If there are many small objects then custom datasets will benefit from training at native or higher resolution. Best inference results are obtained at the same `--img` as the training was run at, i.e. if you train at `--img 1280` you should also test and detect at `--img 1280`.
+- **Batch size.** Use the largest `--batch-size` that your hardware allows for. Small batch sizes produce poor batchnorm statistics and should be avoided.
+- **Hyperparameters.** Default hyperparameters are in [hyp.scratch-low.yaml](https://github.com/ultralytics/yolov5/blob/master/data/hyps/hyp.scratch-low.yaml). We recommend you train with default hyperparameters first before thinking of modifying any. In general, increasing augmentation hyperparameters will reduce and delay overfitting, allowing for longer trainings and higher final mAP. Reduction in loss component gain hyperparameters like `hyp['obj']` will help reduce overfitting in those specific loss components. For an automated method of optimizing these hyperparameters, see our [Hyperparameter Evolution Tutorial](https://docs.ultralytics.com/yolov5/tutorials/hyperparameter_evolution).
+
+## Further Reading
+
+If you'd like to know more, a good place to start is Karpathy's 'Recipe for Training Neural Networks', which has great ideas for training that apply broadly across all ML domains: [http://karpathy.github.io/2019/04/25/recipe/](http://karpathy.github.io/2019/04/25/recipe/)
+
+Good luck 🍀 and let us know if you have any other questions!
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/train_custom_data.md b/yolov8/docs/yolov5/tutorials/train_custom_data.md
new file mode 100644
index 0000000000000000000000000000000000000000..ce11a95f6973306a743bff294545c55b696f2b3a
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/train_custom_data.md
@@ -0,0 +1,237 @@
+---
+comments: true
+description: Train your custom dataset with YOLOv5. Learn to collect, label and annotate images, and train and deploy models. Get started now.
+keywords: YOLOv5, train custom dataset, object detection, artificial intelligence, deep learning, computer vision
+---
+
+📚 This guide explains how to train your own **custom dataset** with [YOLOv5](https://github.com/ultralytics/yolov5) 🚀.
+UPDATED 7 June 2023.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Train On Custom Data
+
+
+
+
+
+
+Creating a custom model to detect your objects is an iterative process of collecting and organizing images, labeling your objects of interest, training a model, deploying it into the wild to make predictions, and then using that deployed model to collect examples of edge cases to repeat and improve.
+
+### 1. Create Dataset
+
+YOLOv5 models must be trained on labelled data in order to learn classes of objects in that data. There are two options for creating your dataset before you start training:
+
+
+Use Roboflow to create your dataset in YOLO format
+
+!!! warning
+
+ Roboflow users can use Ultralytics under the [AGPL license](https://github.com/ultralytics/ultralytics/blob/main/LICENSE) or procure an [Enterprise license](https://ultralytics.com/license) directly from Ultralytics. Be aware that Roboflow does **not** provide Ultralytics licenses, and it is the responsibility of the user to ensure appropriate licensing.
+
+### 1.1 Collect Images
+
+Your model will learn by example. Training on images similar to the ones it will see in the wild is of the utmost importance. Ideally, you will collect a wide variety of images from the same configuration (camera, angle, lighting, etc.) as you will ultimately deploy your project.
+
+If this is not possible, you can start from [a public dataset](https://universe.roboflow.com/?ref=ultralytics) to train your initial model and then [sample images from the wild during inference](https://blog.roboflow.com/computer-vision-active-learning-tips/?ref=ultralytics) to improve your dataset and model iteratively.
+
+### 1.2 Create Labels
+
+Once you have collected images, you will need to annotate the objects of interest to create a ground truth for your model to learn from.
+
+
+
+[Roboflow Annotate](https://roboflow.com/annotate?ref=ultralytics) is a simple
+web-based tool for managing and labeling your images with your team and exporting
+them in [YOLOv5's annotation format](https://roboflow.com/formats/yolov5-pytorch-txt?ref=ultralytics).
+
+### 1.3 Prepare Dataset for YOLOv5
+
+Whether you [label your images with Roboflow](https://roboflow.com/annotate?ref=ultralytics) or not, you can use it to convert your dataset into YOLO format, create a YOLOv5 YAML configuration file, and host it for importing into your training script.
+
+[Create a free Roboflow account](https://app.roboflow.com/?model=yolov5&ref=ultralytics)
+and upload your dataset to a `Public` workspace, label any unannotated images,
+then generate and export a version of your dataset in `YOLOv5 Pytorch` format.
+
+Note: YOLOv5 does online augmentation during training, so we do not recommend
+applying any augmentation steps in Roboflow for training with YOLOv5. But we
+recommend applying the following preprocessing steps:
+
+
+
+* **Auto-Orient** - to strip EXIF orientation from your images.
+* **Resize (Stretch)** - to the square input size of your model (640x640 is the YOLOv5 default).
+
+Generating a version will give you a point in time snapshot of your dataset so
+you can always go back and compare your future model training runs against it,
+even if you add more images or change its configuration later.
+
+
+
+Export in `YOLOv5 Pytorch` format, then copy the snippet into your training
+script or notebook to download your dataset.
+
+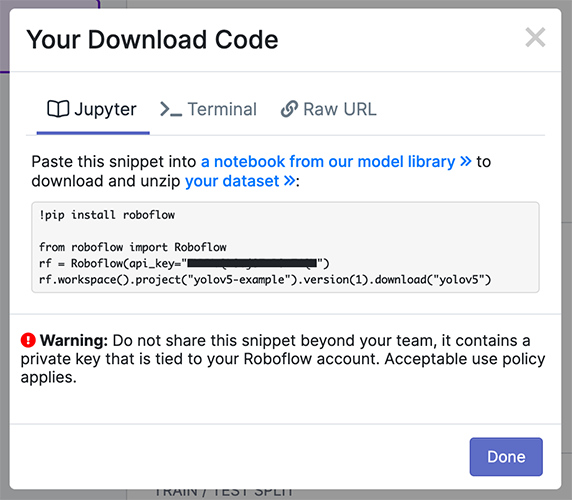
+
+Now continue with `2. Select a Model`.
+
+
+
+Or manually prepare your dataset
+
+### 1.1 Create dataset.yaml
+
+[COCO128](https://www.kaggle.com/ultralytics/coco128) is an example small tutorial dataset composed of the first 128 images in [COCO](http://cocodataset.org/#home) train2017. These same 128 images are used for both training and validation to verify our training pipeline is capable of overfitting. [data/coco128.yaml](https://github.com/ultralytics/yolov5/blob/master/data/coco128.yaml), shown below, is the dataset config file that defines 1) the dataset root directory `path` and relative paths to `train` / `val` / `test` image directories (or *.txt files with image paths) and 2) a class `names` dictionary:
+
+```yaml
+# Train/val/test sets as 1) dir: path/to/imgs, 2) file: path/to/imgs.txt, or 3) list: [path/to/imgs1, path/to/imgs2, ..]
+path: ../datasets/coco128 # dataset root dir
+train: images/train2017 # train images (relative to 'path') 128 images
+val: images/train2017 # val images (relative to 'path') 128 images
+test: # test images (optional)
+
+# Classes (80 COCO classes)
+names:
+ 0: person
+ 1: bicycle
+ 2: car
+ ...
+ 77: teddy bear
+ 78: hair drier
+ 79: toothbrush
+```
+
+### 1.2 Create Labels
+
+After using an annotation tool to label your images, export your labels to **YOLO format**, with one `*.txt` file per image (if no objects in image, no `*.txt` file is required). The `*.txt` file specifications are:
+
+- One row per object
+- Each row is `class x_center y_center width height` format.
+- Box coordinates must be in **normalized xywh** format (from 0 - 1). If your boxes are in pixels, divide `x_center` and `width` by image width, and `y_center` and `height` by image height.
+- Class numbers are zero-indexed (start from 0).
+
+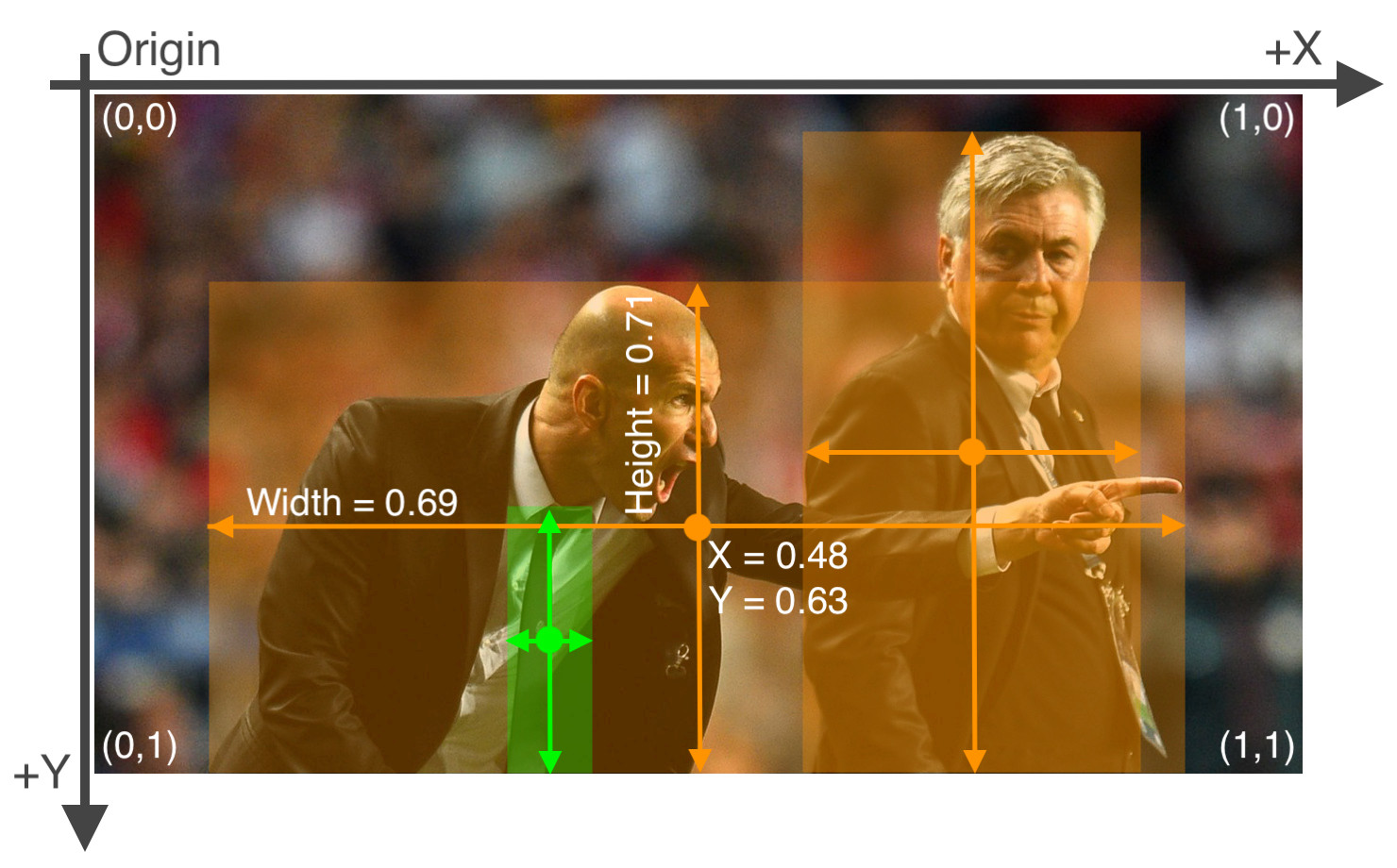
+
+The label file corresponding to the above image contains 2 persons (class `0`) and a tie (class `27`):
+
+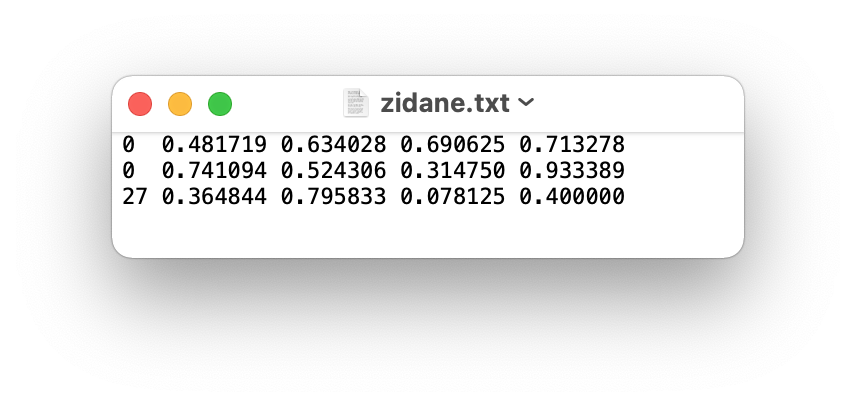
+
+### 1.3 Organize Directories
+
+Organize your train and val images and labels according to the example below. YOLOv5 assumes `/coco128` is inside a `/datasets` directory **next to** the `/yolov5` directory. **YOLOv5 locates labels automatically for each image** by replacing the last instance of `/images/` in each image path with `/labels/`. For example:
+
+```bash
+../datasets/coco128/images/im0.jpg # image
+../datasets/coco128/labels/im0.txt # label
+```
+
+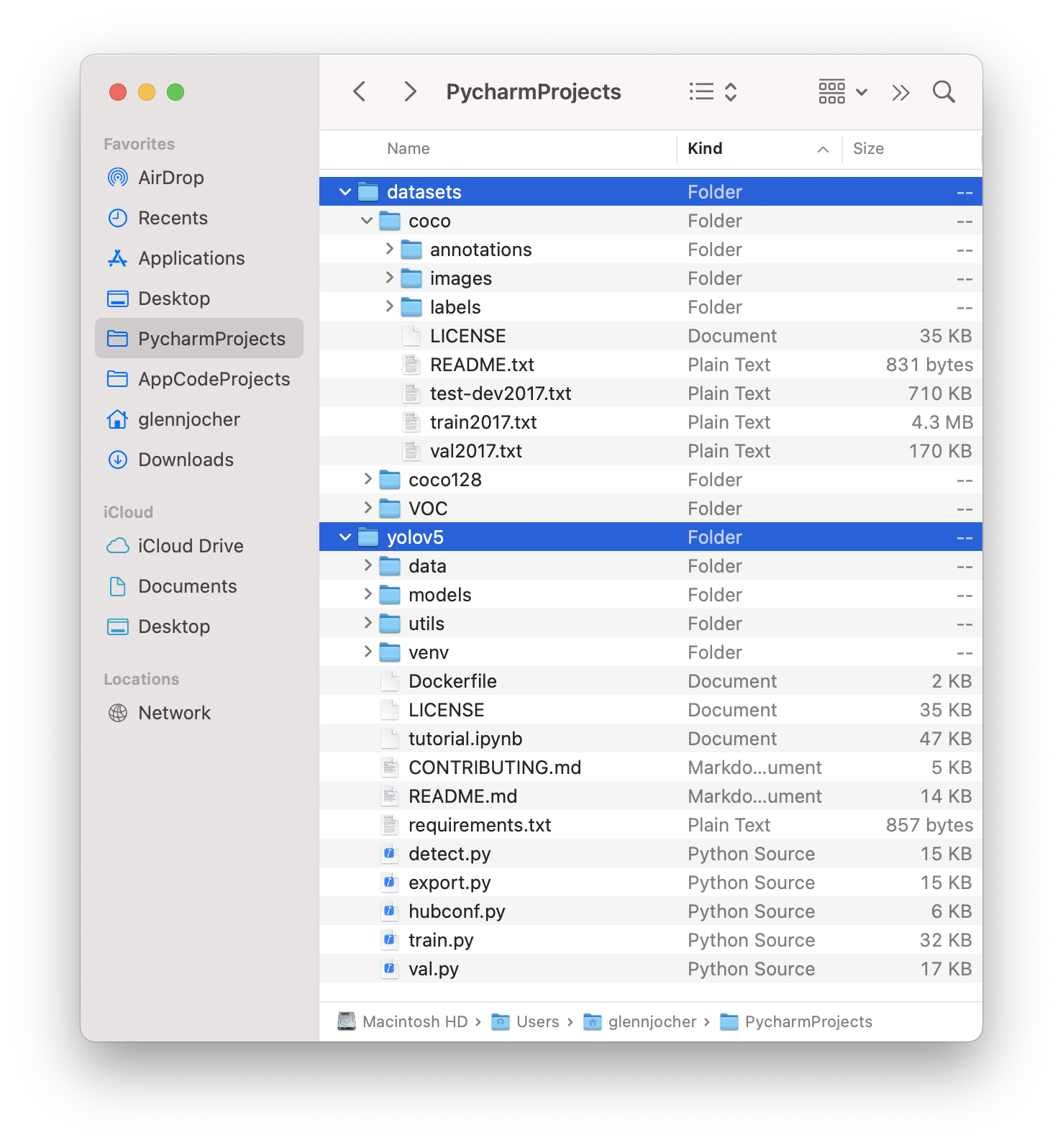
+
+
+### 2. Select a Model
+
+Select a pretrained model to start training from. Here we select [YOLOv5s](https://github.com/ultralytics/yolov5/blob/master/models/yolov5s.yaml), the second-smallest and fastest model available. See our README [table](https://github.com/ultralytics/yolov5#pretrained-checkpoints) for a full comparison of all models.
+
+
+
+### 3. Train
+
+Train a YOLOv5s model on COCO128 by specifying dataset, batch-size, image size and either pretrained `--weights yolov5s.pt` (recommended), or randomly initialized `--weights '' --cfg yolov5s.yaml` (not recommended). Pretrained weights are auto-downloaded from the [latest YOLOv5 release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+python train.py --img 640 --epochs 3 --data coco128.yaml --weights yolov5s.pt
+```
+
+!!! tip "Tip"
+
+ 💡 Add `--cache ram` or `--cache disk` to speed up training (requires significant RAM/disk resources).
+
+!!! tip "Tip"
+
+ 💡 Always train from a local dataset. Mounted or network drives like Google Drive will be very slow.
+
+All training results are saved to `runs/train/` with incrementing run directories, i.e. `runs/train/exp2`, `runs/train/exp3` etc. For more details see the Training section of our tutorial notebook.
+
+### 4. Visualize
+
+#### Comet Logging and Visualization 🌟 NEW
+
+[Comet](https://bit.ly/yolov5-readme-comet) is now fully integrated with YOLOv5. Track and visualize model metrics in real time, save your hyperparameters, datasets, and model checkpoints, and visualize your model predictions with [Comet Custom Panels](https://bit.ly/yolov5-colab-comet-panels)! Comet makes sure you never lose track of your work and makes it easy to share results and collaborate across teams of all sizes!
+
+Getting started is easy:
+
+```shell
+pip install comet_ml # 1. install
+export COMET_API_KEY= # 2. paste API key
+python train.py --img 640 --epochs 3 --data coco128.yaml --weights yolov5s.pt # 3. train
+```
+
+To learn more about all the supported Comet features for this integration, check out the [Comet Tutorial](https://docs.ultralytics.com/yolov5/tutorials/comet_logging_integration). If you'd like to learn more about Comet, head over to our [documentation](https://bit.ly/yolov5-colab-comet-docs). Get started by trying out the Comet Colab Notebook:
+[](https://colab.research.google.com/drive/1RG0WOQyxlDlo5Km8GogJpIEJlg_5lyYO?usp=sharing)
+
+
+
+#### ClearML Logging and Automation 🌟 NEW
+
+[ClearML](https://cutt.ly/yolov5-notebook-clearml) is completely integrated into YOLOv5 to track your experimentation, manage dataset versions and even remotely execute training runs. To enable ClearML:
+
+- `pip install clearml`
+- run `clearml-init` to connect to a ClearML server (**deploy your own open-source server [here](https://github.com/allegroai/clearml-server)**, or use our free hosted server [here](https://cutt.ly/yolov5-notebook-clearml))
+
+You'll get all the great expected features from an experiment manager: live updates, model upload, experiment comparison etc. but ClearML also tracks uncommitted changes and installed packages for example. Thanks to that ClearML Tasks (which is what we call experiments) are also reproducible on different machines! With only 1 extra line, we can schedule a YOLOv5 training task on a queue to be executed by any number of ClearML Agents (workers).
+
+You can use ClearML Data to version your dataset and then pass it to YOLOv5 simply using its unique ID. This will help you keep track of your data without adding extra hassle. Explore the [ClearML Tutorial](https://docs.ultralytics.com/yolov5/tutorials/clearml_logging_integration) for details!
+
+
+
+
+#### Local Logging
+
+Training results are automatically logged with [Tensorboard](https://www.tensorflow.org/tensorboard) and [CSV](https://github.com/ultralytics/yolov5/pull/4148) loggers to `runs/train`, with a new experiment directory created for each new training as `runs/train/exp2`, `runs/train/exp3`, etc.
+
+This directory contains train and val statistics, mosaics, labels, predictions and augmented mosaics, as well as metrics and charts including precision-recall (PR) curves and confusion matrices.
+
+
+
+Results file `results.csv` is updated after each epoch, and then plotted as `results.png` (below) after training completes. You can also plot any `results.csv` file manually:
+
+```python
+from utils.plots import plot_results
+
+plot_results('path/to/results.csv') # plot 'results.csv' as 'results.png'
+```
+
+
+
+## Next Steps
+
+Once your model is trained you can use your best checkpoint `best.pt` to:
+
+* Run [CLI](https://github.com/ultralytics/yolov5#quick-start-examples) or [Python](https://docs.ultralytics.com/yolov5/tutorials/pytorch_hub_model_loading) inference on new images and videos
+* [Validate](https://github.com/ultralytics/yolov5/blob/master/val.py) accuracy on train, val and test splits
+* [Export](https://docs.ultralytics.com/yolov5/tutorials/model_export) to TensorFlow, Keras, ONNX, TFlite, TF.js, CoreML and TensorRT formats
+* [Evolve](https://docs.ultralytics.com/yolov5/tutorials/hyperparameter_evolution) hyperparameters to improve performance
+* [Improve](https://docs.roboflow.com/adding-data/upload-api?ref=ultralytics) your model by sampling real-world images and adding them to your dataset
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/docs/yolov5/tutorials/transfer_learning_with_frozen_layers.md b/yolov8/docs/yolov5/tutorials/transfer_learning_with_frozen_layers.md
new file mode 100644
index 0000000000000000000000000000000000000000..71c0aebf015be373da658f669e8fbb7f1d464085
--- /dev/null
+++ b/yolov8/docs/yolov5/tutorials/transfer_learning_with_frozen_layers.md
@@ -0,0 +1,155 @@
+---
+comments: true
+description: Learn how to freeze YOLOv5 when transfer learning. Retrain a pre-trained model on new data faster and with fewer resources.
+keywords: Freeze YOLOv5, Transfer Learning YOLOv5, Freeze Layers, Reduce Resources, Speed up Training, Increase Accuracy
+---
+
+📚 This guide explains how to **freeze** YOLOv5 🚀 layers when **transfer learning**. Transfer learning is a useful way to quickly retrain a model on new data without having to retrain the entire network. Instead, part of the initial weights are frozen in place, and the rest of the weights are used to compute loss and are updated by the optimizer. This requires less resources than normal training and allows for faster training times, though it may also result in reductions to final trained accuracy.
+UPDATED 25 September 2022.
+
+## Before You Start
+
+Clone repo and install [requirements.txt](https://github.com/ultralytics/yolov5/blob/master/requirements.txt) in a [**Python>=3.7.0**](https://www.python.org/) environment, including [**PyTorch>=1.7**](https://pytorch.org/get-started/locally/). [Models](https://github.com/ultralytics/yolov5/tree/master/models) and [datasets](https://github.com/ultralytics/yolov5/tree/master/data) download automatically from the latest YOLOv5 [release](https://github.com/ultralytics/yolov5/releases).
+
+```bash
+git clone https://github.com/ultralytics/yolov5 # clone
+cd yolov5
+pip install -r requirements.txt # install
+```
+
+## Freeze Backbone
+
+All layers that match the train.py `freeze` list in train.py will be frozen by setting their gradients to zero before training starts.
+
+```python
+ # Freeze
+ freeze = [f'model.{x}.' for x in range(freeze)] # layers to freeze
+ for k, v in model.named_parameters():
+ v.requires_grad = True # train all layers
+ if any(x in k for x in freeze):
+ print(f'freezing {k}')
+ v.requires_grad = False
+```
+
+To see a list of module names:
+
+```python
+for k, v in model.named_parameters():
+ print(k)
+
+# Output
+model.0.conv.conv.weight
+model.0.conv.bn.weight
+model.0.conv.bn.bias
+model.1.conv.weight
+model.1.bn.weight
+model.1.bn.bias
+model.2.cv1.conv.weight
+model.2.cv1.bn.weight
+...
+model.23.m.0.cv2.bn.weight
+model.23.m.0.cv2.bn.bias
+model.24.m.0.weight
+model.24.m.0.bias
+model.24.m.1.weight
+model.24.m.1.bias
+model.24.m.2.weight
+model.24.m.2.bias
+```
+
+Looking at the model architecture we can see that the model backbone is layers 0-9:
+
+```yaml
+# YOLOv5 backbone
+ backbone:
+ # [from, number, module, args]
+ [[-1, 1, Focus, [64, 3]], # 0-P1/2
+ [-1, 1, Conv, [128, 3, 2]], # 1-P2/4
+ [-1, 3, BottleneckCSP, [128]],
+ [-1, 1, Conv, [256, 3, 2]], # 3-P3/8
+ [-1, 9, BottleneckCSP, [256]],
+ [-1, 1, Conv, [512, 3, 2]], # 5-P4/16
+ [-1, 9, BottleneckCSP, [512]],
+ [-1, 1, Conv, [1024, 3, 2]], # 7-P5/32
+ [-1, 1, SPP, [1024, [5, 9, 13]]],
+ [-1, 3, BottleneckCSP, [1024, False]], # 9
+ ]
+
+ # YOLOv5 head
+ head:
+ [[-1, 1, Conv, [512, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 6], 1, Concat, [1]], # cat backbone P4
+ [-1, 3, BottleneckCSP, [512, False]], # 13
+
+ [-1, 1, Conv, [256, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 4], 1, Concat, [1]], # cat backbone P3
+ [-1, 3, BottleneckCSP, [256, False]], # 17 (P3/8-small)
+
+ [-1, 1, Conv, [256, 3, 2]],
+ [[-1, 14], 1, Concat, [1]], # cat head P4
+ [-1, 3, BottleneckCSP, [512, False]], # 20 (P4/16-medium)
+
+ [-1, 1, Conv, [512, 3, 2]],
+ [[-1, 10], 1, Concat, [1]], # cat head P5
+ [-1, 3, BottleneckCSP, [1024, False]], # 23 (P5/32-large)
+
+ [[17, 20, 23], 1, Detect, [nc, anchors]], # Detect(P3, P4, P5)
+ ]
+```
+
+so we can define the freeze list to contain all modules with 'model.0.' - 'model.9.' in their names:
+
+```bash
+python train.py --freeze 10
+```
+
+## Freeze All Layers
+
+To freeze the full model except for the final output convolution layers in Detect(), we set freeze list to contain all modules with 'model.0.' - 'model.23.' in their names:
+
+```bash
+python train.py --freeze 24
+```
+
+## Results
+
+We train YOLOv5m on VOC on both of the above scenarios, along with a default model (no freezing), starting from the official COCO pretrained `--weights yolov5m.pt`:
+
+```python
+train.py --batch 48 --weights yolov5m.pt --data voc.yaml --epochs 50 --cache --img 512 --hyp hyp.finetune.yaml
+```
+
+### Accuracy Comparison
+
+The results show that freezing speeds up training, but reduces final accuracy slightly.
+
+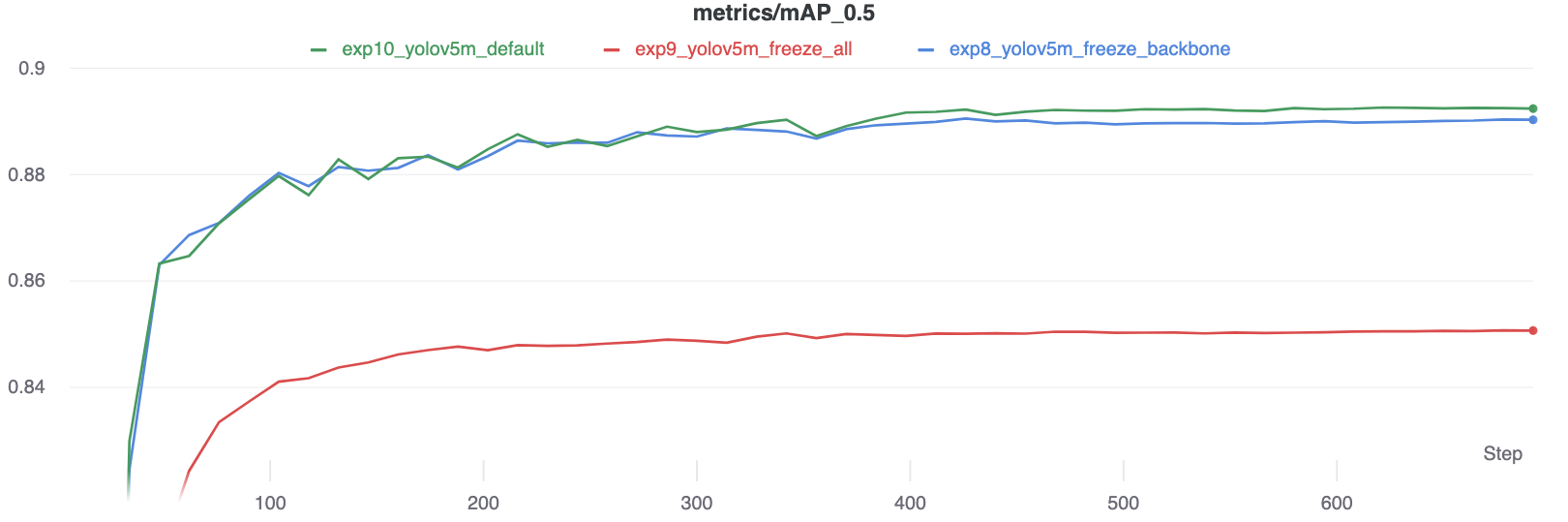
+
+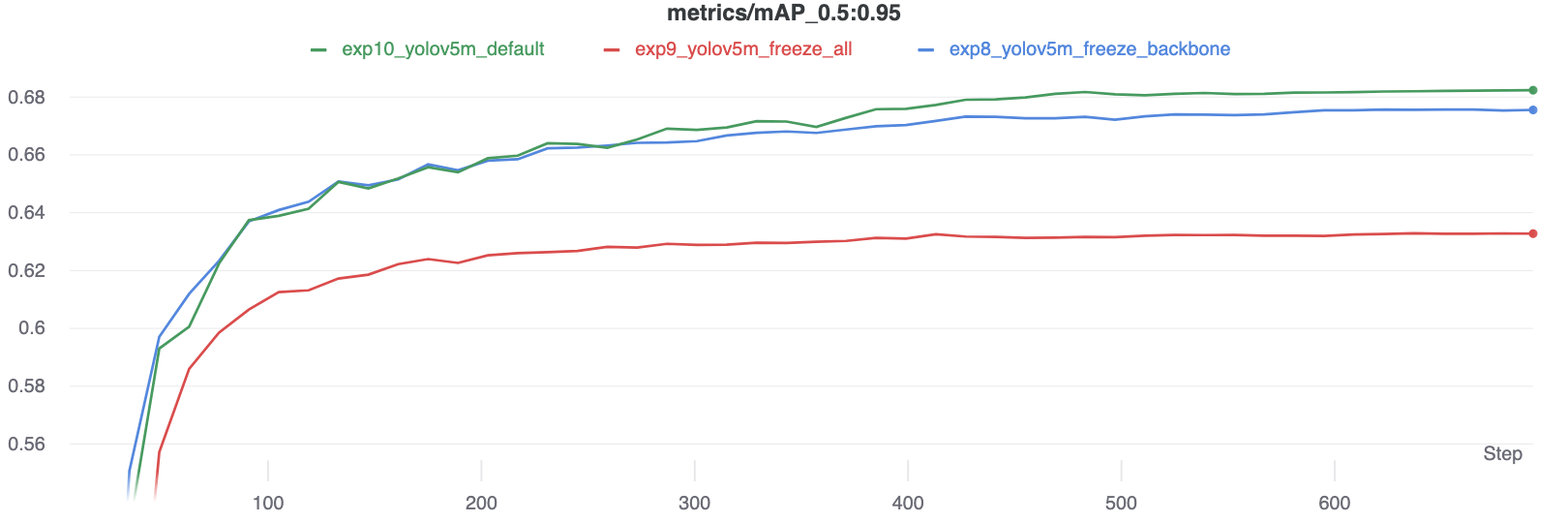
+
+
+
+### GPU Utilization Comparison
+
+Interestingly, the more modules are frozen the less GPU memory is required to train, and the lower GPU utilization. This indicates that larger models, or models trained at larger --image-size may benefit from freezing in order to train faster.
+
+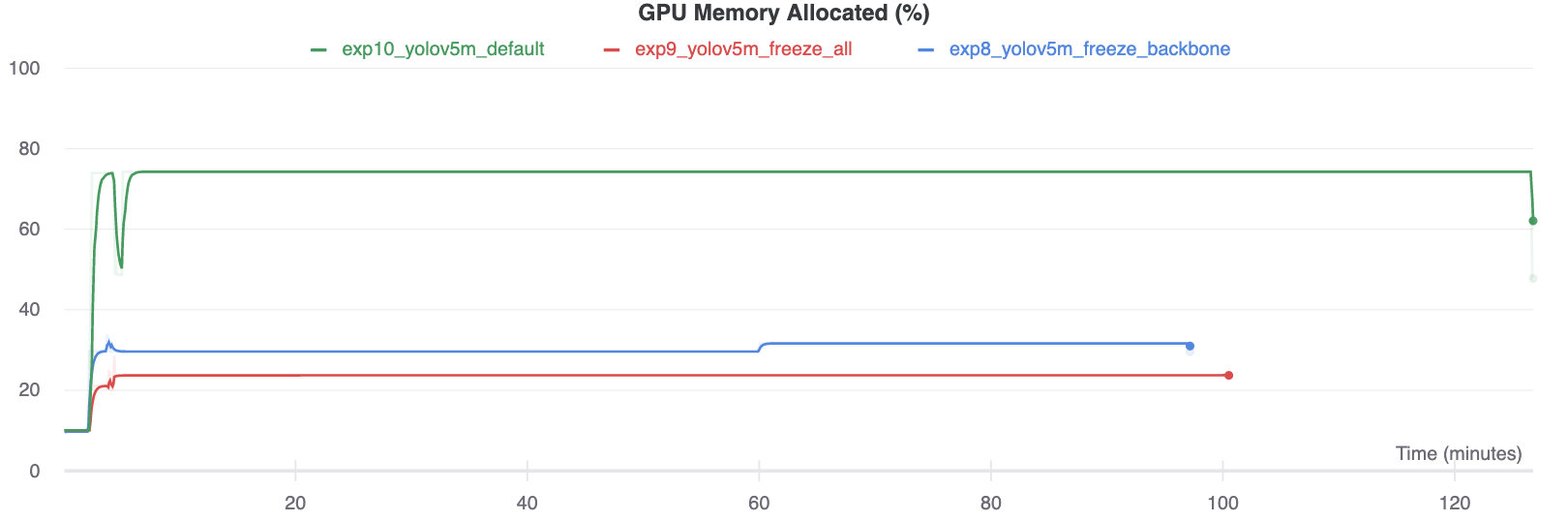
+
+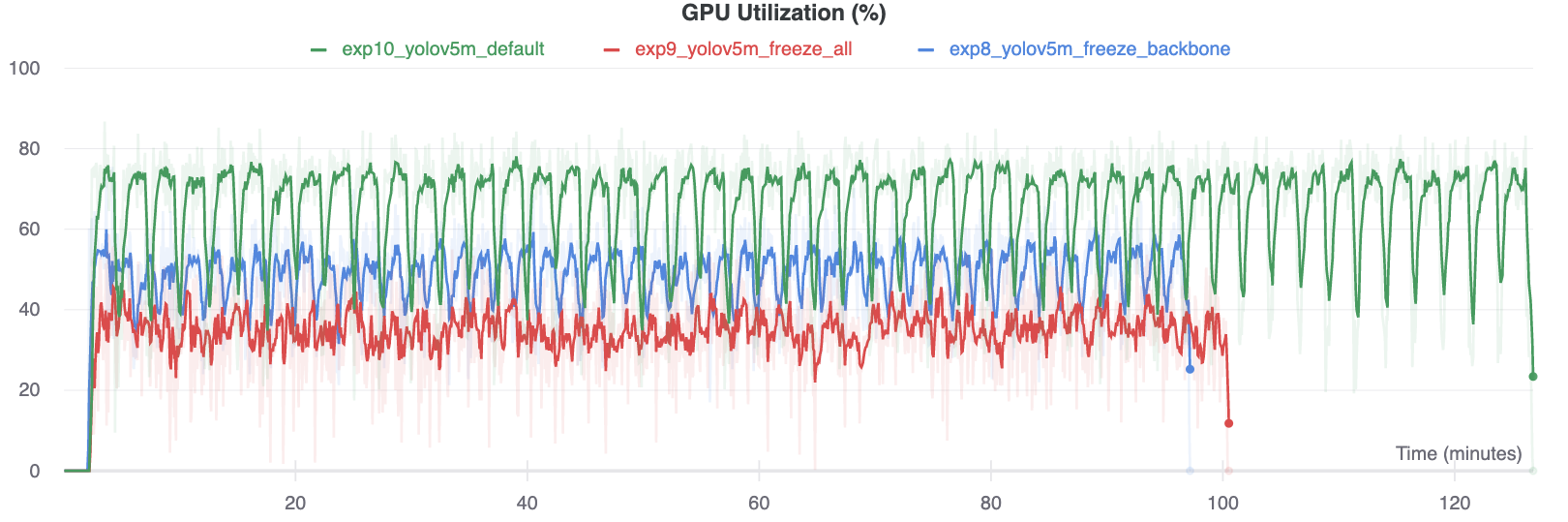
+
+## Environments
+
+YOLOv5 is designed to be run in the following up-to-date verified environments (with all dependencies including [CUDA](https://developer.nvidia.com/cuda)/[CUDNN](https://developer.nvidia.com/cudnn), [Python](https://www.python.org/) and [PyTorch](https://pytorch.org/) preinstalled):
+
+- **Notebooks** with free GPU:
+- **Google Cloud** Deep Learning VM. See [GCP Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/google_cloud_quickstart_tutorial/)
+- **Amazon** Deep Learning AMI. See [AWS Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/aws_quickstart_tutorial/)
+- **Docker Image**. See [Docker Quickstart Guide](https://docs.ultralytics.com/yolov5/environments/docker_image_quickstart_tutorial/)
+
+## Status
+
+
+
+If this badge is green, all [YOLOv5 GitHub Actions](https://github.com/ultralytics/yolov5/actions) Continuous Integration (CI) tests are currently passing. CI tests verify correct operation of YOLOv5 [training](https://github.com/ultralytics/yolov5/blob/master/train.py), [validation](https://github.com/ultralytics/yolov5/blob/master/val.py), [inference](https://github.com/ultralytics/yolov5/blob/master/detect.py), [export](https://github.com/ultralytics/yolov5/blob/master/export.py) and [benchmarks](https://github.com/ultralytics/yolov5/blob/master/benchmarks.py) on macOS, Windows, and Ubuntu every 24 hours and on every commit.
\ No newline at end of file
diff --git a/yolov8/examples/README.md b/yolov8/examples/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..120c04be5f81b7e35a63de3f694545a1337b6384
--- /dev/null
+++ b/yolov8/examples/README.md
@@ -0,0 +1,29 @@
+## Ultralytics YOLOv8 Example Applications
+
+This repository features a collection of real-world applications and walkthroughs, provided as either Python files or notebooks. Explore the examples below to see how YOLOv8 can be integrated into various applications.
+
+### Ultralytics YOLO Example Applications
+
+| Title | Format | Contributor |
+| -------------------------------------------------------------------------------------------------------------- | ------------------ | --------------------------------------------------- |
+| [YOLO ONNX Detection Inference with C++](./YOLOv8-CPP-Inference) | C++/ONNX | [Justas Bartnykas](https://github.com/JustasBart) |
+| [YOLO OpenCV ONNX Detection Python](./YOLOv8-OpenCV-ONNX-Python) | OpenCV/Python/ONNX | [Farid Inawan](https://github.com/frdteknikelektro) |
+| [YOLOv8 .NET ONNX ImageSharp](https://github.com/dme-compunet/YOLOv8) | C#/ONNX/ImageSharp | [Compunet](https://github.com/dme-compunet) |
+| [YOLO .Net ONNX Detection C#](https://www.nuget.org/packages/Yolov8.Net) | C# .Net | [Samuel Stainback](https://github.com/sstainba) |
+| [YOLOv8 on NVIDIA Jetson(TensorRT and DeepStream)](https://wiki.seeedstudio.com/YOLOv8-DeepStream-TRT-Jetson/) | Python | [Lakshantha](https://github.com/lakshanthad) |
+| [YOLOv8 ONNXRuntime Python](./YOLOv8-ONNXRuntime) | Python/ONNXRuntime | [Semih Demirel](https://github.com/semihhdemirel) |
+| [YOLOv8-ONNXRuntime-CPP](./YOLOv8-ONNXRuntime-CPP) | C++/ONNXRuntime | [DennisJcy](https://github.com/DennisJcy) |
+| [RTDETR ONNXRuntime C#](https://github.com/Kayzwer/yolo-cs/blob/master/RTDETR.cs) | C#/ONNX | [Kayzwer](https://github.com/Kayzwer) |
+
+### How to Contribute
+
+We welcome contributions from the community in the form of examples, applications, and guides. To contribute, please follow these steps:
+
+1. Create a pull request (PR) with the `[Example]` prefix in the title, adding your project folder to the `examples/` directory in the repository.
+1. Ensure that your project meets the following criteria:
+ - Utilizes the `ultralytics` package.
+ - Includes a `README.md` file with instructions on how to run the project.
+ - Avoids adding large assets or dependencies unless absolutely necessary.
+ - The contributor is expected to provide support for issues related to their examples.
+
+If you have any questions or concerns about these requirements, please submit a PR, and we will be more than happy to guide you.
diff --git a/yolov8/examples/YOLOv8-CPP-Inference/CMakeLists.txt b/yolov8/examples/YOLOv8-CPP-Inference/CMakeLists.txt
new file mode 100644
index 0000000000000000000000000000000000000000..bc2f33fffd447ffef995a570f01e0aefdd071acb
--- /dev/null
+++ b/yolov8/examples/YOLOv8-CPP-Inference/CMakeLists.txt
@@ -0,0 +1,28 @@
+cmake_minimum_required(VERSION 3.5)
+
+project(Yolov8CPPInference VERSION 0.1)
+
+set(CMAKE_INCLUDE_CURRENT_DIR ON)
+
+# CUDA
+set(CUDA_TOOLKIT_ROOT_DIR "/usr/local/cuda")
+find_package(CUDA 11 REQUIRED)
+
+set(CMAKE_CUDA_STANDARD 11)
+set(CMAKE_CUDA_STANDARD_REQUIRED ON)
+# !CUDA
+
+# OpenCV
+find_package(OpenCV REQUIRED)
+include_directories(${OpenCV_INCLUDE_DIRS})
+# !OpenCV
+
+set(PROJECT_SOURCES
+ main.cpp
+
+ inference.h
+ inference.cpp
+)
+
+add_executable(Yolov8CPPInference ${PROJECT_SOURCES})
+target_link_libraries(Yolov8CPPInference ${OpenCV_LIBS})
diff --git a/yolov8/examples/YOLOv8-CPP-Inference/README.md b/yolov8/examples/YOLOv8-CPP-Inference/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..8e32cbbcaf8eadb298cecbbd3dc57d645003a582
--- /dev/null
+++ b/yolov8/examples/YOLOv8-CPP-Inference/README.md
@@ -0,0 +1,50 @@
+# YOLOv8/YOLOv5 Inference C++
+
+This example demonstrates how to perform inference using YOLOv8 and YOLOv5 models in C++ with OpenCV's DNN API.
+
+## Usage
+
+```bash
+git clone ultralytics
+cd ultralytics
+pip install .
+cd examples/cpp_
+
+# Add a **yolov8\_.onnx** and/or **yolov5\_.onnx** model(s) to the ultralytics folder.
+# Edit the **main.cpp** to change the **projectBasePath** to match your user.
+
+# Note that by default the CMake file will try and import the CUDA library to be used with the OpenCVs dnn (cuDNN) GPU Inference.
+# If your OpenCV build does not use CUDA/cuDNN you can remove that import call and run the example on CPU.
+
+mkdir build
+cd build
+cmake ..
+make
+./Yolov8CPPInference
+```
+
+## Exporting YOLOv8 and YOLOv5 Models
+
+To export YOLOv8 models:
+
+```commandline
+yolo export model=yolov8s.pt imgsz=480,640 format=onnx opset=12
+```
+
+To export YOLOv5 models:
+
+```commandline
+python3 export.py --weights yolov5s.pt --img 480 640 --include onnx --opset 12
+```
+
+yolov8s.onnx:
+
+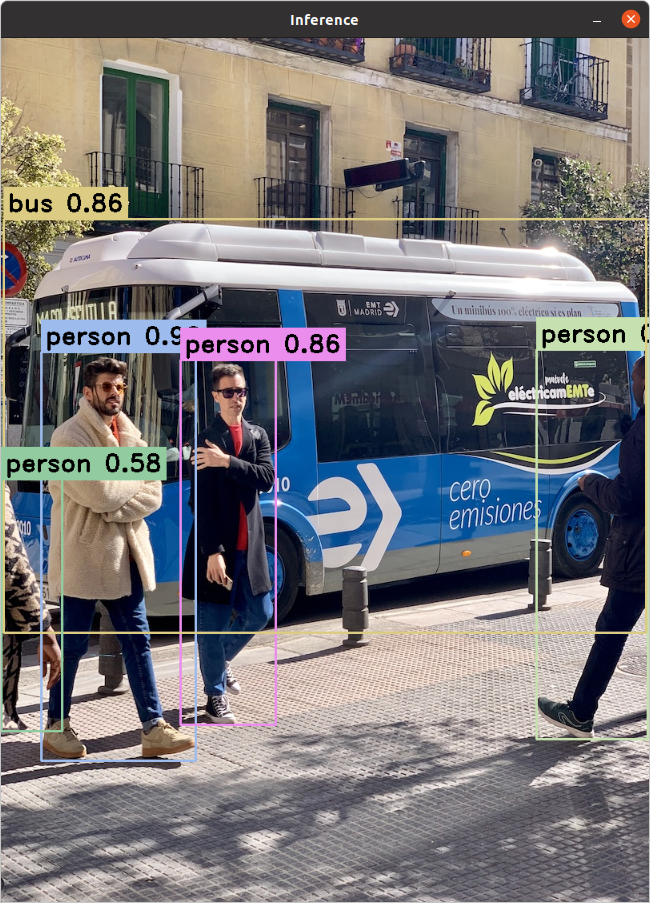
+
+yolov5s.onnx:
+
+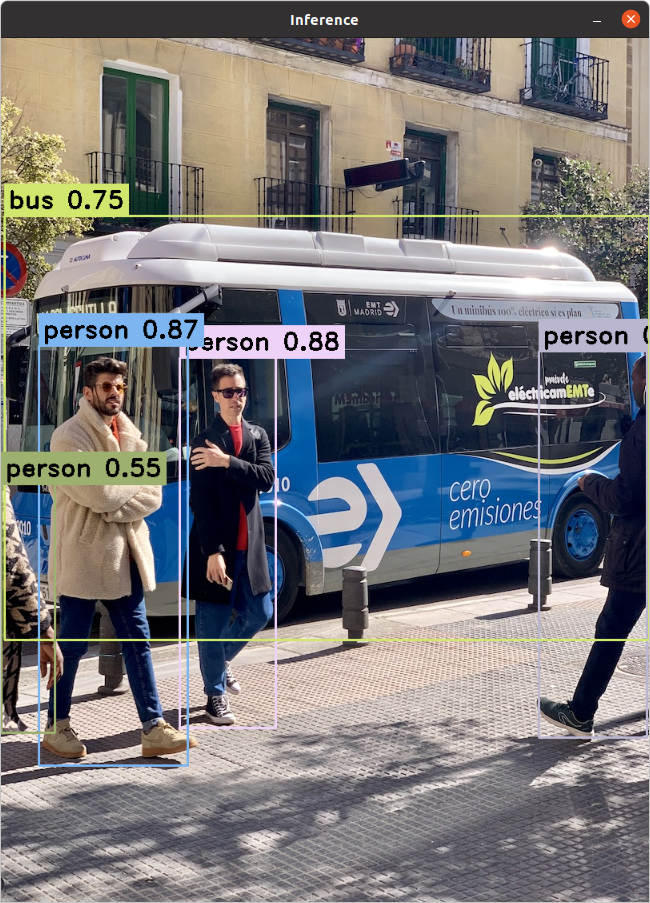
+
+This repository utilizes OpenCV's DNN API to run ONNX exported models of YOLOv5 and YOLOv8. In theory, it should work for YOLOv6 and YOLOv7 as well, but they have not been tested. Note that the example networks are exported with rectangular (640x480) resolutions, but any exported resolution will work. You may want to use the letterbox approach for square images, depending on your use case.
+
+The **main** branch version uses Qt as a GUI wrapper. The primary focus here is the **Inference** class file, which demonstrates how to transpose YOLOv8 models to work as YOLOv5 models.
diff --git a/yolov8/examples/YOLOv8-CPP-Inference/inference.cpp b/yolov8/examples/YOLOv8-CPP-Inference/inference.cpp
new file mode 100644
index 0000000000000000000000000000000000000000..12c26079bcbf1b69b92e2305830dce2474a37288
--- /dev/null
+++ b/yolov8/examples/YOLOv8-CPP-Inference/inference.cpp
@@ -0,0 +1,185 @@
+#include "inference.h"
+
+Inference::Inference(const std::string &onnxModelPath, const cv::Size &modelInputShape, const std::string &classesTxtFile, const bool &runWithCuda)
+{
+ modelPath = onnxModelPath;
+ modelShape = modelInputShape;
+ classesPath = classesTxtFile;
+ cudaEnabled = runWithCuda;
+
+ loadOnnxNetwork();
+ // loadClassesFromFile(); The classes are hard-coded for this example
+}
+
+std::vector Inference::runInference(const cv::Mat &input)
+{
+ cv::Mat modelInput = input;
+ if (letterBoxForSquare && modelShape.width == modelShape.height)
+ modelInput = formatToSquare(modelInput);
+
+ cv::Mat blob;
+ cv::dnn::blobFromImage(modelInput, blob, 1.0/255.0, modelShape, cv::Scalar(), true, false);
+ net.setInput(blob);
+
+ std::vector outputs;
+ net.forward(outputs, net.getUnconnectedOutLayersNames());
+
+ int rows = outputs[0].size[1];
+ int dimensions = outputs[0].size[2];
+
+ bool yolov8 = false;
+ // yolov5 has an output of shape (batchSize, 25200, 85) (Num classes + box[x,y,w,h] + confidence[c])
+ // yolov8 has an output of shape (batchSize, 84, 8400) (Num classes + box[x,y,w,h])
+ if (dimensions > rows) // Check if the shape[2] is more than shape[1] (yolov8)
+ {
+ yolov8 = true;
+ rows = outputs[0].size[2];
+ dimensions = outputs[0].size[1];
+
+ outputs[0] = outputs[0].reshape(1, dimensions);
+ cv::transpose(outputs[0], outputs[0]);
+ }
+ float *data = (float *)outputs[0].data;
+
+ float x_factor = modelInput.cols / modelShape.width;
+ float y_factor = modelInput.rows / modelShape.height;
+
+ std::vector class_ids;
+ std::vector confidences;
+ std::vector boxes;
+
+ for (int i = 0; i < rows; ++i)
+ {
+ if (yolov8)
+ {
+ float *classes_scores = data+4;
+
+ cv::Mat scores(1, classes.size(), CV_32FC1, classes_scores);
+ cv::Point class_id;
+ double maxClassScore;
+
+ minMaxLoc(scores, 0, &maxClassScore, 0, &class_id);
+
+ if (maxClassScore > modelScoreThreshold)
+ {
+ confidences.push_back(maxClassScore);
+ class_ids.push_back(class_id.x);
+
+ float x = data[0];
+ float y = data[1];
+ float w = data[2];
+ float h = data[3];
+
+ int left = int((x - 0.5 * w) * x_factor);
+ int top = int((y - 0.5 * h) * y_factor);
+
+ int width = int(w * x_factor);
+ int height = int(h * y_factor);
+
+ boxes.push_back(cv::Rect(left, top, width, height));
+ }
+ }
+ else // yolov5
+ {
+ float confidence = data[4];
+
+ if (confidence >= modelConfidenceThreshold)
+ {
+ float *classes_scores = data+5;
+
+ cv::Mat scores(1, classes.size(), CV_32FC1, classes_scores);
+ cv::Point class_id;
+ double max_class_score;
+
+ minMaxLoc(scores, 0, &max_class_score, 0, &class_id);
+
+ if (max_class_score > modelScoreThreshold)
+ {
+ confidences.push_back(confidence);
+ class_ids.push_back(class_id.x);
+
+ float x = data[0];
+ float y = data[1];
+ float w = data[2];
+ float h = data[3];
+
+ int left = int((x - 0.5 * w) * x_factor);
+ int top = int((y - 0.5 * h) * y_factor);
+
+ int width = int(w * x_factor);
+ int height = int(h * y_factor);
+
+ boxes.push_back(cv::Rect(left, top, width, height));
+ }
+ }
+ }
+
+ data += dimensions;
+ }
+
+ std::vector nms_result;
+ cv::dnn::NMSBoxes(boxes, confidences, modelScoreThreshold, modelNMSThreshold, nms_result);
+
+ std::vector detections{};
+ for (unsigned long i = 0; i < nms_result.size(); ++i)
+ {
+ int idx = nms_result[i];
+
+ Detection result;
+ result.class_id = class_ids[idx];
+ result.confidence = confidences[idx];
+
+ std::random_device rd;
+ std::mt19937 gen(rd());
+ std::uniform_int_distribution dis(100, 255);
+ result.color = cv::Scalar(dis(gen),
+ dis(gen),
+ dis(gen));
+
+ result.className = classes[result.class_id];
+ result.box = boxes[idx];
+
+ detections.push_back(result);
+ }
+
+ return detections;
+}
+
+void Inference::loadClassesFromFile()
+{
+ std::ifstream inputFile(classesPath);
+ if (inputFile.is_open())
+ {
+ std::string classLine;
+ while (std::getline(inputFile, classLine))
+ classes.push_back(classLine);
+ inputFile.close();
+ }
+}
+
+void Inference::loadOnnxNetwork()
+{
+ net = cv::dnn::readNetFromONNX(modelPath);
+ if (cudaEnabled)
+ {
+ std::cout << "\nRunning on CUDA" << std::endl;
+ net.setPreferableBackend(cv::dnn::DNN_BACKEND_CUDA);
+ net.setPreferableTarget(cv::dnn::DNN_TARGET_CUDA);
+ }
+ else
+ {
+ std::cout << "\nRunning on CPU" << std::endl;
+ net.setPreferableBackend(cv::dnn::DNN_BACKEND_OPENCV);
+ net.setPreferableTarget(cv::dnn::DNN_TARGET_CPU);
+ }
+}
+
+cv::Mat Inference::formatToSquare(const cv::Mat &source)
+{
+ int col = source.cols;
+ int row = source.rows;
+ int _max = MAX(col, row);
+ cv::Mat result = cv::Mat::zeros(_max, _max, CV_8UC3);
+ source.copyTo(result(cv::Rect(0, 0, col, row)));
+ return result;
+}
diff --git a/yolov8/examples/YOLOv8-CPP-Inference/inference.h b/yolov8/examples/YOLOv8-CPP-Inference/inference.h
new file mode 100644
index 0000000000000000000000000000000000000000..dc6149f1875654bf52ccc7497deb5ea8b06f57ca
--- /dev/null
+++ b/yolov8/examples/YOLOv8-CPP-Inference/inference.h
@@ -0,0 +1,52 @@
+#ifndef INFERENCE_H
+#define INFERENCE_H
+
+// Cpp native
+#include
+#include
+#include
+#include
+
+// OpenCV / DNN / Inference
+#include
+#include
+#include
+
+struct Detection
+{
+ int class_id{0};
+ std::string className{};
+ float confidence{0.0};
+ cv::Scalar color{};
+ cv::Rect box{};
+};
+
+class Inference
+{
+public:
+ Inference(const std::string &onnxModelPath, const cv::Size &modelInputShape = {640, 640}, const std::string &classesTxtFile = "", const bool &runWithCuda = true);
+ std::vector runInference(const cv::Mat &input);
+
+private:
+ void loadClassesFromFile();
+ void loadOnnxNetwork();
+ cv::Mat formatToSquare(const cv::Mat &source);
+
+ std::string modelPath{};
+ std::string classesPath{};
+ bool cudaEnabled{};
+
+ std::vector classes{"person", "bicycle", "car", "motorcycle", "airplane", "bus", "train", "truck", "boat", "traffic light", "fire hydrant", "stop sign", "parking meter", "bench", "bird", "cat", "dog", "horse", "sheep", "cow", "elephant", "bear", "zebra", "giraffe", "backpack", "umbrella", "handbag", "tie", "suitcase", "frisbee", "skis", "snowboard", "sports ball", "kite", "baseball bat", "baseball glove", "skateboard", "surfboard", "tennis racket", "bottle", "wine glass", "cup", "fork", "knife", "spoon", "bowl", "banana", "apple", "sandwich", "orange", "broccoli", "carrot", "hot dog", "pizza", "donut", "cake", "chair", "couch", "potted plant", "bed", "dining table", "toilet", "tv", "laptop", "mouse", "remote", "keyboard", "cell phone", "microwave", "oven", "toaster", "sink", "refrigerator", "book", "clock", "vase", "scissors", "teddy bear", "hair drier", "toothbrush"};
+
+ cv::Size2f modelShape{};
+
+ float modelConfidenceThreshold {0.25};
+ float modelScoreThreshold {0.45};
+ float modelNMSThreshold {0.50};
+
+ bool letterBoxForSquare = true;
+
+ cv::dnn::Net net;
+};
+
+#endif // INFERENCE_H
diff --git a/yolov8/examples/YOLOv8-CPP-Inference/main.cpp b/yolov8/examples/YOLOv8-CPP-Inference/main.cpp
new file mode 100644
index 0000000000000000000000000000000000000000..6d1ba988f552b813c0e4fda90dee31cc11bdcceb
--- /dev/null
+++ b/yolov8/examples/YOLOv8-CPP-Inference/main.cpp
@@ -0,0 +1,70 @@
+#include
+#include
+#include
+
+#include
+
+#include "inference.h"
+
+using namespace std;
+using namespace cv;
+
+int main(int argc, char **argv)
+{
+ std::string projectBasePath = "/home/user/ultralytics"; // Set your ultralytics base path
+
+ bool runOnGPU = true;
+
+ //
+ // Pass in either:
+ //
+ // "yolov8s.onnx" or "yolov5s.onnx"
+ //
+ // To run Inference with yolov8/yolov5 (ONNX)
+ //
+
+ // Note that in this example the classes are hard-coded and 'classes.txt' is a place holder.
+ Inference inf(projectBasePath + "/yolov8s.onnx", cv::Size(640, 480), "classes.txt", runOnGPU);
+
+ std::vector imageNames;
+ imageNames.push_back(projectBasePath + "/ultralytics/assets/bus.jpg");
+ imageNames.push_back(projectBasePath + "/ultralytics/assets/zidane.jpg");
+
+ for (int i = 0; i < imageNames.size(); ++i)
+ {
+ cv::Mat frame = cv::imread(imageNames[i]);
+
+ // Inference starts here...
+ std::vector output = inf.runInference(frame);
+
+ int detections = output.size();
+ std::cout << "Number of detections:" << detections << std::endl;
+
+ for (int i = 0; i < detections; ++i)
+ {
+ Detection detection = output[i];
+
+ cv::Rect box = detection.box;
+ cv::Scalar color = detection.color;
+
+ // Detection box
+ cv::rectangle(frame, box, color, 2);
+
+ // Detection box text
+ std::string classString = detection.className + ' ' + std::to_string(detection.confidence).substr(0, 4);
+ cv::Size textSize = cv::getTextSize(classString, cv::FONT_HERSHEY_DUPLEX, 1, 2, 0);
+ cv::Rect textBox(box.x, box.y - 40, textSize.width + 10, textSize.height + 20);
+
+ cv::rectangle(frame, textBox, color, cv::FILLED);
+ cv::putText(frame, classString, cv::Point(box.x + 5, box.y - 10), cv::FONT_HERSHEY_DUPLEX, 1, cv::Scalar(0, 0, 0), 2, 0);
+ }
+ // Inference ends here...
+
+ // This is only for preview purposes
+ float scale = 0.8;
+ cv::resize(frame, frame, cv::Size(frame.cols*scale, frame.rows*scale));
+ cv::imshow("Inference", frame);
+
+ cv::waitKey(-1);
+ }
+}
diff --git a/yolov8/examples/YOLOv8-ONNXRuntime-CPP/README.md b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..c49866497ba05fff6af4755cdf418320895c5b50
--- /dev/null
+++ b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/README.md
@@ -0,0 +1,54 @@
+# YOLOv8 OnnxRuntime C++
+
+This example demonstrates how to perform inference using YOLOv8 in C++ with ONNX Runtime and OpenCV's API.
+
+We recommend using Visual Studio to build the project.
+
+## Benefits
+
+- Friendly for deployment in the industrial sector.
+- Faster than OpenCV's DNN inference on both CPU and GPU.
+- Supports CUDA acceleration.
+- Easy to add FP16 inference (using template functions).
+
+## Exporting YOLOv8 Models
+
+To export YOLOv8 models, use the following Python script:
+
+```python
+from ultralytics import YOLO
+
+# Load a YOLOv8 model
+model = YOLO("yolov8n.pt")
+
+# Export the model
+model.export(format="onnx", opset=12, simplify=True, dynamic=False, imgsz=640)
+```
+
+## Dependencies
+
+| Dependency | Version |
+| ----------------------- | -------- |
+| Onnxruntime-win-x64-gpu | >=1.14.1 |
+| OpenCV | >=4.0.0 |
+| C++ | >=17 |
+
+Note: The dependency on C++17 is due to the usage of the C++17 filesystem feature.
+
+## Usage
+
+```c++
+// CPU inference
+DCSP_INIT_PARAM params{ model_path, YOLO_ORIGIN_V8, {imgsz_w, imgsz_h}, class_num, 0.1, 0.5, false};
+// GPU inference
+DCSP_INIT_PARAM params{ model_path, YOLO_ORIGIN_V8, {imgsz_w, imgsz_h}, class_num, 0.1, 0.5, true};
+
+// Load your image
+cv::Mat img = cv::imread(img_path);
+
+char* ret = p1->CreateSession(params);
+
+ret = p->RunSession(img, res);
+```
+
+This repository should also work for YOLOv5, which needs a permute operator for the output of the YOLOv5 model, but this has not been implemented yet.
diff --git a/yolov8/examples/YOLOv8-ONNXRuntime-CPP/inference.cpp b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/inference.cpp
new file mode 100644
index 0000000000000000000000000000000000000000..5af395ddef39b0eca14297df29f5c09b2c758938
--- /dev/null
+++ b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/inference.cpp
@@ -0,0 +1,271 @@
+#include "inference.h"
+#include
+
+#define benchmark
+#define ELOG
+
+DCSP_CORE::DCSP_CORE()
+{
+
+}
+
+
+DCSP_CORE::~DCSP_CORE()
+{
+ delete session;
+}
+
+
+template
+char* BlobFromImage(cv::Mat& iImg, T& iBlob)
+{
+ int channels = iImg.channels();
+ int imgHeight = iImg.rows;
+ int imgWidth = iImg.cols;
+
+ for (int c = 0; c < channels; c++)
+ {
+ for (int h = 0; h < imgHeight; h++)
+ {
+ for (int w = 0; w < imgWidth; w++)
+ {
+ iBlob[c * imgWidth * imgHeight + h * imgWidth + w] = (std::remove_pointer::type)((iImg.at(h, w)[c]) / 255.0f);
+ }
+ }
+ }
+ return RET_OK;
+}
+
+
+char* PostProcess(cv::Mat& iImg, std::vector iImgSize, cv::Mat& oImg)
+{
+ cv::Mat img = iImg.clone();
+ cv::resize(iImg, oImg, cv::Size(iImgSize.at(0), iImgSize.at(1)));
+ if (img.channels() == 1)
+ {
+ cv::cvtColor(oImg, oImg, cv::COLOR_GRAY2BGR);
+ }
+ cv::cvtColor(oImg, oImg, cv::COLOR_BGR2RGB);
+ return RET_OK;
+}
+
+
+char* DCSP_CORE::CreateSession(DCSP_INIT_PARAM &iParams)
+{
+ char* Ret = RET_OK;
+ std::regex pattern("[\u4e00-\u9fa5]");
+ bool result = std::regex_search(iParams.ModelPath, pattern);
+ if (result)
+ {
+ Ret = "[DCSP_ONNX]:model path error.change your model path without chinese characters.";
+ std::cout << Ret << std::endl;
+ return Ret;
+ }
+ try
+ {
+ rectConfidenceThreshold = iParams.RectConfidenceThreshold;
+ iouThreshold = iParams.iouThreshold;
+ imgSize = iParams.imgSize;
+ modelType = iParams.ModelType;
+ env = Ort::Env(ORT_LOGGING_LEVEL_WARNING, "Yolo");
+ Ort::SessionOptions sessionOption;
+ if (iParams.CudaEnable)
+ {
+ cudaEnable = iParams.CudaEnable;
+ OrtCUDAProviderOptions cudaOption;
+ cudaOption.device_id = 0;
+ sessionOption.AppendExecutionProvider_CUDA(cudaOption);
+ //OrtOpenVINOProviderOptions ovOption;
+ //sessionOption.AppendExecutionProvider_OpenVINO(ovOption);
+ }
+ sessionOption.SetGraphOptimizationLevel(GraphOptimizationLevel::ORT_ENABLE_ALL);
+ sessionOption.SetIntraOpNumThreads(iParams.IntraOpNumThreads);
+ sessionOption.SetLogSeverityLevel(iParams.LogSeverityLevel);
+ int ModelPathSize = MultiByteToWideChar(CP_UTF8, 0, iParams.ModelPath.c_str(), static_cast(iParams.ModelPath.length()), nullptr, 0);
+ wchar_t* wide_cstr = new wchar_t[ModelPathSize + 1];
+ MultiByteToWideChar(CP_UTF8, 0, iParams.ModelPath.c_str(), static_cast(iParams.ModelPath.length()), wide_cstr, ModelPathSize);
+ wide_cstr[ModelPathSize] = L'\0';
+ const wchar_t* modelPath = wide_cstr;
+ session = new Ort::Session(env, modelPath, sessionOption);
+ Ort::AllocatorWithDefaultOptions allocator;
+ size_t inputNodesNum = session->GetInputCount();
+ for (size_t i = 0; i < inputNodesNum; i++)
+ {
+ Ort::AllocatedStringPtr input_node_name = session->GetInputNameAllocated(i, allocator);
+ char* temp_buf = new char[50];
+ strcpy(temp_buf, input_node_name.get());
+ inputNodeNames.push_back(temp_buf);
+ }
+
+ size_t OutputNodesNum = session->GetOutputCount();
+ for (size_t i = 0; i < OutputNodesNum; i++)
+ {
+ Ort::AllocatedStringPtr output_node_name = session->GetOutputNameAllocated(i, allocator);
+ char* temp_buf = new char[10];
+ strcpy(temp_buf, output_node_name.get());
+ outputNodeNames.push_back(temp_buf);
+ }
+ options = Ort::RunOptions{ nullptr };
+ WarmUpSession();
+ //std::cout << OrtGetApiBase()->GetVersionString() << std::endl;;
+ Ret = RET_OK;
+ return Ret;
+ }
+ catch (const std::exception& e)
+ {
+ const char* str1 = "[DCSP_ONNX]:";
+ const char* str2 = e.what();
+ std::string result = std::string(str1) + std::string(str2);
+ char* merged = new char[result.length() + 1];
+ std::strcpy(merged, result.c_str());
+ std::cout << merged << std::endl;
+ delete[] merged;
+ //return merged;
+ return "[DCSP_ONNX]:Create session failed.";
+ }
+
+}
+
+
+char* DCSP_CORE::RunSession(cv::Mat &iImg, std::vector& oResult)
+{
+#ifdef benchmark
+ clock_t starttime_1 = clock();
+#endif // benchmark
+
+ char* Ret = RET_OK;
+ cv::Mat processedImg;
+ PostProcess(iImg, imgSize, processedImg);
+ if (modelType < 4)
+ {
+ float* blob = new float[processedImg.total() * 3];
+ BlobFromImage(processedImg, blob);
+ std::vector inputNodeDims = { 1,3,imgSize.at(0),imgSize.at(1) };
+ TensorProcess(starttime_1, iImg, blob, inputNodeDims, oResult);
+ }
+
+ return Ret;
+}
+
+
+template
+char* DCSP_CORE::TensorProcess(clock_t& starttime_1, cv::Mat& iImg, N& blob, std::vector& inputNodeDims, std::vector& oResult)
+{
+ Ort::Value inputTensor = Ort::Value::CreateTensor::type>(Ort::MemoryInfo::CreateCpu(OrtDeviceAllocator, OrtMemTypeCPU), blob, 3 * imgSize.at(0) * imgSize.at(1), inputNodeDims.data(), inputNodeDims.size());
+#ifdef benchmark
+ clock_t starttime_2 = clock();
+#endif // benchmark
+ auto outputTensor = session->Run(options, inputNodeNames.data(), &inputTensor, 1, outputNodeNames.data(), outputNodeNames.size());
+#ifdef benchmark
+ clock_t starttime_3 = clock();
+#endif // benchmark
+ Ort::TypeInfo typeInfo = outputTensor.front().GetTypeInfo();
+ auto tensor_info = typeInfo.GetTensorTypeAndShapeInfo();
+ std::vectoroutputNodeDims = tensor_info.GetShape();
+ std::remove_pointer::type* output = outputTensor.front().GetTensorMutableData::type>();
+ delete blob;
+ switch (modelType)
+ {
+ case 1:
+ {
+ int strideNum = outputNodeDims[2];
+ int signalResultNum = outputNodeDims[1];
+ std::vector class_ids;
+ std::vector confidences;
+ std::vector boxes;
+ cv::Mat rowData(signalResultNum, strideNum, CV_32F, output);
+ rowData = rowData.t();
+
+ float* data = (float*)rowData.data;
+
+ float x_factor = iImg.cols / 640.;
+ float y_factor = iImg.rows / 640.;
+ for (int i = 0; i < strideNum; ++i)
+ {
+ float* classesScores = data + 4;
+ cv::Mat scores(1, classesNum, CV_32FC1, classesScores);
+ cv::Point class_id;
+ double maxClassScore;
+ cv::minMaxLoc(scores, 0, &maxClassScore, 0, &class_id);
+ if (maxClassScore > rectConfidenceThreshold)
+ {
+ confidences.push_back(maxClassScore);
+ class_ids.push_back(class_id.x);
+
+ float x = data[0];
+ float y = data[1];
+ float w = data[2];
+ float h = data[3];
+
+ int left = int((x - 0.5 * w) * x_factor);
+ int top = int((y - 0.5 * h) * y_factor);
+
+ int width = int(w * x_factor);
+ int height = int(h * y_factor);
+
+ boxes.push_back(cv::Rect(left, top, width, height));
+ }
+ data += signalResultNum;
+ }
+
+ std::vector nmsResult;
+ cv::dnn::NMSBoxes(boxes, confidences, rectConfidenceThreshold, iouThreshold, nmsResult);
+ for (int i = 0; i < nmsResult.size(); ++i)
+ {
+ int idx = nmsResult[i];
+ DCSP_RESULT result;
+ result.classId = class_ids[idx];
+ result.confidence = confidences[idx];
+ result.box = boxes[idx];
+ oResult.push_back(result);
+ }
+
+
+#ifdef benchmark
+ clock_t starttime_4 = clock();
+ double pre_process_time = (double)(starttime_2 - starttime_1) / CLOCKS_PER_SEC * 1000;
+ double process_time = (double)(starttime_3 - starttime_2) / CLOCKS_PER_SEC * 1000;
+ double post_process_time = (double)(starttime_4 - starttime_3) / CLOCKS_PER_SEC * 1000;
+ if (cudaEnable)
+ {
+ std::cout << "[DCSP_ONNX(CUDA)]: " << pre_process_time << "ms pre-process, " << process_time << "ms inference, " << post_process_time << "ms post-process." << std::endl;
+ }
+ else
+ {
+ std::cout << "[DCSP_ONNX(CPU)]: " << pre_process_time << "ms pre-process, " << process_time << "ms inference, " << post_process_time << "ms post-process." << std::endl;
+ }
+#endif // benchmark
+
+ break;
+ }
+ }
+ char* Ret = RET_OK;
+ return Ret;
+}
+
+
+char* DCSP_CORE::WarmUpSession()
+{
+ clock_t starttime_1 = clock();
+ char* Ret = RET_OK;
+ cv::Mat iImg = cv::Mat(cv::Size(imgSize.at(0), imgSize.at(1)), CV_8UC3);
+ cv::Mat processedImg;
+ PostProcess(iImg, imgSize, processedImg);
+ if (modelType < 4)
+ {
+ float* blob = new float[iImg.total() * 3];
+ BlobFromImage(processedImg, blob);
+ std::vector YOLO_input_node_dims = { 1,3,imgSize.at(0),imgSize.at(1) };
+ Ort::Value input_tensor = Ort::Value::CreateTensor(Ort::MemoryInfo::CreateCpu(OrtDeviceAllocator, OrtMemTypeCPU), blob, 3 * imgSize.at(0) * imgSize.at(1), YOLO_input_node_dims.data(), YOLO_input_node_dims.size());
+ auto output_tensors = session->Run(options, inputNodeNames.data(), &input_tensor, 1, outputNodeNames.data(), outputNodeNames.size());
+ delete[] blob;
+ clock_t starttime_4 = clock();
+ double post_process_time = (double)(starttime_4 - starttime_1) / CLOCKS_PER_SEC * 1000;
+ if (cudaEnable)
+ {
+ std::cout << "[DCSP_ONNX(CUDA)]: " << "Cuda warm-up cost " << post_process_time << " ms. " << std::endl;
+ }
+ }
+
+ return Ret;
+}
diff --git a/yolov8/examples/YOLOv8-ONNXRuntime-CPP/inference.h b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/inference.h
new file mode 100644
index 0000000000000000000000000000000000000000..d00fecd12b0ba1423d949d5254a0b74af8eff846
--- /dev/null
+++ b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/inference.h
@@ -0,0 +1,83 @@
+#pragma once
+
+#define _CRT_SECURE_NO_WARNINGS
+#define RET_OK nullptr
+
+#include
+#include
+#include
+#include "io.h"
+#include "direct.h"
+#include "opencv.hpp"
+#include
+#include "onnxruntime_cxx_api.h"
+
+
+enum MODEL_TYPE
+{
+ //FLOAT32 MODEL
+ YOLO_ORIGIN_V5 = 0,
+ YOLO_ORIGIN_V8 = 1,//only support v8 detector currently
+ YOLO_POSE_V8 = 2,
+ YOLO_CLS_V8 = 3
+};
+
+
+typedef struct _DCSP_INIT_PARAM
+{
+ std::string ModelPath;
+ MODEL_TYPE ModelType = YOLO_ORIGIN_V8;
+ std::vector imgSize={640, 640};
+
+ int classesNum=80;
+ float RectConfidenceThreshold = 0.6;
+ float iouThreshold = 0.5;
+ bool CudaEnable = false;
+ int LogSeverityLevel = 3;
+ int IntraOpNumThreads = 1;
+}DCSP_INIT_PARAM;
+
+
+typedef struct _DCSP_RESULT
+{
+ int classId;
+ float confidence;
+ cv::Rect box;
+}DCSP_RESULT;
+
+
+class DCSP_CORE
+{
+public:
+ DCSP_CORE();
+ ~DCSP_CORE();
+
+public:
+ char* CreateSession(DCSP_INIT_PARAM &iParams);
+
+
+ char* RunSession(cv::Mat &iImg, std::vector& oResult);
+
+
+ char* WarmUpSession();
+
+
+ template
+ char* TensorProcess(clock_t& starttime_1, cv::Mat& iImg, N& blob, std::vector& inputNodeDims, std::vector& oResult);
+
+
+private:
+ Ort::Env env;
+ Ort::Session* session;
+ bool cudaEnable;
+ Ort::RunOptions options;
+ std::vector inputNodeNames;
+ std::vector outputNodeNames;
+
+
+ int classesNum;
+ MODEL_TYPE modelType;
+ std::vector imgSize;
+ float rectConfidenceThreshold;
+ float iouThreshold;
+};
diff --git a/yolov8/examples/YOLOv8-ONNXRuntime-CPP/main.cpp b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/main.cpp
new file mode 100644
index 0000000000000000000000000000000000000000..f13d78250abea8c7a48cef0ab78e9bcd1ed0bc3b
--- /dev/null
+++ b/yolov8/examples/YOLOv8-ONNXRuntime-CPP/main.cpp
@@ -0,0 +1,44 @@
+#include
+#include
+#include "inference.h"
+#include
+
+
+
+void file_iterator(DCSP_CORE*& p)
+{
+ std::filesystem::path img_path = R"(E:\project\Project_C++\DCPS_ONNX\TEST_ORIGIN)";
+ int k = 0;
+ for (auto& i : std::filesystem::directory_iterator(img_path))
+ {
+ if (i.path().extension() == ".jpg")
+ {
+ std::string img_path = i.path().string();
+ //std::cout << img_path << std::endl;
+ cv::Mat img = cv::imread(img_path);
+ std::vector res;
+ char* ret = p->RunSession(img, res);
+ for (int i = 0; i < res.size(); i++)
+ {
+ cv::rectangle(img, res.at(i).box, cv::Scalar(125, 123, 0), 3);
+ }
+
+ k++;
+ cv::imshow("TEST_ORIGIN", img);
+ cv::waitKey(0);
+ cv::destroyAllWindows();
+ //cv::imwrite("E:\\output\\" + std::to_string(k) + ".png", img);
+ }
+ }
+}
+
+
+
+int main()
+{
+ DCSP_CORE* p1 = new DCSP_CORE;
+ std::string model_path = "yolov8n.onnx";
+ DCSP_INIT_PARAM params{ model_path, YOLO_ORIGIN_V8, {640, 640}, 80, 0.1, 0.5, false };
+ char* ret = p1->CreateSession(params);
+ file_iterator(p1);
+}
diff --git a/yolov8/examples/YOLOv8-ONNXRuntime/README.md b/yolov8/examples/YOLOv8-ONNXRuntime/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..b206b2e2be97aec2255915c13ef3358ce450a4ba
--- /dev/null
+++ b/yolov8/examples/YOLOv8-ONNXRuntime/README.md
@@ -0,0 +1,43 @@
+# YOLOv8 - ONNX Runtime
+
+This project implements YOLOv8 using ONNX Runtime.
+
+## Installation
+
+To run this project, you need to install the required dependencies. The following instructions will guide you through the installation process.
+
+### Installing Required Dependencies
+
+You can install the required dependencies by running the following command:
+
+```bash
+pip install -r requirements.txt
+```
+
+### Installing `onnxruntime-gpu`
+
+If you have an NVIDIA GPU and want to leverage GPU acceleration, you can install the onnxruntime-gpu package using the following command:
+
+```bash
+pip install onnxruntime-gpu
+```
+
+Note: Make sure you have the appropriate GPU drivers installed on your system.
+
+### Installing `onnxruntime` (CPU version)
+
+If you don't have an NVIDIA GPU or prefer to use the CPU version of onnxruntime, you can install the onnxruntime package using the following command:
+
+```bash
+pip install onnxruntime
+```
+
+### Usage
+
+After successfully installing the required packages, you can run the YOLOv8 implementation using the following command:
+
+```bash
+python main.py --model yolov8n.onnx --img image.jpg --conf-thres 0.5 --iou-thres 0.5
+```
+
+Make sure to replace yolov8n.onnx with the path to your YOLOv8 ONNX model file, image.jpg with the path to your input image, and adjust the confidence threshold (conf-thres) and IoU threshold (iou-thres) values as needed.
diff --git a/yolov8/examples/YOLOv8-ONNXRuntime/main.py b/yolov8/examples/YOLOv8-ONNXRuntime/main.py
new file mode 100644
index 0000000000000000000000000000000000000000..9c5910053324e2ab4b8946c91f31088f1dfd7e7f
--- /dev/null
+++ b/yolov8/examples/YOLOv8-ONNXRuntime/main.py
@@ -0,0 +1,230 @@
+import argparse
+
+import cv2
+import numpy as np
+import onnxruntime as ort
+import torch
+
+from ultralytics.yolo.utils import ROOT, yaml_load
+from ultralytics.yolo.utils.checks import check_requirements, check_yaml
+
+
+class Yolov8:
+
+ def __init__(self, onnx_model, input_image, confidence_thres, iou_thres):
+ """
+ Initializes an instance of the Yolov8 class.
+
+ Args:
+ onnx_model: Path to the ONNX model.
+ input_image: Path to the input image.
+ confidence_thres: Confidence threshold for filtering detections.
+ iou_thres: IoU (Intersection over Union) threshold for non-maximum suppression.
+ """
+ self.onnx_model = onnx_model
+ self.input_image = input_image
+ self.confidence_thres = confidence_thres
+ self.iou_thres = iou_thres
+
+ # Load the class names from the COCO dataset
+ self.classes = yaml_load(check_yaml('coco128.yaml'))['names']
+
+ # Generate a color palette for the classes
+ self.color_palette = np.random.uniform(0, 255, size=(len(self.classes), 3))
+
+ def draw_detections(self, img, box, score, class_id):
+ """
+ Draws bounding boxes and labels on the input image based on the detected objects.
+
+ Args:
+ img: The input image to draw detections on.
+ box: Detected bounding box.
+ score: Corresponding detection score.
+ class_id: Class ID for the detected object.
+
+ Returns:
+ None
+ """
+
+ # Extract the coordinates of the bounding box
+ x1, y1, w, h = box
+
+ # Retrieve the color for the class ID
+ color = self.color_palette[class_id]
+
+ # Draw the bounding box on the image
+ cv2.rectangle(img, (int(x1), int(y1)), (int(x1 + w), int(y1 + h)), color, 2)
+
+ # Create the label text with class name and score
+ label = f'{self.classes[class_id]}: {score:.2f}'
+
+ # Calculate the dimensions of the label text
+ (label_width, label_height), _ = cv2.getTextSize(label, cv2.FONT_HERSHEY_SIMPLEX, 0.5, 1)
+
+ # Calculate the position of the label text
+ label_x = x1
+ label_y = y1 - 10 if y1 - 10 > label_height else y1 + 10
+
+ # Draw a filled rectangle as the background for the label text
+ cv2.rectangle(img, (label_x, label_y - label_height), (label_x + label_width, label_y + label_height), color,
+ cv2.FILLED)
+
+ # Draw the label text on the image
+ cv2.putText(img, label, (label_x, label_y), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 0), 1, cv2.LINE_AA)
+
+ def preprocess(self):
+ """
+ Preprocesses the input image before performing inference.
+
+ Returns:
+ image_data: Preprocessed image data ready for inference.
+ """
+ # Read the input image using OpenCV
+ self.img = cv2.imread(self.input_image)
+
+ # Get the height and width of the input image
+ self.img_height, self.img_width = self.img.shape[:2]
+
+ # Convert the image color space from BGR to RGB
+ img = cv2.cvtColor(self.img, cv2.COLOR_BGR2RGB)
+
+ # Resize the image to match the input shape
+ img = cv2.resize(img, (self.input_width, self.input_height))
+
+ # Normalize the image data by dividing it by 255.0
+ image_data = np.array(img) / 255.0
+
+ # Transpose the image to have the channel dimension as the first dimension
+ image_data = np.transpose(image_data, (2, 0, 1)) # Channel first
+
+ # Expand the dimensions of the image data to match the expected input shape
+ image_data = np.expand_dims(image_data, axis=0).astype(np.float32)
+
+ # Return the preprocessed image data
+ return image_data
+
+ def postprocess(self, input_image, output):
+ """
+ Performs post-processing on the model's output to extract bounding boxes, scores, and class IDs.
+
+ Args:
+ input_image (numpy.ndarray): The input image.
+ output (numpy.ndarray): The output of the model.
+
+ Returns:
+ numpy.ndarray: The input image with detections drawn on it.
+ """
+
+ # Transpose and squeeze the output to match the expected shape
+ outputs = np.transpose(np.squeeze(output[0]))
+
+ # Get the number of rows in the outputs array
+ rows = outputs.shape[0]
+
+ # Lists to store the bounding boxes, scores, and class IDs of the detections
+ boxes = []
+ scores = []
+ class_ids = []
+
+ # Calculate the scaling factors for the bounding box coordinates
+ x_factor = self.img_width / self.input_width
+ y_factor = self.img_height / self.input_height
+
+ # Iterate over each row in the outputs array
+ for i in range(rows):
+ # Extract the class scores from the current row
+ classes_scores = outputs[i][4:]
+
+ # Find the maximum score among the class scores
+ max_score = np.amax(classes_scores)
+
+ # If the maximum score is above the confidence threshold
+ if max_score >= self.confidence_thres:
+ # Get the class ID with the highest score
+ class_id = np.argmax(classes_scores)
+
+ # Extract the bounding box coordinates from the current row
+ x, y, w, h = outputs[i][0], outputs[i][1], outputs[i][2], outputs[i][3]
+
+ # Calculate the scaled coordinates of the bounding box
+ left = int((x - w / 2) * x_factor)
+ top = int((y - h / 2) * y_factor)
+ width = int(w * x_factor)
+ height = int(h * y_factor)
+
+ # Add the class ID, score, and box coordinates to the respective lists
+ class_ids.append(class_id)
+ scores.append(max_score)
+ boxes.append([left, top, width, height])
+
+ # Apply non-maximum suppression to filter out overlapping bounding boxes
+ indices = cv2.dnn.NMSBoxes(boxes, scores, self.confidence_thres, self.iou_thres)
+
+ # Iterate over the selected indices after non-maximum suppression
+ for i in indices:
+ # Get the box, score, and class ID corresponding to the index
+ box = boxes[i]
+ score = scores[i]
+ class_id = class_ids[i]
+
+ # Draw the detection on the input image
+ self.draw_detections(input_image, box, score, class_id)
+
+ # Return the modified input image
+ return input_image
+
+ def main(self):
+ """
+ Performs inference using an ONNX model and returns the output image with drawn detections.
+
+ Returns:
+ output_img: The output image with drawn detections.
+ """
+ # Create an inference session using the ONNX model and specify execution providers
+ session = ort.InferenceSession(self.onnx_model, providers=['CUDAExecutionProvider', 'CPUExecutionProvider'])
+
+ # Get the model inputs
+ model_inputs = session.get_inputs()
+
+ # Store the shape of the input for later use
+ input_shape = model_inputs[0].shape
+ self.input_width = input_shape[2]
+ self.input_height = input_shape[3]
+
+ # Preprocess the image data
+ img_data = self.preprocess()
+
+ # Run inference using the preprocessed image data
+ outputs = session.run(None, {model_inputs[0].name: img_data})
+
+ # Perform post-processing on the outputs to obtain output image.
+ output_img = self.postprocess(self.img, outputs)
+
+ # Return the resulting output image
+ return output_img
+
+
+if __name__ == '__main__':
+ # Create an argument parser to handle command-line arguments
+ parser = argparse.ArgumentParser()
+ parser.add_argument('--model', type=str, default='yolov8n.onnx', help='Input your ONNX model.')
+ parser.add_argument('--img', type=str, default=str(ROOT / 'assets/bus.jpg'), help='Path to input image.')
+ parser.add_argument('--conf-thres', type=float, default=0.5, help='Confidence threshold')
+ parser.add_argument('--iou-thres', type=float, default=0.5, help='NMS IoU threshold')
+ args = parser.parse_args()
+
+ # Check the requirements and select the appropriate backend (CPU or GPU)
+ check_requirements('onnxruntime-gpu' if torch.cuda.is_available() else 'onnxruntime')
+
+ # Create an instance of the Yolov8 class with the specified arguments
+ detection = Yolov8(args.model, args.img, args.conf_thres, args.iou_thres)
+
+ # Perform object detection and obtain the output image
+ output_image = detection.main()
+
+ # Display the output image in a window
+ cv2.namedWindow('Output', cv2.WINDOW_NORMAL)
+ cv2.imshow('Output', output_image)
+
+ # Wait for a key press to exit
+ cv2.waitKey(0)
diff --git a/yolov8/examples/YOLOv8-OpenCV-ONNX-Python/README.md b/yolov8/examples/YOLOv8-OpenCV-ONNX-Python/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..c9076fa55df4c874ac2a8f3705d11917c667d82b
--- /dev/null
+++ b/yolov8/examples/YOLOv8-OpenCV-ONNX-Python/README.md
@@ -0,0 +1,19 @@
+# YOLOv8 - OpenCV
+
+Implementation YOLOv8 on OpenCV using ONNX Format.
+
+Just simply clone and run
+
+```bash
+pip install -r requirements.txt
+python main.py --model yolov8n.onnx --img image.jpg
+```
+
+If you start from scratch:
+
+```bash
+pip install ultralytics
+yolo export model=yolov8n.pt imgsz=640 format=onnx opset=12
+```
+
+_\*Make sure to include "opset=12"_
diff --git a/yolov8/examples/YOLOv8-OpenCV-ONNX-Python/main.py b/yolov8/examples/YOLOv8-OpenCV-ONNX-Python/main.py
new file mode 100644
index 0000000000000000000000000000000000000000..d1f635c274fb292443e65e1c2f3bde488353cf97
--- /dev/null
+++ b/yolov8/examples/YOLOv8-OpenCV-ONNX-Python/main.py
@@ -0,0 +1,80 @@
+import argparse
+
+import cv2.dnn
+import numpy as np
+
+from ultralytics.yolo.utils import ROOT, yaml_load
+from ultralytics.yolo.utils.checks import check_yaml
+
+CLASSES = yaml_load(check_yaml('coco128.yaml'))['names']
+
+colors = np.random.uniform(0, 255, size=(len(CLASSES), 3))
+
+
+def draw_bounding_box(img, class_id, confidence, x, y, x_plus_w, y_plus_h):
+ label = f'{CLASSES[class_id]} ({confidence:.2f})'
+ color = colors[class_id]
+ cv2.rectangle(img, (x, y), (x_plus_w, y_plus_h), color, 2)
+ cv2.putText(img, label, (x - 10, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
+
+
+def main(onnx_model, input_image):
+ model: cv2.dnn.Net = cv2.dnn.readNetFromONNX(onnx_model)
+ original_image: np.ndarray = cv2.imread(input_image)
+ [height, width, _] = original_image.shape
+ length = max((height, width))
+ image = np.zeros((length, length, 3), np.uint8)
+ image[0:height, 0:width] = original_image
+ scale = length / 640
+
+ blob = cv2.dnn.blobFromImage(image, scalefactor=1 / 255, size=(640, 640), swapRB=True)
+ model.setInput(blob)
+ outputs = model.forward()
+
+ outputs = np.array([cv2.transpose(outputs[0])])
+ rows = outputs.shape[1]
+
+ boxes = []
+ scores = []
+ class_ids = []
+
+ for i in range(rows):
+ classes_scores = outputs[0][i][4:]
+ (minScore, maxScore, minClassLoc, (x, maxClassIndex)) = cv2.minMaxLoc(classes_scores)
+ if maxScore >= 0.25:
+ box = [
+ outputs[0][i][0] - (0.5 * outputs[0][i][2]), outputs[0][i][1] - (0.5 * outputs[0][i][3]),
+ outputs[0][i][2], outputs[0][i][3]]
+ boxes.append(box)
+ scores.append(maxScore)
+ class_ids.append(maxClassIndex)
+
+ result_boxes = cv2.dnn.NMSBoxes(boxes, scores, 0.25, 0.45, 0.5)
+
+ detections = []
+ for i in range(len(result_boxes)):
+ index = result_boxes[i]
+ box = boxes[index]
+ detection = {
+ 'class_id': class_ids[index],
+ 'class_name': CLASSES[class_ids[index]],
+ 'confidence': scores[index],
+ 'box': box,
+ 'scale': scale}
+ detections.append(detection)
+ draw_bounding_box(original_image, class_ids[index], scores[index], round(box[0] * scale), round(box[1] * scale),
+ round((box[0] + box[2]) * scale), round((box[1] + box[3]) * scale))
+
+ cv2.imshow('image', original_image)
+ cv2.waitKey(0)
+ cv2.destroyAllWindows()
+
+ return detections
+
+
+if __name__ == '__main__':
+ parser = argparse.ArgumentParser()
+ parser.add_argument('--model', default='yolov8n.onnx', help='Input your onnx model.')
+ parser.add_argument('--img', default=str(ROOT / 'assets/bus.jpg'), help='Path to input image.')
+ args = parser.parse_args()
+ main(args.model, args.img)
diff --git a/yolov8/examples/hub.ipynb b/yolov8/examples/hub.ipynb
new file mode 100644
index 0000000000000000000000000000000000000000..252891424d5f1aef40f7274c9b3672428b9bed70
--- /dev/null
+++ b/yolov8/examples/hub.ipynb
@@ -0,0 +1,103 @@
+{
+ "nbformat": 4,
+ "nbformat_minor": 0,
+ "metadata": {
+ "colab": {
+ "name": "Ultralytics HUB",
+ "provenance": []
+ },
+ "kernelspec": {
+ "name": "python3",
+ "display_name": "Python 3"
+ },
+ "language_info": {
+ "name": "python"
+ },
+ "accelerator": "GPU"
+ },
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "FIzICjaph_Wy"
+ },
+ "source": [
+ "\n",
+ "
\n",
+ "\n",
+ "\n",
+ "
\n",
+ " 
\n",
+ "
\n",
+ " 
\n",
+ "\n",
+ "Welcome to the [Ultralytics](https://ultralytics.com/) HUB notebook! \n",
+ "\n",
+ "This notebook allows you to train [YOLOv5](https://github.com/ultralytics/yolov5) and [YOLOv8](https://github.com/ultralytics/ultralytics) 🚀 models using [HUB](https://hub.ultralytics.com/). Please browse the YOLOv8
Docs for details, raise an issue on
GitHub for support, and join our
Discord community for questions and discussions!\n",
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "eRQ2ow94MiOv"
+ },
+ "source": [
+ "# Setup\n",
+ "\n",
+ "Pip install `ultralytics` and [dependencies](https://github.com/ultralytics/ultralytics/blob/main/requirements.txt) and check software and hardware."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "FyDnXd-n4c7Y",
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "outputId": "22dcbc27-9c6f-44fb-9745-620431f93793"
+ },
+ "source": [
+ "%pip install ultralytics # install\n",
+ "from ultralytics import YOLO, checks, hub\n",
+ "checks() # checks"
+ ],
+ "execution_count": null,
+ "outputs": [
+ {
+ "output_type": "stream",
+ "name": "stderr",
+ "text": [
+ "Ultralytics YOLOv8.0.64 🚀 Python-3.9.16 torch-2.0.0+cu118 CUDA:0 (Tesla T4, 15102MiB)\n",
+ "Setup complete ✅ (2 CPUs, 12.7 GB RAM, 28.3/166.8 GB disk)\n"
+ ]
+ }
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "cQ9BwaAqxAm4"
+ },
+ "source": [
+ "# Start\n",
+ "\n",
+ "Login with your [API key](https://hub.ultralytics.com/settings?tab=api+keys), select your YOLO 🚀 model and start training!"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "XSlZaJ9Iw_iZ"
+ },
+ "source": [
+ "hub.login('API_KEY') # use your API key\n",
+ "\n",
+ "model = YOLO('https://hub.ultralytics.com/MODEL_ID') # use your model URL\n",
+ "model.train() # train model"
+ ],
+ "execution_count": null,
+ "outputs": []
+ }
+ ]
+}
diff --git a/yolov8/examples/tutorial.ipynb b/yolov8/examples/tutorial.ipynb
new file mode 100644
index 0000000000000000000000000000000000000000..818bf7f583e324cb6da759722c8ebc49ddd012ff
--- /dev/null
+++ b/yolov8/examples/tutorial.ipynb
@@ -0,0 +1,704 @@
+{
+ "nbformat": 4,
+ "nbformat_minor": 0,
+ "metadata": {
+ "colab": {
+ "name": "YOLOv8 Tutorial",
+ "provenance": [],
+ "toc_visible": true
+ },
+ "kernelspec": {
+ "name": "python3",
+ "display_name": "Python 3"
+ },
+ "accelerator": "GPU"
+ },
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "t6MPjfT5NrKQ"
+ },
+ "source": [
+ "\n",
+ "\n",
+ "
\n",
+ " 
\n",
+ "\n",
+ "\n",
+ "
\n",
+ "

\n",
+ "

\n",
+ "

\n",
+ "
\n",
+ "\n",
+ "Welcome to the Ultralytics YOLOv8 🚀 notebook!
YOLOv8 is the latest version of the YOLO (You Only Look Once) AI models developed by
Ultralytics. This notebook serves as the starting point for exploring the various resources available to help you get started with YOLOv8 and understand its features and capabilities.\n",
+ "\n",
+ "YOLOv8 models are fast, accurate, and easy to use, making them ideal for various object detection and image segmentation tasks. They can be trained on large datasets and run on diverse hardware platforms, from CPUs to GPUs.\n",
+ "\n",
+ "We hope that the resources in this notebook will help you get the most out of YOLOv8. Please browse the YOLOv8
Docs for details, raise an issue on
GitHub for support, and join our
Discord community for questions and discussions!\n",
+ "\n",
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "7mGmQbAO5pQb"
+ },
+ "source": [
+ "# Setup\n",
+ "\n",
+ "Pip install `ultralytics` and [dependencies](https://github.com/ultralytics/ultralytics/blob/main/requirements.txt) and check software and hardware."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "wbvMlHd_QwMG",
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "outputId": "2ea6e0b9-1a62-4355-c246-5e8b7b1dafff"
+ },
+ "source": [
+ "%pip install ultralytics\n",
+ "import ultralytics\n",
+ "ultralytics.checks()"
+ ],
+ "execution_count": 1,
+ "outputs": [
+ {
+ "output_type": "stream",
+ "name": "stderr",
+ "text": [
+ "Ultralytics YOLOv8.0.71 🚀 Python-3.9.16 torch-2.0.0+cu118 CUDA:0 (Tesla T4, 15102MiB)\n",
+ "Setup complete ✅ (2 CPUs, 12.7 GB RAM, 23.3/166.8 GB disk)\n"
+ ]
+ }
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "4JnkELT0cIJg"
+ },
+ "source": [
+ "# 1. Predict\n",
+ "\n",
+ "YOLOv8 may be used directly in the Command Line Interface (CLI) with a `yolo` command for a variety of tasks and modes and accepts additional arguments, i.e. `imgsz=640`. See a full list of available `yolo` [arguments](https://docs.ultralytics.com/usage/cfg/) and other details in the [YOLOv8 Predict Docs](https://docs.ultralytics.com/modes/train/).\n"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "zR9ZbuQCH7FX",
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "outputId": "c578afbd-47cd-4d11-beec-8b5c31fcfba8"
+ },
+ "source": [
+ "# Run inference on an image with YOLOv8n\n",
+ "!yolo predict model=yolov8n.pt source='https://ultralytics.com/images/zidane.jpg'"
+ ],
+ "execution_count": 2,
+ "outputs": [
+ {
+ "output_type": "stream",
+ "name": "stdout",
+ "text": [
+ "Downloading https://github.com/ultralytics/assets/releases/download/v0.0.0/yolov8n.pt to yolov8n.pt...\n",
+ "100% 6.23M/6.23M [00:00<00:00, 195MB/s]\n",
+ "Ultralytics YOLOv8.0.71 🚀 Python-3.9.16 torch-2.0.0+cu118 CUDA:0 (Tesla T4, 15102MiB)\n",
+ "YOLOv8n summary (fused): 168 layers, 3151904 parameters, 0 gradients, 8.7 GFLOPs\n",
+ "\n",
+ "Downloading https://ultralytics.com/images/zidane.jpg to zidane.jpg...\n",
+ "100% 165k/165k [00:00<00:00, 51.7MB/s]\n",
+ "image 1/1 /content/zidane.jpg: 384x640 2 persons, 1 tie, 60.9ms\n",
+ "Speed: 0.6ms preprocess, 60.9ms inference, 301.3ms postprocess per image at shape (1, 3, 640, 640)\n",
+ "Results saved to \u001b[1mruns/detect/predict\u001b[0m\n"
+ ]
+ }
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "hkAzDWJ7cWTr"
+ },
+ "source": [
+ " \n",
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "0eq1SMWl6Sfn"
+ },
+ "source": [
+ "# 2. Val\n",
+ "Validate a model's accuracy on the [COCO](https://cocodataset.org/#home) dataset's `val` or `test` splits. The latest YOLOv8 [models](https://github.com/ultralytics/ultralytics#models) are downloaded automatically the first time they are used. See [YOLOv8 Val Docs](https://docs.ultralytics.com/modes/val/) for more information."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "WQPtK1QYVaD_"
+ },
+ "source": [
+ "# Download COCO val\n",
+ "import torch\n",
+ "torch.hub.download_url_to_file('https://ultralytics.com/assets/coco2017val.zip', 'tmp.zip') # download (780M - 5000 images)\n",
+ "!unzip -q tmp.zip -d datasets && rm tmp.zip # unzip"
+ ],
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "X58w8JLpMnjH",
+ "outputId": "3e5a9c48-8eba-45eb-d92f-8456cf94b60e",
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ }
+ },
+ "source": [
+ "# Validate YOLOv8n on COCO128 val\n",
+ "!yolo val model=yolov8n.pt data=coco128.yaml"
+ ],
+ "execution_count": 3,
+ "outputs": [
+ {
+ "output_type": "stream",
+ "name": "stdout",
+ "text": [
+ "Ultralytics YOLOv8.0.71 🚀 Python-3.9.16 torch-2.0.0+cu118 CUDA:0 (Tesla T4, 15102MiB)\n",
+ "YOLOv8n summary (fused): 168 layers, 3151904 parameters, 0 gradients, 8.7 GFLOPs\n",
+ "\n",
+ "Dataset 'coco128.yaml' images not found ⚠️, missing paths ['/content/datasets/coco128/images/train2017']\n",
+ "Downloading https://ultralytics.com/assets/coco128.zip to /content/datasets/coco128.zip...\n",
+ "100% 6.66M/6.66M [00:01<00:00, 6.80MB/s]\n",
+ "Unzipping /content/datasets/coco128.zip to /content/datasets...\n",
+ "Dataset download success ✅ (2.2s), saved to \u001b[1m/content/datasets\u001b[0m\n",
+ "\n",
+ "Downloading https://ultralytics.com/assets/Arial.ttf to /root/.config/Ultralytics/Arial.ttf...\n",
+ "100% 755k/755k [00:00<00:00, 107MB/s]\n",
+ "\u001b[34m\u001b[1mval: \u001b[0mScanning /content/datasets/coco128/labels/train2017... 126 images, 2 backgrounds, 80 corrupt: 100% 128/128 [00:00<00:00, 1183.28it/s]\n",
+ "\u001b[34m\u001b[1mval: \u001b[0mNew cache created: /content/datasets/coco128/labels/train2017.cache\n",
+ " Class Images Instances Box(P R mAP50 mAP50-95): 100% 8/8 [00:12<00:00, 1.54s/it]\n",
+ " all 128 929 0.64 0.537 0.605 0.446\n",
+ " person 128 254 0.797 0.677 0.764 0.538\n",
+ " bicycle 128 6 0.514 0.333 0.315 0.264\n",
+ " car 128 46 0.813 0.217 0.273 0.168\n",
+ " motorcycle 128 5 0.687 0.887 0.898 0.685\n",
+ " airplane 128 6 0.82 0.833 0.927 0.675\n",
+ " bus 128 7 0.491 0.714 0.728 0.671\n",
+ " train 128 3 0.534 0.667 0.706 0.604\n",
+ " truck 128 12 1 0.332 0.473 0.297\n",
+ " boat 128 6 0.226 0.167 0.316 0.134\n",
+ " traffic light 128 14 0.734 0.2 0.202 0.139\n",
+ " stop sign 128 2 1 0.992 0.995 0.701\n",
+ " bench 128 9 0.839 0.582 0.62 0.365\n",
+ " bird 128 16 0.921 0.728 0.864 0.51\n",
+ " cat 128 4 0.875 1 0.995 0.791\n",
+ " dog 128 9 0.603 0.889 0.785 0.585\n",
+ " horse 128 2 0.597 1 0.995 0.518\n",
+ " elephant 128 17 0.849 0.765 0.9 0.679\n",
+ " bear 128 1 0.593 1 0.995 0.995\n",
+ " zebra 128 4 0.848 1 0.995 0.965\n",
+ " giraffe 128 9 0.72 1 0.951 0.722\n",
+ " backpack 128 6 0.589 0.333 0.376 0.232\n",
+ " umbrella 128 18 0.804 0.5 0.643 0.414\n",
+ " handbag 128 19 0.424 0.0526 0.165 0.0889\n",
+ " tie 128 7 0.804 0.714 0.674 0.476\n",
+ " suitcase 128 4 0.635 0.883 0.745 0.534\n",
+ " frisbee 128 5 0.675 0.8 0.759 0.688\n",
+ " skis 128 1 0.567 1 0.995 0.497\n",
+ " snowboard 128 7 0.742 0.714 0.747 0.5\n",
+ " sports ball 128 6 0.716 0.433 0.485 0.278\n",
+ " kite 128 10 0.817 0.45 0.569 0.184\n",
+ " baseball bat 128 4 0.551 0.25 0.353 0.175\n",
+ " baseball glove 128 7 0.624 0.429 0.429 0.293\n",
+ " skateboard 128 5 0.846 0.6 0.6 0.41\n",
+ " tennis racket 128 7 0.726 0.387 0.487 0.33\n",
+ " bottle 128 18 0.448 0.389 0.376 0.208\n",
+ " wine glass 128 16 0.743 0.362 0.584 0.333\n",
+ " cup 128 36 0.58 0.278 0.404 0.29\n",
+ " fork 128 6 0.527 0.167 0.246 0.184\n",
+ " knife 128 16 0.564 0.5 0.59 0.36\n",
+ " spoon 128 22 0.597 0.182 0.328 0.19\n",
+ " bowl 128 28 0.648 0.643 0.618 0.491\n",
+ " banana 128 1 0 0 0.124 0.0379\n",
+ " sandwich 128 2 0.249 0.5 0.308 0.308\n",
+ " orange 128 4 1 0.31 0.995 0.623\n",
+ " broccoli 128 11 0.374 0.182 0.249 0.203\n",
+ " carrot 128 24 0.648 0.458 0.572 0.362\n",
+ " hot dog 128 2 0.351 0.553 0.745 0.721\n",
+ " pizza 128 5 0.644 1 0.995 0.843\n",
+ " donut 128 14 0.657 1 0.94 0.864\n",
+ " cake 128 4 0.618 1 0.945 0.845\n",
+ " chair 128 35 0.506 0.514 0.442 0.239\n",
+ " couch 128 6 0.463 0.5 0.706 0.555\n",
+ " potted plant 128 14 0.65 0.643 0.711 0.472\n",
+ " bed 128 3 0.698 0.667 0.789 0.625\n",
+ " dining table 128 13 0.432 0.615 0.485 0.366\n",
+ " toilet 128 2 0.615 0.5 0.695 0.676\n",
+ " tv 128 2 0.373 0.62 0.745 0.696\n",
+ " laptop 128 3 1 0 0.451 0.361\n",
+ " mouse 128 2 1 0 0.0625 0.00625\n",
+ " remote 128 8 0.843 0.5 0.605 0.529\n",
+ " cell phone 128 8 0 0 0.0549 0.0393\n",
+ " microwave 128 3 0.435 0.667 0.806 0.718\n",
+ " oven 128 5 0.412 0.4 0.339 0.27\n",
+ " sink 128 6 0.35 0.167 0.182 0.129\n",
+ " refrigerator 128 5 0.589 0.4 0.604 0.452\n",
+ " book 128 29 0.629 0.103 0.346 0.178\n",
+ " clock 128 9 0.788 0.83 0.875 0.74\n",
+ " vase 128 2 0.376 1 0.828 0.795\n",
+ " scissors 128 1 1 0 0.249 0.0746\n",
+ " teddy bear 128 21 0.877 0.333 0.591 0.394\n",
+ " toothbrush 128 5 0.743 0.6 0.638 0.374\n",
+ "Speed: 5.3ms preprocess, 20.1ms inference, 0.0ms loss, 11.7ms postprocess per image\n",
+ "Results saved to \u001b[1mruns/detect/val\u001b[0m\n"
+ ]
+ }
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "ZY2VXXXu74w5"
+ },
+ "source": [
+ "# 3. Train\n",
+ "\n",
+ "
\n",
+ "\n",
+ "Train YOLOv8 on [Detect](https://docs.ultralytics.com/tasks/detect/), [Segment](https://docs.ultralytics.com/tasks/segment/), [Classify](https://docs.ultralytics.com/tasks/classify/) and [Pose](https://docs.ultralytics.com/tasks/pose/) datasets. See [YOLOv8 Train Docs](https://docs.ultralytics.com/modes/train/) for more information."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "metadata": {
+ "id": "1NcFxRcFdJ_O",
+ "outputId": "b60a1f74-8035-4f9e-b4b0-604f9cf76231",
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ }
+ },
+ "source": [
+ "# Train YOLOv8n on COCO128 for 3 epochs\n",
+ "!yolo train model=yolov8n.pt data=coco128.yaml epochs=3 imgsz=640"
+ ],
+ "execution_count": 4,
+ "outputs": [
+ {
+ "output_type": "stream",
+ "name": "stdout",
+ "text": [
+ "Ultralytics YOLOv8.0.71 🚀 Python-3.9.16 torch-2.0.0+cu118 CUDA:0 (Tesla T4, 15102MiB)\n",
+ "\u001b[34m\u001b[1myolo/engine/trainer: \u001b[0mtask=detect, mode=train, model=yolov8n.pt, data=coco128.yaml, epochs=3, patience=50, batch=16, imgsz=640, save=True, save_period=-1, cache=False, device=None, workers=8, project=None, name=None, exist_ok=False, pretrained=False, optimizer=SGD, verbose=True, seed=0, deterministic=True, single_cls=False, image_weights=False, rect=False, cos_lr=False, close_mosaic=0, resume=False, amp=True, overlap_mask=True, mask_ratio=4, dropout=0.0, val=True, split=val, save_json=False, save_hybrid=False, conf=None, iou=0.7, max_det=300, half=False, dnn=False, plots=True, source=None, show=False, save_txt=False, save_conf=False, save_crop=False, show_labels=True, show_conf=True, vid_stride=1, line_width=3, visualize=False, augment=False, agnostic_nms=False, classes=None, retina_masks=False, boxes=True, format=torchscript, keras=False, optimize=False, int8=False, dynamic=False, simplify=False, opset=None, workspace=4, nms=False, lr0=0.01, lrf=0.01, momentum=0.937, weight_decay=0.0005, warmup_epochs=3.0, warmup_momentum=0.8, warmup_bias_lr=0.1, box=7.5, cls=0.5, dfl=1.5, pose=12.0, kobj=1.0, label_smoothing=0.0, nbs=64, hsv_h=0.015, hsv_s=0.7, hsv_v=0.4, degrees=0.0, translate=0.1, scale=0.5, shear=0.0, perspective=0.0, flipud=0.0, fliplr=0.5, mosaic=1.0, mixup=0.0, copy_paste=0.0, cfg=None, v5loader=False, tracker=botsort.yaml, save_dir=runs/detect/train\n",
+ "\n",
+ " from n params module arguments \n",
+ " 0 -1 1 464 ultralytics.nn.modules.Conv [3, 16, 3, 2] \n",
+ " 1 -1 1 4672 ultralytics.nn.modules.Conv [16, 32, 3, 2] \n",
+ " 2 -1 1 7360 ultralytics.nn.modules.C2f [32, 32, 1, True] \n",
+ " 3 -1 1 18560 ultralytics.nn.modules.Conv [32, 64, 3, 2] \n",
+ " 4 -1 2 49664 ultralytics.nn.modules.C2f [64, 64, 2, True] \n",
+ " 5 -1 1 73984 ultralytics.nn.modules.Conv [64, 128, 3, 2] \n",
+ " 6 -1 2 197632 ultralytics.nn.modules.C2f [128, 128, 2, True] \n",
+ " 7 -1 1 295424 ultralytics.nn.modules.Conv [128, 256, 3, 2] \n",
+ " 8 -1 1 460288 ultralytics.nn.modules.C2f [256, 256, 1, True] \n",
+ " 9 -1 1 164608 ultralytics.nn.modules.SPPF [256, 256, 5] \n",
+ " 10 -1 1 0 torch.nn.modules.upsampling.Upsample [None, 2, 'nearest'] \n",
+ " 11 [-1, 6] 1 0 ultralytics.nn.modules.Concat [1] \n",
+ " 12 -1 1 148224 ultralytics.nn.modules.C2f [384, 128, 1] \n",
+ " 13 -1 1 0 torch.nn.modules.upsampling.Upsample [None, 2, 'nearest'] \n",
+ " 14 [-1, 4] 1 0 ultralytics.nn.modules.Concat [1] \n",
+ " 15 -1 1 37248 ultralytics.nn.modules.C2f [192, 64, 1] \n",
+ " 16 -1 1 36992 ultralytics.nn.modules.Conv [64, 64, 3, 2] \n",
+ " 17 [-1, 12] 1 0 ultralytics.nn.modules.Concat [1] \n",
+ " 18 -1 1 123648 ultralytics.nn.modules.C2f [192, 128, 1] \n",
+ " 19 -1 1 147712 ultralytics.nn.modules.Conv [128, 128, 3, 2] \n",
+ " 20 [-1, 9] 1 0 ultralytics.nn.modules.Concat [1] \n",
+ " 21 -1 1 493056 ultralytics.nn.modules.C2f [384, 256, 1] \n",
+ " 22 [15, 18, 21] 1 897664 ultralytics.nn.modules.Detect [80, [64, 128, 256]] \n",
+ "Model summary: 225 layers, 3157200 parameters, 3157184 gradients, 8.9 GFLOPs\n",
+ "\n",
+ "Transferred 355/355 items from pretrained weights\n",
+ "\u001b[34m\u001b[1mTensorBoard: \u001b[0mStart with 'tensorboard --logdir runs/detect/train', view at http://localhost:6006/\n",
+ "\u001b[34m\u001b[1mAMP: \u001b[0mrunning Automatic Mixed Precision (AMP) checks with YOLOv8n...\n",
+ "\u001b[34m\u001b[1mAMP: \u001b[0mchecks passed ✅\n",
+ "\u001b[34m\u001b[1moptimizer:\u001b[0m SGD(lr=0.01) with parameter groups 57 weight(decay=0.0), 64 weight(decay=0.0005), 63 bias\n",
+ "\u001b[34m\u001b[1mtrain: \u001b[0mScanning /content/datasets/coco128/labels/train2017.cache... 126 images, 2 backgrounds, 80 corrupt: 100% 128/128 [00:00, ?it/s]\n",
+ "\u001b[34m\u001b[1malbumentations: \u001b[0mBlur(p=0.01, blur_limit=(3, 7)), MedianBlur(p=0.01, blur_limit=(3, 7)), ToGray(p=0.01), CLAHE(p=0.01, clip_limit=(1, 4.0), tile_grid_size=(8, 8))\n",
+ "\u001b[34m\u001b[1mval: \u001b[0mScanning /content/datasets/coco128/labels/train2017.cache... 126 images, 2 backgrounds, 80 corrupt: 100% 128/128 [00:00, ?it/s]\n",
+ "Plotting labels to runs/detect/train/labels.jpg... \n",
+ "Image sizes 640 train, 640 val\n",
+ "Using 2 dataloader workers\n",
+ "Logging results to \u001b[1mruns/detect/train\u001b[0m\n",
+ "Starting training for 3 epochs...\n",
+ "\n",
+ " Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size\n",
+ " 1/3 2.78G 1.177 1.338 1.25 230 640: 100% 8/8 [00:06<00:00, 1.21it/s]\n",
+ " Class Images Instances Box(P R mAP50 mAP50-95): 100% 4/4 [00:04<00:00, 1.21s/it]\n",
+ " all 128 929 0.631 0.549 0.614 0.455\n",
+ "\n",
+ " Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size\n",
+ " 2/3 2.69G 1.131 1.405 1.24 179 640: 100% 8/8 [00:02<00:00, 3.13it/s]\n",
+ " Class Images Instances Box(P R mAP50 mAP50-95): 100% 4/4 [00:02<00:00, 1.51it/s]\n",
+ " all 128 929 0.669 0.569 0.634 0.478\n",
+ "\n",
+ " Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size\n",
+ " 3/3 2.84G 1.151 1.281 1.212 214 640: 100% 8/8 [00:02<00:00, 3.27it/s]\n",
+ " Class Images Instances Box(P R mAP50 mAP50-95): 100% 4/4 [00:09<00:00, 2.42s/it]\n",
+ " all 128 929 0.687 0.58 0.65 0.488\n",
+ "\n",
+ "3 epochs completed in 0.010 hours.\n",
+ "Optimizer stripped from runs/detect/train/weights/last.pt, 6.5MB\n",
+ "Optimizer stripped from runs/detect/train/weights/best.pt, 6.5MB\n",
+ "\n",
+ "Validating runs/detect/train/weights/best.pt...\n",
+ "Ultralytics YOLOv8.0.71 🚀 Python-3.9.16 torch-2.0.0+cu118 CUDA:0 (Tesla T4, 15102MiB)\n",
+ "Model summary (fused): 168 layers, 3151904 parameters, 0 gradients, 8.7 GFLOPs\n",
+ " Class Images Instances Box(P R mAP50 mAP50-95): 100% 4/4 [00:06<00:00, 1.63s/it]\n",
+ " all 128 929 0.689 0.578 0.65 0.486\n",
+ " person 128 254 0.763 0.673 0.769 0.544\n",
+ " bicycle 128 6 1 0.328 0.379 0.332\n",
+ " car 128 46 0.84 0.217 0.292 0.18\n",
+ " motorcycle 128 5 0.612 0.8 0.872 0.709\n",
+ " airplane 128 6 0.766 0.833 0.894 0.694\n",
+ " bus 128 7 0.748 0.714 0.721 0.675\n",
+ " train 128 3 0.686 1 0.913 0.83\n",
+ " truck 128 12 0.889 0.5 0.529 0.342\n",
+ " boat 128 6 0.393 0.333 0.44 0.216\n",
+ " traffic light 128 14 1 0.21 0.224 0.142\n",
+ " stop sign 128 2 1 0.977 0.995 0.697\n",
+ " bench 128 9 0.795 0.434 0.658 0.418\n",
+ " bird 128 16 0.933 0.868 0.955 0.656\n",
+ " cat 128 4 0.796 1 0.995 0.786\n",
+ " dog 128 9 0.713 0.889 0.823 0.608\n",
+ " horse 128 2 0.576 1 0.995 0.547\n",
+ " elephant 128 17 0.786 0.824 0.911 0.719\n",
+ " bear 128 1 0.432 1 0.995 0.895\n",
+ " zebra 128 4 0.86 1 0.995 0.935\n",
+ " giraffe 128 9 0.966 1 0.995 0.727\n",
+ " backpack 128 6 0.534 0.333 0.399 0.227\n",
+ " umbrella 128 18 0.757 0.519 0.665 0.447\n",
+ " handbag 128 19 0.939 0.105 0.25 0.14\n",
+ " tie 128 7 0.677 0.602 0.682 0.505\n",
+ " suitcase 128 4 0.636 1 0.995 0.646\n",
+ " frisbee 128 5 1 0.789 0.799 0.689\n",
+ " skis 128 1 0.794 1 0.995 0.497\n",
+ " snowboard 128 7 0.575 0.714 0.762 0.48\n",
+ " sports ball 128 6 0.703 0.407 0.514 0.288\n",
+ " kite 128 10 0.645 0.4 0.506 0.206\n",
+ " baseball bat 128 4 0.436 0.404 0.253 0.125\n",
+ " baseball glove 128 7 0.786 0.429 0.43 0.303\n",
+ " skateboard 128 5 0.752 0.6 0.6 0.433\n",
+ " tennis racket 128 7 0.707 0.286 0.508 0.313\n",
+ " bottle 128 18 0.484 0.389 0.43 0.271\n",
+ " wine glass 128 16 0.471 0.562 0.584 0.327\n",
+ " cup 128 36 0.569 0.278 0.404 0.286\n",
+ " fork 128 6 0.529 0.167 0.207 0.192\n",
+ " knife 128 16 0.697 0.562 0.594 0.377\n",
+ " spoon 128 22 0.68 0.182 0.376 0.213\n",
+ " bowl 128 28 0.623 0.679 0.653 0.536\n",
+ " banana 128 1 0 0 0.142 0.0363\n",
+ " sandwich 128 2 1 0 0.745 0.745\n",
+ " orange 128 4 1 0.457 0.849 0.56\n",
+ " broccoli 128 11 0.465 0.273 0.284 0.246\n",
+ " carrot 128 24 0.581 0.751 0.745 0.489\n",
+ " hot dog 128 2 0.654 0.961 0.828 0.763\n",
+ " pizza 128 5 0.631 1 0.995 0.854\n",
+ " donut 128 14 0.583 1 0.933 0.84\n",
+ " cake 128 4 0.643 1 0.995 0.88\n",
+ " chair 128 35 0.5 0.543 0.459 0.272\n",
+ " couch 128 6 0.488 0.5 0.624 0.47\n",
+ " potted plant 128 14 0.645 0.714 0.747 0.542\n",
+ " bed 128 3 0.718 1 0.995 0.798\n",
+ " dining table 128 13 0.448 0.615 0.538 0.437\n",
+ " toilet 128 2 1 0.884 0.995 0.946\n",
+ " tv 128 2 0.548 0.644 0.828 0.762\n",
+ " laptop 128 3 1 0.563 0.72 0.639\n",
+ " mouse 128 2 1 0 0.0623 0.0125\n",
+ " remote 128 8 0.697 0.5 0.578 0.496\n",
+ " cell phone 128 8 0 0 0.102 0.0471\n",
+ " microwave 128 3 0.651 0.667 0.863 0.738\n",
+ " oven 128 5 0.471 0.4 0.415 0.309\n",
+ " sink 128 6 0.45 0.284 0.268 0.159\n",
+ " refrigerator 128 5 0.679 0.4 0.695 0.537\n",
+ " book 128 29 0.656 0.133 0.424 0.227\n",
+ " clock 128 9 0.878 0.778 0.898 0.759\n",
+ " vase 128 2 0.413 1 0.828 0.745\n",
+ " scissors 128 1 1 0 0.199 0.0597\n",
+ " teddy bear 128 21 0.553 0.472 0.669 0.447\n",
+ " toothbrush 128 5 1 0.518 0.8 0.521\n",
+ "Speed: 2.7ms preprocess, 3.5ms inference, 0.0ms loss, 3.2ms postprocess per image\n",
+ "Results saved to \u001b[1mruns/detect/train\u001b[0m\n"
+ ]
+ }
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "# 4. Export\n",
+ "\n",
+ "Export a YOLOv8 model to any supported format below with the `format` argument, i.e. `format=onnx`. See [YOLOv8 Export Docs](https://docs.ultralytics.com/modes/export/) for more information.\n",
+ "\n",
+ "- 💡 ProTip: Export to [ONNX](https://onnx.ai/) or [OpenVINO](https://docs.openvino.ai/latest/index.html) for up to 3x CPU speedup. \n",
+ "- 💡 ProTip: Export to [TensorRT](https://developer.nvidia.com/tensorrt) for up to 5x GPU speedup.\n",
+ "\n",
+ "\n",
+ "| Format | `format=` | Model |\n",
+ "|----------------------------------------------------------------------------|--------------------|---------------------------|\n",
+ "| [PyTorch](https://pytorch.org/) | - | `yolov8n.pt` |\n",
+ "| [TorchScript](https://pytorch.org/docs/stable/jit.html) | `torchscript` | `yolov8n.torchscript` |\n",
+ "| [ONNX](https://onnx.ai/) | `onnx` | `yolov8n.onnx` |\n",
+ "| [OpenVINO](https://docs.openvino.ai/latest/index.html) | `openvino` | `yolov8n_openvino_model/` |\n",
+ "| [TensorRT](https://developer.nvidia.com/tensorrt) | `engine` | `yolov8n.engine` |\n",
+ "| [CoreML](https://github.com/apple/coremltools) | `coreml` | `yolov8n.mlmodel` |\n",
+ "| [TensorFlow SavedModel](https://www.tensorflow.org/guide/saved_model) | `saved_model` | `yolov8n_saved_model/` |\n",
+ "| [TensorFlow GraphDef](https://www.tensorflow.org/api_docs/python/tf/Graph) | `pb` | `yolov8n.pb` |\n",
+ "| [TensorFlow Lite](https://www.tensorflow.org/lite) | `tflite` | `yolov8n.tflite` |\n",
+ "| [TensorFlow Edge TPU](https://coral.ai/docs/edgetpu/models-intro/) | `edgetpu` | `yolov8n_edgetpu.tflite` |\n",
+ "| [TensorFlow.js](https://www.tensorflow.org/js) | `tfjs` | `yolov8n_web_model/` |\n",
+ "| [PaddlePaddle](https://github.com/PaddlePaddle) | `paddle` | `yolov8n_paddle_model/` |\n",
+ "\n"
+ ],
+ "metadata": {
+ "id": "nPZZeNrLCQG6"
+ }
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "!yolo export model=yolov8n.pt format=torchscript"
+ ],
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "CYIjW4igCjqD",
+ "outputId": "fc41bf7a-0ea2-41a6-9ec5-dd0455af43bc"
+ },
+ "execution_count": 5,
+ "outputs": [
+ {
+ "output_type": "stream",
+ "name": "stdout",
+ "text": [
+ "Ultralytics YOLOv8.0.71 🚀 Python-3.9.16 torch-2.0.0+cu118 CPU\n",
+ "YOLOv8n summary (fused): 168 layers, 3151904 parameters, 0 gradients, 8.7 GFLOPs\n",
+ "\n",
+ "\u001b[34m\u001b[1mPyTorch:\u001b[0m starting from yolov8n.pt with input shape (1, 3, 640, 640) BCHW and output shape(s) (1, 84, 8400) (6.2 MB)\n",
+ "\n",
+ "\u001b[34m\u001b[1mTorchScript:\u001b[0m starting export with torch 2.0.0+cu118...\n",
+ "\u001b[34m\u001b[1mTorchScript:\u001b[0m export success ✅ 2.3s, saved as yolov8n.torchscript (12.4 MB)\n",
+ "\n",
+ "Export complete (3.1s)\n",
+ "Results saved to \u001b[1m/content\u001b[0m\n",
+ "Predict: yolo predict task=detect model=yolov8n.torchscript imgsz=640 \n",
+ "Validate: yolo val task=detect model=yolov8n.torchscript imgsz=640 data=coco.yaml \n",
+ "Visualize: https://netron.app\n"
+ ]
+ }
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "# 5. Python Usage\n",
+ "\n",
+ "YOLOv8 was reimagined using Python-first principles for the most seamless Python YOLO experience yet. YOLOv8 models can be loaded from a trained checkpoint or created from scratch. Then methods are used to train, val, predict, and export the model. See detailed Python usage examples in the [YOLOv8 Python Docs](https://docs.ultralytics.com/usage/python/)."
+ ],
+ "metadata": {
+ "id": "kUMOQ0OeDBJG"
+ }
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "from ultralytics import YOLO\n",
+ "\n",
+ "# Load a model\n",
+ "model = YOLO('yolov8n.yaml') # build a new model from scratch\n",
+ "model = YOLO('yolov8n.pt') # load a pretrained model (recommended for training)\n",
+ "\n",
+ "# Use the model\n",
+ "results = model.train(data='coco128.yaml', epochs=3) # train the model\n",
+ "results = model.val() # evaluate model performance on the validation set\n",
+ "results = model('https://ultralytics.com/images/bus.jpg') # predict on an image\n",
+ "success = model.export(format='onnx') # export the model to ONNX format"
+ ],
+ "metadata": {
+ "id": "bpF9-vS_DAaf"
+ },
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "# 6. Tasks\n",
+ "\n",
+ "YOLOv8 can train, val, predict and export models for the most common tasks in vision AI: [Detect](https://docs.ultralytics.com/tasks/detect/), [Segment](https://docs.ultralytics.com/tasks/segment/), [Classify](https://docs.ultralytics.com/tasks/classify/) and [Pose](https://docs.ultralytics.com/tasks/pose/). See [YOLOv8 Tasks Docs](https://docs.ultralytics.com/tasks/) for more information.\n",
+ "\n",
+ "
\n"
+ ],
+ "metadata": {
+ "id": "Phm9ccmOKye5"
+ }
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "## 1. Detection\n",
+ "\n",
+ "YOLOv8 _detection_ models have no suffix and are the default YOLOv8 models, i.e. `yolov8n.pt` and are pretrained on COCO. See [Detection Docs](https://docs.ultralytics.com/tasks/detect/) for full details.\n"
+ ],
+ "metadata": {
+ "id": "yq26lwpYK1lq"
+ }
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "# Load YOLOv8n, train it on COCO128 for 3 epochs and predict an image with it\n",
+ "from ultralytics import YOLO\n",
+ "\n",
+ "model = YOLO('yolov8n.pt') # load a pretrained YOLOv8n detection model\n",
+ "model.train(data='coco128.yaml', epochs=3) # train the model\n",
+ "model('https://ultralytics.com/images/bus.jpg') # predict on an image"
+ ],
+ "metadata": {
+ "id": "8Go5qqS9LbC5"
+ },
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "## 2. Segmentation\n",
+ "\n",
+ "YOLOv8 _segmentation_ models use the `-seg` suffix, i.e. `yolov8n-seg.pt` and are pretrained on COCO. See [Segmentation Docs](https://docs.ultralytics.com/tasks/segment/) for full details.\n"
+ ],
+ "metadata": {
+ "id": "7ZW58jUzK66B"
+ }
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "# Load YOLOv8n-seg, train it on COCO128-seg for 3 epochs and predict an image with it\n",
+ "from ultralytics import YOLO\n",
+ "\n",
+ "model = YOLO('yolov8n-seg.pt') # load a pretrained YOLOv8n segmentation model\n",
+ "model.train(data='coco128-seg.yaml', epochs=3) # train the model\n",
+ "model('https://ultralytics.com/images/bus.jpg') # predict on an image"
+ ],
+ "metadata": {
+ "id": "WFPJIQl_L5HT"
+ },
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "## 3. Classification\n",
+ "\n",
+ "YOLOv8 _classification_ models use the `-cls` suffix, i.e. `yolov8n-cls.pt` and are pretrained on ImageNet. See [Classification Docs](https://docs.ultralytics.com/tasks/classify/) for full details.\n"
+ ],
+ "metadata": {
+ "id": "ax3p94VNK9zR"
+ }
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "# Load YOLOv8n-cls, train it on mnist160 for 3 epochs and predict an image with it\n",
+ "from ultralytics import YOLO\n",
+ "\n",
+ "model = YOLO('yolov8n-cls.pt') # load a pretrained YOLOv8n classification model\n",
+ "model.train(data='mnist160', epochs=3) # train the model\n",
+ "model('https://ultralytics.com/images/bus.jpg') # predict on an image"
+ ],
+ "metadata": {
+ "id": "5q9Zu6zlL5rS"
+ },
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "markdown",
+ "source": [
+ "## 4. Pose\n",
+ "\n",
+ "YOLOv8 _pose_ models use the `-pose` suffix, i.e. `yolov8n-pose.pt` and are pretrained on COCO Keypoints. See [Pose Docs](https://docs.ultralytics.com/tasks/pose/) for full details."
+ ],
+ "metadata": {
+ "id": "SpIaFLiO11TG"
+ }
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "# Load YOLOv8n-pose, train it on COCO8-pose for 3 epochs and predict an image with it\n",
+ "from ultralytics import YOLO\n",
+ "\n",
+ "model = YOLO('yolov8n-pose.pt') # load a pretrained YOLOv8n classification model\n",
+ "model.train(data='coco8-pose.yaml', epochs=3) # train the model\n",
+ "model('https://ultralytics.com/images/bus.jpg') # predict on an image"
+ ],
+ "metadata": {
+ "id": "si4aKFNg19vX"
+ },
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "IEijrePND_2I"
+ },
+ "source": [
+ "# Appendix\n",
+ "\n",
+ "Additional content below."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "# Git clone and run tests on updates branch\n",
+ "!git clone https://github.com/ultralytics/ultralytics -b updates\n",
+ "%pip install -qe ultralytics\n",
+ "!pytest ultralytics/tests"
+ ],
+ "metadata": {
+ "id": "uRKlwxSJdhd1"
+ },
+ "execution_count": null,
+ "outputs": []
+ },
+ {
+ "cell_type": "code",
+ "source": [
+ "# Validate multiple models\n",
+ "for x in 'nsmlx':\n",
+ " !yolo val model=yolov8{x}.pt data=coco.yaml"
+ ],
+ "metadata": {
+ "id": "Wdc6t_bfzDDk"
+ },
+ "execution_count": null,
+ "outputs": []
+ }
+ ]
+}
diff --git a/yolov8/mkdocs.yml b/yolov8/mkdocs.yml
new file mode 100644
index 0000000000000000000000000000000000000000..c0938946d84a319fa85cc7c72d575ca419a38a55
--- /dev/null
+++ b/yolov8/mkdocs.yml
@@ -0,0 +1,483 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+site_name: Ultralytics YOLOv8 Docs
+site_url: https://docs.ultralytics.com
+site_description: Explore Ultralytics YOLOv8, a cutting-edge real-time object detection and image segmentation model for various applications and hardware platforms.
+site_author: Ultralytics
+repo_url: https://github.com/ultralytics/ultralytics
+edit_uri: https://github.com/ultralytics/ultralytics/tree/main/docs
+repo_name: ultralytics/ultralytics
+remote_name: https://github.com/ultralytics/docs
+
+theme:
+ name: material
+ custom_dir: docs/overrides
+ logo: https://github.com/ultralytics/assets/raw/main/logo/Ultralytics_Logotype_Reverse.svg
+ favicon: assets/favicon.ico
+ icon:
+ repo: fontawesome/brands/github
+ font:
+ text: Helvetica
+ code: Roboto Mono
+
+ palette:
+ # Palette toggle for light mode
+ - scheme: default
+ # primary: grey
+ toggle:
+ icon: material/brightness-7
+ name: Switch to dark mode
+
+ # Palette toggle for dark mode
+ - scheme: slate
+ # primary: black
+ toggle:
+ icon: material/brightness-4
+ name: Switch to light mode
+ features:
+ - content.action.edit
+ - content.code.annotate
+ - content.code.copy
+ - content.tooltips
+ - search.highlight
+ - search.share
+ - search.suggest
+ - toc.follow
+ - toc.integrate
+ - navigation.top
+ - navigation.tabs
+ - navigation.tabs.sticky
+ - navigation.expand
+ - navigation.footer
+ - navigation.tracking
+ - navigation.instant
+ - navigation.indexes
+ - content.tabs.link # all code tabs change simultaneously
+
+# Customization
+copyright: © 2023 Ultralytics Inc. All rights reserved.
+extra:
+ # version:
+ # provider: mike # version drop-down menu
+ robots: robots.txt
+ analytics:
+ provider: google
+ property: G-2M5EHKC0BH
+ # feedback:
+ # title: Was this page helpful?
+ # ratings:
+ # - icon: material/heart
+ # name: This page was helpful
+ # data: 1
+ # note: Thanks for your feedback!
+ # - icon: material/heart-broken
+ # name: This page could be improved
+ # data: 0
+ # note: >-
+ # Thanks for your feedback!
+ # Tell us what we can improve.
+
+ social:
+ - icon: fontawesome/brands/github
+ link: https://github.com/ultralytics
+ - icon: fontawesome/brands/linkedin
+ link: https://www.linkedin.com/company/ultralytics/
+ - icon: fontawesome/brands/twitter
+ link: https://twitter.com/ultralytics
+ - icon: fontawesome/brands/youtube
+ link: https://www.youtube.com/ultralytics
+ - icon: fontawesome/brands/docker
+ link: https://hub.docker.com/r/ultralytics/ultralytics/
+ - icon: fontawesome/brands/python
+ link: https://pypi.org/project/ultralytics/
+ - icon: fontawesome/brands/discord
+ link: https://discord.gg/2wNGbc6g9X
+
+extra_css:
+ - stylesheets/style.css
+ - https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css
+
+markdown_extensions:
+ # Div text decorators
+ - admonition
+ - md_in_html
+ - pymdownx.details
+ - pymdownx.superfences
+ - tables
+ - attr_list
+ - def_list
+ # Syntax highlight
+ - pymdownx.highlight:
+ anchor_linenums: true
+ - pymdownx.inlinehilite
+ - pymdownx.snippets:
+ base_path: ./
+ - pymdownx.emoji:
+ emoji_index: !!python/name:materialx.emoji.twemoji # noqa
+ emoji_generator: !!python/name:materialx.emoji.to_svg
+ - pymdownx.tabbed:
+ alternate_style: true
+
+ # Highlight
+ - pymdownx.critic
+ - pymdownx.caret
+ - pymdownx.keys
+ - pymdownx.mark
+ - pymdownx.tilde
+
+# Primary navigation ---------------------------------------------------------------------------------------------------
+nav:
+ - Home:
+ - Home: index.md
+ - Quickstart: quickstart.md
+ - Modes:
+ - modes/index.md
+ - Train: modes/train.md
+ - Val: modes/val.md
+ - Predict: modes/predict.md
+ - Export: modes/export.md
+ - Track: modes/track.md
+ - Benchmark: modes/benchmark.md
+ - Tasks:
+ - tasks/index.md
+ - Detect: tasks/detect.md
+ - Segment: tasks/segment.md
+ - Classify: tasks/classify.md
+ - Pose: tasks/pose.md
+ - Quickstart: quickstart.md
+ - Modes:
+ - modes/index.md
+ - Train: modes/train.md
+ - Val: modes/val.md
+ - Predict: modes/predict.md
+ - Export: modes/export.md
+ - Track: modes/track.md
+ - Benchmark: modes/benchmark.md
+ - Tasks:
+ - tasks/index.md
+ - Detect: tasks/detect.md
+ - Segment: tasks/segment.md
+ - Classify: tasks/classify.md
+ - Pose: tasks/pose.md
+ - Models:
+ - models/index.md
+ - YOLOv3: models/yolov3.md
+ - YOLOv4: models/yolov4.md
+ - YOLOv5: models/yolov5.md
+ - YOLOv6: models/yolov6.md
+ - YOLOv7: models/yolov7.md
+ - YOLOv8: models/yolov8.md
+ - SAM (Segment Anything Model): models/sam.md
+ - FastSAM (Fast Segment Anything Model): models/fast-sam.md
+ - YOLO-NAS (Neural Architecture Search): models/yolo-nas.md
+ - RT-DETR (Realtime Detection Transformer): models/rtdetr.md
+ - Datasets:
+ - datasets/index.md
+ - Detection:
+ - datasets/detect/index.md
+ - Argoverse: datasets/detect/argoverse.md
+ - COCO: datasets/detect/coco.md
+ - COCO8: datasets/detect/coco8.md
+ - GlobalWheat2020: datasets/detect/globalwheat2020.md
+ - Objects365: datasets/detect/objects365.md
+ - SKU-110K: datasets/detect/sku-110k.md
+ - VisDrone: datasets/detect/visdrone.md
+ - VOC: datasets/detect/voc.md
+ - xView: datasets/detect/xview.md
+ - Segmentation:
+ - datasets/segment/index.md
+ - COCO: datasets/segment/coco.md
+ - COCO8-seg: datasets/segment/coco8-seg.md
+ - Pose:
+ - datasets/pose/index.md
+ - COCO: datasets/pose/coco.md
+ - COCO8-pose: datasets/pose/coco8-pose.md
+ - Classification:
+ - datasets/classify/index.md
+ - Caltech 101: datasets/classify/caltech101.md
+ - Caltech 256: datasets/classify/caltech256.md
+ - CIFAR-10: datasets/classify/cifar10.md
+ - CIFAR-100: datasets/classify/cifar100.md
+ - Fashion-MNIST: datasets/classify/fashion-mnist.md
+ - ImageNet: datasets/classify/imagenet.md
+ - ImageNet-10: datasets/classify/imagenet10.md
+ - Imagenette: datasets/classify/imagenette.md
+ - Imagewoof: datasets/classify/imagewoof.md
+ - MNIST: datasets/classify/mnist.md
+ - Multi-Object Tracking:
+ - datasets/track/index.md
+ - Usage:
+ - CLI: usage/cli.md
+ - Python: usage/python.md
+ - Callbacks: usage/callbacks.md
+ - Configuration: usage/cfg.md
+ - Advanced Customization: usage/engine.md
+ - YOLOv5:
+ - yolov5/index.md
+ - Quickstart: yolov5/quickstart_tutorial.md
+ - Environments:
+ - Amazon Web Services (AWS): yolov5/environments/aws_quickstart_tutorial.md
+ - Google Cloud (GCP): yolov5/environments/google_cloud_quickstart_tutorial.md
+ - Docker Image: yolov5/environments/docker_image_quickstart_tutorial.md
+ - Tutorials:
+ - Train Custom Data: yolov5/tutorials/train_custom_data.md
+ - Tips for Best Training Results: yolov5/tutorials/tips_for_best_training_results.md
+ - Multi-GPU Training: yolov5/tutorials/multi_gpu_training.md
+ - PyTorch Hub: yolov5/tutorials/pytorch_hub_model_loading.md
+ - TFLite, ONNX, CoreML, TensorRT Export: yolov5/tutorials/model_export.md
+ - NVIDIA Jetson Nano Deployment: yolov5/tutorials/running_on_jetson_nano.md
+ - Test-Time Augmentation (TTA): yolov5/tutorials/test_time_augmentation.md
+ - Model Ensembling: yolov5/tutorials/model_ensembling.md
+ - Pruning/Sparsity Tutorial: yolov5/tutorials/model_pruning_and_sparsity.md
+ - Hyperparameter evolution: yolov5/tutorials/hyperparameter_evolution.md
+ - Transfer learning with frozen layers: yolov5/tutorials/transfer_learning_with_frozen_layers.md
+ - Architecture Summary: yolov5/tutorials/architecture_description.md
+ - Roboflow Datasets: yolov5/tutorials/roboflow_datasets_integration.md
+ - Neural Magic's DeepSparse: yolov5/tutorials/neural_magic_pruning_quantization.md
+ - Comet Logging: yolov5/tutorials/comet_logging_integration.md
+ - Clearml Logging: yolov5/tutorials/clearml_logging_integration.md
+ - Ultralytics HUB:
+ - hub/index.md
+ - Quickstart: hub/quickstart.md
+ - Datasets: hub/datasets.md
+ - Projects: hub/projects.md
+ - Models: hub/models.md
+ - Integrations: hub/integrations.md
+ - Ultralytics HUB App:
+ - hub/app/index.md
+ - 'iOS': hub/app/ios.md
+ - 'Android': hub/app/android.md
+ - Inference API: hub/inference_api.md
+ - Reference:
+ - hub:
+ - __init__: reference/hub/__init__.md
+ - auth: reference/hub/auth.md
+ - session: reference/hub/session.md
+ - utils: reference/hub/utils.md
+ - nn:
+ - autobackend: reference/nn/autobackend.md
+ - autoshape: reference/nn/autoshape.md
+ - modules:
+ - block: reference/nn/modules/block.md
+ - conv: reference/nn/modules/conv.md
+ - head: reference/nn/modules/head.md
+ - transformer: reference/nn/modules/transformer.md
+ - utils: reference/nn/modules/utils.md
+ - tasks: reference/nn/tasks.md
+ - tracker:
+ - track: reference/tracker/track.md
+ - trackers:
+ - basetrack: reference/tracker/trackers/basetrack.md
+ - bot_sort: reference/tracker/trackers/bot_sort.md
+ - byte_tracker: reference/tracker/trackers/byte_tracker.md
+ - utils:
+ - gmc: reference/tracker/utils/gmc.md
+ - kalman_filter: reference/tracker/utils/kalman_filter.md
+ - matching: reference/tracker/utils/matching.md
+ - vit:
+ - rtdetr:
+ - model: reference/vit/rtdetr/model.md
+ - predict: reference/vit/rtdetr/predict.md
+ - train: reference/vit/rtdetr/train.md
+ - val: reference/vit/rtdetr/val.md
+ - sam:
+ - amg: reference/vit/sam/amg.md
+ - autosize: reference/vit/sam/autosize.md
+ - build: reference/vit/sam/build.md
+ - model: reference/vit/sam/model.md
+ - modules:
+ - decoders: reference/vit/sam/modules/decoders.md
+ - encoders: reference/vit/sam/modules/encoders.md
+ - mask_generator: reference/vit/sam/modules/mask_generator.md
+ - prompt_predictor: reference/vit/sam/modules/prompt_predictor.md
+ - sam: reference/vit/sam/modules/sam.md
+ - transformer: reference/vit/sam/modules/transformer.md
+ - predict: reference/vit/sam/predict.md
+ - utils:
+ - loss: reference/vit/utils/loss.md
+ - ops: reference/vit/utils/ops.md
+ - yolo:
+ - cfg:
+ - __init__: reference/yolo/cfg/__init__.md
+ - data:
+ - annotator: reference/yolo/data/annotator.md
+ - augment: reference/yolo/data/augment.md
+ - base: reference/yolo/data/base.md
+ - build: reference/yolo/data/build.md
+ - converter: reference/yolo/data/converter.md
+ - dataloaders:
+ - stream_loaders: reference/yolo/data/dataloaders/stream_loaders.md
+ - v5augmentations: reference/yolo/data/dataloaders/v5augmentations.md
+ - v5loader: reference/yolo/data/dataloaders/v5loader.md
+ - dataset: reference/yolo/data/dataset.md
+ - dataset_wrappers: reference/yolo/data/dataset_wrappers.md
+ - utils: reference/yolo/data/utils.md
+ - engine:
+ - exporter: reference/yolo/engine/exporter.md
+ - model: reference/yolo/engine/model.md
+ - predictor: reference/yolo/engine/predictor.md
+ - results: reference/yolo/engine/results.md
+ - trainer: reference/yolo/engine/trainer.md
+ - validator: reference/yolo/engine/validator.md
+ - nas:
+ - model: reference/yolo/nas/model.md
+ - predict: reference/yolo/nas/predict.md
+ - val: reference/yolo/nas/val.md
+ - utils:
+ - __init__: reference/yolo/utils/__init__.md
+ - autobatch: reference/yolo/utils/autobatch.md
+ - benchmarks: reference/yolo/utils/benchmarks.md
+ - callbacks:
+ - base: reference/yolo/utils/callbacks/base.md
+ - clearml: reference/yolo/utils/callbacks/clearml.md
+ - comet: reference/yolo/utils/callbacks/comet.md
+ - dvc: reference/yolo/utils/callbacks/dvc.md
+ - hub: reference/yolo/utils/callbacks/hub.md
+ - mlflow: reference/yolo/utils/callbacks/mlflow.md
+ - neptune: reference/yolo/utils/callbacks/neptune.md
+ - raytune: reference/yolo/utils/callbacks/raytune.md
+ - tensorboard: reference/yolo/utils/callbacks/tensorboard.md
+ - wb: reference/yolo/utils/callbacks/wb.md
+ - checks: reference/yolo/utils/checks.md
+ - dist: reference/yolo/utils/dist.md
+ - downloads: reference/yolo/utils/downloads.md
+ - errors: reference/yolo/utils/errors.md
+ - files: reference/yolo/utils/files.md
+ - instance: reference/yolo/utils/instance.md
+ - loss: reference/yolo/utils/loss.md
+ - metrics: reference/yolo/utils/metrics.md
+ - ops: reference/yolo/utils/ops.md
+ - patches: reference/yolo/utils/patches.md
+ - plotting: reference/yolo/utils/plotting.md
+ - tal: reference/yolo/utils/tal.md
+ - torch_utils: reference/yolo/utils/torch_utils.md
+ - v8:
+ - classify:
+ - predict: reference/yolo/v8/classify/predict.md
+ - train: reference/yolo/v8/classify/train.md
+ - val: reference/yolo/v8/classify/val.md
+ - detect:
+ - predict: reference/yolo/v8/detect/predict.md
+ - train: reference/yolo/v8/detect/train.md
+ - val: reference/yolo/v8/detect/val.md
+ - pose:
+ - predict: reference/yolo/v8/pose/predict.md
+ - train: reference/yolo/v8/pose/train.md
+ - val: reference/yolo/v8/pose/val.md
+ - segment:
+ - predict: reference/yolo/v8/segment/predict.md
+ - train: reference/yolo/v8/segment/train.md
+ - val: reference/yolo/v8/segment/val.md
+
+ - Help:
+ - Help: help/index.md
+ - Frequently Asked Questions (FAQ): help/FAQ.md
+ - Contributing Guide: help/contributing.md
+ - Continuous Integration (CI) Guide: help/CI.md
+ - Contributor License Agreement (CLA): help/CLA.md
+ - Minimum Reproducible Example (MRE) Guide: help/minimum_reproducible_example.md
+ - Code of Conduct: help/code_of_conduct.md
+ - Security Policy: SECURITY.md
+
+# Plugins including 301 redirects navigation ---------------------------------------------------------------------------
+plugins:
+ - mkdocstrings
+ - search
+ - ultralytics:
+ add_desc: False
+ add_image: True
+ add_share_buttons: True
+ default_image: https://github.com/ultralytics/ultralytics/assets/26833433/6d09221c-c52a-4234-9a5d-b862e93c6529
+ - redirects:
+ redirect_maps:
+ callbacks.md: usage/callbacks.md
+ cfg.md: usage/cfg.md
+ cli.md: usage/cli.md
+ config.md: usage/cfg.md
+ engine.md: usage/engine.md
+ environments/AWS-Quickstart.md: yolov5/environments/aws_quickstart_tutorial.md
+ environments/Docker-Quickstart.md: yolov5/environments/docker_image_quickstart_tutorial.md
+ environments/GCP-Quickstart.md: yolov5/environments/google_cloud_quickstart_tutorial.md
+ FAQ/augmentation.md: yolov5/tutorials/tips_for_best_training_results.md
+ package-framework.md: index.md
+ package-framework/mock_detector.md: index.md
+ predict.md: modes/predict.md
+ python.md: usage/python.md
+ quick-start.md: quickstart.md
+ app.md: hub/app/index.md
+ sdk.md: index.md
+ reference/base_pred.md: reference/yolo/engine/predictor.md
+ reference/base_trainer.md: reference/yolo/engine/trainer.md
+ reference/exporter.md: reference/yolo/engine/exporter.md
+ reference/model.md: reference/yolo/engine/model.md
+ reference/nn.md: reference/nn/modules/head.md
+ reference/ops.md: reference/yolo/utils/ops.md
+ reference/results.md: reference/yolo/engine/results.md
+ reference/base_val.md: index.md
+ tasks/classification.md: tasks/classify.md
+ tasks/detection.md: tasks/detect.md
+ tasks/segmentation.md: tasks/segment.md
+ tasks/keypoints.md: tasks/pose.md
+ tasks/tracking.md: modes/track.md
+ tutorials/architecture-summary.md: yolov5/tutorials/architecture_description.md
+ tutorials/clearml-logging.md: yolov5/tutorials/clearml_logging_integration.md
+ tutorials/comet-logging.md: yolov5/tutorials/comet_logging_integration.md
+ tutorials/hyperparameter-evolution.md: yolov5/tutorials/hyperparameter_evolution.md
+ tutorials/model-ensembling.md: yolov5/tutorials/model_ensembling.md
+ tutorials/multi-gpu-training.md: yolov5/tutorials/multi_gpu_training.md
+ tutorials/nvidia-jetson.md: yolov5/tutorials/running_on_jetson_nano.md
+ tutorials/pruning-sparsity.md: yolov5/tutorials/model_pruning_and_sparsity.md
+ tutorials/pytorch-hub.md: yolov5/tutorials/pytorch_hub_model_loading.md
+ tutorials/roboflow.md: yolov5/tutorials/roboflow_datasets_integration.md
+ tutorials/test-time-augmentation.md: yolov5/tutorials/test_time_augmentation.md
+ tutorials/torchscript-onnx-coreml-export.md: yolov5/tutorials/model_export.md
+ tutorials/train-custom-datasets.md: yolov5/tutorials/train_custom_data.md
+ tutorials/training-tips-best-results.md: yolov5/tutorials/tips_for_best_training_results.md
+ tutorials/transfer-learning-froze-layers.md: yolov5/tutorials/transfer_learning_with_frozen_layers.md
+ tutorials/weights-and-biasis-logging.md: yolov5/tutorials/comet_logging_integration.md
+ yolov5/pytorch_hub.md: yolov5/tutorials/pytorch_hub_model_loading.md
+ yolov5/hyp_evolution.md: yolov5/tutorials/hyperparameter_evolution.md
+ yolov5/pruning_sparsity.md: yolov5/tutorials/model_pruning_and_sparsity.md
+ yolov5/roboflow.md: yolov5/tutorials/roboflow_datasets_integration.md
+ yolov5/comet.md: yolov5/tutorials/comet_logging_integration.md
+ yolov5/clearml.md: yolov5/tutorials/clearml_logging_integration.md
+ yolov5/tta.md: yolov5/tutorials/test_time_augmentation.md
+ yolov5/multi_gpu_training.md: yolov5/tutorials/multi_gpu_training.md
+ yolov5/ensemble.md: yolov5/tutorials/model_ensembling.md
+ yolov5/jetson_nano.md: yolov5/tutorials/running_on_jetson_nano.md
+ yolov5/transfer_learn_frozen.md: yolov5/tutorials/transfer_learning_with_frozen_layers.md
+ yolov5/neural_magic.md: yolov5/tutorials/neural_magic_pruning_quantization.md
+ yolov5/train_custom_data.md: yolov5/tutorials/train_custom_data.md
+ yolov5/architecture.md: yolov5/tutorials/architecture_description.md
+ yolov5/export.md: yolov5/tutorials/model_export.md
+ yolov5/yolov5_quickstart_tutorial.md: yolov5/quickstart_tutorial.md
+ yolov5/tips_for_best_training_results.md: yolov5/tutorials/tips_for_best_training_results.md
+ yolov5/tutorials/yolov5_neural_magic_tutorial.md: yolov5/tutorials/neural_magic_pruning_quantization.md
+ yolov5/tutorials/model_ensembling_tutorial.md: yolov5/tutorials/model_ensembling.md
+ yolov5/tutorials/pytorch_hub_tutorial.md: yolov5/tutorials/pytorch_hub_model_loading.md
+ yolov5/tutorials/yolov5_architecture_tutorial.md: yolov5/tutorials/architecture_description.md
+ yolov5/tutorials/multi_gpu_training_tutorial.md: yolov5/tutorials/multi_gpu_training.md
+ yolov5/tutorials/yolov5_pytorch_hub_tutorial.md: yolov5/tutorials/pytorch_hub_model_loading.md
+ yolov5/tutorials/model_export_tutorial.md: yolov5/tutorials/model_export.md
+ yolov5/tutorials/jetson_nano_tutorial.md: yolov5/tutorials/running_on_jetson_nano.md
+ yolov5/tutorials/yolov5_model_ensembling_tutorial.md: yolov5/tutorials/model_ensembling.md
+ yolov5/tutorials/roboflow_integration.md: yolov5/tutorials/roboflow_datasets_integration.md
+ yolov5/tutorials/pruning_and_sparsity_tutorial.md: yolov5/tutorials/model_pruning_and_sparsity.md
+ yolov5/tutorials/yolov5_transfer_learning_with_frozen_layers_tutorial.md: yolov5/tutorials/transfer_learning_with_frozen_layers.md
+ yolov5/tutorials/transfer_learning_with_frozen_layers_tutorial.md: yolov5/tutorials/transfer_learning_with_frozen_layers.md
+ yolov5/tutorials/yolov5_model_export_tutorial.md: yolov5/tutorials/model_export.md
+ yolov5/tutorials/neural_magic_tutorial.md: yolov5/tutorials/neural_magic_pruning_quantization.md
+ yolov5/tutorials/yolov5_clearml_integration_tutorial.md: yolov5/tutorials/clearml_logging_integration.md
+ yolov5/tutorials/yolov5_train_custom_data.md: yolov5/tutorials/train_custom_data.md
+ yolov5/tutorials/comet_integration_tutorial.md: yolov5/tutorials/comet_logging_integration.md
+ yolov5/tutorials/yolov5_pruning_and_sparsity_tutorial.md: yolov5/tutorials/model_pruning_and_sparsity.md
+ yolov5/tutorials/yolov5_jetson_nano_tutorial.md: yolov5/tutorials/running_on_jetson_nano.md
+ yolov5/tutorials/yolov5_roboflow_integration.md: yolov5/tutorials/roboflow_datasets_integration.md
+ yolov5/tutorials/hyperparameter_evolution_tutorial.md: yolov5/tutorials/hyperparameter_evolution.md
+ yolov5/tutorials/yolov5_hyperparameter_evolution_tutorial.md: yolov5/tutorials/hyperparameter_evolution.md
+ yolov5/tutorials/clearml_integration_tutorial.md: yolov5/tutorials/clearml_logging_integration.md
+ yolov5/tutorials/test_time_augmentation_tutorial.md: yolov5/tutorials/test_time_augmentation.md
+ yolov5/tutorials/yolov5_test_time_augmentation_tutorial.md: yolov5/tutorials/test_time_augmentation.md
+ yolov5/environments/yolov5_amazon_web_services_quickstart_tutorial.md: yolov5/environments/aws_quickstart_tutorial.md
+ yolov5/environments/yolov5_google_cloud_platform_quickstart_tutorial.md: yolov5/environments/google_cloud_quickstart_tutorial.md
+ yolov5/environments/yolov5_docker_image_quickstart_tutorial.md: yolov5/environments/docker_image_quickstart_tutorial.md
diff --git a/yolov8/requirements.txt b/yolov8/requirements.txt
new file mode 100644
index 0000000000000000000000000000000000000000..be1fce9b487c3109425ff3ac1c12babed1a1bd7f
--- /dev/null
+++ b/yolov8/requirements.txt
@@ -0,0 +1,43 @@
+# Ultralytics requirements
+# Usage: pip install -r requirements.txt
+
+# Base ----------------------------------------
+matplotlib>=3.2.2
+opencv-python>=4.6.0
+Pillow>=7.1.2
+PyYAML>=5.3.1
+requests>=2.23.0
+scipy>=1.4.1
+torch>=1.7.0
+torchvision>=0.8.1
+tqdm>=4.64.0
+
+# Logging -------------------------------------
+# tensorboard>=2.13.0
+# dvclive>=2.12.0
+# clearml
+# comet
+
+# Plotting ------------------------------------
+pandas>=1.1.4
+seaborn>=0.11.0
+
+# Export --------------------------------------
+# coremltools>=6.0 # CoreML export
+# onnx>=1.12.0 # ONNX export
+# onnxsim>=0.4.1 # ONNX simplifier
+# nvidia-pyindex # TensorRT export
+# nvidia-tensorrt # TensorRT export
+# scikit-learn==0.19.2 # CoreML quantization
+# tensorflow>=2.4.1 # TF exports (-cpu, -aarch64, -macos)
+# tflite-support
+# tensorflowjs>=3.9.0 # TF.js export
+# openvino-dev>=2022.3 # OpenVINO export
+
+# Extras --------------------------------------
+psutil # system utilization
+# thop>=0.1.1 # FLOPs computation
+# ipython # interactive notebook
+# albumentations>=1.0.3
+# pycocotools>=2.0.6 # COCO mAP
+# roboflow
diff --git a/yolov8/setup.cfg b/yolov8/setup.cfg
new file mode 100644
index 0000000000000000000000000000000000000000..2cde6a494836f93681b73aaa7bcf0d0d487de469
--- /dev/null
+++ b/yolov8/setup.cfg
@@ -0,0 +1,56 @@
+# Project-wide configuration file, can be used for package metadata and other toll configurations
+# Example usage: global configuration for PEP8 (via flake8) setting or default pytest arguments
+# Local usage: pip install pre-commit, pre-commit run --all-files
+
+[metadata]
+license_files = LICENSE
+description_file = README.md
+
+[tool:pytest]
+norecursedirs =
+ .git
+ dist
+ build
+addopts =
+ --doctest-modules
+ --durations=25
+ --color=yes
+
+[flake8]
+max-line-length = 120
+exclude = .tox,*.egg,build,temp
+select = E,W,F
+doctests = True
+verbose = 2
+# https://pep8.readthedocs.io/en/latest/intro.html#error-codes
+format = pylint
+# see: https://www.flake8rules.com/
+ignore = E731,F405,E402,W504,E501
+ # E731: Do not assign a lambda expression, use a def
+ # F405: name may be undefined, or defined from star imports: module
+ # E402: module level import not at top of file
+ # W504: line break after binary operator
+ # E501: line too long
+ # removed:
+ # F401: module imported but unused
+ # E231: missing whitespace after ‘,’, ‘;’, or ‘:’
+ # E127: continuation line over-indented for visual indent
+ # F403: ‘from module import *’ used; unable to detect undefined names
+
+
+[isort]
+# https://pycqa.github.io/isort/docs/configuration/options.html
+line_length = 120
+# see: https://pycqa.github.io/isort/docs/configuration/multi_line_output_modes.html
+multi_line_output = 0
+
+[yapf]
+based_on_style = pep8
+spaces_before_comment = 2
+COLUMN_LIMIT = 120
+COALESCE_BRACKETS = True
+SPACES_AROUND_POWER_OPERATOR = True
+SPACE_BETWEEN_ENDING_COMMA_AND_CLOSING_BRACKET = True
+SPLIT_BEFORE_CLOSING_BRACKET = False
+SPLIT_BEFORE_FIRST_ARGUMENT = False
+# EACH_DICT_ENTRY_ON_SEPARATE_LINE = False
diff --git a/yolov8/setup.py b/yolov8/setup.py
new file mode 100644
index 0000000000000000000000000000000000000000..59510c3ed4b8de27220abee3382851c506f339d2
--- /dev/null
+++ b/yolov8/setup.py
@@ -0,0 +1,74 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import re
+from pathlib import Path
+
+import pkg_resources as pkg
+from setuptools import find_packages, setup
+
+# Settings
+FILE = Path(__file__).resolve()
+PARENT = FILE.parent # root directory
+README = (PARENT / 'README.md').read_text(encoding='utf-8')
+REQUIREMENTS = [f'{x.name}{x.specifier}' for x in pkg.parse_requirements((PARENT / 'requirements.txt').read_text())]
+
+
+def get_version():
+ file = PARENT / 'ultralytics/__init__.py'
+ return re.search(r'^__version__ = [\'"]([^\'"]*)[\'"]', file.read_text(encoding='utf-8'), re.M)[1]
+
+
+setup(
+ name='ultralytics', # name of pypi package
+ version=get_version(), # version of pypi package
+ python_requires='>=3.7',
+ license='AGPL-3.0',
+ description=('Ultralytics YOLOv8 for SOTA object detection, multi-object tracking, instance segmentation, '
+ 'pose estimation and image classification.'),
+ long_description=README,
+ long_description_content_type='text/markdown',
+ url='https://github.com/ultralytics/ultralytics',
+ project_urls={
+ 'Bug Reports': 'https://github.com/ultralytics/ultralytics/issues',
+ 'Funding': 'https://ultralytics.com',
+ 'Source': 'https://github.com/ultralytics/ultralytics'},
+ author='Ultralytics',
+ author_email='hello@ultralytics.com',
+ packages=find_packages(), # required
+ include_package_data=True,
+ install_requires=REQUIREMENTS,
+ extras_require={
+ 'dev': [
+ 'check-manifest',
+ 'pytest',
+ 'pytest-cov',
+ 'coverage',
+ 'mkdocs-material',
+ 'mkdocstrings[python]',
+ 'mkdocs-redirects', # for 301 redirects
+ 'mkdocs-ultralytics-plugin', # for meta descriptions and images, dates and authors
+ ],
+ 'export': ['coremltools>=6.0', 'openvino-dev>=2022.3', 'tensorflowjs'], # automatically installs tensorflow
+ },
+ classifiers=[
+ 'Development Status :: 4 - Beta',
+ 'Intended Audience :: Developers',
+ 'Intended Audience :: Education',
+ 'Intended Audience :: Science/Research',
+ 'License :: OSI Approved :: GNU Affero General Public License v3 or later (AGPLv3+)',
+ 'Programming Language :: Python :: 3',
+ 'Programming Language :: Python :: 3.7',
+ 'Programming Language :: Python :: 3.8',
+ 'Programming Language :: Python :: 3.9',
+ 'Programming Language :: Python :: 3.10',
+ 'Programming Language :: Python :: 3.11',
+ 'Topic :: Software Development',
+ 'Topic :: Scientific/Engineering',
+ 'Topic :: Scientific/Engineering :: Artificial Intelligence',
+ 'Topic :: Scientific/Engineering :: Image Recognition',
+ 'Operating System :: POSIX :: Linux',
+ 'Operating System :: MacOS',
+ 'Operating System :: Microsoft :: Windows', ],
+ keywords='machine-learning, deep-learning, vision, ML, DL, AI, YOLO, YOLOv3, YOLOv5, YOLOv8, HUB, Ultralytics',
+ entry_points={
+ 'console_scripts': ['yolo = ultralytics.yolo.cfg:entrypoint', 'ultralytics = ultralytics.yolo.cfg:entrypoint']})
diff --git a/yolov8/tests/conftest.py b/yolov8/tests/conftest.py
new file mode 100644
index 0000000000000000000000000000000000000000..020ac7a3ae449354a21e209b324cbb2899081f4c
--- /dev/null
+++ b/yolov8/tests/conftest.py
@@ -0,0 +1,19 @@
+import pytest
+
+
+def pytest_addoption(parser):
+ parser.addoption('--runslow', action='store_true', default=False, help='run slow tests')
+
+
+def pytest_configure(config):
+ config.addinivalue_line('markers', 'slow: mark test as slow to run')
+
+
+def pytest_collection_modifyitems(config, items):
+ if config.getoption('--runslow'):
+ # --runslow given in cli: do not skip slow tests
+ return
+ skip_slow = pytest.mark.skip(reason='need --runslow option to run')
+ for item in items:
+ if 'slow' in item.keywords:
+ item.add_marker(skip_slow)
diff --git a/yolov8/tests/test_cli.py b/yolov8/tests/test_cli.py
new file mode 100644
index 0000000000000000000000000000000000000000..24e9703d2cbdfea8bbae8749e42aa071f299da47
--- /dev/null
+++ b/yolov8/tests/test_cli.py
@@ -0,0 +1,59 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import subprocess
+from pathlib import Path
+
+import pytest
+
+from ultralytics.yolo.utils import ONLINE, ROOT, SETTINGS
+
+WEIGHT_DIR = Path(SETTINGS['weights_dir'])
+TASK_ARGS = [ # (task, model, data)
+ ('detect', 'yolov8n', 'coco8.yaml'), ('segment', 'yolov8n-seg', 'coco8-seg.yaml'),
+ ('classify', 'yolov8n-cls', 'imagenet10'), ('pose', 'yolov8n-pose', 'coco8-pose.yaml')]
+EXPORT_ARGS = [ # (model, format)
+ ('yolov8n', 'torchscript'), ('yolov8n-seg', 'torchscript'), ('yolov8n-cls', 'torchscript'),
+ ('yolov8n-pose', 'torchscript')]
+
+
+def run(cmd):
+ # Run a subprocess command with check=True
+ subprocess.run(cmd.split(), check=True)
+
+
+def test_special_modes():
+ run('yolo checks')
+ run('yolo settings')
+ run('yolo help')
+
+
+@pytest.mark.parametrize('task,model,data', TASK_ARGS)
+def test_train(task, model, data):
+ run(f'yolo train {task} model={model}.yaml data={data} imgsz=32 epochs=1 cache=disk')
+
+
+@pytest.mark.parametrize('task,model,data', TASK_ARGS)
+def test_val(task, model, data):
+ run(f'yolo val {task} model={model}.pt data={data} imgsz=32')
+
+
+@pytest.mark.parametrize('task,model,data', TASK_ARGS)
+def test_predict(task, model, data):
+ run(f"yolo predict model={model}.pt source={ROOT / 'assets'} imgsz=32 save save_crop save_txt")
+ if ONLINE:
+ run(f'yolo predict model={model}.pt source=https://ultralytics.com/images/bus.jpg imgsz=32')
+ run(f'yolo predict model={model}.pt source=https://ultralytics.com/assets/decelera_landscape_min.mov imgsz=32')
+ run(f'yolo predict model={model}.pt source=https://ultralytics.com/assets/decelera_portrait_min.mov imgsz=32')
+
+
+@pytest.mark.parametrize('model,format', EXPORT_ARGS)
+def test_export(model, format):
+ run(f'yolo export model={model}.pt format={format}')
+
+
+# Slow Tests
+@pytest.mark.slow
+@pytest.mark.parametrize('task,model,data', TASK_ARGS)
+def test_train_gpu(task, model, data):
+ run(f'yolo train {task} model={model}.yaml data={data} imgsz=32 epochs=1 device="0"') # single GPU
+ run(f'yolo train {task} model={model}.pt data={data} imgsz=32 epochs=1 device="0,1"') # Multi GPU
diff --git a/yolov8/tests/test_engine.py b/yolov8/tests/test_engine.py
new file mode 100644
index 0000000000000000000000000000000000000000..b0110442dc7e4488765a695d3febb649db08e54f
--- /dev/null
+++ b/yolov8/tests/test_engine.py
@@ -0,0 +1,125 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from pathlib import Path
+
+from ultralytics import YOLO
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.engine.exporter import Exporter
+from ultralytics.yolo.utils import DEFAULT_CFG, ROOT, SETTINGS
+from ultralytics.yolo.v8 import classify, detect, segment
+
+CFG_DET = 'yolov8n.yaml'
+CFG_SEG = 'yolov8n-seg.yaml'
+CFG_CLS = 'squeezenet1_0'
+CFG = get_cfg(DEFAULT_CFG)
+MODEL = Path(SETTINGS['weights_dir']) / 'yolov8n'
+SOURCE = ROOT / 'assets'
+
+
+def test_func(model=None):
+ print('callback test passed')
+
+
+def test_export():
+ exporter = Exporter()
+ exporter.add_callback('on_export_start', test_func)
+ assert test_func in exporter.callbacks['on_export_start'], 'callback test failed'
+ f = exporter(model=YOLO(CFG_DET).model)
+ YOLO(f)(SOURCE) # exported model inference
+
+
+def test_detect():
+ overrides = {'data': 'coco8.yaml', 'model': CFG_DET, 'imgsz': 32, 'epochs': 1, 'save': False}
+ CFG.data = 'coco8.yaml'
+
+ # Trainer
+ trainer = detect.DetectionTrainer(overrides=overrides)
+ trainer.add_callback('on_train_start', test_func)
+ assert test_func in trainer.callbacks['on_train_start'], 'callback test failed'
+ trainer.train()
+
+ # Validator
+ val = detect.DetectionValidator(args=CFG)
+ val.add_callback('on_val_start', test_func)
+ assert test_func in val.callbacks['on_val_start'], 'callback test failed'
+ val(model=trainer.best) # validate best.pt
+
+ # Predictor
+ pred = detect.DetectionPredictor(overrides={'imgsz': [64, 64]})
+ pred.add_callback('on_predict_start', test_func)
+ assert test_func in pred.callbacks['on_predict_start'], 'callback test failed'
+ result = pred(source=SOURCE, model=f'{MODEL}.pt')
+ assert len(result), 'predictor test failed'
+
+ overrides['resume'] = trainer.last
+ trainer = detect.DetectionTrainer(overrides=overrides)
+ try:
+ trainer.train()
+ except Exception as e:
+ print(f'Expected exception caught: {e}')
+ return
+
+ Exception('Resume test failed!')
+
+
+def test_segment():
+ overrides = {'data': 'coco8-seg.yaml', 'model': CFG_SEG, 'imgsz': 32, 'epochs': 1, 'save': False}
+ CFG.data = 'coco8-seg.yaml'
+ CFG.v5loader = False
+ # YOLO(CFG_SEG).train(**overrides) # works
+
+ # trainer
+ trainer = segment.SegmentationTrainer(overrides=overrides)
+ trainer.add_callback('on_train_start', test_func)
+ assert test_func in trainer.callbacks['on_train_start'], 'callback test failed'
+ trainer.train()
+
+ # Validator
+ val = segment.SegmentationValidator(args=CFG)
+ val.add_callback('on_val_start', test_func)
+ assert test_func in val.callbacks['on_val_start'], 'callback test failed'
+ val(model=trainer.best) # validate best.pt
+
+ # Predictor
+ pred = segment.SegmentationPredictor(overrides={'imgsz': [64, 64]})
+ pred.add_callback('on_predict_start', test_func)
+ assert test_func in pred.callbacks['on_predict_start'], 'callback test failed'
+ result = pred(source=SOURCE, model=f'{MODEL}-seg.pt')
+ assert len(result), 'predictor test failed'
+
+ # Test resume
+ overrides['resume'] = trainer.last
+ trainer = segment.SegmentationTrainer(overrides=overrides)
+ try:
+ trainer.train()
+ except Exception as e:
+ print(f'Expected exception caught: {e}')
+ return
+
+ Exception('Resume test failed!')
+
+
+def test_classify():
+ overrides = {'data': 'imagenet10', 'model': 'yolov8n-cls.yaml', 'imgsz': 32, 'epochs': 1, 'save': False}
+ CFG.data = 'imagenet10'
+ CFG.imgsz = 32
+ # YOLO(CFG_SEG).train(**overrides) # works
+
+ # Trainer
+ trainer = classify.ClassificationTrainer(overrides=overrides)
+ trainer.add_callback('on_train_start', test_func)
+ assert test_func in trainer.callbacks['on_train_start'], 'callback test failed'
+ trainer.train()
+
+ # Validator
+ val = classify.ClassificationValidator(args=CFG)
+ val.add_callback('on_val_start', test_func)
+ assert test_func in val.callbacks['on_val_start'], 'callback test failed'
+ val(model=trainer.best)
+
+ # Predictor
+ pred = classify.ClassificationPredictor(overrides={'imgsz': [64, 64]})
+ pred.add_callback('on_predict_start', test_func)
+ assert test_func in pred.callbacks['on_predict_start'], 'callback test failed'
+ result = pred(source=SOURCE, model=trainer.best)
+ assert len(result), 'predictor test failed'
diff --git a/yolov8/tests/test_python.py b/yolov8/tests/test_python.py
new file mode 100644
index 0000000000000000000000000000000000000000..10f24fd3d97a3658ca3cd0479c74098099ebd7a8
--- /dev/null
+++ b/yolov8/tests/test_python.py
@@ -0,0 +1,245 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from pathlib import Path
+
+import cv2
+import numpy as np
+import torch
+from PIL import Image
+from torchvision.transforms import ToTensor
+
+from ultralytics import RTDETR, YOLO
+from ultralytics.yolo.data.build import load_inference_source
+from ultralytics.yolo.utils import LINUX, ONLINE, ROOT, SETTINGS
+
+MODEL = Path(SETTINGS['weights_dir']) / 'yolov8n.pt'
+CFG = 'yolov8n.yaml'
+SOURCE = ROOT / 'assets/bus.jpg'
+SOURCE_GREYSCALE = Path(f'{SOURCE.parent / SOURCE.stem}_greyscale.jpg')
+SOURCE_RGBA = Path(f'{SOURCE.parent / SOURCE.stem}_4ch.png')
+
+# Convert SOURCE to greyscale and 4-ch
+im = Image.open(SOURCE)
+im.convert('L').save(SOURCE_GREYSCALE) # greyscale
+im.convert('RGBA').save(SOURCE_RGBA) # 4-ch PNG with alpha
+
+
+def test_model_forward():
+ model = YOLO(CFG)
+ model(SOURCE)
+
+
+def test_model_info():
+ model = YOLO(CFG)
+ model.info()
+ model = YOLO(MODEL)
+ model.info(verbose=True)
+
+
+def test_model_fuse():
+ model = YOLO(CFG)
+ model.fuse()
+ model = YOLO(MODEL)
+ model.fuse()
+
+
+def test_predict_dir():
+ model = YOLO(MODEL)
+ model(source=ROOT / 'assets')
+
+
+def test_predict_img():
+ model = YOLO(MODEL)
+ seg_model = YOLO('yolov8n-seg.pt')
+ cls_model = YOLO('yolov8n-cls.pt')
+ pose_model = YOLO('yolov8n-pose.pt')
+ im = cv2.imread(str(SOURCE))
+ assert len(model(source=Image.open(SOURCE), save=True, verbose=True)) == 1 # PIL
+ assert len(model(source=im, save=True, save_txt=True)) == 1 # ndarray
+ assert len(model(source=[im, im], save=True, save_txt=True)) == 2 # batch
+ assert len(list(model(source=[im, im], save=True, stream=True))) == 2 # stream
+ assert len(model(torch.zeros(320, 640, 3).numpy())) == 1 # tensor to numpy
+ batch = [
+ str(SOURCE), # filename
+ Path(SOURCE), # Path
+ 'https://ultralytics.com/images/zidane.jpg' if ONLINE else SOURCE, # URI
+ cv2.imread(str(SOURCE)), # OpenCV
+ Image.open(SOURCE), # PIL
+ np.zeros((320, 640, 3))] # numpy
+ assert len(model(batch, visualize=True)) == len(batch) # multiple sources in a batch
+
+ # Test tensor inference
+ im = cv2.imread(str(SOURCE)) # OpenCV
+ t = cv2.resize(im, (32, 32))
+ t = ToTensor()(t)
+ t = torch.stack([t, t, t, t])
+ results = model(t, visualize=True)
+ assert len(results) == t.shape[0]
+ results = seg_model(t, visualize=True)
+ assert len(results) == t.shape[0]
+ results = cls_model(t, visualize=True)
+ assert len(results) == t.shape[0]
+ results = pose_model(t, visualize=True)
+ assert len(results) == t.shape[0]
+
+
+def test_predict_grey_and_4ch():
+ model = YOLO(MODEL)
+ for f in SOURCE_RGBA, SOURCE_GREYSCALE:
+ for source in Image.open(f), cv2.imread(str(f)), f:
+ model(source, save=True, verbose=True)
+
+
+def test_val():
+ model = YOLO(MODEL)
+ model.val(data='coco8.yaml', imgsz=32)
+
+
+def test_val_scratch():
+ model = YOLO(CFG)
+ model.val(data='coco8.yaml', imgsz=32)
+
+
+def test_amp():
+ if torch.cuda.is_available():
+ from ultralytics.yolo.utils.checks import check_amp
+ model = YOLO(MODEL).model.cuda()
+ assert check_amp(model)
+
+
+def test_train_scratch():
+ model = YOLO(CFG)
+ model.train(data='coco8.yaml', epochs=1, imgsz=32, cache='disk') # test disk caching
+ model(SOURCE)
+
+
+def test_train_pretrained():
+ model = YOLO(MODEL)
+ model.train(data='coco8.yaml', epochs=1, imgsz=32, cache='ram') # test RAM caching
+ model(SOURCE)
+
+
+def test_export_torchscript():
+ model = YOLO(MODEL)
+ f = model.export(format='torchscript')
+ YOLO(f)(SOURCE) # exported model inference
+
+
+def test_export_torchscript_scratch():
+ model = YOLO(CFG)
+ f = model.export(format='torchscript')
+ YOLO(f)(SOURCE) # exported model inference
+
+
+def test_export_onnx():
+ model = YOLO(MODEL)
+ f = model.export(format='onnx')
+ YOLO(f)(SOURCE) # exported model inference
+
+
+def test_export_openvino():
+ model = YOLO(MODEL)
+ f = model.export(format='openvino')
+ YOLO(f)(SOURCE) # exported model inference
+
+
+def test_export_coreml(): # sourcery skip: move-assign
+ model = YOLO(MODEL)
+ model.export(format='coreml')
+ # if MACOS:
+ # YOLO(f)(SOURCE) # model prediction only supported on macOS
+
+
+def test_export_tflite(enabled=False):
+ # TF suffers from install conflicts on Windows and macOS
+ if enabled and LINUX:
+ model = YOLO(MODEL)
+ f = model.export(format='tflite')
+ YOLO(f)(SOURCE)
+
+
+def test_export_pb(enabled=False):
+ # TF suffers from install conflicts on Windows and macOS
+ if enabled and LINUX:
+ model = YOLO(MODEL)
+ f = model.export(format='pb')
+ YOLO(f)(SOURCE)
+
+
+def test_export_paddle(enabled=False):
+ # Paddle protobuf requirements conflicting with onnx protobuf requirements
+ if enabled:
+ model = YOLO(MODEL)
+ model.export(format='paddle')
+
+
+def test_all_model_yamls():
+ for m in list((ROOT / 'models').rglob('yolo*.yaml')):
+ if m.name == 'yolov8-rtdetr.yaml': # except the rtdetr model
+ RTDETR(m.name)
+ else:
+ YOLO(m.name)
+
+
+def test_workflow():
+ model = YOLO(MODEL)
+ model.train(data='coco8.yaml', epochs=1, imgsz=32)
+ model.val()
+ model.predict(SOURCE)
+ model.export(format='onnx') # export a model to ONNX format
+
+
+def test_predict_callback_and_setup():
+ # test callback addition for prediction
+ def on_predict_batch_end(predictor): # results -> List[batch_size]
+ path, im0s, _, _ = predictor.batch
+ # print('on_predict_batch_end', im0s[0].shape)
+ im0s = im0s if isinstance(im0s, list) else [im0s]
+ bs = [predictor.dataset.bs for _ in range(len(path))]
+ predictor.results = zip(predictor.results, im0s, bs)
+
+ model = YOLO(MODEL)
+ model.add_callback('on_predict_batch_end', on_predict_batch_end)
+
+ dataset = load_inference_source(source=SOURCE)
+ bs = dataset.bs # noqa access predictor properties
+ results = model.predict(dataset, stream=True) # source already setup
+ for _, (result, im0, bs) in enumerate(results):
+ print('test_callback', im0.shape)
+ print('test_callback', bs)
+ boxes = result.boxes # Boxes object for bbox outputs
+ print(boxes)
+
+
+def _test_results_api(res):
+ # General apis except plot
+ res = res.cpu().numpy()
+ # res = res.cuda()
+ res = res.to(device='cpu', dtype=torch.float32)
+ res.save_txt('label.txt', save_conf=False)
+ res.save_txt('label.txt', save_conf=True)
+ res.save_crop('crops/')
+ res.tojson(normalize=False)
+ res.tojson(normalize=True)
+ res.plot(pil=True)
+ res.plot(conf=True, boxes=False)
+ res.plot()
+ print(res)
+ print(res.path)
+ for k in res.keys:
+ print(getattr(res, k))
+
+
+def test_results():
+ for m in ['yolov8n-pose.pt', 'yolov8n-seg.pt', 'yolov8n.pt', 'yolov8n-cls.pt']:
+ model = YOLO(m)
+ res = model([SOURCE, SOURCE])
+ _test_results_api(res[0])
+
+
+def test_track():
+ im = cv2.imread(str(SOURCE))
+ for m in ['yolov8n-pose.pt', 'yolov8n-seg.pt', 'yolov8n.pt']:
+ model = YOLO(m)
+ res = model.track(source=im)
+ _test_results_api(res[0])
diff --git a/yolov8/ultralytics/__init__.py b/yolov8/ultralytics/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..5800644cc1c0ae32ffc1fb3a2a8482cea5b4d7a8
--- /dev/null
+++ b/yolov8/ultralytics/__init__.py
@@ -0,0 +1,14 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+__version__ = '8.0.131'
+
+from ultralytics.hub import start
+from ultralytics.vit.rtdetr import RTDETR
+from ultralytics.vit.sam import SAM
+from ultralytics.yolo.engine.model import YOLO
+from ultralytics.yolo.fastsam import FastSAM
+from ultralytics.yolo.nas import NAS
+from ultralytics.yolo.utils.checks import check_yolo as checks
+from ultralytics.yolo.utils.downloads import download
+
+__all__ = '__version__', 'YOLO', 'NAS', 'SAM', 'FastSAM', 'RTDETR', 'checks', 'download', 'start' # allow simpler import
diff --git a/yolov8/ultralytics/assets/bus.jpg b/yolov8/ultralytics/assets/bus.jpg
new file mode 100644
index 0000000000000000000000000000000000000000..40eaaf5c330d0c498fbe1dcacf9bb8bf566797fe
Binary files /dev/null and b/yolov8/ultralytics/assets/bus.jpg differ
diff --git a/yolov8/ultralytics/assets/zidane.jpg b/yolov8/ultralytics/assets/zidane.jpg
new file mode 100644
index 0000000000000000000000000000000000000000..eeab1cdcb282b0e026a57c5bf85df36024b4e1f6
Binary files /dev/null and b/yolov8/ultralytics/assets/zidane.jpg differ
diff --git a/yolov8/ultralytics/hub/__init__.py b/yolov8/ultralytics/hub/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..6059083af3d66d55809442cf49bfc83765e204dc
--- /dev/null
+++ b/yolov8/ultralytics/hub/__init__.py
@@ -0,0 +1,117 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import requests
+
+from ultralytics.hub.auth import Auth
+from ultralytics.hub.utils import PREFIX
+from ultralytics.yolo.data.utils import HUBDatasetStats
+from ultralytics.yolo.utils import LOGGER, SETTINGS, USER_CONFIG_DIR, yaml_save
+
+
+def login(api_key=''):
+ """
+ Log in to the Ultralytics HUB API using the provided API key.
+
+ Args:
+ api_key (str, optional): May be an API key or a combination API key and model ID, i.e. key_id
+
+ Example:
+ from ultralytics import hub
+ hub.login('API_KEY')
+ """
+ Auth(api_key, verbose=True)
+
+
+def logout():
+ """
+ Log out of Ultralytics HUB by removing the API key from the settings file. To log in again, use 'yolo hub login'.
+
+ Example:
+ from ultralytics import hub
+ hub.logout()
+ """
+ SETTINGS['api_key'] = ''
+ yaml_save(USER_CONFIG_DIR / 'settings.yaml', SETTINGS)
+ LOGGER.info(f"{PREFIX}logged out ✅. To log in again, use 'yolo hub login'.")
+
+
+def start(key=''):
+ """
+ Start training models with Ultralytics HUB (DEPRECATED).
+
+ Args:
+ key (str, optional): A string containing either the API key and model ID combination (apikey_modelid),
+ or the full model URL (https://hub.ultralytics.com/models/apikey_modelid).
+ """
+ api_key, model_id = key.split('_')
+ LOGGER.warning(f"""
+WARNING ⚠️ ultralytics.start() is deprecated after 8.0.60. Updated usage to train Ultralytics HUB models is:
+
+from ultralytics import YOLO, hub
+
+hub.login('{api_key}')
+model = YOLO('https://hub.ultralytics.com/models/{model_id}')
+model.train()""")
+
+
+def reset_model(model_id=''):
+ """Reset a trained model to an untrained state."""
+ r = requests.post('https://api.ultralytics.com/model-reset', json={'apiKey': Auth().api_key, 'modelId': model_id})
+ if r.status_code == 200:
+ LOGGER.info(f'{PREFIX}Model reset successfully')
+ return
+ LOGGER.warning(f'{PREFIX}Model reset failure {r.status_code} {r.reason}')
+
+
+def export_fmts_hub():
+ """Returns a list of HUB-supported export formats."""
+ from ultralytics.yolo.engine.exporter import export_formats
+ return list(export_formats()['Argument'][1:]) + ['ultralytics_tflite', 'ultralytics_coreml']
+
+
+def export_model(model_id='', format='torchscript'):
+ """Export a model to all formats."""
+ assert format in export_fmts_hub(), f"Unsupported export format '{format}', valid formats are {export_fmts_hub()}"
+ r = requests.post(f'https://api.ultralytics.com/v1/models/{model_id}/export',
+ json={'format': format},
+ headers={'x-api-key': Auth().api_key})
+ assert r.status_code == 200, f'{PREFIX}{format} export failure {r.status_code} {r.reason}'
+ LOGGER.info(f'{PREFIX}{format} export started ✅')
+
+
+def get_export(model_id='', format='torchscript'):
+ """Get an exported model dictionary with download URL."""
+ assert format in export_fmts_hub(), f"Unsupported export format '{format}', valid formats are {export_fmts_hub()}"
+ r = requests.post('https://api.ultralytics.com/get-export',
+ json={
+ 'apiKey': Auth().api_key,
+ 'modelId': model_id,
+ 'format': format})
+ assert r.status_code == 200, f'{PREFIX}{format} get_export failure {r.status_code} {r.reason}'
+ return r.json()
+
+
+def check_dataset(path='', task='detect'):
+ """
+ Function for error-checking HUB dataset Zip file before upload. It checks a dataset for errors before it is
+ uploaded to the HUB. Usage examples are given below.
+
+ Args:
+ path (str, optional): Path to data.zip (with data.yaml inside data.zip). Defaults to ''.
+ task (str, optional): Dataset task. Options are 'detect', 'segment', 'pose', 'classify'. Defaults to 'detect'.
+
+ Example:
+ ```python
+ from ultralytics.hub import check_dataset
+
+ check_dataset('path/to/coco8.zip', task='detect') # detect dataset
+ check_dataset('path/to/coco8-seg.zip', task='segment') # segment dataset
+ check_dataset('path/to/coco8-pose.zip', task='pose') # pose dataset
+ ```
+ """
+ HUBDatasetStats(path=path, task=task).get_json()
+ LOGGER.info('Checks completed correctly ✅. Upload this dataset to https://hub.ultralytics.com/datasets/.')
+
+
+if __name__ == '__main__':
+ start()
diff --git a/yolov8/ultralytics/hub/auth.py b/yolov8/ultralytics/hub/auth.py
new file mode 100644
index 0000000000000000000000000000000000000000..960b3dc3011a06927e30ec5fbb75b0f593a487f4
--- /dev/null
+++ b/yolov8/ultralytics/hub/auth.py
@@ -0,0 +1,139 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import requests
+
+from ultralytics.hub.utils import HUB_API_ROOT, PREFIX, request_with_credentials
+from ultralytics.yolo.utils import LOGGER, SETTINGS, emojis, is_colab, set_settings
+
+API_KEY_URL = 'https://hub.ultralytics.com/settings?tab=api+keys'
+
+
+class Auth:
+ id_token = api_key = model_key = False
+
+ def __init__(self, api_key='', verbose=False):
+ """
+ Initialize the Auth class with an optional API key.
+
+ Args:
+ api_key (str, optional): May be an API key or a combination API key and model ID, i.e. key_id
+ """
+ # Split the input API key in case it contains a combined key_model and keep only the API key part
+ api_key = api_key.split('_')[0]
+
+ # Set API key attribute as value passed or SETTINGS API key if none passed
+ self.api_key = api_key or SETTINGS.get('api_key', '')
+
+ # If an API key is provided
+ if self.api_key:
+ # If the provided API key matches the API key in the SETTINGS
+ if self.api_key == SETTINGS.get('api_key'):
+ # Log that the user is already logged in
+ if verbose:
+ LOGGER.info(f'{PREFIX}Authenticated ✅')
+ return
+ else:
+ # Attempt to authenticate with the provided API key
+ success = self.authenticate()
+ # If the API key is not provided and the environment is a Google Colab notebook
+ elif is_colab():
+ # Attempt to authenticate using browser cookies
+ success = self.auth_with_cookies()
+ else:
+ # Request an API key
+ success = self.request_api_key()
+
+ # Update SETTINGS with the new API key after successful authentication
+ if success:
+ set_settings({'api_key': self.api_key})
+ # Log that the new login was successful
+ if verbose:
+ LOGGER.info(f'{PREFIX}New authentication successful ✅')
+ elif verbose:
+ LOGGER.info(f'{PREFIX}Retrieve API key from {API_KEY_URL}')
+
+ def request_api_key(self, max_attempts=3):
+ """
+ Prompt the user to input their API key. Returns the model ID.
+ """
+ import getpass
+ for attempts in range(max_attempts):
+ LOGGER.info(f'{PREFIX}Login. Attempt {attempts + 1} of {max_attempts}')
+ input_key = getpass.getpass(f'Enter API key from {API_KEY_URL} ')
+ self.api_key = input_key.split('_')[0] # remove model id if present
+ if self.authenticate():
+ return True
+ raise ConnectionError(emojis(f'{PREFIX}Failed to authenticate ❌'))
+
+ def authenticate(self) -> bool:
+ """
+ Attempt to authenticate with the server using either id_token or API key.
+
+ Returns:
+ bool: True if authentication is successful, False otherwise.
+ """
+ try:
+ header = self.get_auth_header()
+ if header:
+ r = requests.post(f'{HUB_API_ROOT}/v1/auth', headers=header)
+ if not r.json().get('success', False):
+ raise ConnectionError('Unable to authenticate.')
+ return True
+ raise ConnectionError('User has not authenticated locally.')
+ except ConnectionError:
+ self.id_token = self.api_key = False # reset invalid
+ LOGGER.warning(f'{PREFIX}Invalid API key ⚠️')
+ return False
+
+ def auth_with_cookies(self) -> bool:
+ """
+ Attempt to fetch authentication via cookies and set id_token.
+ User must be logged in to HUB and running in a supported browser.
+
+ Returns:
+ bool: True if authentication is successful, False otherwise.
+ """
+ if not is_colab():
+ return False # Currently only works with Colab
+ try:
+ authn = request_with_credentials(f'{HUB_API_ROOT}/v1/auth/auto')
+ if authn.get('success', False):
+ self.id_token = authn.get('data', {}).get('idToken', None)
+ self.authenticate()
+ return True
+ raise ConnectionError('Unable to fetch browser authentication details.')
+ except ConnectionError:
+ self.id_token = False # reset invalid
+ return False
+
+ def get_auth_header(self):
+ """
+ Get the authentication header for making API requests.
+
+ Returns:
+ (dict): The authentication header if id_token or API key is set, None otherwise.
+ """
+ if self.id_token:
+ return {'authorization': f'Bearer {self.id_token}'}
+ elif self.api_key:
+ return {'x-api-key': self.api_key}
+ else:
+ return None
+
+ def get_state(self) -> bool:
+ """
+ Get the authentication state.
+
+ Returns:
+ bool: True if either id_token or API key is set, False otherwise.
+ """
+ return self.id_token or self.api_key
+
+ def set_api_key(self, key: str):
+ """
+ Set the API key for authentication.
+
+ Args:
+ key (str): The API key string.
+ """
+ self.api_key = key
diff --git a/yolov8/ultralytics/hub/session.py b/yolov8/ultralytics/hub/session.py
new file mode 100644
index 0000000000000000000000000000000000000000..01b75fbfaf2c00de29400158ede86f6245f7ab1f
--- /dev/null
+++ b/yolov8/ultralytics/hub/session.py
@@ -0,0 +1,189 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+import signal
+import sys
+from pathlib import Path
+from time import sleep
+
+import requests
+
+from ultralytics.hub.utils import HUB_API_ROOT, PREFIX, smart_request
+from ultralytics.yolo.utils import LOGGER, __version__, checks, emojis, is_colab, threaded
+from ultralytics.yolo.utils.errors import HUBModelError
+
+AGENT_NAME = f'python-{__version__}-colab' if is_colab() else f'python-{__version__}-local'
+
+
+class HUBTrainingSession:
+ """
+ HUB training session for Ultralytics HUB YOLO models. Handles model initialization, heartbeats, and checkpointing.
+
+ Args:
+ url (str): Model identifier used to initialize the HUB training session.
+
+ Attributes:
+ agent_id (str): Identifier for the instance communicating with the server.
+ model_id (str): Identifier for the YOLOv5 model being trained.
+ model_url (str): URL for the model in Ultralytics HUB.
+ api_url (str): API URL for the model in Ultralytics HUB.
+ auth_header (Dict): Authentication header for the Ultralytics HUB API requests.
+ rate_limits (Dict): Rate limits for different API calls (in seconds).
+ timers (Dict): Timers for rate limiting.
+ metrics_queue (Dict): Queue for the model's metrics.
+ model (Dict): Model data fetched from Ultralytics HUB.
+ alive (bool): Indicates if the heartbeat loop is active.
+ """
+
+ def __init__(self, url):
+ """
+ Initialize the HUBTrainingSession with the provided model identifier.
+
+ Args:
+ url (str): Model identifier used to initialize the HUB training session.
+ It can be a URL string or a model key with specific format.
+
+ Raises:
+ ValueError: If the provided model identifier is invalid.
+ ConnectionError: If connecting with global API key is not supported.
+ """
+
+ from ultralytics.hub.auth import Auth
+
+ # Parse input
+ if url.startswith('https://hub.ultralytics.com/models/'):
+ url = url.split('https://hub.ultralytics.com/models/')[-1]
+ if [len(x) for x in url.split('_')] == [42, 20]:
+ key, model_id = url.split('_')
+ elif len(url) == 20:
+ key, model_id = '', url
+ else:
+ raise HUBModelError(f"model='{url}' not found. Check format is correct, i.e. "
+ f"model='https://hub.ultralytics.com/models/MODEL_ID' and try again.")
+
+ # Authorize
+ auth = Auth(key)
+ self.agent_id = None # identifies which instance is communicating with server
+ self.model_id = model_id
+ self.model_url = f'https://hub.ultralytics.com/models/{model_id}'
+ self.api_url = f'{HUB_API_ROOT}/v1/models/{model_id}'
+ self.auth_header = auth.get_auth_header()
+ self.rate_limits = {'metrics': 3.0, 'ckpt': 900.0, 'heartbeat': 300.0} # rate limits (seconds)
+ self.timers = {} # rate limit timers (seconds)
+ self.metrics_queue = {} # metrics queue
+ self.model = self._get_model()
+ self.alive = True
+ self._start_heartbeat() # start heartbeats
+ self._register_signal_handlers()
+ LOGGER.info(f'{PREFIX}View model at {self.model_url} 🚀')
+
+ def _register_signal_handlers(self):
+ """Register signal handlers for SIGTERM and SIGINT signals to gracefully handle termination."""
+ signal.signal(signal.SIGTERM, self._handle_signal)
+ signal.signal(signal.SIGINT, self._handle_signal)
+
+ def _handle_signal(self, signum, frame):
+ """
+ Handle kill signals and prevent heartbeats from being sent on Colab after termination.
+ This method does not use frame, it is included as it is passed by signal.
+ """
+ if self.alive is True:
+ LOGGER.info(f'{PREFIX}Kill signal received! ❌')
+ self._stop_heartbeat()
+ sys.exit(signum)
+
+ def _stop_heartbeat(self):
+ """Terminate the heartbeat loop."""
+ self.alive = False
+
+ def upload_metrics(self):
+ """Upload model metrics to Ultralytics HUB."""
+ payload = {'metrics': self.metrics_queue.copy(), 'type': 'metrics'}
+ smart_request('post', self.api_url, json=payload, headers=self.auth_header, code=2)
+
+ def _get_model(self):
+ """Fetch and return model data from Ultralytics HUB."""
+ api_url = f'{HUB_API_ROOT}/v1/models/{self.model_id}'
+
+ try:
+ response = smart_request('get', api_url, headers=self.auth_header, thread=False, code=0)
+ data = response.json().get('data', None)
+
+ if data.get('status', None) == 'trained':
+ raise ValueError(emojis(f'Model is already trained and uploaded to {self.model_url} 🚀'))
+
+ if not data.get('data', None):
+ raise ValueError('Dataset may still be processing. Please wait a minute and try again.') # RF fix
+ self.model_id = data['id']
+
+ if data['status'] == 'new': # new model to start training
+ self.train_args = {
+ # TODO: deprecate 'batch_size' key for 'batch' in 3Q23
+ 'batch': data['batch' if ('batch' in data) else 'batch_size'],
+ 'epochs': data['epochs'],
+ 'imgsz': data['imgsz'],
+ 'patience': data['patience'],
+ 'device': data['device'],
+ 'cache': data['cache'],
+ 'data': data['data']}
+ self.model_file = data.get('cfg') or data.get('weights') # cfg for pretrained=False
+ self.model_file = checks.check_yolov5u_filename(self.model_file, verbose=False) # YOLOv5->YOLOv5u
+ elif data['status'] == 'training': # existing model to resume training
+ self.train_args = {'data': data['data'], 'resume': True}
+ self.model_file = data['resume']
+
+ return data
+ except requests.exceptions.ConnectionError as e:
+ raise ConnectionRefusedError('ERROR: The HUB server is not online. Please try again later.') from e
+ except Exception:
+ raise
+
+ def upload_model(self, epoch, weights, is_best=False, map=0.0, final=False):
+ """
+ Upload a model checkpoint to Ultralytics HUB.
+
+ Args:
+ epoch (int): The current training epoch.
+ weights (str): Path to the model weights file.
+ is_best (bool): Indicates if the current model is the best one so far.
+ map (float): Mean average precision of the model.
+ final (bool): Indicates if the model is the final model after training.
+ """
+ if Path(weights).is_file():
+ with open(weights, 'rb') as f:
+ file = f.read()
+ else:
+ LOGGER.warning(f'{PREFIX}WARNING ⚠️ Model upload issue. Missing model {weights}.')
+ file = None
+ url = f'{self.api_url}/upload'
+ # url = 'http://httpbin.org/post' # for debug
+ data = {'epoch': epoch}
+ if final:
+ data.update({'type': 'final', 'map': map})
+ smart_request('post',
+ url,
+ data=data,
+ files={'best.pt': file},
+ headers=self.auth_header,
+ retry=10,
+ timeout=3600,
+ thread=False,
+ progress=True,
+ code=4)
+ else:
+ data.update({'type': 'epoch', 'isBest': bool(is_best)})
+ smart_request('post', url, data=data, files={'last.pt': file}, headers=self.auth_header, code=3)
+
+ @threaded
+ def _start_heartbeat(self):
+ """Begin a threaded heartbeat loop to report the agent's status to Ultralytics HUB."""
+ while self.alive:
+ r = smart_request('post',
+ f'{HUB_API_ROOT}/v1/agent/heartbeat/models/{self.model_id}',
+ json={
+ 'agent': AGENT_NAME,
+ 'agentId': self.agent_id},
+ headers=self.auth_header,
+ retry=0,
+ code=5,
+ thread=False) # already in a thread
+ self.agent_id = r.json().get('data', {}).get('agentId', None)
+ sleep(self.rate_limits['heartbeat'])
diff --git a/yolov8/ultralytics/hub/utils.py b/yolov8/ultralytics/hub/utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..ecd64a95e39a62e7a5dcd642f89f38a661afc1c9
--- /dev/null
+++ b/yolov8/ultralytics/hub/utils.py
@@ -0,0 +1,220 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+import platform
+import random
+import sys
+import threading
+import time
+from pathlib import Path
+
+import requests
+from tqdm import tqdm
+
+from ultralytics.yolo.utils import (ENVIRONMENT, LOGGER, ONLINE, RANK, SETTINGS, TESTS_RUNNING, TQDM_BAR_FORMAT,
+ TryExcept, __version__, colorstr, get_git_origin_url, is_colab, is_git_dir,
+ is_pip_package)
+
+PREFIX = colorstr('Ultralytics HUB: ')
+HELP_MSG = 'If this issue persists please visit https://github.com/ultralytics/hub/issues for assistance.'
+HUB_API_ROOT = os.environ.get('ULTRALYTICS_HUB_API', 'https://api.ultralytics.com')
+
+
+def request_with_credentials(url: str) -> any:
+ """
+ Make an AJAX request with cookies attached in a Google Colab environment.
+
+ Args:
+ url (str): The URL to make the request to.
+
+ Returns:
+ (any): The response data from the AJAX request.
+
+ Raises:
+ OSError: If the function is not run in a Google Colab environment.
+ """
+ if not is_colab():
+ raise OSError('request_with_credentials() must run in a Colab environment')
+ from google.colab import output # noqa
+ from IPython import display # noqa
+ display.display(
+ display.Javascript("""
+ window._hub_tmp = new Promise((resolve, reject) => {
+ const timeout = setTimeout(() => reject("Failed authenticating existing browser session"), 5000)
+ fetch("%s", {
+ method: 'POST',
+ credentials: 'include'
+ })
+ .then((response) => resolve(response.json()))
+ .then((json) => {
+ clearTimeout(timeout);
+ }).catch((err) => {
+ clearTimeout(timeout);
+ reject(err);
+ });
+ });
+ """ % url))
+ return output.eval_js('_hub_tmp')
+
+
+def requests_with_progress(method, url, **kwargs):
+ """
+ Make an HTTP request using the specified method and URL, with an optional progress bar.
+
+ Args:
+ method (str): The HTTP method to use (e.g. 'GET', 'POST').
+ url (str): The URL to send the request to.
+ **kwargs (dict): Additional keyword arguments to pass to the underlying `requests.request` function.
+
+ Returns:
+ (requests.Response): The response object from the HTTP request.
+
+ Note:
+ If 'progress' is set to True, the progress bar will display the download progress
+ for responses with a known content length.
+ """
+ progress = kwargs.pop('progress', False)
+ if not progress:
+ return requests.request(method, url, **kwargs)
+ response = requests.request(method, url, stream=True, **kwargs)
+ total = int(response.headers.get('content-length', 0)) # total size
+ try:
+ pbar = tqdm(total=total, unit='B', unit_scale=True, unit_divisor=1024, bar_format=TQDM_BAR_FORMAT)
+ for data in response.iter_content(chunk_size=1024):
+ pbar.update(len(data))
+ pbar.close()
+ except requests.exceptions.ChunkedEncodingError: # avoid 'Connection broken: IncompleteRead' warnings
+ response.close()
+ return response
+
+
+def smart_request(method, url, retry=3, timeout=30, thread=True, code=-1, verbose=True, progress=False, **kwargs):
+ """
+ Makes an HTTP request using the 'requests' library, with exponential backoff retries up to a specified timeout.
+
+ Args:
+ method (str): The HTTP method to use for the request. Choices are 'post' and 'get'.
+ url (str): The URL to make the request to.
+ retry (int, optional): Number of retries to attempt before giving up. Default is 3.
+ timeout (int, optional): Timeout in seconds after which the function will give up retrying. Default is 30.
+ thread (bool, optional): Whether to execute the request in a separate daemon thread. Default is True.
+ code (int, optional): An identifier for the request, used for logging purposes. Default is -1.
+ verbose (bool, optional): A flag to determine whether to print out to console or not. Default is True.
+ progress (bool, optional): Whether to show a progress bar during the request. Default is False.
+ **kwargs (dict): Keyword arguments to be passed to the requests function specified in method.
+
+ Returns:
+ (requests.Response): The HTTP response object. If the request is executed in a separate thread, returns None.
+ """
+ retry_codes = (408, 500) # retry only these codes
+
+ @TryExcept(verbose=verbose)
+ def func(func_method, func_url, **func_kwargs):
+ """Make HTTP requests with retries and timeouts, with optional progress tracking."""
+ r = None # response
+ t0 = time.time() # initial time for timer
+ for i in range(retry + 1):
+ if (time.time() - t0) > timeout:
+ break
+ r = requests_with_progress(func_method, func_url, **func_kwargs) # i.e. get(url, data, json, files)
+ if r.status_code < 300: # return codes in the 2xx range are generally considered "good" or "successful"
+ break
+ try:
+ m = r.json().get('message', 'No JSON message.')
+ except AttributeError:
+ m = 'Unable to read JSON.'
+ if i == 0:
+ if r.status_code in retry_codes:
+ m += f' Retrying {retry}x for {timeout}s.' if retry else ''
+ elif r.status_code == 429: # rate limit
+ h = r.headers # response headers
+ m = f"Rate limit reached ({h['X-RateLimit-Remaining']}/{h['X-RateLimit-Limit']}). " \
+ f"Please retry after {h['Retry-After']}s."
+ if verbose:
+ LOGGER.warning(f'{PREFIX}{m} {HELP_MSG} ({r.status_code} #{code})')
+ if r.status_code not in retry_codes:
+ return r
+ time.sleep(2 ** i) # exponential standoff
+ return r
+
+ args = method, url
+ kwargs['progress'] = progress
+ if thread:
+ threading.Thread(target=func, args=args, kwargs=kwargs, daemon=True).start()
+ else:
+ return func(*args, **kwargs)
+
+
+class Events:
+ """
+ A class for collecting anonymous event analytics. Event analytics are enabled when sync=True in settings and
+ disabled when sync=False. Run 'yolo settings' to see and update settings YAML file.
+
+ Attributes:
+ url (str): The URL to send anonymous events.
+ rate_limit (float): The rate limit in seconds for sending events.
+ metadata (dict): A dictionary containing metadata about the environment.
+ enabled (bool): A flag to enable or disable Events based on certain conditions.
+ """
+
+ url = 'https://www.google-analytics.com/mp/collect?measurement_id=G-X8NCJYTQXM&api_secret=QLQrATrNSwGRFRLE-cbHJw'
+
+ def __init__(self):
+ """
+ Initializes the Events object with default values for events, rate_limit, and metadata.
+ """
+ self.events = [] # events list
+ self.rate_limit = 60.0 # rate limit (seconds)
+ self.t = 0.0 # rate limit timer (seconds)
+ self.metadata = {
+ 'cli': Path(sys.argv[0]).name == 'yolo',
+ 'install': 'git' if is_git_dir() else 'pip' if is_pip_package() else 'other',
+ 'python': '.'.join(platform.python_version_tuple()[:2]), # i.e. 3.10
+ 'version': __version__,
+ 'env': ENVIRONMENT,
+ 'session_id': round(random.random() * 1E15),
+ 'engagement_time_msec': 1000}
+ self.enabled = \
+ SETTINGS['sync'] and \
+ RANK in (-1, 0) and \
+ not TESTS_RUNNING and \
+ ONLINE and \
+ (is_pip_package() or get_git_origin_url() == 'https://github.com/ultralytics/ultralytics.git')
+
+ def __call__(self, cfg):
+ """
+ Attempts to add a new event to the events list and send events if the rate limit is reached.
+
+ Args:
+ cfg (IterableSimpleNamespace): The configuration object containing mode and task information.
+ """
+ if not self.enabled:
+ # Events disabled, do nothing
+ return
+
+ # Attempt to add to events
+ if len(self.events) < 25: # Events list limited to 25 events (drop any events past this)
+ params = {**self.metadata, **{'task': cfg.task}}
+ if cfg.mode == 'export':
+ params['format'] = cfg.format
+ self.events.append({'name': cfg.mode, 'params': params})
+
+ # Check rate limit
+ t = time.time()
+ if (t - self.t) < self.rate_limit:
+ # Time is under rate limiter, wait to send
+ return
+
+ # Time is over rate limiter, send now
+ data = {'client_id': SETTINGS['uuid'], 'events': self.events} # SHA-256 anonymized UUID hash and events list
+
+ # POST equivalent to requests.post(self.url, json=data)
+ smart_request('post', self.url, json=data, retry=0, verbose=False)
+
+ # Reset events and rate limit timer
+ self.events = []
+ self.t = t
+
+
+# Run below code on hub/utils init -------------------------------------------------------------------------------------
+events = Events()
diff --git a/yolov8/ultralytics/models/README.md b/yolov8/ultralytics/models/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..a0edc4b06aa06c454bdd6d97087df6970e689871
--- /dev/null
+++ b/yolov8/ultralytics/models/README.md
@@ -0,0 +1,45 @@
+## Models
+
+Welcome to the Ultralytics Models directory! Here you will find a wide variety of pre-configured model configuration
+files (`*.yaml`s) that can be used to create custom YOLO models. The models in this directory have been expertly crafted
+and fine-tuned by the Ultralytics team to provide the best performance for a wide range of object detection and image
+segmentation tasks.
+
+These model configurations cover a wide range of scenarios, from simple object detection to more complex tasks like
+instance segmentation and object tracking. They are also designed to run efficiently on a variety of hardware platforms,
+from CPUs to GPUs. Whether you are a seasoned machine learning practitioner or just getting started with YOLO, this
+directory provides a great starting point for your custom model development needs.
+
+To get started, simply browse through the models in this directory and find one that best suits your needs. Once you've
+selected a model, you can use the provided `*.yaml` file to train and deploy your custom YOLO model with ease. See full
+details at the Ultralytics [Docs](https://docs.ultralytics.com/models), and if you need help or have any questions, feel free
+to reach out to the Ultralytics team for support. So, don't wait, start creating your custom YOLO model now!
+
+### Usage
+
+Model `*.yaml` files may be used directly in the Command Line Interface (CLI) with a `yolo` command:
+
+```bash
+yolo task=detect mode=train model=yolov8n.yaml data=coco128.yaml epochs=100
+```
+
+They may also be used directly in a Python environment, and accepts the same
+[arguments](https://docs.ultralytics.com/usage/cfg/) as in the CLI example above:
+
+```python
+from ultralytics import YOLO
+
+model = YOLO("model.yaml") # build a YOLOv8n model from scratch
+# YOLO("model.pt") use pre-trained model if available
+model.info() # display model information
+model.train(data="coco128.yaml", epochs=100) # train the model
+```
+
+## Pre-trained Model Architectures
+
+Ultralytics supports many model architectures. Visit https://docs.ultralytics.com/models to view detailed information
+and usage. Any of these models can be used by loading their configs or pretrained checkpoints if available.
+
+## Contributing New Models
+
+If you've developed a new model architecture or have improvements for existing models that you'd like to contribute to the Ultralytics community, please submit your contribution in a new Pull Request. For more details, visit our [Contributing Guide](https://docs.ultralytics.com/help/contributing).
diff --git a/yolov8/ultralytics/models/rt-detr/rtdetr-l.yaml b/yolov8/ultralytics/models/rt-detr/rtdetr-l.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..bd20da16f0828a375f1f469c1e698d6351e67aa8
--- /dev/null
+++ b/yolov8/ultralytics/models/rt-detr/rtdetr-l.yaml
@@ -0,0 +1,50 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# RT-DETR-l object detection model with P3-P5 outputs. For details see https://docs.ultralytics.com/models/rtdetr
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n-cls.yaml' will call yolov8-cls.yaml with scale 'n'
+ # [depth, width, max_channels]
+ l: [1.00, 1.00, 1024]
+
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, HGStem, [32, 48]] # 0-P2/4
+ - [-1, 6, HGBlock, [48, 128, 3]] # stage 1
+
+ - [-1, 1, DWConv, [128, 3, 2, 1, False]] # 2-P3/8
+ - [-1, 6, HGBlock, [96, 512, 3]] # stage 2
+
+ - [-1, 1, DWConv, [512, 3, 2, 1, False]] # 4-P3/16
+ - [-1, 6, HGBlock, [192, 1024, 5, True, False]] # cm, c2, k, light, shortcut
+ - [-1, 6, HGBlock, [192, 1024, 5, True, True]]
+ - [-1, 6, HGBlock, [192, 1024, 5, True, True]] # stage 3
+
+ - [-1, 1, DWConv, [1024, 3, 2, 1, False]] # 8-P4/32
+ - [-1, 6, HGBlock, [384, 2048, 5, True, False]] # stage 4
+
+head:
+ - [-1, 1, Conv, [256, 1, 1, None, 1, 1, False]] # 10 input_proj.2
+ - [-1, 1, AIFI, [1024, 8]]
+ - [-1, 1, Conv, [256, 1, 1]] # 12, Y5, lateral_convs.0
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [7, 1, Conv, [256, 1, 1, None, 1, 1, False]] # 14 input_proj.1
+ - [[-2, -1], 1, Concat, [1]]
+ - [-1, 3, RepC3, [256]] # 16, fpn_blocks.0
+ - [-1, 1, Conv, [256, 1, 1]] # 17, Y4, lateral_convs.1
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [3, 1, Conv, [256, 1, 1, None, 1, 1, False]] # 19 input_proj.0
+ - [[-2, -1], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, RepC3, [256]] # X3 (21), fpn_blocks.1
+
+ - [-1, 1, Conv, [256, 3, 2]] # 22, downsample_convs.0
+ - [[-1, 17], 1, Concat, [1]] # cat Y4
+ - [-1, 3, RepC3, [256]] # F4 (24), pan_blocks.0
+
+ - [-1, 1, Conv, [256, 3, 2]] # 25, downsample_convs.1
+ - [[-1, 12], 1, Concat, [1]] # cat Y5
+ - [-1, 3, RepC3, [256]] # F5 (27), pan_blocks.1
+
+ - [[21, 24, 27], 1, RTDETRDecoder, [nc]] # Detect(P3, P4, P5)
diff --git a/yolov8/ultralytics/models/rt-detr/rtdetr-x.yaml b/yolov8/ultralytics/models/rt-detr/rtdetr-x.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..848cb52b1f7430331ec625f56e496feb31f3f8eb
--- /dev/null
+++ b/yolov8/ultralytics/models/rt-detr/rtdetr-x.yaml
@@ -0,0 +1,54 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# RT-DETR-x object detection model with P3-P5 outputs. For details see https://docs.ultralytics.com/models/rtdetr
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n-cls.yaml' will call yolov8-cls.yaml with scale 'n'
+ # [depth, width, max_channels]
+ x: [1.00, 1.00, 2048]
+
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, HGStem, [32, 64]] # 0-P2/4
+ - [-1, 6, HGBlock, [64, 128, 3]] # stage 1
+
+ - [-1, 1, DWConv, [128, 3, 2, 1, False]] # 2-P3/8
+ - [-1, 6, HGBlock, [128, 512, 3]]
+ - [-1, 6, HGBlock, [128, 512, 3, False, True]] # 4-stage 2
+
+ - [-1, 1, DWConv, [512, 3, 2, 1, False]] # 5-P3/16
+ - [-1, 6, HGBlock, [256, 1024, 5, True, False]] # cm, c2, k, light, shortcut
+ - [-1, 6, HGBlock, [256, 1024, 5, True, True]]
+ - [-1, 6, HGBlock, [256, 1024, 5, True, True]]
+ - [-1, 6, HGBlock, [256, 1024, 5, True, True]]
+ - [-1, 6, HGBlock, [256, 1024, 5, True, True]] # 10-stage 3
+
+ - [-1, 1, DWConv, [1024, 3, 2, 1, False]] # 11-P4/32
+ - [-1, 6, HGBlock, [512, 2048, 5, True, False]]
+ - [-1, 6, HGBlock, [512, 2048, 5, True, True]] # 13-stage 4
+
+head:
+ - [-1, 1, Conv, [384, 1, 1, None, 1, 1, False]] # 14 input_proj.2
+ - [-1, 1, AIFI, [2048, 8]]
+ - [-1, 1, Conv, [384, 1, 1]] # 16, Y5, lateral_convs.0
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [10, 1, Conv, [384, 1, 1, None, 1, 1, False]] # 18 input_proj.1
+ - [[-2, -1], 1, Concat, [1]]
+ - [-1, 3, RepC3, [384]] # 20, fpn_blocks.0
+ - [-1, 1, Conv, [384, 1, 1]] # 21, Y4, lateral_convs.1
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [4, 1, Conv, [384, 1, 1, None, 1, 1, False]] # 23 input_proj.0
+ - [[-2, -1], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, RepC3, [384]] # X3 (25), fpn_blocks.1
+
+ - [-1, 1, Conv, [384, 3, 2]] # 26, downsample_convs.0
+ - [[-1, 21], 1, Concat, [1]] # cat Y4
+ - [-1, 3, RepC3, [384]] # F4 (28), pan_blocks.0
+
+ - [-1, 1, Conv, [384, 3, 2]] # 29, downsample_convs.1
+ - [[-1, 16], 1, Concat, [1]] # cat Y5
+ - [-1, 3, RepC3, [384]] # F5 (31), pan_blocks.1
+
+ - [[25, 28, 31], 1, RTDETRDecoder, [nc]] # Detect(P3, P4, P5)
diff --git a/yolov8/ultralytics/models/v3/yolov3-spp.yaml b/yolov8/ultralytics/models/v3/yolov3-spp.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..406e019b4c84d9b8de5cfc7e9f9d9d49c5eead76
--- /dev/null
+++ b/yolov8/ultralytics/models/v3/yolov3-spp.yaml
@@ -0,0 +1,48 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv3-SPP object detection model with P3-P5 outputs. For details see https://docs.ultralytics.com/models/yolov3
+
+# Parameters
+nc: 80 # number of classes
+depth_multiple: 1.0 # model depth multiple
+width_multiple: 1.0 # layer channel multiple
+
+# darknet53 backbone
+backbone:
+ # [from, number, module, args]
+ [[-1, 1, Conv, [32, 3, 1]], # 0
+ [-1, 1, Conv, [64, 3, 2]], # 1-P1/2
+ [-1, 1, Bottleneck, [64]],
+ [-1, 1, Conv, [128, 3, 2]], # 3-P2/4
+ [-1, 2, Bottleneck, [128]],
+ [-1, 1, Conv, [256, 3, 2]], # 5-P3/8
+ [-1, 8, Bottleneck, [256]],
+ [-1, 1, Conv, [512, 3, 2]], # 7-P4/16
+ [-1, 8, Bottleneck, [512]],
+ [-1, 1, Conv, [1024, 3, 2]], # 9-P5/32
+ [-1, 4, Bottleneck, [1024]], # 10
+ ]
+
+# YOLOv3-SPP head
+head:
+ [[-1, 1, Bottleneck, [1024, False]],
+ [-1, 1, SPP, [512, [5, 9, 13]]],
+ [-1, 1, Conv, [1024, 3, 1]],
+ [-1, 1, Conv, [512, 1, 1]],
+ [-1, 1, Conv, [1024, 3, 1]], # 15 (P5/32-large)
+
+ [-2, 1, Conv, [256, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 8], 1, Concat, [1]], # cat backbone P4
+ [-1, 1, Bottleneck, [512, False]],
+ [-1, 1, Bottleneck, [512, False]],
+ [-1, 1, Conv, [256, 1, 1]],
+ [-1, 1, Conv, [512, 3, 1]], # 22 (P4/16-medium)
+
+ [-2, 1, Conv, [128, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 6], 1, Concat, [1]], # cat backbone P3
+ [-1, 1, Bottleneck, [256, False]],
+ [-1, 2, Bottleneck, [256, False]], # 27 (P3/8-small)
+
+ [[27, 22, 15], 1, Detect, [nc]], # Detect(P3, P4, P5)
+ ]
diff --git a/yolov8/ultralytics/models/v3/yolov3-tiny.yaml b/yolov8/ultralytics/models/v3/yolov3-tiny.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..69d8e42ce68daee04bd777f22ebf8f4c6d60de9a
--- /dev/null
+++ b/yolov8/ultralytics/models/v3/yolov3-tiny.yaml
@@ -0,0 +1,39 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv3-tiny object detection model with P4-P5 outputs. For details see https://docs.ultralytics.com/models/yolov3
+
+# Parameters
+nc: 80 # number of classes
+depth_multiple: 1.0 # model depth multiple
+width_multiple: 1.0 # layer channel multiple
+
+# YOLOv3-tiny backbone
+backbone:
+ # [from, number, module, args]
+ [[-1, 1, Conv, [16, 3, 1]], # 0
+ [-1, 1, nn.MaxPool2d, [2, 2, 0]], # 1-P1/2
+ [-1, 1, Conv, [32, 3, 1]],
+ [-1, 1, nn.MaxPool2d, [2, 2, 0]], # 3-P2/4
+ [-1, 1, Conv, [64, 3, 1]],
+ [-1, 1, nn.MaxPool2d, [2, 2, 0]], # 5-P3/8
+ [-1, 1, Conv, [128, 3, 1]],
+ [-1, 1, nn.MaxPool2d, [2, 2, 0]], # 7-P4/16
+ [-1, 1, Conv, [256, 3, 1]],
+ [-1, 1, nn.MaxPool2d, [2, 2, 0]], # 9-P5/32
+ [-1, 1, Conv, [512, 3, 1]],
+ [-1, 1, nn.ZeroPad2d, [[0, 1, 0, 1]]], # 11
+ [-1, 1, nn.MaxPool2d, [2, 1, 0]], # 12
+ ]
+
+# YOLOv3-tiny head
+head:
+ [[-1, 1, Conv, [1024, 3, 1]],
+ [-1, 1, Conv, [256, 1, 1]],
+ [-1, 1, Conv, [512, 3, 1]], # 15 (P5/32-large)
+
+ [-2, 1, Conv, [128, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 8], 1, Concat, [1]], # cat backbone P4
+ [-1, 1, Conv, [256, 3, 1]], # 19 (P4/16-medium)
+
+ [[19, 15], 1, Detect, [nc]], # Detect(P4, P5)
+ ]
diff --git a/yolov8/ultralytics/models/v3/yolov3.yaml b/yolov8/ultralytics/models/v3/yolov3.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..7cc0afa173e09af9f09f752b45ad8bd113db612f
--- /dev/null
+++ b/yolov8/ultralytics/models/v3/yolov3.yaml
@@ -0,0 +1,48 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv3 object detection model with P3-P5 outputs. For details see https://docs.ultralytics.com/models/yolov3
+
+# Parameters
+nc: 80 # number of classes
+depth_multiple: 1.0 # model depth multiple
+width_multiple: 1.0 # layer channel multiple
+
+# darknet53 backbone
+backbone:
+ # [from, number, module, args]
+ [[-1, 1, Conv, [32, 3, 1]], # 0
+ [-1, 1, Conv, [64, 3, 2]], # 1-P1/2
+ [-1, 1, Bottleneck, [64]],
+ [-1, 1, Conv, [128, 3, 2]], # 3-P2/4
+ [-1, 2, Bottleneck, [128]],
+ [-1, 1, Conv, [256, 3, 2]], # 5-P3/8
+ [-1, 8, Bottleneck, [256]],
+ [-1, 1, Conv, [512, 3, 2]], # 7-P4/16
+ [-1, 8, Bottleneck, [512]],
+ [-1, 1, Conv, [1024, 3, 2]], # 9-P5/32
+ [-1, 4, Bottleneck, [1024]], # 10
+ ]
+
+# YOLOv3 head
+head:
+ [[-1, 1, Bottleneck, [1024, False]],
+ [-1, 1, Conv, [512, 1, 1]],
+ [-1, 1, Conv, [1024, 3, 1]],
+ [-1, 1, Conv, [512, 1, 1]],
+ [-1, 1, Conv, [1024, 3, 1]], # 15 (P5/32-large)
+
+ [-2, 1, Conv, [256, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 8], 1, Concat, [1]], # cat backbone P4
+ [-1, 1, Bottleneck, [512, False]],
+ [-1, 1, Bottleneck, [512, False]],
+ [-1, 1, Conv, [256, 1, 1]],
+ [-1, 1, Conv, [512, 3, 1]], # 22 (P4/16-medium)
+
+ [-2, 1, Conv, [128, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 6], 1, Concat, [1]], # cat backbone P3
+ [-1, 1, Bottleneck, [256, False]],
+ [-1, 2, Bottleneck, [256, False]], # 27 (P3/8-small)
+
+ [[27, 22, 15], 1, Detect, [nc]], # Detect(P3, P4, P5)
+ ]
diff --git a/yolov8/ultralytics/models/v5/yolov5-p6.yaml b/yolov8/ultralytics/models/v5/yolov5-p6.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..d4683771b50057b216aa48c5d8e5722a671d077c
--- /dev/null
+++ b/yolov8/ultralytics/models/v5/yolov5-p6.yaml
@@ -0,0 +1,61 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv5 object detection model with P3-P6 outputs. For details see https://docs.ultralytics.com/models/yolov5
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov5n-p6.yaml' will call yolov5-p6.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 1024]
+ l: [1.00, 1.00, 1024]
+ x: [1.33, 1.25, 1024]
+
+# YOLOv5 v6.0 backbone
+backbone:
+ # [from, number, module, args]
+ [[-1, 1, Conv, [64, 6, 2, 2]], # 0-P1/2
+ [-1, 1, Conv, [128, 3, 2]], # 1-P2/4
+ [-1, 3, C3, [128]],
+ [-1, 1, Conv, [256, 3, 2]], # 3-P3/8
+ [-1, 6, C3, [256]],
+ [-1, 1, Conv, [512, 3, 2]], # 5-P4/16
+ [-1, 9, C3, [512]],
+ [-1, 1, Conv, [768, 3, 2]], # 7-P5/32
+ [-1, 3, C3, [768]],
+ [-1, 1, Conv, [1024, 3, 2]], # 9-P6/64
+ [-1, 3, C3, [1024]],
+ [-1, 1, SPPF, [1024, 5]], # 11
+ ]
+
+# YOLOv5 v6.0 head
+head:
+ [[-1, 1, Conv, [768, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 8], 1, Concat, [1]], # cat backbone P5
+ [-1, 3, C3, [768, False]], # 15
+
+ [-1, 1, Conv, [512, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 6], 1, Concat, [1]], # cat backbone P4
+ [-1, 3, C3, [512, False]], # 19
+
+ [-1, 1, Conv, [256, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 4], 1, Concat, [1]], # cat backbone P3
+ [-1, 3, C3, [256, False]], # 23 (P3/8-small)
+
+ [-1, 1, Conv, [256, 3, 2]],
+ [[-1, 20], 1, Concat, [1]], # cat head P4
+ [-1, 3, C3, [512, False]], # 26 (P4/16-medium)
+
+ [-1, 1, Conv, [512, 3, 2]],
+ [[-1, 16], 1, Concat, [1]], # cat head P5
+ [-1, 3, C3, [768, False]], # 29 (P5/32-large)
+
+ [-1, 1, Conv, [768, 3, 2]],
+ [[-1, 12], 1, Concat, [1]], # cat head P6
+ [-1, 3, C3, [1024, False]], # 32 (P6/64-xlarge)
+
+ [[23, 26, 29, 32], 1, Detect, [nc]], # Detect(P3, P4, P5, P6)
+ ]
diff --git a/yolov8/ultralytics/models/v5/yolov5.yaml b/yolov8/ultralytics/models/v5/yolov5.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..4a3fcedf7c8d8e935c637fe80f52f94310841f45
--- /dev/null
+++ b/yolov8/ultralytics/models/v5/yolov5.yaml
@@ -0,0 +1,50 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv5 object detection model with P3-P5 outputs. For details see https://docs.ultralytics.com/models/yolov5
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov5n.yaml' will call yolov5.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 1024]
+ l: [1.00, 1.00, 1024]
+ x: [1.33, 1.25, 1024]
+
+# YOLOv5 v6.0 backbone
+backbone:
+ # [from, number, module, args]
+ [[-1, 1, Conv, [64, 6, 2, 2]], # 0-P1/2
+ [-1, 1, Conv, [128, 3, 2]], # 1-P2/4
+ [-1, 3, C3, [128]],
+ [-1, 1, Conv, [256, 3, 2]], # 3-P3/8
+ [-1, 6, C3, [256]],
+ [-1, 1, Conv, [512, 3, 2]], # 5-P4/16
+ [-1, 9, C3, [512]],
+ [-1, 1, Conv, [1024, 3, 2]], # 7-P5/32
+ [-1, 3, C3, [1024]],
+ [-1, 1, SPPF, [1024, 5]], # 9
+ ]
+
+# YOLOv5 v6.0 head
+head:
+ [[-1, 1, Conv, [512, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 6], 1, Concat, [1]], # cat backbone P4
+ [-1, 3, C3, [512, False]], # 13
+
+ [-1, 1, Conv, [256, 1, 1]],
+ [-1, 1, nn.Upsample, [None, 2, 'nearest']],
+ [[-1, 4], 1, Concat, [1]], # cat backbone P3
+ [-1, 3, C3, [256, False]], # 17 (P3/8-small)
+
+ [-1, 1, Conv, [256, 3, 2]],
+ [[-1, 14], 1, Concat, [1]], # cat head P4
+ [-1, 3, C3, [512, False]], # 20 (P4/16-medium)
+
+ [-1, 1, Conv, [512, 3, 2]],
+ [[-1, 10], 1, Concat, [1]], # cat head P5
+ [-1, 3, C3, [1024, False]], # 23 (P5/32-large)
+
+ [[17, 20, 23], 1, Detect, [nc]], # Detect(P3, P4, P5)
+ ]
diff --git a/yolov8/ultralytics/models/v6/yolov6.yaml b/yolov8/ultralytics/models/v6/yolov6.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..cb5e32ac3a75d49ddbda9e4cecdc1265ed209401
--- /dev/null
+++ b/yolov8/ultralytics/models/v6/yolov6.yaml
@@ -0,0 +1,53 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv6 object detection model with P3-P5 outputs. For Usage examples see https://docs.ultralytics.com/models/yolov6
+
+# Parameters
+nc: 80 # number of classes
+activation: nn.ReLU() # (optional) model default activation function
+scales: # model compound scaling constants, i.e. 'model=yolov6n.yaml' will call yolov8.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 768]
+ l: [1.00, 1.00, 512]
+ x: [1.00, 1.25, 512]
+
+# YOLOv6-3.0s backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 6, Conv, [128, 3, 1]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 12, Conv, [256, 3, 1]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 18, Conv, [512, 3, 1]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 6, Conv, [1024, 3, 1]]
+ - [-1, 1, SPPF, [1024, 5]] # 9
+
+# YOLOv6-3.0s head
+head:
+ - [-1, 1, Conv, [256, 1, 1]]
+ - [-1, 1, nn.ConvTranspose2d, [256, 2, 2, 0]]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 1, Conv, [256, 3, 1]]
+ - [-1, 9, Conv, [256, 3, 1]] # 14
+
+ - [-1, 1, Conv, [128, 1, 1]]
+ - [-1, 1, nn.ConvTranspose2d, [128, 2, 2, 0]]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 1, Conv, [128, 3, 1]]
+ - [-1, 9, Conv, [128, 3, 1]] # 19
+
+ - [-1, 1, Conv, [128, 3, 2]]
+ - [[-1, 15], 1, Concat, [1]] # cat head P4
+ - [-1, 1, Conv, [256, 3, 1]]
+ - [-1, 9, Conv, [256, 3, 1]] # 23
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 10], 1, Concat, [1]] # cat head P5
+ - [-1, 1, Conv, [512, 3, 1]]
+ - [-1, 9, Conv, [512, 3, 1]] # 27
+
+ - [[19, 23, 27], 1, Detect, [nc]] # Detect(P3, P4, P5)
diff --git a/yolov8/ultralytics/models/v8/yolov8-cls.yaml b/yolov8/ultralytics/models/v8/yolov8-cls.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..5332f1d64ee32bdf8d5cb995e5ef3a3f6f970413
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-cls.yaml
@@ -0,0 +1,29 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8-cls image classification model. For Usage examples see https://docs.ultralytics.com/tasks/classify
+
+# Parameters
+nc: 1000 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n-cls.yaml' will call yolov8-cls.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 1024]
+ l: [1.00, 1.00, 1024]
+ x: [1.00, 1.25, 1024]
+
+# YOLOv8.0n backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [1024, True]]
+
+# YOLOv8.0n head
+head:
+ - [-1, 1, Classify, [nc]] # Classify
diff --git a/yolov8/ultralytics/models/v8/yolov8-p2.yaml b/yolov8/ultralytics/models/v8/yolov8-p2.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..3e286aa96f6f9ba79970c8fead0e83782f70bfca
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-p2.yaml
@@ -0,0 +1,54 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8 object detection model with P2-P5 outputs. For Usage examples see https://docs.ultralytics.com/tasks/detect
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n.yaml' will call yolov8.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 768]
+ l: [1.00, 1.00, 512]
+ x: [1.00, 1.25, 512]
+
+# YOLOv8.0 backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 9
+
+# YOLOv8.0-p2 head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2f, [512]] # 12
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2f, [256]] # 15 (P3/8-small)
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 2], 1, Concat, [1]] # cat backbone P2
+ - [-1, 3, C2f, [128]] # 18 (P2/4-xsmall)
+
+ - [-1, 1, Conv, [128, 3, 2]]
+ - [[-1, 15], 1, Concat, [1]] # cat head P3
+ - [-1, 3, C2f, [256]] # 21 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 12], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2f, [512]] # 24 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 9], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2f, [1024]] # 27 (P5/32-large)
+
+ - [[18, 21, 24, 27], 1, Detect, [nc]] # Detect(P2, P3, P4, P5)
diff --git a/yolov8/ultralytics/models/v8/yolov8-p6.yaml b/yolov8/ultralytics/models/v8/yolov8-p6.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..3635ed97ebc4219188f174be99fa301daeaf49b8
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-p6.yaml
@@ -0,0 +1,56 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8 object detection model with P3-P6 outputs. For Usage examples see https://docs.ultralytics.com/tasks/detect
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n-p6.yaml' will call yolov8-p6.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 768]
+ l: [1.00, 1.00, 512]
+ x: [1.00, 1.25, 512]
+
+# YOLOv8.0x6 backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [768, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [768, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 9-P6/64
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 11
+
+# YOLOv8.0x6 head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 8], 1, Concat, [1]] # cat backbone P5
+ - [-1, 3, C2, [768, False]] # 14
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2, [512, False]] # 17
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2, [256, False]] # 20 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 17], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2, [512, False]] # 23 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 14], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2, [768, False]] # 26 (P5/32-large)
+
+ - [-1, 1, Conv, [768, 3, 2]]
+ - [[-1, 11], 1, Concat, [1]] # cat head P6
+ - [-1, 3, C2, [1024, False]] # 29 (P6/64-xlarge)
+
+ - [[20, 23, 26, 29], 1, Detect, [nc]] # Detect(P3, P4, P5, P6)
diff --git a/yolov8/ultralytics/models/v8/yolov8-pose-p6.yaml b/yolov8/ultralytics/models/v8/yolov8-pose-p6.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..06381fb196673f9751677f9993540fe74e88ba03
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-pose-p6.yaml
@@ -0,0 +1,57 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8-pose keypoints/pose estimation model. For Usage examples see https://docs.ultralytics.com/tasks/pose
+
+# Parameters
+nc: 1 # number of classes
+kpt_shape: [17, 3] # number of keypoints, number of dims (2 for x,y or 3 for x,y,visible)
+scales: # model compound scaling constants, i.e. 'model=yolov8n-p6.yaml' will call yolov8-p6.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 768]
+ l: [1.00, 1.00, 512]
+ x: [1.00, 1.25, 512]
+
+# YOLOv8.0x6 backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [768, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [768, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 9-P6/64
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 11
+
+# YOLOv8.0x6 head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 8], 1, Concat, [1]] # cat backbone P5
+ - [-1, 3, C2, [768, False]] # 14
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2, [512, False]] # 17
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2, [256, False]] # 20 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 17], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2, [512, False]] # 23 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 14], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2, [768, False]] # 26 (P5/32-large)
+
+ - [-1, 1, Conv, [768, 3, 2]]
+ - [[-1, 11], 1, Concat, [1]] # cat head P6
+ - [-1, 3, C2, [1024, False]] # 29 (P6/64-xlarge)
+
+ - [[20, 23, 26, 29], 1, Pose, [nc, kpt_shape]] # Pose(P3, P4, P5, P6)
diff --git a/yolov8/ultralytics/models/v8/yolov8-pose.yaml b/yolov8/ultralytics/models/v8/yolov8-pose.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..9f48e1ead9b223c9a4fcc6836501c6794d431957
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-pose.yaml
@@ -0,0 +1,47 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8-pose keypoints/pose estimation model. For Usage examples see https://docs.ultralytics.com/tasks/pose
+
+# Parameters
+nc: 1 # number of classes
+kpt_shape: [17, 3] # number of keypoints, number of dims (2 for x,y or 3 for x,y,visible)
+scales: # model compound scaling constants, i.e. 'model=yolov8n-pose.yaml' will call yolov8-pose.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 768]
+ l: [1.00, 1.00, 512]
+ x: [1.00, 1.25, 512]
+
+# YOLOv8.0n backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 9
+
+# YOLOv8.0n head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2f, [512]] # 12
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2f, [256]] # 15 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 12], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2f, [512]] # 18 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 9], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2f, [1024]] # 21 (P5/32-large)
+
+ - [[15, 18, 21], 1, Pose, [nc, kpt_shape]] # Pose(P3, P4, P5)
diff --git a/yolov8/ultralytics/models/v8/yolov8-rtdetr.yaml b/yolov8/ultralytics/models/v8/yolov8-rtdetr.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..a0581068f441617d3d215fb4c380f374e4da94d0
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-rtdetr.yaml
@@ -0,0 +1,46 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8 object detection model with P3-P5 outputs. For Usage examples see https://docs.ultralytics.com/tasks/detect
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n.yaml' will call yolov8.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024] # YOLOv8n summary: 225 layers, 3157200 parameters, 3157184 gradients, 8.9 GFLOPs
+ s: [0.33, 0.50, 1024] # YOLOv8s summary: 225 layers, 11166560 parameters, 11166544 gradients, 28.8 GFLOPs
+ m: [0.67, 0.75, 768] # YOLOv8m summary: 295 layers, 25902640 parameters, 25902624 gradients, 79.3 GFLOPs
+ l: [1.00, 1.00, 512] # YOLOv8l summary: 365 layers, 43691520 parameters, 43691504 gradients, 165.7 GFLOPs
+ x: [1.00, 1.25, 512] # YOLOv8x summary: 365 layers, 68229648 parameters, 68229632 gradients, 258.5 GFLOPs
+
+# YOLOv8.0n backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 9
+
+# YOLOv8.0n head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2f, [512]] # 12
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2f, [256]] # 15 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 12], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2f, [512]] # 18 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 9], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2f, [1024]] # 21 (P5/32-large)
+
+ - [[15, 18, 21], 1, RTDETRDecoder, [nc]] # Detect(P3, P4, P5)
diff --git a/yolov8/ultralytics/models/v8/yolov8-seg.yaml b/yolov8/ultralytics/models/v8/yolov8-seg.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..fbb08fc451307ea99dc7fe99f6edfe2eb40c4daf
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8-seg.yaml
@@ -0,0 +1,46 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8-seg instance segmentation model. For Usage examples see https://docs.ultralytics.com/tasks/segment
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n-seg.yaml' will call yolov8-seg.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024]
+ s: [0.33, 0.50, 1024]
+ m: [0.67, 0.75, 768]
+ l: [1.00, 1.00, 512]
+ x: [1.00, 1.25, 512]
+
+# YOLOv8.0n backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 9
+
+# YOLOv8.0n head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2f, [512]] # 12
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2f, [256]] # 15 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 12], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2f, [512]] # 18 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 9], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2f, [1024]] # 21 (P5/32-large)
+
+ - [[15, 18, 21], 1, Segment, [nc, 32, 256]] # Segment(P3, P4, P5)
diff --git a/yolov8/ultralytics/models/v8/yolov8.yaml b/yolov8/ultralytics/models/v8/yolov8.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..2255450f1715a3c244aed909a350f76f38c04170
--- /dev/null
+++ b/yolov8/ultralytics/models/v8/yolov8.yaml
@@ -0,0 +1,46 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# YOLOv8 object detection model with P3-P5 outputs. For Usage examples see https://docs.ultralytics.com/tasks/detect
+
+# Parameters
+nc: 80 # number of classes
+scales: # model compound scaling constants, i.e. 'model=yolov8n.yaml' will call yolov8.yaml with scale 'n'
+ # [depth, width, max_channels]
+ n: [0.33, 0.25, 1024] # YOLOv8n summary: 225 layers, 3157200 parameters, 3157184 gradients, 8.9 GFLOPs
+ s: [0.33, 0.50, 1024] # YOLOv8s summary: 225 layers, 11166560 parameters, 11166544 gradients, 28.8 GFLOPs
+ m: [0.67, 0.75, 768] # YOLOv8m summary: 295 layers, 25902640 parameters, 25902624 gradients, 79.3 GFLOPs
+ l: [1.00, 1.00, 512] # YOLOv8l summary: 365 layers, 43691520 parameters, 43691504 gradients, 165.7 GFLOPs
+ x: [1.00, 1.25, 512] # YOLOv8x summary: 365 layers, 68229648 parameters, 68229632 gradients, 258.5 GFLOPs
+
+# YOLOv8.0n backbone
+backbone:
+ # [from, repeats, module, args]
+ - [-1, 1, Conv, [64, 3, 2]] # 0-P1/2
+ - [-1, 1, Conv, [128, 3, 2]] # 1-P2/4
+ - [-1, 3, C2f, [128, True]]
+ - [-1, 1, Conv, [256, 3, 2]] # 3-P3/8
+ - [-1, 6, C2f, [256, True]]
+ - [-1, 1, Conv, [512, 3, 2]] # 5-P4/16
+ - [-1, 6, C2f, [512, True]]
+ - [-1, 1, Conv, [1024, 3, 2]] # 7-P5/32
+ - [-1, 3, C2f, [1024, True]]
+ - [-1, 1, SPPF, [1024, 5]] # 9
+
+# YOLOv8.0n head
+head:
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 6], 1, Concat, [1]] # cat backbone P4
+ - [-1, 3, C2f, [512]] # 12
+
+ - [-1, 1, nn.Upsample, [None, 2, 'nearest']]
+ - [[-1, 4], 1, Concat, [1]] # cat backbone P3
+ - [-1, 3, C2f, [256]] # 15 (P3/8-small)
+
+ - [-1, 1, Conv, [256, 3, 2]]
+ - [[-1, 12], 1, Concat, [1]] # cat head P4
+ - [-1, 3, C2f, [512]] # 18 (P4/16-medium)
+
+ - [-1, 1, Conv, [512, 3, 2]]
+ - [[-1, 9], 1, Concat, [1]] # cat head P5
+ - [-1, 3, C2f, [1024]] # 21 (P5/32-large)
+
+ - [[15, 18, 21], 1, Detect, [nc]] # Detect(P3, P4, P5)
diff --git a/yolov8/ultralytics/nn/__init__.py b/yolov8/ultralytics/nn/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..9889b7ef218a106eade7e56f381a66c27c53b7c3
--- /dev/null
+++ b/yolov8/ultralytics/nn/__init__.py
@@ -0,0 +1,9 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .tasks import (BaseModel, ClassificationModel, DetectionModel, SegmentationModel, attempt_load_one_weight,
+ attempt_load_weights, guess_model_scale, guess_model_task, parse_model, torch_safe_load,
+ yaml_model_load)
+
+__all__ = ('attempt_load_one_weight', 'attempt_load_weights', 'parse_model', 'yaml_model_load', 'guess_model_task',
+ 'guess_model_scale', 'torch_safe_load', 'DetectionModel', 'SegmentationModel', 'ClassificationModel',
+ 'BaseModel')
diff --git a/yolov8/ultralytics/nn/autobackend.py b/yolov8/ultralytics/nn/autobackend.py
new file mode 100644
index 0000000000000000000000000000000000000000..e277957a7cfc868ee73b3d241ff5fc963f8ff6df
--- /dev/null
+++ b/yolov8/ultralytics/nn/autobackend.py
@@ -0,0 +1,458 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import ast
+import contextlib
+import json
+import platform
+import zipfile
+from collections import OrderedDict, namedtuple
+from pathlib import Path
+from urllib.parse import urlparse
+
+import cv2
+import numpy as np
+import torch
+import torch.nn as nn
+from PIL import Image
+
+from ultralytics.yolo.utils import LINUX, LOGGER, ROOT, yaml_load
+from ultralytics.yolo.utils.checks import check_requirements, check_suffix, check_version, check_yaml
+from ultralytics.yolo.utils.downloads import attempt_download_asset, is_url
+from ultralytics.yolo.utils.ops import xywh2xyxy
+
+
+def check_class_names(names):
+ """Check class names. Map imagenet class codes to human-readable names if required. Convert lists to dicts."""
+ if isinstance(names, list): # names is a list
+ names = dict(enumerate(names)) # convert to dict
+ if isinstance(names, dict):
+ # Convert 1) string keys to int, i.e. '0' to 0, and non-string values to strings, i.e. True to 'True'
+ names = {int(k): str(v) for k, v in names.items()}
+ n = len(names)
+ if max(names.keys()) >= n:
+ raise KeyError(f'{n}-class dataset requires class indices 0-{n - 1}, but you have invalid class indices '
+ f'{min(names.keys())}-{max(names.keys())} defined in your dataset YAML.')
+ if isinstance(names[0], str) and names[0].startswith('n0'): # imagenet class codes, i.e. 'n01440764'
+ map = yaml_load(ROOT / 'datasets/ImageNet.yaml')['map'] # human-readable names
+ names = {k: map[v] for k, v in names.items()}
+ return names
+
+
+class AutoBackend(nn.Module):
+
+ def __init__(self,
+ weights='yolov8n.pt',
+ device=torch.device('cpu'),
+ dnn=False,
+ data=None,
+ fp16=False,
+ fuse=True,
+ verbose=True):
+ """
+ MultiBackend class for python inference on various platforms using Ultralytics YOLO.
+
+ Args:
+ weights (str): The path to the weights file. Default: 'yolov8n.pt'
+ device (torch.device): The device to run the model on.
+ dnn (bool): Use OpenCV DNN module for inference if True, defaults to False.
+ data (str | Path | optional): Additional data.yaml file for class names.
+ fp16 (bool): If True, use half precision. Default: False
+ fuse (bool): Whether to fuse the model or not. Default: True
+ verbose (bool): Whether to run in verbose mode or not. Default: True
+
+ Supported formats and their naming conventions:
+ | Format | Suffix |
+ |-----------------------|------------------|
+ | PyTorch | *.pt |
+ | TorchScript | *.torchscript |
+ | ONNX Runtime | *.onnx |
+ | ONNX OpenCV DNN | *.onnx dnn=True |
+ | OpenVINO | *.xml |
+ | CoreML | *.mlmodel |
+ | TensorRT | *.engine |
+ | TensorFlow SavedModel | *_saved_model |
+ | TensorFlow GraphDef | *.pb |
+ | TensorFlow Lite | *.tflite |
+ | TensorFlow Edge TPU | *_edgetpu.tflite |
+ | PaddlePaddle | *_paddle_model |
+ """
+ super().__init__()
+ w = str(weights[0] if isinstance(weights, list) else weights)
+ nn_module = isinstance(weights, torch.nn.Module)
+ pt, jit, onnx, xml, engine, coreml, saved_model, pb, tflite, edgetpu, tfjs, paddle, ncnn, triton = \
+ self._model_type(w)
+ fp16 &= pt or jit or onnx or engine or nn_module or triton # FP16
+ nhwc = coreml or saved_model or pb or tflite or edgetpu # BHWC formats (vs torch BCWH)
+ stride = 32 # default stride
+ model, metadata = None, None
+ cuda = torch.cuda.is_available() and device.type != 'cpu' # use CUDA
+ if not (pt or triton or nn_module):
+ w = attempt_download_asset(w) # download if not local
+
+ # NOTE: special case: in-memory pytorch model
+ if nn_module:
+ model = weights.to(device)
+ model = model.fuse(verbose=verbose) if fuse else model
+ if hasattr(model, 'kpt_shape'):
+ kpt_shape = model.kpt_shape # pose-only
+ stride = max(int(model.stride.max()), 32) # model stride
+ names = model.module.names if hasattr(model, 'module') else model.names # get class names
+ model.half() if fp16 else model.float()
+ self.model = model # explicitly assign for to(), cpu(), cuda(), half()
+ pt = True
+ elif pt: # PyTorch
+ from ultralytics.nn.tasks import attempt_load_weights
+ model = attempt_load_weights(weights if isinstance(weights, list) else w,
+ device=device,
+ inplace=True,
+ fuse=fuse)
+ if hasattr(model, 'kpt_shape'):
+ kpt_shape = model.kpt_shape # pose-only
+ stride = max(int(model.stride.max()), 32) # model stride
+ names = model.module.names if hasattr(model, 'module') else model.names # get class names
+ model.half() if fp16 else model.float()
+ self.model = model # explicitly assign for to(), cpu(), cuda(), half()
+ elif jit: # TorchScript
+ LOGGER.info(f'Loading {w} for TorchScript inference...')
+ extra_files = {'config.txt': ''} # model metadata
+ model = torch.jit.load(w, _extra_files=extra_files, map_location=device)
+ model.half() if fp16 else model.float()
+ if extra_files['config.txt']: # load metadata dict
+ metadata = json.loads(extra_files['config.txt'], object_hook=lambda x: dict(x.items()))
+ elif dnn: # ONNX OpenCV DNN
+ LOGGER.info(f'Loading {w} for ONNX OpenCV DNN inference...')
+ check_requirements('opencv-python>=4.5.4')
+ net = cv2.dnn.readNetFromONNX(w)
+ elif onnx: # ONNX Runtime
+ LOGGER.info(f'Loading {w} for ONNX Runtime inference...')
+ check_requirements(('onnx', 'onnxruntime-gpu' if cuda else 'onnxruntime'))
+ import onnxruntime
+ providers = ['CUDAExecutionProvider', 'CPUExecutionProvider'] if cuda else ['CPUExecutionProvider']
+ session = onnxruntime.InferenceSession(w, providers=providers)
+ output_names = [x.name for x in session.get_outputs()]
+ metadata = session.get_modelmeta().custom_metadata_map # metadata
+ elif xml: # OpenVINO
+ LOGGER.info(f'Loading {w} for OpenVINO inference...')
+ check_requirements('openvino') # requires openvino-dev: https://pypi.org/project/openvino-dev/
+ from openvino.runtime import Core, Layout, get_batch # noqa
+ ie = Core()
+ w = Path(w)
+ if not w.is_file(): # if not *.xml
+ w = next(w.glob('*.xml')) # get *.xml file from *_openvino_model dir
+ network = ie.read_model(model=str(w), weights=w.with_suffix('.bin'))
+ if network.get_parameters()[0].get_layout().empty:
+ network.get_parameters()[0].set_layout(Layout('NCHW'))
+ batch_dim = get_batch(network)
+ if batch_dim.is_static:
+ batch_size = batch_dim.get_length()
+ executable_network = ie.compile_model(network, device_name='CPU') # device_name="MYRIAD" for NCS2
+ metadata = w.parent / 'metadata.yaml'
+ elif engine: # TensorRT
+ LOGGER.info(f'Loading {w} for TensorRT inference...')
+ try:
+ import tensorrt as trt # noqa https://developer.nvidia.com/nvidia-tensorrt-download
+ except ImportError:
+ if LINUX:
+ check_requirements('nvidia-tensorrt', cmds='-U --index-url https://pypi.ngc.nvidia.com')
+ import tensorrt as trt # noqa
+ check_version(trt.__version__, '7.0.0', hard=True) # require tensorrt>=7.0.0
+ if device.type == 'cpu':
+ device = torch.device('cuda:0')
+ Binding = namedtuple('Binding', ('name', 'dtype', 'shape', 'data', 'ptr'))
+ logger = trt.Logger(trt.Logger.INFO)
+ # Read file
+ with open(w, 'rb') as f, trt.Runtime(logger) as runtime:
+ meta_len = int.from_bytes(f.read(4), byteorder='little') # read metadata length
+ metadata = json.loads(f.read(meta_len).decode('utf-8')) # read metadata
+ model = runtime.deserialize_cuda_engine(f.read()) # read engine
+ context = model.create_execution_context()
+ bindings = OrderedDict()
+ output_names = []
+ fp16 = False # default updated below
+ dynamic = False
+ for i in range(model.num_bindings):
+ name = model.get_binding_name(i)
+ dtype = trt.nptype(model.get_binding_dtype(i))
+ if model.binding_is_input(i):
+ if -1 in tuple(model.get_binding_shape(i)): # dynamic
+ dynamic = True
+ context.set_binding_shape(i, tuple(model.get_profile_shape(0, i)[2]))
+ if dtype == np.float16:
+ fp16 = True
+ else: # output
+ output_names.append(name)
+ shape = tuple(context.get_binding_shape(i))
+ im = torch.from_numpy(np.empty(shape, dtype=dtype)).to(device)
+ bindings[name] = Binding(name, dtype, shape, im, int(im.data_ptr()))
+ binding_addrs = OrderedDict((n, d.ptr) for n, d in bindings.items())
+ batch_size = bindings['images'].shape[0] # if dynamic, this is instead max batch size
+ elif coreml: # CoreML
+ LOGGER.info(f'Loading {w} for CoreML inference...')
+ import coremltools as ct
+ model = ct.models.MLModel(w)
+ metadata = dict(model.user_defined_metadata)
+ elif saved_model: # TF SavedModel
+ LOGGER.info(f'Loading {w} for TensorFlow SavedModel inference...')
+ import tensorflow as tf
+ keras = False # assume TF1 saved_model
+ model = tf.keras.models.load_model(w) if keras else tf.saved_model.load(w)
+ metadata = Path(w) / 'metadata.yaml'
+ elif pb: # GraphDef https://www.tensorflow.org/guide/migrate#a_graphpb_or_graphpbtxt
+ LOGGER.info(f'Loading {w} for TensorFlow GraphDef inference...')
+ import tensorflow as tf
+
+ from ultralytics.yolo.engine.exporter import gd_outputs
+
+ def wrap_frozen_graph(gd, inputs, outputs):
+ """Wrap frozen graphs for deployment."""
+ x = tf.compat.v1.wrap_function(lambda: tf.compat.v1.import_graph_def(gd, name=''), []) # wrapped
+ ge = x.graph.as_graph_element
+ return x.prune(tf.nest.map_structure(ge, inputs), tf.nest.map_structure(ge, outputs))
+
+ gd = tf.Graph().as_graph_def() # TF GraphDef
+ with open(w, 'rb') as f:
+ gd.ParseFromString(f.read())
+ frozen_func = wrap_frozen_graph(gd, inputs='x:0', outputs=gd_outputs(gd))
+ elif tflite or edgetpu: # https://www.tensorflow.org/lite/guide/python#install_tensorflow_lite_for_python
+ try: # https://coral.ai/docs/edgetpu/tflite-python/#update-existing-tf-lite-code-for-the-edge-tpu
+ from tflite_runtime.interpreter import Interpreter, load_delegate
+ except ImportError:
+ import tensorflow as tf
+ Interpreter, load_delegate = tf.lite.Interpreter, tf.lite.experimental.load_delegate
+ if edgetpu: # TF Edge TPU https://coral.ai/software/#edgetpu-runtime
+ LOGGER.info(f'Loading {w} for TensorFlow Lite Edge TPU inference...')
+ delegate = {
+ 'Linux': 'libedgetpu.so.1',
+ 'Darwin': 'libedgetpu.1.dylib',
+ 'Windows': 'edgetpu.dll'}[platform.system()]
+ interpreter = Interpreter(model_path=w, experimental_delegates=[load_delegate(delegate)])
+ else: # TFLite
+ LOGGER.info(f'Loading {w} for TensorFlow Lite inference...')
+ interpreter = Interpreter(model_path=w) # load TFLite model
+ interpreter.allocate_tensors() # allocate
+ input_details = interpreter.get_input_details() # inputs
+ output_details = interpreter.get_output_details() # outputs
+ # Load metadata
+ with contextlib.suppress(zipfile.BadZipFile):
+ with zipfile.ZipFile(w, 'r') as model:
+ meta_file = model.namelist()[0]
+ metadata = ast.literal_eval(model.read(meta_file).decode('utf-8'))
+ elif tfjs: # TF.js
+ raise NotImplementedError('YOLOv8 TF.js inference is not currently supported.')
+ elif paddle: # PaddlePaddle
+ LOGGER.info(f'Loading {w} for PaddlePaddle inference...')
+ check_requirements('paddlepaddle-gpu' if cuda else 'paddlepaddle')
+ import paddle.inference as pdi # noqa
+ w = Path(w)
+ if not w.is_file(): # if not *.pdmodel
+ w = next(w.rglob('*.pdmodel')) # get *.pdmodel file from *_paddle_model dir
+ config = pdi.Config(str(w), str(w.with_suffix('.pdiparams')))
+ if cuda:
+ config.enable_use_gpu(memory_pool_init_size_mb=2048, device_id=0)
+ predictor = pdi.create_predictor(config)
+ input_handle = predictor.get_input_handle(predictor.get_input_names()[0])
+ output_names = predictor.get_output_names()
+ metadata = w.parents[1] / 'metadata.yaml'
+ elif ncnn: # PaddlePaddle
+ raise NotImplementedError('YOLOv8 NCNN inference is not currently supported.')
+ elif triton: # NVIDIA Triton Inference Server
+ LOGGER.info('Triton Inference Server not supported...')
+ '''
+ TODO:
+ check_requirements('tritonclient[all]')
+ from utils.triton import TritonRemoteModel
+ model = TritonRemoteModel(url=w)
+ nhwc = model.runtime.startswith("tensorflow")
+ '''
+ else:
+ from ultralytics.yolo.engine.exporter import export_formats
+ raise TypeError(f"model='{w}' is not a supported model format. "
+ 'See https://docs.ultralytics.com/modes/predict for help.'
+ f'\n\n{export_formats()}')
+
+ # Load external metadata YAML
+ if isinstance(metadata, (str, Path)) and Path(metadata).exists():
+ metadata = yaml_load(metadata)
+ if metadata:
+ for k, v in metadata.items():
+ if k in ('stride', 'batch'):
+ metadata[k] = int(v)
+ elif k in ('imgsz', 'names', 'kpt_shape') and isinstance(v, str):
+ metadata[k] = eval(v)
+ stride = metadata['stride']
+ task = metadata['task']
+ batch = metadata['batch']
+ imgsz = metadata['imgsz']
+ names = metadata['names']
+ kpt_shape = metadata.get('kpt_shape')
+ elif not (pt or triton or nn_module):
+ LOGGER.warning(f"WARNING ⚠️ Metadata not found for 'model={weights}'")
+
+ # Check names
+ if 'names' not in locals(): # names missing
+ names = self._apply_default_class_names(data)
+ names = check_class_names(names)
+
+ self.__dict__.update(locals()) # assign all variables to self
+
+ def forward(self, im, augment=False, visualize=False):
+ """
+ Runs inference on the YOLOv8 MultiBackend model.
+
+ Args:
+ im (torch.Tensor): The image tensor to perform inference on.
+ augment (bool): whether to perform data augmentation during inference, defaults to False
+ visualize (bool): whether to visualize the output predictions, defaults to False
+
+ Returns:
+ (tuple): Tuple containing the raw output tensor, and processed output for visualization (if visualize=True)
+ """
+ b, ch, h, w = im.shape # batch, channel, height, width
+ if self.fp16 and im.dtype != torch.float16:
+ im = im.half() # to FP16
+ if self.nhwc:
+ im = im.permute(0, 2, 3, 1) # torch BCHW to numpy BHWC shape(1,320,192,3)
+
+ if self.pt or self.nn_module: # PyTorch
+ y = self.model(im, augment=augment, visualize=visualize) if augment or visualize else self.model(im)
+ elif self.jit: # TorchScript
+ y = self.model(im)
+ elif self.dnn: # ONNX OpenCV DNN
+ im = im.cpu().numpy() # torch to numpy
+ self.net.setInput(im)
+ y = self.net.forward()
+ elif self.onnx: # ONNX Runtime
+ im = im.cpu().numpy() # torch to numpy
+ y = self.session.run(self.output_names, {self.session.get_inputs()[0].name: im})
+ elif self.xml: # OpenVINO
+ im = im.cpu().numpy() # FP32
+ y = list(self.executable_network([im]).values())
+ elif self.engine: # TensorRT
+ if self.dynamic and im.shape != self.bindings['images'].shape:
+ i = self.model.get_binding_index('images')
+ self.context.set_binding_shape(i, im.shape) # reshape if dynamic
+ self.bindings['images'] = self.bindings['images']._replace(shape=im.shape)
+ for name in self.output_names:
+ i = self.model.get_binding_index(name)
+ self.bindings[name].data.resize_(tuple(self.context.get_binding_shape(i)))
+ s = self.bindings['images'].shape
+ assert im.shape == s, f"input size {im.shape} {'>' if self.dynamic else 'not equal to'} max model size {s}"
+ self.binding_addrs['images'] = int(im.data_ptr())
+ self.context.execute_v2(list(self.binding_addrs.values()))
+ y = [self.bindings[x].data for x in sorted(self.output_names)]
+ elif self.coreml: # CoreML
+ im = im[0].cpu().numpy()
+ im_pil = Image.fromarray((im * 255).astype('uint8'))
+ # im = im.resize((192, 320), Image.BILINEAR)
+ y = self.model.predict({'image': im_pil}) # coordinates are xywh normalized
+ if 'confidence' in y:
+ box = xywh2xyxy(y['coordinates'] * [[w, h, w, h]]) # xyxy pixels
+ conf, cls = y['confidence'].max(1), y['confidence'].argmax(1).astype(np.float)
+ y = np.concatenate((box, conf.reshape(-1, 1), cls.reshape(-1, 1)), 1)
+ elif len(y) == 1: # classification model
+ y = list(y.values())
+ elif len(y) == 2: # segmentation model
+ y = list(reversed(y.values())) # reversed for segmentation models (pred, proto)
+ elif self.paddle: # PaddlePaddle
+ im = im.cpu().numpy().astype(np.float32)
+ self.input_handle.copy_from_cpu(im)
+ self.predictor.run()
+ y = [self.predictor.get_output_handle(x).copy_to_cpu() for x in self.output_names]
+ elif self.triton: # NVIDIA Triton Inference Server
+ y = self.model(im)
+ else: # TensorFlow (SavedModel, GraphDef, Lite, Edge TPU)
+ im = im.cpu().numpy()
+ if self.saved_model: # SavedModel
+ y = self.model(im, training=False) if self.keras else self.model(im)
+ if not isinstance(y, list):
+ y = [y]
+ elif self.pb: # GraphDef
+ y = self.frozen_func(x=self.tf.constant(im))
+ if len(y) == 2 and len(self.names) == 999: # segments and names not defined
+ ip, ib = (0, 1) if len(y[0].shape) == 4 else (1, 0) # index of protos, boxes
+ nc = y[ib].shape[1] - y[ip].shape[3] - 4 # y = (1, 160, 160, 32), (1, 116, 8400)
+ self.names = {i: f'class{i}' for i in range(nc)}
+ else: # Lite or Edge TPU
+ input = self.input_details[0]
+ int8 = input['dtype'] == np.int8 # is TFLite quantized int8 model
+ if int8:
+ scale, zero_point = input['quantization']
+ im = (im / scale + zero_point).astype(np.int8) # de-scale
+ self.interpreter.set_tensor(input['index'], im)
+ self.interpreter.invoke()
+ y = []
+ for output in self.output_details:
+ x = self.interpreter.get_tensor(output['index'])
+ if int8:
+ scale, zero_point = output['quantization']
+ x = (x.astype(np.float32) - zero_point) * scale # re-scale
+ y.append(x)
+ # TF segment fixes: export is reversed vs ONNX export and protos are transposed
+ if len(y) == 2: # segment with (det, proto) output order reversed
+ if len(y[1].shape) != 4:
+ y = list(reversed(y)) # should be y = (1, 116, 8400), (1, 160, 160, 32)
+ y[1] = np.transpose(y[1], (0, 3, 1, 2)) # should be y = (1, 116, 8400), (1, 32, 160, 160)
+ y = [x if isinstance(x, np.ndarray) else x.numpy() for x in y]
+ # y[0][..., :4] *= [w, h, w, h] # xywh normalized to pixels
+
+ # for x in y:
+ # print(type(x), len(x)) if isinstance(x, (list, tuple)) else print(type(x), x.shape) # debug shapes
+ if isinstance(y, (list, tuple)):
+ return self.from_numpy(y[0]) if len(y) == 1 else [self.from_numpy(x) for x in y]
+ else:
+ return self.from_numpy(y)
+
+ def from_numpy(self, x):
+ """
+ Convert a numpy array to a tensor.
+
+ Args:
+ x (np.ndarray): The array to be converted.
+
+ Returns:
+ (torch.Tensor): The converted tensor
+ """
+ return torch.tensor(x).to(self.device) if isinstance(x, np.ndarray) else x
+
+ def warmup(self, imgsz=(1, 3, 640, 640)):
+ """
+ Warm up the model by running one forward pass with a dummy input.
+
+ Args:
+ imgsz (tuple): The shape of the dummy input tensor in the format (batch_size, channels, height, width)
+
+ Returns:
+ (None): This method runs the forward pass and don't return any value
+ """
+ warmup_types = self.pt, self.jit, self.onnx, self.engine, self.saved_model, self.pb, self.triton, self.nn_module
+ if any(warmup_types) and (self.device.type != 'cpu' or self.triton):
+ im = torch.empty(*imgsz, dtype=torch.half if self.fp16 else torch.float, device=self.device) # input
+ for _ in range(2 if self.jit else 1): #
+ self.forward(im) # warmup
+
+ @staticmethod
+ def _apply_default_class_names(data):
+ """Applies default class names to an input YAML file or returns numerical class names."""
+ with contextlib.suppress(Exception):
+ return yaml_load(check_yaml(data))['names']
+ return {i: f'class{i}' for i in range(999)} # return default if above errors
+
+ @staticmethod
+ def _model_type(p='path/to/model.pt'):
+ """
+ This function takes a path to a model file and returns the model type
+
+ Args:
+ p: path to the model file. Defaults to path/to/model.pt
+ """
+ # Return model type from model path, i.e. path='path/to/model.onnx' -> type=onnx
+ # types = [pt, jit, onnx, xml, engine, coreml, saved_model, pb, tflite, edgetpu, tfjs, paddle]
+ from ultralytics.yolo.engine.exporter import export_formats
+ sf = list(export_formats().Suffix) # export suffixes
+ if not is_url(p, check=False) and not isinstance(p, str):
+ check_suffix(p, sf) # checks
+ url = urlparse(p) # if url may be Triton inference server
+ types = [s in Path(p).name for s in sf]
+ types[8] &= not types[9] # tflite &= not edgetpu
+ triton = not any(types) and all([any(s in url.scheme for s in ['http', 'grpc']), url.netloc])
+ return types + [triton]
diff --git a/yolov8/ultralytics/nn/autoshape.py b/yolov8/ultralytics/nn/autoshape.py
new file mode 100644
index 0000000000000000000000000000000000000000..d557f78061ed9e7152e010a96617ccd3409e9511
--- /dev/null
+++ b/yolov8/ultralytics/nn/autoshape.py
@@ -0,0 +1,244 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Common modules
+"""
+
+from copy import copy
+from pathlib import Path
+
+import cv2
+import numpy as np
+import requests
+import torch
+import torch.nn as nn
+from PIL import Image, ImageOps
+from torch.cuda import amp
+
+from ultralytics.nn.autobackend import AutoBackend
+from ultralytics.yolo.data.augment import LetterBox
+from ultralytics.yolo.utils import LOGGER, colorstr
+from ultralytics.yolo.utils.files import increment_path
+from ultralytics.yolo.utils.ops import Profile, make_divisible, non_max_suppression, scale_boxes, xyxy2xywh
+from ultralytics.yolo.utils.plotting import Annotator, colors, save_one_box
+from ultralytics.yolo.utils.torch_utils import copy_attr, smart_inference_mode
+
+
+class AutoShape(nn.Module):
+ """YOLOv8 input-robust model wrapper for passing cv2/np/PIL/torch inputs. Includes preprocessing, inference and NMS."""
+ conf = 0.25 # NMS confidence threshold
+ iou = 0.45 # NMS IoU threshold
+ agnostic = False # NMS class-agnostic
+ multi_label = False # NMS multiple labels per box
+ classes = None # (optional list) filter by class, i.e. = [0, 15, 16] for COCO persons, cats and dogs
+ max_det = 1000 # maximum number of detections per image
+ amp = False # Automatic Mixed Precision (AMP) inference
+
+ def __init__(self, model, verbose=True):
+ """Initializes object and copies attributes from model object."""
+ super().__init__()
+ if verbose:
+ LOGGER.info('Adding AutoShape... ')
+ copy_attr(self, model, include=('yaml', 'nc', 'hyp', 'names', 'stride', 'abc'), exclude=()) # copy attributes
+ self.dmb = isinstance(model, AutoBackend) # DetectMultiBackend() instance
+ self.pt = not self.dmb or model.pt # PyTorch model
+ self.model = model.eval()
+ if self.pt:
+ m = self.model.model.model[-1] if self.dmb else self.model.model[-1] # Detect()
+ m.inplace = False # Detect.inplace=False for safe multithread inference
+ m.export = True # do not output loss values
+
+ def _apply(self, fn):
+ """Apply to(), cpu(), cuda(), half() to model tensors that are not parameters or registered buffers."""
+ self = super()._apply(fn)
+ if self.pt:
+ m = self.model.model.model[-1] if self.dmb else self.model.model[-1] # Detect()
+ m.stride = fn(m.stride)
+ m.grid = list(map(fn, m.grid))
+ if isinstance(m.anchor_grid, list):
+ m.anchor_grid = list(map(fn, m.anchor_grid))
+ return self
+
+ @smart_inference_mode()
+ def forward(self, ims, size=640, augment=False, profile=False):
+ """Inference from various sources. For size(height=640, width=1280), RGB images example inputs are:."""
+ # file: ims = 'data/images/zidane.jpg' # str or PosixPath
+ # URI: = 'https://ultralytics.com/images/zidane.jpg'
+ # OpenCV: = cv2.imread('image.jpg')[:,:,::-1] # HWC BGR to RGB x(640,1280,3)
+ # PIL: = Image.open('image.jpg') or ImageGrab.grab() # HWC x(640,1280,3)
+ # numpy: = np.zeros((640,1280,3)) # HWC
+ # torch: = torch.zeros(16,3,320,640) # BCHW (scaled to size=640, 0-1 values)
+ # multiple: = [Image.open('image1.jpg'), Image.open('image2.jpg'), ...] # list of images
+
+ dt = (Profile(), Profile(), Profile())
+ with dt[0]:
+ if isinstance(size, int): # expand
+ size = (size, size)
+ p = next(self.model.parameters()) if self.pt else torch.empty(1, device=self.model.device) # param
+ autocast = self.amp and (p.device.type != 'cpu') # Automatic Mixed Precision (AMP) inference
+ if isinstance(ims, torch.Tensor): # torch
+ with amp.autocast(autocast):
+ return self.model(ims.to(p.device).type_as(p), augment=augment) # inference
+
+ # Preprocess
+ n, ims = (len(ims), list(ims)) if isinstance(ims, (list, tuple)) else (1, [ims]) # number, list of images
+ shape0, shape1, files = [], [], [] # image and inference shapes, filenames
+ for i, im in enumerate(ims):
+ f = f'image{i}' # filename
+ if isinstance(im, (str, Path)): # filename or uri
+ im, f = Image.open(requests.get(im, stream=True).raw if str(im).startswith('http') else im), im
+ im = np.asarray(ImageOps.exif_transpose(im))
+ elif isinstance(im, Image.Image): # PIL Image
+ im, f = np.asarray(ImageOps.exif_transpose(im)), getattr(im, 'filename', f) or f
+ files.append(Path(f).with_suffix('.jpg').name)
+ if im.shape[0] < 5: # image in CHW
+ im = im.transpose((1, 2, 0)) # reverse dataloader .transpose(2, 0, 1)
+ im = im[..., :3] if im.ndim == 3 else cv2.cvtColor(im, cv2.COLOR_GRAY2BGR) # enforce 3ch input
+ s = im.shape[:2] # HWC
+ shape0.append(s) # image shape
+ g = max(size) / max(s) # gain
+ shape1.append([y * g for y in s])
+ ims[i] = im if im.data.contiguous else np.ascontiguousarray(im) # update
+ shape1 = [make_divisible(x, self.stride) for x in np.array(shape1).max(0)] if self.pt else size # inf shape
+ x = [LetterBox(shape1, auto=False)(image=im)['img'] for im in ims] # pad
+ x = np.ascontiguousarray(np.array(x).transpose((0, 3, 1, 2))) # stack and BHWC to BCHW
+ x = torch.from_numpy(x).to(p.device).type_as(p) / 255 # uint8 to fp16/32
+
+ with amp.autocast(autocast):
+ # Inference
+ with dt[1]:
+ y = self.model(x, augment=augment) # forward
+
+ # Postprocess
+ with dt[2]:
+ y = non_max_suppression(y if self.dmb else y[0],
+ self.conf,
+ self.iou,
+ self.classes,
+ self.agnostic,
+ self.multi_label,
+ max_det=self.max_det) # NMS
+ for i in range(n):
+ scale_boxes(shape1, y[i][:, :4], shape0[i])
+
+ return Detections(ims, y, files, dt, self.names, x.shape)
+
+
+class Detections:
+ """ YOLOv8 detections class for inference results"""
+
+ def __init__(self, ims, pred, files, times=(0, 0, 0), names=None, shape=None):
+ """Initialize object attributes for YOLO detection results."""
+ super().__init__()
+ d = pred[0].device # device
+ gn = [torch.tensor([*(im.shape[i] for i in [1, 0, 1, 0]), 1, 1], device=d) for im in ims] # normalizations
+ self.ims = ims # list of images as numpy arrays
+ self.pred = pred # list of tensors pred[0] = (xyxy, conf, cls)
+ self.names = names # class names
+ self.files = files # image filenames
+ self.times = times # profiling times
+ self.xyxy = pred # xyxy pixels
+ self.xywh = [xyxy2xywh(x) for x in pred] # xywh pixels
+ self.xyxyn = [x / g for x, g in zip(self.xyxy, gn)] # xyxy normalized
+ self.xywhn = [x / g for x, g in zip(self.xywh, gn)] # xywh normalized
+ self.n = len(self.pred) # number of images (batch size)
+ self.t = tuple(x.t / self.n * 1E3 for x in times) # timestamps (ms)
+ self.s = tuple(shape) # inference BCHW shape
+
+ def _run(self, pprint=False, show=False, save=False, crop=False, render=False, labels=True, save_dir=Path('')):
+ """Return performance metrics and optionally cropped/save images or results."""
+ s, crops = '', []
+ for i, (im, pred) in enumerate(zip(self.ims, self.pred)):
+ s += f'\nimage {i + 1}/{len(self.pred)}: {im.shape[0]}x{im.shape[1]} ' # string
+ if pred.shape[0]:
+ for c in pred[:, -1].unique():
+ n = (pred[:, -1] == c).sum() # detections per class
+ s += f"{n} {self.names[int(c)]}{'s' * (n > 1)}, " # add to string
+ s = s.rstrip(', ')
+ if show or save or render or crop:
+ annotator = Annotator(im, example=str(self.names))
+ for *box, conf, cls in reversed(pred): # xyxy, confidence, class
+ label = f'{self.names[int(cls)]} {conf:.2f}'
+ if crop:
+ file = save_dir / 'crops' / self.names[int(cls)] / self.files[i] if save else None
+ crops.append({
+ 'box': box,
+ 'conf': conf,
+ 'cls': cls,
+ 'label': label,
+ 'im': save_one_box(box, im, file=file, save=save)})
+ else: # all others
+ annotator.box_label(box, label if labels else '', color=colors(cls))
+ im = annotator.im
+ else:
+ s += '(no detections)'
+
+ im = Image.fromarray(im.astype(np.uint8)) if isinstance(im, np.ndarray) else im # from np
+ if show:
+ im.show(self.files[i]) # show
+ if save:
+ f = self.files[i]
+ im.save(save_dir / f) # save
+ if i == self.n - 1:
+ LOGGER.info(f"Saved {self.n} image{'s' * (self.n > 1)} to {colorstr('bold', save_dir)}")
+ if render:
+ self.ims[i] = np.asarray(im)
+ if pprint:
+ s = s.lstrip('\n')
+ return f'{s}\nSpeed: %.1fms preprocess, %.1fms inference, %.1fms NMS per image at shape {self.s}' % self.t
+ if crop:
+ if save:
+ LOGGER.info(f'Saved results to {save_dir}\n')
+ return crops
+
+ def show(self, labels=True):
+ """Displays YOLO results with detected bounding boxes."""
+ self._run(show=True, labels=labels) # show results
+
+ def save(self, labels=True, save_dir='runs/detect/exp', exist_ok=False):
+ """Save detection results with optional labels to specified directory."""
+ save_dir = increment_path(save_dir, exist_ok, mkdir=True) # increment save_dir
+ self._run(save=True, labels=labels, save_dir=save_dir) # save results
+
+ def crop(self, save=True, save_dir='runs/detect/exp', exist_ok=False):
+ """Crops images into detections and saves them if 'save' is True."""
+ save_dir = increment_path(save_dir, exist_ok, mkdir=True) if save else None
+ return self._run(crop=True, save=save, save_dir=save_dir) # crop results
+
+ def render(self, labels=True):
+ """Renders detected objects and returns images."""
+ self._run(render=True, labels=labels) # render results
+ return self.ims
+
+ def pandas(self):
+ """Return detections as pandas DataFrames, i.e. print(results.pandas().xyxy[0])."""
+ import pandas
+ new = copy(self) # return copy
+ ca = 'xmin', 'ymin', 'xmax', 'ymax', 'confidence', 'class', 'name' # xyxy columns
+ cb = 'xcenter', 'ycenter', 'width', 'height', 'confidence', 'class', 'name' # xywh columns
+ for k, c in zip(['xyxy', 'xyxyn', 'xywh', 'xywhn'], [ca, ca, cb, cb]):
+ a = [[x[:5] + [int(x[5]), self.names[int(x[5])]] for x in x.tolist()] for x in getattr(self, k)] # update
+ setattr(new, k, [pandas.DataFrame(x, columns=c) for x in a])
+ return new
+
+ def tolist(self):
+ """Return a list of Detections objects, i.e. 'for result in results.tolist():'."""
+ r = range(self.n) # iterable
+ x = [Detections([self.ims[i]], [self.pred[i]], [self.files[i]], self.times, self.names, self.s) for i in r]
+ # for d in x:
+ # for k in ['ims', 'pred', 'xyxy', 'xyxyn', 'xywh', 'xywhn']:
+ # setattr(d, k, getattr(d, k)[0]) # pop out of list
+ return x
+
+ def print(self):
+ """Print the results of the `self._run()` function."""
+ LOGGER.info(self.__str__())
+
+ def __len__(self): # override len(results)
+ return self.n
+
+ def __str__(self): # override print(results)
+ return self._run(pprint=True) # print results
+
+ def __repr__(self):
+ """Returns a printable representation of the object."""
+ return f'YOLOv8 {self.__class__} instance\n' + self.__str__()
diff --git a/yolov8/ultralytics/nn/modules/__init__.py b/yolov8/ultralytics/nn/modules/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..b6dc6c4423631e70146cf1db223e03a3873dec5c
--- /dev/null
+++ b/yolov8/ultralytics/nn/modules/__init__.py
@@ -0,0 +1,29 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Ultralytics modules. Visualize with:
+
+from ultralytics.nn.modules import *
+import torch
+import os
+
+x = torch.ones(1, 128, 40, 40)
+m = Conv(128, 128)
+f = f'{m._get_name()}.onnx'
+torch.onnx.export(m, x, f)
+os.system(f'onnxsim {f} {f} && open {f}')
+"""
+
+from .block import (C1, C2, C3, C3TR, DFL, SPP, SPPF, Bottleneck, BottleneckCSP, C2f, C3Ghost, C3x, GhostBottleneck,
+ HGBlock, HGStem, Proto, RepC3)
+from .conv import (CBAM, ChannelAttention, Concat, Conv, Conv2, ConvTranspose, DWConv, DWConvTranspose2d, Focus,
+ GhostConv, LightConv, RepConv, SpatialAttention)
+from .head import Classify, Detect, Pose, RTDETRDecoder, Segment
+from .transformer import (AIFI, MLP, DeformableTransformerDecoder, DeformableTransformerDecoderLayer, LayerNorm2d,
+ MLPBlock, MSDeformAttn, TransformerBlock, TransformerEncoderLayer, TransformerLayer)
+
+__all__ = ('Conv', 'Conv2', 'LightConv', 'RepConv', 'DWConv', 'DWConvTranspose2d', 'ConvTranspose', 'Focus',
+ 'GhostConv', 'ChannelAttention', 'SpatialAttention', 'CBAM', 'Concat', 'TransformerLayer',
+ 'TransformerBlock', 'MLPBlock', 'LayerNorm2d', 'DFL', 'HGBlock', 'HGStem', 'SPP', 'SPPF', 'C1', 'C2', 'C3',
+ 'C2f', 'C3x', 'C3TR', 'C3Ghost', 'GhostBottleneck', 'Bottleneck', 'BottleneckCSP', 'Proto', 'Detect',
+ 'Segment', 'Pose', 'Classify', 'TransformerEncoderLayer', 'RepC3', 'RTDETRDecoder', 'AIFI',
+ 'DeformableTransformerDecoder', 'DeformableTransformerDecoderLayer', 'MSDeformAttn', 'MLP')
diff --git a/yolov8/ultralytics/nn/modules/block.py b/yolov8/ultralytics/nn/modules/block.py
new file mode 100644
index 0000000000000000000000000000000000000000..508ddb3cb7ee68d6110ac35d7a9c698c2feabb8c
--- /dev/null
+++ b/yolov8/ultralytics/nn/modules/block.py
@@ -0,0 +1,304 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Block modules
+"""
+
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+
+from .conv import Conv, DWConv, GhostConv, LightConv, RepConv
+from .transformer import TransformerBlock
+
+__all__ = ('DFL', 'HGBlock', 'HGStem', 'SPP', 'SPPF', 'C1', 'C2', 'C3', 'C2f', 'C3x', 'C3TR', 'C3Ghost',
+ 'GhostBottleneck', 'Bottleneck', 'BottleneckCSP', 'Proto', 'RepC3')
+
+
+class DFL(nn.Module):
+ """
+ Integral module of Distribution Focal Loss (DFL).
+ Proposed in Generalized Focal Loss https://ieeexplore.ieee.org/document/9792391
+ """
+
+ def __init__(self, c1=16):
+ """Initialize a convolutional layer with a given number of input channels."""
+ super().__init__()
+ self.conv = nn.Conv2d(c1, 1, 1, bias=False).requires_grad_(False)
+ x = torch.arange(c1, dtype=torch.float)
+ self.conv.weight.data[:] = nn.Parameter(x.view(1, c1, 1, 1))
+ self.c1 = c1
+
+ def forward(self, x):
+ """Applies a transformer layer on input tensor 'x' and returns a tensor."""
+ b, c, a = x.shape # batch, channels, anchors
+ return self.conv(x.view(b, 4, self.c1, a).transpose(2, 1).softmax(1)).view(b, 4, a)
+ # return self.conv(x.view(b, self.c1, 4, a).softmax(1)).view(b, 4, a)
+
+
+class Proto(nn.Module):
+ """YOLOv8 mask Proto module for segmentation models."""
+
+ def __init__(self, c1, c_=256, c2=32): # ch_in, number of protos, number of masks
+ super().__init__()
+ self.cv1 = Conv(c1, c_, k=3)
+ self.upsample = nn.ConvTranspose2d(c_, c_, 2, 2, 0, bias=True) # nn.Upsample(scale_factor=2, mode='nearest')
+ self.cv2 = Conv(c_, c_, k=3)
+ self.cv3 = Conv(c_, c2)
+
+ def forward(self, x):
+ """Performs a forward pass through layers using an upsampled input image."""
+ return self.cv3(self.cv2(self.upsample(self.cv1(x))))
+
+
+class HGStem(nn.Module):
+ """StemBlock of PPHGNetV2 with 5 convolutions and one maxpool2d.
+ https://github.com/PaddlePaddle/PaddleDetection/blob/develop/ppdet/modeling/backbones/hgnet_v2.py
+ """
+
+ def __init__(self, c1, cm, c2):
+ super().__init__()
+ self.stem1 = Conv(c1, cm, 3, 2, act=nn.ReLU())
+ self.stem2a = Conv(cm, cm // 2, 2, 1, 0, act=nn.ReLU())
+ self.stem2b = Conv(cm // 2, cm, 2, 1, 0, act=nn.ReLU())
+ self.stem3 = Conv(cm * 2, cm, 3, 2, act=nn.ReLU())
+ self.stem4 = Conv(cm, c2, 1, 1, act=nn.ReLU())
+ self.pool = nn.MaxPool2d(kernel_size=2, stride=1, padding=0, ceil_mode=True)
+
+ def forward(self, x):
+ """Forward pass of a PPHGNetV2 backbone layer."""
+ x = self.stem1(x)
+ x = F.pad(x, [0, 1, 0, 1])
+ x2 = self.stem2a(x)
+ x2 = F.pad(x2, [0, 1, 0, 1])
+ x2 = self.stem2b(x2)
+ x1 = self.pool(x)
+ x = torch.cat([x1, x2], dim=1)
+ x = self.stem3(x)
+ x = self.stem4(x)
+ return x
+
+
+class HGBlock(nn.Module):
+ """HG_Block of PPHGNetV2 with 2 convolutions and LightConv.
+ https://github.com/PaddlePaddle/PaddleDetection/blob/develop/ppdet/modeling/backbones/hgnet_v2.py
+ """
+
+ def __init__(self, c1, cm, c2, k=3, n=6, lightconv=False, shortcut=False, act=nn.ReLU()):
+ super().__init__()
+ block = LightConv if lightconv else Conv
+ self.m = nn.ModuleList(block(c1 if i == 0 else cm, cm, k=k, act=act) for i in range(n))
+ self.sc = Conv(c1 + n * cm, c2 // 2, 1, 1, act=act) # squeeze conv
+ self.ec = Conv(c2 // 2, c2, 1, 1, act=act) # excitation conv
+ self.add = shortcut and c1 == c2
+
+ def forward(self, x):
+ """Forward pass of a PPHGNetV2 backbone layer."""
+ y = [x]
+ y.extend(m(y[-1]) for m in self.m)
+ y = self.ec(self.sc(torch.cat(y, 1)))
+ return y + x if self.add else y
+
+
+class SPP(nn.Module):
+ """Spatial Pyramid Pooling (SPP) layer https://arxiv.org/abs/1406.4729."""
+
+ def __init__(self, c1, c2, k=(5, 9, 13)):
+ """Initialize the SPP layer with input/output channels and pooling kernel sizes."""
+ super().__init__()
+ c_ = c1 // 2 # hidden channels
+ self.cv1 = Conv(c1, c_, 1, 1)
+ self.cv2 = Conv(c_ * (len(k) + 1), c2, 1, 1)
+ self.m = nn.ModuleList([nn.MaxPool2d(kernel_size=x, stride=1, padding=x // 2) for x in k])
+
+ def forward(self, x):
+ """Forward pass of the SPP layer, performing spatial pyramid pooling."""
+ x = self.cv1(x)
+ return self.cv2(torch.cat([x] + [m(x) for m in self.m], 1))
+
+
+class SPPF(nn.Module):
+ """Spatial Pyramid Pooling - Fast (SPPF) layer for YOLOv5 by Glenn Jocher."""
+
+ def __init__(self, c1, c2, k=5): # equivalent to SPP(k=(5, 9, 13))
+ super().__init__()
+ c_ = c1 // 2 # hidden channels
+ self.cv1 = Conv(c1, c_, 1, 1)
+ self.cv2 = Conv(c_ * 4, c2, 1, 1)
+ self.m = nn.MaxPool2d(kernel_size=k, stride=1, padding=k // 2)
+
+ def forward(self, x):
+ """Forward pass through Ghost Convolution block."""
+ x = self.cv1(x)
+ y1 = self.m(x)
+ y2 = self.m(y1)
+ return self.cv2(torch.cat((x, y1, y2, self.m(y2)), 1))
+
+
+class C1(nn.Module):
+ """CSP Bottleneck with 1 convolution."""
+
+ def __init__(self, c1, c2, n=1): # ch_in, ch_out, number
+ super().__init__()
+ self.cv1 = Conv(c1, c2, 1, 1)
+ self.m = nn.Sequential(*(Conv(c2, c2, 3) for _ in range(n)))
+
+ def forward(self, x):
+ """Applies cross-convolutions to input in the C3 module."""
+ y = self.cv1(x)
+ return self.m(y) + y
+
+
+class C2(nn.Module):
+ """CSP Bottleneck with 2 convolutions."""
+
+ def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5): # ch_in, ch_out, number, shortcut, groups, expansion
+ super().__init__()
+ self.c = int(c2 * e) # hidden channels
+ self.cv1 = Conv(c1, 2 * self.c, 1, 1)
+ self.cv2 = Conv(2 * self.c, c2, 1) # optional act=FReLU(c2)
+ # self.attention = ChannelAttention(2 * self.c) # or SpatialAttention()
+ self.m = nn.Sequential(*(Bottleneck(self.c, self.c, shortcut, g, k=((3, 3), (3, 3)), e=1.0) for _ in range(n)))
+
+ def forward(self, x):
+ """Forward pass through the CSP bottleneck with 2 convolutions."""
+ a, b = self.cv1(x).chunk(2, 1)
+ return self.cv2(torch.cat((self.m(a), b), 1))
+
+
+class C2f(nn.Module):
+ """CSP Bottleneck with 2 convolutions."""
+
+ def __init__(self, c1, c2, n=1, shortcut=False, g=1, e=0.5): # ch_in, ch_out, number, shortcut, groups, expansion
+ super().__init__()
+ self.c = int(c2 * e) # hidden channels
+ self.cv1 = Conv(c1, 2 * self.c, 1, 1)
+ self.cv2 = Conv((2 + n) * self.c, c2, 1) # optional act=FReLU(c2)
+ self.m = nn.ModuleList(Bottleneck(self.c, self.c, shortcut, g, k=((3, 3), (3, 3)), e=1.0) for _ in range(n))
+
+ def forward(self, x):
+ """Forward pass through C2f layer."""
+ y = list(self.cv1(x).chunk(2, 1))
+ y.extend(m(y[-1]) for m in self.m)
+ return self.cv2(torch.cat(y, 1))
+
+ def forward_split(self, x):
+ """Forward pass using split() instead of chunk()."""
+ y = list(self.cv1(x).split((self.c, self.c), 1))
+ y.extend(m(y[-1]) for m in self.m)
+ return self.cv2(torch.cat(y, 1))
+
+
+class C3(nn.Module):
+ """CSP Bottleneck with 3 convolutions."""
+
+ def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5): # ch_in, ch_out, number, shortcut, groups, expansion
+ super().__init__()
+ c_ = int(c2 * e) # hidden channels
+ self.cv1 = Conv(c1, c_, 1, 1)
+ self.cv2 = Conv(c1, c_, 1, 1)
+ self.cv3 = Conv(2 * c_, c2, 1) # optional act=FReLU(c2)
+ self.m = nn.Sequential(*(Bottleneck(c_, c_, shortcut, g, k=((1, 1), (3, 3)), e=1.0) for _ in range(n)))
+
+ def forward(self, x):
+ """Forward pass through the CSP bottleneck with 2 convolutions."""
+ return self.cv3(torch.cat((self.m(self.cv1(x)), self.cv2(x)), 1))
+
+
+class C3x(C3):
+ """C3 module with cross-convolutions."""
+
+ def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5):
+ """Initialize C3TR instance and set default parameters."""
+ super().__init__(c1, c2, n, shortcut, g, e)
+ self.c_ = int(c2 * e)
+ self.m = nn.Sequential(*(Bottleneck(self.c_, self.c_, shortcut, g, k=((1, 3), (3, 1)), e=1) for _ in range(n)))
+
+
+class RepC3(nn.Module):
+ """Rep C3."""
+
+ def __init__(self, c1, c2, n=3, e=1.0):
+ super().__init__()
+ c_ = int(c2 * e) # hidden channels
+ self.cv1 = Conv(c1, c2, 1, 1)
+ self.cv2 = Conv(c1, c2, 1, 1)
+ self.m = nn.Sequential(*[RepConv(c_, c_) for _ in range(n)])
+ self.cv3 = Conv(c_, c2, 1, 1) if c_ != c2 else nn.Identity()
+
+ def forward(self, x):
+ """Forward pass of RT-DETR neck layer."""
+ return self.cv3(self.m(self.cv1(x)) + self.cv2(x))
+
+
+class C3TR(C3):
+ """C3 module with TransformerBlock()."""
+
+ def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5):
+ """Initialize C3Ghost module with GhostBottleneck()."""
+ super().__init__(c1, c2, n, shortcut, g, e)
+ c_ = int(c2 * e)
+ self.m = TransformerBlock(c_, c_, 4, n)
+
+
+class C3Ghost(C3):
+ """C3 module with GhostBottleneck()."""
+
+ def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5):
+ """Initialize 'SPP' module with various pooling sizes for spatial pyramid pooling."""
+ super().__init__(c1, c2, n, shortcut, g, e)
+ c_ = int(c2 * e) # hidden channels
+ self.m = nn.Sequential(*(GhostBottleneck(c_, c_) for _ in range(n)))
+
+
+class GhostBottleneck(nn.Module):
+ """Ghost Bottleneck https://github.com/huawei-noah/ghostnet."""
+
+ def __init__(self, c1, c2, k=3, s=1): # ch_in, ch_out, kernel, stride
+ super().__init__()
+ c_ = c2 // 2
+ self.conv = nn.Sequential(
+ GhostConv(c1, c_, 1, 1), # pw
+ DWConv(c_, c_, k, s, act=False) if s == 2 else nn.Identity(), # dw
+ GhostConv(c_, c2, 1, 1, act=False)) # pw-linear
+ self.shortcut = nn.Sequential(DWConv(c1, c1, k, s, act=False), Conv(c1, c2, 1, 1,
+ act=False)) if s == 2 else nn.Identity()
+
+ def forward(self, x):
+ """Applies skip connection and concatenation to input tensor."""
+ return self.conv(x) + self.shortcut(x)
+
+
+class Bottleneck(nn.Module):
+ """Standard bottleneck."""
+
+ def __init__(self, c1, c2, shortcut=True, g=1, k=(3, 3), e=0.5): # ch_in, ch_out, shortcut, groups, kernels, expand
+ super().__init__()
+ c_ = int(c2 * e) # hidden channels
+ self.cv1 = Conv(c1, c_, k[0], 1)
+ self.cv2 = Conv(c_, c2, k[1], 1, g=g)
+ self.add = shortcut and c1 == c2
+
+ def forward(self, x):
+ """'forward()' applies the YOLOv5 FPN to input data."""
+ return x + self.cv2(self.cv1(x)) if self.add else self.cv2(self.cv1(x))
+
+
+class BottleneckCSP(nn.Module):
+ """CSP Bottleneck https://github.com/WongKinYiu/CrossStagePartialNetworks."""
+
+ def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5): # ch_in, ch_out, number, shortcut, groups, expansion
+ super().__init__()
+ c_ = int(c2 * e) # hidden channels
+ self.cv1 = Conv(c1, c_, 1, 1)
+ self.cv2 = nn.Conv2d(c1, c_, 1, 1, bias=False)
+ self.cv3 = nn.Conv2d(c_, c_, 1, 1, bias=False)
+ self.cv4 = Conv(2 * c_, c2, 1, 1)
+ self.bn = nn.BatchNorm2d(2 * c_) # applied to cat(cv2, cv3)
+ self.act = nn.SiLU()
+ self.m = nn.Sequential(*(Bottleneck(c_, c_, shortcut, g, e=1.0) for _ in range(n)))
+
+ def forward(self, x):
+ """Applies a CSP bottleneck with 3 convolutions."""
+ y1 = self.cv3(self.m(self.cv1(x)))
+ y2 = self.cv2(x)
+ return self.cv4(self.act(self.bn(torch.cat((y1, y2), 1))))
diff --git a/yolov8/ultralytics/nn/modules/conv.py b/yolov8/ultralytics/nn/modules/conv.py
new file mode 100644
index 0000000000000000000000000000000000000000..38ee3f5f3f40618e5fc0f7f836a3ecc595496bec
--- /dev/null
+++ b/yolov8/ultralytics/nn/modules/conv.py
@@ -0,0 +1,297 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Convolution modules
+"""
+
+import math
+
+import numpy as np
+import torch
+import torch.nn as nn
+
+__all__ = ('Conv', 'LightConv', 'DWConv', 'DWConvTranspose2d', 'ConvTranspose', 'Focus', 'GhostConv',
+ 'ChannelAttention', 'SpatialAttention', 'CBAM', 'Concat', 'RepConv')
+
+
+def autopad(k, p=None, d=1): # kernel, padding, dilation
+ """Pad to 'same' shape outputs."""
+ if d > 1:
+ k = d * (k - 1) + 1 if isinstance(k, int) else [d * (x - 1) + 1 for x in k] # actual kernel-size
+ if p is None:
+ p = k // 2 if isinstance(k, int) else [x // 2 for x in k] # auto-pad
+ return p
+
+
+class Conv(nn.Module):
+ """Standard convolution with args(ch_in, ch_out, kernel, stride, padding, groups, dilation, activation)."""
+ default_act = nn.SiLU() # default activation
+
+ def __init__(self, c1, c2, k=1, s=1, p=None, g=1, d=1, act=True):
+ """Initialize Conv layer with given arguments including activation."""
+ super().__init__()
+ self.conv = nn.Conv2d(c1, c2, k, s, autopad(k, p, d), groups=g, dilation=d, bias=False)
+ self.bn = nn.BatchNorm2d(c2)
+ self.act = self.default_act if act is True else act if isinstance(act, nn.Module) else nn.Identity()
+
+ def forward(self, x):
+ """Apply convolution, batch normalization and activation to input tensor."""
+ return self.act(self.bn(self.conv(x)))
+
+ def forward_fuse(self, x):
+ """Perform transposed convolution of 2D data."""
+ return self.act(self.conv(x))
+
+
+class Conv2(Conv):
+ """Simplified RepConv module with Conv fusing."""
+
+ def __init__(self, c1, c2, k=3, s=1, p=None, g=1, d=1, act=True):
+ """Initialize Conv layer with given arguments including activation."""
+ super().__init__(c1, c2, k, s, p, g=g, d=d, act=act)
+ self.cv2 = nn.Conv2d(c1, c2, 1, s, autopad(1, p, d), groups=g, dilation=d, bias=False) # add 1x1 conv
+
+ def forward(self, x):
+ """Apply convolution, batch normalization and activation to input tensor."""
+ return self.act(self.bn(self.conv(x) + self.cv2(x)))
+
+ def fuse_convs(self):
+ """Fuse parallel convolutions."""
+ w = torch.zeros_like(self.conv.weight.data)
+ i = [x // 2 for x in w.shape[2:]]
+ w[:, :, i[0]:i[0] + 1, i[1]:i[1] + 1] = self.cv2.weight.data.clone()
+ self.conv.weight.data += w
+ self.__delattr__('cv2')
+
+
+class LightConv(nn.Module):
+ """Light convolution with args(ch_in, ch_out, kernel).
+ https://github.com/PaddlePaddle/PaddleDetection/blob/develop/ppdet/modeling/backbones/hgnet_v2.py
+ """
+
+ def __init__(self, c1, c2, k=1, act=nn.ReLU()):
+ """Initialize Conv layer with given arguments including activation."""
+ super().__init__()
+ self.conv1 = Conv(c1, c2, 1, act=False)
+ self.conv2 = DWConv(c2, c2, k, act=act)
+
+ def forward(self, x):
+ """Apply 2 convolutions to input tensor."""
+ return self.conv2(self.conv1(x))
+
+
+class DWConv(Conv):
+ """Depth-wise convolution."""
+
+ def __init__(self, c1, c2, k=1, s=1, d=1, act=True): # ch_in, ch_out, kernel, stride, dilation, activation
+ super().__init__(c1, c2, k, s, g=math.gcd(c1, c2), d=d, act=act)
+
+
+class DWConvTranspose2d(nn.ConvTranspose2d):
+ """Depth-wise transpose convolution."""
+
+ def __init__(self, c1, c2, k=1, s=1, p1=0, p2=0): # ch_in, ch_out, kernel, stride, padding, padding_out
+ super().__init__(c1, c2, k, s, p1, p2, groups=math.gcd(c1, c2))
+
+
+class ConvTranspose(nn.Module):
+ """Convolution transpose 2d layer."""
+ default_act = nn.SiLU() # default activation
+
+ def __init__(self, c1, c2, k=2, s=2, p=0, bn=True, act=True):
+ """Initialize ConvTranspose2d layer with batch normalization and activation function."""
+ super().__init__()
+ self.conv_transpose = nn.ConvTranspose2d(c1, c2, k, s, p, bias=not bn)
+ self.bn = nn.BatchNorm2d(c2) if bn else nn.Identity()
+ self.act = self.default_act if act is True else act if isinstance(act, nn.Module) else nn.Identity()
+
+ def forward(self, x):
+ """Applies transposed convolutions, batch normalization and activation to input."""
+ return self.act(self.bn(self.conv_transpose(x)))
+
+ def forward_fuse(self, x):
+ """Applies activation and convolution transpose operation to input."""
+ return self.act(self.conv_transpose(x))
+
+
+class Focus(nn.Module):
+ """Focus wh information into c-space."""
+
+ def __init__(self, c1, c2, k=1, s=1, p=None, g=1, act=True): # ch_in, ch_out, kernel, stride, padding, groups
+ super().__init__()
+ self.conv = Conv(c1 * 4, c2, k, s, p, g, act=act)
+ # self.contract = Contract(gain=2)
+
+ def forward(self, x): # x(b,c,w,h) -> y(b,4c,w/2,h/2)
+ return self.conv(torch.cat((x[..., ::2, ::2], x[..., 1::2, ::2], x[..., ::2, 1::2], x[..., 1::2, 1::2]), 1))
+ # return self.conv(self.contract(x))
+
+
+class GhostConv(nn.Module):
+ """Ghost Convolution https://github.com/huawei-noah/ghostnet."""
+
+ def __init__(self, c1, c2, k=1, s=1, g=1, act=True): # ch_in, ch_out, kernel, stride, groups
+ super().__init__()
+ c_ = c2 // 2 # hidden channels
+ self.cv1 = Conv(c1, c_, k, s, None, g, act=act)
+ self.cv2 = Conv(c_, c_, 5, 1, None, c_, act=act)
+
+ def forward(self, x):
+ """Forward propagation through a Ghost Bottleneck layer with skip connection."""
+ y = self.cv1(x)
+ return torch.cat((y, self.cv2(y)), 1)
+
+
+class RepConv(nn.Module):
+ """RepConv is a basic rep-style block, including training and deploy status
+ This code is based on https://github.com/DingXiaoH/RepVGG/blob/main/repvgg.py
+ """
+ default_act = nn.SiLU() # default activation
+
+ def __init__(self, c1, c2, k=3, s=1, p=1, g=1, d=1, act=True, bn=False, deploy=False):
+ super().__init__()
+ assert k == 3 and p == 1
+ self.g = g
+ self.c1 = c1
+ self.c2 = c2
+ self.act = self.default_act if act is True else act if isinstance(act, nn.Module) else nn.Identity()
+
+ self.bn = nn.BatchNorm2d(num_features=c1) if bn and c2 == c1 and s == 1 else None
+ self.conv1 = Conv(c1, c2, k, s, p=p, g=g, act=False)
+ self.conv2 = Conv(c1, c2, 1, s, p=(p - k // 2), g=g, act=False)
+
+ def forward_fuse(self, x):
+ """Forward process"""
+ return self.act(self.conv(x))
+
+ def forward(self, x):
+ """Forward process"""
+ id_out = 0 if self.bn is None else self.bn(x)
+ return self.act(self.conv1(x) + self.conv2(x) + id_out)
+
+ def get_equivalent_kernel_bias(self):
+ kernel3x3, bias3x3 = self._fuse_bn_tensor(self.conv1)
+ kernel1x1, bias1x1 = self._fuse_bn_tensor(self.conv2)
+ kernelid, biasid = self._fuse_bn_tensor(self.bn)
+ return kernel3x3 + self._pad_1x1_to_3x3_tensor(kernel1x1) + kernelid, bias3x3 + bias1x1 + biasid
+
+ def _avg_to_3x3_tensor(self, avgp):
+ channels = self.c1
+ groups = self.g
+ kernel_size = avgp.kernel_size
+ input_dim = channels // groups
+ k = torch.zeros((channels, input_dim, kernel_size, kernel_size))
+ k[np.arange(channels), np.tile(np.arange(input_dim), groups), :, :] = 1.0 / kernel_size ** 2
+ return k
+
+ def _pad_1x1_to_3x3_tensor(self, kernel1x1):
+ if kernel1x1 is None:
+ return 0
+ else:
+ return torch.nn.functional.pad(kernel1x1, [1, 1, 1, 1])
+
+ def _fuse_bn_tensor(self, branch):
+ if branch is None:
+ return 0, 0
+ if isinstance(branch, Conv):
+ kernel = branch.conv.weight
+ running_mean = branch.bn.running_mean
+ running_var = branch.bn.running_var
+ gamma = branch.bn.weight
+ beta = branch.bn.bias
+ eps = branch.bn.eps
+ elif isinstance(branch, nn.BatchNorm2d):
+ if not hasattr(self, 'id_tensor'):
+ input_dim = self.c1 // self.g
+ kernel_value = np.zeros((self.c1, input_dim, 3, 3), dtype=np.float32)
+ for i in range(self.c1):
+ kernel_value[i, i % input_dim, 1, 1] = 1
+ self.id_tensor = torch.from_numpy(kernel_value).to(branch.weight.device)
+ kernel = self.id_tensor
+ running_mean = branch.running_mean
+ running_var = branch.running_var
+ gamma = branch.weight
+ beta = branch.bias
+ eps = branch.eps
+ std = (running_var + eps).sqrt()
+ t = (gamma / std).reshape(-1, 1, 1, 1)
+ return kernel * t, beta - running_mean * gamma / std
+
+ def fuse_convs(self):
+ if hasattr(self, 'conv'):
+ return
+ kernel, bias = self.get_equivalent_kernel_bias()
+ self.conv = nn.Conv2d(in_channels=self.conv1.conv.in_channels,
+ out_channels=self.conv1.conv.out_channels,
+ kernel_size=self.conv1.conv.kernel_size,
+ stride=self.conv1.conv.stride,
+ padding=self.conv1.conv.padding,
+ dilation=self.conv1.conv.dilation,
+ groups=self.conv1.conv.groups,
+ bias=True).requires_grad_(False)
+ self.conv.weight.data = kernel
+ self.conv.bias.data = bias
+ for para in self.parameters():
+ para.detach_()
+ self.__delattr__('conv1')
+ self.__delattr__('conv2')
+ if hasattr(self, 'nm'):
+ self.__delattr__('nm')
+ if hasattr(self, 'bn'):
+ self.__delattr__('bn')
+ if hasattr(self, 'id_tensor'):
+ self.__delattr__('id_tensor')
+
+
+class ChannelAttention(nn.Module):
+ """Channel-attention module https://github.com/open-mmlab/mmdetection/tree/v3.0.0rc1/configs/rtmdet."""
+
+ def __init__(self, channels: int) -> None:
+ super().__init__()
+ self.pool = nn.AdaptiveAvgPool2d(1)
+ self.fc = nn.Conv2d(channels, channels, 1, 1, 0, bias=True)
+ self.act = nn.Sigmoid()
+
+ def forward(self, x: torch.Tensor) -> torch.Tensor:
+ return x * self.act(self.fc(self.pool(x)))
+
+
+class SpatialAttention(nn.Module):
+ """Spatial-attention module."""
+
+ def __init__(self, kernel_size=7):
+ """Initialize Spatial-attention module with kernel size argument."""
+ super().__init__()
+ assert kernel_size in (3, 7), 'kernel size must be 3 or 7'
+ padding = 3 if kernel_size == 7 else 1
+ self.cv1 = nn.Conv2d(2, 1, kernel_size, padding=padding, bias=False)
+ self.act = nn.Sigmoid()
+
+ def forward(self, x):
+ """Apply channel and spatial attention on input for feature recalibration."""
+ return x * self.act(self.cv1(torch.cat([torch.mean(x, 1, keepdim=True), torch.max(x, 1, keepdim=True)[0]], 1)))
+
+
+class CBAM(nn.Module):
+ """Convolutional Block Attention Module."""
+
+ def __init__(self, c1, kernel_size=7): # ch_in, kernels
+ super().__init__()
+ self.channel_attention = ChannelAttention(c1)
+ self.spatial_attention = SpatialAttention(kernel_size)
+
+ def forward(self, x):
+ """Applies the forward pass through C1 module."""
+ return self.spatial_attention(self.channel_attention(x))
+
+
+class Concat(nn.Module):
+ """Concatenate a list of tensors along dimension."""
+
+ def __init__(self, dimension=1):
+ """Concatenates a list of tensors along a specified dimension."""
+ super().__init__()
+ self.d = dimension
+
+ def forward(self, x):
+ """Forward pass for the YOLOv8 mask Proto module."""
+ return torch.cat(x, self.d)
diff --git a/yolov8/ultralytics/nn/modules/head.py b/yolov8/ultralytics/nn/modules/head.py
new file mode 100644
index 0000000000000000000000000000000000000000..3b70d702516e927e982d578c7e2176d5d4056497
--- /dev/null
+++ b/yolov8/ultralytics/nn/modules/head.py
@@ -0,0 +1,353 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Model head modules
+"""
+
+import math
+
+import torch
+import torch.nn as nn
+from torch.nn.init import constant_, xavier_uniform_
+
+from ultralytics.yolo.utils.tal import dist2bbox, make_anchors
+
+from .block import DFL, Proto
+from .conv import Conv
+from .transformer import MLP, DeformableTransformerDecoder, DeformableTransformerDecoderLayer
+from .utils import bias_init_with_prob, linear_init_
+
+__all__ = 'Detect', 'Segment', 'Pose', 'Classify', 'RTDETRDecoder'
+
+
+class Detect(nn.Module):
+ """YOLOv8 Detect head for detection models."""
+ dynamic = False # force grid reconstruction
+ export = False # export mode
+ shape = None
+ anchors = torch.empty(0) # init
+ strides = torch.empty(0) # init
+
+ def __init__(self, nc=80, ch=()): # detection layer
+ super().__init__()
+ self.nc = nc # number of classes
+ self.nl = len(ch) # number of detection layers
+ self.reg_max = 16 # DFL channels (ch[0] // 16 to scale 4/8/12/16/20 for n/s/m/l/x)
+ self.no = nc + self.reg_max * 4 # number of outputs per anchor
+ self.stride = torch.zeros(self.nl) # strides computed during build
+ c2, c3 = max((16, ch[0] // 4, self.reg_max * 4)), max(ch[0], min(self.nc, 100)) # channels
+ self.cv2 = nn.ModuleList(
+ nn.Sequential(Conv(x, c2, 3), Conv(c2, c2, 3), nn.Conv2d(c2, 4 * self.reg_max, 1)) for x in ch)
+ self.cv3 = nn.ModuleList(nn.Sequential(Conv(x, c3, 3), Conv(c3, c3, 3), nn.Conv2d(c3, self.nc, 1)) for x in ch)
+ self.dfl = DFL(self.reg_max) if self.reg_max > 1 else nn.Identity()
+
+ def forward(self, x):
+ """Concatenates and returns predicted bounding boxes and class probabilities."""
+ shape = x[0].shape # BCHW
+ for i in range(self.nl):
+ x[i] = torch.cat((self.cv2[i](x[i]), self.cv3[i](x[i])), 1)
+ if self.training:
+ return x
+ elif self.dynamic or self.shape != shape:
+ self.anchors, self.strides = (x.transpose(0, 1) for x in make_anchors(x, self.stride, 0.5))
+ self.shape = shape
+
+ x_cat = torch.cat([xi.view(shape[0], self.no, -1) for xi in x], 2)
+ if self.export and self.format in ('saved_model', 'pb', 'tflite', 'edgetpu', 'tfjs'): # avoid TF FlexSplitV ops
+ box = x_cat[:, :self.reg_max * 4]
+ cls = x_cat[:, self.reg_max * 4:]
+ else:
+ box, cls = x_cat.split((self.reg_max * 4, self.nc), 1)
+ dbox = dist2bbox(self.dfl(box), self.anchors.unsqueeze(0), xywh=True, dim=1) * self.strides
+ y = torch.cat((dbox, cls.sigmoid()), 1)
+ return y if self.export else (y, x)
+
+ def bias_init(self):
+ """Initialize Detect() biases, WARNING: requires stride availability."""
+ m = self # self.model[-1] # Detect() module
+ # cf = torch.bincount(torch.tensor(np.concatenate(dataset.labels, 0)[:, 0]).long(), minlength=nc) + 1
+ # ncf = math.log(0.6 / (m.nc - 0.999999)) if cf is None else torch.log(cf / cf.sum()) # nominal class frequency
+ for a, b, s in zip(m.cv2, m.cv3, m.stride): # from
+ a[-1].bias.data[:] = 1.0 # box
+ b[-1].bias.data[:m.nc] = math.log(5 / m.nc / (640 / s) ** 2) # cls (.01 objects, 80 classes, 640 img)
+
+
+class Segment(Detect):
+ """YOLOv8 Segment head for segmentation models."""
+
+ def __init__(self, nc=80, nm=32, npr=256, ch=()):
+ """Initialize the YOLO model attributes such as the number of masks, prototypes, and the convolution layers."""
+ super().__init__(nc, ch)
+ self.nm = nm # number of masks
+ self.npr = npr # number of protos
+ self.proto = Proto(ch[0], self.npr, self.nm) # protos
+ self.detect = Detect.forward
+
+ c4 = max(ch[0] // 4, self.nm)
+ self.cv4 = nn.ModuleList(nn.Sequential(Conv(x, c4, 3), Conv(c4, c4, 3), nn.Conv2d(c4, self.nm, 1)) for x in ch)
+
+ def forward(self, x):
+ """Return model outputs and mask coefficients if training, otherwise return outputs and mask coefficients."""
+ p = self.proto(x[0]) # mask protos
+ bs = p.shape[0] # batch size
+
+ mc = torch.cat([self.cv4[i](x[i]).view(bs, self.nm, -1) for i in range(self.nl)], 2) # mask coefficients
+ x = self.detect(self, x)
+ if self.training:
+ return x, mc, p
+ return (torch.cat([x, mc], 1), p) if self.export else (torch.cat([x[0], mc], 1), (x[1], mc, p))
+
+
+class Pose(Detect):
+ """YOLOv8 Pose head for keypoints models."""
+
+ def __init__(self, nc=80, kpt_shape=(17, 3), ch=()):
+ """Initialize YOLO network with default parameters and Convolutional Layers."""
+ super().__init__(nc, ch)
+ self.kpt_shape = kpt_shape # number of keypoints, number of dims (2 for x,y or 3 for x,y,visible)
+ self.nk = kpt_shape[0] * kpt_shape[1] # number of keypoints total
+ self.detect = Detect.forward
+
+ c4 = max(ch[0] // 4, self.nk)
+ self.cv4 = nn.ModuleList(nn.Sequential(Conv(x, c4, 3), Conv(c4, c4, 3), nn.Conv2d(c4, self.nk, 1)) for x in ch)
+
+ def forward(self, x):
+ """Perform forward pass through YOLO model and return predictions."""
+ bs = x[0].shape[0] # batch size
+ kpt = torch.cat([self.cv4[i](x[i]).view(bs, self.nk, -1) for i in range(self.nl)], -1) # (bs, 17*3, h*w)
+ x = self.detect(self, x)
+ if self.training:
+ return x, kpt
+ pred_kpt = self.kpts_decode(bs, kpt)
+ return torch.cat([x, pred_kpt], 1) if self.export else (torch.cat([x[0], pred_kpt], 1), (x[1], kpt))
+
+ def kpts_decode(self, bs, kpts):
+ """Decodes keypoints."""
+ ndim = self.kpt_shape[1]
+ if self.export: # required for TFLite export to avoid 'PLACEHOLDER_FOR_GREATER_OP_CODES' bug
+ y = kpts.view(bs, *self.kpt_shape, -1)
+ a = (y[:, :, :2] * 2.0 + (self.anchors - 0.5)) * self.strides
+ if ndim == 3:
+ a = torch.cat((a, y[:, :, 2:3].sigmoid()), 2)
+ return a.view(bs, self.nk, -1)
+ else:
+ y = kpts.clone()
+ if ndim == 3:
+ y[:, 2::3].sigmoid_() # inplace sigmoid
+ y[:, 0::ndim] = (y[:, 0::ndim] * 2.0 + (self.anchors[0] - 0.5)) * self.strides
+ y[:, 1::ndim] = (y[:, 1::ndim] * 2.0 + (self.anchors[1] - 0.5)) * self.strides
+ return y
+
+
+class Classify(nn.Module):
+ """YOLOv8 classification head, i.e. x(b,c1,20,20) to x(b,c2)."""
+
+ def __init__(self, c1, c2, k=1, s=1, p=None, g=1): # ch_in, ch_out, kernel, stride, padding, groups
+ super().__init__()
+ c_ = 1280 # efficientnet_b0 size
+ self.conv = Conv(c1, c_, k, s, p, g)
+ self.pool = nn.AdaptiveAvgPool2d(1) # to x(b,c_,1,1)
+ self.drop = nn.Dropout(p=0.0, inplace=True)
+ self.linear = nn.Linear(c_, c2) # to x(b,c2)
+
+ def forward(self, x):
+ """Performs a forward pass of the YOLO model on input image data."""
+ if isinstance(x, list):
+ x = torch.cat(x, 1)
+ x = self.linear(self.drop(self.pool(self.conv(x)).flatten(1)))
+ return x if self.training else x.softmax(1)
+
+
+class RTDETRDecoder(nn.Module):
+ export = False # export mode
+
+ def __init__(
+ self,
+ nc=80,
+ ch=(512, 1024, 2048),
+ hd=256, # hidden dim
+ nq=300, # num queries
+ ndp=4, # num decoder points
+ nh=8, # num head
+ ndl=6, # num decoder layers
+ d_ffn=1024, # dim of feedforward
+ dropout=0.,
+ act=nn.ReLU(),
+ eval_idx=-1,
+ # training args
+ nd=100, # num denoising
+ label_noise_ratio=0.5,
+ box_noise_scale=1.0,
+ learnt_init_query=False):
+ super().__init__()
+ self.hidden_dim = hd
+ self.nhead = nh
+ self.nl = len(ch) # num level
+ self.nc = nc
+ self.num_queries = nq
+ self.num_decoder_layers = ndl
+
+ # backbone feature projection
+ self.input_proj = nn.ModuleList(nn.Sequential(nn.Conv2d(x, hd, 1, bias=False), nn.BatchNorm2d(hd)) for x in ch)
+ # NOTE: simplified version but it's not consistent with .pt weights.
+ # self.input_proj = nn.ModuleList(Conv(x, hd, act=False) for x in ch)
+
+ # Transformer module
+ decoder_layer = DeformableTransformerDecoderLayer(hd, nh, d_ffn, dropout, act, self.nl, ndp)
+ self.decoder = DeformableTransformerDecoder(hd, decoder_layer, ndl, eval_idx)
+
+ # denoising part
+ self.denoising_class_embed = nn.Embedding(nc, hd)
+ self.num_denoising = nd
+ self.label_noise_ratio = label_noise_ratio
+ self.box_noise_scale = box_noise_scale
+
+ # decoder embedding
+ self.learnt_init_query = learnt_init_query
+ if learnt_init_query:
+ self.tgt_embed = nn.Embedding(nq, hd)
+ self.query_pos_head = MLP(4, 2 * hd, hd, num_layers=2)
+
+ # encoder head
+ self.enc_output = nn.Sequential(nn.Linear(hd, hd), nn.LayerNorm(hd))
+ self.enc_score_head = nn.Linear(hd, nc)
+ self.enc_bbox_head = MLP(hd, hd, 4, num_layers=3)
+
+ # decoder head
+ self.dec_score_head = nn.ModuleList([nn.Linear(hd, nc) for _ in range(ndl)])
+ self.dec_bbox_head = nn.ModuleList([MLP(hd, hd, 4, num_layers=3) for _ in range(ndl)])
+
+ self._reset_parameters()
+
+ def forward(self, x, batch=None):
+ from ultralytics.vit.utils.ops import get_cdn_group
+
+ # input projection and embedding
+ feats, shapes = self._get_encoder_input(x)
+
+ # prepare denoising training
+ dn_embed, dn_bbox, attn_mask, dn_meta = \
+ get_cdn_group(batch,
+ self.nc,
+ self.num_queries,
+ self.denoising_class_embed.weight,
+ self.num_denoising,
+ self.label_noise_ratio,
+ self.box_noise_scale,
+ self.training)
+
+ embed, refer_bbox, enc_bboxes, enc_scores = \
+ self._get_decoder_input(feats, shapes, dn_embed, dn_bbox)
+
+ # decoder
+ dec_bboxes, dec_scores = self.decoder(embed,
+ refer_bbox,
+ feats,
+ shapes,
+ self.dec_bbox_head,
+ self.dec_score_head,
+ self.query_pos_head,
+ attn_mask=attn_mask)
+ x = dec_bboxes, dec_scores, enc_bboxes, enc_scores, dn_meta
+ if self.training:
+ return x
+ # (bs, 300, 4+nc)
+ y = torch.cat((dec_bboxes.squeeze(0), dec_scores.squeeze(0).sigmoid()), -1)
+ return y if self.export else (y, x)
+
+ def _generate_anchors(self, shapes, grid_size=0.05, dtype=torch.float32, device='cpu', eps=1e-2):
+ anchors = []
+ for i, (h, w) in enumerate(shapes):
+ grid_y, grid_x = torch.meshgrid(torch.arange(end=h, dtype=dtype, device=device),
+ torch.arange(end=w, dtype=dtype, device=device),
+ indexing='ij')
+ grid_xy = torch.stack([grid_x, grid_y], -1) # (h, w, 2)
+
+ valid_WH = torch.tensor([h, w], dtype=dtype, device=device)
+ grid_xy = (grid_xy.unsqueeze(0) + 0.5) / valid_WH # (1, h, w, 2)
+ wh = torch.ones_like(grid_xy, dtype=dtype, device=device) * grid_size * (2.0 ** i)
+ anchors.append(torch.cat([grid_xy, wh], -1).view(-1, h * w, 4)) # (1, h*w, 4)
+
+ anchors = torch.cat(anchors, 1) # (1, h*w*nl, 4)
+ valid_mask = ((anchors > eps) * (anchors < 1 - eps)).all(-1, keepdim=True) # 1, h*w*nl, 1
+ anchors = torch.log(anchors / (1 - anchors))
+ anchors = anchors.masked_fill(~valid_mask, float('inf'))
+ return anchors, valid_mask
+
+ def _get_encoder_input(self, x):
+ # get projection features
+ x = [self.input_proj[i](feat) for i, feat in enumerate(x)]
+ # get encoder inputs
+ feats = []
+ shapes = []
+ for feat in x:
+ h, w = feat.shape[2:]
+ # [b, c, h, w] -> [b, h*w, c]
+ feats.append(feat.flatten(2).permute(0, 2, 1))
+ # [nl, 2]
+ shapes.append([h, w])
+
+ # [b, h*w, c]
+ feats = torch.cat(feats, 1)
+ return feats, shapes
+
+ def _get_decoder_input(self, feats, shapes, dn_embed=None, dn_bbox=None):
+ bs = len(feats)
+ # prepare input for decoder
+ anchors, valid_mask = self._generate_anchors(shapes, dtype=feats.dtype, device=feats.device)
+ features = self.enc_output(valid_mask * feats) # bs, h*w, 256
+
+ enc_outputs_scores = self.enc_score_head(features) # (bs, h*w, nc)
+ # dynamic anchors + static content
+ enc_outputs_bboxes = self.enc_bbox_head(features) + anchors # (bs, h*w, 4)
+
+ # query selection
+ # (bs, num_queries)
+ topk_ind = torch.topk(enc_outputs_scores.max(-1).values, self.num_queries, dim=1).indices.view(-1)
+ # (bs, num_queries)
+ batch_ind = torch.arange(end=bs, dtype=topk_ind.dtype).unsqueeze(-1).repeat(1, self.num_queries).view(-1)
+
+ # Unsigmoided
+ refer_bbox = enc_outputs_bboxes[batch_ind, topk_ind].view(bs, self.num_queries, -1)
+ # refer_bbox = torch.gather(enc_outputs_bboxes, 1, topk_ind.reshape(bs, self.num_queries).unsqueeze(-1).repeat(1, 1, 4))
+
+ enc_bboxes = refer_bbox.sigmoid()
+ if dn_bbox is not None:
+ refer_bbox = torch.cat([dn_bbox, refer_bbox], 1)
+ if self.training:
+ refer_bbox = refer_bbox.detach()
+ enc_scores = enc_outputs_scores[batch_ind, topk_ind].view(bs, self.num_queries, -1)
+
+ if self.learnt_init_query:
+ embeddings = self.tgt_embed.weight.unsqueeze(0).repeat(bs, 1, 1)
+ else:
+ embeddings = features[batch_ind, topk_ind].view(bs, self.num_queries, -1)
+ if self.training:
+ embeddings = embeddings.detach()
+ if dn_embed is not None:
+ embeddings = torch.cat([dn_embed, embeddings], 1)
+
+ return embeddings, refer_bbox, enc_bboxes, enc_scores
+
+ # TODO
+ def _reset_parameters(self):
+ # class and bbox head init
+ bias_cls = bias_init_with_prob(0.01) / 80 * self.nc
+ # NOTE: the weight initialization in `linear_init_` would cause NaN when training with custom datasets.
+ # linear_init_(self.enc_score_head)
+ constant_(self.enc_score_head.bias, bias_cls)
+ constant_(self.enc_bbox_head.layers[-1].weight, 0.)
+ constant_(self.enc_bbox_head.layers[-1].bias, 0.)
+ for cls_, reg_ in zip(self.dec_score_head, self.dec_bbox_head):
+ # linear_init_(cls_)
+ constant_(cls_.bias, bias_cls)
+ constant_(reg_.layers[-1].weight, 0.)
+ constant_(reg_.layers[-1].bias, 0.)
+
+ linear_init_(self.enc_output[0])
+ xavier_uniform_(self.enc_output[0].weight)
+ if self.learnt_init_query:
+ xavier_uniform_(self.tgt_embed.weight)
+ xavier_uniform_(self.query_pos_head.layers[0].weight)
+ xavier_uniform_(self.query_pos_head.layers[1].weight)
+ for layer in self.input_proj:
+ xavier_uniform_(layer[0].weight)
diff --git a/yolov8/ultralytics/nn/modules/transformer.py b/yolov8/ultralytics/nn/modules/transformer.py
new file mode 100644
index 0000000000000000000000000000000000000000..b3304cc8d8f8ba7f60493bc1d800f496053ed434
--- /dev/null
+++ b/yolov8/ultralytics/nn/modules/transformer.py
@@ -0,0 +1,378 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Transformer modules
+"""
+
+import math
+
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+from torch.nn.init import constant_, xavier_uniform_
+
+from .conv import Conv
+from .utils import _get_clones, inverse_sigmoid, multi_scale_deformable_attn_pytorch
+
+__all__ = ('TransformerEncoderLayer', 'TransformerLayer', 'TransformerBlock', 'MLPBlock', 'LayerNorm2d', 'AIFI',
+ 'DeformableTransformerDecoder', 'DeformableTransformerDecoderLayer', 'MSDeformAttn', 'MLP')
+
+
+class TransformerEncoderLayer(nn.Module):
+ """Transformer Encoder."""
+
+ def __init__(self, c1, cm=2048, num_heads=8, dropout=0.0, act=nn.GELU(), normalize_before=False):
+ super().__init__()
+ self.ma = nn.MultiheadAttention(c1, num_heads, dropout=dropout, batch_first=True)
+ # Implementation of Feedforward model
+ self.fc1 = nn.Linear(c1, cm)
+ self.fc2 = nn.Linear(cm, c1)
+
+ self.norm1 = nn.LayerNorm(c1)
+ self.norm2 = nn.LayerNorm(c1)
+ self.dropout = nn.Dropout(dropout)
+ self.dropout1 = nn.Dropout(dropout)
+ self.dropout2 = nn.Dropout(dropout)
+
+ self.act = act
+ self.normalize_before = normalize_before
+
+ def with_pos_embed(self, tensor, pos=None):
+ """Add position embeddings if given."""
+ return tensor if pos is None else tensor + pos
+
+ def forward_post(self, src, src_mask=None, src_key_padding_mask=None, pos=None):
+ q = k = self.with_pos_embed(src, pos)
+ src2 = self.ma(q, k, value=src, attn_mask=src_mask, key_padding_mask=src_key_padding_mask)[0]
+ src = src + self.dropout1(src2)
+ src = self.norm1(src)
+ src2 = self.fc2(self.dropout(self.act(self.fc1(src))))
+ src = src + self.dropout2(src2)
+ src = self.norm2(src)
+ return src
+
+ def forward_pre(self, src, src_mask=None, src_key_padding_mask=None, pos=None):
+ src2 = self.norm1(src)
+ q = k = self.with_pos_embed(src2, pos)
+ src2 = self.ma(q, k, value=src2, attn_mask=src_mask, key_padding_mask=src_key_padding_mask)[0]
+ src = src + self.dropout1(src2)
+ src2 = self.norm2(src)
+ src2 = self.fc2(self.dropout(self.act(self.fc1(src2))))
+ src = src + self.dropout2(src2)
+ return src
+
+ def forward(self, src, src_mask=None, src_key_padding_mask=None, pos=None):
+ """Forward propagates the input through the encoder module."""
+ if self.normalize_before:
+ return self.forward_pre(src, src_mask, src_key_padding_mask, pos)
+ return self.forward_post(src, src_mask, src_key_padding_mask, pos)
+
+
+class AIFI(TransformerEncoderLayer):
+
+ def __init__(self, c1, cm=2048, num_heads=8, dropout=0, act=nn.GELU(), normalize_before=False):
+ super().__init__(c1, cm, num_heads, dropout, act, normalize_before)
+
+ def forward(self, x):
+ c, h, w = x.shape[1:]
+ pos_embed = self.build_2d_sincos_position_embedding(w, h, c)
+ # flatten [B, C, H, W] to [B, HxW, C]
+ x = super().forward(x.flatten(2).permute(0, 2, 1), pos=pos_embed.to(device=x.device, dtype=x.dtype))
+ return x.permute(0, 2, 1).view([-1, c, h, w]).contiguous()
+
+ @staticmethod
+ def build_2d_sincos_position_embedding(w, h, embed_dim=256, temperature=10000.):
+ grid_w = torch.arange(int(w), dtype=torch.float32)
+ grid_h = torch.arange(int(h), dtype=torch.float32)
+ grid_w, grid_h = torch.meshgrid(grid_w, grid_h, indexing='ij')
+ assert embed_dim % 4 == 0, \
+ 'Embed dimension must be divisible by 4 for 2D sin-cos position embedding'
+ pos_dim = embed_dim // 4
+ omega = torch.arange(pos_dim, dtype=torch.float32) / pos_dim
+ omega = 1. / (temperature ** omega)
+
+ out_w = grid_w.flatten()[..., None] @ omega[None]
+ out_h = grid_h.flatten()[..., None] @ omega[None]
+
+ return torch.concat([torch.sin(out_w), torch.cos(out_w),
+ torch.sin(out_h), torch.cos(out_h)], axis=1)[None, :, :]
+
+
+class TransformerLayer(nn.Module):
+ """Transformer layer https://arxiv.org/abs/2010.11929 (LayerNorm layers removed for better performance)."""
+
+ def __init__(self, c, num_heads):
+ """Initializes a self-attention mechanism using linear transformations and multi-head attention."""
+ super().__init__()
+ self.q = nn.Linear(c, c, bias=False)
+ self.k = nn.Linear(c, c, bias=False)
+ self.v = nn.Linear(c, c, bias=False)
+ self.ma = nn.MultiheadAttention(embed_dim=c, num_heads=num_heads)
+ self.fc1 = nn.Linear(c, c, bias=False)
+ self.fc2 = nn.Linear(c, c, bias=False)
+
+ def forward(self, x):
+ """Apply a transformer block to the input x and return the output."""
+ x = self.ma(self.q(x), self.k(x), self.v(x))[0] + x
+ x = self.fc2(self.fc1(x)) + x
+ return x
+
+
+class TransformerBlock(nn.Module):
+ """Vision Transformer https://arxiv.org/abs/2010.11929."""
+
+ def __init__(self, c1, c2, num_heads, num_layers):
+ """Initialize a Transformer module with position embedding and specified number of heads and layers."""
+ super().__init__()
+ self.conv = None
+ if c1 != c2:
+ self.conv = Conv(c1, c2)
+ self.linear = nn.Linear(c2, c2) # learnable position embedding
+ self.tr = nn.Sequential(*(TransformerLayer(c2, num_heads) for _ in range(num_layers)))
+ self.c2 = c2
+
+ def forward(self, x):
+ """Forward propagates the input through the bottleneck module."""
+ if self.conv is not None:
+ x = self.conv(x)
+ b, _, w, h = x.shape
+ p = x.flatten(2).permute(2, 0, 1)
+ return self.tr(p + self.linear(p)).permute(1, 2, 0).reshape(b, self.c2, w, h)
+
+
+class MLPBlock(nn.Module):
+
+ def __init__(self, embedding_dim, mlp_dim, act=nn.GELU):
+ super().__init__()
+ self.lin1 = nn.Linear(embedding_dim, mlp_dim)
+ self.lin2 = nn.Linear(mlp_dim, embedding_dim)
+ self.act = act()
+
+ def forward(self, x: torch.Tensor) -> torch.Tensor:
+ return self.lin2(self.act(self.lin1(x)))
+
+
+class MLP(nn.Module):
+ """ Very simple multi-layer perceptron (also called FFN)"""
+
+ def __init__(self, input_dim, hidden_dim, output_dim, num_layers):
+ super().__init__()
+ self.num_layers = num_layers
+ h = [hidden_dim] * (num_layers - 1)
+ self.layers = nn.ModuleList(nn.Linear(n, k) for n, k in zip([input_dim] + h, h + [output_dim]))
+
+ def forward(self, x):
+ for i, layer in enumerate(self.layers):
+ x = F.relu(layer(x)) if i < self.num_layers - 1 else layer(x)
+ return x
+
+
+# From https://github.com/facebookresearch/detectron2/blob/main/detectron2/layers/batch_norm.py # noqa
+# Itself from https://github.com/facebookresearch/ConvNeXt/blob/d1fa8f6fef0a165b27399986cc2bdacc92777e40/models/convnext.py#L119 # noqa
+class LayerNorm2d(nn.Module):
+
+ def __init__(self, num_channels, eps=1e-6):
+ super().__init__()
+ self.weight = nn.Parameter(torch.ones(num_channels))
+ self.bias = nn.Parameter(torch.zeros(num_channels))
+ self.eps = eps
+
+ def forward(self, x):
+ u = x.mean(1, keepdim=True)
+ s = (x - u).pow(2).mean(1, keepdim=True)
+ x = (x - u) / torch.sqrt(s + self.eps)
+ x = self.weight[:, None, None] * x + self.bias[:, None, None]
+ return x
+
+
+class MSDeformAttn(nn.Module):
+ """
+ Original Multi-Scale Deformable Attention Module.
+ https://github.com/fundamentalvision/Deformable-DETR/blob/main/models/ops/modules/ms_deform_attn.py
+ """
+
+ def __init__(self, d_model=256, n_levels=4, n_heads=8, n_points=4):
+ super().__init__()
+ if d_model % n_heads != 0:
+ raise ValueError(f'd_model must be divisible by n_heads, but got {d_model} and {n_heads}')
+ _d_per_head = d_model // n_heads
+ # you'd better set _d_per_head to a power of 2 which is more efficient in our CUDA implementation
+ assert _d_per_head * n_heads == d_model, '`d_model` must be divisible by `n_heads`'
+
+ self.im2col_step = 64
+
+ self.d_model = d_model
+ self.n_levels = n_levels
+ self.n_heads = n_heads
+ self.n_points = n_points
+
+ self.sampling_offsets = nn.Linear(d_model, n_heads * n_levels * n_points * 2)
+ self.attention_weights = nn.Linear(d_model, n_heads * n_levels * n_points)
+ self.value_proj = nn.Linear(d_model, d_model)
+ self.output_proj = nn.Linear(d_model, d_model)
+
+ self._reset_parameters()
+
+ def _reset_parameters(self):
+ constant_(self.sampling_offsets.weight.data, 0.)
+ thetas = torch.arange(self.n_heads, dtype=torch.float32) * (2.0 * math.pi / self.n_heads)
+ grid_init = torch.stack([thetas.cos(), thetas.sin()], -1)
+ grid_init = (grid_init / grid_init.abs().max(-1, keepdim=True)[0]).view(self.n_heads, 1, 1, 2).repeat(
+ 1, self.n_levels, self.n_points, 1)
+ for i in range(self.n_points):
+ grid_init[:, :, i, :] *= i + 1
+ with torch.no_grad():
+ self.sampling_offsets.bias = nn.Parameter(grid_init.view(-1))
+ constant_(self.attention_weights.weight.data, 0.)
+ constant_(self.attention_weights.bias.data, 0.)
+ xavier_uniform_(self.value_proj.weight.data)
+ constant_(self.value_proj.bias.data, 0.)
+ xavier_uniform_(self.output_proj.weight.data)
+ constant_(self.output_proj.bias.data, 0.)
+
+ def forward(self, query, refer_bbox, value, value_shapes, value_mask=None):
+ """
+ https://github.com/PaddlePaddle/PaddleDetection/blob/develop/ppdet/modeling/transformers/deformable_transformer.py
+ Args:
+ query (torch.Tensor): [bs, query_length, C]
+ refer_bbox (torch.Tensor): [bs, query_length, n_levels, 2], range in [0, 1], top-left (0,0),
+ bottom-right (1, 1), including padding area
+ value (torch.Tensor): [bs, value_length, C]
+ value_shapes (List): [n_levels, 2], [(H_0, W_0), (H_1, W_1), ..., (H_{L-1}, W_{L-1})]
+ value_mask (Tensor): [bs, value_length], True for non-padding elements, False for padding elements
+
+ Returns:
+ output (Tensor): [bs, Length_{query}, C]
+ """
+ bs, len_q = query.shape[:2]
+ len_v = value.shape[1]
+ assert sum(s[0] * s[1] for s in value_shapes) == len_v
+
+ value = self.value_proj(value)
+ if value_mask is not None:
+ value = value.masked_fill(value_mask[..., None], float(0))
+ value = value.view(bs, len_v, self.n_heads, self.d_model // self.n_heads)
+ sampling_offsets = self.sampling_offsets(query).view(bs, len_q, self.n_heads, self.n_levels, self.n_points, 2)
+ attention_weights = self.attention_weights(query).view(bs, len_q, self.n_heads, self.n_levels * self.n_points)
+ attention_weights = F.softmax(attention_weights, -1).view(bs, len_q, self.n_heads, self.n_levels, self.n_points)
+ # N, Len_q, n_heads, n_levels, n_points, 2
+ num_points = refer_bbox.shape[-1]
+ if num_points == 2:
+ offset_normalizer = torch.as_tensor(value_shapes, dtype=query.dtype, device=query.device).flip(-1)
+ add = sampling_offsets / offset_normalizer[None, None, None, :, None, :]
+ sampling_locations = refer_bbox[:, :, None, :, None, :] + add
+ elif num_points == 4:
+ add = sampling_offsets / self.n_points * refer_bbox[:, :, None, :, None, 2:] * 0.5
+ sampling_locations = refer_bbox[:, :, None, :, None, :2] + add
+ else:
+ raise ValueError(f'Last dim of reference_points must be 2 or 4, but got {num_points}.')
+ output = multi_scale_deformable_attn_pytorch(value, value_shapes, sampling_locations, attention_weights)
+ output = self.output_proj(output)
+ return output
+
+
+class DeformableTransformerDecoderLayer(nn.Module):
+ """
+ https://github.com/PaddlePaddle/PaddleDetection/blob/develop/ppdet/modeling/transformers/deformable_transformer.py
+ https://github.com/fundamentalvision/Deformable-DETR/blob/main/models/deformable_transformer.py
+ """
+
+ def __init__(self, d_model=256, n_heads=8, d_ffn=1024, dropout=0., act=nn.ReLU(), n_levels=4, n_points=4):
+ super().__init__()
+
+ # self attention
+ self.self_attn = nn.MultiheadAttention(d_model, n_heads, dropout=dropout)
+ self.dropout1 = nn.Dropout(dropout)
+ self.norm1 = nn.LayerNorm(d_model)
+
+ # cross attention
+ self.cross_attn = MSDeformAttn(d_model, n_levels, n_heads, n_points)
+ self.dropout2 = nn.Dropout(dropout)
+ self.norm2 = nn.LayerNorm(d_model)
+
+ # ffn
+ self.linear1 = nn.Linear(d_model, d_ffn)
+ self.act = act
+ self.dropout3 = nn.Dropout(dropout)
+ self.linear2 = nn.Linear(d_ffn, d_model)
+ self.dropout4 = nn.Dropout(dropout)
+ self.norm3 = nn.LayerNorm(d_model)
+
+ @staticmethod
+ def with_pos_embed(tensor, pos):
+ return tensor if pos is None else tensor + pos
+
+ def forward_ffn(self, tgt):
+ tgt2 = self.linear2(self.dropout3(self.act(self.linear1(tgt))))
+ tgt = tgt + self.dropout4(tgt2)
+ tgt = self.norm3(tgt)
+ return tgt
+
+ def forward(self, embed, refer_bbox, feats, shapes, padding_mask=None, attn_mask=None, query_pos=None):
+ # self attention
+ q = k = self.with_pos_embed(embed, query_pos)
+ tgt = self.self_attn(q.transpose(0, 1), k.transpose(0, 1), embed.transpose(0, 1),
+ attn_mask=attn_mask)[0].transpose(0, 1)
+ embed = embed + self.dropout1(tgt)
+ embed = self.norm1(embed)
+
+ # cross attention
+ tgt = self.cross_attn(self.with_pos_embed(embed, query_pos), refer_bbox.unsqueeze(2), feats, shapes,
+ padding_mask)
+ embed = embed + self.dropout2(tgt)
+ embed = self.norm2(embed)
+
+ # ffn
+ embed = self.forward_ffn(embed)
+
+ return embed
+
+
+class DeformableTransformerDecoder(nn.Module):
+ """
+ https://github.com/PaddlePaddle/PaddleDetection/blob/develop/ppdet/modeling/transformers/deformable_transformer.py
+ """
+
+ def __init__(self, hidden_dim, decoder_layer, num_layers, eval_idx=-1):
+ super().__init__()
+ self.layers = _get_clones(decoder_layer, num_layers)
+ self.num_layers = num_layers
+ self.hidden_dim = hidden_dim
+ self.eval_idx = eval_idx if eval_idx >= 0 else num_layers + eval_idx
+
+ def forward(
+ self,
+ embed, # decoder embeddings
+ refer_bbox, # anchor
+ feats, # image features
+ shapes, # feature shapes
+ bbox_head,
+ score_head,
+ pos_mlp,
+ attn_mask=None,
+ padding_mask=None):
+ output = embed
+ dec_bboxes = []
+ dec_cls = []
+ last_refined_bbox = None
+ refer_bbox = refer_bbox.sigmoid()
+ for i, layer in enumerate(self.layers):
+ output = layer(output, refer_bbox, feats, shapes, padding_mask, attn_mask, pos_mlp(refer_bbox))
+
+ # refine bboxes, (bs, num_queries+num_denoising, 4)
+ refined_bbox = torch.sigmoid(bbox_head[i](output) + inverse_sigmoid(refer_bbox))
+
+ if self.training:
+ dec_cls.append(score_head[i](output))
+ if i == 0:
+ dec_bboxes.append(refined_bbox)
+ else:
+ dec_bboxes.append(torch.sigmoid(bbox_head[i](output) + inverse_sigmoid(last_refined_bbox)))
+ elif i == self.eval_idx:
+ dec_cls.append(score_head[i](output))
+ dec_bboxes.append(refined_bbox)
+ break
+
+ last_refined_bbox = refined_bbox
+ refer_bbox = refined_bbox.detach() if self.training else refined_bbox
+
+ return torch.stack(dec_bboxes), torch.stack(dec_cls)
diff --git a/yolov8/ultralytics/nn/modules/utils.py b/yolov8/ultralytics/nn/modules/utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..f8636dc479d242e982fb969a313f7fd786cc3107
--- /dev/null
+++ b/yolov8/ultralytics/nn/modules/utils.py
@@ -0,0 +1,78 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Module utils
+"""
+
+import copy
+import math
+
+import numpy as np
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+from torch.nn.init import uniform_
+
+__all__ = 'multi_scale_deformable_attn_pytorch', 'inverse_sigmoid'
+
+
+def _get_clones(module, n):
+ return nn.ModuleList([copy.deepcopy(module) for _ in range(n)])
+
+
+def bias_init_with_prob(prior_prob=0.01):
+ """initialize conv/fc bias value according to a given probability value."""
+ return float(-np.log((1 - prior_prob) / prior_prob)) # return bias_init
+
+
+def linear_init_(module):
+ bound = 1 / math.sqrt(module.weight.shape[0])
+ uniform_(module.weight, -bound, bound)
+ if hasattr(module, 'bias') and module.bias is not None:
+ uniform_(module.bias, -bound, bound)
+
+
+def inverse_sigmoid(x, eps=1e-5):
+ x = x.clamp(min=0, max=1)
+ x1 = x.clamp(min=eps)
+ x2 = (1 - x).clamp(min=eps)
+ return torch.log(x1 / x2)
+
+
+def multi_scale_deformable_attn_pytorch(value: torch.Tensor, value_spatial_shapes: torch.Tensor,
+ sampling_locations: torch.Tensor,
+ attention_weights: torch.Tensor) -> torch.Tensor:
+ """
+ Multi-scale deformable attention.
+ https://github.com/IDEA-Research/detrex/blob/main/detrex/layers/multi_scale_deform_attn.py
+ """
+
+ bs, _, num_heads, embed_dims = value.shape
+ _, num_queries, num_heads, num_levels, num_points, _ = sampling_locations.shape
+ value_list = value.split([H_ * W_ for H_, W_ in value_spatial_shapes], dim=1)
+ sampling_grids = 2 * sampling_locations - 1
+ sampling_value_list = []
+ for level, (H_, W_) in enumerate(value_spatial_shapes):
+ # bs, H_*W_, num_heads, embed_dims ->
+ # bs, H_*W_, num_heads*embed_dims ->
+ # bs, num_heads*embed_dims, H_*W_ ->
+ # bs*num_heads, embed_dims, H_, W_
+ value_l_ = (value_list[level].flatten(2).transpose(1, 2).reshape(bs * num_heads, embed_dims, H_, W_))
+ # bs, num_queries, num_heads, num_points, 2 ->
+ # bs, num_heads, num_queries, num_points, 2 ->
+ # bs*num_heads, num_queries, num_points, 2
+ sampling_grid_l_ = sampling_grids[:, :, :, level].transpose(1, 2).flatten(0, 1)
+ # bs*num_heads, embed_dims, num_queries, num_points
+ sampling_value_l_ = F.grid_sample(value_l_,
+ sampling_grid_l_,
+ mode='bilinear',
+ padding_mode='zeros',
+ align_corners=False)
+ sampling_value_list.append(sampling_value_l_)
+ # (bs, num_queries, num_heads, num_levels, num_points) ->
+ # (bs, num_heads, num_queries, num_levels, num_points) ->
+ # (bs, num_heads, 1, num_queries, num_levels*num_points)
+ attention_weights = attention_weights.transpose(1, 2).reshape(bs * num_heads, 1, num_queries,
+ num_levels * num_points)
+ output = ((torch.stack(sampling_value_list, dim=-2).flatten(-2) * attention_weights).sum(-1).view(
+ bs, num_heads * embed_dims, num_queries))
+ return output.transpose(1, 2).contiguous()
diff --git a/yolov8/ultralytics/nn/tasks.py b/yolov8/ultralytics/nn/tasks.py
new file mode 100644
index 0000000000000000000000000000000000000000..e05c53f795aa54291aae9b4839970b53a22a15d8
--- /dev/null
+++ b/yolov8/ultralytics/nn/tasks.py
@@ -0,0 +1,780 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+from copy import deepcopy
+from pathlib import Path
+
+import torch
+import torch.nn as nn
+
+from ultralytics.nn.modules import (AIFI, C1, C2, C3, C3TR, SPP, SPPF, Bottleneck, BottleneckCSP, C2f, C3Ghost, C3x,
+ Classify, Concat, Conv, Conv2, ConvTranspose, Detect, DWConv, DWConvTranspose2d,
+ Focus, GhostBottleneck, GhostConv, HGBlock, HGStem, Pose, RepC3, RepConv,
+ RTDETRDecoder, Segment)
+from ultralytics.yolo.utils import DEFAULT_CFG_DICT, DEFAULT_CFG_KEYS, LOGGER, colorstr, emojis, yaml_load
+from ultralytics.yolo.utils.checks import check_requirements, check_suffix, check_yaml
+from ultralytics.yolo.utils.loss import v8ClassificationLoss, v8DetectionLoss, v8PoseLoss, v8SegmentationLoss
+from ultralytics.yolo.utils.plotting import feature_visualization
+from ultralytics.yolo.utils.torch_utils import (fuse_conv_and_bn, fuse_deconv_and_bn, initialize_weights,
+ intersect_dicts, make_divisible, model_info, scale_img, time_sync)
+
+try:
+ import thop
+except ImportError:
+ thop = None
+
+
+class BaseModel(nn.Module):
+ """
+ The BaseModel class serves as a base class for all the models in the Ultralytics YOLO family.
+ """
+
+ def forward(self, x, *args, **kwargs):
+ """
+ Forward pass of the model on a single scale.
+ Wrapper for `_forward_once` method.
+
+ Args:
+ x (torch.Tensor | dict): The input image tensor or a dict including image tensor and gt labels.
+
+ Returns:
+ (torch.Tensor): The output of the network.
+ """
+ if isinstance(x, dict): # for cases of training and validating while training.
+ return self.loss(x, *args, **kwargs)
+ return self.predict(x, *args, **kwargs)
+
+ def predict(self, x, profile=False, visualize=False, augment=False):
+ """
+ Perform a forward pass through the network.
+
+ Args:
+ x (torch.Tensor): The input tensor to the model.
+ profile (bool): Print the computation time of each layer if True, defaults to False.
+ visualize (bool): Save the feature maps of the model if True, defaults to False.
+ augment (bool): Augment image during prediction, defaults to False.
+
+ Returns:
+ (torch.Tensor): The last output of the model.
+ """
+ if augment:
+ return self._predict_augment(x)
+ return self._predict_once(x, profile, visualize)
+
+ def _predict_once(self, x, profile=False, visualize=False):
+ """
+ Perform a forward pass through the network.
+
+ Args:
+ x (torch.Tensor): The input tensor to the model.
+ profile (bool): Print the computation time of each layer if True, defaults to False.
+ visualize (bool): Save the feature maps of the model if True, defaults to False.
+
+ Returns:
+ (torch.Tensor): The last output of the model.
+ """
+ y, dt = [], [] # outputs
+ for m in self.model:
+ if m.f != -1: # if not from previous layer
+ x = y[m.f] if isinstance(m.f, int) else [x if j == -1 else y[j] for j in m.f] # from earlier layers
+ if profile:
+ self._profile_one_layer(m, x, dt)
+ x = m(x) # run
+ y.append(x if m.i in self.save else None) # save output
+ if visualize:
+ feature_visualization(x, m.type, m.i, save_dir=visualize)
+ return x
+
+ def _predict_augment(self, x):
+ """Perform augmentations on input image x and return augmented inference."""
+ LOGGER.warning(
+ f'WARNING ⚠️ {self.__class__.__name__} has not supported augment inference yet! Now using single-scale inference instead.'
+ )
+ return self._predict_once(x)
+
+ def _profile_one_layer(self, m, x, dt):
+ """
+ Profile the computation time and FLOPs of a single layer of the model on a given input.
+ Appends the results to the provided list.
+
+ Args:
+ m (nn.Module): The layer to be profiled.
+ x (torch.Tensor): The input data to the layer.
+ dt (list): A list to store the computation time of the layer.
+
+ Returns:
+ None
+ """
+ c = m == self.model[-1] # is final layer, copy input as inplace fix
+ o = thop.profile(m, inputs=[x.clone() if c else x], verbose=False)[0] / 1E9 * 2 if thop else 0 # FLOPs
+ t = time_sync()
+ for _ in range(10):
+ m(x.clone() if c else x)
+ dt.append((time_sync() - t) * 100)
+ if m == self.model[0]:
+ LOGGER.info(f"{'time (ms)':>10s} {'GFLOPs':>10s} {'params':>10s} module")
+ LOGGER.info(f'{dt[-1]:10.2f} {o:10.2f} {m.np:10.0f} {m.type}')
+ if c:
+ LOGGER.info(f"{sum(dt):10.2f} {'-':>10s} {'-':>10s} Total")
+
+ def fuse(self, verbose=True):
+ """
+ Fuse the `Conv2d()` and `BatchNorm2d()` layers of the model into a single layer, in order to improve the
+ computation efficiency.
+
+ Returns:
+ (nn.Module): The fused model is returned.
+ """
+ if not self.is_fused():
+ for m in self.model.modules():
+ if isinstance(m, (Conv, Conv2, DWConv)) and hasattr(m, 'bn'):
+ if isinstance(m, Conv2):
+ m.fuse_convs()
+ m.conv = fuse_conv_and_bn(m.conv, m.bn) # update conv
+ delattr(m, 'bn') # remove batchnorm
+ m.forward = m.forward_fuse # update forward
+ if isinstance(m, ConvTranspose) and hasattr(m, 'bn'):
+ m.conv_transpose = fuse_deconv_and_bn(m.conv_transpose, m.bn)
+ delattr(m, 'bn') # remove batchnorm
+ m.forward = m.forward_fuse # update forward
+ if isinstance(m, RepConv):
+ m.fuse_convs()
+ m.forward = m.forward_fuse # update forward
+ self.info(verbose=verbose)
+
+ return self
+
+ def is_fused(self, thresh=10):
+ """
+ Check if the model has less than a certain threshold of BatchNorm layers.
+
+ Args:
+ thresh (int, optional): The threshold number of BatchNorm layers. Default is 10.
+
+ Returns:
+ (bool): True if the number of BatchNorm layers in the model is less than the threshold, False otherwise.
+ """
+ bn = tuple(v for k, v in nn.__dict__.items() if 'Norm' in k) # normalization layers, i.e. BatchNorm2d()
+ return sum(isinstance(v, bn) for v in self.modules()) < thresh # True if < 'thresh' BatchNorm layers in model
+
+ def info(self, detailed=False, verbose=True, imgsz=640):
+ """
+ Prints model information
+
+ Args:
+ verbose (bool): if True, prints out the model information. Defaults to False
+ imgsz (int): the size of the image that the model will be trained on. Defaults to 640
+ """
+ return model_info(self, detailed=detailed, verbose=verbose, imgsz=imgsz)
+
+ def _apply(self, fn):
+ """
+ `_apply()` is a function that applies a function to all the tensors in the model that are not
+ parameters or registered buffers
+
+ Args:
+ fn: the function to apply to the model
+
+ Returns:
+ A model that is a Detect() object.
+ """
+ self = super()._apply(fn)
+ m = self.model[-1] # Detect()
+ if isinstance(m, (Detect, Segment)):
+ m.stride = fn(m.stride)
+ m.anchors = fn(m.anchors)
+ m.strides = fn(m.strides)
+ return self
+
+ def load(self, weights, verbose=True):
+ """Load the weights into the model.
+
+ Args:
+ weights (dict | torch.nn.Module): The pre-trained weights to be loaded.
+ verbose (bool, optional): Whether to log the transfer progress. Defaults to True.
+ """
+ model = weights['model'] if isinstance(weights, dict) else weights # torchvision models are not dicts
+ csd = model.float().state_dict() # checkpoint state_dict as FP32
+ csd = intersect_dicts(csd, self.state_dict()) # intersect
+ self.load_state_dict(csd, strict=False) # load
+ if verbose:
+ LOGGER.info(f'Transferred {len(csd)}/{len(self.model.state_dict())} items from pretrained weights')
+
+ def loss(self, batch, preds=None):
+ """
+ Compute loss
+
+ Args:
+ batch (dict): Batch to compute loss on
+ preds (torch.Tensor | List[torch.Tensor]): Predictions.
+ """
+ if not hasattr(self, 'criterion'):
+ self.criterion = self.init_criterion()
+
+ preds = self.forward(batch['img']) if preds is None else preds
+ return self.criterion(preds, batch)
+
+ def init_criterion(self):
+ raise NotImplementedError('compute_loss() needs to be implemented by task heads')
+
+
+class DetectionModel(BaseModel):
+ """YOLOv8 detection model."""
+
+ def __init__(self, cfg='yolov8n.yaml', ch=3, nc=None, verbose=True): # model, input channels, number of classes
+ super().__init__()
+ self.yaml = cfg if isinstance(cfg, dict) else yaml_model_load(cfg) # cfg dict
+
+ # Define model
+ ch = self.yaml['ch'] = self.yaml.get('ch', ch) # input channels
+ if nc and nc != self.yaml['nc']:
+ LOGGER.info(f"Overriding model.yaml nc={self.yaml['nc']} with nc={nc}")
+ self.yaml['nc'] = nc # override yaml value
+ self.model, self.save = parse_model(deepcopy(self.yaml), ch=ch, verbose=verbose) # model, savelist
+ self.names = {i: f'{i}' for i in range(self.yaml['nc'])} # default names dict
+ self.inplace = self.yaml.get('inplace', True)
+
+ # Build strides
+ m = self.model[-1] # Detect()
+ if isinstance(m, (Detect, Segment, Pose)):
+ s = 256 # 2x min stride
+ m.inplace = self.inplace
+ forward = lambda x: self.forward(x)[0] if isinstance(m, (Segment, Pose)) else self.forward(x)
+ m.stride = torch.tensor([s / x.shape[-2] for x in forward(torch.zeros(1, ch, s, s))]) # forward
+ self.stride = m.stride
+ m.bias_init() # only run once
+ else:
+ self.stride = torch.Tensor([32]) # default stride for i.e. RTDETR
+
+ # Init weights, biases
+ initialize_weights(self)
+ if verbose:
+ self.info()
+ LOGGER.info('')
+
+ def _predict_augment(self, x):
+ """Perform augmentations on input image x and return augmented inference and train outputs."""
+ img_size = x.shape[-2:] # height, width
+ s = [1, 0.83, 0.67] # scales
+ f = [None, 3, None] # flips (2-ud, 3-lr)
+ y = [] # outputs
+ for si, fi in zip(s, f):
+ xi = scale_img(x.flip(fi) if fi else x, si, gs=int(self.stride.max()))
+ yi = super().predict(xi)[0] # forward
+ # cv2.imwrite(f'img_{si}.jpg', 255 * xi[0].cpu().numpy().transpose((1, 2, 0))[:, :, ::-1]) # save
+ yi = self._descale_pred(yi, fi, si, img_size)
+ y.append(yi)
+ y = self._clip_augmented(y) # clip augmented tails
+ return torch.cat(y, -1), None # augmented inference, train
+
+ @staticmethod
+ def _descale_pred(p, flips, scale, img_size, dim=1):
+ """De-scale predictions following augmented inference (inverse operation)."""
+ p[:, :4] /= scale # de-scale
+ x, y, wh, cls = p.split((1, 1, 2, p.shape[dim] - 4), dim)
+ if flips == 2:
+ y = img_size[0] - y # de-flip ud
+ elif flips == 3:
+ x = img_size[1] - x # de-flip lr
+ return torch.cat((x, y, wh, cls), dim)
+
+ def _clip_augmented(self, y):
+ """Clip YOLOv5 augmented inference tails."""
+ nl = self.model[-1].nl # number of detection layers (P3-P5)
+ g = sum(4 ** x for x in range(nl)) # grid points
+ e = 1 # exclude layer count
+ i = (y[0].shape[-1] // g) * sum(4 ** x for x in range(e)) # indices
+ y[0] = y[0][..., :-i] # large
+ i = (y[-1].shape[-1] // g) * sum(4 ** (nl - 1 - x) for x in range(e)) # indices
+ y[-1] = y[-1][..., i:] # small
+ return y
+
+ def init_criterion(self):
+ return v8DetectionLoss(self)
+
+
+class SegmentationModel(DetectionModel):
+ """YOLOv8 segmentation model."""
+
+ def __init__(self, cfg='yolov8n-seg.yaml', ch=3, nc=None, verbose=True):
+ """Initialize YOLOv8 segmentation model with given config and parameters."""
+ super().__init__(cfg=cfg, ch=ch, nc=nc, verbose=verbose)
+
+ def init_criterion(self):
+ return v8SegmentationLoss(self)
+
+ def _predict_augment(self, x):
+ """Perform augmentations on input image x and return augmented inference."""
+ LOGGER.warning(
+ f'WARNING ⚠️ {self.__class__.__name__} has not supported augment inference yet! Now using single-scale inference instead.'
+ )
+ return self._predict_once(x)
+
+
+class PoseModel(DetectionModel):
+ """YOLOv8 pose model."""
+
+ def __init__(self, cfg='yolov8n-pose.yaml', ch=3, nc=None, data_kpt_shape=(None, None), verbose=True):
+ """Initialize YOLOv8 Pose model."""
+ if not isinstance(cfg, dict):
+ cfg = yaml_model_load(cfg) # load model YAML
+ if any(data_kpt_shape) and list(data_kpt_shape) != list(cfg['kpt_shape']):
+ LOGGER.info(f"Overriding model.yaml kpt_shape={cfg['kpt_shape']} with kpt_shape={data_kpt_shape}")
+ cfg['kpt_shape'] = data_kpt_shape
+ super().__init__(cfg=cfg, ch=ch, nc=nc, verbose=verbose)
+
+ def init_criterion(self):
+ return v8PoseLoss(self)
+
+ def _predict_augment(self, x):
+ """Perform augmentations on input image x and return augmented inference."""
+ LOGGER.warning(
+ f'WARNING ⚠️ {self.__class__.__name__} has not supported augment inference yet! Now using single-scale inference instead.'
+ )
+ return self._predict_once(x)
+
+
+class ClassificationModel(BaseModel):
+ """YOLOv8 classification model."""
+
+ def __init__(self,
+ cfg=None,
+ model=None,
+ ch=3,
+ nc=None,
+ cutoff=10,
+ verbose=True): # yaml, model, channels, number of classes, cutoff index, verbose flag
+ super().__init__()
+ self._from_detection_model(model, nc, cutoff) if model is not None else self._from_yaml(cfg, ch, nc, verbose)
+
+ def _from_detection_model(self, model, nc=1000, cutoff=10):
+ """Create a YOLOv5 classification model from a YOLOv5 detection model."""
+ from ultralytics.nn.autobackend import AutoBackend
+ if isinstance(model, AutoBackend):
+ model = model.model # unwrap DetectMultiBackend
+ model.model = model.model[:cutoff] # backbone
+ m = model.model[-1] # last layer
+ ch = m.conv.in_channels if hasattr(m, 'conv') else m.cv1.conv.in_channels # ch into module
+ c = Classify(ch, nc) # Classify()
+ c.i, c.f, c.type = m.i, m.f, 'models.common.Classify' # index, from, type
+ model.model[-1] = c # replace
+ self.model = model.model
+ self.stride = model.stride
+ self.save = []
+ self.nc = nc
+
+ def _from_yaml(self, cfg, ch, nc, verbose):
+ """Set YOLOv8 model configurations and define the model architecture."""
+ self.yaml = cfg if isinstance(cfg, dict) else yaml_model_load(cfg) # cfg dict
+
+ # Define model
+ ch = self.yaml['ch'] = self.yaml.get('ch', ch) # input channels
+ if nc and nc != self.yaml['nc']:
+ LOGGER.info(f"Overriding model.yaml nc={self.yaml['nc']} with nc={nc}")
+ self.yaml['nc'] = nc # override yaml value
+ elif not nc and not self.yaml.get('nc', None):
+ raise ValueError('nc not specified. Must specify nc in model.yaml or function arguments.')
+ self.model, self.save = parse_model(deepcopy(self.yaml), ch=ch, verbose=verbose) # model, savelist
+ self.stride = torch.Tensor([1]) # no stride constraints
+ self.names = {i: f'{i}' for i in range(self.yaml['nc'])} # default names dict
+ self.info()
+
+ @staticmethod
+ def reshape_outputs(model, nc):
+ """Update a TorchVision classification model to class count 'n' if required."""
+ name, m = list((model.model if hasattr(model, 'model') else model).named_children())[-1] # last module
+ if isinstance(m, Classify): # YOLO Classify() head
+ if m.linear.out_features != nc:
+ m.linear = nn.Linear(m.linear.in_features, nc)
+ elif isinstance(m, nn.Linear): # ResNet, EfficientNet
+ if m.out_features != nc:
+ setattr(model, name, nn.Linear(m.in_features, nc))
+ elif isinstance(m, nn.Sequential):
+ types = [type(x) for x in m]
+ if nn.Linear in types:
+ i = types.index(nn.Linear) # nn.Linear index
+ if m[i].out_features != nc:
+ m[i] = nn.Linear(m[i].in_features, nc)
+ elif nn.Conv2d in types:
+ i = types.index(nn.Conv2d) # nn.Conv2d index
+ if m[i].out_channels != nc:
+ m[i] = nn.Conv2d(m[i].in_channels, nc, m[i].kernel_size, m[i].stride, bias=m[i].bias is not None)
+
+ def init_criterion(self):
+ """Compute the classification loss between predictions and true labels."""
+ return v8ClassificationLoss()
+
+
+class RTDETRDetectionModel(DetectionModel):
+
+ def __init__(self, cfg='rtdetr-l.yaml', ch=3, nc=None, verbose=True):
+ super().__init__(cfg=cfg, ch=ch, nc=nc, verbose=verbose)
+
+ def init_criterion(self):
+ """Compute the classification loss between predictions and true labels."""
+ from ultralytics.vit.utils.loss import RTDETRDetectionLoss
+
+ return RTDETRDetectionLoss(nc=self.nc, use_vfl=True)
+
+ def loss(self, batch, preds=None):
+ if not hasattr(self, 'criterion'):
+ self.criterion = self.init_criterion()
+
+ img = batch['img']
+ # NOTE: preprocess gt_bbox and gt_labels to list.
+ bs = len(img)
+ batch_idx = batch['batch_idx']
+ gt_groups = [(batch_idx == i).sum().item() for i in range(bs)]
+ targets = {
+ 'cls': batch['cls'].to(img.device, dtype=torch.long).view(-1),
+ 'bboxes': batch['bboxes'].to(device=img.device),
+ 'batch_idx': batch_idx.to(img.device, dtype=torch.long).view(-1),
+ 'gt_groups': gt_groups}
+
+ preds = self.predict(img, batch=targets) if preds is None else preds
+ dec_bboxes, dec_scores, enc_bboxes, enc_scores, dn_meta = preds if self.training else preds[1]
+ if dn_meta is None:
+ dn_bboxes, dn_scores = None, None
+ else:
+ dn_bboxes, dec_bboxes = torch.split(dec_bboxes, dn_meta['dn_num_split'], dim=2)
+ dn_scores, dec_scores = torch.split(dec_scores, dn_meta['dn_num_split'], dim=2)
+
+ dec_bboxes = torch.cat([enc_bboxes.unsqueeze(0), dec_bboxes]) # (7, bs, 300, 4)
+ dec_scores = torch.cat([enc_scores.unsqueeze(0), dec_scores])
+
+ loss = self.criterion((dec_bboxes, dec_scores),
+ targets,
+ dn_bboxes=dn_bboxes,
+ dn_scores=dn_scores,
+ dn_meta=dn_meta)
+ # NOTE: There are like 12 losses in RTDETR, backward with all losses but only show the main three losses.
+ return sum(loss.values()), torch.as_tensor([loss[k].detach() for k in ['loss_giou', 'loss_class', 'loss_bbox']],
+ device=img.device)
+
+ def predict(self, x, profile=False, visualize=False, batch=None, augment=False):
+ """
+ Perform a forward pass through the network.
+
+ Args:
+ x (torch.Tensor): The input tensor to the model
+ profile (bool): Print the computation time of each layer if True, defaults to False.
+ visualize (bool): Save the feature maps of the model if True, defaults to False
+ batch (dict): A dict including gt boxes and labels from dataloader.
+
+ Returns:
+ (torch.Tensor): The last output of the model.
+ """
+ y, dt = [], [] # outputs
+ for m in self.model[:-1]: # except the head part
+ if m.f != -1: # if not from previous layer
+ x = y[m.f] if isinstance(m.f, int) else [x if j == -1 else y[j] for j in m.f] # from earlier layers
+ if profile:
+ self._profile_one_layer(m, x, dt)
+ x = m(x) # run
+ y.append(x if m.i in self.save else None) # save output
+ if visualize:
+ feature_visualization(x, m.type, m.i, save_dir=visualize)
+ head = self.model[-1]
+ x = head([y[j] for j in head.f], batch) # head inference
+ return x
+
+
+class Ensemble(nn.ModuleList):
+ """Ensemble of models."""
+
+ def __init__(self):
+ """Initialize an ensemble of models."""
+ super().__init__()
+
+ def forward(self, x, augment=False, profile=False, visualize=False):
+ """Function generates the YOLOv5 network's final layer."""
+ y = [module(x, augment, profile, visualize)[0] for module in self]
+ # y = torch.stack(y).max(0)[0] # max ensemble
+ # y = torch.stack(y).mean(0) # mean ensemble
+ y = torch.cat(y, 2) # nms ensemble, y shape(B, HW, C)
+ return y, None # inference, train output
+
+
+# Functions ------------------------------------------------------------------------------------------------------------
+
+
+def torch_safe_load(weight):
+ """
+ This function attempts to load a PyTorch model with the torch.load() function. If a ModuleNotFoundError is raised,
+ it catches the error, logs a warning message, and attempts to install the missing module via the
+ check_requirements() function. After installation, the function again attempts to load the model using torch.load().
+
+ Args:
+ weight (str): The file path of the PyTorch model.
+
+ Returns:
+ (dict): The loaded PyTorch model.
+ """
+ from ultralytics.yolo.utils.downloads import attempt_download_asset
+
+ check_suffix(file=weight, suffix='.pt')
+ file = attempt_download_asset(weight) # search online if missing locally
+ try:
+ return torch.load(file, map_location='cpu'), file # load
+ except ModuleNotFoundError as e: # e.name is missing module name
+ if e.name == 'models':
+ raise TypeError(
+ emojis(f'ERROR ❌️ {weight} appears to be an Ultralytics YOLOv5 model originally trained '
+ f'with https://github.com/ultralytics/yolov5.\nThis model is NOT forwards compatible with '
+ f'YOLOv8 at https://github.com/ultralytics/ultralytics.'
+ f"\nRecommend fixes are to train a new model using the latest 'ultralytics' package or to "
+ f"run a command with an official YOLOv8 model, i.e. 'yolo predict model=yolov8n.pt'")) from e
+ LOGGER.warning(f"WARNING ⚠️ {weight} appears to require '{e.name}', which is not in ultralytics requirements."
+ f"\nAutoInstall will run now for '{e.name}' but this feature will be removed in the future."
+ f"\nRecommend fixes are to train a new model using the latest 'ultralytics' package or to "
+ f"run a command with an official YOLOv8 model, i.e. 'yolo predict model=yolov8n.pt'")
+ check_requirements(e.name) # install missing module
+
+ return torch.load(file, map_location='cpu'), file # load
+
+
+def attempt_load_weights(weights, device=None, inplace=True, fuse=False):
+ """Loads an ensemble of models weights=[a,b,c] or a single model weights=[a] or weights=a."""
+
+ ensemble = Ensemble()
+ for w in weights if isinstance(weights, list) else [weights]:
+ ckpt, w = torch_safe_load(w) # load ckpt
+ args = {**DEFAULT_CFG_DICT, **ckpt['train_args']} if 'train_args' in ckpt else None # combined args
+ model = (ckpt.get('ema') or ckpt['model']).to(device).float() # FP32 model
+
+ # Model compatibility updates
+ model.args = args # attach args to model
+ model.pt_path = w # attach *.pt file path to model
+ model.task = guess_model_task(model)
+ if not hasattr(model, 'stride'):
+ model.stride = torch.tensor([32.])
+
+ # Append
+ ensemble.append(model.fuse().eval() if fuse and hasattr(model, 'fuse') else model.eval()) # model in eval mode
+
+ # Module compatibility updates
+ for m in ensemble.modules():
+ t = type(m)
+ if t in (nn.Hardswish, nn.LeakyReLU, nn.ReLU, nn.ReLU6, nn.SiLU, Detect, Segment):
+ m.inplace = inplace # torch 1.7.0 compatibility
+ elif t is nn.Upsample and not hasattr(m, 'recompute_scale_factor'):
+ m.recompute_scale_factor = None # torch 1.11.0 compatibility
+
+ # Return model
+ if len(ensemble) == 1:
+ return ensemble[-1]
+
+ # Return ensemble
+ LOGGER.info(f'Ensemble created with {weights}\n')
+ for k in 'names', 'nc', 'yaml':
+ setattr(ensemble, k, getattr(ensemble[0], k))
+ ensemble.stride = ensemble[torch.argmax(torch.tensor([m.stride.max() for m in ensemble])).int()].stride
+ assert all(ensemble[0].nc == m.nc for m in ensemble), f'Models differ in class counts {[m.nc for m in ensemble]}'
+ return ensemble
+
+
+def attempt_load_one_weight(weight, device=None, inplace=True, fuse=False):
+ """Loads a single model weights."""
+ ckpt, weight = torch_safe_load(weight) # load ckpt
+ args = {**DEFAULT_CFG_DICT, **(ckpt.get('train_args', {}))} # combine model and default args, preferring model args
+ model = (ckpt.get('ema') or ckpt['model']).to(device).float() # FP32 model
+
+ # Model compatibility updates
+ model.args = {k: v for k, v in args.items() if k in DEFAULT_CFG_KEYS} # attach args to model
+ model.pt_path = weight # attach *.pt file path to model
+ model.task = guess_model_task(model)
+ if not hasattr(model, 'stride'):
+ model.stride = torch.tensor([32.])
+
+ model = model.fuse().eval() if fuse and hasattr(model, 'fuse') else model.eval() # model in eval mode
+
+ # Module compatibility updates
+ for m in model.modules():
+ t = type(m)
+ if t in (nn.Hardswish, nn.LeakyReLU, nn.ReLU, nn.ReLU6, nn.SiLU, Detect, Segment):
+ m.inplace = inplace # torch 1.7.0 compatibility
+ elif t is nn.Upsample and not hasattr(m, 'recompute_scale_factor'):
+ m.recompute_scale_factor = None # torch 1.11.0 compatibility
+
+ # Return model and ckpt
+ return model, ckpt
+
+
+def parse_model(d, ch, verbose=True): # model_dict, input_channels(3)
+ # Parse a YOLO model.yaml dictionary into a PyTorch model
+ import ast
+
+ # Args
+ max_channels = float('inf')
+ nc, act, scales = (d.get(x) for x in ('nc', 'activation', 'scales'))
+ depth, width, kpt_shape = (d.get(x, 1.0) for x in ('depth_multiple', 'width_multiple', 'kpt_shape'))
+ if scales:
+ scale = d.get('scale')
+ if not scale:
+ scale = tuple(scales.keys())[0]
+ LOGGER.warning(f"WARNING ⚠️ no model scale passed. Assuming scale='{scale}'.")
+ depth, width, max_channels = scales[scale]
+
+ if act:
+ Conv.default_act = eval(act) # redefine default activation, i.e. Conv.default_act = nn.SiLU()
+ if verbose:
+ LOGGER.info(f"{colorstr('activation:')} {act}") # print
+
+ if verbose:
+ LOGGER.info(f"\n{'':>3}{'from':>20}{'n':>3}{'params':>10} {'module':<45}{'arguments':<30}")
+ ch = [ch]
+ layers, save, c2 = [], [], ch[-1] # layers, savelist, ch out
+ for i, (f, n, m, args) in enumerate(d['backbone'] + d['head']): # from, number, module, args
+ m = getattr(torch.nn, m[3:]) if 'nn.' in m else globals()[m] # get module
+ for j, a in enumerate(args):
+ if isinstance(a, str):
+ with contextlib.suppress(ValueError):
+ args[j] = locals()[a] if a in locals() else ast.literal_eval(a)
+
+ n = n_ = max(round(n * depth), 1) if n > 1 else n # depth gain
+ if m in (Classify, Conv, ConvTranspose, GhostConv, Bottleneck, GhostBottleneck, SPP, SPPF, DWConv, Focus,
+ BottleneckCSP, C1, C2, C2f, C3, C3TR, C3Ghost, nn.ConvTranspose2d, DWConvTranspose2d, C3x, RepC3):
+ c1, c2 = ch[f], args[0]
+ if c2 != nc: # if c2 not equal to number of classes (i.e. for Classify() output)
+ c2 = make_divisible(min(c2, max_channels) * width, 8)
+
+ args = [c1, c2, *args[1:]]
+ if m in (BottleneckCSP, C1, C2, C2f, C3, C3TR, C3Ghost, C3x, RepC3):
+ args.insert(2, n) # number of repeats
+ n = 1
+ elif m is AIFI:
+ args = [ch[f], *args]
+ elif m in (HGStem, HGBlock):
+ c1, cm, c2 = ch[f], args[0], args[1]
+ args = [c1, cm, c2, *args[2:]]
+ if m is HGBlock:
+ args.insert(4, n) # number of repeats
+ n = 1
+
+ elif m is nn.BatchNorm2d:
+ args = [ch[f]]
+ elif m is Concat:
+ c2 = sum(ch[x] for x in f)
+ elif m in (Detect, Segment, Pose, RTDETRDecoder):
+ args.append([ch[x] for x in f])
+ if m is Segment:
+ args[2] = make_divisible(min(args[2], max_channels) * width, 8)
+ else:
+ c2 = ch[f]
+
+ m_ = nn.Sequential(*(m(*args) for _ in range(n))) if n > 1 else m(*args) # module
+ t = str(m)[8:-2].replace('__main__.', '') # module type
+ m.np = sum(x.numel() for x in m_.parameters()) # number params
+ m_.i, m_.f, m_.type = i, f, t # attach index, 'from' index, type
+ if verbose:
+ LOGGER.info(f'{i:>3}{str(f):>20}{n_:>3}{m.np:10.0f} {t:<45}{str(args):<30}') # print
+ save.extend(x % i for x in ([f] if isinstance(f, int) else f) if x != -1) # append to savelist
+ layers.append(m_)
+ if i == 0:
+ ch = []
+ ch.append(c2)
+ return nn.Sequential(*layers), sorted(save)
+
+
+def yaml_model_load(path):
+ """Load a YOLOv8 model from a YAML file."""
+ import re
+
+ path = Path(path)
+ if path.stem in (f'yolov{d}{x}6' for x in 'nsmlx' for d in (5, 8)):
+ new_stem = re.sub(r'(\d+)([nslmx])6(.+)?$', r'\1\2-p6\3', path.stem)
+ LOGGER.warning(f'WARNING ⚠️ Ultralytics YOLO P6 models now use -p6 suffix. Renaming {path.stem} to {new_stem}.')
+ path = path.with_name(new_stem + path.suffix)
+
+ unified_path = re.sub(r'(\d+)([nslmx])(.+)?$', r'\1\3', str(path)) # i.e. yolov8x.yaml -> yolov8.yaml
+ yaml_file = check_yaml(unified_path, hard=False) or check_yaml(path)
+ d = yaml_load(yaml_file) # model dict
+ d['scale'] = guess_model_scale(path)
+ d['yaml_file'] = str(path)
+ return d
+
+
+def guess_model_scale(model_path):
+ """
+ Takes a path to a YOLO model's YAML file as input and extracts the size character of the model's scale.
+ The function uses regular expression matching to find the pattern of the model scale in the YAML file name,
+ which is denoted by n, s, m, l, or x. The function returns the size character of the model scale as a string.
+
+ Args:
+ model_path (str | Path): The path to the YOLO model's YAML file.
+
+ Returns:
+ (str): The size character of the model's scale, which can be n, s, m, l, or x.
+ """
+ with contextlib.suppress(AttributeError):
+ import re
+ return re.search(r'yolov\d+([nslmx])', Path(model_path).stem).group(1) # n, s, m, l, or x
+ return ''
+
+
+def guess_model_task(model):
+ """
+ Guess the task of a PyTorch model from its architecture or configuration.
+
+ Args:
+ model (nn.Module | dict): PyTorch model or model configuration in YAML format.
+
+ Returns:
+ (str): Task of the model ('detect', 'segment', 'classify', 'pose').
+
+ Raises:
+ SyntaxError: If the task of the model could not be determined.
+ """
+
+ def cfg2task(cfg):
+ """Guess from YAML dictionary."""
+ m = cfg['head'][-1][-2].lower() # output module name
+ if m in ('classify', 'classifier', 'cls', 'fc'):
+ return 'classify'
+ if m == 'detect':
+ return 'detect'
+ if m == 'segment':
+ return 'segment'
+ if m == 'pose':
+ return 'pose'
+
+ # Guess from model cfg
+ if isinstance(model, dict):
+ with contextlib.suppress(Exception):
+ return cfg2task(model)
+
+ # Guess from PyTorch model
+ if isinstance(model, nn.Module): # PyTorch model
+ for x in 'model.args', 'model.model.args', 'model.model.model.args':
+ with contextlib.suppress(Exception):
+ return eval(x)['task']
+ for x in 'model.yaml', 'model.model.yaml', 'model.model.model.yaml':
+ with contextlib.suppress(Exception):
+ return cfg2task(eval(x))
+
+ for m in model.modules():
+ if isinstance(m, Detect):
+ return 'detect'
+ elif isinstance(m, Segment):
+ return 'segment'
+ elif isinstance(m, Classify):
+ return 'classify'
+ elif isinstance(m, Pose):
+ return 'pose'
+
+ # Guess from model filename
+ if isinstance(model, (str, Path)):
+ model = Path(model)
+ if '-seg' in model.stem or 'segment' in model.parts:
+ return 'segment'
+ elif '-cls' in model.stem or 'classify' in model.parts:
+ return 'classify'
+ elif '-pose' in model.stem or 'pose' in model.parts:
+ return 'pose'
+ elif 'detect' in model.parts:
+ return 'detect'
+
+ # Unable to determine task from model
+ LOGGER.warning("WARNING ⚠️ Unable to automatically guess model task, assuming 'task=detect'. "
+ "Explicitly define task for your model, i.e. 'task=detect', 'segment', 'classify', or 'pose'.")
+ return 'detect' # assume detect
diff --git a/yolov8/ultralytics/tracker/README.md b/yolov8/ultralytics/tracker/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..26ec0c3246b3e2caf8c499fb476d7da6ede60386
--- /dev/null
+++ b/yolov8/ultralytics/tracker/README.md
@@ -0,0 +1,86 @@
+# Tracker
+
+## Supported Trackers
+
+- [x] ByteTracker
+- [x] BoT-SORT
+
+## Usage
+
+### python interface:
+
+You can use the Python interface to track objects using the YOLO model.
+
+```python
+from ultralytics import YOLO
+
+model = YOLO("yolov8n.pt") # or a segmentation model .i.e yolov8n-seg.pt
+model.track(
+ source="video/streams",
+ stream=True,
+ tracker="botsort.yaml", # or 'bytetrack.yaml'
+ show=True,
+)
+```
+
+You can get the IDs of the tracked objects using the following code:
+
+```python
+from ultralytics import YOLO
+
+model = YOLO("yolov8n.pt")
+
+for result in model.track(source="video.mp4"):
+ print(
+ result.boxes.id.cpu().numpy().astype(int)
+ ) # this will print the IDs of the tracked objects in the frame
+```
+
+If you want to use the tracker with a folder of images or when you loop on the video frames, you should use the `persist` parameter to tell the model that these frames are related to each other so the IDs will be fixed for the same objects. Otherwise, the IDs will be different in each frame because in each loop, the model creates a new object for tracking, but the `persist` parameter makes it use the same object for tracking.
+
+```python
+import cv2
+from ultralytics import YOLO
+
+cap = cv2.VideoCapture("video.mp4")
+model = YOLO("yolov8n.pt")
+while True:
+ ret, frame = cap.read()
+ if not ret:
+ break
+ results = model.track(frame, persist=True)
+ boxes = results[0].boxes.xyxy.cpu().numpy().astype(int)
+ ids = results[0].boxes.id.cpu().numpy().astype(int)
+ for box, id in zip(boxes, ids):
+ cv2.rectangle(frame, (box[0], box[1]), (box[2], box[3]), (0, 255, 0), 2)
+ cv2.putText(
+ frame,
+ f"Id {id}",
+ (box[0], box[1]),
+ cv2.FONT_HERSHEY_SIMPLEX,
+ 1,
+ (0, 0, 255),
+ 2,
+ )
+ cv2.imshow("frame", frame)
+ if cv2.waitKey(1) & 0xFF == ord("q"):
+ break
+```
+
+## Change tracker parameters
+
+You can change the tracker parameters by eding the `tracker.yaml` file which is located in the ultralytics/tracker/cfg folder.
+
+## Command Line Interface (CLI)
+
+You can also use the command line interface to track objects using the YOLO model.
+
+```bash
+yolo detect track source=... tracker=...
+yolo segment track source=... tracker=...
+yolo pose track source=... tracker=...
+```
+
+By default, trackers will use the configuration in `ultralytics/tracker/cfg`.
+We also support using a modified tracker config file. Please refer to the tracker config files
+in `ultralytics/tracker/cfg`.
diff --git a/yolov8/ultralytics/tracker/__init__.py b/yolov8/ultralytics/tracker/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..13d3903e763e1fbb21c1064f6bffdafcca08e9e6
--- /dev/null
+++ b/yolov8/ultralytics/tracker/__init__.py
@@ -0,0 +1,6 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .track import register_tracker
+from .trackers import BOTSORT, BYTETracker
+
+__all__ = 'register_tracker', 'BOTSORT', 'BYTETracker' # allow simpler import
diff --git a/yolov8/ultralytics/tracker/cfg/botsort.yaml b/yolov8/ultralytics/tracker/cfg/botsort.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..d4947c6079cb5628d80a0134a9f72bc5c3ab360e
--- /dev/null
+++ b/yolov8/ultralytics/tracker/cfg/botsort.yaml
@@ -0,0 +1,18 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# Default YOLO tracker settings for BoT-SORT tracker https://github.com/NirAharon/BoT-SORT
+
+tracker_type: botsort # tracker type, ['botsort', 'bytetrack']
+track_high_thresh: 0.5 # threshold for the first association
+track_low_thresh: 0.1 # threshold for the second association
+new_track_thresh: 0.6 # threshold for init new track if the detection does not match any tracks
+track_buffer: 30 # buffer to calculate the time when to remove tracks
+match_thresh: 0.8 # threshold for matching tracks
+# min_box_area: 10 # threshold for min box areas(for tracker evaluation, not used for now)
+# mot20: False # for tracker evaluation(not used for now)
+
+# BoT-SORT settings
+cmc_method: sparseOptFlow # method of global motion compensation
+# ReID model related thresh (not supported yet)
+proximity_thresh: 0.5
+appearance_thresh: 0.25
+with_reid: False
diff --git a/yolov8/ultralytics/tracker/cfg/bytetrack.yaml b/yolov8/ultralytics/tracker/cfg/bytetrack.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..5060f92622a9873dbdd0596e29172277c1cdbb99
--- /dev/null
+++ b/yolov8/ultralytics/tracker/cfg/bytetrack.yaml
@@ -0,0 +1,11 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# Default YOLO tracker settings for ByteTrack tracker https://github.com/ifzhang/ByteTrack
+
+tracker_type: bytetrack # tracker type, ['botsort', 'bytetrack']
+track_high_thresh: 0.5 # threshold for the first association
+track_low_thresh: 0.1 # threshold for the second association
+new_track_thresh: 0.6 # threshold for init new track if the detection does not match any tracks
+track_buffer: 30 # buffer to calculate the time when to remove tracks
+match_thresh: 0.8 # threshold for matching tracks
+# min_box_area: 10 # threshold for min box areas(for tracker evaluation, not used for now)
+# mot20: False # for tracker evaluation(not used for now)
diff --git a/yolov8/ultralytics/tracker/track.py b/yolov8/ultralytics/tracker/track.py
new file mode 100644
index 0000000000000000000000000000000000000000..d08abfc7ad94465d5c7283df4c960580065ffec9
--- /dev/null
+++ b/yolov8/ultralytics/tracker/track.py
@@ -0,0 +1,65 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from functools import partial
+
+import torch
+
+from ultralytics.yolo.utils import IterableSimpleNamespace, yaml_load
+from ultralytics.yolo.utils.checks import check_yaml
+
+from .trackers import BOTSORT, BYTETracker
+
+TRACKER_MAP = {'bytetrack': BYTETracker, 'botsort': BOTSORT}
+
+
+def on_predict_start(predictor, persist=False):
+ """
+ Initialize trackers for object tracking during prediction.
+
+ Args:
+ predictor (object): The predictor object to initialize trackers for.
+ persist (bool, optional): Whether to persist the trackers if they already exist. Defaults to False.
+
+ Raises:
+ AssertionError: If the tracker_type is not 'bytetrack' or 'botsort'.
+ """
+ if hasattr(predictor, 'trackers') and persist:
+ return
+ tracker = check_yaml(predictor.args.tracker)
+ cfg = IterableSimpleNamespace(**yaml_load(tracker))
+ assert cfg.tracker_type in ['bytetrack', 'botsort'], \
+ f"Only support 'bytetrack' and 'botsort' for now, but got '{cfg.tracker_type}'"
+ trackers = []
+ for _ in range(predictor.dataset.bs):
+ tracker = TRACKER_MAP[cfg.tracker_type](args=cfg, frame_rate=30)
+ trackers.append(tracker)
+ predictor.trackers = trackers
+
+
+def on_predict_postprocess_end(predictor):
+ """Postprocess detected boxes and update with object tracking."""
+ bs = predictor.dataset.bs
+ im0s = predictor.batch[1]
+ for i in range(bs):
+ det = predictor.results[i].boxes.cpu().numpy()
+ if len(det) == 0:
+ continue
+ tracks = predictor.trackers[i].update(det, im0s[i])
+ if len(tracks) == 0:
+ continue
+ idx = tracks[:, -1].astype(int)
+ predictor.results[i] = predictor.results[i][idx]
+ predictor.results[i].update(boxes=torch.as_tensor(tracks[:, :-1]))
+
+
+def register_tracker(model, persist):
+ """
+ Register tracking callbacks to the model for object tracking during prediction.
+
+ Args:
+ model (object): The model object to register tracking callbacks for.
+ persist (bool): Whether to persist the trackers if they already exist.
+
+ """
+ model.add_callback('on_predict_start', partial(on_predict_start, persist=persist))
+ model.add_callback('on_predict_postprocess_end', on_predict_postprocess_end)
diff --git a/yolov8/ultralytics/tracker/trackers/__init__.py b/yolov8/ultralytics/tracker/trackers/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..a0fd890e95dfd40c1d025e8d8ed97495b9c33c4e
--- /dev/null
+++ b/yolov8/ultralytics/tracker/trackers/__init__.py
@@ -0,0 +1,6 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .bot_sort import BOTSORT
+from .byte_tracker import BYTETracker
+
+__all__ = 'BOTSORT', 'BYTETracker' # allow simpler import
diff --git a/yolov8/ultralytics/tracker/trackers/basetrack.py b/yolov8/ultralytics/tracker/trackers/basetrack.py
new file mode 100644
index 0000000000000000000000000000000000000000..3c7b0f707508d92699b9a2f5c3d4500006e9faa5
--- /dev/null
+++ b/yolov8/ultralytics/tracker/trackers/basetrack.py
@@ -0,0 +1,71 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from collections import OrderedDict
+
+import numpy as np
+
+
+class TrackState:
+ """Enumeration of possible object tracking states."""
+
+ New = 0
+ Tracked = 1
+ Lost = 2
+ Removed = 3
+
+
+class BaseTrack:
+ """Base class for object tracking, handling basic track attributes and operations."""
+
+ _count = 0
+
+ track_id = 0
+ is_activated = False
+ state = TrackState.New
+
+ history = OrderedDict()
+ features = []
+ curr_feature = None
+ score = 0
+ start_frame = 0
+ frame_id = 0
+ time_since_update = 0
+
+ # Multi-camera
+ location = (np.inf, np.inf)
+
+ @property
+ def end_frame(self):
+ """Return the last frame ID of the track."""
+ return self.frame_id
+
+ @staticmethod
+ def next_id():
+ """Increment and return the global track ID counter."""
+ BaseTrack._count += 1
+ return BaseTrack._count
+
+ def activate(self, *args):
+ """Activate the track with the provided arguments."""
+ raise NotImplementedError
+
+ def predict(self):
+ """Predict the next state of the track."""
+ raise NotImplementedError
+
+ def update(self, *args, **kwargs):
+ """Update the track with new observations."""
+ raise NotImplementedError
+
+ def mark_lost(self):
+ """Mark the track as lost."""
+ self.state = TrackState.Lost
+
+ def mark_removed(self):
+ """Mark the track as removed."""
+ self.state = TrackState.Removed
+
+ @staticmethod
+ def reset_id():
+ """Reset the global track ID counter."""
+ BaseTrack._count = 0
diff --git a/yolov8/ultralytics/tracker/trackers/bot_sort.py b/yolov8/ultralytics/tracker/trackers/bot_sort.py
new file mode 100644
index 0000000000000000000000000000000000000000..10e88682d91c72b3b7671ee00e19fd42a4cd6c64
--- /dev/null
+++ b/yolov8/ultralytics/tracker/trackers/bot_sort.py
@@ -0,0 +1,148 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from collections import deque
+
+import numpy as np
+
+from ..utils import matching
+from ..utils.gmc import GMC
+from ..utils.kalman_filter import KalmanFilterXYWH
+from .basetrack import TrackState
+from .byte_tracker import BYTETracker, STrack
+
+
+class BOTrack(STrack):
+ shared_kalman = KalmanFilterXYWH()
+
+ def __init__(self, tlwh, score, cls, feat=None, feat_history=50):
+ """Initialize YOLOv8 object with temporal parameters, such as feature history, alpha and current features."""
+ super().__init__(tlwh, score, cls)
+
+ self.smooth_feat = None
+ self.curr_feat = None
+ if feat is not None:
+ self.update_features(feat)
+ self.features = deque([], maxlen=feat_history)
+ self.alpha = 0.9
+
+ def update_features(self, feat):
+ """Update features vector and smooth it using exponential moving average."""
+ feat /= np.linalg.norm(feat)
+ self.curr_feat = feat
+ if self.smooth_feat is None:
+ self.smooth_feat = feat
+ else:
+ self.smooth_feat = self.alpha * self.smooth_feat + (1 - self.alpha) * feat
+ self.features.append(feat)
+ self.smooth_feat /= np.linalg.norm(self.smooth_feat)
+
+ def predict(self):
+ """Predicts the mean and covariance using Kalman filter."""
+ mean_state = self.mean.copy()
+ if self.state != TrackState.Tracked:
+ mean_state[6] = 0
+ mean_state[7] = 0
+
+ self.mean, self.covariance = self.kalman_filter.predict(mean_state, self.covariance)
+
+ def re_activate(self, new_track, frame_id, new_id=False):
+ """Reactivates a track with updated features and optionally assigns a new ID."""
+ if new_track.curr_feat is not None:
+ self.update_features(new_track.curr_feat)
+ super().re_activate(new_track, frame_id, new_id)
+
+ def update(self, new_track, frame_id):
+ """Update the YOLOv8 instance with new track and frame ID."""
+ if new_track.curr_feat is not None:
+ self.update_features(new_track.curr_feat)
+ super().update(new_track, frame_id)
+
+ @property
+ def tlwh(self):
+ """Get current position in bounding box format `(top left x, top left y,
+ width, height)`.
+ """
+ if self.mean is None:
+ return self._tlwh.copy()
+ ret = self.mean[:4].copy()
+ ret[:2] -= ret[2:] / 2
+ return ret
+
+ @staticmethod
+ def multi_predict(stracks):
+ """Predicts the mean and covariance of multiple object tracks using shared Kalman filter."""
+ if len(stracks) <= 0:
+ return
+ multi_mean = np.asarray([st.mean.copy() for st in stracks])
+ multi_covariance = np.asarray([st.covariance for st in stracks])
+ for i, st in enumerate(stracks):
+ if st.state != TrackState.Tracked:
+ multi_mean[i][6] = 0
+ multi_mean[i][7] = 0
+ multi_mean, multi_covariance = BOTrack.shared_kalman.multi_predict(multi_mean, multi_covariance)
+ for i, (mean, cov) in enumerate(zip(multi_mean, multi_covariance)):
+ stracks[i].mean = mean
+ stracks[i].covariance = cov
+
+ def convert_coords(self, tlwh):
+ """Converts Top-Left-Width-Height bounding box coordinates to X-Y-Width-Height format."""
+ return self.tlwh_to_xywh(tlwh)
+
+ @staticmethod
+ def tlwh_to_xywh(tlwh):
+ """Convert bounding box to format `(center x, center y, width,
+ height)`.
+ """
+ ret = np.asarray(tlwh).copy()
+ ret[:2] += ret[2:] / 2
+ return ret
+
+
+class BOTSORT(BYTETracker):
+
+ def __init__(self, args, frame_rate=30):
+ """Initialize YOLOv8 object with ReID module and GMC algorithm."""
+ super().__init__(args, frame_rate)
+ # ReID module
+ self.proximity_thresh = args.proximity_thresh
+ self.appearance_thresh = args.appearance_thresh
+
+ if args.with_reid:
+ # Haven't supported BoT-SORT(reid) yet
+ self.encoder = None
+ # self.gmc = GMC(method=args.cmc_method, verbose=[args.name, args.ablation])
+ self.gmc = GMC(method=args.cmc_method)
+
+ def get_kalmanfilter(self):
+ """Returns an instance of KalmanFilterXYWH for object tracking."""
+ return KalmanFilterXYWH()
+
+ def init_track(self, dets, scores, cls, img=None):
+ """Initialize track with detections, scores, and classes."""
+ if len(dets) == 0:
+ return []
+ if self.args.with_reid and self.encoder is not None:
+ features_keep = self.encoder.inference(img, dets)
+ return [BOTrack(xyxy, s, c, f) for (xyxy, s, c, f) in zip(dets, scores, cls, features_keep)] # detections
+ else:
+ return [BOTrack(xyxy, s, c) for (xyxy, s, c) in zip(dets, scores, cls)] # detections
+
+ def get_dists(self, tracks, detections):
+ """Get distances between tracks and detections using IoU and (optionally) ReID embeddings."""
+ dists = matching.iou_distance(tracks, detections)
+ dists_mask = (dists > self.proximity_thresh)
+
+ # TODO: mot20
+ # if not self.args.mot20:
+ dists = matching.fuse_score(dists, detections)
+
+ if self.args.with_reid and self.encoder is not None:
+ emb_dists = matching.embedding_distance(tracks, detections) / 2.0
+ emb_dists[emb_dists > self.appearance_thresh] = 1.0
+ emb_dists[dists_mask] = 1.0
+ dists = np.minimum(dists, emb_dists)
+ return dists
+
+ def multi_predict(self, tracks):
+ """Predict and track multiple objects with YOLOv8 model."""
+ BOTrack.multi_predict(tracks)
diff --git a/yolov8/ultralytics/tracker/trackers/byte_tracker.py b/yolov8/ultralytics/tracker/trackers/byte_tracker.py
new file mode 100644
index 0000000000000000000000000000000000000000..6034cdc9dc6f29de331f2767ba83fe8bd7c617a5
--- /dev/null
+++ b/yolov8/ultralytics/tracker/trackers/byte_tracker.py
@@ -0,0 +1,364 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import numpy as np
+
+from ..utils import matching
+from ..utils.kalman_filter import KalmanFilterXYAH
+from .basetrack import BaseTrack, TrackState
+
+
+class STrack(BaseTrack):
+ shared_kalman = KalmanFilterXYAH()
+
+ def __init__(self, tlwh, score, cls):
+ """wait activate."""
+ self._tlwh = np.asarray(self.tlbr_to_tlwh(tlwh[:-1]), dtype=np.float32)
+ self.kalman_filter = None
+ self.mean, self.covariance = None, None
+ self.is_activated = False
+
+ self.score = score
+ self.tracklet_len = 0
+ self.cls = cls
+ self.idx = tlwh[-1]
+
+ def predict(self):
+ """Predicts mean and covariance using Kalman filter."""
+ mean_state = self.mean.copy()
+ if self.state != TrackState.Tracked:
+ mean_state[7] = 0
+ self.mean, self.covariance = self.kalman_filter.predict(mean_state, self.covariance)
+
+ @staticmethod
+ def multi_predict(stracks):
+ """Perform multi-object predictive tracking using Kalman filter for given stracks."""
+ if len(stracks) <= 0:
+ return
+ multi_mean = np.asarray([st.mean.copy() for st in stracks])
+ multi_covariance = np.asarray([st.covariance for st in stracks])
+ for i, st in enumerate(stracks):
+ if st.state != TrackState.Tracked:
+ multi_mean[i][7] = 0
+ multi_mean, multi_covariance = STrack.shared_kalman.multi_predict(multi_mean, multi_covariance)
+ for i, (mean, cov) in enumerate(zip(multi_mean, multi_covariance)):
+ stracks[i].mean = mean
+ stracks[i].covariance = cov
+
+ @staticmethod
+ def multi_gmc(stracks, H=np.eye(2, 3)):
+ """Update state tracks positions and covariances using a homography matrix."""
+ if len(stracks) > 0:
+ multi_mean = np.asarray([st.mean.copy() for st in stracks])
+ multi_covariance = np.asarray([st.covariance for st in stracks])
+
+ R = H[:2, :2]
+ R8x8 = np.kron(np.eye(4, dtype=float), R)
+ t = H[:2, 2]
+
+ for i, (mean, cov) in enumerate(zip(multi_mean, multi_covariance)):
+ mean = R8x8.dot(mean)
+ mean[:2] += t
+ cov = R8x8.dot(cov).dot(R8x8.transpose())
+
+ stracks[i].mean = mean
+ stracks[i].covariance = cov
+
+ def activate(self, kalman_filter, frame_id):
+ """Start a new tracklet."""
+ self.kalman_filter = kalman_filter
+ self.track_id = self.next_id()
+ self.mean, self.covariance = self.kalman_filter.initiate(self.convert_coords(self._tlwh))
+
+ self.tracklet_len = 0
+ self.state = TrackState.Tracked
+ if frame_id == 1:
+ self.is_activated = True
+ self.frame_id = frame_id
+ self.start_frame = frame_id
+
+ def re_activate(self, new_track, frame_id, new_id=False):
+ """Reactivates a previously lost track with a new detection."""
+ self.mean, self.covariance = self.kalman_filter.update(self.mean, self.covariance,
+ self.convert_coords(new_track.tlwh))
+ self.tracklet_len = 0
+ self.state = TrackState.Tracked
+ self.is_activated = True
+ self.frame_id = frame_id
+ if new_id:
+ self.track_id = self.next_id()
+ self.score = new_track.score
+ self.cls = new_track.cls
+ self.idx = new_track.idx
+
+ def update(self, new_track, frame_id):
+ """
+ Update a matched track
+ :type new_track: STrack
+ :type frame_id: int
+ :return:
+ """
+ self.frame_id = frame_id
+ self.tracklet_len += 1
+
+ new_tlwh = new_track.tlwh
+ self.mean, self.covariance = self.kalman_filter.update(self.mean, self.covariance,
+ self.convert_coords(new_tlwh))
+ self.state = TrackState.Tracked
+ self.is_activated = True
+
+ self.score = new_track.score
+ self.cls = new_track.cls
+ self.idx = new_track.idx
+
+ def convert_coords(self, tlwh):
+ """Convert a bounding box's top-left-width-height format to its x-y-angle-height equivalent."""
+ return self.tlwh_to_xyah(tlwh)
+
+ @property
+ def tlwh(self):
+ """Get current position in bounding box format `(top left x, top left y,
+ width, height)`.
+ """
+ if self.mean is None:
+ return self._tlwh.copy()
+ ret = self.mean[:4].copy()
+ ret[2] *= ret[3]
+ ret[:2] -= ret[2:] / 2
+ return ret
+
+ @property
+ def tlbr(self):
+ """Convert bounding box to format `(min x, min y, max x, max y)`, i.e.,
+ `(top left, bottom right)`.
+ """
+ ret = self.tlwh.copy()
+ ret[2:] += ret[:2]
+ return ret
+
+ @staticmethod
+ def tlwh_to_xyah(tlwh):
+ """Convert bounding box to format `(center x, center y, aspect ratio,
+ height)`, where the aspect ratio is `width / height`.
+ """
+ ret = np.asarray(tlwh).copy()
+ ret[:2] += ret[2:] / 2
+ ret[2] /= ret[3]
+ return ret
+
+ @staticmethod
+ def tlbr_to_tlwh(tlbr):
+ """Converts top-left bottom-right format to top-left width height format."""
+ ret = np.asarray(tlbr).copy()
+ ret[2:] -= ret[:2]
+ return ret
+
+ @staticmethod
+ def tlwh_to_tlbr(tlwh):
+ """Converts tlwh bounding box format to tlbr format."""
+ ret = np.asarray(tlwh).copy()
+ ret[2:] += ret[:2]
+ return ret
+
+ def __repr__(self):
+ """Return a string representation of the BYTETracker object with start and end frames and track ID."""
+ return f'OT_{self.track_id}_({self.start_frame}-{self.end_frame})'
+
+
+class BYTETracker:
+
+ def __init__(self, args, frame_rate=30):
+ """Initialize a YOLOv8 object to track objects with given arguments and frame rate."""
+ self.tracked_stracks = [] # type: list[STrack]
+ self.lost_stracks = [] # type: list[STrack]
+ self.removed_stracks = [] # type: list[STrack]
+
+ self.frame_id = 0
+ self.args = args
+ self.max_time_lost = int(frame_rate / 30.0 * args.track_buffer)
+ self.kalman_filter = self.get_kalmanfilter()
+ self.reset_id()
+
+ def update(self, results, img=None):
+ """Updates object tracker with new detections and returns tracked object bounding boxes."""
+ self.frame_id += 1
+ activated_stracks = []
+ refind_stracks = []
+ lost_stracks = []
+ removed_stracks = []
+
+ scores = results.conf
+ bboxes = results.xyxy
+ # Add index
+ bboxes = np.concatenate([bboxes, np.arange(len(bboxes)).reshape(-1, 1)], axis=-1)
+ cls = results.cls
+
+ remain_inds = scores > self.args.track_high_thresh
+ inds_low = scores > self.args.track_low_thresh
+ inds_high = scores < self.args.track_high_thresh
+
+ inds_second = np.logical_and(inds_low, inds_high)
+ dets_second = bboxes[inds_second]
+ dets = bboxes[remain_inds]
+ scores_keep = scores[remain_inds]
+ scores_second = scores[inds_second]
+ cls_keep = cls[remain_inds]
+ cls_second = cls[inds_second]
+
+ detections = self.init_track(dets, scores_keep, cls_keep, img)
+ # Add newly detected tracklets to tracked_stracks
+ unconfirmed = []
+ tracked_stracks = [] # type: list[STrack]
+ for track in self.tracked_stracks:
+ if not track.is_activated:
+ unconfirmed.append(track)
+ else:
+ tracked_stracks.append(track)
+ # Step 2: First association, with high score detection boxes
+ strack_pool = self.joint_stracks(tracked_stracks, self.lost_stracks)
+ # Predict the current location with KF
+ self.multi_predict(strack_pool)
+ if hasattr(self, 'gmc') and img is not None:
+ warp = self.gmc.apply(img, dets)
+ STrack.multi_gmc(strack_pool, warp)
+ STrack.multi_gmc(unconfirmed, warp)
+
+ dists = self.get_dists(strack_pool, detections)
+ matches, u_track, u_detection = matching.linear_assignment(dists, thresh=self.args.match_thresh)
+
+ for itracked, idet in matches:
+ track = strack_pool[itracked]
+ det = detections[idet]
+ if track.state == TrackState.Tracked:
+ track.update(det, self.frame_id)
+ activated_stracks.append(track)
+ else:
+ track.re_activate(det, self.frame_id, new_id=False)
+ refind_stracks.append(track)
+ # Step 3: Second association, with low score detection boxes
+ # association the untrack to the low score detections
+ detections_second = self.init_track(dets_second, scores_second, cls_second, img)
+ r_tracked_stracks = [strack_pool[i] for i in u_track if strack_pool[i].state == TrackState.Tracked]
+ # TODO
+ dists = matching.iou_distance(r_tracked_stracks, detections_second)
+ matches, u_track, u_detection_second = matching.linear_assignment(dists, thresh=0.5)
+ for itracked, idet in matches:
+ track = r_tracked_stracks[itracked]
+ det = detections_second[idet]
+ if track.state == TrackState.Tracked:
+ track.update(det, self.frame_id)
+ activated_stracks.append(track)
+ else:
+ track.re_activate(det, self.frame_id, new_id=False)
+ refind_stracks.append(track)
+
+ for it in u_track:
+ track = r_tracked_stracks[it]
+ if track.state != TrackState.Lost:
+ track.mark_lost()
+ lost_stracks.append(track)
+ # Deal with unconfirmed tracks, usually tracks with only one beginning frame
+ detections = [detections[i] for i in u_detection]
+ dists = self.get_dists(unconfirmed, detections)
+ matches, u_unconfirmed, u_detection = matching.linear_assignment(dists, thresh=0.7)
+ for itracked, idet in matches:
+ unconfirmed[itracked].update(detections[idet], self.frame_id)
+ activated_stracks.append(unconfirmed[itracked])
+ for it in u_unconfirmed:
+ track = unconfirmed[it]
+ track.mark_removed()
+ removed_stracks.append(track)
+ # Step 4: Init new stracks
+ for inew in u_detection:
+ track = detections[inew]
+ if track.score < self.args.new_track_thresh:
+ continue
+ track.activate(self.kalman_filter, self.frame_id)
+ activated_stracks.append(track)
+ # Step 5: Update state
+ for track in self.lost_stracks:
+ if self.frame_id - track.end_frame > self.max_time_lost:
+ track.mark_removed()
+ removed_stracks.append(track)
+
+ self.tracked_stracks = [t for t in self.tracked_stracks if t.state == TrackState.Tracked]
+ self.tracked_stracks = self.joint_stracks(self.tracked_stracks, activated_stracks)
+ self.tracked_stracks = self.joint_stracks(self.tracked_stracks, refind_stracks)
+ self.lost_stracks = self.sub_stracks(self.lost_stracks, self.tracked_stracks)
+ self.lost_stracks.extend(lost_stracks)
+ self.lost_stracks = self.sub_stracks(self.lost_stracks, self.removed_stracks)
+ self.tracked_stracks, self.lost_stracks = self.remove_duplicate_stracks(self.tracked_stracks, self.lost_stracks)
+ self.removed_stracks.extend(removed_stracks)
+ if len(self.removed_stracks) > 1000:
+ self.removed_stracks = self.removed_stracks[-999:] # clip remove stracks to 1000 maximum
+ return np.asarray(
+ [x.tlbr.tolist() + [x.track_id, x.score, x.cls, x.idx] for x in self.tracked_stracks if x.is_activated],
+ dtype=np.float32)
+
+ def get_kalmanfilter(self):
+ """Returns a Kalman filter object for tracking bounding boxes."""
+ return KalmanFilterXYAH()
+
+ def init_track(self, dets, scores, cls, img=None):
+ """Initialize object tracking with detections and scores using STrack algorithm."""
+ return [STrack(xyxy, s, c) for (xyxy, s, c) in zip(dets, scores, cls)] if len(dets) else [] # detections
+
+ def get_dists(self, tracks, detections):
+ """Calculates the distance between tracks and detections using IOU and fuses scores."""
+ dists = matching.iou_distance(tracks, detections)
+ # TODO: mot20
+ # if not self.args.mot20:
+ dists = matching.fuse_score(dists, detections)
+ return dists
+
+ def multi_predict(self, tracks):
+ """Returns the predicted tracks using the YOLOv8 network."""
+ STrack.multi_predict(tracks)
+
+ def reset_id(self):
+ """Resets the ID counter of STrack."""
+ STrack.reset_id()
+
+ @staticmethod
+ def joint_stracks(tlista, tlistb):
+ """Combine two lists of stracks into a single one."""
+ exists = {}
+ res = []
+ for t in tlista:
+ exists[t.track_id] = 1
+ res.append(t)
+ for t in tlistb:
+ tid = t.track_id
+ if not exists.get(tid, 0):
+ exists[tid] = 1
+ res.append(t)
+ return res
+
+ @staticmethod
+ def sub_stracks(tlista, tlistb):
+ """DEPRECATED CODE in https://github.com/ultralytics/ultralytics/pull/1890/
+ stracks = {t.track_id: t for t in tlista}
+ for t in tlistb:
+ tid = t.track_id
+ if stracks.get(tid, 0):
+ del stracks[tid]
+ return list(stracks.values())
+ """
+ track_ids_b = {t.track_id for t in tlistb}
+ return [t for t in tlista if t.track_id not in track_ids_b]
+
+ @staticmethod
+ def remove_duplicate_stracks(stracksa, stracksb):
+ """Remove duplicate stracks with non-maximum IOU distance."""
+ pdist = matching.iou_distance(stracksa, stracksb)
+ pairs = np.where(pdist < 0.15)
+ dupa, dupb = [], []
+ for p, q in zip(*pairs):
+ timep = stracksa[p].frame_id - stracksa[p].start_frame
+ timeq = stracksb[q].frame_id - stracksb[q].start_frame
+ if timep > timeq:
+ dupb.append(q)
+ else:
+ dupa.append(p)
+ resa = [t for i, t in enumerate(stracksa) if i not in dupa]
+ resb = [t for i, t in enumerate(stracksb) if i not in dupb]
+ return resa, resb
diff --git a/yolov8/ultralytics/tracker/utils/__init__.py b/yolov8/ultralytics/tracker/utils/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/yolov8/ultralytics/tracker/utils/gmc.py b/yolov8/ultralytics/tracker/utils/gmc.py
new file mode 100644
index 0000000000000000000000000000000000000000..a5c910d3b55153deefd7b3de1bb53285594153e1
--- /dev/null
+++ b/yolov8/ultralytics/tracker/utils/gmc.py
@@ -0,0 +1,319 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import copy
+
+import cv2
+import numpy as np
+
+from ultralytics.yolo.utils import LOGGER
+
+
+class GMC:
+
+ def __init__(self, method='sparseOptFlow', downscale=2, verbose=None):
+ """Initialize a video tracker with specified parameters."""
+ super().__init__()
+
+ self.method = method
+ self.downscale = max(1, int(downscale))
+
+ if self.method == 'orb':
+ self.detector = cv2.FastFeatureDetector_create(20)
+ self.extractor = cv2.ORB_create()
+ self.matcher = cv2.BFMatcher(cv2.NORM_HAMMING)
+
+ elif self.method == 'sift':
+ self.detector = cv2.SIFT_create(nOctaveLayers=3, contrastThreshold=0.02, edgeThreshold=20)
+ self.extractor = cv2.SIFT_create(nOctaveLayers=3, contrastThreshold=0.02, edgeThreshold=20)
+ self.matcher = cv2.BFMatcher(cv2.NORM_L2)
+
+ elif self.method == 'ecc':
+ number_of_iterations = 5000
+ termination_eps = 1e-6
+ self.warp_mode = cv2.MOTION_EUCLIDEAN
+ self.criteria = (cv2.TERM_CRITERIA_EPS | cv2.TERM_CRITERIA_COUNT, number_of_iterations, termination_eps)
+
+ elif self.method == 'sparseOptFlow':
+ self.feature_params = dict(maxCorners=1000,
+ qualityLevel=0.01,
+ minDistance=1,
+ blockSize=3,
+ useHarrisDetector=False,
+ k=0.04)
+ # self.gmc_file = open('GMC_results.txt', 'w')
+
+ elif self.method in ['file', 'files']:
+ seqName = verbose[0]
+ ablation = verbose[1]
+ if ablation:
+ filePath = r'tracker/GMC_files/MOT17_ablation'
+ else:
+ filePath = r'tracker/GMC_files/MOTChallenge'
+
+ if '-FRCNN' in seqName:
+ seqName = seqName[:-6]
+ elif '-DPM' in seqName or '-SDP' in seqName:
+ seqName = seqName[:-4]
+ self.gmcFile = open(f'{filePath}/GMC-{seqName}.txt')
+
+ if self.gmcFile is None:
+ raise ValueError(f'Error: Unable to open GMC file in directory:{filePath}')
+ elif self.method in ['none', 'None']:
+ self.method = 'none'
+ else:
+ raise ValueError(f'Error: Unknown CMC method:{method}')
+
+ self.prevFrame = None
+ self.prevKeyPoints = None
+ self.prevDescriptors = None
+
+ self.initializedFirstFrame = False
+
+ def apply(self, raw_frame, detections=None):
+ """Apply object detection on a raw frame using specified method."""
+ if self.method in ['orb', 'sift']:
+ return self.applyFeatures(raw_frame, detections)
+ elif self.method == 'ecc':
+ return self.applyEcc(raw_frame, detections)
+ elif self.method == 'sparseOptFlow':
+ return self.applySparseOptFlow(raw_frame, detections)
+ elif self.method == 'file':
+ return self.applyFile(raw_frame, detections)
+ elif self.method == 'none':
+ return np.eye(2, 3)
+ else:
+ return np.eye(2, 3)
+
+ def applyEcc(self, raw_frame, detections=None):
+ """Initialize."""
+ height, width, _ = raw_frame.shape
+ frame = cv2.cvtColor(raw_frame, cv2.COLOR_BGR2GRAY)
+ H = np.eye(2, 3, dtype=np.float32)
+
+ # Downscale image (TODO: consider using pyramids)
+ if self.downscale > 1.0:
+ frame = cv2.GaussianBlur(frame, (3, 3), 1.5)
+ frame = cv2.resize(frame, (width // self.downscale, height // self.downscale))
+ width = width // self.downscale
+ height = height // self.downscale
+
+ # Handle first frame
+ if not self.initializedFirstFrame:
+ # Initialize data
+ self.prevFrame = frame.copy()
+
+ # Initialization done
+ self.initializedFirstFrame = True
+
+ return H
+
+ # Run the ECC algorithm. The results are stored in warp_matrix.
+ # (cc, H) = cv2.findTransformECC(self.prevFrame, frame, H, self.warp_mode, self.criteria)
+ try:
+ (cc, H) = cv2.findTransformECC(self.prevFrame, frame, H, self.warp_mode, self.criteria, None, 1)
+ except Exception as e:
+ LOGGER.warning(f'WARNING: find transform failed. Set warp as identity {e}')
+
+ return H
+
+ def applyFeatures(self, raw_frame, detections=None):
+ """Initialize."""
+ height, width, _ = raw_frame.shape
+ frame = cv2.cvtColor(raw_frame, cv2.COLOR_BGR2GRAY)
+ H = np.eye(2, 3)
+
+ # Downscale image (TODO: consider using pyramids)
+ if self.downscale > 1.0:
+ # frame = cv2.GaussianBlur(frame, (3, 3), 1.5)
+ frame = cv2.resize(frame, (width // self.downscale, height // self.downscale))
+ width = width // self.downscale
+ height = height // self.downscale
+
+ # Find the keypoints
+ mask = np.zeros_like(frame)
+ # mask[int(0.05 * height): int(0.95 * height), int(0.05 * width): int(0.95 * width)] = 255
+ mask[int(0.02 * height):int(0.98 * height), int(0.02 * width):int(0.98 * width)] = 255
+ if detections is not None:
+ for det in detections:
+ tlbr = (det[:4] / self.downscale).astype(np.int_)
+ mask[tlbr[1]:tlbr[3], tlbr[0]:tlbr[2]] = 0
+
+ keypoints = self.detector.detect(frame, mask)
+
+ # Compute the descriptors
+ keypoints, descriptors = self.extractor.compute(frame, keypoints)
+
+ # Handle first frame
+ if not self.initializedFirstFrame:
+ # Initialize data
+ self.prevFrame = frame.copy()
+ self.prevKeyPoints = copy.copy(keypoints)
+ self.prevDescriptors = copy.copy(descriptors)
+
+ # Initialization done
+ self.initializedFirstFrame = True
+
+ return H
+
+ # Match descriptors.
+ knnMatches = self.matcher.knnMatch(self.prevDescriptors, descriptors, 2)
+
+ # Filtered matches based on smallest spatial distance
+ matches = []
+ spatialDistances = []
+
+ maxSpatialDistance = 0.25 * np.array([width, height])
+
+ # Handle empty matches case
+ if len(knnMatches) == 0:
+ # Store to next iteration
+ self.prevFrame = frame.copy()
+ self.prevKeyPoints = copy.copy(keypoints)
+ self.prevDescriptors = copy.copy(descriptors)
+
+ return H
+
+ for m, n in knnMatches:
+ if m.distance < 0.9 * n.distance:
+ prevKeyPointLocation = self.prevKeyPoints[m.queryIdx].pt
+ currKeyPointLocation = keypoints[m.trainIdx].pt
+
+ spatialDistance = (prevKeyPointLocation[0] - currKeyPointLocation[0],
+ prevKeyPointLocation[1] - currKeyPointLocation[1])
+
+ if (np.abs(spatialDistance[0]) < maxSpatialDistance[0]) and \
+ (np.abs(spatialDistance[1]) < maxSpatialDistance[1]):
+ spatialDistances.append(spatialDistance)
+ matches.append(m)
+
+ meanSpatialDistances = np.mean(spatialDistances, 0)
+ stdSpatialDistances = np.std(spatialDistances, 0)
+
+ inliers = (spatialDistances - meanSpatialDistances) < 2.5 * stdSpatialDistances
+
+ goodMatches = []
+ prevPoints = []
+ currPoints = []
+ for i in range(len(matches)):
+ if inliers[i, 0] and inliers[i, 1]:
+ goodMatches.append(matches[i])
+ prevPoints.append(self.prevKeyPoints[matches[i].queryIdx].pt)
+ currPoints.append(keypoints[matches[i].trainIdx].pt)
+
+ prevPoints = np.array(prevPoints)
+ currPoints = np.array(currPoints)
+
+ # Draw the keypoint matches on the output image
+ # if False:
+ # import matplotlib.pyplot as plt
+ # matches_img = np.hstack((self.prevFrame, frame))
+ # matches_img = cv2.cvtColor(matches_img, cv2.COLOR_GRAY2BGR)
+ # W = np.size(self.prevFrame, 1)
+ # for m in goodMatches:
+ # prev_pt = np.array(self.prevKeyPoints[m.queryIdx].pt, dtype=np.int_)
+ # curr_pt = np.array(keypoints[m.trainIdx].pt, dtype=np.int_)
+ # curr_pt[0] += W
+ # color = np.random.randint(0, 255, 3)
+ # color = (int(color[0]), int(color[1]), int(color[2]))
+ #
+ # matches_img = cv2.line(matches_img, prev_pt, curr_pt, tuple(color), 1, cv2.LINE_AA)
+ # matches_img = cv2.circle(matches_img, prev_pt, 2, tuple(color), -1)
+ # matches_img = cv2.circle(matches_img, curr_pt, 2, tuple(color), -1)
+ #
+ # plt.figure()
+ # plt.imshow(matches_img)
+ # plt.show()
+
+ # Find rigid matrix
+ if (np.size(prevPoints, 0) > 4) and (np.size(prevPoints, 0) == np.size(prevPoints, 0)):
+ H, inliers = cv2.estimateAffinePartial2D(prevPoints, currPoints, cv2.RANSAC)
+
+ # Handle downscale
+ if self.downscale > 1.0:
+ H[0, 2] *= self.downscale
+ H[1, 2] *= self.downscale
+ else:
+ LOGGER.warning('WARNING: not enough matching points')
+
+ # Store to next iteration
+ self.prevFrame = frame.copy()
+ self.prevKeyPoints = copy.copy(keypoints)
+ self.prevDescriptors = copy.copy(descriptors)
+
+ return H
+
+ def applySparseOptFlow(self, raw_frame, detections=None):
+ """Initialize."""
+ # t0 = time.time()
+ height, width, _ = raw_frame.shape
+ frame = cv2.cvtColor(raw_frame, cv2.COLOR_BGR2GRAY)
+ H = np.eye(2, 3)
+
+ # Downscale image
+ if self.downscale > 1.0:
+ # frame = cv2.GaussianBlur(frame, (3, 3), 1.5)
+ frame = cv2.resize(frame, (width // self.downscale, height // self.downscale))
+
+ # Find the keypoints
+ keypoints = cv2.goodFeaturesToTrack(frame, mask=None, **self.feature_params)
+
+ # Handle first frame
+ if not self.initializedFirstFrame:
+ # Initialize data
+ self.prevFrame = frame.copy()
+ self.prevKeyPoints = copy.copy(keypoints)
+
+ # Initialization done
+ self.initializedFirstFrame = True
+
+ return H
+
+ # Find correspondences
+ matchedKeypoints, status, err = cv2.calcOpticalFlowPyrLK(self.prevFrame, frame, self.prevKeyPoints, None)
+
+ # Leave good correspondences only
+ prevPoints = []
+ currPoints = []
+
+ for i in range(len(status)):
+ if status[i]:
+ prevPoints.append(self.prevKeyPoints[i])
+ currPoints.append(matchedKeypoints[i])
+
+ prevPoints = np.array(prevPoints)
+ currPoints = np.array(currPoints)
+
+ # Find rigid matrix
+ if (np.size(prevPoints, 0) > 4) and (np.size(prevPoints, 0) == np.size(prevPoints, 0)):
+ H, inliers = cv2.estimateAffinePartial2D(prevPoints, currPoints, cv2.RANSAC)
+
+ # Handle downscale
+ if self.downscale > 1.0:
+ H[0, 2] *= self.downscale
+ H[1, 2] *= self.downscale
+ else:
+ LOGGER.warning('WARNING: not enough matching points')
+
+ # Store to next iteration
+ self.prevFrame = frame.copy()
+ self.prevKeyPoints = copy.copy(keypoints)
+
+ # gmc_line = str(1000 * (time.time() - t0)) + "\t" + str(H[0, 0]) + "\t" + str(H[0, 1]) + "\t" + str(
+ # H[0, 2]) + "\t" + str(H[1, 0]) + "\t" + str(H[1, 1]) + "\t" + str(H[1, 2]) + "\n"
+ # self.gmc_file.write(gmc_line)
+
+ return H
+
+ def applyFile(self, raw_frame, detections=None):
+ """Return the homography matrix based on the GCPs in the next line of the input GMC file."""
+ line = self.gmcFile.readline()
+ tokens = line.split('\t')
+ H = np.eye(2, 3, dtype=np.float_)
+ H[0, 0] = float(tokens[1])
+ H[0, 1] = float(tokens[2])
+ H[0, 2] = float(tokens[3])
+ H[1, 0] = float(tokens[4])
+ H[1, 1] = float(tokens[5])
+ H[1, 2] = float(tokens[6])
+
+ return H
diff --git a/yolov8/ultralytics/tracker/utils/kalman_filter.py b/yolov8/ultralytics/tracker/utils/kalman_filter.py
new file mode 100644
index 0000000000000000000000000000000000000000..a0ee4980db9882add92ef15725ca3f86c18893c7
--- /dev/null
+++ b/yolov8/ultralytics/tracker/utils/kalman_filter.py
@@ -0,0 +1,462 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import numpy as np
+import scipy.linalg
+
+# Table for the 0.95 quantile of the chi-square distribution with N degrees of freedom (contains values for N=1, ..., 9)
+# Taken from MATLAB/Octave's chi2inv function and used as Mahalanobis gating threshold.
+chi2inv95 = {1: 3.8415, 2: 5.9915, 3: 7.8147, 4: 9.4877, 5: 11.070, 6: 12.592, 7: 14.067, 8: 15.507, 9: 16.919}
+
+
+class KalmanFilterXYAH:
+ """
+ For bytetrack
+ A simple Kalman filter for tracking bounding boxes in image space.
+
+ The 8-dimensional state space
+
+ x, y, a, h, vx, vy, va, vh
+
+ contains the bounding box center position (x, y), aspect ratio a, height h,
+ and their respective velocities.
+
+ Object motion follows a constant velocity model. The bounding box location
+ (x, y, a, h) is taken as direct observation of the state space (linear
+ observation model).
+
+ """
+
+ def __init__(self):
+ """Initialize Kalman filter model matrices with motion and observation uncertainty weights."""
+ ndim, dt = 4, 1.
+
+ # Create Kalman filter model matrices.
+ self._motion_mat = np.eye(2 * ndim, 2 * ndim)
+ for i in range(ndim):
+ self._motion_mat[i, ndim + i] = dt
+ self._update_mat = np.eye(ndim, 2 * ndim)
+
+ # Motion and observation uncertainty are chosen relative to the current
+ # state estimate. These weights control the amount of uncertainty in
+ # the model. This is a bit hacky.
+ self._std_weight_position = 1. / 20
+ self._std_weight_velocity = 1. / 160
+
+ def initiate(self, measurement):
+ """Create track from unassociated measurement.
+
+ Parameters
+ ----------
+ measurement : ndarray
+ Bounding box coordinates (x, y, a, h) with center position (x, y),
+ aspect ratio a, and height h.
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the mean vector (8 dimensional) and covariance matrix (8x8
+ dimensional) of the new track. Unobserved velocities are initialized
+ to 0 mean.
+
+ """
+ mean_pos = measurement
+ mean_vel = np.zeros_like(mean_pos)
+ mean = np.r_[mean_pos, mean_vel]
+
+ std = [
+ 2 * self._std_weight_position * measurement[3], 2 * self._std_weight_position * measurement[3], 1e-2,
+ 2 * self._std_weight_position * measurement[3], 10 * self._std_weight_velocity * measurement[3],
+ 10 * self._std_weight_velocity * measurement[3], 1e-5, 10 * self._std_weight_velocity * measurement[3]]
+ covariance = np.diag(np.square(std))
+ return mean, covariance
+
+ def predict(self, mean, covariance):
+ """Run Kalman filter prediction step.
+
+ Parameters
+ ----------
+ mean : ndarray
+ The 8 dimensional mean vector of the object state at the previous
+ time step.
+ covariance : ndarray
+ The 8x8 dimensional covariance matrix of the object state at the
+ previous time step.
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the mean vector and covariance matrix of the predicted
+ state. Unobserved velocities are initialized to 0 mean.
+
+ """
+ std_pos = [
+ self._std_weight_position * mean[3], self._std_weight_position * mean[3], 1e-2,
+ self._std_weight_position * mean[3]]
+ std_vel = [
+ self._std_weight_velocity * mean[3], self._std_weight_velocity * mean[3], 1e-5,
+ self._std_weight_velocity * mean[3]]
+ motion_cov = np.diag(np.square(np.r_[std_pos, std_vel]))
+
+ # mean = np.dot(self._motion_mat, mean)
+ mean = np.dot(mean, self._motion_mat.T)
+ covariance = np.linalg.multi_dot((self._motion_mat, covariance, self._motion_mat.T)) + motion_cov
+
+ return mean, covariance
+
+ def project(self, mean, covariance):
+ """Project state distribution to measurement space.
+
+ Parameters
+ ----------
+ mean : ndarray
+ The state's mean vector (8 dimensional array).
+ covariance : ndarray
+ The state's covariance matrix (8x8 dimensional).
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the projected mean and covariance matrix of the given state
+ estimate.
+
+ """
+ std = [
+ self._std_weight_position * mean[3], self._std_weight_position * mean[3], 1e-1,
+ self._std_weight_position * mean[3]]
+ innovation_cov = np.diag(np.square(std))
+
+ mean = np.dot(self._update_mat, mean)
+ covariance = np.linalg.multi_dot((self._update_mat, covariance, self._update_mat.T))
+ return mean, covariance + innovation_cov
+
+ def multi_predict(self, mean, covariance):
+ """Run Kalman filter prediction step (Vectorized version).
+ Parameters
+ ----------
+ mean : ndarray
+ The Nx8 dimensional mean matrix of the object states at the previous
+ time step.
+ covariance : ndarray
+ The Nx8x8 dimensional covariance matrix of the object states at the
+ previous time step.
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the mean vector and covariance matrix of the predicted
+ state. Unobserved velocities are initialized to 0 mean.
+ """
+ std_pos = [
+ self._std_weight_position * mean[:, 3], self._std_weight_position * mean[:, 3],
+ 1e-2 * np.ones_like(mean[:, 3]), self._std_weight_position * mean[:, 3]]
+ std_vel = [
+ self._std_weight_velocity * mean[:, 3], self._std_weight_velocity * mean[:, 3],
+ 1e-5 * np.ones_like(mean[:, 3]), self._std_weight_velocity * mean[:, 3]]
+ sqr = np.square(np.r_[std_pos, std_vel]).T
+
+ motion_cov = [np.diag(sqr[i]) for i in range(len(mean))]
+ motion_cov = np.asarray(motion_cov)
+
+ mean = np.dot(mean, self._motion_mat.T)
+ left = np.dot(self._motion_mat, covariance).transpose((1, 0, 2))
+ covariance = np.dot(left, self._motion_mat.T) + motion_cov
+
+ return mean, covariance
+
+ def update(self, mean, covariance, measurement):
+ """Run Kalman filter correction step.
+
+ Parameters
+ ----------
+ mean : ndarray
+ The predicted state's mean vector (8 dimensional).
+ covariance : ndarray
+ The state's covariance matrix (8x8 dimensional).
+ measurement : ndarray
+ The 4 dimensional measurement vector (x, y, a, h), where (x, y)
+ is the center position, a the aspect ratio, and h the height of the
+ bounding box.
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the measurement-corrected state distribution.
+
+ """
+ projected_mean, projected_cov = self.project(mean, covariance)
+
+ chol_factor, lower = scipy.linalg.cho_factor(projected_cov, lower=True, check_finite=False)
+ kalman_gain = scipy.linalg.cho_solve((chol_factor, lower),
+ np.dot(covariance, self._update_mat.T).T,
+ check_finite=False).T
+ innovation = measurement - projected_mean
+
+ new_mean = mean + np.dot(innovation, kalman_gain.T)
+ new_covariance = covariance - np.linalg.multi_dot((kalman_gain, projected_cov, kalman_gain.T))
+ return new_mean, new_covariance
+
+ def gating_distance(self, mean, covariance, measurements, only_position=False, metric='maha'):
+ """Compute gating distance between state distribution and measurements.
+ A suitable distance threshold can be obtained from `chi2inv95`. If
+ `only_position` is False, the chi-square distribution has 4 degrees of
+ freedom, otherwise 2.
+ Parameters
+ ----------
+ mean : ndarray
+ Mean vector over the state distribution (8 dimensional).
+ covariance : ndarray
+ Covariance of the state distribution (8x8 dimensional).
+ measurements : ndarray
+ An Nx4 dimensional matrix of N measurements, each in
+ format (x, y, a, h) where (x, y) is the bounding box center
+ position, a the aspect ratio, and h the height.
+ only_position : Optional[bool]
+ If True, distance computation is done with respect to the bounding
+ box center position only.
+ Returns
+ -------
+ ndarray
+ Returns an array of length N, where the i-th element contains the
+ squared Mahalanobis distance between (mean, covariance) and
+ `measurements[i]`.
+ """
+ mean, covariance = self.project(mean, covariance)
+ if only_position:
+ mean, covariance = mean[:2], covariance[:2, :2]
+ measurements = measurements[:, :2]
+
+ d = measurements - mean
+ if metric == 'gaussian':
+ return np.sum(d * d, axis=1)
+ elif metric == 'maha':
+ cholesky_factor = np.linalg.cholesky(covariance)
+ z = scipy.linalg.solve_triangular(cholesky_factor, d.T, lower=True, check_finite=False, overwrite_b=True)
+ return np.sum(z * z, axis=0) # square maha
+ else:
+ raise ValueError('invalid distance metric')
+
+
+class KalmanFilterXYWH:
+ """
+ For BoT-SORT
+ A simple Kalman filter for tracking bounding boxes in image space.
+
+ The 8-dimensional state space
+
+ x, y, w, h, vx, vy, vw, vh
+
+ contains the bounding box center position (x, y), width w, height h,
+ and their respective velocities.
+
+ Object motion follows a constant velocity model. The bounding box location
+ (x, y, w, h) is taken as direct observation of the state space (linear
+ observation model).
+
+ """
+
+ def __init__(self):
+ """Initialize Kalman filter model matrices with motion and observation uncertainties."""
+ ndim, dt = 4, 1.
+
+ # Create Kalman filter model matrices.
+ self._motion_mat = np.eye(2 * ndim, 2 * ndim)
+ for i in range(ndim):
+ self._motion_mat[i, ndim + i] = dt
+ self._update_mat = np.eye(ndim, 2 * ndim)
+
+ # Motion and observation uncertainty are chosen relative to the current
+ # state estimate. These weights control the amount of uncertainty in
+ # the model. This is a bit hacky.
+ self._std_weight_position = 1. / 20
+ self._std_weight_velocity = 1. / 160
+
+ def initiate(self, measurement):
+ """Create track from unassociated measurement.
+
+ Parameters
+ ----------
+ measurement : ndarray
+ Bounding box coordinates (x, y, w, h) with center position (x, y),
+ width w, and height h.
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the mean vector (8 dimensional) and covariance matrix (8x8
+ dimensional) of the new track. Unobserved velocities are initialized
+ to 0 mean.
+
+ """
+ mean_pos = measurement
+ mean_vel = np.zeros_like(mean_pos)
+ mean = np.r_[mean_pos, mean_vel]
+
+ std = [
+ 2 * self._std_weight_position * measurement[2], 2 * self._std_weight_position * measurement[3],
+ 2 * self._std_weight_position * measurement[2], 2 * self._std_weight_position * measurement[3],
+ 10 * self._std_weight_velocity * measurement[2], 10 * self._std_weight_velocity * measurement[3],
+ 10 * self._std_weight_velocity * measurement[2], 10 * self._std_weight_velocity * measurement[3]]
+ covariance = np.diag(np.square(std))
+ return mean, covariance
+
+ def predict(self, mean, covariance):
+ """Run Kalman filter prediction step.
+
+ Parameters
+ ----------
+ mean : ndarray
+ The 8 dimensional mean vector of the object state at the previous
+ time step.
+ covariance : ndarray
+ The 8x8 dimensional covariance matrix of the object state at the
+ previous time step.
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the mean vector and covariance matrix of the predicted
+ state. Unobserved velocities are initialized to 0 mean.
+
+ """
+ std_pos = [
+ self._std_weight_position * mean[2], self._std_weight_position * mean[3],
+ self._std_weight_position * mean[2], self._std_weight_position * mean[3]]
+ std_vel = [
+ self._std_weight_velocity * mean[2], self._std_weight_velocity * mean[3],
+ self._std_weight_velocity * mean[2], self._std_weight_velocity * mean[3]]
+ motion_cov = np.diag(np.square(np.r_[std_pos, std_vel]))
+
+ mean = np.dot(mean, self._motion_mat.T)
+ covariance = np.linalg.multi_dot((self._motion_mat, covariance, self._motion_mat.T)) + motion_cov
+
+ return mean, covariance
+
+ def project(self, mean, covariance):
+ """Project state distribution to measurement space.
+
+ Parameters
+ ----------
+ mean : ndarray
+ The state's mean vector (8 dimensional array).
+ covariance : ndarray
+ The state's covariance matrix (8x8 dimensional).
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the projected mean and covariance matrix of the given state
+ estimate.
+
+ """
+ std = [
+ self._std_weight_position * mean[2], self._std_weight_position * mean[3],
+ self._std_weight_position * mean[2], self._std_weight_position * mean[3]]
+ innovation_cov = np.diag(np.square(std))
+
+ mean = np.dot(self._update_mat, mean)
+ covariance = np.linalg.multi_dot((self._update_mat, covariance, self._update_mat.T))
+ return mean, covariance + innovation_cov
+
+ def multi_predict(self, mean, covariance):
+ """Run Kalman filter prediction step (Vectorized version).
+ Parameters
+ ----------
+ mean : ndarray
+ The Nx8 dimensional mean matrix of the object states at the previous
+ time step.
+ covariance : ndarray
+ The Nx8x8 dimensional covariance matrix of the object states at the
+ previous time step.
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the mean vector and covariance matrix of the predicted
+ state. Unobserved velocities are initialized to 0 mean.
+ """
+ std_pos = [
+ self._std_weight_position * mean[:, 2], self._std_weight_position * mean[:, 3],
+ self._std_weight_position * mean[:, 2], self._std_weight_position * mean[:, 3]]
+ std_vel = [
+ self._std_weight_velocity * mean[:, 2], self._std_weight_velocity * mean[:, 3],
+ self._std_weight_velocity * mean[:, 2], self._std_weight_velocity * mean[:, 3]]
+ sqr = np.square(np.r_[std_pos, std_vel]).T
+
+ motion_cov = [np.diag(sqr[i]) for i in range(len(mean))]
+ motion_cov = np.asarray(motion_cov)
+
+ mean = np.dot(mean, self._motion_mat.T)
+ left = np.dot(self._motion_mat, covariance).transpose((1, 0, 2))
+ covariance = np.dot(left, self._motion_mat.T) + motion_cov
+
+ return mean, covariance
+
+ def update(self, mean, covariance, measurement):
+ """Run Kalman filter correction step.
+
+ Parameters
+ ----------
+ mean : ndarray
+ The predicted state's mean vector (8 dimensional).
+ covariance : ndarray
+ The state's covariance matrix (8x8 dimensional).
+ measurement : ndarray
+ The 4 dimensional measurement vector (x, y, w, h), where (x, y)
+ is the center position, w the width, and h the height of the
+ bounding box.
+
+ Returns
+ -------
+ (ndarray, ndarray)
+ Returns the measurement-corrected state distribution.
+
+ """
+ projected_mean, projected_cov = self.project(mean, covariance)
+
+ chol_factor, lower = scipy.linalg.cho_factor(projected_cov, lower=True, check_finite=False)
+ kalman_gain = scipy.linalg.cho_solve((chol_factor, lower),
+ np.dot(covariance, self._update_mat.T).T,
+ check_finite=False).T
+ innovation = measurement - projected_mean
+
+ new_mean = mean + np.dot(innovation, kalman_gain.T)
+ new_covariance = covariance - np.linalg.multi_dot((kalman_gain, projected_cov, kalman_gain.T))
+ return new_mean, new_covariance
+
+ def gating_distance(self, mean, covariance, measurements, only_position=False, metric='maha'):
+ """Compute gating distance between state distribution and measurements.
+ A suitable distance threshold can be obtained from `chi2inv95`. If
+ `only_position` is False, the chi-square distribution has 4 degrees of
+ freedom, otherwise 2.
+ Parameters
+ ----------
+ mean : ndarray
+ Mean vector over the state distribution (8 dimensional).
+ covariance : ndarray
+ Covariance of the state distribution (8x8 dimensional).
+ measurements : ndarray
+ An Nx4 dimensional matrix of N measurements, each in
+ format (x, y, a, h) where (x, y) is the bounding box center
+ position, a the aspect ratio, and h the height.
+ only_position : Optional[bool]
+ If True, distance computation is done with respect to the bounding
+ box center position only.
+ Returns
+ -------
+ ndarray
+ Returns an array of length N, where the i-th element contains the
+ squared Mahalanobis distance between (mean, covariance) and
+ `measurements[i]`.
+ """
+ mean, covariance = self.project(mean, covariance)
+ if only_position:
+ mean, covariance = mean[:2], covariance[:2, :2]
+ measurements = measurements[:, :2]
+
+ d = measurements - mean
+ if metric == 'gaussian':
+ return np.sum(d * d, axis=1)
+ elif metric == 'maha':
+ cholesky_factor = np.linalg.cholesky(covariance)
+ z = scipy.linalg.solve_triangular(cholesky_factor, d.T, lower=True, check_finite=False, overwrite_b=True)
+ return np.sum(z * z, axis=0) # square maha
+ else:
+ raise ValueError('invalid distance metric')
diff --git a/yolov8/ultralytics/tracker/utils/matching.py b/yolov8/ultralytics/tracker/utils/matching.py
new file mode 100644
index 0000000000000000000000000000000000000000..0b22b3de80988e5774487d698ae6b5b3267e7746
--- /dev/null
+++ b/yolov8/ultralytics/tracker/utils/matching.py
@@ -0,0 +1,229 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import numpy as np
+import scipy
+from scipy.spatial.distance import cdist
+
+from .kalman_filter import chi2inv95
+
+try:
+ import lap # for linear_assignment
+
+ assert lap.__version__ # verify package is not directory
+except (ImportError, AssertionError, AttributeError):
+ from ultralytics.yolo.utils.checks import check_requirements
+
+ check_requirements('lapx>=0.5.2') # update to lap package from https://github.com/rathaROG/lapx
+ import lap
+
+
+def merge_matches(m1, m2, shape):
+ """Merge two sets of matches and return matched and unmatched indices."""
+ O, P, Q = shape
+ m1 = np.asarray(m1)
+ m2 = np.asarray(m2)
+
+ M1 = scipy.sparse.coo_matrix((np.ones(len(m1)), (m1[:, 0], m1[:, 1])), shape=(O, P))
+ M2 = scipy.sparse.coo_matrix((np.ones(len(m2)), (m2[:, 0], m2[:, 1])), shape=(P, Q))
+
+ mask = M1 * M2
+ match = mask.nonzero()
+ match = list(zip(match[0], match[1]))
+ unmatched_O = tuple(set(range(O)) - {i for i, j in match})
+ unmatched_Q = tuple(set(range(Q)) - {j for i, j in match})
+
+ return match, unmatched_O, unmatched_Q
+
+
+def _indices_to_matches(cost_matrix, indices, thresh):
+ """_indices_to_matches: Return matched and unmatched indices given a cost matrix, indices, and a threshold."""
+ matched_cost = cost_matrix[tuple(zip(*indices))]
+ matched_mask = (matched_cost <= thresh)
+
+ matches = indices[matched_mask]
+ unmatched_a = tuple(set(range(cost_matrix.shape[0])) - set(matches[:, 0]))
+ unmatched_b = tuple(set(range(cost_matrix.shape[1])) - set(matches[:, 1]))
+
+ return matches, unmatched_a, unmatched_b
+
+
+def linear_assignment(cost_matrix, thresh, use_lap=True):
+ """Linear assignment implementations with scipy and lap.lapjv."""
+ if cost_matrix.size == 0:
+ return np.empty((0, 2), dtype=int), tuple(range(cost_matrix.shape[0])), tuple(range(cost_matrix.shape[1]))
+
+ if use_lap:
+ _, x, y = lap.lapjv(cost_matrix, extend_cost=True, cost_limit=thresh)
+ matches = [[ix, mx] for ix, mx in enumerate(x) if mx >= 0]
+ unmatched_a = np.where(x < 0)[0]
+ unmatched_b = np.where(y < 0)[0]
+ else:
+ # Scipy linear sum assignment is NOT working correctly, DO NOT USE
+ y, x = scipy.optimize.linear_sum_assignment(cost_matrix) # row y, col x
+ matches = np.asarray([[i, x] for i, x in enumerate(x) if cost_matrix[i, x] <= thresh])
+ unmatched = np.ones(cost_matrix.shape)
+ for i, xi in matches:
+ unmatched[i, xi] = 0.0
+ unmatched_a = np.where(unmatched.all(1))[0]
+ unmatched_b = np.where(unmatched.all(0))[0]
+
+ return matches, unmatched_a, unmatched_b
+
+
+def ious(atlbrs, btlbrs):
+ """
+ Compute cost based on IoU
+ :type atlbrs: list[tlbr] | np.ndarray
+ :type atlbrs: list[tlbr] | np.ndarray
+
+ :rtype ious np.ndarray
+ """
+ ious = np.zeros((len(atlbrs), len(btlbrs)), dtype=np.float32)
+ if ious.size == 0:
+ return ious
+
+ ious = bbox_ious(np.ascontiguousarray(atlbrs, dtype=np.float32), np.ascontiguousarray(btlbrs, dtype=np.float32))
+ return ious
+
+
+def iou_distance(atracks, btracks):
+ """
+ Compute cost based on IoU
+ :type atracks: list[STrack]
+ :type btracks: list[STrack]
+
+ :rtype cost_matrix np.ndarray
+ """
+
+ if (len(atracks) > 0 and isinstance(atracks[0], np.ndarray)) \
+ or (len(btracks) > 0 and isinstance(btracks[0], np.ndarray)):
+ atlbrs = atracks
+ btlbrs = btracks
+ else:
+ atlbrs = [track.tlbr for track in atracks]
+ btlbrs = [track.tlbr for track in btracks]
+ _ious = ious(atlbrs, btlbrs)
+ return 1 - _ious # cost matrix
+
+
+def v_iou_distance(atracks, btracks):
+ """
+ Compute cost based on IoU
+ :type atracks: list[STrack]
+ :type btracks: list[STrack]
+
+ :rtype cost_matrix np.ndarray
+ """
+
+ if (len(atracks) > 0 and isinstance(atracks[0], np.ndarray)) \
+ or (len(btracks) > 0 and isinstance(btracks[0], np.ndarray)):
+ atlbrs = atracks
+ btlbrs = btracks
+ else:
+ atlbrs = [track.tlwh_to_tlbr(track.pred_bbox) for track in atracks]
+ btlbrs = [track.tlwh_to_tlbr(track.pred_bbox) for track in btracks]
+ _ious = ious(atlbrs, btlbrs)
+ return 1 - _ious # cost matrix
+
+
+def embedding_distance(tracks, detections, metric='cosine'):
+ """
+ :param tracks: list[STrack]
+ :param detections: list[BaseTrack]
+ :param metric:
+ :return: cost_matrix np.ndarray
+ """
+
+ cost_matrix = np.zeros((len(tracks), len(detections)), dtype=np.float32)
+ if cost_matrix.size == 0:
+ return cost_matrix
+ det_features = np.asarray([track.curr_feat for track in detections], dtype=np.float32)
+ # for i, track in enumerate(tracks):
+ # cost_matrix[i, :] = np.maximum(0.0, cdist(track.smooth_feat.reshape(1,-1), det_features, metric))
+ track_features = np.asarray([track.smooth_feat for track in tracks], dtype=np.float32)
+ cost_matrix = np.maximum(0.0, cdist(track_features, det_features, metric)) # Normalized features
+ return cost_matrix
+
+
+def gate_cost_matrix(kf, cost_matrix, tracks, detections, only_position=False):
+ """Apply gating to the cost matrix based on predicted tracks and detected objects."""
+ if cost_matrix.size == 0:
+ return cost_matrix
+ gating_dim = 2 if only_position else 4
+ gating_threshold = chi2inv95[gating_dim]
+ measurements = np.asarray([det.to_xyah() for det in detections])
+ for row, track in enumerate(tracks):
+ gating_distance = kf.gating_distance(track.mean, track.covariance, measurements, only_position)
+ cost_matrix[row, gating_distance > gating_threshold] = np.inf
+ return cost_matrix
+
+
+def fuse_motion(kf, cost_matrix, tracks, detections, only_position=False, lambda_=0.98):
+ """Fuse motion between tracks and detections with gating and Kalman filtering."""
+ if cost_matrix.size == 0:
+ return cost_matrix
+ gating_dim = 2 if only_position else 4
+ gating_threshold = chi2inv95[gating_dim]
+ measurements = np.asarray([det.to_xyah() for det in detections])
+ for row, track in enumerate(tracks):
+ gating_distance = kf.gating_distance(track.mean, track.covariance, measurements, only_position, metric='maha')
+ cost_matrix[row, gating_distance > gating_threshold] = np.inf
+ cost_matrix[row] = lambda_ * cost_matrix[row] + (1 - lambda_) * gating_distance
+ return cost_matrix
+
+
+def fuse_iou(cost_matrix, tracks, detections):
+ """Fuses ReID and IoU similarity matrices to yield a cost matrix for object tracking."""
+ if cost_matrix.size == 0:
+ return cost_matrix
+ reid_sim = 1 - cost_matrix
+ iou_dist = iou_distance(tracks, detections)
+ iou_sim = 1 - iou_dist
+ fuse_sim = reid_sim * (1 + iou_sim) / 2
+ # det_scores = np.array([det.score for det in detections])
+ # det_scores = np.expand_dims(det_scores, axis=0).repeat(cost_matrix.shape[0], axis=0)
+ return 1 - fuse_sim # fuse cost
+
+
+def fuse_score(cost_matrix, detections):
+ """Fuses cost matrix with detection scores to produce a single similarity matrix."""
+ if cost_matrix.size == 0:
+ return cost_matrix
+ iou_sim = 1 - cost_matrix
+ det_scores = np.array([det.score for det in detections])
+ det_scores = np.expand_dims(det_scores, axis=0).repeat(cost_matrix.shape[0], axis=0)
+ fuse_sim = iou_sim * det_scores
+ return 1 - fuse_sim # fuse_cost
+
+
+def bbox_ious(box1, box2, eps=1e-7):
+ """
+ Calculate the Intersection over Union (IoU) between pairs of bounding boxes.
+
+ Args:
+ box1 (np.array): A numpy array of shape (n, 4) representing 'n' bounding boxes.
+ Each row is in the format (x1, y1, x2, y2).
+ box2 (np.array): A numpy array of shape (m, 4) representing 'm' bounding boxes.
+ Each row is in the format (x1, y1, x2, y2).
+ eps (float, optional): A small constant to prevent division by zero. Defaults to 1e-7.
+
+ Returns:
+ (np.array): A numpy array of shape (n, m) representing the IoU scores for each pair
+ of bounding boxes from box1 and box2.
+
+ Note:
+ The bounding box coordinates are expected to be in the format (x1, y1, x2, y2).
+ """
+
+ # Get the coordinates of bounding boxes
+ b1_x1, b1_y1, b1_x2, b1_y2 = box1.T
+ b2_x1, b2_y1, b2_x2, b2_y2 = box2.T
+
+ # Intersection area
+ inter_area = (np.minimum(b1_x2[:, None], b2_x2) - np.maximum(b1_x1[:, None], b2_x1)).clip(0) * \
+ (np.minimum(b1_y2[:, None], b2_y2) - np.maximum(b1_y1[:, None], b2_y1)).clip(0)
+
+ # box2 area
+ box1_area = (b1_x2 - b1_x1) * (b1_y2 - b1_y1)
+ box2_area = (b2_x2 - b2_x1) * (b2_y2 - b2_y1)
+ return inter_area / (box2_area + box1_area[:, None] - inter_area + eps)
diff --git a/yolov8/ultralytics/vit/__init__.py b/yolov8/ultralytics/vit/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..8e96f915d0d670a2140850d13e9d72ae78a7d3d8
--- /dev/null
+++ b/yolov8/ultralytics/vit/__init__.py
@@ -0,0 +1,6 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .rtdetr import RTDETR
+from .sam import SAM
+
+__all__ = 'RTDETR', 'SAM' # allow simpler import
diff --git a/yolov8/ultralytics/vit/rtdetr/__init__.py b/yolov8/ultralytics/vit/rtdetr/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..4d12115616a9e637857da368d5ace3098bbb96d1
--- /dev/null
+++ b/yolov8/ultralytics/vit/rtdetr/__init__.py
@@ -0,0 +1,7 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .model import RTDETR
+from .predict import RTDETRPredictor
+from .val import RTDETRValidator
+
+__all__ = 'RTDETRPredictor', 'RTDETRValidator', 'RTDETR'
diff --git a/yolov8/ultralytics/vit/rtdetr/model.py b/yolov8/ultralytics/vit/rtdetr/model.py
new file mode 100644
index 0000000000000000000000000000000000000000..259c7c97689b328348863f64c88a36fd078ef0be
--- /dev/null
+++ b/yolov8/ultralytics/vit/rtdetr/model.py
@@ -0,0 +1,173 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+RT-DETR model interface
+"""
+
+from pathlib import Path
+
+import torch.nn as nn
+
+from ultralytics.nn.tasks import RTDETRDetectionModel, attempt_load_one_weight, yaml_model_load
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.engine.exporter import Exporter
+from ultralytics.yolo.utils import DEFAULT_CFG, DEFAULT_CFG_DICT, LOGGER, RANK, ROOT, is_git_dir
+from ultralytics.yolo.utils.checks import check_imgsz
+from ultralytics.yolo.utils.torch_utils import model_info, smart_inference_mode
+
+from .predict import RTDETRPredictor
+from .train import RTDETRTrainer
+from .val import RTDETRValidator
+
+
+class RTDETR:
+
+ def __init__(self, model='rtdetr-l.pt') -> None:
+ if model and not model.endswith('.pt') and not model.endswith('.yaml'):
+ raise NotImplementedError('RT-DETR only supports creating from pt file or yaml file.')
+ # Load or create new YOLO model
+ self.predictor = None
+ self.ckpt = None
+ suffix = Path(model).suffix
+ if suffix == '.yaml':
+ self._new(model)
+ else:
+ self._load(model)
+
+ def _new(self, cfg: str, verbose=True):
+ cfg_dict = yaml_model_load(cfg)
+ self.cfg = cfg
+ self.task = 'detect'
+ self.model = RTDETRDetectionModel(cfg_dict, verbose=verbose) # build model
+
+ # Below added to allow export from YAMLs
+ self.model.args = DEFAULT_CFG_DICT # attach args to model
+ self.model.task = self.task
+
+ @smart_inference_mode()
+ def _load(self, weights: str):
+ self.model, self.ckpt = attempt_load_one_weight(weights)
+ self.model.args = DEFAULT_CFG_DICT # attach args to model
+ self.task = self.model.args['task']
+
+ @smart_inference_mode()
+ def load(self, weights='yolov8n.pt'):
+ """
+ Transfers parameters with matching names and shapes from 'weights' to model.
+ """
+ if isinstance(weights, (str, Path)):
+ weights, self.ckpt = attempt_load_one_weight(weights)
+ self.model.load(weights)
+ return self
+
+ @smart_inference_mode()
+ def predict(self, source=None, stream=False, **kwargs):
+ """
+ Perform prediction using the YOLO model.
+
+ Args:
+ source (str | int | PIL | np.ndarray): The source of the image to make predictions on.
+ Accepts all source types accepted by the YOLO model.
+ stream (bool): Whether to stream the predictions or not. Defaults to False.
+ **kwargs : Additional keyword arguments passed to the predictor.
+ Check the 'configuration' section in the documentation for all available options.
+
+ Returns:
+ (List[ultralytics.yolo.engine.results.Results]): The prediction results.
+ """
+ if source is None:
+ source = ROOT / 'assets' if is_git_dir() else 'https://ultralytics.com/images/bus.jpg'
+ LOGGER.warning(f"WARNING ⚠️ 'source' is missing. Using 'source={source}'.")
+ overrides = dict(conf=0.25, task='detect', mode='predict')
+ overrides.update(kwargs) # prefer kwargs
+ if not self.predictor:
+ self.predictor = RTDETRPredictor(overrides=overrides)
+ self.predictor.setup_model(model=self.model)
+ else: # only update args if predictor is already setup
+ self.predictor.args = get_cfg(self.predictor.args, overrides)
+ return self.predictor(source, stream=stream)
+
+ def train(self, **kwargs):
+ """
+ Trains the model on a given dataset.
+
+ Args:
+ **kwargs (Any): Any number of arguments representing the training configuration.
+ """
+ overrides = dict(task='detect', mode='train')
+ overrides.update(kwargs)
+ overrides['deterministic'] = False
+ if not overrides.get('data'):
+ raise AttributeError("Dataset required but missing, i.e. pass 'data=coco128.yaml'")
+ if overrides.get('resume'):
+ overrides['resume'] = self.ckpt_path
+ self.task = overrides.get('task') or self.task
+ self.trainer = RTDETRTrainer(overrides=overrides)
+ if not overrides.get('resume'): # manually set model only if not resuming
+ self.trainer.model = self.trainer.get_model(weights=self.model if self.ckpt else None, cfg=self.model.yaml)
+ self.model = self.trainer.model
+ self.trainer.train()
+ # Update model and cfg after training
+ if RANK in (-1, 0):
+ self.model, _ = attempt_load_one_weight(str(self.trainer.best))
+ self.overrides = self.model.args
+ self.metrics = getattr(self.trainer.validator, 'metrics', None) # TODO: no metrics returned by DDP
+
+ def val(self, **kwargs):
+ """Run validation given dataset."""
+ overrides = dict(task='detect', mode='val')
+ overrides.update(kwargs) # prefer kwargs
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.imgsz = check_imgsz(args.imgsz, max_dim=1)
+ validator = RTDETRValidator(args=args)
+ validator(model=self.model)
+ self.metrics = validator.metrics
+ return validator.metrics
+
+ def info(self, verbose=True):
+ """Get model info"""
+ return model_info(self.model, verbose=verbose)
+
+ def _check_is_pytorch_model(self):
+ """
+ Raises TypeError is model is not a PyTorch model
+ """
+ pt_str = isinstance(self.model, (str, Path)) and Path(self.model).suffix == '.pt'
+ pt_module = isinstance(self.model, nn.Module)
+ if not (pt_module or pt_str):
+ raise TypeError(f"model='{self.model}' must be a *.pt PyTorch model, but is a different type. "
+ f'PyTorch models can be used to train, val, predict and export, i.e. '
+ f"'yolo export model=yolov8n.pt', but exported formats like ONNX, TensorRT etc. only "
+ f"support 'predict' and 'val' modes, i.e. 'yolo predict model=yolov8n.onnx'.")
+
+ def fuse(self):
+ """Fuse PyTorch Conv2d and BatchNorm2d layers."""
+ self._check_is_pytorch_model()
+ self.model.fuse()
+
+ @smart_inference_mode()
+ def export(self, **kwargs):
+ """
+ Export model.
+
+ Args:
+ **kwargs : Any other args accepted by the predictors. To see all args check 'configuration' section in docs
+ """
+ overrides = dict(task='detect')
+ overrides.update(kwargs)
+ overrides['mode'] = 'export'
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.task = self.task
+ if args.imgsz == DEFAULT_CFG.imgsz:
+ args.imgsz = self.model.args['imgsz'] # use trained imgsz unless custom value is passed
+ if args.batch == DEFAULT_CFG.batch:
+ args.batch = 1 # default to 1 if not modified
+ return Exporter(overrides=args)(model=self.model)
+
+ def __call__(self, source=None, stream=False, **kwargs):
+ """Calls the 'predict' function with given arguments to perform object detection."""
+ return self.predict(source, stream, **kwargs)
+
+ def __getattr__(self, attr):
+ """Raises error if object has no requested attribute."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
diff --git a/yolov8/ultralytics/vit/rtdetr/predict.py b/yolov8/ultralytics/vit/rtdetr/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..77c02c24d037076be6d39d6ccaf5148d8835bf66
--- /dev/null
+++ b/yolov8/ultralytics/vit/rtdetr/predict.py
@@ -0,0 +1,44 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.data.augment import LetterBox
+from ultralytics.yolo.engine.predictor import BasePredictor
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils import ops
+
+
+class RTDETRPredictor(BasePredictor):
+
+ def postprocess(self, preds, img, orig_imgs):
+ """Postprocess predictions and returns a list of Results objects."""
+ nd = preds[0].shape[-1]
+ bboxes, scores = preds[0].split((4, nd - 4), dim=-1)
+ results = []
+ for i, bbox in enumerate(bboxes): # (300, 4)
+ bbox = ops.xywh2xyxy(bbox)
+ score, cls = scores[i].max(-1, keepdim=True) # (300, 1)
+ idx = score.squeeze(-1) > self.args.conf # (300, )
+ if self.args.classes is not None:
+ idx = (cls == torch.tensor(self.args.classes, device=cls.device)).any(1) & idx
+ pred = torch.cat([bbox, score, cls], dim=-1)[idx] # filter
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ oh, ow = orig_img.shape[:2]
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[..., [0, 2]] *= ow
+ pred[..., [1, 3]] *= oh
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ results.append(Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred))
+ return results
+
+ def pre_transform(self, im):
+ """Pre-transform input image before inference.
+
+ Args:
+ im (List(np.ndarray)): (N, 3, h, w) for tensor, [(h, w, 3) x N] for list.
+
+ Return: A list of transformed imgs.
+ """
+ # The size must be square(640) and scaleFilled.
+ return [LetterBox(self.imgsz, auto=False, scaleFill=True)(image=x) for x in im]
diff --git a/yolov8/ultralytics/vit/rtdetr/train.py b/yolov8/ultralytics/vit/rtdetr/train.py
new file mode 100644
index 0000000000000000000000000000000000000000..54eeaf4aa79a9f22fd5d04d60482d52d7155cfc8
--- /dev/null
+++ b/yolov8/ultralytics/vit/rtdetr/train.py
@@ -0,0 +1,80 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from copy import copy
+
+import torch
+
+from ultralytics.nn.tasks import RTDETRDetectionModel
+from ultralytics.yolo.utils import DEFAULT_CFG, RANK, colorstr
+from ultralytics.yolo.v8.detect import DetectionTrainer
+
+from .val import RTDETRDataset, RTDETRValidator
+
+
+class RTDETRTrainer(DetectionTrainer):
+
+ def get_model(self, cfg=None, weights=None, verbose=True):
+ """Return a YOLO detection model."""
+ model = RTDETRDetectionModel(cfg, nc=self.data['nc'], verbose=verbose and RANK == -1)
+ if weights:
+ model.load(weights)
+ return model
+
+ def build_dataset(self, img_path, mode='val', batch=None):
+ """Build RTDETR Dataset
+
+ Args:
+ img_path (str): Path to the folder containing images.
+ mode (str): `train` mode or `val` mode, users are able to customize different augmentations for each mode.
+ batch (int, optional): Size of batches, this is for `rect`. Defaults to None.
+ """
+ return RTDETRDataset(
+ img_path=img_path,
+ imgsz=self.args.imgsz,
+ batch_size=batch,
+ augment=mode == 'train', # no augmentation
+ hyp=self.args,
+ rect=False, # no rect
+ cache=self.args.cache or None,
+ prefix=colorstr(f'{mode}: '),
+ data=self.data)
+
+ def get_validator(self):
+ """Returns a DetectionValidator for RTDETR model validation."""
+ self.loss_names = 'giou_loss', 'cls_loss', 'l1_loss'
+ return RTDETRValidator(self.test_loader, save_dir=self.save_dir, args=copy(self.args))
+
+ def preprocess_batch(self, batch):
+ """Preprocesses a batch of images by scaling and converting to float."""
+ batch = super().preprocess_batch(batch)
+ bs = len(batch['img'])
+ batch_idx = batch['batch_idx']
+ gt_bbox, gt_class = [], []
+ for i in range(bs):
+ gt_bbox.append(batch['bboxes'][batch_idx == i].to(batch_idx.device))
+ gt_class.append(batch['cls'][batch_idx == i].to(device=batch_idx.device, dtype=torch.long))
+ return batch
+
+
+def train(cfg=DEFAULT_CFG, use_python=False):
+ """Train and optimize RTDETR model given training data and device."""
+ model = 'rtdetr-l.yaml'
+ data = cfg.data or 'coco128.yaml' # or yolo.ClassificationDataset("mnist")
+ device = cfg.device if cfg.device is not None else ''
+
+ # NOTE: F.grid_sample which is in rt-detr does not support deterministic=True
+ # NOTE: amp training causes nan outputs and end with error while doing bipartite graph matching
+ args = dict(model=model,
+ data=data,
+ device=device,
+ imgsz=640,
+ exist_ok=True,
+ batch=4,
+ deterministic=False,
+ amp=False)
+ trainer = RTDETRTrainer(overrides=args)
+ trainer.train()
+
+
+if __name__ == '__main__':
+ train()
diff --git a/yolov8/ultralytics/vit/rtdetr/val.py b/yolov8/ultralytics/vit/rtdetr/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..cfee2925352e49019ae0c17ae6d9636a9dd02790
--- /dev/null
+++ b/yolov8/ultralytics/vit/rtdetr/val.py
@@ -0,0 +1,151 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from pathlib import Path
+
+import cv2
+import numpy as np
+import torch
+
+from ultralytics.yolo.data import YOLODataset
+from ultralytics.yolo.data.augment import Compose, Format, v8_transforms
+from ultralytics.yolo.utils import colorstr, ops
+from ultralytics.yolo.v8.detect import DetectionValidator
+
+__all__ = 'RTDETRValidator', # tuple or list
+
+
+# TODO: Temporarily, RT-DETR does not need padding.
+class RTDETRDataset(YOLODataset):
+
+ def __init__(self, *args, data=None, **kwargs):
+ super().__init__(*args, data=data, use_segments=False, use_keypoints=False, **kwargs)
+
+ # NOTE: add stretch version load_image for rtdetr mosaic
+ def load_image(self, i):
+ """Loads 1 image from dataset index 'i', returns (im, resized hw)."""
+ im, f, fn = self.ims[i], self.im_files[i], self.npy_files[i]
+ if im is None: # not cached in RAM
+ if fn.exists(): # load npy
+ im = np.load(fn)
+ else: # read image
+ im = cv2.imread(f) # BGR
+ if im is None:
+ raise FileNotFoundError(f'Image Not Found {f}')
+ h0, w0 = im.shape[:2] # orig hw
+ im = cv2.resize(im, (self.imgsz, self.imgsz), interpolation=cv2.INTER_LINEAR)
+
+ # Add to buffer if training with augmentations
+ if self.augment:
+ self.ims[i], self.im_hw0[i], self.im_hw[i] = im, (h0, w0), im.shape[:2] # im, hw_original, hw_resized
+ self.buffer.append(i)
+ if len(self.buffer) >= self.max_buffer_length:
+ j = self.buffer.pop(0)
+ self.ims[j], self.im_hw0[j], self.im_hw[j] = None, None, None
+
+ return im, (h0, w0), im.shape[:2]
+
+ return self.ims[i], self.im_hw0[i], self.im_hw[i]
+
+ def build_transforms(self, hyp=None):
+ """Temporarily, only for evaluation."""
+ if self.augment:
+ hyp.mosaic = hyp.mosaic if self.augment and not self.rect else 0.0
+ hyp.mixup = hyp.mixup if self.augment and not self.rect else 0.0
+ transforms = v8_transforms(self, self.imgsz, hyp, stretch=True)
+ else:
+ # transforms = Compose([LetterBox(new_shape=(self.imgsz, self.imgsz), auto=False, scaleFill=True)])
+ transforms = Compose([])
+ transforms.append(
+ Format(bbox_format='xywh',
+ normalize=True,
+ return_mask=self.use_segments,
+ return_keypoint=self.use_keypoints,
+ batch_idx=True,
+ mask_ratio=hyp.mask_ratio,
+ mask_overlap=hyp.overlap_mask))
+ return transforms
+
+
+class RTDETRValidator(DetectionValidator):
+
+ def build_dataset(self, img_path, mode='val', batch=None):
+ """Build YOLO Dataset
+
+ Args:
+ img_path (str): Path to the folder containing images.
+ mode (str): `train` mode or `val` mode, users are able to customize different augmentations for each mode.
+ batch (int, optional): Size of batches, this is for `rect`. Defaults to None.
+ """
+ return RTDETRDataset(
+ img_path=img_path,
+ imgsz=self.args.imgsz,
+ batch_size=batch,
+ augment=False, # no augmentation
+ hyp=self.args,
+ rect=False, # no rect
+ cache=self.args.cache or None,
+ prefix=colorstr(f'{mode}: '),
+ data=self.data)
+
+ def postprocess(self, preds):
+ """Apply Non-maximum suppression to prediction outputs."""
+ bs, _, nd = preds[0].shape
+ bboxes, scores = preds[0].split((4, nd - 4), dim=-1)
+ bboxes *= self.args.imgsz
+ outputs = [torch.zeros((0, 6), device=bboxes.device)] * bs
+ for i, bbox in enumerate(bboxes): # (300, 4)
+ bbox = ops.xywh2xyxy(bbox)
+ score, cls = scores[i].max(-1) # (300, )
+ # Do not need threshold for evaluation as only got 300 boxes here.
+ # idx = score > self.args.conf
+ pred = torch.cat([bbox, score[..., None], cls[..., None]], dim=-1) # filter
+ # sort by confidence to correctly get internal metrics.
+ pred = pred[score.argsort(descending=True)]
+ outputs[i] = pred # [idx]
+
+ return outputs
+
+ def update_metrics(self, preds, batch):
+ """Metrics."""
+ for si, pred in enumerate(preds):
+ idx = batch['batch_idx'] == si
+ cls = batch['cls'][idx]
+ bbox = batch['bboxes'][idx]
+ nl, npr = cls.shape[0], pred.shape[0] # number of labels, predictions
+ shape = batch['ori_shape'][si]
+ correct_bboxes = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ self.seen += 1
+
+ if npr == 0:
+ if nl:
+ self.stats.append((correct_bboxes, *torch.zeros((2, 0), device=self.device), cls.squeeze(-1)))
+ if self.args.plots:
+ self.confusion_matrix.process_batch(detections=None, labels=cls.squeeze(-1))
+ continue
+
+ # Predictions
+ if self.args.single_cls:
+ pred[:, 5] = 0
+ predn = pred.clone()
+ predn[..., [0, 2]] *= shape[1] / self.args.imgsz # native-space pred
+ predn[..., [1, 3]] *= shape[0] / self.args.imgsz # native-space pred
+
+ # Evaluate
+ if nl:
+ tbox = ops.xywh2xyxy(bbox) # target boxes
+ tbox[..., [0, 2]] *= shape[1] # native-space pred
+ tbox[..., [1, 3]] *= shape[0] # native-space pred
+ labelsn = torch.cat((cls, tbox), 1) # native-space labels
+ # NOTE: To get correct metrics, the inputs of `_process_batch` should always be float32 type.
+ correct_bboxes = self._process_batch(predn.float(), labelsn)
+ # TODO: maybe remove these `self.` arguments as they already are member variable
+ if self.args.plots:
+ self.confusion_matrix.process_batch(predn, labelsn)
+ self.stats.append((correct_bboxes, pred[:, 4], pred[:, 5], cls.squeeze(-1))) # (conf, pcls, tcls)
+
+ # Save
+ if self.args.save_json:
+ self.pred_to_json(predn, batch['im_file'][si])
+ if self.args.save_txt:
+ file = self.save_dir / 'labels' / f'{Path(batch["im_file"][si]).stem}.txt'
+ self.save_one_txt(predn, self.args.save_conf, shape, file)
diff --git a/yolov8/ultralytics/vit/sam/__init__.py b/yolov8/ultralytics/vit/sam/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..b47c04364e5f9d344b767a349864f3970130d6b9
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/__init__.py
@@ -0,0 +1,5 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .build import build_sam # noqa
+from .model import SAM # noqa
+from .modules.prompt_predictor import PromptPredictor # noqa
diff --git a/yolov8/ultralytics/vit/sam/amg.py b/yolov8/ultralytics/vit/sam/amg.py
new file mode 100644
index 0000000000000000000000000000000000000000..29f0bcf84d041cf7c00963156d04408a955152d8
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/amg.py
@@ -0,0 +1,311 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import math
+from copy import deepcopy
+from itertools import product
+from typing import Any, Dict, Generator, ItemsView, List, Tuple
+
+import numpy as np
+import torch
+
+
+class MaskData:
+ """
+ A structure for storing masks and their related data in batched format.
+ Implements basic filtering and concatenation.
+ """
+
+ def __init__(self, **kwargs) -> None:
+ """Initialize a MaskData object, ensuring all values are supported types."""
+ for v in kwargs.values():
+ assert isinstance(
+ v, (list, np.ndarray, torch.Tensor)), 'MaskData only supports list, numpy arrays, and torch tensors.'
+ self._stats = dict(**kwargs)
+
+ def __setitem__(self, key: str, item: Any) -> None:
+ """Set an item in the MaskData object, ensuring it is a supported type."""
+ assert isinstance(
+ item, (list, np.ndarray, torch.Tensor)), 'MaskData only supports list, numpy arrays, and torch tensors.'
+ self._stats[key] = item
+
+ def __delitem__(self, key: str) -> None:
+ """Delete an item from the MaskData object."""
+ del self._stats[key]
+
+ def __getitem__(self, key: str) -> Any:
+ """Get an item from the MaskData object."""
+ return self._stats[key]
+
+ def items(self) -> ItemsView[str, Any]:
+ """Return an ItemsView of the MaskData object."""
+ return self._stats.items()
+
+ def filter(self, keep: torch.Tensor) -> None:
+ """Filter the MaskData object based on the given boolean tensor."""
+ for k, v in self._stats.items():
+ if v is None:
+ self._stats[k] = None
+ elif isinstance(v, torch.Tensor):
+ self._stats[k] = v[torch.as_tensor(keep, device=v.device)]
+ elif isinstance(v, np.ndarray):
+ self._stats[k] = v[keep.detach().cpu().numpy()]
+ elif isinstance(v, list) and keep.dtype == torch.bool:
+ self._stats[k] = [a for i, a in enumerate(v) if keep[i]]
+ elif isinstance(v, list):
+ self._stats[k] = [v[i] for i in keep]
+ else:
+ raise TypeError(f'MaskData key {k} has an unsupported type {type(v)}.')
+
+ def cat(self, new_stats: 'MaskData') -> None:
+ """Concatenate a new MaskData object to the current one."""
+ for k, v in new_stats.items():
+ if k not in self._stats or self._stats[k] is None:
+ self._stats[k] = deepcopy(v)
+ elif isinstance(v, torch.Tensor):
+ self._stats[k] = torch.cat([self._stats[k], v], dim=0)
+ elif isinstance(v, np.ndarray):
+ self._stats[k] = np.concatenate([self._stats[k], v], axis=0)
+ elif isinstance(v, list):
+ self._stats[k] = self._stats[k] + deepcopy(v)
+ else:
+ raise TypeError(f'MaskData key {k} has an unsupported type {type(v)}.')
+
+ def to_numpy(self) -> None:
+ """Convert all torch tensors in the MaskData object to numpy arrays."""
+ for k, v in self._stats.items():
+ if isinstance(v, torch.Tensor):
+ self._stats[k] = v.detach().cpu().numpy()
+
+
+def is_box_near_crop_edge(boxes: torch.Tensor,
+ crop_box: List[int],
+ orig_box: List[int],
+ atol: float = 20.0) -> torch.Tensor:
+ """Return a boolean tensor indicating if boxes are near the crop edge."""
+ crop_box_torch = torch.as_tensor(crop_box, dtype=torch.float, device=boxes.device)
+ orig_box_torch = torch.as_tensor(orig_box, dtype=torch.float, device=boxes.device)
+ boxes = uncrop_boxes_xyxy(boxes, crop_box).float()
+ near_crop_edge = torch.isclose(boxes, crop_box_torch[None, :], atol=atol, rtol=0)
+ near_image_edge = torch.isclose(boxes, orig_box_torch[None, :], atol=atol, rtol=0)
+ near_crop_edge = torch.logical_and(near_crop_edge, ~near_image_edge)
+ return torch.any(near_crop_edge, dim=1)
+
+
+def box_xyxy_to_xywh(box_xyxy: torch.Tensor) -> torch.Tensor:
+ """Convert bounding boxes from XYXY format to XYWH format."""
+ box_xywh = deepcopy(box_xyxy)
+ box_xywh[2] = box_xywh[2] - box_xywh[0]
+ box_xywh[3] = box_xywh[3] - box_xywh[1]
+ return box_xywh
+
+
+def batch_iterator(batch_size: int, *args) -> Generator[List[Any], None, None]:
+ """Yield batches of data from the input arguments."""
+ assert args and all(len(a) == len(args[0]) for a in args), 'Batched iteration must have same-size inputs.'
+ n_batches = len(args[0]) // batch_size + int(len(args[0]) % batch_size != 0)
+ for b in range(n_batches):
+ yield [arg[b * batch_size:(b + 1) * batch_size] for arg in args]
+
+
+def mask_to_rle_pytorch(tensor: torch.Tensor) -> List[Dict[str, Any]]:
+ """Encode masks as uncompressed RLEs in the format expected by pycocotools."""
+ # Put in fortran order and flatten h,w
+ b, h, w = tensor.shape
+ tensor = tensor.permute(0, 2, 1).flatten(1)
+
+ # Compute change indices
+ diff = tensor[:, 1:] ^ tensor[:, :-1]
+ change_indices = diff.nonzero()
+
+ # Encode run length
+ out = []
+ for i in range(b):
+ cur_idxs = change_indices[change_indices[:, 0] == i, 1]
+ cur_idxs = torch.cat([
+ torch.tensor([0], dtype=cur_idxs.dtype, device=cur_idxs.device),
+ cur_idxs + 1,
+ torch.tensor([h * w], dtype=cur_idxs.dtype, device=cur_idxs.device), ])
+ btw_idxs = cur_idxs[1:] - cur_idxs[:-1]
+ counts = [] if tensor[i, 0] == 0 else [0]
+ counts.extend(btw_idxs.detach().cpu().tolist())
+ out.append({'size': [h, w], 'counts': counts})
+ return out
+
+
+def rle_to_mask(rle: Dict[str, Any]) -> np.ndarray:
+ """Compute a binary mask from an uncompressed RLE."""
+ h, w = rle['size']
+ mask = np.empty(h * w, dtype=bool)
+ idx = 0
+ parity = False
+ for count in rle['counts']:
+ mask[idx:idx + count] = parity
+ idx += count
+ parity ^= True
+ mask = mask.reshape(w, h)
+ return mask.transpose() # Put in C order
+
+
+def area_from_rle(rle: Dict[str, Any]) -> int:
+ """Calculate the area of a mask from its uncompressed RLE."""
+ return sum(rle['counts'][1::2])
+
+
+def calculate_stability_score(masks: torch.Tensor, mask_threshold: float, threshold_offset: float) -> torch.Tensor:
+ """
+ Computes the stability score for a batch of masks. The stability
+ score is the IoU between the binary masks obtained by thresholding
+ the predicted mask logits at high and low values.
+ """
+ # One mask is always contained inside the other.
+ # Save memory by preventing unnecessary cast to torch.int64
+ intersections = ((masks > (mask_threshold + threshold_offset)).sum(-1, dtype=torch.int16).sum(-1,
+ dtype=torch.int32))
+ unions = ((masks > (mask_threshold - threshold_offset)).sum(-1, dtype=torch.int16).sum(-1, dtype=torch.int32))
+ return intersections / unions
+
+
+def build_point_grid(n_per_side: int) -> np.ndarray:
+ """Generate a 2D grid of evenly spaced points in the range [0,1]x[0,1]."""
+ offset = 1 / (2 * n_per_side)
+ points_one_side = np.linspace(offset, 1 - offset, n_per_side)
+ points_x = np.tile(points_one_side[None, :], (n_per_side, 1))
+ points_y = np.tile(points_one_side[:, None], (1, n_per_side))
+ return np.stack([points_x, points_y], axis=-1).reshape(-1, 2)
+
+
+def build_all_layer_point_grids(n_per_side: int, n_layers: int, scale_per_layer: int) -> List[np.ndarray]:
+ """Generate point grids for all crop layers."""
+ return [build_point_grid(int(n_per_side / (scale_per_layer ** i))) for i in range(n_layers + 1)]
+
+
+def generate_crop_boxes(im_size: Tuple[int, ...], n_layers: int,
+ overlap_ratio: float) -> Tuple[List[List[int]], List[int]]:
+ """Generates a list of crop boxes of different sizes. Each layer has (2**i)**2 boxes for the ith layer."""
+ crop_boxes, layer_idxs = [], []
+ im_h, im_w = im_size
+ short_side = min(im_h, im_w)
+
+ # Original image
+ crop_boxes.append([0, 0, im_w, im_h])
+ layer_idxs.append(0)
+
+ def crop_len(orig_len, n_crops, overlap):
+ """Crops bounding boxes to the size of the input image."""
+ return int(math.ceil((overlap * (n_crops - 1) + orig_len) / n_crops))
+
+ for i_layer in range(n_layers):
+ n_crops_per_side = 2 ** (i_layer + 1)
+ overlap = int(overlap_ratio * short_side * (2 / n_crops_per_side))
+
+ crop_w = crop_len(im_w, n_crops_per_side, overlap)
+ crop_h = crop_len(im_h, n_crops_per_side, overlap)
+
+ crop_box_x0 = [int((crop_w - overlap) * i) for i in range(n_crops_per_side)]
+ crop_box_y0 = [int((crop_h - overlap) * i) for i in range(n_crops_per_side)]
+
+ # Crops in XYWH format
+ for x0, y0 in product(crop_box_x0, crop_box_y0):
+ box = [x0, y0, min(x0 + crop_w, im_w), min(y0 + crop_h, im_h)]
+ crop_boxes.append(box)
+ layer_idxs.append(i_layer + 1)
+
+ return crop_boxes, layer_idxs
+
+
+def uncrop_boxes_xyxy(boxes: torch.Tensor, crop_box: List[int]) -> torch.Tensor:
+ """Uncrop bounding boxes by adding the crop box offset."""
+ x0, y0, _, _ = crop_box
+ offset = torch.tensor([[x0, y0, x0, y0]], device=boxes.device)
+ # Check if boxes has a channel dimension
+ if len(boxes.shape) == 3:
+ offset = offset.unsqueeze(1)
+ return boxes + offset
+
+
+def uncrop_points(points: torch.Tensor, crop_box: List[int]) -> torch.Tensor:
+ """Uncrop points by adding the crop box offset."""
+ x0, y0, _, _ = crop_box
+ offset = torch.tensor([[x0, y0]], device=points.device)
+ # Check if points has a channel dimension
+ if len(points.shape) == 3:
+ offset = offset.unsqueeze(1)
+ return points + offset
+
+
+def uncrop_masks(masks: torch.Tensor, crop_box: List[int], orig_h: int, orig_w: int) -> torch.Tensor:
+ """Uncrop masks by padding them to the original image size."""
+ x0, y0, x1, y1 = crop_box
+ if x0 == 0 and y0 == 0 and x1 == orig_w and y1 == orig_h:
+ return masks
+ # Coordinate transform masks
+ pad_x, pad_y = orig_w - (x1 - x0), orig_h - (y1 - y0)
+ pad = (x0, pad_x - x0, y0, pad_y - y0)
+ return torch.nn.functional.pad(masks, pad, value=0)
+
+
+def remove_small_regions(mask: np.ndarray, area_thresh: float, mode: str) -> Tuple[np.ndarray, bool]:
+ """Remove small disconnected regions or holes in a mask, returning the mask and a modification indicator."""
+ import cv2 # type: ignore
+
+ assert mode in {'holes', 'islands'}
+ correct_holes = mode == 'holes'
+ working_mask = (correct_holes ^ mask).astype(np.uint8)
+ n_labels, regions, stats, _ = cv2.connectedComponentsWithStats(working_mask, 8)
+ sizes = stats[:, -1][1:] # Row 0 is background label
+ small_regions = [i + 1 for i, s in enumerate(sizes) if s < area_thresh]
+ if not small_regions:
+ return mask, False
+ fill_labels = [0] + small_regions
+ if not correct_holes:
+ # If every region is below threshold, keep largest
+ fill_labels = [i for i in range(n_labels) if i not in fill_labels] or [int(np.argmax(sizes)) + 1]
+ mask = np.isin(regions, fill_labels)
+ return mask, True
+
+
+def coco_encode_rle(uncompressed_rle: Dict[str, Any]) -> Dict[str, Any]:
+ """Encode uncompressed RLE (run-length encoding) to COCO RLE format."""
+ from pycocotools import mask as mask_utils # type: ignore
+
+ h, w = uncompressed_rle['size']
+ rle = mask_utils.frPyObjects(uncompressed_rle, h, w)
+ rle['counts'] = rle['counts'].decode('utf-8') # Necessary to serialize with json
+ return rle
+
+
+def batched_mask_to_box(masks: torch.Tensor) -> torch.Tensor:
+ """
+ Calculates boxes in XYXY format around masks. Return [0,0,0,0] for
+ an empty mask. For input shape C1xC2x...xHxW, the output shape is C1xC2x...x4.
+ """
+ # torch.max below raises an error on empty inputs, just skip in this case
+ if torch.numel(masks) == 0:
+ return torch.zeros(*masks.shape[:-2], 4, device=masks.device)
+
+ # Normalize shape to CxHxW
+ shape = masks.shape
+ h, w = shape[-2:]
+ masks = masks.flatten(0, -3) if len(shape) > 2 else masks.unsqueeze(0)
+ # Get top and bottom edges
+ in_height, _ = torch.max(masks, dim=-1)
+ in_height_coords = in_height * torch.arange(h, device=in_height.device)[None, :]
+ bottom_edges, _ = torch.max(in_height_coords, dim=-1)
+ in_height_coords = in_height_coords + h * (~in_height)
+ top_edges, _ = torch.min(in_height_coords, dim=-1)
+
+ # Get left and right edges
+ in_width, _ = torch.max(masks, dim=-2)
+ in_width_coords = in_width * torch.arange(w, device=in_width.device)[None, :]
+ right_edges, _ = torch.max(in_width_coords, dim=-1)
+ in_width_coords = in_width_coords + w * (~in_width)
+ left_edges, _ = torch.min(in_width_coords, dim=-1)
+
+ # If the mask is empty the right edge will be to the left of the left edge.
+ # Replace these boxes with [0, 0, 0, 0]
+ empty_filter = (right_edges < left_edges) | (bottom_edges < top_edges)
+ out = torch.stack([left_edges, top_edges, right_edges, bottom_edges], dim=-1)
+ out = out * (~empty_filter).unsqueeze(-1)
+
+ # Return to original shape
+ return out.reshape(*shape[:-2], 4) if len(shape) > 2 else out[0]
diff --git a/yolov8/ultralytics/vit/sam/autosize.py b/yolov8/ultralytics/vit/sam/autosize.py
new file mode 100644
index 0000000000000000000000000000000000000000..ef3364454022edc85ae9b856aeaf8aaf4bc187b2
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/autosize.py
@@ -0,0 +1,94 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+# Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+from copy import deepcopy
+from typing import Tuple
+
+import numpy as np
+import torch
+from torch.nn import functional as F
+from torchvision.transforms.functional import resize, to_pil_image # type: ignore
+
+
+class ResizeLongestSide:
+ """
+ Resizes images to the longest side 'target_length', as well as provides
+ methods for resizing coordinates and boxes. Provides methods for
+ transforming both numpy array and batched torch tensors.
+ """
+
+ def __init__(self, target_length: int) -> None:
+ self.target_length = target_length
+
+ def apply_image(self, image: np.ndarray) -> np.ndarray:
+ """
+ Expects a numpy array with shape HxWxC in uint8 format.
+ """
+ target_size = self.get_preprocess_shape(image.shape[0], image.shape[1], self.target_length)
+ return np.array(resize(to_pil_image(image), target_size))
+
+ def apply_coords(self, coords: np.ndarray, original_size: Tuple[int, ...]) -> np.ndarray:
+ """
+ Expects a numpy array of length 2 in the final dimension. Requires the
+ original image size in (H, W) format.
+ """
+ old_h, old_w = original_size
+ new_h, new_w = self.get_preprocess_shape(original_size[0], original_size[1], self.target_length)
+ coords = deepcopy(coords).astype(float)
+ coords[..., 0] = coords[..., 0] * (new_w / old_w)
+ coords[..., 1] = coords[..., 1] * (new_h / old_h)
+ return coords
+
+ def apply_boxes(self, boxes: np.ndarray, original_size: Tuple[int, ...]) -> np.ndarray:
+ """
+ Expects a numpy array shape Bx4. Requires the original image size
+ in (H, W) format.
+ """
+ boxes = self.apply_coords(boxes.reshape(-1, 2, 2), original_size)
+ return boxes.reshape(-1, 4)
+
+ def apply_image_torch(self, image: torch.Tensor) -> torch.Tensor:
+ """
+ Expects batched images with shape BxCxHxW and float format. This
+ transformation may not exactly match apply_image. apply_image is
+ the transformation expected by the model.
+ """
+ # Expects an image in BCHW format. May not exactly match apply_image.
+ target_size = self.get_preprocess_shape(image.shape[2], image.shape[3], self.target_length)
+ return F.interpolate(image, target_size, mode='bilinear', align_corners=False, antialias=True)
+
+ def apply_coords_torch(self, coords: torch.Tensor, original_size: Tuple[int, ...]) -> torch.Tensor:
+ """
+ Expects a torch tensor with length 2 in the last dimension. Requires the
+ original image size in (H, W) format.
+ """
+ old_h, old_w = original_size
+ new_h, new_w = self.get_preprocess_shape(original_size[0], original_size[1], self.target_length)
+ coords = deepcopy(coords).to(torch.float)
+ coords[..., 0] = coords[..., 0] * (new_w / old_w)
+ coords[..., 1] = coords[..., 1] * (new_h / old_h)
+ return coords
+
+ def apply_boxes_torch(self, boxes: torch.Tensor, original_size: Tuple[int, ...]) -> torch.Tensor:
+ """
+ Expects a torch tensor with shape Bx4. Requires the original image
+ size in (H, W) format.
+ """
+ boxes = self.apply_coords_torch(boxes.reshape(-1, 2, 2), original_size)
+ return boxes.reshape(-1, 4)
+
+ @staticmethod
+ def get_preprocess_shape(oldh: int, oldw: int, long_side_length: int) -> Tuple[int, int]:
+ """
+ Compute the output size given input size and target long side length.
+ """
+ scale = long_side_length * 1.0 / max(oldh, oldw)
+ newh, neww = oldh * scale, oldw * scale
+ neww = int(neww + 0.5)
+ newh = int(newh + 0.5)
+ return (newh, neww)
diff --git a/yolov8/ultralytics/vit/sam/build.py b/yolov8/ultralytics/vit/sam/build.py
new file mode 100644
index 0000000000000000000000000000000000000000..b2e098649d08d44c9542cfd63cee803d4247d11f
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/build.py
@@ -0,0 +1,127 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+# Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+from functools import partial
+
+import torch
+
+from ...yolo.utils.downloads import attempt_download_asset
+from .modules.decoders import MaskDecoder
+from .modules.encoders import ImageEncoderViT, PromptEncoder
+from .modules.sam import Sam
+from .modules.transformer import TwoWayTransformer
+
+
+def build_sam_vit_h(checkpoint=None):
+ """Build and return a Segment Anything Model (SAM) h-size model."""
+ return _build_sam(
+ encoder_embed_dim=1280,
+ encoder_depth=32,
+ encoder_num_heads=16,
+ encoder_global_attn_indexes=[7, 15, 23, 31],
+ checkpoint=checkpoint,
+ )
+
+
+def build_sam_vit_l(checkpoint=None):
+ """Build and return a Segment Anything Model (SAM) l-size model."""
+ return _build_sam(
+ encoder_embed_dim=1024,
+ encoder_depth=24,
+ encoder_num_heads=16,
+ encoder_global_attn_indexes=[5, 11, 17, 23],
+ checkpoint=checkpoint,
+ )
+
+
+def build_sam_vit_b(checkpoint=None):
+ """Build and return a Segment Anything Model (SAM) b-size model."""
+ return _build_sam(
+ encoder_embed_dim=768,
+ encoder_depth=12,
+ encoder_num_heads=12,
+ encoder_global_attn_indexes=[2, 5, 8, 11],
+ checkpoint=checkpoint,
+ )
+
+
+def _build_sam(
+ encoder_embed_dim,
+ encoder_depth,
+ encoder_num_heads,
+ encoder_global_attn_indexes,
+ checkpoint=None,
+):
+ """Builds the selected SAM model architecture."""
+ prompt_embed_dim = 256
+ image_size = 1024
+ vit_patch_size = 16
+ image_embedding_size = image_size // vit_patch_size
+ sam = Sam(
+ image_encoder=ImageEncoderViT(
+ depth=encoder_depth,
+ embed_dim=encoder_embed_dim,
+ img_size=image_size,
+ mlp_ratio=4,
+ norm_layer=partial(torch.nn.LayerNorm, eps=1e-6),
+ num_heads=encoder_num_heads,
+ patch_size=vit_patch_size,
+ qkv_bias=True,
+ use_rel_pos=True,
+ global_attn_indexes=encoder_global_attn_indexes,
+ window_size=14,
+ out_chans=prompt_embed_dim,
+ ),
+ prompt_encoder=PromptEncoder(
+ embed_dim=prompt_embed_dim,
+ image_embedding_size=(image_embedding_size, image_embedding_size),
+ input_image_size=(image_size, image_size),
+ mask_in_chans=16,
+ ),
+ mask_decoder=MaskDecoder(
+ num_multimask_outputs=3,
+ transformer=TwoWayTransformer(
+ depth=2,
+ embedding_dim=prompt_embed_dim,
+ mlp_dim=2048,
+ num_heads=8,
+ ),
+ transformer_dim=prompt_embed_dim,
+ iou_head_depth=3,
+ iou_head_hidden_dim=256,
+ ),
+ pixel_mean=[123.675, 116.28, 103.53],
+ pixel_std=[58.395, 57.12, 57.375],
+ )
+ sam.eval()
+ if checkpoint is not None:
+ attempt_download_asset(checkpoint)
+ with open(checkpoint, 'rb') as f:
+ state_dict = torch.load(f)
+ sam.load_state_dict(state_dict)
+ return sam
+
+
+sam_model_map = {
+ # "default": build_sam_vit_h,
+ 'sam_h.pt': build_sam_vit_h,
+ 'sam_l.pt': build_sam_vit_l,
+ 'sam_b.pt': build_sam_vit_b, }
+
+
+def build_sam(ckpt='sam_b.pt'):
+ """Build a SAM model specified by ckpt."""
+ model_builder = None
+ for k in sam_model_map.keys():
+ if ckpt.endswith(k):
+ model_builder = sam_model_map.get(k)
+
+ if not model_builder:
+ raise FileNotFoundError(f'{ckpt} is not a supported sam model. Available models are: \n {sam_model_map.keys()}')
+
+ return model_builder(ckpt)
diff --git a/yolov8/ultralytics/vit/sam/model.py b/yolov8/ultralytics/vit/sam/model.py
new file mode 100644
index 0000000000000000000000000000000000000000..83861f4b9cafaf323a1a690b3443ffeb6c891fbd
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/model.py
@@ -0,0 +1,59 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+SAM model interface
+"""
+
+from ultralytics.yolo.cfg import get_cfg
+
+from ...yolo.utils.torch_utils import model_info
+from .build import build_sam
+from .predict import Predictor
+
+
+class SAM:
+
+ def __init__(self, model='sam_b.pt') -> None:
+ if model and not model.endswith('.pt') and not model.endswith('.pth'):
+ # Should raise AssertionError instead?
+ raise NotImplementedError('Segment anything prediction requires pre-trained checkpoint')
+ self.model = build_sam(model)
+ self.task = 'segment' # required
+ self.predictor = None # reuse predictor
+
+ def predict(self, source, stream=False, **kwargs):
+ """Predicts and returns segmentation masks for given image or video source."""
+ overrides = dict(conf=0.25, task='segment', mode='predict')
+ overrides.update(kwargs) # prefer kwargs
+ if not self.predictor:
+ self.predictor = Predictor(overrides=overrides)
+ self.predictor.setup_model(model=self.model)
+ else: # only update args if predictor is already setup
+ self.predictor.args = get_cfg(self.predictor.args, overrides)
+ return self.predictor(source, stream=stream)
+
+ def train(self, **kwargs):
+ """Function trains models but raises an error as SAM models do not support training."""
+ raise NotImplementedError("SAM models don't support training")
+
+ def val(self, **kwargs):
+ """Run validation given dataset."""
+ raise NotImplementedError("SAM models don't support validation")
+
+ def __call__(self, source=None, stream=False, **kwargs):
+ """Calls the 'predict' function with given arguments to perform object detection."""
+ return self.predict(source, stream, **kwargs)
+
+ def __getattr__(self, attr):
+ """Raises error if object has no requested attribute."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
+
+ def info(self, detailed=False, verbose=True):
+ """
+ Logs model info.
+
+ Args:
+ detailed (bool): Show detailed information about model.
+ verbose (bool): Controls verbosity.
+ """
+ return model_info(self.model, detailed=detailed, verbose=verbose)
diff --git a/yolov8/ultralytics/vit/sam/modules/__init__.py b/yolov8/ultralytics/vit/sam/modules/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..9e68dc12245afb4f72ba5f7c1227df74613a427d
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/__init__.py
@@ -0,0 +1 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
diff --git a/yolov8/ultralytics/vit/sam/modules/decoders.py b/yolov8/ultralytics/vit/sam/modules/decoders.py
new file mode 100644
index 0000000000000000000000000000000000000000..cadc0f0fc29bb0e36a7385bcc8cbedde9cb87b62
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/decoders.py
@@ -0,0 +1,159 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from typing import List, Tuple, Type
+
+import torch
+from torch import nn
+from torch.nn import functional as F
+
+from ultralytics.nn.modules import LayerNorm2d
+
+
+class MaskDecoder(nn.Module):
+
+ def __init__(
+ self,
+ *,
+ transformer_dim: int,
+ transformer: nn.Module,
+ num_multimask_outputs: int = 3,
+ activation: Type[nn.Module] = nn.GELU,
+ iou_head_depth: int = 3,
+ iou_head_hidden_dim: int = 256,
+ ) -> None:
+ """
+ Predicts masks given an image and prompt embeddings, using a transformer architecture.
+
+ Arguments:
+ transformer_dim (int): the channel dimension of the transformer module
+ transformer (nn.Module): the transformer used to predict masks
+ num_multimask_outputs (int): the number of masks to predict when disambiguating masks
+ activation (nn.Module): the type of activation to use when upscaling masks
+ iou_head_depth (int): the depth of the MLP used to predict mask quality
+ iou_head_hidden_dim (int): the hidden dimension of the MLP used to predict mask quality
+ """
+ super().__init__()
+ self.transformer_dim = transformer_dim
+ self.transformer = transformer
+
+ self.num_multimask_outputs = num_multimask_outputs
+
+ self.iou_token = nn.Embedding(1, transformer_dim)
+ self.num_mask_tokens = num_multimask_outputs + 1
+ self.mask_tokens = nn.Embedding(self.num_mask_tokens, transformer_dim)
+
+ self.output_upscaling = nn.Sequential(
+ nn.ConvTranspose2d(transformer_dim, transformer_dim // 4, kernel_size=2, stride=2),
+ LayerNorm2d(transformer_dim // 4),
+ activation(),
+ nn.ConvTranspose2d(transformer_dim // 4, transformer_dim // 8, kernel_size=2, stride=2),
+ activation(),
+ )
+ self.output_hypernetworks_mlps = nn.ModuleList([
+ MLP(transformer_dim, transformer_dim, transformer_dim // 8, 3) for _ in range(self.num_mask_tokens)])
+
+ self.iou_prediction_head = MLP(transformer_dim, iou_head_hidden_dim, self.num_mask_tokens, iou_head_depth)
+
+ def forward(
+ self,
+ image_embeddings: torch.Tensor,
+ image_pe: torch.Tensor,
+ sparse_prompt_embeddings: torch.Tensor,
+ dense_prompt_embeddings: torch.Tensor,
+ multimask_output: bool,
+ ) -> Tuple[torch.Tensor, torch.Tensor]:
+ """
+ Predict masks given image and prompt embeddings.
+
+ Arguments:
+ image_embeddings (torch.Tensor): the embeddings from the image encoder
+ image_pe (torch.Tensor): positional encoding with the shape of image_embeddings
+ sparse_prompt_embeddings (torch.Tensor): the embeddings of the points and boxes
+ dense_prompt_embeddings (torch.Tensor): the embeddings of the mask inputs
+ multimask_output (bool): Whether to return multiple masks or a single mask.
+
+ Returns:
+ torch.Tensor: batched predicted masks
+ torch.Tensor: batched predictions of mask quality
+ """
+ masks, iou_pred = self.predict_masks(
+ image_embeddings=image_embeddings,
+ image_pe=image_pe,
+ sparse_prompt_embeddings=sparse_prompt_embeddings,
+ dense_prompt_embeddings=dense_prompt_embeddings,
+ )
+
+ # Select the correct mask or masks for output
+ mask_slice = slice(1, None) if multimask_output else slice(0, 1)
+ masks = masks[:, mask_slice, :, :]
+ iou_pred = iou_pred[:, mask_slice]
+
+ # Prepare output
+ return masks, iou_pred
+
+ def predict_masks(
+ self,
+ image_embeddings: torch.Tensor,
+ image_pe: torch.Tensor,
+ sparse_prompt_embeddings: torch.Tensor,
+ dense_prompt_embeddings: torch.Tensor,
+ ) -> Tuple[torch.Tensor, torch.Tensor]:
+ """Predicts masks. See 'forward' for more details."""
+ # Concatenate output tokens
+ output_tokens = torch.cat([self.iou_token.weight, self.mask_tokens.weight], dim=0)
+ output_tokens = output_tokens.unsqueeze(0).expand(sparse_prompt_embeddings.size(0), -1, -1)
+ tokens = torch.cat((output_tokens, sparse_prompt_embeddings), dim=1)
+
+ # Expand per-image data in batch direction to be per-mask
+ src = torch.repeat_interleave(image_embeddings, tokens.shape[0], dim=0)
+ src = src + dense_prompt_embeddings
+ pos_src = torch.repeat_interleave(image_pe, tokens.shape[0], dim=0)
+ b, c, h, w = src.shape
+
+ # Run the transformer
+ hs, src = self.transformer(src, pos_src, tokens)
+ iou_token_out = hs[:, 0, :]
+ mask_tokens_out = hs[:, 1:(1 + self.num_mask_tokens), :]
+
+ # Upscale mask embeddings and predict masks using the mask tokens
+ src = src.transpose(1, 2).view(b, c, h, w)
+ upscaled_embedding = self.output_upscaling(src)
+ hyper_in_list: List[torch.Tensor] = [
+ self.output_hypernetworks_mlps[i](mask_tokens_out[:, i, :]) for i in range(self.num_mask_tokens)]
+ hyper_in = torch.stack(hyper_in_list, dim=1)
+ b, c, h, w = upscaled_embedding.shape
+ masks = (hyper_in @ upscaled_embedding.view(b, c, h * w)).view(b, -1, h, w)
+
+ # Generate mask quality predictions
+ iou_pred = self.iou_prediction_head(iou_token_out)
+
+ return masks, iou_pred
+
+
+class MLP(nn.Module):
+ """
+ Lightly adapted from
+ https://github.com/facebookresearch/MaskFormer/blob/main/mask_former/modeling/transformer/transformer_predictor.py
+ """
+
+ def __init__(
+ self,
+ input_dim: int,
+ hidden_dim: int,
+ output_dim: int,
+ num_layers: int,
+ sigmoid_output: bool = False,
+ ) -> None:
+ super().__init__()
+ self.num_layers = num_layers
+ h = [hidden_dim] * (num_layers - 1)
+ self.layers = nn.ModuleList(nn.Linear(n, k) for n, k in zip([input_dim] + h, h + [output_dim]))
+ self.sigmoid_output = sigmoid_output
+
+ def forward(self, x):
+ """Executes feedforward within the neural network module and applies activation."""
+ for i, layer in enumerate(self.layers):
+ x = F.relu(layer(x)) if i < self.num_layers - 1 else layer(x)
+ if self.sigmoid_output:
+ x = torch.sigmoid(x)
+ return x
diff --git a/yolov8/ultralytics/vit/sam/modules/encoders.py b/yolov8/ultralytics/vit/sam/modules/encoders.py
new file mode 100644
index 0000000000000000000000000000000000000000..0da032dddb15d3d104b2173e5835189dfa72f1b6
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/encoders.py
@@ -0,0 +1,583 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from typing import Any, Optional, Tuple, Type
+
+import numpy as np
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+
+from ultralytics.nn.modules import LayerNorm2d, MLPBlock
+
+
+# This class and its supporting functions below lightly adapted from the ViTDet backbone available at: https://github.com/facebookresearch/detectron2/blob/main/detectron2/modeling/backbone/vit.py # noqa
+class ImageEncoderViT(nn.Module):
+
+ def __init__(
+ self,
+ img_size: int = 1024,
+ patch_size: int = 16,
+ in_chans: int = 3,
+ embed_dim: int = 768,
+ depth: int = 12,
+ num_heads: int = 12,
+ mlp_ratio: float = 4.0,
+ out_chans: int = 256,
+ qkv_bias: bool = True,
+ norm_layer: Type[nn.Module] = nn.LayerNorm,
+ act_layer: Type[nn.Module] = nn.GELU,
+ use_abs_pos: bool = True,
+ use_rel_pos: bool = False,
+ rel_pos_zero_init: bool = True,
+ window_size: int = 0,
+ global_attn_indexes: Tuple[int, ...] = (),
+ ) -> None:
+ """
+ Args:
+ img_size (int): Input image size.
+ patch_size (int): Patch size.
+ in_chans (int): Number of input image channels.
+ embed_dim (int): Patch embedding dimension.
+ depth (int): Depth of ViT.
+ num_heads (int): Number of attention heads in each ViT block.
+ mlp_ratio (float): Ratio of mlp hidden dim to embedding dim.
+ qkv_bias (bool): If True, add a learnable bias to query, key, value.
+ norm_layer (nn.Module): Normalization layer.
+ act_layer (nn.Module): Activation layer.
+ use_abs_pos (bool): If True, use absolute positional embeddings.
+ use_rel_pos (bool): If True, add relative positional embeddings to the attention map.
+ rel_pos_zero_init (bool): If True, zero initialize relative positional parameters.
+ window_size (int): Window size for window attention blocks.
+ global_attn_indexes (list): Indexes for blocks using global attention.
+ """
+ super().__init__()
+ self.img_size = img_size
+
+ self.patch_embed = PatchEmbed(
+ kernel_size=(patch_size, patch_size),
+ stride=(patch_size, patch_size),
+ in_chans=in_chans,
+ embed_dim=embed_dim,
+ )
+
+ self.pos_embed: Optional[nn.Parameter] = None
+ if use_abs_pos:
+ # Initialize absolute positional embedding with pretrain image size.
+ self.pos_embed = nn.Parameter(torch.zeros(1, img_size // patch_size, img_size // patch_size, embed_dim))
+
+ self.blocks = nn.ModuleList()
+ for i in range(depth):
+ block = Block(
+ dim=embed_dim,
+ num_heads=num_heads,
+ mlp_ratio=mlp_ratio,
+ qkv_bias=qkv_bias,
+ norm_layer=norm_layer,
+ act_layer=act_layer,
+ use_rel_pos=use_rel_pos,
+ rel_pos_zero_init=rel_pos_zero_init,
+ window_size=window_size if i not in global_attn_indexes else 0,
+ input_size=(img_size // patch_size, img_size // patch_size),
+ )
+ self.blocks.append(block)
+
+ self.neck = nn.Sequential(
+ nn.Conv2d(
+ embed_dim,
+ out_chans,
+ kernel_size=1,
+ bias=False,
+ ),
+ LayerNorm2d(out_chans),
+ nn.Conv2d(
+ out_chans,
+ out_chans,
+ kernel_size=3,
+ padding=1,
+ bias=False,
+ ),
+ LayerNorm2d(out_chans),
+ )
+
+ def forward(self, x: torch.Tensor) -> torch.Tensor:
+ x = self.patch_embed(x)
+ if self.pos_embed is not None:
+ x = x + self.pos_embed
+
+ for blk in self.blocks:
+ x = blk(x)
+
+ x = self.neck(x.permute(0, 3, 1, 2))
+
+ return x
+
+
+class PromptEncoder(nn.Module):
+
+ def __init__(
+ self,
+ embed_dim: int,
+ image_embedding_size: Tuple[int, int],
+ input_image_size: Tuple[int, int],
+ mask_in_chans: int,
+ activation: Type[nn.Module] = nn.GELU,
+ ) -> None:
+ """
+ Encodes prompts for input to SAM's mask decoder.
+
+ Arguments:
+ embed_dim (int): The prompts' embedding dimension
+ image_embedding_size (tuple(int, int)): The spatial size of the
+ image embedding, as (H, W).
+ input_image_size (int): The padded size of the image as input
+ to the image encoder, as (H, W).
+ mask_in_chans (int): The number of hidden channels used for
+ encoding input masks.
+ activation (nn.Module): The activation to use when encoding
+ input masks.
+ """
+ super().__init__()
+ self.embed_dim = embed_dim
+ self.input_image_size = input_image_size
+ self.image_embedding_size = image_embedding_size
+ self.pe_layer = PositionEmbeddingRandom(embed_dim // 2)
+
+ self.num_point_embeddings: int = 4 # pos/neg point + 2 box corners
+ point_embeddings = [nn.Embedding(1, embed_dim) for _ in range(self.num_point_embeddings)]
+ self.point_embeddings = nn.ModuleList(point_embeddings)
+ self.not_a_point_embed = nn.Embedding(1, embed_dim)
+
+ self.mask_input_size = (4 * image_embedding_size[0], 4 * image_embedding_size[1])
+ self.mask_downscaling = nn.Sequential(
+ nn.Conv2d(1, mask_in_chans // 4, kernel_size=2, stride=2),
+ LayerNorm2d(mask_in_chans // 4),
+ activation(),
+ nn.Conv2d(mask_in_chans // 4, mask_in_chans, kernel_size=2, stride=2),
+ LayerNorm2d(mask_in_chans),
+ activation(),
+ nn.Conv2d(mask_in_chans, embed_dim, kernel_size=1),
+ )
+ self.no_mask_embed = nn.Embedding(1, embed_dim)
+
+ def get_dense_pe(self) -> torch.Tensor:
+ """
+ Returns the positional encoding used to encode point prompts,
+ applied to a dense set of points the shape of the image encoding.
+
+ Returns:
+ torch.Tensor: Positional encoding with shape
+ 1x(embed_dim)x(embedding_h)x(embedding_w)
+ """
+ return self.pe_layer(self.image_embedding_size).unsqueeze(0)
+
+ def _embed_points(
+ self,
+ points: torch.Tensor,
+ labels: torch.Tensor,
+ pad: bool,
+ ) -> torch.Tensor:
+ """Embeds point prompts."""
+ points = points + 0.5 # Shift to center of pixel
+ if pad:
+ padding_point = torch.zeros((points.shape[0], 1, 2), device=points.device)
+ padding_label = -torch.ones((labels.shape[0], 1), device=labels.device)
+ points = torch.cat([points, padding_point], dim=1)
+ labels = torch.cat([labels, padding_label], dim=1)
+ point_embedding = self.pe_layer.forward_with_coords(points, self.input_image_size)
+ point_embedding[labels == -1] = 0.0
+ point_embedding[labels == -1] += self.not_a_point_embed.weight
+ point_embedding[labels == 0] += self.point_embeddings[0].weight
+ point_embedding[labels == 1] += self.point_embeddings[1].weight
+ return point_embedding
+
+ def _embed_boxes(self, boxes: torch.Tensor) -> torch.Tensor:
+ """Embeds box prompts."""
+ boxes = boxes + 0.5 # Shift to center of pixel
+ coords = boxes.reshape(-1, 2, 2)
+ corner_embedding = self.pe_layer.forward_with_coords(coords, self.input_image_size)
+ corner_embedding[:, 0, :] += self.point_embeddings[2].weight
+ corner_embedding[:, 1, :] += self.point_embeddings[3].weight
+ return corner_embedding
+
+ def _embed_masks(self, masks: torch.Tensor) -> torch.Tensor:
+ """Embeds mask inputs."""
+ return self.mask_downscaling(masks)
+
+ def _get_batch_size(
+ self,
+ points: Optional[Tuple[torch.Tensor, torch.Tensor]],
+ boxes: Optional[torch.Tensor],
+ masks: Optional[torch.Tensor],
+ ) -> int:
+ """
+ Gets the batch size of the output given the batch size of the input prompts.
+ """
+ if points is not None:
+ return points[0].shape[0]
+ elif boxes is not None:
+ return boxes.shape[0]
+ elif masks is not None:
+ return masks.shape[0]
+ else:
+ return 1
+
+ def _get_device(self) -> torch.device:
+ return self.point_embeddings[0].weight.device
+
+ def forward(
+ self,
+ points: Optional[Tuple[torch.Tensor, torch.Tensor]],
+ boxes: Optional[torch.Tensor],
+ masks: Optional[torch.Tensor],
+ ) -> Tuple[torch.Tensor, torch.Tensor]:
+ """
+ Embeds different types of prompts, returning both sparse and dense
+ embeddings.
+
+ Arguments:
+ points (tuple(torch.Tensor, torch.Tensor), None): point coordinates
+ and labels to embed.
+ boxes (torch.Tensor, None): boxes to embed
+ masks (torch.Tensor, None): masks to embed
+
+ Returns:
+ torch.Tensor: sparse embeddings for the points and boxes, with shape
+ BxNx(embed_dim), where N is determined by the number of input points
+ and boxes.
+ torch.Tensor: dense embeddings for the masks, in the shape
+ Bx(embed_dim)x(embed_H)x(embed_W)
+ """
+ bs = self._get_batch_size(points, boxes, masks)
+ sparse_embeddings = torch.empty((bs, 0, self.embed_dim), device=self._get_device())
+ if points is not None:
+ coords, labels = points
+ point_embeddings = self._embed_points(coords, labels, pad=(boxes is None))
+ sparse_embeddings = torch.cat([sparse_embeddings, point_embeddings], dim=1)
+ if boxes is not None:
+ box_embeddings = self._embed_boxes(boxes)
+ sparse_embeddings = torch.cat([sparse_embeddings, box_embeddings], dim=1)
+
+ if masks is not None:
+ dense_embeddings = self._embed_masks(masks)
+ else:
+ dense_embeddings = self.no_mask_embed.weight.reshape(1, -1, 1,
+ 1).expand(bs, -1, self.image_embedding_size[0],
+ self.image_embedding_size[1])
+
+ return sparse_embeddings, dense_embeddings
+
+
+class PositionEmbeddingRandom(nn.Module):
+ """
+ Positional encoding using random spatial frequencies.
+ """
+
+ def __init__(self, num_pos_feats: int = 64, scale: Optional[float] = None) -> None:
+ super().__init__()
+ if scale is None or scale <= 0.0:
+ scale = 1.0
+ self.register_buffer(
+ 'positional_encoding_gaussian_matrix',
+ scale * torch.randn((2, num_pos_feats)),
+ )
+
+ def _pe_encoding(self, coords: torch.Tensor) -> torch.Tensor:
+ """Positionally encode points that are normalized to [0,1]."""
+ # assuming coords are in [0, 1]^2 square and have d_1 x ... x d_n x 2 shape
+ coords = 2 * coords - 1
+ coords = coords @ self.positional_encoding_gaussian_matrix
+ coords = 2 * np.pi * coords
+ # outputs d_1 x ... x d_n x C shape
+ return torch.cat([torch.sin(coords), torch.cos(coords)], dim=-1)
+
+ def forward(self, size: Tuple[int, int]) -> torch.Tensor:
+ """Generate positional encoding for a grid of the specified size."""
+ h, w = size
+ device: Any = self.positional_encoding_gaussian_matrix.device
+ grid = torch.ones((h, w), device=device, dtype=torch.float32)
+ y_embed = grid.cumsum(dim=0) - 0.5
+ x_embed = grid.cumsum(dim=1) - 0.5
+ y_embed = y_embed / h
+ x_embed = x_embed / w
+
+ pe = self._pe_encoding(torch.stack([x_embed, y_embed], dim=-1))
+ return pe.permute(2, 0, 1) # C x H x W
+
+ def forward_with_coords(self, coords_input: torch.Tensor, image_size: Tuple[int, int]) -> torch.Tensor:
+ """Positionally encode points that are not normalized to [0,1]."""
+ coords = coords_input.clone()
+ coords[:, :, 0] = coords[:, :, 0] / image_size[1]
+ coords[:, :, 1] = coords[:, :, 1] / image_size[0]
+ return self._pe_encoding(coords.to(torch.float)) # B x N x C
+
+
+class Block(nn.Module):
+ """Transformer blocks with support of window attention and residual propagation blocks"""
+
+ def __init__(
+ self,
+ dim: int,
+ num_heads: int,
+ mlp_ratio: float = 4.0,
+ qkv_bias: bool = True,
+ norm_layer: Type[nn.Module] = nn.LayerNorm,
+ act_layer: Type[nn.Module] = nn.GELU,
+ use_rel_pos: bool = False,
+ rel_pos_zero_init: bool = True,
+ window_size: int = 0,
+ input_size: Optional[Tuple[int, int]] = None,
+ ) -> None:
+ """
+ Args:
+ dim (int): Number of input channels.
+ num_heads (int): Number of attention heads in each ViT block.
+ mlp_ratio (float): Ratio of mlp hidden dim to embedding dim.
+ qkv_bias (bool): If True, add a learnable bias to query, key, value.
+ norm_layer (nn.Module): Normalization layer.
+ act_layer (nn.Module): Activation layer.
+ use_rel_pos (bool): If True, add relative positional embeddings to the attention map.
+ rel_pos_zero_init (bool): If True, zero initialize relative positional parameters.
+ window_size (int): Window size for window attention blocks. If it equals 0, then
+ use global attention.
+ input_size (tuple(int, int), None): Input resolution for calculating the relative
+ positional parameter size.
+ """
+ super().__init__()
+ self.norm1 = norm_layer(dim)
+ self.attn = Attention(
+ dim,
+ num_heads=num_heads,
+ qkv_bias=qkv_bias,
+ use_rel_pos=use_rel_pos,
+ rel_pos_zero_init=rel_pos_zero_init,
+ input_size=input_size if window_size == 0 else (window_size, window_size),
+ )
+
+ self.norm2 = norm_layer(dim)
+ self.mlp = MLPBlock(embedding_dim=dim, mlp_dim=int(dim * mlp_ratio), act=act_layer)
+
+ self.window_size = window_size
+
+ def forward(self, x: torch.Tensor) -> torch.Tensor:
+ shortcut = x
+ x = self.norm1(x)
+ # Window partition
+ if self.window_size > 0:
+ H, W = x.shape[1], x.shape[2]
+ x, pad_hw = window_partition(x, self.window_size)
+
+ x = self.attn(x)
+ # Reverse window partition
+ if self.window_size > 0:
+ x = window_unpartition(x, self.window_size, pad_hw, (H, W))
+
+ x = shortcut + x
+ x = x + self.mlp(self.norm2(x))
+
+ return x
+
+
+class Attention(nn.Module):
+ """Multi-head Attention block with relative position embeddings."""
+
+ def __init__(
+ self,
+ dim: int,
+ num_heads: int = 8,
+ qkv_bias: bool = True,
+ use_rel_pos: bool = False,
+ rel_pos_zero_init: bool = True,
+ input_size: Optional[Tuple[int, int]] = None,
+ ) -> None:
+ """
+ Args:
+ dim (int): Number of input channels.
+ num_heads (int): Number of attention heads.
+ qkv_bias (bool): If True, add a learnable bias to query, key, value.
+ rel_pos_zero_init (bool): If True, zero initialize relative positional parameters.
+ input_size (tuple(int, int), None): Input resolution for calculating the relative
+ positional parameter size.
+ """
+ super().__init__()
+ self.num_heads = num_heads
+ head_dim = dim // num_heads
+ self.scale = head_dim ** -0.5
+
+ self.qkv = nn.Linear(dim, dim * 3, bias=qkv_bias)
+ self.proj = nn.Linear(dim, dim)
+
+ self.use_rel_pos = use_rel_pos
+ if self.use_rel_pos:
+ assert (input_size is not None), 'Input size must be provided if using relative positional encoding.'
+ # initialize relative positional embeddings
+ self.rel_pos_h = nn.Parameter(torch.zeros(2 * input_size[0] - 1, head_dim))
+ self.rel_pos_w = nn.Parameter(torch.zeros(2 * input_size[1] - 1, head_dim))
+
+ def forward(self, x: torch.Tensor) -> torch.Tensor:
+ B, H, W, _ = x.shape
+ # qkv with shape (3, B, nHead, H * W, C)
+ qkv = self.qkv(x).reshape(B, H * W, 3, self.num_heads, -1).permute(2, 0, 3, 1, 4)
+ # q, k, v with shape (B * nHead, H * W, C)
+ q, k, v = qkv.reshape(3, B * self.num_heads, H * W, -1).unbind(0)
+
+ attn = (q * self.scale) @ k.transpose(-2, -1)
+
+ if self.use_rel_pos:
+ attn = add_decomposed_rel_pos(attn, q, self.rel_pos_h, self.rel_pos_w, (H, W), (H, W))
+
+ attn = attn.softmax(dim=-1)
+ x = (attn @ v).view(B, self.num_heads, H, W, -1).permute(0, 2, 3, 1, 4).reshape(B, H, W, -1)
+ x = self.proj(x)
+
+ return x
+
+
+def window_partition(x: torch.Tensor, window_size: int) -> Tuple[torch.Tensor, Tuple[int, int]]:
+ """
+ Partition into non-overlapping windows with padding if needed.
+ Args:
+ x (tensor): input tokens with [B, H, W, C].
+ window_size (int): window size.
+
+ Returns:
+ windows: windows after partition with [B * num_windows, window_size, window_size, C].
+ (Hp, Wp): padded height and width before partition
+ """
+ B, H, W, C = x.shape
+
+ pad_h = (window_size - H % window_size) % window_size
+ pad_w = (window_size - W % window_size) % window_size
+ if pad_h > 0 or pad_w > 0:
+ x = F.pad(x, (0, 0, 0, pad_w, 0, pad_h))
+ Hp, Wp = H + pad_h, W + pad_w
+
+ x = x.view(B, Hp // window_size, window_size, Wp // window_size, window_size, C)
+ windows = x.permute(0, 1, 3, 2, 4, 5).contiguous().view(-1, window_size, window_size, C)
+ return windows, (Hp, Wp)
+
+
+def window_unpartition(windows: torch.Tensor, window_size: int, pad_hw: Tuple[int, int],
+ hw: Tuple[int, int]) -> torch.Tensor:
+ """
+ Window unpartition into original sequences and removing padding.
+ Args:
+ windows (tensor): input tokens with [B * num_windows, window_size, window_size, C].
+ window_size (int): window size.
+ pad_hw (Tuple): padded height and width (Hp, Wp).
+ hw (Tuple): original height and width (H, W) before padding.
+
+ Returns:
+ x: unpartitioned sequences with [B, H, W, C].
+ """
+ Hp, Wp = pad_hw
+ H, W = hw
+ B = windows.shape[0] // (Hp * Wp // window_size // window_size)
+ x = windows.view(B, Hp // window_size, Wp // window_size, window_size, window_size, -1)
+ x = x.permute(0, 1, 3, 2, 4, 5).contiguous().view(B, Hp, Wp, -1)
+
+ if Hp > H or Wp > W:
+ x = x[:, :H, :W, :].contiguous()
+ return x
+
+
+def get_rel_pos(q_size: int, k_size: int, rel_pos: torch.Tensor) -> torch.Tensor:
+ """
+ Get relative positional embeddings according to the relative positions of
+ query and key sizes.
+ Args:
+ q_size (int): size of query q.
+ k_size (int): size of key k.
+ rel_pos (Tensor): relative position embeddings (L, C).
+
+ Returns:
+ Extracted positional embeddings according to relative positions.
+ """
+ max_rel_dist = int(2 * max(q_size, k_size) - 1)
+ # Interpolate rel pos if needed.
+ if rel_pos.shape[0] != max_rel_dist:
+ # Interpolate rel pos.
+ rel_pos_resized = F.interpolate(
+ rel_pos.reshape(1, rel_pos.shape[0], -1).permute(0, 2, 1),
+ size=max_rel_dist,
+ mode='linear',
+ )
+ rel_pos_resized = rel_pos_resized.reshape(-1, max_rel_dist).permute(1, 0)
+ else:
+ rel_pos_resized = rel_pos
+
+ # Scale the coords with short length if shapes for q and k are different.
+ q_coords = torch.arange(q_size)[:, None] * max(k_size / q_size, 1.0)
+ k_coords = torch.arange(k_size)[None, :] * max(q_size / k_size, 1.0)
+ relative_coords = (q_coords - k_coords) + (k_size - 1) * max(q_size / k_size, 1.0)
+
+ return rel_pos_resized[relative_coords.long()]
+
+
+def add_decomposed_rel_pos(
+ attn: torch.Tensor,
+ q: torch.Tensor,
+ rel_pos_h: torch.Tensor,
+ rel_pos_w: torch.Tensor,
+ q_size: Tuple[int, int],
+ k_size: Tuple[int, int],
+) -> torch.Tensor:
+ """
+ Calculate decomposed Relative Positional Embeddings from :paper:`mvitv2`.
+ https://github.com/facebookresearch/mvit/blob/19786631e330df9f3622e5402b4a419a263a2c80/mvit/models/attention.py # noqa B950
+ Args:
+ attn (Tensor): attention map.
+ q (Tensor): query q in the attention layer with shape (B, q_h * q_w, C).
+ rel_pos_h (Tensor): relative position embeddings (Lh, C) for height axis.
+ rel_pos_w (Tensor): relative position embeddings (Lw, C) for width axis.
+ q_size (Tuple): spatial sequence size of query q with (q_h, q_w).
+ k_size (Tuple): spatial sequence size of key k with (k_h, k_w).
+
+ Returns:
+ attn (Tensor): attention map with added relative positional embeddings.
+ """
+ q_h, q_w = q_size
+ k_h, k_w = k_size
+ Rh = get_rel_pos(q_h, k_h, rel_pos_h)
+ Rw = get_rel_pos(q_w, k_w, rel_pos_w)
+
+ B, _, dim = q.shape
+ r_q = q.reshape(B, q_h, q_w, dim)
+ rel_h = torch.einsum('bhwc,hkc->bhwk', r_q, Rh)
+ rel_w = torch.einsum('bhwc,wkc->bhwk', r_q, Rw)
+
+ attn = (attn.view(B, q_h, q_w, k_h, k_w) + rel_h[:, :, :, :, None] + rel_w[:, :, :, None, :]).view(
+ B, q_h * q_w, k_h * k_w)
+
+ return attn
+
+
+class PatchEmbed(nn.Module):
+ """
+ Image to Patch Embedding.
+ """
+
+ def __init__(
+ self,
+ kernel_size: Tuple[int, int] = (16, 16),
+ stride: Tuple[int, int] = (16, 16),
+ padding: Tuple[int, int] = (0, 0),
+ in_chans: int = 3,
+ embed_dim: int = 768,
+ ) -> None:
+ """
+ Args:
+ kernel_size (Tuple): kernel size of the projection layer.
+ stride (Tuple): stride of the projection layer.
+ padding (Tuple): padding size of the projection layer.
+ in_chans (int): Number of input image channels.
+ embed_dim (int): Patch embedding dimension.
+ """
+ super().__init__()
+
+ self.proj = nn.Conv2d(in_chans, embed_dim, kernel_size=kernel_size, stride=stride, padding=padding)
+
+ def forward(self, x: torch.Tensor) -> torch.Tensor:
+ x = self.proj(x)
+ # B C H W -> B H W C
+ x = x.permute(0, 2, 3, 1)
+ return x
diff --git a/yolov8/ultralytics/vit/sam/modules/mask_generator.py b/yolov8/ultralytics/vit/sam/modules/mask_generator.py
new file mode 100644
index 0000000000000000000000000000000000000000..8c1e00ea172d28bb7f0bb3fe92e32a482924f7e7
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/mask_generator.py
@@ -0,0 +1,353 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+# Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+from typing import Any, Dict, List, Optional, Tuple
+
+import numpy as np
+import torch
+from torchvision.ops.boxes import batched_nms, box_area # type: ignore
+
+from ..amg import (MaskData, area_from_rle, batch_iterator, batched_mask_to_box, box_xyxy_to_xywh,
+ build_all_layer_point_grids, calculate_stability_score, coco_encode_rle, generate_crop_boxes,
+ is_box_near_crop_edge, mask_to_rle_pytorch, remove_small_regions, rle_to_mask, uncrop_boxes_xyxy,
+ uncrop_masks, uncrop_points)
+from .prompt_predictor import PromptPredictor
+from .sam import Sam
+
+
+class SamAutomaticMaskGenerator:
+
+ def __init__(
+ self,
+ model: Sam,
+ points_per_side: Optional[int] = 32,
+ points_per_batch: int = 64,
+ pred_iou_thresh: float = 0.88,
+ stability_score_thresh: float = 0.95,
+ stability_score_offset: float = 1.0,
+ box_nms_thresh: float = 0.7,
+ crop_n_layers: int = 0,
+ crop_nms_thresh: float = 0.7,
+ crop_overlap_ratio: float = 512 / 1500,
+ crop_n_points_downscale_factor: int = 1,
+ point_grids: Optional[List[np.ndarray]] = None,
+ min_mask_region_area: int = 0,
+ output_mode: str = 'binary_mask',
+ ) -> None:
+ """
+ Using a SAM model, generates masks for the entire image.
+ Generates a grid of point prompts over the image, then filters
+ low quality and duplicate masks. The default settings are chosen
+ for SAM with a ViT-H backbone.
+
+ Arguments:
+ model (Sam): The SAM model to use for mask prediction.
+ points_per_side (int, None): The number of points to be sampled
+ along one side of the image. The total number of points is
+ points_per_side**2. If None, 'point_grids' must provide explicit
+ point sampling.
+ points_per_batch (int): Sets the number of points run simultaneously
+ by the model. Higher numbers may be faster but use more GPU memory.
+ pred_iou_thresh (float): A filtering threshold in [0,1], using the
+ model's predicted mask quality.
+ stability_score_thresh (float): A filtering threshold in [0,1], using
+ the stability of the mask under changes to the cutoff used to binarize
+ the model's mask predictions.
+ stability_score_offset (float): The amount to shift the cutoff when
+ calculated the stability score.
+ box_nms_thresh (float): The box IoU cutoff used by non-maximal
+ suppression to filter duplicate masks.
+ crop_n_layers (int): If >0, mask prediction will be run again on
+ crops of the image. Sets the number of layers to run, where each
+ layer has 2**i_layer number of image crops.
+ crop_nms_thresh (float): The box IoU cutoff used by non-maximal
+ suppression to filter duplicate masks between different crops.
+ crop_overlap_ratio (float): Sets the degree to which crops overlap.
+ In the first crop layer, crops will overlap by this fraction of
+ the image length. Later layers with more crops scale down this overlap.
+ crop_n_points_downscale_factor (int): The number of points-per-side
+ sampled in layer n is scaled down by crop_n_points_downscale_factor**n.
+ point_grids (list(np.ndarray), None): A list over explicit grids
+ of points used for sampling, normalized to [0,1]. The nth grid in the
+ list is used in the nth crop layer. Exclusive with points_per_side.
+ min_mask_region_area (int): If >0, postprocessing will be applied
+ to remove disconnected regions and holes in masks with area smaller
+ than min_mask_region_area. Requires opencv.
+ output_mode (str): The form masks are returned in. Can be 'binary_mask',
+ 'uncompressed_rle', or 'coco_rle'. 'coco_rle' requires pycocotools.
+ For large resolutions, 'binary_mask' may consume large amounts of
+ memory.
+ """
+
+ assert (points_per_side is None) != (point_grids is None), \
+ 'Exactly one of points_per_side or point_grid must be provided.'
+ if points_per_side is not None:
+ self.point_grids = build_all_layer_point_grids(
+ points_per_side,
+ crop_n_layers,
+ crop_n_points_downscale_factor,
+ )
+ elif point_grids is not None:
+ self.point_grids = point_grids
+ else:
+ raise ValueError("Can't have both points_per_side and point_grid be None.")
+
+ assert output_mode in {'binary_mask', 'uncompressed_rle', 'coco_rle'}, f'Unknown output_mode {output_mode}.'
+ if output_mode == 'coco_rle':
+ from pycocotools import mask as mask_utils # type: ignore # noqa: F401
+
+ if min_mask_region_area > 0:
+ import cv2 # type: ignore # noqa: F401
+
+ self.predictor = PromptPredictor(model)
+ self.points_per_batch = points_per_batch
+ self.pred_iou_thresh = pred_iou_thresh
+ self.stability_score_thresh = stability_score_thresh
+ self.stability_score_offset = stability_score_offset
+ self.box_nms_thresh = box_nms_thresh
+ self.crop_n_layers = crop_n_layers
+ self.crop_nms_thresh = crop_nms_thresh
+ self.crop_overlap_ratio = crop_overlap_ratio
+ self.crop_n_points_downscale_factor = crop_n_points_downscale_factor
+ self.min_mask_region_area = min_mask_region_area
+ self.output_mode = output_mode
+
+ # TODO: Temporary implementation for compatibility
+ def __call__(self, image: np.ndarray, augment=False, visualize=False) -> List[Dict[str, Any]]:
+ return self.generate(image)
+
+ @torch.no_grad()
+ def generate(self, image: np.ndarray) -> List[Dict[str, Any]]:
+ """
+ Generates masks for the given image.
+
+ Arguments:
+ image (np.ndarray): The image to generate masks for, in HWC uint8 format.
+
+ Returns:
+ list(dict(str, any)): A list over records for masks. Each record is a dict containing the following keys:
+ segmentation (dict(str, any), np.ndarray): The mask. If
+ output_mode='binary_mask', is an array of shape HW. Otherwise,
+ is a dictionary containing the RLE.
+ bbox (list(float)): The box around the mask, in XYWH format.
+ area (int): The area in pixels of the mask.
+ predicted_iou (float): The model's own prediction of the mask's
+ quality. This is filtered by the pred_iou_thresh parameter.
+ point_coords (list(list(float))): The point coordinates input
+ to the model to generate this mask.
+ stability_score (float): A measure of the mask's quality. This
+ is filtered on using the stability_score_thresh parameter.
+ crop_box (list(float)): The crop of the image used to generate
+ the mask, given in XYWH format.
+ """
+
+ # Generate masks
+ mask_data = self._generate_masks(image)
+
+ # Filter small disconnected regions and holes in masks
+ if self.min_mask_region_area > 0:
+ mask_data = self.postprocess_small_regions(
+ mask_data,
+ self.min_mask_region_area,
+ max(self.box_nms_thresh, self.crop_nms_thresh),
+ )
+
+ # Encode masks
+ if self.output_mode == 'coco_rle':
+ mask_data['segmentations'] = [coco_encode_rle(rle) for rle in mask_data['rles']]
+ elif self.output_mode == 'binary_mask':
+ mask_data['segmentations'] = [rle_to_mask(rle) for rle in mask_data['rles']]
+ else:
+ mask_data['segmentations'] = mask_data['rles']
+
+ # Write mask records
+ curr_anns = []
+ for idx in range(len(mask_data['segmentations'])):
+ ann = {
+ 'segmentation': mask_data['segmentations'][idx],
+ 'area': area_from_rle(mask_data['rles'][idx]),
+ 'bbox': box_xyxy_to_xywh(mask_data['boxes'][idx]).tolist(),
+ 'predicted_iou': mask_data['iou_preds'][idx].item(),
+ 'point_coords': [mask_data['points'][idx].tolist()],
+ 'stability_score': mask_data['stability_score'][idx].item(),
+ 'crop_box': box_xyxy_to_xywh(mask_data['crop_boxes'][idx]).tolist(), }
+ curr_anns.append(ann)
+
+ return curr_anns
+
+ def _generate_masks(self, image: np.ndarray) -> MaskData:
+ orig_size = image.shape[:2]
+ crop_boxes, layer_idxs = generate_crop_boxes(orig_size, self.crop_n_layers, self.crop_overlap_ratio)
+
+ # Iterate over image crops
+ data = MaskData()
+ for crop_box, layer_idx in zip(crop_boxes, layer_idxs):
+ crop_data = self._process_crop(image, crop_box, layer_idx, orig_size)
+ data.cat(crop_data)
+
+ # Remove duplicate masks between crops
+ if len(crop_boxes) > 1:
+ # Prefer masks from smaller crops
+ scores = 1 / box_area(data['crop_boxes'])
+ scores = scores.to(data['boxes'].device)
+ keep_by_nms = batched_nms(
+ data['boxes'].float(),
+ scores,
+ torch.zeros_like(data['boxes'][:, 0]), # categories
+ iou_threshold=self.crop_nms_thresh,
+ )
+ data.filter(keep_by_nms)
+
+ data.to_numpy()
+ return data
+
+ def _process_crop(
+ self,
+ image: np.ndarray,
+ crop_box: List[int],
+ crop_layer_idx: int,
+ orig_size: Tuple[int, ...],
+ ) -> MaskData:
+ # Crop the image and calculate embeddings
+ x0, y0, x1, y1 = crop_box
+ cropped_im = image[y0:y1, x0:x1, :]
+ cropped_im_size = cropped_im.shape[:2]
+ self.predictor.set_image(cropped_im)
+
+ # Get points for this crop
+ points_scale = np.array(cropped_im_size)[None, ::-1]
+ points_for_image = self.point_grids[crop_layer_idx] * points_scale
+
+ # Generate masks for this crop in batches
+ data = MaskData()
+ for (points, ) in batch_iterator(self.points_per_batch, points_for_image):
+ batch_data = self._process_batch(points, cropped_im_size, crop_box, orig_size)
+ data.cat(batch_data)
+ del batch_data
+ self.predictor.reset_image()
+
+ # Remove duplicates within this crop.
+ keep_by_nms = batched_nms(
+ data['boxes'].float(),
+ data['iou_preds'],
+ torch.zeros_like(data['boxes'][:, 0]), # categories
+ iou_threshold=self.box_nms_thresh,
+ )
+ data.filter(keep_by_nms)
+
+ # Return to the original image frame
+ data['boxes'] = uncrop_boxes_xyxy(data['boxes'], crop_box)
+ data['points'] = uncrop_points(data['points'], crop_box)
+ data['crop_boxes'] = torch.tensor([crop_box for _ in range(len(data['rles']))])
+
+ return data
+
+ def _process_batch(
+ self,
+ points: np.ndarray,
+ im_size: Tuple[int, ...],
+ crop_box: List[int],
+ orig_size: Tuple[int, ...],
+ ) -> MaskData:
+ orig_h, orig_w = orig_size
+
+ # Run model on this batch
+ transformed_points = self.predictor.transform.apply_coords(points, im_size)
+ in_points = torch.as_tensor(transformed_points, device=self.predictor.device)
+ in_labels = torch.ones(in_points.shape[0], dtype=torch.int, device=in_points.device)
+ masks, iou_preds, _ = self.predictor.predict_torch(
+ in_points[:, None, :],
+ in_labels[:, None],
+ multimask_output=True,
+ return_logits=True,
+ )
+
+ # Serialize predictions and store in MaskData
+ data = MaskData(
+ masks=masks.flatten(0, 1),
+ iou_preds=iou_preds.flatten(0, 1),
+ points=torch.as_tensor(points.repeat(masks.shape[1], axis=0)),
+ )
+ del masks
+
+ # Filter by predicted IoU
+ if self.pred_iou_thresh > 0.0:
+ keep_mask = data['iou_preds'] > self.pred_iou_thresh
+ data.filter(keep_mask)
+
+ # Calculate stability score
+ data['stability_score'] = calculate_stability_score(data['masks'], self.predictor.model.mask_threshold,
+ self.stability_score_offset)
+ if self.stability_score_thresh > 0.0:
+ keep_mask = data['stability_score'] >= self.stability_score_thresh
+ data.filter(keep_mask)
+
+ # Threshold masks and calculate boxes
+ data['masks'] = data['masks'] > self.predictor.model.mask_threshold
+ data['boxes'] = batched_mask_to_box(data['masks'])
+
+ # Filter boxes that touch crop boundaries
+ keep_mask = ~is_box_near_crop_edge(data['boxes'], crop_box, [0, 0, orig_w, orig_h])
+ if not torch.all(keep_mask):
+ data.filter(keep_mask)
+
+ # Compress to RLE
+ data['masks'] = uncrop_masks(data['masks'], crop_box, orig_h, orig_w)
+ data['rles'] = mask_to_rle_pytorch(data['masks'])
+ del data['masks']
+
+ return data
+
+ @staticmethod
+ def postprocess_small_regions(mask_data: MaskData, min_area: int, nms_thresh: float) -> MaskData:
+ """
+ Removes small disconnected regions and holes in masks, then reruns
+ box NMS to remove any new duplicates.
+
+ Edits mask_data in place.
+
+ Requires open-cv as a dependency.
+ """
+ if len(mask_data['rles']) == 0:
+ return mask_data
+
+ # Filter small disconnected regions and holes
+ new_masks = []
+ scores = []
+ for rle in mask_data['rles']:
+ mask = rle_to_mask(rle)
+
+ mask, changed = remove_small_regions(mask, min_area, mode='holes')
+ unchanged = not changed
+ mask, changed = remove_small_regions(mask, min_area, mode='islands')
+ unchanged = unchanged and not changed
+
+ new_masks.append(torch.as_tensor(mask).unsqueeze(0))
+ # Give score=0 to changed masks and score=1 to unchanged masks
+ # so NMS will prefer ones that didn't need postprocessing
+ scores.append(float(unchanged))
+
+ # Recalculate boxes and remove any new duplicates
+ masks = torch.cat(new_masks, dim=0)
+ boxes = batched_mask_to_box(masks)
+ keep_by_nms = batched_nms(
+ boxes.float(),
+ torch.as_tensor(scores),
+ torch.zeros_like(boxes[:, 0]), # categories
+ iou_threshold=nms_thresh,
+ )
+
+ # Only recalculate RLEs for masks that have changed
+ for i_mask in keep_by_nms:
+ if scores[i_mask] == 0.0:
+ mask_torch = masks[i_mask].unsqueeze(0)
+ mask_data['rles'][i_mask] = mask_to_rle_pytorch(mask_torch)[0]
+ mask_data['boxes'][i_mask] = boxes[i_mask] # update res directly
+ mask_data.filter(keep_by_nms)
+
+ return mask_data
diff --git a/yolov8/ultralytics/vit/sam/modules/prompt_predictor.py b/yolov8/ultralytics/vit/sam/modules/prompt_predictor.py
new file mode 100644
index 0000000000000000000000000000000000000000..bf89893458532c928568693707b16312f19237f7
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/prompt_predictor.py
@@ -0,0 +1,242 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from typing import Optional, Tuple
+
+import numpy as np
+import torch
+
+from ..autosize import ResizeLongestSide
+from .sam import Sam
+
+
+class PromptPredictor:
+
+ def __init__(self, sam_model: Sam) -> None:
+ """
+ Uses SAM to calculate the image embedding for an image, and then
+ allow repeated, efficient mask prediction given prompts.
+
+ Arguments:
+ sam_model (Sam): The model to use for mask prediction.
+ """
+ super().__init__()
+ self.model = sam_model
+ self.transform = ResizeLongestSide(sam_model.image_encoder.img_size)
+ self.reset_image()
+
+ def set_image(self, image: np.ndarray, image_format: str = 'RGB') -> None:
+ """
+ Calculates the image embeddings for the provided image, allowing
+ masks to be predicted with the 'predict' method.
+
+ Arguments:
+ image (np.ndarray): The image for calculating masks. Expects an
+ image in HWC uint8 format, with pixel values in [0, 255].
+ image_format (str): The color format of the image, in ['RGB', 'BGR'].
+ """
+ assert image_format in {'RGB', 'BGR'}, f"image_format must be in ['RGB', 'BGR'], is {image_format}."
+ if image_format != self.model.image_format:
+ image = image[..., ::-1]
+
+ # Transform the image to the form expected by the model
+ input_image = self.transform.apply_image(image)
+ input_image_torch = torch.as_tensor(input_image, device=self.device)
+ input_image_torch = input_image_torch.permute(2, 0, 1).contiguous()[None, :, :, :]
+
+ self.set_torch_image(input_image_torch, image.shape[:2])
+
+ @torch.no_grad()
+ def set_torch_image(self, transformed_image: torch.Tensor, original_image_size: Tuple[int, ...]) -> None:
+ """
+ Calculates the image embeddings for the provided image, allowing
+ masks to be predicted with the 'predict' method. Expects the input
+ image to be already transformed to the format expected by the model.
+
+ Arguments:
+ transformed_image (torch.Tensor): The input image, with shape
+ 1x3xHxW, which has been transformed with ResizeLongestSide.
+ original_image_size (tuple(int, int)): The size of the image
+ before transformation, in (H, W) format.
+ """
+ if len(transformed_image.shape) != 4 \
+ or transformed_image.shape[1] != 3 \
+ or max(*transformed_image.shape[2:]) != self.model.image_encoder.img_size:
+ raise ValueError('set_torch_image input must be BCHW with long side {self.model.image_encoder.img_size}.')
+ self.reset_image()
+
+ self.original_size = original_image_size
+ self.input_size = tuple(transformed_image.shape[-2:])
+ input_image = self.model.preprocess(transformed_image)
+ self.features = self.model.image_encoder(input_image)
+ self.is_image_set = True
+
+ def predict(
+ self,
+ point_coords: Optional[np.ndarray] = None,
+ point_labels: Optional[np.ndarray] = None,
+ box: Optional[np.ndarray] = None,
+ mask_input: Optional[np.ndarray] = None,
+ multimask_output: bool = True,
+ return_logits: bool = False,
+ ) -> Tuple[np.ndarray, np.ndarray, np.ndarray]:
+ """
+ Predict masks for the given input prompts, using the currently set image.
+
+ Arguments:
+ point_coords (np.ndarray, None): A Nx2 array of point prompts to the
+ model. Each point is in (X,Y) in pixels.
+ point_labels (np.ndarray, None): A length N array of labels for the
+ point prompts. 1 indicates a foreground point and 0 indicates a
+ background point.
+ box (np.ndarray, None): A length 4 array given a box prompt to the
+ model, in XYXY format.
+ mask_input (np.ndarray): A low resolution mask input to the model, typically
+ coming from a previous prediction iteration. Has form 1xHxW, where
+ for SAM, H=W=256.
+ multimask_output (bool): If true, the model will return three masks.
+ For ambiguous input prompts (such as a single click), this will often
+ produce better masks than a single prediction. If only a single
+ mask is needed, the model's predicted quality score can be used
+ to select the best mask. For non-ambiguous prompts, such as multiple
+ input prompts, multimask_output=False can give better results.
+ return_logits (bool): If true, returns un-thresholded masks logits
+ instead of a binary mask.
+
+ Returns:
+ (np.ndarray): The output masks in CxHxW format, where C is the
+ number of masks, and (H, W) is the original image size.
+ (np.ndarray): An array of length C containing the model's
+ predictions for the quality of each mask.
+ (np.ndarray): An array of shape CxHxW, where C is the number
+ of masks and H=W=256. These low resolution logits can be passed to
+ a subsequent iteration as mask input.
+ """
+ if not self.is_image_set:
+ raise RuntimeError('An image must be set with .set_image(...) before mask prediction.')
+
+ # Transform input prompts
+ coords_torch, labels_torch, box_torch, mask_input_torch = None, None, None, None
+ if point_coords is not None:
+ assert (point_labels is not None), 'point_labels must be supplied if point_coords is supplied.'
+ point_coords = self.transform.apply_coords(point_coords, self.original_size)
+ coords_torch = torch.as_tensor(point_coords, dtype=torch.float, device=self.device)
+ labels_torch = torch.as_tensor(point_labels, dtype=torch.int, device=self.device)
+ coords_torch, labels_torch = coords_torch[None, :, :], labels_torch[None, :]
+ if box is not None:
+ box = self.transform.apply_boxes(box, self.original_size)
+ box_torch = torch.as_tensor(box, dtype=torch.float, device=self.device)
+ box_torch = box_torch[None, :]
+ if mask_input is not None:
+ mask_input_torch = torch.as_tensor(mask_input, dtype=torch.float, device=self.device)
+ mask_input_torch = mask_input_torch[None, :, :, :]
+
+ masks, iou_predictions, low_res_masks = self.predict_torch(
+ coords_torch,
+ labels_torch,
+ box_torch,
+ mask_input_torch,
+ multimask_output,
+ return_logits=return_logits,
+ )
+
+ masks_np = masks[0].detach().cpu().numpy()
+ iou_predictions_np = iou_predictions[0].detach().cpu().numpy()
+ low_res_masks_np = low_res_masks[0].detach().cpu().numpy()
+ return masks_np, iou_predictions_np, low_res_masks_np
+
+ @torch.no_grad()
+ def predict_torch(
+ self,
+ point_coords: Optional[torch.Tensor],
+ point_labels: Optional[torch.Tensor],
+ boxes: Optional[torch.Tensor] = None,
+ mask_input: Optional[torch.Tensor] = None,
+ multimask_output: bool = True,
+ return_logits: bool = False,
+ ) -> Tuple[torch.Tensor, torch.Tensor, torch.Tensor]:
+ """
+ Predict masks for the given input prompts, using the currently set image.
+ Input prompts are batched torch tensors and are expected to already be
+ transformed to the input frame using ResizeLongestSide.
+
+ Arguments:
+ point_coords (torch.Tensor, None): A BxNx2 array of point prompts to the
+ model. Each point is in (X,Y) in pixels.
+ point_labels (torch.Tensor, None): A BxN array of labels for the
+ point prompts. 1 indicates a foreground point and 0 indicates a
+ background point.
+ boxes (np.ndarray, None): A Bx4 array given a box prompt to the
+ model, in XYXY format.
+ mask_input (np.ndarray): A low resolution mask input to the model, typically
+ coming from a previous prediction iteration. Has form Bx1xHxW, where
+ for SAM, H=W=256. Masks returned by a previous iteration of the
+ predict method do not need further transformation.
+ multimask_output (bool): If true, the model will return three masks.
+ For ambiguous input prompts (such as a single click), this will often
+ produce better masks than a single prediction. If only a single
+ mask is needed, the model's predicted quality score can be used
+ to select the best mask. For non-ambiguous prompts, such as multiple
+ input prompts, multimask_output=False can give better results.
+ return_logits (bool): If true, returns un-thresholded masks logits
+ instead of a binary mask.
+
+ Returns:
+ (torch.Tensor): The output masks in BxCxHxW format, where C is the
+ number of masks, and (H, W) is the original image size.
+ (torch.Tensor): An array of shape BxC containing the model's
+ predictions for the quality of each mask.
+ (torch.Tensor): An array of shape BxCxHxW, where C is the number
+ of masks and H=W=256. These low res logits can be passed to
+ a subsequent iteration as mask input.
+ """
+ if not self.is_image_set:
+ raise RuntimeError('An image must be set with .set_image(...) before mask prediction.')
+
+ points = (point_coords, point_labels) if point_coords is not None else None
+ # Embed prompts
+ sparse_embeddings, dense_embeddings = self.model.prompt_encoder(
+ points=points,
+ boxes=boxes,
+ masks=mask_input,
+ )
+
+ # Predict masks
+ low_res_masks, iou_predictions = self.model.mask_decoder(
+ image_embeddings=self.features,
+ image_pe=self.model.prompt_encoder.get_dense_pe(),
+ sparse_prompt_embeddings=sparse_embeddings,
+ dense_prompt_embeddings=dense_embeddings,
+ multimask_output=multimask_output,
+ )
+
+ # Upscale the masks to the original image resolution
+ masks = self.model.postprocess_masks(low_res_masks, self.input_size, self.original_size)
+
+ if not return_logits:
+ masks = masks > self.model.mask_threshold
+
+ return masks, iou_predictions, low_res_masks
+
+ def get_image_embedding(self) -> torch.Tensor:
+ """
+ Returns the image embeddings for the currently set image, with
+ shape 1xCxHxW, where C is the embedding dimension and (H,W) are
+ the embedding spatial dimension of SAM (typically C=256, H=W=64).
+ """
+ if not self.is_image_set:
+ raise RuntimeError('An image must be set with .set_image(...) to generate an embedding.')
+ assert self.features is not None, 'Features must exist if an image has been set.'
+ return self.features
+
+ @property
+ def device(self) -> torch.device:
+ return self.model.device
+
+ def reset_image(self) -> None:
+ """Resets the currently set image."""
+ self.is_image_set = False
+ self.features = None
+ self.orig_h = None
+ self.orig_w = None
+ self.input_h = None
+ self.input_w = None
diff --git a/yolov8/ultralytics/vit/sam/modules/sam.py b/yolov8/ultralytics/vit/sam/modules/sam.py
new file mode 100644
index 0000000000000000000000000000000000000000..49f4bfcb0f502b8014972473a8925b2da89d482c
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/sam.py
@@ -0,0 +1,173 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+# Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+from typing import Any, Dict, List, Tuple
+
+import torch
+from torch import nn
+from torch.nn import functional as F
+
+from .decoders import MaskDecoder
+from .encoders import ImageEncoderViT, PromptEncoder
+
+
+class Sam(nn.Module):
+ mask_threshold: float = 0.0
+ image_format: str = 'RGB'
+
+ def __init__(self,
+ image_encoder: ImageEncoderViT,
+ prompt_encoder: PromptEncoder,
+ mask_decoder: MaskDecoder,
+ pixel_mean: List[float] = None,
+ pixel_std: List[float] = None) -> None:
+ """
+ SAM predicts object masks from an image and input prompts.
+
+ Arguments:
+ image_encoder (ImageEncoderViT): The backbone used to encode the
+ image into image embeddings that allow for efficient mask prediction.
+ prompt_encoder (PromptEncoder): Encodes various types of input prompts.
+ mask_decoder (MaskDecoder): Predicts masks from the image embeddings
+ and encoded prompts.
+ pixel_mean (list(float)): Mean values for normalizing pixels in the input image.
+ pixel_std (list(float)): Std values for normalizing pixels in the input image.
+ """
+ if pixel_mean is None:
+ pixel_mean = [123.675, 116.28, 103.53]
+ if pixel_std is None:
+ pixel_std = [58.395, 57.12, 57.375]
+ super().__init__()
+ self.image_encoder = image_encoder
+ self.prompt_encoder = prompt_encoder
+ self.mask_decoder = mask_decoder
+ self.register_buffer('pixel_mean', torch.Tensor(pixel_mean).view(-1, 1, 1), False)
+ self.register_buffer('pixel_std', torch.Tensor(pixel_std).view(-1, 1, 1), False)
+
+ @property
+ def device(self) -> Any:
+ return self.pixel_mean.device
+
+ @torch.no_grad()
+ def forward(
+ self,
+ batched_input: List[Dict[str, Any]],
+ multimask_output: bool,
+ ) -> List[Dict[str, torch.Tensor]]:
+ """
+ Predicts masks end-to-end from provided images and prompts.
+ If prompts are not known in advance, using SamPredictor is
+ recommended over calling the model directly.
+
+ Arguments:
+ batched_input (list(dict)): A list over input images, each a
+ dictionary with the following keys. A prompt key can be
+ excluded if it is not present.
+ 'image': The image as a torch tensor in 3xHxW format,
+ already transformed for input to the model.
+ 'original_size': (tuple(int, int)) The original size of
+ the image before transformation, as (H, W).
+ 'point_coords': (torch.Tensor) Batched point prompts for
+ this image, with shape BxNx2. Already transformed to the
+ input frame of the model.
+ 'point_labels': (torch.Tensor) Batched labels for point prompts,
+ with shape BxN.
+ 'boxes': (torch.Tensor) Batched box inputs, with shape Bx4.
+ Already transformed to the input frame of the model.
+ 'mask_inputs': (torch.Tensor) Batched mask inputs to the model,
+ in the form Bx1xHxW.
+ multimask_output (bool): Whether the model should predict multiple
+ disambiguating masks, or return a single mask.
+
+ Returns:
+ (list(dict)): A list over input images, where each element is
+ as dictionary with the following keys.
+ 'masks': (torch.Tensor) Batched binary mask predictions,
+ with shape BxCxHxW, where B is the number of input prompts,
+ C is determined by multimask_output, and (H, W) is the
+ original size of the image.
+ 'iou_predictions': (torch.Tensor) The model's predictions
+ of mask quality, in shape BxC.
+ 'low_res_logits': (torch.Tensor) Low resolution logits with
+ shape BxCxHxW, where H=W=256. Can be passed as mask input
+ to subsequent iterations of prediction.
+ """
+ input_images = torch.stack([self.preprocess(x['image']) for x in batched_input], dim=0)
+ image_embeddings = self.image_encoder(input_images)
+
+ outputs = []
+ for image_record, curr_embedding in zip(batched_input, image_embeddings):
+ if 'point_coords' in image_record:
+ points = (image_record['point_coords'], image_record['point_labels'])
+ else:
+ points = None
+ sparse_embeddings, dense_embeddings = self.prompt_encoder(
+ points=points,
+ boxes=image_record.get('boxes', None),
+ masks=image_record.get('mask_inputs', None),
+ )
+ low_res_masks, iou_predictions = self.mask_decoder(
+ image_embeddings=curr_embedding.unsqueeze(0),
+ image_pe=self.prompt_encoder.get_dense_pe(),
+ sparse_prompt_embeddings=sparse_embeddings,
+ dense_prompt_embeddings=dense_embeddings,
+ multimask_output=multimask_output,
+ )
+ masks = self.postprocess_masks(
+ low_res_masks,
+ input_size=image_record['image'].shape[-2:],
+ original_size=image_record['original_size'],
+ )
+ masks = masks > self.mask_threshold
+ outputs.append({
+ 'masks': masks,
+ 'iou_predictions': iou_predictions,
+ 'low_res_logits': low_res_masks, })
+ return outputs
+
+ def postprocess_masks(
+ self,
+ masks: torch.Tensor,
+ input_size: Tuple[int, ...],
+ original_size: Tuple[int, ...],
+ ) -> torch.Tensor:
+ """
+ Remove padding and upscale masks to the original image size.
+
+ Arguments:
+ masks (torch.Tensor): Batched masks from the mask_decoder,
+ in BxCxHxW format.
+ input_size (tuple(int, int)): The size of the image input to the
+ model, in (H, W) format. Used to remove padding.
+ original_size (tuple(int, int)): The original size of the image
+ before resizing for input to the model, in (H, W) format.
+
+ Returns:
+ (torch.Tensor): Batched masks in BxCxHxW format, where (H, W)
+ is given by original_size.
+ """
+ masks = F.interpolate(
+ masks,
+ (self.image_encoder.img_size, self.image_encoder.img_size),
+ mode='bilinear',
+ align_corners=False,
+ )
+ masks = masks[..., :input_size[0], :input_size[1]]
+ masks = F.interpolate(masks, original_size, mode='bilinear', align_corners=False)
+ return masks
+
+ def preprocess(self, x: torch.Tensor) -> torch.Tensor:
+ """Normalize pixel values and pad to a square input."""
+ # Normalize colors
+ x = (x - self.pixel_mean) / self.pixel_std
+
+ # Pad
+ h, w = x.shape[-2:]
+ padh = self.image_encoder.img_size - h
+ padw = self.image_encoder.img_size - w
+ return F.pad(x, (0, padw, 0, padh))
diff --git a/yolov8/ultralytics/vit/sam/modules/transformer.py b/yolov8/ultralytics/vit/sam/modules/transformer.py
new file mode 100644
index 0000000000000000000000000000000000000000..d5275bf9836410f41c89e20c3cb07003c486d727
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/modules/transformer.py
@@ -0,0 +1,235 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import math
+from typing import Tuple, Type
+
+import torch
+from torch import Tensor, nn
+
+from ultralytics.nn.modules import MLPBlock
+
+
+class TwoWayTransformer(nn.Module):
+
+ def __init__(
+ self,
+ depth: int,
+ embedding_dim: int,
+ num_heads: int,
+ mlp_dim: int,
+ activation: Type[nn.Module] = nn.ReLU,
+ attention_downsample_rate: int = 2,
+ ) -> None:
+ """
+ A transformer decoder that attends to an input image using
+ queries whose positional embedding is supplied.
+
+ Args:
+ depth (int): number of layers in the transformer
+ embedding_dim (int): the channel dimension for the input embeddings
+ num_heads (int): the number of heads for multihead attention. Must
+ divide embedding_dim
+ mlp_dim (int): the channel dimension internal to the MLP block
+ activation (nn.Module): the activation to use in the MLP block
+ """
+ super().__init__()
+ self.depth = depth
+ self.embedding_dim = embedding_dim
+ self.num_heads = num_heads
+ self.mlp_dim = mlp_dim
+ self.layers = nn.ModuleList()
+
+ for i in range(depth):
+ self.layers.append(
+ TwoWayAttentionBlock(
+ embedding_dim=embedding_dim,
+ num_heads=num_heads,
+ mlp_dim=mlp_dim,
+ activation=activation,
+ attention_downsample_rate=attention_downsample_rate,
+ skip_first_layer_pe=(i == 0),
+ ))
+
+ self.final_attn_token_to_image = Attention(embedding_dim, num_heads, downsample_rate=attention_downsample_rate)
+ self.norm_final_attn = nn.LayerNorm(embedding_dim)
+
+ def forward(
+ self,
+ image_embedding: Tensor,
+ image_pe: Tensor,
+ point_embedding: Tensor,
+ ) -> Tuple[Tensor, Tensor]:
+ """
+ Args:
+ image_embedding (torch.Tensor): image to attend to. Should be shape
+ B x embedding_dim x h x w for any h and w.
+ image_pe (torch.Tensor): the positional encoding to add to the image. Must
+ have the same shape as image_embedding.
+ point_embedding (torch.Tensor): the embedding to add to the query points.
+ Must have shape B x N_points x embedding_dim for any N_points.
+
+ Returns:
+ torch.Tensor: the processed point_embedding
+ torch.Tensor: the processed image_embedding
+ """
+ # BxCxHxW -> BxHWxC == B x N_image_tokens x C
+ bs, c, h, w = image_embedding.shape
+ image_embedding = image_embedding.flatten(2).permute(0, 2, 1)
+ image_pe = image_pe.flatten(2).permute(0, 2, 1)
+
+ # Prepare queries
+ queries = point_embedding
+ keys = image_embedding
+
+ # Apply transformer blocks and final layernorm
+ for layer in self.layers:
+ queries, keys = layer(
+ queries=queries,
+ keys=keys,
+ query_pe=point_embedding,
+ key_pe=image_pe,
+ )
+
+ # Apply the final attention layer from the points to the image
+ q = queries + point_embedding
+ k = keys + image_pe
+ attn_out = self.final_attn_token_to_image(q=q, k=k, v=keys)
+ queries = queries + attn_out
+ queries = self.norm_final_attn(queries)
+
+ return queries, keys
+
+
+class TwoWayAttentionBlock(nn.Module):
+
+ def __init__(
+ self,
+ embedding_dim: int,
+ num_heads: int,
+ mlp_dim: int = 2048,
+ activation: Type[nn.Module] = nn.ReLU,
+ attention_downsample_rate: int = 2,
+ skip_first_layer_pe: bool = False,
+ ) -> None:
+ """
+ A transformer block with four layers: (1) self-attention of sparse
+ inputs, (2) cross attention of sparse inputs to dense inputs, (3) mlp
+ block on sparse inputs, and (4) cross attention of dense inputs to sparse
+ inputs.
+
+ Arguments:
+ embedding_dim (int): the channel dimension of the embeddings
+ num_heads (int): the number of heads in the attention layers
+ mlp_dim (int): the hidden dimension of the mlp block
+ activation (nn.Module): the activation of the mlp block
+ skip_first_layer_pe (bool): skip the PE on the first layer
+ """
+ super().__init__()
+ self.self_attn = Attention(embedding_dim, num_heads)
+ self.norm1 = nn.LayerNorm(embedding_dim)
+
+ self.cross_attn_token_to_image = Attention(embedding_dim, num_heads, downsample_rate=attention_downsample_rate)
+ self.norm2 = nn.LayerNorm(embedding_dim)
+
+ self.mlp = MLPBlock(embedding_dim, mlp_dim, activation)
+ self.norm3 = nn.LayerNorm(embedding_dim)
+
+ self.norm4 = nn.LayerNorm(embedding_dim)
+ self.cross_attn_image_to_token = Attention(embedding_dim, num_heads, downsample_rate=attention_downsample_rate)
+
+ self.skip_first_layer_pe = skip_first_layer_pe
+
+ def forward(self, queries: Tensor, keys: Tensor, query_pe: Tensor, key_pe: Tensor) -> Tuple[Tensor, Tensor]:
+ """Apply self-attention and cross-attention to queries and keys and return the processed embeddings."""
+
+ # Self attention block
+ if self.skip_first_layer_pe:
+ queries = self.self_attn(q=queries, k=queries, v=queries)
+ else:
+ q = queries + query_pe
+ attn_out = self.self_attn(q=q, k=q, v=queries)
+ queries = queries + attn_out
+ queries = self.norm1(queries)
+
+ # Cross attention block, tokens attending to image embedding
+ q = queries + query_pe
+ k = keys + key_pe
+ attn_out = self.cross_attn_token_to_image(q=q, k=k, v=keys)
+ queries = queries + attn_out
+ queries = self.norm2(queries)
+
+ # MLP block
+ mlp_out = self.mlp(queries)
+ queries = queries + mlp_out
+ queries = self.norm3(queries)
+
+ # Cross attention block, image embedding attending to tokens
+ q = queries + query_pe
+ k = keys + key_pe
+ attn_out = self.cross_attn_image_to_token(q=k, k=q, v=queries)
+ keys = keys + attn_out
+ keys = self.norm4(keys)
+
+ return queries, keys
+
+
+class Attention(nn.Module):
+ """
+ An attention layer that allows for downscaling the size of the embedding
+ after projection to queries, keys, and values.
+ """
+
+ def __init__(
+ self,
+ embedding_dim: int,
+ num_heads: int,
+ downsample_rate: int = 1,
+ ) -> None:
+ super().__init__()
+ self.embedding_dim = embedding_dim
+ self.internal_dim = embedding_dim // downsample_rate
+ self.num_heads = num_heads
+ assert self.internal_dim % num_heads == 0, 'num_heads must divide embedding_dim.'
+
+ self.q_proj = nn.Linear(embedding_dim, self.internal_dim)
+ self.k_proj = nn.Linear(embedding_dim, self.internal_dim)
+ self.v_proj = nn.Linear(embedding_dim, self.internal_dim)
+ self.out_proj = nn.Linear(self.internal_dim, embedding_dim)
+
+ def _separate_heads(self, x: Tensor, num_heads: int) -> Tensor:
+ """Separate the input tensor into the specified number of attention heads."""
+ b, n, c = x.shape
+ x = x.reshape(b, n, num_heads, c // num_heads)
+ return x.transpose(1, 2) # B x N_heads x N_tokens x C_per_head
+
+ def _recombine_heads(self, x: Tensor) -> Tensor:
+ """Recombine the separated attention heads into a single tensor."""
+ b, n_heads, n_tokens, c_per_head = x.shape
+ x = x.transpose(1, 2)
+ return x.reshape(b, n_tokens, n_heads * c_per_head) # B x N_tokens x C
+
+ def forward(self, q: Tensor, k: Tensor, v: Tensor) -> Tensor:
+ """Compute the attention output given the input query, key, and value tensors."""
+
+ # Input projections
+ q = self.q_proj(q)
+ k = self.k_proj(k)
+ v = self.v_proj(v)
+
+ # Separate into heads
+ q = self._separate_heads(q, self.num_heads)
+ k = self._separate_heads(k, self.num_heads)
+ v = self._separate_heads(v, self.num_heads)
+
+ # Attention
+ _, _, _, c_per_head = q.shape
+ attn = q @ k.permute(0, 1, 3, 2) # B x N_heads x N_tokens x N_tokens
+ attn = attn / math.sqrt(c_per_head)
+ attn = torch.softmax(attn, dim=-1)
+
+ # Get output
+ out = attn @ v
+ out = self._recombine_heads(out)
+ out = self.out_proj(out)
+
+ return out
diff --git a/yolov8/ultralytics/vit/sam/predict.py b/yolov8/ultralytics/vit/sam/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..063955de798f7dc43077ff3331b3c04bc3377e7a
--- /dev/null
+++ b/yolov8/ultralytics/vit/sam/predict.py
@@ -0,0 +1,54 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import numpy as np
+import torch
+
+from ultralytics.yolo.engine.predictor import BasePredictor
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils.torch_utils import select_device
+
+from .modules.mask_generator import SamAutomaticMaskGenerator
+
+
+class Predictor(BasePredictor):
+
+ def preprocess(self, im):
+ """Prepares input image for inference."""
+ # TODO: Only support bs=1 for now
+ # im = ResizeLongestSide(1024).apply_image(im[0])
+ # im = torch.as_tensor(im, device=self.device)
+ # im = im.permute(2, 0, 1).contiguous()[None, :, :, :]
+ return im[0]
+
+ def setup_model(self, model):
+ """Set up YOLO model with specified thresholds and device."""
+ device = select_device(self.args.device)
+ model.eval()
+ self.model = SamAutomaticMaskGenerator(model.to(device),
+ pred_iou_thresh=self.args.conf,
+ box_nms_thresh=self.args.iou)
+ self.device = device
+ # TODO: Temporary settings for compatibility
+ self.model.pt = False
+ self.model.triton = False
+ self.model.stride = 32
+ self.model.fp16 = False
+ self.done_warmup = True
+
+ def postprocess(self, preds, path, orig_imgs):
+ """Postprocesses inference output predictions to create detection masks for objects."""
+ names = dict(enumerate(list(range(len(preds)))))
+ results = []
+ # TODO
+ for i, pred in enumerate([preds]):
+ masks = torch.from_numpy(np.stack([p['segmentation'] for p in pred], axis=0))
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ results.append(Results(orig_img=orig_img, path=img_path, names=names, masks=masks))
+ return results
+
+ # def __call__(self, source=None, model=None, stream=False):
+ # frame = cv2.imread(source)
+ # preds = self.model.generate(frame)
+ # return self.postprocess(preds, source, frame)
diff --git a/yolov8/ultralytics/vit/utils/__init__.py b/yolov8/ultralytics/vit/utils/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..9e68dc12245afb4f72ba5f7c1227df74613a427d
--- /dev/null
+++ b/yolov8/ultralytics/vit/utils/__init__.py
@@ -0,0 +1 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
diff --git a/yolov8/ultralytics/vit/utils/loss.py b/yolov8/ultralytics/vit/utils/loss.py
new file mode 100644
index 0000000000000000000000000000000000000000..cb2de206f31e165270f5d74e7e3e3dac9642d897
--- /dev/null
+++ b/yolov8/ultralytics/vit/utils/loss.py
@@ -0,0 +1,294 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+
+from ultralytics.vit.utils.ops import HungarianMatcher
+from ultralytics.yolo.utils.loss import FocalLoss, VarifocalLoss
+from ultralytics.yolo.utils.metrics import bbox_iou
+
+
+class DETRLoss(nn.Module):
+
+ def __init__(self,
+ nc=80,
+ loss_gain=None,
+ aux_loss=True,
+ use_fl=True,
+ use_vfl=False,
+ use_uni_match=False,
+ uni_match_ind=0):
+ """
+ DETR loss function.
+
+ Args:
+ nc (int): The number of classes.
+ loss_gain (dict): The coefficient of loss.
+ aux_loss (bool): If 'aux_loss = True', loss at each decoder layer are to be used.
+ use_vfl (bool): Use VarifocalLoss or not.
+ use_uni_match (bool): Whether to use a fixed layer to assign labels for auxiliary branch.
+ uni_match_ind (int): The fixed indices of a layer.
+ """
+ super().__init__()
+
+ if loss_gain is None:
+ loss_gain = {'class': 1, 'bbox': 5, 'giou': 2, 'no_object': 0.1, 'mask': 1, 'dice': 1}
+ self.nc = nc
+ self.matcher = HungarianMatcher(cost_gain={'class': 2, 'bbox': 5, 'giou': 2})
+ self.loss_gain = loss_gain
+ self.aux_loss = aux_loss
+ self.fl = FocalLoss() if use_fl else None
+ self.vfl = VarifocalLoss() if use_vfl else None
+
+ self.use_uni_match = use_uni_match
+ self.uni_match_ind = uni_match_ind
+ self.device = None
+
+ def _get_loss_class(self, pred_scores, targets, gt_scores, num_gts, postfix=''):
+ # logits: [b, query, num_classes], gt_class: list[[n, 1]]
+ name_class = f'loss_class{postfix}'
+ bs, nq = pred_scores.shape[:2]
+ # one_hot = F.one_hot(targets, self.nc + 1)[..., :-1] # (bs, num_queries, num_classes)
+ one_hot = torch.zeros((bs, nq, self.nc + 1), dtype=torch.int64, device=targets.device)
+ one_hot.scatter_(2, targets.unsqueeze(-1), 1)
+ one_hot = one_hot[..., :-1]
+ gt_scores = gt_scores.view(bs, nq, 1) * one_hot
+
+ if self.fl:
+ if num_gts and self.vfl:
+ loss_cls = self.vfl(pred_scores, gt_scores, one_hot)
+ else:
+ loss_cls = self.fl(pred_scores, one_hot.float())
+ loss_cls /= max(num_gts, 1) / nq
+ else:
+ loss_cls = nn.BCEWithLogitsLoss(reduction='none')(pred_scores, gt_scores).mean(1).sum() # YOLO CLS loss
+
+ return {name_class: loss_cls.squeeze() * self.loss_gain['class']}
+
+ def _get_loss_bbox(self, pred_bboxes, gt_bboxes, postfix=''):
+ # boxes: [b, query, 4], gt_bbox: list[[n, 4]]
+ name_bbox = f'loss_bbox{postfix}'
+ name_giou = f'loss_giou{postfix}'
+
+ loss = {}
+ if len(gt_bboxes) == 0:
+ loss[name_bbox] = torch.tensor(0., device=self.device)
+ loss[name_giou] = torch.tensor(0., device=self.device)
+ return loss
+
+ loss[name_bbox] = self.loss_gain['bbox'] * F.l1_loss(pred_bboxes, gt_bboxes, reduction='sum') / len(gt_bboxes)
+ loss[name_giou] = 1.0 - bbox_iou(pred_bboxes, gt_bboxes, xywh=True, GIoU=True)
+ loss[name_giou] = loss[name_giou].sum() / len(gt_bboxes)
+ loss[name_giou] = self.loss_gain['giou'] * loss[name_giou]
+ loss = {k: v.squeeze() for k, v in loss.items()}
+ return loss
+
+ def _get_loss_mask(self, masks, gt_mask, match_indices, postfix=''):
+ # masks: [b, query, h, w], gt_mask: list[[n, H, W]]
+ name_mask = f'loss_mask{postfix}'
+ name_dice = f'loss_dice{postfix}'
+
+ loss = {}
+ if sum(len(a) for a in gt_mask) == 0:
+ loss[name_mask] = torch.tensor(0., device=self.device)
+ loss[name_dice] = torch.tensor(0., device=self.device)
+ return loss
+
+ num_gts = len(gt_mask)
+ src_masks, target_masks = self._get_assigned_bboxes(masks, gt_mask, match_indices)
+ src_masks = F.interpolate(src_masks.unsqueeze(0), size=target_masks.shape[-2:], mode='bilinear')[0]
+ # TODO: torch does not have `sigmoid_focal_loss`, but it's not urgent since we don't use mask branch for now.
+ loss[name_mask] = self.loss_gain['mask'] * F.sigmoid_focal_loss(src_masks, target_masks,
+ torch.tensor([num_gts], dtype=torch.float32))
+ loss[name_dice] = self.loss_gain['dice'] * self._dice_loss(src_masks, target_masks, num_gts)
+ return loss
+
+ def _dice_loss(self, inputs, targets, num_gts):
+ inputs = F.sigmoid(inputs)
+ inputs = inputs.flatten(1)
+ targets = targets.flatten(1)
+ numerator = 2 * (inputs * targets).sum(1)
+ denominator = inputs.sum(-1) + targets.sum(-1)
+ loss = 1 - (numerator + 1) / (denominator + 1)
+ return loss.sum() / num_gts
+
+ def _get_loss_aux(self,
+ pred_bboxes,
+ pred_scores,
+ gt_bboxes,
+ gt_cls,
+ gt_groups,
+ match_indices=None,
+ postfix='',
+ masks=None,
+ gt_mask=None):
+ """Get auxiliary losses"""
+ # NOTE: loss class, bbox, giou, mask, dice
+ loss = torch.zeros(5 if masks is not None else 3, device=pred_bboxes.device)
+ if match_indices is None and self.use_uni_match:
+ match_indices = self.matcher(pred_bboxes[self.uni_match_ind],
+ pred_scores[self.uni_match_ind],
+ gt_bboxes,
+ gt_cls,
+ gt_groups,
+ masks=masks[self.uni_match_ind] if masks is not None else None,
+ gt_mask=gt_mask)
+ for i, (aux_bboxes, aux_scores) in enumerate(zip(pred_bboxes, pred_scores)):
+ aux_masks = masks[i] if masks is not None else None
+ loss_ = self._get_loss(aux_bboxes,
+ aux_scores,
+ gt_bboxes,
+ gt_cls,
+ gt_groups,
+ masks=aux_masks,
+ gt_mask=gt_mask,
+ postfix=postfix,
+ match_indices=match_indices)
+ loss[0] += loss_[f'loss_class{postfix}']
+ loss[1] += loss_[f'loss_bbox{postfix}']
+ loss[2] += loss_[f'loss_giou{postfix}']
+ # if masks is not None and gt_mask is not None:
+ # loss_ = self._get_loss_mask(aux_masks, gt_mask, match_indices, postfix)
+ # loss[3] += loss_[f'loss_mask{postfix}']
+ # loss[4] += loss_[f'loss_dice{postfix}']
+
+ loss = {
+ f'loss_class_aux{postfix}': loss[0],
+ f'loss_bbox_aux{postfix}': loss[1],
+ f'loss_giou_aux{postfix}': loss[2]}
+ # if masks is not None and gt_mask is not None:
+ # loss[f'loss_mask_aux{postfix}'] = loss[3]
+ # loss[f'loss_dice_aux{postfix}'] = loss[4]
+ return loss
+
+ def _get_index(self, match_indices):
+ batch_idx = torch.cat([torch.full_like(src, i) for i, (src, _) in enumerate(match_indices)])
+ src_idx = torch.cat([src for (src, _) in match_indices])
+ dst_idx = torch.cat([dst for (_, dst) in match_indices])
+ return (batch_idx, src_idx), dst_idx
+
+ def _get_assigned_bboxes(self, pred_bboxes, gt_bboxes, match_indices):
+ pred_assigned = torch.cat([
+ t[I] if len(I) > 0 else torch.zeros(0, t.shape[-1], device=self.device)
+ for t, (I, _) in zip(pred_bboxes, match_indices)])
+ gt_assigned = torch.cat([
+ t[J] if len(J) > 0 else torch.zeros(0, t.shape[-1], device=self.device)
+ for t, (_, J) in zip(gt_bboxes, match_indices)])
+ return pred_assigned, gt_assigned
+
+ def _get_loss(self,
+ pred_bboxes,
+ pred_scores,
+ gt_bboxes,
+ gt_cls,
+ gt_groups,
+ masks=None,
+ gt_mask=None,
+ postfix='',
+ match_indices=None):
+ """Get losses"""
+ if match_indices is None:
+ match_indices = self.matcher(pred_bboxes,
+ pred_scores,
+ gt_bboxes,
+ gt_cls,
+ gt_groups,
+ masks=masks,
+ gt_mask=gt_mask)
+
+ idx, gt_idx = self._get_index(match_indices)
+ pred_bboxes, gt_bboxes = pred_bboxes[idx], gt_bboxes[gt_idx]
+
+ bs, nq = pred_scores.shape[:2]
+ targets = torch.full((bs, nq), self.nc, device=pred_scores.device, dtype=gt_cls.dtype)
+ targets[idx] = gt_cls[gt_idx]
+
+ gt_scores = torch.zeros([bs, nq], device=pred_scores.device)
+ if len(gt_bboxes):
+ gt_scores[idx] = bbox_iou(pred_bboxes.detach(), gt_bboxes, xywh=True).squeeze(-1)
+
+ loss = {}
+ loss.update(self._get_loss_class(pred_scores, targets, gt_scores, len(gt_bboxes), postfix))
+ loss.update(self._get_loss_bbox(pred_bboxes, gt_bboxes, postfix))
+ # if masks is not None and gt_mask is not None:
+ # loss.update(self._get_loss_mask(masks, gt_mask, match_indices, postfix))
+ return loss
+
+ def forward(self, pred_bboxes, pred_scores, batch, postfix='', **kwargs):
+ """
+ Args:
+ pred_bboxes (torch.Tensor): [l, b, query, 4]
+ pred_scores (torch.Tensor): [l, b, query, num_classes]
+ batch (dict): A dict includes:
+ gt_cls (torch.Tensor) with shape [num_gts, ],
+ gt_bboxes (torch.Tensor): [num_gts, 4],
+ gt_groups (List(int)): a list of batch size length includes the number of gts of each image.
+ postfix (str): postfix of loss name.
+ """
+ self.device = pred_bboxes.device
+ match_indices = kwargs.get('match_indices', None)
+ gt_cls, gt_bboxes, gt_groups = batch['cls'], batch['bboxes'], batch['gt_groups']
+
+ total_loss = self._get_loss(pred_bboxes[-1],
+ pred_scores[-1],
+ gt_bboxes,
+ gt_cls,
+ gt_groups,
+ postfix=postfix,
+ match_indices=match_indices)
+
+ if self.aux_loss:
+ total_loss.update(
+ self._get_loss_aux(pred_bboxes[:-1], pred_scores[:-1], gt_bboxes, gt_cls, gt_groups, match_indices,
+ postfix))
+
+ return total_loss
+
+
+class RTDETRDetectionLoss(DETRLoss):
+
+ def forward(self, preds, batch, dn_bboxes=None, dn_scores=None, dn_meta=None):
+ pred_bboxes, pred_scores = preds
+ total_loss = super().forward(pred_bboxes, pred_scores, batch)
+
+ if dn_meta is not None:
+ dn_pos_idx, dn_num_group = dn_meta['dn_pos_idx'], dn_meta['dn_num_group']
+ assert len(batch['gt_groups']) == len(dn_pos_idx)
+
+ # denoising match indices
+ match_indices = self.get_dn_match_indices(dn_pos_idx, dn_num_group, batch['gt_groups'])
+
+ # compute denoising training loss
+ dn_loss = super().forward(dn_bboxes, dn_scores, batch, postfix='_dn', match_indices=match_indices)
+ total_loss.update(dn_loss)
+ else:
+ total_loss.update({f'{k}_dn': torch.tensor(0., device=self.device) for k in total_loss.keys()})
+
+ return total_loss
+
+ @staticmethod
+ def get_dn_match_indices(dn_pos_idx, dn_num_group, gt_groups):
+ """Get the match indices for denoising.
+
+ Args:
+ dn_pos_idx (List[torch.Tensor]): A list includes positive indices of denoising.
+ dn_num_group (int): The number of groups of denoising.
+ gt_groups (List(int)): a list of batch size length includes the number of gts of each image.
+
+ Returns:
+ dn_match_indices (List(tuple)): Matched indices.
+
+ """
+ dn_match_indices = []
+ idx_groups = torch.as_tensor([0, *gt_groups[:-1]]).cumsum_(0)
+ for i, num_gt in enumerate(gt_groups):
+ if num_gt > 0:
+ gt_idx = torch.arange(end=num_gt, dtype=torch.long) + idx_groups[i]
+ gt_idx = gt_idx.repeat(dn_num_group)
+ assert len(dn_pos_idx[i]) == len(gt_idx), 'Expected the same length, '
+ f'but got {len(dn_pos_idx[i])} and {len(gt_idx)} respectively.'
+ dn_match_indices.append((dn_pos_idx[i], gt_idx))
+ else:
+ dn_match_indices.append((torch.zeros([0], dtype=torch.long), torch.zeros([0], dtype=torch.long)))
+ return dn_match_indices
diff --git a/yolov8/ultralytics/vit/utils/ops.py b/yolov8/ultralytics/vit/utils/ops.py
new file mode 100644
index 0000000000000000000000000000000000000000..4b37931927884bc975ffb066932d9284c09dd3f8
--- /dev/null
+++ b/yolov8/ultralytics/vit/utils/ops.py
@@ -0,0 +1,260 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+from scipy.optimize import linear_sum_assignment
+
+from ultralytics.yolo.utils.metrics import bbox_iou
+from ultralytics.yolo.utils.ops import xywh2xyxy, xyxy2xywh
+
+
+class HungarianMatcher(nn.Module):
+ """
+ A module implementing the HungarianMatcher, which is a differentiable module to solve the assignment problem in
+ an end-to-end fashion.
+
+ HungarianMatcher performs optimal assignment over predicted and ground truth bounding boxes using a cost function
+ that considers classification scores, bounding box coordinates, and optionally, mask predictions.
+
+ Attributes:
+ cost_gain (dict): Dictionary of cost coefficients for different components: 'class', 'bbox', 'giou', 'mask', and 'dice'.
+ use_fl (bool): Indicates whether to use Focal Loss for the classification cost calculation.
+ with_mask (bool): Indicates whether the model makes mask predictions.
+ num_sample_points (int): The number of sample points used in mask cost calculation.
+ alpha (float): The alpha factor in Focal Loss calculation.
+ gamma (float): The gamma factor in Focal Loss calculation.
+
+ Methods:
+ forward(pred_bboxes, pred_scores, gt_bboxes, gt_cls, gt_groups, masks=None, gt_mask=None): Computes the assignment
+ between predictions and ground truths for a batch.
+ _cost_mask(bs, num_gts, masks=None, gt_mask=None): Computes the mask cost and dice cost if masks are predicted.
+ """
+
+ def __init__(self, cost_gain=None, use_fl=True, with_mask=False, num_sample_points=12544, alpha=0.25, gamma=2.0):
+ super().__init__()
+ if cost_gain is None:
+ cost_gain = {'class': 1, 'bbox': 5, 'giou': 2, 'mask': 1, 'dice': 1}
+ self.cost_gain = cost_gain
+ self.use_fl = use_fl
+ self.with_mask = with_mask
+ self.num_sample_points = num_sample_points
+ self.alpha = alpha
+ self.gamma = gamma
+
+ def forward(self, pred_bboxes, pred_scores, gt_bboxes, gt_cls, gt_groups, masks=None, gt_mask=None):
+ """
+ Forward pass for HungarianMatcher. This function computes costs based on prediction and ground truth
+ (classification cost, L1 cost between boxes and GIoU cost between boxes) and finds the optimal matching
+ between predictions and ground truth based on these costs.
+
+ Args:
+ pred_bboxes (Tensor): Predicted bounding boxes with shape [batch_size, num_queries, 4].
+ pred_scores (Tensor): Predicted scores with shape [batch_size, num_queries, num_classes].
+ gt_cls (torch.Tensor): Ground truth classes with shape [num_gts, ].
+ gt_bboxes (torch.Tensor): Ground truth bounding boxes with shape [num_gts, 4].
+ gt_groups (List[int]): List of length equal to batch size, containing the number of ground truths for
+ each image.
+ masks (Tensor, optional): Predicted masks with shape [batch_size, num_queries, height, width].
+ Defaults to None.
+ gt_mask (List[Tensor], optional): List of ground truth masks, each with shape [num_masks, Height, Width].
+ Defaults to None.
+
+ Returns:
+ (List[Tuple[Tensor, Tensor]]): A list of size batch_size, each element is a tuple (index_i, index_j), where:
+ - index_i is the tensor of indices of the selected predictions (in order)
+ - index_j is the tensor of indices of the corresponding selected ground truth targets (in order)
+ For each batch element, it holds:
+ len(index_i) = len(index_j) = min(num_queries, num_target_boxes)
+ """
+
+ bs, nq, nc = pred_scores.shape
+
+ if sum(gt_groups) == 0:
+ return [(torch.tensor([], dtype=torch.long), torch.tensor([], dtype=torch.long)) for _ in range(bs)]
+
+ # We flatten to compute the cost matrices in a batch
+ # [batch_size * num_queries, num_classes]
+ pred_scores = pred_scores.detach().view(-1, nc)
+ pred_scores = F.sigmoid(pred_scores) if self.use_fl else F.softmax(pred_scores, dim=-1)
+ # [batch_size * num_queries, 4]
+ pred_bboxes = pred_bboxes.detach().view(-1, 4)
+
+ # Compute the classification cost
+ pred_scores = pred_scores[:, gt_cls]
+ if self.use_fl:
+ neg_cost_class = (1 - self.alpha) * (pred_scores ** self.gamma) * (-(1 - pred_scores + 1e-8).log())
+ pos_cost_class = self.alpha * ((1 - pred_scores) ** self.gamma) * (-(pred_scores + 1e-8).log())
+ cost_class = pos_cost_class - neg_cost_class
+ else:
+ cost_class = -pred_scores
+
+ # Compute the L1 cost between boxes
+ cost_bbox = (pred_bboxes.unsqueeze(1) - gt_bboxes.unsqueeze(0)).abs().sum(-1) # (bs*num_queries, num_gt)
+
+ # Compute the GIoU cost between boxes, (bs*num_queries, num_gt)
+ cost_giou = 1.0 - bbox_iou(pred_bboxes.unsqueeze(1), gt_bboxes.unsqueeze(0), xywh=True, GIoU=True).squeeze(-1)
+
+ # Final cost matrix
+ C = self.cost_gain['class'] * cost_class + \
+ self.cost_gain['bbox'] * cost_bbox + \
+ self.cost_gain['giou'] * cost_giou
+ # Compute the mask cost and dice cost
+ if self.with_mask:
+ C += self._cost_mask(bs, gt_groups, masks, gt_mask)
+
+ C = C.view(bs, nq, -1).cpu()
+ indices = [linear_sum_assignment(c[i]) for i, c in enumerate(C.split(gt_groups, -1))]
+ gt_groups = torch.as_tensor([0, *gt_groups[:-1]]).cumsum_(0)
+ # (idx for queries, idx for gt)
+ return [(torch.tensor(i, dtype=torch.long), torch.tensor(j, dtype=torch.long) + gt_groups[k])
+ for k, (i, j) in enumerate(indices)]
+
+ def _cost_mask(self, bs, num_gts, masks=None, gt_mask=None):
+ assert masks is not None and gt_mask is not None, 'Make sure the input has `mask` and `gt_mask`'
+ # all masks share the same set of points for efficient matching
+ sample_points = torch.rand([bs, 1, self.num_sample_points, 2])
+ sample_points = 2.0 * sample_points - 1.0
+
+ out_mask = F.grid_sample(masks.detach(), sample_points, align_corners=False).squeeze(-2)
+ out_mask = out_mask.flatten(0, 1)
+
+ tgt_mask = torch.cat(gt_mask).unsqueeze(1)
+ sample_points = torch.cat([a.repeat(b, 1, 1, 1) for a, b in zip(sample_points, num_gts) if b > 0])
+ tgt_mask = F.grid_sample(tgt_mask, sample_points, align_corners=False).squeeze([1, 2])
+
+ with torch.cuda.amp.autocast(False):
+ # binary cross entropy cost
+ pos_cost_mask = F.binary_cross_entropy_with_logits(out_mask, torch.ones_like(out_mask), reduction='none')
+ neg_cost_mask = F.binary_cross_entropy_with_logits(out_mask, torch.zeros_like(out_mask), reduction='none')
+ cost_mask = torch.matmul(pos_cost_mask, tgt_mask.T) + torch.matmul(neg_cost_mask, 1 - tgt_mask.T)
+ cost_mask /= self.num_sample_points
+
+ # dice cost
+ out_mask = F.sigmoid(out_mask)
+ numerator = 2 * torch.matmul(out_mask, tgt_mask.T)
+ denominator = out_mask.sum(-1, keepdim=True) + tgt_mask.sum(-1).unsqueeze(0)
+ cost_dice = 1 - (numerator + 1) / (denominator + 1)
+
+ C = self.cost_gain['mask'] * cost_mask + self.cost_gain['dice'] * cost_dice
+ return C
+
+
+def get_cdn_group(batch,
+ num_classes,
+ num_queries,
+ class_embed,
+ num_dn=100,
+ cls_noise_ratio=0.5,
+ box_noise_scale=1.0,
+ training=False):
+ """
+ Get contrastive denoising training group. This function creates a contrastive denoising training group with
+ positive and negative samples from the ground truths (gt). It applies noise to the class labels and bounding
+ box coordinates, and returns the modified labels, bounding boxes, attention mask and meta information.
+
+ Args:
+ batch (dict): A dict that includes 'gt_cls' (torch.Tensor with shape [num_gts, ]), 'gt_bboxes'
+ (torch.Tensor with shape [num_gts, 4]), 'gt_groups' (List(int)) which is a list of batch size length
+ indicating the number of gts of each image.
+ num_classes (int): Number of classes.
+ num_queries (int): Number of queries.
+ class_embed (torch.Tensor): Embedding weights to map class labels to embedding space.
+ num_dn (int, optional): Number of denoising. Defaults to 100.
+ cls_noise_ratio (float, optional): Noise ratio for class labels. Defaults to 0.5.
+ box_noise_scale (float, optional): Noise scale for bounding box coordinates. Defaults to 1.0.
+ training (bool, optional): If it's in training mode. Defaults to False.
+
+ Returns:
+ (Tuple[Optional[Tensor], Optional[Tensor], Optional[Tensor], Optional[Dict]]): The modified class embeddings,
+ bounding boxes, attention mask and meta information for denoising. If not in training mode or 'num_dn'
+ is less than or equal to 0, the function returns None for all elements in the tuple.
+ """
+
+ if (not training) or num_dn <= 0:
+ return None, None, None, None
+ gt_groups = batch['gt_groups']
+ total_num = sum(gt_groups)
+ max_nums = max(gt_groups)
+ if max_nums == 0:
+ return None, None, None, None
+
+ num_group = num_dn // max_nums
+ num_group = 1 if num_group == 0 else num_group
+ # pad gt to max_num of a batch
+ bs = len(gt_groups)
+ gt_cls = batch['cls'] # (bs*num, )
+ gt_bbox = batch['bboxes'] # bs*num, 4
+ b_idx = batch['batch_idx']
+
+ # each group has positive and negative queries.
+ dn_cls = gt_cls.repeat(2 * num_group) # (2*num_group*bs*num, )
+ dn_bbox = gt_bbox.repeat(2 * num_group, 1) # 2*num_group*bs*num, 4
+ dn_b_idx = b_idx.repeat(2 * num_group).view(-1) # (2*num_group*bs*num, )
+
+ # positive and negative mask
+ # (bs*num*num_group, ), the second total_num*num_group part as negative samples
+ neg_idx = torch.arange(total_num * num_group, dtype=torch.long, device=gt_bbox.device) + num_group * total_num
+
+ if cls_noise_ratio > 0:
+ # half of bbox prob
+ mask = torch.rand(dn_cls.shape) < (cls_noise_ratio * 0.5)
+ idx = torch.nonzero(mask).squeeze(-1)
+ # randomly put a new one here
+ new_label = torch.randint_like(idx, 0, num_classes, dtype=dn_cls.dtype, device=dn_cls.device)
+ dn_cls[idx] = new_label
+
+ if box_noise_scale > 0:
+ known_bbox = xywh2xyxy(dn_bbox)
+
+ diff = (dn_bbox[..., 2:] * 0.5).repeat(1, 2) * box_noise_scale # 2*num_group*bs*num, 4
+
+ rand_sign = torch.randint_like(dn_bbox, 0, 2) * 2.0 - 1.0
+ rand_part = torch.rand_like(dn_bbox)
+ rand_part[neg_idx] += 1.0
+ rand_part *= rand_sign
+ known_bbox += rand_part * diff
+ known_bbox.clip_(min=0.0, max=1.0)
+ dn_bbox = xyxy2xywh(known_bbox)
+ dn_bbox = inverse_sigmoid(dn_bbox)
+
+ # total denoising queries
+ num_dn = int(max_nums * 2 * num_group)
+ # class_embed = torch.cat([class_embed, torch.zeros([1, class_embed.shape[-1]], device=class_embed.device)])
+ dn_cls_embed = class_embed[dn_cls] # bs*num * 2 * num_group, 256
+ padding_cls = torch.zeros(bs, num_dn, dn_cls_embed.shape[-1], device=gt_cls.device)
+ padding_bbox = torch.zeros(bs, num_dn, 4, device=gt_bbox.device)
+
+ map_indices = torch.cat([torch.tensor(range(num), dtype=torch.long) for num in gt_groups])
+ pos_idx = torch.stack([map_indices + max_nums * i for i in range(num_group)], dim=0)
+
+ map_indices = torch.cat([map_indices + max_nums * i for i in range(2 * num_group)])
+ padding_cls[(dn_b_idx, map_indices)] = dn_cls_embed
+ padding_bbox[(dn_b_idx, map_indices)] = dn_bbox
+
+ tgt_size = num_dn + num_queries
+ attn_mask = torch.zeros([tgt_size, tgt_size], dtype=torch.bool)
+ # match query cannot see the reconstruct
+ attn_mask[num_dn:, :num_dn] = True
+ # reconstruct cannot see each other
+ for i in range(num_group):
+ if i == 0:
+ attn_mask[max_nums * 2 * i:max_nums * 2 * (i + 1), max_nums * 2 * (i + 1):num_dn] = True
+ if i == num_group - 1:
+ attn_mask[max_nums * 2 * i:max_nums * 2 * (i + 1), :max_nums * i * 2] = True
+ else:
+ attn_mask[max_nums * 2 * i:max_nums * 2 * (i + 1), max_nums * 2 * (i + 1):num_dn] = True
+ attn_mask[max_nums * 2 * i:max_nums * 2 * (i + 1), :max_nums * 2 * i] = True
+ dn_meta = {
+ 'dn_pos_idx': [p.reshape(-1) for p in pos_idx.cpu().split(list(gt_groups), dim=1)],
+ 'dn_num_group': num_group,
+ 'dn_num_split': [num_dn, num_queries]}
+
+ return padding_cls.to(class_embed.device), padding_bbox.to(class_embed.device), attn_mask.to(
+ class_embed.device), dn_meta
+
+
+def inverse_sigmoid(x, eps=1e-6):
+ """Inverse sigmoid function."""
+ x = x.clip(min=0., max=1.)
+ return torch.log(x / (1 - x + eps) + eps)
diff --git a/yolov8/ultralytics/yolo/__init__.py b/yolov8/ultralytics/yolo/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..d1fa55845814759a36b040edb2cfcd930d2229f3
--- /dev/null
+++ b/yolov8/ultralytics/yolo/__init__.py
@@ -0,0 +1,5 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from . import v8
+
+__all__ = 'v8', # tuple or list
diff --git a/yolov8/ultralytics/yolo/cfg/__init__.py b/yolov8/ultralytics/yolo/cfg/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..5908fa1dbc6620228a8cffe2842eb6f511d7c705
--- /dev/null
+++ b/yolov8/ultralytics/yolo/cfg/__init__.py
@@ -0,0 +1,421 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+import re
+import shutil
+import sys
+from difflib import get_close_matches
+from pathlib import Path
+from types import SimpleNamespace
+from typing import Dict, List, Union
+
+from ultralytics.yolo.utils import (DEFAULT_CFG, DEFAULT_CFG_DICT, DEFAULT_CFG_PATH, LOGGER, ROOT, USER_CONFIG_DIR,
+ IterableSimpleNamespace, __version__, checks, colorstr, deprecation_warn,
+ get_settings, yaml_load, yaml_print)
+
+# Define valid tasks and modes
+MODES = 'train', 'val', 'predict', 'export', 'track', 'benchmark'
+TASKS = 'detect', 'segment', 'classify', 'pose'
+TASK2DATA = {'detect': 'coco8.yaml', 'segment': 'coco8-seg.yaml', 'classify': 'imagenet100', 'pose': 'coco8-pose.yaml'}
+TASK2MODEL = {
+ 'detect': 'yolov8n.pt',
+ 'segment': 'yolov8n-seg.pt',
+ 'classify': 'yolov8n-cls.pt',
+ 'pose': 'yolov8n-pose.pt'}
+TASK2METRIC = {
+ 'detect': 'metrics/mAP50-95(B)',
+ 'segment': 'metrics/mAP50-95(M)',
+ 'classify': 'metrics/accuracy_top1',
+ 'pose': 'metrics/mAP50-95(P)'}
+
+
+CLI_HELP_MSG = \
+ f"""
+ Arguments received: {str(['yolo'] + sys.argv[1:])}. Ultralytics 'yolo' commands use the following syntax:
+
+ yolo TASK MODE ARGS
+
+ Where TASK (optional) is one of {TASKS}
+ MODE (required) is one of {MODES}
+ ARGS (optional) are any number of custom 'arg=value' pairs like 'imgsz=320' that override defaults.
+ See all ARGS at https://docs.ultralytics.com/usage/cfg or with 'yolo cfg'
+
+ 1. Train a detection model for 10 epochs with an initial learning_rate of 0.01
+ yolo train data=coco128.yaml model=yolov8n.pt epochs=10 lr0=0.01
+
+ 2. Predict a YouTube video using a pretrained segmentation model at image size 320:
+ yolo predict model=yolov8n-seg.pt source='https://youtu.be/Zgi9g1ksQHc' imgsz=320
+
+ 3. Val a pretrained detection model at batch-size 1 and image size 640:
+ yolo val model=yolov8n.pt data=coco128.yaml batch=1 imgsz=640
+
+ 4. Export a YOLOv8n classification model to ONNX format at image size 224 by 128 (no TASK required)
+ yolo export model=yolov8n-cls.pt format=onnx imgsz=224,128
+
+ 5. Run special commands:
+ yolo help
+ yolo checks
+ yolo version
+ yolo settings
+ yolo copy-cfg
+ yolo cfg
+
+ Docs: https://docs.ultralytics.com
+ Community: https://community.ultralytics.com
+ GitHub: https://github.com/ultralytics/ultralytics
+ """
+
+# Define keys for arg type checks
+CFG_FLOAT_KEYS = 'warmup_epochs', 'box', 'cls', 'dfl', 'degrees', 'shear'
+CFG_FRACTION_KEYS = ('dropout', 'iou', 'lr0', 'lrf', 'momentum', 'weight_decay', 'warmup_momentum', 'warmup_bias_lr',
+ 'label_smoothing', 'hsv_h', 'hsv_s', 'hsv_v', 'translate', 'scale', 'perspective', 'flipud',
+ 'fliplr', 'mosaic', 'mixup', 'copy_paste', 'conf', 'iou', 'fraction') # fraction floats 0.0 - 1.0
+CFG_INT_KEYS = ('epochs', 'patience', 'batch', 'workers', 'seed', 'close_mosaic', 'mask_ratio', 'max_det', 'vid_stride',
+ 'line_width', 'workspace', 'nbs', 'save_period')
+CFG_BOOL_KEYS = ('save', 'exist_ok', 'verbose', 'deterministic', 'single_cls', 'rect', 'cos_lr', 'overlap_mask', 'val',
+ 'save_json', 'save_hybrid', 'half', 'dnn', 'plots', 'show', 'save_txt', 'save_conf', 'save_crop',
+ 'show_labels', 'show_conf', 'visualize', 'augment', 'agnostic_nms', 'retina_masks', 'boxes', 'keras',
+ 'optimize', 'int8', 'dynamic', 'simplify', 'nms', 'v5loader', 'profile')
+
+
+def cfg2dict(cfg):
+ """
+ Convert a configuration object to a dictionary, whether it is a file path, a string, or a SimpleNamespace object.
+
+ Args:
+ cfg (str | Path | SimpleNamespace): Configuration object to be converted to a dictionary.
+
+ Returns:
+ cfg (dict): Configuration object in dictionary format.
+ """
+ if isinstance(cfg, (str, Path)):
+ cfg = yaml_load(cfg) # load dict
+ elif isinstance(cfg, SimpleNamespace):
+ cfg = vars(cfg) # convert to dict
+ return cfg
+
+
+def get_cfg(cfg: Union[str, Path, Dict, SimpleNamespace] = DEFAULT_CFG_DICT, overrides: Dict = None):
+ """
+ Load and merge configuration data from a file or dictionary.
+
+ Args:
+ cfg (str | Path | Dict | SimpleNamespace): Configuration data.
+ overrides (str | Dict | optional): Overrides in the form of a file name or a dictionary. Default is None.
+
+ Returns:
+ (SimpleNamespace): Training arguments namespace.
+ """
+ cfg = cfg2dict(cfg)
+
+ # Merge overrides
+ if overrides:
+ overrides = cfg2dict(overrides)
+ check_cfg_mismatch(cfg, overrides)
+ cfg = {**cfg, **overrides} # merge cfg and overrides dicts (prefer overrides)
+
+ # Special handling for numeric project/name
+ for k in 'project', 'name':
+ if k in cfg and isinstance(cfg[k], (int, float)):
+ cfg[k] = str(cfg[k])
+ if cfg.get('name') == 'model': # assign model to 'name' arg
+ cfg['name'] = cfg.get('model', '').split('.')[0]
+ LOGGER.warning(f"WARNING ⚠️ 'name=model' automatically updated to 'name={cfg['name']}'.")
+
+ # Type and Value checks
+ for k, v in cfg.items():
+ if v is not None: # None values may be from optional args
+ if k in CFG_FLOAT_KEYS and not isinstance(v, (int, float)):
+ raise TypeError(f"'{k}={v}' is of invalid type {type(v).__name__}. "
+ f"Valid '{k}' types are int (i.e. '{k}=0') or float (i.e. '{k}=0.5')")
+ elif k in CFG_FRACTION_KEYS:
+ if not isinstance(v, (int, float)):
+ raise TypeError(f"'{k}={v}' is of invalid type {type(v).__name__}. "
+ f"Valid '{k}' types are int (i.e. '{k}=0') or float (i.e. '{k}=0.5')")
+ if not (0.0 <= v <= 1.0):
+ raise ValueError(f"'{k}={v}' is an invalid value. "
+ f"Valid '{k}' values are between 0.0 and 1.0.")
+ elif k in CFG_INT_KEYS and not isinstance(v, int):
+ raise TypeError(f"'{k}={v}' is of invalid type {type(v).__name__}. "
+ f"'{k}' must be an int (i.e. '{k}=8')")
+ elif k in CFG_BOOL_KEYS and not isinstance(v, bool):
+ raise TypeError(f"'{k}={v}' is of invalid type {type(v).__name__}. "
+ f"'{k}' must be a bool (i.e. '{k}=True' or '{k}=False')")
+
+ # Return instance
+ return IterableSimpleNamespace(**cfg)
+
+
+def _handle_deprecation(custom):
+ """
+ Hardcoded function to handle deprecated config keys
+ """
+
+ for key in custom.copy().keys():
+ if key == 'hide_labels':
+ deprecation_warn(key, 'show_labels')
+ custom['show_labels'] = custom.pop('hide_labels') == 'False'
+ if key == 'hide_conf':
+ deprecation_warn(key, 'show_conf')
+ custom['show_conf'] = custom.pop('hide_conf') == 'False'
+ if key == 'line_thickness':
+ deprecation_warn(key, 'line_width')
+ custom['line_width'] = custom.pop('line_thickness')
+
+ return custom
+
+
+def check_cfg_mismatch(base: Dict, custom: Dict, e=None):
+ """
+ This function checks for any mismatched keys between a custom configuration list and a base configuration list.
+ If any mismatched keys are found, the function prints out similar keys from the base list and exits the program.
+
+ Args:
+ custom (Dict): a dictionary of custom configuration options
+ base (Dict): a dictionary of base configuration options
+ """
+ custom = _handle_deprecation(custom)
+ base, custom = (set(x.keys()) for x in (base, custom))
+ mismatched = [x for x in custom if x not in base]
+ if mismatched:
+ string = ''
+ for x in mismatched:
+ matches = get_close_matches(x, base) # key list
+ matches = [f'{k}={DEFAULT_CFG_DICT[k]}' if DEFAULT_CFG_DICT.get(k) is not None else k for k in matches]
+ match_str = f'Similar arguments are i.e. {matches}.' if matches else ''
+ string += f"'{colorstr('red', 'bold', x)}' is not a valid YOLO argument. {match_str}\n"
+ raise SyntaxError(string + CLI_HELP_MSG) from e
+
+
+def merge_equals_args(args: List[str]) -> List[str]:
+ """
+ Merges arguments around isolated '=' args in a list of strings.
+ The function considers cases where the first argument ends with '=' or the second starts with '=',
+ as well as when the middle one is an equals sign.
+
+ Args:
+ args (List[str]): A list of strings where each element is an argument.
+
+ Returns:
+ List[str]: A list of strings where the arguments around isolated '=' are merged.
+ """
+ new_args = []
+ for i, arg in enumerate(args):
+ if arg == '=' and 0 < i < len(args) - 1: # merge ['arg', '=', 'val']
+ new_args[-1] += f'={args[i + 1]}'
+ del args[i + 1]
+ elif arg.endswith('=') and i < len(args) - 1 and '=' not in args[i + 1]: # merge ['arg=', 'val']
+ new_args.append(f'{arg}{args[i + 1]}')
+ del args[i + 1]
+ elif arg.startswith('=') and i > 0: # merge ['arg', '=val']
+ new_args[-1] += arg
+ else:
+ new_args.append(arg)
+ return new_args
+
+
+def handle_yolo_hub(args: List[str]) -> None:
+ """
+ Handle Ultralytics HUB command-line interface (CLI) commands.
+
+ This function processes Ultralytics HUB CLI commands such as login and logout.
+ It should be called when executing a script with arguments related to HUB authentication.
+
+ Args:
+ args (List[str]): A list of command line arguments
+
+ Example:
+ python my_script.py hub login your_api_key
+ """
+ from ultralytics import hub
+
+ if args[0] == 'login':
+ key = args[1] if len(args) > 1 else ''
+ # Log in to Ultralytics HUB using the provided API key
+ hub.login(key)
+ elif args[0] == 'logout':
+ # Log out from Ultralytics HUB
+ hub.logout()
+
+
+def handle_yolo_settings(args: List[str]) -> None:
+ """
+ Handle YOLO settings command-line interface (CLI) commands.
+
+ This function processes YOLO settings CLI commands such as reset.
+ It should be called when executing a script with arguments related to YOLO settings management.
+
+ Args:
+ args (List[str]): A list of command line arguments for YOLO settings management.
+
+ Example:
+ python my_script.py yolo settings reset
+ """
+ path = USER_CONFIG_DIR / 'settings.yaml' # get SETTINGS YAML file path
+ if any(args) and args[0] == 'reset':
+ path.unlink() # delete the settings file
+ get_settings() # create new settings
+ LOGGER.info('Settings reset successfully') # inform the user that settings have been reset
+ yaml_print(path) # print the current settings
+
+
+def entrypoint(debug=''):
+ """
+ This function is the ultralytics package entrypoint, it's responsible for parsing the command line arguments passed
+ to the package.
+
+ This function allows for:
+ - passing mandatory YOLO args as a list of strings
+ - specifying the task to be performed, either 'detect', 'segment' or 'classify'
+ - specifying the mode, either 'train', 'val', 'test', or 'predict'
+ - running special modes like 'checks'
+ - passing overrides to the package's configuration
+
+ It uses the package's default cfg and initializes it using the passed overrides.
+ Then it calls the CLI function with the composed cfg
+ """
+ args = (debug.split(' ') if debug else sys.argv)[1:]
+ if not args: # no arguments passed
+ LOGGER.info(CLI_HELP_MSG)
+ return
+
+ special = {
+ 'help': lambda: LOGGER.info(CLI_HELP_MSG),
+ 'checks': checks.check_yolo,
+ 'version': lambda: LOGGER.info(__version__),
+ 'settings': lambda: handle_yolo_settings(args[1:]),
+ 'cfg': lambda: yaml_print(DEFAULT_CFG_PATH),
+ 'hub': lambda: handle_yolo_hub(args[1:]),
+ 'login': lambda: handle_yolo_hub(args),
+ 'copy-cfg': copy_default_cfg}
+ full_args_dict = {**DEFAULT_CFG_DICT, **{k: None for k in TASKS}, **{k: None for k in MODES}, **special}
+
+ # Define common mis-uses of special commands, i.e. -h, -help, --help
+ special.update({k[0]: v for k, v in special.items()}) # singular
+ special.update({k[:-1]: v for k, v in special.items() if len(k) > 1 and k.endswith('s')}) # singular
+ special = {**special, **{f'-{k}': v for k, v in special.items()}, **{f'--{k}': v for k, v in special.items()}}
+
+ overrides = {} # basic overrides, i.e. imgsz=320
+ for a in merge_equals_args(args): # merge spaces around '=' sign
+ if a.startswith('--'):
+ LOGGER.warning(f"WARNING ⚠️ '{a}' does not require leading dashes '--', updating to '{a[2:]}'.")
+ a = a[2:]
+ if a.endswith(','):
+ LOGGER.warning(f"WARNING ⚠️ '{a}' does not require trailing comma ',', updating to '{a[:-1]}'.")
+ a = a[:-1]
+ if '=' in a:
+ try:
+ re.sub(r' *= *', '=', a) # remove spaces around equals sign
+ k, v = a.split('=', 1) # split on first '=' sign
+ assert v, f"missing '{k}' value"
+ if k == 'cfg': # custom.yaml passed
+ LOGGER.info(f'Overriding {DEFAULT_CFG_PATH} with {v}')
+ overrides = {k: val for k, val in yaml_load(checks.check_yaml(v)).items() if k != 'cfg'}
+ else:
+ if v.lower() == 'none':
+ v = None
+ elif v.lower() == 'true':
+ v = True
+ elif v.lower() == 'false':
+ v = False
+ else:
+ with contextlib.suppress(Exception):
+ v = eval(v)
+ overrides[k] = v
+ except (NameError, SyntaxError, ValueError, AssertionError) as e:
+ check_cfg_mismatch(full_args_dict, {a: ''}, e)
+
+ elif a in TASKS:
+ overrides['task'] = a
+ elif a in MODES:
+ overrides['mode'] = a
+ elif a.lower() in special:
+ special[a.lower()]()
+ return
+ elif a in DEFAULT_CFG_DICT and isinstance(DEFAULT_CFG_DICT[a], bool):
+ overrides[a] = True # auto-True for default bool args, i.e. 'yolo show' sets show=True
+ elif a in DEFAULT_CFG_DICT:
+ raise SyntaxError(f"'{colorstr('red', 'bold', a)}' is a valid YOLO argument but is missing an '=' sign "
+ f"to set its value, i.e. try '{a}={DEFAULT_CFG_DICT[a]}'\n{CLI_HELP_MSG}")
+ else:
+ check_cfg_mismatch(full_args_dict, {a: ''})
+
+ # Check keys
+ check_cfg_mismatch(full_args_dict, overrides)
+
+ # Mode
+ mode = overrides.get('mode', None)
+ if mode is None:
+ mode = DEFAULT_CFG.mode or 'predict'
+ LOGGER.warning(f"WARNING ⚠️ 'mode' is missing. Valid modes are {MODES}. Using default 'mode={mode}'.")
+ elif mode not in MODES:
+ if mode not in ('checks', checks):
+ raise ValueError(f"Invalid 'mode={mode}'. Valid modes are {MODES}.\n{CLI_HELP_MSG}")
+ LOGGER.warning("WARNING ⚠️ 'yolo mode=checks' is deprecated. Use 'yolo checks' instead.")
+ checks.check_yolo()
+ return
+
+ # Task
+ task = overrides.pop('task', None)
+ if task:
+ if task not in TASKS:
+ raise ValueError(f"Invalid 'task={task}'. Valid tasks are {TASKS}.\n{CLI_HELP_MSG}")
+ if 'model' not in overrides:
+ overrides['model'] = TASK2MODEL[task]
+
+ # Model
+ model = overrides.pop('model', DEFAULT_CFG.model)
+ if model is None:
+ model = 'yolov8n.pt'
+ LOGGER.warning(f"WARNING ⚠️ 'model' is missing. Using default 'model={model}'.")
+ overrides['model'] = model
+ if 'rtdetr' in model.lower(): # guess architecture
+ from ultralytics import RTDETR
+ model = RTDETR(model) # no task argument
+ elif 'sam' in model.lower():
+ from ultralytics import SAM
+ model = SAM(model)
+ else:
+ from ultralytics import YOLO
+ model = YOLO(model, task=task)
+ if isinstance(overrides.get('pretrained'), str):
+ model.load(overrides['pretrained'])
+
+ # Task Update
+ if task != model.task:
+ if task:
+ LOGGER.warning(f"WARNING ⚠️ conflicting 'task={task}' passed with 'task={model.task}' model. "
+ f"Ignoring 'task={task}' and updating to 'task={model.task}' to match model.")
+ task = model.task
+
+ # Mode
+ if mode in ('predict', 'track') and 'source' not in overrides:
+ overrides['source'] = DEFAULT_CFG.source or ROOT / 'assets' if (ROOT / 'assets').exists() \
+ else 'https://ultralytics.com/images/bus.jpg'
+ LOGGER.warning(f"WARNING ⚠️ 'source' is missing. Using default 'source={overrides['source']}'.")
+ elif mode in ('train', 'val'):
+ if 'data' not in overrides:
+ overrides['data'] = TASK2DATA.get(task or DEFAULT_CFG.task, DEFAULT_CFG.data)
+ LOGGER.warning(f"WARNING ⚠️ 'data' is missing. Using default 'data={overrides['data']}'.")
+ elif mode == 'export':
+ if 'format' not in overrides:
+ overrides['format'] = DEFAULT_CFG.format or 'torchscript'
+ LOGGER.warning(f"WARNING ⚠️ 'format' is missing. Using default 'format={overrides['format']}'.")
+
+ # Run command in python
+ # getattr(model, mode)(**vars(get_cfg(overrides=overrides))) # default args using default.yaml
+ getattr(model, mode)(**overrides) # default args from model
+
+
+# Special modes --------------------------------------------------------------------------------------------------------
+def copy_default_cfg():
+ """Copy and create a new default configuration file with '_copy' appended to its name."""
+ new_file = Path.cwd() / DEFAULT_CFG_PATH.name.replace('.yaml', '_copy.yaml')
+ shutil.copy2(DEFAULT_CFG_PATH, new_file)
+ LOGGER.info(f'{DEFAULT_CFG_PATH} copied to {new_file}\n'
+ f"Example YOLO command with this new custom cfg:\n yolo cfg='{new_file}' imgsz=320 batch=8")
+
+
+if __name__ == '__main__':
+ # Example Usage: entrypoint(debug='yolo predict model=yolov8n.pt')
+ entrypoint(debug='')
diff --git a/yolov8/ultralytics/yolo/cfg/default.yaml b/yolov8/ultralytics/yolo/cfg/default.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..25e4001aeaea233bf8cd6b1b21c74d4263c4c503
--- /dev/null
+++ b/yolov8/ultralytics/yolo/cfg/default.yaml
@@ -0,0 +1,117 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# Default training settings and hyperparameters for medium-augmentation COCO training
+
+task: detect # (str) YOLO task, i.e. detect, segment, classify, pose
+mode: train # (str) YOLO mode, i.e. train, val, predict, export, track, benchmark
+
+# Train settings -------------------------------------------------------------------------------------------------------
+model: # (str, optional) path to model file, i.e. yolov8n.pt, yolov8n.yaml
+data: # (str, optional) path to data file, i.e. coco128.yaml
+epochs: 100 # (int) number of epochs to train for
+patience: 50 # (int) epochs to wait for no observable improvement for early stopping of training
+batch: 16 # (int) number of images per batch (-1 for AutoBatch)
+imgsz: 640 # (int | list) input images size as int for train and val modes, or list[w,h] for predict and export modes
+save: True # (bool) save train checkpoints and predict results
+save_period: -1 # (int) Save checkpoint every x epochs (disabled if < 1)
+cache: False # (bool) True/ram, disk or False. Use cache for data loading
+device: # (int | str | list, optional) device to run on, i.e. cuda device=0 or device=0,1,2,3 or device=cpu
+workers: 8 # (int) number of worker threads for data loading (per RANK if DDP)
+project: # (str, optional) project name
+name: # (str, optional) experiment name, results saved to 'project/name' directory
+exist_ok: False # (bool) whether to overwrite existing experiment
+pretrained: True # (bool | str) whether to use a pretrained model (bool) or a model to load weights from (str)
+optimizer: auto # (str) optimizer to use, choices=[SGD, Adam, Adamax, AdamW, NAdam, RAdam, RMSProp, auto]
+verbose: True # (bool) whether to print verbose output
+seed: 0 # (int) random seed for reproducibility
+deterministic: True # (bool) whether to enable deterministic mode
+single_cls: False # (bool) train multi-class data as single-class
+rect: False # (bool) rectangular training if mode='train' or rectangular validation if mode='val'
+cos_lr: False # (bool) use cosine learning rate scheduler
+close_mosaic: 10 # (int) disable mosaic augmentation for final epochs
+resume: False # (bool) resume training from last checkpoint
+amp: True # (bool) Automatic Mixed Precision (AMP) training, choices=[True, False], True runs AMP check
+fraction: 1.0 # (float) dataset fraction to train on (default is 1.0, all images in train set)
+profile: False # (bool) profile ONNX and TensorRT speeds during training for loggers
+# Segmentation
+overlap_mask: True # (bool) masks should overlap during training (segment train only)
+mask_ratio: 4 # (int) mask downsample ratio (segment train only)
+# Classification
+dropout: 0.0 # (float) use dropout regularization (classify train only)
+
+# Val/Test settings ----------------------------------------------------------------------------------------------------
+val: True # (bool) validate/test during training
+split: val # (str) dataset split to use for validation, i.e. 'val', 'test' or 'train'
+save_json: False # (bool) save results to JSON file
+save_hybrid: False # (bool) save hybrid version of labels (labels + additional predictions)
+conf: # (float, optional) object confidence threshold for detection (default 0.25 predict, 0.001 val)
+iou: 0.7 # (float) intersection over union (IoU) threshold for NMS
+max_det: 300 # (int) maximum number of detections per image
+half: False # (bool) use half precision (FP16)
+dnn: False # (bool) use OpenCV DNN for ONNX inference
+plots: True # (bool) save plots during train/val
+
+# Prediction settings --------------------------------------------------------------------------------------------------
+source: # (str, optional) source directory for images or videos
+show: False # (bool) show results if possible
+save_txt: False # (bool) save results as .txt file
+save_conf: False # (bool) save results with confidence scores
+save_crop: False # (bool) save cropped images with results
+show_labels: True # (bool) show object labels in plots
+show_conf: True # (bool) show object confidence scores in plots
+vid_stride: 1 # (int) video frame-rate stride
+line_width: # (int, optional) line width of the bounding boxes, auto if missing
+visualize: False # (bool) visualize model features
+augment: False # (bool) apply image augmentation to prediction sources
+agnostic_nms: False # (bool) class-agnostic NMS
+classes: # (int | list[int], optional) filter results by class, i.e. class=0, or class=[0,2,3]
+retina_masks: False # (bool) use high-resolution segmentation masks
+boxes: True # (bool) Show boxes in segmentation predictions
+
+# Export settings ------------------------------------------------------------------------------------------------------
+format: torchscript # (str) format to export to, choices at https://docs.ultralytics.com/modes/export/#export-formats
+keras: False # (bool) use Kera=s
+optimize: False # (bool) TorchScript: optimize for mobile
+int8: False # (bool) CoreML/TF INT8 quantization
+dynamic: False # (bool) ONNX/TF/TensorRT: dynamic axes
+simplify: False # (bool) ONNX: simplify model
+opset: # (int, optional) ONNX: opset version
+workspace: 4 # (int) TensorRT: workspace size (GB)
+nms: False # (bool) CoreML: add NMS
+
+# Hyperparameters ------------------------------------------------------------------------------------------------------
+lr0: 0.01 # (float) initial learning rate (i.e. SGD=1E-2, Adam=1E-3)
+lrf: 0.01 # (float) final learning rate (lr0 * lrf)
+momentum: 0.937 # (float) SGD momentum/Adam beta1
+weight_decay: 0.0005 # (float) optimizer weight decay 5e-4
+warmup_epochs: 3.0 # (float) warmup epochs (fractions ok)
+warmup_momentum: 0.8 # (float) warmup initial momentum
+warmup_bias_lr: 0.1 # (float) warmup initial bias lr
+box: 7.5 # (float) box loss gain
+cls: 0.5 # (float) cls loss gain (scale with pixels)
+dfl: 1.5 # (float) dfl loss gain
+pose: 12.0 # (float) pose loss gain
+kobj: 1.0 # (float) keypoint obj loss gain
+label_smoothing: 0.0 # (float) label smoothing (fraction)
+nbs: 64 # (int) nominal batch size
+hsv_h: 0.015 # (float) image HSV-Hue augmentation (fraction)
+hsv_s: 0.7 # (float) image HSV-Saturation augmentation (fraction)
+hsv_v: 0.4 # (float) image HSV-Value augmentation (fraction)
+degrees: 0.0 # (float) image rotation (+/- deg)
+translate: 0.1 # (float) image translation (+/- fraction)
+scale: 0.5 # (float) image scale (+/- gain)
+shear: 0.0 # (float) image shear (+/- deg)
+perspective: 0.0 # (float) image perspective (+/- fraction), range 0-0.001
+flipud: 0.0 # (float) image flip up-down (probability)
+fliplr: 0.5 # (float) image flip left-right (probability)
+mosaic: 1.0 # (float) image mosaic (probability)
+mixup: 0.0 # (float) image mixup (probability)
+copy_paste: 0.0 # (float) segment copy-paste (probability)
+
+# Custom config.yaml ---------------------------------------------------------------------------------------------------
+cfg: # (str, optional) for overriding defaults.yaml
+
+# Debug, do not modify -------------------------------------------------------------------------------------------------
+v5loader: False # (bool) use legacy YOLOv5 dataloader (deprecated)
+
+# Tracker settings ------------------------------------------------------------------------------------------------------
+tracker: botsort.yaml # (str) tracker type, choices=[botsort.yaml, bytetrack.yaml]
diff --git a/yolov8/ultralytics/yolo/data/__init__.py b/yolov8/ultralytics/yolo/data/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..f1d9deeed93b8cfbf167930016ef0702208c6a0b
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/__init__.py
@@ -0,0 +1,9 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .base import BaseDataset
+from .build import build_dataloader, build_yolo_dataset, load_inference_source
+from .dataset import ClassificationDataset, SemanticDataset, YOLODataset
+from .dataset_wrappers import MixAndRectDataset
+
+__all__ = ('BaseDataset', 'ClassificationDataset', 'MixAndRectDataset', 'SemanticDataset', 'YOLODataset',
+ 'build_yolo_dataset', 'build_dataloader', 'load_inference_source')
diff --git a/yolov8/ultralytics/yolo/data/annotator.py b/yolov8/ultralytics/yolo/data/annotator.py
new file mode 100644
index 0000000000000000000000000000000000000000..e841df631aeef6b3c25b62f5f8e0052e42df32bf
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/annotator.py
@@ -0,0 +1,53 @@
+from pathlib import Path
+
+from ultralytics import YOLO
+from ultralytics.vit.sam import PromptPredictor, build_sam
+from ultralytics.yolo.utils.torch_utils import select_device
+
+
+def auto_annotate(data, det_model='yolov8x.pt', sam_model='sam_b.pt', device='', output_dir=None):
+ """
+ Automatically annotates images using a YOLO object detection model and a SAM segmentation model.
+ Args:
+ data (str): Path to a folder containing images to be annotated.
+ det_model (str, optional): Pre-trained YOLO detection model. Defaults to 'yolov8x.pt'.
+ sam_model (str, optional): Pre-trained SAM segmentation model. Defaults to 'sam_b.pt'.
+ device (str, optional): Device to run the models on. Defaults to an empty string (CPU or GPU, if available).
+ output_dir (str | None | optional): Directory to save the annotated results.
+ Defaults to a 'labels' folder in the same directory as 'data'.
+ """
+ device = select_device(device)
+ det_model = YOLO(det_model)
+ sam_model = build_sam(sam_model)
+ det_model.to(device)
+ sam_model.to(device)
+
+ if not output_dir:
+ output_dir = Path(str(data)).parent / 'labels'
+ Path(output_dir).mkdir(exist_ok=True, parents=True)
+
+ prompt_predictor = PromptPredictor(sam_model)
+ det_results = det_model(data, stream=True)
+
+ for result in det_results:
+ boxes = result.boxes.xyxy # Boxes object for bbox outputs
+ class_ids = result.boxes.cls.int().tolist() # noqa
+ if len(class_ids):
+ prompt_predictor.set_image(result.orig_img)
+ masks, _, _ = prompt_predictor.predict_torch(
+ point_coords=None,
+ point_labels=None,
+ boxes=prompt_predictor.transform.apply_boxes_torch(boxes, result.orig_shape[:2]),
+ multimask_output=False,
+ )
+
+ result.update(masks=masks.squeeze(1))
+ segments = result.masks.xyn # noqa
+
+ with open(str(Path(output_dir) / Path(result.path).stem) + '.txt', 'w') as f:
+ for i in range(len(segments)):
+ s = segments[i]
+ if len(s) == 0:
+ continue
+ segment = map(str, segments[i].reshape(-1).tolist())
+ f.write(f'{class_ids[i]} ' + ' '.join(segment) + '\n')
diff --git a/yolov8/ultralytics/yolo/data/augment.py b/yolov8/ultralytics/yolo/data/augment.py
new file mode 100644
index 0000000000000000000000000000000000000000..f3c44834c10a828cb796cb7675a4dab6b8c6c854
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/augment.py
@@ -0,0 +1,899 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import math
+import random
+from copy import deepcopy
+
+import cv2
+import numpy as np
+import torch
+import torchvision.transforms as T
+
+from ..utils import LOGGER, colorstr
+from ..utils.checks import check_version
+from ..utils.instance import Instances
+from ..utils.metrics import bbox_ioa
+from ..utils.ops import segment2box
+from .utils import polygons2masks, polygons2masks_overlap
+
+POSE_FLIPLR_INDEX = [0, 2, 1, 4, 3, 6, 5, 8, 7, 10, 9, 12, 11, 14, 13, 16, 15]
+
+
+# TODO: we might need a BaseTransform to make all these augments be compatible with both classification and semantic
+class BaseTransform:
+
+ def __init__(self) -> None:
+ pass
+
+ def apply_image(self, labels):
+ """Applies image transformation to labels."""
+ pass
+
+ def apply_instances(self, labels):
+ """Applies transformations to input 'labels' and returns object instances."""
+ pass
+
+ def apply_semantic(self, labels):
+ """Applies semantic segmentation to an image."""
+ pass
+
+ def __call__(self, labels):
+ """Applies label transformations to an image, instances and semantic masks."""
+ self.apply_image(labels)
+ self.apply_instances(labels)
+ self.apply_semantic(labels)
+
+
+class Compose:
+
+ def __init__(self, transforms):
+ """Initializes the Compose object with a list of transforms."""
+ self.transforms = transforms
+
+ def __call__(self, data):
+ """Applies a series of transformations to input data."""
+ for t in self.transforms:
+ data = t(data)
+ return data
+
+ def append(self, transform):
+ """Appends a new transform to the existing list of transforms."""
+ self.transforms.append(transform)
+
+ def tolist(self):
+ """Converts list of transforms to a standard Python list."""
+ return self.transforms
+
+ def __repr__(self):
+ """Return string representation of object."""
+ format_string = f'{self.__class__.__name__}('
+ for t in self.transforms:
+ format_string += '\n'
+ format_string += f' {t}'
+ format_string += '\n)'
+ return format_string
+
+
+class BaseMixTransform:
+ """This implementation is from mmyolo."""
+
+ def __init__(self, dataset, pre_transform=None, p=0.0) -> None:
+ self.dataset = dataset
+ self.pre_transform = pre_transform
+ self.p = p
+
+ def __call__(self, labels):
+ """Applies pre-processing transforms and mixup/mosaic transforms to labels data."""
+ if random.uniform(0, 1) > self.p:
+ return labels
+
+ # Get index of one or three other images
+ indexes = self.get_indexes()
+ if isinstance(indexes, int):
+ indexes = [indexes]
+
+ # Get images information will be used for Mosaic or MixUp
+ mix_labels = [self.dataset.get_image_and_label(i) for i in indexes]
+
+ if self.pre_transform is not None:
+ for i, data in enumerate(mix_labels):
+ mix_labels[i] = self.pre_transform(data)
+ labels['mix_labels'] = mix_labels
+
+ # Mosaic or MixUp
+ labels = self._mix_transform(labels)
+ labels.pop('mix_labels', None)
+ return labels
+
+ def _mix_transform(self, labels):
+ """Applies MixUp or Mosaic augmentation to the label dictionary."""
+ raise NotImplementedError
+
+ def get_indexes(self):
+ """Gets a list of shuffled indexes for mosaic augmentation."""
+ raise NotImplementedError
+
+
+class Mosaic(BaseMixTransform):
+ """
+ Mosaic augmentation.
+
+ This class performs mosaic augmentation by combining multiple (4 or 9) images into a single mosaic image.
+ The augmentation is applied to a dataset with a given probability.
+
+ Attributes:
+ dataset: The dataset on which the mosaic augmentation is applied.
+ imgsz (int, optional): Image size (height and width) after mosaic pipeline of a single image. Default to 640.
+ p (float, optional): Probability of applying the mosaic augmentation. Must be in the range 0-1. Default to 1.0.
+ n (int, optional): The grid size, either 4 (for 2x2) or 9 (for 3x3).
+ """
+
+ def __init__(self, dataset, imgsz=640, p=1.0, n=4):
+ """Initializes the object with a dataset, image size, probability, and border."""
+ assert 0 <= p <= 1.0, f'The probability should be in range [0, 1], but got {p}.'
+ assert n in (4, 9), 'grid must be equal to 4 or 9.'
+ super().__init__(dataset=dataset, p=p)
+ self.dataset = dataset
+ self.imgsz = imgsz
+ self.border = (-imgsz // 2, -imgsz // 2) # width, height
+ self.n = n
+
+ def get_indexes(self, buffer=True):
+ """Return a list of random indexes from the dataset."""
+ if buffer: # select images from buffer
+ return random.choices(list(self.dataset.buffer), k=self.n - 1)
+ else: # select any images
+ return [random.randint(0, len(self.dataset) - 1) for _ in range(self.n - 1)]
+
+ def _mix_transform(self, labels):
+ """Apply mixup transformation to the input image and labels."""
+ assert labels.get('rect_shape', None) is None, 'rect and mosaic are mutually exclusive.'
+ assert len(labels.get('mix_labels', [])), 'There are no other images for mosaic augment.'
+ return self._mosaic4(labels) if self.n == 4 else self._mosaic9(labels)
+
+ def _mosaic4(self, labels):
+ """Create a 2x2 image mosaic."""
+ mosaic_labels = []
+ s = self.imgsz
+ yc, xc = (int(random.uniform(-x, 2 * s + x)) for x in self.border) # mosaic center x, y
+ for i in range(4):
+ labels_patch = labels if i == 0 else labels['mix_labels'][i - 1]
+ # Load image
+ img = labels_patch['img']
+ h, w = labels_patch.pop('resized_shape')
+
+ # Place img in img4
+ if i == 0: # top left
+ img4 = np.full((s * 2, s * 2, img.shape[2]), 114, dtype=np.uint8) # base image with 4 tiles
+ x1a, y1a, x2a, y2a = max(xc - w, 0), max(yc - h, 0), xc, yc # xmin, ymin, xmax, ymax (large image)
+ x1b, y1b, x2b, y2b = w - (x2a - x1a), h - (y2a - y1a), w, h # xmin, ymin, xmax, ymax (small image)
+ elif i == 1: # top right
+ x1a, y1a, x2a, y2a = xc, max(yc - h, 0), min(xc + w, s * 2), yc
+ x1b, y1b, x2b, y2b = 0, h - (y2a - y1a), min(w, x2a - x1a), h
+ elif i == 2: # bottom left
+ x1a, y1a, x2a, y2a = max(xc - w, 0), yc, xc, min(s * 2, yc + h)
+ x1b, y1b, x2b, y2b = w - (x2a - x1a), 0, w, min(y2a - y1a, h)
+ elif i == 3: # bottom right
+ x1a, y1a, x2a, y2a = xc, yc, min(xc + w, s * 2), min(s * 2, yc + h)
+ x1b, y1b, x2b, y2b = 0, 0, min(w, x2a - x1a), min(y2a - y1a, h)
+
+ img4[y1a:y2a, x1a:x2a] = img[y1b:y2b, x1b:x2b] # img4[ymin:ymax, xmin:xmax]
+ padw = x1a - x1b
+ padh = y1a - y1b
+
+ labels_patch = self._update_labels(labels_patch, padw, padh)
+ mosaic_labels.append(labels_patch)
+ final_labels = self._cat_labels(mosaic_labels)
+ final_labels['img'] = img4
+ return final_labels
+
+ def _mosaic9(self, labels):
+ """Create a 3x3 image mosaic."""
+ mosaic_labels = []
+ s = self.imgsz
+ hp, wp = -1, -1 # height, width previous
+ for i in range(9):
+ labels_patch = labels if i == 0 else labels['mix_labels'][i - 1]
+ # Load image
+ img = labels_patch['img']
+ h, w = labels_patch.pop('resized_shape')
+
+ # Place img in img9
+ if i == 0: # center
+ img9 = np.full((s * 3, s * 3, img.shape[2]), 114, dtype=np.uint8) # base image with 4 tiles
+ h0, w0 = h, w
+ c = s, s, s + w, s + h # xmin, ymin, xmax, ymax (base) coordinates
+ elif i == 1: # top
+ c = s, s - h, s + w, s
+ elif i == 2: # top right
+ c = s + wp, s - h, s + wp + w, s
+ elif i == 3: # right
+ c = s + w0, s, s + w0 + w, s + h
+ elif i == 4: # bottom right
+ c = s + w0, s + hp, s + w0 + w, s + hp + h
+ elif i == 5: # bottom
+ c = s + w0 - w, s + h0, s + w0, s + h0 + h
+ elif i == 6: # bottom left
+ c = s + w0 - wp - w, s + h0, s + w0 - wp, s + h0 + h
+ elif i == 7: # left
+ c = s - w, s + h0 - h, s, s + h0
+ elif i == 8: # top left
+ c = s - w, s + h0 - hp - h, s, s + h0 - hp
+
+ padw, padh = c[:2]
+ x1, y1, x2, y2 = (max(x, 0) for x in c) # allocate coords
+
+ # Image
+ img9[y1:y2, x1:x2] = img[y1 - padh:, x1 - padw:] # img9[ymin:ymax, xmin:xmax]
+ hp, wp = h, w # height, width previous for next iteration
+
+ # Labels assuming imgsz*2 mosaic size
+ labels_patch = self._update_labels(labels_patch, padw + self.border[0], padh + self.border[1])
+ mosaic_labels.append(labels_patch)
+ final_labels = self._cat_labels(mosaic_labels)
+
+ final_labels['img'] = img9[-self.border[0]:self.border[0], -self.border[1]:self.border[1]]
+ return final_labels
+
+ @staticmethod
+ def _update_labels(labels, padw, padh):
+ """Update labels."""
+ nh, nw = labels['img'].shape[:2]
+ labels['instances'].convert_bbox(format='xyxy')
+ labels['instances'].denormalize(nw, nh)
+ labels['instances'].add_padding(padw, padh)
+ return labels
+
+ def _cat_labels(self, mosaic_labels):
+ """Return labels with mosaic border instances clipped."""
+ if len(mosaic_labels) == 0:
+ return {}
+ cls = []
+ instances = []
+ imgsz = self.imgsz * 2 # mosaic imgsz
+ for labels in mosaic_labels:
+ cls.append(labels['cls'])
+ instances.append(labels['instances'])
+ final_labels = {
+ 'im_file': mosaic_labels[0]['im_file'],
+ 'ori_shape': mosaic_labels[0]['ori_shape'],
+ 'resized_shape': (imgsz, imgsz),
+ 'cls': np.concatenate(cls, 0),
+ 'instances': Instances.concatenate(instances, axis=0),
+ 'mosaic_border': self.border} # final_labels
+ final_labels['instances'].clip(imgsz, imgsz)
+ good = final_labels['instances'].remove_zero_area_boxes()
+ final_labels['cls'] = final_labels['cls'][good]
+ return final_labels
+
+
+class MixUp(BaseMixTransform):
+
+ def __init__(self, dataset, pre_transform=None, p=0.0) -> None:
+ super().__init__(dataset=dataset, pre_transform=pre_transform, p=p)
+
+ def get_indexes(self):
+ """Get a random index from the dataset."""
+ return random.randint(0, len(self.dataset) - 1)
+
+ def _mix_transform(self, labels):
+ """Applies MixUp augmentation https://arxiv.org/pdf/1710.09412.pdf."""
+ r = np.random.beta(32.0, 32.0) # mixup ratio, alpha=beta=32.0
+ labels2 = labels['mix_labels'][0]
+ labels['img'] = (labels['img'] * r + labels2['img'] * (1 - r)).astype(np.uint8)
+ labels['instances'] = Instances.concatenate([labels['instances'], labels2['instances']], axis=0)
+ labels['cls'] = np.concatenate([labels['cls'], labels2['cls']], 0)
+ return labels
+
+
+class RandomPerspective:
+
+ def __init__(self,
+ degrees=0.0,
+ translate=0.1,
+ scale=0.5,
+ shear=0.0,
+ perspective=0.0,
+ border=(0, 0),
+ pre_transform=None):
+ self.degrees = degrees
+ self.translate = translate
+ self.scale = scale
+ self.shear = shear
+ self.perspective = perspective
+ # Mosaic border
+ self.border = border
+ self.pre_transform = pre_transform
+
+ def affine_transform(self, img, border):
+ """Center."""
+ C = np.eye(3, dtype=np.float32)
+
+ C[0, 2] = -img.shape[1] / 2 # x translation (pixels)
+ C[1, 2] = -img.shape[0] / 2 # y translation (pixels)
+
+ # Perspective
+ P = np.eye(3, dtype=np.float32)
+ P[2, 0] = random.uniform(-self.perspective, self.perspective) # x perspective (about y)
+ P[2, 1] = random.uniform(-self.perspective, self.perspective) # y perspective (about x)
+
+ # Rotation and Scale
+ R = np.eye(3, dtype=np.float32)
+ a = random.uniform(-self.degrees, self.degrees)
+ # a += random.choice([-180, -90, 0, 90]) # add 90deg rotations to small rotations
+ s = random.uniform(1 - self.scale, 1 + self.scale)
+ # s = 2 ** random.uniform(-scale, scale)
+ R[:2] = cv2.getRotationMatrix2D(angle=a, center=(0, 0), scale=s)
+
+ # Shear
+ S = np.eye(3, dtype=np.float32)
+ S[0, 1] = math.tan(random.uniform(-self.shear, self.shear) * math.pi / 180) # x shear (deg)
+ S[1, 0] = math.tan(random.uniform(-self.shear, self.shear) * math.pi / 180) # y shear (deg)
+
+ # Translation
+ T = np.eye(3, dtype=np.float32)
+ T[0, 2] = random.uniform(0.5 - self.translate, 0.5 + self.translate) * self.size[0] # x translation (pixels)
+ T[1, 2] = random.uniform(0.5 - self.translate, 0.5 + self.translate) * self.size[1] # y translation (pixels)
+
+ # Combined rotation matrix
+ M = T @ S @ R @ P @ C # order of operations (right to left) is IMPORTANT
+ # Affine image
+ if (border[0] != 0) or (border[1] != 0) or (M != np.eye(3)).any(): # image changed
+ if self.perspective:
+ img = cv2.warpPerspective(img, M, dsize=self.size, borderValue=(114, 114, 114))
+ else: # affine
+ img = cv2.warpAffine(img, M[:2], dsize=self.size, borderValue=(114, 114, 114))
+ return img, M, s
+
+ def apply_bboxes(self, bboxes, M):
+ """
+ Apply affine to bboxes only.
+
+ Args:
+ bboxes (ndarray): list of bboxes, xyxy format, with shape (num_bboxes, 4).
+ M (ndarray): affine matrix.
+
+ Returns:
+ new_bboxes (ndarray): bboxes after affine, [num_bboxes, 4].
+ """
+ n = len(bboxes)
+ if n == 0:
+ return bboxes
+
+ xy = np.ones((n * 4, 3), dtype=bboxes.dtype)
+ xy[:, :2] = bboxes[:, [0, 1, 2, 3, 0, 3, 2, 1]].reshape(n * 4, 2) # x1y1, x2y2, x1y2, x2y1
+ xy = xy @ M.T # transform
+ xy = (xy[:, :2] / xy[:, 2:3] if self.perspective else xy[:, :2]).reshape(n, 8) # perspective rescale or affine
+
+ # Create new boxes
+ x = xy[:, [0, 2, 4, 6]]
+ y = xy[:, [1, 3, 5, 7]]
+ return np.concatenate((x.min(1), y.min(1), x.max(1), y.max(1)), dtype=bboxes.dtype).reshape(4, n).T
+
+ def apply_segments(self, segments, M):
+ """
+ Apply affine to segments and generate new bboxes from segments.
+
+ Args:
+ segments (ndarray): list of segments, [num_samples, 500, 2].
+ M (ndarray): affine matrix.
+
+ Returns:
+ new_segments (ndarray): list of segments after affine, [num_samples, 500, 2].
+ new_bboxes (ndarray): bboxes after affine, [N, 4].
+ """
+ n, num = segments.shape[:2]
+ if n == 0:
+ return [], segments
+
+ xy = np.ones((n * num, 3), dtype=segments.dtype)
+ segments = segments.reshape(-1, 2)
+ xy[:, :2] = segments
+ xy = xy @ M.T # transform
+ xy = xy[:, :2] / xy[:, 2:3]
+ segments = xy.reshape(n, -1, 2)
+ bboxes = np.stack([segment2box(xy, self.size[0], self.size[1]) for xy in segments], 0)
+ return bboxes, segments
+
+ def apply_keypoints(self, keypoints, M):
+ """
+ Apply affine to keypoints.
+
+ Args:
+ keypoints (ndarray): keypoints, [N, 17, 3].
+ M (ndarray): affine matrix.
+
+ Return:
+ new_keypoints (ndarray): keypoints after affine, [N, 17, 3].
+ """
+ n, nkpt = keypoints.shape[:2]
+ if n == 0:
+ return keypoints
+ xy = np.ones((n * nkpt, 3), dtype=keypoints.dtype)
+ visible = keypoints[..., 2].reshape(n * nkpt, 1)
+ xy[:, :2] = keypoints[..., :2].reshape(n * nkpt, 2)
+ xy = xy @ M.T # transform
+ xy = xy[:, :2] / xy[:, 2:3] # perspective rescale or affine
+ out_mask = (xy[:, 0] < 0) | (xy[:, 1] < 0) | (xy[:, 0] > self.size[0]) | (xy[:, 1] > self.size[1])
+ visible[out_mask] = 0
+ return np.concatenate([xy, visible], axis=-1).reshape(n, nkpt, 3)
+
+ def __call__(self, labels):
+ """
+ Affine images and targets.
+
+ Args:
+ labels (dict): a dict of `bboxes`, `segments`, `keypoints`.
+ """
+ if self.pre_transform and 'mosaic_border' not in labels:
+ labels = self.pre_transform(labels)
+ labels.pop('ratio_pad', None) # do not need ratio pad
+
+ img = labels['img']
+ cls = labels['cls']
+ instances = labels.pop('instances')
+ # Make sure the coord formats are right
+ instances.convert_bbox(format='xyxy')
+ instances.denormalize(*img.shape[:2][::-1])
+
+ border = labels.pop('mosaic_border', self.border)
+ self.size = img.shape[1] + border[1] * 2, img.shape[0] + border[0] * 2 # w, h
+ # M is affine matrix
+ # scale for func:`box_candidates`
+ img, M, scale = self.affine_transform(img, border)
+
+ bboxes = self.apply_bboxes(instances.bboxes, M)
+
+ segments = instances.segments
+ keypoints = instances.keypoints
+ # Update bboxes if there are segments.
+ if len(segments):
+ bboxes, segments = self.apply_segments(segments, M)
+
+ if keypoints is not None:
+ keypoints = self.apply_keypoints(keypoints, M)
+ new_instances = Instances(bboxes, segments, keypoints, bbox_format='xyxy', normalized=False)
+ # Clip
+ new_instances.clip(*self.size)
+
+ # Filter instances
+ instances.scale(scale_w=scale, scale_h=scale, bbox_only=True)
+ # Make the bboxes have the same scale with new_bboxes
+ i = self.box_candidates(box1=instances.bboxes.T,
+ box2=new_instances.bboxes.T,
+ area_thr=0.01 if len(segments) else 0.10)
+ labels['instances'] = new_instances[i]
+ labels['cls'] = cls[i]
+ labels['img'] = img
+ labels['resized_shape'] = img.shape[:2]
+ return labels
+
+ def box_candidates(self, box1, box2, wh_thr=2, ar_thr=100, area_thr=0.1, eps=1e-16): # box1(4,n), box2(4,n)
+ # Compute box candidates: box1 before augment, box2 after augment, wh_thr (pixels), aspect_ratio_thr, area_ratio
+ w1, h1 = box1[2] - box1[0], box1[3] - box1[1]
+ w2, h2 = box2[2] - box2[0], box2[3] - box2[1]
+ ar = np.maximum(w2 / (h2 + eps), h2 / (w2 + eps)) # aspect ratio
+ return (w2 > wh_thr) & (h2 > wh_thr) & (w2 * h2 / (w1 * h1 + eps) > area_thr) & (ar < ar_thr) # candidates
+
+
+class RandomHSV:
+
+ def __init__(self, hgain=0.5, sgain=0.5, vgain=0.5) -> None:
+ self.hgain = hgain
+ self.sgain = sgain
+ self.vgain = vgain
+
+ def __call__(self, labels):
+ """Applies random horizontal or vertical flip to an image with a given probability."""
+ img = labels['img']
+ if self.hgain or self.sgain or self.vgain:
+ r = np.random.uniform(-1, 1, 3) * [self.hgain, self.sgain, self.vgain] + 1 # random gains
+ hue, sat, val = cv2.split(cv2.cvtColor(img, cv2.COLOR_BGR2HSV))
+ dtype = img.dtype # uint8
+
+ x = np.arange(0, 256, dtype=r.dtype)
+ lut_hue = ((x * r[0]) % 180).astype(dtype)
+ lut_sat = np.clip(x * r[1], 0, 255).astype(dtype)
+ lut_val = np.clip(x * r[2], 0, 255).astype(dtype)
+
+ im_hsv = cv2.merge((cv2.LUT(hue, lut_hue), cv2.LUT(sat, lut_sat), cv2.LUT(val, lut_val)))
+ cv2.cvtColor(im_hsv, cv2.COLOR_HSV2BGR, dst=img) # no return needed
+ return labels
+
+
+class RandomFlip:
+
+ def __init__(self, p=0.5, direction='horizontal', flip_idx=None) -> None:
+ assert direction in ['horizontal', 'vertical'], f'Support direction `horizontal` or `vertical`, got {direction}'
+ assert 0 <= p <= 1.0
+
+ self.p = p
+ self.direction = direction
+ self.flip_idx = flip_idx
+
+ def __call__(self, labels):
+ """Resize image and padding for detection, instance segmentation, pose."""
+ img = labels['img']
+ instances = labels.pop('instances')
+ instances.convert_bbox(format='xywh')
+ h, w = img.shape[:2]
+ h = 1 if instances.normalized else h
+ w = 1 if instances.normalized else w
+
+ # Flip up-down
+ if self.direction == 'vertical' and random.random() < self.p:
+ img = np.flipud(img)
+ instances.flipud(h)
+ if self.direction == 'horizontal' and random.random() < self.p:
+ img = np.fliplr(img)
+ instances.fliplr(w)
+ # For keypoints
+ if self.flip_idx is not None and instances.keypoints is not None:
+ instances.keypoints = np.ascontiguousarray(instances.keypoints[:, self.flip_idx, :])
+ labels['img'] = np.ascontiguousarray(img)
+ labels['instances'] = instances
+ return labels
+
+
+class LetterBox:
+ """Resize image and padding for detection, instance segmentation, pose."""
+
+ def __init__(self, new_shape=(640, 640), auto=False, scaleFill=False, scaleup=True, stride=32):
+ """Initialize LetterBox object with specific parameters."""
+ self.new_shape = new_shape
+ self.auto = auto
+ self.scaleFill = scaleFill
+ self.scaleup = scaleup
+ self.stride = stride
+
+ def __call__(self, labels=None, image=None):
+ """Return updated labels and image with added border."""
+ if labels is None:
+ labels = {}
+ img = labels.get('img') if image is None else image
+ shape = img.shape[:2] # current shape [height, width]
+ new_shape = labels.pop('rect_shape', self.new_shape)
+ if isinstance(new_shape, int):
+ new_shape = (new_shape, new_shape)
+
+ # Scale ratio (new / old)
+ r = min(new_shape[0] / shape[0], new_shape[1] / shape[1])
+ if not self.scaleup: # only scale down, do not scale up (for better val mAP)
+ r = min(r, 1.0)
+
+ # Compute padding
+ ratio = r, r # width, height ratios
+ new_unpad = int(round(shape[1] * r)), int(round(shape[0] * r))
+ dw, dh = new_shape[1] - new_unpad[0], new_shape[0] - new_unpad[1] # wh padding
+ if self.auto: # minimum rectangle
+ dw, dh = np.mod(dw, self.stride), np.mod(dh, self.stride) # wh padding
+ elif self.scaleFill: # stretch
+ dw, dh = 0.0, 0.0
+ new_unpad = (new_shape[1], new_shape[0])
+ ratio = new_shape[1] / shape[1], new_shape[0] / shape[0] # width, height ratios
+
+ dw /= 2 # divide padding into 2 sides
+ dh /= 2
+ if labels.get('ratio_pad'):
+ labels['ratio_pad'] = (labels['ratio_pad'], (dw, dh)) # for evaluation
+
+ if shape[::-1] != new_unpad: # resize
+ img = cv2.resize(img, new_unpad, interpolation=cv2.INTER_LINEAR)
+ top, bottom = int(round(dh - 0.1)), int(round(dh + 0.1))
+ left, right = int(round(dw - 0.1)), int(round(dw + 0.1))
+ img = cv2.copyMakeBorder(img, top, bottom, left, right, cv2.BORDER_CONSTANT,
+ value=(114, 114, 114)) # add border
+
+ if len(labels):
+ labels = self._update_labels(labels, ratio, dw, dh)
+ labels['img'] = img
+ labels['resized_shape'] = new_shape
+ return labels
+ else:
+ return img
+
+ def _update_labels(self, labels, ratio, padw, padh):
+ """Update labels."""
+ labels['instances'].convert_bbox(format='xyxy')
+ labels['instances'].denormalize(*labels['img'].shape[:2][::-1])
+ labels['instances'].scale(*ratio)
+ labels['instances'].add_padding(padw, padh)
+ return labels
+
+
+class CopyPaste:
+
+ def __init__(self, p=0.5) -> None:
+ self.p = p
+
+ def __call__(self, labels):
+ """Implement Copy-Paste augmentation https://arxiv.org/abs/2012.07177, labels as nx5 np.array(cls, xyxy)."""
+ im = labels['img']
+ cls = labels['cls']
+ h, w = im.shape[:2]
+ instances = labels.pop('instances')
+ instances.convert_bbox(format='xyxy')
+ instances.denormalize(w, h)
+ if self.p and len(instances.segments):
+ n = len(instances)
+ _, w, _ = im.shape # height, width, channels
+ im_new = np.zeros(im.shape, np.uint8)
+
+ # Calculate ioa first then select indexes randomly
+ ins_flip = deepcopy(instances)
+ ins_flip.fliplr(w)
+
+ ioa = bbox_ioa(ins_flip.bboxes, instances.bboxes) # intersection over area, (N, M)
+ indexes = np.nonzero((ioa < 0.30).all(1))[0] # (N, )
+ n = len(indexes)
+ for j in random.sample(list(indexes), k=round(self.p * n)):
+ cls = np.concatenate((cls, cls[[j]]), axis=0)
+ instances = Instances.concatenate((instances, ins_flip[[j]]), axis=0)
+ cv2.drawContours(im_new, instances.segments[[j]].astype(np.int32), -1, (1, 1, 1), cv2.FILLED)
+
+ result = cv2.flip(im, 1) # augment segments (flip left-right)
+ i = cv2.flip(im_new, 1).astype(bool)
+ im[i] = result[i] # cv2.imwrite('debug.jpg', im) # debug
+
+ labels['img'] = im
+ labels['cls'] = cls
+ labels['instances'] = instances
+ return labels
+
+
+class Albumentations:
+ # YOLOv8 Albumentations class (optional, only used if package is installed)
+ def __init__(self, p=1.0):
+ """Initialize the transform object for YOLO bbox formatted params."""
+ self.p = p
+ self.transform = None
+ prefix = colorstr('albumentations: ')
+ try:
+ import albumentations as A
+
+ check_version(A.__version__, '1.0.3', hard=True) # version requirement
+
+ T = [
+ A.Blur(p=0.01),
+ A.MedianBlur(p=0.01),
+ A.ToGray(p=0.01),
+ A.CLAHE(p=0.01),
+ A.RandomBrightnessContrast(p=0.0),
+ A.RandomGamma(p=0.0),
+ A.ImageCompression(quality_lower=75, p=0.0)] # transforms
+ self.transform = A.Compose(T, bbox_params=A.BboxParams(format='yolo', label_fields=['class_labels']))
+
+ LOGGER.info(prefix + ', '.join(f'{x}'.replace('always_apply=False, ', '') for x in T if x.p))
+ except ImportError: # package not installed, skip
+ pass
+ except Exception as e:
+ LOGGER.info(f'{prefix}{e}')
+
+ def __call__(self, labels):
+ """Generates object detections and returns a dictionary with detection results."""
+ im = labels['img']
+ cls = labels['cls']
+ if len(cls):
+ labels['instances'].convert_bbox('xywh')
+ labels['instances'].normalize(*im.shape[:2][::-1])
+ bboxes = labels['instances'].bboxes
+ # TODO: add supports of segments and keypoints
+ if self.transform and random.random() < self.p:
+ new = self.transform(image=im, bboxes=bboxes, class_labels=cls) # transformed
+ if len(new['class_labels']) > 0: # skip update if no bbox in new im
+ labels['img'] = new['image']
+ labels['cls'] = np.array(new['class_labels'])
+ bboxes = np.array(new['bboxes'], dtype=np.float32)
+ labels['instances'].update(bboxes=bboxes)
+ return labels
+
+
+# TODO: technically this is not an augmentation, maybe we should put this to another files
+class Format:
+
+ def __init__(self,
+ bbox_format='xywh',
+ normalize=True,
+ return_mask=False,
+ return_keypoint=False,
+ mask_ratio=4,
+ mask_overlap=True,
+ batch_idx=True):
+ self.bbox_format = bbox_format
+ self.normalize = normalize
+ self.return_mask = return_mask # set False when training detection only
+ self.return_keypoint = return_keypoint
+ self.mask_ratio = mask_ratio
+ self.mask_overlap = mask_overlap
+ self.batch_idx = batch_idx # keep the batch indexes
+
+ def __call__(self, labels):
+ """Return formatted image, classes, bounding boxes & keypoints to be used by 'collate_fn'."""
+ img = labels.pop('img')
+ h, w = img.shape[:2]
+ cls = labels.pop('cls')
+ instances = labels.pop('instances')
+ instances.convert_bbox(format=self.bbox_format)
+ instances.denormalize(w, h)
+ nl = len(instances)
+
+ if self.return_mask:
+ if nl:
+ masks, instances, cls = self._format_segments(instances, cls, w, h)
+ masks = torch.from_numpy(masks)
+ else:
+ masks = torch.zeros(1 if self.mask_overlap else nl, img.shape[0] // self.mask_ratio,
+ img.shape[1] // self.mask_ratio)
+ labels['masks'] = masks
+ if self.normalize:
+ instances.normalize(w, h)
+ labels['img'] = self._format_img(img)
+ labels['cls'] = torch.from_numpy(cls) if nl else torch.zeros(nl)
+ labels['bboxes'] = torch.from_numpy(instances.bboxes) if nl else torch.zeros((nl, 4))
+ if self.return_keypoint:
+ labels['keypoints'] = torch.from_numpy(instances.keypoints)
+ # Then we can use collate_fn
+ if self.batch_idx:
+ labels['batch_idx'] = torch.zeros(nl)
+ return labels
+
+ def _format_img(self, img):
+ """Format the image for YOLOv5 from Numpy array to PyTorch tensor."""
+ if len(img.shape) < 3:
+ img = np.expand_dims(img, -1)
+ img = np.ascontiguousarray(img.transpose(2, 0, 1)[::-1])
+ img = torch.from_numpy(img)
+ return img
+
+ def _format_segments(self, instances, cls, w, h):
+ """convert polygon points to bitmap."""
+ segments = instances.segments
+ if self.mask_overlap:
+ masks, sorted_idx = polygons2masks_overlap((h, w), segments, downsample_ratio=self.mask_ratio)
+ masks = masks[None] # (640, 640) -> (1, 640, 640)
+ instances = instances[sorted_idx]
+ cls = cls[sorted_idx]
+ else:
+ masks = polygons2masks((h, w), segments, color=1, downsample_ratio=self.mask_ratio)
+
+ return masks, instances, cls
+
+
+def v8_transforms(dataset, imgsz, hyp, stretch=False):
+ """Convert images to a size suitable for YOLOv8 training."""
+ pre_transform = Compose([
+ Mosaic(dataset, imgsz=imgsz, p=hyp.mosaic),
+ CopyPaste(p=hyp.copy_paste),
+ RandomPerspective(
+ degrees=hyp.degrees,
+ translate=hyp.translate,
+ scale=hyp.scale,
+ shear=hyp.shear,
+ perspective=hyp.perspective,
+ pre_transform=None if stretch else LetterBox(new_shape=(imgsz, imgsz)),
+ )])
+ flip_idx = dataset.data.get('flip_idx', []) # for keypoints augmentation
+ if dataset.use_keypoints:
+ kpt_shape = dataset.data.get('kpt_shape', None)
+ if len(flip_idx) == 0 and hyp.fliplr > 0.0:
+ hyp.fliplr = 0.0
+ LOGGER.warning("WARNING ⚠️ No 'flip_idx' array defined in data.yaml, setting augmentation 'fliplr=0.0'")
+ elif flip_idx and (len(flip_idx) != kpt_shape[0]):
+ raise ValueError(f'data.yaml flip_idx={flip_idx} length must be equal to kpt_shape[0]={kpt_shape[0]}')
+
+ return Compose([
+ pre_transform,
+ MixUp(dataset, pre_transform=pre_transform, p=hyp.mixup),
+ Albumentations(p=1.0),
+ RandomHSV(hgain=hyp.hsv_h, sgain=hyp.hsv_s, vgain=hyp.hsv_v),
+ RandomFlip(direction='vertical', p=hyp.flipud),
+ RandomFlip(direction='horizontal', p=hyp.fliplr, flip_idx=flip_idx)]) # transforms
+
+
+# Classification augmentations -----------------------------------------------------------------------------------------
+def classify_transforms(size=224, mean=(0.0, 0.0, 0.0), std=(1.0, 1.0, 1.0)): # IMAGENET_MEAN, IMAGENET_STD
+ # Transforms to apply if albumentations not installed
+ if not isinstance(size, int):
+ raise TypeError(f'classify_transforms() size {size} must be integer, not (list, tuple)')
+ if any(mean) or any(std):
+ return T.Compose([CenterCrop(size), ToTensor(), T.Normalize(mean, std, inplace=True)])
+ else:
+ return T.Compose([CenterCrop(size), ToTensor()])
+
+
+def hsv2colorjitter(h, s, v):
+ """Map HSV (hue, saturation, value) jitter into ColorJitter values (brightness, contrast, saturation, hue)"""
+ return v, v, s, h
+
+
+def classify_albumentations(
+ augment=True,
+ size=224,
+ scale=(0.08, 1.0),
+ hflip=0.5,
+ vflip=0.0,
+ hsv_h=0.015, # image HSV-Hue augmentation (fraction)
+ hsv_s=0.7, # image HSV-Saturation augmentation (fraction)
+ hsv_v=0.4, # image HSV-Value augmentation (fraction)
+ mean=(0.0, 0.0, 0.0), # IMAGENET_MEAN
+ std=(1.0, 1.0, 1.0), # IMAGENET_STD
+ auto_aug=False,
+):
+ # YOLOv8 classification Albumentations (optional, only used if package is installed)
+ prefix = colorstr('albumentations: ')
+ try:
+ import albumentations as A
+ from albumentations.pytorch import ToTensorV2
+
+ check_version(A.__version__, '1.0.3', hard=True) # version requirement
+ if augment: # Resize and crop
+ T = [A.RandomResizedCrop(height=size, width=size, scale=scale)]
+ if auto_aug:
+ # TODO: implement AugMix, AutoAug & RandAug in albumentations
+ LOGGER.info(f'{prefix}auto augmentations are currently not supported')
+ else:
+ if hflip > 0:
+ T += [A.HorizontalFlip(p=hflip)]
+ if vflip > 0:
+ T += [A.VerticalFlip(p=vflip)]
+ if any((hsv_h, hsv_s, hsv_v)):
+ T += [A.ColorJitter(*hsv2colorjitter(hsv_h, hsv_s, hsv_v))] # brightness, contrast, saturation, hue
+ else: # Use fixed crop for eval set (reproducibility)
+ T = [A.SmallestMaxSize(max_size=size), A.CenterCrop(height=size, width=size)]
+ T += [A.Normalize(mean=mean, std=std), ToTensorV2()] # Normalize and convert to Tensor
+ LOGGER.info(prefix + ', '.join(f'{x}'.replace('always_apply=False, ', '') for x in T if x.p))
+ return A.Compose(T)
+
+ except ImportError: # package not installed, skip
+ pass
+ except Exception as e:
+ LOGGER.info(f'{prefix}{e}')
+
+
+class ClassifyLetterBox:
+ # YOLOv8 LetterBox class for image preprocessing, i.e. T.Compose([LetterBox(size), ToTensor()])
+ def __init__(self, size=(640, 640), auto=False, stride=32):
+ """Resizes image and crops it to center with max dimensions 'h' and 'w'."""
+ super().__init__()
+ self.h, self.w = (size, size) if isinstance(size, int) else size
+ self.auto = auto # pass max size integer, automatically solve for short side using stride
+ self.stride = stride # used with auto
+
+ def __call__(self, im): # im = np.array HWC
+ imh, imw = im.shape[:2]
+ r = min(self.h / imh, self.w / imw) # ratio of new/old
+ h, w = round(imh * r), round(imw * r) # resized image
+ hs, ws = (math.ceil(x / self.stride) * self.stride for x in (h, w)) if self.auto else self.h, self.w
+ top, left = round((hs - h) / 2 - 0.1), round((ws - w) / 2 - 0.1)
+ im_out = np.full((self.h, self.w, 3), 114, dtype=im.dtype)
+ im_out[top:top + h, left:left + w] = cv2.resize(im, (w, h), interpolation=cv2.INTER_LINEAR)
+ return im_out
+
+
+class CenterCrop:
+ # YOLOv8 CenterCrop class for image preprocessing, i.e. T.Compose([CenterCrop(size), ToTensor()])
+ def __init__(self, size=640):
+ """Converts an image from numpy array to PyTorch tensor."""
+ super().__init__()
+ self.h, self.w = (size, size) if isinstance(size, int) else size
+
+ def __call__(self, im): # im = np.array HWC
+ imh, imw = im.shape[:2]
+ m = min(imh, imw) # min dimension
+ top, left = (imh - m) // 2, (imw - m) // 2
+ return cv2.resize(im[top:top + m, left:left + m], (self.w, self.h), interpolation=cv2.INTER_LINEAR)
+
+
+class ToTensor:
+ # YOLOv8 ToTensor class for image preprocessing, i.e. T.Compose([LetterBox(size), ToTensor()])
+ def __init__(self, half=False):
+ """Initialize YOLOv8 ToTensor object with optional half-precision support."""
+ super().__init__()
+ self.half = half
+
+ def __call__(self, im): # im = np.array HWC in BGR order
+ im = np.ascontiguousarray(im.transpose((2, 0, 1))[::-1]) # HWC to CHW -> BGR to RGB -> contiguous
+ im = torch.from_numpy(im) # to torch
+ im = im.half() if self.half else im.float() # uint8 to fp16/32
+ im /= 255.0 # 0-255 to 0.0-1.0
+ return im
diff --git a/yolov8/ultralytics/yolo/data/base.py b/yolov8/ultralytics/yolo/data/base.py
new file mode 100644
index 0000000000000000000000000000000000000000..d2e9793c13dd12715ee96243c16e31006aa3d8be
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/base.py
@@ -0,0 +1,286 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import glob
+import math
+import os
+import random
+from copy import deepcopy
+from multiprocessing.pool import ThreadPool
+from pathlib import Path
+from typing import Optional
+
+import cv2
+import numpy as np
+import psutil
+from torch.utils.data import Dataset
+from tqdm import tqdm
+
+from ..utils import DEFAULT_CFG, LOCAL_RANK, LOGGER, NUM_THREADS, TQDM_BAR_FORMAT
+from .utils import HELP_URL, IMG_FORMATS
+
+
+class BaseDataset(Dataset):
+ """
+ Base dataset class for loading and processing image data.
+
+ Args:
+ img_path (str): Path to the folder containing images.
+ imgsz (int, optional): Image size. Defaults to 640.
+ cache (bool, optional): Cache images to RAM or disk during training. Defaults to False.
+ augment (bool, optional): If True, data augmentation is applied. Defaults to True.
+ hyp (dict, optional): Hyperparameters to apply data augmentation. Defaults to None.
+ prefix (str, optional): Prefix to print in log messages. Defaults to ''.
+ rect (bool, optional): If True, rectangular training is used. Defaults to False.
+ batch_size (int, optional): Size of batches. Defaults to None.
+ stride (int, optional): Stride. Defaults to 32.
+ pad (float, optional): Padding. Defaults to 0.0.
+ single_cls (bool, optional): If True, single class training is used. Defaults to False.
+ classes (list): List of included classes. Default is None.
+ fraction (float): Fraction of dataset to utilize. Default is 1.0 (use all data).
+
+ Attributes:
+ im_files (list): List of image file paths.
+ labels (list): List of label data dictionaries.
+ ni (int): Number of images in the dataset.
+ ims (list): List of loaded images.
+ npy_files (list): List of numpy file paths.
+ transforms (callable): Image transformation function.
+ """
+
+ def __init__(self,
+ img_path,
+ imgsz=640,
+ cache=False,
+ augment=True,
+ hyp=DEFAULT_CFG,
+ prefix='',
+ rect=False,
+ batch_size=16,
+ stride=32,
+ pad=0.5,
+ single_cls=False,
+ classes=None,
+ fraction=1.0):
+ super().__init__()
+ self.img_path = img_path
+ self.imgsz = imgsz
+ self.augment = augment
+ self.single_cls = single_cls
+ self.prefix = prefix
+ self.fraction = fraction
+ self.im_files = self.get_img_files(self.img_path)
+ self.labels = self.get_labels()
+ self.update_labels(include_class=classes) # single_cls and include_class
+ self.ni = len(self.labels) # number of images
+ self.rect = rect
+ self.batch_size = batch_size
+ self.stride = stride
+ self.pad = pad
+ if self.rect:
+ assert self.batch_size is not None
+ self.set_rectangle()
+
+ # Buffer thread for mosaic images
+ self.buffer = [] # buffer size = batch size
+ self.max_buffer_length = min((self.ni, self.batch_size * 8, 1000)) if self.augment else 0
+
+ # Cache stuff
+ if cache == 'ram' and not self.check_cache_ram():
+ cache = False
+ self.ims, self.im_hw0, self.im_hw = [None] * self.ni, [None] * self.ni, [None] * self.ni
+ self.npy_files = [Path(f).with_suffix('.npy') for f in self.im_files]
+ if cache:
+ self.cache_images(cache)
+
+ # Transforms
+ self.transforms = self.build_transforms(hyp=hyp)
+
+ def get_img_files(self, img_path):
+ """Read image files."""
+ try:
+ f = [] # image files
+ for p in img_path if isinstance(img_path, list) else [img_path]:
+ p = Path(p) # os-agnostic
+ if p.is_dir(): # dir
+ f += glob.glob(str(p / '**' / '*.*'), recursive=True)
+ # F = list(p.rglob('*.*')) # pathlib
+ elif p.is_file(): # file
+ with open(p) as t:
+ t = t.read().strip().splitlines()
+ parent = str(p.parent) + os.sep
+ f += [x.replace('./', parent) if x.startswith('./') else x for x in t] # local to global path
+ # F += [p.parent / x.lstrip(os.sep) for x in t] # local to global path (pathlib)
+ else:
+ raise FileNotFoundError(f'{self.prefix}{p} does not exist')
+ im_files = sorted(x.replace('/', os.sep) for x in f if x.split('.')[-1].lower() in IMG_FORMATS)
+ # self.img_files = sorted([x for x in f if x.suffix[1:].lower() in IMG_FORMATS]) # pathlib
+ assert im_files, f'{self.prefix}No images found'
+ except Exception as e:
+ raise FileNotFoundError(f'{self.prefix}Error loading data from {img_path}\n{HELP_URL}') from e
+ if self.fraction < 1:
+ im_files = im_files[:round(len(im_files) * self.fraction)]
+ return im_files
+
+ def update_labels(self, include_class: Optional[list]):
+ """include_class, filter labels to include only these classes (optional)."""
+ include_class_array = np.array(include_class).reshape(1, -1)
+ for i in range(len(self.labels)):
+ if include_class is not None:
+ cls = self.labels[i]['cls']
+ bboxes = self.labels[i]['bboxes']
+ segments = self.labels[i]['segments']
+ keypoints = self.labels[i]['keypoints']
+ j = (cls == include_class_array).any(1)
+ self.labels[i]['cls'] = cls[j]
+ self.labels[i]['bboxes'] = bboxes[j]
+ if segments:
+ self.labels[i]['segments'] = [segments[si] for si, idx in enumerate(j) if idx]
+ if keypoints is not None:
+ self.labels[i]['keypoints'] = keypoints[j]
+ if self.single_cls:
+ self.labels[i]['cls'][:, 0] = 0
+
+ def load_image(self, i):
+ """Loads 1 image from dataset index 'i', returns (im, resized hw)."""
+ im, f, fn = self.ims[i], self.im_files[i], self.npy_files[i]
+ if im is None: # not cached in RAM
+ if fn.exists(): # load npy
+ im = np.load(fn)
+ else: # read image
+ im = cv2.imread(f) # BGR
+ if im is None:
+ raise FileNotFoundError(f'Image Not Found {f}')
+ h0, w0 = im.shape[:2] # orig hw
+ r = self.imgsz / max(h0, w0) # ratio
+ if r != 1: # if sizes are not equal
+ interp = cv2.INTER_LINEAR if (self.augment or r > 1) else cv2.INTER_AREA
+ im = cv2.resize(im, (min(math.ceil(w0 * r), self.imgsz), min(math.ceil(h0 * r), self.imgsz)),
+ interpolation=interp)
+
+ # Add to buffer if training with augmentations
+ if self.augment:
+ self.ims[i], self.im_hw0[i], self.im_hw[i] = im, (h0, w0), im.shape[:2] # im, hw_original, hw_resized
+ self.buffer.append(i)
+ if len(self.buffer) >= self.max_buffer_length:
+ j = self.buffer.pop(0)
+ self.ims[j], self.im_hw0[j], self.im_hw[j] = None, None, None
+
+ return im, (h0, w0), im.shape[:2]
+
+ return self.ims[i], self.im_hw0[i], self.im_hw[i]
+
+ def cache_images(self, cache):
+ """Cache images to memory or disk."""
+ b, gb = 0, 1 << 30 # bytes of cached images, bytes per gigabytes
+ fcn = self.cache_images_to_disk if cache == 'disk' else self.load_image
+ with ThreadPool(NUM_THREADS) as pool:
+ results = pool.imap(fcn, range(self.ni))
+ pbar = tqdm(enumerate(results), total=self.ni, bar_format=TQDM_BAR_FORMAT, disable=LOCAL_RANK > 0)
+ for i, x in pbar:
+ if cache == 'disk':
+ b += self.npy_files[i].stat().st_size
+ else: # 'ram'
+ self.ims[i], self.im_hw0[i], self.im_hw[i] = x # im, hw_orig, hw_resized = load_image(self, i)
+ b += self.ims[i].nbytes
+ pbar.desc = f'{self.prefix}Caching images ({b / gb:.1f}GB {cache})'
+ pbar.close()
+
+ def cache_images_to_disk(self, i):
+ """Saves an image as an *.npy file for faster loading."""
+ f = self.npy_files[i]
+ if not f.exists():
+ np.save(f.as_posix(), cv2.imread(self.im_files[i]))
+
+ def check_cache_ram(self, safety_margin=0.5):
+ """Check image caching requirements vs available memory."""
+ b, gb = 0, 1 << 30 # bytes of cached images, bytes per gigabytes
+ n = min(self.ni, 30) # extrapolate from 30 random images
+ for _ in range(n):
+ im = cv2.imread(random.choice(self.im_files)) # sample image
+ ratio = self.imgsz / max(im.shape[0], im.shape[1]) # max(h, w) # ratio
+ b += im.nbytes * ratio ** 2
+ mem_required = b * self.ni / n * (1 + safety_margin) # GB required to cache dataset into RAM
+ mem = psutil.virtual_memory()
+ cache = mem_required < mem.available # to cache or not to cache, that is the question
+ if not cache:
+ LOGGER.info(f'{self.prefix}{mem_required / gb:.1f}GB RAM required to cache images '
+ f'with {int(safety_margin * 100)}% safety margin but only '
+ f'{mem.available / gb:.1f}/{mem.total / gb:.1f}GB available, '
+ f"{'caching images ✅' if cache else 'not caching images ⚠️'}")
+ return cache
+
+ def set_rectangle(self):
+ """Sets the shape of bounding boxes for YOLO detections as rectangles."""
+ bi = np.floor(np.arange(self.ni) / self.batch_size).astype(int) # batch index
+ nb = bi[-1] + 1 # number of batches
+
+ s = np.array([x.pop('shape') for x in self.labels]) # hw
+ ar = s[:, 0] / s[:, 1] # aspect ratio
+ irect = ar.argsort()
+ self.im_files = [self.im_files[i] for i in irect]
+ self.labels = [self.labels[i] for i in irect]
+ ar = ar[irect]
+
+ # Set training image shapes
+ shapes = [[1, 1]] * nb
+ for i in range(nb):
+ ari = ar[bi == i]
+ mini, maxi = ari.min(), ari.max()
+ if maxi < 1:
+ shapes[i] = [maxi, 1]
+ elif mini > 1:
+ shapes[i] = [1, 1 / mini]
+
+ self.batch_shapes = np.ceil(np.array(shapes) * self.imgsz / self.stride + self.pad).astype(int) * self.stride
+ self.batch = bi # batch index of image
+
+ def __getitem__(self, index):
+ """Returns transformed label information for given index."""
+ return self.transforms(self.get_image_and_label(index))
+
+ def get_image_and_label(self, index):
+ """Get and return label information from the dataset."""
+ label = deepcopy(self.labels[index]) # requires deepcopy() https://github.com/ultralytics/ultralytics/pull/1948
+ label.pop('shape', None) # shape is for rect, remove it
+ label['img'], label['ori_shape'], label['resized_shape'] = self.load_image(index)
+ label['ratio_pad'] = (label['resized_shape'][0] / label['ori_shape'][0],
+ label['resized_shape'][1] / label['ori_shape'][1]) # for evaluation
+ if self.rect:
+ label['rect_shape'] = self.batch_shapes[self.batch[index]]
+ return self.update_labels_info(label)
+
+ def __len__(self):
+ """Returns the length of the labels list for the dataset."""
+ return len(self.labels)
+
+ def update_labels_info(self, label):
+ """custom your label format here."""
+ return label
+
+ def build_transforms(self, hyp=None):
+ """Users can custom augmentations here
+ like:
+ if self.augment:
+ # Training transforms
+ return Compose([])
+ else:
+ # Val transforms
+ return Compose([])
+ """
+ raise NotImplementedError
+
+ def get_labels(self):
+ """Users can custom their own format here.
+ Make sure your output is a list with each element like below:
+ dict(
+ im_file=im_file,
+ shape=shape, # format: (height, width)
+ cls=cls,
+ bboxes=bboxes, # xywh
+ segments=segments, # xy
+ keypoints=keypoints, # xy
+ normalized=True, # or False
+ bbox_format="xyxy", # or xywh, ltwh
+ )
+ """
+ raise NotImplementedError
diff --git a/yolov8/ultralytics/yolo/data/build.py b/yolov8/ultralytics/yolo/data/build.py
new file mode 100644
index 0000000000000000000000000000000000000000..54d2d16fc84c89ff311edced4b0e5b9e341dce3c
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/build.py
@@ -0,0 +1,170 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+import random
+from pathlib import Path
+
+import numpy as np
+import torch
+from PIL import Image
+from torch.utils.data import dataloader, distributed
+
+from ultralytics.yolo.data.dataloaders.stream_loaders import (LOADERS, LoadImages, LoadPilAndNumpy, LoadScreenshots,
+ LoadStreams, LoadTensor, SourceTypes, autocast_list)
+from ultralytics.yolo.data.utils import IMG_FORMATS, VID_FORMATS
+from ultralytics.yolo.utils.checks import check_file
+
+from ..utils import RANK, colorstr
+from .dataset import YOLODataset
+from .utils import PIN_MEMORY
+
+
+class InfiniteDataLoader(dataloader.DataLoader):
+ """Dataloader that reuses workers. Uses same syntax as vanilla DataLoader."""
+
+ def __init__(self, *args, **kwargs):
+ """Dataloader that infinitely recycles workers, inherits from DataLoader."""
+ super().__init__(*args, **kwargs)
+ object.__setattr__(self, 'batch_sampler', _RepeatSampler(self.batch_sampler))
+ self.iterator = super().__iter__()
+
+ def __len__(self):
+ """Returns the length of the batch sampler's sampler."""
+ return len(self.batch_sampler.sampler)
+
+ def __iter__(self):
+ """Creates a sampler that repeats indefinitely."""
+ for _ in range(len(self)):
+ yield next(self.iterator)
+
+ def reset(self):
+ """Reset iterator.
+ This is useful when we want to modify settings of dataset while training.
+ """
+ self.iterator = self._get_iterator()
+
+
+class _RepeatSampler:
+ """
+ Sampler that repeats forever.
+
+ Args:
+ sampler (Dataset.sampler): The sampler to repeat.
+ """
+
+ def __init__(self, sampler):
+ """Initializes an object that repeats a given sampler indefinitely."""
+ self.sampler = sampler
+
+ def __iter__(self):
+ """Iterates over the 'sampler' and yields its contents."""
+ while True:
+ yield from iter(self.sampler)
+
+
+def seed_worker(worker_id): # noqa
+ # Set dataloader worker seed https://pytorch.org/docs/stable/notes/randomness.html#dataloader
+ worker_seed = torch.initial_seed() % 2 ** 32
+ np.random.seed(worker_seed)
+ random.seed(worker_seed)
+
+
+def build_yolo_dataset(cfg, img_path, batch, data, mode='train', rect=False, stride=32):
+ """Build YOLO Dataset"""
+ return YOLODataset(
+ img_path=img_path,
+ imgsz=cfg.imgsz,
+ batch_size=batch,
+ augment=mode == 'train', # augmentation
+ hyp=cfg, # TODO: probably add a get_hyps_from_cfg function
+ rect=cfg.rect or rect, # rectangular batches
+ cache=cfg.cache or None,
+ single_cls=cfg.single_cls or False,
+ stride=int(stride),
+ pad=0.0 if mode == 'train' else 0.5,
+ prefix=colorstr(f'{mode}: '),
+ use_segments=cfg.task == 'segment',
+ use_keypoints=cfg.task == 'pose',
+ classes=cfg.classes,
+ data=data,
+ fraction=cfg.fraction if mode == 'train' else 1.0)
+
+
+def build_dataloader(dataset, batch, workers, shuffle=True, rank=-1):
+ """Return an InfiniteDataLoader or DataLoader for training or validation set."""
+ batch = min(batch, len(dataset))
+ nd = torch.cuda.device_count() # number of CUDA devices
+ nw = min([os.cpu_count() // max(nd, 1), batch if batch > 1 else 0, workers]) # number of workers
+ sampler = None if rank == -1 else distributed.DistributedSampler(dataset, shuffle=shuffle)
+ generator = torch.Generator()
+ generator.manual_seed(6148914691236517205 + RANK)
+ return InfiniteDataLoader(dataset=dataset,
+ batch_size=batch,
+ shuffle=shuffle and sampler is None,
+ num_workers=nw,
+ sampler=sampler,
+ pin_memory=PIN_MEMORY,
+ collate_fn=getattr(dataset, 'collate_fn', None),
+ worker_init_fn=seed_worker,
+ generator=generator)
+
+
+def check_source(source):
+ """Check source type and return corresponding flag values."""
+ webcam, screenshot, from_img, in_memory, tensor = False, False, False, False, False
+ if isinstance(source, (str, int, Path)): # int for local usb camera
+ source = str(source)
+ is_file = Path(source).suffix[1:] in (IMG_FORMATS + VID_FORMATS)
+ is_url = source.lower().startswith(('https://', 'http://', 'rtsp://', 'rtmp://'))
+ webcam = source.isnumeric() or source.endswith('.streams') or (is_url and not is_file)
+ screenshot = source.lower() == 'screen'
+ if is_url and is_file:
+ source = check_file(source) # download
+ elif isinstance(source, tuple(LOADERS)):
+ in_memory = True
+ elif isinstance(source, (list, tuple)):
+ source = autocast_list(source) # convert all list elements to PIL or np arrays
+ from_img = True
+ elif isinstance(source, (Image.Image, np.ndarray)):
+ from_img = True
+ elif isinstance(source, torch.Tensor):
+ tensor = True
+ else:
+ raise TypeError('Unsupported image type. For supported types see https://docs.ultralytics.com/modes/predict')
+
+ return source, webcam, screenshot, from_img, in_memory, tensor
+
+
+def load_inference_source(source=None, imgsz=640, vid_stride=1):
+ """
+ Loads an inference source for object detection and applies necessary transformations.
+
+ Args:
+ source (str, Path, Tensor, PIL.Image, np.ndarray): The input source for inference.
+ imgsz (int, optional): The size of the image for inference. Default is 640.
+ vid_stride (int, optional): The frame interval for video sources. Default is 1.
+
+ Returns:
+ dataset (Dataset): A dataset object for the specified input source.
+ """
+ source, webcam, screenshot, from_img, in_memory, tensor = check_source(source)
+ source_type = source.source_type if in_memory else SourceTypes(webcam, screenshot, from_img, tensor)
+
+ # Dataloader
+ if tensor:
+ dataset = LoadTensor(source)
+ elif in_memory:
+ dataset = source
+ elif webcam:
+ dataset = LoadStreams(source, imgsz=imgsz, vid_stride=vid_stride)
+ elif screenshot:
+ dataset = LoadScreenshots(source, imgsz=imgsz)
+ elif from_img:
+ dataset = LoadPilAndNumpy(source, imgsz=imgsz)
+ else:
+ dataset = LoadImages(source, imgsz=imgsz, vid_stride=vid_stride)
+
+ # Attach source types to the dataset
+ setattr(dataset, 'source_type', source_type)
+
+ return dataset
diff --git a/yolov8/ultralytics/yolo/data/converter.py b/yolov8/ultralytics/yolo/data/converter.py
new file mode 100644
index 0000000000000000000000000000000000000000..c1278dd952381faa1b6a88e03c8cc962d8cadea9
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/converter.py
@@ -0,0 +1,230 @@
+import json
+from collections import defaultdict
+from pathlib import Path
+
+import cv2
+import numpy as np
+from tqdm import tqdm
+
+from ultralytics.yolo.utils.checks import check_requirements
+from ultralytics.yolo.utils.files import make_dirs
+
+
+def coco91_to_coco80_class():
+ """Converts 91-index COCO class IDs to 80-index COCO class IDs.
+
+ Returns:
+ (list): A list of 91 class IDs where the index represents the 80-index class ID and the value is the
+ corresponding 91-index class ID.
+
+ """
+ return [
+ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, None, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, None, 24, 25, None,
+ None, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, None, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50,
+ 51, 52, 53, 54, 55, 56, 57, 58, 59, None, 60, None, None, 61, None, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72,
+ None, 73, 74, 75, 76, 77, 78, 79, None]
+
+
+def convert_coco(labels_dir='../coco/annotations/', use_segments=False, use_keypoints=False, cls91to80=True):
+ """Converts COCO dataset annotations to a format suitable for training YOLOv5 models.
+
+ Args:
+ labels_dir (str, optional): Path to directory containing COCO dataset annotation files.
+ use_segments (bool, optional): Whether to include segmentation masks in the output.
+ use_keypoints (bool, optional): Whether to include keypoint annotations in the output.
+ cls91to80 (bool, optional): Whether to map 91 COCO class IDs to the corresponding 80 COCO class IDs.
+
+ Raises:
+ FileNotFoundError: If the labels_dir path does not exist.
+
+ Example Usage:
+ convert_coco(labels_dir='../coco/annotations/', use_segments=True, use_keypoints=True, cls91to80=True)
+
+ Output:
+ Generates output files in the specified output directory.
+ """
+
+ save_dir = make_dirs('yolo_labels') # output directory
+ coco80 = coco91_to_coco80_class()
+
+ # Import json
+ for json_file in sorted(Path(labels_dir).resolve().glob('*.json')):
+ fn = Path(save_dir) / 'labels' / json_file.stem.replace('instances_', '') # folder name
+ fn.mkdir(parents=True, exist_ok=True)
+ with open(json_file) as f:
+ data = json.load(f)
+
+ # Create image dict
+ images = {f'{x["id"]:d}': x for x in data['images']}
+ # Create image-annotations dict
+ imgToAnns = defaultdict(list)
+ for ann in data['annotations']:
+ imgToAnns[ann['image_id']].append(ann)
+
+ # Write labels file
+ for img_id, anns in tqdm(imgToAnns.items(), desc=f'Annotations {json_file}'):
+ img = images[f'{img_id:d}']
+ h, w, f = img['height'], img['width'], img['file_name']
+
+ bboxes = []
+ segments = []
+ keypoints = []
+ for ann in anns:
+ if ann['iscrowd']:
+ continue
+ # The COCO box format is [top left x, top left y, width, height]
+ box = np.array(ann['bbox'], dtype=np.float64)
+ box[:2] += box[2:] / 2 # xy top-left corner to center
+ box[[0, 2]] /= w # normalize x
+ box[[1, 3]] /= h # normalize y
+ if box[2] <= 0 or box[3] <= 0: # if w <= 0 and h <= 0
+ continue
+
+ cls = coco80[ann['category_id'] - 1] if cls91to80 else ann['category_id'] - 1 # class
+ box = [cls] + box.tolist()
+ if box not in bboxes:
+ bboxes.append(box)
+ if use_segments and ann.get('segmentation') is not None:
+ if len(ann['segmentation']) == 0:
+ segments.append([])
+ continue
+ if isinstance(ann['segmentation'], dict):
+ ann['segmentation'] = rle2polygon(ann['segmentation'])
+ if len(ann['segmentation']) > 1:
+ s = merge_multi_segment(ann['segmentation'])
+ s = (np.concatenate(s, axis=0) / np.array([w, h])).reshape(-1).tolist()
+ else:
+ s = [j for i in ann['segmentation'] for j in i] # all segments concatenated
+ s = (np.array(s).reshape(-1, 2) / np.array([w, h])).reshape(-1).tolist()
+ s = [cls] + s
+ if s not in segments:
+ segments.append(s)
+ if use_keypoints and ann.get('keypoints') is not None:
+ k = (np.array(ann['keypoints']).reshape(-1, 3) / np.array([w, h, 1])).reshape(-1).tolist()
+ k = box + k
+ keypoints.append(k)
+
+ # Write
+ with open((fn / f).with_suffix('.txt'), 'a') as file:
+ for i in range(len(bboxes)):
+ if use_keypoints:
+ line = *(keypoints[i]), # cls, box, keypoints
+ else:
+ line = *(segments[i]
+ if use_segments and len(segments[i]) > 0 else bboxes[i]), # cls, box or segments
+ file.write(('%g ' * len(line)).rstrip() % line + '\n')
+
+
+def rle2polygon(segmentation):
+ """
+ Convert Run-Length Encoding (RLE) mask to polygon coordinates.
+
+ Args:
+ segmentation (dict, list): RLE mask representation of the object segmentation.
+
+ Returns:
+ (list): A list of lists representing the polygon coordinates for each contour.
+
+ Note:
+ Requires the 'pycocotools' package to be installed.
+ """
+ check_requirements('pycocotools')
+ from pycocotools import mask
+
+ m = mask.decode(segmentation)
+ m[m > 0] = 255
+ contours, _ = cv2.findContours(m, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_TC89_KCOS)
+ polygons = []
+ for contour in contours:
+ epsilon = 0.001 * cv2.arcLength(contour, True)
+ contour_approx = cv2.approxPolyDP(contour, epsilon, True)
+ polygon = contour_approx.flatten().tolist()
+ polygons.append(polygon)
+ return polygons
+
+
+def min_index(arr1, arr2):
+ """
+ Find a pair of indexes with the shortest distance between two arrays of 2D points.
+
+ Args:
+ arr1 (np.array): A NumPy array of shape (N, 2) representing N 2D points.
+ arr2 (np.array): A NumPy array of shape (M, 2) representing M 2D points.
+
+ Returns:
+ (tuple): A tuple containing the indexes of the points with the shortest distance in arr1 and arr2 respectively.
+ """
+ dis = ((arr1[:, None, :] - arr2[None, :, :]) ** 2).sum(-1)
+ return np.unravel_index(np.argmin(dis, axis=None), dis.shape)
+
+
+def merge_multi_segment(segments):
+ """
+ Merge multiple segments into one list by connecting the coordinates with the minimum distance between each segment.
+ This function connects these coordinates with a thin line to merge all segments into one.
+
+ Args:
+ segments (List[List]): Original segmentations in COCO's JSON file.
+ Each element is a list of coordinates, like [segmentation1, segmentation2,...].
+
+ Returns:
+ s (List[np.ndarray]): A list of connected segments represented as NumPy arrays.
+ """
+ s = []
+ segments = [np.array(i).reshape(-1, 2) for i in segments]
+ idx_list = [[] for _ in range(len(segments))]
+
+ # record the indexes with min distance between each segment
+ for i in range(1, len(segments)):
+ idx1, idx2 = min_index(segments[i - 1], segments[i])
+ idx_list[i - 1].append(idx1)
+ idx_list[i].append(idx2)
+
+ # use two round to connect all the segments
+ for k in range(2):
+ # forward connection
+ if k == 0:
+ for i, idx in enumerate(idx_list):
+ # middle segments have two indexes
+ # reverse the index of middle segments
+ if len(idx) == 2 and idx[0] > idx[1]:
+ idx = idx[::-1]
+ segments[i] = segments[i][::-1, :]
+
+ segments[i] = np.roll(segments[i], -idx[0], axis=0)
+ segments[i] = np.concatenate([segments[i], segments[i][:1]])
+ # deal with the first segment and the last one
+ if i in [0, len(idx_list) - 1]:
+ s.append(segments[i])
+ else:
+ idx = [0, idx[1] - idx[0]]
+ s.append(segments[i][idx[0]:idx[1] + 1])
+
+ else:
+ for i in range(len(idx_list) - 1, -1, -1):
+ if i not in [0, len(idx_list) - 1]:
+ idx = idx_list[i]
+ nidx = abs(idx[1] - idx[0])
+ s.append(segments[i][nidx:])
+ return s
+
+
+def delete_dsstore(path='../datasets'):
+ """Delete Apple .DS_Store files in the specified directory and its subdirectories."""
+ from pathlib import Path
+
+ files = list(Path(path).rglob('.DS_store'))
+ print(files)
+ for f in files:
+ f.unlink()
+
+
+if __name__ == '__main__':
+ source = 'COCO'
+
+ if source == 'COCO':
+ convert_coco(
+ '../datasets/coco/annotations', # directory with *.json
+ use_segments=False,
+ use_keypoints=True,
+ cls91to80=False)
diff --git a/yolov8/ultralytics/yolo/data/dataloaders/__init__.py b/yolov8/ultralytics/yolo/data/dataloaders/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/yolov8/ultralytics/yolo/data/dataloaders/stream_loaders.py b/yolov8/ultralytics/yolo/data/dataloaders/stream_loaders.py
new file mode 100644
index 0000000000000000000000000000000000000000..f497cb1c1b1f2e32a5d7c1570f5fa83deed4c08d
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/dataloaders/stream_loaders.py
@@ -0,0 +1,401 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import glob
+import math
+import os
+import time
+from dataclasses import dataclass
+from pathlib import Path
+from threading import Thread
+from urllib.parse import urlparse
+
+import cv2
+import numpy as np
+import requests
+import torch
+from PIL import Image
+
+from ultralytics.yolo.data.utils import IMG_FORMATS, VID_FORMATS
+from ultralytics.yolo.utils import LOGGER, ROOT, is_colab, is_kaggle, ops
+from ultralytics.yolo.utils.checks import check_requirements
+
+
+@dataclass
+class SourceTypes:
+ webcam: bool = False
+ screenshot: bool = False
+ from_img: bool = False
+ tensor: bool = False
+
+
+class LoadStreams:
+ # YOLOv8 streamloader, i.e. `yolo predict source='rtsp://example.com/media.mp4' # RTSP, RTMP, HTTP streams`
+ def __init__(self, sources='file.streams', imgsz=640, vid_stride=1):
+ """Initialize instance variables and check for consistent input stream shapes."""
+ torch.backends.cudnn.benchmark = True # faster for fixed-size inference
+ self.mode = 'stream'
+ self.imgsz = imgsz
+ self.vid_stride = vid_stride # video frame-rate stride
+ sources = Path(sources).read_text().rsplit() if os.path.isfile(sources) else [sources]
+ n = len(sources)
+ self.sources = [ops.clean_str(x) for x in sources] # clean source names for later
+ self.imgs, self.fps, self.frames, self.threads, self.shape = [[]] * n, [0] * n, [0] * n, [None] * n, [None] * n
+ for i, s in enumerate(sources): # index, source
+ # Start thread to read frames from video stream
+ st = f'{i + 1}/{n}: {s}... '
+ if urlparse(s).hostname in ('www.youtube.com', 'youtube.com', 'youtu.be'): # if source is YouTube video
+ # YouTube format i.e. 'https://www.youtube.com/watch?v=Zgi9g1ksQHc' or 'https://youtu.be/Zgi9g1ksQHc'
+ s = get_best_youtube_url(s)
+ s = eval(s) if s.isnumeric() else s # i.e. s = '0' local webcam
+ if s == 0 and (is_colab() or is_kaggle()):
+ raise NotImplementedError("'source=0' webcam not supported in Colab and Kaggle notebooks. "
+ "Try running 'source=0' in a local environment.")
+ cap = cv2.VideoCapture(s)
+ if not cap.isOpened():
+ raise ConnectionError(f'{st}Failed to open {s}')
+ w = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
+ h = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
+ fps = cap.get(cv2.CAP_PROP_FPS) # warning: may return 0 or nan
+ self.frames[i] = max(int(cap.get(cv2.CAP_PROP_FRAME_COUNT)), 0) or float('inf') # infinite stream fallback
+ self.fps[i] = max((fps if math.isfinite(fps) else 0) % 100, 0) or 30 # 30 FPS fallback
+
+ success, im = cap.read() # guarantee first frame
+ if not success or im is None:
+ raise ConnectionError(f'{st}Failed to read images from {s}')
+ self.imgs[i].append(im)
+ self.shape[i] = im.shape
+ self.threads[i] = Thread(target=self.update, args=([i, cap, s]), daemon=True)
+ LOGGER.info(f'{st}Success ✅ ({self.frames[i]} frames of shape {w}x{h} at {self.fps[i]:.2f} FPS)')
+ self.threads[i].start()
+ LOGGER.info('') # newline
+
+ # Check for common shapes
+ self.bs = self.__len__()
+
+ def update(self, i, cap, stream):
+ """Read stream `i` frames in daemon thread."""
+ n, f = 0, self.frames[i] # frame number, frame array
+ while cap.isOpened() and n < f:
+ # Only read a new frame if the buffer is empty
+ if not self.imgs[i]:
+ n += 1
+ cap.grab() # .read() = .grab() followed by .retrieve()
+ if n % self.vid_stride == 0:
+ success, im = cap.retrieve()
+ if success:
+ self.imgs[i].append(im) # add image to buffer
+ else:
+ LOGGER.warning('WARNING ⚠️ Video stream unresponsive, please check your IP camera connection.')
+ self.imgs[i].append(np.zeros(self.shape[i]))
+ cap.open(stream) # re-open stream if signal was lost
+ else:
+ time.sleep(0.01) # wait until the buffer is empty
+
+ def __iter__(self):
+ """Iterates through YOLO image feed and re-opens unresponsive streams."""
+ self.count = -1
+ return self
+
+ def __next__(self):
+ """Returns source paths, transformed and original images for processing."""
+ self.count += 1
+
+ # Wait until a frame is available in each buffer
+ while not all(self.imgs):
+ if not all(x.is_alive() for x in self.threads) or cv2.waitKey(1) == ord('q'): # q to quit
+ cv2.destroyAllWindows()
+ raise StopIteration
+ time.sleep(1 / min(self.fps))
+
+ # Get and remove the next frame from imgs buffer
+ return self.sources, [x.pop(0) for x in self.imgs], None, ''
+
+ def __len__(self):
+ """Return the length of the sources object."""
+ return len(self.sources) # 1E12 frames = 32 streams at 30 FPS for 30 years
+
+
+class LoadScreenshots:
+ # YOLOv8 screenshot dataloader, i.e. `yolo predict source=screen`
+ def __init__(self, source, imgsz=640):
+ """source = [screen_number left top width height] (pixels)."""
+ check_requirements('mss')
+ import mss # noqa
+
+ source, *params = source.split()
+ self.screen, left, top, width, height = 0, None, None, None, None # default to full screen 0
+ if len(params) == 1:
+ self.screen = int(params[0])
+ elif len(params) == 4:
+ left, top, width, height = (int(x) for x in params)
+ elif len(params) == 5:
+ self.screen, left, top, width, height = (int(x) for x in params)
+ self.imgsz = imgsz
+ self.mode = 'stream'
+ self.frame = 0
+ self.sct = mss.mss()
+ self.bs = 1
+
+ # Parse monitor shape
+ monitor = self.sct.monitors[self.screen]
+ self.top = monitor['top'] if top is None else (monitor['top'] + top)
+ self.left = monitor['left'] if left is None else (monitor['left'] + left)
+ self.width = width or monitor['width']
+ self.height = height or monitor['height']
+ self.monitor = {'left': self.left, 'top': self.top, 'width': self.width, 'height': self.height}
+
+ def __iter__(self):
+ """Returns an iterator of the object."""
+ return self
+
+ def __next__(self):
+ """mss screen capture: get raw pixels from the screen as np array."""
+ im0 = np.array(self.sct.grab(self.monitor))[:, :, :3] # [:, :, :3] BGRA to BGR
+ s = f'screen {self.screen} (LTWH): {self.left},{self.top},{self.width},{self.height}: '
+
+ self.frame += 1
+ return str(self.screen), im0, None, s # screen, img, original img, im0s, s
+
+
+class LoadImages:
+ # YOLOv8 image/video dataloader, i.e. `yolo predict source=image.jpg/vid.mp4`
+ def __init__(self, path, imgsz=640, vid_stride=1):
+ """Initialize the Dataloader and raise FileNotFoundError if file not found."""
+ if isinstance(path, str) and Path(path).suffix == '.txt': # *.txt file with img/vid/dir on each line
+ path = Path(path).read_text().rsplit()
+ files = []
+ for p in sorted(path) if isinstance(path, (list, tuple)) else [path]:
+ p = str(Path(p).absolute()) # do not use .resolve() https://github.com/ultralytics/ultralytics/issues/2912
+ if '*' in p:
+ files.extend(sorted(glob.glob(p, recursive=True))) # glob
+ elif os.path.isdir(p):
+ files.extend(sorted(glob.glob(os.path.join(p, '*.*')))) # dir
+ elif os.path.isfile(p):
+ files.append(p) # files
+ else:
+ raise FileNotFoundError(f'{p} does not exist')
+
+ images = [x for x in files if x.split('.')[-1].lower() in IMG_FORMATS]
+ videos = [x for x in files if x.split('.')[-1].lower() in VID_FORMATS]
+ ni, nv = len(images), len(videos)
+
+ self.imgsz = imgsz
+ self.files = images + videos
+ self.nf = ni + nv # number of files
+ self.video_flag = [False] * ni + [True] * nv
+ self.mode = 'image'
+ self.vid_stride = vid_stride # video frame-rate stride
+ self.bs = 1
+ if any(videos):
+ self.orientation = None # rotation degrees
+ self._new_video(videos[0]) # new video
+ else:
+ self.cap = None
+ if self.nf == 0:
+ raise FileNotFoundError(f'No images or videos found in {p}. '
+ f'Supported formats are:\nimages: {IMG_FORMATS}\nvideos: {VID_FORMATS}')
+
+ def __iter__(self):
+ """Returns an iterator object for VideoStream or ImageFolder."""
+ self.count = 0
+ return self
+
+ def __next__(self):
+ """Return next image, path and metadata from dataset."""
+ if self.count == self.nf:
+ raise StopIteration
+ path = self.files[self.count]
+
+ if self.video_flag[self.count]:
+ # Read video
+ self.mode = 'video'
+ for _ in range(self.vid_stride):
+ self.cap.grab()
+ success, im0 = self.cap.retrieve()
+ while not success:
+ self.count += 1
+ self.cap.release()
+ if self.count == self.nf: # last video
+ raise StopIteration
+ path = self.files[self.count]
+ self._new_video(path)
+ success, im0 = self.cap.read()
+
+ self.frame += 1
+ # im0 = self._cv2_rotate(im0) # for use if cv2 autorotation is False
+ s = f'video {self.count + 1}/{self.nf} ({self.frame}/{self.frames}) {path}: '
+
+ else:
+ # Read image
+ self.count += 1
+ im0 = cv2.imread(path) # BGR
+ if im0 is None:
+ raise FileNotFoundError(f'Image Not Found {path}')
+ s = f'image {self.count}/{self.nf} {path}: '
+
+ return [path], [im0], self.cap, s
+
+ def _new_video(self, path):
+ """Create a new video capture object."""
+ self.frame = 0
+ self.cap = cv2.VideoCapture(path)
+ self.frames = int(self.cap.get(cv2.CAP_PROP_FRAME_COUNT) / self.vid_stride)
+ if hasattr(cv2, 'CAP_PROP_ORIENTATION_META'): # cv2<4.6.0 compatibility
+ self.orientation = int(self.cap.get(cv2.CAP_PROP_ORIENTATION_META)) # rotation degrees
+ # Disable auto-orientation due to known issues in https://github.com/ultralytics/yolov5/issues/8493
+ # self.cap.set(cv2.CAP_PROP_ORIENTATION_AUTO, 0)
+
+ def _cv2_rotate(self, im):
+ """Rotate a cv2 video manually."""
+ if self.orientation == 0:
+ return cv2.rotate(im, cv2.ROTATE_90_CLOCKWISE)
+ elif self.orientation == 180:
+ return cv2.rotate(im, cv2.ROTATE_90_COUNTERCLOCKWISE)
+ elif self.orientation == 90:
+ return cv2.rotate(im, cv2.ROTATE_180)
+ return im
+
+ def __len__(self):
+ """Returns the number of files in the object."""
+ return self.nf # number of files
+
+
+class LoadPilAndNumpy:
+
+ def __init__(self, im0, imgsz=640):
+ """Initialize PIL and Numpy Dataloader."""
+ if not isinstance(im0, list):
+ im0 = [im0]
+ self.paths = [getattr(im, 'filename', f'image{i}.jpg') for i, im in enumerate(im0)]
+ self.im0 = [self._single_check(im) for im in im0]
+ self.imgsz = imgsz
+ self.mode = 'image'
+ # Generate fake paths
+ self.bs = len(self.im0)
+
+ @staticmethod
+ def _single_check(im):
+ """Validate and format an image to numpy array."""
+ assert isinstance(im, (Image.Image, np.ndarray)), f'Expected PIL/np.ndarray image type, but got {type(im)}'
+ if isinstance(im, Image.Image):
+ if im.mode != 'RGB':
+ im = im.convert('RGB')
+ im = np.asarray(im)[:, :, ::-1]
+ im = np.ascontiguousarray(im) # contiguous
+ return im
+
+ def __len__(self):
+ """Returns the length of the 'im0' attribute."""
+ return len(self.im0)
+
+ def __next__(self):
+ """Returns batch paths, images, processed images, None, ''."""
+ if self.count == 1: # loop only once as it's batch inference
+ raise StopIteration
+ self.count += 1
+ return self.paths, self.im0, None, ''
+
+ def __iter__(self):
+ """Enables iteration for class LoadPilAndNumpy."""
+ self.count = 0
+ return self
+
+
+class LoadTensor:
+
+ def __init__(self, im0) -> None:
+ self.im0 = self._single_check(im0)
+ self.bs = self.im0.shape[0]
+ self.mode = 'image'
+ self.paths = [getattr(im, 'filename', f'image{i}.jpg') for i, im in enumerate(im0)]
+
+ @staticmethod
+ def _single_check(im, stride=32):
+ """Validate and format an image to torch.Tensor."""
+ s = f'WARNING ⚠️ torch.Tensor inputs should be BCHW i.e. shape(1, 3, 640, 640) ' \
+ f'divisible by stride {stride}. Input shape{tuple(im.shape)} is incompatible.'
+ if len(im.shape) != 4:
+ if len(im.shape) == 3:
+ LOGGER.warning(s)
+ im = im.unsqueeze(0)
+ else:
+ raise ValueError(s)
+ if im.shape[2] % stride or im.shape[3] % stride:
+ raise ValueError(s)
+ if im.max() > 1.0:
+ LOGGER.warning(f'WARNING ⚠️ torch.Tensor inputs should be normalized 0.0-1.0 but max value is {im.max()}. '
+ f'Dividing input by 255.')
+ im = im.float() / 255.0
+
+ return im
+
+ def __iter__(self):
+ """Returns an iterator object."""
+ self.count = 0
+ return self
+
+ def __next__(self):
+ """Return next item in the iterator."""
+ if self.count == 1:
+ raise StopIteration
+ self.count += 1
+ return self.paths, self.im0, None, ''
+
+ def __len__(self):
+ """Returns the batch size."""
+ return self.bs
+
+
+def autocast_list(source):
+ """
+ Merges a list of source of different types into a list of numpy arrays or PIL images
+ """
+ files = []
+ for im in source:
+ if isinstance(im, (str, Path)): # filename or uri
+ files.append(Image.open(requests.get(im, stream=True).raw if str(im).startswith('http') else im))
+ elif isinstance(im, (Image.Image, np.ndarray)): # PIL or np Image
+ files.append(im)
+ else:
+ raise TypeError(f'type {type(im).__name__} is not a supported Ultralytics prediction source type. \n'
+ f'See https://docs.ultralytics.com/modes/predict for supported source types.')
+
+ return files
+
+
+LOADERS = [LoadStreams, LoadPilAndNumpy, LoadImages, LoadScreenshots]
+
+
+def get_best_youtube_url(url, use_pafy=True):
+ """
+ Retrieves the URL of the best quality MP4 video stream from a given YouTube video.
+
+ This function uses the pafy or yt_dlp library to extract the video info from YouTube. It then finds the highest
+ quality MP4 format that has video codec but no audio codec, and returns the URL of this video stream.
+
+ Args:
+ url (str): The URL of the YouTube video.
+ use_pafy (bool): Use the pafy package, default=True, otherwise use yt_dlp package.
+
+ Returns:
+ (str): The URL of the best quality MP4 video stream, or None if no suitable stream is found.
+ """
+ if use_pafy:
+ check_requirements(('pafy', 'youtube_dl==2020.12.2'))
+ import pafy # noqa
+ return pafy.new(url).getbest(preftype='mp4').url
+ else:
+ check_requirements('yt-dlp')
+ import yt_dlp
+ with yt_dlp.YoutubeDL({'quiet': True}) as ydl:
+ info_dict = ydl.extract_info(url, download=False) # extract info
+ for f in info_dict.get('formats', None):
+ if f['vcodec'] != 'none' and f['acodec'] == 'none' and f['ext'] == 'mp4':
+ return f.get('url', None)
+
+
+if __name__ == '__main__':
+ img = cv2.imread(str(ROOT / 'assets/bus.jpg'))
+ dataset = LoadPilAndNumpy(im0=img)
+ for d in dataset:
+ print(d[0])
diff --git a/yolov8/ultralytics/yolo/data/dataloaders/v5augmentations.py b/yolov8/ultralytics/yolo/data/dataloaders/v5augmentations.py
new file mode 100644
index 0000000000000000000000000000000000000000..8e0b3e2fd1ef4446f7b00ac51cf1668fa625205d
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/dataloaders/v5augmentations.py
@@ -0,0 +1,407 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Image augmentation functions
+"""
+
+import math
+import random
+
+import cv2
+import numpy as np
+import torch
+import torchvision.transforms as T
+import torchvision.transforms.functional as TF
+
+from ultralytics.yolo.utils import LOGGER, colorstr
+from ultralytics.yolo.utils.checks import check_version
+from ultralytics.yolo.utils.metrics import bbox_ioa
+from ultralytics.yolo.utils.ops import resample_segments, segment2box, xywhn2xyxy
+
+IMAGENET_MEAN = 0.485, 0.456, 0.406 # RGB mean
+IMAGENET_STD = 0.229, 0.224, 0.225 # RGB standard deviation
+
+
+class Albumentations:
+ # YOLOv5 Albumentations class (optional, only used if package is installed)
+ def __init__(self, size=640):
+ """Instantiate object with image augmentations for YOLOv5."""
+ self.transform = None
+ prefix = colorstr('albumentations: ')
+ try:
+ import albumentations as A
+ check_version(A.__version__, '1.0.3', hard=True) # version requirement
+
+ T = [
+ A.RandomResizedCrop(height=size, width=size, scale=(0.8, 1.0), ratio=(0.9, 1.11), p=0.0),
+ A.Blur(p=0.01),
+ A.MedianBlur(p=0.01),
+ A.ToGray(p=0.01),
+ A.CLAHE(p=0.01),
+ A.RandomBrightnessContrast(p=0.0),
+ A.RandomGamma(p=0.0),
+ A.ImageCompression(quality_lower=75, p=0.0)] # transforms
+ self.transform = A.Compose(T, bbox_params=A.BboxParams(format='yolo', label_fields=['class_labels']))
+
+ LOGGER.info(prefix + ', '.join(f'{x}'.replace('always_apply=False, ', '') for x in T if x.p))
+ except ImportError: # package not installed, skip
+ pass
+ except Exception as e:
+ LOGGER.info(f'{prefix}{e}')
+
+ def __call__(self, im, labels, p=1.0):
+ """Transforms input image and labels with probability 'p'."""
+ if self.transform and random.random() < p:
+ new = self.transform(image=im, bboxes=labels[:, 1:], class_labels=labels[:, 0]) # transformed
+ im, labels = new['image'], np.array([[c, *b] for c, b in zip(new['class_labels'], new['bboxes'])])
+ return im, labels
+
+
+def normalize(x, mean=IMAGENET_MEAN, std=IMAGENET_STD, inplace=False):
+ """Denormalize RGB images x per ImageNet stats in BCHW format, i.e. = (x - mean) / std."""
+ return TF.normalize(x, mean, std, inplace=inplace)
+
+
+def denormalize(x, mean=IMAGENET_MEAN, std=IMAGENET_STD):
+ """Denormalize RGB images x per ImageNet stats in BCHW format, i.e. = x * std + mean."""
+ for i in range(3):
+ x[:, i] = x[:, i] * std[i] + mean[i]
+ return x
+
+
+def augment_hsv(im, hgain=0.5, sgain=0.5, vgain=0.5):
+ """HSV color-space augmentation."""
+ if hgain or sgain or vgain:
+ r = np.random.uniform(-1, 1, 3) * [hgain, sgain, vgain] + 1 # random gains
+ hue, sat, val = cv2.split(cv2.cvtColor(im, cv2.COLOR_BGR2HSV))
+ dtype = im.dtype # uint8
+
+ x = np.arange(0, 256, dtype=r.dtype)
+ lut_hue = ((x * r[0]) % 180).astype(dtype)
+ lut_sat = np.clip(x * r[1], 0, 255).astype(dtype)
+ lut_val = np.clip(x * r[2], 0, 255).astype(dtype)
+
+ im_hsv = cv2.merge((cv2.LUT(hue, lut_hue), cv2.LUT(sat, lut_sat), cv2.LUT(val, lut_val)))
+ cv2.cvtColor(im_hsv, cv2.COLOR_HSV2BGR, dst=im) # no return needed
+
+
+def hist_equalize(im, clahe=True, bgr=False):
+ """Equalize histogram on BGR image 'im' with im.shape(n,m,3) and range 0-255."""
+ yuv = cv2.cvtColor(im, cv2.COLOR_BGR2YUV if bgr else cv2.COLOR_RGB2YUV)
+ if clahe:
+ c = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8, 8))
+ yuv[:, :, 0] = c.apply(yuv[:, :, 0])
+ else:
+ yuv[:, :, 0] = cv2.equalizeHist(yuv[:, :, 0]) # equalize Y channel histogram
+ return cv2.cvtColor(yuv, cv2.COLOR_YUV2BGR if bgr else cv2.COLOR_YUV2RGB) # convert YUV image to RGB
+
+
+def replicate(im, labels):
+ """Replicate labels."""
+ h, w = im.shape[:2]
+ boxes = labels[:, 1:].astype(int)
+ x1, y1, x2, y2 = boxes.T
+ s = ((x2 - x1) + (y2 - y1)) / 2 # side length (pixels)
+ for i in s.argsort()[:round(s.size * 0.5)]: # smallest indices
+ x1b, y1b, x2b, y2b = boxes[i]
+ bh, bw = y2b - y1b, x2b - x1b
+ yc, xc = int(random.uniform(0, h - bh)), int(random.uniform(0, w - bw)) # offset x, y
+ x1a, y1a, x2a, y2a = [xc, yc, xc + bw, yc + bh]
+ im[y1a:y2a, x1a:x2a] = im[y1b:y2b, x1b:x2b] # im4[ymin:ymax, xmin:xmax]
+ labels = np.append(labels, [[labels[i, 0], x1a, y1a, x2a, y2a]], axis=0)
+
+ return im, labels
+
+
+def letterbox(im, new_shape=(640, 640), color=(114, 114, 114), auto=True, scaleFill=False, scaleup=True, stride=32):
+ """Resize and pad image while meeting stride-multiple constraints."""
+ shape = im.shape[:2] # current shape [height, width]
+ if isinstance(new_shape, int):
+ new_shape = (new_shape, new_shape)
+
+ # Scale ratio (new / old)
+ r = min(new_shape[0] / shape[0], new_shape[1] / shape[1])
+ if not scaleup: # only scale down, do not scale up (for better val mAP)
+ r = min(r, 1.0)
+
+ # Compute padding
+ ratio = r, r # width, height ratios
+ new_unpad = int(round(shape[1] * r)), int(round(shape[0] * r))
+ dw, dh = new_shape[1] - new_unpad[0], new_shape[0] - new_unpad[1] # wh padding
+ if auto: # minimum rectangle
+ dw, dh = np.mod(dw, stride), np.mod(dh, stride) # wh padding
+ elif scaleFill: # stretch
+ dw, dh = 0.0, 0.0
+ new_unpad = (new_shape[1], new_shape[0])
+ ratio = new_shape[1] / shape[1], new_shape[0] / shape[0] # width, height ratios
+
+ dw /= 2 # divide padding into 2 sides
+ dh /= 2
+
+ if shape[::-1] != new_unpad: # resize
+ im = cv2.resize(im, new_unpad, interpolation=cv2.INTER_LINEAR)
+ top, bottom = int(round(dh - 0.1)), int(round(dh + 0.1))
+ left, right = int(round(dw - 0.1)), int(round(dw + 0.1))
+ im = cv2.copyMakeBorder(im, top, bottom, left, right, cv2.BORDER_CONSTANT, value=color) # add border
+ return im, ratio, (dw, dh)
+
+
+def random_perspective(im,
+ targets=(),
+ segments=(),
+ degrees=10,
+ translate=.1,
+ scale=.1,
+ shear=10,
+ perspective=0.0,
+ border=(0, 0)):
+ # torchvision.transforms.RandomAffine(degrees=(-10, 10), translate=(0.1, 0.1), scale=(0.9, 1.1), shear=(-10, 10))
+ # targets = [cls, xyxy]
+
+ height = im.shape[0] + border[0] * 2 # shape(h,w,c)
+ width = im.shape[1] + border[1] * 2
+
+ # Center
+ C = np.eye(3)
+ C[0, 2] = -im.shape[1] / 2 # x translation (pixels)
+ C[1, 2] = -im.shape[0] / 2 # y translation (pixels)
+
+ # Perspective
+ P = np.eye(3)
+ P[2, 0] = random.uniform(-perspective, perspective) # x perspective (about y)
+ P[2, 1] = random.uniform(-perspective, perspective) # y perspective (about x)
+
+ # Rotation and Scale
+ R = np.eye(3)
+ a = random.uniform(-degrees, degrees)
+ # a += random.choice([-180, -90, 0, 90]) # add 90deg rotations to small rotations
+ s = random.uniform(1 - scale, 1 + scale)
+ # s = 2 ** random.uniform(-scale, scale)
+ R[:2] = cv2.getRotationMatrix2D(angle=a, center=(0, 0), scale=s)
+
+ # Shear
+ S = np.eye(3)
+ S[0, 1] = math.tan(random.uniform(-shear, shear) * math.pi / 180) # x shear (deg)
+ S[1, 0] = math.tan(random.uniform(-shear, shear) * math.pi / 180) # y shear (deg)
+
+ # Translation
+ T = np.eye(3)
+ T[0, 2] = random.uniform(0.5 - translate, 0.5 + translate) * width # x translation (pixels)
+ T[1, 2] = random.uniform(0.5 - translate, 0.5 + translate) * height # y translation (pixels)
+
+ # Combined rotation matrix
+ M = T @ S @ R @ P @ C # order of operations (right to left) is IMPORTANT
+ if (border[0] != 0) or (border[1] != 0) or (M != np.eye(3)).any(): # image changed
+ if perspective:
+ im = cv2.warpPerspective(im, M, dsize=(width, height), borderValue=(114, 114, 114))
+ else: # affine
+ im = cv2.warpAffine(im, M[:2], dsize=(width, height), borderValue=(114, 114, 114))
+
+ # Visualize
+ # import matplotlib.pyplot as plt
+ # ax = plt.subplots(1, 2, figsize=(12, 6))[1].ravel()
+ # ax[0].imshow(im[:, :, ::-1]) # base
+ # ax[1].imshow(im2[:, :, ::-1]) # warped
+
+ # Transform label coordinates
+ n = len(targets)
+ if n:
+ use_segments = any(x.any() for x in segments)
+ new = np.zeros((n, 4))
+ if use_segments: # warp segments
+ segments = resample_segments(segments) # upsample
+ for i, segment in enumerate(segments):
+ xy = np.ones((len(segment), 3))
+ xy[:, :2] = segment
+ xy = xy @ M.T # transform
+ xy = xy[:, :2] / xy[:, 2:3] if perspective else xy[:, :2] # perspective rescale or affine
+
+ # Clip
+ new[i] = segment2box(xy, width, height)
+
+ else: # warp boxes
+ xy = np.ones((n * 4, 3))
+ xy[:, :2] = targets[:, [1, 2, 3, 4, 1, 4, 3, 2]].reshape(n * 4, 2) # x1y1, x2y2, x1y2, x2y1
+ xy = xy @ M.T # transform
+ xy = (xy[:, :2] / xy[:, 2:3] if perspective else xy[:, :2]).reshape(n, 8) # perspective rescale or affine
+
+ # Create new boxes
+ x = xy[:, [0, 2, 4, 6]]
+ y = xy[:, [1, 3, 5, 7]]
+ new = np.concatenate((x.min(1), y.min(1), x.max(1), y.max(1))).reshape(4, n).T
+
+ # Clip
+ new[:, [0, 2]] = new[:, [0, 2]].clip(0, width)
+ new[:, [1, 3]] = new[:, [1, 3]].clip(0, height)
+
+ # Filter candidates
+ i = box_candidates(box1=targets[:, 1:5].T * s, box2=new.T, area_thr=0.01 if use_segments else 0.10)
+ targets = targets[i]
+ targets[:, 1:5] = new[i]
+
+ return im, targets
+
+
+def copy_paste(im, labels, segments, p=0.5):
+ """Implement Copy-Paste augmentation https://arxiv.org/abs/2012.07177, labels as nx5 np.array(cls, xyxy)."""
+ n = len(segments)
+ if p and n:
+ h, w, c = im.shape # height, width, channels
+ im_new = np.zeros(im.shape, np.uint8)
+
+ # Calculate ioa first then select indexes randomly
+ boxes = np.stack([w - labels[:, 3], labels[:, 2], w - labels[:, 1], labels[:, 4]], axis=-1) # (n, 4)
+ ioa = bbox_ioa(boxes, labels[:, 1:5]) # intersection over area
+ indexes = np.nonzero((ioa < 0.30).all(1))[0] # (N, )
+ n = len(indexes)
+ for j in random.sample(list(indexes), k=round(p * n)):
+ l, box, s = labels[j], boxes[j], segments[j]
+ labels = np.concatenate((labels, [[l[0], *box]]), 0)
+ segments.append(np.concatenate((w - s[:, 0:1], s[:, 1:2]), 1))
+ cv2.drawContours(im_new, [segments[j].astype(np.int32)], -1, (1, 1, 1), cv2.FILLED)
+
+ result = cv2.flip(im, 1) # augment segments (flip left-right)
+ i = cv2.flip(im_new, 1).astype(bool)
+ im[i] = result[i] # cv2.imwrite('debug.jpg', im) # debug
+
+ return im, labels, segments
+
+
+def cutout(im, labels, p=0.5):
+ """Applies image cutout augmentation https://arxiv.org/abs/1708.04552."""
+ if random.random() < p:
+ h, w = im.shape[:2]
+ scales = [0.5] * 1 + [0.25] * 2 + [0.125] * 4 + [0.0625] * 8 + [0.03125] * 16 # image size fraction
+ for s in scales:
+ mask_h = random.randint(1, int(h * s)) # create random masks
+ mask_w = random.randint(1, int(w * s))
+
+ # Box
+ xmin = max(0, random.randint(0, w) - mask_w // 2)
+ ymin = max(0, random.randint(0, h) - mask_h // 2)
+ xmax = min(w, xmin + mask_w)
+ ymax = min(h, ymin + mask_h)
+
+ # Apply random color mask
+ im[ymin:ymax, xmin:xmax] = [random.randint(64, 191) for _ in range(3)]
+
+ # Return unobscured labels
+ if len(labels) and s > 0.03:
+ box = np.array([[xmin, ymin, xmax, ymax]], dtype=np.float32)
+ ioa = bbox_ioa(box, xywhn2xyxy(labels[:, 1:5], w, h))[0] # intersection over area
+ labels = labels[ioa < 0.60] # remove >60% obscured labels
+
+ return labels
+
+
+def mixup(im, labels, im2, labels2):
+ """Applies MixUp augmentation https://arxiv.org/pdf/1710.09412.pdf."""
+ r = np.random.beta(32.0, 32.0) # mixup ratio, alpha=beta=32.0
+ im = (im * r + im2 * (1 - r)).astype(np.uint8)
+ labels = np.concatenate((labels, labels2), 0)
+ return im, labels
+
+
+def box_candidates(box1, box2, wh_thr=2, ar_thr=100, area_thr=0.1, eps=1e-16): # box1(4,n), box2(4,n)
+ # Compute candidate boxes: box1 before augment, box2 after augment, wh_thr (pixels), aspect_ratio_thr, area_ratio
+ w1, h1 = box1[2] - box1[0], box1[3] - box1[1]
+ w2, h2 = box2[2] - box2[0], box2[3] - box2[1]
+ ar = np.maximum(w2 / (h2 + eps), h2 / (w2 + eps)) # aspect ratio
+ return (w2 > wh_thr) & (h2 > wh_thr) & (w2 * h2 / (w1 * h1 + eps) > area_thr) & (ar < ar_thr) # candidates
+
+
+def classify_albumentations(
+ augment=True,
+ size=224,
+ scale=(0.08, 1.0),
+ ratio=(0.75, 1.0 / 0.75), # 0.75, 1.33
+ hflip=0.5,
+ vflip=0.0,
+ jitter=0.4,
+ mean=IMAGENET_MEAN,
+ std=IMAGENET_STD,
+ auto_aug=False):
+ # YOLOv5 classification Albumentations (optional, only used if package is installed)
+ prefix = colorstr('albumentations: ')
+ try:
+ import albumentations as A
+ from albumentations.pytorch import ToTensorV2
+ check_version(A.__version__, '1.0.3', hard=True) # version requirement
+ if augment: # Resize and crop
+ T = [A.RandomResizedCrop(height=size, width=size, scale=scale, ratio=ratio)]
+ if auto_aug:
+ # TODO: implement AugMix, AutoAug & RandAug in albumentation
+ LOGGER.info(f'{prefix}auto augmentations are currently not supported')
+ else:
+ if hflip > 0:
+ T += [A.HorizontalFlip(p=hflip)]
+ if vflip > 0:
+ T += [A.VerticalFlip(p=vflip)]
+ if jitter > 0:
+ jitter = float(jitter)
+ T += [A.ColorJitter(jitter, jitter, jitter, 0)] # brightness, contrast, satuaration, 0 hue
+ else: # Use fixed crop for eval set (reproducibility)
+ T = [A.SmallestMaxSize(max_size=size), A.CenterCrop(height=size, width=size)]
+ T += [A.Normalize(mean=mean, std=std), ToTensorV2()] # Normalize and convert to Tensor
+ LOGGER.info(prefix + ', '.join(f'{x}'.replace('always_apply=False, ', '') for x in T if x.p))
+ return A.Compose(T)
+
+ except ImportError: # package not installed, skip
+ LOGGER.warning(f'{prefix}⚠️ not found, install with `pip install albumentations` (recommended)')
+ except Exception as e:
+ LOGGER.info(f'{prefix}{e}')
+
+
+def classify_transforms(size=224):
+ """Transforms to apply if albumentations not installed."""
+ assert isinstance(size, int), f'ERROR: classify_transforms size {size} must be integer, not (list, tuple)'
+ # T.Compose([T.ToTensor(), T.Resize(size), T.CenterCrop(size), T.Normalize(IMAGENET_MEAN, IMAGENET_STD)])
+ return T.Compose([CenterCrop(size), ToTensor(), T.Normalize(IMAGENET_MEAN, IMAGENET_STD)])
+
+
+class LetterBox:
+ # YOLOv5 LetterBox class for image preprocessing, i.e. T.Compose([LetterBox(size), ToTensor()])
+ def __init__(self, size=(640, 640), auto=False, stride=32):
+ """Resizes and crops an image to a specified size for YOLOv5 preprocessing."""
+ super().__init__()
+ self.h, self.w = (size, size) if isinstance(size, int) else size
+ self.auto = auto # pass max size integer, automatically solve for short side using stride
+ self.stride = stride # used with auto
+
+ def __call__(self, im): # im = np.array HWC
+ imh, imw = im.shape[:2]
+ r = min(self.h / imh, self.w / imw) # ratio of new/old
+ h, w = round(imh * r), round(imw * r) # resized image
+ hs, ws = (math.ceil(x / self.stride) * self.stride for x in (h, w)) if self.auto else self.h, self.w
+ top, left = round((hs - h) / 2 - 0.1), round((ws - w) / 2 - 0.1)
+ im_out = np.full((self.h, self.w, 3), 114, dtype=im.dtype)
+ im_out[top:top + h, left:left + w] = cv2.resize(im, (w, h), interpolation=cv2.INTER_LINEAR)
+ return im_out
+
+
+class CenterCrop:
+ # YOLOv5 CenterCrop class for image preprocessing, i.e. T.Compose([CenterCrop(size), ToTensor()])
+ def __init__(self, size=640):
+ """Converts input image into tensor for YOLOv5 processing."""
+ super().__init__()
+ self.h, self.w = (size, size) if isinstance(size, int) else size
+
+ def __call__(self, im): # im = np.array HWC
+ imh, imw = im.shape[:2]
+ m = min(imh, imw) # min dimension
+ top, left = (imh - m) // 2, (imw - m) // 2
+ return cv2.resize(im[top:top + m, left:left + m], (self.w, self.h), interpolation=cv2.INTER_LINEAR)
+
+
+class ToTensor:
+ # YOLOv5 ToTensor class for image preprocessing, i.e. T.Compose([LetterBox(size), ToTensor()])
+ def __init__(self, half=False):
+ """Initialize ToTensor class for YOLOv5 image preprocessing."""
+ super().__init__()
+ self.half = half
+
+ def __call__(self, im): # im = np.array HWC in BGR order
+ im = np.ascontiguousarray(im.transpose((2, 0, 1))[::-1]) # HWC to CHW -> BGR to RGB -> contiguous
+ im = torch.from_numpy(im) # to torch
+ im = im.half() if self.half else im.float() # uint8 to fp16/32
+ im /= 255.0 # 0-255 to 0.0-1.0
+ return im
diff --git a/yolov8/ultralytics/yolo/data/dataloaders/v5loader.py b/yolov8/ultralytics/yolo/data/dataloaders/v5loader.py
new file mode 100644
index 0000000000000000000000000000000000000000..96549ddebe359cacaa0bfdbecc7f36bf71fce8ab
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/dataloaders/v5loader.py
@@ -0,0 +1,1109 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Dataloaders and dataset utils
+"""
+
+import contextlib
+import glob
+import hashlib
+import math
+import os
+import random
+import shutil
+import time
+from itertools import repeat
+from multiprocessing.pool import ThreadPool
+from pathlib import Path
+from threading import Thread
+from urllib.parse import urlparse
+
+import cv2
+import numpy as np
+import psutil
+import torch
+import torchvision
+from PIL import ExifTags, Image, ImageOps
+from torch.utils.data import DataLoader, Dataset, dataloader, distributed
+from tqdm import tqdm
+
+from ultralytics.yolo.utils import (DATASETS_DIR, LOGGER, NUM_THREADS, TQDM_BAR_FORMAT, is_colab, is_dir_writeable,
+ is_kaggle)
+from ultralytics.yolo.utils.checks import check_requirements
+from ultralytics.yolo.utils.ops import clean_str, segments2boxes, xyn2xy, xywh2xyxy, xywhn2xyxy, xyxy2xywhn
+from ultralytics.yolo.utils.torch_utils import torch_distributed_zero_first
+
+from .v5augmentations import (Albumentations, augment_hsv, classify_albumentations, classify_transforms, copy_paste,
+ letterbox, mixup, random_perspective)
+
+# Parameters
+HELP_URL = 'See https://docs.ultralytics.com/yolov5/tutorials/train_custom_data'
+IMG_FORMATS = 'bmp', 'dng', 'jpeg', 'jpg', 'mpo', 'png', 'tif', 'tiff', 'webp', 'pfm' # include image suffixes
+VID_FORMATS = 'asf', 'avi', 'gif', 'm4v', 'mkv', 'mov', 'mp4', 'mpeg', 'mpg', 'ts', 'wmv' # include video suffixes
+LOCAL_RANK = int(os.getenv('LOCAL_RANK', -1)) # https://pytorch.org/docs/stable/elastic/run.html
+RANK = int(os.getenv('RANK', -1))
+PIN_MEMORY = str(os.getenv('PIN_MEMORY', True)).lower() == 'true' # global pin_memory for dataloaders
+
+# Get orientation exif tag
+for orientation in ExifTags.TAGS.keys():
+ if ExifTags.TAGS[orientation] == 'Orientation':
+ break
+
+
+def get_hash(paths):
+ """Returns a single hash value of a list of paths (files or dirs)."""
+ size = sum(os.path.getsize(p) for p in paths if os.path.exists(p)) # sizes
+ h = hashlib.sha256(str(size).encode()) # hash sizes
+ h.update(''.join(paths).encode()) # hash paths
+ return h.hexdigest() # return hash
+
+
+def exif_size(img):
+ """Returns exif-corrected PIL size."""
+ s = img.size # (width, height)
+ with contextlib.suppress(Exception):
+ rotation = dict(img._getexif().items())[orientation]
+ if rotation in [6, 8]: # rotation 270 or 90
+ s = (s[1], s[0])
+ return s
+
+
+def exif_transpose(image):
+ """
+ Transpose a PIL image accordingly if it has an EXIF Orientation tag.
+ Inplace version of https://github.com/python-pillow/Pillow/blob/master/src/PIL/ImageOps.py exif_transpose()
+
+ :param image: The image to transpose.
+ :return: An image.
+ """
+ exif = image.getexif()
+ orientation = exif.get(0x0112, 1) # default 1
+ if orientation > 1:
+ method = {
+ 2: Image.FLIP_LEFT_RIGHT,
+ 3: Image.ROTATE_180,
+ 4: Image.FLIP_TOP_BOTTOM,
+ 5: Image.TRANSPOSE,
+ 6: Image.ROTATE_270,
+ 7: Image.TRANSVERSE,
+ 8: Image.ROTATE_90}.get(orientation)
+ if method is not None:
+ image = image.transpose(method)
+ del exif[0x0112]
+ image.info['exif'] = exif.tobytes()
+ return image
+
+
+def seed_worker(worker_id):
+ """Set dataloader worker seed https://pytorch.org/docs/stable/notes/randomness.html#dataloader."""
+ worker_seed = torch.initial_seed() % 2 ** 32
+ np.random.seed(worker_seed)
+ random.seed(worker_seed)
+
+
+def create_dataloader(path,
+ imgsz,
+ batch_size,
+ stride,
+ single_cls=False,
+ hyp=None,
+ augment=False,
+ cache=False,
+ pad=0.0,
+ rect=False,
+ rank=-1,
+ workers=8,
+ image_weights=False,
+ close_mosaic=False,
+ min_items=0,
+ prefix='',
+ shuffle=False,
+ seed=0):
+ if rect and shuffle:
+ LOGGER.warning('WARNING ⚠️ --rect is incompatible with DataLoader shuffle, setting shuffle=False')
+ shuffle = False
+ with torch_distributed_zero_first(rank): # init dataset *.cache only once if DDP
+ dataset = LoadImagesAndLabels(
+ path,
+ imgsz,
+ batch_size,
+ augment=augment, # augmentation
+ hyp=hyp, # hyperparameters
+ rect=rect, # rectangular batches
+ cache_images=cache,
+ single_cls=single_cls,
+ stride=int(stride),
+ pad=pad,
+ image_weights=image_weights,
+ min_items=min_items,
+ prefix=prefix)
+
+ batch_size = min(batch_size, len(dataset))
+ nd = torch.cuda.device_count() # number of CUDA devices
+ nw = min([os.cpu_count() // max(nd, 1), batch_size if batch_size > 1 else 0, workers]) # number of workers
+ sampler = None if rank == -1 else distributed.DistributedSampler(dataset, shuffle=shuffle)
+ loader = DataLoader if image_weights or close_mosaic else InfiniteDataLoader # DataLoader allows attribute updates
+ generator = torch.Generator()
+ generator.manual_seed(6148914691236517205 + seed + RANK)
+ return loader(dataset,
+ batch_size=batch_size,
+ shuffle=shuffle and sampler is None,
+ num_workers=nw,
+ sampler=sampler,
+ pin_memory=PIN_MEMORY,
+ collate_fn=LoadImagesAndLabels.collate_fn,
+ worker_init_fn=seed_worker,
+ generator=generator), dataset
+
+
+class InfiniteDataLoader(dataloader.DataLoader):
+ """Dataloader that reuses workers
+
+ Uses same syntax as vanilla DataLoader
+ """
+
+ def __init__(self, *args, **kwargs):
+ """Dataloader that reuses workers for same syntax as vanilla DataLoader."""
+ super().__init__(*args, **kwargs)
+ object.__setattr__(self, 'batch_sampler', _RepeatSampler(self.batch_sampler))
+ self.iterator = super().__iter__()
+
+ def __len__(self):
+ """Returns the length of batch_sampler's sampler."""
+ return len(self.batch_sampler.sampler)
+
+ def __iter__(self):
+ """Creates a sampler that infinitely repeats."""
+ for _ in range(len(self)):
+ yield next(self.iterator)
+
+
+class _RepeatSampler:
+ """Sampler that repeats forever
+
+ Args:
+ sampler (Dataset.sampler): The sampler to repeat.
+ """
+
+ def __init__(self, sampler):
+ """Sampler that repeats dataset samples infinitely."""
+ self.sampler = sampler
+
+ def __iter__(self):
+ """Infinite loop iterating over a given sampler."""
+ while True:
+ yield from iter(self.sampler)
+
+
+class LoadScreenshots:
+ # YOLOv5 screenshot dataloader, i.e. `python detect.py --source "screen 0 100 100 512 256"`
+ def __init__(self, source, img_size=640, stride=32, auto=True, transforms=None):
+ """source = [screen_number left top width height] (pixels)."""
+ check_requirements('mss')
+ import mss
+
+ source, *params = source.split()
+ self.screen, left, top, width, height = 0, None, None, None, None # default to full screen 0
+ if len(params) == 1:
+ self.screen = int(params[0])
+ elif len(params) == 4:
+ left, top, width, height = (int(x) for x in params)
+ elif len(params) == 5:
+ self.screen, left, top, width, height = (int(x) for x in params)
+ self.img_size = img_size
+ self.stride = stride
+ self.transforms = transforms
+ self.auto = auto
+ self.mode = 'stream'
+ self.frame = 0
+ self.sct = mss.mss()
+
+ # Parse monitor shape
+ monitor = self.sct.monitors[self.screen]
+ self.top = monitor['top'] if top is None else (monitor['top'] + top)
+ self.left = monitor['left'] if left is None else (monitor['left'] + left)
+ self.width = width or monitor['width']
+ self.height = height or monitor['height']
+ self.monitor = {'left': self.left, 'top': self.top, 'width': self.width, 'height': self.height}
+
+ def __iter__(self):
+ """Iterates over objects with the same structure as the monitor attribute."""
+ return self
+
+ def __next__(self):
+ """mss screen capture: get raw pixels from the screen as np array."""
+ im0 = np.array(self.sct.grab(self.monitor))[:, :, :3] # [:, :, :3] BGRA to BGR
+ s = f'screen {self.screen} (LTWH): {self.left},{self.top},{self.width},{self.height}: '
+
+ if self.transforms:
+ im = self.transforms(im0) # transforms
+ else:
+ im = letterbox(im0, self.img_size, stride=self.stride, auto=self.auto)[0] # padded resize
+ im = im.transpose((2, 0, 1))[::-1] # HWC to CHW, BGR to RGB
+ im = np.ascontiguousarray(im) # contiguous
+ self.frame += 1
+ return str(self.screen), im, im0, None, s # screen, img, original img, im0s, s
+
+
+class LoadImages:
+ # YOLOv5 image/video dataloader, i.e. `python detect.py --source image.jpg/vid.mp4`
+ def __init__(self, path, img_size=640, stride=32, auto=True, transforms=None, vid_stride=1):
+ """Initialize instance variables and check for valid input."""
+ if isinstance(path, str) and Path(path).suffix == '.txt': # *.txt file with img/vid/dir on each line
+ path = Path(path).read_text().rsplit()
+ files = []
+ for p in sorted(path) if isinstance(path, (list, tuple)) else [path]:
+ p = str(Path(p).resolve())
+ if '*' in p:
+ files.extend(sorted(glob.glob(p, recursive=True))) # glob
+ elif os.path.isdir(p):
+ files.extend(sorted(glob.glob(os.path.join(p, '*.*')))) # dir
+ elif os.path.isfile(p):
+ files.append(p) # files
+ else:
+ raise FileNotFoundError(f'{p} does not exist')
+
+ images = [x for x in files if x.split('.')[-1].lower() in IMG_FORMATS]
+ videos = [x for x in files if x.split('.')[-1].lower() in VID_FORMATS]
+ ni, nv = len(images), len(videos)
+
+ self.img_size = img_size
+ self.stride = stride
+ self.files = images + videos
+ self.nf = ni + nv # number of files
+ self.video_flag = [False] * ni + [True] * nv
+ self.mode = 'image'
+ self.auto = auto
+ self.transforms = transforms # optional
+ self.vid_stride = vid_stride # video frame-rate stride
+ if any(videos):
+ self._new_video(videos[0]) # new video
+ else:
+ self.cap = None
+ assert self.nf > 0, f'No images or videos found in {p}. ' \
+ f'Supported formats are:\nimages: {IMG_FORMATS}\nvideos: {VID_FORMATS}'
+
+ def __iter__(self):
+ """Returns an iterator object for iterating over images or videos found in a directory."""
+ self.count = 0
+ return self
+
+ def __next__(self):
+ """Iterator's next item, performs transformation on image and returns path, transformed image, original image, capture and size."""
+ if self.count == self.nf:
+ raise StopIteration
+ path = self.files[self.count]
+
+ if self.video_flag[self.count]:
+ # Read video
+ self.mode = 'video'
+ for _ in range(self.vid_stride):
+ self.cap.grab()
+ ret_val, im0 = self.cap.retrieve()
+ while not ret_val:
+ self.count += 1
+ self.cap.release()
+ if self.count == self.nf: # last video
+ raise StopIteration
+ path = self.files[self.count]
+ self._new_video(path)
+ ret_val, im0 = self.cap.read()
+
+ self.frame += 1
+ # im0 = self._cv2_rotate(im0) # for use if cv2 autorotation is False
+ s = f'video {self.count + 1}/{self.nf} ({self.frame}/{self.frames}) {path}: '
+
+ else:
+ # Read image
+ self.count += 1
+ im0 = cv2.imread(path) # BGR
+ assert im0 is not None, f'Image Not Found {path}'
+ s = f'image {self.count}/{self.nf} {path}: '
+
+ if self.transforms:
+ im = self.transforms(im0) # transforms
+ else:
+ im = letterbox(im0, self.img_size, stride=self.stride, auto=self.auto)[0] # padded resize
+ im = im.transpose((2, 0, 1))[::-1] # HWC to CHW, BGR to RGB
+ im = np.ascontiguousarray(im) # contiguous
+
+ return path, im, im0, self.cap, s
+
+ def _new_video(self, path):
+ """Create a new video capture object."""
+ self.frame = 0
+ self.cap = cv2.VideoCapture(path)
+ self.frames = int(self.cap.get(cv2.CAP_PROP_FRAME_COUNT) / self.vid_stride)
+ self.orientation = int(self.cap.get(cv2.CAP_PROP_ORIENTATION_META)) # rotation degrees
+ # self.cap.set(cv2.CAP_PROP_ORIENTATION_AUTO, 0) # disable https://github.com/ultralytics/yolov5/issues/8493
+
+ def _cv2_rotate(self, im):
+ """Rotate a cv2 video manually."""
+ if self.orientation == 0:
+ return cv2.rotate(im, cv2.ROTATE_90_CLOCKWISE)
+ elif self.orientation == 180:
+ return cv2.rotate(im, cv2.ROTATE_90_COUNTERCLOCKWISE)
+ elif self.orientation == 90:
+ return cv2.rotate(im, cv2.ROTATE_180)
+ return im
+
+ def __len__(self):
+ """Returns the number of files in the class instance."""
+ return self.nf # number of files
+
+
+class LoadStreams:
+ # YOLOv5 streamloader, i.e. `python detect.py --source 'rtsp://example.com/media.mp4' # RTSP, RTMP, HTTP streams`
+ def __init__(self, sources='file.streams', img_size=640, stride=32, auto=True, transforms=None, vid_stride=1):
+ """Initialize YOLO detector with optional transforms and check input shapes."""
+ torch.backends.cudnn.benchmark = True # faster for fixed-size inference
+ self.mode = 'stream'
+ self.img_size = img_size
+ self.stride = stride
+ self.vid_stride = vid_stride # video frame-rate stride
+ sources = Path(sources).read_text().rsplit() if os.path.isfile(sources) else [sources]
+ n = len(sources)
+ self.sources = [clean_str(x) for x in sources] # clean source names for later
+ self.imgs, self.fps, self.frames, self.threads = [None] * n, [0] * n, [0] * n, [None] * n
+ for i, s in enumerate(sources): # index, source
+ # Start thread to read frames from video stream
+ st = f'{i + 1}/{n}: {s}... '
+ if urlparse(s).hostname in ('www.youtube.com', 'youtube.com', 'youtu.be'): # if source is YouTube video
+ # YouTube format i.e. 'https://www.youtube.com/watch?v=Zgi9g1ksQHc' or 'https://youtu.be/Zgi9g1ksQHc'
+ check_requirements(('pafy', 'youtube_dl==2020.12.2'))
+ import pafy
+ s = pafy.new(s).getbest(preftype='mp4').url # YouTube URL
+ s = eval(s) if s.isnumeric() else s # i.e. s = '0' local webcam
+ if s == 0:
+ assert not is_colab(), '--source 0 webcam unsupported on Colab. Rerun command in a local environment.'
+ assert not is_kaggle(), '--source 0 webcam unsupported on Kaggle. Rerun command in a local environment.'
+ cap = cv2.VideoCapture(s)
+ assert cap.isOpened(), f'{st}Failed to open {s}'
+ w = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
+ h = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
+ fps = cap.get(cv2.CAP_PROP_FPS) # warning: may return 0 or nan
+ self.frames[i] = max(int(cap.get(cv2.CAP_PROP_FRAME_COUNT)), 0) or float('inf') # infinite stream fallback
+ self.fps[i] = max((fps if math.isfinite(fps) else 0) % 100, 0) or 30 # 30 FPS fallback
+
+ _, self.imgs[i] = cap.read() # guarantee first frame
+ self.threads[i] = Thread(target=self.update, args=([i, cap, s]), daemon=True)
+ LOGGER.info(f'{st} Success ({self.frames[i]} frames {w}x{h} at {self.fps[i]:.2f} FPS)')
+ self.threads[i].start()
+ LOGGER.info('') # newline
+
+ # Check for common shapes
+ s = np.stack([letterbox(x, img_size, stride=stride, auto=auto)[0].shape for x in self.imgs])
+ self.rect = np.unique(s, axis=0).shape[0] == 1 # rect inference if all shapes equal
+ self.auto = auto and self.rect
+ self.transforms = transforms # optional
+ if not self.rect:
+ LOGGER.warning('WARNING ⚠️ Stream shapes differ. For optimal performance supply similarly-shaped streams.')
+
+ def update(self, i, cap, stream):
+ """Read stream `i` frames in daemon thread."""
+ n, f = 0, self.frames[i] # frame number, frame array
+ while cap.isOpened() and n < f:
+ n += 1
+ cap.grab() # .read() = .grab() followed by .retrieve()
+ if n % self.vid_stride == 0:
+ success, im = cap.retrieve()
+ if success:
+ self.imgs[i] = im
+ else:
+ LOGGER.warning('WARNING ⚠️ Video stream unresponsive, please check your IP camera connection.')
+ self.imgs[i] = np.zeros_like(self.imgs[i])
+ cap.open(stream) # re-open stream if signal was lost
+ time.sleep(0.0) # wait time
+
+ def __iter__(self):
+ """Iterator that returns the class instance."""
+ self.count = -1
+ return self
+
+ def __next__(self):
+ """Return a tuple containing transformed and resized image data."""
+ self.count += 1
+ if not all(x.is_alive() for x in self.threads) or cv2.waitKey(1) == ord('q'): # q to quit
+ cv2.destroyAllWindows()
+ raise StopIteration
+
+ im0 = self.imgs.copy()
+ if self.transforms:
+ im = np.stack([self.transforms(x) for x in im0]) # transforms
+ else:
+ im = np.stack([letterbox(x, self.img_size, stride=self.stride, auto=self.auto)[0] for x in im0]) # resize
+ im = im[..., ::-1].transpose((0, 3, 1, 2)) # BGR to RGB, BHWC to BCHW
+ im = np.ascontiguousarray(im) # contiguous
+
+ return self.sources, im, im0, None, ''
+
+ def __len__(self):
+ """Returns the number of sources as the length of the object."""
+ return len(self.sources) # 1E12 frames = 32 streams at 30 FPS for 30 years
+
+
+def img2label_paths(img_paths):
+ """Define label paths as a function of image paths."""
+ sa, sb = f'{os.sep}images{os.sep}', f'{os.sep}labels{os.sep}' # /images/, /labels/ substrings
+ return [sb.join(x.rsplit(sa, 1)).rsplit('.', 1)[0] + '.txt' for x in img_paths]
+
+
+class LoadImagesAndLabels(Dataset):
+ """YOLOv5 train_loader/val_loader, loads images and labels for training and validation."""
+ cache_version = 0.6 # dataset labels *.cache version
+ rand_interp_methods = [cv2.INTER_NEAREST, cv2.INTER_LINEAR, cv2.INTER_CUBIC, cv2.INTER_AREA, cv2.INTER_LANCZOS4]
+
+ def __init__(self,
+ path,
+ img_size=640,
+ batch_size=16,
+ augment=False,
+ hyp=None,
+ rect=False,
+ image_weights=False,
+ cache_images=False,
+ single_cls=False,
+ stride=32,
+ pad=0.0,
+ min_items=0,
+ prefix=''):
+ self.img_size = img_size
+ self.augment = augment
+ self.hyp = hyp
+ self.image_weights = image_weights
+ self.rect = False if image_weights else rect
+ self.mosaic = self.augment and not self.rect # load 4 images at a time into a mosaic (only during training)
+ self.mosaic_border = [-img_size // 2, -img_size // 2]
+ self.stride = stride
+ self.path = path
+ self.albumentations = Albumentations(size=img_size) if augment else None
+
+ try:
+ f = [] # image files
+ for p in path if isinstance(path, list) else [path]:
+ p = Path(p) # os-agnostic
+ if p.is_dir(): # dir
+ f += glob.glob(str(p / '**' / '*.*'), recursive=True)
+ # f = list(p.rglob('*.*')) # pathlib
+ elif p.is_file(): # file
+ with open(p) as t:
+ t = t.read().strip().splitlines()
+ parent = str(p.parent) + os.sep
+ f += [x.replace('./', parent, 1) if x.startswith('./') else x for x in t] # to global path
+ # f += [p.parent / x.lstrip(os.sep) for x in t] # to global path (pathlib)
+ else:
+ raise FileNotFoundError(f'{prefix}{p} does not exist')
+ self.im_files = sorted(x.replace('/', os.sep) for x in f if x.split('.')[-1].lower() in IMG_FORMATS)
+ # self.img_files = sorted([x for x in f if x.suffix[1:].lower() in IMG_FORMATS]) # pathlib
+ assert self.im_files, f'{prefix}No images found'
+ except Exception as e:
+ raise FileNotFoundError(f'{prefix}Error loading data from {path}: {e}\n{HELP_URL}') from e
+
+ # Check cache
+ self.label_files = img2label_paths(self.im_files) # labels
+ cache_path = (p if p.is_file() else Path(self.label_files[0]).parent).with_suffix('.cache')
+ try:
+ cache, exists = np.load(cache_path, allow_pickle=True).item(), True # load dict
+ assert cache['version'] == self.cache_version # matches current version
+ assert cache['hash'] == get_hash(self.label_files + self.im_files) # identical hash
+ except (FileNotFoundError, AssertionError, AttributeError):
+ cache, exists = self.cache_labels(cache_path, prefix), False # run cache ops
+
+ # Display cache
+ nf, nm, ne, nc, n = cache.pop('results') # found, missing, empty, corrupt, total
+ if exists and LOCAL_RANK in (-1, 0):
+ d = f'Scanning {cache_path}... {nf} images, {nm + ne} backgrounds, {nc} corrupt'
+ tqdm(None, desc=prefix + d, total=n, initial=n, bar_format=TQDM_BAR_FORMAT) # display cache results
+ if cache['msgs']:
+ LOGGER.info('\n'.join(cache['msgs'])) # display warnings
+ assert nf > 0 or not augment, f'{prefix}No labels found in {cache_path}, can not start training. {HELP_URL}'
+
+ # Read cache
+ [cache.pop(k) for k in ('hash', 'version', 'msgs')] # remove items
+ labels, shapes, self.segments = zip(*cache.values())
+ nl = len(np.concatenate(labels, 0)) # number of labels
+ assert nl > 0 or not augment, f'{prefix}All labels empty in {cache_path}, can not start training. {HELP_URL}'
+ self.labels = list(labels)
+ self.shapes = np.array(shapes)
+ self.im_files = list(cache.keys()) # update
+ self.label_files = img2label_paths(cache.keys()) # update
+
+ # Filter images
+ if min_items:
+ include = np.array([len(x) >= min_items for x in self.labels]).nonzero()[0].astype(int)
+ LOGGER.info(f'{prefix}{n - len(include)}/{n} images filtered from dataset')
+ self.im_files = [self.im_files[i] for i in include]
+ self.label_files = [self.label_files[i] for i in include]
+ self.labels = [self.labels[i] for i in include]
+ self.segments = [self.segments[i] for i in include]
+ self.shapes = self.shapes[include] # wh
+
+ # Create indices
+ n = len(self.shapes) # number of images
+ bi = np.floor(np.arange(n) / batch_size).astype(int) # batch index
+ nb = bi[-1] + 1 # number of batches
+ self.batch = bi # batch index of image
+ self.n = n
+ self.indices = range(n)
+
+ # Update labels
+ include_class = [] # filter labels to include only these classes (optional)
+ include_class_array = np.array(include_class).reshape(1, -1)
+ for i, (label, segment) in enumerate(zip(self.labels, self.segments)):
+ if include_class:
+ j = (label[:, 0:1] == include_class_array).any(1)
+ self.labels[i] = label[j]
+ if segment:
+ self.segments[i] = [segment[si] for si, idx in enumerate(j) if idx]
+ if single_cls: # single-class training, merge all classes into 0
+ self.labels[i][:, 0] = 0
+
+ # Rectangular Training
+ if self.rect:
+ # Sort by aspect ratio
+ s = self.shapes # wh
+ ar = s[:, 1] / s[:, 0] # aspect ratio
+ irect = ar.argsort()
+ self.im_files = [self.im_files[i] for i in irect]
+ self.label_files = [self.label_files[i] for i in irect]
+ self.labels = [self.labels[i] for i in irect]
+ self.segments = [self.segments[i] for i in irect]
+ self.shapes = s[irect] # wh
+ ar = ar[irect]
+
+ # Set training image shapes
+ shapes = [[1, 1]] * nb
+ for i in range(nb):
+ ari = ar[bi == i]
+ mini, maxi = ari.min(), ari.max()
+ if maxi < 1:
+ shapes[i] = [maxi, 1]
+ elif mini > 1:
+ shapes[i] = [1, 1 / mini]
+
+ self.batch_shapes = np.ceil(np.array(shapes) * img_size / stride + pad).astype(int) * stride
+
+ # Cache images into RAM/disk for faster training
+ if cache_images == 'ram' and not self.check_cache_ram(prefix=prefix):
+ cache_images = False
+ self.ims = [None] * n
+ self.npy_files = [Path(f).with_suffix('.npy') for f in self.im_files]
+ if cache_images:
+ b, gb = 0, 1 << 30 # bytes of cached images, bytes per gigabytes
+ self.im_hw0, self.im_hw = [None] * n, [None] * n
+ fcn = self.cache_images_to_disk if cache_images == 'disk' else self.load_image
+ with ThreadPool(NUM_THREADS) as pool:
+ results = pool.imap(fcn, range(n))
+ pbar = tqdm(enumerate(results), total=n, bar_format=TQDM_BAR_FORMAT, disable=LOCAL_RANK > 0)
+ for i, x in pbar:
+ if cache_images == 'disk':
+ b += self.npy_files[i].stat().st_size
+ else: # 'ram'
+ self.ims[i], self.im_hw0[i], self.im_hw[i] = x # im, hw_orig, hw_resized = load_image(self, i)
+ b += self.ims[i].nbytes
+ pbar.desc = f'{prefix}Caching images ({b / gb:.1f}GB {cache_images})'
+ pbar.close()
+
+ def check_cache_ram(self, safety_margin=0.1, prefix=''):
+ """Check image caching requirements vs available memory."""
+ b, gb = 0, 1 << 30 # bytes of cached images, bytes per gigabytes
+ n = min(self.n, 30) # extrapolate from 30 random images
+ for _ in range(n):
+ im = cv2.imread(random.choice(self.im_files)) # sample image
+ ratio = self.img_size / max(im.shape[0], im.shape[1]) # max(h, w) # ratio
+ b += im.nbytes * ratio ** 2
+ mem_required = b * self.n / n # GB required to cache dataset into RAM
+ mem = psutil.virtual_memory()
+ cache = mem_required * (1 + safety_margin) < mem.available # to cache or not to cache, that is the question
+ if not cache:
+ LOGGER.info(f'{prefix}{mem_required / gb:.1f}GB RAM required, '
+ f'{mem.available / gb:.1f}/{mem.total / gb:.1f}GB available, '
+ f"{'caching images ✅' if cache else 'not caching images ⚠️'}")
+ return cache
+
+ def cache_labels(self, path=Path('./labels.cache'), prefix=''):
+ """Cache labels and save as numpy file for next time."""
+ # Cache dataset labels, check images and read shapes
+ if path.exists():
+ path.unlink() # remove *.cache file if exists
+ x = {} # dict
+ nm, nf, ne, nc, msgs = 0, 0, 0, 0, [] # number missing, found, empty, corrupt, messages
+ desc = f'{prefix}Scanning {path.parent / path.stem}...'
+ total = len(self.im_files)
+ with ThreadPool(NUM_THREADS) as pool:
+ results = pool.imap(verify_image_label, zip(self.im_files, self.label_files, repeat(prefix)))
+ pbar = tqdm(results, desc=desc, total=total, bar_format=TQDM_BAR_FORMAT)
+ for im_file, lb, shape, segments, nm_f, nf_f, ne_f, nc_f, msg in pbar:
+ nm += nm_f
+ nf += nf_f
+ ne += ne_f
+ nc += nc_f
+ if im_file:
+ x[im_file] = [lb, shape, segments]
+ if msg:
+ msgs.append(msg)
+ pbar.desc = f'{desc} {nf} images, {nm + ne} backgrounds, {nc} corrupt'
+ pbar.close()
+
+ if msgs:
+ LOGGER.info('\n'.join(msgs))
+ if nf == 0:
+ LOGGER.warning(f'{prefix}WARNING ⚠️ No labels found in {path}. {HELP_URL}')
+ x['hash'] = get_hash(self.label_files + self.im_files)
+ x['results'] = nf, nm, ne, nc, len(self.im_files)
+ x['msgs'] = msgs # warnings
+ x['version'] = self.cache_version # cache version
+ if is_dir_writeable(path.parent):
+ np.save(str(path), x) # save cache for next time
+ path.with_suffix('.cache.npy').rename(path) # remove .npy suffix
+ LOGGER.info(f'{prefix}New cache created: {path}')
+ else:
+ LOGGER.warning(f'{prefix}WARNING ⚠️ Cache directory {path.parent} is not writeable') # not writeable
+ return x
+
+ def __len__(self):
+ """Returns the length of 'im_files' attribute."""
+ return len(self.im_files)
+
+ def __getitem__(self, index):
+ """Get a sample and its corresponding label, filename and shape from the dataset."""
+ index = self.indices[index] # linear, shuffled, or image_weights
+
+ hyp = self.hyp
+ mosaic = self.mosaic and random.random() < hyp['mosaic']
+ if mosaic:
+ # Load mosaic
+ img, labels = self.load_mosaic(index)
+ shapes = None
+
+ # MixUp augmentation
+ if random.random() < hyp['mixup']:
+ img, labels = mixup(img, labels, *self.load_mosaic(random.randint(0, self.n - 1)))
+
+ else:
+ # Load image
+ img, (h0, w0), (h, w) = self.load_image(index)
+
+ # Letterbox
+ shape = self.batch_shapes[self.batch[index]] if self.rect else self.img_size # final letterboxed shape
+ img, ratio, pad = letterbox(img, shape, auto=False, scaleup=self.augment)
+ shapes = (h0, w0), ((h / h0, w / w0), pad) # for COCO mAP rescaling
+
+ labels = self.labels[index].copy()
+ if labels.size: # normalized xywh to pixel xyxy format
+ labels[:, 1:] = xywhn2xyxy(labels[:, 1:], ratio[0] * w, ratio[1] * h, padw=pad[0], padh=pad[1])
+
+ if self.augment:
+ img, labels = random_perspective(img,
+ labels,
+ degrees=hyp['degrees'],
+ translate=hyp['translate'],
+ scale=hyp['scale'],
+ shear=hyp['shear'],
+ perspective=hyp['perspective'])
+
+ nl = len(labels) # number of labels
+ if nl:
+ labels[:, 1:5] = xyxy2xywhn(labels[:, 1:5], w=img.shape[1], h=img.shape[0], clip=True, eps=1E-3)
+
+ if self.augment:
+ # Albumentations
+ img, labels = self.albumentations(img, labels)
+ nl = len(labels) # update after albumentations
+
+ # HSV color-space
+ augment_hsv(img, hgain=hyp['hsv_h'], sgain=hyp['hsv_s'], vgain=hyp['hsv_v'])
+
+ # Flip up-down
+ if random.random() < hyp['flipud']:
+ img = np.flipud(img)
+ if nl:
+ labels[:, 2] = 1 - labels[:, 2]
+
+ # Flip left-right
+ if random.random() < hyp['fliplr']:
+ img = np.fliplr(img)
+ if nl:
+ labels[:, 1] = 1 - labels[:, 1]
+
+ # Cutouts
+ # labels = cutout(img, labels, p=0.5)
+ # nl = len(labels) # update after cutout
+
+ labels_out = torch.zeros((nl, 6))
+ if nl:
+ labels_out[:, 1:] = torch.from_numpy(labels)
+
+ # Convert
+ img = img.transpose((2, 0, 1))[::-1] # HWC to CHW, BGR to RGB
+ img = np.ascontiguousarray(img)
+
+ return torch.from_numpy(img), labels_out, self.im_files[index], shapes
+
+ def load_image(self, i):
+ """Loads 1 image from dataset index 'i', returns (im, original hw, resized hw)."""
+ im, f, fn = self.ims[i], self.im_files[i], self.npy_files[i],
+ if im is None: # not cached in RAM
+ if fn.exists(): # load npy
+ im = np.load(fn)
+ else: # read image
+ im = cv2.imread(f) # BGR
+ assert im is not None, f'Image Not Found {f}'
+ h0, w0 = im.shape[:2] # orig hw
+ r = self.img_size / max(h0, w0) # ratio
+ if r != 1: # if sizes are not equal
+ interp = cv2.INTER_LINEAR if (self.augment or r > 1) else cv2.INTER_AREA
+ im = cv2.resize(im, (math.ceil(w0 * r), math.ceil(h0 * r)), interpolation=interp)
+ return im, (h0, w0), im.shape[:2] # im, hw_original, hw_resized
+ return self.ims[i], self.im_hw0[i], self.im_hw[i] # im, hw_original, hw_resized
+
+ def cache_images_to_disk(self, i):
+ """Saves an image as an *.npy file for faster loading."""
+ f = self.npy_files[i]
+ if not f.exists():
+ np.save(f.as_posix(), cv2.imread(self.im_files[i]))
+
+ def load_mosaic(self, index):
+ """YOLOv5 4-mosaic loader. Loads 1 image + 3 random images into a 4-image mosaic."""
+ labels4, segments4 = [], []
+ s = self.img_size
+ yc, xc = (int(random.uniform(-x, 2 * s + x)) for x in self.mosaic_border) # mosaic center x, y
+ indices = [index] + random.choices(self.indices, k=3) # 3 additional image indices
+ random.shuffle(indices)
+ for i, index in enumerate(indices):
+ # Load image
+ img, _, (h, w) = self.load_image(index)
+
+ # Place img in img4
+ if i == 0: # top left
+ img4 = np.full((s * 2, s * 2, img.shape[2]), 114, dtype=np.uint8) # base image with 4 tiles
+ x1a, y1a, x2a, y2a = max(xc - w, 0), max(yc - h, 0), xc, yc # xmin, ymin, xmax, ymax (large image)
+ x1b, y1b, x2b, y2b = w - (x2a - x1a), h - (y2a - y1a), w, h # xmin, ymin, xmax, ymax (small image)
+ elif i == 1: # top right
+ x1a, y1a, x2a, y2a = xc, max(yc - h, 0), min(xc + w, s * 2), yc
+ x1b, y1b, x2b, y2b = 0, h - (y2a - y1a), min(w, x2a - x1a), h
+ elif i == 2: # bottom left
+ x1a, y1a, x2a, y2a = max(xc - w, 0), yc, xc, min(s * 2, yc + h)
+ x1b, y1b, x2b, y2b = w - (x2a - x1a), 0, w, min(y2a - y1a, h)
+ elif i == 3: # bottom right
+ x1a, y1a, x2a, y2a = xc, yc, min(xc + w, s * 2), min(s * 2, yc + h)
+ x1b, y1b, x2b, y2b = 0, 0, min(w, x2a - x1a), min(y2a - y1a, h)
+
+ img4[y1a:y2a, x1a:x2a] = img[y1b:y2b, x1b:x2b] # img4[ymin:ymax, xmin:xmax]
+ padw = x1a - x1b
+ padh = y1a - y1b
+
+ # Labels
+ labels, segments = self.labels[index].copy(), self.segments[index].copy()
+ if labels.size:
+ labels[:, 1:] = xywhn2xyxy(labels[:, 1:], w, h, padw, padh) # normalized xywh to pixel xyxy format
+ segments = [xyn2xy(x, w, h, padw, padh) for x in segments]
+ labels4.append(labels)
+ segments4.extend(segments)
+
+ # Concat/clip labels
+ labels4 = np.concatenate(labels4, 0)
+ for x in (labels4[:, 1:], *segments4):
+ np.clip(x, 0, 2 * s, out=x) # clip when using random_perspective()
+ # img4, labels4 = replicate(img4, labels4) # replicate
+
+ # Augment
+ img4, labels4, segments4 = copy_paste(img4, labels4, segments4, p=self.hyp['copy_paste'])
+ img4, labels4 = random_perspective(img4,
+ labels4,
+ segments4,
+ degrees=self.hyp['degrees'],
+ translate=self.hyp['translate'],
+ scale=self.hyp['scale'],
+ shear=self.hyp['shear'],
+ perspective=self.hyp['perspective'],
+ border=self.mosaic_border) # border to remove
+
+ return img4, labels4
+
+ def load_mosaic9(self, index):
+ """YOLOv5 9-mosaic loader. Loads 1 image + 8 random images into a 9-image mosaic."""
+ labels9, segments9 = [], []
+ s = self.img_size
+ indices = [index] + random.choices(self.indices, k=8) # 8 additional image indices
+ random.shuffle(indices)
+ hp, wp = -1, -1 # height, width previous
+ for i, index in enumerate(indices):
+ # Load image
+ img, _, (h, w) = self.load_image(index)
+
+ # Place img in img9
+ if i == 0: # center
+ img9 = np.full((s * 3, s * 3, img.shape[2]), 114, dtype=np.uint8) # base image with 4 tiles
+ h0, w0 = h, w
+ c = s, s, s + w, s + h # xmin, ymin, xmax, ymax (base) coordinates
+ elif i == 1: # top
+ c = s, s - h, s + w, s
+ elif i == 2: # top right
+ c = s + wp, s - h, s + wp + w, s
+ elif i == 3: # right
+ c = s + w0, s, s + w0 + w, s + h
+ elif i == 4: # bottom right
+ c = s + w0, s + hp, s + w0 + w, s + hp + h
+ elif i == 5: # bottom
+ c = s + w0 - w, s + h0, s + w0, s + h0 + h
+ elif i == 6: # bottom left
+ c = s + w0 - wp - w, s + h0, s + w0 - wp, s + h0 + h
+ elif i == 7: # left
+ c = s - w, s + h0 - h, s, s + h0
+ elif i == 8: # top left
+ c = s - w, s + h0 - hp - h, s, s + h0 - hp
+
+ padx, pady = c[:2]
+ x1, y1, x2, y2 = (max(x, 0) for x in c) # allocate coords
+
+ # Labels
+ labels, segments = self.labels[index].copy(), self.segments[index].copy()
+ if labels.size:
+ labels[:, 1:] = xywhn2xyxy(labels[:, 1:], w, h, padx, pady) # normalized xywh to pixel xyxy format
+ segments = [xyn2xy(x, w, h, padx, pady) for x in segments]
+ labels9.append(labels)
+ segments9.extend(segments)
+
+ # Image
+ img9[y1:y2, x1:x2] = img[y1 - pady:, x1 - padx:] # img9[ymin:ymax, xmin:xmax]
+ hp, wp = h, w # height, width previous
+
+ # Offset
+ yc, xc = (int(random.uniform(0, s)) for _ in self.mosaic_border) # mosaic center x, y
+ img9 = img9[yc:yc + 2 * s, xc:xc + 2 * s]
+
+ # Concat/clip labels
+ labels9 = np.concatenate(labels9, 0)
+ labels9[:, [1, 3]] -= xc
+ labels9[:, [2, 4]] -= yc
+ c = np.array([xc, yc]) # centers
+ segments9 = [x - c for x in segments9]
+
+ for x in (labels9[:, 1:], *segments9):
+ np.clip(x, 0, 2 * s, out=x) # clip when using random_perspective()
+ # img9, labels9 = replicate(img9, labels9) # replicate
+
+ # Augment
+ img9, labels9, segments9 = copy_paste(img9, labels9, segments9, p=self.hyp['copy_paste'])
+ img9, labels9 = random_perspective(img9,
+ labels9,
+ segments9,
+ degrees=self.hyp['degrees'],
+ translate=self.hyp['translate'],
+ scale=self.hyp['scale'],
+ shear=self.hyp['shear'],
+ perspective=self.hyp['perspective'],
+ border=self.mosaic_border) # border to remove
+
+ return img9, labels9
+
+ @staticmethod
+ def collate_fn(batch):
+ """YOLOv8 collate function, outputs dict."""
+ im, label, path, shapes = zip(*batch) # transposed
+ for i, lb in enumerate(label):
+ lb[:, 0] = i # add target image index for build_targets()
+ batch_idx, cls, bboxes = torch.cat(label, 0).split((1, 1, 4), dim=1)
+ return {
+ 'ori_shape': tuple((x[0] if x else None) for x in shapes),
+ 'ratio_pad': tuple((x[1] if x else None) for x in shapes),
+ 'im_file': path,
+ 'img': torch.stack(im, 0),
+ 'cls': cls,
+ 'bboxes': bboxes,
+ 'batch_idx': batch_idx.view(-1)}
+
+ @staticmethod
+ def collate_fn_old(batch):
+ """YOLOv5 original collate function."""
+ im, label, path, shapes = zip(*batch) # transposed
+ for i, lb in enumerate(label):
+ lb[:, 0] = i # add target image index for build_targets()
+ return torch.stack(im, 0), torch.cat(label, 0), path, shapes
+
+
+# Ancillary functions --------------------------------------------------------------------------------------------------
+def flatten_recursive(path=DATASETS_DIR / 'coco128'):
+ """Flatten a recursive directory by bringing all files to top level."""
+ new_path = Path(f'{str(path)}_flat')
+ if os.path.exists(new_path):
+ shutil.rmtree(new_path) # delete output folder
+ os.makedirs(new_path) # make new output folder
+ for file in tqdm(glob.glob(f'{str(Path(path))}/**/*.*', recursive=True)):
+ shutil.copyfile(file, new_path / Path(file).name)
+
+
+def extract_boxes(path=DATASETS_DIR / 'coco128'): # from utils.dataloaders import *; extract_boxes()
+ # Convert detection dataset into classification dataset, with one directory per class
+ path = Path(path) # images dir
+ shutil.rmtree(path / 'classification') if (path / 'classification').is_dir() else None # remove existing
+ files = list(path.rglob('*.*'))
+ n = len(files) # number of files
+ for im_file in tqdm(files, total=n):
+ if im_file.suffix[1:] in IMG_FORMATS:
+ # Image
+ im = cv2.imread(str(im_file))[..., ::-1] # BGR to RGB
+ h, w = im.shape[:2]
+
+ # Labels
+ lb_file = Path(img2label_paths([str(im_file)])[0])
+ if Path(lb_file).exists():
+ with open(lb_file) as f:
+ lb = np.array([x.split() for x in f.read().strip().splitlines()], dtype=np.float32) # labels
+
+ for j, x in enumerate(lb):
+ c = int(x[0]) # class
+ f = (path / 'classifier') / f'{c}' / f'{path.stem}_{im_file.stem}_{j}.jpg' # new filename
+ if not f.parent.is_dir():
+ f.parent.mkdir(parents=True)
+
+ b = x[1:] * [w, h, w, h] # box
+ # B[2:] = b[2:].max() # rectangle to square
+ b[2:] = b[2:] * 1.2 + 3 # pad
+ b = xywh2xyxy(b.reshape(-1, 4)).ravel().astype(int)
+
+ b[[0, 2]] = np.clip(b[[0, 2]], 0, w) # clip boxes outside of image
+ b[[1, 3]] = np.clip(b[[1, 3]], 0, h)
+ assert cv2.imwrite(str(f), im[b[1]:b[3], b[0]:b[2]]), f'box failure in {f}'
+
+
+def autosplit(path=DATASETS_DIR / 'coco128/images', weights=(0.9, 0.1, 0.0), annotated_only=False):
+ """Autosplit a dataset into train/val/test splits and save path/autosplit_*.txt files
+ Usage: from utils.dataloaders import *; autosplit()
+ Arguments
+ path: Path to images directory
+ weights: Train, val, test weights (list, tuple)
+ annotated_only: Only use images with an annotated txt file
+ """
+ path = Path(path) # images dir
+ files = sorted(x for x in path.rglob('*.*') if x.suffix[1:].lower() in IMG_FORMATS) # image files only
+ n = len(files) # number of files
+ random.seed(0) # for reproducibility
+ indices = random.choices([0, 1, 2], weights=weights, k=n) # assign each image to a split
+
+ txt = ['autosplit_train.txt', 'autosplit_val.txt', 'autosplit_test.txt'] # 3 txt files
+ for x in txt:
+ if (path.parent / x).exists():
+ (path.parent / x).unlink() # remove existing
+
+ print(f'Autosplitting images from {path}' + ', using *.txt labeled images only' * annotated_only)
+ for i, img in tqdm(zip(indices, files), total=n):
+ if not annotated_only or Path(img2label_paths([str(img)])[0]).exists(): # check label
+ with open(path.parent / txt[i], 'a') as f:
+ f.write(f'./{img.relative_to(path.parent).as_posix()}' + '\n') # add image to txt file
+
+
+def verify_image_label(args):
+ """Verify one image-label pair."""
+ im_file, lb_file, prefix = args
+ nm, nf, ne, nc, msg, segments = 0, 0, 0, 0, '', [] # number (missing, found, empty, corrupt), message, segments
+ try:
+ # Verify images
+ im = Image.open(im_file)
+ im.verify() # PIL verify
+ shape = exif_size(im) # image size
+ assert (shape[0] > 9) & (shape[1] > 9), f'image size {shape} <10 pixels'
+ assert im.format.lower() in IMG_FORMATS, f'invalid image format {im.format}'
+ if im.format.lower() in ('jpg', 'jpeg'):
+ with open(im_file, 'rb') as f:
+ f.seek(-2, 2)
+ if f.read() != b'\xff\xd9': # corrupt JPEG
+ ImageOps.exif_transpose(Image.open(im_file)).save(im_file, 'JPEG', subsampling=0, quality=100)
+ msg = f'{prefix}WARNING ⚠️ {im_file}: corrupt JPEG restored and saved'
+
+ # Verify labels
+ if os.path.isfile(lb_file):
+ nf = 1 # label found
+ with open(lb_file) as f:
+ lb = [x.split() for x in f.read().strip().splitlines() if len(x)]
+ if any(len(x) > 6 for x in lb): # is segment
+ classes = np.array([x[0] for x in lb], dtype=np.float32)
+ segments = [np.array(x[1:], dtype=np.float32).reshape(-1, 2) for x in lb] # (cls, xy1...)
+ lb = np.concatenate((classes.reshape(-1, 1), segments2boxes(segments)), 1) # (cls, xywh)
+ lb = np.array(lb, dtype=np.float32)
+ nl = len(lb)
+ if nl:
+ assert lb.shape[1] == 5, f'labels require 5 columns, {lb.shape[1]} columns detected'
+ assert (lb >= 0).all(), f'negative label values {lb[lb < 0]}'
+ assert (lb[:, 1:] <= 1).all(), f'non-normalized or out of bounds coordinates {lb[:, 1:][lb[:, 1:] > 1]}'
+ _, i = np.unique(lb, axis=0, return_index=True)
+ if len(i) < nl: # duplicate row check
+ lb = lb[i] # remove duplicates
+ if segments:
+ segments = [segments[x] for x in i]
+ msg = f'{prefix}WARNING ⚠️ {im_file}: {nl - len(i)} duplicate labels removed'
+ else:
+ ne = 1 # label empty
+ lb = np.zeros((0, 5), dtype=np.float32)
+ else:
+ nm = 1 # label missing
+ lb = np.zeros((0, 5), dtype=np.float32)
+ return im_file, lb, shape, segments, nm, nf, ne, nc, msg
+ except Exception as e:
+ nc = 1
+ msg = f'{prefix}WARNING ⚠️ {im_file}: ignoring corrupt image/label: {e}'
+ return [None, None, None, None, nm, nf, ne, nc, msg]
+
+
+# Classification dataloaders -------------------------------------------------------------------------------------------
+class ClassificationDataset(torchvision.datasets.ImageFolder):
+ """
+ YOLOv5 Classification Dataset.
+ Arguments
+ root: Dataset path
+ transform: torchvision transforms, used by default
+ album_transform: Albumentations transforms, used if installed
+ """
+
+ def __init__(self, root, augment, imgsz, cache=False):
+ """Initialize YOLO dataset with root, augmentation, image size, and cache parameters."""
+ super().__init__(root=root)
+ self.torch_transforms = classify_transforms(imgsz)
+ self.album_transforms = classify_albumentations(augment, imgsz) if augment else None
+ self.cache_ram = cache is True or cache == 'ram'
+ self.cache_disk = cache == 'disk'
+ self.samples = [list(x) + [Path(x[0]).with_suffix('.npy'), None] for x in self.samples] # file, index, npy, im
+
+ def __getitem__(self, i):
+ """Retrieves data items of 'dataset' via indices & creates InfiniteDataLoader."""
+ f, j, fn, im = self.samples[i] # filename, index, filename.with_suffix('.npy'), image
+ if self.cache_ram and im is None:
+ im = self.samples[i][3] = cv2.imread(f)
+ elif self.cache_disk:
+ if not fn.exists(): # load npy
+ np.save(fn.as_posix(), cv2.imread(f))
+ im = np.load(fn)
+ else: # read image
+ im = cv2.imread(f) # BGR
+ if self.album_transforms:
+ sample = self.album_transforms(image=cv2.cvtColor(im, cv2.COLOR_BGR2RGB))['image']
+ else:
+ sample = self.torch_transforms(im)
+ return sample, j
+
+
+def create_classification_dataloader(path,
+ imgsz=224,
+ batch_size=16,
+ augment=True,
+ cache=False,
+ rank=-1,
+ workers=8,
+ shuffle=True):
+ """Returns Dataloader object to be used with YOLOv5 Classifier."""
+ with torch_distributed_zero_first(rank): # init dataset *.cache only once if DDP
+ dataset = ClassificationDataset(root=path, imgsz=imgsz, augment=augment, cache=cache)
+ batch_size = min(batch_size, len(dataset))
+ nd = torch.cuda.device_count()
+ nw = min([os.cpu_count() // max(nd, 1), batch_size if batch_size > 1 else 0, workers])
+ sampler = None if rank == -1 else distributed.DistributedSampler(dataset, shuffle=shuffle)
+ generator = torch.Generator()
+ generator.manual_seed(6148914691236517205 + RANK)
+ return InfiniteDataLoader(dataset,
+ batch_size=batch_size,
+ shuffle=shuffle and sampler is None,
+ num_workers=nw,
+ sampler=sampler,
+ pin_memory=PIN_MEMORY,
+ worker_init_fn=seed_worker,
+ generator=generator) # or DataLoader(persistent_workers=True)
diff --git a/yolov8/ultralytics/yolo/data/dataset.py b/yolov8/ultralytics/yolo/data/dataset.py
new file mode 100644
index 0000000000000000000000000000000000000000..17e6d47c109f9f9088ce01602dc9b06e0a89fde9
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/dataset.py
@@ -0,0 +1,274 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from itertools import repeat
+from multiprocessing.pool import ThreadPool
+from pathlib import Path
+
+import cv2
+import numpy as np
+import torch
+import torchvision
+from tqdm import tqdm
+
+from ..utils import LOCAL_RANK, NUM_THREADS, TQDM_BAR_FORMAT, is_dir_writeable
+from .augment import Compose, Format, Instances, LetterBox, classify_albumentations, classify_transforms, v8_transforms
+from .base import BaseDataset
+from .utils import HELP_URL, LOGGER, get_hash, img2label_paths, verify_image_label
+
+
+class YOLODataset(BaseDataset):
+ """
+ Dataset class for loading object detection and/or segmentation labels in YOLO format.
+
+ Args:
+ data (dict, optional): A dataset YAML dictionary. Defaults to None.
+ use_segments (bool, optional): If True, segmentation masks are used as labels. Defaults to False.
+ use_keypoints (bool, optional): If True, keypoints are used as labels. Defaults to False.
+
+ Returns:
+ (torch.utils.data.Dataset): A PyTorch dataset object that can be used for training an object detection model.
+ """
+ cache_version = '1.0.2' # dataset labels *.cache version, >= 1.0.0 for YOLOv8
+ rand_interp_methods = [cv2.INTER_NEAREST, cv2.INTER_LINEAR, cv2.INTER_CUBIC, cv2.INTER_AREA, cv2.INTER_LANCZOS4]
+
+ def __init__(self, *args, data=None, use_segments=False, use_keypoints=False, **kwargs):
+ self.use_segments = use_segments
+ self.use_keypoints = use_keypoints
+ self.data = data
+ assert not (self.use_segments and self.use_keypoints), 'Can not use both segments and keypoints.'
+ super().__init__(*args, **kwargs)
+
+ def cache_labels(self, path=Path('./labels.cache')):
+ """Cache dataset labels, check images and read shapes.
+ Args:
+ path (Path): path where to save the cache file (default: Path('./labels.cache')).
+ Returns:
+ (dict): labels.
+ """
+ x = {'labels': []}
+ nm, nf, ne, nc, msgs = 0, 0, 0, 0, [] # number missing, found, empty, corrupt, messages
+ desc = f'{self.prefix}Scanning {path.parent / path.stem}...'
+ total = len(self.im_files)
+ nkpt, ndim = self.data.get('kpt_shape', (0, 0))
+ if self.use_keypoints and (nkpt <= 0 or ndim not in (2, 3)):
+ raise ValueError("'kpt_shape' in data.yaml missing or incorrect. Should be a list with [number of "
+ "keypoints, number of dims (2 for x,y or 3 for x,y,visible)], i.e. 'kpt_shape: [17, 3]'")
+ with ThreadPool(NUM_THREADS) as pool:
+ results = pool.imap(func=verify_image_label,
+ iterable=zip(self.im_files, self.label_files, repeat(self.prefix),
+ repeat(self.use_keypoints), repeat(len(self.data['names'])), repeat(nkpt),
+ repeat(ndim)))
+ pbar = tqdm(results, desc=desc, total=total, bar_format=TQDM_BAR_FORMAT)
+ for im_file, lb, shape, segments, keypoint, nm_f, nf_f, ne_f, nc_f, msg in pbar:
+ nm += nm_f
+ nf += nf_f
+ ne += ne_f
+ nc += nc_f
+ if im_file:
+ x['labels'].append(
+ dict(
+ im_file=im_file,
+ shape=shape,
+ cls=lb[:, 0:1], # n, 1
+ bboxes=lb[:, 1:], # n, 4
+ segments=segments,
+ keypoints=keypoint,
+ normalized=True,
+ bbox_format='xywh'))
+ if msg:
+ msgs.append(msg)
+ pbar.desc = f'{desc} {nf} images, {nm + ne} backgrounds, {nc} corrupt'
+ pbar.close()
+
+ if msgs:
+ LOGGER.info('\n'.join(msgs))
+ if nf == 0:
+ LOGGER.warning(f'{self.prefix}WARNING ⚠️ No labels found in {path}. {HELP_URL}')
+ x['hash'] = get_hash(self.label_files + self.im_files)
+ x['results'] = nf, nm, ne, nc, len(self.im_files)
+ x['msgs'] = msgs # warnings
+ x['version'] = self.cache_version # cache version
+ if is_dir_writeable(path.parent):
+ if path.exists():
+ path.unlink() # remove *.cache file if exists
+ np.save(str(path), x) # save cache for next time
+ path.with_suffix('.cache.npy').rename(path) # remove .npy suffix
+ LOGGER.info(f'{self.prefix}New cache created: {path}')
+ else:
+ LOGGER.warning(f'{self.prefix}WARNING ⚠️ Cache directory {path.parent} is not writeable, cache not saved.')
+ return x
+
+ def get_labels(self):
+ """Returns dictionary of labels for YOLO training."""
+ self.label_files = img2label_paths(self.im_files)
+ cache_path = Path(self.label_files[0]).parent.with_suffix('.cache')
+ try:
+ import gc
+ gc.disable() # reduce pickle load time https://github.com/ultralytics/ultralytics/pull/1585
+ cache, exists = np.load(str(cache_path), allow_pickle=True).item(), True # load dict
+ gc.enable()
+ assert cache['version'] == self.cache_version # matches current version
+ assert cache['hash'] == get_hash(self.label_files + self.im_files) # identical hash
+ except (FileNotFoundError, AssertionError, AttributeError):
+ cache, exists = self.cache_labels(cache_path), False # run cache ops
+
+ # Display cache
+ nf, nm, ne, nc, n = cache.pop('results') # found, missing, empty, corrupt, total
+ if exists and LOCAL_RANK in (-1, 0):
+ d = f'Scanning {cache_path}... {nf} images, {nm + ne} backgrounds, {nc} corrupt'
+ tqdm(None, desc=self.prefix + d, total=n, initial=n, bar_format=TQDM_BAR_FORMAT) # display cache results
+ if cache['msgs']:
+ LOGGER.info('\n'.join(cache['msgs'])) # display warnings
+ if nf == 0: # number of labels found
+ raise FileNotFoundError(f'{self.prefix}No labels found in {cache_path}, can not start training. {HELP_URL}')
+
+ # Read cache
+ [cache.pop(k) for k in ('hash', 'version', 'msgs')] # remove items
+ labels = cache['labels']
+ self.im_files = [lb['im_file'] for lb in labels] # update im_files
+
+ # Check if the dataset is all boxes or all segments
+ lengths = ((len(lb['cls']), len(lb['bboxes']), len(lb['segments'])) for lb in labels)
+ len_cls, len_boxes, len_segments = (sum(x) for x in zip(*lengths))
+ if len_segments and len_boxes != len_segments:
+ LOGGER.warning(
+ f'WARNING ⚠️ Box and segment counts should be equal, but got len(segments) = {len_segments}, '
+ f'len(boxes) = {len_boxes}. To resolve this only boxes will be used and all segments will be removed. '
+ 'To avoid this please supply either a detect or segment dataset, not a detect-segment mixed dataset.')
+ for lb in labels:
+ lb['segments'] = []
+ if len_cls == 0:
+ raise ValueError(f'All labels empty in {cache_path}, can not start training without labels. {HELP_URL}')
+ return labels
+
+ # TODO: use hyp config to set all these augmentations
+ def build_transforms(self, hyp=None):
+ """Builds and appends transforms to the list."""
+ if self.augment:
+ hyp.mosaic = hyp.mosaic if self.augment and not self.rect else 0.0
+ hyp.mixup = hyp.mixup if self.augment and not self.rect else 0.0
+ transforms = v8_transforms(self, self.imgsz, hyp)
+ else:
+ transforms = Compose([LetterBox(new_shape=(self.imgsz, self.imgsz), scaleup=False)])
+ transforms.append(
+ Format(bbox_format='xywh',
+ normalize=True,
+ return_mask=self.use_segments,
+ return_keypoint=self.use_keypoints,
+ batch_idx=True,
+ mask_ratio=hyp.mask_ratio,
+ mask_overlap=hyp.overlap_mask))
+ return transforms
+
+ def close_mosaic(self, hyp):
+ """Sets mosaic, copy_paste and mixup options to 0.0 and builds transformations."""
+ hyp.mosaic = 0.0 # set mosaic ratio=0.0
+ hyp.copy_paste = 0.0 # keep the same behavior as previous v8 close-mosaic
+ hyp.mixup = 0.0 # keep the same behavior as previous v8 close-mosaic
+ self.transforms = self.build_transforms(hyp)
+
+ def update_labels_info(self, label):
+ """custom your label format here."""
+ # NOTE: cls is not with bboxes now, classification and semantic segmentation need an independent cls label
+ # we can make it also support classification and semantic segmentation by add or remove some dict keys there.
+ bboxes = label.pop('bboxes')
+ segments = label.pop('segments')
+ keypoints = label.pop('keypoints', None)
+ bbox_format = label.pop('bbox_format')
+ normalized = label.pop('normalized')
+ label['instances'] = Instances(bboxes, segments, keypoints, bbox_format=bbox_format, normalized=normalized)
+ return label
+
+ @staticmethod
+ def collate_fn(batch):
+ """Collates data samples into batches."""
+ new_batch = {}
+ keys = batch[0].keys()
+ values = list(zip(*[list(b.values()) for b in batch]))
+ for i, k in enumerate(keys):
+ value = values[i]
+ if k == 'img':
+ value = torch.stack(value, 0)
+ if k in ['masks', 'keypoints', 'bboxes', 'cls']:
+ value = torch.cat(value, 0)
+ new_batch[k] = value
+ new_batch['batch_idx'] = list(new_batch['batch_idx'])
+ for i in range(len(new_batch['batch_idx'])):
+ new_batch['batch_idx'][i] += i # add target image index for build_targets()
+ new_batch['batch_idx'] = torch.cat(new_batch['batch_idx'], 0)
+ return new_batch
+
+
+# Classification dataloaders -------------------------------------------------------------------------------------------
+class ClassificationDataset(torchvision.datasets.ImageFolder):
+ """
+ YOLO Classification Dataset.
+
+ Args:
+ root (str): Dataset path.
+
+ Attributes:
+ cache_ram (bool): True if images should be cached in RAM, False otherwise.
+ cache_disk (bool): True if images should be cached on disk, False otherwise.
+ samples (list): List of samples containing file, index, npy, and im.
+ torch_transforms (callable): torchvision transforms applied to the dataset.
+ album_transforms (callable, optional): Albumentations transforms applied to the dataset if augment is True.
+ """
+
+ def __init__(self, root, args, augment=False, cache=False):
+ """
+ Initialize YOLO object with root, image size, augmentations, and cache settings.
+
+ Args:
+ root (str): Dataset path.
+ args (Namespace): Argument parser containing dataset related settings.
+ augment (bool, optional): True if dataset should be augmented, False otherwise. Defaults to False.
+ cache (bool | str | optional): Cache setting, can be True, False, 'ram' or 'disk'. Defaults to False.
+ """
+ super().__init__(root=root)
+ if augment and args.fraction < 1.0: # reduce training fraction
+ self.samples = self.samples[:round(len(self.samples) * args.fraction)]
+ self.cache_ram = cache is True or cache == 'ram'
+ self.cache_disk = cache == 'disk'
+ self.samples = [list(x) + [Path(x[0]).with_suffix('.npy'), None] for x in self.samples] # file, index, npy, im
+ self.torch_transforms = classify_transforms(args.imgsz)
+ self.album_transforms = classify_albumentations(
+ augment=augment,
+ size=args.imgsz,
+ scale=(1.0 - args.scale, 1.0), # (0.08, 1.0)
+ hflip=args.fliplr,
+ vflip=args.flipud,
+ hsv_h=args.hsv_h, # HSV-Hue augmentation (fraction)
+ hsv_s=args.hsv_s, # HSV-Saturation augmentation (fraction)
+ hsv_v=args.hsv_v, # HSV-Value augmentation (fraction)
+ mean=(0.0, 0.0, 0.0), # IMAGENET_MEAN
+ std=(1.0, 1.0, 1.0), # IMAGENET_STD
+ auto_aug=False) if augment else None
+
+ def __getitem__(self, i):
+ """Returns subset of data and targets corresponding to given indices."""
+ f, j, fn, im = self.samples[i] # filename, index, filename.with_suffix('.npy'), image
+ if self.cache_ram and im is None:
+ im = self.samples[i][3] = cv2.imread(f)
+ elif self.cache_disk:
+ if not fn.exists(): # load npy
+ np.save(fn.as_posix(), cv2.imread(f))
+ im = np.load(fn)
+ else: # read image
+ im = cv2.imread(f) # BGR
+ if self.album_transforms:
+ sample = self.album_transforms(image=cv2.cvtColor(im, cv2.COLOR_BGR2RGB))['image']
+ else:
+ sample = self.torch_transforms(im)
+ return {'img': sample, 'cls': j}
+
+ def __len__(self) -> int:
+ return len(self.samples)
+
+
+# TODO: support semantic segmentation
+class SemanticDataset(BaseDataset):
+
+ def __init__(self):
+ """Initialize a SemanticDataset object."""
+ super().__init__()
diff --git a/yolov8/ultralytics/yolo/data/dataset_wrappers.py b/yolov8/ultralytics/yolo/data/dataset_wrappers.py
new file mode 100644
index 0000000000000000000000000000000000000000..72a6fb57a373cb9fbfd2a4facde7dfb427452a64
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/dataset_wrappers.py
@@ -0,0 +1,53 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import collections
+from copy import deepcopy
+
+from .augment import LetterBox
+
+
+class MixAndRectDataset:
+ """
+ A dataset class that applies mosaic and mixup transformations as well as rectangular training.
+
+ Attributes:
+ dataset: The base dataset.
+ imgsz: The size of the images in the dataset.
+ """
+
+ def __init__(self, dataset):
+ """
+ Args:
+ dataset (BaseDataset): The base dataset to apply transformations to.
+ """
+ self.dataset = dataset
+ self.imgsz = dataset.imgsz
+
+ def __len__(self):
+ """Returns the number of items in the dataset."""
+ return len(self.dataset)
+
+ def __getitem__(self, index):
+ """
+ Applies mosaic, mixup and rectangular training transformations to an item in the dataset.
+
+ Args:
+ index (int): Index of the item in the dataset.
+
+ Returns:
+ (dict): A dictionary containing the transformed item data.
+ """
+ labels = deepcopy(self.dataset[index])
+ for transform in self.dataset.transforms.tolist():
+ # Mosaic and mixup
+ if hasattr(transform, 'get_indexes'):
+ indexes = transform.get_indexes(self.dataset)
+ if not isinstance(indexes, collections.abc.Sequence):
+ indexes = [indexes]
+ labels['mix_labels'] = [deepcopy(self.dataset[index]) for index in indexes]
+ if self.dataset.rect and isinstance(transform, LetterBox):
+ transform.new_shape = self.dataset.batch_shapes[self.dataset.batch[index]]
+ labels = transform(labels)
+ if 'mix_labels' in labels:
+ labels.pop('mix_labels')
+ return labels
diff --git a/yolov8/ultralytics/yolo/data/scripts/download_weights.sh b/yolov8/ultralytics/yolo/data/scripts/download_weights.sh
new file mode 100755
index 0000000000000000000000000000000000000000..72502a366eaee7dff539fc806e00c0d1bcc5e404
--- /dev/null
+++ b/yolov8/ultralytics/yolo/data/scripts/download_weights.sh
@@ -0,0 +1,18 @@
+#!/bin/bash
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+# Download latest models from https://github.com/ultralytics/assets/releases
+# Example usage: bash ultralytics/yolo/data/scripts/download_weights.sh
+# parent
+# └── weights
+# ├── yolov8n.pt ← downloads here
+# ├── yolov8s.pt
+# └── ...
+
+python - < 9) & (shape[1] > 9), f'image size {shape} <10 pixels'
+ assert im.format.lower() in IMG_FORMATS, f'invalid image format {im.format}'
+ if im.format.lower() in ('jpg', 'jpeg'):
+ with open(im_file, 'rb') as f:
+ f.seek(-2, 2)
+ if f.read() != b'\xff\xd9': # corrupt JPEG
+ ImageOps.exif_transpose(Image.open(im_file)).save(im_file, 'JPEG', subsampling=0, quality=100)
+ msg = f'{prefix}WARNING ⚠️ {im_file}: corrupt JPEG restored and saved'
+
+ # Verify labels
+ if os.path.isfile(lb_file):
+ nf = 1 # label found
+ with open(lb_file) as f:
+ lb = [x.split() for x in f.read().strip().splitlines() if len(x)]
+ if any(len(x) > 6 for x in lb) and (not keypoint): # is segment
+ classes = np.array([x[0] for x in lb], dtype=np.float32)
+ segments = [np.array(x[1:], dtype=np.float32).reshape(-1, 2) for x in lb] # (cls, xy1...)
+ lb = np.concatenate((classes.reshape(-1, 1), segments2boxes(segments)), 1) # (cls, xywh)
+ lb = np.array(lb, dtype=np.float32)
+ nl = len(lb)
+ if nl:
+ if keypoint:
+ assert lb.shape[1] == (5 + nkpt * ndim), f'labels require {(5 + nkpt * ndim)} columns each'
+ assert (lb[:, 5::ndim] <= 1).all(), 'non-normalized or out of bounds coordinate labels'
+ assert (lb[:, 6::ndim] <= 1).all(), 'non-normalized or out of bounds coordinate labels'
+ else:
+ assert lb.shape[1] == 5, f'labels require 5 columns, {lb.shape[1]} columns detected'
+ assert (lb[:, 1:] <= 1).all(), \
+ f'non-normalized or out of bounds coordinates {lb[:, 1:][lb[:, 1:] > 1]}'
+ assert (lb >= 0).all(), f'negative label values {lb[lb < 0]}'
+ # All labels
+ max_cls = int(lb[:, 0].max()) # max label count
+ assert max_cls <= num_cls, \
+ f'Label class {max_cls} exceeds dataset class count {num_cls}. ' \
+ f'Possible class labels are 0-{num_cls - 1}'
+ _, i = np.unique(lb, axis=0, return_index=True)
+ if len(i) < nl: # duplicate row check
+ lb = lb[i] # remove duplicates
+ if segments:
+ segments = [segments[x] for x in i]
+ msg = f'{prefix}WARNING ⚠️ {im_file}: {nl - len(i)} duplicate labels removed'
+ else:
+ ne = 1 # label empty
+ lb = np.zeros((0, (5 + nkpt * ndim)), dtype=np.float32) if keypoint else np.zeros(
+ (0, 5), dtype=np.float32)
+ else:
+ nm = 1 # label missing
+ lb = np.zeros((0, (5 + nkpt * ndim)), dtype=np.float32) if keypoint else np.zeros((0, 5), dtype=np.float32)
+ if keypoint:
+ keypoints = lb[:, 5:].reshape(-1, nkpt, ndim)
+ if ndim == 2:
+ kpt_mask = np.ones(keypoints.shape[:2], dtype=np.float32)
+ kpt_mask = np.where(keypoints[..., 0] < 0, 0.0, kpt_mask)
+ kpt_mask = np.where(keypoints[..., 1] < 0, 0.0, kpt_mask)
+ keypoints = np.concatenate([keypoints, kpt_mask[..., None]], axis=-1) # (nl, nkpt, 3)
+ lb = lb[:, :5]
+ return im_file, lb, shape, segments, keypoints, nm, nf, ne, nc, msg
+ except Exception as e:
+ nc = 1
+ msg = f'{prefix}WARNING ⚠️ {im_file}: ignoring corrupt image/label: {e}'
+ return [None, None, None, None, None, nm, nf, ne, nc, msg]
+
+
+def polygon2mask(imgsz, polygons, color=1, downsample_ratio=1):
+ """
+ Args:
+ imgsz (tuple): The image size.
+ polygons (list[np.ndarray]): [N, M], N is the number of polygons, M is the number of points(Be divided by 2).
+ color (int): color
+ downsample_ratio (int): downsample ratio
+ """
+ mask = np.zeros(imgsz, dtype=np.uint8)
+ polygons = np.asarray(polygons)
+ polygons = polygons.astype(np.int32)
+ shape = polygons.shape
+ polygons = polygons.reshape(shape[0], -1, 2)
+ cv2.fillPoly(mask, polygons, color=color)
+ nh, nw = (imgsz[0] // downsample_ratio, imgsz[1] // downsample_ratio)
+ # NOTE: fillPoly firstly then resize is trying the keep the same way
+ # of loss calculation when mask-ratio=1.
+ mask = cv2.resize(mask, (nw, nh))
+ return mask
+
+
+def polygons2masks(imgsz, polygons, color, downsample_ratio=1):
+ """
+ Args:
+ imgsz (tuple): The image size.
+ polygons (list[np.ndarray]): each polygon is [N, M], N is number of polygons, M is number of points (M % 2 = 0)
+ color (int): color
+ downsample_ratio (int): downsample ratio
+ """
+ masks = []
+ for si in range(len(polygons)):
+ mask = polygon2mask(imgsz, [polygons[si].reshape(-1)], color, downsample_ratio)
+ masks.append(mask)
+ return np.array(masks)
+
+
+def polygons2masks_overlap(imgsz, segments, downsample_ratio=1):
+ """Return a (640, 640) overlap mask."""
+ masks = np.zeros((imgsz[0] // downsample_ratio, imgsz[1] // downsample_ratio),
+ dtype=np.int32 if len(segments) > 255 else np.uint8)
+ areas = []
+ ms = []
+ for si in range(len(segments)):
+ mask = polygon2mask(imgsz, [segments[si].reshape(-1)], downsample_ratio=downsample_ratio, color=1)
+ ms.append(mask)
+ areas.append(mask.sum())
+ areas = np.asarray(areas)
+ index = np.argsort(-areas)
+ ms = np.array(ms)[index]
+ for i in range(len(segments)):
+ mask = ms[i] * (i + 1)
+ masks = masks + mask
+ masks = np.clip(masks, a_min=0, a_max=i + 1)
+ return masks, index
+
+
+def check_det_dataset(dataset, autodownload=True):
+ """Download, check and/or unzip dataset if not found locally."""
+ data = check_file(dataset)
+
+ # Download (optional)
+ extract_dir = ''
+ if isinstance(data, (str, Path)) and (zipfile.is_zipfile(data) or is_tarfile(data)):
+ new_dir = safe_download(data, dir=DATASETS_DIR, unzip=True, delete=False, curl=False)
+ data = next((DATASETS_DIR / new_dir).rglob('*.yaml'))
+ extract_dir, autodownload = data.parent, False
+
+ # Read yaml (optional)
+ if isinstance(data, (str, Path)):
+ data = yaml_load(data, append_filename=True) # dictionary
+
+ # Checks
+ for k in 'train', 'val':
+ if k not in data:
+ raise SyntaxError(
+ emojis(f"{dataset} '{k}:' key missing ❌.\n'train' and 'val' are required in all data YAMLs."))
+ if 'names' not in data and 'nc' not in data:
+ raise SyntaxError(emojis(f"{dataset} key missing ❌.\n either 'names' or 'nc' are required in all data YAMLs."))
+ if 'names' in data and 'nc' in data and len(data['names']) != data['nc']:
+ raise SyntaxError(emojis(f"{dataset} 'names' length {len(data['names'])} and 'nc: {data['nc']}' must match."))
+ if 'names' not in data:
+ data['names'] = [f'class_{i}' for i in range(data['nc'])]
+ else:
+ data['nc'] = len(data['names'])
+
+ data['names'] = check_class_names(data['names'])
+
+ # Resolve paths
+ path = Path(extract_dir or data.get('path') or Path(data.get('yaml_file', '')).parent) # dataset root
+
+ if not path.is_absolute():
+ path = (DATASETS_DIR / path).resolve()
+ data['path'] = path # download scripts
+ for k in 'train', 'val', 'test':
+ if data.get(k): # prepend path
+ if isinstance(data[k], str):
+ x = (path / data[k]).resolve()
+ if not x.exists() and data[k].startswith('../'):
+ x = (path / data[k][3:]).resolve()
+ data[k] = str(x)
+ else:
+ data[k] = [str((path / x).resolve()) for x in data[k]]
+
+ # Parse yaml
+ train, val, test, s = (data.get(x) for x in ('train', 'val', 'test', 'download'))
+ if val:
+ val = [Path(x).resolve() for x in (val if isinstance(val, list) else [val])] # val path
+ if not all(x.exists() for x in val):
+ name = clean_url(dataset) # dataset name with URL auth stripped
+ m = f"\nDataset '{name}' images not found ⚠️, missing paths %s" % [str(x) for x in val if not x.exists()]
+ if s and autodownload:
+ LOGGER.warning(m)
+ else:
+ m += f"\nNote dataset download directory is '{DATASETS_DIR}'. You can update this in '{SETTINGS_YAML}'"
+ raise FileNotFoundError(m)
+ t = time.time()
+ if s.startswith('http') and s.endswith('.zip'): # URL
+ safe_download(url=s, dir=DATASETS_DIR, delete=True)
+ r = None # success
+ elif s.startswith('bash '): # bash script
+ LOGGER.info(f'Running {s} ...')
+ r = os.system(s)
+ else: # python script
+ r = exec(s, {'yaml': data}) # return None
+ dt = f'({round(time.time() - t, 1)}s)'
+ s = f"success ✅ {dt}, saved to {colorstr('bold', DATASETS_DIR)}" if r in (0, None) else f'failure {dt} ❌'
+ LOGGER.info(f'Dataset download {s}\n')
+ check_font('Arial.ttf' if is_ascii(data['names']) else 'Arial.Unicode.ttf') # download fonts
+
+ return data # dictionary
+
+
+def check_cls_dataset(dataset: str, split=''):
+ """
+ Check a classification dataset such as Imagenet.
+
+ This function takes a `dataset` name as input and returns a dictionary containing information about the dataset.
+ If the dataset is not found, it attempts to download the dataset from the internet and save it locally.
+
+ Args:
+ dataset (str): Name of the dataset.
+ split (str, optional): Dataset split, either 'val', 'test', or ''. Defaults to ''.
+
+ Returns:
+ data (dict): A dictionary containing the following keys and values:
+ 'train': Path object for the directory containing the training set of the dataset
+ 'val': Path object for the directory containing the validation set of the dataset
+ 'test': Path object for the directory containing the test set of the dataset
+ 'nc': Number of classes in the dataset
+ 'names': List of class names in the dataset
+ """
+ data_dir = (DATASETS_DIR / dataset).resolve()
+ if not data_dir.is_dir():
+ LOGGER.info(f'\nDataset not found ⚠️, missing path {data_dir}, attempting download...')
+ t = time.time()
+ if dataset == 'imagenet':
+ subprocess.run(f"bash {ROOT / 'yolo/data/scripts/get_imagenet.sh'}", shell=True, check=True)
+ else:
+ url = f'https://github.com/ultralytics/yolov5/releases/download/v1.0/{dataset}.zip'
+ download(url, dir=data_dir.parent)
+ s = f"Dataset download success ✅ ({time.time() - t:.1f}s), saved to {colorstr('bold', data_dir)}\n"
+ LOGGER.info(s)
+ train_set = data_dir / 'train'
+ val_set = data_dir / 'val' if (data_dir / 'val').exists() else None # data/test or data/val
+ test_set = data_dir / 'test' if (data_dir / 'test').exists() else None # data/val or data/test
+ if split == 'val' and not val_set:
+ LOGGER.info("WARNING ⚠️ Dataset 'split=val' not found, using 'split=test' instead.")
+ elif split == 'test' and not test_set:
+ LOGGER.info("WARNING ⚠️ Dataset 'split=test' not found, using 'split=val' instead.")
+
+ nc = len([x for x in (data_dir / 'train').glob('*') if x.is_dir()]) # number of classes
+ names = [x.name for x in (data_dir / 'train').iterdir() if x.is_dir()] # class names list
+ names = dict(enumerate(sorted(names)))
+ return {'train': train_set, 'val': val_set or test_set, 'test': test_set or val_set, 'nc': nc, 'names': names}
+
+
+class HUBDatasetStats():
+ """
+ Class for generating HUB dataset JSON and `-hub` dataset directory
+
+ Arguments
+ path: Path to data.yaml or data.zip (with data.yaml inside data.zip)
+ task: Dataset task. Options are 'detect', 'segment', 'pose', 'classify'.
+ autodownload: Attempt to download dataset if not found locally
+
+ Usage
+ from ultralytics.yolo.data.utils import HUBDatasetStats
+ stats = HUBDatasetStats('/Users/glennjocher/Downloads/coco8.zip', task='detect') # detect dataset
+ stats = HUBDatasetStats('/Users/glennjocher/Downloads/coco8-seg.zip', task='segment') # segment dataset
+ stats = HUBDatasetStats('/Users/glennjocher/Downloads/coco8-pose.zip', task='pose') # pose dataset
+ stats.get_json(save=False)
+ stats.process_images()
+ """
+
+ def __init__(self, path='coco128.yaml', task='detect', autodownload=False):
+ """Initialize class."""
+ LOGGER.info(f'Starting HUB dataset checks for {path}....')
+ zipped, data_dir, yaml_path = self._unzip(Path(path))
+ try:
+ # data = yaml_load(check_yaml(yaml_path)) # data dict
+ data = check_det_dataset(yaml_path, autodownload) # data dict
+ if zipped:
+ data['path'] = data_dir
+ except Exception as e:
+ raise Exception('error/HUB/dataset_stats/yaml_load') from e
+
+ self.hub_dir = Path(str(data['path']) + '-hub')
+ self.im_dir = self.hub_dir / 'images'
+ self.im_dir.mkdir(parents=True, exist_ok=True) # makes /images
+ self.stats = {'nc': len(data['names']), 'names': list(data['names'].values())} # statistics dictionary
+ self.data = data
+ self.task = task # detect, segment, pose, classify
+
+ @staticmethod
+ def _find_yaml(dir):
+ """Return data.yaml file."""
+ files = list(dir.glob('*.yaml')) or list(dir.rglob('*.yaml')) # try root level first and then recursive
+ assert files, f'No *.yaml file found in {dir}'
+ if len(files) > 1:
+ files = [f for f in files if f.stem == dir.stem] # prefer *.yaml files that match dir name
+ assert files, f'Multiple *.yaml files found in {dir}, only 1 *.yaml file allowed'
+ assert len(files) == 1, f'Multiple *.yaml files found: {files}, only 1 *.yaml file allowed in {dir}'
+ return files[0]
+
+ def _unzip(self, path):
+ """Unzip data.zip."""
+ if not str(path).endswith('.zip'): # path is data.yaml
+ return False, None, path
+ unzip_dir = unzip_file(path, path=path.parent)
+ assert unzip_dir.is_dir(), f'Error unzipping {path}, {unzip_dir} not found. ' \
+ f'path/to/abc.zip MUST unzip to path/to/abc/'
+ return True, str(unzip_dir), self._find_yaml(unzip_dir) # zipped, data_dir, yaml_path
+
+ def _hub_ops(self, f):
+ """Saves a compressed image for HUB previews."""
+ compress_one_image(f, self.im_dir / Path(f).name) # save to dataset-hub
+
+ def get_json(self, save=False, verbose=False):
+ """Return dataset JSON for Ultralytics HUB."""
+ from ultralytics.yolo.data import YOLODataset # ClassificationDataset
+
+ def _round(labels):
+ """Update labels to integer class and 4 decimal place floats."""
+ if self.task == 'detect':
+ coordinates = labels['bboxes']
+ elif self.task == 'segment':
+ coordinates = [x.flatten() for x in labels['segments']]
+ elif self.task == 'pose':
+ n = labels['keypoints'].shape[0]
+ coordinates = np.concatenate((labels['bboxes'], labels['keypoints'].reshape(n, -1)), 1)
+ else:
+ raise ValueError('Undefined dataset task.')
+ zipped = zip(labels['cls'], coordinates)
+ return [[int(c), *(round(float(x), 4) for x in points)] for c, points in zipped]
+
+ for split in 'train', 'val', 'test':
+ if self.data.get(split) is None:
+ self.stats[split] = None # i.e. no test set
+ continue
+
+ dataset = YOLODataset(img_path=self.data[split],
+ data=self.data,
+ use_segments=self.task == 'segment',
+ use_keypoints=self.task == 'pose')
+ x = np.array([
+ np.bincount(label['cls'].astype(int).flatten(), minlength=self.data['nc'])
+ for label in tqdm(dataset.labels, total=len(dataset), desc='Statistics')]) # shape(128x80)
+ self.stats[split] = {
+ 'instance_stats': {
+ 'total': int(x.sum()),
+ 'per_class': x.sum(0).tolist()},
+ 'image_stats': {
+ 'total': len(dataset),
+ 'unlabelled': int(np.all(x == 0, 1).sum()),
+ 'per_class': (x > 0).sum(0).tolist()},
+ 'labels': [{
+ Path(k).name: _round(v)} for k, v in zip(dataset.im_files, dataset.labels)]}
+
+ # Save, print and return
+ if save:
+ stats_path = self.hub_dir / 'stats.json'
+ LOGGER.info(f'Saving {stats_path.resolve()}...')
+ with open(stats_path, 'w') as f:
+ json.dump(self.stats, f) # save stats.json
+ if verbose:
+ LOGGER.info(json.dumps(self.stats, indent=2, sort_keys=False))
+ return self.stats
+
+ def process_images(self):
+ """Compress images for Ultralytics HUB."""
+ from ultralytics.yolo.data import YOLODataset # ClassificationDataset
+
+ for split in 'train', 'val', 'test':
+ if self.data.get(split) is None:
+ continue
+ dataset = YOLODataset(img_path=self.data[split], data=self.data)
+ with ThreadPool(NUM_THREADS) as pool:
+ for _ in tqdm(pool.imap(self._hub_ops, dataset.im_files), total=len(dataset), desc=f'{split} images'):
+ pass
+ LOGGER.info(f'Done. All images saved to {self.im_dir}')
+ return self.im_dir
+
+
+def compress_one_image(f, f_new=None, max_dim=1920, quality=50):
+ """
+ Compresses a single image file to reduced size while preserving its aspect ratio and quality using either the
+ Python Imaging Library (PIL) or OpenCV library. If the input image is smaller than the maximum dimension, it will
+ not be resized.
+
+ Args:
+ f (str): The path to the input image file.
+ f_new (str, optional): The path to the output image file. If not specified, the input file will be overwritten.
+ max_dim (int, optional): The maximum dimension (width or height) of the output image. Default is 1920 pixels.
+ quality (int, optional): The image compression quality as a percentage. Default is 50%.
+
+ Usage:
+ from pathlib import Path
+ from ultralytics.yolo.data.utils import compress_one_image
+ for f in Path('/Users/glennjocher/Downloads/dataset').rglob('*.jpg'):
+ compress_one_image(f)
+ """
+ try: # use PIL
+ im = Image.open(f)
+ r = max_dim / max(im.height, im.width) # ratio
+ if r < 1.0: # image too large
+ im = im.resize((int(im.width * r), int(im.height * r)))
+ im.save(f_new or f, 'JPEG', quality=quality, optimize=True) # save
+ except Exception as e: # use OpenCV
+ LOGGER.info(f'WARNING ⚠️ HUB ops PIL failure {f}: {e}')
+ im = cv2.imread(f)
+ im_height, im_width = im.shape[:2]
+ r = max_dim / max(im_height, im_width) # ratio
+ if r < 1.0: # image too large
+ im = cv2.resize(im, (int(im_width * r), int(im_height * r)), interpolation=cv2.INTER_AREA)
+ cv2.imwrite(str(f_new or f), im)
+
+
+def delete_dsstore(path):
+ """
+ Deletes all ".DS_store" files under a specified directory.
+
+ Args:
+ path (str, optional): The directory path where the ".DS_store" files should be deleted.
+
+ Usage:
+ from ultralytics.yolo.data.utils import delete_dsstore
+ delete_dsstore('/Users/glennjocher/Downloads/dataset')
+
+ Note:
+ ".DS_store" files are created by the Apple operating system and contain metadata about folders and files. They
+ are hidden system files and can cause issues when transferring files between different operating systems.
+ """
+ # Delete Apple .DS_store files
+ files = list(Path(path).rglob('.DS_store'))
+ LOGGER.info(f'Deleting *.DS_store files: {files}')
+ for f in files:
+ f.unlink()
+
+
+def zip_directory(dir, use_zipfile_library=True):
+ """
+ Zips a directory and saves the archive to the specified output path.
+
+ Args:
+ dir (str): The path to the directory to be zipped.
+ use_zipfile_library (bool): Whether to use zipfile library or shutil for zipping.
+
+ Usage:
+ from ultralytics.yolo.data.utils import zip_directory
+ zip_directory('/Users/glennjocher/Downloads/playground')
+
+ zip -r coco8-pose.zip coco8-pose
+ """
+ delete_dsstore(dir)
+ if use_zipfile_library:
+ dir = Path(dir)
+ with zipfile.ZipFile(dir.with_suffix('.zip'), 'w', zipfile.ZIP_DEFLATED) as zip_file:
+ for file_path in dir.glob('**/*'):
+ if file_path.is_file():
+ zip_file.write(file_path, file_path.relative_to(dir))
+ else:
+ import shutil
+ shutil.make_archive(dir, 'zip', dir)
diff --git a/yolov8/ultralytics/yolo/engine/__init__.py b/yolov8/ultralytics/yolo/engine/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/yolov8/ultralytics/yolo/engine/exporter.py b/yolov8/ultralytics/yolo/engine/exporter.py
new file mode 100644
index 0000000000000000000000000000000000000000..5d236272bc33fdaf5354feb7f7176473c83dbe49
--- /dev/null
+++ b/yolov8/ultralytics/yolo/engine/exporter.py
@@ -0,0 +1,922 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Export a YOLOv8 PyTorch model to other formats. TensorFlow exports authored by https://github.com/zldrobit
+
+Format | `format=argument` | Model
+--- | --- | ---
+PyTorch | - | yolov8n.pt
+TorchScript | `torchscript` | yolov8n.torchscript
+ONNX | `onnx` | yolov8n.onnx
+OpenVINO | `openvino` | yolov8n_openvino_model/
+TensorRT | `engine` | yolov8n.engine
+CoreML | `coreml` | yolov8n.mlmodel
+TensorFlow SavedModel | `saved_model` | yolov8n_saved_model/
+TensorFlow GraphDef | `pb` | yolov8n.pb
+TensorFlow Lite | `tflite` | yolov8n.tflite
+TensorFlow Edge TPU | `edgetpu` | yolov8n_edgetpu.tflite
+TensorFlow.js | `tfjs` | yolov8n_web_model/
+PaddlePaddle | `paddle` | yolov8n_paddle_model/
+NCNN | `ncnn` | yolov8n_ncnn_model/
+
+Requirements:
+ $ pip install ultralytics[export]
+
+Python:
+ from ultralytics import YOLO
+ model = YOLO('yolov8n.pt')
+ results = model.export(format='onnx')
+
+CLI:
+ $ yolo mode=export model=yolov8n.pt format=onnx
+
+Inference:
+ $ yolo predict model=yolov8n.pt # PyTorch
+ yolov8n.torchscript # TorchScript
+ yolov8n.onnx # ONNX Runtime or OpenCV DNN with --dnn
+ yolov8n_openvino_model # OpenVINO
+ yolov8n.engine # TensorRT
+ yolov8n.mlmodel # CoreML (macOS-only)
+ yolov8n_saved_model # TensorFlow SavedModel
+ yolov8n.pb # TensorFlow GraphDef
+ yolov8n.tflite # TensorFlow Lite
+ yolov8n_edgetpu.tflite # TensorFlow Edge TPU
+ yolov8n_paddle_model # PaddlePaddle
+
+TensorFlow.js:
+ $ cd .. && git clone https://github.com/zldrobit/tfjs-yolov5-example.git && cd tfjs-yolov5-example
+ $ npm install
+ $ ln -s ../../yolov5/yolov8n_web_model public/yolov8n_web_model
+ $ npm start
+"""
+import json
+import os
+import shutil
+import subprocess
+import time
+import warnings
+from copy import deepcopy
+from pathlib import Path
+
+import torch
+
+from ultralytics.nn.autobackend import check_class_names
+from ultralytics.nn.modules import C2f, Detect, RTDETRDecoder
+from ultralytics.nn.tasks import DetectionModel, SegmentationModel
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.utils import (ARM64, DEFAULT_CFG, LINUX, LOGGER, MACOS, ROOT, __version__, callbacks, colorstr,
+ get_default_args, yaml_save)
+from ultralytics.yolo.utils.checks import check_imgsz, check_requirements, check_version
+from ultralytics.yolo.utils.downloads import attempt_download_asset, get_github_assets
+from ultralytics.yolo.utils.files import file_size
+from ultralytics.yolo.utils.ops import Profile
+from ultralytics.yolo.utils.torch_utils import get_latest_opset, select_device, smart_inference_mode
+
+
+def export_formats():
+ """YOLOv8 export formats."""
+ import pandas
+ x = [
+ ['PyTorch', '-', '.pt', True, True],
+ ['TorchScript', 'torchscript', '.torchscript', True, True],
+ ['ONNX', 'onnx', '.onnx', True, True],
+ ['OpenVINO', 'openvino', '_openvino_model', True, False],
+ ['TensorRT', 'engine', '.engine', False, True],
+ ['CoreML', 'coreml', '.mlmodel', True, False],
+ ['TensorFlow SavedModel', 'saved_model', '_saved_model', True, True],
+ ['TensorFlow GraphDef', 'pb', '.pb', True, True],
+ ['TensorFlow Lite', 'tflite', '.tflite', True, False],
+ ['TensorFlow Edge TPU', 'edgetpu', '_edgetpu.tflite', True, False],
+ ['TensorFlow.js', 'tfjs', '_web_model', True, False],
+ ['PaddlePaddle', 'paddle', '_paddle_model', True, True],
+ ['NCNN', 'ncnn', '_ncnn_model', True, True], ]
+ return pandas.DataFrame(x, columns=['Format', 'Argument', 'Suffix', 'CPU', 'GPU'])
+
+
+def gd_outputs(gd):
+ """TensorFlow GraphDef model output node names."""
+ name_list, input_list = [], []
+ for node in gd.node: # tensorflow.core.framework.node_def_pb2.NodeDef
+ name_list.append(node.name)
+ input_list.extend(node.input)
+ return sorted(f'{x}:0' for x in list(set(name_list) - set(input_list)) if not x.startswith('NoOp'))
+
+
+def try_export(inner_func):
+ """YOLOv8 export decorator, i..e @try_export."""
+ inner_args = get_default_args(inner_func)
+
+ def outer_func(*args, **kwargs):
+ """Export a model."""
+ prefix = inner_args['prefix']
+ try:
+ with Profile() as dt:
+ f, model = inner_func(*args, **kwargs)
+ LOGGER.info(f'{prefix} export success ✅ {dt.t:.1f}s, saved as {f} ({file_size(f):.1f} MB)')
+ return f, model
+ except Exception as e:
+ LOGGER.info(f'{prefix} export failure ❌ {dt.t:.1f}s: {e}')
+ return None, None
+
+ return outer_func
+
+
+class Exporter:
+ """
+ A class for exporting a model.
+
+ Attributes:
+ args (SimpleNamespace): Configuration for the exporter.
+ save_dir (Path): Directory to save results.
+ """
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ """
+ Initializes the Exporter class.
+
+ Args:
+ cfg (str, optional): Path to a configuration file. Defaults to DEFAULT_CFG.
+ overrides (dict, optional): Configuration overrides. Defaults to None.
+ _callbacks (list, optional): List of callback functions. Defaults to None.
+ """
+ self.args = get_cfg(cfg, overrides)
+ self.callbacks = _callbacks or callbacks.get_default_callbacks()
+ callbacks.add_integration_callbacks(self)
+
+ @smart_inference_mode()
+ def __call__(self, model=None):
+ """Returns list of exported files/dirs after running callbacks."""
+ self.run_callbacks('on_export_start')
+ t = time.time()
+ format = self.args.format.lower() # to lowercase
+ if format in ('tensorrt', 'trt'): # engine aliases
+ format = 'engine'
+ fmts = tuple(export_formats()['Argument'][1:]) # available export formats
+ flags = [x == format for x in fmts]
+ if sum(flags) != 1:
+ raise ValueError(f"Invalid export format='{format}'. Valid formats are {fmts}")
+ jit, onnx, xml, engine, coreml, saved_model, pb, tflite, edgetpu, tfjs, paddle, ncnn = flags # export booleans
+
+ # Load PyTorch model
+ self.device = select_device('cpu' if self.args.device is None else self.args.device)
+
+ # Checks
+ model.names = check_class_names(model.names)
+ if self.args.half and onnx and self.device.type == 'cpu':
+ LOGGER.warning('WARNING ⚠️ half=True only compatible with GPU export, i.e. use device=0')
+ self.args.half = False
+ assert not self.args.dynamic, 'half=True not compatible with dynamic=True, i.e. use only one.'
+ self.imgsz = check_imgsz(self.args.imgsz, stride=model.stride, min_dim=2) # check image size
+ if self.args.optimize:
+ assert not ncnn, "optimize=True not compatible with format='ncnn', i.e. use optimize=False"
+ assert self.device.type == 'cpu', "optimize=True not compatible with cuda devices, i.e. use device='cpu'"
+ if edgetpu and not LINUX:
+ raise SystemError('Edge TPU export only supported on Linux. See https://coral.ai/docs/edgetpu/compiler/')
+
+ # Input
+ im = torch.zeros(self.args.batch, 3, *self.imgsz).to(self.device)
+ file = Path(
+ getattr(model, 'pt_path', None) or getattr(model, 'yaml_file', None) or model.yaml.get('yaml_file', ''))
+ if file.suffix == '.yaml':
+ file = Path(file.name)
+
+ # Update model
+ model = deepcopy(model).to(self.device)
+ for p in model.parameters():
+ p.requires_grad = False
+ model.eval()
+ model.float()
+ model = model.fuse()
+ for k, m in model.named_modules():
+ if isinstance(m, (Detect, RTDETRDecoder)): # Segment and Pose use Detect base class
+ m.dynamic = self.args.dynamic
+ m.export = True
+ m.format = self.args.format
+ elif isinstance(m, C2f) and not any((saved_model, pb, tflite, edgetpu, tfjs)):
+ # EdgeTPU does not support FlexSplitV while split provides cleaner ONNX graph
+ m.forward = m.forward_split
+
+ y = None
+ for _ in range(2):
+ y = model(im) # dry runs
+ if self.args.half and (engine or onnx) and self.device.type != 'cpu':
+ im, model = im.half(), model.half() # to FP16
+
+ # Filter warnings
+ warnings.filterwarnings('ignore', category=torch.jit.TracerWarning) # suppress TracerWarning
+ warnings.filterwarnings('ignore', category=UserWarning) # suppress shape prim::Constant missing ONNX warning
+ warnings.filterwarnings('ignore', category=DeprecationWarning) # suppress CoreML np.bool deprecation warning
+
+ # Assign
+ self.im = im
+ self.model = model
+ self.file = file
+ self.output_shape = tuple(y.shape) if isinstance(y, torch.Tensor) else \
+ tuple(tuple(x.shape if isinstance(x, torch.Tensor) else []) for x in y)
+ self.pretty_name = Path(self.model.yaml.get('yaml_file', self.file)).stem.replace('yolo', 'YOLO')
+ trained_on = f'trained on {Path(self.args.data).name}' if self.args.data else '(untrained)'
+ description = f'Ultralytics {self.pretty_name} model {trained_on}'
+ self.metadata = {
+ 'description': description,
+ 'author': 'Ultralytics',
+ 'license': 'AGPL-3.0 https://ultralytics.com/license',
+ 'version': __version__,
+ 'stride': int(max(model.stride)),
+ 'task': model.task,
+ 'batch': self.args.batch,
+ 'imgsz': self.imgsz,
+ 'names': model.names} # model metadata
+ if model.task == 'pose':
+ self.metadata['kpt_shape'] = model.model[-1].kpt_shape
+
+ LOGGER.info(f"\n{colorstr('PyTorch:')} starting from {file} with input shape {tuple(im.shape)} BCHW and "
+ f'output shape(s) {self.output_shape} ({file_size(file):.1f} MB)')
+
+ # Exports
+ f = [''] * len(fmts) # exported filenames
+ if jit or ncnn: # TorchScript
+ f[0], _ = self.export_torchscript()
+ if engine: # TensorRT required before ONNX
+ f[1], _ = self.export_engine()
+ if onnx or xml: # OpenVINO requires ONNX
+ f[2], _ = self.export_onnx()
+ if xml: # OpenVINO
+ f[3], _ = self.export_openvino()
+ if coreml: # CoreML
+ f[4], _ = self.export_coreml()
+ if any((saved_model, pb, tflite, edgetpu, tfjs)): # TensorFlow formats
+ self.args.int8 |= edgetpu
+ f[5], s_model = self.export_saved_model()
+ if pb or tfjs: # pb prerequisite to tfjs
+ f[6], _ = self.export_pb(s_model)
+ if tflite:
+ f[7], _ = self.export_tflite(s_model, nms=False, agnostic_nms=self.args.agnostic_nms)
+ if edgetpu:
+ f[8], _ = self.export_edgetpu(tflite_model=Path(f[5]) / f'{self.file.stem}_full_integer_quant.tflite')
+ if tfjs:
+ f[9], _ = self.export_tfjs()
+ if paddle: # PaddlePaddle
+ f[10], _ = self.export_paddle()
+ if ncnn: # NCNN
+ f[11], _ = self.export_ncnn()
+
+ # Finish
+ f = [str(x) for x in f if x] # filter out '' and None
+ if any(f):
+ f = str(Path(f[-1]))
+ square = self.imgsz[0] == self.imgsz[1]
+ s = '' if square else f"WARNING ⚠️ non-PyTorch val requires square images, 'imgsz={self.imgsz}' will not " \
+ f"work. Use export 'imgsz={max(self.imgsz)}' if val is required."
+ imgsz = self.imgsz[0] if square else str(self.imgsz)[1:-1].replace(' ', '')
+ data = f'data={self.args.data}' if model.task == 'segment' and format == 'pb' else ''
+ LOGGER.info(
+ f'\nExport complete ({time.time() - t:.1f}s)'
+ f"\nResults saved to {colorstr('bold', file.parent.resolve())}"
+ f'\nPredict: yolo predict task={model.task} model={f} imgsz={imgsz} {data}'
+ f'\nValidate: yolo val task={model.task} model={f} imgsz={imgsz} data={self.args.data} {s}'
+ f'\nVisualize: https://netron.app')
+
+ self.run_callbacks('on_export_end')
+ return f # return list of exported files/dirs
+
+ @try_export
+ def export_torchscript(self, prefix=colorstr('TorchScript:')):
+ """YOLOv8 TorchScript model export."""
+ LOGGER.info(f'\n{prefix} starting export with torch {torch.__version__}...')
+ f = self.file.with_suffix('.torchscript')
+
+ ts = torch.jit.trace(self.model, self.im, strict=False)
+ extra_files = {'config.txt': json.dumps(self.metadata)} # torch._C.ExtraFilesMap()
+ if self.args.optimize: # https://pytorch.org/tutorials/recipes/mobile_interpreter.html
+ LOGGER.info(f'{prefix} optimizing for mobile...')
+ from torch.utils.mobile_optimizer import optimize_for_mobile
+ optimize_for_mobile(ts)._save_for_lite_interpreter(str(f), _extra_files=extra_files)
+ else:
+ ts.save(str(f), _extra_files=extra_files)
+ return f, None
+
+ @try_export
+ def export_onnx(self, prefix=colorstr('ONNX:')):
+ """YOLOv8 ONNX export."""
+ requirements = ['onnx>=1.12.0']
+ if self.args.simplify:
+ requirements += ['onnxsim>=0.4.17', 'onnxruntime-gpu' if torch.cuda.is_available() else 'onnxruntime']
+ check_requirements(requirements)
+ import onnx # noqa
+
+ opset_version = self.args.opset or get_latest_opset()
+ LOGGER.info(f'\n{prefix} starting export with onnx {onnx.__version__} opset {opset_version}...')
+ f = str(self.file.with_suffix('.onnx'))
+
+ output_names = ['output0', 'output1'] if isinstance(self.model, SegmentationModel) else ['output0']
+ dynamic = self.args.dynamic
+ if dynamic:
+ dynamic = {'images': {0: 'batch', 2: 'height', 3: 'width'}} # shape(1,3,640,640)
+ if isinstance(self.model, SegmentationModel):
+ dynamic['output0'] = {0: 'batch', 1: 'anchors'} # shape(1,25200,85)
+ dynamic['output1'] = {0: 'batch', 2: 'mask_height', 3: 'mask_width'} # shape(1,32,160,160)
+ elif isinstance(self.model, DetectionModel):
+ dynamic['output0'] = {0: 'batch', 1: 'anchors'} # shape(1,25200,85)
+
+ torch.onnx.export(
+ self.model.cpu() if dynamic else self.model, # --dynamic only compatible with cpu
+ self.im.cpu() if dynamic else self.im,
+ f,
+ verbose=False,
+ opset_version=opset_version,
+ do_constant_folding=True, # WARNING: DNN inference with torch>=1.12 may require do_constant_folding=False
+ input_names=['images'],
+ output_names=output_names,
+ dynamic_axes=dynamic or None)
+
+ # Checks
+ model_onnx = onnx.load(f) # load onnx model
+ # onnx.checker.check_model(model_onnx) # check onnx model
+
+ # Simplify
+ if self.args.simplify:
+ try:
+ import onnxsim
+
+ LOGGER.info(f'{prefix} simplifying with onnxsim {onnxsim.__version__}...')
+ # subprocess.run(f'onnxsim {f} {f}', shell=True)
+ model_onnx, check = onnxsim.simplify(model_onnx)
+ assert check, 'Simplified ONNX model could not be validated'
+ except Exception as e:
+ LOGGER.info(f'{prefix} simplifier failure: {e}')
+
+ # Metadata
+ for k, v in self.metadata.items():
+ meta = model_onnx.metadata_props.add()
+ meta.key, meta.value = k, str(v)
+
+ onnx.save(model_onnx, f)
+ return f, model_onnx
+
+ @try_export
+ def export_openvino(self, prefix=colorstr('OpenVINO:')):
+ """YOLOv8 OpenVINO export."""
+ check_requirements('openvino-dev>=2022.3') # requires openvino-dev: https://pypi.org/project/openvino-dev/
+ import openvino.runtime as ov # noqa
+ from openvino.tools import mo # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with openvino {ov.__version__}...')
+ f = str(self.file).replace(self.file.suffix, f'_openvino_model{os.sep}')
+ f_onnx = self.file.with_suffix('.onnx')
+ f_ov = str(Path(f) / self.file.with_suffix('.xml').name)
+
+ ov_model = mo.convert_model(f_onnx,
+ model_name=self.pretty_name,
+ framework='onnx',
+ compress_to_fp16=self.args.half) # export
+
+ # Set RT info
+ ov_model.set_rt_info('YOLOv8', ['model_info', 'model_type'])
+ ov_model.set_rt_info(True, ['model_info', 'reverse_input_channels'])
+ ov_model.set_rt_info(114, ['model_info', 'pad_value'])
+ ov_model.set_rt_info([255.0], ['model_info', 'scale_values'])
+ ov_model.set_rt_info(self.args.iou, ['model_info', 'iou_threshold'])
+ ov_model.set_rt_info([v.replace(' ', '_') for k, v in sorted(self.model.names.items())],
+ ['model_info', 'labels'])
+ if self.model.task != 'classify':
+ ov_model.set_rt_info('fit_to_window_letterbox', ['model_info', 'resize_type'])
+
+ ov.serialize(ov_model, f_ov) # save
+ yaml_save(Path(f) / 'metadata.yaml', self.metadata) # add metadata.yaml
+ return f, None
+
+ @try_export
+ def export_paddle(self, prefix=colorstr('PaddlePaddle:')):
+ """YOLOv8 Paddle export."""
+ check_requirements(('paddlepaddle', 'x2paddle'))
+ import x2paddle # noqa
+ from x2paddle.convert import pytorch2paddle # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with X2Paddle {x2paddle.__version__}...')
+ f = str(self.file).replace(self.file.suffix, f'_paddle_model{os.sep}')
+
+ pytorch2paddle(module=self.model, save_dir=f, jit_type='trace', input_examples=[self.im]) # export
+ yaml_save(Path(f) / 'metadata.yaml', self.metadata) # add metadata.yaml
+ return f, None
+
+ @try_export
+ def export_ncnn(self, prefix=colorstr('NCNN:')):
+ """
+ YOLOv8 NCNN export using PNNX https://github.com/pnnx/pnnx.
+ """
+ check_requirements('git+https://github.com/Tencent/ncnn.git' if ARM64 else 'ncnn') # requires NCNN
+ import ncnn # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with NCNN {ncnn.__version__}...')
+ f = Path(str(self.file).replace(self.file.suffix, f'_ncnn_model{os.sep}'))
+ f_ts = str(self.file.with_suffix('.torchscript'))
+
+ if Path('./pnnx').is_file():
+ pnnx = './pnnx'
+ elif (ROOT / 'pnnx').is_file():
+ pnnx = ROOT / 'pnnx'
+ else:
+ LOGGER.warning(
+ f'{prefix} WARNING ⚠️ PNNX not found. Attempting to download binary file from '
+ 'https://github.com/pnnx/pnnx/.\nNote PNNX Binary file must be placed in current working directory '
+ f'or in {ROOT}. See PNNX repo for full installation instructions.')
+ _, assets = get_github_assets(repo='pnnx/pnnx')
+ asset = [x for x in assets if ('macos' if MACOS else 'ubuntu' if LINUX else 'windows') in x][0]
+ attempt_download_asset(asset, repo='pnnx/pnnx', release='latest')
+ unzip_dir = Path(asset).with_suffix('')
+ pnnx = ROOT / 'pnnx' # new location
+ (unzip_dir / 'pnnx').rename(pnnx) # move binary to ROOT
+ shutil.rmtree(unzip_dir) # delete unzip dir
+ Path(asset).unlink() # delete zip
+ pnnx.chmod(0o777) # set read, write, and execute permissions for everyone
+
+ cmd = [
+ str(pnnx),
+ f_ts,
+ f'pnnxparam={f / "model.pnnx.param"}',
+ f'pnnxbin={f / "model.pnnx.bin"}',
+ f'pnnxpy={f / "model_pnnx.py"}',
+ f'pnnxonnx={f / "model.pnnx.onnx"}',
+ f'ncnnparam={f / "model.ncnn.param"}',
+ f'ncnnbin={f / "model.ncnn.bin"}',
+ f'ncnnpy={f / "model_ncnn.py"}',
+ f'fp16={int(self.args.half)}',
+ f'device={self.device.type}',
+ f'inputshape="{[self.args.batch, 3, *self.imgsz]}"', ]
+ f.mkdir(exist_ok=True) # make ncnn_model directory
+ LOGGER.info(f"{prefix} running '{' '.join(cmd)}'")
+ subprocess.run(cmd, check=True)
+
+ yaml_save(f / 'metadata.yaml', self.metadata) # add metadata.yaml
+ return str(f), None
+
+ @try_export
+ def export_coreml(self, prefix=colorstr('CoreML:')):
+ """YOLOv8 CoreML export."""
+ check_requirements('coremltools>=6.0')
+ import coremltools as ct # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with coremltools {ct.__version__}...')
+ f = self.file.with_suffix('.mlmodel')
+
+ bias = [0.0, 0.0, 0.0]
+ scale = 1 / 255
+ classifier_config = None
+ if self.model.task == 'classify':
+ classifier_config = ct.ClassifierConfig(list(self.model.names.values())) if self.args.nms else None
+ model = self.model
+ elif self.model.task == 'detect':
+ model = iOSDetectModel(self.model, self.im) if self.args.nms else self.model
+ else:
+ # TODO CoreML Segment and Pose model pipelining
+ model = self.model
+
+ ts = torch.jit.trace(model.eval(), self.im, strict=False) # TorchScript model
+ ct_model = ct.convert(ts,
+ inputs=[ct.ImageType('image', shape=self.im.shape, scale=scale, bias=bias)],
+ classifier_config=classifier_config)
+ bits, mode = (8, 'kmeans_lut') if self.args.int8 else (16, 'linear') if self.args.half else (32, None)
+ if bits < 32:
+ if 'kmeans' in mode:
+ check_requirements('scikit-learn') # scikit-learn package required for k-means quantization
+ ct_model = ct.models.neural_network.quantization_utils.quantize_weights(ct_model, bits, mode)
+ if self.args.nms and self.model.task == 'detect':
+ ct_model = self._pipeline_coreml(ct_model)
+
+ m = self.metadata # metadata dict
+ ct_model.short_description = m.pop('description')
+ ct_model.author = m.pop('author')
+ ct_model.license = m.pop('license')
+ ct_model.version = m.pop('version')
+ ct_model.user_defined_metadata.update({k: str(v) for k, v in m.items()})
+ ct_model.save(str(f))
+ return f, ct_model
+
+ @try_export
+ def export_engine(self, prefix=colorstr('TensorRT:')):
+ """YOLOv8 TensorRT export https://developer.nvidia.com/tensorrt."""
+ assert self.im.device.type != 'cpu', "export running on CPU but must be on GPU, i.e. use 'device=0'"
+ try:
+ import tensorrt as trt # noqa
+ except ImportError:
+ if LINUX:
+ check_requirements('nvidia-tensorrt', cmds='-U --index-url https://pypi.ngc.nvidia.com')
+ import tensorrt as trt # noqa
+
+ check_version(trt.__version__, '7.0.0', hard=True) # require tensorrt>=7.0.0
+ self.args.simplify = True
+ f_onnx, _ = self.export_onnx()
+
+ LOGGER.info(f'\n{prefix} starting export with TensorRT {trt.__version__}...')
+ assert Path(f_onnx).exists(), f'failed to export ONNX file: {f_onnx}'
+ f = self.file.with_suffix('.engine') # TensorRT engine file
+ logger = trt.Logger(trt.Logger.INFO)
+ if self.args.verbose:
+ logger.min_severity = trt.Logger.Severity.VERBOSE
+
+ builder = trt.Builder(logger)
+ config = builder.create_builder_config()
+ config.max_workspace_size = self.args.workspace * 1 << 30
+ # config.set_memory_pool_limit(trt.MemoryPoolType.WORKSPACE, workspace << 30) # fix TRT 8.4 deprecation notice
+
+ flag = (1 << int(trt.NetworkDefinitionCreationFlag.EXPLICIT_BATCH))
+ network = builder.create_network(flag)
+ parser = trt.OnnxParser(network, logger)
+ if not parser.parse_from_file(f_onnx):
+ raise RuntimeError(f'failed to load ONNX file: {f_onnx}')
+
+ inputs = [network.get_input(i) for i in range(network.num_inputs)]
+ outputs = [network.get_output(i) for i in range(network.num_outputs)]
+ for inp in inputs:
+ LOGGER.info(f'{prefix} input "{inp.name}" with shape{inp.shape} {inp.dtype}')
+ for out in outputs:
+ LOGGER.info(f'{prefix} output "{out.name}" with shape{out.shape} {out.dtype}')
+
+ if self.args.dynamic:
+ shape = self.im.shape
+ if shape[0] <= 1:
+ LOGGER.warning(f'{prefix} WARNING ⚠️ --dynamic model requires maximum --batch-size argument')
+ profile = builder.create_optimization_profile()
+ for inp in inputs:
+ profile.set_shape(inp.name, (1, *shape[1:]), (max(1, shape[0] // 2), *shape[1:]), shape)
+ config.add_optimization_profile(profile)
+
+ LOGGER.info(
+ f'{prefix} building FP{16 if builder.platform_has_fast_fp16 and self.args.half else 32} engine as {f}')
+ if builder.platform_has_fast_fp16 and self.args.half:
+ config.set_flag(trt.BuilderFlag.FP16)
+
+ # Write file
+ with builder.build_engine(network, config) as engine, open(f, 'wb') as t:
+ # Metadata
+ meta = json.dumps(self.metadata)
+ t.write(len(meta).to_bytes(4, byteorder='little', signed=True))
+ t.write(meta.encode())
+ # Model
+ t.write(engine.serialize())
+
+ return f, None
+
+ @try_export
+ def export_saved_model(self, prefix=colorstr('TensorFlow SavedModel:')):
+ """YOLOv8 TensorFlow SavedModel export."""
+ try:
+ import tensorflow as tf # noqa
+ except ImportError:
+ cuda = torch.cuda.is_available()
+ check_requirements(f"tensorflow{'-macos' if MACOS else '-aarch64' if ARM64 else '' if cuda else '-cpu'}")
+ import tensorflow as tf # noqa
+ check_requirements(('onnx', 'onnx2tf>=1.7.7', 'sng4onnx>=1.0.1', 'onnxsim>=0.4.17', 'onnx_graphsurgeon>=0.3.26',
+ 'tflite_support', 'onnxruntime-gpu' if torch.cuda.is_available() else 'onnxruntime'),
+ cmds='--extra-index-url https://pypi.ngc.nvidia.com')
+
+ LOGGER.info(f'\n{prefix} starting export with tensorflow {tf.__version__}...')
+ f = Path(str(self.file).replace(self.file.suffix, '_saved_model'))
+ if f.is_dir():
+ import shutil
+ shutil.rmtree(f) # delete output folder
+
+ # Export to ONNX
+ self.args.simplify = True
+ f_onnx, _ = self.export_onnx()
+
+ # Export to TF
+ int8 = '-oiqt -qt per-tensor' if self.args.int8 else ''
+ cmd = f'onnx2tf -i {f_onnx} -o {f} -nuo --non_verbose {int8}'
+ LOGGER.info(f"\n{prefix} running '{cmd.strip()}'")
+ subprocess.run(cmd, shell=True)
+ yaml_save(f / 'metadata.yaml', self.metadata) # add metadata.yaml
+
+ # Remove/rename TFLite models
+ if self.args.int8:
+ for file in f.rglob('*_dynamic_range_quant.tflite'):
+ file.rename(file.with_name(file.stem.replace('_dynamic_range_quant', '_int8') + file.suffix))
+ for file in f.rglob('*_integer_quant_with_int16_act.tflite'):
+ file.unlink() # delete extra fp16 activation TFLite files
+
+ # Add TFLite metadata
+ for file in f.rglob('*.tflite'):
+ f.unlink() if 'quant_with_int16_act.tflite' in str(f) else self._add_tflite_metadata(file)
+
+ # Load saved_model
+ keras_model = tf.saved_model.load(f, tags=None, options=None)
+
+ return str(f), keras_model
+
+ @try_export
+ def export_pb(self, keras_model, prefix=colorstr('TensorFlow GraphDef:')):
+ """YOLOv8 TensorFlow GraphDef *.pb export https://github.com/leimao/Frozen_Graph_TensorFlow."""
+ import tensorflow as tf # noqa
+ from tensorflow.python.framework.convert_to_constants import convert_variables_to_constants_v2 # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with tensorflow {tf.__version__}...')
+ f = self.file.with_suffix('.pb')
+
+ m = tf.function(lambda x: keras_model(x)) # full model
+ m = m.get_concrete_function(tf.TensorSpec(keras_model.inputs[0].shape, keras_model.inputs[0].dtype))
+ frozen_func = convert_variables_to_constants_v2(m)
+ frozen_func.graph.as_graph_def()
+ tf.io.write_graph(graph_or_graph_def=frozen_func.graph, logdir=str(f.parent), name=f.name, as_text=False)
+ return f, None
+
+ @try_export
+ def export_tflite(self, keras_model, nms, agnostic_nms, prefix=colorstr('TensorFlow Lite:')):
+ """YOLOv8 TensorFlow Lite export."""
+ import tensorflow as tf # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with tensorflow {tf.__version__}...')
+ saved_model = Path(str(self.file).replace(self.file.suffix, '_saved_model'))
+ if self.args.int8:
+ f = saved_model / f'{self.file.stem}_int8.tflite' # fp32 in/out
+ elif self.args.half:
+ f = saved_model / f'{self.file.stem}_float16.tflite' # fp32 in/out
+ else:
+ f = saved_model / f'{self.file.stem}_float32.tflite'
+ return str(f), None
+
+ @try_export
+ def export_edgetpu(self, tflite_model='', prefix=colorstr('Edge TPU:')):
+ """YOLOv8 Edge TPU export https://coral.ai/docs/edgetpu/models-intro/."""
+ LOGGER.warning(f'{prefix} WARNING ⚠️ Edge TPU known bug https://github.com/ultralytics/ultralytics/issues/1185')
+
+ cmd = 'edgetpu_compiler --version'
+ help_url = 'https://coral.ai/docs/edgetpu/compiler/'
+ assert LINUX, f'export only supported on Linux. See {help_url}'
+ if subprocess.run(cmd, stdout=subprocess.DEVNULL, stderr=subprocess.DEVNULL, shell=True).returncode != 0:
+ LOGGER.info(f'\n{prefix} export requires Edge TPU compiler. Attempting install from {help_url}')
+ sudo = subprocess.run('sudo --version >/dev/null', shell=True).returncode == 0 # sudo installed on system
+ for c in (
+ 'curl https://packages.cloud.google.com/apt/doc/apt-key.gpg | sudo apt-key add -',
+ 'echo "deb https://packages.cloud.google.com/apt coral-edgetpu-stable main" | sudo tee /etc/apt/sources.list.d/coral-edgetpu.list',
+ 'sudo apt-get update', 'sudo apt-get install edgetpu-compiler'):
+ subprocess.run(c if sudo else c.replace('sudo ', ''), shell=True, check=True)
+ ver = subprocess.run(cmd, shell=True, capture_output=True, check=True).stdout.decode().split()[-1]
+
+ LOGGER.info(f'\n{prefix} starting export with Edge TPU compiler {ver}...')
+ f = str(tflite_model).replace('.tflite', '_edgetpu.tflite') # Edge TPU model
+
+ cmd = f'edgetpu_compiler -s -d -k 10 --out_dir {Path(f).parent} {tflite_model}'
+ LOGGER.info(f"{prefix} running '{cmd}'")
+ subprocess.run(cmd.split(), check=True)
+ self._add_tflite_metadata(f)
+ return f, None
+
+ @try_export
+ def export_tfjs(self, prefix=colorstr('TensorFlow.js:')):
+ """YOLOv8 TensorFlow.js export."""
+ check_requirements('tensorflowjs')
+ import tensorflow as tf
+ import tensorflowjs as tfjs # noqa
+
+ LOGGER.info(f'\n{prefix} starting export with tensorflowjs {tfjs.__version__}...')
+ f = str(self.file).replace(self.file.suffix, '_web_model') # js dir
+ f_pb = self.file.with_suffix('.pb') # *.pb path
+
+ gd = tf.Graph().as_graph_def() # TF GraphDef
+ with open(f_pb, 'rb') as file:
+ gd.ParseFromString(file.read())
+ outputs = ','.join(gd_outputs(gd))
+ LOGGER.info(f'\n{prefix} output node names: {outputs}')
+
+ cmd = f'tensorflowjs_converter --input_format=tf_frozen_model --output_node_names={outputs} {f_pb} {f}'
+ subprocess.run(cmd.split(), check=True)
+
+ # f_json = Path(f) / 'model.json' # *.json path
+ # with open(f_json, 'w') as j: # sort JSON Identity_* in ascending order
+ # subst = re.sub(
+ # r'{"outputs": {"Identity.?.?": {"name": "Identity.?.?"}, '
+ # r'"Identity.?.?": {"name": "Identity.?.?"}, '
+ # r'"Identity.?.?": {"name": "Identity.?.?"}, '
+ # r'"Identity.?.?": {"name": "Identity.?.?"}}}',
+ # r'{"outputs": {"Identity": {"name": "Identity"}, '
+ # r'"Identity_1": {"name": "Identity_1"}, '
+ # r'"Identity_2": {"name": "Identity_2"}, '
+ # r'"Identity_3": {"name": "Identity_3"}}}',
+ # f_json.read_text(),
+ # )
+ # j.write(subst)
+ yaml_save(Path(f) / 'metadata.yaml', self.metadata) # add metadata.yaml
+ return f, None
+
+ def _add_tflite_metadata(self, file):
+ """Add metadata to *.tflite models per https://www.tensorflow.org/lite/models/convert/metadata."""
+ from tflite_support import flatbuffers # noqa
+ from tflite_support import metadata as _metadata # noqa
+ from tflite_support import metadata_schema_py_generated as _metadata_fb # noqa
+
+ # Create model info
+ model_meta = _metadata_fb.ModelMetadataT()
+ model_meta.name = self.metadata['description']
+ model_meta.version = self.metadata['version']
+ model_meta.author = self.metadata['author']
+ model_meta.license = self.metadata['license']
+
+ # Label file
+ tmp_file = Path(file).parent / 'temp_meta.txt'
+ with open(tmp_file, 'w') as f:
+ f.write(str(self.metadata))
+
+ label_file = _metadata_fb.AssociatedFileT()
+ label_file.name = tmp_file.name
+ label_file.type = _metadata_fb.AssociatedFileType.TENSOR_AXIS_LABELS
+
+ # Create input info
+ input_meta = _metadata_fb.TensorMetadataT()
+ input_meta.name = 'image'
+ input_meta.description = 'Input image to be detected.'
+ input_meta.content = _metadata_fb.ContentT()
+ input_meta.content.contentProperties = _metadata_fb.ImagePropertiesT()
+ input_meta.content.contentProperties.colorSpace = _metadata_fb.ColorSpaceType.RGB
+ input_meta.content.contentPropertiesType = _metadata_fb.ContentProperties.ImageProperties
+
+ # Create output info
+ output1 = _metadata_fb.TensorMetadataT()
+ output1.name = 'output'
+ output1.description = 'Coordinates of detected objects, class labels, and confidence score'
+ output1.associatedFiles = [label_file]
+ if self.model.task == 'segment':
+ output2 = _metadata_fb.TensorMetadataT()
+ output2.name = 'output'
+ output2.description = 'Mask protos'
+ output2.associatedFiles = [label_file]
+
+ # Create subgraph info
+ subgraph = _metadata_fb.SubGraphMetadataT()
+ subgraph.inputTensorMetadata = [input_meta]
+ subgraph.outputTensorMetadata = [output1, output2] if self.model.task == 'segment' else [output1]
+ model_meta.subgraphMetadata = [subgraph]
+
+ b = flatbuffers.Builder(0)
+ b.Finish(model_meta.Pack(b), _metadata.MetadataPopulator.METADATA_FILE_IDENTIFIER)
+ metadata_buf = b.Output()
+
+ populator = _metadata.MetadataPopulator.with_model_file(str(file))
+ populator.load_metadata_buffer(metadata_buf)
+ populator.load_associated_files([str(tmp_file)])
+ populator.populate()
+ tmp_file.unlink()
+
+ def _pipeline_coreml(self, model, prefix=colorstr('CoreML Pipeline:')):
+ """YOLOv8 CoreML pipeline."""
+ import coremltools as ct # noqa
+
+ LOGGER.info(f'{prefix} starting pipeline with coremltools {ct.__version__}...')
+ batch_size, ch, h, w = list(self.im.shape) # BCHW
+
+ # Output shapes
+ spec = model.get_spec()
+ out0, out1 = iter(spec.description.output)
+ if MACOS:
+ from PIL import Image
+ img = Image.new('RGB', (w, h)) # img(192 width, 320 height)
+ # img = torch.zeros((*opt.img_size, 3)).numpy() # img size(320,192,3) iDetection
+ out = model.predict({'image': img})
+ out0_shape = out[out0.name].shape
+ out1_shape = out[out1.name].shape
+ else: # linux and windows can not run model.predict(), get sizes from pytorch output y
+ out0_shape = self.output_shape[2], self.output_shape[1] - 4 # (3780, 80)
+ out1_shape = self.output_shape[2], 4 # (3780, 4)
+
+ # Checks
+ names = self.metadata['names']
+ nx, ny = spec.description.input[0].type.imageType.width, spec.description.input[0].type.imageType.height
+ na, nc = out0_shape
+ # na, nc = out0.type.multiArrayType.shape # number anchors, classes
+ assert len(names) == nc, f'{len(names)} names found for nc={nc}' # check
+
+ # Define output shapes (missing)
+ out0.type.multiArrayType.shape[:] = out0_shape # (3780, 80)
+ out1.type.multiArrayType.shape[:] = out1_shape # (3780, 4)
+ # spec.neuralNetwork.preprocessing[0].featureName = '0'
+
+ # Flexible input shapes
+ # from coremltools.models.neural_network import flexible_shape_utils
+ # s = [] # shapes
+ # s.append(flexible_shape_utils.NeuralNetworkImageSize(320, 192))
+ # s.append(flexible_shape_utils.NeuralNetworkImageSize(640, 384)) # (height, width)
+ # flexible_shape_utils.add_enumerated_image_sizes(spec, feature_name='image', sizes=s)
+ # r = flexible_shape_utils.NeuralNetworkImageSizeRange() # shape ranges
+ # r.add_height_range((192, 640))
+ # r.add_width_range((192, 640))
+ # flexible_shape_utils.update_image_size_range(spec, feature_name='image', size_range=r)
+
+ # Print
+ # print(spec.description)
+
+ # Model from spec
+ model = ct.models.MLModel(spec)
+
+ # 3. Create NMS protobuf
+ nms_spec = ct.proto.Model_pb2.Model()
+ nms_spec.specificationVersion = 5
+ for i in range(2):
+ decoder_output = model._spec.description.output[i].SerializeToString()
+ nms_spec.description.input.add()
+ nms_spec.description.input[i].ParseFromString(decoder_output)
+ nms_spec.description.output.add()
+ nms_spec.description.output[i].ParseFromString(decoder_output)
+
+ nms_spec.description.output[0].name = 'confidence'
+ nms_spec.description.output[1].name = 'coordinates'
+
+ output_sizes = [nc, 4]
+ for i in range(2):
+ ma_type = nms_spec.description.output[i].type.multiArrayType
+ ma_type.shapeRange.sizeRanges.add()
+ ma_type.shapeRange.sizeRanges[0].lowerBound = 0
+ ma_type.shapeRange.sizeRanges[0].upperBound = -1
+ ma_type.shapeRange.sizeRanges.add()
+ ma_type.shapeRange.sizeRanges[1].lowerBound = output_sizes[i]
+ ma_type.shapeRange.sizeRanges[1].upperBound = output_sizes[i]
+ del ma_type.shape[:]
+
+ nms = nms_spec.nonMaximumSuppression
+ nms.confidenceInputFeatureName = out0.name # 1x507x80
+ nms.coordinatesInputFeatureName = out1.name # 1x507x4
+ nms.confidenceOutputFeatureName = 'confidence'
+ nms.coordinatesOutputFeatureName = 'coordinates'
+ nms.iouThresholdInputFeatureName = 'iouThreshold'
+ nms.confidenceThresholdInputFeatureName = 'confidenceThreshold'
+ nms.iouThreshold = 0.45
+ nms.confidenceThreshold = 0.25
+ nms.pickTop.perClass = True
+ nms.stringClassLabels.vector.extend(names.values())
+ nms_model = ct.models.MLModel(nms_spec)
+
+ # 4. Pipeline models together
+ pipeline = ct.models.pipeline.Pipeline(input_features=[('image', ct.models.datatypes.Array(3, ny, nx)),
+ ('iouThreshold', ct.models.datatypes.Double()),
+ ('confidenceThreshold', ct.models.datatypes.Double())],
+ output_features=['confidence', 'coordinates'])
+ pipeline.add_model(model)
+ pipeline.add_model(nms_model)
+
+ # Correct datatypes
+ pipeline.spec.description.input[0].ParseFromString(model._spec.description.input[0].SerializeToString())
+ pipeline.spec.description.output[0].ParseFromString(nms_model._spec.description.output[0].SerializeToString())
+ pipeline.spec.description.output[1].ParseFromString(nms_model._spec.description.output[1].SerializeToString())
+
+ # Update metadata
+ pipeline.spec.specificationVersion = 5
+ pipeline.spec.description.metadata.userDefined.update({
+ 'IoU threshold': str(nms.iouThreshold),
+ 'Confidence threshold': str(nms.confidenceThreshold)})
+
+ # Save the model
+ model = ct.models.MLModel(pipeline.spec)
+ model.input_description['image'] = 'Input image'
+ model.input_description['iouThreshold'] = f'(optional) IOU threshold override (default: {nms.iouThreshold})'
+ model.input_description['confidenceThreshold'] = \
+ f'(optional) Confidence threshold override (default: {nms.confidenceThreshold})'
+ model.output_description['confidence'] = 'Boxes × Class confidence (see user-defined metadata "classes")'
+ model.output_description['coordinates'] = 'Boxes × [x, y, width, height] (relative to image size)'
+ LOGGER.info(f'{prefix} pipeline success')
+ return model
+
+ def add_callback(self, event: str, callback):
+ """
+ Appends the given callback.
+ """
+ self.callbacks[event].append(callback)
+
+ def run_callbacks(self, event: str):
+ """Execute all callbacks for a given event."""
+ for callback in self.callbacks.get(event, []):
+ callback(self)
+
+
+class iOSDetectModel(torch.nn.Module):
+ """Wrap an Ultralytics YOLO model for iOS export."""
+
+ def __init__(self, model, im):
+ """Initialize the iOSDetectModel class with a YOLO model and example image."""
+ super().__init__()
+ b, c, h, w = im.shape # batch, channel, height, width
+ self.model = model
+ self.nc = len(model.names) # number of classes
+ if w == h:
+ self.normalize = 1.0 / w # scalar
+ else:
+ self.normalize = torch.tensor([1.0 / w, 1.0 / h, 1.0 / w, 1.0 / h]) # broadcast (slower, smaller)
+
+ def forward(self, x):
+ """Normalize predictions of object detection model with input size-dependent factors."""
+ xywh, cls = self.model(x)[0].transpose(0, 1).split((4, self.nc), 1)
+ return cls, xywh * self.normalize # confidence (3780, 80), coordinates (3780, 4)
+
+
+def export(cfg=DEFAULT_CFG):
+ """Export a YOLOv model to a specific format."""
+ cfg.model = cfg.model or 'yolov8n.yaml'
+ cfg.format = cfg.format or 'torchscript'
+
+ from ultralytics import YOLO
+ model = YOLO(cfg.model)
+ model.export(**vars(cfg))
+
+
+if __name__ == '__main__':
+ """
+ CLI:
+ yolo mode=export model=yolov8n.yaml format=onnx
+ """
+ export()
diff --git a/yolov8/ultralytics/yolo/engine/model.py b/yolov8/ultralytics/yolo/engine/model.py
new file mode 100644
index 0000000000000000000000000000000000000000..2019566fb453085f7938b2fa3fc32d972dda131e
--- /dev/null
+++ b/yolov8/ultralytics/yolo/engine/model.py
@@ -0,0 +1,444 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import sys
+from pathlib import Path
+from typing import Union
+
+from ultralytics import yolo # noqa
+from ultralytics.nn.tasks import (ClassificationModel, DetectionModel, PoseModel, SegmentationModel,
+ attempt_load_one_weight, guess_model_task, nn, yaml_model_load)
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.engine.exporter import Exporter
+from ultralytics.yolo.utils import (DEFAULT_CFG, DEFAULT_CFG_DICT, DEFAULT_CFG_KEYS, LOGGER, RANK, ROOT, callbacks,
+ is_git_dir, yaml_load)
+from ultralytics.yolo.utils.checks import check_file, check_imgsz, check_pip_update_available, check_yaml
+from ultralytics.yolo.utils.downloads import GITHUB_ASSET_STEMS
+from ultralytics.yolo.utils.torch_utils import smart_inference_mode
+
+# Map head to model, trainer, validator, and predictor classes
+TASK_MAP = {
+ 'classify': [
+ ClassificationModel, yolo.v8.classify.ClassificationTrainer, yolo.v8.classify.ClassificationValidator,
+ yolo.v8.classify.ClassificationPredictor],
+ 'detect': [
+ DetectionModel, yolo.v8.detect.DetectionTrainer, yolo.v8.detect.DetectionValidator,
+ yolo.v8.detect.DetectionPredictor],
+ 'segment': [
+ SegmentationModel, yolo.v8.segment.SegmentationTrainer, yolo.v8.segment.SegmentationValidator,
+ yolo.v8.segment.SegmentationPredictor],
+ 'pose': [PoseModel, yolo.v8.pose.PoseTrainer, yolo.v8.pose.PoseValidator, yolo.v8.pose.PosePredictor]}
+
+
+class YOLO:
+ """
+ YOLO (You Only Look Once) object detection model.
+
+ Args:
+ model (str, Path): Path to the model file to load or create.
+ task (Any, optional): Task type for the YOLO model. Defaults to None.
+
+ Attributes:
+ predictor (Any): The predictor object.
+ model (Any): The model object.
+ trainer (Any): The trainer object.
+ task (str): The type of model task.
+ ckpt (Any): The checkpoint object if the model loaded from *.pt file.
+ cfg (str): The model configuration if loaded from *.yaml file.
+ ckpt_path (str): The checkpoint file path.
+ overrides (dict): Overrides for the trainer object.
+ metrics (Any): The data for metrics.
+
+ Methods:
+ __call__(source=None, stream=False, **kwargs):
+ Alias for the predict method.
+ _new(cfg:str, verbose:bool=True) -> None:
+ Initializes a new model and infers the task type from the model definitions.
+ _load(weights:str, task:str='') -> None:
+ Initializes a new model and infers the task type from the model head.
+ _check_is_pytorch_model() -> None:
+ Raises TypeError if the model is not a PyTorch model.
+ reset() -> None:
+ Resets the model modules.
+ info(verbose:bool=False) -> None:
+ Logs the model info.
+ fuse() -> None:
+ Fuses the model for faster inference.
+ predict(source=None, stream=False, **kwargs) -> List[ultralytics.yolo.engine.results.Results]:
+ Performs prediction using the YOLO model.
+
+ Returns:
+ list(ultralytics.yolo.engine.results.Results): The prediction results.
+ """
+
+ def __init__(self, model: Union[str, Path] = 'yolov8n.pt', task=None) -> None:
+ """
+ Initializes the YOLO model.
+
+ Args:
+ model (Union[str, Path], optional): Path or name of the model to load or create. Defaults to 'yolov8n.pt'.
+ task (Any, optional): Task type for the YOLO model. Defaults to None.
+ """
+ self.callbacks = callbacks.get_default_callbacks()
+ self.predictor = None # reuse predictor
+ self.model = None # model object
+ self.trainer = None # trainer object
+ self.task = None # task type
+ self.ckpt = None # if loaded from *.pt
+ self.cfg = None # if loaded from *.yaml
+ self.ckpt_path = None
+ self.overrides = {} # overrides for trainer object
+ self.metrics = None # validation/training metrics
+ self.session = None # HUB session
+ model = str(model).strip() # strip spaces
+
+ # Check if Ultralytics HUB model from https://hub.ultralytics.com
+ if self.is_hub_model(model):
+ from ultralytics.hub.session import HUBTrainingSession
+ self.session = HUBTrainingSession(model)
+ model = self.session.model_file
+
+ # Load or create new YOLO model
+ suffix = Path(model).suffix
+ if not suffix and Path(model).stem in GITHUB_ASSET_STEMS:
+ model, suffix = Path(model).with_suffix('.pt'), '.pt' # add suffix, i.e. yolov8n -> yolov8n.pt
+ if suffix == '.yaml':
+ self._new(model, task)
+ else:
+ self._load(model, task)
+
+ def __call__(self, source=None, stream=False, **kwargs):
+ """Calls the 'predict' function with given arguments to perform object detection."""
+ return self.predict(source, stream, **kwargs)
+
+ def __getattr__(self, attr):
+ """Raises error if object has no requested attribute."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
+
+ @staticmethod
+ def is_hub_model(model):
+ """Check if the provided model is a HUB model."""
+ return any((
+ model.startswith('https://hub.ultralytics.com/models/'), # i.e. https://hub.ultralytics.com/models/MODEL_ID
+ [len(x) for x in model.split('_')] == [42, 20], # APIKEY_MODELID
+ len(model) == 20 and not Path(model).exists() and all(x not in model for x in './\\'))) # MODELID
+
+ def _new(self, cfg: str, task=None, verbose=True):
+ """
+ Initializes a new model and infers the task type from the model definitions.
+
+ Args:
+ cfg (str): model configuration file
+ task (str | None): model task
+ verbose (bool): display model info on load
+ """
+ cfg_dict = yaml_model_load(cfg)
+ self.cfg = cfg
+ self.task = task or guess_model_task(cfg_dict)
+ self.model = TASK_MAP[self.task][0](cfg_dict, verbose=verbose and RANK == -1) # build model
+ self.overrides['model'] = self.cfg
+
+ # Below added to allow export from yamls
+ args = {**DEFAULT_CFG_DICT, **self.overrides} # combine model and default args, preferring model args
+ self.model.args = {k: v for k, v in args.items() if k in DEFAULT_CFG_KEYS} # attach args to model
+ self.model.task = self.task
+
+ def _load(self, weights: str, task=None):
+ """
+ Initializes a new model and infers the task type from the model head.
+
+ Args:
+ weights (str): model checkpoint to be loaded
+ task (str | None): model task
+ """
+ suffix = Path(weights).suffix
+ if suffix == '.pt':
+ self.model, self.ckpt = attempt_load_one_weight(weights)
+ self.task = self.model.args['task']
+ self.overrides = self.model.args = self._reset_ckpt_args(self.model.args)
+ self.ckpt_path = self.model.pt_path
+ else:
+ weights = check_file(weights)
+ self.model, self.ckpt = weights, None
+ self.task = task or guess_model_task(weights)
+ self.ckpt_path = weights
+ self.overrides['model'] = weights
+ self.overrides['task'] = self.task
+
+ def _check_is_pytorch_model(self):
+ """
+ Raises TypeError is model is not a PyTorch model
+ """
+ pt_str = isinstance(self.model, (str, Path)) and Path(self.model).suffix == '.pt'
+ pt_module = isinstance(self.model, nn.Module)
+ if not (pt_module or pt_str):
+ raise TypeError(f"model='{self.model}' must be a *.pt PyTorch model, but is a different type. "
+ f'PyTorch models can be used to train, val, predict and export, i.e. '
+ f"'yolo export model=yolov8n.pt', but exported formats like ONNX, TensorRT etc. only "
+ f"support 'predict' and 'val' modes, i.e. 'yolo predict model=yolov8n.onnx'.")
+
+ @smart_inference_mode()
+ def reset_weights(self):
+ """
+ Resets the model modules parameters to randomly initialized values, losing all training information.
+ """
+ self._check_is_pytorch_model()
+ for m in self.model.modules():
+ if hasattr(m, 'reset_parameters'):
+ m.reset_parameters()
+ for p in self.model.parameters():
+ p.requires_grad = True
+ return self
+
+ @smart_inference_mode()
+ def load(self, weights='yolov8n.pt'):
+ """
+ Transfers parameters with matching names and shapes from 'weights' to model.
+ """
+ self._check_is_pytorch_model()
+ if isinstance(weights, (str, Path)):
+ weights, self.ckpt = attempt_load_one_weight(weights)
+ self.model.load(weights)
+ return self
+
+ def info(self, detailed=False, verbose=True):
+ """
+ Logs model info.
+
+ Args:
+ detailed (bool): Show detailed information about model.
+ verbose (bool): Controls verbosity.
+ """
+ self._check_is_pytorch_model()
+ return self.model.info(detailed=detailed, verbose=verbose)
+
+ def fuse(self):
+ """Fuse PyTorch Conv2d and BatchNorm2d layers."""
+ self._check_is_pytorch_model()
+ self.model.fuse()
+
+ @smart_inference_mode()
+ def predict(self, source=None, stream=False, **kwargs):
+ """
+ Perform prediction using the YOLO model.
+
+ Args:
+ source (str | int | PIL | np.ndarray): The source of the image to make predictions on.
+ Accepts all source types accepted by the YOLO model.
+ stream (bool): Whether to stream the predictions or not. Defaults to False.
+ **kwargs : Additional keyword arguments passed to the predictor.
+ Check the 'configuration' section in the documentation for all available options.
+
+ Returns:
+ (List[ultralytics.yolo.engine.results.Results]): The prediction results.
+ """
+ if source is None:
+ source = ROOT / 'assets' if is_git_dir() else 'https://ultralytics.com/images/bus.jpg'
+ LOGGER.warning(f"WARNING ⚠️ 'source' is missing. Using 'source={source}'.")
+ is_cli = (sys.argv[0].endswith('yolo') or sys.argv[0].endswith('ultralytics')) and any(
+ x in sys.argv for x in ('predict', 'track', 'mode=predict', 'mode=track'))
+ overrides = self.overrides.copy()
+ overrides['conf'] = 0.25
+ overrides.update(kwargs) # prefer kwargs
+ overrides['mode'] = kwargs.get('mode', 'predict')
+ assert overrides['mode'] in ['track', 'predict']
+ if not is_cli:
+ overrides['save'] = kwargs.get('save', False) # do not save by default if called in Python
+ if not self.predictor:
+ self.task = overrides.get('task') or self.task
+ self.predictor = TASK_MAP[self.task][3](overrides=overrides, _callbacks=self.callbacks)
+ self.predictor.setup_model(model=self.model, verbose=is_cli)
+ else: # only update args if predictor is already setup
+ self.predictor.args = get_cfg(self.predictor.args, overrides)
+ if 'project' in overrides or 'name' in overrides:
+ self.predictor.save_dir = self.predictor.get_save_dir()
+ return self.predictor.predict_cli(source=source) if is_cli else self.predictor(source=source, stream=stream)
+
+ def track(self, source=None, stream=False, persist=False, **kwargs):
+ """
+ Perform object tracking on the input source using the registered trackers.
+
+ Args:
+ source (str, optional): The input source for object tracking. Can be a file path or a video stream.
+ stream (bool, optional): Whether the input source is a video stream. Defaults to False.
+ persist (bool, optional): Whether to persist the trackers if they already exist. Defaults to False.
+ **kwargs (optional): Additional keyword arguments for the tracking process.
+
+ Returns:
+ (List[ultralytics.yolo.engine.results.Results]): The tracking results.
+
+ """
+ if not hasattr(self.predictor, 'trackers'):
+ from ultralytics.tracker import register_tracker
+ register_tracker(self, persist)
+ # ByteTrack-based method needs low confidence predictions as input
+ conf = kwargs.get('conf') or 0.1
+ kwargs['conf'] = conf
+ kwargs['mode'] = 'track'
+ return self.predict(source=source, stream=stream, **kwargs)
+
+ @smart_inference_mode()
+ def val(self, data=None, **kwargs):
+ """
+ Validate a model on a given dataset.
+
+ Args:
+ data (str): The dataset to validate on. Accepts all formats accepted by yolo
+ **kwargs : Any other args accepted by the validators. To see all args check 'configuration' section in docs
+ """
+ overrides = self.overrides.copy()
+ overrides['rect'] = True # rect batches as default
+ overrides.update(kwargs)
+ overrides['mode'] = 'val'
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.data = data or args.data
+ if 'task' in overrides:
+ self.task = args.task
+ else:
+ args.task = self.task
+ if args.imgsz == DEFAULT_CFG.imgsz and not isinstance(self.model, (str, Path)):
+ args.imgsz = self.model.args['imgsz'] # use trained imgsz unless custom value is passed
+ args.imgsz = check_imgsz(args.imgsz, max_dim=1)
+
+ validator = TASK_MAP[self.task][2](args=args, _callbacks=self.callbacks)
+ validator(model=self.model)
+ self.metrics = validator.metrics
+
+ return validator.metrics
+
+ @smart_inference_mode()
+ def benchmark(self, **kwargs):
+ """
+ Benchmark a model on all export formats.
+
+ Args:
+ **kwargs : Any other args accepted by the validators. To see all args check 'configuration' section in docs
+ """
+ self._check_is_pytorch_model()
+ from ultralytics.yolo.utils.benchmarks import benchmark
+ overrides = self.model.args.copy()
+ overrides.update(kwargs)
+ overrides['mode'] = 'benchmark'
+ overrides = {**DEFAULT_CFG_DICT, **overrides} # fill in missing overrides keys with defaults
+ return benchmark(model=self, imgsz=overrides['imgsz'], half=overrides['half'], device=overrides['device'])
+
+ def export(self, **kwargs):
+ """
+ Export model.
+
+ Args:
+ **kwargs : Any other args accepted by the predictors. To see all args check 'configuration' section in docs
+ """
+ self._check_is_pytorch_model()
+ overrides = self.overrides.copy()
+ overrides.update(kwargs)
+ overrides['mode'] = 'export'
+ if overrides.get('imgsz') is None:
+ overrides['imgsz'] = self.model.args['imgsz'] # use trained imgsz unless custom value is passed
+ if 'batch' not in kwargs:
+ overrides['batch'] = 1 # default to 1 if not modified
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.task = self.task
+ return Exporter(overrides=args, _callbacks=self.callbacks)(model=self.model)
+
+ def train(self, **kwargs):
+ """
+ Trains the model on a given dataset.
+
+ Args:
+ **kwargs (Any): Any number of arguments representing the training configuration.
+ """
+ self._check_is_pytorch_model()
+ if self.session: # Ultralytics HUB session
+ if any(kwargs):
+ LOGGER.warning('WARNING ⚠️ using HUB training arguments, ignoring local training arguments.')
+ kwargs = self.session.train_args
+ check_pip_update_available()
+ overrides = self.overrides.copy()
+ if kwargs.get('cfg'):
+ LOGGER.info(f"cfg file passed. Overriding default params with {kwargs['cfg']}.")
+ overrides = yaml_load(check_yaml(kwargs['cfg']))
+ overrides.update(kwargs)
+ overrides['mode'] = 'train'
+ if not overrides.get('data'):
+ raise AttributeError("Dataset required but missing, i.e. pass 'data=coco128.yaml'")
+ if overrides.get('resume'):
+ overrides['resume'] = self.ckpt_path
+ self.task = overrides.get('task') or self.task
+ self.trainer = TASK_MAP[self.task][1](overrides=overrides, _callbacks=self.callbacks)
+ if not overrides.get('resume'): # manually set model only if not resuming
+ self.trainer.model = self.trainer.get_model(weights=self.model if self.ckpt else None, cfg=self.model.yaml)
+ self.model = self.trainer.model
+ self.trainer.hub_session = self.session # attach optional HUB session
+ self.trainer.train()
+ # Update model and cfg after training
+ if RANK in (-1, 0):
+ self.model, _ = attempt_load_one_weight(str(self.trainer.best))
+ self.overrides = self.model.args
+ self.metrics = getattr(self.trainer.validator, 'metrics', None) # TODO: no metrics returned by DDP
+
+ def to(self, device):
+ """
+ Sends the model to the given device.
+
+ Args:
+ device (str): device
+ """
+ self._check_is_pytorch_model()
+ self.model.to(device)
+
+ def tune(self, *args, **kwargs):
+ """
+ Runs hyperparameter tuning using Ray Tune.
+
+ Args:
+ data (str): The dataset to run the tuner on.
+ space (dict, optional): The hyperparameter search space. Defaults to None.
+ grace_period (int, optional): The grace period in epochs of the ASHA scheduler. Defaults to 10.
+ gpu_per_trial (int, optional): The number of GPUs to allocate per trial. Defaults to None.
+ max_samples (int, optional): The maximum number of trials to run. Defaults to 10.
+ train_args (dict, optional): Additional arguments to pass to the `train()` method. Defaults to {}.
+
+ Returns:
+ (dict): A dictionary containing the results of the hyperparameter search.
+
+ Raises:
+ ModuleNotFoundError: If Ray Tune is not installed.
+ """
+ self._check_is_pytorch_model()
+ from ultralytics.yolo.utils.tuner import run_ray_tune
+ return run_ray_tune(self, *args, **kwargs)
+
+ @property
+ def names(self):
+ """Returns class names of the loaded model."""
+ return self.model.names if hasattr(self.model, 'names') else None
+
+ @property
+ def device(self):
+ """Returns device if PyTorch model."""
+ return next(self.model.parameters()).device if isinstance(self.model, nn.Module) else None
+
+ @property
+ def transforms(self):
+ """Returns transform of the loaded model."""
+ return self.model.transforms if hasattr(self.model, 'transforms') else None
+
+ def add_callback(self, event: str, func):
+ """Add a callback."""
+ self.callbacks[event].append(func)
+
+ def clear_callback(self, event: str):
+ """Clear all event callbacks."""
+ self.callbacks[event] = []
+
+ @staticmethod
+ def _reset_ckpt_args(args):
+ """Reset arguments when loading a PyTorch model."""
+ include = {'imgsz', 'data', 'task', 'single_cls'} # only remember these arguments when loading a PyTorch model
+ return {k: v for k, v in args.items() if k in include}
+
+ def _reset_callbacks(self):
+ """Reset all registered callbacks."""
+ for event in callbacks.default_callbacks.keys():
+ self.callbacks[event] = [callbacks.default_callbacks[event][0]]
diff --git a/yolov8/ultralytics/yolo/engine/predictor.py b/yolov8/ultralytics/yolo/engine/predictor.py
new file mode 100644
index 0000000000000000000000000000000000000000..0f6eb0d3812cb74f6a5a6369c8d0c4772861208b
--- /dev/null
+++ b/yolov8/ultralytics/yolo/engine/predictor.py
@@ -0,0 +1,355 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Run prediction on images, videos, directories, globs, YouTube, webcam, streams, etc.
+
+Usage - sources:
+ $ yolo mode=predict model=yolov8n.pt source=0 # webcam
+ img.jpg # image
+ vid.mp4 # video
+ screen # screenshot
+ path/ # directory
+ list.txt # list of images
+ list.streams # list of streams
+ 'path/*.jpg' # glob
+ 'https://youtu.be/Zgi9g1ksQHc' # YouTube
+ 'rtsp://example.com/media.mp4' # RTSP, RTMP, HTTP stream
+
+Usage - formats:
+ $ yolo mode=predict model=yolov8n.pt # PyTorch
+ yolov8n.torchscript # TorchScript
+ yolov8n.onnx # ONNX Runtime or OpenCV DNN with dnn=True
+ yolov8n_openvino_model # OpenVINO
+ yolov8n.engine # TensorRT
+ yolov8n.mlmodel # CoreML (macOS-only)
+ yolov8n_saved_model # TensorFlow SavedModel
+ yolov8n.pb # TensorFlow GraphDef
+ yolov8n.tflite # TensorFlow Lite
+ yolov8n_edgetpu.tflite # TensorFlow Edge TPU
+ yolov8n_paddle_model # PaddlePaddle
+"""
+import platform
+from pathlib import Path
+
+import cv2
+import numpy as np
+import torch
+
+from ultralytics.nn.autobackend import AutoBackend
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.data import load_inference_source
+from ultralytics.yolo.data.augment import LetterBox, classify_transforms
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, MACOS, SETTINGS, WINDOWS, callbacks, colorstr, ops
+from ultralytics.yolo.utils.checks import check_imgsz, check_imshow
+from ultralytics.yolo.utils.files import increment_path
+from ultralytics.yolo.utils.torch_utils import select_device, smart_inference_mode
+
+STREAM_WARNING = """
+ WARNING ⚠️ stream/video/webcam/dir predict source will accumulate results in RAM unless `stream=True` is passed,
+ causing potential out-of-memory errors for large sources or long-running streams/videos.
+
+ Usage:
+ results = model(source=..., stream=True) # generator of Results objects
+ for r in results:
+ boxes = r.boxes # Boxes object for bbox outputs
+ masks = r.masks # Masks object for segment masks outputs
+ probs = r.probs # Class probabilities for classification outputs
+"""
+
+
+class BasePredictor:
+ """
+ BasePredictor
+
+ A base class for creating predictors.
+
+ Attributes:
+ args (SimpleNamespace): Configuration for the predictor.
+ save_dir (Path): Directory to save results.
+ done_warmup (bool): Whether the predictor has finished setup.
+ model (nn.Module): Model used for prediction.
+ data (dict): Data configuration.
+ device (torch.device): Device used for prediction.
+ dataset (Dataset): Dataset used for prediction.
+ vid_path (str): Path to video file.
+ vid_writer (cv2.VideoWriter): Video writer for saving video output.
+ data_path (str): Path to data.
+ """
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ """
+ Initializes the BasePredictor class.
+
+ Args:
+ cfg (str, optional): Path to a configuration file. Defaults to DEFAULT_CFG.
+ overrides (dict, optional): Configuration overrides. Defaults to None.
+ """
+ self.args = get_cfg(cfg, overrides)
+ self.save_dir = self.get_save_dir()
+ if self.args.conf is None:
+ self.args.conf = 0.25 # default conf=0.25
+ self.done_warmup = False
+ if self.args.show:
+ self.args.show = check_imshow(warn=True)
+
+ # Usable if setup is done
+ self.model = None
+ self.data = self.args.data # data_dict
+ self.imgsz = None
+ self.device = None
+ self.dataset = None
+ self.vid_path, self.vid_writer = None, None
+ self.plotted_img = None
+ self.data_path = None
+ self.source_type = None
+ self.batch = None
+ self.results = None
+ self.transforms = None
+ self.callbacks = _callbacks or callbacks.get_default_callbacks()
+ callbacks.add_integration_callbacks(self)
+
+ def get_save_dir(self):
+ project = self.args.project or Path(SETTINGS['runs_dir']) / self.args.task
+ name = self.args.name or f'{self.args.mode}'
+ return increment_path(Path(project) / name, exist_ok=self.args.exist_ok)
+
+ def preprocess(self, im):
+ """Prepares input image before inference.
+
+ Args:
+ im (torch.Tensor | List(np.ndarray)): BCHW for tensor, [(HWC) x B] for list.
+ """
+ not_tensor = not isinstance(im, torch.Tensor)
+ if not_tensor:
+ im = np.stack(self.pre_transform(im))
+ im = im[..., ::-1].transpose((0, 3, 1, 2)) # BGR to RGB, BHWC to BCHW, (n, 3, h, w)
+ im = np.ascontiguousarray(im) # contiguous
+ im = torch.from_numpy(im)
+
+ img = im.to(self.device)
+ img = img.half() if self.model.fp16 else img.float() # uint8 to fp16/32
+ if not_tensor:
+ img /= 255 # 0 - 255 to 0.0 - 1.0
+ return img
+
+ def pre_transform(self, im):
+ """Pre-transform input image before inference.
+
+ Args:
+ im (List(np.ndarray)): (N, 3, h, w) for tensor, [(h, w, 3) x N] for list.
+
+ Return: A list of transformed imgs.
+ """
+ same_shapes = all(x.shape == im[0].shape for x in im)
+ auto = same_shapes and self.model.pt
+ return [LetterBox(self.imgsz, auto=auto, stride=self.model.stride)(image=x) for x in im]
+
+ def write_results(self, idx, results, batch):
+ """Write inference results to a file or directory."""
+ p, im, _ = batch
+ log_string = ''
+ if len(im.shape) == 3:
+ im = im[None] # expand for batch dim
+ if self.source_type.webcam or self.source_type.from_img or self.source_type.tensor: # batch_size >= 1
+ log_string += f'{idx}: '
+ frame = self.dataset.count
+ else:
+ frame = getattr(self.dataset, 'frame', 0)
+ self.data_path = p
+ self.txt_path = str(self.save_dir / 'labels' / p.stem) + ('' if self.dataset.mode == 'image' else f'_{frame}')
+ log_string += '%gx%g ' % im.shape[2:] # print string
+ result = results[idx]
+ log_string += result.verbose()
+
+ if self.args.save or self.args.show: # Add bbox to image
+ plot_args = {
+ 'line_width': self.args.line_width,
+ 'boxes': self.args.boxes,
+ 'conf': self.args.show_conf,
+ 'labels': self.args.show_labels}
+ if not self.args.retina_masks:
+ plot_args['im_gpu'] = im[idx]
+ self.plotted_img = result.plot(**plot_args)
+ # Write
+ if self.args.save_txt:
+ result.save_txt(f'{self.txt_path}.txt', save_conf=self.args.save_conf)
+ if self.args.save_crop:
+ result.save_crop(save_dir=self.save_dir / 'crops', file_name=self.data_path.stem)
+
+ return log_string
+
+ def postprocess(self, preds, img, orig_imgs):
+ """Post-processes predictions for an image and returns them."""
+ return preds
+
+ def __call__(self, source=None, model=None, stream=False):
+ """Performs inference on an image or stream."""
+ self.stream = stream
+ if stream:
+ return self.stream_inference(source, model)
+ else:
+ return list(self.stream_inference(source, model)) # merge list of Result into one
+
+ def predict_cli(self, source=None, model=None):
+ """Method used for CLI prediction. It uses always generator as outputs as not required by CLI mode."""
+ gen = self.stream_inference(source, model)
+ for _ in gen: # running CLI inference without accumulating any outputs (do not modify)
+ pass
+
+ def setup_source(self, source):
+ """Sets up source and inference mode."""
+ self.imgsz = check_imgsz(self.args.imgsz, stride=self.model.stride, min_dim=2) # check image size
+ self.transforms = getattr(self.model.model, 'transforms', classify_transforms(
+ self.imgsz[0])) if self.args.task == 'classify' else None
+ self.dataset = load_inference_source(source=source, imgsz=self.imgsz, vid_stride=self.args.vid_stride)
+ self.source_type = self.dataset.source_type
+ if not getattr(self, 'stream', True) and (self.dataset.mode == 'stream' or # streams
+ len(self.dataset) > 1000 or # images
+ any(getattr(self.dataset, 'video_flag', [False]))): # videos
+ LOGGER.warning(STREAM_WARNING)
+ self.vid_path, self.vid_writer = [None] * self.dataset.bs, [None] * self.dataset.bs
+
+ @smart_inference_mode()
+ def stream_inference(self, source=None, model=None):
+ """Streams real-time inference on camera feed and saves results to file."""
+ if self.args.verbose:
+ LOGGER.info('')
+
+ # Setup model
+ if not self.model:
+ self.setup_model(model)
+
+ # Setup source every time predict is called
+ self.setup_source(source if source is not None else self.args.source)
+
+ # Check if save_dir/ label file exists
+ if self.args.save or self.args.save_txt:
+ (self.save_dir / 'labels' if self.args.save_txt else self.save_dir).mkdir(parents=True, exist_ok=True)
+
+ # Warmup model
+ if not self.done_warmup:
+ self.model.warmup(imgsz=(1 if self.model.pt or self.model.triton else self.dataset.bs, 3, *self.imgsz))
+ self.done_warmup = True
+
+ self.seen, self.windows, self.batch, profilers = 0, [], None, (ops.Profile(), ops.Profile(), ops.Profile())
+ self.run_callbacks('on_predict_start')
+ for batch in self.dataset:
+ self.run_callbacks('on_predict_batch_start')
+ self.batch = batch
+ path, im0s, vid_cap, s = batch
+ visualize = increment_path(self.save_dir / Path(path[0]).stem,
+ mkdir=True) if self.args.visualize and (not self.source_type.tensor) else False
+
+ # Preprocess
+ with profilers[0]:
+ im = self.preprocess(im0s)
+
+ # Inference
+ with profilers[1]:
+ preds = self.model(im, augment=self.args.augment, visualize=visualize)
+
+ # Postprocess
+ with profilers[2]:
+ self.results = self.postprocess(preds, im, im0s)
+ self.run_callbacks('on_predict_postprocess_end')
+
+ # Visualize, save, write results
+ n = len(im0s)
+ for i in range(n):
+ self.seen += 1
+ self.results[i].speed = {
+ 'preprocess': profilers[0].dt * 1E3 / n,
+ 'inference': profilers[1].dt * 1E3 / n,
+ 'postprocess': profilers[2].dt * 1E3 / n}
+ p, im0 = path[i], None if self.source_type.tensor else im0s[i].copy()
+ p = Path(p)
+
+ if self.args.verbose or self.args.save or self.args.save_txt or self.args.show:
+ s += self.write_results(i, self.results, (p, im, im0))
+ if self.args.save or self.args.save_txt:
+ self.results[i].save_dir = self.save_dir.__str__()
+ if self.args.show and self.plotted_img is not None:
+ self.show(p)
+ if self.args.save and self.plotted_img is not None:
+ self.save_preds(vid_cap, i, str(self.save_dir / p.name))
+
+ self.run_callbacks('on_predict_batch_end')
+ yield from self.results
+
+ # Print time (inference-only)
+ if self.args.verbose:
+ LOGGER.info(f'{s}{profilers[1].dt * 1E3:.1f}ms')
+
+ # Release assets
+ if isinstance(self.vid_writer[-1], cv2.VideoWriter):
+ self.vid_writer[-1].release() # release final video writer
+
+ # Print results
+ if self.args.verbose and self.seen:
+ t = tuple(x.t / self.seen * 1E3 for x in profilers) # speeds per image
+ LOGGER.info(f'Speed: %.1fms preprocess, %.1fms inference, %.1fms postprocess per image at shape '
+ f'{(1, 3, *im.shape[2:])}' % t)
+ if self.args.save or self.args.save_txt or self.args.save_crop:
+ nl = len(list(self.save_dir.glob('labels/*.txt'))) # number of labels
+ s = f"\n{nl} label{'s' * (nl > 1)} saved to {self.save_dir / 'labels'}" if self.args.save_txt else ''
+ LOGGER.info(f"Results saved to {colorstr('bold', self.save_dir)}{s}")
+
+ self.run_callbacks('on_predict_end')
+
+ def setup_model(self, model, verbose=True):
+ """Initialize YOLO model with given parameters and set it to evaluation mode."""
+ device = select_device(self.args.device, verbose=verbose)
+ model = model or self.args.model
+ self.args.half &= device.type != 'cpu' # half precision only supported on CUDA
+ self.model = AutoBackend(model,
+ device=device,
+ dnn=self.args.dnn,
+ data=self.args.data,
+ fp16=self.args.half,
+ fuse=True,
+ verbose=verbose)
+ self.device = device
+ self.model.eval()
+
+ def show(self, p):
+ """Display an image in a window using OpenCV imshow()."""
+ im0 = self.plotted_img
+ if platform.system() == 'Linux' and p not in self.windows:
+ self.windows.append(p)
+ cv2.namedWindow(str(p), cv2.WINDOW_NORMAL | cv2.WINDOW_KEEPRATIO) # allow window resize (Linux)
+ cv2.resizeWindow(str(p), im0.shape[1], im0.shape[0])
+ cv2.imshow(str(p), im0)
+ cv2.waitKey(500 if self.batch[3].startswith('image') else 1) # 1 millisecond
+
+ def save_preds(self, vid_cap, idx, save_path):
+ """Save video predictions as mp4 at specified path."""
+ im0 = self.plotted_img
+ # Save imgs
+ if self.dataset.mode == 'image':
+ cv2.imwrite(save_path, im0)
+ else: # 'video' or 'stream'
+ if self.vid_path[idx] != save_path: # new video
+ self.vid_path[idx] = save_path
+ if isinstance(self.vid_writer[idx], cv2.VideoWriter):
+ self.vid_writer[idx].release() # release previous video writer
+ if vid_cap: # video
+ fps = int(vid_cap.get(cv2.CAP_PROP_FPS)) # integer required, floats produce error in MP4 codec
+ w = int(vid_cap.get(cv2.CAP_PROP_FRAME_WIDTH))
+ h = int(vid_cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
+ else: # stream
+ fps, w, h = 30, im0.shape[1], im0.shape[0]
+ suffix = '.mp4' if MACOS else '.avi' if WINDOWS else '.avi'
+ fourcc = 'avc1' if MACOS else 'WMV2' if WINDOWS else 'MJPG'
+ save_path = str(Path(save_path).with_suffix(suffix))
+ self.vid_writer[idx] = cv2.VideoWriter(save_path, cv2.VideoWriter_fourcc(*fourcc), fps, (w, h))
+ self.vid_writer[idx].write(im0)
+
+ def run_callbacks(self, event: str):
+ """Runs all registered callbacks for a specific event."""
+ for callback in self.callbacks.get(event, []):
+ callback(self)
+
+ def add_callback(self, event: str, func):
+ """
+ Add callback
+ """
+ self.callbacks[event].append(func)
diff --git a/yolov8/ultralytics/yolo/engine/results.py b/yolov8/ultralytics/yolo/engine/results.py
new file mode 100644
index 0000000000000000000000000000000000000000..4e9f6f317894eeff0fbc3f1c5f9a1b5728aa49fc
--- /dev/null
+++ b/yolov8/ultralytics/yolo/engine/results.py
@@ -0,0 +1,608 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Ultralytics Results, Boxes and Masks classes for handling inference results
+
+Usage: See https://docs.ultralytics.com/modes/predict/
+"""
+
+from copy import deepcopy
+from functools import lru_cache
+from pathlib import Path
+
+import numpy as np
+import torch
+
+from ultralytics.yolo.data.augment import LetterBox
+from ultralytics.yolo.utils import LOGGER, SimpleClass, deprecation_warn, ops
+from ultralytics.yolo.utils.plotting import Annotator, colors, save_one_box
+
+
+class BaseTensor(SimpleClass):
+ """
+ Base tensor class with additional methods for easy manipulation and device handling.
+ """
+
+ def __init__(self, data, orig_shape) -> None:
+ """Initialize BaseTensor with data and original shape.
+
+ Args:
+ data (torch.Tensor | np.ndarray): Predictions, such as bboxes, masks and keypoints.
+ orig_shape (tuple): Original shape of image.
+ """
+ assert isinstance(data, (torch.Tensor, np.ndarray))
+ self.data = data
+ self.orig_shape = orig_shape
+
+ @property
+ def shape(self):
+ """Return the shape of the data tensor."""
+ return self.data.shape
+
+ def cpu(self):
+ """Return a copy of the tensor on CPU memory."""
+ return self if isinstance(self.data, np.ndarray) else self.__class__(self.data.cpu(), self.orig_shape)
+
+ def numpy(self):
+ """Return a copy of the tensor as a numpy array."""
+ return self if isinstance(self.data, np.ndarray) else self.__class__(self.data.numpy(), self.orig_shape)
+
+ def cuda(self):
+ """Return a copy of the tensor on GPU memory."""
+ return self.__class__(torch.as_tensor(self.data).cuda(), self.orig_shape)
+
+ def to(self, *args, **kwargs):
+ """Return a copy of the tensor with the specified device and dtype."""
+ return self.__class__(torch.as_tensor(self.data).to(*args, **kwargs), self.orig_shape)
+
+ def __len__(self): # override len(results)
+ """Return the length of the data tensor."""
+ return len(self.data)
+
+ def __getitem__(self, idx):
+ """Return a BaseTensor with the specified index of the data tensor."""
+ return self.__class__(self.data[idx], self.orig_shape)
+
+
+class Results(SimpleClass):
+ """
+ A class for storing and manipulating inference results.
+
+ Args:
+ orig_img (numpy.ndarray): The original image as a numpy array.
+ path (str): The path to the image file.
+ names (dict): A dictionary of class names.
+ boxes (torch.tensor, optional): A 2D tensor of bounding box coordinates for each detection.
+ masks (torch.tensor, optional): A 3D tensor of detection masks, where each mask is a binary image.
+ probs (torch.tensor, optional): A 1D tensor of probabilities of each class for classification task.
+ keypoints (List[List[float]], optional): A list of detected keypoints for each object.
+
+
+ Attributes:
+ orig_img (numpy.ndarray): The original image as a numpy array.
+ orig_shape (tuple): The original image shape in (height, width) format.
+ boxes (Boxes, optional): A Boxes object containing the detection bounding boxes.
+ masks (Masks, optional): A Masks object containing the detection masks.
+ probs (Probs, optional): A Probs object containing probabilities of each class for classification task.
+ names (dict): A dictionary of class names.
+ path (str): The path to the image file.
+ keypoints (Keypoints, optional): A Keypoints object containing detected keypoints for each object.
+ speed (dict): A dictionary of preprocess, inference and postprocess speeds in milliseconds per image.
+ _keys (tuple): A tuple of attribute names for non-empty attributes.
+ """
+
+ def __init__(self, orig_img, path, names, boxes=None, masks=None, probs=None, keypoints=None) -> None:
+ """Initialize the Results class."""
+ self.orig_img = orig_img
+ self.orig_shape = orig_img.shape[:2]
+ self.boxes = Boxes(boxes, self.orig_shape) if boxes is not None else None # native size boxes
+ self.masks = Masks(masks, self.orig_shape) if masks is not None else None # native size or imgsz masks
+ self.probs = Probs(probs) if probs is not None else None
+ self.keypoints = Keypoints(keypoints, self.orig_shape) if keypoints is not None else None
+ self.speed = {'preprocess': None, 'inference': None, 'postprocess': None} # milliseconds per image
+ self.names = names
+ self.path = path
+ self.save_dir = None
+ self._keys = ('boxes', 'masks', 'probs', 'keypoints')
+
+ def __getitem__(self, idx):
+ """Return a Results object for the specified index."""
+ r = self.new()
+ for k in self.keys:
+ setattr(r, k, getattr(self, k)[idx])
+ return r
+
+ def update(self, boxes=None, masks=None, probs=None):
+ """Update the boxes, masks, and probs attributes of the Results object."""
+ if boxes is not None:
+ self.boxes = Boxes(boxes, self.orig_shape)
+ if masks is not None:
+ self.masks = Masks(masks, self.orig_shape)
+ if probs is not None:
+ self.probs = probs
+
+ def cpu(self):
+ """Return a copy of the Results object with all tensors on CPU memory."""
+ r = self.new()
+ for k in self.keys:
+ setattr(r, k, getattr(self, k).cpu())
+ return r
+
+ def numpy(self):
+ """Return a copy of the Results object with all tensors as numpy arrays."""
+ r = self.new()
+ for k in self.keys:
+ setattr(r, k, getattr(self, k).numpy())
+ return r
+
+ def cuda(self):
+ """Return a copy of the Results object with all tensors on GPU memory."""
+ r = self.new()
+ for k in self.keys:
+ setattr(r, k, getattr(self, k).cuda())
+ return r
+
+ def to(self, *args, **kwargs):
+ """Return a copy of the Results object with tensors on the specified device and dtype."""
+ r = self.new()
+ for k in self.keys:
+ setattr(r, k, getattr(self, k).to(*args, **kwargs))
+ return r
+
+ def __len__(self):
+ """Return the number of detections in the Results object."""
+ for k in self.keys:
+ return len(getattr(self, k))
+
+ def new(self):
+ """Return a new Results object with the same image, path, and names."""
+ return Results(orig_img=self.orig_img, path=self.path, names=self.names)
+
+ @property
+ def keys(self):
+ """Return a list of non-empty attribute names."""
+ return [k for k in self._keys if getattr(self, k) is not None]
+
+ def plot(
+ self,
+ conf=True,
+ line_width=None,
+ font_size=None,
+ font='Arial.ttf',
+ pil=False,
+ img=None,
+ img_gpu=None,
+ kpt_line=True,
+ labels=True,
+ boxes=True,
+ masks=True,
+ probs=True,
+ **kwargs # deprecated args TODO: remove support in 8.2
+ ):
+ """
+ Plots the detection results on an input RGB image. Accepts a numpy array (cv2) or a PIL Image.
+
+ Args:
+ conf (bool): Whether to plot the detection confidence score.
+ line_width (float, optional): The line width of the bounding boxes. If None, it is scaled to the image size.
+ font_size (float, optional): The font size of the text. If None, it is scaled to the image size.
+ font (str): The font to use for the text.
+ pil (bool): Whether to return the image as a PIL Image.
+ img (numpy.ndarray): Plot to another image. if not, plot to original image.
+ img_gpu (torch.Tensor): Normalized image in gpu with shape (1, 3, 640, 640), for faster mask plotting.
+ kpt_line (bool): Whether to draw lines connecting keypoints.
+ labels (bool): Whether to plot the label of bounding boxes.
+ boxes (bool): Whether to plot the bounding boxes.
+ masks (bool): Whether to plot the masks.
+ probs (bool): Whether to plot classification probability
+
+ Returns:
+ (numpy.ndarray): A numpy array of the annotated image.
+ """
+ if img is None and isinstance(self.orig_img, torch.Tensor):
+ img = np.ascontiguousarray(self.orig_img[0].permute(1, 2, 0).cpu().detach().numpy()) * 255
+
+ # Deprecation warn TODO: remove in 8.2
+ if 'show_conf' in kwargs:
+ deprecation_warn('show_conf', 'conf')
+ conf = kwargs['show_conf']
+ assert type(conf) == bool, '`show_conf` should be of boolean type, i.e, show_conf=True/False'
+
+ if 'line_thickness' in kwargs:
+ deprecation_warn('line_thickness', 'line_width')
+ line_width = kwargs['line_thickness']
+ assert type(line_width) == int, '`line_width` should be of int type, i.e, line_width=3'
+
+ names = self.names
+ annotator = Annotator(deepcopy(self.orig_img if img is None else img),
+ line_width,
+ font_size,
+ font,
+ pil,
+ example=names)
+ pred_boxes, show_boxes = self.boxes, boxes
+ pred_masks, show_masks = self.masks, masks
+ pred_probs, show_probs = self.probs, probs
+ keypoints = self.keypoints
+ if pred_masks and show_masks:
+ if img_gpu is None:
+ img = LetterBox(pred_masks.shape[1:])(image=annotator.result())
+ img_gpu = torch.as_tensor(img, dtype=torch.float16, device=pred_masks.data.device).permute(
+ 2, 0, 1).flip(0).contiguous() / 255
+ idx = pred_boxes.cls if pred_boxes else range(len(pred_masks))
+ annotator.masks(pred_masks.data, colors=[colors(x, True) for x in idx], im_gpu=img_gpu)
+
+ if pred_boxes and show_boxes:
+ for d in reversed(pred_boxes):
+ c, conf, id = int(d.cls), float(d.conf) if conf else None, None if d.id is None else int(d.id.item())
+ name = ('' if id is None else f'id:{id} ') + names[c]
+ label = (f'{name} {conf:.2f}' if conf else name) if labels else None
+ annotator.box_label(d.xyxy.squeeze(), label, color=colors(c, True))
+
+ if pred_probs is not None and show_probs:
+ text = f"{', '.join(f'{names[j] if names else j} {pred_probs.data[j]:.2f}' for j in pred_probs.top5)}, "
+ annotator.text((32, 32), text, txt_color=(255, 255, 255)) # TODO: allow setting colors
+
+ if keypoints is not None:
+ for k in reversed(keypoints.data):
+ annotator.kpts(k, self.orig_shape, kpt_line=kpt_line)
+
+ return annotator.result()
+
+ def verbose(self):
+ """
+ Return log string for each task.
+ """
+ log_string = ''
+ probs = self.probs
+ boxes = self.boxes
+ if len(self) == 0:
+ return log_string if probs is not None else f'{log_string}(no detections), '
+ if probs is not None:
+ log_string += f"{', '.join(f'{self.names[j]} {probs.data[j]:.2f}' for j in probs.top5)}, "
+ if boxes:
+ for c in boxes.cls.unique():
+ n = (boxes.cls == c).sum() # detections per class
+ log_string += f"{n} {self.names[int(c)]}{'s' * (n > 1)}, "
+ return log_string
+
+ def save_txt(self, txt_file, save_conf=False):
+ """
+ Save predictions into txt file.
+
+ Args:
+ txt_file (str): txt file path.
+ save_conf (bool): save confidence score or not.
+ """
+ boxes = self.boxes
+ masks = self.masks
+ probs = self.probs
+ kpts = self.keypoints
+ texts = []
+ if probs is not None:
+ # Classify
+ [texts.append(f'{probs.data[j]:.2f} {self.names[j]}') for j in probs.top5]
+ elif boxes:
+ # Detect/segment/pose
+ for j, d in enumerate(boxes):
+ c, conf, id = int(d.cls), float(d.conf), None if d.id is None else int(d.id.item())
+ line = (c, *d.xywhn.view(-1))
+ if masks:
+ seg = masks[j].xyn[0].copy().reshape(-1) # reversed mask.xyn, (n,2) to (n*2)
+ line = (c, *seg)
+ if kpts is not None:
+ kpt = torch.cat((kpts[j].xyn, kpts[j].conf[..., None]), 2) if kpts[j].has_visible else kpts[j].xyn
+ line += (*kpt.reshape(-1).tolist(), )
+ line += (conf, ) * save_conf + (() if id is None else (id, ))
+ texts.append(('%g ' * len(line)).rstrip() % line)
+
+ if texts:
+ with open(txt_file, 'a') as f:
+ f.writelines(text + '\n' for text in texts)
+
+ def save_crop(self, save_dir, file_name=Path('im.jpg')):
+ """
+ Save cropped predictions to `save_dir/cls/file_name.jpg`.
+
+ Args:
+ save_dir (str | pathlib.Path): Save path.
+ file_name (str | pathlib.Path): File name.
+ """
+ if self.probs is not None:
+ LOGGER.warning('WARNING ⚠️ Classify task do not support `save_crop`.')
+ return
+ if isinstance(save_dir, str):
+ save_dir = Path(save_dir)
+ if isinstance(file_name, str):
+ file_name = Path(file_name)
+ for d in self.boxes:
+ save_one_box(d.xyxy,
+ self.orig_img.copy(),
+ file=save_dir / self.names[int(d.cls)] / f'{file_name.stem}.jpg',
+ BGR=True)
+
+ def pandas(self):
+ """Convert the object to a pandas DataFrame (not yet implemented)."""
+ LOGGER.warning("WARNING ⚠️ 'Results.pandas' method is not yet implemented.")
+
+ def tojson(self, normalize=False):
+ """Convert the object to JSON format."""
+ if self.probs is not None:
+ LOGGER.warning('Warning: Classify task do not support `tojson` yet.')
+ return
+
+ import json
+
+ # Create list of detection dictionaries
+ results = []
+ data = self.boxes.data.cpu().tolist()
+ h, w = self.orig_shape if normalize else (1, 1)
+ for i, row in enumerate(data):
+ box = {'x1': row[0] / w, 'y1': row[1] / h, 'x2': row[2] / w, 'y2': row[3] / h}
+ conf = row[4]
+ id = int(row[5])
+ name = self.names[id]
+ result = {'name': name, 'class': id, 'confidence': conf, 'box': box}
+ if self.masks:
+ x, y = self.masks.xy[i][:, 0], self.masks.xy[i][:, 1] # numpy array
+ result['segments'] = {'x': (x / w).tolist(), 'y': (y / h).tolist()}
+ if self.keypoints is not None:
+ x, y, visible = self.keypoints[i].data[0].cpu().unbind(dim=1) # torch Tensor
+ result['keypoints'] = {'x': (x / w).tolist(), 'y': (y / h).tolist(), 'visible': visible.tolist()}
+ results.append(result)
+
+ # Convert detections to JSON
+ return json.dumps(results, indent=2)
+
+
+class Boxes(BaseTensor):
+ """
+ A class for storing and manipulating detection boxes.
+
+ Args:
+ boxes (torch.Tensor | numpy.ndarray): A tensor or numpy array containing the detection boxes,
+ with shape (num_boxes, 6). The last two columns should contain confidence and class values.
+ orig_shape (tuple): Original image size, in the format (height, width).
+
+ Attributes:
+ boxes (torch.Tensor | numpy.ndarray): The detection boxes with shape (num_boxes, 6).
+ orig_shape (torch.Tensor | numpy.ndarray): Original image size, in the format (height, width).
+ is_track (bool): True if the boxes also include track IDs, False otherwise.
+
+ Properties:
+ xyxy (torch.Tensor | numpy.ndarray): The boxes in xyxy format.
+ conf (torch.Tensor | numpy.ndarray): The confidence values of the boxes.
+ cls (torch.Tensor | numpy.ndarray): The class values of the boxes.
+ id (torch.Tensor | numpy.ndarray): The track IDs of the boxes (if available).
+ xywh (torch.Tensor | numpy.ndarray): The boxes in xywh format.
+ xyxyn (torch.Tensor | numpy.ndarray): The boxes in xyxy format normalized by original image size.
+ xywhn (torch.Tensor | numpy.ndarray): The boxes in xywh format normalized by original image size.
+ data (torch.Tensor): The raw bboxes tensor
+
+ Methods:
+ cpu(): Move the object to CPU memory.
+ numpy(): Convert the object to a numpy array.
+ cuda(): Move the object to CUDA memory.
+ to(*args, **kwargs): Move the object to the specified device.
+ pandas(): Convert the object to a pandas DataFrame (not yet implemented).
+ """
+
+ def __init__(self, boxes, orig_shape) -> None:
+ """Initialize the Boxes class."""
+ if boxes.ndim == 1:
+ boxes = boxes[None, :]
+ n = boxes.shape[-1]
+ assert n in (6, 7), f'expected `n` in [6, 7], but got {n}' # xyxy, (track_id), conf, cls
+ super().__init__(boxes, orig_shape)
+ self.is_track = n == 7
+ self.orig_shape = orig_shape
+
+ @property
+ def xyxy(self):
+ """Return the boxes in xyxy format."""
+ return self.data[:, :4]
+
+ @property
+ def conf(self):
+ """Return the confidence values of the boxes."""
+ return self.data[:, -2]
+
+ @property
+ def cls(self):
+ """Return the class values of the boxes."""
+ return self.data[:, -1]
+
+ @property
+ def id(self):
+ """Return the track IDs of the boxes (if available)."""
+ return self.data[:, -3] if self.is_track else None
+
+ @property
+ @lru_cache(maxsize=2) # maxsize 1 should suffice
+ def xywh(self):
+ """Return the boxes in xywh format."""
+ return ops.xyxy2xywh(self.xyxy)
+
+ @property
+ @lru_cache(maxsize=2)
+ def xyxyn(self):
+ """Return the boxes in xyxy format normalized by original image size."""
+ xyxy = self.xyxy.clone() if isinstance(self.xyxy, torch.Tensor) else np.copy(self.xyxy)
+ xyxy[..., [0, 2]] /= self.orig_shape[1]
+ xyxy[..., [1, 3]] /= self.orig_shape[0]
+ return xyxy
+
+ @property
+ @lru_cache(maxsize=2)
+ def xywhn(self):
+ """Return the boxes in xywh format normalized by original image size."""
+ xywh = ops.xyxy2xywh(self.xyxy)
+ xywh[..., [0, 2]] /= self.orig_shape[1]
+ xywh[..., [1, 3]] /= self.orig_shape[0]
+ return xywh
+
+ @property
+ def boxes(self):
+ """Return the raw bboxes tensor (deprecated)."""
+ LOGGER.warning("WARNING ⚠️ 'Boxes.boxes' is deprecated. Use 'Boxes.data' instead.")
+ return self.data
+
+
+class Masks(BaseTensor):
+ """
+ A class for storing and manipulating detection masks.
+
+ Args:
+ masks (torch.Tensor | np.ndarray): A tensor containing the detection masks, with shape (num_masks, height, width).
+ orig_shape (tuple): Original image size, in the format (height, width).
+
+ Attributes:
+ masks (torch.Tensor | np.ndarray): A tensor containing the detection masks, with shape (num_masks, height, width).
+ orig_shape (tuple): Original image size, in the format (height, width).
+
+ Properties:
+ xy (list): A list of segments (pixels) which includes x, y segments of each detection.
+ xyn (list): A list of segments (normalized) which includes x, y segments of each detection.
+
+ Methods:
+ cpu(): Returns a copy of the masks tensor on CPU memory.
+ numpy(): Returns a copy of the masks tensor as a numpy array.
+ cuda(): Returns a copy of the masks tensor on GPU memory.
+ to(): Returns a copy of the masks tensor with the specified device and dtype.
+ """
+
+ def __init__(self, masks, orig_shape) -> None:
+ """Initialize the Masks class."""
+ if masks.ndim == 2:
+ masks = masks[None, :]
+ super().__init__(masks, orig_shape)
+
+ @property
+ @lru_cache(maxsize=1)
+ def segments(self):
+ """Return segments (deprecated; normalized)."""
+ LOGGER.warning("WARNING ⚠️ 'Masks.segments' is deprecated. Use 'Masks.xyn' for segments (normalized) and "
+ "'Masks.xy' for segments (pixels) instead.")
+ return self.xyn
+
+ @property
+ @lru_cache(maxsize=1)
+ def xyn(self):
+ """Return segments (normalized)."""
+ return [
+ ops.scale_coords(self.data.shape[1:], x, self.orig_shape, normalize=True)
+ for x in ops.masks2segments(self.data)]
+
+ @property
+ @lru_cache(maxsize=1)
+ def xy(self):
+ """Return segments (pixels)."""
+ return [
+ ops.scale_coords(self.data.shape[1:], x, self.orig_shape, normalize=False)
+ for x in ops.masks2segments(self.data)]
+
+ @property
+ def masks(self):
+ """Return the raw masks tensor (deprecated)."""
+ LOGGER.warning("WARNING ⚠️ 'Masks.masks' is deprecated. Use 'Masks.data' instead.")
+ return self.data
+
+ def pandas(self):
+ """Convert the object to a pandas DataFrame (not yet implemented)."""
+ LOGGER.warning("WARNING ⚠️ 'Masks.pandas' method is not yet implemented.")
+
+
+class Keypoints(BaseTensor):
+ """
+ A class for storing and manipulating detection keypoints.
+
+ Args:
+ keypoints (torch.Tensor | np.ndarray): A tensor containing the detection keypoints, with shape (num_dets, num_kpts, 2/3).
+ orig_shape (tuple): Original image size, in the format (height, width).
+
+ Attributes:
+ keypoints (torch.Tensor | np.ndarray): A tensor containing the detection keypoints, with shape (num_dets, num_kpts, 2/3).
+ orig_shape (tuple): Original image size, in the format (height, width).
+
+ Properties:
+ xy (list): A list of keypoints (pixels) which includes x, y keypoints of each detection.
+ xyn (list): A list of keypoints (normalized) which includes x, y keypoints of each detection.
+
+ Methods:
+ cpu(): Returns a copy of the keypoints tensor on CPU memory.
+ numpy(): Returns a copy of the keypoints tensor as a numpy array.
+ cuda(): Returns a copy of the keypoints tensor on GPU memory.
+ to(): Returns a copy of the keypoints tensor with the specified device and dtype.
+ """
+
+ def __init__(self, keypoints, orig_shape) -> None:
+ if keypoints.ndim == 2:
+ keypoints = keypoints[None, :]
+ super().__init__(keypoints, orig_shape)
+ self.has_visible = self.data.shape[-1] == 3
+
+ @property
+ @lru_cache(maxsize=1)
+ def xy(self):
+ return self.data[..., :2]
+
+ @property
+ @lru_cache(maxsize=1)
+ def xyn(self):
+ xy = self.xy.clone() if isinstance(self.xy, torch.Tensor) else np.copy(self.xy)
+ xy[..., 0] /= self.orig_shape[1]
+ xy[..., 1] /= self.orig_shape[0]
+ return xy
+
+ @property
+ @lru_cache(maxsize=1)
+ def conf(self):
+ return self.data[..., 2] if self.has_visible else None
+
+
+class Probs(BaseTensor):
+ """
+ A class for storing and manipulating classify predictions.
+
+ Args:
+ probs (torch.Tensor | np.ndarray): A tensor containing the detection keypoints, with shape (num_class, ).
+
+ Attributes:
+ probs (torch.Tensor | np.ndarray): A tensor containing the detection keypoints, with shape (num_class).
+
+ Properties:
+ top5 (list[int]): Top 1 indice.
+ top1 (int): Top 5 indices.
+
+ Methods:
+ cpu(): Returns a copy of the probs tensor on CPU memory.
+ numpy(): Returns a copy of the probs tensor as a numpy array.
+ cuda(): Returns a copy of the probs tensor on GPU memory.
+ to(): Returns a copy of the probs tensor with the specified device and dtype.
+ """
+
+ def __init__(self, probs, orig_shape=None) -> None:
+ super().__init__(probs, orig_shape)
+
+ @property
+ @lru_cache(maxsize=1)
+ def top5(self):
+ """Return the indices of top 5."""
+ return (-self.data).argsort(0)[:5].tolist() # this way works with both torch and numpy.
+
+ @property
+ @lru_cache(maxsize=1)
+ def top1(self):
+ """Return the indices of top 1."""
+ return int(self.data.argmax())
+
+ @property
+ @lru_cache(maxsize=1)
+ def top5conf(self):
+ """Return the confidences of top 5."""
+ return self.data[self.top5]
+
+ @property
+ @lru_cache(maxsize=1)
+ def top1conf(self):
+ """Return the confidences of top 1."""
+ return self.data[self.top1]
diff --git a/yolov8/ultralytics/yolo/engine/trainer.py b/yolov8/ultralytics/yolo/engine/trainer.py
new file mode 100644
index 0000000000000000000000000000000000000000..144be9c8df16e42f0e0b72743e0784fc045fa17f
--- /dev/null
+++ b/yolov8/ultralytics/yolo/engine/trainer.py
@@ -0,0 +1,664 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Train a model on a dataset
+
+Usage:
+ $ yolo mode=train model=yolov8n.pt data=coco128.yaml imgsz=640 epochs=100 batch=16
+"""
+import math
+import os
+import subprocess
+import time
+from copy import deepcopy
+from datetime import datetime, timedelta
+from pathlib import Path
+
+import numpy as np
+import torch
+from torch import distributed as dist
+from torch import nn, optim
+from torch.cuda import amp
+from torch.nn.parallel import DistributedDataParallel as DDP
+from tqdm import tqdm
+
+from ultralytics.nn.tasks import attempt_load_one_weight, attempt_load_weights
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.data.utils import check_cls_dataset, check_det_dataset
+from ultralytics.yolo.utils import (DEFAULT_CFG, LOGGER, RANK, SETTINGS, TQDM_BAR_FORMAT, __version__, callbacks,
+ clean_url, colorstr, emojis, yaml_save)
+from ultralytics.yolo.utils.autobatch import check_train_batch_size
+from ultralytics.yolo.utils.checks import check_amp, check_file, check_imgsz, print_args
+from ultralytics.yolo.utils.dist import ddp_cleanup, generate_ddp_command
+from ultralytics.yolo.utils.files import get_latest_run, increment_path
+from ultralytics.yolo.utils.torch_utils import (EarlyStopping, ModelEMA, de_parallel, init_seeds, one_cycle,
+ select_device, strip_optimizer)
+
+
+class BaseTrainer:
+ """
+ BaseTrainer
+
+ A base class for creating trainers.
+
+ Attributes:
+ args (SimpleNamespace): Configuration for the trainer.
+ check_resume (method): Method to check if training should be resumed from a saved checkpoint.
+ validator (BaseValidator): Validator instance.
+ model (nn.Module): Model instance.
+ callbacks (defaultdict): Dictionary of callbacks.
+ save_dir (Path): Directory to save results.
+ wdir (Path): Directory to save weights.
+ last (Path): Path to last checkpoint.
+ best (Path): Path to best checkpoint.
+ save_period (int): Save checkpoint every x epochs (disabled if < 1).
+ batch_size (int): Batch size for training.
+ epochs (int): Number of epochs to train for.
+ start_epoch (int): Starting epoch for training.
+ device (torch.device): Device to use for training.
+ amp (bool): Flag to enable AMP (Automatic Mixed Precision).
+ scaler (amp.GradScaler): Gradient scaler for AMP.
+ data (str): Path to data.
+ trainset (torch.utils.data.Dataset): Training dataset.
+ testset (torch.utils.data.Dataset): Testing dataset.
+ ema (nn.Module): EMA (Exponential Moving Average) of the model.
+ lf (nn.Module): Loss function.
+ scheduler (torch.optim.lr_scheduler._LRScheduler): Learning rate scheduler.
+ best_fitness (float): The best fitness value achieved.
+ fitness (float): Current fitness value.
+ loss (float): Current loss value.
+ tloss (float): Total loss value.
+ loss_names (list): List of loss names.
+ csv (Path): Path to results CSV file.
+ """
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ """
+ Initializes the BaseTrainer class.
+
+ Args:
+ cfg (str, optional): Path to a configuration file. Defaults to DEFAULT_CFG.
+ overrides (dict, optional): Configuration overrides. Defaults to None.
+ """
+ self.args = get_cfg(cfg, overrides)
+ self.device = select_device(self.args.device, self.args.batch)
+ self.check_resume()
+ self.validator = None
+ self.model = None
+ self.metrics = None
+ self.plots = {}
+ init_seeds(self.args.seed + 1 + RANK, deterministic=self.args.deterministic)
+
+ # Dirs
+ project = self.args.project or Path(SETTINGS['runs_dir']) / self.args.task
+ name = self.args.name or f'{self.args.mode}'
+ if hasattr(self.args, 'save_dir'):
+ self.save_dir = Path(self.args.save_dir)
+ else:
+ self.save_dir = Path(
+ increment_path(Path(project) / name, exist_ok=self.args.exist_ok if RANK in (-1, 0) else True))
+ self.wdir = self.save_dir / 'weights' # weights dir
+ if RANK in (-1, 0):
+ self.wdir.mkdir(parents=True, exist_ok=True) # make dir
+ self.args.save_dir = str(self.save_dir)
+ yaml_save(self.save_dir / 'args.yaml', vars(self.args)) # save run args
+ self.last, self.best = self.wdir / 'last.pt', self.wdir / 'best.pt' # checkpoint paths
+ self.save_period = self.args.save_period
+
+ self.batch_size = self.args.batch
+ self.epochs = self.args.epochs
+ self.start_epoch = 0
+ if RANK == -1:
+ print_args(vars(self.args))
+
+ # Device
+ if self.device.type == 'cpu':
+ self.args.workers = 0 # faster CPU training as time dominated by inference, not dataloading
+
+ # Model and Dataset
+ self.model = self.args.model
+ try:
+ if self.args.task == 'classify':
+ self.data = check_cls_dataset(self.args.data)
+ elif self.args.data.endswith('.yaml') or self.args.task in ('detect', 'segment'):
+ self.data = check_det_dataset(self.args.data)
+ if 'yaml_file' in self.data:
+ self.args.data = self.data['yaml_file'] # for validating 'yolo train data=url.zip' usage
+ except Exception as e:
+ raise RuntimeError(emojis(f"Dataset '{clean_url(self.args.data)}' error ❌ {e}")) from e
+
+ self.trainset, self.testset = self.get_dataset(self.data)
+ self.ema = None
+
+ # Optimization utils init
+ self.lf = None
+ self.scheduler = None
+
+ # Epoch level metrics
+ self.best_fitness = None
+ self.fitness = None
+ self.loss = None
+ self.tloss = None
+ self.loss_names = ['Loss']
+ self.csv = self.save_dir / 'results.csv'
+ self.plot_idx = [0, 1, 2]
+
+ # Callbacks
+ self.callbacks = _callbacks or callbacks.get_default_callbacks()
+ if RANK in (-1, 0):
+ callbacks.add_integration_callbacks(self)
+
+ def add_callback(self, event: str, callback):
+ """
+ Appends the given callback.
+ """
+ self.callbacks[event].append(callback)
+
+ def set_callback(self, event: str, callback):
+ """
+ Overrides the existing callbacks with the given callback.
+ """
+ self.callbacks[event] = [callback]
+
+ def run_callbacks(self, event: str):
+ """Run all existing callbacks associated with a particular event."""
+ for callback in self.callbacks.get(event, []):
+ callback(self)
+
+ def train(self):
+ """Allow device='', device=None on Multi-GPU systems to default to device=0."""
+ if isinstance(self.args.device, int) or self.args.device: # i.e. device=0 or device=[0,1,2,3]
+ world_size = torch.cuda.device_count()
+ elif torch.cuda.is_available(): # i.e. device=None or device=''
+ world_size = 1 # default to device 0
+ else: # i.e. device='cpu' or 'mps'
+ world_size = 0
+
+ # Run subprocess if DDP training, else train normally
+ if world_size > 1 and 'LOCAL_RANK' not in os.environ:
+ # Argument checks
+ if self.args.rect:
+ LOGGER.warning("WARNING ⚠️ 'rect=True' is incompatible with Multi-GPU training, setting rect=False")
+ self.args.rect = False
+ # Command
+ cmd, file = generate_ddp_command(world_size, self)
+ try:
+ LOGGER.info(f'DDP command: {cmd}')
+ subprocess.run(cmd, check=True)
+ except Exception as e:
+ raise e
+ finally:
+ ddp_cleanup(self, str(file))
+ else:
+ self._do_train(world_size)
+
+ def _setup_ddp(self, world_size):
+ """Initializes and sets the DistributedDataParallel parameters for training."""
+ torch.cuda.set_device(RANK)
+ self.device = torch.device('cuda', RANK)
+ LOGGER.info(f'DDP info: RANK {RANK}, WORLD_SIZE {world_size}, DEVICE {self.device}')
+ os.environ['NCCL_BLOCKING_WAIT'] = '1' # set to enforce timeout
+ dist.init_process_group(
+ 'nccl' if dist.is_nccl_available() else 'gloo',
+ timeout=timedelta(seconds=10800), # 3 hours
+ rank=RANK,
+ world_size=world_size)
+
+ def _setup_train(self, world_size):
+ """
+ Builds dataloaders and optimizer on correct rank process.
+ """
+ # Model
+ self.run_callbacks('on_pretrain_routine_start')
+ ckpt = self.setup_model()
+ self.model = self.model.to(self.device)
+ self.set_model_attributes()
+ # Check AMP
+ self.amp = torch.tensor(self.args.amp).to(self.device) # True or False
+ if self.amp and RANK in (-1, 0): # Single-GPU and DDP
+ callbacks_backup = callbacks.default_callbacks.copy() # backup callbacks as check_amp() resets them
+ self.amp = torch.tensor(check_amp(self.model), device=self.device)
+ callbacks.default_callbacks = callbacks_backup # restore callbacks
+ if RANK > -1 and world_size > 1: # DDP
+ dist.broadcast(self.amp, src=0) # broadcast the tensor from rank 0 to all other ranks (returns None)
+ self.amp = bool(self.amp) # as boolean
+ self.scaler = amp.GradScaler(enabled=self.amp)
+ if world_size > 1:
+ self.model = DDP(self.model, device_ids=[RANK])
+ # Check imgsz
+ gs = max(int(self.model.stride.max() if hasattr(self.model, 'stride') else 32), 32) # grid size (max stride)
+ self.args.imgsz = check_imgsz(self.args.imgsz, stride=gs, floor=gs, max_dim=1)
+ # Batch size
+ if self.batch_size == -1:
+ if RANK == -1: # single-GPU only, estimate best batch size
+ self.args.batch = self.batch_size = check_train_batch_size(self.model, self.args.imgsz, self.amp)
+ else:
+ SyntaxError('batch=-1 to use AutoBatch is only available in Single-GPU training. '
+ 'Please pass a valid batch size value for Multi-GPU DDP training, i.e. batch=16')
+
+ # Dataloaders
+ batch_size = self.batch_size // max(world_size, 1)
+ self.train_loader = self.get_dataloader(self.trainset, batch_size=batch_size, rank=RANK, mode='train')
+ if RANK in (-1, 0):
+ self.test_loader = self.get_dataloader(self.testset, batch_size=batch_size * 2, rank=-1, mode='val')
+ self.validator = self.get_validator()
+ metric_keys = self.validator.metrics.keys + self.label_loss_items(prefix='val')
+ self.metrics = dict(zip(metric_keys, [0] * len(metric_keys))) # TODO: init metrics for plot_results()?
+ self.ema = ModelEMA(self.model)
+ if self.args.plots and not self.args.v5loader:
+ self.plot_training_labels()
+
+ # Optimizer
+ self.accumulate = max(round(self.args.nbs / self.batch_size), 1) # accumulate loss before optimizing
+ weight_decay = self.args.weight_decay * self.batch_size * self.accumulate / self.args.nbs # scale weight_decay
+ iterations = math.ceil(len(self.train_loader.dataset) / max(self.batch_size, self.args.nbs)) * self.epochs
+ self.optimizer = self.build_optimizer(model=self.model,
+ name=self.args.optimizer,
+ lr=self.args.lr0,
+ momentum=self.args.momentum,
+ decay=weight_decay,
+ iterations=iterations)
+ # Scheduler
+ if self.args.cos_lr:
+ self.lf = one_cycle(1, self.args.lrf, self.epochs) # cosine 1->hyp['lrf']
+ else:
+ self.lf = lambda x: (1 - x / self.epochs) * (1.0 - self.args.lrf) + self.args.lrf # linear
+ self.scheduler = optim.lr_scheduler.LambdaLR(self.optimizer, lr_lambda=self.lf)
+ self.stopper, self.stop = EarlyStopping(patience=self.args.patience), False
+ self.resume_training(ckpt)
+ self.scheduler.last_epoch = self.start_epoch - 1 # do not move
+ self.run_callbacks('on_pretrain_routine_end')
+
+ def _do_train(self, world_size=1):
+ """Train completed, evaluate and plot if specified by arguments."""
+ if world_size > 1:
+ self._setup_ddp(world_size)
+
+ self._setup_train(world_size)
+
+ self.epoch_time = None
+ self.epoch_time_start = time.time()
+ self.train_time_start = time.time()
+ nb = len(self.train_loader) # number of batches
+ nw = max(round(self.args.warmup_epochs *
+ nb), 100) if self.args.warmup_epochs > 0 else -1 # number of warmup iterations
+ last_opt_step = -1
+ self.run_callbacks('on_train_start')
+ LOGGER.info(f'Image sizes {self.args.imgsz} train, {self.args.imgsz} val\n'
+ f'Using {self.train_loader.num_workers * (world_size or 1)} dataloader workers\n'
+ f"Logging results to {colorstr('bold', self.save_dir)}\n"
+ f'Starting training for {self.epochs} epochs...')
+ if self.args.close_mosaic:
+ base_idx = (self.epochs - self.args.close_mosaic) * nb
+ self.plot_idx.extend([base_idx, base_idx + 1, base_idx + 2])
+ epoch = self.epochs # predefine for resume fully trained model edge cases
+ for epoch in range(self.start_epoch, self.epochs):
+ self.epoch = epoch
+ self.run_callbacks('on_train_epoch_start')
+ self.model.train()
+ if RANK != -1:
+ self.train_loader.sampler.set_epoch(epoch)
+ pbar = enumerate(self.train_loader)
+ # Update dataloader attributes (optional)
+ if epoch == (self.epochs - self.args.close_mosaic):
+ LOGGER.info('Closing dataloader mosaic')
+ if hasattr(self.train_loader.dataset, 'mosaic'):
+ self.train_loader.dataset.mosaic = False
+ if hasattr(self.train_loader.dataset, 'close_mosaic'):
+ self.train_loader.dataset.close_mosaic(hyp=self.args)
+ self.train_loader.reset()
+
+ if RANK in (-1, 0):
+ LOGGER.info(self.progress_string())
+ pbar = tqdm(enumerate(self.train_loader), total=nb, bar_format=TQDM_BAR_FORMAT)
+ self.tloss = None
+ self.optimizer.zero_grad()
+ for i, batch in pbar:
+ self.run_callbacks('on_train_batch_start')
+ # Warmup
+ ni = i + nb * epoch
+ if ni <= nw:
+ xi = [0, nw] # x interp
+ self.accumulate = max(1, np.interp(ni, xi, [1, self.args.nbs / self.batch_size]).round())
+ for j, x in enumerate(self.optimizer.param_groups):
+ # Bias lr falls from 0.1 to lr0, all other lrs rise from 0.0 to lr0
+ x['lr'] = np.interp(
+ ni, xi, [self.args.warmup_bias_lr if j == 0 else 0.0, x['initial_lr'] * self.lf(epoch)])
+ if 'momentum' in x:
+ x['momentum'] = np.interp(ni, xi, [self.args.warmup_momentum, self.args.momentum])
+
+ # Forward
+ with torch.cuda.amp.autocast(self.amp):
+ batch = self.preprocess_batch(batch)
+ self.loss, self.loss_items = self.model(batch)
+ if RANK != -1:
+ self.loss *= world_size
+ self.tloss = (self.tloss * i + self.loss_items) / (i + 1) if self.tloss is not None \
+ else self.loss_items
+
+ # Backward
+ self.scaler.scale(self.loss).backward()
+
+ # Optimize - https://pytorch.org/docs/master/notes/amp_examples.html
+ if ni - last_opt_step >= self.accumulate:
+ self.optimizer_step()
+ last_opt_step = ni
+
+ # Log
+ mem = f'{torch.cuda.memory_reserved() / 1E9 if torch.cuda.is_available() else 0:.3g}G' # (GB)
+ loss_len = self.tloss.shape[0] if len(self.tloss.size()) else 1
+ losses = self.tloss if loss_len > 1 else torch.unsqueeze(self.tloss, 0)
+ if RANK in (-1, 0):
+ pbar.set_description(
+ ('%11s' * 2 + '%11.4g' * (2 + loss_len)) %
+ (f'{epoch + 1}/{self.epochs}', mem, *losses, batch['cls'].shape[0], batch['img'].shape[-1]))
+ self.run_callbacks('on_batch_end')
+ if self.args.plots and ni in self.plot_idx:
+ self.plot_training_samples(batch, ni)
+
+ self.run_callbacks('on_train_batch_end')
+
+ self.lr = {f'lr/pg{ir}': x['lr'] for ir, x in enumerate(self.optimizer.param_groups)} # for loggers
+
+ self.scheduler.step()
+ self.run_callbacks('on_train_epoch_end')
+
+ if RANK in (-1, 0):
+
+ # Validation
+ self.ema.update_attr(self.model, include=['yaml', 'nc', 'args', 'names', 'stride', 'class_weights'])
+ final_epoch = (epoch + 1 == self.epochs) or self.stopper.possible_stop
+
+ if self.args.val or final_epoch:
+ self.metrics, self.fitness = self.validate()
+ self.save_metrics(metrics={**self.label_loss_items(self.tloss), **self.metrics, **self.lr})
+ self.stop = self.stopper(epoch + 1, self.fitness)
+
+ # Save model
+ if self.args.save or (epoch + 1 == self.epochs):
+ self.save_model()
+ self.run_callbacks('on_model_save')
+
+ tnow = time.time()
+ self.epoch_time = tnow - self.epoch_time_start
+ self.epoch_time_start = tnow
+ self.run_callbacks('on_fit_epoch_end')
+ torch.cuda.empty_cache() # clears GPU vRAM at end of epoch, can help with out of memory errors
+
+ # Early Stopping
+ if RANK != -1: # if DDP training
+ broadcast_list = [self.stop if RANK == 0 else None]
+ dist.broadcast_object_list(broadcast_list, 0) # broadcast 'stop' to all ranks
+ if RANK != 0:
+ self.stop = broadcast_list[0]
+ if self.stop:
+ break # must break all DDP ranks
+
+ if RANK in (-1, 0):
+ # Do final val with best.pt
+ LOGGER.info(f'\n{epoch - self.start_epoch + 1} epochs completed in '
+ f'{(time.time() - self.train_time_start) / 3600:.3f} hours.')
+ self.final_eval()
+ if self.args.plots:
+ self.plot_metrics()
+ self.run_callbacks('on_train_end')
+ torch.cuda.empty_cache()
+ self.run_callbacks('teardown')
+
+ def save_model(self):
+ """Save model checkpoints based on various conditions."""
+ ckpt = {
+ 'epoch': self.epoch,
+ 'best_fitness': self.best_fitness,
+ 'model': deepcopy(de_parallel(self.model)).half(),
+ 'ema': deepcopy(self.ema.ema).half(),
+ 'updates': self.ema.updates,
+ 'optimizer': self.optimizer.state_dict(),
+ 'train_args': vars(self.args), # save as dict
+ 'date': datetime.now().isoformat(),
+ 'version': __version__}
+
+ # Use dill (if exists) to serialize the lambda functions where pickle does not do this
+ try:
+ import dill as pickle
+ except ImportError:
+ import pickle
+
+ # Save last, best and delete
+ torch.save(ckpt, self.last, pickle_module=pickle)
+ if self.best_fitness == self.fitness:
+ torch.save(ckpt, self.best, pickle_module=pickle)
+ if (self.epoch > 0) and (self.save_period > 0) and (self.epoch % self.save_period == 0):
+ torch.save(ckpt, self.wdir / f'epoch{self.epoch}.pt', pickle_module=pickle)
+ del ckpt
+
+ @staticmethod
+ def get_dataset(data):
+ """
+ Get train, val path from data dict if it exists. Returns None if data format is not recognized.
+ """
+ return data['train'], data.get('val') or data.get('test')
+
+ def setup_model(self):
+ """
+ load/create/download model for any task.
+ """
+ if isinstance(self.model, torch.nn.Module): # if model is loaded beforehand. No setup needed
+ return
+
+ model, weights = self.model, None
+ ckpt = None
+ if str(model).endswith('.pt'):
+ weights, ckpt = attempt_load_one_weight(model)
+ cfg = ckpt['model'].yaml
+ else:
+ cfg = model
+ self.model = self.get_model(cfg=cfg, weights=weights, verbose=RANK == -1) # calls Model(cfg, weights)
+ return ckpt
+
+ def optimizer_step(self):
+ """Perform a single step of the training optimizer with gradient clipping and EMA update."""
+ self.scaler.unscale_(self.optimizer) # unscale gradients
+ torch.nn.utils.clip_grad_norm_(self.model.parameters(), max_norm=10.0) # clip gradients
+ self.scaler.step(self.optimizer)
+ self.scaler.update()
+ self.optimizer.zero_grad()
+ if self.ema:
+ self.ema.update(self.model)
+
+ def preprocess_batch(self, batch):
+ """
+ Allows custom preprocessing model inputs and ground truths depending on task type.
+ """
+ return batch
+
+ def validate(self):
+ """
+ Runs validation on test set using self.validator. The returned dict is expected to contain "fitness" key.
+ """
+ metrics = self.validator(self)
+ fitness = metrics.pop('fitness', -self.loss.detach().cpu().numpy()) # use loss as fitness measure if not found
+ if not self.best_fitness or self.best_fitness < fitness:
+ self.best_fitness = fitness
+ return metrics, fitness
+
+ def get_model(self, cfg=None, weights=None, verbose=True):
+ """Get model and raise NotImplementedError for loading cfg files."""
+ raise NotImplementedError("This task trainer doesn't support loading cfg files")
+
+ def get_validator(self):
+ """Returns a NotImplementedError when the get_validator function is called."""
+ raise NotImplementedError('get_validator function not implemented in trainer')
+
+ def get_dataloader(self, dataset_path, batch_size=16, rank=0, mode='train'):
+ """
+ Returns dataloader derived from torch.data.Dataloader.
+ """
+ raise NotImplementedError('get_dataloader function not implemented in trainer')
+
+ def build_dataset(self, img_path, mode='train', batch=None):
+ """Build dataset"""
+ raise NotImplementedError('build_dataset function not implemented in trainer')
+
+ def label_loss_items(self, loss_items=None, prefix='train'):
+ """
+ Returns a loss dict with labelled training loss items tensor
+ """
+ # Not needed for classification but necessary for segmentation & detection
+ return {'loss': loss_items} if loss_items is not None else ['loss']
+
+ def set_model_attributes(self):
+ """
+ To set or update model parameters before training.
+ """
+ self.model.names = self.data['names']
+
+ def build_targets(self, preds, targets):
+ """Builds target tensors for training YOLO model."""
+ pass
+
+ def progress_string(self):
+ """Returns a string describing training progress."""
+ return ''
+
+ # TODO: may need to put these following functions into callback
+ def plot_training_samples(self, batch, ni):
+ """Plots training samples during YOLOv5 training."""
+ pass
+
+ def plot_training_labels(self):
+ """Plots training labels for YOLO model."""
+ pass
+
+ def save_metrics(self, metrics):
+ """Saves training metrics to a CSV file."""
+ keys, vals = list(metrics.keys()), list(metrics.values())
+ n = len(metrics) + 1 # number of cols
+ s = '' if self.csv.exists() else (('%23s,' * n % tuple(['epoch'] + keys)).rstrip(',') + '\n') # header
+ with open(self.csv, 'a') as f:
+ f.write(s + ('%23.5g,' * n % tuple([self.epoch] + vals)).rstrip(',') + '\n')
+
+ def plot_metrics(self):
+ """Plot and display metrics visually."""
+ pass
+
+ def on_plot(self, name, data=None):
+ """Registers plots (e.g. to be consumed in callbacks)"""
+ self.plots[name] = {'data': data, 'timestamp': time.time()}
+
+ def final_eval(self):
+ """Performs final evaluation and validation for object detection YOLO model."""
+ for f in self.last, self.best:
+ if f.exists():
+ strip_optimizer(f) # strip optimizers
+ if f is self.best:
+ LOGGER.info(f'\nValidating {f}...')
+ self.metrics = self.validator(model=f)
+ self.metrics.pop('fitness', None)
+ self.run_callbacks('on_fit_epoch_end')
+
+ def check_resume(self):
+ """Check if resume checkpoint exists and update arguments accordingly."""
+ resume = self.args.resume
+ if resume:
+ try:
+ exists = isinstance(resume, (str, Path)) and Path(resume).exists()
+ last = Path(check_file(resume) if exists else get_latest_run())
+
+ # Check that resume data YAML exists, otherwise strip to force re-download of dataset
+ ckpt_args = attempt_load_weights(last).args
+ if not Path(ckpt_args['data']).exists():
+ ckpt_args['data'] = self.args.data
+
+ self.args = get_cfg(ckpt_args)
+ self.args.model, resume = str(last), True # reinstate
+ except Exception as e:
+ raise FileNotFoundError('Resume checkpoint not found. Please pass a valid checkpoint to resume from, '
+ "i.e. 'yolo train resume model=path/to/last.pt'") from e
+ self.resume = resume
+
+ def resume_training(self, ckpt):
+ """Resume YOLO training from given epoch and best fitness."""
+ if ckpt is None:
+ return
+ best_fitness = 0.0
+ start_epoch = ckpt['epoch'] + 1
+ if ckpt['optimizer'] is not None:
+ self.optimizer.load_state_dict(ckpt['optimizer']) # optimizer
+ best_fitness = ckpt['best_fitness']
+ if self.ema and ckpt.get('ema'):
+ self.ema.ema.load_state_dict(ckpt['ema'].float().state_dict()) # EMA
+ self.ema.updates = ckpt['updates']
+ if self.resume:
+ assert start_epoch > 0, \
+ f'{self.args.model} training to {self.epochs} epochs is finished, nothing to resume.\n' \
+ f"Start a new training without resuming, i.e. 'yolo train model={self.args.model}'"
+ LOGGER.info(
+ f'Resuming training from {self.args.model} from epoch {start_epoch + 1} to {self.epochs} total epochs')
+ if self.epochs < start_epoch:
+ LOGGER.info(
+ f"{self.model} has been trained for {ckpt['epoch']} epochs. Fine-tuning for {self.epochs} more epochs.")
+ self.epochs += ckpt['epoch'] # finetune additional epochs
+ self.best_fitness = best_fitness
+ self.start_epoch = start_epoch
+ if start_epoch > (self.epochs - self.args.close_mosaic):
+ LOGGER.info('Closing dataloader mosaic')
+ if hasattr(self.train_loader.dataset, 'mosaic'):
+ self.train_loader.dataset.mosaic = False
+ if hasattr(self.train_loader.dataset, 'close_mosaic'):
+ self.train_loader.dataset.close_mosaic(hyp=self.args)
+
+ def build_optimizer(self, model, name='auto', lr=0.001, momentum=0.9, decay=1e-5, iterations=1e5):
+ """
+ Constructs an optimizer for the given model, based on the specified optimizer name, learning rate,
+ momentum, weight decay, and number of iterations.
+
+ Args:
+ model (torch.nn.Module): The model for which to build an optimizer.
+ name (str, optional): The name of the optimizer to use. If 'auto', the optimizer is selected
+ based on the number of iterations. Default: 'auto'.
+ lr (float, optional): The learning rate for the optimizer. Default: 0.001.
+ momentum (float, optional): The momentum factor for the optimizer. Default: 0.9.
+ decay (float, optional): The weight decay for the optimizer. Default: 1e-5.
+ iterations (float, optional): The number of iterations, which determines the optimizer if
+ name is 'auto'. Default: 1e5.
+
+ Returns:
+ (torch.optim.Optimizer): The constructed optimizer.
+ """
+
+ g = [], [], [] # optimizer parameter groups
+ bn = tuple(v for k, v in nn.__dict__.items() if 'Norm' in k) # normalization layers, i.e. BatchNorm2d()
+ if name == 'auto':
+ nc = getattr(model, 'nc', 10) # number of classes
+ lr_fit = round(0.002 * 5 / (4 + nc), 6) # lr0 fit equation to 6 decimal places
+ name, lr, momentum = ('SGD', 0.01, 0.9) if iterations > 10000 else ('AdamW', lr_fit, 0.9)
+ self.args.warmup_bias_lr = 0.0 # no higher than 0.01 for Adam
+
+ for module_name, module in model.named_modules():
+ for param_name, param in module.named_parameters(recurse=False):
+ fullname = f'{module_name}.{param_name}' if module_name else param_name
+ if 'bias' in fullname: # bias (no decay)
+ g[2].append(param)
+ elif isinstance(module, bn): # weight (no decay)
+ g[1].append(param)
+ else: # weight (with decay)
+ g[0].append(param)
+
+ if name in ('Adam', 'Adamax', 'AdamW', 'NAdam', 'RAdam'):
+ optimizer = getattr(optim, name, optim.Adam)(g[2], lr=lr, betas=(momentum, 0.999), weight_decay=0.0)
+ elif name == 'RMSProp':
+ optimizer = optim.RMSprop(g[2], lr=lr, momentum=momentum)
+ elif name == 'SGD':
+ optimizer = optim.SGD(g[2], lr=lr, momentum=momentum, nesterov=True)
+ else:
+ raise NotImplementedError(
+ f"Optimizer '{name}' not found in list of available optimizers "
+ f'[Adam, AdamW, NAdam, RAdam, RMSProp, SGD, auto].'
+ 'To request support for addition optimizers please visit https://github.com/ultralytics/ultralytics.')
+
+ optimizer.add_param_group({'params': g[0], 'weight_decay': decay}) # add g0 with weight_decay
+ optimizer.add_param_group({'params': g[1], 'weight_decay': 0.0}) # add g1 (BatchNorm2d weights)
+ LOGGER.info(
+ f"{colorstr('optimizer:')} {type(optimizer).__name__}(lr={lr}, momentum={momentum}) with parameter groups "
+ f'{len(g[1])} weight(decay=0.0), {len(g[0])} weight(decay={decay}), {len(g[2])} bias(decay=0.0)')
+ return optimizer
diff --git a/yolov8/ultralytics/yolo/engine/validator.py b/yolov8/ultralytics/yolo/engine/validator.py
new file mode 100644
index 0000000000000000000000000000000000000000..f84c8d0b0fb7274454625b87111056f83a30963a
--- /dev/null
+++ b/yolov8/ultralytics/yolo/engine/validator.py
@@ -0,0 +1,276 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Check a model's accuracy on a test or val split of a dataset
+
+Usage:
+ $ yolo mode=val model=yolov8n.pt data=coco128.yaml imgsz=640
+
+Usage - formats:
+ $ yolo mode=val model=yolov8n.pt # PyTorch
+ yolov8n.torchscript # TorchScript
+ yolov8n.onnx # ONNX Runtime or OpenCV DNN with dnn=True
+ yolov8n_openvino_model # OpenVINO
+ yolov8n.engine # TensorRT
+ yolov8n.mlmodel # CoreML (macOS-only)
+ yolov8n_saved_model # TensorFlow SavedModel
+ yolov8n.pb # TensorFlow GraphDef
+ yolov8n.tflite # TensorFlow Lite
+ yolov8n_edgetpu.tflite # TensorFlow Edge TPU
+ yolov8n_paddle_model # PaddlePaddle
+"""
+import json
+import time
+from pathlib import Path
+
+import torch
+from tqdm import tqdm
+
+from ultralytics.nn.autobackend import AutoBackend
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.data.utils import check_cls_dataset, check_det_dataset
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, RANK, SETTINGS, TQDM_BAR_FORMAT, callbacks, colorstr, emojis
+from ultralytics.yolo.utils.checks import check_imgsz
+from ultralytics.yolo.utils.files import increment_path
+from ultralytics.yolo.utils.ops import Profile
+from ultralytics.yolo.utils.torch_utils import de_parallel, select_device, smart_inference_mode
+
+
+class BaseValidator:
+ """
+ BaseValidator
+
+ A base class for creating validators.
+
+ Attributes:
+ dataloader (DataLoader): Dataloader to use for validation.
+ pbar (tqdm): Progress bar to update during validation.
+ args (SimpleNamespace): Configuration for the validator.
+ model (nn.Module): Model to validate.
+ data (dict): Data dictionary.
+ device (torch.device): Device to use for validation.
+ batch_i (int): Current batch index.
+ training (bool): Whether the model is in training mode.
+ speed (float): Batch processing speed in seconds.
+ jdict (dict): Dictionary to store validation results.
+ save_dir (Path): Directory to save results.
+ """
+
+ def __init__(self, dataloader=None, save_dir=None, pbar=None, args=None, _callbacks=None):
+ """
+ Initializes a BaseValidator instance.
+
+ Args:
+ dataloader (torch.utils.data.DataLoader): Dataloader to be used for validation.
+ save_dir (Path): Directory to save results.
+ pbar (tqdm.tqdm): Progress bar for displaying progress.
+ args (SimpleNamespace): Configuration for the validator.
+ """
+ self.dataloader = dataloader
+ self.pbar = pbar
+ self.args = args or get_cfg(DEFAULT_CFG)
+ self.model = None
+ self.data = None
+ self.device = None
+ self.batch_i = None
+ self.training = True
+ self.speed = {'preprocess': 0.0, 'inference': 0.0, 'loss': 0.0, 'postprocess': 0.0}
+ self.jdict = None
+
+ project = self.args.project or Path(SETTINGS['runs_dir']) / self.args.task
+ name = self.args.name or f'{self.args.mode}'
+ self.save_dir = save_dir or increment_path(Path(project) / name,
+ exist_ok=self.args.exist_ok if RANK in (-1, 0) else True)
+ (self.save_dir / 'labels' if self.args.save_txt else self.save_dir).mkdir(parents=True, exist_ok=True)
+
+ if self.args.conf is None:
+ self.args.conf = 0.001 # default conf=0.001
+
+ self.plots = {}
+ self.callbacks = _callbacks or callbacks.get_default_callbacks()
+
+ @smart_inference_mode()
+ def __call__(self, trainer=None, model=None):
+ """
+ Supports validation of a pre-trained model if passed or a model being trained
+ if trainer is passed (trainer gets priority).
+ """
+ self.training = trainer is not None
+ if self.training:
+ self.device = trainer.device
+ self.data = trainer.data
+ model = trainer.ema.ema or trainer.model
+ self.args.half = self.device.type != 'cpu' # force FP16 val during training
+ model = model.half() if self.args.half else model.float()
+ self.model = model
+ self.loss = torch.zeros_like(trainer.loss_items, device=trainer.device)
+ self.args.plots = trainer.stopper.possible_stop or (trainer.epoch == trainer.epochs - 1)
+ model.eval()
+ else:
+ callbacks.add_integration_callbacks(self)
+ self.run_callbacks('on_val_start')
+ assert model is not None, 'Either trainer or model is needed for validation'
+ self.device = select_device(self.args.device, self.args.batch)
+ self.args.half &= self.device.type != 'cpu'
+ model = AutoBackend(model, device=self.device, dnn=self.args.dnn, data=self.args.data, fp16=self.args.half)
+ self.model = model
+ stride, pt, jit, engine = model.stride, model.pt, model.jit, model.engine
+ imgsz = check_imgsz(self.args.imgsz, stride=stride)
+ if engine:
+ self.args.batch = model.batch_size
+ else:
+ self.device = model.device
+ if not pt and not jit:
+ self.args.batch = 1 # export.py models default to batch-size 1
+ LOGGER.info(f'Forcing batch=1 square inference (1,3,{imgsz},{imgsz}) for non-PyTorch models')
+
+ if isinstance(self.args.data, str) and self.args.data.endswith('.yaml'):
+ self.data = check_det_dataset(self.args.data)
+ elif self.args.task == 'classify':
+ self.data = check_cls_dataset(self.args.data, split=self.args.split)
+ else:
+ raise FileNotFoundError(emojis(f"Dataset '{self.args.data}' for task={self.args.task} not found ❌"))
+
+ if self.device.type == 'cpu':
+ self.args.workers = 0 # faster CPU val as time dominated by inference, not dataloading
+ if not pt:
+ self.args.rect = False
+ self.dataloader = self.dataloader or self.get_dataloader(self.data.get(self.args.split), self.args.batch)
+
+ model.eval()
+ model.warmup(imgsz=(1 if pt else self.args.batch, 3, imgsz, imgsz)) # warmup
+
+ dt = Profile(), Profile(), Profile(), Profile()
+ n_batches = len(self.dataloader)
+ desc = self.get_desc()
+ # NOTE: keeping `not self.training` in tqdm will eliminate pbar after segmentation evaluation during training,
+ # which may affect classification task since this arg is in yolov5/classify/val.py.
+ # bar = tqdm(self.dataloader, desc, n_batches, not self.training, bar_format=TQDM_BAR_FORMAT)
+ bar = tqdm(self.dataloader, desc, n_batches, bar_format=TQDM_BAR_FORMAT)
+ self.init_metrics(de_parallel(model))
+ self.jdict = [] # empty before each val
+ for batch_i, batch in enumerate(bar):
+ self.run_callbacks('on_val_batch_start')
+ self.batch_i = batch_i
+ # Preprocess
+ with dt[0]:
+ batch = self.preprocess(batch)
+
+ # Inference
+ with dt[1]:
+ preds = model(batch['img'], augment=self.args.augment)
+
+ # Loss
+ with dt[2]:
+ if self.training:
+ self.loss += model.loss(batch, preds)[1]
+
+ # Postprocess
+ with dt[3]:
+ preds = self.postprocess(preds)
+
+ self.update_metrics(preds, batch)
+ if self.args.plots and batch_i < 3:
+ self.plot_val_samples(batch, batch_i)
+ self.plot_predictions(batch, preds, batch_i)
+
+ self.run_callbacks('on_val_batch_end')
+ stats = self.get_stats()
+ self.check_stats(stats)
+ self.speed = dict(zip(self.speed.keys(), (x.t / len(self.dataloader.dataset) * 1E3 for x in dt)))
+ self.finalize_metrics()
+ self.print_results()
+ self.run_callbacks('on_val_end')
+ if self.training:
+ model.float()
+ results = {**stats, **trainer.label_loss_items(self.loss.cpu() / len(self.dataloader), prefix='val')}
+ return {k: round(float(v), 5) for k, v in results.items()} # return results as 5 decimal place floats
+ else:
+ LOGGER.info('Speed: %.1fms preprocess, %.1fms inference, %.1fms loss, %.1fms postprocess per image' %
+ tuple(self.speed.values()))
+ if self.args.save_json and self.jdict:
+ with open(str(self.save_dir / 'predictions.json'), 'w') as f:
+ LOGGER.info(f'Saving {f.name}...')
+ json.dump(self.jdict, f) # flatten and save
+ stats = self.eval_json(stats) # update stats
+ if self.args.plots or self.args.save_json:
+ LOGGER.info(f"Results saved to {colorstr('bold', self.save_dir)}")
+ return stats
+
+ def add_callback(self, event: str, callback):
+ """Appends the given callback."""
+ self.callbacks[event].append(callback)
+
+ def run_callbacks(self, event: str):
+ """Runs all callbacks associated with a specified event."""
+ for callback in self.callbacks.get(event, []):
+ callback(self)
+
+ def get_dataloader(self, dataset_path, batch_size):
+ """Get data loader from dataset path and batch size."""
+ raise NotImplementedError('get_dataloader function not implemented for this validator')
+
+ def build_dataset(self, img_path):
+ """Build dataset"""
+ raise NotImplementedError('build_dataset function not implemented in validator')
+
+ def preprocess(self, batch):
+ """Preprocesses an input batch."""
+ return batch
+
+ def postprocess(self, preds):
+ """Describes and summarizes the purpose of 'postprocess()' but no details mentioned."""
+ return preds
+
+ def init_metrics(self, model):
+ """Initialize performance metrics for the YOLO model."""
+ pass
+
+ def update_metrics(self, preds, batch):
+ """Updates metrics based on predictions and batch."""
+ pass
+
+ def finalize_metrics(self, *args, **kwargs):
+ """Finalizes and returns all metrics."""
+ pass
+
+ def get_stats(self):
+ """Returns statistics about the model's performance."""
+ return {}
+
+ def check_stats(self, stats):
+ """Checks statistics."""
+ pass
+
+ def print_results(self):
+ """Prints the results of the model's predictions."""
+ pass
+
+ def get_desc(self):
+ """Get description of the YOLO model."""
+ pass
+
+ @property
+ def metric_keys(self):
+ """Returns the metric keys used in YOLO training/validation."""
+ return []
+
+ def on_plot(self, name, data=None):
+ """Registers plots (e.g. to be consumed in callbacks)"""
+ self.plots[name] = {'data': data, 'timestamp': time.time()}
+
+ # TODO: may need to put these following functions into callback
+ def plot_val_samples(self, batch, ni):
+ """Plots validation samples during training."""
+ pass
+
+ def plot_predictions(self, batch, preds, ni):
+ """Plots YOLO model predictions on batch images."""
+ pass
+
+ def pred_to_json(self, preds, batch):
+ """Convert predictions to JSON format."""
+ pass
+
+ def eval_json(self, stats):
+ """Evaluate and return JSON format of prediction statistics."""
+ pass
diff --git a/yolov8/ultralytics/yolo/fastsam/__init__.py b/yolov8/ultralytics/yolo/fastsam/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..8f47772fd5eec97ea6776d7fdee00a9ea79dac17
--- /dev/null
+++ b/yolov8/ultralytics/yolo/fastsam/__init__.py
@@ -0,0 +1,8 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .model import FastSAM
+from .predict import FastSAMPredictor
+from .prompt import FastSAMPrompt
+from .val import FastSAMValidator
+
+__all__ = 'FastSAMPredictor', 'FastSAM', 'FastSAMPrompt', 'FastSAMValidator'
diff --git a/yolov8/ultralytics/yolo/fastsam/model.py b/yolov8/ultralytics/yolo/fastsam/model.py
new file mode 100644
index 0000000000000000000000000000000000000000..36c7d427059750ba4b00523d0dee05db646fefc4
--- /dev/null
+++ b/yolov8/ultralytics/yolo/fastsam/model.py
@@ -0,0 +1,111 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+FastSAM model interface.
+
+Usage - Predict:
+ from ultralytics import FastSAM
+
+ model = FastSAM('last.pt')
+ results = model.predict('ultralytics/assets/bus.jpg')
+"""
+
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.engine.exporter import Exporter
+from ultralytics.yolo.engine.model import YOLO
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, ROOT, is_git_dir
+from ultralytics.yolo.utils.checks import check_imgsz
+
+from ...yolo.utils.torch_utils import model_info, smart_inference_mode
+from .predict import FastSAMPredictor
+
+
+class FastSAM(YOLO):
+
+ def __init__(self, model='FastSAM-x.pt'):
+ """Call the __init__ method of the parent class (YOLO) with the updated default model"""
+ if model == 'FastSAM.pt':
+ model = 'FastSAM-x.pt'
+ super().__init__(model=model)
+ # any additional initialization code for FastSAM
+
+ @smart_inference_mode()
+ def predict(self, source=None, stream=False, **kwargs):
+ """
+ Perform prediction using the YOLO model.
+
+ Args:
+ source (str | int | PIL | np.ndarray): The source of the image to make predictions on.
+ Accepts all source types accepted by the YOLO model.
+ stream (bool): Whether to stream the predictions or not. Defaults to False.
+ **kwargs : Additional keyword arguments passed to the predictor.
+ Check the 'configuration' section in the documentation for all available options.
+
+ Returns:
+ (List[ultralytics.yolo.engine.results.Results]): The prediction results.
+ """
+ if source is None:
+ source = ROOT / 'assets' if is_git_dir() else 'https://ultralytics.com/images/bus.jpg'
+ LOGGER.warning(f"WARNING ⚠️ 'source' is missing. Using 'source={source}'.")
+ overrides = self.overrides.copy()
+ overrides['conf'] = 0.25
+ overrides.update(kwargs) # prefer kwargs
+ overrides['mode'] = kwargs.get('mode', 'predict')
+ assert overrides['mode'] in ['track', 'predict']
+ overrides['save'] = kwargs.get('save', False) # do not save by default if called in Python
+ self.predictor = FastSAMPredictor(overrides=overrides)
+ self.predictor.setup_model(model=self.model, verbose=False)
+
+ return self.predictor(source, stream=stream)
+
+ def train(self, **kwargs):
+ """Function trains models but raises an error as FastSAM models do not support training."""
+ raise NotImplementedError("FastSAM models don't support training")
+
+ def val(self, **kwargs):
+ """Run validation given dataset."""
+ overrides = dict(task='segment', mode='val')
+ overrides.update(kwargs) # prefer kwargs
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.imgsz = check_imgsz(args.imgsz, max_dim=1)
+ validator = FastSAM(args=args)
+ validator(model=self.model)
+ self.metrics = validator.metrics
+ return validator.metrics
+
+ @smart_inference_mode()
+ def export(self, **kwargs):
+ """
+ Export model.
+
+ Args:
+ **kwargs : Any other args accepted by the predictors. To see all args check 'configuration' section in docs
+ """
+ overrides = dict(task='detect')
+ overrides.update(kwargs)
+ overrides['mode'] = 'export'
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.task = self.task
+ if args.imgsz == DEFAULT_CFG.imgsz:
+ args.imgsz = self.model.args['imgsz'] # use trained imgsz unless custom value is passed
+ if args.batch == DEFAULT_CFG.batch:
+ args.batch = 1 # default to 1 if not modified
+ return Exporter(overrides=args)(model=self.model)
+
+ def info(self, detailed=False, verbose=True):
+ """
+ Logs model info.
+
+ Args:
+ detailed (bool): Show detailed information about model.
+ verbose (bool): Controls verbosity.
+ """
+ return model_info(self.model, detailed=detailed, verbose=verbose, imgsz=640)
+
+ def __call__(self, source=None, stream=False, **kwargs):
+ """Calls the 'predict' function with given arguments to perform object detection."""
+ return self.predict(source, stream, **kwargs)
+
+ def __getattr__(self, attr):
+ """Raises error if object has no requested attribute."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
diff --git a/yolov8/ultralytics/yolo/fastsam/predict.py b/yolov8/ultralytics/yolo/fastsam/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..0a6ac277cf842af41e382aff9164ae5579e68426
--- /dev/null
+++ b/yolov8/ultralytics/yolo/fastsam/predict.py
@@ -0,0 +1,53 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.fastsam.utils import bbox_iou
+from ultralytics.yolo.utils import DEFAULT_CFG, ops
+from ultralytics.yolo.v8.detect.predict import DetectionPredictor
+
+
+class FastSAMPredictor(DetectionPredictor):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ super().__init__(cfg, overrides, _callbacks)
+ self.args.task = 'segment'
+
+ def postprocess(self, preds, img, orig_imgs):
+ """TODO: filter by classes."""
+ p = ops.non_max_suppression(preds[0],
+ self.args.conf,
+ self.args.iou,
+ agnostic=self.args.agnostic_nms,
+ max_det=self.args.max_det,
+ nc=len(self.model.names),
+ classes=self.args.classes)
+ full_box = torch.zeros_like(p[0][0])
+ full_box[2], full_box[3], full_box[4], full_box[6:] = img.shape[3], img.shape[2], 1.0, 1.0
+ full_box = full_box.view(1, -1)
+ critical_iou_index = bbox_iou(full_box[0][:4], p[0][:, :4], iou_thres=0.9, image_shape=img.shape[2:])
+ if critical_iou_index.numel() != 0:
+ full_box[0][4] = p[0][critical_iou_index][:, 4]
+ full_box[0][6:] = p[0][critical_iou_index][:, 6:]
+ p[0][critical_iou_index] = full_box
+ results = []
+ proto = preds[1][-1] if len(preds[1]) == 3 else preds[1] # second output is len 3 if pt, but only 1 if exported
+ for i, pred in enumerate(p):
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ if not len(pred): # save empty boxes
+ results.append(Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred[:, :6]))
+ continue
+ if self.args.retina_masks:
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], orig_img.shape)
+ masks = ops.process_mask_native(proto[i], pred[:, 6:], pred[:, :4], orig_img.shape[:2]) # HWC
+ else:
+ masks = ops.process_mask(proto[i], pred[:, 6:], pred[:, :4], img.shape[2:], upsample=True) # HWC
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], orig_img.shape)
+ results.append(
+ Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred[:, :6], masks=masks))
+ return results
diff --git a/yolov8/ultralytics/yolo/fastsam/prompt.py b/yolov8/ultralytics/yolo/fastsam/prompt.py
new file mode 100644
index 0000000000000000000000000000000000000000..d34968d8ea900d12e4183b84d80f5c7135b2f5d2
--- /dev/null
+++ b/yolov8/ultralytics/yolo/fastsam/prompt.py
@@ -0,0 +1,406 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+
+import cv2
+import matplotlib.pyplot as plt
+import numpy as np
+import torch
+from PIL import Image
+
+
+class FastSAMPrompt:
+
+ def __init__(self, img_path, results, device='cuda') -> None:
+ # self.img_path = img_path
+ self.device = device
+ self.results = results
+ self.img_path = img_path
+ self.ori_img = cv2.imread(img_path)
+
+ # Import and assign clip
+ try:
+ import clip # for linear_assignment
+ except ImportError:
+ from ultralytics.yolo.utils.checks import check_requirements
+ check_requirements('git+https://github.com/openai/CLIP.git') # required before installing lap from source
+ import clip
+ self.clip = clip
+
+ @staticmethod
+ def _segment_image(image, bbox):
+ image_array = np.array(image)
+ segmented_image_array = np.zeros_like(image_array)
+ x1, y1, x2, y2 = bbox
+ segmented_image_array[y1:y2, x1:x2] = image_array[y1:y2, x1:x2]
+ segmented_image = Image.fromarray(segmented_image_array)
+ black_image = Image.new('RGB', image.size, (255, 255, 255))
+ # transparency_mask = np.zeros_like((), dtype=np.uint8)
+ transparency_mask = np.zeros((image_array.shape[0], image_array.shape[1]), dtype=np.uint8)
+ transparency_mask[y1:y2, x1:x2] = 255
+ transparency_mask_image = Image.fromarray(transparency_mask, mode='L')
+ black_image.paste(segmented_image, mask=transparency_mask_image)
+ return black_image
+
+ @staticmethod
+ def _format_results(result, filter=0):
+ annotations = []
+ n = len(result.masks.data)
+ for i in range(n):
+ mask = result.masks.data[i] == 1.0
+
+ if torch.sum(mask) < filter:
+ continue
+ annotation = {
+ 'id': i,
+ 'segmentation': mask.cpu().numpy(),
+ 'bbox': result.boxes.data[i],
+ 'score': result.boxes.conf[i]}
+ annotation['area'] = annotation['segmentation'].sum()
+ annotations.append(annotation)
+ return annotations
+
+ @staticmethod
+ def filter_masks(annotations): # filter the overlap mask
+ annotations.sort(key=lambda x: x['area'], reverse=True)
+ to_remove = set()
+ for i in range(len(annotations)):
+ a = annotations[i]
+ for j in range(i + 1, len(annotations)):
+ b = annotations[j]
+ if i != j and j not in to_remove and b['area'] < a['area'] and \
+ (a['segmentation'] & b['segmentation']).sum() / b['segmentation'].sum() > 0.8:
+ to_remove.add(j)
+
+ return [a for i, a in enumerate(annotations) if i not in to_remove], to_remove
+
+ @staticmethod
+ def _get_bbox_from_mask(mask):
+ mask = mask.astype(np.uint8)
+ contours, hierarchy = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
+ x1, y1, w, h = cv2.boundingRect(contours[0])
+ x2, y2 = x1 + w, y1 + h
+ if len(contours) > 1:
+ for b in contours:
+ x_t, y_t, w_t, h_t = cv2.boundingRect(b)
+ # 将多个bbox合并成一个
+ x1 = min(x1, x_t)
+ y1 = min(y1, y_t)
+ x2 = max(x2, x_t + w_t)
+ y2 = max(y2, y_t + h_t)
+ h = y2 - y1
+ w = x2 - x1
+ return [x1, y1, x2, y2]
+
+ def plot(self,
+ annotations,
+ output,
+ bbox=None,
+ points=None,
+ point_label=None,
+ mask_random_color=True,
+ better_quality=True,
+ retina=False,
+ withContours=True):
+ if isinstance(annotations[0], dict):
+ annotations = [annotation['segmentation'] for annotation in annotations]
+ result_name = os.path.basename(self.img_path)
+ image = self.ori_img
+ image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
+ original_h = image.shape[0]
+ original_w = image.shape[1]
+ # for macOS only
+ # plt.switch_backend('TkAgg')
+ plt.figure(figsize=(original_w / 100, original_h / 100))
+ # Add subplot with no margin.
+ plt.subplots_adjust(top=1, bottom=0, right=1, left=0, hspace=0, wspace=0)
+ plt.margins(0, 0)
+ plt.gca().xaxis.set_major_locator(plt.NullLocator())
+ plt.gca().yaxis.set_major_locator(plt.NullLocator())
+
+ plt.imshow(image)
+ if better_quality:
+ if isinstance(annotations[0], torch.Tensor):
+ annotations = np.array(annotations.cpu())
+ for i, mask in enumerate(annotations):
+ mask = cv2.morphologyEx(mask.astype(np.uint8), cv2.MORPH_CLOSE, np.ones((3, 3), np.uint8))
+ annotations[i] = cv2.morphologyEx(mask.astype(np.uint8), cv2.MORPH_OPEN, np.ones((8, 8), np.uint8))
+ if self.device == 'cpu':
+ annotations = np.array(annotations)
+ self.fast_show_mask(
+ annotations,
+ plt.gca(),
+ random_color=mask_random_color,
+ bbox=bbox,
+ points=points,
+ pointlabel=point_label,
+ retinamask=retina,
+ target_height=original_h,
+ target_width=original_w,
+ )
+ else:
+ if isinstance(annotations[0], np.ndarray):
+ annotations = torch.from_numpy(annotations)
+ self.fast_show_mask_gpu(
+ annotations,
+ plt.gca(),
+ random_color=mask_random_color,
+ bbox=bbox,
+ points=points,
+ pointlabel=point_label,
+ retinamask=retina,
+ target_height=original_h,
+ target_width=original_w,
+ )
+ if isinstance(annotations, torch.Tensor):
+ annotations = annotations.cpu().numpy()
+ if withContours:
+ contour_all = []
+ temp = np.zeros((original_h, original_w, 1))
+ for i, mask in enumerate(annotations):
+ if type(mask) == dict:
+ mask = mask['segmentation']
+ annotation = mask.astype(np.uint8)
+ if not retina:
+ annotation = cv2.resize(
+ annotation,
+ (original_w, original_h),
+ interpolation=cv2.INTER_NEAREST,
+ )
+ contours, hierarchy = cv2.findContours(annotation, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
+ contour_all.extend(iter(contours))
+ cv2.drawContours(temp, contour_all, -1, (255, 255, 255), 2)
+ color = np.array([0 / 255, 0 / 255, 1.0, 0.8])
+ contour_mask = temp / 255 * color.reshape(1, 1, -1)
+ plt.imshow(contour_mask)
+
+ save_path = output
+ if not os.path.exists(save_path):
+ os.makedirs(save_path)
+ plt.axis('off')
+ fig = plt.gcf()
+ plt.draw()
+
+ try:
+ buf = fig.canvas.tostring_rgb()
+ except AttributeError:
+ fig.canvas.draw()
+ buf = fig.canvas.tostring_rgb()
+ cols, rows = fig.canvas.get_width_height()
+ img_array = np.frombuffer(buf, dtype=np.uint8).reshape(rows, cols, 3)
+ cv2.imwrite(os.path.join(save_path, result_name), cv2.cvtColor(img_array, cv2.COLOR_RGB2BGR))
+
+ # CPU post process
+ def fast_show_mask(
+ self,
+ annotation,
+ ax,
+ random_color=False,
+ bbox=None,
+ points=None,
+ pointlabel=None,
+ retinamask=True,
+ target_height=960,
+ target_width=960,
+ ):
+ msak_sum = annotation.shape[0]
+ height = annotation.shape[1]
+ weight = annotation.shape[2]
+ # 将annotation 按照面积 排序
+ areas = np.sum(annotation, axis=(1, 2))
+ sorted_indices = np.argsort(areas)
+ annotation = annotation[sorted_indices]
+
+ index = (annotation != 0).argmax(axis=0)
+ if random_color:
+ color = np.random.random((msak_sum, 1, 1, 3))
+ else:
+ color = np.ones((msak_sum, 1, 1, 3)) * np.array([30 / 255, 144 / 255, 1.0])
+ transparency = np.ones((msak_sum, 1, 1, 1)) * 0.6
+ visual = np.concatenate([color, transparency], axis=-1)
+ mask_image = np.expand_dims(annotation, -1) * visual
+
+ show = np.zeros((height, weight, 4))
+ h_indices, w_indices = np.meshgrid(np.arange(height), np.arange(weight), indexing='ij')
+ indices = (index[h_indices, w_indices], h_indices, w_indices, slice(None))
+ # 使用向量化索引更新show的值
+ show[h_indices, w_indices, :] = mask_image[indices]
+ if bbox is not None:
+ x1, y1, x2, y2 = bbox
+ ax.add_patch(plt.Rectangle((x1, y1), x2 - x1, y2 - y1, fill=False, edgecolor='b', linewidth=1))
+ # draw point
+ if points is not None:
+ plt.scatter(
+ [point[0] for i, point in enumerate(points) if pointlabel[i] == 1],
+ [point[1] for i, point in enumerate(points) if pointlabel[i] == 1],
+ s=20,
+ c='y',
+ )
+ plt.scatter(
+ [point[0] for i, point in enumerate(points) if pointlabel[i] == 0],
+ [point[1] for i, point in enumerate(points) if pointlabel[i] == 0],
+ s=20,
+ c='m',
+ )
+
+ if not retinamask:
+ show = cv2.resize(show, (target_width, target_height), interpolation=cv2.INTER_NEAREST)
+ ax.imshow(show)
+
+ def fast_show_mask_gpu(
+ self,
+ annotation,
+ ax,
+ random_color=False,
+ bbox=None,
+ points=None,
+ pointlabel=None,
+ retinamask=True,
+ target_height=960,
+ target_width=960,
+ ):
+ msak_sum = annotation.shape[0]
+ height = annotation.shape[1]
+ weight = annotation.shape[2]
+ areas = torch.sum(annotation, dim=(1, 2))
+ sorted_indices = torch.argsort(areas, descending=False)
+ annotation = annotation[sorted_indices]
+ # 找每个位置第一个非零值下标
+ index = (annotation != 0).to(torch.long).argmax(dim=0)
+ if random_color:
+ color = torch.rand((msak_sum, 1, 1, 3)).to(annotation.device)
+ else:
+ color = torch.ones((msak_sum, 1, 1, 3)).to(annotation.device) * torch.tensor([30 / 255, 144 / 255, 1.0]).to(
+ annotation.device)
+ transparency = torch.ones((msak_sum, 1, 1, 1)).to(annotation.device) * 0.6
+ visual = torch.cat([color, transparency], dim=-1)
+ mask_image = torch.unsqueeze(annotation, -1) * visual
+ # 按index取数,index指每个位置选哪个batch的数,把mask_image转成一个batch的形式
+ show = torch.zeros((height, weight, 4)).to(annotation.device)
+ h_indices, w_indices = torch.meshgrid(torch.arange(height), torch.arange(weight), indexing='ij')
+ indices = (index[h_indices, w_indices], h_indices, w_indices, slice(None))
+ # 使用向量化索引更新show的值
+ show[h_indices, w_indices, :] = mask_image[indices]
+ show_cpu = show.cpu().numpy()
+ if bbox is not None:
+ x1, y1, x2, y2 = bbox
+ ax.add_patch(plt.Rectangle((x1, y1), x2 - x1, y2 - y1, fill=False, edgecolor='b', linewidth=1))
+ # draw point
+ if points is not None:
+ plt.scatter(
+ [point[0] for i, point in enumerate(points) if pointlabel[i] == 1],
+ [point[1] for i, point in enumerate(points) if pointlabel[i] == 1],
+ s=20,
+ c='y',
+ )
+ plt.scatter(
+ [point[0] for i, point in enumerate(points) if pointlabel[i] == 0],
+ [point[1] for i, point in enumerate(points) if pointlabel[i] == 0],
+ s=20,
+ c='m',
+ )
+ if not retinamask:
+ show_cpu = cv2.resize(show_cpu, (target_width, target_height), interpolation=cv2.INTER_NEAREST)
+ ax.imshow(show_cpu)
+
+ # clip
+ @torch.no_grad()
+ def retrieve(self, model, preprocess, elements, search_text: str, device) -> int:
+ preprocessed_images = [preprocess(image).to(device) for image in elements]
+ tokenized_text = self.clip.tokenize([search_text]).to(device)
+ stacked_images = torch.stack(preprocessed_images)
+ image_features = model.encode_image(stacked_images)
+ text_features = model.encode_text(tokenized_text)
+ image_features /= image_features.norm(dim=-1, keepdim=True)
+ text_features /= text_features.norm(dim=-1, keepdim=True)
+ probs = 100.0 * image_features @ text_features.T
+ return probs[:, 0].softmax(dim=0)
+
+ def _crop_image(self, format_results):
+
+ image = Image.fromarray(cv2.cvtColor(self.ori_img, cv2.COLOR_BGR2RGB))
+ ori_w, ori_h = image.size
+ annotations = format_results
+ mask_h, mask_w = annotations[0]['segmentation'].shape
+ if ori_w != mask_w or ori_h != mask_h:
+ image = image.resize((mask_w, mask_h))
+ cropped_boxes = []
+ cropped_images = []
+ not_crop = []
+ filter_id = []
+ # annotations, _ = filter_masks(annotations)
+ # filter_id = list(_)
+ for _, mask in enumerate(annotations):
+ if np.sum(mask['segmentation']) <= 100:
+ filter_id.append(_)
+ continue
+ bbox = self._get_bbox_from_mask(mask['segmentation']) # mask 的 bbox
+ cropped_boxes.append(self._segment_image(image, bbox)) # 保存裁剪的图片
+ # cropped_boxes.append(segment_image(image,mask["segmentation"]))
+ cropped_images.append(bbox) # 保存裁剪的图片的bbox
+
+ return cropped_boxes, cropped_images, not_crop, filter_id, annotations
+
+ def box_prompt(self, bbox):
+
+ assert (bbox[2] != 0 and bbox[3] != 0)
+ masks = self.results[0].masks.data
+ target_height = self.ori_img.shape[0]
+ target_width = self.ori_img.shape[1]
+ h = masks.shape[1]
+ w = masks.shape[2]
+ if h != target_height or w != target_width:
+ bbox = [
+ int(bbox[0] * w / target_width),
+ int(bbox[1] * h / target_height),
+ int(bbox[2] * w / target_width),
+ int(bbox[3] * h / target_height), ]
+ bbox[0] = max(round(bbox[0]), 0)
+ bbox[1] = max(round(bbox[1]), 0)
+ bbox[2] = min(round(bbox[2]), w)
+ bbox[3] = min(round(bbox[3]), h)
+
+ # IoUs = torch.zeros(len(masks), dtype=torch.float32)
+ bbox_area = (bbox[3] - bbox[1]) * (bbox[2] - bbox[0])
+
+ masks_area = torch.sum(masks[:, bbox[1]:bbox[3], bbox[0]:bbox[2]], dim=(1, 2))
+ orig_masks_area = torch.sum(masks, dim=(1, 2))
+
+ union = bbox_area + orig_masks_area - masks_area
+ IoUs = masks_area / union
+ max_iou_index = torch.argmax(IoUs)
+
+ return np.array([masks[max_iou_index].cpu().numpy()])
+
+ def point_prompt(self, points, pointlabel): # numpy 处理
+
+ masks = self._format_results(self.results[0], 0)
+ target_height = self.ori_img.shape[0]
+ target_width = self.ori_img.shape[1]
+ h = masks[0]['segmentation'].shape[0]
+ w = masks[0]['segmentation'].shape[1]
+ if h != target_height or w != target_width:
+ points = [[int(point[0] * w / target_width), int(point[1] * h / target_height)] for point in points]
+ onemask = np.zeros((h, w))
+ for i, annotation in enumerate(masks):
+ mask = annotation['segmentation'] if type(annotation) == dict else annotation
+ for i, point in enumerate(points):
+ if mask[point[1], point[0]] == 1 and pointlabel[i] == 1:
+ onemask += mask
+ if mask[point[1], point[0]] == 1 and pointlabel[i] == 0:
+ onemask -= mask
+ onemask = onemask >= 1
+ return np.array([onemask])
+
+ def text_prompt(self, text):
+ format_results = self._format_results(self.results[0], 0)
+ cropped_boxes, cropped_images, not_crop, filter_id, annotations = self._crop_image(format_results)
+ clip_model, preprocess = self.clip.load('ViT-B/32', device=self.device)
+ scores = self.retrieve(clip_model, preprocess, cropped_boxes, text, device=self.device)
+ max_idx = scores.argsort()
+ max_idx = max_idx[-1]
+ max_idx += sum(np.array(filter_id) <= int(max_idx))
+ return np.array([annotations[max_idx]['segmentation']])
+
+ def everything_prompt(self):
+ return self.results[0].masks.data
diff --git a/yolov8/ultralytics/yolo/fastsam/utils.py b/yolov8/ultralytics/yolo/fastsam/utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..c5b6cc235e3a758c344f4aca29f050633d96dbc7
--- /dev/null
+++ b/yolov8/ultralytics/yolo/fastsam/utils.py
@@ -0,0 +1,64 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+
+def adjust_bboxes_to_image_border(boxes, image_shape, threshold=20):
+ """
+ Adjust bounding boxes to stick to image border if they are within a certain threshold.
+
+ Args:
+ boxes: (n, 4)
+ image_shape: (height, width)
+ threshold: pixel threshold
+
+ Returns:
+ adjusted_boxes: adjusted bounding boxes
+ """
+
+ # Image dimensions
+ h, w = image_shape
+
+ # Adjust boxes
+ boxes[boxes[:, 0] < threshold, 0] = 0 # x1
+ boxes[boxes[:, 1] < threshold, 1] = 0 # y1
+ boxes[boxes[:, 2] > w - threshold, 2] = w # x2
+ boxes[boxes[:, 3] > h - threshold, 3] = h # y2
+ return boxes
+
+
+def bbox_iou(box1, boxes, iou_thres=0.9, image_shape=(640, 640), raw_output=False):
+ """
+ Compute the Intersection-Over-Union of a bounding box with respect to an array of other bounding boxes.
+
+ Args:
+ box1: (4, )
+ boxes: (n, 4)
+
+ Returns:
+ high_iou_indices: Indices of boxes with IoU > thres
+ """
+ boxes = adjust_bboxes_to_image_border(boxes, image_shape)
+ # obtain coordinates for intersections
+ x1 = torch.max(box1[0], boxes[:, 0])
+ y1 = torch.max(box1[1], boxes[:, 1])
+ x2 = torch.min(box1[2], boxes[:, 2])
+ y2 = torch.min(box1[3], boxes[:, 3])
+
+ # compute the area of intersection
+ intersection = (x2 - x1).clamp(0) * (y2 - y1).clamp(0)
+
+ # compute the area of both individual boxes
+ box1_area = (box1[2] - box1[0]) * (box1[3] - box1[1])
+ box2_area = (boxes[:, 2] - boxes[:, 0]) * (boxes[:, 3] - boxes[:, 1])
+
+ # compute the area of union
+ union = box1_area + box2_area - intersection
+
+ # compute the IoU
+ iou = intersection / union # Should be shape (n, )
+ if raw_output:
+ return 0 if iou.numel() == 0 else iou
+
+ # return indices of boxes with IoU > thres
+ return torch.nonzero(iou > iou_thres).flatten()
diff --git a/yolov8/ultralytics/yolo/fastsam/val.py b/yolov8/ultralytics/yolo/fastsam/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..250bd5e41a89af4e72a7b1d4f38c4eda7b5ee649
--- /dev/null
+++ b/yolov8/ultralytics/yolo/fastsam/val.py
@@ -0,0 +1,244 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from multiprocessing.pool import ThreadPool
+from pathlib import Path
+
+import numpy as np
+import torch
+import torch.nn.functional as F
+
+from ultralytics.yolo.utils import LOGGER, NUM_THREADS, ops
+from ultralytics.yolo.utils.checks import check_requirements
+from ultralytics.yolo.utils.metrics import SegmentMetrics, box_iou, mask_iou
+from ultralytics.yolo.utils.plotting import output_to_target, plot_images
+from ultralytics.yolo.v8.detect import DetectionValidator
+
+
+class FastSAMValidator(DetectionValidator):
+
+ def __init__(self, dataloader=None, save_dir=None, pbar=None, args=None, _callbacks=None):
+ """Initialize SegmentationValidator and set task to 'segment', metrics to SegmentMetrics."""
+ super().__init__(dataloader, save_dir, pbar, args, _callbacks)
+ self.args.task = 'segment'
+ self.metrics = SegmentMetrics(save_dir=self.save_dir, on_plot=self.on_plot)
+
+ def preprocess(self, batch):
+ """Preprocesses batch by converting masks to float and sending to device."""
+ batch = super().preprocess(batch)
+ batch['masks'] = batch['masks'].to(self.device).float()
+ return batch
+
+ def init_metrics(self, model):
+ """Initialize metrics and select mask processing function based on save_json flag."""
+ super().init_metrics(model)
+ self.plot_masks = []
+ if self.args.save_json:
+ check_requirements('pycocotools>=2.0.6')
+ self.process = ops.process_mask_upsample # more accurate
+ else:
+ self.process = ops.process_mask # faster
+
+ def get_desc(self):
+ """Return a formatted description of evaluation metrics."""
+ return ('%22s' + '%11s' * 10) % ('Class', 'Images', 'Instances', 'Box(P', 'R', 'mAP50', 'mAP50-95)', 'Mask(P',
+ 'R', 'mAP50', 'mAP50-95)')
+
+ def postprocess(self, preds):
+ """Postprocesses YOLO predictions and returns output detections with proto."""
+ p = ops.non_max_suppression(preds[0],
+ self.args.conf,
+ self.args.iou,
+ labels=self.lb,
+ multi_label=True,
+ agnostic=self.args.single_cls,
+ max_det=self.args.max_det,
+ nc=self.nc)
+ proto = preds[1][-1] if len(preds[1]) == 3 else preds[1] # second output is len 3 if pt, but only 1 if exported
+ return p, proto
+
+ def update_metrics(self, preds, batch):
+ """Metrics."""
+ for si, (pred, proto) in enumerate(zip(preds[0], preds[1])):
+ idx = batch['batch_idx'] == si
+ cls = batch['cls'][idx]
+ bbox = batch['bboxes'][idx]
+ nl, npr = cls.shape[0], pred.shape[0] # number of labels, predictions
+ shape = batch['ori_shape'][si]
+ correct_masks = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ correct_bboxes = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ self.seen += 1
+
+ if npr == 0:
+ if nl:
+ self.stats.append((correct_bboxes, correct_masks, *torch.zeros(
+ (2, 0), device=self.device), cls.squeeze(-1)))
+ if self.args.plots:
+ self.confusion_matrix.process_batch(detections=None, labels=cls.squeeze(-1))
+ continue
+
+ # Masks
+ midx = [si] if self.args.overlap_mask else idx
+ gt_masks = batch['masks'][midx]
+ pred_masks = self.process(proto, pred[:, 6:], pred[:, :4], shape=batch['img'][si].shape[1:])
+
+ # Predictions
+ if self.args.single_cls:
+ pred[:, 5] = 0
+ predn = pred.clone()
+ ops.scale_boxes(batch['img'][si].shape[1:], predn[:, :4], shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space pred
+
+ # Evaluate
+ if nl:
+ height, width = batch['img'].shape[2:]
+ tbox = ops.xywh2xyxy(bbox) * torch.tensor(
+ (width, height, width, height), device=self.device) # target boxes
+ ops.scale_boxes(batch['img'][si].shape[1:], tbox, shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space labels
+ labelsn = torch.cat((cls, tbox), 1) # native-space labels
+ correct_bboxes = self._process_batch(predn, labelsn)
+ # TODO: maybe remove these `self.` arguments as they already are member variable
+ correct_masks = self._process_batch(predn,
+ labelsn,
+ pred_masks,
+ gt_masks,
+ overlap=self.args.overlap_mask,
+ masks=True)
+ if self.args.plots:
+ self.confusion_matrix.process_batch(predn, labelsn)
+
+ # Append correct_masks, correct_boxes, pconf, pcls, tcls
+ self.stats.append((correct_bboxes, correct_masks, pred[:, 4], pred[:, 5], cls.squeeze(-1)))
+
+ pred_masks = torch.as_tensor(pred_masks, dtype=torch.uint8)
+ if self.args.plots and self.batch_i < 3:
+ self.plot_masks.append(pred_masks[:15].cpu()) # filter top 15 to plot
+
+ # Save
+ if self.args.save_json:
+ pred_masks = ops.scale_image(pred_masks.permute(1, 2, 0).contiguous().cpu().numpy(),
+ shape,
+ ratio_pad=batch['ratio_pad'][si])
+ self.pred_to_json(predn, batch['im_file'][si], pred_masks)
+ # if self.args.save_txt:
+ # save_one_txt(predn, save_conf, shape, file=save_dir / 'labels' / f'{path.stem}.txt')
+
+ def finalize_metrics(self, *args, **kwargs):
+ """Sets speed and confusion matrix for evaluation metrics."""
+ self.metrics.speed = self.speed
+ self.metrics.confusion_matrix = self.confusion_matrix
+
+ def _process_batch(self, detections, labels, pred_masks=None, gt_masks=None, overlap=False, masks=False):
+ """
+ Return correct prediction matrix
+ Arguments:
+ detections (array[N, 6]), x1, y1, x2, y2, conf, class
+ labels (array[M, 5]), class, x1, y1, x2, y2
+ Returns:
+ correct (array[N, 10]), for 10 IoU levels
+ """
+ if masks:
+ if overlap:
+ nl = len(labels)
+ index = torch.arange(nl, device=gt_masks.device).view(nl, 1, 1) + 1
+ gt_masks = gt_masks.repeat(nl, 1, 1) # shape(1,640,640) -> (n,640,640)
+ gt_masks = torch.where(gt_masks == index, 1.0, 0.0)
+ if gt_masks.shape[1:] != pred_masks.shape[1:]:
+ gt_masks = F.interpolate(gt_masks[None], pred_masks.shape[1:], mode='bilinear', align_corners=False)[0]
+ gt_masks = gt_masks.gt_(0.5)
+ iou = mask_iou(gt_masks.view(gt_masks.shape[0], -1), pred_masks.view(pred_masks.shape[0], -1))
+ else: # boxes
+ iou = box_iou(labels[:, 1:], detections[:, :4])
+
+ correct = np.zeros((detections.shape[0], self.iouv.shape[0])).astype(bool)
+ correct_class = labels[:, 0:1] == detections[:, 5]
+ for i in range(len(self.iouv)):
+ x = torch.where((iou >= self.iouv[i]) & correct_class) # IoU > threshold and classes match
+ if x[0].shape[0]:
+ matches = torch.cat((torch.stack(x, 1), iou[x[0], x[1]][:, None]),
+ 1).cpu().numpy() # [label, detect, iou]
+ if x[0].shape[0] > 1:
+ matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 1], return_index=True)[1]]
+ # matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 0], return_index=True)[1]]
+ correct[matches[:, 1].astype(int), i] = True
+ return torch.tensor(correct, dtype=torch.bool, device=detections.device)
+
+ def plot_val_samples(self, batch, ni):
+ """Plots validation samples with bounding box labels."""
+ plot_images(batch['img'],
+ batch['batch_idx'],
+ batch['cls'].squeeze(-1),
+ batch['bboxes'],
+ batch['masks'],
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_labels.jpg',
+ names=self.names,
+ on_plot=self.on_plot)
+
+ def plot_predictions(self, batch, preds, ni):
+ """Plots batch predictions with masks and bounding boxes."""
+ plot_images(
+ batch['img'],
+ *output_to_target(preds[0], max_det=15), # not set to self.args.max_det due to slow plotting speed
+ torch.cat(self.plot_masks, dim=0) if len(self.plot_masks) else self.plot_masks,
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_pred.jpg',
+ names=self.names,
+ on_plot=self.on_plot) # pred
+ self.plot_masks.clear()
+
+ def pred_to_json(self, predn, filename, pred_masks):
+ """Save one JSON result."""
+ # Example result = {"image_id": 42, "category_id": 18, "bbox": [258.15, 41.29, 348.26, 243.78], "score": 0.236}
+ from pycocotools.mask import encode # noqa
+
+ def single_encode(x):
+ """Encode predicted masks as RLE and append results to jdict."""
+ rle = encode(np.asarray(x[:, :, None], order='F', dtype='uint8'))[0]
+ rle['counts'] = rle['counts'].decode('utf-8')
+ return rle
+
+ stem = Path(filename).stem
+ image_id = int(stem) if stem.isnumeric() else stem
+ box = ops.xyxy2xywh(predn[:, :4]) # xywh
+ box[:, :2] -= box[:, 2:] / 2 # xy center to top-left corner
+ pred_masks = np.transpose(pred_masks, (2, 0, 1))
+ with ThreadPool(NUM_THREADS) as pool:
+ rles = pool.map(single_encode, pred_masks)
+ for i, (p, b) in enumerate(zip(predn.tolist(), box.tolist())):
+ self.jdict.append({
+ 'image_id': image_id,
+ 'category_id': self.class_map[int(p[5])],
+ 'bbox': [round(x, 3) for x in b],
+ 'score': round(p[4], 5),
+ 'segmentation': rles[i]})
+
+ def eval_json(self, stats):
+ """Return COCO-style object detection evaluation metrics."""
+ if self.args.save_json and self.is_coco and len(self.jdict):
+ anno_json = self.data['path'] / 'annotations/instances_val2017.json' # annotations
+ pred_json = self.save_dir / 'predictions.json' # predictions
+ LOGGER.info(f'\nEvaluating pycocotools mAP using {pred_json} and {anno_json}...')
+ try: # https://github.com/cocodataset/cocoapi/blob/master/PythonAPI/pycocoEvalDemo.ipynb
+ check_requirements('pycocotools>=2.0.6')
+ from pycocotools.coco import COCO # noqa
+ from pycocotools.cocoeval import COCOeval # noqa
+
+ for x in anno_json, pred_json:
+ assert x.is_file(), f'{x} file not found'
+ anno = COCO(str(anno_json)) # init annotations api
+ pred = anno.loadRes(str(pred_json)) # init predictions api (must pass string, not Path)
+ for i, eval in enumerate([COCOeval(anno, pred, 'bbox'), COCOeval(anno, pred, 'segm')]):
+ if self.is_coco:
+ eval.params.imgIds = [int(Path(x).stem) for x in self.dataloader.dataset.im_files] # im to eval
+ eval.evaluate()
+ eval.accumulate()
+ eval.summarize()
+ idx = i * 4 + 2
+ stats[self.metrics.keys[idx + 1]], stats[
+ self.metrics.keys[idx]] = eval.stats[:2] # update mAP50-95 and mAP50
+ except Exception as e:
+ LOGGER.warning(f'pycocotools unable to run: {e}')
+ return stats
diff --git a/yolov8/ultralytics/yolo/nas/__init__.py b/yolov8/ultralytics/yolo/nas/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..eec3837d492b08db2c8be6a033b7f3870dd6e0df
--- /dev/null
+++ b/yolov8/ultralytics/yolo/nas/__init__.py
@@ -0,0 +1,7 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .model import NAS
+from .predict import NASPredictor
+from .val import NASValidator
+
+__all__ = 'NASPredictor', 'NASValidator', 'NAS'
diff --git a/yolov8/ultralytics/yolo/nas/model.py b/yolov8/ultralytics/yolo/nas/model.py
new file mode 100644
index 0000000000000000000000000000000000000000..bfe7dcdfd86274edf75634ef588c3e7eb184fa3b
--- /dev/null
+++ b/yolov8/ultralytics/yolo/nas/model.py
@@ -0,0 +1,133 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+YOLO-NAS model interface.
+
+Usage - Predict:
+ from ultralytics import NAS
+
+ model = NAS('yolo_nas_s')
+ results = model.predict('ultralytics/assets/bus.jpg')
+"""
+
+from pathlib import Path
+
+import torch
+
+from ultralytics.yolo.cfg import get_cfg
+from ultralytics.yolo.engine.exporter import Exporter
+from ultralytics.yolo.utils import DEFAULT_CFG, DEFAULT_CFG_DICT, LOGGER, ROOT, is_git_dir
+from ultralytics.yolo.utils.checks import check_imgsz
+
+from ...yolo.utils.torch_utils import model_info, smart_inference_mode
+from .predict import NASPredictor
+from .val import NASValidator
+
+
+class NAS:
+
+ def __init__(self, model='yolo_nas_s.pt') -> None:
+ # Load or create new NAS model
+ import super_gradients
+
+ self.predictor = None
+ suffix = Path(model).suffix
+ if suffix == '.pt':
+ self._load(model)
+ elif suffix == '':
+ self.model = super_gradients.training.models.get(model, pretrained_weights='coco')
+ self.task = 'detect'
+ self.model.args = DEFAULT_CFG_DICT # attach args to model
+
+ # Standardize model
+ self.model.fuse = lambda verbose=True: self.model
+ self.model.stride = torch.tensor([32])
+ self.model.names = dict(enumerate(self.model._class_names))
+ self.model.is_fused = lambda: False # for info()
+ self.model.yaml = {} # for info()
+ self.model.pt_path = model # for export()
+ self.model.task = 'detect' # for export()
+ self.info()
+
+ @smart_inference_mode()
+ def _load(self, weights: str):
+ self.model = torch.load(weights)
+
+ @smart_inference_mode()
+ def predict(self, source=None, stream=False, **kwargs):
+ """
+ Perform prediction using the YOLO model.
+
+ Args:
+ source (str | int | PIL | np.ndarray): The source of the image to make predictions on.
+ Accepts all source types accepted by the YOLO model.
+ stream (bool): Whether to stream the predictions or not. Defaults to False.
+ **kwargs : Additional keyword arguments passed to the predictor.
+ Check the 'configuration' section in the documentation for all available options.
+
+ Returns:
+ (List[ultralytics.yolo.engine.results.Results]): The prediction results.
+ """
+ if source is None:
+ source = ROOT / 'assets' if is_git_dir() else 'https://ultralytics.com/images/bus.jpg'
+ LOGGER.warning(f"WARNING ⚠️ 'source' is missing. Using 'source={source}'.")
+ overrides = dict(conf=0.25, task='detect', mode='predict')
+ overrides.update(kwargs) # prefer kwargs
+ if not self.predictor:
+ self.predictor = NASPredictor(overrides=overrides)
+ self.predictor.setup_model(model=self.model)
+ else: # only update args if predictor is already setup
+ self.predictor.args = get_cfg(self.predictor.args, overrides)
+ return self.predictor(source, stream=stream)
+
+ def train(self, **kwargs):
+ """Function trains models but raises an error as NAS models do not support training."""
+ raise NotImplementedError("NAS models don't support training")
+
+ def val(self, **kwargs):
+ """Run validation given dataset."""
+ overrides = dict(task='detect', mode='val')
+ overrides.update(kwargs) # prefer kwargs
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.imgsz = check_imgsz(args.imgsz, max_dim=1)
+ validator = NASValidator(args=args)
+ validator(model=self.model)
+ self.metrics = validator.metrics
+ return validator.metrics
+
+ @smart_inference_mode()
+ def export(self, **kwargs):
+ """
+ Export model.
+
+ Args:
+ **kwargs : Any other args accepted by the predictors. To see all args check 'configuration' section in docs
+ """
+ overrides = dict(task='detect')
+ overrides.update(kwargs)
+ overrides['mode'] = 'export'
+ args = get_cfg(cfg=DEFAULT_CFG, overrides=overrides)
+ args.task = self.task
+ if args.imgsz == DEFAULT_CFG.imgsz:
+ args.imgsz = self.model.args['imgsz'] # use trained imgsz unless custom value is passed
+ if args.batch == DEFAULT_CFG.batch:
+ args.batch = 1 # default to 1 if not modified
+ return Exporter(overrides=args)(model=self.model)
+
+ def info(self, detailed=False, verbose=True):
+ """
+ Logs model info.
+
+ Args:
+ detailed (bool): Show detailed information about model.
+ verbose (bool): Controls verbosity.
+ """
+ return model_info(self.model, detailed=detailed, verbose=verbose, imgsz=640)
+
+ def __call__(self, source=None, stream=False, **kwargs):
+ """Calls the 'predict' function with given arguments to perform object detection."""
+ return self.predict(source, stream, **kwargs)
+
+ def __getattr__(self, attr):
+ """Raises error if object has no requested attribute."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
diff --git a/yolov8/ultralytics/yolo/nas/predict.py b/yolov8/ultralytics/yolo/nas/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..e135bc1ef37fe32fcdf5947b69b2bb34096d12b7
--- /dev/null
+++ b/yolov8/ultralytics/yolo/nas/predict.py
@@ -0,0 +1,35 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.engine.predictor import BasePredictor
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils import ops
+from ultralytics.yolo.utils.ops import xyxy2xywh
+
+
+class NASPredictor(BasePredictor):
+
+ def postprocess(self, preds_in, img, orig_imgs):
+ """Postprocesses predictions and returns a list of Results objects."""
+
+ # Cat boxes and class scores
+ boxes = xyxy2xywh(preds_in[0][0])
+ preds = torch.cat((boxes, preds_in[0][1]), -1).permute(0, 2, 1)
+
+ preds = ops.non_max_suppression(preds,
+ self.args.conf,
+ self.args.iou,
+ agnostic=self.args.agnostic_nms,
+ max_det=self.args.max_det,
+ classes=self.args.classes)
+
+ results = []
+ for i, pred in enumerate(preds):
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], orig_img.shape)
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ results.append(Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred))
+ return results
diff --git a/yolov8/ultralytics/yolo/nas/val.py b/yolov8/ultralytics/yolo/nas/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..474cf6bd04d30563784fa21e469f91df53f0b3e0
--- /dev/null
+++ b/yolov8/ultralytics/yolo/nas/val.py
@@ -0,0 +1,25 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.utils import ops
+from ultralytics.yolo.utils.ops import xyxy2xywh
+from ultralytics.yolo.v8.detect import DetectionValidator
+
+__all__ = ['NASValidator']
+
+
+class NASValidator(DetectionValidator):
+
+ def postprocess(self, preds_in):
+ """Apply Non-maximum suppression to prediction outputs."""
+ boxes = xyxy2xywh(preds_in[0][0])
+ preds = torch.cat((boxes, preds_in[0][1]), -1).permute(0, 2, 1)
+ return ops.non_max_suppression(preds,
+ self.args.conf,
+ self.args.iou,
+ labels=self.lb,
+ multi_label=False,
+ agnostic=self.args.single_cls,
+ max_det=self.args.max_det,
+ max_time_img=0.5)
diff --git a/yolov8/ultralytics/yolo/utils/__init__.py b/yolov8/ultralytics/yolo/utils/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..bcfce51eb93e38843a9843f3daeb8f4085300ba9
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/__init__.py
@@ -0,0 +1,779 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+import inspect
+import logging.config
+import os
+import platform
+import re
+import subprocess
+import sys
+import threading
+import urllib
+import uuid
+from pathlib import Path
+from types import SimpleNamespace
+from typing import Union
+
+import cv2
+import matplotlib.pyplot as plt
+import numpy as np
+import torch
+import yaml
+
+from ultralytics import __version__
+
+# PyTorch Multi-GPU DDP Constants
+RANK = int(os.getenv('RANK', -1))
+LOCAL_RANK = int(os.getenv('LOCAL_RANK', -1)) # https://pytorch.org/docs/stable/elastic/run.html
+WORLD_SIZE = int(os.getenv('WORLD_SIZE', 1))
+
+# Other Constants
+FILE = Path(__file__).resolve()
+ROOT = FILE.parents[2] # YOLO
+DEFAULT_CFG_PATH = ROOT / 'yolo/cfg/default.yaml'
+NUM_THREADS = min(8, max(1, os.cpu_count() - 1)) # number of YOLOv5 multiprocessing threads
+AUTOINSTALL = str(os.getenv('YOLO_AUTOINSTALL', True)).lower() == 'true' # global auto-install mode
+VERBOSE = str(os.getenv('YOLO_VERBOSE', True)).lower() == 'true' # global verbose mode
+TQDM_BAR_FORMAT = '{l_bar}{bar:10}{r_bar}' # tqdm bar format
+LOGGING_NAME = 'ultralytics'
+MACOS, LINUX, WINDOWS = (platform.system() == x for x in ['Darwin', 'Linux', 'Windows']) # environment booleans
+ARM64 = platform.machine() in ('arm64', 'aarch64') # ARM64 booleans
+HELP_MSG = \
+ """
+ Usage examples for running YOLOv8:
+
+ 1. Install the ultralytics package:
+
+ pip install ultralytics
+
+ 2. Use the Python SDK:
+
+ from ultralytics import YOLO
+
+ # Load a model
+ model = YOLO('yolov8n.yaml') # build a new model from scratch
+ model = YOLO("yolov8n.pt") # load a pretrained model (recommended for training)
+
+ # Use the model
+ results = model.train(data="coco128.yaml", epochs=3) # train the model
+ results = model.val() # evaluate model performance on the validation set
+ results = model('https://ultralytics.com/images/bus.jpg') # predict on an image
+ success = model.export(format='onnx') # export the model to ONNX format
+
+ 3. Use the command line interface (CLI):
+
+ YOLOv8 'yolo' CLI commands use the following syntax:
+
+ yolo TASK MODE ARGS
+
+ Where TASK (optional) is one of [detect, segment, classify]
+ MODE (required) is one of [train, val, predict, export]
+ ARGS (optional) are any number of custom 'arg=value' pairs like 'imgsz=320' that override defaults.
+ See all ARGS at https://docs.ultralytics.com/usage/cfg or with 'yolo cfg'
+
+ - Train a detection model for 10 epochs with an initial learning_rate of 0.01
+ yolo detect train data=coco128.yaml model=yolov8n.pt epochs=10 lr0=0.01
+
+ - Predict a YouTube video using a pretrained segmentation model at image size 320:
+ yolo segment predict model=yolov8n-seg.pt source='https://youtu.be/Zgi9g1ksQHc' imgsz=320
+
+ - Val a pretrained detection model at batch-size 1 and image size 640:
+ yolo detect val model=yolov8n.pt data=coco128.yaml batch=1 imgsz=640
+
+ - Export a YOLOv8n classification model to ONNX format at image size 224 by 128 (no TASK required)
+ yolo export model=yolov8n-cls.pt format=onnx imgsz=224,128
+
+ - Run special commands:
+ yolo help
+ yolo checks
+ yolo version
+ yolo settings
+ yolo copy-cfg
+ yolo cfg
+
+ Docs: https://docs.ultralytics.com
+ Community: https://community.ultralytics.com
+ GitHub: https://github.com/ultralytics/ultralytics
+ """
+
+# Settings
+torch.set_printoptions(linewidth=320, precision=4, profile='default')
+np.set_printoptions(linewidth=320, formatter={'float_kind': '{:11.5g}'.format}) # format short g, %precision=5
+cv2.setNumThreads(0) # prevent OpenCV from multithreading (incompatible with PyTorch DataLoader)
+os.environ['NUMEXPR_MAX_THREADS'] = str(NUM_THREADS) # NumExpr max threads
+os.environ['CUBLAS_WORKSPACE_CONFIG'] = ':4096:8' # for deterministic training
+os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2' # suppress verbose TF compiler warnings in Colab
+
+
+class SimpleClass:
+ """
+ Ultralytics SimpleClass is a base class providing helpful string representation, error reporting, and attribute
+ access methods for easier debugging and usage.
+ """
+
+ def __str__(self):
+ """Return a human-readable string representation of the object."""
+ attr = []
+ for a in dir(self):
+ v = getattr(self, a)
+ if not callable(v) and not a.startswith('_'):
+ if isinstance(v, SimpleClass):
+ # Display only the module and class name for subclasses
+ s = f'{a}: {v.__module__}.{v.__class__.__name__} object'
+ else:
+ s = f'{a}: {repr(v)}'
+ attr.append(s)
+ return f'{self.__module__}.{self.__class__.__name__} object with attributes:\n\n' + '\n'.join(attr)
+
+ def __repr__(self):
+ """Return a machine-readable string representation of the object."""
+ return self.__str__()
+
+ def __getattr__(self, attr):
+ """Custom attribute access error message with helpful information."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
+
+
+class IterableSimpleNamespace(SimpleNamespace):
+ """
+ Ultralytics IterableSimpleNamespace is an extension class of SimpleNamespace that adds iterable functionality and
+ enables usage with dict() and for loops.
+ """
+
+ def __iter__(self):
+ """Return an iterator of key-value pairs from the namespace's attributes."""
+ return iter(vars(self).items())
+
+ def __str__(self):
+ """Return a human-readable string representation of the object."""
+ return '\n'.join(f'{k}={v}' for k, v in vars(self).items())
+
+ def __getattr__(self, attr):
+ """Custom attribute access error message with helpful information."""
+ name = self.__class__.__name__
+ raise AttributeError(f"""
+ '{name}' object has no attribute '{attr}'. This may be caused by a modified or out of date ultralytics
+ 'default.yaml' file.\nPlease update your code with 'pip install -U ultralytics' and if necessary replace
+ {DEFAULT_CFG_PATH} with the latest version from
+ https://github.com/ultralytics/ultralytics/blob/main/ultralytics/yolo/cfg/default.yaml
+ """)
+
+ def get(self, key, default=None):
+ """Return the value of the specified key if it exists; otherwise, return the default value."""
+ return getattr(self, key, default)
+
+
+def plt_settings(rcparams=None, backend='Agg'):
+ """
+ Decorator to temporarily set rc parameters and the backend for a plotting function.
+
+ Usage:
+ decorator: @plt_settings({"font.size": 12})
+ context manager: with plt_settings({"font.size": 12}):
+
+ Args:
+ rcparams (dict): Dictionary of rc parameters to set.
+ backend (str, optional): Name of the backend to use. Defaults to 'Agg'.
+
+ Returns:
+ (Callable): Decorated function with temporarily set rc parameters and backend. This decorator can be
+ applied to any function that needs to have specific matplotlib rc parameters and backend for its execution.
+ """
+
+ if rcparams is None:
+ rcparams = {'font.size': 11}
+
+ def decorator(func):
+ """Decorator to apply temporary rc parameters and backend to a function."""
+
+ def wrapper(*args, **kwargs):
+ """Sets rc parameters and backend, calls the original function, and restores the settings."""
+ original_backend = plt.get_backend()
+ plt.switch_backend(backend)
+
+ with plt.rc_context(rcparams):
+ result = func(*args, **kwargs)
+
+ plt.switch_backend(original_backend)
+ return result
+
+ return wrapper
+
+ return decorator
+
+
+def set_logging(name=LOGGING_NAME, verbose=True):
+ """Sets up logging for the given name."""
+ rank = int(os.getenv('RANK', -1)) # rank in world for Multi-GPU trainings
+ level = logging.INFO if verbose and rank in {-1, 0} else logging.ERROR
+ logging.config.dictConfig({
+ 'version': 1,
+ 'disable_existing_loggers': False,
+ 'formatters': {
+ name: {
+ 'format': '%(message)s'}},
+ 'handlers': {
+ name: {
+ 'class': 'logging.StreamHandler',
+ 'formatter': name,
+ 'level': level}},
+ 'loggers': {
+ name: {
+ 'level': level,
+ 'handlers': [name],
+ 'propagate': False}}})
+
+
+def emojis(string=''):
+ """Return platform-dependent emoji-safe version of string."""
+ return string.encode().decode('ascii', 'ignore') if WINDOWS else string
+
+
+class EmojiFilter(logging.Filter):
+ """
+ A custom logging filter class for removing emojis in log messages.
+
+ This filter is particularly useful for ensuring compatibility with Windows terminals
+ that may not support the display of emojis in log messages.
+ """
+
+ def filter(self, record):
+ """Filter logs by emoji unicode characters on windows."""
+ record.msg = emojis(record.msg)
+ return super().filter(record)
+
+
+# Set logger
+set_logging(LOGGING_NAME, verbose=VERBOSE) # run before defining LOGGER
+LOGGER = logging.getLogger(LOGGING_NAME) # define globally (used in train.py, val.py, detect.py, etc.)
+if WINDOWS: # emoji-safe logging
+ LOGGER.addFilter(EmojiFilter())
+
+
+def yaml_save(file='data.yaml', data=None):
+ """
+ Save YAML data to a file.
+
+ Args:
+ file (str, optional): File name. Default is 'data.yaml'.
+ data (dict): Data to save in YAML format.
+
+ Returns:
+ (None): Data is saved to the specified file.
+ """
+ if data is None:
+ data = {}
+ file = Path(file)
+ if not file.parent.exists():
+ # Create parent directories if they don't exist
+ file.parent.mkdir(parents=True, exist_ok=True)
+
+ # Convert Path objects to strings
+ for k, v in data.items():
+ if isinstance(v, Path):
+ data[k] = str(v)
+
+ # Dump data to file in YAML format
+ with open(file, 'w') as f:
+ yaml.safe_dump(data, f, sort_keys=False, allow_unicode=True)
+
+
+def yaml_load(file='data.yaml', append_filename=False):
+ """
+ Load YAML data from a file.
+
+ Args:
+ file (str, optional): File name. Default is 'data.yaml'.
+ append_filename (bool): Add the YAML filename to the YAML dictionary. Default is False.
+
+ Returns:
+ (dict): YAML data and file name.
+ """
+ with open(file, errors='ignore', encoding='utf-8') as f:
+ s = f.read() # string
+
+ # Remove special characters
+ if not s.isprintable():
+ s = re.sub(r'[^\x09\x0A\x0D\x20-\x7E\x85\xA0-\uD7FF\uE000-\uFFFD\U00010000-\U0010ffff]+', '', s)
+
+ # Add YAML filename to dict and return
+ return {**yaml.safe_load(s), 'yaml_file': str(file)} if append_filename else yaml.safe_load(s)
+
+
+def yaml_print(yaml_file: Union[str, Path, dict]) -> None:
+ """
+ Pretty prints a yaml file or a yaml-formatted dictionary.
+
+ Args:
+ yaml_file: The file path of the yaml file or a yaml-formatted dictionary.
+
+ Returns:
+ None
+ """
+ yaml_dict = yaml_load(yaml_file) if isinstance(yaml_file, (str, Path)) else yaml_file
+ dump = yaml.dump(yaml_dict, sort_keys=False, allow_unicode=True)
+ LOGGER.info(f"Printing '{colorstr('bold', 'black', yaml_file)}'\n\n{dump}")
+
+
+# Default configuration
+DEFAULT_CFG_DICT = yaml_load(DEFAULT_CFG_PATH)
+for k, v in DEFAULT_CFG_DICT.items():
+ if isinstance(v, str) and v.lower() == 'none':
+ DEFAULT_CFG_DICT[k] = None
+DEFAULT_CFG_KEYS = DEFAULT_CFG_DICT.keys()
+DEFAULT_CFG = IterableSimpleNamespace(**DEFAULT_CFG_DICT)
+
+
+def is_colab():
+ """
+ Check if the current script is running inside a Google Colab notebook.
+
+ Returns:
+ (bool): True if running inside a Colab notebook, False otherwise.
+ """
+ return 'COLAB_RELEASE_TAG' in os.environ or 'COLAB_BACKEND_VERSION' in os.environ
+
+
+def is_kaggle():
+ """
+ Check if the current script is running inside a Kaggle kernel.
+
+ Returns:
+ (bool): True if running inside a Kaggle kernel, False otherwise.
+ """
+ return os.environ.get('PWD') == '/kaggle/working' and os.environ.get('KAGGLE_URL_BASE') == 'https://www.kaggle.com'
+
+
+def is_jupyter():
+ """
+ Check if the current script is running inside a Jupyter Notebook.
+ Verified on Colab, Jupyterlab, Kaggle, Paperspace.
+
+ Returns:
+ (bool): True if running inside a Jupyter Notebook, False otherwise.
+ """
+ with contextlib.suppress(Exception):
+ from IPython import get_ipython
+ return get_ipython() is not None
+ return False
+
+
+def is_docker() -> bool:
+ """
+ Determine if the script is running inside a Docker container.
+
+ Returns:
+ (bool): True if the script is running inside a Docker container, False otherwise.
+ """
+ file = Path('/proc/self/cgroup')
+ if file.exists():
+ with open(file) as f:
+ return 'docker' in f.read()
+ else:
+ return False
+
+
+def is_online() -> bool:
+ """
+ Check internet connectivity by attempting to connect to a known online host.
+
+ Returns:
+ (bool): True if connection is successful, False otherwise.
+ """
+ import socket
+
+ for host in '1.1.1.1', '8.8.8.8', '223.5.5.5': # Cloudflare, Google, AliDNS:
+ try:
+ test_connection = socket.create_connection(address=(host, 53), timeout=2)
+ except (socket.timeout, socket.gaierror, OSError):
+ continue
+ else:
+ # If the connection was successful, close it to avoid a ResourceWarning
+ test_connection.close()
+ return True
+ return False
+
+
+ONLINE = is_online()
+
+
+def is_pip_package(filepath: str = __name__) -> bool:
+ """
+ Determines if the file at the given filepath is part of a pip package.
+
+ Args:
+ filepath (str): The filepath to check.
+
+ Returns:
+ (bool): True if the file is part of a pip package, False otherwise.
+ """
+ import importlib.util
+
+ # Get the spec for the module
+ spec = importlib.util.find_spec(filepath)
+
+ # Return whether the spec is not None and the origin is not None (indicating it is a package)
+ return spec is not None and spec.origin is not None
+
+
+def is_dir_writeable(dir_path: Union[str, Path]) -> bool:
+ """
+ Check if a directory is writeable.
+
+ Args:
+ dir_path (str | Path): The path to the directory.
+
+ Returns:
+ (bool): True if the directory is writeable, False otherwise.
+ """
+ return os.access(str(dir_path), os.W_OK)
+
+
+def is_pytest_running():
+ """
+ Determines whether pytest is currently running or not.
+
+ Returns:
+ (bool): True if pytest is running, False otherwise.
+ """
+ return ('PYTEST_CURRENT_TEST' in os.environ) or ('pytest' in sys.modules) or ('pytest' in Path(sys.argv[0]).stem)
+
+
+def is_github_actions_ci() -> bool:
+ """
+ Determine if the current environment is a GitHub Actions CI Python runner.
+
+ Returns:
+ (bool): True if the current environment is a GitHub Actions CI Python runner, False otherwise.
+ """
+ return 'GITHUB_ACTIONS' in os.environ and 'RUNNER_OS' in os.environ and 'RUNNER_TOOL_CACHE' in os.environ
+
+
+def is_git_dir():
+ """
+ Determines whether the current file is part of a git repository.
+ If the current file is not part of a git repository, returns None.
+
+ Returns:
+ (bool): True if current file is part of a git repository.
+ """
+ return get_git_dir() is not None
+
+
+def get_git_dir():
+ """
+ Determines whether the current file is part of a git repository and if so, returns the repository root directory.
+ If the current file is not part of a git repository, returns None.
+
+ Returns:
+ (Path | None): Git root directory if found or None if not found.
+ """
+ for d in Path(__file__).parents:
+ if (d / '.git').is_dir():
+ return d
+ return None # no .git dir found
+
+
+def get_git_origin_url():
+ """
+ Retrieves the origin URL of a git repository.
+
+ Returns:
+ (str | None): The origin URL of the git repository.
+ """
+ if is_git_dir():
+ with contextlib.suppress(subprocess.CalledProcessError):
+ origin = subprocess.check_output(['git', 'config', '--get', 'remote.origin.url'])
+ return origin.decode().strip()
+ return None # if not git dir or on error
+
+
+def get_git_branch():
+ """
+ Returns the current git branch name. If not in a git repository, returns None.
+
+ Returns:
+ (str | None): The current git branch name.
+ """
+ if is_git_dir():
+ with contextlib.suppress(subprocess.CalledProcessError):
+ origin = subprocess.check_output(['git', 'rev-parse', '--abbrev-ref', 'HEAD'])
+ return origin.decode().strip()
+ return None # if not git dir or on error
+
+
+def get_default_args(func):
+ """Returns a dictionary of default arguments for a function.
+
+ Args:
+ func (callable): The function to inspect.
+
+ Returns:
+ (dict): A dictionary where each key is a parameter name, and each value is the default value of that parameter.
+ """
+ signature = inspect.signature(func)
+ return {k: v.default for k, v in signature.parameters.items() if v.default is not inspect.Parameter.empty}
+
+
+def get_user_config_dir(sub_dir='Ultralytics'):
+ """
+ Get the user config directory.
+
+ Args:
+ sub_dir (str): The name of the subdirectory to create.
+
+ Returns:
+ (Path): The path to the user config directory.
+ """
+ # Return the appropriate config directory for each operating system
+ if WINDOWS:
+ path = Path.home() / 'AppData' / 'Roaming' / sub_dir
+ elif MACOS: # macOS
+ path = Path.home() / 'Library' / 'Application Support' / sub_dir
+ elif LINUX:
+ path = Path.home() / '.config' / sub_dir
+ else:
+ raise ValueError(f'Unsupported operating system: {platform.system()}')
+
+ # GCP and AWS lambda fix, only /tmp is writeable
+ if not is_dir_writeable(str(path.parent)):
+ path = Path('/tmp') / sub_dir
+ LOGGER.warning(f"WARNING ⚠️ user config directory is not writeable, defaulting to '{path}'.")
+
+ # Create the subdirectory if it does not exist
+ path.mkdir(parents=True, exist_ok=True)
+
+ return path
+
+
+USER_CONFIG_DIR = Path(os.getenv('YOLO_CONFIG_DIR', get_user_config_dir())) # Ultralytics settings dir
+SETTINGS_YAML = USER_CONFIG_DIR / 'settings.yaml'
+
+
+def colorstr(*input):
+ """Colors a string https://en.wikipedia.org/wiki/ANSI_escape_code, i.e. colorstr('blue', 'hello world')."""
+ *args, string = input if len(input) > 1 else ('blue', 'bold', input[0]) # color arguments, string
+ colors = {
+ 'black': '\033[30m', # basic colors
+ 'red': '\033[31m',
+ 'green': '\033[32m',
+ 'yellow': '\033[33m',
+ 'blue': '\033[34m',
+ 'magenta': '\033[35m',
+ 'cyan': '\033[36m',
+ 'white': '\033[37m',
+ 'bright_black': '\033[90m', # bright colors
+ 'bright_red': '\033[91m',
+ 'bright_green': '\033[92m',
+ 'bright_yellow': '\033[93m',
+ 'bright_blue': '\033[94m',
+ 'bright_magenta': '\033[95m',
+ 'bright_cyan': '\033[96m',
+ 'bright_white': '\033[97m',
+ 'end': '\033[0m', # misc
+ 'bold': '\033[1m',
+ 'underline': '\033[4m'}
+ return ''.join(colors[x] for x in args) + f'{string}' + colors['end']
+
+
+class TryExcept(contextlib.ContextDecorator):
+ """YOLOv8 TryExcept class. Usage: @TryExcept() decorator or 'with TryExcept():' context manager."""
+
+ def __init__(self, msg='', verbose=True):
+ """Initialize TryExcept class with optional message and verbosity settings."""
+ self.msg = msg
+ self.verbose = verbose
+
+ def __enter__(self):
+ """Executes when entering TryExcept context, initializes instance."""
+ pass
+
+ def __exit__(self, exc_type, value, traceback):
+ """Defines behavior when exiting a 'with' block, prints error message if necessary."""
+ if self.verbose and value:
+ print(emojis(f"{self.msg}{': ' if self.msg else ''}{value}"))
+ return True
+
+
+def threaded(func):
+ """Multi-threads a target function and returns thread. Usage: @threaded decorator."""
+
+ def wrapper(*args, **kwargs):
+ """Multi-threads a given function and returns the thread."""
+ thread = threading.Thread(target=func, args=args, kwargs=kwargs, daemon=True)
+ thread.start()
+ return thread
+
+ return wrapper
+
+
+def set_sentry():
+ """
+ Initialize the Sentry SDK for error tracking and reporting. Only used if sentry_sdk package is installed and
+ sync=True in settings. Run 'yolo settings' to see and update settings YAML file.
+
+ Conditions required to send errors (ALL conditions must be met or no errors will be reported):
+ - sentry_sdk package is installed
+ - sync=True in YOLO settings
+ - pytest is not running
+ - running in a pip package installation
+ - running in a non-git directory
+ - running with rank -1 or 0
+ - online environment
+ - CLI used to run package (checked with 'yolo' as the name of the main CLI command)
+
+ The function also configures Sentry SDK to ignore KeyboardInterrupt and FileNotFoundError
+ exceptions and to exclude events with 'out of memory' in their exception message.
+
+ Additionally, the function sets custom tags and user information for Sentry events.
+ """
+
+ def before_send(event, hint):
+ """
+ Modify the event before sending it to Sentry based on specific exception types and messages.
+
+ Args:
+ event (dict): The event dictionary containing information about the error.
+ hint (dict): A dictionary containing additional information about the error.
+
+ Returns:
+ dict: The modified event or None if the event should not be sent to Sentry.
+ """
+ if 'exc_info' in hint:
+ exc_type, exc_value, tb = hint['exc_info']
+ if exc_type in (KeyboardInterrupt, FileNotFoundError) \
+ or 'out of memory' in str(exc_value):
+ return None # do not send event
+
+ event['tags'] = {
+ 'sys_argv': sys.argv[0],
+ 'sys_argv_name': Path(sys.argv[0]).name,
+ 'install': 'git' if is_git_dir() else 'pip' if is_pip_package() else 'other',
+ 'os': ENVIRONMENT}
+ return event
+
+ if SETTINGS['sync'] and \
+ RANK in (-1, 0) and \
+ Path(sys.argv[0]).name == 'yolo' and \
+ not TESTS_RUNNING and \
+ ONLINE and \
+ is_pip_package() and \
+ not is_git_dir():
+
+ # If sentry_sdk package is not installed then return and do not use Sentry
+ try:
+ import sentry_sdk # noqa
+ except ImportError:
+ return
+
+ sentry_sdk.init(
+ dsn='https://5ff1556b71594bfea135ff0203a0d290@o4504521589325824.ingest.sentry.io/4504521592406016',
+ debug=False,
+ traces_sample_rate=1.0,
+ release=__version__,
+ environment='production', # 'dev' or 'production'
+ before_send=before_send,
+ ignore_errors=[KeyboardInterrupt, FileNotFoundError])
+ sentry_sdk.set_user({'id': SETTINGS['uuid']}) # SHA-256 anonymized UUID hash
+
+ # Disable all sentry logging
+ for logger in 'sentry_sdk', 'sentry_sdk.errors':
+ logging.getLogger(logger).setLevel(logging.CRITICAL)
+
+
+def get_settings(file=SETTINGS_YAML, version='0.0.3'):
+ """
+ Loads a global Ultralytics settings YAML file or creates one with default values if it does not exist.
+
+ Args:
+ file (Path): Path to the Ultralytics settings YAML file. Defaults to 'settings.yaml' in the USER_CONFIG_DIR.
+ version (str): Settings version. If min settings version not met, new default settings will be saved.
+
+ Returns:
+ (dict): Dictionary of settings key-value pairs.
+ """
+ import hashlib
+
+ from ultralytics.yolo.utils.checks import check_version
+ from ultralytics.yolo.utils.torch_utils import torch_distributed_zero_first
+
+ git_dir = get_git_dir()
+ root = git_dir or Path()
+ datasets_root = (root.parent if git_dir and is_dir_writeable(root.parent) else root).resolve()
+ defaults = {
+ 'datasets_dir': str(datasets_root / 'datasets'), # default datasets directory.
+ 'weights_dir': str(root / 'weights'), # default weights directory.
+ 'runs_dir': str(root / 'runs'), # default runs directory.
+ 'uuid': hashlib.sha256(str(uuid.getnode()).encode()).hexdigest(), # SHA-256 anonymized UUID hash
+ 'sync': True, # sync analytics to help with YOLO development
+ 'api_key': '', # Ultralytics HUB API key (https://hub.ultralytics.com/)
+ 'settings_version': version} # Ultralytics settings version
+
+ with torch_distributed_zero_first(RANK):
+ if not file.exists():
+ yaml_save(file, defaults)
+ settings = yaml_load(file)
+
+ # Check that settings keys and types match defaults
+ correct = \
+ settings \
+ and settings.keys() == defaults.keys() \
+ and all(type(a) == type(b) for a, b in zip(settings.values(), defaults.values())) \
+ and check_version(settings['settings_version'], version)
+ if not correct:
+ LOGGER.warning('WARNING ⚠️ Ultralytics settings reset to defaults. This is normal and may be due to a '
+ 'recent ultralytics package update, but may have overwritten previous settings. '
+ f"\nView and update settings with 'yolo settings' or at '{file}'")
+ settings = defaults # merge **defaults with **settings (prefer **settings)
+ yaml_save(file, settings) # save updated defaults
+
+ return settings
+
+
+def set_settings(kwargs, file=SETTINGS_YAML):
+ """
+ Function that runs on a first-time ultralytics package installation to set up global settings and create necessary
+ directories.
+ """
+ SETTINGS.update(kwargs)
+ yaml_save(file, SETTINGS)
+
+
+def deprecation_warn(arg, new_arg, version=None):
+ """Issue a deprecation warning when a deprecated argument is used, suggesting an updated argument."""
+ if not version:
+ version = float(__version__[:3]) + 0.2 # deprecate after 2nd major release
+ LOGGER.warning(f"WARNING ⚠️ '{arg}' is deprecated and will be removed in 'ultralytics {version}' in the future. "
+ f"Please use '{new_arg}' instead.")
+
+
+def clean_url(url):
+ """Strip auth from URL, i.e. https://url.com/file.txt?auth -> https://url.com/file.txt."""
+ url = str(Path(url)).replace(':/', '://') # Pathlib turns :// -> :/
+ return urllib.parse.unquote(url).split('?')[0] # '%2F' to '/', split https://url.com/file.txt?auth
+
+
+def url2file(url):
+ """Convert URL to filename, i.e. https://url.com/file.txt?auth -> file.txt."""
+ return Path(clean_url(url)).name
+
+
+# Run below code on yolo/utils init ------------------------------------------------------------------------------------
+
+# Check first-install steps
+PREFIX = colorstr('Ultralytics: ')
+SETTINGS = get_settings()
+DATASETS_DIR = Path(SETTINGS['datasets_dir']) # global datasets directory
+ENVIRONMENT = 'Colab' if is_colab() else 'Kaggle' if is_kaggle() else 'Jupyter' if is_jupyter() else \
+ 'Docker' if is_docker() else platform.system()
+TESTS_RUNNING = is_pytest_running() or is_github_actions_ci()
+set_sentry()
+
+# Apply monkey patches if the script is being run from within the parent directory of the script's location
+from .patches import imread, imshow, imwrite
+
+# torch.save = torch_save
+if Path(inspect.stack()[0].filename).parent.parent.as_posix() in inspect.stack()[-1].filename:
+ cv2.imread, cv2.imwrite, cv2.imshow = imread, imwrite, imshow
diff --git a/yolov8/ultralytics/yolo/utils/autobatch.py b/yolov8/ultralytics/yolo/utils/autobatch.py
new file mode 100644
index 0000000000000000000000000000000000000000..0645f81ebfe7ff50776e18f8e8f03ce5f7aa0998
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/autobatch.py
@@ -0,0 +1,90 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Functions for estimating the best YOLO batch size to use a fraction of the available CUDA memory in PyTorch.
+"""
+
+from copy import deepcopy
+
+import numpy as np
+import torch
+
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, colorstr
+from ultralytics.yolo.utils.torch_utils import profile
+
+
+def check_train_batch_size(model, imgsz=640, amp=True):
+ """
+ Check YOLO training batch size using the autobatch() function.
+
+ Args:
+ model (torch.nn.Module): YOLO model to check batch size for.
+ imgsz (int): Image size used for training.
+ amp (bool): If True, use automatic mixed precision (AMP) for training.
+
+ Returns:
+ (int): Optimal batch size computed using the autobatch() function.
+ """
+
+ with torch.cuda.amp.autocast(amp):
+ return autobatch(deepcopy(model).train(), imgsz) # compute optimal batch size
+
+
+def autobatch(model, imgsz=640, fraction=0.67, batch_size=DEFAULT_CFG.batch):
+ """
+ Automatically estimate the best YOLO batch size to use a fraction of the available CUDA memory.
+
+ Args:
+ model (torch.nn.module): YOLO model to compute batch size for.
+ imgsz (int, optional): The image size used as input for the YOLO model. Defaults to 640.
+ fraction (float, optional): The fraction of available CUDA memory to use. Defaults to 0.67.
+ batch_size (int, optional): The default batch size to use if an error is detected. Defaults to 16.
+
+ Returns:
+ (int): The optimal batch size.
+ """
+
+ # Check device
+ prefix = colorstr('AutoBatch: ')
+ LOGGER.info(f'{prefix}Computing optimal batch size for imgsz={imgsz}')
+ device = next(model.parameters()).device # get model device
+ if device.type == 'cpu':
+ LOGGER.info(f'{prefix}CUDA not detected, using default CPU batch-size {batch_size}')
+ return batch_size
+ if torch.backends.cudnn.benchmark:
+ LOGGER.info(f'{prefix} ⚠️ Requires torch.backends.cudnn.benchmark=False, using default batch-size {batch_size}')
+ return batch_size
+
+ # Inspect CUDA memory
+ gb = 1 << 30 # bytes to GiB (1024 ** 3)
+ d = str(device).upper() # 'CUDA:0'
+ properties = torch.cuda.get_device_properties(device) # device properties
+ t = properties.total_memory / gb # GiB total
+ r = torch.cuda.memory_reserved(device) / gb # GiB reserved
+ a = torch.cuda.memory_allocated(device) / gb # GiB allocated
+ f = t - (r + a) # GiB free
+ LOGGER.info(f'{prefix}{d} ({properties.name}) {t:.2f}G total, {r:.2f}G reserved, {a:.2f}G allocated, {f:.2f}G free')
+
+ # Profile batch sizes
+ batch_sizes = [1, 2, 4, 8, 16]
+ try:
+ img = [torch.empty(b, 3, imgsz, imgsz) for b in batch_sizes]
+ results = profile(img, model, n=3, device=device)
+
+ # Fit a solution
+ y = [x[2] for x in results if x] # memory [2]
+ p = np.polyfit(batch_sizes[:len(y)], y, deg=1) # first degree polynomial fit
+ b = int((f * fraction - p[1]) / p[0]) # y intercept (optimal batch size)
+ if None in results: # some sizes failed
+ i = results.index(None) # first fail index
+ if b >= batch_sizes[i]: # y intercept above failure point
+ b = batch_sizes[max(i - 1, 0)] # select prior safe point
+ if b < 1 or b > 1024: # b outside of safe range
+ b = batch_size
+ LOGGER.info(f'{prefix}WARNING ⚠️ CUDA anomaly detected, using default batch-size {batch_size}.')
+
+ fraction = (np.polyval(p, b) + r + a) / t # actual fraction predicted
+ LOGGER.info(f'{prefix}Using batch-size {b} for {d} {t * fraction:.2f}G/{t:.2f}G ({fraction * 100:.0f}%) ✅')
+ return b
+ except Exception as e:
+ LOGGER.warning(f'{prefix}WARNING ⚠️ error detected: {e}, using default batch-size {batch_size}.')
+ return batch_size
diff --git a/yolov8/ultralytics/yolo/utils/benchmarks.py b/yolov8/ultralytics/yolo/utils/benchmarks.py
new file mode 100644
index 0000000000000000000000000000000000000000..f9e8f00e75276ca95631ab18b2de73bf74a3fc04
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/benchmarks.py
@@ -0,0 +1,358 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Benchmark a YOLO model formats for speed and accuracy
+
+Usage:
+ from ultralytics.yolo.utils.benchmarks import ProfileModels, benchmark
+ ProfileModels(['yolov8n.yaml', 'yolov8s.yaml']).profile()
+ run_benchmarks(model='yolov8n.pt', imgsz=160)
+
+Format | `format=argument` | Model
+--- | --- | ---
+PyTorch | - | yolov8n.pt
+TorchScript | `torchscript` | yolov8n.torchscript
+ONNX | `onnx` | yolov8n.onnx
+OpenVINO | `openvino` | yolov8n_openvino_model/
+TensorRT | `engine` | yolov8n.engine
+CoreML | `coreml` | yolov8n.mlmodel
+TensorFlow SavedModel | `saved_model` | yolov8n_saved_model/
+TensorFlow GraphDef | `pb` | yolov8n.pb
+TensorFlow Lite | `tflite` | yolov8n.tflite
+TensorFlow Edge TPU | `edgetpu` | yolov8n_edgetpu.tflite
+TensorFlow.js | `tfjs` | yolov8n_web_model/
+PaddlePaddle | `paddle` | yolov8n_paddle_model/
+NCNN | `ncnn` | yolov8n_ncnn_model/
+"""
+
+import glob
+import platform
+import time
+from pathlib import Path
+
+import numpy as np
+import torch.cuda
+from tqdm import tqdm
+
+from ultralytics import YOLO
+from ultralytics.yolo.cfg import TASK2DATA, TASK2METRIC
+from ultralytics.yolo.engine.exporter import export_formats
+from ultralytics.yolo.utils import LINUX, LOGGER, MACOS, ROOT, SETTINGS
+from ultralytics.yolo.utils.checks import check_requirements, check_yolo
+from ultralytics.yolo.utils.downloads import download
+from ultralytics.yolo.utils.files import file_size
+from ultralytics.yolo.utils.torch_utils import select_device
+
+
+def benchmark(model=Path(SETTINGS['weights_dir']) / 'yolov8n.pt',
+ imgsz=160,
+ half=False,
+ int8=False,
+ device='cpu',
+ hard_fail=False):
+ """
+ Benchmark a YOLO model across different formats for speed and accuracy.
+
+ Args:
+ model (str | Path | optional): Path to the model file or directory. Default is
+ Path(SETTINGS['weights_dir']) / 'yolov8n.pt'.
+ imgsz (int, optional): Image size for the benchmark. Default is 160.
+ half (bool, optional): Use half-precision for the model if True. Default is False.
+ int8 (bool, optional): Use int8-precision for the model if True. Default is False.
+ device (str, optional): Device to run the benchmark on, either 'cpu' or 'cuda'. Default is 'cpu'.
+ hard_fail (bool | float | optional): If True or a float, assert benchmarks pass with given metric.
+ Default is False.
+
+ Returns:
+ df (pandas.DataFrame): A pandas DataFrame with benchmark results for each format, including file size,
+ metric, and inference time.
+ """
+
+ import pandas as pd
+ pd.options.display.max_columns = 10
+ pd.options.display.width = 120
+ device = select_device(device, verbose=False)
+ if isinstance(model, (str, Path)):
+ model = YOLO(model)
+
+ y = []
+ t0 = time.time()
+ for i, (name, format, suffix, cpu, gpu) in export_formats().iterrows(): # index, (name, format, suffix, CPU, GPU)
+ emoji, filename = '❌', None # export defaults
+ try:
+ assert i != 9 or LINUX, 'Edge TPU export only supported on Linux'
+ if i == 10:
+ assert MACOS or LINUX, 'TF.js export only supported on macOS and Linux'
+ if 'cpu' in device.type:
+ assert cpu, 'inference not supported on CPU'
+ if 'cuda' in device.type:
+ assert gpu, 'inference not supported on GPU'
+
+ # Export
+ if format == '-':
+ filename = model.ckpt_path or model.cfg
+ export = model # PyTorch format
+ else:
+ filename = model.export(imgsz=imgsz, format=format, half=half, int8=int8, device=device, verbose=False)
+ export = YOLO(filename, task=model.task)
+ assert suffix in str(filename), 'export failed'
+ emoji = '❎' # indicates export succeeded
+
+ # Predict
+ assert model.task != 'pose' or i != 7, 'GraphDef Pose inference is not supported'
+ assert i not in (9, 10, 12), 'inference not supported' # Edge TPU, TF.js and NCNN are unsupported
+ assert i != 5 or platform.system() == 'Darwin', 'inference only supported on macOS>=10.13' # CoreML
+ if not (ROOT / 'assets/bus.jpg').exists():
+ download(url='https://ultralytics.com/images/bus.jpg', dir=ROOT / 'assets')
+ export.predict(ROOT / 'assets/bus.jpg', imgsz=imgsz, device=device, half=half)
+
+ # Validate
+ data = TASK2DATA[model.task] # task to dataset, i.e. coco8.yaml for task=detect
+ key = TASK2METRIC[model.task] # task to metric, i.e. metrics/mAP50-95(B) for task=detect
+ results = export.val(data=data,
+ batch=1,
+ imgsz=imgsz,
+ plots=False,
+ device=device,
+ half=half,
+ int8=int8,
+ verbose=False)
+ metric, speed = results.results_dict[key], results.speed['inference']
+ y.append([name, '✅', round(file_size(filename), 1), round(metric, 4), round(speed, 2)])
+ except Exception as e:
+ if hard_fail:
+ assert type(e) is AssertionError, f'Benchmark hard_fail for {name}: {e}'
+ LOGGER.warning(f'ERROR ❌️ Benchmark failure for {name}: {e}')
+ y.append([name, emoji, round(file_size(filename), 1), None, None]) # mAP, t_inference
+
+ # Print results
+ check_yolo(device=device) # print system info
+ df = pd.DataFrame(y, columns=['Format', 'Status❔', 'Size (MB)', key, 'Inference time (ms/im)'])
+
+ name = Path(model.ckpt_path).name
+ s = f'\nBenchmarks complete for {name} on {data} at imgsz={imgsz} ({time.time() - t0:.2f}s)\n{df}\n'
+ LOGGER.info(s)
+ with open('benchmarks.log', 'a', errors='ignore', encoding='utf-8') as f:
+ f.write(s)
+
+ if hard_fail and isinstance(hard_fail, float):
+ metrics = df[key].array # values to compare to floor
+ floor = hard_fail # minimum metric floor to pass, i.e. = 0.29 mAP for YOLOv5n
+ assert all(x > floor for x in metrics if pd.notna(x)), f'HARD FAIL: one or more metric(s) < floor {floor}'
+
+ return df
+
+
+class ProfileModels:
+ """
+ ProfileModels class for profiling different models on ONNX and TensorRT.
+
+ This class profiles the performance of different models, provided their paths. The profiling includes parameters such as
+ model speed and FLOPs.
+
+ Attributes:
+ paths (list): Paths of the models to profile.
+ num_timed_runs (int): Number of timed runs for the profiling. Default is 100.
+ num_warmup_runs (int): Number of warmup runs before profiling. Default is 10.
+ min_time (float): Minimum number of seconds to profile for. Default is 60.
+ imgsz (int): Image size used in the models. Default is 640.
+
+ Methods:
+ profile(): Profiles the models and prints the result.
+ """
+
+ def __init__(self,
+ paths: list,
+ num_timed_runs=100,
+ num_warmup_runs=10,
+ min_time=60,
+ imgsz=640,
+ trt=True,
+ device=None):
+ self.paths = paths
+ self.num_timed_runs = num_timed_runs
+ self.num_warmup_runs = num_warmup_runs
+ self.min_time = min_time
+ self.imgsz = imgsz
+ self.trt = trt # run TensorRT profiling
+ self.device = device or torch.device(0 if torch.cuda.is_available() else 'cpu')
+
+ def profile(self):
+ files = self.get_files()
+
+ if not files:
+ print('No matching *.pt or *.onnx files found.')
+ return
+
+ table_rows = []
+ output = []
+ for file in files:
+ engine_file = file.with_suffix('.engine')
+ if file.suffix in ('.pt', '.yaml'):
+ model = YOLO(str(file))
+ model.fuse() # to report correct params and GFLOPs in model.info()
+ model_info = model.info()
+ if self.trt and self.device.type != 'cpu' and not engine_file.is_file():
+ engine_file = model.export(format='engine',
+ half=True,
+ imgsz=self.imgsz,
+ device=self.device,
+ verbose=False)
+ onnx_file = model.export(format='onnx',
+ half=True,
+ imgsz=self.imgsz,
+ simplify=True,
+ device=self.device,
+ verbose=False)
+ elif file.suffix == '.onnx':
+ model_info = self.get_onnx_model_info(file)
+ onnx_file = file
+ else:
+ continue
+
+ t_engine = self.profile_tensorrt_model(str(engine_file))
+ t_onnx = self.profile_onnx_model(str(onnx_file))
+ table_rows.append(self.generate_table_row(file.stem, t_onnx, t_engine, model_info))
+ output.append(self.generate_results_dict(file.stem, t_onnx, t_engine, model_info))
+
+ self.print_table(table_rows)
+ return output
+
+ def get_files(self):
+ files = []
+ for path in self.paths:
+ path = Path(path)
+ if path.is_dir():
+ extensions = ['*.pt', '*.onnx', '*.yaml']
+ files.extend([file for ext in extensions for file in glob.glob(str(path / ext))])
+ elif path.suffix in {'.pt', '.yaml'}: # add non-existing
+ files.append(str(path))
+ else:
+ files.extend(glob.glob(str(path)))
+
+ print(f'Profiling: {sorted(files)}')
+ return [Path(file) for file in sorted(files)]
+
+ def get_onnx_model_info(self, onnx_file: str):
+ # return (num_layers, num_params, num_gradients, num_flops)
+ return 0.0, 0.0, 0.0, 0.0
+
+ def iterative_sigma_clipping(self, data, sigma=2, max_iters=3):
+ data = np.array(data)
+ for _ in range(max_iters):
+ mean, std = np.mean(data), np.std(data)
+ clipped_data = data[(data > mean - sigma * std) & (data < mean + sigma * std)]
+ if len(clipped_data) == len(data):
+ break
+ data = clipped_data
+ return data
+
+ def profile_tensorrt_model(self, engine_file: str):
+ if not self.trt or not Path(engine_file).is_file():
+ return 0.0, 0.0
+
+ # Model and input
+ model = YOLO(engine_file)
+ input_data = np.random.rand(self.imgsz, self.imgsz, 3).astype(np.float32) # must be FP32
+
+ # Warmup runs
+ elapsed = 0.0
+ for _ in range(3):
+ start_time = time.time()
+ for _ in range(self.num_warmup_runs):
+ model(input_data, imgsz=self.imgsz, verbose=False)
+ elapsed = time.time() - start_time
+
+ # Compute number of runs as higher of min_time or num_timed_runs
+ num_runs = max(round(self.min_time / elapsed * self.num_warmup_runs), self.num_timed_runs * 50)
+
+ # Timed runs
+ run_times = []
+ for _ in tqdm(range(num_runs), desc=engine_file):
+ results = model(input_data, imgsz=self.imgsz, verbose=False)
+ run_times.append(results[0].speed['inference']) # Convert to milliseconds
+
+ run_times = self.iterative_sigma_clipping(np.array(run_times), sigma=2, max_iters=3) # sigma clipping
+ return np.mean(run_times), np.std(run_times)
+
+ def profile_onnx_model(self, onnx_file: str):
+ check_requirements('onnxruntime')
+ import onnxruntime as ort
+
+ # Session with either 'TensorrtExecutionProvider', 'CUDAExecutionProvider', 'CPUExecutionProvider'
+ sess_options = ort.SessionOptions()
+ sess_options.graph_optimization_level = ort.GraphOptimizationLevel.ORT_ENABLE_ALL
+ sess_options.intra_op_num_threads = 8 # Limit the number of threads
+ sess = ort.InferenceSession(onnx_file, sess_options, providers=['CPUExecutionProvider'])
+
+ input_tensor = sess.get_inputs()[0]
+ input_type = input_tensor.type
+
+ # Mapping ONNX datatype to numpy datatype
+ if 'float16' in input_type:
+ input_dtype = np.float16
+ elif 'float' in input_type:
+ input_dtype = np.float32
+ elif 'double' in input_type:
+ input_dtype = np.float64
+ elif 'int64' in input_type:
+ input_dtype = np.int64
+ elif 'int32' in input_type:
+ input_dtype = np.int32
+ else:
+ raise ValueError(f'Unsupported ONNX datatype {input_type}')
+
+ input_data = np.random.rand(*input_tensor.shape).astype(input_dtype)
+ input_name = input_tensor.name
+ output_name = sess.get_outputs()[0].name
+
+ # Warmup runs
+ elapsed = 0.0
+ for _ in range(3):
+ start_time = time.time()
+ for _ in range(self.num_warmup_runs):
+ sess.run([output_name], {input_name: input_data})
+ elapsed = time.time() - start_time
+
+ # Compute number of runs as higher of min_time or num_timed_runs
+ num_runs = max(round(self.min_time / elapsed * self.num_warmup_runs), self.num_timed_runs)
+
+ # Timed runs
+ run_times = []
+ for _ in tqdm(range(num_runs), desc=onnx_file):
+ start_time = time.time()
+ sess.run([output_name], {input_name: input_data})
+ run_times.append((time.time() - start_time) * 1000) # Convert to milliseconds
+
+ run_times = self.iterative_sigma_clipping(np.array(run_times), sigma=2, max_iters=5) # sigma clipping
+ return np.mean(run_times), np.std(run_times)
+
+ def generate_table_row(self, model_name, t_onnx, t_engine, model_info):
+ layers, params, gradients, flops = model_info
+ return f'| {model_name:18s} | {self.imgsz} | - | {t_onnx[0]:.2f} ± {t_onnx[1]:.2f} ms | {t_engine[0]:.2f} ± {t_engine[1]:.2f} ms | {params / 1e6:.1f} | {flops:.1f} |'
+
+ def generate_results_dict(self, model_name, t_onnx, t_engine, model_info):
+ layers, params, gradients, flops = model_info
+ return {
+ 'model/name': model_name,
+ 'model/parameters': params,
+ 'model/GFLOPs': round(flops, 3),
+ 'model/speed_ONNX(ms)': round(t_onnx[0], 3),
+ 'model/speed_TensorRT(ms)': round(t_engine[0], 3)}
+
+ def print_table(self, table_rows):
+ gpu = torch.cuda.get_device_name(0) if torch.cuda.is_available() else 'GPU'
+ header = f'| Model | size
(pixels) | mAPval
50-95 | Speed
CPU ONNX
(ms) | Speed
{gpu} TensorRT
(ms) | params
(M) | FLOPs
(B) |'
+ separator = '|-------------|---------------------|--------------------|------------------------------|-----------------------------------|------------------|-----------------|'
+
+ print(f'\n\n{header}')
+ print(separator)
+ for row in table_rows:
+ print(row)
+
+
+if __name__ == '__main__':
+ # Benchmark all export formats
+ benchmark()
+
+ # Profiling models on ONNX and TensorRT
+ ProfileModels(['yolov8n.yaml', 'yolov8s.yaml'])
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/__init__.py b/yolov8/ultralytics/yolo/utils/callbacks/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..8ad4ad6e7b4726d5c8405a217ab2a3cf9cb3440d
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/__init__.py
@@ -0,0 +1,5 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .base import add_integration_callbacks, default_callbacks, get_default_callbacks
+
+__all__ = 'add_integration_callbacks', 'default_callbacks', 'get_default_callbacks'
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/base.py b/yolov8/ultralytics/yolo/utils/callbacks/base.py
new file mode 100644
index 0000000000000000000000000000000000000000..0b1734798ffb760f92c9ea098e996d4637b082ea
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/base.py
@@ -0,0 +1,212 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Base callbacks
+"""
+
+from collections import defaultdict
+from copy import deepcopy
+
+# Trainer callbacks ----------------------------------------------------------------------------------------------------
+
+
+def on_pretrain_routine_start(trainer):
+ """Called before the pretraining routine starts."""
+ pass
+
+
+def on_pretrain_routine_end(trainer):
+ """Called after the pretraining routine ends."""
+ pass
+
+
+def on_train_start(trainer):
+ """Called when the training starts."""
+ pass
+
+
+def on_train_epoch_start(trainer):
+ """Called at the start of each training epoch."""
+ pass
+
+
+def on_train_batch_start(trainer):
+ """Called at the start of each training batch."""
+ pass
+
+
+def optimizer_step(trainer):
+ """Called when the optimizer takes a step."""
+ pass
+
+
+def on_before_zero_grad(trainer):
+ """Called before the gradients are set to zero."""
+ pass
+
+
+def on_train_batch_end(trainer):
+ """Called at the end of each training batch."""
+ pass
+
+
+def on_train_epoch_end(trainer):
+ """Called at the end of each training epoch."""
+ pass
+
+
+def on_fit_epoch_end(trainer):
+ """Called at the end of each fit epoch (train + val)."""
+ pass
+
+
+def on_model_save(trainer):
+ """Called when the model is saved."""
+ pass
+
+
+def on_train_end(trainer):
+ """Called when the training ends."""
+ pass
+
+
+def on_params_update(trainer):
+ """Called when the model parameters are updated."""
+ pass
+
+
+def teardown(trainer):
+ """Called during the teardown of the training process."""
+ pass
+
+
+# Validator callbacks --------------------------------------------------------------------------------------------------
+
+
+def on_val_start(validator):
+ """Called when the validation starts."""
+ pass
+
+
+def on_val_batch_start(validator):
+ """Called at the start of each validation batch."""
+ pass
+
+
+def on_val_batch_end(validator):
+ """Called at the end of each validation batch."""
+ pass
+
+
+def on_val_end(validator):
+ """Called when the validation ends."""
+ pass
+
+
+# Predictor callbacks --------------------------------------------------------------------------------------------------
+
+
+def on_predict_start(predictor):
+ """Called when the prediction starts."""
+ pass
+
+
+def on_predict_batch_start(predictor):
+ """Called at the start of each prediction batch."""
+ pass
+
+
+def on_predict_batch_end(predictor):
+ """Called at the end of each prediction batch."""
+ pass
+
+
+def on_predict_postprocess_end(predictor):
+ """Called after the post-processing of the prediction ends."""
+ pass
+
+
+def on_predict_end(predictor):
+ """Called when the prediction ends."""
+ pass
+
+
+# Exporter callbacks ---------------------------------------------------------------------------------------------------
+
+
+def on_export_start(exporter):
+ """Called when the model export starts."""
+ pass
+
+
+def on_export_end(exporter):
+ """Called when the model export ends."""
+ pass
+
+
+default_callbacks = {
+ # Run in trainer
+ 'on_pretrain_routine_start': [on_pretrain_routine_start],
+ 'on_pretrain_routine_end': [on_pretrain_routine_end],
+ 'on_train_start': [on_train_start],
+ 'on_train_epoch_start': [on_train_epoch_start],
+ 'on_train_batch_start': [on_train_batch_start],
+ 'optimizer_step': [optimizer_step],
+ 'on_before_zero_grad': [on_before_zero_grad],
+ 'on_train_batch_end': [on_train_batch_end],
+ 'on_train_epoch_end': [on_train_epoch_end],
+ 'on_fit_epoch_end': [on_fit_epoch_end], # fit = train + val
+ 'on_model_save': [on_model_save],
+ 'on_train_end': [on_train_end],
+ 'on_params_update': [on_params_update],
+ 'teardown': [teardown],
+
+ # Run in validator
+ 'on_val_start': [on_val_start],
+ 'on_val_batch_start': [on_val_batch_start],
+ 'on_val_batch_end': [on_val_batch_end],
+ 'on_val_end': [on_val_end],
+
+ # Run in predictor
+ 'on_predict_start': [on_predict_start],
+ 'on_predict_batch_start': [on_predict_batch_start],
+ 'on_predict_postprocess_end': [on_predict_postprocess_end],
+ 'on_predict_batch_end': [on_predict_batch_end],
+ 'on_predict_end': [on_predict_end],
+
+ # Run in exporter
+ 'on_export_start': [on_export_start],
+ 'on_export_end': [on_export_end]}
+
+
+def get_default_callbacks():
+ """
+ Return a copy of the default_callbacks dictionary with lists as default values.
+
+ Returns:
+ (defaultdict): A defaultdict with keys from default_callbacks and empty lists as default values.
+ """
+ return defaultdict(list, deepcopy(default_callbacks))
+
+
+def add_integration_callbacks(instance):
+ """
+ Add integration callbacks from various sources to the instance's callbacks.
+
+ Args:
+ instance (Trainer, Predictor, Validator, Exporter): An object with a 'callbacks' attribute that is a dictionary
+ of callback lists.
+ """
+ from .clearml import callbacks as clearml_cb
+ from .comet import callbacks as comet_cb
+ from .dvc import callbacks as dvc_cb
+ from .hub import callbacks as hub_cb
+ from .mlflow import callbacks as mlflow_cb
+ from .neptune import callbacks as neptune_cb
+ from .raytune import callbacks as tune_cb
+ from .tensorboard import callbacks as tensorboard_cb
+ from .wb import callbacks as wb_cb
+
+ for x in clearml_cb, comet_cb, hub_cb, mlflow_cb, neptune_cb, tune_cb, tensorboard_cb, wb_cb, dvc_cb:
+ for k, v in x.items():
+ if v not in instance.callbacks[k]: # prevent duplicate callbacks addition
+ instance.callbacks[k].append(v) # callback[name].append(func)
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/clearml.py b/yolov8/ultralytics/yolo/utils/callbacks/clearml.py
new file mode 100644
index 0000000000000000000000000000000000000000..2cfdd73e0e58d913fca0c6caf599fbb27060c732
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/clearml.py
@@ -0,0 +1,143 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import re
+
+import matplotlib.image as mpimg
+import matplotlib.pyplot as plt
+
+from ultralytics.yolo.utils import LOGGER, TESTS_RUNNING
+from ultralytics.yolo.utils.torch_utils import model_info_for_loggers
+
+try:
+ import clearml
+ from clearml import Task
+ from clearml.binding.frameworks.pytorch_bind import PatchPyTorchModelIO
+ from clearml.binding.matplotlib_bind import PatchedMatplotlib
+
+ assert hasattr(clearml, '__version__') # verify package is not directory
+ assert not TESTS_RUNNING # do not log pytest
+except (ImportError, AssertionError):
+ clearml = None
+
+
+def _log_debug_samples(files, title='Debug Samples') -> None:
+ """
+ Log files (images) as debug samples in the ClearML task.
+
+ Args:
+ files (list): A list of file paths in PosixPath format.
+ title (str): A title that groups together images with the same values.
+ """
+ task = Task.current_task()
+ if task:
+ for f in files:
+ if f.exists():
+ it = re.search(r'_batch(\d+)', f.name)
+ iteration = int(it.groups()[0]) if it else 0
+ task.get_logger().report_image(title=title,
+ series=f.name.replace(it.group(), ''),
+ local_path=str(f),
+ iteration=iteration)
+
+
+def _log_plot(title, plot_path) -> None:
+ """
+ Log an image as a plot in the plot section of ClearML.
+
+ Args:
+ title (str): The title of the plot.
+ plot_path (str): The path to the saved image file.
+ """
+ img = mpimg.imread(plot_path)
+ fig = plt.figure()
+ ax = fig.add_axes([0, 0, 1, 1], frameon=False, aspect='auto', xticks=[], yticks=[]) # no ticks
+ ax.imshow(img)
+
+ Task.current_task().get_logger().report_matplotlib_figure(title=title,
+ series='',
+ figure=fig,
+ report_interactive=False)
+
+
+def on_pretrain_routine_start(trainer):
+ """Runs at start of pretraining routine; initializes and connects/ logs task to ClearML."""
+ try:
+ task = Task.current_task()
+ if task:
+ # Make sure the automatic pytorch and matplotlib bindings are disabled!
+ # We are logging these plots and model files manually in the integration
+ PatchPyTorchModelIO.update_current_task(None)
+ PatchedMatplotlib.update_current_task(None)
+ else:
+ task = Task.init(project_name=trainer.args.project or 'YOLOv8',
+ task_name=trainer.args.name,
+ tags=['YOLOv8'],
+ output_uri=True,
+ reuse_last_task_id=False,
+ auto_connect_frameworks={
+ 'pytorch': False,
+ 'matplotlib': False})
+ LOGGER.warning('ClearML Initialized a new task. If you want to run remotely, '
+ 'please add clearml-init and connect your arguments before initializing YOLO.')
+ task.connect(vars(trainer.args), name='General')
+ except Exception as e:
+ LOGGER.warning(f'WARNING ⚠️ ClearML installed but not initialized correctly, not logging this run. {e}')
+
+
+def on_train_epoch_end(trainer):
+ task = Task.current_task()
+
+ if task:
+ """Logs debug samples for the first epoch of YOLO training."""
+ if trainer.epoch == 1:
+ _log_debug_samples(sorted(trainer.save_dir.glob('train_batch*.jpg')), 'Mosaic')
+ """Report the current training progress."""
+ for k, v in trainer.validator.metrics.results_dict.items():
+ task.get_logger().report_scalar('train', k, v, iteration=trainer.epoch)
+
+
+def on_fit_epoch_end(trainer):
+ """Reports model information to logger at the end of an epoch."""
+ task = Task.current_task()
+ if task:
+ # You should have access to the validation bboxes under jdict
+ task.get_logger().report_scalar(title='Epoch Time',
+ series='Epoch Time',
+ value=trainer.epoch_time,
+ iteration=trainer.epoch)
+ if trainer.epoch == 0:
+ for k, v in model_info_for_loggers(trainer).items():
+ task.get_logger().report_single_value(k, v)
+
+
+def on_val_end(validator):
+ """Logs validation results including labels and predictions."""
+ if Task.current_task():
+ # Log val_labels and val_pred
+ _log_debug_samples(sorted(validator.save_dir.glob('val*.jpg')), 'Validation')
+
+
+def on_train_end(trainer):
+ """Logs final model and its name on training completion."""
+ task = Task.current_task()
+ if task:
+ # Log final results, CM matrix + PR plots
+ files = [
+ 'results.png', 'confusion_matrix.png', 'confusion_matrix_normalized.png',
+ *(f'{x}_curve.png' for x in ('F1', 'PR', 'P', 'R'))]
+ files = [(trainer.save_dir / f) for f in files if (trainer.save_dir / f).exists()] # filter
+ for f in files:
+ _log_plot(title=f.stem, plot_path=f)
+ # Report final metrics
+ for k, v in trainer.validator.metrics.results_dict.items():
+ task.get_logger().report_single_value(k, v)
+ # Log the final model
+ task.update_output_model(model_path=str(trainer.best), model_name=trainer.args.name, auto_delete_file=False)
+
+
+callbacks = {
+ 'on_pretrain_routine_start': on_pretrain_routine_start,
+ 'on_train_epoch_end': on_train_epoch_end,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_val_end': on_val_end,
+ 'on_train_end': on_train_end} if clearml else {}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/comet.py b/yolov8/ultralytics/yolo/utils/callbacks/comet.py
new file mode 100644
index 0000000000000000000000000000000000000000..94aeb8f64c8abc39564d1cac25c3c6eb55ad3dce
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/comet.py
@@ -0,0 +1,368 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+from pathlib import Path
+
+from ultralytics.yolo.utils import LOGGER, RANK, TESTS_RUNNING, ops
+from ultralytics.yolo.utils.torch_utils import model_info_for_loggers
+
+try:
+ import comet_ml
+
+ assert not TESTS_RUNNING # do not log pytest
+ assert hasattr(comet_ml, '__version__') # verify package is not directory
+except (ImportError, AssertionError):
+ comet_ml = None
+
+# Ensures certain logging functions only run for supported tasks
+COMET_SUPPORTED_TASKS = ['detect']
+
+# Names of plots created by YOLOv8 that are logged to Comet
+EVALUATION_PLOT_NAMES = 'F1_curve', 'P_curve', 'R_curve', 'PR_curve', 'confusion_matrix'
+LABEL_PLOT_NAMES = 'labels', 'labels_correlogram'
+
+_comet_image_prediction_count = 0
+
+
+def _get_comet_mode():
+ return os.getenv('COMET_MODE', 'online')
+
+
+def _get_comet_model_name():
+ return os.getenv('COMET_MODEL_NAME', 'YOLOv8')
+
+
+def _get_eval_batch_logging_interval():
+ return int(os.getenv('COMET_EVAL_BATCH_LOGGING_INTERVAL', 1))
+
+
+def _get_max_image_predictions_to_log():
+ return int(os.getenv('COMET_MAX_IMAGE_PREDICTIONS', 100))
+
+
+def _scale_confidence_score(score):
+ scale = float(os.getenv('COMET_MAX_CONFIDENCE_SCORE', 100.0))
+ return score * scale
+
+
+def _should_log_confusion_matrix():
+ return os.getenv('COMET_EVAL_LOG_CONFUSION_MATRIX', 'false').lower() == 'true'
+
+
+def _should_log_image_predictions():
+ return os.getenv('COMET_EVAL_LOG_IMAGE_PREDICTIONS', 'true').lower() == 'true'
+
+
+def _get_experiment_type(mode, project_name):
+ """Return an experiment based on mode and project name."""
+ if mode == 'offline':
+ return comet_ml.OfflineExperiment(project_name=project_name)
+
+ return comet_ml.Experiment(project_name=project_name)
+
+
+def _create_experiment(args):
+ """Ensures that the experiment object is only created in a single process during distributed training."""
+ if RANK not in (-1, 0):
+ return
+ try:
+ comet_mode = _get_comet_mode()
+ _project_name = os.getenv('COMET_PROJECT_NAME', args.project)
+ experiment = _get_experiment_type(comet_mode, _project_name)
+ experiment.log_parameters(vars(args))
+ experiment.log_others({
+ 'eval_batch_logging_interval': _get_eval_batch_logging_interval(),
+ 'log_confusion_matrix_on_eval': _should_log_confusion_matrix(),
+ 'log_image_predictions': _should_log_image_predictions(),
+ 'max_image_predictions': _get_max_image_predictions_to_log(), })
+ experiment.log_other('Created from', 'yolov8')
+
+ except Exception as e:
+ LOGGER.warning(f'WARNING ⚠️ Comet installed but not initialized correctly, not logging this run. {e}')
+
+
+def _fetch_trainer_metadata(trainer):
+ """Returns metadata for YOLO training including epoch and asset saving status."""
+ curr_epoch = trainer.epoch + 1
+
+ train_num_steps_per_epoch = len(trainer.train_loader.dataset) // trainer.batch_size
+ curr_step = curr_epoch * train_num_steps_per_epoch
+ final_epoch = curr_epoch == trainer.epochs
+
+ save = trainer.args.save
+ save_period = trainer.args.save_period
+ save_interval = curr_epoch % save_period == 0
+ save_assets = save and save_period > 0 and save_interval and not final_epoch
+
+ return dict(
+ curr_epoch=curr_epoch,
+ curr_step=curr_step,
+ save_assets=save_assets,
+ final_epoch=final_epoch,
+ )
+
+
+def _scale_bounding_box_to_original_image_shape(box, resized_image_shape, original_image_shape, ratio_pad):
+ """YOLOv8 resizes images during training and the label values
+ are normalized based on this resized shape. This function rescales the
+ bounding box labels to the original image shape.
+ """
+
+ resized_image_height, resized_image_width = resized_image_shape
+
+ # Convert normalized xywh format predictions to xyxy in resized scale format
+ box = ops.xywhn2xyxy(box, h=resized_image_height, w=resized_image_width)
+ # Scale box predictions from resized image scale back to original image scale
+ box = ops.scale_boxes(resized_image_shape, box, original_image_shape, ratio_pad)
+ # Convert bounding box format from xyxy to xywh for Comet logging
+ box = ops.xyxy2xywh(box)
+ # Adjust xy center to correspond top-left corner
+ box[:2] -= box[2:] / 2
+ box = box.tolist()
+
+ return box
+
+
+def _format_ground_truth_annotations_for_detection(img_idx, image_path, batch, class_name_map=None):
+ """Format ground truth annotations for detection."""
+ indices = batch['batch_idx'] == img_idx
+ bboxes = batch['bboxes'][indices]
+ if len(bboxes) == 0:
+ LOGGER.debug(f'COMET WARNING: Image: {image_path} has no bounding boxes labels')
+ return None
+
+ cls_labels = batch['cls'][indices].squeeze(1).tolist()
+ if class_name_map:
+ cls_labels = [str(class_name_map[label]) for label in cls_labels]
+
+ original_image_shape = batch['ori_shape'][img_idx]
+ resized_image_shape = batch['resized_shape'][img_idx]
+ ratio_pad = batch['ratio_pad'][img_idx]
+
+ data = []
+ for box, label in zip(bboxes, cls_labels):
+ box = _scale_bounding_box_to_original_image_shape(box, resized_image_shape, original_image_shape, ratio_pad)
+ data.append({
+ 'boxes': [box],
+ 'label': f'gt_{label}',
+ 'score': _scale_confidence_score(1.0), })
+
+ return {'name': 'ground_truth', 'data': data}
+
+
+def _format_prediction_annotations_for_detection(image_path, metadata, class_label_map=None):
+ """Format YOLO predictions for object detection visualization."""
+ stem = image_path.stem
+ image_id = int(stem) if stem.isnumeric() else stem
+
+ predictions = metadata.get(image_id)
+ if not predictions:
+ LOGGER.debug(f'COMET WARNING: Image: {image_path} has no bounding boxes predictions')
+ return None
+
+ data = []
+ for prediction in predictions:
+ boxes = prediction['bbox']
+ score = _scale_confidence_score(prediction['score'])
+ cls_label = prediction['category_id']
+ if class_label_map:
+ cls_label = str(class_label_map[cls_label])
+
+ data.append({'boxes': [boxes], 'label': cls_label, 'score': score})
+
+ return {'name': 'prediction', 'data': data}
+
+
+def _fetch_annotations(img_idx, image_path, batch, prediction_metadata_map, class_label_map):
+ """Join the ground truth and prediction annotations if they exist."""
+ ground_truth_annotations = _format_ground_truth_annotations_for_detection(img_idx, image_path, batch,
+ class_label_map)
+ prediction_annotations = _format_prediction_annotations_for_detection(image_path, prediction_metadata_map,
+ class_label_map)
+
+ annotations = [
+ annotation for annotation in [ground_truth_annotations, prediction_annotations] if annotation is not None]
+ return [annotations] if annotations else None
+
+
+def _create_prediction_metadata_map(model_predictions):
+ """Create metadata map for model predictions by groupings them based on image ID."""
+ pred_metadata_map = {}
+ for prediction in model_predictions:
+ pred_metadata_map.setdefault(prediction['image_id'], [])
+ pred_metadata_map[prediction['image_id']].append(prediction)
+
+ return pred_metadata_map
+
+
+def _log_confusion_matrix(experiment, trainer, curr_step, curr_epoch):
+ """Log the confusion matrix to Comet experiment."""
+ conf_mat = trainer.validator.confusion_matrix.matrix
+ names = list(trainer.data['names'].values()) + ['background']
+ experiment.log_confusion_matrix(
+ matrix=conf_mat,
+ labels=names,
+ max_categories=len(names),
+ epoch=curr_epoch,
+ step=curr_step,
+ )
+
+
+def _log_images(experiment, image_paths, curr_step, annotations=None):
+ """Logs images to the experiment with optional annotations."""
+ if annotations:
+ for image_path, annotation in zip(image_paths, annotations):
+ experiment.log_image(image_path, name=image_path.stem, step=curr_step, annotations=annotation)
+
+ else:
+ for image_path in image_paths:
+ experiment.log_image(image_path, name=image_path.stem, step=curr_step)
+
+
+def _log_image_predictions(experiment, validator, curr_step):
+ """Logs predicted boxes for a single image during training."""
+ global _comet_image_prediction_count
+
+ task = validator.args.task
+ if task not in COMET_SUPPORTED_TASKS:
+ return
+
+ jdict = validator.jdict
+ if not jdict:
+ return
+
+ predictions_metadata_map = _create_prediction_metadata_map(jdict)
+ dataloader = validator.dataloader
+ class_label_map = validator.names
+
+ batch_logging_interval = _get_eval_batch_logging_interval()
+ max_image_predictions = _get_max_image_predictions_to_log()
+
+ for batch_idx, batch in enumerate(dataloader):
+ if (batch_idx + 1) % batch_logging_interval != 0:
+ continue
+
+ image_paths = batch['im_file']
+ for img_idx, image_path in enumerate(image_paths):
+ if _comet_image_prediction_count >= max_image_predictions:
+ return
+
+ image_path = Path(image_path)
+ annotations = _fetch_annotations(
+ img_idx,
+ image_path,
+ batch,
+ predictions_metadata_map,
+ class_label_map,
+ )
+ _log_images(
+ experiment,
+ [image_path],
+ curr_step,
+ annotations=annotations,
+ )
+ _comet_image_prediction_count += 1
+
+
+def _log_plots(experiment, trainer):
+ """Logs evaluation plots and label plots for the experiment."""
+ plot_filenames = [trainer.save_dir / f'{plots}.png' for plots in EVALUATION_PLOT_NAMES]
+ _log_images(experiment, plot_filenames, None)
+
+ label_plot_filenames = [trainer.save_dir / f'{labels}.jpg' for labels in LABEL_PLOT_NAMES]
+ _log_images(experiment, label_plot_filenames, None)
+
+
+def _log_model(experiment, trainer):
+ """Log the best-trained model to Comet.ml."""
+ model_name = _get_comet_model_name()
+ experiment.log_model(
+ model_name,
+ file_or_folder=str(trainer.best),
+ file_name='best.pt',
+ overwrite=True,
+ )
+
+
+def on_pretrain_routine_start(trainer):
+ """Creates or resumes a CometML experiment at the start of a YOLO pre-training routine."""
+ experiment = comet_ml.get_global_experiment()
+ is_alive = getattr(experiment, 'alive', False)
+ if not experiment or not is_alive:
+ _create_experiment(trainer.args)
+
+
+def on_train_epoch_end(trainer):
+ """Log metrics and save batch images at the end of training epochs."""
+ experiment = comet_ml.get_global_experiment()
+ if not experiment:
+ return
+
+ metadata = _fetch_trainer_metadata(trainer)
+ curr_epoch = metadata['curr_epoch']
+ curr_step = metadata['curr_step']
+
+ experiment.log_metrics(
+ trainer.label_loss_items(trainer.tloss, prefix='train'),
+ step=curr_step,
+ epoch=curr_epoch,
+ )
+
+ if curr_epoch == 1:
+ _log_images(experiment, trainer.save_dir.glob('train_batch*.jpg'), curr_step)
+
+
+def on_fit_epoch_end(trainer):
+ """Logs model assets at the end of each epoch."""
+ experiment = comet_ml.get_global_experiment()
+ if not experiment:
+ return
+
+ metadata = _fetch_trainer_metadata(trainer)
+ curr_epoch = metadata['curr_epoch']
+ curr_step = metadata['curr_step']
+ save_assets = metadata['save_assets']
+
+ experiment.log_metrics(trainer.metrics, step=curr_step, epoch=curr_epoch)
+ experiment.log_metrics(trainer.lr, step=curr_step, epoch=curr_epoch)
+ if curr_epoch == 1:
+ experiment.log_metrics(model_info_for_loggers(trainer), step=curr_step, epoch=curr_epoch)
+
+ if not save_assets:
+ return
+
+ _log_model(experiment, trainer)
+ if _should_log_confusion_matrix():
+ _log_confusion_matrix(experiment, trainer, curr_step, curr_epoch)
+ if _should_log_image_predictions():
+ _log_image_predictions(experiment, trainer.validator, curr_step)
+
+
+def on_train_end(trainer):
+ """Perform operations at the end of training."""
+ experiment = comet_ml.get_global_experiment()
+ if not experiment:
+ return
+
+ metadata = _fetch_trainer_metadata(trainer)
+ curr_epoch = metadata['curr_epoch']
+ curr_step = metadata['curr_step']
+ plots = trainer.args.plots
+
+ _log_model(experiment, trainer)
+ if plots:
+ _log_plots(experiment, trainer)
+
+ _log_confusion_matrix(experiment, trainer, curr_step, curr_epoch)
+ _log_image_predictions(experiment, trainer.validator, curr_step)
+ experiment.end()
+
+ global _comet_image_prediction_count
+ _comet_image_prediction_count = 0
+
+
+callbacks = {
+ 'on_pretrain_routine_start': on_pretrain_routine_start,
+ 'on_train_epoch_end': on_train_epoch_end,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_train_end': on_train_end} if comet_ml else {}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/dvc.py b/yolov8/ultralytics/yolo/utils/callbacks/dvc.py
new file mode 100644
index 0000000000000000000000000000000000000000..138100c8d64331cdfea8bdcc64c4451d131bf930
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/dvc.py
@@ -0,0 +1,136 @@
+# Ultralytics YOLO 🚀, GPL-3.0 license
+import os
+
+import pkg_resources as pkg
+
+from ultralytics.yolo.utils import LOGGER, TESTS_RUNNING
+from ultralytics.yolo.utils.torch_utils import model_info_for_loggers
+
+try:
+ from importlib.metadata import version
+
+ import dvclive
+
+ assert not TESTS_RUNNING # do not log pytest
+
+ ver = version('dvclive')
+ if pkg.parse_version(ver) < pkg.parse_version('2.11.0'):
+ LOGGER.debug(f'DVCLive is detected but version {ver} is incompatible (>=2.11 required).')
+ dvclive = None # noqa: F811
+except (ImportError, AssertionError, TypeError):
+ dvclive = None
+
+# DVCLive logger instance
+live = None
+_processed_plots = {}
+
+# `on_fit_epoch_end` is called on final validation (probably need to be fixed)
+# for now this is the way we distinguish final evaluation of the best model vs
+# last epoch validation
+_training_epoch = False
+
+
+def _logger_disabled():
+ return os.getenv('ULTRALYTICS_DVC_DISABLED', 'false').lower() == 'true'
+
+
+def _log_images(image_path, prefix=''):
+ if live:
+ live.log_image(os.path.join(prefix, image_path.name), image_path)
+
+
+def _log_plots(plots, prefix=''):
+ for name, params in plots.items():
+ timestamp = params['timestamp']
+ if _processed_plots.get(name) != timestamp:
+ _log_images(name, prefix)
+ _processed_plots[name] = timestamp
+
+
+def _log_confusion_matrix(validator):
+ targets = []
+ preds = []
+ matrix = validator.confusion_matrix.matrix
+ names = list(validator.names.values())
+ if validator.confusion_matrix.task == 'detect':
+ names += ['background']
+
+ for ti, pred in enumerate(matrix.T.astype(int)):
+ for pi, num in enumerate(pred):
+ targets.extend([names[ti]] * num)
+ preds.extend([names[pi]] * num)
+
+ live.log_sklearn_plot('confusion_matrix', targets, preds, name='cf.json', normalized=True)
+
+
+def on_pretrain_routine_start(trainer):
+ try:
+ global live
+ if not _logger_disabled():
+ live = dvclive.Live(save_dvc_exp=True, cache_images=True)
+ LOGGER.info(
+ 'DVCLive is detected and auto logging is enabled (can be disabled with `ULTRALYTICS_DVC_DISABLED=true`).'
+ )
+ else:
+ LOGGER.debug('DVCLive is detected and auto logging is disabled via `ULTRALYTICS_DVC_DISABLED`.')
+ live = None
+ except Exception as e:
+ LOGGER.warning(f'WARNING ⚠️ DVCLive installed but not initialized correctly, not logging this run. {e}')
+
+
+def on_pretrain_routine_end(trainer):
+ _log_plots(trainer.plots, 'train')
+
+
+def on_train_start(trainer):
+ if live:
+ live.log_params(trainer.args)
+
+
+def on_train_epoch_start(trainer):
+ global _training_epoch
+ _training_epoch = True
+
+
+def on_fit_epoch_end(trainer):
+ global _training_epoch
+ if live and _training_epoch:
+ all_metrics = {**trainer.label_loss_items(trainer.tloss, prefix='train'), **trainer.metrics, **trainer.lr}
+ for metric, value in all_metrics.items():
+ live.log_metric(metric, value)
+
+ if trainer.epoch == 0:
+ for metric, value in model_info_for_loggers(trainer).items():
+ live.log_metric(metric, value, plot=False)
+
+ _log_plots(trainer.plots, 'train')
+ _log_plots(trainer.validator.plots, 'val')
+
+ live.next_step()
+ _training_epoch = False
+
+
+def on_train_end(trainer):
+ if live:
+ # At the end log the best metrics. It runs validator on the best model internally.
+ all_metrics = {**trainer.label_loss_items(trainer.tloss, prefix='train'), **trainer.metrics, **trainer.lr}
+ for metric, value in all_metrics.items():
+ live.log_metric(metric, value, plot=False)
+
+ _log_plots(trainer.plots, 'eval')
+ _log_plots(trainer.validator.plots, 'eval')
+ _log_confusion_matrix(trainer.validator)
+
+ if trainer.best.exists():
+ live.log_artifact(trainer.best, copy=True)
+
+ live.end()
+
+
+callbacks = {
+ 'on_pretrain_routine_start': on_pretrain_routine_start,
+ 'on_pretrain_routine_end': on_pretrain_routine_end,
+ 'on_train_start': on_train_start,
+ 'on_train_epoch_start': on_train_epoch_start,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_train_end': on_train_end} if dvclive else {}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/hub.py b/yolov8/ultralytics/yolo/utils/callbacks/hub.py
new file mode 100644
index 0000000000000000000000000000000000000000..e3b34272ea725951e1d40a33f8fd8ff6b0e9315a
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/hub.py
@@ -0,0 +1,87 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import json
+from time import time
+
+from ultralytics.hub.utils import PREFIX, events
+from ultralytics.yolo.utils import LOGGER
+from ultralytics.yolo.utils.torch_utils import model_info_for_loggers
+
+
+def on_pretrain_routine_end(trainer):
+ """Logs info before starting timer for upload rate limit."""
+ session = getattr(trainer, 'hub_session', None)
+ if session:
+ # Start timer for upload rate limit
+ LOGGER.info(f'{PREFIX}View model at https://hub.ultralytics.com/models/{session.model_id} 🚀')
+ session.timers = {'metrics': time(), 'ckpt': time()} # start timer on session.rate_limit
+
+
+def on_fit_epoch_end(trainer):
+ """Uploads training progress metrics at the end of each epoch."""
+ session = getattr(trainer, 'hub_session', None)
+ if session:
+ # Upload metrics after val end
+ all_plots = {**trainer.label_loss_items(trainer.tloss, prefix='train'), **trainer.metrics}
+ if trainer.epoch == 0:
+ all_plots = {**all_plots, **model_info_for_loggers(trainer)}
+ session.metrics_queue[trainer.epoch] = json.dumps(all_plots)
+ if time() - session.timers['metrics'] > session.rate_limits['metrics']:
+ session.upload_metrics()
+ session.timers['metrics'] = time() # reset timer
+ session.metrics_queue = {} # reset queue
+
+
+def on_model_save(trainer):
+ """Saves checkpoints to Ultralytics HUB with rate limiting."""
+ session = getattr(trainer, 'hub_session', None)
+ if session:
+ # Upload checkpoints with rate limiting
+ is_best = trainer.best_fitness == trainer.fitness
+ if time() - session.timers['ckpt'] > session.rate_limits['ckpt']:
+ LOGGER.info(f'{PREFIX}Uploading checkpoint https://hub.ultralytics.com/models/{session.model_id}')
+ session.upload_model(trainer.epoch, trainer.last, is_best)
+ session.timers['ckpt'] = time() # reset timer
+
+
+def on_train_end(trainer):
+ """Upload final model and metrics to Ultralytics HUB at the end of training."""
+ session = getattr(trainer, 'hub_session', None)
+ if session:
+ # Upload final model and metrics with exponential standoff
+ LOGGER.info(f'{PREFIX}Syncing final model...')
+ session.upload_model(trainer.epoch, trainer.best, map=trainer.metrics.get('metrics/mAP50-95(B)', 0), final=True)
+ session.alive = False # stop heartbeats
+ LOGGER.info(f'{PREFIX}Done ✅\n'
+ f'{PREFIX}View model at https://hub.ultralytics.com/models/{session.model_id} 🚀')
+
+
+def on_train_start(trainer):
+ """Run events on train start."""
+ events(trainer.args)
+
+
+def on_val_start(validator):
+ """Runs events on validation start."""
+ events(validator.args)
+
+
+def on_predict_start(predictor):
+ """Run events on predict start."""
+ events(predictor.args)
+
+
+def on_export_start(exporter):
+ """Run events on export start."""
+ events(exporter.args)
+
+
+callbacks = {
+ 'on_pretrain_routine_end': on_pretrain_routine_end,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_model_save': on_model_save,
+ 'on_train_end': on_train_end,
+ 'on_train_start': on_train_start,
+ 'on_val_start': on_val_start,
+ 'on_predict_start': on_predict_start,
+ 'on_export_start': on_export_start}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/mlflow.py b/yolov8/ultralytics/yolo/utils/callbacks/mlflow.py
new file mode 100644
index 0000000000000000000000000000000000000000..e61ca3db0bdf939abebf9fd2661a604e6d023325
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/mlflow.py
@@ -0,0 +1,71 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+import re
+from pathlib import Path
+
+from ultralytics.yolo.utils import LOGGER, TESTS_RUNNING, colorstr
+
+try:
+ import mlflow
+
+ assert not TESTS_RUNNING # do not log pytest
+ assert hasattr(mlflow, '__version__') # verify package is not directory
+except (ImportError, AssertionError):
+ mlflow = None
+
+
+def on_pretrain_routine_end(trainer):
+ """Logs training parameters to MLflow."""
+ global mlflow, run, run_id, experiment_name
+
+ if os.environ.get('MLFLOW_TRACKING_URI') is None:
+ mlflow = None
+
+ if mlflow:
+ mlflow_location = os.environ['MLFLOW_TRACKING_URI'] # "http://192.168.xxx.xxx:5000"
+ mlflow.set_tracking_uri(mlflow_location)
+
+ experiment_name = os.environ.get('MLFLOW_EXPERIMENT') or trainer.args.project or '/Shared/YOLOv8'
+ run_name = os.environ.get('MLFLOW_RUN') or trainer.args.name
+ experiment = mlflow.get_experiment_by_name(experiment_name)
+ if experiment is None:
+ mlflow.create_experiment(experiment_name)
+ mlflow.set_experiment(experiment_name)
+
+ prefix = colorstr('MLFlow: ')
+ try:
+ run, active_run = mlflow, mlflow.active_run()
+ if not active_run:
+ active_run = mlflow.start_run(experiment_id=experiment.experiment_id, run_name=run_name)
+ run_id = active_run.info.run_id
+ LOGGER.info(f'{prefix}Using run_id({run_id}) at {mlflow_location}')
+ run.log_params(vars(trainer.model.args))
+ except Exception as err:
+ LOGGER.error(f'{prefix}Failing init - {repr(err)}')
+ LOGGER.warning(f'{prefix}Continuing without Mlflow')
+
+
+def on_fit_epoch_end(trainer):
+ """Logs training metrics to Mlflow."""
+ if mlflow:
+ metrics_dict = {f"{re.sub('[()]', '', k)}": float(v) for k, v in trainer.metrics.items()}
+ run.log_metrics(metrics=metrics_dict, step=trainer.epoch)
+
+
+def on_train_end(trainer):
+ """Called at end of train loop to log model artifact info."""
+ if mlflow:
+ root_dir = Path(__file__).resolve().parents[3]
+ run.log_artifact(trainer.last)
+ run.log_artifact(trainer.best)
+ run.pyfunc.log_model(artifact_path=experiment_name,
+ code_path=[str(root_dir)],
+ artifacts={'model_path': str(trainer.save_dir)},
+ python_model=run.pyfunc.PythonModel())
+
+
+callbacks = {
+ 'on_pretrain_routine_end': on_pretrain_routine_end,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_train_end': on_train_end} if mlflow else {}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/neptune.py b/yolov8/ultralytics/yolo/utils/callbacks/neptune.py
new file mode 100644
index 0000000000000000000000000000000000000000..be6434124283b352db8ca040ee85ba892f5264b1
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/neptune.py
@@ -0,0 +1,103 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import matplotlib.image as mpimg
+import matplotlib.pyplot as plt
+
+from ultralytics.yolo.utils import LOGGER, TESTS_RUNNING
+from ultralytics.yolo.utils.torch_utils import model_info_for_loggers
+
+try:
+ import neptune
+ from neptune.types import File
+
+ assert not TESTS_RUNNING # do not log pytest
+ assert hasattr(neptune, '__version__')
+except (ImportError, AssertionError):
+ neptune = None
+
+run = None # NeptuneAI experiment logger instance
+
+
+def _log_scalars(scalars, step=0):
+ """Log scalars to the NeptuneAI experiment logger."""
+ if run:
+ for k, v in scalars.items():
+ run[k].append(value=v, step=step)
+
+
+def _log_images(imgs_dict, group=''):
+ """Log scalars to the NeptuneAI experiment logger."""
+ if run:
+ for k, v in imgs_dict.items():
+ run[f'{group}/{k}'].upload(File(v))
+
+
+def _log_plot(title, plot_path):
+ """Log plots to the NeptuneAI experiment logger."""
+ """
+ Log image as plot in the plot section of NeptuneAI
+
+ arguments:
+ title (str) Title of the plot
+ plot_path (PosixPath or str) Path to the saved image file
+ """
+ img = mpimg.imread(plot_path)
+ fig = plt.figure()
+ ax = fig.add_axes([0, 0, 1, 1], frameon=False, aspect='auto', xticks=[], yticks=[]) # no ticks
+ ax.imshow(img)
+ run[f'Plots/{title}'].upload(fig)
+
+
+def on_pretrain_routine_start(trainer):
+ """Callback function called before the training routine starts."""
+ try:
+ global run
+ run = neptune.init_run(project=trainer.args.project or 'YOLOv8', name=trainer.args.name, tags=['YOLOv8'])
+ run['Configuration/Hyperparameters'] = {k: '' if v is None else v for k, v in vars(trainer.args).items()}
+ except Exception as e:
+ LOGGER.warning(f'WARNING ⚠️ NeptuneAI installed but not initialized correctly, not logging this run. {e}')
+
+
+def on_train_epoch_end(trainer):
+ """Callback function called at end of each training epoch."""
+ _log_scalars(trainer.label_loss_items(trainer.tloss, prefix='train'), trainer.epoch + 1)
+ _log_scalars(trainer.lr, trainer.epoch + 1)
+ if trainer.epoch == 1:
+ _log_images({f.stem: str(f) for f in trainer.save_dir.glob('train_batch*.jpg')}, 'Mosaic')
+
+
+def on_fit_epoch_end(trainer):
+ """Callback function called at end of each fit (train+val) epoch."""
+ if run and trainer.epoch == 0:
+ run['Configuration/Model'] = model_info_for_loggers(trainer)
+ _log_scalars(trainer.metrics, trainer.epoch + 1)
+
+
+def on_val_end(validator):
+ """Callback function called at end of each validation."""
+ if run:
+ # Log val_labels and val_pred
+ _log_images({f.stem: str(f) for f in validator.save_dir.glob('val*.jpg')}, 'Validation')
+
+
+def on_train_end(trainer):
+ """Callback function called at end of training."""
+ if run:
+ # Log final results, CM matrix + PR plots
+ files = [
+ 'results.png', 'confusion_matrix.png', 'confusion_matrix_normalized.png',
+ *(f'{x}_curve.png' for x in ('F1', 'PR', 'P', 'R'))]
+ files = [(trainer.save_dir / f) for f in files if (trainer.save_dir / f).exists()] # filter
+ for f in files:
+ _log_plot(title=f.stem, plot_path=f)
+ # Log the final model
+ run[f'weights/{trainer.args.name or trainer.args.task}/{str(trainer.best.name)}'].upload(File(str(
+ trainer.best)))
+
+
+callbacks = {
+ 'on_pretrain_routine_start': on_pretrain_routine_start,
+ 'on_train_epoch_end': on_train_epoch_end,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_val_end': on_val_end,
+ 'on_train_end': on_train_end} if neptune else {}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/raytune.py b/yolov8/ultralytics/yolo/utils/callbacks/raytune.py
new file mode 100644
index 0000000000000000000000000000000000000000..1f532252a552e6813f8a4cdb835f85b8071daff0
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/raytune.py
@@ -0,0 +1,20 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+try:
+ import ray
+ from ray import tune
+ from ray.air import session
+except (ImportError, AssertionError):
+ tune = None
+
+
+def on_fit_epoch_end(trainer):
+ """Sends training metrics to Ray Tune at end of each epoch."""
+ if ray.tune.is_session_enabled():
+ metrics = trainer.metrics
+ metrics['epoch'] = trainer.epoch
+ session.report(metrics)
+
+
+callbacks = {
+ 'on_fit_epoch_end': on_fit_epoch_end, } if tune else {}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/tensorboard.py b/yolov8/ultralytics/yolo/utils/callbacks/tensorboard.py
new file mode 100644
index 0000000000000000000000000000000000000000..a436b9ce90993c8d8ff993719c1662d6e481ba75
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/tensorboard.py
@@ -0,0 +1,47 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from ultralytics.yolo.utils import LOGGER, TESTS_RUNNING, colorstr
+
+try:
+ from torch.utils.tensorboard import SummaryWriter
+
+ assert not TESTS_RUNNING # do not log pytest
+except (ImportError, AssertionError):
+ SummaryWriter = None
+
+writer = None # TensorBoard SummaryWriter instance
+
+
+def _log_scalars(scalars, step=0):
+ """Logs scalar values to TensorBoard."""
+ if writer:
+ for k, v in scalars.items():
+ writer.add_scalar(k, v, step)
+
+
+def on_pretrain_routine_start(trainer):
+ """Initialize TensorBoard logging with SummaryWriter."""
+ if SummaryWriter:
+ try:
+ global writer
+ writer = SummaryWriter(str(trainer.save_dir))
+ prefix = colorstr('TensorBoard: ')
+ LOGGER.info(f"{prefix}Start with 'tensorboard --logdir {trainer.save_dir}', view at http://localhost:6006/")
+ except Exception as e:
+ LOGGER.warning(f'WARNING ⚠️ TensorBoard not initialized correctly, not logging this run. {e}')
+
+
+def on_batch_end(trainer):
+ """Logs scalar statistics at the end of a training batch."""
+ _log_scalars(trainer.label_loss_items(trainer.tloss, prefix='train'), trainer.epoch + 1)
+
+
+def on_fit_epoch_end(trainer):
+ """Logs epoch metrics at end of training epoch."""
+ _log_scalars(trainer.metrics, trainer.epoch + 1)
+
+
+callbacks = {
+ 'on_pretrain_routine_start': on_pretrain_routine_start,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_batch_end': on_batch_end}
diff --git a/yolov8/ultralytics/yolo/utils/callbacks/wb.py b/yolov8/ultralytics/yolo/utils/callbacks/wb.py
new file mode 100644
index 0000000000000000000000000000000000000000..4b4c29b77d6c193c29f01224fdd102366afd22f1
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/callbacks/wb.py
@@ -0,0 +1,60 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+from ultralytics.yolo.utils import TESTS_RUNNING
+from ultralytics.yolo.utils.torch_utils import model_info_for_loggers
+
+try:
+ import wandb as wb
+
+ assert hasattr(wb, '__version__')
+ assert not TESTS_RUNNING # do not log pytest
+except (ImportError, AssertionError):
+ wb = None
+
+_processed_plots = {}
+
+
+def _log_plots(plots, step):
+ for name, params in plots.items():
+ timestamp = params['timestamp']
+ if _processed_plots.get(name, None) != timestamp:
+ wb.run.log({name.stem: wb.Image(str(name))}, step=step)
+ _processed_plots[name] = timestamp
+
+
+def on_pretrain_routine_start(trainer):
+ """Initiate and start project if module is present."""
+ wb.run or wb.init(project=trainer.args.project or 'YOLOv8', name=trainer.args.name, config=vars(trainer.args))
+
+
+def on_fit_epoch_end(trainer):
+ """Logs training metrics and model information at the end of an epoch."""
+ wb.run.log(trainer.metrics, step=trainer.epoch + 1)
+ _log_plots(trainer.plots, step=trainer.epoch + 1)
+ _log_plots(trainer.validator.plots, step=trainer.epoch + 1)
+ if trainer.epoch == 0:
+ wb.run.log(model_info_for_loggers(trainer), step=trainer.epoch + 1)
+
+
+def on_train_epoch_end(trainer):
+ """Log metrics and save images at the end of each training epoch."""
+ wb.run.log(trainer.label_loss_items(trainer.tloss, prefix='train'), step=trainer.epoch + 1)
+ wb.run.log(trainer.lr, step=trainer.epoch + 1)
+ if trainer.epoch == 1:
+ _log_plots(trainer.plots, step=trainer.epoch + 1)
+
+
+def on_train_end(trainer):
+ """Save the best model as an artifact at end of training."""
+ _log_plots(trainer.validator.plots, step=trainer.epoch + 1)
+ _log_plots(trainer.plots, step=trainer.epoch + 1)
+ art = wb.Artifact(type='model', name=f'run_{wb.run.id}_model')
+ if trainer.best.exists():
+ art.add_file(trainer.best)
+ wb.run.log_artifact(art, aliases=['best'])
+
+
+callbacks = {
+ 'on_pretrain_routine_start': on_pretrain_routine_start,
+ 'on_train_epoch_end': on_train_epoch_end,
+ 'on_fit_epoch_end': on_fit_epoch_end,
+ 'on_train_end': on_train_end} if wb else {}
diff --git a/yolov8/ultralytics/yolo/utils/checks.py b/yolov8/ultralytics/yolo/utils/checks.py
new file mode 100644
index 0000000000000000000000000000000000000000..0ea860c10db25ba1af8b9f63491c1e7273fe4b1b
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/checks.py
@@ -0,0 +1,430 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+import contextlib
+import glob
+import inspect
+import math
+import os
+import platform
+import re
+import shutil
+import subprocess
+import time
+from pathlib import Path
+from typing import Optional
+
+import cv2
+import numpy as np
+import pkg_resources as pkg
+import psutil
+import requests
+import torch
+from matplotlib import font_manager
+
+from ultralytics.yolo.utils import (AUTOINSTALL, LOGGER, ONLINE, ROOT, USER_CONFIG_DIR, TryExcept, clean_url, colorstr,
+ downloads, emojis, is_colab, is_docker, is_jupyter, is_kaggle, is_online,
+ is_pip_package, url2file)
+
+
+def is_ascii(s) -> bool:
+ """
+ Check if a string is composed of only ASCII characters.
+
+ Args:
+ s (str): String to be checked.
+
+ Returns:
+ bool: True if the string is composed only of ASCII characters, False otherwise.
+ """
+ # Convert list, tuple, None, etc. to string
+ s = str(s)
+
+ # Check if the string is composed of only ASCII characters
+ return all(ord(c) < 128 for c in s)
+
+
+def check_imgsz(imgsz, stride=32, min_dim=1, max_dim=2, floor=0):
+ """
+ Verify image size is a multiple of the given stride in each dimension. If the image size is not a multiple of the
+ stride, update it to the nearest multiple of the stride that is greater than or equal to the given floor value.
+
+ Args:
+ imgsz (int | cList[int]): Image size.
+ stride (int): Stride value.
+ min_dim (int): Minimum number of dimensions.
+ floor (int): Minimum allowed value for image size.
+
+ Returns:
+ (List[int]): Updated image size.
+ """
+ # Convert stride to integer if it is a tensor
+ stride = int(stride.max() if isinstance(stride, torch.Tensor) else stride)
+
+ # Convert image size to list if it is an integer
+ if isinstance(imgsz, int):
+ imgsz = [imgsz]
+ elif isinstance(imgsz, (list, tuple)):
+ imgsz = list(imgsz)
+ else:
+ raise TypeError(f"'imgsz={imgsz}' is of invalid type {type(imgsz).__name__}. "
+ f"Valid imgsz types are int i.e. 'imgsz=640' or list i.e. 'imgsz=[640,640]'")
+
+ # Apply max_dim
+ if len(imgsz) > max_dim:
+ msg = "'train' and 'val' imgsz must be an integer, while 'predict' and 'export' imgsz may be a [h, w] list " \
+ "or an integer, i.e. 'yolo export imgsz=640,480' or 'yolo export imgsz=640'"
+ if max_dim != 1:
+ raise ValueError(f'imgsz={imgsz} is not a valid image size. {msg}')
+ LOGGER.warning(f"WARNING ⚠️ updating to 'imgsz={max(imgsz)}'. {msg}")
+ imgsz = [max(imgsz)]
+ # Make image size a multiple of the stride
+ sz = [max(math.ceil(x / stride) * stride, floor) for x in imgsz]
+
+ # Print warning message if image size was updated
+ if sz != imgsz:
+ LOGGER.warning(f'WARNING ⚠️ imgsz={imgsz} must be multiple of max stride {stride}, updating to {sz}')
+
+ # Add missing dimensions if necessary
+ sz = [sz[0], sz[0]] if min_dim == 2 and len(sz) == 1 else sz[0] if min_dim == 1 and len(sz) == 1 else sz
+
+ return sz
+
+
+def check_version(current: str = '0.0.0',
+ minimum: str = '0.0.0',
+ name: str = 'version ',
+ pinned: bool = False,
+ hard: bool = False,
+ verbose: bool = False) -> bool:
+ """
+ Check current version against the required minimum version.
+
+ Args:
+ current (str): Current version.
+ minimum (str): Required minimum version.
+ name (str): Name to be used in warning message.
+ pinned (bool): If True, versions must match exactly. If False, minimum version must be satisfied.
+ hard (bool): If True, raise an AssertionError if the minimum version is not met.
+ verbose (bool): If True, print warning message if minimum version is not met.
+
+ Returns:
+ (bool): True if minimum version is met, False otherwise.
+ """
+ current, minimum = (pkg.parse_version(x) for x in (current, minimum))
+ result = (current == minimum) if pinned else (current >= minimum) # bool
+ warning_message = f'WARNING ⚠️ {name}{minimum} is required by YOLOv8, but {name}{current} is currently installed'
+ if hard:
+ assert result, emojis(warning_message) # assert min requirements met
+ if verbose and not result:
+ LOGGER.warning(warning_message)
+ return result
+
+
+def check_latest_pypi_version(package_name='ultralytics'):
+ """
+ Returns the latest version of a PyPI package without downloading or installing it.
+
+ Parameters:
+ package_name (str): The name of the package to find the latest version for.
+
+ Returns:
+ (str): The latest version of the package.
+ """
+ with contextlib.suppress(Exception):
+ requests.packages.urllib3.disable_warnings() # Disable the InsecureRequestWarning
+ response = requests.get(f'https://pypi.org/pypi/{package_name}/json', timeout=3)
+ if response.status_code == 200:
+ return response.json()['info']['version']
+ return None
+
+
+def check_pip_update_available():
+ """
+ Checks if a new version of the ultralytics package is available on PyPI.
+
+ Returns:
+ (bool): True if an update is available, False otherwise.
+ """
+ if ONLINE and is_pip_package():
+ with contextlib.suppress(Exception):
+ from ultralytics import __version__
+ latest = check_latest_pypi_version()
+ if pkg.parse_version(__version__) < pkg.parse_version(latest): # update is available
+ LOGGER.info(f'New https://pypi.org/project/ultralytics/{latest} available 😃 '
+ f"Update with 'pip install -U ultralytics'")
+ return True
+ return False
+
+
+def check_font(font='Arial.ttf'):
+ """
+ Find font locally or download to user's configuration directory if it does not already exist.
+
+ Args:
+ font (str): Path or name of font.
+
+ Returns:
+ file (Path): Resolved font file path.
+ """
+ name = Path(font).name
+
+ # Check USER_CONFIG_DIR
+ file = USER_CONFIG_DIR / name
+ if file.exists():
+ return file
+
+ # Check system fonts
+ matches = [s for s in font_manager.findSystemFonts() if font in s]
+ if any(matches):
+ return matches[0]
+
+ # Download to USER_CONFIG_DIR if missing
+ url = f'https://ultralytics.com/assets/{name}'
+ if downloads.is_url(url):
+ downloads.safe_download(url=url, file=file)
+ return file
+
+
+def check_python(minimum: str = '3.7.0') -> bool:
+ """
+ Check current python version against the required minimum version.
+
+ Args:
+ minimum (str): Required minimum version of python.
+
+ Returns:
+ None
+ """
+ return check_version(platform.python_version(), minimum, name='Python ', hard=True)
+
+
+@TryExcept()
+def check_requirements(requirements=ROOT.parent / 'requirements.txt', exclude=(), install=True, cmds=''):
+ """
+ Check if installed dependencies meet YOLOv8 requirements and attempt to auto-update if needed.
+
+ Args:
+ requirements (Union[Path, str, List[str]]): Path to a requirements.txt file, a single package requirement as a
+ string, or a list of package requirements as strings.
+ exclude (Tuple[str]): Tuple of package names to exclude from checking.
+ install (bool): If True, attempt to auto-update packages that don't meet requirements.
+ cmds (str): Additional commands to pass to the pip install command when auto-updating.
+ """
+ prefix = colorstr('red', 'bold', 'requirements:')
+ check_python() # check python version
+ file = None
+ if isinstance(requirements, Path): # requirements.txt file
+ file = requirements.resolve()
+ assert file.exists(), f'{prefix} {file} not found, check failed.'
+ with file.open() as f:
+ requirements = [f'{x.name}{x.specifier}' for x in pkg.parse_requirements(f) if x.name not in exclude]
+ elif isinstance(requirements, str):
+ requirements = [requirements]
+
+ s = '' # console string
+ n = 0 # number of packages updates
+ for r in requirements:
+ rmin = r.split('/')[-1].replace('.git', '') # replace git+https://org/repo.git -> 'repo'
+ try:
+ pkg.require(rmin)
+ except (pkg.VersionConflict, pkg.DistributionNotFound): # exception if requirements not met
+ try: # attempt to import (slower but more accurate)
+ import importlib
+ importlib.import_module(next(pkg.parse_requirements(rmin)).name)
+ except ImportError:
+ s += f'"{r}" '
+ n += 1
+
+ if s:
+ if install and AUTOINSTALL: # check environment variable
+ pkgs = file or requirements # missing packages
+ LOGGER.info(f"{prefix} Ultralytics requirement{'s' * (n > 1)} {pkgs} not found, attempting AutoUpdate...")
+ try:
+ t = time.time()
+ assert is_online(), 'AutoUpdate skipped (offline)'
+ LOGGER.info(subprocess.check_output(f'pip install --no-cache {s} {cmds}', shell=True).decode())
+ dt = time.time() - t
+ LOGGER.info(
+ f"{prefix} AutoUpdate success ✅ {dt:.1f}s, installed {n} package{'s' * (n > 1)}: {pkgs}\n"
+ f"{prefix} ⚠️ {colorstr('bold', 'Restart runtime or rerun command for updates to take effect')}\n")
+ except Exception as e:
+ LOGGER.warning(f'{prefix} ❌ {e}')
+ return False
+ else:
+ return False
+
+ return True
+
+
+def check_suffix(file='yolov8n.pt', suffix='.pt', msg=''):
+ """Check file(s) for acceptable suffix."""
+ if file and suffix:
+ if isinstance(suffix, str):
+ suffix = (suffix, )
+ for f in file if isinstance(file, (list, tuple)) else [file]:
+ s = Path(f).suffix.lower().strip() # file suffix
+ if len(s):
+ assert s in suffix, f'{msg}{f} acceptable suffix is {suffix}, not {s}'
+
+
+def check_yolov5u_filename(file: str, verbose: bool = True):
+ """Replace legacy YOLOv5 filenames with updated YOLOv5u filenames."""
+ if ('yolov3' in file or 'yolov5' in file) and 'u' not in file:
+ original_file = file
+ file = re.sub(r'(.*yolov5([nsmlx]))\.pt', '\\1u.pt', file) # i.e. yolov5n.pt -> yolov5nu.pt
+ file = re.sub(r'(.*yolov5([nsmlx])6)\.pt', '\\1u.pt', file) # i.e. yolov5n6.pt -> yolov5n6u.pt
+ file = re.sub(r'(.*yolov3(|-tiny|-spp))\.pt', '\\1u.pt', file) # i.e. yolov3-spp.pt -> yolov3-sppu.pt
+ if file != original_file and verbose:
+ LOGGER.info(f"PRO TIP 💡 Replace 'model={original_file}' with new 'model={file}'.\nYOLOv5 'u' models are "
+ f'trained with https://github.com/ultralytics/ultralytics and feature improved performance vs '
+ f'standard YOLOv5 models trained with https://github.com/ultralytics/yolov5.\n')
+ return file
+
+
+def check_file(file, suffix='', download=True, hard=True):
+ """Search/download file (if necessary) and return path."""
+ check_suffix(file, suffix) # optional
+ file = str(file).strip() # convert to string and strip spaces
+ file = check_yolov5u_filename(file) # yolov5n -> yolov5nu
+ if not file or ('://' not in file and Path(file).exists()): # exists ('://' check required in Windows Python<3.10)
+ return file
+ elif download and file.lower().startswith(('https://', 'http://', 'rtsp://', 'rtmp://')): # download
+ url = file # warning: Pathlib turns :// -> :/
+ file = url2file(file) # '%2F' to '/', split https://url.com/file.txt?auth
+ if Path(file).exists():
+ LOGGER.info(f'Found {clean_url(url)} locally at {file}') # file already exists
+ else:
+ downloads.safe_download(url=url, file=file, unzip=False)
+ return file
+ else: # search
+ files = []
+ for d in 'models', 'datasets', 'tracker/cfg', 'yolo/cfg': # search directories
+ files.extend(glob.glob(str(ROOT / d / '**' / file), recursive=True)) # find file
+ if not files and hard:
+ raise FileNotFoundError(f"'{file}' does not exist")
+ elif len(files) > 1 and hard:
+ raise FileNotFoundError(f"Multiple files match '{file}', specify exact path: {files}")
+ return files[0] if len(files) else [] # return file
+
+
+def check_yaml(file, suffix=('.yaml', '.yml'), hard=True):
+ """Search/download YAML file (if necessary) and return path, checking suffix."""
+ return check_file(file, suffix, hard=hard)
+
+
+def check_imshow(warn=False):
+ """Check if environment supports image displays."""
+ try:
+ assert not any((is_colab(), is_kaggle(), is_docker()))
+ cv2.imshow('test', np.zeros((1, 1, 3)))
+ cv2.waitKey(1)
+ cv2.destroyAllWindows()
+ cv2.waitKey(1)
+ return True
+ except Exception as e:
+ if warn:
+ LOGGER.warning(f'WARNING ⚠️ Environment does not support cv2.imshow() or PIL Image.show()\n{e}')
+ return False
+
+
+def check_yolo(verbose=True, device=''):
+ """Return a human-readable YOLO software and hardware summary."""
+ from ultralytics.yolo.utils.torch_utils import select_device
+
+ if is_jupyter():
+ if check_requirements('wandb', install=False):
+ os.system('pip uninstall -y wandb') # uninstall wandb: unwanted account creation prompt with infinite hang
+ if is_colab():
+ shutil.rmtree('sample_data', ignore_errors=True) # remove colab /sample_data directory
+
+ if verbose:
+ # System info
+ gib = 1 << 30 # bytes per GiB
+ ram = psutil.virtual_memory().total
+ total, used, free = shutil.disk_usage('/')
+ s = f'({os.cpu_count()} CPUs, {ram / gib:.1f} GB RAM, {(total - free) / gib:.1f}/{total / gib:.1f} GB disk)'
+ with contextlib.suppress(Exception): # clear display if ipython is installed
+ from IPython import display
+ display.clear_output()
+ else:
+ s = ''
+
+ select_device(device=device, newline=False)
+ LOGGER.info(f'Setup complete ✅ {s}')
+
+
+def check_amp(model):
+ """
+ This function checks the PyTorch Automatic Mixed Precision (AMP) functionality of a YOLOv8 model.
+ If the checks fail, it means there are anomalies with AMP on the system that may cause NaN losses or zero-mAP
+ results, so AMP will be disabled during training.
+
+ Args:
+ model (nn.Module): A YOLOv8 model instance.
+
+ Returns:
+ (bool): Returns True if the AMP functionality works correctly with YOLOv8 model, else False.
+
+ Raises:
+ AssertionError: If the AMP checks fail, indicating anomalies with the AMP functionality on the system.
+ """
+ device = next(model.parameters()).device # get model device
+ if device.type in ('cpu', 'mps'):
+ return False # AMP only used on CUDA devices
+
+ def amp_allclose(m, im):
+ """All close FP32 vs AMP results."""
+ a = m(im, device=device, verbose=False)[0].boxes.data # FP32 inference
+ with torch.cuda.amp.autocast(True):
+ b = m(im, device=device, verbose=False)[0].boxes.data # AMP inference
+ del m
+ return a.shape == b.shape and torch.allclose(a, b.float(), atol=0.5) # close to 0.5 absolute tolerance
+
+ f = ROOT / 'assets/bus.jpg' # image to check
+ im = f if f.exists() else 'https://ultralytics.com/images/bus.jpg' if ONLINE else np.ones((640, 640, 3))
+ prefix = colorstr('AMP: ')
+ LOGGER.info(f'{prefix}running Automatic Mixed Precision (AMP) checks with YOLOv8n...')
+ warning_msg = "Setting 'amp=True'. If you experience zero-mAP or NaN losses you can disable AMP with amp=False."
+ try:
+ from ultralytics import YOLO
+ assert amp_allclose(YOLO('yolov8n.pt'), im)
+ LOGGER.info(f'{prefix}checks passed ✅')
+ except ConnectionError:
+ LOGGER.warning(f'{prefix}checks skipped ⚠️, offline and unable to download YOLOv8n. {warning_msg}')
+ except (AttributeError, ModuleNotFoundError):
+ LOGGER.warning(
+ f'{prefix}checks skipped ⚠️. Unable to load YOLOv8n due to possible Ultralytics package modifications. {warning_msg}'
+ )
+ except AssertionError:
+ LOGGER.warning(f'{prefix}checks failed ❌. Anomalies were detected with AMP on your system that may lead to '
+ f'NaN losses or zero-mAP results, so AMP will be disabled during training.')
+ return False
+ return True
+
+
+def git_describe(path=ROOT): # path must be a directory
+ # Return human-readable git description, i.e. v5.0-5-g3e25f1e https://git-scm.com/docs/git-describe
+ try:
+ assert (Path(path) / '.git').is_dir()
+ return subprocess.check_output(f'git -C {path} describe --tags --long --always', shell=True).decode()[:-1]
+ except AssertionError:
+ return ''
+
+
+def print_args(args: Optional[dict] = None, show_file=True, show_func=False):
+ """Print function arguments (optional args dict)."""
+
+ def strip_auth(v):
+ """Clean longer Ultralytics HUB URLs by stripping potential authentication information."""
+ return clean_url(v) if (isinstance(v, str) and v.startswith('http') and len(v) > 100) else v
+
+ x = inspect.currentframe().f_back # previous frame
+ file, _, func, _, _ = inspect.getframeinfo(x)
+ if args is None: # get args automatically
+ args, _, _, frm = inspect.getargvalues(x)
+ args = {k: v for k, v in frm.items() if k in args}
+ try:
+ file = Path(file).resolve().relative_to(ROOT).with_suffix('')
+ except ValueError:
+ file = Path(file).stem
+ s = (f'{file}: ' if show_file else '') + (f'{func}: ' if show_func else '')
+ LOGGER.info(colorstr(s) + ', '.join(f'{k}={strip_auth(v)}' for k, v in args.items()))
diff --git a/yolov8/ultralytics/yolo/utils/dist.py b/yolov8/ultralytics/yolo/utils/dist.py
new file mode 100644
index 0000000000000000000000000000000000000000..6de029f5c96ea237d8b9e4fc5f8e1d605f506d35
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/dist.py
@@ -0,0 +1,67 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+import re
+import shutil
+import socket
+import sys
+import tempfile
+from pathlib import Path
+
+from . import USER_CONFIG_DIR
+from .torch_utils import TORCH_1_9
+
+
+def find_free_network_port() -> int:
+ """Finds a free port on localhost.
+
+ It is useful in single-node training when we don't want to connect to a real main node but have to set the
+ `MASTER_PORT` environment variable.
+ """
+ with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
+ s.bind(('127.0.0.1', 0))
+ return s.getsockname()[1] # port
+
+
+def generate_ddp_file(trainer):
+ """Generates a DDP file and returns its file name."""
+ module, name = f'{trainer.__class__.__module__}.{trainer.__class__.__name__}'.rsplit('.', 1)
+
+ content = f'''overrides = {vars(trainer.args)} \nif __name__ == "__main__":
+ from {module} import {name}
+ from ultralytics.yolo.utils import DEFAULT_CFG_DICT
+
+ cfg = DEFAULT_CFG_DICT.copy()
+ cfg.update(save_dir='') # handle the extra key 'save_dir'
+ trainer = {name}(cfg=cfg, overrides=overrides)
+ trainer.train()'''
+ (USER_CONFIG_DIR / 'DDP').mkdir(exist_ok=True)
+ with tempfile.NamedTemporaryFile(prefix='_temp_',
+ suffix=f'{id(trainer)}.py',
+ mode='w+',
+ encoding='utf-8',
+ dir=USER_CONFIG_DIR / 'DDP',
+ delete=False) as file:
+ file.write(content)
+ return file.name
+
+
+def generate_ddp_command(world_size, trainer):
+ """Generates and returns command for distributed training."""
+ import __main__ # noqa local import to avoid https://github.com/Lightning-AI/lightning/issues/15218
+ if not trainer.resume:
+ shutil.rmtree(trainer.save_dir) # remove the save_dir
+ file = str(Path(sys.argv[0]).resolve())
+ safe_pattern = re.compile(r'^[a-zA-Z0-9_. /\\-]{1,128}$') # allowed characters and maximum of 100 characters
+ if not (safe_pattern.match(file) and Path(file).exists() and file.endswith('.py')): # using CLI
+ file = generate_ddp_file(trainer)
+ dist_cmd = 'torch.distributed.run' if TORCH_1_9 else 'torch.distributed.launch'
+ port = find_free_network_port()
+ cmd = [sys.executable, '-m', dist_cmd, '--nproc_per_node', f'{world_size}', '--master_port', f'{port}', file]
+ return cmd, file
+
+
+def ddp_cleanup(trainer, file):
+ """Delete temp file if created."""
+ if f'{id(trainer)}.py' in file: # if temp_file suffix in file
+ os.remove(file)
diff --git a/yolov8/ultralytics/yolo/utils/downloads.py b/yolov8/ultralytics/yolo/utils/downloads.py
new file mode 100644
index 0000000000000000000000000000000000000000..53f58cfddcc42a745ff7672560e0d3f1b3c1398c
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/downloads.py
@@ -0,0 +1,270 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+import shutil
+import subprocess
+from itertools import repeat
+from multiprocessing.pool import ThreadPool
+from pathlib import Path
+from urllib import parse, request
+from zipfile import BadZipFile, ZipFile, is_zipfile
+
+import requests
+import torch
+from tqdm import tqdm
+
+from ultralytics.yolo.utils import LOGGER, checks, clean_url, emojis, is_online, url2file
+
+GITHUB_ASSET_NAMES = [f'yolov8{k}{suffix}.pt' for k in 'nsmlx' for suffix in ('', '6', '-cls', '-seg', '-pose')] + \
+ [f'yolov5{k}u.pt' for k in 'nsmlx'] + \
+ [f'yolov3{k}u.pt' for k in ('', '-spp', '-tiny')] + \
+ [f'yolo_nas_{k}.pt' for k in 'sml'] + \
+ [f'sam_{k}.pt' for k in 'bl'] + \
+ [f'FastSAM-{k}.pt' for k in 'sx'] + \
+ [f'rtdetr-{k}.pt' for k in 'lx']
+GITHUB_ASSET_STEMS = [Path(k).stem for k in GITHUB_ASSET_NAMES]
+
+
+def is_url(url, check=True):
+ """Check if string is URL and check if URL exists."""
+ with contextlib.suppress(Exception):
+ url = str(url)
+ result = parse.urlparse(url)
+ assert all([result.scheme, result.netloc]) # check if is url
+ if check:
+ with request.urlopen(url) as response:
+ return response.getcode() == 200 # check if exists online
+ return True
+ return False
+
+
+def unzip_file(file, path=None, exclude=('.DS_Store', '__MACOSX'), exist_ok=False):
+ """
+ Unzips a *.zip file to the specified path, excluding files containing strings in the exclude list.
+
+ If the zipfile does not contain a single top-level directory, the function will create a new
+ directory with the same name as the zipfile (without the extension) to extract its contents.
+ If a path is not provided, the function will use the parent directory of the zipfile as the default path.
+
+ Args:
+ file (str): The path to the zipfile to be extracted.
+ path (str, optional): The path to extract the zipfile to. Defaults to None.
+ exclude (tuple, optional): A tuple of filename strings to be excluded. Defaults to ('.DS_Store', '__MACOSX').
+ exist_ok (bool, optional): Whether to overwrite existing contents if they exist. Defaults to False.
+
+ Raises:
+ BadZipFile: If the provided file does not exist or is not a valid zipfile.
+
+ Returns:
+ (Path): The path to the directory where the zipfile was extracted.
+ """
+ if not (Path(file).exists() and is_zipfile(file)):
+ raise BadZipFile(f"File '{file}' does not exist or is a bad zip file.")
+ if path is None:
+ path = Path(file).parent # default path
+
+ # Unzip the file contents
+ with ZipFile(file) as zipObj:
+ file_list = [f for f in zipObj.namelist() if all(x not in f for x in exclude)]
+ top_level_dirs = {Path(f).parts[0] for f in file_list}
+
+ if len(top_level_dirs) > 1 or not file_list[0].endswith('/'):
+ path = Path(path) / Path(file).stem # define new unzip directory
+
+ # Check if destination directory already exists and contains files
+ extract_path = Path(path) / list(top_level_dirs)[0]
+ if extract_path.exists() and any(extract_path.iterdir()) and not exist_ok:
+ # If it exists and is not empty, return the path without unzipping
+ LOGGER.info(f'Skipping {file} unzip (already unzipped)')
+ return path
+
+ for f in file_list:
+ zipObj.extract(f, path=path)
+
+ return path # return unzip dir
+
+
+def check_disk_space(url='https://ultralytics.com/assets/coco128.zip', sf=1.5, hard=True):
+ """
+ Check if there is sufficient disk space to download and store a file.
+
+ Args:
+ url (str, optional): The URL to the file. Defaults to 'https://ultralytics.com/assets/coco128.zip'.
+ sf (float, optional): Safety factor, the multiplier for the required free space. Defaults to 2.0.
+ hard (bool, optional): Whether to throw an error or not on insufficient disk space. Defaults to True.
+
+ Returns:
+ (bool): True if there is sufficient disk space, False otherwise.
+ """
+ with contextlib.suppress(Exception):
+ gib = 1 << 30 # bytes per GiB
+ data = int(requests.head(url).headers['Content-Length']) / gib # file size (GB)
+ total, used, free = (x / gib for x in shutil.disk_usage('/')) # bytes
+ if data * sf < free:
+ return True # sufficient space
+
+ # Insufficient space
+ text = (f'WARNING ⚠️ Insufficient free disk space {free:.1f} GB < {data * sf:.3f} GB required, '
+ f'Please free {data * sf - free:.1f} GB additional disk space and try again.')
+ if hard:
+ raise MemoryError(text)
+ else:
+ LOGGER.warning(text)
+ return False
+
+ # Pass if error
+ return True
+
+
+def safe_download(url,
+ file=None,
+ dir=None,
+ unzip=True,
+ delete=False,
+ curl=False,
+ retry=3,
+ min_bytes=1E0,
+ progress=True):
+ """
+ Downloads files from a URL, with options for retrying, unzipping, and deleting the downloaded file.
+
+ Args:
+ url (str): The URL of the file to be downloaded.
+ file (str, optional): The filename of the downloaded file.
+ If not provided, the file will be saved with the same name as the URL.
+ dir (str, optional): The directory to save the downloaded file.
+ If not provided, the file will be saved in the current working directory.
+ unzip (bool, optional): Whether to unzip the downloaded file. Default: True.
+ delete (bool, optional): Whether to delete the downloaded file after unzipping. Default: False.
+ curl (bool, optional): Whether to use curl command line tool for downloading. Default: False.
+ retry (int, optional): The number of times to retry the download in case of failure. Default: 3.
+ min_bytes (float, optional): The minimum number of bytes that the downloaded file should have, to be considered
+ a successful download. Default: 1E0.
+ progress (bool, optional): Whether to display a progress bar during the download. Default: True.
+ """
+ f = dir / url2file(url) if dir else Path(file) # URL converted to filename
+ if '://' not in str(url) and Path(url).is_file(): # URL exists ('://' check required in Windows Python<3.10)
+ f = Path(url) # filename
+ elif not f.is_file(): # URL and file do not exist
+ assert dir or file, 'dir or file required for download'
+ f = dir / url2file(url) if dir else Path(file)
+ desc = f'Downloading {clean_url(url)} to {f}'
+ LOGGER.info(f'{desc}...')
+ f.parent.mkdir(parents=True, exist_ok=True) # make directory if missing
+ check_disk_space(url)
+ for i in range(retry + 1):
+ try:
+ if curl or i > 0: # curl download with retry, continue
+ s = 'sS' * (not progress) # silent
+ r = subprocess.run(['curl', '-#', f'-{s}L', url, '-o', f, '--retry', '3', '-C', '-']).returncode
+ assert r == 0, f'Curl return value {r}'
+ else: # urllib download
+ method = 'torch'
+ if method == 'torch':
+ torch.hub.download_url_to_file(url, f, progress=progress)
+ else:
+ from ultralytics.yolo.utils import TQDM_BAR_FORMAT
+ with request.urlopen(url) as response, tqdm(total=int(response.getheader('Content-Length', 0)),
+ desc=desc,
+ disable=not progress,
+ unit='B',
+ unit_scale=True,
+ unit_divisor=1024,
+ bar_format=TQDM_BAR_FORMAT) as pbar:
+ with open(f, 'wb') as f_opened:
+ for data in response:
+ f_opened.write(data)
+ pbar.update(len(data))
+
+ if f.exists():
+ if f.stat().st_size > min_bytes:
+ break # success
+ f.unlink() # remove partial downloads
+ except Exception as e:
+ if i == 0 and not is_online():
+ raise ConnectionError(emojis(f'❌ Download failure for {url}. Environment is not online.')) from e
+ elif i >= retry:
+ raise ConnectionError(emojis(f'❌ Download failure for {url}. Retry limit reached.')) from e
+ LOGGER.warning(f'⚠️ Download failure, retrying {i + 1}/{retry} {url}...')
+
+ if unzip and f.exists() and f.suffix in ('', '.zip', '.tar', '.gz'):
+ unzip_dir = dir or f.parent # unzip to dir if provided else unzip in place
+ LOGGER.info(f'Unzipping {f} to {unzip_dir.absolute()}...')
+ if is_zipfile(f):
+ unzip_dir = unzip_file(file=f, path=unzip_dir) # unzip
+ elif f.suffix == '.tar':
+ subprocess.run(['tar', 'xf', f, '--directory', unzip_dir], check=True) # unzip
+ elif f.suffix == '.gz':
+ subprocess.run(['tar', 'xfz', f, '--directory', unzip_dir], check=True) # unzip
+ if delete:
+ f.unlink() # remove zip
+ return unzip_dir
+
+
+def get_github_assets(repo='ultralytics/assets', version='latest'):
+ """Return GitHub repo tag and assets (i.e. ['yolov8n.pt', 'yolov8s.pt', ...])."""
+ if version != 'latest':
+ version = f'tags/{version}' # i.e. tags/v6.2
+ response = requests.get(f'https://api.github.com/repos/{repo}/releases/{version}').json() # github api
+ return response['tag_name'], [x['name'] for x in response['assets']] # tag, assets
+
+
+def attempt_download_asset(file, repo='ultralytics/assets', release='v0.0.0'):
+ """Attempt file download from GitHub release assets if not found locally. release = 'latest', 'v6.2', etc."""
+ from ultralytics.yolo.utils import SETTINGS # scoped for circular import
+
+ # YOLOv3/5u updates
+ file = str(file)
+ file = checks.check_yolov5u_filename(file)
+ file = Path(file.strip().replace("'", ''))
+ if file.exists():
+ return str(file)
+ elif (SETTINGS['weights_dir'] / file).exists():
+ return str(SETTINGS['weights_dir'] / file)
+ else:
+ # URL specified
+ name = Path(parse.unquote(str(file))).name # decode '%2F' to '/' etc.
+ if str(file).startswith(('http:/', 'https:/')): # download
+ url = str(file).replace(':/', '://') # Pathlib turns :// -> :/
+ file = url2file(name) # parse authentication https://url.com/file.txt?auth...
+ if Path(file).is_file():
+ LOGGER.info(f'Found {clean_url(url)} locally at {file}') # file already exists
+ else:
+ safe_download(url=url, file=file, min_bytes=1E5)
+ return file
+
+ # GitHub assets
+ assets = GITHUB_ASSET_NAMES
+ try:
+ tag, assets = get_github_assets(repo, release)
+ except Exception:
+ try:
+ tag, assets = get_github_assets(repo) # latest release
+ except Exception:
+ try:
+ tag = subprocess.check_output(['git', 'tag']).decode().split()[-1]
+ except Exception:
+ tag = release
+
+ file.parent.mkdir(parents=True, exist_ok=True) # make parent dir (if required)
+ if name in assets:
+ safe_download(url=f'https://github.com/{repo}/releases/download/{tag}/{name}', file=file, min_bytes=1E5)
+
+ return str(file)
+
+
+def download(url, dir=Path.cwd(), unzip=True, delete=False, curl=False, threads=1, retry=3):
+ """Downloads and unzips files concurrently if threads > 1, else sequentially."""
+ dir = Path(dir)
+ dir.mkdir(parents=True, exist_ok=True) # make directory
+ if threads > 1:
+ with ThreadPool(threads) as pool:
+ pool.map(
+ lambda x: safe_download(
+ url=x[0], dir=x[1], unzip=unzip, delete=delete, curl=curl, retry=retry, progress=threads <= 1),
+ zip(url, repeat(dir)))
+ pool.close()
+ pool.join()
+ else:
+ for u in [url] if isinstance(url, (str, Path)) else url:
+ safe_download(url=u, dir=dir, unzip=unzip, delete=delete, curl=curl, retry=retry)
diff --git a/yolov8/ultralytics/yolo/utils/errors.py b/yolov8/ultralytics/yolo/utils/errors.py
new file mode 100644
index 0000000000000000000000000000000000000000..7163d4d2576fc78ed25f36bf3a4ec06981ea94e6
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/errors.py
@@ -0,0 +1,10 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from ultralytics.yolo.utils import emojis
+
+
+class HUBModelError(Exception):
+
+ def __init__(self, message='Model not found. Please check model URL and try again.'):
+ """Create an exception for when a model is not found."""
+ super().__init__(emojis(message))
diff --git a/yolov8/ultralytics/yolo/utils/files.py b/yolov8/ultralytics/yolo/utils/files.py
new file mode 100644
index 0000000000000000000000000000000000000000..2a13c4eb2bdad8a2ca8672ceb08c39c19ba59679
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/files.py
@@ -0,0 +1,100 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+import glob
+import os
+import shutil
+from datetime import datetime
+from pathlib import Path
+
+
+class WorkingDirectory(contextlib.ContextDecorator):
+ """Usage: @WorkingDirectory(dir) decorator or 'with WorkingDirectory(dir):' context manager."""
+
+ def __init__(self, new_dir):
+ """Sets the working directory to 'new_dir' upon instantiation."""
+ self.dir = new_dir # new dir
+ self.cwd = Path.cwd().resolve() # current dir
+
+ def __enter__(self):
+ """Changes the current directory to the specified directory."""
+ os.chdir(self.dir)
+
+ def __exit__(self, exc_type, exc_val, exc_tb):
+ """Restore the current working directory on context exit."""
+ os.chdir(self.cwd)
+
+
+def increment_path(path, exist_ok=False, sep='', mkdir=False):
+ """
+ Increments a file or directory path, i.e. runs/exp --> runs/exp{sep}2, runs/exp{sep}3, ... etc.
+
+ If the path exists and exist_ok is not set to True, the path will be incremented by appending a number and sep to
+ the end of the path. If the path is a file, the file extension will be preserved. If the path is a directory, the
+ number will be appended directly to the end of the path. If mkdir is set to True, the path will be created as a
+ directory if it does not already exist.
+
+ Args:
+ path (str, pathlib.Path): Path to increment.
+ exist_ok (bool, optional): If True, the path will not be incremented and returned as-is. Defaults to False.
+ sep (str, optional): Separator to use between the path and the incrementation number. Defaults to ''.
+ mkdir (bool, optional): Create a directory if it does not exist. Defaults to False.
+
+ Returns:
+ (pathlib.Path): Incremented path.
+ """
+ path = Path(path) # os-agnostic
+ if path.exists() and not exist_ok:
+ path, suffix = (path.with_suffix(''), path.suffix) if path.is_file() else (path, '')
+
+ # Method 1
+ for n in range(2, 9999):
+ p = f'{path}{sep}{n}{suffix}' # increment path
+ if not os.path.exists(p): #
+ break
+ path = Path(p)
+
+ if mkdir:
+ path.mkdir(parents=True, exist_ok=True) # make directory
+
+ return path
+
+
+def file_age(path=__file__):
+ """Return days since last file update."""
+ dt = (datetime.now() - datetime.fromtimestamp(Path(path).stat().st_mtime)) # delta
+ return dt.days # + dt.seconds / 86400 # fractional days
+
+
+def file_date(path=__file__):
+ """Return human-readable file modification date, i.e. '2021-3-26'."""
+ t = datetime.fromtimestamp(Path(path).stat().st_mtime)
+ return f'{t.year}-{t.month}-{t.day}'
+
+
+def file_size(path):
+ """Return file/dir size (MB)."""
+ if isinstance(path, (str, Path)):
+ mb = 1 << 20 # bytes to MiB (1024 ** 2)
+ path = Path(path)
+ if path.is_file():
+ return path.stat().st_size / mb
+ elif path.is_dir():
+ return sum(f.stat().st_size for f in path.glob('**/*') if f.is_file()) / mb
+ return 0.0
+
+
+def get_latest_run(search_dir='.'):
+ """Return path to most recent 'last.pt' in /runs (i.e. to --resume from)."""
+ last_list = glob.glob(f'{search_dir}/**/last*.pt', recursive=True)
+ return max(last_list, key=os.path.getctime) if last_list else ''
+
+
+def make_dirs(dir='new_dir/'):
+ # Create folders
+ dir = Path(dir)
+ if dir.exists():
+ shutil.rmtree(dir) # delete dir
+ for p in dir, dir / 'labels', dir / 'images':
+ p.mkdir(parents=True, exist_ok=True) # make dir
+ return dir
diff --git a/yolov8/ultralytics/yolo/utils/instance.py b/yolov8/ultralytics/yolo/utils/instance.py
new file mode 100644
index 0000000000000000000000000000000000000000..3566f6e2ea70cc7ac1db46a04a9d1546e3792ac9
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/instance.py
@@ -0,0 +1,391 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from collections import abc
+from itertools import repeat
+from numbers import Number
+from typing import List
+
+import numpy as np
+
+from .ops import ltwh2xywh, ltwh2xyxy, resample_segments, xywh2ltwh, xywh2xyxy, xyxy2ltwh, xyxy2xywh
+
+
+def _ntuple(n):
+ """From PyTorch internals."""
+
+ def parse(x):
+ """Parse bounding boxes format between XYWH and LTWH."""
+ return x if isinstance(x, abc.Iterable) else tuple(repeat(x, n))
+
+ return parse
+
+
+to_4tuple = _ntuple(4)
+
+# `xyxy` means left top and right bottom
+# `xywh` means center x, center y and width, height(yolo format)
+# `ltwh` means left top and width, height(coco format)
+_formats = ['xyxy', 'xywh', 'ltwh']
+
+__all__ = 'Bboxes', # tuple or list
+
+
+class Bboxes:
+ """Now only numpy is supported."""
+
+ def __init__(self, bboxes, format='xyxy') -> None:
+ assert format in _formats, f'Invalid bounding box format: {format}, format must be one of {_formats}'
+ bboxes = bboxes[None, :] if bboxes.ndim == 1 else bboxes
+ assert bboxes.ndim == 2
+ assert bboxes.shape[1] == 4
+ self.bboxes = bboxes
+ self.format = format
+ # self.normalized = normalized
+
+ # def convert(self, format):
+ # assert format in _formats
+ # if self.format == format:
+ # bboxes = self.bboxes
+ # elif self.format == "xyxy":
+ # if format == "xywh":
+ # bboxes = xyxy2xywh(self.bboxes)
+ # else:
+ # bboxes = xyxy2ltwh(self.bboxes)
+ # elif self.format == "xywh":
+ # if format == "xyxy":
+ # bboxes = xywh2xyxy(self.bboxes)
+ # else:
+ # bboxes = xywh2ltwh(self.bboxes)
+ # else:
+ # if format == "xyxy":
+ # bboxes = ltwh2xyxy(self.bboxes)
+ # else:
+ # bboxes = ltwh2xywh(self.bboxes)
+ #
+ # return Bboxes(bboxes, format)
+
+ def convert(self, format):
+ """Converts bounding box format from one type to another."""
+ assert format in _formats, f'Invalid bounding box format: {format}, format must be one of {_formats}'
+ if self.format == format:
+ return
+ elif self.format == 'xyxy':
+ bboxes = xyxy2xywh(self.bboxes) if format == 'xywh' else xyxy2ltwh(self.bboxes)
+ elif self.format == 'xywh':
+ bboxes = xywh2xyxy(self.bboxes) if format == 'xyxy' else xywh2ltwh(self.bboxes)
+ else:
+ bboxes = ltwh2xyxy(self.bboxes) if format == 'xyxy' else ltwh2xywh(self.bboxes)
+ self.bboxes = bboxes
+ self.format = format
+
+ def areas(self):
+ """Return box areas."""
+ self.convert('xyxy')
+ return (self.bboxes[:, 2] - self.bboxes[:, 0]) * (self.bboxes[:, 3] - self.bboxes[:, 1])
+
+ # def denormalize(self, w, h):
+ # if not self.normalized:
+ # return
+ # assert (self.bboxes <= 1.0).all()
+ # self.bboxes[:, 0::2] *= w
+ # self.bboxes[:, 1::2] *= h
+ # self.normalized = False
+ #
+ # def normalize(self, w, h):
+ # if self.normalized:
+ # return
+ # assert (self.bboxes > 1.0).any()
+ # self.bboxes[:, 0::2] /= w
+ # self.bboxes[:, 1::2] /= h
+ # self.normalized = True
+
+ def mul(self, scale):
+ """
+ Args:
+ scale (tuple | list | int): the scale for four coords.
+ """
+ if isinstance(scale, Number):
+ scale = to_4tuple(scale)
+ assert isinstance(scale, (tuple, list))
+ assert len(scale) == 4
+ self.bboxes[:, 0] *= scale[0]
+ self.bboxes[:, 1] *= scale[1]
+ self.bboxes[:, 2] *= scale[2]
+ self.bboxes[:, 3] *= scale[3]
+
+ def add(self, offset):
+ """
+ Args:
+ offset (tuple | list | int): the offset for four coords.
+ """
+ if isinstance(offset, Number):
+ offset = to_4tuple(offset)
+ assert isinstance(offset, (tuple, list))
+ assert len(offset) == 4
+ self.bboxes[:, 0] += offset[0]
+ self.bboxes[:, 1] += offset[1]
+ self.bboxes[:, 2] += offset[2]
+ self.bboxes[:, 3] += offset[3]
+
+ def __len__(self):
+ """Return the number of boxes."""
+ return len(self.bboxes)
+
+ @classmethod
+ def concatenate(cls, boxes_list: List['Bboxes'], axis=0) -> 'Bboxes':
+ """
+ Concatenate a list of Bboxes objects into a single Bboxes object.
+
+ Args:
+ boxes_list (List[Bboxes]): A list of Bboxes objects to concatenate.
+ axis (int, optional): The axis along which to concatenate the bounding boxes.
+ Defaults to 0.
+
+ Returns:
+ Bboxes: A new Bboxes object containing the concatenated bounding boxes.
+
+ Note:
+ The input should be a list or tuple of Bboxes objects.
+ """
+ assert isinstance(boxes_list, (list, tuple))
+ if not boxes_list:
+ return cls(np.empty(0))
+ assert all(isinstance(box, Bboxes) for box in boxes_list)
+
+ if len(boxes_list) == 1:
+ return boxes_list[0]
+ return cls(np.concatenate([b.bboxes for b in boxes_list], axis=axis))
+
+ def __getitem__(self, index) -> 'Bboxes':
+ """
+ Retrieve a specific bounding box or a set of bounding boxes using indexing.
+
+ Args:
+ index (int, slice, or np.ndarray): The index, slice, or boolean array to select
+ the desired bounding boxes.
+
+ Returns:
+ Bboxes: A new Bboxes object containing the selected bounding boxes.
+
+ Raises:
+ AssertionError: If the indexed bounding boxes do not form a 2-dimensional matrix.
+
+ Note:
+ When using boolean indexing, make sure to provide a boolean array with the same
+ length as the number of bounding boxes.
+ """
+ if isinstance(index, int):
+ return Bboxes(self.bboxes[index].view(1, -1))
+ b = self.bboxes[index]
+ assert b.ndim == 2, f'Indexing on Bboxes with {index} failed to return a matrix!'
+ return Bboxes(b)
+
+
+class Instances:
+
+ def __init__(self, bboxes, segments=None, keypoints=None, bbox_format='xywh', normalized=True) -> None:
+ """
+ Args:
+ bboxes (ndarray): bboxes with shape [N, 4].
+ segments (list | ndarray): segments.
+ keypoints (ndarray): keypoints(x, y, visible) with shape [N, 17, 3].
+ """
+ if segments is None:
+ segments = []
+ self._bboxes = Bboxes(bboxes=bboxes, format=bbox_format)
+ self.keypoints = keypoints
+ self.normalized = normalized
+
+ if len(segments) > 0:
+ # list[np.array(1000, 2)] * num_samples
+ segments = resample_segments(segments)
+ # (N, 1000, 2)
+ segments = np.stack(segments, axis=0)
+ else:
+ segments = np.zeros((0, 1000, 2), dtype=np.float32)
+ self.segments = segments
+
+ def convert_bbox(self, format):
+ """Convert bounding box format."""
+ self._bboxes.convert(format=format)
+
+ @property
+ def bbox_areas(self):
+ """Calculate the area of bounding boxes."""
+ return self._bboxes.areas()
+
+ def scale(self, scale_w, scale_h, bbox_only=False):
+ """this might be similar with denormalize func but without normalized sign."""
+ self._bboxes.mul(scale=(scale_w, scale_h, scale_w, scale_h))
+ if bbox_only:
+ return
+ self.segments[..., 0] *= scale_w
+ self.segments[..., 1] *= scale_h
+ if self.keypoints is not None:
+ self.keypoints[..., 0] *= scale_w
+ self.keypoints[..., 1] *= scale_h
+
+ def denormalize(self, w, h):
+ """Denormalizes boxes, segments, and keypoints from normalized coordinates."""
+ if not self.normalized:
+ return
+ self._bboxes.mul(scale=(w, h, w, h))
+ self.segments[..., 0] *= w
+ self.segments[..., 1] *= h
+ if self.keypoints is not None:
+ self.keypoints[..., 0] *= w
+ self.keypoints[..., 1] *= h
+ self.normalized = False
+
+ def normalize(self, w, h):
+ """Normalize bounding boxes, segments, and keypoints to image dimensions."""
+ if self.normalized:
+ return
+ self._bboxes.mul(scale=(1 / w, 1 / h, 1 / w, 1 / h))
+ self.segments[..., 0] /= w
+ self.segments[..., 1] /= h
+ if self.keypoints is not None:
+ self.keypoints[..., 0] /= w
+ self.keypoints[..., 1] /= h
+ self.normalized = True
+
+ def add_padding(self, padw, padh):
+ """Handle rect and mosaic situation."""
+ assert not self.normalized, 'you should add padding with absolute coordinates.'
+ self._bboxes.add(offset=(padw, padh, padw, padh))
+ self.segments[..., 0] += padw
+ self.segments[..., 1] += padh
+ if self.keypoints is not None:
+ self.keypoints[..., 0] += padw
+ self.keypoints[..., 1] += padh
+
+ def __getitem__(self, index) -> 'Instances':
+ """
+ Retrieve a specific instance or a set of instances using indexing.
+
+ Args:
+ index (int, slice, or np.ndarray): The index, slice, or boolean array to select
+ the desired instances.
+
+ Returns:
+ Instances: A new Instances object containing the selected bounding boxes,
+ segments, and keypoints if present.
+
+ Note:
+ When using boolean indexing, make sure to provide a boolean array with the same
+ length as the number of instances.
+ """
+ segments = self.segments[index] if len(self.segments) else self.segments
+ keypoints = self.keypoints[index] if self.keypoints is not None else None
+ bboxes = self.bboxes[index]
+ bbox_format = self._bboxes.format
+ return Instances(
+ bboxes=bboxes,
+ segments=segments,
+ keypoints=keypoints,
+ bbox_format=bbox_format,
+ normalized=self.normalized,
+ )
+
+ def flipud(self, h):
+ """Flips the coordinates of bounding boxes, segments, and keypoints vertically."""
+ if self._bboxes.format == 'xyxy':
+ y1 = self.bboxes[:, 1].copy()
+ y2 = self.bboxes[:, 3].copy()
+ self.bboxes[:, 1] = h - y2
+ self.bboxes[:, 3] = h - y1
+ else:
+ self.bboxes[:, 1] = h - self.bboxes[:, 1]
+ self.segments[..., 1] = h - self.segments[..., 1]
+ if self.keypoints is not None:
+ self.keypoints[..., 1] = h - self.keypoints[..., 1]
+
+ def fliplr(self, w):
+ """Reverses the order of the bounding boxes and segments horizontally."""
+ if self._bboxes.format == 'xyxy':
+ x1 = self.bboxes[:, 0].copy()
+ x2 = self.bboxes[:, 2].copy()
+ self.bboxes[:, 0] = w - x2
+ self.bboxes[:, 2] = w - x1
+ else:
+ self.bboxes[:, 0] = w - self.bboxes[:, 0]
+ self.segments[..., 0] = w - self.segments[..., 0]
+ if self.keypoints is not None:
+ self.keypoints[..., 0] = w - self.keypoints[..., 0]
+
+ def clip(self, w, h):
+ """Clips bounding boxes, segments, and keypoints values to stay within image boundaries."""
+ ori_format = self._bboxes.format
+ self.convert_bbox(format='xyxy')
+ self.bboxes[:, [0, 2]] = self.bboxes[:, [0, 2]].clip(0, w)
+ self.bboxes[:, [1, 3]] = self.bboxes[:, [1, 3]].clip(0, h)
+ if ori_format != 'xyxy':
+ self.convert_bbox(format=ori_format)
+ self.segments[..., 0] = self.segments[..., 0].clip(0, w)
+ self.segments[..., 1] = self.segments[..., 1].clip(0, h)
+ if self.keypoints is not None:
+ self.keypoints[..., 0] = self.keypoints[..., 0].clip(0, w)
+ self.keypoints[..., 1] = self.keypoints[..., 1].clip(0, h)
+
+ def remove_zero_area_boxes(self):
+ """Remove zero-area boxes, i.e. after clipping some boxes may have zero width or height. This removes them."""
+ good = self.bbox_areas > 0
+ if not all(good):
+ self._bboxes = self._bboxes[good]
+ if len(self.segments):
+ self.segments = self.segments[good]
+ if self.keypoints is not None:
+ self.keypoints = self.keypoints[good]
+ return good
+
+ def update(self, bboxes, segments=None, keypoints=None):
+ """Updates instance variables."""
+ self._bboxes = Bboxes(bboxes, format=self._bboxes.format)
+ if segments is not None:
+ self.segments = segments
+ if keypoints is not None:
+ self.keypoints = keypoints
+
+ def __len__(self):
+ """Return the length of the instance list."""
+ return len(self.bboxes)
+
+ @classmethod
+ def concatenate(cls, instances_list: List['Instances'], axis=0) -> 'Instances':
+ """
+ Concatenates a list of Instances objects into a single Instances object.
+
+ Args:
+ instances_list (List[Instances]): A list of Instances objects to concatenate.
+ axis (int, optional): The axis along which the arrays will be concatenated. Defaults to 0.
+
+ Returns:
+ Instances: A new Instances object containing the concatenated bounding boxes,
+ segments, and keypoints if present.
+
+ Note:
+ The `Instances` objects in the list should have the same properties, such as
+ the format of the bounding boxes, whether keypoints are present, and if the
+ coordinates are normalized.
+ """
+ assert isinstance(instances_list, (list, tuple))
+ if not instances_list:
+ return cls(np.empty(0))
+ assert all(isinstance(instance, Instances) for instance in instances_list)
+
+ if len(instances_list) == 1:
+ return instances_list[0]
+
+ use_keypoint = instances_list[0].keypoints is not None
+ bbox_format = instances_list[0]._bboxes.format
+ normalized = instances_list[0].normalized
+
+ cat_boxes = np.concatenate([ins.bboxes for ins in instances_list], axis=axis)
+ cat_segments = np.concatenate([b.segments for b in instances_list], axis=axis)
+ cat_keypoints = np.concatenate([b.keypoints for b in instances_list], axis=axis) if use_keypoint else None
+ return cls(cat_boxes, cat_segments, cat_keypoints, bbox_format, normalized)
+
+ @property
+ def bboxes(self):
+ """Return bounding boxes."""
+ return self._bboxes.bboxes
diff --git a/yolov8/ultralytics/yolo/utils/loss.py b/yolov8/ultralytics/yolo/utils/loss.py
new file mode 100644
index 0000000000000000000000000000000000000000..71ed0a5263df16163173a5fd4f84815c1de3cd57
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/loss.py
@@ -0,0 +1,392 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+
+from ultralytics.yolo.utils.metrics import OKS_SIGMA
+from ultralytics.yolo.utils.ops import crop_mask, xywh2xyxy, xyxy2xywh
+from ultralytics.yolo.utils.tal import TaskAlignedAssigner, dist2bbox, make_anchors
+
+from .metrics import bbox_iou
+from .tal import bbox2dist
+
+
+class VarifocalLoss(nn.Module):
+ """Varifocal loss by Zhang et al. https://arxiv.org/abs/2008.13367."""
+
+ def __init__(self):
+ """Initialize the VarifocalLoss class."""
+ super().__init__()
+
+ def forward(self, pred_score, gt_score, label, alpha=0.75, gamma=2.0):
+ """Computes varfocal loss."""
+ weight = alpha * pred_score.sigmoid().pow(gamma) * (1 - label) + gt_score * label
+ with torch.cuda.amp.autocast(enabled=False):
+ loss = (F.binary_cross_entropy_with_logits(pred_score.float(), gt_score.float(), reduction='none') *
+ weight).mean(1).sum()
+ return loss
+
+
+# Losses
+class FocalLoss(nn.Module):
+ """Wraps focal loss around existing loss_fcn(), i.e. criteria = FocalLoss(nn.BCEWithLogitsLoss(), gamma=1.5)."""
+
+ def __init__(self, ):
+ super().__init__()
+
+ def forward(self, pred, label, gamma=1.5, alpha=0.25):
+ """Calculates and updates confusion matrix for object detection/classification tasks."""
+ loss = F.binary_cross_entropy_with_logits(pred, label, reduction='none')
+ # p_t = torch.exp(-loss)
+ # loss *= self.alpha * (1.000001 - p_t) ** self.gamma # non-zero power for gradient stability
+
+ # TF implementation https://github.com/tensorflow/addons/blob/v0.7.1/tensorflow_addons/losses/focal_loss.py
+ pred_prob = pred.sigmoid() # prob from logits
+ p_t = label * pred_prob + (1 - label) * (1 - pred_prob)
+ modulating_factor = (1.0 - p_t) ** gamma
+ loss *= modulating_factor
+ if alpha > 0:
+ alpha_factor = label * alpha + (1 - label) * (1 - alpha)
+ loss *= alpha_factor
+ return loss.mean(1).sum()
+
+
+class BboxLoss(nn.Module):
+
+ def __init__(self, reg_max, use_dfl=False):
+ """Initialize the BboxLoss module with regularization maximum and DFL settings."""
+ super().__init__()
+ self.reg_max = reg_max
+ self.use_dfl = use_dfl
+
+ def forward(self, pred_dist, pred_bboxes, anchor_points, target_bboxes, target_scores, target_scores_sum, fg_mask):
+ """IoU loss."""
+ weight = target_scores.sum(-1)[fg_mask].unsqueeze(-1)
+ iou = bbox_iou(pred_bboxes[fg_mask], target_bboxes[fg_mask], xywh=False, CIoU=True)
+ loss_iou = ((1.0 - iou) * weight).sum() / target_scores_sum
+
+ # DFL loss
+ if self.use_dfl:
+ target_ltrb = bbox2dist(anchor_points, target_bboxes, self.reg_max)
+ loss_dfl = self._df_loss(pred_dist[fg_mask].view(-1, self.reg_max + 1), target_ltrb[fg_mask]) * weight
+ loss_dfl = loss_dfl.sum() / target_scores_sum
+ else:
+ loss_dfl = torch.tensor(0.0).to(pred_dist.device)
+
+ return loss_iou, loss_dfl
+
+ @staticmethod
+ def _df_loss(pred_dist, target):
+ """Return sum of left and right DFL losses."""
+ # Distribution Focal Loss (DFL) proposed in Generalized Focal Loss https://ieeexplore.ieee.org/document/9792391
+ tl = target.long() # target left
+ tr = tl + 1 # target right
+ wl = tr - target # weight left
+ wr = 1 - wl # weight right
+ return (F.cross_entropy(pred_dist, tl.view(-1), reduction='none').view(tl.shape) * wl +
+ F.cross_entropy(pred_dist, tr.view(-1), reduction='none').view(tl.shape) * wr).mean(-1, keepdim=True)
+
+
+class KeypointLoss(nn.Module):
+
+ def __init__(self, sigmas) -> None:
+ super().__init__()
+ self.sigmas = sigmas
+
+ def forward(self, pred_kpts, gt_kpts, kpt_mask, area):
+ """Calculates keypoint loss factor and Euclidean distance loss for predicted and actual keypoints."""
+ d = (pred_kpts[..., 0] - gt_kpts[..., 0]) ** 2 + (pred_kpts[..., 1] - gt_kpts[..., 1]) ** 2
+ kpt_loss_factor = (torch.sum(kpt_mask != 0) + torch.sum(kpt_mask == 0)) / (torch.sum(kpt_mask != 0) + 1e-9)
+ # e = d / (2 * (area * self.sigmas) ** 2 + 1e-9) # from formula
+ e = d / (2 * self.sigmas) ** 2 / (area + 1e-9) / 2 # from cocoeval
+ return kpt_loss_factor * ((1 - torch.exp(-e)) * kpt_mask).mean()
+
+
+# Criterion class for computing Detection training losses
+class v8DetectionLoss:
+
+ def __init__(self, model): # model must be de-paralleled
+
+ device = next(model.parameters()).device # get model device
+ h = model.args # hyperparameters
+
+ m = model.model[-1] # Detect() module
+ self.bce = nn.BCEWithLogitsLoss(reduction='none')
+ self.hyp = h
+ self.stride = m.stride # model strides
+ self.nc = m.nc # number of classes
+ self.no = m.no
+ self.reg_max = m.reg_max
+ self.device = device
+
+ self.use_dfl = m.reg_max > 1
+
+ self.assigner = TaskAlignedAssigner(topk=10, num_classes=self.nc, alpha=0.5, beta=6.0)
+ self.bbox_loss = BboxLoss(m.reg_max - 1, use_dfl=self.use_dfl).to(device)
+ self.proj = torch.arange(m.reg_max, dtype=torch.float, device=device)
+
+ def preprocess(self, targets, batch_size, scale_tensor):
+ """Preprocesses the target counts and matches with the input batch size to output a tensor."""
+ if targets.shape[0] == 0:
+ out = torch.zeros(batch_size, 0, 5, device=self.device)
+ else:
+ i = targets[:, 0] # image index
+ _, counts = i.unique(return_counts=True)
+ counts = counts.to(dtype=torch.int32)
+ out = torch.zeros(batch_size, counts.max(), 5, device=self.device)
+ for j in range(batch_size):
+ matches = i == j
+ n = matches.sum()
+ if n:
+ out[j, :n] = targets[matches, 1:]
+ out[..., 1:5] = xywh2xyxy(out[..., 1:5].mul_(scale_tensor))
+ return out
+
+ def bbox_decode(self, anchor_points, pred_dist):
+ """Decode predicted object bounding box coordinates from anchor points and distribution."""
+ if self.use_dfl:
+ b, a, c = pred_dist.shape # batch, anchors, channels
+ pred_dist = pred_dist.view(b, a, 4, c // 4).softmax(3).matmul(self.proj.type(pred_dist.dtype))
+ # pred_dist = pred_dist.view(b, a, c // 4, 4).transpose(2,3).softmax(3).matmul(self.proj.type(pred_dist.dtype))
+ # pred_dist = (pred_dist.view(b, a, c // 4, 4).softmax(2) * self.proj.type(pred_dist.dtype).view(1, 1, -1, 1)).sum(2)
+ return dist2bbox(pred_dist, anchor_points, xywh=False)
+
+ def __call__(self, preds, batch):
+ """Calculate the sum of the loss for box, cls and dfl multiplied by batch size."""
+ loss = torch.zeros(3, device=self.device) # box, cls, dfl
+ feats = preds[1] if isinstance(preds, tuple) else preds
+ pred_distri, pred_scores = torch.cat([xi.view(feats[0].shape[0], self.no, -1) for xi in feats], 2).split(
+ (self.reg_max * 4, self.nc), 1)
+
+ pred_scores = pred_scores.permute(0, 2, 1).contiguous()
+ pred_distri = pred_distri.permute(0, 2, 1).contiguous()
+
+ dtype = pred_scores.dtype
+ batch_size = pred_scores.shape[0]
+ imgsz = torch.tensor(feats[0].shape[2:], device=self.device, dtype=dtype) * self.stride[0] # image size (h,w)
+ anchor_points, stride_tensor = make_anchors(feats, self.stride, 0.5)
+
+ # targets
+ targets = torch.cat((batch['batch_idx'].view(-1, 1), batch['cls'].view(-1, 1), batch['bboxes']), 1)
+ targets = self.preprocess(targets.to(self.device), batch_size, scale_tensor=imgsz[[1, 0, 1, 0]])
+ gt_labels, gt_bboxes = targets.split((1, 4), 2) # cls, xyxy
+ mask_gt = gt_bboxes.sum(2, keepdim=True).gt_(0)
+
+ # pboxes
+ pred_bboxes = self.bbox_decode(anchor_points, pred_distri) # xyxy, (b, h*w, 4)
+
+ _, target_bboxes, target_scores, fg_mask, _ = self.assigner(
+ pred_scores.detach().sigmoid(), (pred_bboxes.detach() * stride_tensor).type(gt_bboxes.dtype),
+ anchor_points * stride_tensor, gt_labels, gt_bboxes, mask_gt)
+
+ target_scores_sum = max(target_scores.sum(), 1)
+
+ # cls loss
+ # loss[1] = self.varifocal_loss(pred_scores, target_scores, target_labels) / target_scores_sum # VFL way
+ loss[1] = self.bce(pred_scores, target_scores.to(dtype)).sum() / target_scores_sum # BCE
+
+ # bbox loss
+ if fg_mask.sum():
+ target_bboxes /= stride_tensor
+ loss[0], loss[2] = self.bbox_loss(pred_distri, pred_bboxes, anchor_points, target_bboxes, target_scores,
+ target_scores_sum, fg_mask)
+
+ loss[0] *= self.hyp.box # box gain
+ loss[1] *= self.hyp.cls # cls gain
+ loss[2] *= self.hyp.dfl # dfl gain
+
+ return loss.sum() * batch_size, loss.detach() # loss(box, cls, dfl)
+
+
+# Criterion class for computing training losses
+class v8SegmentationLoss(v8DetectionLoss):
+
+ def __init__(self, model): # model must be de-paralleled
+ super().__init__(model)
+ self.nm = model.model[-1].nm # number of masks
+ self.overlap = model.args.overlap_mask
+
+ def __call__(self, preds, batch):
+ """Calculate and return the loss for the YOLO model."""
+ loss = torch.zeros(4, device=self.device) # box, cls, dfl
+ feats, pred_masks, proto = preds if len(preds) == 3 else preds[1]
+ batch_size, _, mask_h, mask_w = proto.shape # batch size, number of masks, mask height, mask width
+ pred_distri, pred_scores = torch.cat([xi.view(feats[0].shape[0], self.no, -1) for xi in feats], 2).split(
+ (self.reg_max * 4, self.nc), 1)
+
+ # b, grids, ..
+ pred_scores = pred_scores.permute(0, 2, 1).contiguous()
+ pred_distri = pred_distri.permute(0, 2, 1).contiguous()
+ pred_masks = pred_masks.permute(0, 2, 1).contiguous()
+
+ dtype = pred_scores.dtype
+ imgsz = torch.tensor(feats[0].shape[2:], device=self.device, dtype=dtype) * self.stride[0] # image size (h,w)
+ anchor_points, stride_tensor = make_anchors(feats, self.stride, 0.5)
+
+ # targets
+ try:
+ batch_idx = batch['batch_idx'].view(-1, 1)
+ targets = torch.cat((batch_idx, batch['cls'].view(-1, 1), batch['bboxes']), 1)
+ targets = self.preprocess(targets.to(self.device), batch_size, scale_tensor=imgsz[[1, 0, 1, 0]])
+ gt_labels, gt_bboxes = targets.split((1, 4), 2) # cls, xyxy
+ mask_gt = gt_bboxes.sum(2, keepdim=True).gt_(0)
+ except RuntimeError as e:
+ raise TypeError('ERROR ❌ segment dataset incorrectly formatted or not a segment dataset.\n'
+ "This error can occur when incorrectly training a 'segment' model on a 'detect' dataset, "
+ "i.e. 'yolo train model=yolov8n-seg.pt data=coco128.yaml'.\nVerify your dataset is a "
+ "correctly formatted 'segment' dataset using 'data=coco128-seg.yaml' "
+ 'as an example.\nSee https://docs.ultralytics.com/tasks/segment/ for help.') from e
+
+ # pboxes
+ pred_bboxes = self.bbox_decode(anchor_points, pred_distri) # xyxy, (b, h*w, 4)
+
+ _, target_bboxes, target_scores, fg_mask, target_gt_idx = self.assigner(
+ pred_scores.detach().sigmoid(), (pred_bboxes.detach() * stride_tensor).type(gt_bboxes.dtype),
+ anchor_points * stride_tensor, gt_labels, gt_bboxes, mask_gt)
+
+ target_scores_sum = max(target_scores.sum(), 1)
+
+ # cls loss
+ # loss[1] = self.varifocal_loss(pred_scores, target_scores, target_labels) / target_scores_sum # VFL way
+ loss[2] = self.bce(pred_scores, target_scores.to(dtype)).sum() / target_scores_sum # BCE
+
+ if fg_mask.sum():
+ # bbox loss
+ loss[0], loss[3] = self.bbox_loss(pred_distri, pred_bboxes, anchor_points, target_bboxes / stride_tensor,
+ target_scores, target_scores_sum, fg_mask)
+ # masks loss
+ masks = batch['masks'].to(self.device).float()
+ if tuple(masks.shape[-2:]) != (mask_h, mask_w): # downsample
+ masks = F.interpolate(masks[None], (mask_h, mask_w), mode='nearest')[0]
+
+ for i in range(batch_size):
+ if fg_mask[i].sum():
+ mask_idx = target_gt_idx[i][fg_mask[i]]
+ if self.overlap:
+ gt_mask = torch.where(masks[[i]] == (mask_idx + 1).view(-1, 1, 1), 1.0, 0.0)
+ else:
+ gt_mask = masks[batch_idx.view(-1) == i][mask_idx]
+ xyxyn = target_bboxes[i][fg_mask[i]] / imgsz[[1, 0, 1, 0]]
+ marea = xyxy2xywh(xyxyn)[:, 2:].prod(1)
+ mxyxy = xyxyn * torch.tensor([mask_w, mask_h, mask_w, mask_h], device=self.device)
+ loss[1] += self.single_mask_loss(gt_mask, pred_masks[i][fg_mask[i]], proto[i], mxyxy, marea) # seg
+
+ # WARNING: lines below prevents Multi-GPU DDP 'unused gradient' PyTorch errors, do not remove
+ else:
+ loss[1] += (proto * 0).sum() + (pred_masks * 0).sum() # inf sums may lead to nan loss
+
+ # WARNING: lines below prevent Multi-GPU DDP 'unused gradient' PyTorch errors, do not remove
+ else:
+ loss[1] += (proto * 0).sum() + (pred_masks * 0).sum() # inf sums may lead to nan loss
+
+ loss[0] *= self.hyp.box # box gain
+ loss[1] *= self.hyp.box / batch_size # seg gain
+ loss[2] *= self.hyp.cls # cls gain
+ loss[3] *= self.hyp.dfl # dfl gain
+
+ return loss.sum() * batch_size, loss.detach() # loss(box, cls, dfl)
+
+ def single_mask_loss(self, gt_mask, pred, proto, xyxy, area):
+ """Mask loss for one image."""
+ pred_mask = (pred @ proto.view(self.nm, -1)).view(-1, *proto.shape[1:]) # (n, 32) @ (32,80,80) -> (n,80,80)
+ loss = F.binary_cross_entropy_with_logits(pred_mask, gt_mask, reduction='none')
+ return (crop_mask(loss, xyxy).mean(dim=(1, 2)) / area).mean()
+
+
+# Criterion class for computing training losses
+class v8PoseLoss(v8DetectionLoss):
+
+ def __init__(self, model): # model must be de-paralleled
+ super().__init__(model)
+ self.kpt_shape = model.model[-1].kpt_shape
+ self.bce_pose = nn.BCEWithLogitsLoss()
+ is_pose = self.kpt_shape == [17, 3]
+ nkpt = self.kpt_shape[0] # number of keypoints
+ sigmas = torch.from_numpy(OKS_SIGMA).to(self.device) if is_pose else torch.ones(nkpt, device=self.device) / nkpt
+ self.keypoint_loss = KeypointLoss(sigmas=sigmas)
+
+ def __call__(self, preds, batch):
+ """Calculate the total loss and detach it."""
+ loss = torch.zeros(5, device=self.device) # box, cls, dfl, kpt_location, kpt_visibility
+ feats, pred_kpts = preds if isinstance(preds[0], list) else preds[1]
+ pred_distri, pred_scores = torch.cat([xi.view(feats[0].shape[0], self.no, -1) for xi in feats], 2).split(
+ (self.reg_max * 4, self.nc), 1)
+
+ # b, grids, ..
+ pred_scores = pred_scores.permute(0, 2, 1).contiguous()
+ pred_distri = pred_distri.permute(0, 2, 1).contiguous()
+ pred_kpts = pred_kpts.permute(0, 2, 1).contiguous()
+
+ dtype = pred_scores.dtype
+ imgsz = torch.tensor(feats[0].shape[2:], device=self.device, dtype=dtype) * self.stride[0] # image size (h,w)
+ anchor_points, stride_tensor = make_anchors(feats, self.stride, 0.5)
+
+ # targets
+ batch_size = pred_scores.shape[0]
+ batch_idx = batch['batch_idx'].view(-1, 1)
+ targets = torch.cat((batch_idx, batch['cls'].view(-1, 1), batch['bboxes']), 1)
+ targets = self.preprocess(targets.to(self.device), batch_size, scale_tensor=imgsz[[1, 0, 1, 0]])
+ gt_labels, gt_bboxes = targets.split((1, 4), 2) # cls, xyxy
+ mask_gt = gt_bboxes.sum(2, keepdim=True).gt_(0)
+
+ # pboxes
+ pred_bboxes = self.bbox_decode(anchor_points, pred_distri) # xyxy, (b, h*w, 4)
+ pred_kpts = self.kpts_decode(anchor_points, pred_kpts.view(batch_size, -1, *self.kpt_shape)) # (b, h*w, 17, 3)
+
+ _, target_bboxes, target_scores, fg_mask, target_gt_idx = self.assigner(
+ pred_scores.detach().sigmoid(), (pred_bboxes.detach() * stride_tensor).type(gt_bboxes.dtype),
+ anchor_points * stride_tensor, gt_labels, gt_bboxes, mask_gt)
+
+ target_scores_sum = max(target_scores.sum(), 1)
+
+ # cls loss
+ # loss[1] = self.varifocal_loss(pred_scores, target_scores, target_labels) / target_scores_sum # VFL way
+ loss[3] = self.bce(pred_scores, target_scores.to(dtype)).sum() / target_scores_sum # BCE
+
+ # bbox loss
+ if fg_mask.sum():
+ target_bboxes /= stride_tensor
+ loss[0], loss[4] = self.bbox_loss(pred_distri, pred_bboxes, anchor_points, target_bboxes, target_scores,
+ target_scores_sum, fg_mask)
+ keypoints = batch['keypoints'].to(self.device).float().clone()
+ keypoints[..., 0] *= imgsz[1]
+ keypoints[..., 1] *= imgsz[0]
+ for i in range(batch_size):
+ if fg_mask[i].sum():
+ idx = target_gt_idx[i][fg_mask[i]]
+ gt_kpt = keypoints[batch_idx.view(-1) == i][idx] # (n, 51)
+ gt_kpt[..., 0] /= stride_tensor[fg_mask[i]]
+ gt_kpt[..., 1] /= stride_tensor[fg_mask[i]]
+ area = xyxy2xywh(target_bboxes[i][fg_mask[i]])[:, 2:].prod(1, keepdim=True)
+ pred_kpt = pred_kpts[i][fg_mask[i]]
+ kpt_mask = gt_kpt[..., 2] != 0
+ loss[1] += self.keypoint_loss(pred_kpt, gt_kpt, kpt_mask, area) # pose loss
+ # kpt_score loss
+ if pred_kpt.shape[-1] == 3:
+ loss[2] += self.bce_pose(pred_kpt[..., 2], kpt_mask.float()) # keypoint obj loss
+
+ loss[0] *= self.hyp.box # box gain
+ loss[1] *= self.hyp.pose / batch_size # pose gain
+ loss[2] *= self.hyp.kobj / batch_size # kobj gain
+ loss[3] *= self.hyp.cls # cls gain
+ loss[4] *= self.hyp.dfl # dfl gain
+
+ return loss.sum() * batch_size, loss.detach() # loss(box, cls, dfl)
+
+ def kpts_decode(self, anchor_points, pred_kpts):
+ """Decodes predicted keypoints to image coordinates."""
+ y = pred_kpts.clone()
+ y[..., :2] *= 2.0
+ y[..., 0] += anchor_points[:, [0]] - 0.5
+ y[..., 1] += anchor_points[:, [1]] - 0.5
+ return y
+
+
+class v8ClassificationLoss:
+
+ def __call__(self, preds, batch):
+ """Compute the classification loss between predictions and true labels."""
+ loss = torch.nn.functional.cross_entropy(preds, batch['cls'], reduction='sum') / 64
+ loss_items = loss.detach()
+ return loss, loss_items
diff --git a/yolov8/ultralytics/yolo/utils/metrics.py b/yolov8/ultralytics/yolo/utils/metrics.py
new file mode 100644
index 0000000000000000000000000000000000000000..cd903213feb85e8e2dd517194c30f10a26bac359
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/metrics.py
@@ -0,0 +1,977 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Model validation metrics
+"""
+import math
+import warnings
+from pathlib import Path
+
+import matplotlib.pyplot as plt
+import numpy as np
+import torch
+
+from ultralytics.yolo.utils import LOGGER, SimpleClass, TryExcept, plt_settings
+
+OKS_SIGMA = np.array([.26, .25, .25, .35, .35, .79, .79, .72, .72, .62, .62, 1.07, 1.07, .87, .87, .89, .89]) / 10.0
+
+
+# Boxes
+def box_area(box):
+ """Return box area, where box shape is xyxy(4,n)."""
+ return (box[2] - box[0]) * (box[3] - box[1])
+
+
+def bbox_ioa(box1, box2, eps=1e-7):
+ """
+ Calculate the intersection over box2 area given box1 and box2. Boxes are in x1y1x2y2 format.
+
+ Args:
+ box1 (np.array): A numpy array of shape (n, 4) representing n bounding boxes.
+ box2 (np.array): A numpy array of shape (m, 4) representing m bounding boxes.
+ eps (float, optional): A small value to avoid division by zero. Defaults to 1e-7.
+
+ Returns:
+ (np.array): A numpy array of shape (n, m) representing the intersection over box2 area.
+ """
+
+ # Get the coordinates of bounding boxes
+ b1_x1, b1_y1, b1_x2, b1_y2 = box1.T
+ b2_x1, b2_y1, b2_x2, b2_y2 = box2.T
+
+ # Intersection area
+ inter_area = (np.minimum(b1_x2[:, None], b2_x2) - np.maximum(b1_x1[:, None], b2_x1)).clip(0) * \
+ (np.minimum(b1_y2[:, None], b2_y2) - np.maximum(b1_y1[:, None], b2_y1)).clip(0)
+
+ # box2 area
+ box2_area = (b2_x2 - b2_x1) * (b2_y2 - b2_y1) + eps
+
+ # Intersection over box2 area
+ return inter_area / box2_area
+
+
+def box_iou(box1, box2, eps=1e-7):
+ """
+ Calculate intersection-over-union (IoU) of boxes.
+ Both sets of boxes are expected to be in (x1, y1, x2, y2) format.
+ Based on https://github.com/pytorch/vision/blob/master/torchvision/ops/boxes.py
+
+ Args:
+ box1 (torch.Tensor): A tensor of shape (N, 4) representing N bounding boxes.
+ box2 (torch.Tensor): A tensor of shape (M, 4) representing M bounding boxes.
+ eps (float, optional): A small value to avoid division by zero. Defaults to 1e-7.
+
+ Returns:
+ (torch.Tensor): An NxM tensor containing the pairwise IoU values for every element in box1 and box2.
+ """
+
+ # inter(N,M) = (rb(N,M,2) - lt(N,M,2)).clamp(0).prod(2)
+ (a1, a2), (b1, b2) = box1.unsqueeze(1).chunk(2, 2), box2.unsqueeze(0).chunk(2, 2)
+ inter = (torch.min(a2, b2) - torch.max(a1, b1)).clamp_(0).prod(2)
+
+ # IoU = inter / (area1 + area2 - inter)
+ return inter / ((a2 - a1).prod(2) + (b2 - b1).prod(2) - inter + eps)
+
+
+def bbox_iou(box1, box2, xywh=True, GIoU=False, DIoU=False, CIoU=False, eps=1e-7):
+ """
+ Calculate Intersection over Union (IoU) of box1(1, 4) to box2(n, 4).
+
+ Args:
+ box1 (torch.Tensor): A tensor representing a single bounding box with shape (1, 4).
+ box2 (torch.Tensor): A tensor representing n bounding boxes with shape (n, 4).
+ xywh (bool, optional): If True, input boxes are in (x, y, w, h) format. If False, input boxes are in
+ (x1, y1, x2, y2) format. Defaults to True.
+ GIoU (bool, optional): If True, calculate Generalized IoU. Defaults to False.
+ DIoU (bool, optional): If True, calculate Distance IoU. Defaults to False.
+ CIoU (bool, optional): If True, calculate Complete IoU. Defaults to False.
+ eps (float, optional): A small value to avoid division by zero. Defaults to 1e-7.
+
+ Returns:
+ (torch.Tensor): IoU, GIoU, DIoU, or CIoU values depending on the specified flags.
+ """
+
+ # Get the coordinates of bounding boxes
+ if xywh: # transform from xywh to xyxy
+ (x1, y1, w1, h1), (x2, y2, w2, h2) = box1.chunk(4, -1), box2.chunk(4, -1)
+ w1_, h1_, w2_, h2_ = w1 / 2, h1 / 2, w2 / 2, h2 / 2
+ b1_x1, b1_x2, b1_y1, b1_y2 = x1 - w1_, x1 + w1_, y1 - h1_, y1 + h1_
+ b2_x1, b2_x2, b2_y1, b2_y2 = x2 - w2_, x2 + w2_, y2 - h2_, y2 + h2_
+ else: # x1, y1, x2, y2 = box1
+ b1_x1, b1_y1, b1_x2, b1_y2 = box1.chunk(4, -1)
+ b2_x1, b2_y1, b2_x2, b2_y2 = box2.chunk(4, -1)
+ w1, h1 = b1_x2 - b1_x1, b1_y2 - b1_y1 + eps
+ w2, h2 = b2_x2 - b2_x1, b2_y2 - b2_y1 + eps
+
+ # Intersection area
+ inter = (b1_x2.minimum(b2_x2) - b1_x1.maximum(b2_x1)).clamp_(0) * \
+ (b1_y2.minimum(b2_y2) - b1_y1.maximum(b2_y1)).clamp_(0)
+
+ # Union Area
+ union = w1 * h1 + w2 * h2 - inter + eps
+
+ # IoU
+ iou = inter / union
+ if CIoU or DIoU or GIoU:
+ cw = b1_x2.maximum(b2_x2) - b1_x1.minimum(b2_x1) # convex (smallest enclosing box) width
+ ch = b1_y2.maximum(b2_y2) - b1_y1.minimum(b2_y1) # convex height
+ if CIoU or DIoU: # Distance or Complete IoU https://arxiv.org/abs/1911.08287v1
+ c2 = cw ** 2 + ch ** 2 + eps # convex diagonal squared
+ rho2 = ((b2_x1 + b2_x2 - b1_x1 - b1_x2) ** 2 + (b2_y1 + b2_y2 - b1_y1 - b1_y2) ** 2) / 4 # center dist ** 2
+ if CIoU: # https://github.com/Zzh-tju/DIoU-SSD-pytorch/blob/master/utils/box/box_utils.py#L47
+ v = (4 / math.pi ** 2) * (torch.atan(w2 / h2) - torch.atan(w1 / h1)).pow(2)
+ with torch.no_grad():
+ alpha = v / (v - iou + (1 + eps))
+ return iou - (rho2 / c2 + v * alpha) # CIoU
+ return iou - rho2 / c2 # DIoU
+ c_area = cw * ch + eps # convex area
+ return iou - (c_area - union) / c_area # GIoU https://arxiv.org/pdf/1902.09630.pdf
+ return iou # IoU
+
+
+def mask_iou(mask1, mask2, eps=1e-7):
+ """
+ Calculate masks IoU.
+
+ Args:
+ mask1 (torch.Tensor): A tensor of shape (N, n) where N is the number of ground truth objects and n is the
+ product of image width and height.
+ mask2 (torch.Tensor): A tensor of shape (M, n) where M is the number of predicted objects and n is the
+ product of image width and height.
+ eps (float, optional): A small value to avoid division by zero. Defaults to 1e-7.
+
+ Returns:
+ (torch.Tensor): A tensor of shape (N, M) representing masks IoU.
+ """
+ intersection = torch.matmul(mask1, mask2.T).clamp_(0)
+ union = (mask1.sum(1)[:, None] + mask2.sum(1)[None]) - intersection # (area1 + area2) - intersection
+ return intersection / (union + eps)
+
+
+def kpt_iou(kpt1, kpt2, area, sigma, eps=1e-7):
+ """
+ Calculate Object Keypoint Similarity (OKS).
+
+ Args:
+ kpt1 (torch.Tensor): A tensor of shape (N, 17, 3) representing ground truth keypoints.
+ kpt2 (torch.Tensor): A tensor of shape (M, 17, 3) representing predicted keypoints.
+ area (torch.Tensor): A tensor of shape (N,) representing areas from ground truth.
+ sigma (list): A list containing 17 values representing keypoint scales.
+ eps (float, optional): A small value to avoid division by zero. Defaults to 1e-7.
+
+ Returns:
+ (torch.Tensor): A tensor of shape (N, M) representing keypoint similarities.
+ """
+ d = (kpt1[:, None, :, 0] - kpt2[..., 0]) ** 2 + (kpt1[:, None, :, 1] - kpt2[..., 1]) ** 2 # (N, M, 17)
+ sigma = torch.tensor(sigma, device=kpt1.device, dtype=kpt1.dtype) # (17, )
+ kpt_mask = kpt1[..., 2] != 0 # (N, 17)
+ e = d / (2 * sigma) ** 2 / (area[:, None, None] + eps) / 2 # from cocoeval
+ # e = d / ((area[None, :, None] + eps) * sigma) ** 2 / 2 # from formula
+ return (torch.exp(-e) * kpt_mask[:, None]).sum(-1) / (kpt_mask.sum(-1)[:, None] + eps)
+
+
+def smooth_BCE(eps=0.1): # https://github.com/ultralytics/yolov3/issues/238#issuecomment-598028441
+ # return positive, negative label smoothing BCE targets
+ return 1.0 - 0.5 * eps, 0.5 * eps
+
+
+class ConfusionMatrix:
+ """
+ A class for calculating and updating a confusion matrix for object detection and classification tasks.
+
+ Attributes:
+ task (str): The type of task, either 'detect' or 'classify'.
+ matrix (np.array): The confusion matrix, with dimensions depending on the task.
+ nc (int): The number of classes.
+ conf (float): The confidence threshold for detections.
+ iou_thres (float): The Intersection over Union threshold.
+ """
+
+ def __init__(self, nc, conf=0.25, iou_thres=0.45, task='detect'):
+ """Initialize attributes for the YOLO model."""
+ self.task = task
+ self.matrix = np.zeros((nc + 1, nc + 1)) if self.task == 'detect' else np.zeros((nc, nc))
+ self.nc = nc # number of classes
+ self.conf = conf
+ self.iou_thres = iou_thres
+
+ def process_cls_preds(self, preds, targets):
+ """
+ Update confusion matrix for classification task
+
+ Args:
+ preds (Array[N, min(nc,5)]): Predicted class labels.
+ targets (Array[N, 1]): Ground truth class labels.
+ """
+ preds, targets = torch.cat(preds)[:, 0], torch.cat(targets)
+ for p, t in zip(preds.cpu().numpy(), targets.cpu().numpy()):
+ self.matrix[p][t] += 1
+
+ def process_batch(self, detections, labels):
+ """
+ Update confusion matrix for object detection task.
+
+ Args:
+ detections (Array[N, 6]): Detected bounding boxes and their associated information.
+ Each row should contain (x1, y1, x2, y2, conf, class).
+ labels (Array[M, 5]): Ground truth bounding boxes and their associated class labels.
+ Each row should contain (class, x1, y1, x2, y2).
+ """
+ if detections is None:
+ gt_classes = labels.int()
+ for gc in gt_classes:
+ self.matrix[self.nc, gc] += 1 # background FN
+ return
+
+ detections = detections[detections[:, 4] > self.conf]
+ gt_classes = labels[:, 0].int()
+ detection_classes = detections[:, 5].int()
+ iou = box_iou(labels[:, 1:], detections[:, :4])
+
+ x = torch.where(iou > self.iou_thres)
+ if x[0].shape[0]:
+ matches = torch.cat((torch.stack(x, 1), iou[x[0], x[1]][:, None]), 1).cpu().numpy()
+ if x[0].shape[0] > 1:
+ matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 1], return_index=True)[1]]
+ matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 0], return_index=True)[1]]
+ else:
+ matches = np.zeros((0, 3))
+
+ n = matches.shape[0] > 0
+ m0, m1, _ = matches.transpose().astype(int)
+ for i, gc in enumerate(gt_classes):
+ j = m0 == i
+ if n and sum(j) == 1:
+ self.matrix[detection_classes[m1[j]], gc] += 1 # correct
+ else:
+ self.matrix[self.nc, gc] += 1 # true background
+
+ if n:
+ for i, dc in enumerate(detection_classes):
+ if not any(m1 == i):
+ self.matrix[dc, self.nc] += 1 # predicted background
+
+ def matrix(self):
+ """Returns the confusion matrix."""
+ return self.matrix
+
+ def tp_fp(self):
+ """Returns true positives and false positives."""
+ tp = self.matrix.diagonal() # true positives
+ fp = self.matrix.sum(1) - tp # false positives
+ # fn = self.matrix.sum(0) - tp # false negatives (missed detections)
+ return (tp[:-1], fp[:-1]) if self.task == 'detect' else (tp, fp) # remove background class if task=detect
+
+ @TryExcept('WARNING ⚠️ ConfusionMatrix plot failure')
+ @plt_settings()
+ def plot(self, normalize=True, save_dir='', names=(), on_plot=None):
+ """
+ Plot the confusion matrix using seaborn and save it to a file.
+
+ Args:
+ normalize (bool): Whether to normalize the confusion matrix.
+ save_dir (str): Directory where the plot will be saved.
+ names (tuple): Names of classes, used as labels on the plot.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered.
+ """
+ import seaborn as sn
+
+ array = self.matrix / ((self.matrix.sum(0).reshape(1, -1) + 1E-9) if normalize else 1) # normalize columns
+ array[array < 0.005] = np.nan # don't annotate (would appear as 0.00)
+
+ fig, ax = plt.subplots(1, 1, figsize=(12, 9), tight_layout=True)
+ nc, nn = self.nc, len(names) # number of classes, names
+ sn.set(font_scale=1.0 if nc < 50 else 0.8) # for label size
+ labels = (0 < nn < 99) and (nn == nc) # apply names to ticklabels
+ ticklabels = (list(names) + ['background']) if labels else 'auto'
+ with warnings.catch_warnings():
+ warnings.simplefilter('ignore') # suppress empty matrix RuntimeWarning: All-NaN slice encountered
+ sn.heatmap(array,
+ ax=ax,
+ annot=nc < 30,
+ annot_kws={
+ 'size': 8},
+ cmap='Blues',
+ fmt='.2f' if normalize else '.0f',
+ square=True,
+ vmin=0.0,
+ xticklabels=ticklabels,
+ yticklabels=ticklabels).set_facecolor((1, 1, 1))
+ title = 'Confusion Matrix' + ' Normalized' * normalize
+ ax.set_xlabel('True')
+ ax.set_ylabel('Predicted')
+ ax.set_title(title)
+ plot_fname = Path(save_dir) / f'{title.lower().replace(" ", "_")}.png'
+ fig.savefig(plot_fname, dpi=250)
+ plt.close(fig)
+ if on_plot:
+ on_plot(plot_fname)
+
+ def print(self):
+ """
+ Print the confusion matrix to the console.
+ """
+ for i in range(self.nc + 1):
+ LOGGER.info(' '.join(map(str, self.matrix[i])))
+
+
+def smooth(y, f=0.05):
+ """Box filter of fraction f."""
+ nf = round(len(y) * f * 2) // 2 + 1 # number of filter elements (must be odd)
+ p = np.ones(nf // 2) # ones padding
+ yp = np.concatenate((p * y[0], y, p * y[-1]), 0) # y padded
+ return np.convolve(yp, np.ones(nf) / nf, mode='valid') # y-smoothed
+
+
+@plt_settings()
+def plot_pr_curve(px, py, ap, save_dir=Path('pr_curve.png'), names=(), on_plot=None):
+ """Plots a precision-recall curve."""
+ fig, ax = plt.subplots(1, 1, figsize=(9, 6), tight_layout=True)
+ py = np.stack(py, axis=1)
+
+ if 0 < len(names) < 21: # display per-class legend if < 21 classes
+ for i, y in enumerate(py.T):
+ ax.plot(px, y, linewidth=1, label=f'{names[i]} {ap[i, 0]:.3f}') # plot(recall, precision)
+ else:
+ ax.plot(px, py, linewidth=1, color='grey') # plot(recall, precision)
+
+ ax.plot(px, py.mean(1), linewidth=3, color='blue', label='all classes %.3f mAP@0.5' % ap[:, 0].mean())
+ ax.set_xlabel('Recall')
+ ax.set_ylabel('Precision')
+ ax.set_xlim(0, 1)
+ ax.set_ylim(0, 1)
+ ax.legend(bbox_to_anchor=(1.04, 1), loc='upper left')
+ ax.set_title('Precision-Recall Curve')
+ fig.savefig(save_dir, dpi=250)
+ plt.close(fig)
+ if on_plot:
+ on_plot(save_dir)
+
+
+@plt_settings()
+def plot_mc_curve(px, py, save_dir=Path('mc_curve.png'), names=(), xlabel='Confidence', ylabel='Metric', on_plot=None):
+ """Plots a metric-confidence curve."""
+ fig, ax = plt.subplots(1, 1, figsize=(9, 6), tight_layout=True)
+
+ if 0 < len(names) < 21: # display per-class legend if < 21 classes
+ for i, y in enumerate(py):
+ ax.plot(px, y, linewidth=1, label=f'{names[i]}') # plot(confidence, metric)
+ else:
+ ax.plot(px, py.T, linewidth=1, color='grey') # plot(confidence, metric)
+
+ y = smooth(py.mean(0), 0.05)
+ ax.plot(px, y, linewidth=3, color='blue', label=f'all classes {y.max():.2f} at {px[y.argmax()]:.3f}')
+ ax.set_xlabel(xlabel)
+ ax.set_ylabel(ylabel)
+ ax.set_xlim(0, 1)
+ ax.set_ylim(0, 1)
+ ax.legend(bbox_to_anchor=(1.04, 1), loc='upper left')
+ ax.set_title(f'{ylabel}-Confidence Curve')
+ fig.savefig(save_dir, dpi=250)
+ plt.close(fig)
+ if on_plot:
+ on_plot(save_dir)
+
+
+def compute_ap(recall, precision):
+ """
+ Compute the average precision (AP) given the recall and precision curves.
+
+ Arguments:
+ recall (list): The recall curve.
+ precision (list): The precision curve.
+
+ Returns:
+ (float): Average precision.
+ (np.ndarray): Precision envelope curve.
+ (np.ndarray): Modified recall curve with sentinel values added at the beginning and end.
+ """
+
+ # Append sentinel values to beginning and end
+ mrec = np.concatenate(([0.0], recall, [1.0]))
+ mpre = np.concatenate(([1.0], precision, [0.0]))
+
+ # Compute the precision envelope
+ mpre = np.flip(np.maximum.accumulate(np.flip(mpre)))
+
+ # Integrate area under curve
+ method = 'interp' # methods: 'continuous', 'interp'
+ if method == 'interp':
+ x = np.linspace(0, 1, 101) # 101-point interp (COCO)
+ ap = np.trapz(np.interp(x, mrec, mpre), x) # integrate
+ else: # 'continuous'
+ i = np.where(mrec[1:] != mrec[:-1])[0] # points where x-axis (recall) changes
+ ap = np.sum((mrec[i + 1] - mrec[i]) * mpre[i + 1]) # area under curve
+
+ return ap, mpre, mrec
+
+
+def ap_per_class(tp,
+ conf,
+ pred_cls,
+ target_cls,
+ plot=False,
+ on_plot=None,
+ save_dir=Path(),
+ names=(),
+ eps=1e-16,
+ prefix=''):
+ """
+ Computes the average precision per class for object detection evaluation.
+
+ Args:
+ tp (np.ndarray): Binary array indicating whether the detection is correct (True) or not (False).
+ conf (np.ndarray): Array of confidence scores of the detections.
+ pred_cls (np.ndarray): Array of predicted classes of the detections.
+ target_cls (np.ndarray): Array of true classes of the detections.
+ plot (bool, optional): Whether to plot PR curves or not. Defaults to False.
+ on_plot (func, optional): A callback to pass plots path and data when they are rendered. Defaults to None.
+ save_dir (Path, optional): Directory to save the PR curves. Defaults to an empty path.
+ names (tuple, optional): Tuple of class names to plot PR curves. Defaults to an empty tuple.
+ eps (float, optional): A small value to avoid division by zero. Defaults to 1e-16.
+ prefix (str, optional): A prefix string for saving the plot files. Defaults to an empty string.
+
+ Returns:
+ (tuple): A tuple of six arrays and one array of unique classes, where:
+ tp (np.ndarray): True positive counts for each class.
+ fp (np.ndarray): False positive counts for each class.
+ p (np.ndarray): Precision values at each confidence threshold.
+ r (np.ndarray): Recall values at each confidence threshold.
+ f1 (np.ndarray): F1-score values at each confidence threshold.
+ ap (np.ndarray): Average precision for each class at different IoU thresholds.
+ unique_classes (np.ndarray): An array of unique classes that have data.
+
+ """
+
+ # Sort by objectness
+ i = np.argsort(-conf)
+ tp, conf, pred_cls = tp[i], conf[i], pred_cls[i]
+
+ # Find unique classes
+ unique_classes, nt = np.unique(target_cls, return_counts=True)
+ nc = unique_classes.shape[0] # number of classes, number of detections
+
+ # Create Precision-Recall curve and compute AP for each class
+ px, py = np.linspace(0, 1, 1000), [] # for plotting
+ ap, p, r = np.zeros((nc, tp.shape[1])), np.zeros((nc, 1000)), np.zeros((nc, 1000))
+ for ci, c in enumerate(unique_classes):
+ i = pred_cls == c
+ n_l = nt[ci] # number of labels
+ n_p = i.sum() # number of predictions
+ if n_p == 0 or n_l == 0:
+ continue
+
+ # Accumulate FPs and TPs
+ fpc = (1 - tp[i]).cumsum(0)
+ tpc = tp[i].cumsum(0)
+
+ # Recall
+ recall = tpc / (n_l + eps) # recall curve
+ r[ci] = np.interp(-px, -conf[i], recall[:, 0], left=0) # negative x, xp because xp decreases
+
+ # Precision
+ precision = tpc / (tpc + fpc) # precision curve
+ p[ci] = np.interp(-px, -conf[i], precision[:, 0], left=1) # p at pr_score
+
+ # AP from recall-precision curve
+ for j in range(tp.shape[1]):
+ ap[ci, j], mpre, mrec = compute_ap(recall[:, j], precision[:, j])
+ if plot and j == 0:
+ py.append(np.interp(px, mrec, mpre)) # precision at mAP@0.5
+
+ # Compute F1 (harmonic mean of precision and recall)
+ f1 = 2 * p * r / (p + r + eps)
+ names = [v for k, v in names.items() if k in unique_classes] # list: only classes that have data
+ names = dict(enumerate(names)) # to dict
+ if plot:
+ plot_pr_curve(px, py, ap, save_dir / f'{prefix}PR_curve.png', names, on_plot=on_plot)
+ plot_mc_curve(px, f1, save_dir / f'{prefix}F1_curve.png', names, ylabel='F1', on_plot=on_plot)
+ plot_mc_curve(px, p, save_dir / f'{prefix}P_curve.png', names, ylabel='Precision', on_plot=on_plot)
+ plot_mc_curve(px, r, save_dir / f'{prefix}R_curve.png', names, ylabel='Recall', on_plot=on_plot)
+
+ i = smooth(f1.mean(0), 0.1).argmax() # max F1 index
+ p, r, f1 = p[:, i], r[:, i], f1[:, i]
+ tp = (r * nt).round() # true positives
+ fp = (tp / (p + eps) - tp).round() # false positives
+ return tp, fp, p, r, f1, ap, unique_classes.astype(int)
+
+
+class Metric(SimpleClass):
+ """
+ Class for computing evaluation metrics for YOLOv8 model.
+
+ Attributes:
+ p (list): Precision for each class. Shape: (nc,).
+ r (list): Recall for each class. Shape: (nc,).
+ f1 (list): F1 score for each class. Shape: (nc,).
+ all_ap (list): AP scores for all classes and all IoU thresholds. Shape: (nc, 10).
+ ap_class_index (list): Index of class for each AP score. Shape: (nc,).
+ nc (int): Number of classes.
+
+ Methods:
+ ap50(): AP at IoU threshold of 0.5 for all classes. Returns: List of AP scores. Shape: (nc,) or [].
+ ap(): AP at IoU thresholds from 0.5 to 0.95 for all classes. Returns: List of AP scores. Shape: (nc,) or [].
+ mp(): Mean precision of all classes. Returns: Float.
+ mr(): Mean recall of all classes. Returns: Float.
+ map50(): Mean AP at IoU threshold of 0.5 for all classes. Returns: Float.
+ map75(): Mean AP at IoU threshold of 0.75 for all classes. Returns: Float.
+ map(): Mean AP at IoU thresholds from 0.5 to 0.95 for all classes. Returns: Float.
+ mean_results(): Mean of results, returns mp, mr, map50, map.
+ class_result(i): Class-aware result, returns p[i], r[i], ap50[i], ap[i].
+ maps(): mAP of each class. Returns: Array of mAP scores, shape: (nc,).
+ fitness(): Model fitness as a weighted combination of metrics. Returns: Float.
+ update(results): Update metric attributes with new evaluation results.
+
+ """
+
+ def __init__(self) -> None:
+ self.p = [] # (nc, )
+ self.r = [] # (nc, )
+ self.f1 = [] # (nc, )
+ self.all_ap = [] # (nc, 10)
+ self.ap_class_index = [] # (nc, )
+ self.nc = 0
+
+ @property
+ def ap50(self):
+ """
+ Returns the Average Precision (AP) at an IoU threshold of 0.5 for all classes.
+
+ Returns:
+ (np.ndarray, list): Array of shape (nc,) with AP50 values per class, or an empty list if not available.
+ """
+ return self.all_ap[:, 0] if len(self.all_ap) else []
+
+ @property
+ def ap(self):
+ """
+ Returns the Average Precision (AP) at an IoU threshold of 0.5-0.95 for all classes.
+
+ Returns:
+ (np.ndarray, list): Array of shape (nc,) with AP50-95 values per class, or an empty list if not available.
+ """
+ return self.all_ap.mean(1) if len(self.all_ap) else []
+
+ @property
+ def mp(self):
+ """
+ Returns the Mean Precision of all classes.
+
+ Returns:
+ (float): The mean precision of all classes.
+ """
+ return self.p.mean() if len(self.p) else 0.0
+
+ @property
+ def mr(self):
+ """
+ Returns the Mean Recall of all classes.
+
+ Returns:
+ (float): The mean recall of all classes.
+ """
+ return self.r.mean() if len(self.r) else 0.0
+
+ @property
+ def map50(self):
+ """
+ Returns the mean Average Precision (mAP) at an IoU threshold of 0.5.
+
+ Returns:
+ (float): The mAP50 at an IoU threshold of 0.5.
+ """
+ return self.all_ap[:, 0].mean() if len(self.all_ap) else 0.0
+
+ @property
+ def map75(self):
+ """
+ Returns the mean Average Precision (mAP) at an IoU threshold of 0.75.
+
+ Returns:
+ (float): The mAP50 at an IoU threshold of 0.75.
+ """
+ return self.all_ap[:, 5].mean() if len(self.all_ap) else 0.0
+
+ @property
+ def map(self):
+ """
+ Returns the mean Average Precision (mAP) over IoU thresholds of 0.5 - 0.95 in steps of 0.05.
+
+ Returns:
+ (float): The mAP over IoU thresholds of 0.5 - 0.95 in steps of 0.05.
+ """
+ return self.all_ap.mean() if len(self.all_ap) else 0.0
+
+ def mean_results(self):
+ """Mean of results, return mp, mr, map50, map."""
+ return [self.mp, self.mr, self.map50, self.map]
+
+ def class_result(self, i):
+ """class-aware result, return p[i], r[i], ap50[i], ap[i]."""
+ return self.p[i], self.r[i], self.ap50[i], self.ap[i]
+
+ @property
+ def maps(self):
+ """mAP of each class."""
+ maps = np.zeros(self.nc) + self.map
+ for i, c in enumerate(self.ap_class_index):
+ maps[c] = self.ap[i]
+ return maps
+
+ def fitness(self):
+ """Model fitness as a weighted combination of metrics."""
+ w = [0.0, 0.0, 0.1, 0.9] # weights for [P, R, mAP@0.5, mAP@0.5:0.95]
+ return (np.array(self.mean_results()) * w).sum()
+
+ def update(self, results):
+ """
+ Args:
+ results (tuple): A tuple of (p, r, ap, f1, ap_class)
+ """
+ self.p, self.r, self.f1, self.all_ap, self.ap_class_index = results
+
+
+class DetMetrics(SimpleClass):
+ """
+ This class is a utility class for computing detection metrics such as precision, recall, and mean average precision
+ (mAP) of an object detection model.
+
+ Args:
+ save_dir (Path): A path to the directory where the output plots will be saved. Defaults to current directory.
+ plot (bool): A flag that indicates whether to plot precision-recall curves for each class. Defaults to False.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered. Defaults to None.
+ names (tuple of str): A tuple of strings that represents the names of the classes. Defaults to an empty tuple.
+
+ Attributes:
+ save_dir (Path): A path to the directory where the output plots will be saved.
+ plot (bool): A flag that indicates whether to plot the precision-recall curves for each class.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered.
+ names (tuple of str): A tuple of strings that represents the names of the classes.
+ box (Metric): An instance of the Metric class for storing the results of the detection metrics.
+ speed (dict): A dictionary for storing the execution time of different parts of the detection process.
+
+ Methods:
+ process(tp, conf, pred_cls, target_cls): Updates the metric results with the latest batch of predictions.
+ keys: Returns a list of keys for accessing the computed detection metrics.
+ mean_results: Returns a list of mean values for the computed detection metrics.
+ class_result(i): Returns a list of values for the computed detection metrics for a specific class.
+ maps: Returns a dictionary of mean average precision (mAP) values for different IoU thresholds.
+ fitness: Computes the fitness score based on the computed detection metrics.
+ ap_class_index: Returns a list of class indices sorted by their average precision (AP) values.
+ results_dict: Returns a dictionary that maps detection metric keys to their computed values.
+ """
+
+ def __init__(self, save_dir=Path('.'), plot=False, on_plot=None, names=()) -> None:
+ self.save_dir = save_dir
+ self.plot = plot
+ self.on_plot = on_plot
+ self.names = names
+ self.box = Metric()
+ self.speed = {'preprocess': 0.0, 'inference': 0.0, 'loss': 0.0, 'postprocess': 0.0}
+
+ def process(self, tp, conf, pred_cls, target_cls):
+ """Process predicted results for object detection and update metrics."""
+ results = ap_per_class(tp,
+ conf,
+ pred_cls,
+ target_cls,
+ plot=self.plot,
+ save_dir=self.save_dir,
+ names=self.names,
+ on_plot=self.on_plot)[2:]
+ self.box.nc = len(self.names)
+ self.box.update(results)
+
+ @property
+ def keys(self):
+ """Returns a list of keys for accessing specific metrics."""
+ return ['metrics/precision(B)', 'metrics/recall(B)', 'metrics/mAP50(B)', 'metrics/mAP50-95(B)']
+
+ def mean_results(self):
+ """Calculate mean of detected objects & return precision, recall, mAP50, and mAP50-95."""
+ return self.box.mean_results()
+
+ def class_result(self, i):
+ """Return the result of evaluating the performance of an object detection model on a specific class."""
+ return self.box.class_result(i)
+
+ @property
+ def maps(self):
+ """Returns mean Average Precision (mAP) scores per class."""
+ return self.box.maps
+
+ @property
+ def fitness(self):
+ """Returns the fitness of box object."""
+ return self.box.fitness()
+
+ @property
+ def ap_class_index(self):
+ """Returns the average precision index per class."""
+ return self.box.ap_class_index
+
+ @property
+ def results_dict(self):
+ """Returns dictionary of computed performance metrics and statistics."""
+ return dict(zip(self.keys + ['fitness'], self.mean_results() + [self.fitness]))
+
+
+class SegmentMetrics(SimpleClass):
+ """
+ Calculates and aggregates detection and segmentation metrics over a given set of classes.
+
+ Args:
+ save_dir (Path): Path to the directory where the output plots should be saved. Default is the current directory.
+ plot (bool): Whether to save the detection and segmentation plots. Default is False.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered. Defaults to None.
+ names (list): List of class names. Default is an empty list.
+
+ Attributes:
+ save_dir (Path): Path to the directory where the output plots should be saved.
+ plot (bool): Whether to save the detection and segmentation plots.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered.
+ names (list): List of class names.
+ box (Metric): An instance of the Metric class to calculate box detection metrics.
+ seg (Metric): An instance of the Metric class to calculate mask segmentation metrics.
+ speed (dict): Dictionary to store the time taken in different phases of inference.
+
+ Methods:
+ process(tp_m, tp_b, conf, pred_cls, target_cls): Processes metrics over the given set of predictions.
+ mean_results(): Returns the mean of the detection and segmentation metrics over all the classes.
+ class_result(i): Returns the detection and segmentation metrics of class `i`.
+ maps: Returns the mean Average Precision (mAP) scores for IoU thresholds ranging from 0.50 to 0.95.
+ fitness: Returns the fitness scores, which are a single weighted combination of metrics.
+ ap_class_index: Returns the list of indices of classes used to compute Average Precision (AP).
+ results_dict: Returns the dictionary containing all the detection and segmentation metrics and fitness score.
+ """
+
+ def __init__(self, save_dir=Path('.'), plot=False, on_plot=None, names=()) -> None:
+ self.save_dir = save_dir
+ self.plot = plot
+ self.on_plot = on_plot
+ self.names = names
+ self.box = Metric()
+ self.seg = Metric()
+ self.speed = {'preprocess': 0.0, 'inference': 0.0, 'loss': 0.0, 'postprocess': 0.0}
+
+ def process(self, tp_b, tp_m, conf, pred_cls, target_cls):
+ """
+ Processes the detection and segmentation metrics over the given set of predictions.
+
+ Args:
+ tp_b (list): List of True Positive boxes.
+ tp_m (list): List of True Positive masks.
+ conf (list): List of confidence scores.
+ pred_cls (list): List of predicted classes.
+ target_cls (list): List of target classes.
+ """
+
+ results_mask = ap_per_class(tp_m,
+ conf,
+ pred_cls,
+ target_cls,
+ plot=self.plot,
+ on_plot=self.on_plot,
+ save_dir=self.save_dir,
+ names=self.names,
+ prefix='Mask')[2:]
+ self.seg.nc = len(self.names)
+ self.seg.update(results_mask)
+ results_box = ap_per_class(tp_b,
+ conf,
+ pred_cls,
+ target_cls,
+ plot=self.plot,
+ on_plot=self.on_plot,
+ save_dir=self.save_dir,
+ names=self.names,
+ prefix='Box')[2:]
+ self.box.nc = len(self.names)
+ self.box.update(results_box)
+
+ @property
+ def keys(self):
+ """Returns a list of keys for accessing metrics."""
+ return [
+ 'metrics/precision(B)', 'metrics/recall(B)', 'metrics/mAP50(B)', 'metrics/mAP50-95(B)',
+ 'metrics/precision(M)', 'metrics/recall(M)', 'metrics/mAP50(M)', 'metrics/mAP50-95(M)']
+
+ def mean_results(self):
+ """Return the mean metrics for bounding box and segmentation results."""
+ return self.box.mean_results() + self.seg.mean_results()
+
+ def class_result(self, i):
+ """Returns classification results for a specified class index."""
+ return self.box.class_result(i) + self.seg.class_result(i)
+
+ @property
+ def maps(self):
+ """Returns mAP scores for object detection and semantic segmentation models."""
+ return self.box.maps + self.seg.maps
+
+ @property
+ def fitness(self):
+ """Get the fitness score for both segmentation and bounding box models."""
+ return self.seg.fitness() + self.box.fitness()
+
+ @property
+ def ap_class_index(self):
+ """Boxes and masks have the same ap_class_index."""
+ return self.box.ap_class_index
+
+ @property
+ def results_dict(self):
+ """Returns results of object detection model for evaluation."""
+ return dict(zip(self.keys + ['fitness'], self.mean_results() + [self.fitness]))
+
+
+class PoseMetrics(SegmentMetrics):
+ """
+ Calculates and aggregates detection and pose metrics over a given set of classes.
+
+ Args:
+ save_dir (Path): Path to the directory where the output plots should be saved. Default is the current directory.
+ plot (bool): Whether to save the detection and segmentation plots. Default is False.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered. Defaults to None.
+ names (list): List of class names. Default is an empty list.
+
+ Attributes:
+ save_dir (Path): Path to the directory where the output plots should be saved.
+ plot (bool): Whether to save the detection and segmentation plots.
+ on_plot (func): An optional callback to pass plots path and data when they are rendered.
+ names (list): List of class names.
+ box (Metric): An instance of the Metric class to calculate box detection metrics.
+ pose (Metric): An instance of the Metric class to calculate mask segmentation metrics.
+ speed (dict): Dictionary to store the time taken in different phases of inference.
+
+ Methods:
+ process(tp_m, tp_b, conf, pred_cls, target_cls): Processes metrics over the given set of predictions.
+ mean_results(): Returns the mean of the detection and segmentation metrics over all the classes.
+ class_result(i): Returns the detection and segmentation metrics of class `i`.
+ maps: Returns the mean Average Precision (mAP) scores for IoU thresholds ranging from 0.50 to 0.95.
+ fitness: Returns the fitness scores, which are a single weighted combination of metrics.
+ ap_class_index: Returns the list of indices of classes used to compute Average Precision (AP).
+ results_dict: Returns the dictionary containing all the detection and segmentation metrics and fitness score.
+ """
+
+ def __init__(self, save_dir=Path('.'), plot=False, on_plot=None, names=()) -> None:
+ super().__init__(save_dir, plot, names)
+ self.save_dir = save_dir
+ self.plot = plot
+ self.on_plot = on_plot
+ self.names = names
+ self.box = Metric()
+ self.pose = Metric()
+ self.speed = {'preprocess': 0.0, 'inference': 0.0, 'loss': 0.0, 'postprocess': 0.0}
+
+ def __getattr__(self, attr):
+ """Raises an AttributeError if an invalid attribute is accessed."""
+ name = self.__class__.__name__
+ raise AttributeError(f"'{name}' object has no attribute '{attr}'. See valid attributes below.\n{self.__doc__}")
+
+ def process(self, tp_b, tp_p, conf, pred_cls, target_cls):
+ """
+ Processes the detection and pose metrics over the given set of predictions.
+
+ Args:
+ tp_b (list): List of True Positive boxes.
+ tp_p (list): List of True Positive keypoints.
+ conf (list): List of confidence scores.
+ pred_cls (list): List of predicted classes.
+ target_cls (list): List of target classes.
+ """
+
+ results_pose = ap_per_class(tp_p,
+ conf,
+ pred_cls,
+ target_cls,
+ plot=self.plot,
+ on_plot=self.on_plot,
+ save_dir=self.save_dir,
+ names=self.names,
+ prefix='Pose')[2:]
+ self.pose.nc = len(self.names)
+ self.pose.update(results_pose)
+ results_box = ap_per_class(tp_b,
+ conf,
+ pred_cls,
+ target_cls,
+ plot=self.plot,
+ on_plot=self.on_plot,
+ save_dir=self.save_dir,
+ names=self.names,
+ prefix='Box')[2:]
+ self.box.nc = len(self.names)
+ self.box.update(results_box)
+
+ @property
+ def keys(self):
+ """Returns list of evaluation metric keys."""
+ return [
+ 'metrics/precision(B)', 'metrics/recall(B)', 'metrics/mAP50(B)', 'metrics/mAP50-95(B)',
+ 'metrics/precision(P)', 'metrics/recall(P)', 'metrics/mAP50(P)', 'metrics/mAP50-95(P)']
+
+ def mean_results(self):
+ """Return the mean results of box and pose."""
+ return self.box.mean_results() + self.pose.mean_results()
+
+ def class_result(self, i):
+ """Return the class-wise detection results for a specific class i."""
+ return self.box.class_result(i) + self.pose.class_result(i)
+
+ @property
+ def maps(self):
+ """Returns the mean average precision (mAP) per class for both box and pose detections."""
+ return self.box.maps + self.pose.maps
+
+ @property
+ def fitness(self):
+ """Computes classification metrics and speed using the `targets` and `pred` inputs."""
+ return self.pose.fitness() + self.box.fitness()
+
+
+class ClassifyMetrics(SimpleClass):
+ """
+ Class for computing classification metrics including top-1 and top-5 accuracy.
+
+ Attributes:
+ top1 (float): The top-1 accuracy.
+ top5 (float): The top-5 accuracy.
+ speed (Dict[str, float]): A dictionary containing the time taken for each step in the pipeline.
+
+ Properties:
+ fitness (float): The fitness of the model, which is equal to top-5 accuracy.
+ results_dict (Dict[str, Union[float, str]]): A dictionary containing the classification metrics and fitness.
+ keys (List[str]): A list of keys for the results_dict.
+
+ Methods:
+ process(targets, pred): Processes the targets and predictions to compute classification metrics.
+ """
+
+ def __init__(self) -> None:
+ self.top1 = 0
+ self.top5 = 0
+ self.speed = {'preprocess': 0.0, 'inference': 0.0, 'loss': 0.0, 'postprocess': 0.0}
+
+ def process(self, targets, pred):
+ """Target classes and predicted classes."""
+ pred, targets = torch.cat(pred), torch.cat(targets)
+ correct = (targets[:, None] == pred).float()
+ acc = torch.stack((correct[:, 0], correct.max(1).values), dim=1) # (top1, top5) accuracy
+ self.top1, self.top5 = acc.mean(0).tolist()
+
+ @property
+ def fitness(self):
+ """Returns top-5 accuracy as fitness score."""
+ return self.top5
+
+ @property
+ def results_dict(self):
+ """Returns a dictionary with model's performance metrics and fitness score."""
+ return dict(zip(self.keys + ['fitness'], [self.top1, self.top5, self.fitness]))
+
+ @property
+ def keys(self):
+ """Returns a list of keys for the results_dict property."""
+ return ['metrics/accuracy_top1', 'metrics/accuracy_top5']
diff --git a/yolov8/ultralytics/yolo/utils/ops.py b/yolov8/ultralytics/yolo/utils/ops.py
new file mode 100644
index 0000000000000000000000000000000000000000..9135117ff17c7265b8543d5e760c7f37d46c3c16
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/ops.py
@@ -0,0 +1,710 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+import math
+import re
+import time
+
+import cv2
+import numpy as np
+import torch
+import torch.nn.functional as F
+import torchvision
+
+from ultralytics.yolo.utils import LOGGER
+
+from .metrics import box_iou
+
+
+class Profile(contextlib.ContextDecorator):
+ """
+ YOLOv8 Profile class.
+ Usage: as a decorator with @Profile() or as a context manager with 'with Profile():'
+ """
+
+ def __init__(self, t=0.0):
+ """
+ Initialize the Profile class.
+
+ Args:
+ t (float): Initial time. Defaults to 0.0.
+ """
+ self.t = t
+ self.cuda = torch.cuda.is_available()
+
+ def __enter__(self):
+ """
+ Start timing.
+ """
+ self.start = self.time()
+ return self
+
+ def __exit__(self, type, value, traceback):
+ """
+ Stop timing.
+ """
+ self.dt = self.time() - self.start # delta-time
+ self.t += self.dt # accumulate dt
+
+ def time(self):
+ """
+ Get current time.
+ """
+ if self.cuda:
+ torch.cuda.synchronize()
+ return time.time()
+
+
+def coco80_to_coco91_class(): # converts 80-index (val2014) to 91-index (paper)
+ # https://tech.amikelive.com/node-718/what-object-categories-labels-are-in-coco-dataset/
+ # a = np.loadtxt('data/coco.names', dtype='str', delimiter='\n')
+ # b = np.loadtxt('data/coco_paper.names', dtype='str', delimiter='\n')
+ # x1 = [list(a[i] == b).index(True) + 1 for i in range(80)] # darknet to coco
+ # x2 = [list(b[i] == a).index(True) if any(b[i] == a) else None for i in range(91)] # coco to darknet
+ return [
+ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 27, 28, 31, 32, 33, 34,
+ 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63,
+ 64, 65, 67, 70, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 84, 85, 86, 87, 88, 89, 90]
+
+
+def segment2box(segment, width=640, height=640):
+ """
+ Convert 1 segment label to 1 box label, applying inside-image constraint, i.e. (xy1, xy2, ...) to (xyxy)
+
+ Args:
+ segment (torch.Tensor): the segment label
+ width (int): the width of the image. Defaults to 640
+ height (int): The height of the image. Defaults to 640
+
+ Returns:
+ (np.ndarray): the minimum and maximum x and y values of the segment.
+ """
+ # Convert 1 segment label to 1 box label, applying inside-image constraint, i.e. (xy1, xy2, ...) to (xyxy)
+ x, y = segment.T # segment xy
+ inside = (x >= 0) & (y >= 0) & (x <= width) & (y <= height)
+ x, y, = x[inside], y[inside]
+ return np.array([x.min(), y.min(), x.max(), y.max()], dtype=segment.dtype) if any(x) else np.zeros(
+ 4, dtype=segment.dtype) # xyxy
+
+
+def scale_boxes(img1_shape, boxes, img0_shape, ratio_pad=None):
+ """
+ Rescales bounding boxes (in the format of xyxy) from the shape of the image they were originally specified in
+ (img1_shape) to the shape of a different image (img0_shape).
+
+ Args:
+ img1_shape (tuple): The shape of the image that the bounding boxes are for, in the format of (height, width).
+ boxes (torch.Tensor): the bounding boxes of the objects in the image, in the format of (x1, y1, x2, y2)
+ img0_shape (tuple): the shape of the target image, in the format of (height, width).
+ ratio_pad (tuple): a tuple of (ratio, pad) for scaling the boxes. If not provided, the ratio and pad will be
+ calculated based on the size difference between the two images.
+
+ Returns:
+ boxes (torch.Tensor): The scaled bounding boxes, in the format of (x1, y1, x2, y2)
+ """
+ if ratio_pad is None: # calculate from img0_shape
+ gain = min(img1_shape[0] / img0_shape[0], img1_shape[1] / img0_shape[1]) # gain = old / new
+ pad = round((img1_shape[1] - img0_shape[1] * gain) / 2 - 0.1), round(
+ (img1_shape[0] - img0_shape[0] * gain) / 2 - 0.1) # wh padding
+ else:
+ gain = ratio_pad[0][0]
+ pad = ratio_pad[1]
+
+ boxes[..., [0, 2]] -= pad[0] # x padding
+ boxes[..., [1, 3]] -= pad[1] # y padding
+ boxes[..., :4] /= gain
+ clip_boxes(boxes, img0_shape)
+ return boxes
+
+
+def make_divisible(x, divisor):
+ """
+ Returns the nearest number that is divisible by the given divisor.
+
+ Args:
+ x (int): The number to make divisible.
+ divisor (int | torch.Tensor): The divisor.
+
+ Returns:
+ (int): The nearest number divisible by the divisor.
+ """
+ if isinstance(divisor, torch.Tensor):
+ divisor = int(divisor.max()) # to int
+ return math.ceil(x / divisor) * divisor
+
+
+def non_max_suppression(
+ prediction,
+ conf_thres=0.25,
+ iou_thres=0.45,
+ classes=None,
+ agnostic=False,
+ multi_label=False,
+ labels=(),
+ max_det=300,
+ nc=0, # number of classes (optional)
+ max_time_img=0.05,
+ max_nms=30000,
+ max_wh=7680,
+):
+ """
+ Perform non-maximum suppression (NMS) on a set of boxes, with support for masks and multiple labels per box.
+
+ Arguments:
+ prediction (torch.Tensor): A tensor of shape (batch_size, num_classes + 4 + num_masks, num_boxes)
+ containing the predicted boxes, classes, and masks. The tensor should be in the format
+ output by a model, such as YOLO.
+ conf_thres (float): The confidence threshold below which boxes will be filtered out.
+ Valid values are between 0.0 and 1.0.
+ iou_thres (float): The IoU threshold below which boxes will be filtered out during NMS.
+ Valid values are between 0.0 and 1.0.
+ classes (List[int]): A list of class indices to consider. If None, all classes will be considered.
+ agnostic (bool): If True, the model is agnostic to the number of classes, and all
+ classes will be considered as one.
+ multi_label (bool): If True, each box may have multiple labels.
+ labels (List[List[Union[int, float, torch.Tensor]]]): A list of lists, where each inner
+ list contains the apriori labels for a given image. The list should be in the format
+ output by a dataloader, with each label being a tuple of (class_index, x1, y1, x2, y2).
+ max_det (int): The maximum number of boxes to keep after NMS.
+ nc (int, optional): The number of classes output by the model. Any indices after this will be considered masks.
+ max_time_img (float): The maximum time (seconds) for processing one image.
+ max_nms (int): The maximum number of boxes into torchvision.ops.nms().
+ max_wh (int): The maximum box width and height in pixels
+
+ Returns:
+ (List[torch.Tensor]): A list of length batch_size, where each element is a tensor of
+ shape (num_boxes, 6 + num_masks) containing the kept boxes, with columns
+ (x1, y1, x2, y2, confidence, class, mask1, mask2, ...).
+ """
+
+ # Checks
+ assert 0 <= conf_thres <= 1, f'Invalid Confidence threshold {conf_thres}, valid values are between 0.0 and 1.0'
+ assert 0 <= iou_thres <= 1, f'Invalid IoU {iou_thres}, valid values are between 0.0 and 1.0'
+ if isinstance(prediction, (list, tuple)): # YOLOv8 model in validation model, output = (inference_out, loss_out)
+ prediction = prediction[0] # select only inference output
+
+ device = prediction.device
+ mps = 'mps' in device.type # Apple MPS
+ if mps: # MPS not fully supported yet, convert tensors to CPU before NMS
+ prediction = prediction.cpu()
+ bs = prediction.shape[0] # batch size
+ nc = nc or (prediction.shape[1] - 4) # number of classes
+ nm = prediction.shape[1] - nc - 4
+ mi = 4 + nc # mask start index
+ xc = prediction[:, 4:mi].amax(1) > conf_thres # candidates
+
+ # Settings
+ # min_wh = 2 # (pixels) minimum box width and height
+ time_limit = 0.5 + max_time_img * bs # seconds to quit after
+ redundant = True # require redundant detections
+ multi_label &= nc > 1 # multiple labels per box (adds 0.5ms/img)
+ merge = False # use merge-NMS
+
+ prediction = prediction.transpose(-1, -2) # shape(1,84,6300) to shape(1,6300,84)
+ prediction[..., :4] = xywh2xyxy(prediction[..., :4]) # xywh to xyxy
+
+ t = time.time()
+ output = [torch.zeros((0, 6 + nm), device=prediction.device)] * bs
+ for xi, x in enumerate(prediction): # image index, image inference
+ # Apply constraints
+ # x[((x[:, 2:4] < min_wh) | (x[:, 2:4] > max_wh)).any(1), 4] = 0 # width-height
+ x = x[xc[xi]] # confidence
+
+ # Cat apriori labels if autolabelling
+ if labels and len(labels[xi]):
+ lb = labels[xi]
+ v = torch.zeros((len(lb), nc + nm + 5), device=x.device)
+ v[:, :4] = lb[:, 1:5] # box
+ v[range(len(lb)), lb[:, 0].long() + 4] = 1.0 # cls
+ x = torch.cat((x, v), 0)
+
+ # If none remain process next image
+ if not x.shape[0]:
+ continue
+
+ # Detections matrix nx6 (xyxy, conf, cls)
+ box, cls, mask = x.split((4, nc, nm), 1)
+
+ if multi_label:
+ i, j = torch.where(cls > conf_thres)
+ x = torch.cat((box[i], x[i, 4 + j, None], j[:, None].float(), mask[i]), 1)
+ else: # best class only
+ conf, j = cls.max(1, keepdim=True)
+ x = torch.cat((box, conf, j.float(), mask), 1)[conf.view(-1) > conf_thres]
+
+ # Filter by class
+ if classes is not None:
+ x = x[(x[:, 5:6] == torch.tensor(classes, device=x.device)).any(1)]
+
+ # Apply finite constraint
+ # if not torch.isfinite(x).all():
+ # x = x[torch.isfinite(x).all(1)]
+
+ # Check shape
+ n = x.shape[0] # number of boxes
+ if not n: # no boxes
+ continue
+ if n > max_nms: # excess boxes
+ x = x[x[:, 4].argsort(descending=True)[:max_nms]] # sort by confidence and remove excess boxes
+
+ # Batched NMS
+ c = x[:, 5:6] * (0 if agnostic else max_wh) # classes
+ boxes, scores = x[:, :4] + c, x[:, 4] # boxes (offset by class), scores
+ i = torchvision.ops.nms(boxes, scores, iou_thres) # NMS
+ i = i[:max_det] # limit detections
+ if merge and (1 < n < 3E3): # Merge NMS (boxes merged using weighted mean)
+ # Update boxes as boxes(i,4) = weights(i,n) * boxes(n,4)
+ iou = box_iou(boxes[i], boxes) > iou_thres # iou matrix
+ weights = iou * scores[None] # box weights
+ x[i, :4] = torch.mm(weights, x[:, :4]).float() / weights.sum(1, keepdim=True) # merged boxes
+ if redundant:
+ i = i[iou.sum(1) > 1] # require redundancy
+
+ output[xi] = x[i]
+ if mps:
+ output[xi] = output[xi].to(device)
+ if (time.time() - t) > time_limit:
+ LOGGER.warning(f'WARNING ⚠️ NMS time limit {time_limit:.3f}s exceeded')
+ break # time limit exceeded
+
+ return output
+
+
+def clip_boxes(boxes, shape):
+ """
+ It takes a list of bounding boxes and a shape (height, width) and clips the bounding boxes to the
+ shape
+
+ Args:
+ boxes (torch.Tensor): the bounding boxes to clip
+ shape (tuple): the shape of the image
+ """
+ if isinstance(boxes, torch.Tensor): # faster individually
+ boxes[..., 0].clamp_(0, shape[1]) # x1
+ boxes[..., 1].clamp_(0, shape[0]) # y1
+ boxes[..., 2].clamp_(0, shape[1]) # x2
+ boxes[..., 3].clamp_(0, shape[0]) # y2
+ else: # np.array (faster grouped)
+ boxes[..., [0, 2]] = boxes[..., [0, 2]].clip(0, shape[1]) # x1, x2
+ boxes[..., [1, 3]] = boxes[..., [1, 3]].clip(0, shape[0]) # y1, y2
+
+
+def clip_coords(coords, shape):
+ """
+ Clip line coordinates to the image boundaries.
+
+ Args:
+ coords (torch.Tensor | numpy.ndarray): A list of line coordinates.
+ shape (tuple): A tuple of integers representing the size of the image in the format (height, width).
+
+ Returns:
+ (None): The function modifies the input `coordinates` in place, by clipping each coordinate to the image boundaries.
+ """
+ if isinstance(coords, torch.Tensor): # faster individually
+ coords[..., 0].clamp_(0, shape[1]) # x
+ coords[..., 1].clamp_(0, shape[0]) # y
+ else: # np.array (faster grouped)
+ coords[..., 0] = coords[..., 0].clip(0, shape[1]) # x
+ coords[..., 1] = coords[..., 1].clip(0, shape[0]) # y
+
+
+def scale_image(masks, im0_shape, ratio_pad=None):
+ """
+ Takes a mask, and resizes it to the original image size
+
+ Args:
+ masks (torch.Tensor): resized and padded masks/images, [h, w, num]/[h, w, 3].
+ im0_shape (tuple): the original image shape
+ ratio_pad (tuple): the ratio of the padding to the original image.
+
+ Returns:
+ masks (torch.Tensor): The masks that are being returned.
+ """
+ # Rescale coordinates (xyxy) from im1_shape to im0_shape
+ im1_shape = masks.shape
+ if im1_shape[:2] == im0_shape[:2]:
+ return masks
+ if ratio_pad is None: # calculate from im0_shape
+ gain = min(im1_shape[0] / im0_shape[0], im1_shape[1] / im0_shape[1]) # gain = old / new
+ pad = (im1_shape[1] - im0_shape[1] * gain) / 2, (im1_shape[0] - im0_shape[0] * gain) / 2 # wh padding
+ else:
+ gain = ratio_pad[0][0]
+ pad = ratio_pad[1]
+ top, left = int(pad[1]), int(pad[0]) # y, x
+ bottom, right = int(im1_shape[0] - pad[1]), int(im1_shape[1] - pad[0])
+
+ if len(masks.shape) < 2:
+ raise ValueError(f'"len of masks shape" should be 2 or 3, but got {len(masks.shape)}')
+ masks = masks[top:bottom, left:right]
+ # masks = masks.permute(2, 0, 1).contiguous()
+ # masks = F.interpolate(masks[None], im0_shape[:2], mode='bilinear', align_corners=False)[0]
+ # masks = masks.permute(1, 2, 0).contiguous()
+ masks = cv2.resize(masks, (im0_shape[1], im0_shape[0]))
+ if len(masks.shape) == 2:
+ masks = masks[:, :, None]
+
+ return masks
+
+
+def xyxy2xywh(x):
+ """
+ Convert bounding box coordinates from (x1, y1, x2, y2) format to (x, y, width, height) format.
+
+ Args:
+ x (np.ndarray | torch.Tensor): The input bounding box coordinates in (x1, y1, x2, y2) format.
+ Returns:
+ y (np.ndarray | torch.Tensor): The bounding box coordinates in (x, y, width, height) format.
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[..., 0] = (x[..., 0] + x[..., 2]) / 2 # x center
+ y[..., 1] = (x[..., 1] + x[..., 3]) / 2 # y center
+ y[..., 2] = x[..., 2] - x[..., 0] # width
+ y[..., 3] = x[..., 3] - x[..., 1] # height
+ return y
+
+
+def xywh2xyxy(x):
+ """
+ Convert bounding box coordinates from (x, y, width, height) format to (x1, y1, x2, y2) format where (x1, y1) is the
+ top-left corner and (x2, y2) is the bottom-right corner.
+
+ Args:
+ x (np.ndarray | torch.Tensor): The input bounding box coordinates in (x, y, width, height) format.
+ Returns:
+ y (np.ndarray | torch.Tensor): The bounding box coordinates in (x1, y1, x2, y2) format.
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[..., 0] = x[..., 0] - x[..., 2] / 2 # top left x
+ y[..., 1] = x[..., 1] - x[..., 3] / 2 # top left y
+ y[..., 2] = x[..., 0] + x[..., 2] / 2 # bottom right x
+ y[..., 3] = x[..., 1] + x[..., 3] / 2 # bottom right y
+ return y
+
+
+def xywhn2xyxy(x, w=640, h=640, padw=0, padh=0):
+ """
+ Convert normalized bounding box coordinates to pixel coordinates.
+
+ Args:
+ x (np.ndarray | torch.Tensor): The bounding box coordinates.
+ w (int): Width of the image. Defaults to 640
+ h (int): Height of the image. Defaults to 640
+ padw (int): Padding width. Defaults to 0
+ padh (int): Padding height. Defaults to 0
+ Returns:
+ y (np.ndarray | torch.Tensor): The coordinates of the bounding box in the format [x1, y1, x2, y2] where
+ x1,y1 is the top-left corner, x2,y2 is the bottom-right corner of the bounding box.
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[..., 0] = w * (x[..., 0] - x[..., 2] / 2) + padw # top left x
+ y[..., 1] = h * (x[..., 1] - x[..., 3] / 2) + padh # top left y
+ y[..., 2] = w * (x[..., 0] + x[..., 2] / 2) + padw # bottom right x
+ y[..., 3] = h * (x[..., 1] + x[..., 3] / 2) + padh # bottom right y
+ return y
+
+
+def xyxy2xywhn(x, w=640, h=640, clip=False, eps=0.0):
+ """
+ Convert bounding box coordinates from (x1, y1, x2, y2) format to (x, y, width, height, normalized) format.
+ x, y, width and height are normalized to image dimensions
+
+ Args:
+ x (np.ndarray | torch.Tensor): The input bounding box coordinates in (x1, y1, x2, y2) format.
+ w (int): The width of the image. Defaults to 640
+ h (int): The height of the image. Defaults to 640
+ clip (bool): If True, the boxes will be clipped to the image boundaries. Defaults to False
+ eps (float): The minimum value of the box's width and height. Defaults to 0.0
+ Returns:
+ y (np.ndarray | torch.Tensor): The bounding box coordinates in (x, y, width, height, normalized) format
+ """
+ if clip:
+ clip_boxes(x, (h - eps, w - eps)) # warning: inplace clip
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[..., 0] = ((x[..., 0] + x[..., 2]) / 2) / w # x center
+ y[..., 1] = ((x[..., 1] + x[..., 3]) / 2) / h # y center
+ y[..., 2] = (x[..., 2] - x[..., 0]) / w # width
+ y[..., 3] = (x[..., 3] - x[..., 1]) / h # height
+ return y
+
+
+def xyn2xy(x, w=640, h=640, padw=0, padh=0):
+ """
+ Convert normalized coordinates to pixel coordinates of shape (n,2)
+
+ Args:
+ x (np.ndarray | torch.Tensor): The input tensor of normalized bounding box coordinates
+ w (int): The width of the image. Defaults to 640
+ h (int): The height of the image. Defaults to 640
+ padw (int): The width of the padding. Defaults to 0
+ padh (int): The height of the padding. Defaults to 0
+ Returns:
+ y (np.ndarray | torch.Tensor): The x and y coordinates of the top left corner of the bounding box
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[..., 0] = w * x[..., 0] + padw # top left x
+ y[..., 1] = h * x[..., 1] + padh # top left y
+ return y
+
+
+def xywh2ltwh(x):
+ """
+ Convert the bounding box format from [x, y, w, h] to [x1, y1, w, h], where x1, y1 are the top-left coordinates.
+
+ Args:
+ x (np.ndarray | torch.Tensor): The input tensor with the bounding box coordinates in the xywh format
+ Returns:
+ y (np.ndarray | torch.Tensor): The bounding box coordinates in the xyltwh format
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[:, 0] = x[:, 0] - x[:, 2] / 2 # top left x
+ y[:, 1] = x[:, 1] - x[:, 3] / 2 # top left y
+ return y
+
+
+def xyxy2ltwh(x):
+ """
+ Convert nx4 bounding boxes from [x1, y1, x2, y2] to [x1, y1, w, h], where xy1=top-left, xy2=bottom-right
+
+ Args:
+ x (np.ndarray | torch.Tensor): The input tensor with the bounding boxes coordinates in the xyxy format
+ Returns:
+ y (np.ndarray | torch.Tensor): The bounding box coordinates in the xyltwh format.
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[:, 2] = x[:, 2] - x[:, 0] # width
+ y[:, 3] = x[:, 3] - x[:, 1] # height
+ return y
+
+
+def ltwh2xywh(x):
+ """
+ Convert nx4 boxes from [x1, y1, w, h] to [x, y, w, h] where xy1=top-left, xy=center
+
+ Args:
+ x (torch.Tensor): the input tensor
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[:, 0] = x[:, 0] + x[:, 2] / 2 # center x
+ y[:, 1] = x[:, 1] + x[:, 3] / 2 # center y
+ return y
+
+
+def ltwh2xyxy(x):
+ """
+ It converts the bounding box from [x1, y1, w, h] to [x1, y1, x2, y2] where xy1=top-left, xy2=bottom-right
+
+ Args:
+ x (np.ndarray | torch.Tensor): the input image
+
+ Returns:
+ y (np.ndarray | torch.Tensor): the xyxy coordinates of the bounding boxes.
+ """
+ y = x.clone() if isinstance(x, torch.Tensor) else np.copy(x)
+ y[:, 2] = x[:, 2] + x[:, 0] # width
+ y[:, 3] = x[:, 3] + x[:, 1] # height
+ return y
+
+
+def segments2boxes(segments):
+ """
+ It converts segment labels to box labels, i.e. (cls, xy1, xy2, ...) to (cls, xywh)
+
+ Args:
+ segments (list): list of segments, each segment is a list of points, each point is a list of x, y coordinates
+
+ Returns:
+ (np.ndarray): the xywh coordinates of the bounding boxes.
+ """
+ boxes = []
+ for s in segments:
+ x, y = s.T # segment xy
+ boxes.append([x.min(), y.min(), x.max(), y.max()]) # cls, xyxy
+ return xyxy2xywh(np.array(boxes)) # cls, xywh
+
+
+def resample_segments(segments, n=1000):
+ """
+ Inputs a list of segments (n,2) and returns a list of segments (n,2) up-sampled to n points each.
+
+ Args:
+ segments (list): a list of (n,2) arrays, where n is the number of points in the segment.
+ n (int): number of points to resample the segment to. Defaults to 1000
+
+ Returns:
+ segments (list): the resampled segments.
+ """
+ for i, s in enumerate(segments):
+ s = np.concatenate((s, s[0:1, :]), axis=0)
+ x = np.linspace(0, len(s) - 1, n)
+ xp = np.arange(len(s))
+ segments[i] = np.concatenate([np.interp(x, xp, s[:, i]) for i in range(2)],
+ dtype=np.float32).reshape(2, -1).T # segment xy
+ return segments
+
+
+def crop_mask(masks, boxes):
+ """
+ It takes a mask and a bounding box, and returns a mask that is cropped to the bounding box
+
+ Args:
+ masks (torch.Tensor): [h, w, n] tensor of masks
+ boxes (torch.Tensor): [n, 4] tensor of bbox coordinates in relative point form
+
+ Returns:
+ (torch.Tensor): The masks are being cropped to the bounding box.
+ """
+ n, h, w = masks.shape
+ x1, y1, x2, y2 = torch.chunk(boxes[:, :, None], 4, 1) # x1 shape(n,1,1)
+ r = torch.arange(w, device=masks.device, dtype=x1.dtype)[None, None, :] # rows shape(1,1,w)
+ c = torch.arange(h, device=masks.device, dtype=x1.dtype)[None, :, None] # cols shape(1,h,1)
+
+ return masks * ((r >= x1) * (r < x2) * (c >= y1) * (c < y2))
+
+
+def process_mask_upsample(protos, masks_in, bboxes, shape):
+ """
+ It takes the output of the mask head, and applies the mask to the bounding boxes. This produces masks of higher
+ quality but is slower.
+
+ Args:
+ protos (torch.Tensor): [mask_dim, mask_h, mask_w]
+ masks_in (torch.Tensor): [n, mask_dim], n is number of masks after nms
+ bboxes (torch.Tensor): [n, 4], n is number of masks after nms
+ shape (tuple): the size of the input image (h,w)
+
+ Returns:
+ (torch.Tensor): The upsampled masks.
+ """
+ c, mh, mw = protos.shape # CHW
+ masks = (masks_in @ protos.float().view(c, -1)).sigmoid().view(-1, mh, mw)
+ masks = F.interpolate(masks[None], shape, mode='bilinear', align_corners=False)[0] # CHW
+ masks = crop_mask(masks, bboxes) # CHW
+ return masks.gt_(0.5)
+
+
+def process_mask(protos, masks_in, bboxes, shape, upsample=False):
+ """
+ Apply masks to bounding boxes using the output of the mask head.
+
+ Args:
+ protos (torch.Tensor): A tensor of shape [mask_dim, mask_h, mask_w].
+ masks_in (torch.Tensor): A tensor of shape [n, mask_dim], where n is the number of masks after NMS.
+ bboxes (torch.Tensor): A tensor of shape [n, 4], where n is the number of masks after NMS.
+ shape (tuple): A tuple of integers representing the size of the input image in the format (h, w).
+ upsample (bool): A flag to indicate whether to upsample the mask to the original image size. Default is False.
+
+ Returns:
+ (torch.Tensor): A binary mask tensor of shape [n, h, w], where n is the number of masks after NMS, and h and w
+ are the height and width of the input image. The mask is applied to the bounding boxes.
+ """
+
+ c, mh, mw = protos.shape # CHW
+ ih, iw = shape
+ masks = (masks_in @ protos.float().view(c, -1)).sigmoid().view(-1, mh, mw) # CHW
+
+ downsampled_bboxes = bboxes.clone()
+ downsampled_bboxes[:, 0] *= mw / iw
+ downsampled_bboxes[:, 2] *= mw / iw
+ downsampled_bboxes[:, 3] *= mh / ih
+ downsampled_bboxes[:, 1] *= mh / ih
+
+ masks = crop_mask(masks, downsampled_bboxes) # CHW
+ if upsample:
+ masks = F.interpolate(masks[None], shape, mode='bilinear', align_corners=False)[0] # CHW
+ return masks.gt_(0.5)
+
+
+def process_mask_native(protos, masks_in, bboxes, shape):
+ """
+ It takes the output of the mask head, and crops it after upsampling to the bounding boxes.
+
+ Args:
+ protos (torch.Tensor): [mask_dim, mask_h, mask_w]
+ masks_in (torch.Tensor): [n, mask_dim], n is number of masks after nms
+ bboxes (torch.Tensor): [n, 4], n is number of masks after nms
+ shape (tuple): the size of the input image (h,w)
+
+ Returns:
+ masks (torch.Tensor): The returned masks with dimensions [h, w, n]
+ """
+ c, mh, mw = protos.shape # CHW
+ masks = (masks_in @ protos.float().view(c, -1)).sigmoid().view(-1, mh, mw)
+ gain = min(mh / shape[0], mw / shape[1]) # gain = old / new
+ pad = (mw - shape[1] * gain) / 2, (mh - shape[0] * gain) / 2 # wh padding
+ top, left = int(pad[1]), int(pad[0]) # y, x
+ bottom, right = int(mh - pad[1]), int(mw - pad[0])
+ masks = masks[:, top:bottom, left:right]
+
+ masks = F.interpolate(masks[None], shape, mode='bilinear', align_corners=False)[0] # CHW
+ masks = crop_mask(masks, bboxes) # CHW
+ return masks.gt_(0.5)
+
+
+def scale_coords(img1_shape, coords, img0_shape, ratio_pad=None, normalize=False):
+ """
+ Rescale segment coordinates (xyxy) from img1_shape to img0_shape
+
+ Args:
+ img1_shape (tuple): The shape of the image that the coords are from.
+ coords (torch.Tensor): the coords to be scaled
+ img0_shape (tuple): the shape of the image that the segmentation is being applied to
+ ratio_pad (tuple): the ratio of the image size to the padded image size.
+ normalize (bool): If True, the coordinates will be normalized to the range [0, 1]. Defaults to False
+
+ Returns:
+ coords (torch.Tensor): the segmented image.
+ """
+ if ratio_pad is None: # calculate from img0_shape
+ gain = min(img1_shape[0] / img0_shape[0], img1_shape[1] / img0_shape[1]) # gain = old / new
+ pad = (img1_shape[1] - img0_shape[1] * gain) / 2, (img1_shape[0] - img0_shape[0] * gain) / 2 # wh padding
+ else:
+ gain = ratio_pad[0][0]
+ pad = ratio_pad[1]
+
+ coords[..., 0] -= pad[0] # x padding
+ coords[..., 1] -= pad[1] # y padding
+ coords[..., 0] /= gain
+ coords[..., 1] /= gain
+ clip_coords(coords, img0_shape)
+ if normalize:
+ coords[..., 0] /= img0_shape[1] # width
+ coords[..., 1] /= img0_shape[0] # height
+ return coords
+
+
+def masks2segments(masks, strategy='largest'):
+ """
+ It takes a list of masks(n,h,w) and returns a list of segments(n,xy)
+
+ Args:
+ masks (torch.Tensor): the output of the model, which is a tensor of shape (batch_size, 160, 160)
+ strategy (str): 'concat' or 'largest'. Defaults to largest
+
+ Returns:
+ segments (List): list of segment masks
+ """
+ segments = []
+ for x in masks.int().cpu().numpy().astype('uint8'):
+ c = cv2.findContours(x, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[0]
+ if c:
+ if strategy == 'concat': # concatenate all segments
+ c = np.concatenate([x.reshape(-1, 2) for x in c])
+ elif strategy == 'largest': # select largest segment
+ c = np.array(c[np.array([len(x) for x in c]).argmax()]).reshape(-1, 2)
+ else:
+ c = np.zeros((0, 2)) # no segments found
+ segments.append(c.astype('float32'))
+ return segments
+
+
+def clean_str(s):
+ """
+ Cleans a string by replacing special characters with underscore _
+
+ Args:
+ s (str): a string needing special characters replaced
+
+ Returns:
+ (str): a string with special characters replaced by an underscore _
+ """
+ return re.sub(pattern='[|@#!¡·$€%&()=?¿^*;:,¨´><+]', repl='_', string=s)
diff --git a/yolov8/ultralytics/yolo/utils/patches.py b/yolov8/ultralytics/yolo/utils/patches.py
new file mode 100644
index 0000000000000000000000000000000000000000..2b023b9072f99f590b8f9082bb8bff900e66ab00
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/patches.py
@@ -0,0 +1,45 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+"""
+Monkey patches to update/extend functionality of existing functions
+"""
+
+from pathlib import Path
+
+import cv2
+import numpy as np
+import torch
+
+# OpenCV Multilanguage-friendly functions ------------------------------------------------------------------------------
+_imshow = cv2.imshow # copy to avoid recursion errors
+
+
+def imread(filename, flags=cv2.IMREAD_COLOR):
+ return cv2.imdecode(np.fromfile(filename, np.uint8), flags)
+
+
+def imwrite(filename, img):
+ try:
+ cv2.imencode(Path(filename).suffix, img)[1].tofile(filename)
+ return True
+ except Exception:
+ return False
+
+
+def imshow(path, im):
+ _imshow(path.encode('unicode_escape').decode(), im)
+
+
+# PyTorch functions ----------------------------------------------------------------------------------------------------
+_torch_save = torch.save # copy to avoid recursion errors
+
+
+def torch_save(*args, **kwargs):
+ # Use dill (if exists) to serialize the lambda functions where pickle does not do this
+ try:
+ import dill as pickle
+ except ImportError:
+ import pickle
+
+ if 'pickle_module' not in kwargs:
+ kwargs['pickle_module'] = pickle
+ return _torch_save(*args, **kwargs)
diff --git a/yolov8/ultralytics/yolo/utils/plotting.py b/yolov8/ultralytics/yolo/utils/plotting.py
new file mode 100644
index 0000000000000000000000000000000000000000..89320621332e2905a5581d33a63bfdcfd1ecc0ca
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/plotting.py
@@ -0,0 +1,518 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import contextlib
+import math
+import warnings
+from pathlib import Path
+
+import cv2
+import matplotlib.pyplot as plt
+import numpy as np
+import torch
+from PIL import Image, ImageDraw, ImageFont
+from PIL import __version__ as pil_version
+from scipy.ndimage import gaussian_filter1d
+
+from ultralytics.yolo.utils import LOGGER, TryExcept, plt_settings, threaded
+
+from .checks import check_font, check_version, is_ascii
+from .files import increment_path
+from .ops import clip_boxes, scale_image, xywh2xyxy, xyxy2xywh
+
+
+class Colors:
+ # Ultralytics color palette https://ultralytics.com/
+ def __init__(self):
+ """Initialize colors as hex = matplotlib.colors.TABLEAU_COLORS.values()."""
+ hexs = ('FF3838', 'FF9D97', 'FF701F', 'FFB21D', 'CFD231', '48F90A', '92CC17', '3DDB86', '1A9334', '00D4BB',
+ '2C99A8', '00C2FF', '344593', '6473FF', '0018EC', '8438FF', '520085', 'CB38FF', 'FF95C8', 'FF37C7')
+ self.palette = [self.hex2rgb(f'#{c}') for c in hexs]
+ self.n = len(self.palette)
+ self.pose_palette = np.array([[255, 128, 0], [255, 153, 51], [255, 178, 102], [230, 230, 0], [255, 153, 255],
+ [153, 204, 255], [255, 102, 255], [255, 51, 255], [102, 178, 255], [51, 153, 255],
+ [255, 153, 153], [255, 102, 102], [255, 51, 51], [153, 255, 153], [102, 255, 102],
+ [51, 255, 51], [0, 255, 0], [0, 0, 255], [255, 0, 0], [255, 255, 255]],
+ dtype=np.uint8)
+
+ def __call__(self, i, bgr=False):
+ """Converts hex color codes to rgb values."""
+ c = self.palette[int(i) % self.n]
+ return (c[2], c[1], c[0]) if bgr else c
+
+ @staticmethod
+ def hex2rgb(h): # rgb order (PIL)
+ return tuple(int(h[1 + i:1 + i + 2], 16) for i in (0, 2, 4))
+
+
+colors = Colors() # create instance for 'from utils.plots import colors'
+
+
+class Annotator:
+ # YOLOv8 Annotator for train/val mosaics and jpgs and detect/hub inference annotations
+ def __init__(self, im, line_width=None, font_size=None, font='Arial.ttf', pil=False, example='abc'):
+ """Initialize the Annotator class with image and line width along with color palette for keypoints and limbs."""
+ assert im.data.contiguous, 'Image not contiguous. Apply np.ascontiguousarray(im) to Annotator() input images.'
+ non_ascii = not is_ascii(example) # non-latin labels, i.e. asian, arabic, cyrillic
+ self.pil = pil or non_ascii
+ if self.pil: # use PIL
+ self.im = im if isinstance(im, Image.Image) else Image.fromarray(im)
+ self.draw = ImageDraw.Draw(self.im)
+ try:
+ font = check_font('Arial.Unicode.ttf' if non_ascii else font)
+ size = font_size or max(round(sum(self.im.size) / 2 * 0.035), 12)
+ self.font = ImageFont.truetype(str(font), size)
+ except Exception:
+ self.font = ImageFont.load_default()
+ # Deprecation fix for w, h = getsize(string) -> _, _, w, h = getbox(string)
+ if check_version(pil_version, '9.2.0'):
+ self.font.getsize = lambda x: self.font.getbbox(x)[2:4] # text width, height
+ else: # use cv2
+ self.im = im
+ self.lw = line_width or max(round(sum(im.shape) / 2 * 0.003), 2) # line width
+ # Pose
+ self.skeleton = [[16, 14], [14, 12], [17, 15], [15, 13], [12, 13], [6, 12], [7, 13], [6, 7], [6, 8], [7, 9],
+ [8, 10], [9, 11], [2, 3], [1, 2], [1, 3], [2, 4], [3, 5], [4, 6], [5, 7]]
+
+ self.limb_color = colors.pose_palette[[9, 9, 9, 9, 7, 7, 7, 0, 0, 0, 0, 0, 16, 16, 16, 16, 16, 16, 16]]
+ self.kpt_color = colors.pose_palette[[16, 16, 16, 16, 16, 0, 0, 0, 0, 0, 0, 9, 9, 9, 9, 9, 9]]
+
+ def box_label(self, box, label='', color=(128, 128, 128), txt_color=(255, 255, 255)):
+ """Add one xyxy box to image with label."""
+ if isinstance(box, torch.Tensor):
+ box = box.tolist()
+ if self.pil or not is_ascii(label):
+ self.draw.rectangle(box, width=self.lw, outline=color) # box
+ if label:
+ w, h = self.font.getsize(label) # text width, height
+ outside = box[1] - h >= 0 # label fits outside box
+ self.draw.rectangle(
+ (box[0], box[1] - h if outside else box[1], box[0] + w + 1,
+ box[1] + 1 if outside else box[1] + h + 1),
+ fill=color,
+ )
+ # self.draw.text((box[0], box[1]), label, fill=txt_color, font=self.font, anchor='ls') # for PIL>8.0
+ self.draw.text((box[0], box[1] - h if outside else box[1]), label, fill=txt_color, font=self.font)
+ else: # cv2
+ p1, p2 = (int(box[0]), int(box[1])), (int(box[2]), int(box[3]))
+ cv2.rectangle(self.im, p1, p2, color, thickness=self.lw, lineType=cv2.LINE_AA)
+ if label:
+ tf = max(self.lw - 1, 1) # font thickness
+ w, h = cv2.getTextSize(label, 0, fontScale=self.lw / 3, thickness=tf)[0] # text width, height
+ outside = p1[1] - h >= 3
+ p2 = p1[0] + w, p1[1] - h - 3 if outside else p1[1] + h + 3
+ cv2.rectangle(self.im, p1, p2, color, -1, cv2.LINE_AA) # filled
+ cv2.putText(self.im,
+ label, (p1[0], p1[1] - 2 if outside else p1[1] + h + 2),
+ 0,
+ self.lw / 3,
+ txt_color,
+ thickness=tf,
+ lineType=cv2.LINE_AA)
+
+ def masks(self, masks, colors, im_gpu, alpha=0.5, retina_masks=False):
+ """Plot masks at once.
+ Args:
+ masks (tensor): predicted masks on cuda, shape: [n, h, w]
+ colors (List[List[Int]]): colors for predicted masks, [[r, g, b] * n]
+ im_gpu (tensor): img is in cuda, shape: [3, h, w], range: [0, 1]
+ alpha (float): mask transparency: 0.0 fully transparent, 1.0 opaque
+ """
+ if self.pil:
+ # Convert to numpy first
+ self.im = np.asarray(self.im).copy()
+ if len(masks) == 0:
+ self.im[:] = im_gpu.permute(1, 2, 0).contiguous().cpu().numpy() * 255
+ if im_gpu.device != masks.device:
+ im_gpu = im_gpu.to(masks.device)
+ colors = torch.tensor(colors, device=masks.device, dtype=torch.float32) / 255.0 # shape(n,3)
+ colors = colors[:, None, None] # shape(n,1,1,3)
+ masks = masks.unsqueeze(3) # shape(n,h,w,1)
+ masks_color = masks * (colors * alpha) # shape(n,h,w,3)
+
+ inv_alph_masks = (1 - masks * alpha).cumprod(0) # shape(n,h,w,1)
+ mcs = masks_color.max(dim=0).values # shape(n,h,w,3)
+
+ im_gpu = im_gpu.flip(dims=[0]) # flip channel
+ im_gpu = im_gpu.permute(1, 2, 0).contiguous() # shape(h,w,3)
+ im_gpu = im_gpu * inv_alph_masks[-1] + mcs
+ im_mask = (im_gpu * 255)
+ im_mask_np = im_mask.byte().cpu().numpy()
+ self.im[:] = im_mask_np if retina_masks else scale_image(im_mask_np, self.im.shape)
+ if self.pil:
+ # Convert im back to PIL and update draw
+ self.fromarray(self.im)
+
+ def kpts(self, kpts, shape=(640, 640), radius=5, kpt_line=True):
+ """Plot keypoints on the image.
+
+ Args:
+ kpts (tensor): Predicted keypoints with shape [17, 3]. Each keypoint has (x, y, confidence).
+ shape (tuple): Image shape as a tuple (h, w), where h is the height and w is the width.
+ radius (int, optional): Radius of the drawn keypoints. Default is 5.
+ kpt_line (bool, optional): If True, the function will draw lines connecting keypoints
+ for human pose. Default is True.
+
+ Note: `kpt_line=True` currently only supports human pose plotting.
+ """
+ if self.pil:
+ # Convert to numpy first
+ self.im = np.asarray(self.im).copy()
+ nkpt, ndim = kpts.shape
+ is_pose = nkpt == 17 and ndim == 3
+ kpt_line &= is_pose # `kpt_line=True` for now only supports human pose plotting
+ for i, k in enumerate(kpts):
+ color_k = [int(x) for x in self.kpt_color[i]] if is_pose else colors(i)
+ x_coord, y_coord = k[0], k[1]
+ if x_coord % shape[1] != 0 and y_coord % shape[0] != 0:
+ if len(k) == 3:
+ conf = k[2]
+ if conf < 0.5:
+ continue
+ cv2.circle(self.im, (int(x_coord), int(y_coord)), radius, color_k, -1, lineType=cv2.LINE_AA)
+
+ if kpt_line:
+ ndim = kpts.shape[-1]
+ for i, sk in enumerate(self.skeleton):
+ pos1 = (int(kpts[(sk[0] - 1), 0]), int(kpts[(sk[0] - 1), 1]))
+ pos2 = (int(kpts[(sk[1] - 1), 0]), int(kpts[(sk[1] - 1), 1]))
+ if ndim == 3:
+ conf1 = kpts[(sk[0] - 1), 2]
+ conf2 = kpts[(sk[1] - 1), 2]
+ if conf1 < 0.5 or conf2 < 0.5:
+ continue
+ if pos1[0] % shape[1] == 0 or pos1[1] % shape[0] == 0 or pos1[0] < 0 or pos1[1] < 0:
+ continue
+ if pos2[0] % shape[1] == 0 or pos2[1] % shape[0] == 0 or pos2[0] < 0 or pos2[1] < 0:
+ continue
+ cv2.line(self.im, pos1, pos2, [int(x) for x in self.limb_color[i]], thickness=2, lineType=cv2.LINE_AA)
+ if self.pil:
+ # Convert im back to PIL and update draw
+ self.fromarray(self.im)
+
+ def rectangle(self, xy, fill=None, outline=None, width=1):
+ """Add rectangle to image (PIL-only)."""
+ self.draw.rectangle(xy, fill, outline, width)
+
+ def text(self, xy, text, txt_color=(255, 255, 255), anchor='top', box_style=False):
+ """Adds text to an image using PIL or cv2."""
+ if anchor == 'bottom': # start y from font bottom
+ w, h = self.font.getsize(text) # text width, height
+ xy[1] += 1 - h
+ if self.pil:
+ if box_style:
+ w, h = self.font.getsize(text)
+ self.draw.rectangle((xy[0], xy[1], xy[0] + w + 1, xy[1] + h + 1), fill=txt_color)
+ # Using `txt_color` for background and draw fg with white color
+ txt_color = (255, 255, 255)
+ self.draw.text(xy, text, fill=txt_color, font=self.font)
+ else:
+ if box_style:
+ tf = max(self.lw - 1, 1) # font thickness
+ w, h = cv2.getTextSize(text, 0, fontScale=self.lw / 3, thickness=tf)[0] # text width, height
+ outside = xy[1] - h >= 3
+ p2 = xy[0] + w, xy[1] - h - 3 if outside else xy[1] + h + 3
+ cv2.rectangle(self.im, xy, p2, txt_color, -1, cv2.LINE_AA) # filled
+ # Using `txt_color` for background and draw fg with white color
+ txt_color = (255, 255, 255)
+ tf = max(self.lw - 1, 1) # font thickness
+ cv2.putText(self.im, text, xy, 0, self.lw / 3, txt_color, thickness=tf, lineType=cv2.LINE_AA)
+
+ def fromarray(self, im):
+ """Update self.im from a numpy array."""
+ self.im = im if isinstance(im, Image.Image) else Image.fromarray(im)
+ self.draw = ImageDraw.Draw(self.im)
+
+ def result(self):
+ """Return annotated image as array."""
+ return np.asarray(self.im)
+
+
+@TryExcept() # known issue https://github.com/ultralytics/yolov5/issues/5395
+@plt_settings()
+def plot_labels(boxes, cls, names=(), save_dir=Path(''), on_plot=None):
+ """Save and plot image with no axis or spines."""
+ import pandas as pd
+ import seaborn as sn
+
+ # Filter matplotlib>=3.7.2 warning
+ warnings.filterwarnings('ignore', category=UserWarning, message='The figure layout has changed to tight')
+
+ # Plot dataset labels
+ LOGGER.info(f"Plotting labels to {save_dir / 'labels.jpg'}... ")
+ b = boxes.transpose() # classes, boxes
+ nc = int(cls.max() + 1) # number of classes
+ x = pd.DataFrame(b.transpose(), columns=['x', 'y', 'width', 'height'])
+
+ # Seaborn correlogram
+ sn.pairplot(x, corner=True, diag_kind='auto', kind='hist', diag_kws=dict(bins=50), plot_kws=dict(pmax=0.9))
+ plt.savefig(save_dir / 'labels_correlogram.jpg', dpi=200)
+ plt.close()
+
+ # Matplotlib labels
+ ax = plt.subplots(2, 2, figsize=(8, 8), tight_layout=True)[1].ravel()
+ y = ax[0].hist(cls, bins=np.linspace(0, nc, nc + 1) - 0.5, rwidth=0.8)
+ with contextlib.suppress(Exception): # color histogram bars by class
+ [y[2].patches[i].set_color([x / 255 for x in colors(i)]) for i in range(nc)] # known issue #3195
+ ax[0].set_ylabel('instances')
+ if 0 < len(names) < 30:
+ ax[0].set_xticks(range(len(names)))
+ ax[0].set_xticklabels(list(names.values()), rotation=90, fontsize=10)
+ else:
+ ax[0].set_xlabel('classes')
+ sn.histplot(x, x='x', y='y', ax=ax[2], bins=50, pmax=0.9)
+ sn.histplot(x, x='width', y='height', ax=ax[3], bins=50, pmax=0.9)
+
+ # Rectangles
+ boxes[:, 0:2] = 0.5 # center
+ boxes = xywh2xyxy(boxes) * 1000
+ img = Image.fromarray(np.ones((1000, 1000, 3), dtype=np.uint8) * 255)
+ for cls, box in zip(cls[:500], boxes[:500]):
+ ImageDraw.Draw(img).rectangle(box, width=1, outline=colors(cls)) # plot
+ ax[1].imshow(img)
+ ax[1].axis('off')
+
+ for a in [0, 1, 2, 3]:
+ for s in ['top', 'right', 'left', 'bottom']:
+ ax[a].spines[s].set_visible(False)
+
+ fname = save_dir / 'labels.jpg'
+ plt.savefig(fname, dpi=200)
+ plt.close()
+ if on_plot:
+ on_plot(fname)
+
+
+def save_one_box(xyxy, im, file=Path('im.jpg'), gain=1.02, pad=10, square=False, BGR=False, save=True):
+ """Save image crop as {file} with crop size multiple {gain} and {pad} pixels. Save and/or return crop."""
+ b = xyxy2xywh(xyxy.view(-1, 4)) # boxes
+ if square:
+ b[:, 2:] = b[:, 2:].max(1)[0].unsqueeze(1) # attempt rectangle to square
+ b[:, 2:] = b[:, 2:] * gain + pad # box wh * gain + pad
+ xyxy = xywh2xyxy(b).long()
+ clip_boxes(xyxy, im.shape)
+ crop = im[int(xyxy[0, 1]):int(xyxy[0, 3]), int(xyxy[0, 0]):int(xyxy[0, 2]), ::(1 if BGR else -1)]
+ if save:
+ file.parent.mkdir(parents=True, exist_ok=True) # make directory
+ f = str(increment_path(file).with_suffix('.jpg'))
+ # cv2.imwrite(f, crop) # save BGR, https://github.com/ultralytics/yolov5/issues/7007 chroma subsampling issue
+ Image.fromarray(crop[..., ::-1]).save(f, quality=95, subsampling=0) # save RGB
+ return crop
+
+
+@threaded
+def plot_images(images,
+ batch_idx,
+ cls,
+ bboxes=np.zeros(0, dtype=np.float32),
+ masks=np.zeros(0, dtype=np.uint8),
+ kpts=np.zeros((0, 51), dtype=np.float32),
+ paths=None,
+ fname='images.jpg',
+ names=None,
+ on_plot=None):
+ # Plot image grid with labels
+ if isinstance(images, torch.Tensor):
+ images = images.cpu().float().numpy()
+ if isinstance(cls, torch.Tensor):
+ cls = cls.cpu().numpy()
+ if isinstance(bboxes, torch.Tensor):
+ bboxes = bboxes.cpu().numpy()
+ if isinstance(masks, torch.Tensor):
+ masks = masks.cpu().numpy().astype(int)
+ if isinstance(kpts, torch.Tensor):
+ kpts = kpts.cpu().numpy()
+ if isinstance(batch_idx, torch.Tensor):
+ batch_idx = batch_idx.cpu().numpy()
+
+ max_size = 1920 # max image size
+ max_subplots = 16 # max image subplots, i.e. 4x4
+ bs, _, h, w = images.shape # batch size, _, height, width
+ bs = min(bs, max_subplots) # limit plot images
+ ns = np.ceil(bs ** 0.5) # number of subplots (square)
+ if np.max(images[0]) <= 1:
+ images *= 255 # de-normalise (optional)
+
+ # Build Image
+ mosaic = np.full((int(ns * h), int(ns * w), 3), 255, dtype=np.uint8) # init
+ for i, im in enumerate(images):
+ if i == max_subplots: # if last batch has fewer images than we expect
+ break
+ x, y = int(w * (i // ns)), int(h * (i % ns)) # block origin
+ im = im.transpose(1, 2, 0)
+ mosaic[y:y + h, x:x + w, :] = im
+
+ # Resize (optional)
+ scale = max_size / ns / max(h, w)
+ if scale < 1:
+ h = math.ceil(scale * h)
+ w = math.ceil(scale * w)
+ mosaic = cv2.resize(mosaic, tuple(int(x * ns) for x in (w, h)))
+
+ # Annotate
+ fs = int((h + w) * ns * 0.01) # font size
+ annotator = Annotator(mosaic, line_width=round(fs / 10), font_size=fs, pil=True, example=names)
+ for i in range(i + 1):
+ x, y = int(w * (i // ns)), int(h * (i % ns)) # block origin
+ annotator.rectangle([x, y, x + w, y + h], None, (255, 255, 255), width=2) # borders
+ if paths:
+ annotator.text((x + 5, y + 5), text=Path(paths[i]).name[:40], txt_color=(220, 220, 220)) # filenames
+ if len(cls) > 0:
+ idx = batch_idx == i
+ classes = cls[idx].astype('int')
+
+ if len(bboxes):
+ boxes = xywh2xyxy(bboxes[idx, :4]).T
+ labels = bboxes.shape[1] == 4 # labels if no conf column
+ conf = None if labels else bboxes[idx, 4] # check for confidence presence (label vs pred)
+
+ if boxes.shape[1]:
+ if boxes.max() <= 1.01: # if normalized with tolerance 0.01
+ boxes[[0, 2]] *= w # scale to pixels
+ boxes[[1, 3]] *= h
+ elif scale < 1: # absolute coords need scale if image scales
+ boxes *= scale
+ boxes[[0, 2]] += x
+ boxes[[1, 3]] += y
+ for j, box in enumerate(boxes.T.tolist()):
+ c = classes[j]
+ color = colors(c)
+ c = names.get(c, c) if names else c
+ if labels or conf[j] > 0.25: # 0.25 conf thresh
+ label = f'{c}' if labels else f'{c} {conf[j]:.1f}'
+ annotator.box_label(box, label, color=color)
+ elif len(classes):
+ for c in classes:
+ color = colors(c)
+ c = names.get(c, c) if names else c
+ annotator.text((x, y), f'{c}', txt_color=color, box_style=True)
+
+ # Plot keypoints
+ if len(kpts):
+ kpts_ = kpts[idx].copy()
+ if len(kpts_):
+ if kpts_[..., 0].max() <= 1.01 or kpts_[..., 1].max() <= 1.01: # if normalized with tolerance .01
+ kpts_[..., 0] *= w # scale to pixels
+ kpts_[..., 1] *= h
+ elif scale < 1: # absolute coords need scale if image scales
+ kpts_ *= scale
+ kpts_[..., 0] += x
+ kpts_[..., 1] += y
+ for j in range(len(kpts_)):
+ if labels or conf[j] > 0.25: # 0.25 conf thresh
+ annotator.kpts(kpts_[j])
+
+ # Plot masks
+ if len(masks):
+ if idx.shape[0] == masks.shape[0]: # overlap_masks=False
+ image_masks = masks[idx]
+ else: # overlap_masks=True
+ image_masks = masks[[i]] # (1, 640, 640)
+ nl = idx.sum()
+ index = np.arange(nl).reshape((nl, 1, 1)) + 1
+ image_masks = np.repeat(image_masks, nl, axis=0)
+ image_masks = np.where(image_masks == index, 1.0, 0.0)
+
+ im = np.asarray(annotator.im).copy()
+ for j, box in enumerate(boxes.T.tolist()):
+ if labels or conf[j] > 0.25: # 0.25 conf thresh
+ color = colors(classes[j])
+ mh, mw = image_masks[j].shape
+ if mh != h or mw != w:
+ mask = image_masks[j].astype(np.uint8)
+ mask = cv2.resize(mask, (w, h))
+ mask = mask.astype(bool)
+ else:
+ mask = image_masks[j].astype(bool)
+ with contextlib.suppress(Exception):
+ im[y:y + h, x:x + w, :][mask] = im[y:y + h, x:x + w, :][mask] * 0.4 + np.array(color) * 0.6
+ annotator.fromarray(im)
+ annotator.im.save(fname) # save
+ if on_plot:
+ on_plot(fname)
+
+
+@plt_settings()
+def plot_results(file='path/to/results.csv', dir='', segment=False, pose=False, classify=False, on_plot=None):
+ """Plot training results.csv. Usage: from utils.plots import *; plot_results('path/to/results.csv')."""
+ import pandas as pd
+ save_dir = Path(file).parent if file else Path(dir)
+ if classify:
+ fig, ax = plt.subplots(2, 2, figsize=(6, 6), tight_layout=True)
+ index = [1, 4, 2, 3]
+ elif segment:
+ fig, ax = plt.subplots(2, 8, figsize=(18, 6), tight_layout=True)
+ index = [1, 2, 3, 4, 5, 6, 9, 10, 13, 14, 15, 16, 7, 8, 11, 12]
+ elif pose:
+ fig, ax = plt.subplots(2, 9, figsize=(21, 6), tight_layout=True)
+ index = [1, 2, 3, 4, 5, 6, 7, 10, 11, 14, 15, 16, 17, 18, 8, 9, 12, 13]
+ else:
+ fig, ax = plt.subplots(2, 5, figsize=(12, 6), tight_layout=True)
+ index = [1, 2, 3, 4, 5, 8, 9, 10, 6, 7]
+ ax = ax.ravel()
+ files = list(save_dir.glob('results*.csv'))
+ assert len(files), f'No results.csv files found in {save_dir.resolve()}, nothing to plot.'
+ for f in files:
+ try:
+ data = pd.read_csv(f)
+ s = [x.strip() for x in data.columns]
+ x = data.values[:, 0]
+ for i, j in enumerate(index):
+ y = data.values[:, j].astype('float')
+ # y[y == 0] = np.nan # don't show zero values
+ ax[i].plot(x, y, marker='.', label=f.stem, linewidth=2, markersize=8) # actual results
+ ax[i].plot(x, gaussian_filter1d(y, sigma=3), ':', label='smooth', linewidth=2) # smoothing line
+ ax[i].set_title(s[j], fontsize=12)
+ # if j in [8, 9, 10]: # share train and val loss y axes
+ # ax[i].get_shared_y_axes().join(ax[i], ax[i - 5])
+ except Exception as e:
+ LOGGER.warning(f'WARNING: Plotting error for {f}: {e}')
+ ax[1].legend()
+ fname = save_dir / 'results.png'
+ fig.savefig(fname, dpi=200)
+ plt.close()
+ if on_plot:
+ on_plot(fname)
+
+
+def output_to_target(output, max_det=300):
+ """Convert model output to target format [batch_id, class_id, x, y, w, h, conf] for plotting."""
+ targets = []
+ for i, o in enumerate(output):
+ box, conf, cls = o[:max_det, :6].cpu().split((4, 1, 1), 1)
+ j = torch.full((conf.shape[0], 1), i)
+ targets.append(torch.cat((j, cls, xyxy2xywh(box), conf), 1))
+ targets = torch.cat(targets, 0).numpy()
+ return targets[:, 0], targets[:, 1], targets[:, 2:]
+
+
+def feature_visualization(x, module_type, stage, n=32, save_dir=Path('runs/detect/exp')):
+ """
+ Visualize feature maps of a given model module during inference.
+
+ Args:
+ x (torch.Tensor): Features to be visualized.
+ module_type (str): Module type.
+ stage (int): Module stage within the model.
+ n (int, optional): Maximum number of feature maps to plot. Defaults to 32.
+ save_dir (Path, optional): Directory to save results. Defaults to Path('runs/detect/exp').
+ """
+ for m in ['Detect', 'Pose', 'Segment']:
+ if m in module_type:
+ return
+ batch, channels, height, width = x.shape # batch, channels, height, width
+ if height > 1 and width > 1:
+ f = save_dir / f"stage{stage}_{module_type.split('.')[-1]}_features.png" # filename
+
+ blocks = torch.chunk(x[0].cpu(), channels, dim=0) # select batch index 0, block by channels
+ n = min(n, channels) # number of plots
+ fig, ax = plt.subplots(math.ceil(n / 8), 8, tight_layout=True) # 8 rows x n/8 cols
+ ax = ax.ravel()
+ plt.subplots_adjust(wspace=0.05, hspace=0.05)
+ for i in range(n):
+ ax[i].imshow(blocks[i].squeeze()) # cmap='gray'
+ ax[i].axis('off')
+
+ LOGGER.info(f'Saving {f}... ({n}/{channels})')
+ plt.savefig(f, dpi=300, bbox_inches='tight')
+ plt.close()
+ np.save(str(f.with_suffix('.npy')), x[0].cpu().numpy()) # npy save
diff --git a/yolov8/ultralytics/yolo/utils/tal.py b/yolov8/ultralytics/yolo/utils/tal.py
new file mode 100644
index 0000000000000000000000000000000000000000..aea8918ce8cca4a78d1ddf16e4d98dc6a6cd145f
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/tal.py
@@ -0,0 +1,276 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+import torch.nn as nn
+
+from .checks import check_version
+from .metrics import bbox_iou
+
+TORCH_1_10 = check_version(torch.__version__, '1.10.0')
+
+
+def select_candidates_in_gts(xy_centers, gt_bboxes, eps=1e-9):
+ """select the positive anchor center in gt
+
+ Args:
+ xy_centers (Tensor): shape(h*w, 4)
+ gt_bboxes (Tensor): shape(b, n_boxes, 4)
+ Return:
+ (Tensor): shape(b, n_boxes, h*w)
+ """
+ n_anchors = xy_centers.shape[0]
+ bs, n_boxes, _ = gt_bboxes.shape
+ lt, rb = gt_bboxes.view(-1, 1, 4).chunk(2, 2) # left-top, right-bottom
+ bbox_deltas = torch.cat((xy_centers[None] - lt, rb - xy_centers[None]), dim=2).view(bs, n_boxes, n_anchors, -1)
+ # return (bbox_deltas.min(3)[0] > eps).to(gt_bboxes.dtype)
+ return bbox_deltas.amin(3).gt_(eps)
+
+
+def select_highest_overlaps(mask_pos, overlaps, n_max_boxes):
+ """if an anchor box is assigned to multiple gts,
+ the one with the highest iou will be selected.
+
+ Args:
+ mask_pos (Tensor): shape(b, n_max_boxes, h*w)
+ overlaps (Tensor): shape(b, n_max_boxes, h*w)
+ Return:
+ target_gt_idx (Tensor): shape(b, h*w)
+ fg_mask (Tensor): shape(b, h*w)
+ mask_pos (Tensor): shape(b, n_max_boxes, h*w)
+ """
+ # (b, n_max_boxes, h*w) -> (b, h*w)
+ fg_mask = mask_pos.sum(-2)
+ if fg_mask.max() > 1: # one anchor is assigned to multiple gt_bboxes
+ mask_multi_gts = (fg_mask.unsqueeze(1) > 1).expand(-1, n_max_boxes, -1) # (b, n_max_boxes, h*w)
+ max_overlaps_idx = overlaps.argmax(1) # (b, h*w)
+
+ is_max_overlaps = torch.zeros(mask_pos.shape, dtype=mask_pos.dtype, device=mask_pos.device)
+ is_max_overlaps.scatter_(1, max_overlaps_idx.unsqueeze(1), 1)
+
+ mask_pos = torch.where(mask_multi_gts, is_max_overlaps, mask_pos).float() # (b, n_max_boxes, h*w)
+ fg_mask = mask_pos.sum(-2)
+ # Find each grid serve which gt(index)
+ target_gt_idx = mask_pos.argmax(-2) # (b, h*w)
+ return target_gt_idx, fg_mask, mask_pos
+
+
+class TaskAlignedAssigner(nn.Module):
+ """
+ A task-aligned assigner for object detection.
+
+ This class assigns ground-truth (gt) objects to anchors based on the task-aligned metric,
+ which combines both classification and localization information.
+
+ Attributes:
+ topk (int): The number of top candidates to consider.
+ num_classes (int): The number of object classes.
+ alpha (float): The alpha parameter for the classification component of the task-aligned metric.
+ beta (float): The beta parameter for the localization component of the task-aligned metric.
+ eps (float): A small value to prevent division by zero.
+ """
+
+ def __init__(self, topk=13, num_classes=80, alpha=1.0, beta=6.0, eps=1e-9):
+ """Initialize a TaskAlignedAssigner object with customizable hyperparameters."""
+ super().__init__()
+ self.topk = topk
+ self.num_classes = num_classes
+ self.bg_idx = num_classes
+ self.alpha = alpha
+ self.beta = beta
+ self.eps = eps
+
+ @torch.no_grad()
+ def forward(self, pd_scores, pd_bboxes, anc_points, gt_labels, gt_bboxes, mask_gt):
+ """
+ Compute the task-aligned assignment.
+ Reference https://github.com/Nioolek/PPYOLOE_pytorch/blob/master/ppyoloe/assigner/tal_assigner.py
+
+ Args:
+ pd_scores (Tensor): shape(bs, num_total_anchors, num_classes)
+ pd_bboxes (Tensor): shape(bs, num_total_anchors, 4)
+ anc_points (Tensor): shape(num_total_anchors, 2)
+ gt_labels (Tensor): shape(bs, n_max_boxes, 1)
+ gt_bboxes (Tensor): shape(bs, n_max_boxes, 4)
+ mask_gt (Tensor): shape(bs, n_max_boxes, 1)
+
+ Returns:
+ target_labels (Tensor): shape(bs, num_total_anchors)
+ target_bboxes (Tensor): shape(bs, num_total_anchors, 4)
+ target_scores (Tensor): shape(bs, num_total_anchors, num_classes)
+ fg_mask (Tensor): shape(bs, num_total_anchors)
+ target_gt_idx (Tensor): shape(bs, num_total_anchors)
+ """
+ self.bs = pd_scores.size(0)
+ self.n_max_boxes = gt_bboxes.size(1)
+
+ if self.n_max_boxes == 0:
+ device = gt_bboxes.device
+ return (torch.full_like(pd_scores[..., 0], self.bg_idx).to(device), torch.zeros_like(pd_bboxes).to(device),
+ torch.zeros_like(pd_scores).to(device), torch.zeros_like(pd_scores[..., 0]).to(device),
+ torch.zeros_like(pd_scores[..., 0]).to(device))
+
+ mask_pos, align_metric, overlaps = self.get_pos_mask(pd_scores, pd_bboxes, gt_labels, gt_bboxes, anc_points,
+ mask_gt)
+
+ target_gt_idx, fg_mask, mask_pos = select_highest_overlaps(mask_pos, overlaps, self.n_max_boxes)
+
+ # Assigned target
+ target_labels, target_bboxes, target_scores = self.get_targets(gt_labels, gt_bboxes, target_gt_idx, fg_mask)
+
+ # Normalize
+ align_metric *= mask_pos
+ pos_align_metrics = align_metric.amax(axis=-1, keepdim=True) # b, max_num_obj
+ pos_overlaps = (overlaps * mask_pos).amax(axis=-1, keepdim=True) # b, max_num_obj
+ norm_align_metric = (align_metric * pos_overlaps / (pos_align_metrics + self.eps)).amax(-2).unsqueeze(-1)
+ target_scores = target_scores * norm_align_metric
+
+ return target_labels, target_bboxes, target_scores, fg_mask.bool(), target_gt_idx
+
+ def get_pos_mask(self, pd_scores, pd_bboxes, gt_labels, gt_bboxes, anc_points, mask_gt):
+ """Get in_gts mask, (b, max_num_obj, h*w)."""
+ mask_in_gts = select_candidates_in_gts(anc_points, gt_bboxes)
+ # Get anchor_align metric, (b, max_num_obj, h*w)
+ align_metric, overlaps = self.get_box_metrics(pd_scores, pd_bboxes, gt_labels, gt_bboxes, mask_in_gts * mask_gt)
+ # Get topk_metric mask, (b, max_num_obj, h*w)
+ mask_topk = self.select_topk_candidates(align_metric, topk_mask=mask_gt.expand(-1, -1, self.topk).bool())
+ # Merge all mask to a final mask, (b, max_num_obj, h*w)
+ mask_pos = mask_topk * mask_in_gts * mask_gt
+
+ return mask_pos, align_metric, overlaps
+
+ def get_box_metrics(self, pd_scores, pd_bboxes, gt_labels, gt_bboxes, mask_gt):
+ """Compute alignment metric given predicted and ground truth bounding boxes."""
+ na = pd_bboxes.shape[-2]
+ mask_gt = mask_gt.bool() # b, max_num_obj, h*w
+ overlaps = torch.zeros([self.bs, self.n_max_boxes, na], dtype=pd_bboxes.dtype, device=pd_bboxes.device)
+ bbox_scores = torch.zeros([self.bs, self.n_max_boxes, na], dtype=pd_scores.dtype, device=pd_scores.device)
+
+ ind = torch.zeros([2, self.bs, self.n_max_boxes], dtype=torch.long) # 2, b, max_num_obj
+ ind[0] = torch.arange(end=self.bs).view(-1, 1).expand(-1, self.n_max_boxes) # b, max_num_obj
+ ind[1] = gt_labels.squeeze(-1) # b, max_num_obj
+ # Get the scores of each grid for each gt cls
+ bbox_scores[mask_gt] = pd_scores[ind[0], :, ind[1]][mask_gt] # b, max_num_obj, h*w
+
+ # (b, max_num_obj, 1, 4), (b, 1, h*w, 4)
+ pd_boxes = pd_bboxes.unsqueeze(1).expand(-1, self.n_max_boxes, -1, -1)[mask_gt]
+ gt_boxes = gt_bboxes.unsqueeze(2).expand(-1, -1, na, -1)[mask_gt]
+ overlaps[mask_gt] = bbox_iou(gt_boxes, pd_boxes, xywh=False, CIoU=True).squeeze(-1).clamp_(0)
+
+ align_metric = bbox_scores.pow(self.alpha) * overlaps.pow(self.beta)
+ return align_metric, overlaps
+
+ def select_topk_candidates(self, metrics, largest=True, topk_mask=None):
+ """
+ Select the top-k candidates based on the given metrics.
+
+ Args:
+ metrics (Tensor): A tensor of shape (b, max_num_obj, h*w), where b is the batch size,
+ max_num_obj is the maximum number of objects, and h*w represents the
+ total number of anchor points.
+ largest (bool): If True, select the largest values; otherwise, select the smallest values.
+ topk_mask (Tensor): An optional boolean tensor of shape (b, max_num_obj, topk), where
+ topk is the number of top candidates to consider. If not provided,
+ the top-k values are automatically computed based on the given metrics.
+
+ Returns:
+ (Tensor): A tensor of shape (b, max_num_obj, h*w) containing the selected top-k candidates.
+ """
+
+ # (b, max_num_obj, topk)
+ topk_metrics, topk_idxs = torch.topk(metrics, self.topk, dim=-1, largest=largest)
+ if topk_mask is None:
+ topk_mask = (topk_metrics.max(-1, keepdim=True)[0] > self.eps).expand_as(topk_idxs)
+ # (b, max_num_obj, topk)
+ topk_idxs.masked_fill_(~topk_mask, 0)
+
+ # (b, max_num_obj, topk, h*w) -> (b, max_num_obj, h*w)
+ count_tensor = torch.zeros(metrics.shape, dtype=torch.int8, device=topk_idxs.device)
+ ones = torch.ones_like(topk_idxs[:, :, :1], dtype=torch.int8, device=topk_idxs.device)
+ for k in range(self.topk):
+ # Expand topk_idxs for each value of k and add 1 at the specified positions
+ count_tensor.scatter_add_(-1, topk_idxs[:, :, k:k + 1], ones)
+ # count_tensor.scatter_add_(-1, topk_idxs, torch.ones_like(topk_idxs, dtype=torch.int8, device=topk_idxs.device))
+ # filter invalid bboxes
+ count_tensor.masked_fill_(count_tensor > 1, 0)
+
+ return count_tensor.to(metrics.dtype)
+
+ def get_targets(self, gt_labels, gt_bboxes, target_gt_idx, fg_mask):
+ """
+ Compute target labels, target bounding boxes, and target scores for the positive anchor points.
+
+ Args:
+ gt_labels (Tensor): Ground truth labels of shape (b, max_num_obj, 1), where b is the
+ batch size and max_num_obj is the maximum number of objects.
+ gt_bboxes (Tensor): Ground truth bounding boxes of shape (b, max_num_obj, 4).
+ target_gt_idx (Tensor): Indices of the assigned ground truth objects for positive
+ anchor points, with shape (b, h*w), where h*w is the total
+ number of anchor points.
+ fg_mask (Tensor): A boolean tensor of shape (b, h*w) indicating the positive
+ (foreground) anchor points.
+
+ Returns:
+ (Tuple[Tensor, Tensor, Tensor]): A tuple containing the following tensors:
+ - target_labels (Tensor): Shape (b, h*w), containing the target labels for
+ positive anchor points.
+ - target_bboxes (Tensor): Shape (b, h*w, 4), containing the target bounding boxes
+ for positive anchor points.
+ - target_scores (Tensor): Shape (b, h*w, num_classes), containing the target scores
+ for positive anchor points, where num_classes is the number
+ of object classes.
+ """
+
+ # Assigned target labels, (b, 1)
+ batch_ind = torch.arange(end=self.bs, dtype=torch.int64, device=gt_labels.device)[..., None]
+ target_gt_idx = target_gt_idx + batch_ind * self.n_max_boxes # (b, h*w)
+ target_labels = gt_labels.long().flatten()[target_gt_idx] # (b, h*w)
+
+ # Assigned target boxes, (b, max_num_obj, 4) -> (b, h*w)
+ target_bboxes = gt_bboxes.view(-1, 4)[target_gt_idx]
+
+ # Assigned target scores
+ target_labels.clamp_(0)
+
+ # 10x faster than F.one_hot()
+ target_scores = torch.zeros((target_labels.shape[0], target_labels.shape[1], self.num_classes),
+ dtype=torch.int64,
+ device=target_labels.device) # (b, h*w, 80)
+ target_scores.scatter_(2, target_labels.unsqueeze(-1), 1)
+
+ fg_scores_mask = fg_mask[:, :, None].repeat(1, 1, self.num_classes) # (b, h*w, 80)
+ target_scores = torch.where(fg_scores_mask > 0, target_scores, 0)
+
+ return target_labels, target_bboxes, target_scores
+
+
+def make_anchors(feats, strides, grid_cell_offset=0.5):
+ """Generate anchors from features."""
+ anchor_points, stride_tensor = [], []
+ assert feats is not None
+ dtype, device = feats[0].dtype, feats[0].device
+ for i, stride in enumerate(strides):
+ _, _, h, w = feats[i].shape
+ sx = torch.arange(end=w, device=device, dtype=dtype) + grid_cell_offset # shift x
+ sy = torch.arange(end=h, device=device, dtype=dtype) + grid_cell_offset # shift y
+ sy, sx = torch.meshgrid(sy, sx, indexing='ij') if TORCH_1_10 else torch.meshgrid(sy, sx)
+ anchor_points.append(torch.stack((sx, sy), -1).view(-1, 2))
+ stride_tensor.append(torch.full((h * w, 1), stride, dtype=dtype, device=device))
+ return torch.cat(anchor_points), torch.cat(stride_tensor)
+
+
+def dist2bbox(distance, anchor_points, xywh=True, dim=-1):
+ """Transform distance(ltrb) to box(xywh or xyxy)."""
+ lt, rb = distance.chunk(2, dim)
+ x1y1 = anchor_points - lt
+ x2y2 = anchor_points + rb
+ if xywh:
+ c_xy = (x1y1 + x2y2) / 2
+ wh = x2y2 - x1y1
+ return torch.cat((c_xy, wh), dim) # xywh bbox
+ return torch.cat((x1y1, x2y2), dim) # xyxy bbox
+
+
+def bbox2dist(anchor_points, bbox, reg_max):
+ """Transform bbox(xyxy) to dist(ltrb)."""
+ x1y1, x2y2 = bbox.chunk(2, -1)
+ return torch.cat((anchor_points - x1y1, x2y2 - anchor_points), -1).clamp_(0, reg_max - 0.01) # dist (lt, rb)
diff --git a/yolov8/ultralytics/yolo/utils/torch_utils.py b/yolov8/ultralytics/yolo/utils/torch_utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..e5a62dc5ed5a8bb80e6cb12b44fa8a56a47abc6a
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/torch_utils.py
@@ -0,0 +1,505 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import math
+import os
+import platform
+import random
+import time
+from contextlib import contextmanager
+from copy import deepcopy
+from pathlib import Path
+from typing import Union
+
+import numpy as np
+import torch
+import torch.distributed as dist
+import torch.nn as nn
+import torch.nn.functional as F
+import torchvision
+
+from ultralytics.yolo.utils import DEFAULT_CFG_DICT, DEFAULT_CFG_KEYS, LOGGER, RANK, __version__
+from ultralytics.yolo.utils.checks import check_version
+
+try:
+ import thop
+except ImportError:
+ thop = None
+
+TORCHVISION_0_10 = check_version(torchvision.__version__, '0.10.0')
+TORCH_1_9 = check_version(torch.__version__, '1.9.0')
+TORCH_1_11 = check_version(torch.__version__, '1.11.0')
+TORCH_1_12 = check_version(torch.__version__, '1.12.0')
+TORCH_2_0 = check_version(torch.__version__, minimum='2.0')
+
+
+@contextmanager
+def torch_distributed_zero_first(local_rank: int):
+ """Decorator to make all processes in distributed training wait for each local_master to do something."""
+ initialized = torch.distributed.is_available() and torch.distributed.is_initialized()
+ if initialized and local_rank not in (-1, 0):
+ dist.barrier(device_ids=[local_rank])
+ yield
+ if initialized and local_rank == 0:
+ dist.barrier(device_ids=[0])
+
+
+def smart_inference_mode():
+ """Applies torch.inference_mode() decorator if torch>=1.9.0 else torch.no_grad() decorator."""
+
+ def decorate(fn):
+ """Applies appropriate torch decorator for inference mode based on torch version."""
+ return (torch.inference_mode if TORCH_1_9 else torch.no_grad)()(fn)
+
+ return decorate
+
+
+def select_device(device='', batch=0, newline=False, verbose=True):
+ """Selects PyTorch Device. Options are device = None or 'cpu' or 0 or '0' or '0,1,2,3'."""
+ s = f'Ultralytics YOLOv{__version__} 🚀 Python-{platform.python_version()} torch-{torch.__version__} '
+ device = str(device).lower()
+ for remove in 'cuda:', 'none', '(', ')', '[', ']', "'", ' ':
+ device = device.replace(remove, '') # to string, 'cuda:0' -> '0' and '(0, 1)' -> '0,1'
+ cpu = device == 'cpu'
+ mps = device == 'mps' # Apple Metal Performance Shaders (MPS)
+ if cpu or mps:
+ os.environ['CUDA_VISIBLE_DEVICES'] = '-1' # force torch.cuda.is_available() = False
+ elif device: # non-cpu device requested
+ if device == 'cuda':
+ device = '0'
+ visible = os.environ.get('CUDA_VISIBLE_DEVICES', None)
+ os.environ['CUDA_VISIBLE_DEVICES'] = device # set environment variable - must be before assert is_available()
+ if not (torch.cuda.is_available() and torch.cuda.device_count() >= len(device.replace(',', ''))):
+ LOGGER.info(s)
+ install = 'See https://pytorch.org/get-started/locally/ for up-to-date torch install instructions if no ' \
+ 'CUDA devices are seen by torch.\n' if torch.cuda.device_count() == 0 else ''
+ raise ValueError(f"Invalid CUDA 'device={device}' requested."
+ f" Use 'device=cpu' or pass valid CUDA device(s) if available,"
+ f" i.e. 'device=0' or 'device=0,1,2,3' for Multi-GPU.\n"
+ f'\ntorch.cuda.is_available(): {torch.cuda.is_available()}'
+ f'\ntorch.cuda.device_count(): {torch.cuda.device_count()}'
+ f"\nos.environ['CUDA_VISIBLE_DEVICES']: {visible}\n"
+ f'{install}')
+
+ if not cpu and not mps and torch.cuda.is_available(): # prefer GPU if available
+ devices = device.split(',') if device else '0' # range(torch.cuda.device_count()) # i.e. 0,1,6,7
+ n = len(devices) # device count
+ if n > 1 and batch > 0 and batch % n != 0: # check batch_size is divisible by device_count
+ raise ValueError(f"'batch={batch}' must be a multiple of GPU count {n}. Try 'batch={batch // n * n}' or "
+ f"'batch={batch // n * n + n}', the nearest batch sizes evenly divisible by {n}.")
+ space = ' ' * (len(s) + 1)
+ for i, d in enumerate(devices):
+ p = torch.cuda.get_device_properties(i)
+ s += f"{'' if i == 0 else space}CUDA:{d} ({p.name}, {p.total_memory / (1 << 20):.0f}MiB)\n" # bytes to MB
+ arg = 'cuda:0'
+ elif mps and getattr(torch, 'has_mps', False) and torch.backends.mps.is_available() and TORCH_2_0:
+ # Prefer MPS if available
+ s += 'MPS\n'
+ arg = 'mps'
+ else: # revert to CPU
+ s += 'CPU\n'
+ arg = 'cpu'
+
+ if verbose and RANK == -1:
+ LOGGER.info(s if newline else s.rstrip())
+ return torch.device(arg)
+
+
+def time_sync():
+ """PyTorch-accurate time."""
+ if torch.cuda.is_available():
+ torch.cuda.synchronize()
+ return time.time()
+
+
+def fuse_conv_and_bn(conv, bn):
+ """Fuse Conv2d() and BatchNorm2d() layers https://tehnokv.com/posts/fusing-batchnorm-and-conv/."""
+ fusedconv = nn.Conv2d(conv.in_channels,
+ conv.out_channels,
+ kernel_size=conv.kernel_size,
+ stride=conv.stride,
+ padding=conv.padding,
+ dilation=conv.dilation,
+ groups=conv.groups,
+ bias=True).requires_grad_(False).to(conv.weight.device)
+
+ # Prepare filters
+ w_conv = conv.weight.clone().view(conv.out_channels, -1)
+ w_bn = torch.diag(bn.weight.div(torch.sqrt(bn.eps + bn.running_var)))
+ fusedconv.weight.copy_(torch.mm(w_bn, w_conv).view(fusedconv.weight.shape))
+
+ # Prepare spatial bias
+ b_conv = torch.zeros(conv.weight.size(0), device=conv.weight.device) if conv.bias is None else conv.bias
+ b_bn = bn.bias - bn.weight.mul(bn.running_mean).div(torch.sqrt(bn.running_var + bn.eps))
+ fusedconv.bias.copy_(torch.mm(w_bn, b_conv.reshape(-1, 1)).reshape(-1) + b_bn)
+
+ return fusedconv
+
+
+def fuse_deconv_and_bn(deconv, bn):
+ """Fuse ConvTranspose2d() and BatchNorm2d() layers."""
+ fuseddconv = nn.ConvTranspose2d(deconv.in_channels,
+ deconv.out_channels,
+ kernel_size=deconv.kernel_size,
+ stride=deconv.stride,
+ padding=deconv.padding,
+ output_padding=deconv.output_padding,
+ dilation=deconv.dilation,
+ groups=deconv.groups,
+ bias=True).requires_grad_(False).to(deconv.weight.device)
+
+ # Prepare filters
+ w_deconv = deconv.weight.clone().view(deconv.out_channels, -1)
+ w_bn = torch.diag(bn.weight.div(torch.sqrt(bn.eps + bn.running_var)))
+ fuseddconv.weight.copy_(torch.mm(w_bn, w_deconv).view(fuseddconv.weight.shape))
+
+ # Prepare spatial bias
+ b_conv = torch.zeros(deconv.weight.size(1), device=deconv.weight.device) if deconv.bias is None else deconv.bias
+ b_bn = bn.bias - bn.weight.mul(bn.running_mean).div(torch.sqrt(bn.running_var + bn.eps))
+ fuseddconv.bias.copy_(torch.mm(w_bn, b_conv.reshape(-1, 1)).reshape(-1) + b_bn)
+
+ return fuseddconv
+
+
+def model_info(model, detailed=False, verbose=True, imgsz=640):
+ """Model information. imgsz may be int or list, i.e. imgsz=640 or imgsz=[640, 320]."""
+ if not verbose:
+ return
+ n_p = get_num_params(model) # number of parameters
+ n_g = get_num_gradients(model) # number of gradients
+ n_l = len(list(model.modules())) # number of layers
+ if detailed:
+ LOGGER.info(
+ f"{'layer':>5} {'name':>40} {'gradient':>9} {'parameters':>12} {'shape':>20} {'mu':>10} {'sigma':>10}")
+ for i, (name, p) in enumerate(model.named_parameters()):
+ name = name.replace('module_list.', '')
+ LOGGER.info('%5g %40s %9s %12g %20s %10.3g %10.3g %10s' %
+ (i, name, p.requires_grad, p.numel(), list(p.shape), p.mean(), p.std(), p.dtype))
+
+ flops = get_flops(model, imgsz)
+ fused = ' (fused)' if getattr(model, 'is_fused', lambda: False)() else ''
+ fs = f', {flops:.1f} GFLOPs' if flops else ''
+ yaml_file = getattr(model, 'yaml_file', '') or getattr(model, 'yaml', {}).get('yaml_file', '')
+ model_name = Path(yaml_file).stem.replace('yolo', 'YOLO') or 'Model'
+ LOGGER.info(f'{model_name} summary{fused}: {n_l} layers, {n_p} parameters, {n_g} gradients{fs}')
+ return n_l, n_p, n_g, flops
+
+
+def get_num_params(model):
+ """Return the total number of parameters in a YOLO model."""
+ return sum(x.numel() for x in model.parameters())
+
+
+def get_num_gradients(model):
+ """Return the total number of parameters with gradients in a YOLO model."""
+ return sum(x.numel() for x in model.parameters() if x.requires_grad)
+
+
+def model_info_for_loggers(trainer):
+ """
+ Return model info dict with useful model information.
+
+ Example for YOLOv8n:
+ {'model/parameters': 3151904,
+ 'model/GFLOPs': 8.746,
+ 'model/speed_ONNX(ms)': 41.244,
+ 'model/speed_TensorRT(ms)': 3.211,
+ 'model/speed_PyTorch(ms)': 18.755}
+ """
+ if trainer.args.profile: # profile ONNX and TensorRT times
+ from ultralytics.yolo.utils.benchmarks import ProfileModels
+ results = ProfileModels([trainer.last], device=trainer.device).profile()[0]
+ results.pop('model/name')
+ else: # only return PyTorch times from most recent validation
+ results = {
+ 'model/parameters': get_num_params(trainer.model),
+ 'model/GFLOPs': round(get_flops(trainer.model), 3)}
+ results['model/speed_PyTorch(ms)'] = round(trainer.validator.speed['inference'], 3)
+ return results
+
+
+def get_flops(model, imgsz=640):
+ """Return a YOLO model's FLOPs."""
+ try:
+ model = de_parallel(model)
+ p = next(model.parameters())
+ stride = max(int(model.stride.max()), 32) if hasattr(model, 'stride') else 32 # max stride
+ im = torch.empty((1, p.shape[1], stride, stride), device=p.device) # input image in BCHW format
+ flops = thop.profile(deepcopy(model), inputs=[im], verbose=False)[0] / 1E9 * 2 if thop else 0 # stride GFLOPs
+ imgsz = imgsz if isinstance(imgsz, list) else [imgsz, imgsz] # expand if int/float
+ return flops * imgsz[0] / stride * imgsz[1] / stride # 640x640 GFLOPs
+ except Exception:
+ return 0
+
+
+def get_flops_with_torch_profiler(model, imgsz=640):
+ # Compute model FLOPs (thop alternative)
+ model = de_parallel(model)
+ p = next(model.parameters())
+ stride = (max(int(model.stride.max()), 32) if hasattr(model, 'stride') else 32) * 2 # max stride
+ im = torch.zeros((1, p.shape[1], stride, stride), device=p.device) # input image in BCHW format
+ with torch.profiler.profile(with_flops=True) as prof:
+ model(im)
+ flops = sum(x.flops for x in prof.key_averages()) / 1E9
+ imgsz = imgsz if isinstance(imgsz, list) else [imgsz, imgsz] # expand if int/float
+ flops = flops * imgsz[0] / stride * imgsz[1] / stride # 640x640 GFLOPs
+ return flops
+
+
+def initialize_weights(model):
+ """Initialize model weights to random values."""
+ for m in model.modules():
+ t = type(m)
+ if t is nn.Conv2d:
+ pass # nn.init.kaiming_normal_(m.weight, mode='fan_out', nonlinearity='relu')
+ elif t is nn.BatchNorm2d:
+ m.eps = 1e-3
+ m.momentum = 0.03
+ elif t in [nn.Hardswish, nn.LeakyReLU, nn.ReLU, nn.ReLU6, nn.SiLU]:
+ m.inplace = True
+
+
+def scale_img(img, ratio=1.0, same_shape=False, gs=32): # img(16,3,256,416)
+ # Scales img(bs,3,y,x) by ratio constrained to gs-multiple
+ if ratio == 1.0:
+ return img
+ h, w = img.shape[2:]
+ s = (int(h * ratio), int(w * ratio)) # new size
+ img = F.interpolate(img, size=s, mode='bilinear', align_corners=False) # resize
+ if not same_shape: # pad/crop img
+ h, w = (math.ceil(x * ratio / gs) * gs for x in (h, w))
+ return F.pad(img, [0, w - s[1], 0, h - s[0]], value=0.447) # value = imagenet mean
+
+
+def make_divisible(x, divisor):
+ """Returns nearest x divisible by divisor."""
+ if isinstance(divisor, torch.Tensor):
+ divisor = int(divisor.max()) # to int
+ return math.ceil(x / divisor) * divisor
+
+
+def copy_attr(a, b, include=(), exclude=()):
+ """Copies attributes from object 'b' to object 'a', with options to include/exclude certain attributes."""
+ for k, v in b.__dict__.items():
+ if (len(include) and k not in include) or k.startswith('_') or k in exclude:
+ continue
+ else:
+ setattr(a, k, v)
+
+
+def get_latest_opset():
+ """Return second-most (for maturity) recently supported ONNX opset by this version of torch."""
+ return max(int(k[14:]) for k in vars(torch.onnx) if 'symbolic_opset' in k) - 1 # opset
+
+
+def intersect_dicts(da, db, exclude=()):
+ """Returns a dictionary of intersecting keys with matching shapes, excluding 'exclude' keys, using da values."""
+ return {k: v for k, v in da.items() if k in db and all(x not in k for x in exclude) and v.shape == db[k].shape}
+
+
+def is_parallel(model):
+ """Returns True if model is of type DP or DDP."""
+ return isinstance(model, (nn.parallel.DataParallel, nn.parallel.DistributedDataParallel))
+
+
+def de_parallel(model):
+ """De-parallelize a model: returns single-GPU model if model is of type DP or DDP."""
+ return model.module if is_parallel(model) else model
+
+
+def one_cycle(y1=0.0, y2=1.0, steps=100):
+ """Returns a lambda function for sinusoidal ramp from y1 to y2 https://arxiv.org/pdf/1812.01187.pdf."""
+ return lambda x: ((1 - math.cos(x * math.pi / steps)) / 2) * (y2 - y1) + y1
+
+
+def init_seeds(seed=0, deterministic=False):
+ """Initialize random number generator (RNG) seeds https://pytorch.org/docs/stable/notes/randomness.html."""
+ random.seed(seed)
+ np.random.seed(seed)
+ torch.manual_seed(seed)
+ torch.cuda.manual_seed(seed)
+ torch.cuda.manual_seed_all(seed) # for Multi-GPU, exception safe
+ # torch.backends.cudnn.benchmark = True # AutoBatch problem https://github.com/ultralytics/yolov5/issues/9287
+ if deterministic:
+ if TORCH_2_0:
+ torch.use_deterministic_algorithms(True, warn_only=True) # warn if deterministic is not possible
+ torch.backends.cudnn.deterministic = True
+ os.environ['CUBLAS_WORKSPACE_CONFIG'] = ':4096:8'
+ os.environ['PYTHONHASHSEED'] = str(seed)
+ else:
+ LOGGER.warning('WARNING ⚠️ Upgrade to torch>=2.0.0 for deterministic training.')
+ else:
+ torch.use_deterministic_algorithms(False)
+ torch.backends.cudnn.deterministic = False
+
+
+class ModelEMA:
+ """Updated Exponential Moving Average (EMA) from https://github.com/rwightman/pytorch-image-models
+ Keeps a moving average of everything in the model state_dict (parameters and buffers)
+ For EMA details see https://www.tensorflow.org/api_docs/python/tf/train/ExponentialMovingAverage
+ To disable EMA set the `enabled` attribute to `False`.
+ """
+
+ def __init__(self, model, decay=0.9999, tau=2000, updates=0):
+ """Create EMA."""
+ self.ema = deepcopy(de_parallel(model)).eval() # FP32 EMA
+ self.updates = updates # number of EMA updates
+ self.decay = lambda x: decay * (1 - math.exp(-x / tau)) # decay exponential ramp (to help early epochs)
+ for p in self.ema.parameters():
+ p.requires_grad_(False)
+ self.enabled = True
+
+ def update(self, model):
+ """Update EMA parameters."""
+ if self.enabled:
+ self.updates += 1
+ d = self.decay(self.updates)
+
+ msd = de_parallel(model).state_dict() # model state_dict
+ for k, v in self.ema.state_dict().items():
+ if v.dtype.is_floating_point: # true for FP16 and FP32
+ v *= d
+ v += (1 - d) * msd[k].detach()
+ # assert v.dtype == msd[k].dtype == torch.float32, f'{k}: EMA {v.dtype}, model {msd[k].dtype}'
+
+ def update_attr(self, model, include=(), exclude=('process_group', 'reducer')):
+ """Updates attributes and saves stripped model with optimizer removed."""
+ if self.enabled:
+ copy_attr(self.ema, model, include, exclude)
+
+
+def strip_optimizer(f: Union[str, Path] = 'best.pt', s: str = '') -> None:
+ """
+ Strip optimizer from 'f' to finalize training, optionally save as 's'.
+
+ Args:
+ f (str): file path to model to strip the optimizer from. Default is 'best.pt'.
+ s (str): file path to save the model with stripped optimizer to. If not provided, 'f' will be overwritten.
+
+ Returns:
+ None
+
+ Usage:
+ from pathlib import Path
+ from ultralytics.yolo.utils.torch_utils import strip_optimizer
+ for f in Path('/Users/glennjocher/Downloads/weights').rglob('*.pt'):
+ strip_optimizer(f)
+ """
+ # Use dill (if exists) to serialize the lambda functions where pickle does not do this
+ try:
+ import dill as pickle
+ except ImportError:
+ import pickle
+
+ x = torch.load(f, map_location=torch.device('cpu'))
+ args = {**DEFAULT_CFG_DICT, **x['train_args']} if 'train_args' in x else None # combine args
+ if x.get('ema'):
+ x['model'] = x['ema'] # replace model with ema
+ for k in 'optimizer', 'best_fitness', 'ema', 'updates': # keys
+ x[k] = None
+ x['epoch'] = -1
+ x['model'].half() # to FP16
+ for p in x['model'].parameters():
+ p.requires_grad = False
+ x['train_args'] = {k: v for k, v in args.items() if k in DEFAULT_CFG_KEYS} # strip non-default keys
+ # x['model'].args = x['train_args']
+ torch.save(x, s or f, pickle_module=pickle)
+ mb = os.path.getsize(s or f) / 1E6 # filesize
+ LOGGER.info(f"Optimizer stripped from {f},{f' saved as {s},' if s else ''} {mb:.1f}MB")
+
+
+def profile(input, ops, n=10, device=None):
+ """
+ YOLOv8 speed/memory/FLOPs profiler
+
+ Usage:
+ input = torch.randn(16, 3, 640, 640)
+ m1 = lambda x: x * torch.sigmoid(x)
+ m2 = nn.SiLU()
+ profile(input, [m1, m2], n=100) # profile over 100 iterations
+ """
+ results = []
+ if not isinstance(device, torch.device):
+ device = select_device(device)
+ LOGGER.info(f"{'Params':>12s}{'GFLOPs':>12s}{'GPU_mem (GB)':>14s}{'forward (ms)':>14s}{'backward (ms)':>14s}"
+ f"{'input':>24s}{'output':>24s}")
+
+ for x in input if isinstance(input, list) else [input]:
+ x = x.to(device)
+ x.requires_grad = True
+ for m in ops if isinstance(ops, list) else [ops]:
+ m = m.to(device) if hasattr(m, 'to') else m # device
+ m = m.half() if hasattr(m, 'half') and isinstance(x, torch.Tensor) and x.dtype is torch.float16 else m
+ tf, tb, t = 0, 0, [0, 0, 0] # dt forward, backward
+ try:
+ flops = thop.profile(m, inputs=[x], verbose=False)[0] / 1E9 * 2 if thop else 0 # GFLOPs
+ except Exception:
+ flops = 0
+
+ try:
+ for _ in range(n):
+ t[0] = time_sync()
+ y = m(x)
+ t[1] = time_sync()
+ try:
+ _ = (sum(yi.sum() for yi in y) if isinstance(y, list) else y).sum().backward()
+ t[2] = time_sync()
+ except Exception: # no backward method
+ # print(e) # for debug
+ t[2] = float('nan')
+ tf += (t[1] - t[0]) * 1000 / n # ms per op forward
+ tb += (t[2] - t[1]) * 1000 / n # ms per op backward
+ mem = torch.cuda.memory_reserved() / 1E9 if torch.cuda.is_available() else 0 # (GB)
+ s_in, s_out = (tuple(x.shape) if isinstance(x, torch.Tensor) else 'list' for x in (x, y)) # shapes
+ p = sum(x.numel() for x in m.parameters()) if isinstance(m, nn.Module) else 0 # parameters
+ LOGGER.info(f'{p:12}{flops:12.4g}{mem:>14.3f}{tf:14.4g}{tb:14.4g}{str(s_in):>24s}{str(s_out):>24s}')
+ results.append([p, flops, mem, tf, tb, s_in, s_out])
+ except Exception as e:
+ LOGGER.info(e)
+ results.append(None)
+ torch.cuda.empty_cache()
+ return results
+
+
+class EarlyStopping:
+ """
+ Early stopping class that stops training when a specified number of epochs have passed without improvement.
+ """
+
+ def __init__(self, patience=50):
+ """
+ Initialize early stopping object
+
+ Args:
+ patience (int, optional): Number of epochs to wait after fitness stops improving before stopping.
+ """
+ self.best_fitness = 0.0 # i.e. mAP
+ self.best_epoch = 0
+ self.patience = patience or float('inf') # epochs to wait after fitness stops improving to stop
+ self.possible_stop = False # possible stop may occur next epoch
+
+ def __call__(self, epoch, fitness):
+ """
+ Check whether to stop training
+
+ Args:
+ epoch (int): Current epoch of training
+ fitness (float): Fitness value of current epoch
+
+ Returns:
+ (bool): True if training should stop, False otherwise
+ """
+ if fitness is None: # check if fitness=None (happens when val=False)
+ return False
+
+ if fitness >= self.best_fitness: # >= 0 to allow for early zero-fitness stage of training
+ self.best_epoch = epoch
+ self.best_fitness = fitness
+ delta = epoch - self.best_epoch # epochs without improvement
+ self.possible_stop = delta >= (self.patience - 1) # possible stop may occur next epoch
+ stop = delta >= self.patience # stop training if patience exceeded
+ if stop:
+ LOGGER.info(f'Stopping training early as no improvement observed in last {self.patience} epochs. '
+ f'Best results observed at epoch {self.best_epoch}, best model saved as best.pt.\n'
+ f'To update EarlyStopping(patience={self.patience}) pass a new patience value, '
+ f'i.e. `patience=300` or use `patience=0` to disable EarlyStopping.')
+ return stop
diff --git a/yolov8/ultralytics/yolo/utils/tuner.py b/yolov8/ultralytics/yolo/utils/tuner.py
new file mode 100644
index 0000000000000000000000000000000000000000..54f10b054308d2e98228a4d752929204b092dae4
--- /dev/null
+++ b/yolov8/ultralytics/yolo/utils/tuner.py
@@ -0,0 +1,120 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+from ultralytics.yolo.cfg import TASK2DATA, TASK2METRIC
+from ultralytics.yolo.utils import DEFAULT_CFG_DICT, LOGGER, NUM_THREADS
+
+
+def run_ray_tune(model,
+ space: dict = None,
+ grace_period: int = 10,
+ gpu_per_trial: int = None,
+ max_samples: int = 10,
+ **train_args):
+ """
+ Runs hyperparameter tuning using Ray Tune.
+
+ Args:
+ model (YOLO): Model to run the tuner on.
+ space (dict, optional): The hyperparameter search space. Defaults to None.
+ grace_period (int, optional): The grace period in epochs of the ASHA scheduler. Defaults to 10.
+ gpu_per_trial (int, optional): The number of GPUs to allocate per trial. Defaults to None.
+ max_samples (int, optional): The maximum number of trials to run. Defaults to 10.
+ train_args (dict, optional): Additional arguments to pass to the `train()` method. Defaults to {}.
+
+ Returns:
+ (dict): A dictionary containing the results of the hyperparameter search.
+
+ Raises:
+ ModuleNotFoundError: If Ray Tune is not installed.
+ """
+ if train_args is None:
+ train_args = {}
+
+ try:
+ from ray import tune
+ from ray.air import RunConfig
+ from ray.air.integrations.wandb import WandbLoggerCallback
+ from ray.tune.schedulers import ASHAScheduler
+ except ImportError:
+ raise ModuleNotFoundError("Tuning hyperparameters requires Ray Tune. Install with: pip install 'ray[tune]'")
+
+ try:
+ import wandb
+
+ assert hasattr(wandb, '__version__')
+ except (ImportError, AssertionError):
+ wandb = False
+
+ default_space = {
+ # 'optimizer': tune.choice(['SGD', 'Adam', 'AdamW', 'NAdam', 'RAdam', 'RMSProp']),
+ 'lr0': tune.uniform(1e-5, 1e-1),
+ 'lrf': tune.uniform(0.01, 1.0), # final OneCycleLR learning rate (lr0 * lrf)
+ 'momentum': tune.uniform(0.6, 0.98), # SGD momentum/Adam beta1
+ 'weight_decay': tune.uniform(0.0, 0.001), # optimizer weight decay 5e-4
+ 'warmup_epochs': tune.uniform(0.0, 5.0), # warmup epochs (fractions ok)
+ 'warmup_momentum': tune.uniform(0.0, 0.95), # warmup initial momentum
+ 'box': tune.uniform(0.02, 0.2), # box loss gain
+ 'cls': tune.uniform(0.2, 4.0), # cls loss gain (scale with pixels)
+ 'hsv_h': tune.uniform(0.0, 0.1), # image HSV-Hue augmentation (fraction)
+ 'hsv_s': tune.uniform(0.0, 0.9), # image HSV-Saturation augmentation (fraction)
+ 'hsv_v': tune.uniform(0.0, 0.9), # image HSV-Value augmentation (fraction)
+ 'degrees': tune.uniform(0.0, 45.0), # image rotation (+/- deg)
+ 'translate': tune.uniform(0.0, 0.9), # image translation (+/- fraction)
+ 'scale': tune.uniform(0.0, 0.9), # image scale (+/- gain)
+ 'shear': tune.uniform(0.0, 10.0), # image shear (+/- deg)
+ 'perspective': tune.uniform(0.0, 0.001), # image perspective (+/- fraction), range 0-0.001
+ 'flipud': tune.uniform(0.0, 1.0), # image flip up-down (probability)
+ 'fliplr': tune.uniform(0.0, 1.0), # image flip left-right (probability)
+ 'mosaic': tune.uniform(0.0, 1.0), # image mixup (probability)
+ 'mixup': tune.uniform(0.0, 1.0), # image mixup (probability)
+ 'copy_paste': tune.uniform(0.0, 1.0)} # segment copy-paste (probability)
+
+ def _tune(config):
+ """
+ Trains the YOLO model with the specified hyperparameters and additional arguments.
+
+ Args:
+ config (dict): A dictionary of hyperparameters to use for training.
+
+ Returns:
+ None.
+ """
+ model._reset_callbacks()
+ config.update(train_args)
+ model.train(**config)
+
+ # Get search space
+ if not space:
+ space = default_space
+ LOGGER.warning('WARNING ⚠️ search space not provided, using default search space.')
+
+ # Get dataset
+ data = train_args.get('data', TASK2DATA[model.task])
+ space['data'] = data
+ if 'data' not in train_args:
+ LOGGER.warning(f'WARNING ⚠️ data not provided, using default "data={data}".')
+
+ # Define the trainable function with allocated resources
+ trainable_with_resources = tune.with_resources(_tune, {'cpu': NUM_THREADS, 'gpu': gpu_per_trial or 0})
+
+ # Define the ASHA scheduler for hyperparameter search
+ asha_scheduler = ASHAScheduler(time_attr='epoch',
+ metric=TASK2METRIC[model.task],
+ mode='max',
+ max_t=train_args.get('epochs') or DEFAULT_CFG_DICT['epochs'] or 100,
+ grace_period=grace_period,
+ reduction_factor=3)
+
+ # Define the callbacks for the hyperparameter search
+ tuner_callbacks = [WandbLoggerCallback(project='YOLOv8-tune')] if wandb else []
+
+ # Create the Ray Tune hyperparameter search tuner
+ tuner = tune.Tuner(trainable_with_resources,
+ param_space=space,
+ tune_config=tune.TuneConfig(scheduler=asha_scheduler, num_samples=max_samples),
+ run_config=RunConfig(callbacks=tuner_callbacks, storage_path='./runs/tune'))
+
+ # Run the hyperparameter search
+ tuner.fit()
+
+ # Return the results of the hyperparameter search
+ return tuner.get_results()
diff --git a/yolov8/ultralytics/yolo/v8/__init__.py b/yolov8/ultralytics/yolo/v8/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..adc0351ba42fe55a71d3e8cf236809e2408da207
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/__init__.py
@@ -0,0 +1,5 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from ultralytics.yolo.v8 import classify, detect, pose, segment
+
+__all__ = 'classify', 'segment', 'detect', 'pose'
diff --git a/yolov8/ultralytics/yolo/v8/classify/__init__.py b/yolov8/ultralytics/yolo/v8/classify/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..2f049ed33b2ac74535c3b4a52a7e88b3c9299ed4
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/classify/__init__.py
@@ -0,0 +1,7 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from ultralytics.yolo.v8.classify.predict import ClassificationPredictor, predict
+from ultralytics.yolo.v8.classify.train import ClassificationTrainer, train
+from ultralytics.yolo.v8.classify.val import ClassificationValidator, val
+
+__all__ = 'ClassificationPredictor', 'predict', 'ClassificationTrainer', 'train', 'ClassificationValidator', 'val'
diff --git a/yolov8/ultralytics/yolo/v8/classify/predict.py b/yolov8/ultralytics/yolo/v8/classify/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..fb486e292e40671a410199b7de27e05213a57341
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/classify/predict.py
@@ -0,0 +1,51 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.engine.predictor import BasePredictor
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils import DEFAULT_CFG, ROOT
+
+
+class ClassificationPredictor(BasePredictor):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ super().__init__(cfg, overrides, _callbacks)
+ self.args.task = 'classify'
+
+ def preprocess(self, img):
+ """Converts input image to model-compatible data type."""
+ if not isinstance(img, torch.Tensor):
+ img = torch.stack([self.transforms(im) for im in img], dim=0)
+ img = (img if isinstance(img, torch.Tensor) else torch.from_numpy(img)).to(self.model.device)
+ return img.half() if self.model.fp16 else img.float() # uint8 to fp16/32
+
+ def postprocess(self, preds, img, orig_imgs):
+ """Postprocesses predictions to return Results objects."""
+ results = []
+ for i, pred in enumerate(preds):
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ results.append(Results(orig_img=orig_img, path=img_path, names=self.model.names, probs=pred))
+
+ return results
+
+
+def predict(cfg=DEFAULT_CFG, use_python=False):
+ """Run YOLO model predictions on input images/videos."""
+ model = cfg.model or 'yolov8n-cls.pt' # or "resnet18"
+ source = cfg.source if cfg.source is not None else ROOT / 'assets' if (ROOT / 'assets').exists() \
+ else 'https://ultralytics.com/images/bus.jpg'
+
+ args = dict(model=model, source=source)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model)(**args)
+ else:
+ predictor = ClassificationPredictor(overrides=args)
+ predictor.predict_cli()
+
+
+if __name__ == '__main__':
+ predict()
diff --git a/yolov8/ultralytics/yolo/v8/classify/train.py b/yolov8/ultralytics/yolo/v8/classify/train.py
new file mode 100644
index 0000000000000000000000000000000000000000..72feb55913d2eabc097ab78f628533eb315857f3
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/classify/train.py
@@ -0,0 +1,161 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+import torchvision
+
+from ultralytics.nn.tasks import ClassificationModel, attempt_load_one_weight
+from ultralytics.yolo import v8
+from ultralytics.yolo.data import ClassificationDataset, build_dataloader
+from ultralytics.yolo.engine.trainer import BaseTrainer
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, RANK, colorstr
+from ultralytics.yolo.utils.plotting import plot_images, plot_results
+from ultralytics.yolo.utils.torch_utils import is_parallel, strip_optimizer, torch_distributed_zero_first
+
+
+class ClassificationTrainer(BaseTrainer):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ """Initialize a ClassificationTrainer object with optional configuration overrides and callbacks."""
+ if overrides is None:
+ overrides = {}
+ overrides['task'] = 'classify'
+ if overrides.get('imgsz') is None:
+ overrides['imgsz'] = 224
+ super().__init__(cfg, overrides, _callbacks)
+
+ def set_model_attributes(self):
+ """Set the YOLO model's class names from the loaded dataset."""
+ self.model.names = self.data['names']
+
+ def get_model(self, cfg=None, weights=None, verbose=True):
+ """Returns a modified PyTorch model configured for training YOLO."""
+ model = ClassificationModel(cfg, nc=self.data['nc'], verbose=verbose and RANK == -1)
+ if weights:
+ model.load(weights)
+
+ for m in model.modules():
+ if not self.args.pretrained and hasattr(m, 'reset_parameters'):
+ m.reset_parameters()
+ if isinstance(m, torch.nn.Dropout) and self.args.dropout:
+ m.p = self.args.dropout # set dropout
+ for p in model.parameters():
+ p.requires_grad = True # for training
+ return model
+
+ def setup_model(self):
+ """
+ load/create/download model for any task
+ """
+ # Classification models require special handling
+
+ if isinstance(self.model, torch.nn.Module): # if model is loaded beforehand. No setup needed
+ return
+
+ model = str(self.model)
+ # Load a YOLO model locally, from torchvision, or from Ultralytics assets
+ if model.endswith('.pt'):
+ self.model, _ = attempt_load_one_weight(model, device='cpu')
+ for p in self.model.parameters():
+ p.requires_grad = True # for training
+ elif model.endswith('.yaml'):
+ self.model = self.get_model(cfg=model)
+ elif model in torchvision.models.__dict__:
+ self.model = torchvision.models.__dict__[model](weights='IMAGENET1K_V1' if self.args.pretrained else None)
+ else:
+ FileNotFoundError(f'ERROR: model={model} not found locally or online. Please check model name.')
+ ClassificationModel.reshape_outputs(self.model, self.data['nc'])
+
+ return # dont return ckpt. Classification doesn't support resume
+
+ def build_dataset(self, img_path, mode='train', batch=None):
+ return ClassificationDataset(root=img_path, args=self.args, augment=mode == 'train')
+
+ def get_dataloader(self, dataset_path, batch_size=16, rank=0, mode='train'):
+ """Returns PyTorch DataLoader with transforms to preprocess images for inference."""
+ with torch_distributed_zero_first(rank): # init dataset *.cache only once if DDP
+ dataset = self.build_dataset(dataset_path, mode)
+
+ loader = build_dataloader(dataset, batch_size, self.args.workers, rank=rank)
+ # Attach inference transforms
+ if mode != 'train':
+ if is_parallel(self.model):
+ self.model.module.transforms = loader.dataset.torch_transforms
+ else:
+ self.model.transforms = loader.dataset.torch_transforms
+ return loader
+
+ def preprocess_batch(self, batch):
+ """Preprocesses a batch of images and classes."""
+ batch['img'] = batch['img'].to(self.device)
+ batch['cls'] = batch['cls'].to(self.device)
+ return batch
+
+ def progress_string(self):
+ """Returns a formatted string showing training progress."""
+ return ('\n' + '%11s' * (4 + len(self.loss_names))) % \
+ ('Epoch', 'GPU_mem', *self.loss_names, 'Instances', 'Size')
+
+ def get_validator(self):
+ """Returns an instance of ClassificationValidator for validation."""
+ self.loss_names = ['loss']
+ return v8.classify.ClassificationValidator(self.test_loader, self.save_dir)
+
+ def label_loss_items(self, loss_items=None, prefix='train'):
+ """
+ Returns a loss dict with labelled training loss items tensor
+ """
+ # Not needed for classification but necessary for segmentation & detection
+ keys = [f'{prefix}/{x}' for x in self.loss_names]
+ if loss_items is None:
+ return keys
+ loss_items = [round(float(loss_items), 5)]
+ return dict(zip(keys, loss_items))
+
+ def resume_training(self, ckpt):
+ """Resumes training from a given checkpoint."""
+ pass
+
+ def plot_metrics(self):
+ """Plots metrics from a CSV file."""
+ plot_results(file=self.csv, classify=True, on_plot=self.on_plot) # save results.png
+
+ def final_eval(self):
+ """Evaluate trained model and save validation results."""
+ for f in self.last, self.best:
+ if f.exists():
+ strip_optimizer(f) # strip optimizers
+ # TODO: validate best.pt after training completes
+ # if f is self.best:
+ # LOGGER.info(f'\nValidating {f}...')
+ # self.validator.args.save_json = True
+ # self.metrics = self.validator(model=f)
+ # self.metrics.pop('fitness', None)
+ # self.run_callbacks('on_fit_epoch_end')
+ LOGGER.info(f"Results saved to {colorstr('bold', self.save_dir)}")
+
+ def plot_training_samples(self, batch, ni):
+ """Plots training samples with their annotations."""
+ plot_images(images=batch['img'],
+ batch_idx=torch.arange(len(batch['img'])),
+ cls=batch['cls'].squeeze(-1),
+ fname=self.save_dir / f'train_batch{ni}.jpg',
+ on_plot=self.on_plot)
+
+
+def train(cfg=DEFAULT_CFG, use_python=False):
+ """Train the YOLO classification model."""
+ model = cfg.model or 'yolov8n-cls.pt' # or "resnet18"
+ data = cfg.data or 'mnist160' # or yolo.ClassificationDataset("mnist")
+ device = cfg.device if cfg.device is not None else ''
+
+ args = dict(model=model, data=data, device=device)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).train(**args)
+ else:
+ trainer = ClassificationTrainer(overrides=args)
+ trainer.train()
+
+
+if __name__ == '__main__':
+ train()
diff --git a/yolov8/ultralytics/yolo/v8/classify/val.py b/yolov8/ultralytics/yolo/v8/classify/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..f56dea0a2d7af0d88d3185a05aed566c0a8ba8a3
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/classify/val.py
@@ -0,0 +1,109 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.data import ClassificationDataset, build_dataloader
+from ultralytics.yolo.engine.validator import BaseValidator
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER
+from ultralytics.yolo.utils.metrics import ClassifyMetrics, ConfusionMatrix
+from ultralytics.yolo.utils.plotting import plot_images
+
+
+class ClassificationValidator(BaseValidator):
+
+ def __init__(self, dataloader=None, save_dir=None, pbar=None, args=None, _callbacks=None):
+ """Initializes ClassificationValidator instance with args, dataloader, save_dir, and progress bar."""
+ super().__init__(dataloader, save_dir, pbar, args, _callbacks)
+ self.args.task = 'classify'
+ self.metrics = ClassifyMetrics()
+
+ def get_desc(self):
+ """Returns a formatted string summarizing classification metrics."""
+ return ('%22s' + '%11s' * 2) % ('classes', 'top1_acc', 'top5_acc')
+
+ def init_metrics(self, model):
+ """Initialize confusion matrix, class names, and top-1 and top-5 accuracy."""
+ self.names = model.names
+ self.nc = len(model.names)
+ self.confusion_matrix = ConfusionMatrix(nc=self.nc, task='classify')
+ self.pred = []
+ self.targets = []
+
+ def preprocess(self, batch):
+ """Preprocesses input batch and returns it."""
+ batch['img'] = batch['img'].to(self.device, non_blocking=True)
+ batch['img'] = batch['img'].half() if self.args.half else batch['img'].float()
+ batch['cls'] = batch['cls'].to(self.device)
+ return batch
+
+ def update_metrics(self, preds, batch):
+ """Updates running metrics with model predictions and batch targets."""
+ n5 = min(len(self.model.names), 5)
+ self.pred.append(preds.argsort(1, descending=True)[:, :n5])
+ self.targets.append(batch['cls'])
+
+ def finalize_metrics(self, *args, **kwargs):
+ """Finalizes metrics of the model such as confusion_matrix and speed."""
+ self.confusion_matrix.process_cls_preds(self.pred, self.targets)
+ if self.args.plots:
+ for normalize in True, False:
+ self.confusion_matrix.plot(save_dir=self.save_dir,
+ names=self.names.values(),
+ normalize=normalize,
+ on_plot=self.on_plot)
+ self.metrics.speed = self.speed
+ self.metrics.confusion_matrix = self.confusion_matrix
+
+ def get_stats(self):
+ """Returns a dictionary of metrics obtained by processing targets and predictions."""
+ self.metrics.process(self.targets, self.pred)
+ return self.metrics.results_dict
+
+ def build_dataset(self, img_path):
+ return ClassificationDataset(root=img_path, args=self.args, augment=False)
+
+ def get_dataloader(self, dataset_path, batch_size):
+ """Builds and returns a data loader for classification tasks with given parameters."""
+ dataset = self.build_dataset(dataset_path)
+ return build_dataloader(dataset, batch_size, self.args.workers, rank=-1)
+
+ def print_results(self):
+ """Prints evaluation metrics for YOLO object detection model."""
+ pf = '%22s' + '%11.3g' * len(self.metrics.keys) # print format
+ LOGGER.info(pf % ('all', self.metrics.top1, self.metrics.top5))
+
+ def plot_val_samples(self, batch, ni):
+ """Plot validation image samples."""
+ plot_images(images=batch['img'],
+ batch_idx=torch.arange(len(batch['img'])),
+ cls=batch['cls'].squeeze(-1),
+ fname=self.save_dir / f'val_batch{ni}_labels.jpg',
+ names=self.names,
+ on_plot=self.on_plot)
+
+ def plot_predictions(self, batch, preds, ni):
+ """Plots predicted bounding boxes on input images and saves the result."""
+ plot_images(batch['img'],
+ batch_idx=torch.arange(len(batch['img'])),
+ cls=torch.argmax(preds, dim=1),
+ fname=self.save_dir / f'val_batch{ni}_pred.jpg',
+ names=self.names,
+ on_plot=self.on_plot) # pred
+
+
+def val(cfg=DEFAULT_CFG, use_python=False):
+ """Validate YOLO model using custom data."""
+ model = cfg.model or 'yolov8n-cls.pt' # or "resnet18"
+ data = cfg.data or 'mnist160'
+
+ args = dict(model=model, data=data)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).val(**args)
+ else:
+ validator = ClassificationValidator(args=args)
+ validator(model=args['model'])
+
+
+if __name__ == '__main__':
+ val()
diff --git a/yolov8/ultralytics/yolo/v8/detect/__init__.py b/yolov8/ultralytics/yolo/v8/detect/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..481951a9c79e54bb1720b3d174717973fc999059
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/detect/__init__.py
@@ -0,0 +1,7 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .predict import DetectionPredictor, predict
+from .train import DetectionTrainer, train
+from .val import DetectionValidator, val
+
+__all__ = 'DetectionPredictor', 'predict', 'DetectionTrainer', 'train', 'DetectionValidator', 'val'
diff --git a/yolov8/ultralytics/yolo/v8/detect/predict.py b/yolov8/ultralytics/yolo/v8/detect/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..31e8a9f28aa93f23e17eb70be4738a465b8fcee0
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/detect/predict.py
@@ -0,0 +1,48 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.engine.predictor import BasePredictor
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils import DEFAULT_CFG, ROOT, ops
+
+
+class DetectionPredictor(BasePredictor):
+
+ def postprocess(self, preds, img, orig_imgs):
+ """Postprocesses predictions and returns a list of Results objects."""
+ preds = ops.non_max_suppression(preds,
+ self.args.conf,
+ self.args.iou,
+ agnostic=self.args.agnostic_nms,
+ max_det=self.args.max_det,
+ classes=self.args.classes)
+
+ results = []
+ for i, pred in enumerate(preds):
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], orig_img.shape)
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ results.append(Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred))
+ return results
+
+
+def predict(cfg=DEFAULT_CFG, use_python=False):
+ """Runs YOLO model inference on input image(s)."""
+ model = cfg.model or 'yolov8n.pt'
+ source = cfg.source if cfg.source is not None else ROOT / 'assets' if (ROOT / 'assets').exists() \
+ else 'https://ultralytics.com/images/bus.jpg'
+
+ args = dict(model=model, source=source)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model)(**args)
+ else:
+ predictor = DetectionPredictor(overrides=args)
+ predictor.predict_cli()
+
+
+if __name__ == '__main__':
+ predict()
diff --git a/yolov8/ultralytics/yolo/v8/detect/train.py b/yolov8/ultralytics/yolo/v8/detect/train.py
new file mode 100644
index 0000000000000000000000000000000000000000..1b475ed0d600c38d530c5ab4055fbb760130794d
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/detect/train.py
@@ -0,0 +1,143 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+from copy import copy
+
+import numpy as np
+
+from ultralytics.nn.tasks import DetectionModel
+from ultralytics.yolo import v8
+from ultralytics.yolo.data import build_dataloader, build_yolo_dataset
+from ultralytics.yolo.data.dataloaders.v5loader import create_dataloader
+from ultralytics.yolo.engine.trainer import BaseTrainer
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, RANK, colorstr
+from ultralytics.yolo.utils.plotting import plot_images, plot_labels, plot_results
+from ultralytics.yolo.utils.torch_utils import de_parallel, torch_distributed_zero_first
+
+
+# BaseTrainer python usage
+class DetectionTrainer(BaseTrainer):
+
+ def build_dataset(self, img_path, mode='train', batch=None):
+ """Build YOLO Dataset
+
+ Args:
+ img_path (str): Path to the folder containing images.
+ mode (str): `train` mode or `val` mode, users are able to customize different augmentations for each mode.
+ batch (int, optional): Size of batches, this is for `rect`. Defaults to None.
+ """
+ gs = max(int(de_parallel(self.model).stride.max() if self.model else 0), 32)
+ return build_yolo_dataset(self.args, img_path, batch, self.data, mode=mode, rect=mode == 'val', stride=gs)
+
+ def get_dataloader(self, dataset_path, batch_size=16, rank=0, mode='train'):
+ """TODO: manage splits differently."""
+ # Calculate stride - check if model is initialized
+ if self.args.v5loader:
+ LOGGER.warning("WARNING ⚠️ 'v5loader' feature is deprecated and will be removed soon. You can train using "
+ 'the default YOLOv8 dataloader instead, no argument is needed.')
+ gs = max(int(de_parallel(self.model).stride.max() if self.model else 0), 32)
+ return create_dataloader(path=dataset_path,
+ imgsz=self.args.imgsz,
+ batch_size=batch_size,
+ stride=gs,
+ hyp=vars(self.args),
+ augment=mode == 'train',
+ cache=self.args.cache,
+ pad=0 if mode == 'train' else 0.5,
+ rect=self.args.rect or mode == 'val',
+ rank=rank,
+ workers=self.args.workers,
+ close_mosaic=self.args.close_mosaic != 0,
+ prefix=colorstr(f'{mode}: '),
+ shuffle=mode == 'train',
+ seed=self.args.seed)[0]
+ assert mode in ['train', 'val']
+ with torch_distributed_zero_first(rank): # init dataset *.cache only once if DDP
+ dataset = self.build_dataset(dataset_path, mode, batch_size)
+ shuffle = mode == 'train'
+ if getattr(dataset, 'rect', False) and shuffle:
+ LOGGER.warning("WARNING ⚠️ 'rect=True' is incompatible with DataLoader shuffle, setting shuffle=False")
+ shuffle = False
+ workers = self.args.workers if mode == 'train' else self.args.workers * 2
+ return build_dataloader(dataset, batch_size, workers, shuffle, rank) # return dataloader
+
+ def preprocess_batch(self, batch):
+ """Preprocesses a batch of images by scaling and converting to float."""
+ batch['img'] = batch['img'].to(self.device, non_blocking=True).float() / 255
+ return batch
+
+ def set_model_attributes(self):
+ """nl = de_parallel(self.model).model[-1].nl # number of detection layers (to scale hyps)."""
+ # self.args.box *= 3 / nl # scale to layers
+ # self.args.cls *= self.data["nc"] / 80 * 3 / nl # scale to classes and layers
+ # self.args.cls *= (self.args.imgsz / 640) ** 2 * 3 / nl # scale to image size and layers
+ self.model.nc = self.data['nc'] # attach number of classes to model
+ self.model.names = self.data['names'] # attach class names to model
+ self.model.args = self.args # attach hyperparameters to model
+ # TODO: self.model.class_weights = labels_to_class_weights(dataset.labels, nc).to(device) * nc
+
+ def get_model(self, cfg=None, weights=None, verbose=True):
+ """Return a YOLO detection model."""
+ model = DetectionModel(cfg, nc=self.data['nc'], verbose=verbose and RANK == -1)
+ if weights:
+ model.load(weights)
+ return model
+
+ def get_validator(self):
+ """Returns a DetectionValidator for YOLO model validation."""
+ self.loss_names = 'box_loss', 'cls_loss', 'dfl_loss'
+ return v8.detect.DetectionValidator(self.test_loader, save_dir=self.save_dir, args=copy(self.args))
+
+ def label_loss_items(self, loss_items=None, prefix='train'):
+ """
+ Returns a loss dict with labelled training loss items tensor
+ """
+ # Not needed for classification but necessary for segmentation & detection
+ keys = [f'{prefix}/{x}' for x in self.loss_names]
+ if loss_items is not None:
+ loss_items = [round(float(x), 5) for x in loss_items] # convert tensors to 5 decimal place floats
+ return dict(zip(keys, loss_items))
+ else:
+ return keys
+
+ def progress_string(self):
+ """Returns a formatted string of training progress with epoch, GPU memory, loss, instances and size."""
+ return ('\n' + '%11s' *
+ (4 + len(self.loss_names))) % ('Epoch', 'GPU_mem', *self.loss_names, 'Instances', 'Size')
+
+ def plot_training_samples(self, batch, ni):
+ """Plots training samples with their annotations."""
+ plot_images(images=batch['img'],
+ batch_idx=batch['batch_idx'],
+ cls=batch['cls'].squeeze(-1),
+ bboxes=batch['bboxes'],
+ paths=batch['im_file'],
+ fname=self.save_dir / f'train_batch{ni}.jpg',
+ on_plot=self.on_plot)
+
+ def plot_metrics(self):
+ """Plots metrics from a CSV file."""
+ plot_results(file=self.csv, on_plot=self.on_plot) # save results.png
+
+ def plot_training_labels(self):
+ """Create a labeled training plot of the YOLO model."""
+ boxes = np.concatenate([lb['bboxes'] for lb in self.train_loader.dataset.labels], 0)
+ cls = np.concatenate([lb['cls'] for lb in self.train_loader.dataset.labels], 0)
+ plot_labels(boxes, cls.squeeze(), names=self.data['names'], save_dir=self.save_dir, on_plot=self.on_plot)
+
+
+def train(cfg=DEFAULT_CFG, use_python=False):
+ """Train and optimize YOLO model given training data and device."""
+ model = cfg.model or 'yolov8n.pt'
+ data = cfg.data or 'coco128.yaml' # or yolo.ClassificationDataset("mnist")
+ device = cfg.device if cfg.device is not None else ''
+
+ args = dict(model=model, data=data, device=device)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).train(**args)
+ else:
+ trainer = DetectionTrainer(overrides=args)
+ trainer.train()
+
+
+if __name__ == '__main__':
+ train()
diff --git a/yolov8/ultralytics/yolo/v8/detect/val.py b/yolov8/ultralytics/yolo/v8/detect/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..77d346ca4b98c13ea31dc3a6499bce64e28dda21
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/detect/val.py
@@ -0,0 +1,296 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import os
+from pathlib import Path
+
+import numpy as np
+import torch
+
+from ultralytics.yolo.data import build_dataloader, build_yolo_dataset
+from ultralytics.yolo.data.dataloaders.v5loader import create_dataloader
+from ultralytics.yolo.engine.validator import BaseValidator
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, colorstr, ops
+from ultralytics.yolo.utils.checks import check_requirements
+from ultralytics.yolo.utils.metrics import ConfusionMatrix, DetMetrics, box_iou
+from ultralytics.yolo.utils.plotting import output_to_target, plot_images
+from ultralytics.yolo.utils.torch_utils import de_parallel
+
+
+class DetectionValidator(BaseValidator):
+
+ def __init__(self, dataloader=None, save_dir=None, pbar=None, args=None, _callbacks=None):
+ """Initialize detection model with necessary variables and settings."""
+ super().__init__(dataloader, save_dir, pbar, args, _callbacks)
+ self.args.task = 'detect'
+ self.is_coco = False
+ self.class_map = None
+ self.metrics = DetMetrics(save_dir=self.save_dir, on_plot=self.on_plot)
+ self.iouv = torch.linspace(0.5, 0.95, 10) # iou vector for mAP@0.5:0.95
+ self.niou = self.iouv.numel()
+
+ def preprocess(self, batch):
+ """Preprocesses batch of images for YOLO training."""
+ batch['img'] = batch['img'].to(self.device, non_blocking=True)
+ batch['img'] = (batch['img'].half() if self.args.half else batch['img'].float()) / 255
+ for k in ['batch_idx', 'cls', 'bboxes']:
+ batch[k] = batch[k].to(self.device)
+
+ nb = len(batch['img'])
+ self.lb = [torch.cat([batch['cls'], batch['bboxes']], dim=-1)[batch['batch_idx'] == i]
+ for i in range(nb)] if self.args.save_hybrid else [] # for autolabelling
+
+ return batch
+
+ def init_metrics(self, model):
+ """Initialize evaluation metrics for YOLO."""
+ val = self.data.get(self.args.split, '') # validation path
+ self.is_coco = isinstance(val, str) and 'coco' in val and val.endswith(f'{os.sep}val2017.txt') # is COCO
+ self.class_map = ops.coco80_to_coco91_class() if self.is_coco else list(range(1000))
+ self.args.save_json |= self.is_coco and not self.training # run on final val if training COCO
+ self.names = model.names
+ self.nc = len(model.names)
+ self.metrics.names = self.names
+ self.metrics.plot = self.args.plots
+ self.confusion_matrix = ConfusionMatrix(nc=self.nc)
+ self.seen = 0
+ self.jdict = []
+ self.stats = []
+
+ def get_desc(self):
+ """Return a formatted string summarizing class metrics of YOLO model."""
+ return ('%22s' + '%11s' * 6) % ('Class', 'Images', 'Instances', 'Box(P', 'R', 'mAP50', 'mAP50-95)')
+
+ def postprocess(self, preds):
+ """Apply Non-maximum suppression to prediction outputs."""
+ return ops.non_max_suppression(preds,
+ self.args.conf,
+ self.args.iou,
+ labels=self.lb,
+ multi_label=True,
+ agnostic=self.args.single_cls,
+ max_det=self.args.max_det)
+
+ def update_metrics(self, preds, batch):
+ """Metrics."""
+ for si, pred in enumerate(preds):
+ idx = batch['batch_idx'] == si
+ cls = batch['cls'][idx]
+ bbox = batch['bboxes'][idx]
+ nl, npr = cls.shape[0], pred.shape[0] # number of labels, predictions
+ shape = batch['ori_shape'][si]
+ correct_bboxes = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ self.seen += 1
+
+ if npr == 0:
+ if nl:
+ self.stats.append((correct_bboxes, *torch.zeros((2, 0), device=self.device), cls.squeeze(-1)))
+ if self.args.plots:
+ self.confusion_matrix.process_batch(detections=None, labels=cls.squeeze(-1))
+ continue
+
+ # Predictions
+ if self.args.single_cls:
+ pred[:, 5] = 0
+ predn = pred.clone()
+ ops.scale_boxes(batch['img'][si].shape[1:], predn[:, :4], shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space pred
+
+ # Evaluate
+ if nl:
+ height, width = batch['img'].shape[2:]
+ tbox = ops.xywh2xyxy(bbox) * torch.tensor(
+ (width, height, width, height), device=self.device) # target boxes
+ ops.scale_boxes(batch['img'][si].shape[1:], tbox, shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space labels
+ labelsn = torch.cat((cls, tbox), 1) # native-space labels
+ correct_bboxes = self._process_batch(predn, labelsn)
+ # TODO: maybe remove these `self.` arguments as they already are member variable
+ if self.args.plots:
+ self.confusion_matrix.process_batch(predn, labelsn)
+ self.stats.append((correct_bboxes, pred[:, 4], pred[:, 5], cls.squeeze(-1))) # (conf, pcls, tcls)
+
+ # Save
+ if self.args.save_json:
+ self.pred_to_json(predn, batch['im_file'][si])
+ if self.args.save_txt:
+ file = self.save_dir / 'labels' / f'{Path(batch["im_file"][si]).stem}.txt'
+ self.save_one_txt(predn, self.args.save_conf, shape, file)
+
+ def finalize_metrics(self, *args, **kwargs):
+ """Set final values for metrics speed and confusion matrix."""
+ self.metrics.speed = self.speed
+ self.metrics.confusion_matrix = self.confusion_matrix
+
+ def get_stats(self):
+ """Returns metrics statistics and results dictionary."""
+ stats = [torch.cat(x, 0).cpu().numpy() for x in zip(*self.stats)] # to numpy
+ if len(stats) and stats[0].any():
+ self.metrics.process(*stats)
+ self.nt_per_class = np.bincount(stats[-1].astype(int), minlength=self.nc) # number of targets per class
+ return self.metrics.results_dict
+
+ def print_results(self):
+ """Prints training/validation set metrics per class."""
+ pf = '%22s' + '%11i' * 2 + '%11.3g' * len(self.metrics.keys) # print format
+ LOGGER.info(pf % ('all', self.seen, self.nt_per_class.sum(), *self.metrics.mean_results()))
+ if self.nt_per_class.sum() == 0:
+ LOGGER.warning(
+ f'WARNING ⚠️ no labels found in {self.args.task} set, can not compute metrics without labels')
+
+ # Print results per class
+ if self.args.verbose and not self.training and self.nc > 1 and len(self.stats):
+ for i, c in enumerate(self.metrics.ap_class_index):
+ LOGGER.info(pf % (self.names[c], self.seen, self.nt_per_class[c], *self.metrics.class_result(i)))
+
+ if self.args.plots:
+ for normalize in True, False:
+ self.confusion_matrix.plot(save_dir=self.save_dir,
+ names=self.names.values(),
+ normalize=normalize,
+ on_plot=self.on_plot)
+
+ def _process_batch(self, detections, labels):
+ """
+ Return correct prediction matrix
+ Arguments:
+ detections (array[N, 6]), x1, y1, x2, y2, conf, class
+ labels (array[M, 5]), class, x1, y1, x2, y2
+ Returns:
+ correct (array[N, 10]), for 10 IoU levels
+ """
+ iou = box_iou(labels[:, 1:], detections[:, :4])
+ correct = np.zeros((detections.shape[0], self.iouv.shape[0])).astype(bool)
+ correct_class = labels[:, 0:1] == detections[:, 5]
+ for i in range(len(self.iouv)):
+ x = torch.where((iou >= self.iouv[i]) & correct_class) # IoU > threshold and classes match
+ if x[0].shape[0]:
+ matches = torch.cat((torch.stack(x, 1), iou[x[0], x[1]][:, None]),
+ 1).cpu().numpy() # [label, detect, iou]
+ if x[0].shape[0] > 1:
+ matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 1], return_index=True)[1]]
+ # matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 0], return_index=True)[1]]
+ correct[matches[:, 1].astype(int), i] = True
+ return torch.tensor(correct, dtype=torch.bool, device=detections.device)
+
+ def build_dataset(self, img_path, mode='val', batch=None):
+ """Build YOLO Dataset
+
+ Args:
+ img_path (str): Path to the folder containing images.
+ mode (str): `train` mode or `val` mode, users are able to customize different augmentations for each mode.
+ batch (int, optional): Size of batches, this is for `rect`. Defaults to None.
+ """
+ gs = max(int(de_parallel(self.model).stride if self.model else 0), 32)
+ return build_yolo_dataset(self.args, img_path, batch, self.data, mode=mode, stride=gs)
+
+ def get_dataloader(self, dataset_path, batch_size):
+ """TODO: manage splits differently."""
+ # Calculate stride - check if model is initialized
+ if self.args.v5loader:
+ LOGGER.warning("WARNING ⚠️ 'v5loader' feature is deprecated and will be removed soon. You can train using "
+ 'the default YOLOv8 dataloader instead, no argument is needed.')
+ gs = max(int(de_parallel(self.model).stride if self.model else 0), 32)
+ return create_dataloader(path=dataset_path,
+ imgsz=self.args.imgsz,
+ batch_size=batch_size,
+ stride=gs,
+ hyp=vars(self.args),
+ cache=False,
+ pad=0.5,
+ rect=self.args.rect,
+ workers=self.args.workers,
+ prefix=colorstr(f'{self.args.mode}: '),
+ shuffle=False,
+ seed=self.args.seed)[0]
+
+ dataset = self.build_dataset(dataset_path, batch=batch_size, mode='val')
+ dataloader = build_dataloader(dataset, batch_size, self.args.workers, shuffle=False, rank=-1)
+ return dataloader
+
+ def plot_val_samples(self, batch, ni):
+ """Plot validation image samples."""
+ plot_images(batch['img'],
+ batch['batch_idx'],
+ batch['cls'].squeeze(-1),
+ batch['bboxes'],
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_labels.jpg',
+ names=self.names,
+ on_plot=self.on_plot)
+
+ def plot_predictions(self, batch, preds, ni):
+ """Plots predicted bounding boxes on input images and saves the result."""
+ plot_images(batch['img'],
+ *output_to_target(preds, max_det=self.args.max_det),
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_pred.jpg',
+ names=self.names,
+ on_plot=self.on_plot) # pred
+
+ def save_one_txt(self, predn, save_conf, shape, file):
+ """Save YOLO detections to a txt file in normalized coordinates in a specific format."""
+ gn = torch.tensor(shape)[[1, 0, 1, 0]] # normalization gain whwh
+ for *xyxy, conf, cls in predn.tolist():
+ xywh = (ops.xyxy2xywh(torch.tensor(xyxy).view(1, 4)) / gn).view(-1).tolist() # normalized xywh
+ line = (cls, *xywh, conf) if save_conf else (cls, *xywh) # label format
+ with open(file, 'a') as f:
+ f.write(('%g ' * len(line)).rstrip() % line + '\n')
+
+ def pred_to_json(self, predn, filename):
+ """Serialize YOLO predictions to COCO json format."""
+ stem = Path(filename).stem
+ image_id = int(stem) if stem.isnumeric() else stem
+ box = ops.xyxy2xywh(predn[:, :4]) # xywh
+ box[:, :2] -= box[:, 2:] / 2 # xy center to top-left corner
+ for p, b in zip(predn.tolist(), box.tolist()):
+ self.jdict.append({
+ 'image_id': image_id,
+ 'category_id': self.class_map[int(p[5])],
+ 'bbox': [round(x, 3) for x in b],
+ 'score': round(p[4], 5)})
+
+ def eval_json(self, stats):
+ """Evaluates YOLO output in JSON format and returns performance statistics."""
+ if self.args.save_json and self.is_coco and len(self.jdict):
+ anno_json = self.data['path'] / 'annotations/instances_val2017.json' # annotations
+ pred_json = self.save_dir / 'predictions.json' # predictions
+ LOGGER.info(f'\nEvaluating pycocotools mAP using {pred_json} and {anno_json}...')
+ try: # https://github.com/cocodataset/cocoapi/blob/master/PythonAPI/pycocoEvalDemo.ipynb
+ check_requirements('pycocotools>=2.0.6')
+ from pycocotools.coco import COCO # noqa
+ from pycocotools.cocoeval import COCOeval # noqa
+
+ for x in anno_json, pred_json:
+ assert x.is_file(), f'{x} file not found'
+ anno = COCO(str(anno_json)) # init annotations api
+ pred = anno.loadRes(str(pred_json)) # init predictions api (must pass string, not Path)
+ eval = COCOeval(anno, pred, 'bbox')
+ if self.is_coco:
+ eval.params.imgIds = [int(Path(x).stem) for x in self.dataloader.dataset.im_files] # images to eval
+ eval.evaluate()
+ eval.accumulate()
+ eval.summarize()
+ stats[self.metrics.keys[-1]], stats[self.metrics.keys[-2]] = eval.stats[:2] # update mAP50-95 and mAP50
+ except Exception as e:
+ LOGGER.warning(f'pycocotools unable to run: {e}')
+ return stats
+
+
+def val(cfg=DEFAULT_CFG, use_python=False):
+ """Validate trained YOLO model on validation dataset."""
+ model = cfg.model or 'yolov8n.pt'
+ data = cfg.data or 'coco128.yaml'
+
+ args = dict(model=model, data=data)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).val(**args)
+ else:
+ validator = DetectionValidator(args=args)
+ validator(model=args['model'])
+
+
+if __name__ == '__main__':
+ val()
diff --git a/yolov8/ultralytics/yolo/v8/pose/__init__.py b/yolov8/ultralytics/yolo/v8/pose/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..8ec6d58771ab6dd02633eba23a0c7032e7cc02d9
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/pose/__init__.py
@@ -0,0 +1,7 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .predict import PosePredictor, predict
+from .train import PoseTrainer, train
+from .val import PoseValidator, val
+
+__all__ = 'PoseTrainer', 'train', 'PoseValidator', 'val', 'PosePredictor', 'predict'
diff --git a/yolov8/ultralytics/yolo/v8/pose/predict.py b/yolov8/ultralytics/yolo/v8/pose/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..ad3246e11a55a0696b54af2b91bd2beb30352352
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/pose/predict.py
@@ -0,0 +1,58 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils import DEFAULT_CFG, ROOT, ops
+from ultralytics.yolo.v8.detect.predict import DetectionPredictor
+
+
+class PosePredictor(DetectionPredictor):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ super().__init__(cfg, overrides, _callbacks)
+ self.args.task = 'pose'
+
+ def postprocess(self, preds, img, orig_imgs):
+ """Return detection results for a given input image or list of images."""
+ preds = ops.non_max_suppression(preds,
+ self.args.conf,
+ self.args.iou,
+ agnostic=self.args.agnostic_nms,
+ max_det=self.args.max_det,
+ classes=self.args.classes,
+ nc=len(self.model.names))
+
+ results = []
+ for i, pred in enumerate(preds):
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ shape = orig_img.shape
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], shape).round()
+ pred_kpts = pred[:, 6:].view(len(pred), *self.model.kpt_shape) if len(pred) else pred[:, 6:]
+ pred_kpts = ops.scale_coords(img.shape[2:], pred_kpts, shape)
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ results.append(
+ Results(orig_img=orig_img,
+ path=img_path,
+ names=self.model.names,
+ boxes=pred[:, :6],
+ keypoints=pred_kpts))
+ return results
+
+
+def predict(cfg=DEFAULT_CFG, use_python=False):
+ """Runs YOLO to predict objects in an image or video."""
+ model = cfg.model or 'yolov8n-pose.pt'
+ source = cfg.source if cfg.source is not None else ROOT / 'assets' if (ROOT / 'assets').exists() \
+ else 'https://ultralytics.com/images/bus.jpg'
+
+ args = dict(model=model, source=source)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model)(**args)
+ else:
+ predictor = PosePredictor(overrides=args)
+ predictor.predict_cli()
+
+
+if __name__ == '__main__':
+ predict()
diff --git a/yolov8/ultralytics/yolo/v8/pose/train.py b/yolov8/ultralytics/yolo/v8/pose/train.py
new file mode 100644
index 0000000000000000000000000000000000000000..af3043c1942ffe88ae8eedcb45ef7bf5bb70a45a
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/pose/train.py
@@ -0,0 +1,77 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from copy import copy
+
+from ultralytics.nn.tasks import PoseModel
+from ultralytics.yolo import v8
+from ultralytics.yolo.utils import DEFAULT_CFG
+from ultralytics.yolo.utils.plotting import plot_images, plot_results
+
+
+# BaseTrainer python usage
+class PoseTrainer(v8.detect.DetectionTrainer):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ """Initialize a PoseTrainer object with specified configurations and overrides."""
+ if overrides is None:
+ overrides = {}
+ overrides['task'] = 'pose'
+ super().__init__(cfg, overrides, _callbacks)
+
+ def get_model(self, cfg=None, weights=None, verbose=True):
+ """Get pose estimation model with specified configuration and weights."""
+ model = PoseModel(cfg, ch=3, nc=self.data['nc'], data_kpt_shape=self.data['kpt_shape'], verbose=verbose)
+ if weights:
+ model.load(weights)
+
+ return model
+
+ def set_model_attributes(self):
+ """Sets keypoints shape attribute of PoseModel."""
+ super().set_model_attributes()
+ self.model.kpt_shape = self.data['kpt_shape']
+
+ def get_validator(self):
+ """Returns an instance of the PoseValidator class for validation."""
+ self.loss_names = 'box_loss', 'pose_loss', 'kobj_loss', 'cls_loss', 'dfl_loss'
+ return v8.pose.PoseValidator(self.test_loader, save_dir=self.save_dir, args=copy(self.args))
+
+ def plot_training_samples(self, batch, ni):
+ """Plot a batch of training samples with annotated class labels, bounding boxes, and keypoints."""
+ images = batch['img']
+ kpts = batch['keypoints']
+ cls = batch['cls'].squeeze(-1)
+ bboxes = batch['bboxes']
+ paths = batch['im_file']
+ batch_idx = batch['batch_idx']
+ plot_images(images,
+ batch_idx,
+ cls,
+ bboxes,
+ kpts=kpts,
+ paths=paths,
+ fname=self.save_dir / f'train_batch{ni}.jpg',
+ on_plot=self.on_plot)
+
+ def plot_metrics(self):
+ """Plots training/val metrics."""
+ plot_results(file=self.csv, pose=True, on_plot=self.on_plot) # save results.png
+
+
+def train(cfg=DEFAULT_CFG, use_python=False):
+ """Train the YOLO model on the given data and device."""
+ model = cfg.model or 'yolov8n-pose.yaml'
+ data = cfg.data or 'coco8-pose.yaml'
+ device = cfg.device if cfg.device is not None else ''
+
+ args = dict(model=model, data=data, device=device)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).train(**args)
+ else:
+ trainer = PoseTrainer(overrides=args)
+ trainer.train()
+
+
+if __name__ == '__main__':
+ train()
diff --git a/yolov8/ultralytics/yolo/v8/pose/val.py b/yolov8/ultralytics/yolo/v8/pose/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..f3fc1ac871e4becbe5950ab6556591f7a637af3c
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/pose/val.py
@@ -0,0 +1,224 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from pathlib import Path
+
+import numpy as np
+import torch
+
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, ops
+from ultralytics.yolo.utils.checks import check_requirements
+from ultralytics.yolo.utils.metrics import OKS_SIGMA, PoseMetrics, box_iou, kpt_iou
+from ultralytics.yolo.utils.plotting import output_to_target, plot_images
+from ultralytics.yolo.v8.detect import DetectionValidator
+
+
+class PoseValidator(DetectionValidator):
+
+ def __init__(self, dataloader=None, save_dir=None, pbar=None, args=None, _callbacks=None):
+ """Initialize a 'PoseValidator' object with custom parameters and assigned attributes."""
+ super().__init__(dataloader, save_dir, pbar, args, _callbacks)
+ self.args.task = 'pose'
+ self.metrics = PoseMetrics(save_dir=self.save_dir, on_plot=self.on_plot)
+
+ def preprocess(self, batch):
+ """Preprocesses the batch by converting the 'keypoints' data into a float and moving it to the device."""
+ batch = super().preprocess(batch)
+ batch['keypoints'] = batch['keypoints'].to(self.device).float()
+ return batch
+
+ def get_desc(self):
+ """Returns description of evaluation metrics in string format."""
+ return ('%22s' + '%11s' * 10) % ('Class', 'Images', 'Instances', 'Box(P', 'R', 'mAP50', 'mAP50-95)', 'Pose(P',
+ 'R', 'mAP50', 'mAP50-95)')
+
+ def postprocess(self, preds):
+ """Apply non-maximum suppression and return detections with high confidence scores."""
+ return ops.non_max_suppression(preds,
+ self.args.conf,
+ self.args.iou,
+ labels=self.lb,
+ multi_label=True,
+ agnostic=self.args.single_cls,
+ max_det=self.args.max_det,
+ nc=self.nc)
+
+ def init_metrics(self, model):
+ """Initiate pose estimation metrics for YOLO model."""
+ super().init_metrics(model)
+ self.kpt_shape = self.data['kpt_shape']
+ is_pose = self.kpt_shape == [17, 3]
+ nkpt = self.kpt_shape[0]
+ self.sigma = OKS_SIGMA if is_pose else np.ones(nkpt) / nkpt
+
+ def update_metrics(self, preds, batch):
+ """Metrics."""
+ for si, pred in enumerate(preds):
+ idx = batch['batch_idx'] == si
+ cls = batch['cls'][idx]
+ bbox = batch['bboxes'][idx]
+ kpts = batch['keypoints'][idx]
+ nl, npr = cls.shape[0], pred.shape[0] # number of labels, predictions
+ nk = kpts.shape[1] # number of keypoints
+ shape = batch['ori_shape'][si]
+ correct_kpts = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ correct_bboxes = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ self.seen += 1
+
+ if npr == 0:
+ if nl:
+ self.stats.append((correct_bboxes, correct_kpts, *torch.zeros(
+ (2, 0), device=self.device), cls.squeeze(-1)))
+ if self.args.plots:
+ self.confusion_matrix.process_batch(detections=None, labels=cls.squeeze(-1))
+ continue
+
+ # Predictions
+ if self.args.single_cls:
+ pred[:, 5] = 0
+ predn = pred.clone()
+ ops.scale_boxes(batch['img'][si].shape[1:], predn[:, :4], shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space pred
+ pred_kpts = predn[:, 6:].view(npr, nk, -1)
+ ops.scale_coords(batch['img'][si].shape[1:], pred_kpts, shape, ratio_pad=batch['ratio_pad'][si])
+
+ # Evaluate
+ if nl:
+ height, width = batch['img'].shape[2:]
+ tbox = ops.xywh2xyxy(bbox) * torch.tensor(
+ (width, height, width, height), device=self.device) # target boxes
+ ops.scale_boxes(batch['img'][si].shape[1:], tbox, shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space labels
+ tkpts = kpts.clone()
+ tkpts[..., 0] *= width
+ tkpts[..., 1] *= height
+ tkpts = ops.scale_coords(batch['img'][si].shape[1:], tkpts, shape, ratio_pad=batch['ratio_pad'][si])
+ labelsn = torch.cat((cls, tbox), 1) # native-space labels
+ correct_bboxes = self._process_batch(predn[:, :6], labelsn)
+ correct_kpts = self._process_batch(predn[:, :6], labelsn, pred_kpts, tkpts)
+ if self.args.plots:
+ self.confusion_matrix.process_batch(predn, labelsn)
+
+ # Append correct_masks, correct_boxes, pconf, pcls, tcls
+ self.stats.append((correct_bboxes, correct_kpts, pred[:, 4], pred[:, 5], cls.squeeze(-1)))
+
+ # Save
+ if self.args.save_json:
+ self.pred_to_json(predn, batch['im_file'][si])
+ # if self.args.save_txt:
+ # save_one_txt(predn, save_conf, shape, file=save_dir / 'labels' / f'{path.stem}.txt')
+
+ def _process_batch(self, detections, labels, pred_kpts=None, gt_kpts=None):
+ """
+ Return correct prediction matrix
+ Arguments:
+ detections (array[N, 6]), x1, y1, x2, y2, conf, class
+ labels (array[M, 5]), class, x1, y1, x2, y2
+ pred_kpts (array[N, 51]), 51 = 17 * 3
+ gt_kpts (array[N, 51])
+ Returns:
+ correct (array[N, 10]), for 10 IoU levels
+ """
+ if pred_kpts is not None and gt_kpts is not None:
+ # `0.53` is from https://github.com/jin-s13/xtcocoapi/blob/master/xtcocotools/cocoeval.py#L384
+ area = ops.xyxy2xywh(labels[:, 1:])[:, 2:].prod(1) * 0.53
+ iou = kpt_iou(gt_kpts, pred_kpts, sigma=self.sigma, area=area)
+ else: # boxes
+ iou = box_iou(labels[:, 1:], detections[:, :4])
+
+ correct = np.zeros((detections.shape[0], self.iouv.shape[0])).astype(bool)
+ correct_class = labels[:, 0:1] == detections[:, 5]
+ for i in range(len(self.iouv)):
+ x = torch.where((iou >= self.iouv[i]) & correct_class) # IoU > threshold and classes match
+ if x[0].shape[0]:
+ matches = torch.cat((torch.stack(x, 1), iou[x[0], x[1]][:, None]),
+ 1).cpu().numpy() # [label, detect, iou]
+ if x[0].shape[0] > 1:
+ matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 1], return_index=True)[1]]
+ # matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 0], return_index=True)[1]]
+ correct[matches[:, 1].astype(int), i] = True
+ return torch.tensor(correct, dtype=torch.bool, device=detections.device)
+
+ def plot_val_samples(self, batch, ni):
+ """Plots and saves validation set samples with predicted bounding boxes and keypoints."""
+ plot_images(batch['img'],
+ batch['batch_idx'],
+ batch['cls'].squeeze(-1),
+ batch['bboxes'],
+ kpts=batch['keypoints'],
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_labels.jpg',
+ names=self.names,
+ on_plot=self.on_plot)
+
+ def plot_predictions(self, batch, preds, ni):
+ """Plots predictions for YOLO model."""
+ pred_kpts = torch.cat([p[:, 6:].view(-1, *self.kpt_shape) for p in preds], 0)
+ plot_images(batch['img'],
+ *output_to_target(preds, max_det=self.args.max_det),
+ kpts=pred_kpts,
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_pred.jpg',
+ names=self.names,
+ on_plot=self.on_plot) # pred
+
+ def pred_to_json(self, predn, filename):
+ """Converts YOLO predictions to COCO JSON format."""
+ stem = Path(filename).stem
+ image_id = int(stem) if stem.isnumeric() else stem
+ box = ops.xyxy2xywh(predn[:, :4]) # xywh
+ box[:, :2] -= box[:, 2:] / 2 # xy center to top-left corner
+ for p, b in zip(predn.tolist(), box.tolist()):
+ self.jdict.append({
+ 'image_id': image_id,
+ 'category_id': self.class_map[int(p[5])],
+ 'bbox': [round(x, 3) for x in b],
+ 'keypoints': p[6:],
+ 'score': round(p[4], 5)})
+
+ def eval_json(self, stats):
+ """Evaluates object detection model using COCO JSON format."""
+ if self.args.save_json and self.is_coco and len(self.jdict):
+ anno_json = self.data['path'] / 'annotations/person_keypoints_val2017.json' # annotations
+ pred_json = self.save_dir / 'predictions.json' # predictions
+ LOGGER.info(f'\nEvaluating pycocotools mAP using {pred_json} and {anno_json}...')
+ try: # https://github.com/cocodataset/cocoapi/blob/master/PythonAPI/pycocoEvalDemo.ipynb
+ check_requirements('pycocotools>=2.0.6')
+ from pycocotools.coco import COCO # noqa
+ from pycocotools.cocoeval import COCOeval # noqa
+
+ for x in anno_json, pred_json:
+ assert x.is_file(), f'{x} file not found'
+ anno = COCO(str(anno_json)) # init annotations api
+ pred = anno.loadRes(str(pred_json)) # init predictions api (must pass string, not Path)
+ for i, eval in enumerate([COCOeval(anno, pred, 'bbox'), COCOeval(anno, pred, 'keypoints')]):
+ if self.is_coco:
+ eval.params.imgIds = [int(Path(x).stem) for x in self.dataloader.dataset.im_files] # im to eval
+ eval.evaluate()
+ eval.accumulate()
+ eval.summarize()
+ idx = i * 4 + 2
+ stats[self.metrics.keys[idx + 1]], stats[
+ self.metrics.keys[idx]] = eval.stats[:2] # update mAP50-95 and mAP50
+ except Exception as e:
+ LOGGER.warning(f'pycocotools unable to run: {e}')
+ return stats
+
+
+def val(cfg=DEFAULT_CFG, use_python=False):
+ """Performs validation on YOLO model using given data."""
+ model = cfg.model or 'yolov8n-pose.pt'
+ data = cfg.data or 'coco8-pose.yaml'
+
+ args = dict(model=model, data=data)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).val(**args)
+ else:
+ validator = PoseValidator(args=args)
+ validator(model=args['model'])
+
+
+if __name__ == '__main__':
+ val()
diff --git a/yolov8/ultralytics/yolo/v8/segment/__init__.py b/yolov8/ultralytics/yolo/v8/segment/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..61a9efe98d484814996b03f3386653c3b2e44bf6
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/segment/__init__.py
@@ -0,0 +1,7 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from .predict import SegmentationPredictor, predict
+from .train import SegmentationTrainer, train
+from .val import SegmentationValidator, val
+
+__all__ = 'SegmentationPredictor', 'predict', 'SegmentationTrainer', 'train', 'SegmentationValidator', 'val'
diff --git a/yolov8/ultralytics/yolo/v8/segment/predict.py b/yolov8/ultralytics/yolo/v8/segment/predict.py
new file mode 100644
index 0000000000000000000000000000000000000000..0b6ebc494d22bffc6cc3a4f5607d4691b425db24
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/segment/predict.py
@@ -0,0 +1,63 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+import torch
+
+from ultralytics.yolo.engine.results import Results
+from ultralytics.yolo.utils import DEFAULT_CFG, ROOT, ops
+from ultralytics.yolo.v8.detect.predict import DetectionPredictor
+
+
+class SegmentationPredictor(DetectionPredictor):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ super().__init__(cfg, overrides, _callbacks)
+ self.args.task = 'segment'
+
+ def postprocess(self, preds, img, orig_imgs):
+ """TODO: filter by classes."""
+ p = ops.non_max_suppression(preds[0],
+ self.args.conf,
+ self.args.iou,
+ agnostic=self.args.agnostic_nms,
+ max_det=self.args.max_det,
+ nc=len(self.model.names),
+ classes=self.args.classes)
+ results = []
+ proto = preds[1][-1] if len(preds[1]) == 3 else preds[1] # second output is len 3 if pt, but only 1 if exported
+ for i, pred in enumerate(p):
+ orig_img = orig_imgs[i] if isinstance(orig_imgs, list) else orig_imgs
+ path = self.batch[0]
+ img_path = path[i] if isinstance(path, list) else path
+ if not len(pred): # save empty boxes
+ results.append(Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred[:, :6]))
+ continue
+ if self.args.retina_masks:
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], orig_img.shape)
+ masks = ops.process_mask_native(proto[i], pred[:, 6:], pred[:, :4], orig_img.shape[:2]) # HWC
+ else:
+ masks = ops.process_mask(proto[i], pred[:, 6:], pred[:, :4], img.shape[2:], upsample=True) # HWC
+ if not isinstance(orig_imgs, torch.Tensor):
+ pred[:, :4] = ops.scale_boxes(img.shape[2:], pred[:, :4], orig_img.shape)
+ results.append(
+ Results(orig_img=orig_img, path=img_path, names=self.model.names, boxes=pred[:, :6], masks=masks))
+ return results
+
+
+def predict(cfg=DEFAULT_CFG, use_python=False):
+ """Runs YOLO object detection on an image or video source."""
+ model = cfg.model or 'yolov8n-seg.pt'
+ source = cfg.source if cfg.source is not None else ROOT / 'assets' if (ROOT / 'assets').exists() \
+ else 'https://ultralytics.com/images/bus.jpg'
+
+ args = dict(model=model, source=source)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model)(**args)
+ else:
+ predictor = SegmentationPredictor(overrides=args)
+ predictor.predict_cli()
+
+
+if __name__ == '__main__':
+ predict()
diff --git a/yolov8/ultralytics/yolo/v8/segment/train.py b/yolov8/ultralytics/yolo/v8/segment/train.py
new file mode 100644
index 0000000000000000000000000000000000000000..ab66cf061ac2bdd6ac86b8a8139ba2f81999d62d
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/segment/train.py
@@ -0,0 +1,65 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+from copy import copy
+
+from ultralytics.nn.tasks import SegmentationModel
+from ultralytics.yolo import v8
+from ultralytics.yolo.utils import DEFAULT_CFG, RANK
+from ultralytics.yolo.utils.plotting import plot_images, plot_results
+
+
+# BaseTrainer python usage
+class SegmentationTrainer(v8.detect.DetectionTrainer):
+
+ def __init__(self, cfg=DEFAULT_CFG, overrides=None, _callbacks=None):
+ """Initialize a SegmentationTrainer object with given arguments."""
+ if overrides is None:
+ overrides = {}
+ overrides['task'] = 'segment'
+ super().__init__(cfg, overrides, _callbacks)
+
+ def get_model(self, cfg=None, weights=None, verbose=True):
+ """Return SegmentationModel initialized with specified config and weights."""
+ model = SegmentationModel(cfg, ch=3, nc=self.data['nc'], verbose=verbose and RANK == -1)
+ if weights:
+ model.load(weights)
+
+ return model
+
+ def get_validator(self):
+ """Return an instance of SegmentationValidator for validation of YOLO model."""
+ self.loss_names = 'box_loss', 'seg_loss', 'cls_loss', 'dfl_loss'
+ return v8.segment.SegmentationValidator(self.test_loader, save_dir=self.save_dir, args=copy(self.args))
+
+ def plot_training_samples(self, batch, ni):
+ """Creates a plot of training sample images with labels and box coordinates."""
+ plot_images(batch['img'],
+ batch['batch_idx'],
+ batch['cls'].squeeze(-1),
+ batch['bboxes'],
+ batch['masks'],
+ paths=batch['im_file'],
+ fname=self.save_dir / f'train_batch{ni}.jpg',
+ on_plot=self.on_plot)
+
+ def plot_metrics(self):
+ """Plots training/val metrics."""
+ plot_results(file=self.csv, segment=True, on_plot=self.on_plot) # save results.png
+
+
+def train(cfg=DEFAULT_CFG, use_python=False):
+ """Train a YOLO segmentation model based on passed arguments."""
+ model = cfg.model or 'yolov8n-seg.pt'
+ data = cfg.data or 'coco128-seg.yaml' # or yolo.ClassificationDataset("mnist")
+ device = cfg.device if cfg.device is not None else ''
+
+ args = dict(model=model, data=data, device=device)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).train(**args)
+ else:
+ trainer = SegmentationTrainer(overrides=args)
+ trainer.train()
+
+
+if __name__ == '__main__':
+ train()
diff --git a/yolov8/ultralytics/yolo/v8/segment/val.py b/yolov8/ultralytics/yolo/v8/segment/val.py
new file mode 100644
index 0000000000000000000000000000000000000000..73c2fe834fc16daf5435f5db90046f5d7698e20e
--- /dev/null
+++ b/yolov8/ultralytics/yolo/v8/segment/val.py
@@ -0,0 +1,262 @@
+# Ultralytics YOLO 🚀, AGPL-3.0 license
+
+from multiprocessing.pool import ThreadPool
+from pathlib import Path
+
+import numpy as np
+import torch
+import torch.nn.functional as F
+
+from ultralytics.yolo.utils import DEFAULT_CFG, LOGGER, NUM_THREADS, ops
+from ultralytics.yolo.utils.checks import check_requirements
+from ultralytics.yolo.utils.metrics import SegmentMetrics, box_iou, mask_iou
+from ultralytics.yolo.utils.plotting import output_to_target, plot_images
+from ultralytics.yolo.v8.detect import DetectionValidator
+
+
+class SegmentationValidator(DetectionValidator):
+
+ def __init__(self, dataloader=None, save_dir=None, pbar=None, args=None, _callbacks=None):
+ """Initialize SegmentationValidator and set task to 'segment', metrics to SegmentMetrics."""
+ super().__init__(dataloader, save_dir, pbar, args, _callbacks)
+ self.args.task = 'segment'
+ self.metrics = SegmentMetrics(save_dir=self.save_dir, on_plot=self.on_plot)
+
+ def preprocess(self, batch):
+ """Preprocesses batch by converting masks to float and sending to device."""
+ batch = super().preprocess(batch)
+ batch['masks'] = batch['masks'].to(self.device).float()
+ return batch
+
+ def init_metrics(self, model):
+ """Initialize metrics and select mask processing function based on save_json flag."""
+ super().init_metrics(model)
+ self.plot_masks = []
+ if self.args.save_json:
+ check_requirements('pycocotools>=2.0.6')
+ self.process = ops.process_mask_upsample # more accurate
+ else:
+ self.process = ops.process_mask # faster
+
+ def get_desc(self):
+ """Return a formatted description of evaluation metrics."""
+ return ('%22s' + '%11s' * 10) % ('Class', 'Images', 'Instances', 'Box(P', 'R', 'mAP50', 'mAP50-95)', 'Mask(P',
+ 'R', 'mAP50', 'mAP50-95)')
+
+ def postprocess(self, preds):
+ """Postprocesses YOLO predictions and returns output detections with proto."""
+ p = ops.non_max_suppression(preds[0],
+ self.args.conf,
+ self.args.iou,
+ labels=self.lb,
+ multi_label=True,
+ agnostic=self.args.single_cls,
+ max_det=self.args.max_det,
+ nc=self.nc)
+ proto = preds[1][-1] if len(preds[1]) == 3 else preds[1] # second output is len 3 if pt, but only 1 if exported
+ return p, proto
+
+ def update_metrics(self, preds, batch):
+ """Metrics."""
+ for si, (pred, proto) in enumerate(zip(preds[0], preds[1])):
+ idx = batch['batch_idx'] == si
+ cls = batch['cls'][idx]
+ bbox = batch['bboxes'][idx]
+ nl, npr = cls.shape[0], pred.shape[0] # number of labels, predictions
+ shape = batch['ori_shape'][si]
+ correct_masks = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ correct_bboxes = torch.zeros(npr, self.niou, dtype=torch.bool, device=self.device) # init
+ self.seen += 1
+
+ if npr == 0:
+ if nl:
+ self.stats.append((correct_bboxes, correct_masks, *torch.zeros(
+ (2, 0), device=self.device), cls.squeeze(-1)))
+ if self.args.plots:
+ self.confusion_matrix.process_batch(detections=None, labels=cls.squeeze(-1))
+ continue
+
+ # Masks
+ midx = [si] if self.args.overlap_mask else idx
+ gt_masks = batch['masks'][midx]
+ pred_masks = self.process(proto, pred[:, 6:], pred[:, :4], shape=batch['img'][si].shape[1:])
+
+ # Predictions
+ if self.args.single_cls:
+ pred[:, 5] = 0
+ predn = pred.clone()
+ ops.scale_boxes(batch['img'][si].shape[1:], predn[:, :4], shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space pred
+
+ # Evaluate
+ if nl:
+ height, width = batch['img'].shape[2:]
+ tbox = ops.xywh2xyxy(bbox) * torch.tensor(
+ (width, height, width, height), device=self.device) # target boxes
+ ops.scale_boxes(batch['img'][si].shape[1:], tbox, shape,
+ ratio_pad=batch['ratio_pad'][si]) # native-space labels
+ labelsn = torch.cat((cls, tbox), 1) # native-space labels
+ correct_bboxes = self._process_batch(predn, labelsn)
+ # TODO: maybe remove these `self.` arguments as they already are member variable
+ correct_masks = self._process_batch(predn,
+ labelsn,
+ pred_masks,
+ gt_masks,
+ overlap=self.args.overlap_mask,
+ masks=True)
+ if self.args.plots:
+ self.confusion_matrix.process_batch(predn, labelsn)
+
+ # Append correct_masks, correct_boxes, pconf, pcls, tcls
+ self.stats.append((correct_bboxes, correct_masks, pred[:, 4], pred[:, 5], cls.squeeze(-1)))
+
+ pred_masks = torch.as_tensor(pred_masks, dtype=torch.uint8)
+ if self.args.plots and self.batch_i < 3:
+ self.plot_masks.append(pred_masks[:15].cpu()) # filter top 15 to plot
+
+ # Save
+ if self.args.save_json:
+ pred_masks = ops.scale_image(pred_masks.permute(1, 2, 0).contiguous().cpu().numpy(),
+ shape,
+ ratio_pad=batch['ratio_pad'][si])
+ self.pred_to_json(predn, batch['im_file'][si], pred_masks)
+ # if self.args.save_txt:
+ # save_one_txt(predn, save_conf, shape, file=save_dir / 'labels' / f'{path.stem}.txt')
+
+ def finalize_metrics(self, *args, **kwargs):
+ """Sets speed and confusion matrix for evaluation metrics."""
+ self.metrics.speed = self.speed
+ self.metrics.confusion_matrix = self.confusion_matrix
+
+ def _process_batch(self, detections, labels, pred_masks=None, gt_masks=None, overlap=False, masks=False):
+ """
+ Return correct prediction matrix
+ Arguments:
+ detections (array[N, 6]), x1, y1, x2, y2, conf, class
+ labels (array[M, 5]), class, x1, y1, x2, y2
+ Returns:
+ correct (array[N, 10]), for 10 IoU levels
+ """
+ if masks:
+ if overlap:
+ nl = len(labels)
+ index = torch.arange(nl, device=gt_masks.device).view(nl, 1, 1) + 1
+ gt_masks = gt_masks.repeat(nl, 1, 1) # shape(1,640,640) -> (n,640,640)
+ gt_masks = torch.where(gt_masks == index, 1.0, 0.0)
+ if gt_masks.shape[1:] != pred_masks.shape[1:]:
+ gt_masks = F.interpolate(gt_masks[None], pred_masks.shape[1:], mode='bilinear', align_corners=False)[0]
+ gt_masks = gt_masks.gt_(0.5)
+ iou = mask_iou(gt_masks.view(gt_masks.shape[0], -1), pred_masks.view(pred_masks.shape[0], -1))
+ else: # boxes
+ iou = box_iou(labels[:, 1:], detections[:, :4])
+
+ correct = np.zeros((detections.shape[0], self.iouv.shape[0])).astype(bool)
+ correct_class = labels[:, 0:1] == detections[:, 5]
+ for i in range(len(self.iouv)):
+ x = torch.where((iou >= self.iouv[i]) & correct_class) # IoU > threshold and classes match
+ if x[0].shape[0]:
+ matches = torch.cat((torch.stack(x, 1), iou[x[0], x[1]][:, None]),
+ 1).cpu().numpy() # [label, detect, iou]
+ if x[0].shape[0] > 1:
+ matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 1], return_index=True)[1]]
+ # matches = matches[matches[:, 2].argsort()[::-1]]
+ matches = matches[np.unique(matches[:, 0], return_index=True)[1]]
+ correct[matches[:, 1].astype(int), i] = True
+ return torch.tensor(correct, dtype=torch.bool, device=detections.device)
+
+ def plot_val_samples(self, batch, ni):
+ """Plots validation samples with bounding box labels."""
+ plot_images(batch['img'],
+ batch['batch_idx'],
+ batch['cls'].squeeze(-1),
+ batch['bboxes'],
+ batch['masks'],
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_labels.jpg',
+ names=self.names,
+ on_plot=self.on_plot)
+
+ def plot_predictions(self, batch, preds, ni):
+ """Plots batch predictions with masks and bounding boxes."""
+ plot_images(
+ batch['img'],
+ *output_to_target(preds[0], max_det=15), # not set to self.args.max_det due to slow plotting speed
+ torch.cat(self.plot_masks, dim=0) if len(self.plot_masks) else self.plot_masks,
+ paths=batch['im_file'],
+ fname=self.save_dir / f'val_batch{ni}_pred.jpg',
+ names=self.names,
+ on_plot=self.on_plot) # pred
+ self.plot_masks.clear()
+
+ def pred_to_json(self, predn, filename, pred_masks):
+ """Save one JSON result."""
+ # Example result = {"image_id": 42, "category_id": 18, "bbox": [258.15, 41.29, 348.26, 243.78], "score": 0.236}
+ from pycocotools.mask import encode # noqa
+
+ def single_encode(x):
+ """Encode predicted masks as RLE and append results to jdict."""
+ rle = encode(np.asarray(x[:, :, None], order='F', dtype='uint8'))[0]
+ rle['counts'] = rle['counts'].decode('utf-8')
+ return rle
+
+ stem = Path(filename).stem
+ image_id = int(stem) if stem.isnumeric() else stem
+ box = ops.xyxy2xywh(predn[:, :4]) # xywh
+ box[:, :2] -= box[:, 2:] / 2 # xy center to top-left corner
+ pred_masks = np.transpose(pred_masks, (2, 0, 1))
+ with ThreadPool(NUM_THREADS) as pool:
+ rles = pool.map(single_encode, pred_masks)
+ for i, (p, b) in enumerate(zip(predn.tolist(), box.tolist())):
+ self.jdict.append({
+ 'image_id': image_id,
+ 'category_id': self.class_map[int(p[5])],
+ 'bbox': [round(x, 3) for x in b],
+ 'score': round(p[4], 5),
+ 'segmentation': rles[i]})
+
+ def eval_json(self, stats):
+ """Return COCO-style object detection evaluation metrics."""
+ if self.args.save_json and self.is_coco and len(self.jdict):
+ anno_json = self.data['path'] / 'annotations/instances_val2017.json' # annotations
+ pred_json = self.save_dir / 'predictions.json' # predictions
+ LOGGER.info(f'\nEvaluating pycocotools mAP using {pred_json} and {anno_json}...')
+ try: # https://github.com/cocodataset/cocoapi/blob/master/PythonAPI/pycocoEvalDemo.ipynb
+ check_requirements('pycocotools>=2.0.6')
+ from pycocotools.coco import COCO # noqa
+ from pycocotools.cocoeval import COCOeval # noqa
+
+ for x in anno_json, pred_json:
+ assert x.is_file(), f'{x} file not found'
+ anno = COCO(str(anno_json)) # init annotations api
+ pred = anno.loadRes(str(pred_json)) # init predictions api (must pass string, not Path)
+ for i, eval in enumerate([COCOeval(anno, pred, 'bbox'), COCOeval(anno, pred, 'segm')]):
+ if self.is_coco:
+ eval.params.imgIds = [int(Path(x).stem) for x in self.dataloader.dataset.im_files] # im to eval
+ eval.evaluate()
+ eval.accumulate()
+ eval.summarize()
+ idx = i * 4 + 2
+ stats[self.metrics.keys[idx + 1]], stats[
+ self.metrics.keys[idx]] = eval.stats[:2] # update mAP50-95 and mAP50
+ except Exception as e:
+ LOGGER.warning(f'pycocotools unable to run: {e}')
+ return stats
+
+
+def val(cfg=DEFAULT_CFG, use_python=False):
+ """Validate trained YOLO model on validation data."""
+ model = cfg.model or 'yolov8n-seg.pt'
+ data = cfg.data or 'coco128-seg.yaml'
+
+ args = dict(model=model, data=data)
+ if use_python:
+ from ultralytics import YOLO
+ YOLO(model).val(**args)
+ else:
+ validator = SegmentationValidator(args=args)
+ validator(model=args['model'])
+
+
+if __name__ == '__main__':
+ val()