diff --git a/app.py b/app.py
new file mode 100644
index 0000000000000000000000000000000000000000..bbf6c454b0dc228f3a27a304d1b28047f539a952
--- /dev/null
+++ b/app.py
@@ -0,0 +1,249 @@
+"""
+Adapted from: https://github.com/Vision-CAIR/MiniGPT-4/blob/main/demo.py
+"""
+import argparse
+import os
+import sys
+import random
+
+import numpy as np
+import torch
+import torch.backends.cudnn as cudnn
+import gradio as gr
+
+from global_local.common.config import Config
+from global_local.common.dist_utils import get_rank
+from global_local.common.registry import registry
+from global_local.conversation.conversation_video import Chat, Conversation, default_conversation,SeparatorStyle,conv_llava_llama_2
+import decord
+decord.bridge.set_bridge('torch')
+
+#%%
+# imports modules for registration
+from global_local.datasets.builders import *
+from global_local.models import *
+from global_local.processors import *
+from global_local.runners import *
+from global_local.tasks import *
+
+#%%
+def parse_args():
+ parser = argparse.ArgumentParser(description="Demo")
+ #parser.add_argument("--cfg-path", required=True, help="path to configuration file.")
+ parser.add_argument("--cfg-path", type=str, default='./eval_configs/conversation_demo.yaml', help="path to configuration file.")
+ parser.add_argument("--gpu-id", type=int, default=0, help="specify the gpu to load the model.")
+ parser.add_argument("--model_type", type=str, default='llama_v2', help="specify LLM")
+ parser.add_argument('--pretrained_weight_path', type=str, default="./ckpt/finetuned_model.pth", metavar='PWP',
+ help='path to pretrained weight path')
+ parser.add_argument('--num_frames_per_clip', type=int, default=16, metavar='NPPC',
+ help='specify how frames to use per clip')
+ parser.add_argument('--num_segments', type=int, default=4, metavar='NS',
+ help='specify number of video segments')
+ parser.add_argument('--hierarchical_agg_function', type=str, default="without-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned", metavar='HAF',
+ help='specify function to merge global and clip visual representations')
+
+ parser.add_argument(
+ "--options",
+ nargs="+",
+ help="override some settings in the used config, the key-value pair "
+ "in xxx=yyy format will be merged into config file (deprecate), "
+ "change to --cfg-options instead.",
+ )
+ args = parser.parse_args()
+ return args
+
+
+def setup_seeds(config):
+ seed = config.run_cfg.seed + get_rank()
+
+ random.seed(seed)
+ np.random.seed(seed)
+ torch.manual_seed(seed)
+
+ cudnn.benchmark = False
+ cudnn.deterministic = True
+
+
+# ========================================
+# Model Initialization
+# ========================================
+
+print('Initializing Chat')
+args = parse_args()
+cfg = Config(args)
+
+model_config = cfg.model_cfg
+model_config.device_8bit = args.gpu_id
+model_cls = registry.get_model_class(model_config.arch)
+model = model_cls.from_config(model_config).to('cuda:{}'.format(args.gpu_id))
+
+model.num_frames_per_clip = args.num_frames_per_clip
+model.num_segments = args.num_segments
+model.hierarchical_agg_function = args.hierarchical_agg_function
+model.global_region_embed_weight = None
+
+model.initialize_visual_agg_function()
+
+best_checkpoint = torch.load(args.pretrained_weight_path, map_location='cpu')['model_state_dict']
+pretrained_dict = {}
+for k, v in best_checkpoint.items():
+ pretrained_dict[k.replace('module.', '')] = v
+
+model_dict = model.state_dict()
+model_dict.update(pretrained_dict)
+model.load_state_dict(model_dict)
+model.cuda().eval()
+
+#vis_processor_cfg = cfg.datasets_cfg.cc_sbu_align.vis_processor.train
+vis_processor_cfg = cfg.datasets_cfg.webvid.vis_processor.train
+vis_processor = registry.get_processor_class(vis_processor_cfg.name).from_config(vis_processor_cfg)
+chat = Chat(model, vis_processor, device='cuda:{}'.format(args.gpu_id))
+print('Initialization Finished')
+
+# ========================================
+# Gradio Setting
+# ========================================
+
+def gradio_reset(chat_state, img_list):
+ if chat_state is not None:
+ chat_state.messages = []
+ if img_list is not None:
+ img_list = []
+ return None, gr.update(value=None, interactive=True), gr.update(value=None, interactive=True), gr.update(placeholder='Please upload your video first', interactive=False),gr.update(value="Upload & Start Chat", interactive=True), chat_state, img_list
+
+def upload_imgorvideo(gr_video, gr_img, text_input, chat_state,chatbot):
+ if args.model_type == 'vicuna':
+ chat_state = default_conversation.copy()
+ else:
+ chat_state = conv_llava_llama_2.copy()
+ if gr_img is None and gr_video is None:
+ return None, None, None, gr.update(interactive=True), chat_state, None
+ elif gr_img is not None and gr_video is None:
+ print(gr_img)
+ chatbot = chatbot + [((gr_img,), None)]
+ chat_state.system = "You are able to understand the visual content that the user provides. Follow the instructions carefully and explain your answers in detail."
+ img_list = []
+ llm_message = chat.upload_img(gr_img, chat_state, img_list)
+ return gr.update(interactive=False), gr.update(interactive=False), gr.update(interactive=True, placeholder='Type and press Enter'), gr.update(value="Start Chatting", interactive=False), chat_state, img_list,chatbot
+ elif gr_video is not None and gr_img is None:
+ print(gr_video)
+ chatbot = chatbot + [((gr_video,), None)]
+ chat_state.system = "You are able to understand the visual content that the user provides. Follow the instructions carefully and explain your answers in detail."
+ img_list = []
+ llm_message = chat.upload_video_without_audio(gr_video, chat_state, img_list)
+ return gr.update(interactive=False), gr.update(interactive=False), gr.update(interactive=True, placeholder='Type and press Enter'), gr.update(value="Start Chatting", interactive=False), chat_state, img_list,chatbot
+ else:
+ # img_list = []
+ return gr.update(interactive=False), gr.update(interactive=False, placeholder='Currently, only one input is supported'), gr.update(value="Currently, only one input is supported", interactive=False), chat_state, None,chatbot
+
+def gradio_ask(user_message, chatbot, chat_state):
+ if len(user_message) == 0:
+ return gr.update(interactive=True, placeholder='Input should not be empty!'), chatbot, chat_state
+ chat.ask(user_message, chat_state)
+ chatbot = chatbot + [[user_message, None]]
+ return '', chatbot, chat_state
+
+
+def gradio_answer(chatbot, chat_state, img_list, num_beams, temperature):
+ llm_message = chat.answer(conv=chat_state,
+ img_list=img_list,
+ num_beams=num_beams,
+ temperature=temperature,
+ max_new_tokens=300,
+ max_length=2000)[0]
+ chatbot[-1][1] = llm_message
+ print(chat_state.get_prompt())
+ print(chat_state)
+ return chatbot, chat_state, img_list
+
+title = """
+
Global-Local QFormer for Long Video Understanding with LLMs
+
+ Introduction: We introduce a Global-Local QFormer video model that is connected with a Large Language Model to understand \
+ and answer questions about long videos.
+
+
+

+

+
+
+
+Thank you for using the Global-Local QFormer Demo Page! If you have any questions or feedback, feel free to contact us.
+Current online demo uses the 7B version of Llama-2 due to resource limitations.
+
+
+"""
+
+Note_markdown = ("""
+### We note that our Global-Local QFormer model may be limited at understanding videos from rare domains. Due to the pretraining data, the \
+ model may be susceptible to hallucinations
+We would like to acknowledge the Video-LLama repository which we copied the demo layout from.
+
+**Boston University**
+""")
+
+cite_markdown = ("""
+""")
+
+#case_note_upload = ("""
+### We provide some examples at the bottom of the page. Simply click on them to try them out directly.
+#""")
+
+#TODO show examples below
+
+with gr.Blocks() as demo:
+ gr.Markdown(title)
+
+ with gr.Row():
+ with gr.Column(scale=0.5):
+ video = gr.Video()
+ #image = gr.Image(type="filepath")
+ #gr.Markdown(case_note_upload)
+
+ upload_button = gr.Button(value="Upload & Start Chat", interactive=True, variant="primary")
+ clear = gr.Button("Restart")
+
+ num_beams = gr.Slider(
+ minimum=1,
+ maximum=10,
+ value=1,
+ step=1,
+ interactive=True,
+ label="beam search numbers)",
+ )
+
+ temperature = gr.Slider(
+ minimum=0.1,
+ maximum=2.0,
+ value=1.0,
+ step=0.1,
+ interactive=True,
+ label="Temperature",
+ )
+
+ audio = gr.Checkbox(interactive=True, value=False, label="Audio")
+ gr.Markdown(Note_markdown)
+ with gr.Column():
+ chat_state = gr.State()
+ img_list = gr.State()
+ chatbot = gr.Chatbot(label='Global-Local QFormer')
+ text_input = gr.Textbox(label='User', placeholder='Please upload your video first.', interactive=False)
+
+
+ '''with gr.Column():
+ gr.Examples(examples=[
+ [f"examples/skateboarding_dog.mp4", "What is the dog doing? "],
+ [f"examples/birthday.mp4", "What is the boy doing? "],
+ [f"examples/IronMan.mp4", "Is the guy in the video Iron Man? "],
+ ], inputs=[video, text_input])'''
+
+ gr.Markdown(cite_markdown)
+ upload_button.click(upload_imgorvideo, [video, text_input, chat_state,chatbot], [video, text_input, upload_button, chat_state, img_list,chatbot])
+
+ text_input.submit(gradio_ask, [text_input, chatbot, chat_state], [text_input, chatbot, chat_state]).then(
+ gradio_answer, [chatbot, chat_state, img_list, num_beams, temperature], [chatbot, chat_state, img_list]
+ )
+ clear.click(gradio_reset, [chat_state, img_list], [chatbot, video, text_input, upload_button, chat_state, img_list], queue=False)
+
+#demo.launch(share=False, enable_queue=True, debug=True)
+demo.launch(share=False, debug=True)
\ No newline at end of file
diff --git a/ckpt/VL_LLaMA_2_7B_Finetuned.pth b/ckpt/VL_LLaMA_2_7B_Finetuned.pth
new file mode 100644
index 0000000000000000000000000000000000000000..f82c60f73916ba70b757a13de56b325e362fd5d7
--- /dev/null
+++ b/ckpt/VL_LLaMA_2_7B_Finetuned.pth
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:3cec0e2979ed7656e08ecc5b185c2229a3c577b4b7a4721a94bd461ba0447c6e
+size 265559201
diff --git a/ckpt/finetuned_model.pth b/ckpt/finetuned_model.pth
new file mode 100644
index 0000000000000000000000000000000000000000..3a4175736d0d65d03a2830133cce04fa5d3fb3f4
--- /dev/null
+++ b/ckpt/finetuned_model.pth
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:3795447e3459f467aae141873ba5f666efcc0f1478ddd9316437d3ba56aa72fd
+size 38852011
diff --git a/demo_video.py b/demo_video.py
new file mode 100644
index 0000000000000000000000000000000000000000..bbf6c454b0dc228f3a27a304d1b28047f539a952
--- /dev/null
+++ b/demo_video.py
@@ -0,0 +1,249 @@
+"""
+Adapted from: https://github.com/Vision-CAIR/MiniGPT-4/blob/main/demo.py
+"""
+import argparse
+import os
+import sys
+import random
+
+import numpy as np
+import torch
+import torch.backends.cudnn as cudnn
+import gradio as gr
+
+from global_local.common.config import Config
+from global_local.common.dist_utils import get_rank
+from global_local.common.registry import registry
+from global_local.conversation.conversation_video import Chat, Conversation, default_conversation,SeparatorStyle,conv_llava_llama_2
+import decord
+decord.bridge.set_bridge('torch')
+
+#%%
+# imports modules for registration
+from global_local.datasets.builders import *
+from global_local.models import *
+from global_local.processors import *
+from global_local.runners import *
+from global_local.tasks import *
+
+#%%
+def parse_args():
+ parser = argparse.ArgumentParser(description="Demo")
+ #parser.add_argument("--cfg-path", required=True, help="path to configuration file.")
+ parser.add_argument("--cfg-path", type=str, default='./eval_configs/conversation_demo.yaml', help="path to configuration file.")
+ parser.add_argument("--gpu-id", type=int, default=0, help="specify the gpu to load the model.")
+ parser.add_argument("--model_type", type=str, default='llama_v2', help="specify LLM")
+ parser.add_argument('--pretrained_weight_path', type=str, default="./ckpt/finetuned_model.pth", metavar='PWP',
+ help='path to pretrained weight path')
+ parser.add_argument('--num_frames_per_clip', type=int, default=16, metavar='NPPC',
+ help='specify how frames to use per clip')
+ parser.add_argument('--num_segments', type=int, default=4, metavar='NS',
+ help='specify number of video segments')
+ parser.add_argument('--hierarchical_agg_function', type=str, default="without-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned", metavar='HAF',
+ help='specify function to merge global and clip visual representations')
+
+ parser.add_argument(
+ "--options",
+ nargs="+",
+ help="override some settings in the used config, the key-value pair "
+ "in xxx=yyy format will be merged into config file (deprecate), "
+ "change to --cfg-options instead.",
+ )
+ args = parser.parse_args()
+ return args
+
+
+def setup_seeds(config):
+ seed = config.run_cfg.seed + get_rank()
+
+ random.seed(seed)
+ np.random.seed(seed)
+ torch.manual_seed(seed)
+
+ cudnn.benchmark = False
+ cudnn.deterministic = True
+
+
+# ========================================
+# Model Initialization
+# ========================================
+
+print('Initializing Chat')
+args = parse_args()
+cfg = Config(args)
+
+model_config = cfg.model_cfg
+model_config.device_8bit = args.gpu_id
+model_cls = registry.get_model_class(model_config.arch)
+model = model_cls.from_config(model_config).to('cuda:{}'.format(args.gpu_id))
+
+model.num_frames_per_clip = args.num_frames_per_clip
+model.num_segments = args.num_segments
+model.hierarchical_agg_function = args.hierarchical_agg_function
+model.global_region_embed_weight = None
+
+model.initialize_visual_agg_function()
+
+best_checkpoint = torch.load(args.pretrained_weight_path, map_location='cpu')['model_state_dict']
+pretrained_dict = {}
+for k, v in best_checkpoint.items():
+ pretrained_dict[k.replace('module.', '')] = v
+
+model_dict = model.state_dict()
+model_dict.update(pretrained_dict)
+model.load_state_dict(model_dict)
+model.cuda().eval()
+
+#vis_processor_cfg = cfg.datasets_cfg.cc_sbu_align.vis_processor.train
+vis_processor_cfg = cfg.datasets_cfg.webvid.vis_processor.train
+vis_processor = registry.get_processor_class(vis_processor_cfg.name).from_config(vis_processor_cfg)
+chat = Chat(model, vis_processor, device='cuda:{}'.format(args.gpu_id))
+print('Initialization Finished')
+
+# ========================================
+# Gradio Setting
+# ========================================
+
+def gradio_reset(chat_state, img_list):
+ if chat_state is not None:
+ chat_state.messages = []
+ if img_list is not None:
+ img_list = []
+ return None, gr.update(value=None, interactive=True), gr.update(value=None, interactive=True), gr.update(placeholder='Please upload your video first', interactive=False),gr.update(value="Upload & Start Chat", interactive=True), chat_state, img_list
+
+def upload_imgorvideo(gr_video, gr_img, text_input, chat_state,chatbot):
+ if args.model_type == 'vicuna':
+ chat_state = default_conversation.copy()
+ else:
+ chat_state = conv_llava_llama_2.copy()
+ if gr_img is None and gr_video is None:
+ return None, None, None, gr.update(interactive=True), chat_state, None
+ elif gr_img is not None and gr_video is None:
+ print(gr_img)
+ chatbot = chatbot + [((gr_img,), None)]
+ chat_state.system = "You are able to understand the visual content that the user provides. Follow the instructions carefully and explain your answers in detail."
+ img_list = []
+ llm_message = chat.upload_img(gr_img, chat_state, img_list)
+ return gr.update(interactive=False), gr.update(interactive=False), gr.update(interactive=True, placeholder='Type and press Enter'), gr.update(value="Start Chatting", interactive=False), chat_state, img_list,chatbot
+ elif gr_video is not None and gr_img is None:
+ print(gr_video)
+ chatbot = chatbot + [((gr_video,), None)]
+ chat_state.system = "You are able to understand the visual content that the user provides. Follow the instructions carefully and explain your answers in detail."
+ img_list = []
+ llm_message = chat.upload_video_without_audio(gr_video, chat_state, img_list)
+ return gr.update(interactive=False), gr.update(interactive=False), gr.update(interactive=True, placeholder='Type and press Enter'), gr.update(value="Start Chatting", interactive=False), chat_state, img_list,chatbot
+ else:
+ # img_list = []
+ return gr.update(interactive=False), gr.update(interactive=False, placeholder='Currently, only one input is supported'), gr.update(value="Currently, only one input is supported", interactive=False), chat_state, None,chatbot
+
+def gradio_ask(user_message, chatbot, chat_state):
+ if len(user_message) == 0:
+ return gr.update(interactive=True, placeholder='Input should not be empty!'), chatbot, chat_state
+ chat.ask(user_message, chat_state)
+ chatbot = chatbot + [[user_message, None]]
+ return '', chatbot, chat_state
+
+
+def gradio_answer(chatbot, chat_state, img_list, num_beams, temperature):
+ llm_message = chat.answer(conv=chat_state,
+ img_list=img_list,
+ num_beams=num_beams,
+ temperature=temperature,
+ max_new_tokens=300,
+ max_length=2000)[0]
+ chatbot[-1][1] = llm_message
+ print(chat_state.get_prompt())
+ print(chat_state)
+ return chatbot, chat_state, img_list
+
+title = """
+Global-Local QFormer for Long Video Understanding with LLMs
+
+ Introduction: We introduce a Global-Local QFormer video model that is connected with a Large Language Model to understand \
+ and answer questions about long videos.
+
+
+

+

+
+
+
+Thank you for using the Global-Local QFormer Demo Page! If you have any questions or feedback, feel free to contact us.
+Current online demo uses the 7B version of Llama-2 due to resource limitations.
+
+
+"""
+
+Note_markdown = ("""
+### We note that our Global-Local QFormer model may be limited at understanding videos from rare domains. Due to the pretraining data, the \
+ model may be susceptible to hallucinations
+We would like to acknowledge the Video-LLama repository which we copied the demo layout from.
+
+**Boston University**
+""")
+
+cite_markdown = ("""
+""")
+
+#case_note_upload = ("""
+### We provide some examples at the bottom of the page. Simply click on them to try them out directly.
+#""")
+
+#TODO show examples below
+
+with gr.Blocks() as demo:
+ gr.Markdown(title)
+
+ with gr.Row():
+ with gr.Column(scale=0.5):
+ video = gr.Video()
+ #image = gr.Image(type="filepath")
+ #gr.Markdown(case_note_upload)
+
+ upload_button = gr.Button(value="Upload & Start Chat", interactive=True, variant="primary")
+ clear = gr.Button("Restart")
+
+ num_beams = gr.Slider(
+ minimum=1,
+ maximum=10,
+ value=1,
+ step=1,
+ interactive=True,
+ label="beam search numbers)",
+ )
+
+ temperature = gr.Slider(
+ minimum=0.1,
+ maximum=2.0,
+ value=1.0,
+ step=0.1,
+ interactive=True,
+ label="Temperature",
+ )
+
+ audio = gr.Checkbox(interactive=True, value=False, label="Audio")
+ gr.Markdown(Note_markdown)
+ with gr.Column():
+ chat_state = gr.State()
+ img_list = gr.State()
+ chatbot = gr.Chatbot(label='Global-Local QFormer')
+ text_input = gr.Textbox(label='User', placeholder='Please upload your video first.', interactive=False)
+
+
+ '''with gr.Column():
+ gr.Examples(examples=[
+ [f"examples/skateboarding_dog.mp4", "What is the dog doing? "],
+ [f"examples/birthday.mp4", "What is the boy doing? "],
+ [f"examples/IronMan.mp4", "Is the guy in the video Iron Man? "],
+ ], inputs=[video, text_input])'''
+
+ gr.Markdown(cite_markdown)
+ upload_button.click(upload_imgorvideo, [video, text_input, chat_state,chatbot], [video, text_input, upload_button, chat_state, img_list,chatbot])
+
+ text_input.submit(gradio_ask, [text_input, chatbot, chat_state], [text_input, chatbot, chat_state]).then(
+ gradio_answer, [chatbot, chat_state, img_list, num_beams, temperature], [chatbot, chat_state, img_list]
+ )
+ clear.click(gradio_reset, [chat_state, img_list], [chatbot, video, text_input, upload_button, chat_state, img_list], queue=False)
+
+#demo.launch(share=False, enable_queue=True, debug=True)
+demo.launch(share=False, debug=True)
\ No newline at end of file
diff --git a/eval_configs/conversation_demo.yaml b/eval_configs/conversation_demo.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..e13495759baa5a35d4b7526813393f8202d6f930
--- /dev/null
+++ b/eval_configs/conversation_demo.yaml
@@ -0,0 +1,78 @@
+model:
+ arch: video_instruction_llama
+ model_type: pretrain_vicuna
+ freeze_vit: True
+ freeze_qformer: True
+
+
+ # Q-Former
+ num_query_token: 32
+
+ # If you want train models based on LLaMA-2-chat,
+ # some ckpts could be download from our provided huggingface repo
+ # i.e. https://huggingface.co/DAMO-NLP-SG/Video-LLaMA-2-13B-Finetuned llama-2-7b-chat-hf
+ #llama_model: "/projectnb/ivc-ml/rxtan/llama-2-7b-chat-hf/"
+ llama_model: "Video-LLaMA-2-7B-Finetuned/llama-2-7b-chat-hf/"
+ imagebind_ckpt_path: "ckpt/imagebind_path/"
+
+ # The ckpt of vision branch after stage1 pretrained,
+ ckpt: 'ckpt/VL_LLaMA_2_7B_Finetuned.pth' # you can use our pretrained ckpt from https://huggingface.co/DAMO-NLP-SG/Video-LLaMA-2-13B-Pretrained/
+
+
+ # only train vision branch
+ equip_audio_branch: False # whether equips the audio branch
+ frozen_llama_proj: False
+ frozen_video_Qformer: True
+ frozen_audio_Qformer: True
+
+ fusion_head_layers: 2
+ max_frame_pos: 32
+ fusion_header_type: "seqTransf"
+
+ max_txt_len: 320
+
+ # for llama_2_chat:
+ end_sym: ""
+ prompt_path: "prompts/alignment_image.txt"
+ prompt_template: '[INST] <>\n \n<>\n\n{} [/INST] '
+
+datasets:
+ webvid:
+ vis_processor:
+ train:
+ name: "alpro_video_eval"
+ n_frms: 8
+ image_size: 224
+ text_processor:
+ train:
+ name: "blip_caption"
+
+run:
+ task: video_text_pretrain
+ # optimizer
+ lr_sched: "linear_warmup_cosine_lr"
+ init_lr: 3e-5
+ min_lr: 1e-5
+ warmup_lr: 1e-6
+
+ weight_decay: 0.05
+ max_epoch: 3
+ iters_per_epoch: 1000
+ batch_size_train: 4
+ batch_size_eval: 4
+ num_workers: 4
+ warmup_steps: 1000
+
+ seed: 42
+ output_dir: "output/videollama_stage2_finetune"
+
+ amp: True
+ resume_ckpt_path: null
+
+ evaluate: False
+ train_splits: ["train"]
+
+ device: "cuda"
+ world_size: 1
+ dist_url: "env://"
+ distributed: True
\ No newline at end of file
diff --git a/global_local/__init__.py b/global_local/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..cee783478304563969f33d926fc4f7965d337d33
--- /dev/null
+++ b/global_local/__init__.py
@@ -0,0 +1,31 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import os
+import sys
+
+from omegaconf import OmegaConf
+
+from global_local.common.registry import registry
+
+from global_local.datasets.builders import *
+from global_local.models import *
+from global_local.processors import *
+from global_local.tasks import *
+
+
+root_dir = os.path.dirname(os.path.abspath(__file__))
+default_cfg = OmegaConf.load(os.path.join(root_dir, "configs/default.yaml"))
+
+registry.register_path("library_root", root_dir)
+repo_root = os.path.join(root_dir, "..")
+registry.register_path("repo_root", repo_root)
+cache_root = os.path.join(repo_root, default_cfg.env.cache_root)
+registry.register_path("cache_root", cache_root)
+
+registry.register("MAX_INT", sys.maxsize)
+registry.register("SPLIT_NAMES", ["train", "val", "test"])
diff --git a/global_local/__pycache__/__init__.cpython-39.pyc b/global_local/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..cd6460a0831d2a9e0b3488351448849000bcbddc
Binary files /dev/null and b/global_local/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/common/__init__.py b/global_local/common/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/global_local/common/__pycache__/__init__.cpython-39.pyc b/global_local/common/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..b3d55707bebfec431f14307c519507218b1c5708
Binary files /dev/null and b/global_local/common/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/common/__pycache__/config.cpython-39.pyc b/global_local/common/__pycache__/config.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..63e0447efcaa9137696ffc083a9c49781b26a747
Binary files /dev/null and b/global_local/common/__pycache__/config.cpython-39.pyc differ
diff --git a/global_local/common/__pycache__/dist_utils.cpython-39.pyc b/global_local/common/__pycache__/dist_utils.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..79f8264df7e14969428a751110b68935aea23fc8
Binary files /dev/null and b/global_local/common/__pycache__/dist_utils.cpython-39.pyc differ
diff --git a/global_local/common/__pycache__/logger.cpython-39.pyc b/global_local/common/__pycache__/logger.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..dc35b86674a4d7e7487554a0c7941aad13874b4a
Binary files /dev/null and b/global_local/common/__pycache__/logger.cpython-39.pyc differ
diff --git a/global_local/common/__pycache__/optims.cpython-39.pyc b/global_local/common/__pycache__/optims.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..ad736d956db280833b5ae7c9bb33922fe6d5cb1d
Binary files /dev/null and b/global_local/common/__pycache__/optims.cpython-39.pyc differ
diff --git a/global_local/common/__pycache__/registry.cpython-39.pyc b/global_local/common/__pycache__/registry.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..ed0a25742f0d00fc671701d792d0b0fefa82cdaf
Binary files /dev/null and b/global_local/common/__pycache__/registry.cpython-39.pyc differ
diff --git a/global_local/common/__pycache__/utils.cpython-39.pyc b/global_local/common/__pycache__/utils.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..f4c7e10c08e44620b7b00c1b8d12af5571b396f9
Binary files /dev/null and b/global_local/common/__pycache__/utils.cpython-39.pyc differ
diff --git a/global_local/common/config.py b/global_local/common/config.py
new file mode 100644
index 0000000000000000000000000000000000000000..6cec1bafb79cc4e49ba4ccb88c00fafe7724368e
--- /dev/null
+++ b/global_local/common/config.py
@@ -0,0 +1,468 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import logging
+import json
+from typing import Dict
+
+from omegaconf import OmegaConf
+from global_local.common.registry import registry
+
+
+class Config:
+ def __init__(self, args):
+ self.config = {}
+
+ self.args = args
+
+ # Register the config and configuration for setup
+ registry.register("configuration", self)
+
+ user_config = self._build_opt_list(self.args.options)
+
+ config = OmegaConf.load(self.args.cfg_path)
+
+ runner_config = self.build_runner_config(config)
+ model_config = self.build_model_config(config, **user_config)
+ dataset_config = self.build_dataset_config(config)
+
+ # Validate the user-provided runner configuration
+ # model and dataset configuration are supposed to be validated by the respective classes
+ # [TODO] validate the model/dataset configuration
+ # self._validate_runner_config(runner_config)
+
+ # Override the default configuration with user options.
+ self.config = OmegaConf.merge(
+ runner_config, model_config, dataset_config, user_config
+ )
+
+ def _validate_runner_config(self, runner_config):
+ """
+ This method validates the configuration, such that
+ 1) all the user specified options are valid;
+ 2) no type mismatches between the user specified options and the config.
+ """
+ runner_config_validator = create_runner_config_validator()
+ runner_config_validator.validate(runner_config)
+
+ def _build_opt_list(self, opts):
+ opts_dot_list = self._convert_to_dot_list(opts)
+ return OmegaConf.from_dotlist(opts_dot_list)
+
+ @staticmethod
+ def build_model_config(config, **kwargs):
+ model = config.get("model", None)
+ assert model is not None, "Missing model configuration file."
+
+ model_cls = registry.get_model_class(model.arch)
+ assert model_cls is not None, f"Model '{model.arch}' has not been registered."
+
+ model_type = kwargs.get("model.model_type", None)
+ if not model_type:
+ model_type = model.get("model_type", None)
+ # else use the model type selected by user.
+
+ assert model_type is not None, "Missing model_type."
+
+ model_config_path = model_cls.default_config_path(model_type=model_type)
+
+ model_config = OmegaConf.create()
+ # hierarchy override, customized config > default config
+ model_config = OmegaConf.merge(
+ model_config,
+ OmegaConf.load(model_config_path),
+ {"model": config["model"]},
+ )
+
+ return model_config
+
+ @staticmethod
+ def build_runner_config(config):
+ return {"run": config.run}
+
+ @staticmethod
+ def build_dataset_config(config):
+ datasets = config.get("datasets", None)
+ if datasets is None:
+ raise KeyError(
+ "Expecting 'datasets' as the root key for dataset configuration."
+ )
+
+ dataset_config = OmegaConf.create()
+
+ for dataset_name in datasets:
+ builder_cls = registry.get_builder_class(dataset_name)
+
+ dataset_config_type = datasets[dataset_name].get("type", "default")
+ dataset_config_path = builder_cls.default_config_path(
+ type=dataset_config_type
+ )
+
+ # hierarchy override, customized config > default config
+ dataset_config = OmegaConf.merge(
+ dataset_config,
+ OmegaConf.load(dataset_config_path),
+ {"datasets": {dataset_name: config["datasets"][dataset_name]}},
+ )
+
+ return dataset_config
+
+ def _convert_to_dot_list(self, opts):
+ if opts is None:
+ opts = []
+
+ if len(opts) == 0:
+ return opts
+
+ has_equal = opts[0].find("=") != -1
+
+ if has_equal:
+ return opts
+
+ return [(opt + "=" + value) for opt, value in zip(opts[0::2], opts[1::2])]
+
+ def get_config(self):
+ return self.config
+
+ @property
+ def run_cfg(self):
+ return self.config.run
+
+ @property
+ def datasets_cfg(self):
+ return self.config.datasets
+
+ @property
+ def model_cfg(self):
+ return self.config.model
+
+ def pretty_print(self):
+ logging.info("\n===== Running Parameters =====")
+ logging.info(self._convert_node_to_json(self.config.run))
+
+ logging.info("\n====== Dataset Attributes ======")
+ datasets = self.config.datasets
+
+ for dataset in datasets:
+ if dataset in self.config.datasets:
+ logging.info(f"\n======== {dataset} =======")
+ dataset_config = self.config.datasets[dataset]
+ logging.info(self._convert_node_to_json(dataset_config))
+ else:
+ logging.warning(f"No dataset named '{dataset}' in config. Skipping")
+
+ logging.info(f"\n====== Model Attributes ======")
+ logging.info(self._convert_node_to_json(self.config.model))
+
+ def _convert_node_to_json(self, node):
+ container = OmegaConf.to_container(node, resolve=True)
+ return json.dumps(container, indent=4, sort_keys=True)
+
+ def to_dict(self):
+ return OmegaConf.to_container(self.config)
+
+
+def node_to_dict(node):
+ return OmegaConf.to_container(node)
+
+
+class ConfigValidator:
+ """
+ This is a preliminary implementation to centralize and validate the configuration.
+ May be altered in the future.
+
+ A helper class to validate configurations from yaml file.
+
+ This serves the following purposes:
+ 1. Ensure all the options in the yaml are defined, raise error if not.
+ 2. when type mismatches are found, the validator will raise an error.
+ 3. a central place to store and display helpful messages for supported configurations.
+
+ """
+
+ class _Argument:
+ def __init__(self, name, choices=None, type=None, help=None):
+ self.name = name
+ self.val = None
+ self.choices = choices
+ self.type = type
+ self.help = help
+
+ def __str__(self):
+ s = f"{self.name}={self.val}"
+ if self.type is not None:
+ s += f", ({self.type})"
+ if self.choices is not None:
+ s += f", choices: {self.choices}"
+ if self.help is not None:
+ s += f", ({self.help})"
+ return s
+
+ def __init__(self, description):
+ self.description = description
+
+ self.arguments = dict()
+
+ self.parsed_args = None
+
+ def __getitem__(self, key):
+ assert self.parsed_args is not None, "No arguments parsed yet."
+
+ return self.parsed_args[key]
+
+ def __str__(self) -> str:
+ return self.format_help()
+
+ def add_argument(self, *args, **kwargs):
+ """
+ Assume the first argument is the name of the argument.
+ """
+ self.arguments[args[0]] = self._Argument(*args, **kwargs)
+
+ def validate(self, config=None):
+ """
+ Convert yaml config (dict-like) to list, required by argparse.
+ """
+ for k, v in config.items():
+ assert (
+ k in self.arguments
+ ), f"""{k} is not a valid argument. Support arguments are {self.format_arguments()}."""
+
+ if self.arguments[k].type is not None:
+ try:
+ self.arguments[k].val = self.arguments[k].type(v)
+ except ValueError:
+ raise ValueError(f"{k} is not a valid {self.arguments[k].type}.")
+
+ if self.arguments[k].choices is not None:
+ assert (
+ v in self.arguments[k].choices
+ ), f"""{k} must be one of {self.arguments[k].choices}."""
+
+ return config
+
+ def format_arguments(self):
+ return str([f"{k}" for k in sorted(self.arguments.keys())])
+
+ def format_help(self):
+ # description + key-value pair string for each argument
+ help_msg = str(self.description)
+ return help_msg + ", available arguments: " + self.format_arguments()
+
+ def print_help(self):
+ # display help message
+ print(self.format_help())
+
+
+def create_runner_config_validator():
+ validator = ConfigValidator(description="Runner configurations")
+
+ validator.add_argument(
+ "runner",
+ type=str,
+ choices=["runner_base", "runner_iter"],
+ help="""Runner to use. The "runner_base" uses epoch-based training while iter-based
+ runner runs based on iters. Default: runner_base""",
+ )
+ # add argumetns for training dataset ratios
+ validator.add_argument(
+ "train_dataset_ratios",
+ type=Dict[str, float],
+ help="""Ratios of training dataset. This is used in iteration-based runner.
+ Do not support for epoch-based runner because how to define an epoch becomes tricky.
+ Default: None""",
+ )
+ validator.add_argument(
+ "max_iters",
+ type=float,
+ help="Maximum number of iterations to run.",
+ )
+ validator.add_argument(
+ "max_epoch",
+ type=int,
+ help="Maximum number of epochs to run.",
+ )
+ # add arguments for iters_per_inner_epoch
+ validator.add_argument(
+ "iters_per_inner_epoch",
+ type=float,
+ help="Number of iterations per inner epoch. This is required when runner is runner_iter.",
+ )
+ lr_scheds_choices = registry.list_lr_schedulers()
+ validator.add_argument(
+ "lr_sched",
+ type=str,
+ choices=lr_scheds_choices,
+ help="Learning rate scheduler to use, from {}".format(lr_scheds_choices),
+ )
+ task_choices = registry.list_tasks()
+ validator.add_argument(
+ "task",
+ type=str,
+ choices=task_choices,
+ help="Task to use, from {}".format(task_choices),
+ )
+ # add arguments for init_lr
+ validator.add_argument(
+ "init_lr",
+ type=float,
+ help="Initial learning rate. This will be the learning rate after warmup and before decay.",
+ )
+ # add arguments for min_lr
+ validator.add_argument(
+ "min_lr",
+ type=float,
+ help="Minimum learning rate (after decay).",
+ )
+ # add arguments for warmup_lr
+ validator.add_argument(
+ "warmup_lr",
+ type=float,
+ help="Starting learning rate for warmup.",
+ )
+ # add arguments for learning rate decay rate
+ validator.add_argument(
+ "lr_decay_rate",
+ type=float,
+ help="Learning rate decay rate. Required if using a decaying learning rate scheduler.",
+ )
+ # add arguments for weight decay
+ validator.add_argument(
+ "weight_decay",
+ type=float,
+ help="Weight decay rate.",
+ )
+ # add arguments for training batch size
+ validator.add_argument(
+ "batch_size_train",
+ type=int,
+ help="Training batch size.",
+ )
+ # add arguments for evaluation batch size
+ validator.add_argument(
+ "batch_size_eval",
+ type=int,
+ help="Evaluation batch size, including validation and testing.",
+ )
+ # add arguments for number of workers for data loading
+ validator.add_argument(
+ "num_workers",
+ help="Number of workers for data loading.",
+ )
+ # add arguments for warm up steps
+ validator.add_argument(
+ "warmup_steps",
+ type=int,
+ help="Number of warmup steps. Required if a warmup schedule is used.",
+ )
+ # add arguments for random seed
+ validator.add_argument(
+ "seed",
+ type=int,
+ help="Random seed.",
+ )
+ # add arguments for output directory
+ validator.add_argument(
+ "output_dir",
+ type=str,
+ help="Output directory to save checkpoints and logs.",
+ )
+ # add arguments for whether only use evaluation
+ validator.add_argument(
+ "evaluate",
+ help="Whether to only evaluate the model. If true, training will not be performed.",
+ )
+ # add arguments for splits used for training, e.g. ["train", "val"]
+ validator.add_argument(
+ "train_splits",
+ type=list,
+ help="Splits to use for training.",
+ )
+ # add arguments for splits used for validation, e.g. ["val"]
+ validator.add_argument(
+ "valid_splits",
+ type=list,
+ help="Splits to use for validation. If not provided, will skip the validation.",
+ )
+ # add arguments for splits used for testing, e.g. ["test"]
+ validator.add_argument(
+ "test_splits",
+ type=list,
+ help="Splits to use for testing. If not provided, will skip the testing.",
+ )
+ # add arguments for accumulating gradient for iterations
+ validator.add_argument(
+ "accum_grad_iters",
+ type=int,
+ help="Number of iterations to accumulate gradient for.",
+ )
+
+ # ====== distributed training ======
+ validator.add_argument(
+ "device",
+ type=str,
+ choices=["cpu", "cuda"],
+ help="Device to use. Support 'cuda' or 'cpu' as for now.",
+ )
+ validator.add_argument(
+ "world_size",
+ type=int,
+ help="Number of processes participating in the job.",
+ )
+ validator.add_argument("dist_url", type=str)
+ validator.add_argument("distributed", type=bool)
+ # add arguments to opt using distributed sampler during evaluation or not
+ validator.add_argument(
+ "use_dist_eval_sampler",
+ type=bool,
+ help="Whether to use distributed sampler during evaluation or not.",
+ )
+
+ # ====== task specific ======
+ # generation task specific arguments
+ # add arguments for maximal length of text output
+ validator.add_argument(
+ "max_len",
+ type=int,
+ help="Maximal length of text output.",
+ )
+ # add arguments for minimal length of text output
+ validator.add_argument(
+ "min_len",
+ type=int,
+ help="Minimal length of text output.",
+ )
+ # add arguments number of beams
+ validator.add_argument(
+ "num_beams",
+ type=int,
+ help="Number of beams used for beam search.",
+ )
+
+ # vqa task specific arguments
+ # add arguments for number of answer candidates
+ validator.add_argument(
+ "num_ans_candidates",
+ type=int,
+ help="""For ALBEF and BLIP, these models first rank answers according to likelihood to select answer candidates.""",
+ )
+ # add arguments for inference method
+ validator.add_argument(
+ "inference_method",
+ type=str,
+ choices=["genearte", "rank"],
+ help="""Inference method to use for question answering. If rank, requires a answer list.""",
+ )
+
+ # ====== model specific ======
+ validator.add_argument(
+ "k_test",
+ type=int,
+ help="Number of top k most similar samples from ITC/VTC selection to be tested.",
+ )
+
+ return validator
diff --git a/global_local/common/dist_utils.py b/global_local/common/dist_utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..74e315180be1caaaaddbe48add6a46d0e2ee4c08
--- /dev/null
+++ b/global_local/common/dist_utils.py
@@ -0,0 +1,156 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import datetime
+import functools
+import os
+
+import torch
+import torch.distributed as dist
+import timm.models.hub as timm_hub
+
+
+def setup_for_distributed(is_master):
+ """
+ This function disables printing when not in master process
+ """
+ import builtins as __builtin__
+
+ builtin_print = __builtin__.print
+
+ def print(*args, **kwargs):
+ force = kwargs.pop("force", False)
+ if is_master or force:
+ builtin_print(*args, **kwargs)
+
+ __builtin__.print = print
+
+
+def is_dist_avail_and_initialized():
+ if not dist.is_available():
+ return False
+ if not dist.is_initialized():
+ return False
+ return True
+
+
+def get_world_size():
+ if not is_dist_avail_and_initialized():
+ return 1
+ return dist.get_world_size()
+
+
+def get_rank():
+ if not is_dist_avail_and_initialized():
+ return 0
+ return dist.get_rank()
+
+
+def is_main_process():
+ return get_rank() == 0
+
+
+def init_distributed_mode(args):
+ if "RANK" in os.environ and "WORLD_SIZE" in os.environ:
+ args.rank = int(os.environ["RANK"])
+ args.world_size = int(os.environ["WORLD_SIZE"])
+ args.gpu = int(os.environ["LOCAL_RANK"])
+ elif "SLURM_PROCID" in os.environ:
+ args.rank = int(os.environ["SLURM_PROCID"])
+ args.gpu = args.rank % torch.cuda.device_count()
+ else:
+ print("Not using distributed mode")
+ args.distributed = False
+ return
+
+ args.distributed = True
+
+ torch.cuda.set_device(args.gpu)
+ args.dist_backend = "nccl"
+ print(
+ "| distributed init (rank {}, world {}): {}".format(
+ args.rank, args.world_size, args.dist_url
+ ),
+ flush=True,
+ )
+ torch.distributed.init_process_group(
+ backend=args.dist_backend,
+ init_method=args.dist_url,
+ world_size=args.world_size,
+ rank=args.rank,
+ timeout=datetime.timedelta(
+ days=365
+ ), # allow auto-downloading and de-compressing
+ )
+ torch.distributed.barrier()
+ setup_for_distributed(args.rank == 0)
+
+
+def get_dist_info():
+ if torch.__version__ < "1.0":
+ initialized = dist._initialized
+ else:
+ initialized = dist.is_initialized()
+ if initialized:
+ rank = dist.get_rank()
+ world_size = dist.get_world_size()
+ else: # non-distributed training
+ rank = 0
+ world_size = 1
+ return rank, world_size
+
+def no_grad_all_gather(tensors):
+ """
+ All gathers the provided tensors from all processes across machines.
+ Args:
+ tensors (list): tensors to perform all gather across all processes in
+ all machines.
+ """
+
+ gather_list = []
+ output_tensor = []
+ world_size = dist.get_world_size()
+
+ for tensor in tensors:
+ tensor_placeholder = [torch.ones_like(tensor) for _ in range(world_size)]
+ dist.all_gather(tensor_placeholder, tensor, async_op=False)
+ gather_list.append(tensor_placeholder)
+ for gathered_tensor in gather_list:
+ output_tensor.append(torch.cat(gathered_tensor, dim=0))
+ return output_tensor
+
+def main_process(func):
+ @functools.wraps(func)
+ def wrapper(*args, **kwargs):
+ rank, _ = get_dist_info()
+ if rank == 0:
+ return func(*args, **kwargs)
+
+ return wrapper
+
+
+def download_cached_file(url, check_hash=True, progress=False):
+ """
+ Download a file from a URL and cache it locally. If the file already exists, it is not downloaded again.
+ If distributed, only the main process downloads the file, and the other processes wait for the file to be downloaded.
+ """
+
+ def get_cached_file_path():
+ # a hack to sync the file path across processes
+ parts = torch.hub.urlparse(url)
+ filename = os.path.basename(parts.path)
+ cached_file = os.path.join(timm_hub.get_cache_dir(), filename)
+
+ return cached_file
+
+ if is_main_process():
+ timm_hub.download_cached_file(url, check_hash, progress)
+
+ #if is_dist_avail_and_initialized():
+ # dist.barrier()
+
+ return get_cached_file_path()
diff --git a/global_local/common/gradcam.py b/global_local/common/gradcam.py
new file mode 100644
index 0000000000000000000000000000000000000000..d53a5254d4b319eaf2cbfbd081b0ca8e38c5c7a0
--- /dev/null
+++ b/global_local/common/gradcam.py
@@ -0,0 +1,24 @@
+import numpy as np
+from matplotlib import pyplot as plt
+from scipy.ndimage import filters
+from skimage import transform as skimage_transform
+
+
+def getAttMap(img, attMap, blur=True, overlap=True):
+ attMap -= attMap.min()
+ if attMap.max() > 0:
+ attMap /= attMap.max()
+ attMap = skimage_transform.resize(attMap, (img.shape[:2]), order=3, mode="constant")
+ if blur:
+ attMap = filters.gaussian_filter(attMap, 0.02 * max(img.shape[:2]))
+ attMap -= attMap.min()
+ attMap /= attMap.max()
+ cmap = plt.get_cmap("jet")
+ attMapV = cmap(attMap)
+ attMapV = np.delete(attMapV, 3, 2)
+ if overlap:
+ attMap = (
+ 1 * (1 - attMap**0.7).reshape(attMap.shape + (1,)) * img
+ + (attMap**0.7).reshape(attMap.shape + (1,)) * attMapV
+ )
+ return attMap
diff --git a/global_local/common/logger.py b/global_local/common/logger.py
new file mode 100644
index 0000000000000000000000000000000000000000..5c98243eaa27ed3318a19b162e485642e4c61057
--- /dev/null
+++ b/global_local/common/logger.py
@@ -0,0 +1,195 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import datetime
+import logging
+import time
+from collections import defaultdict, deque
+
+import torch
+import torch.distributed as dist
+
+from global_local.common import dist_utils
+
+
+class SmoothedValue(object):
+ """Track a series of values and provide access to smoothed values over a
+ window or the global series average.
+ """
+
+ def __init__(self, window_size=20, fmt=None):
+ if fmt is None:
+ fmt = "{median:.4f} ({global_avg:.4f})"
+ self.deque = deque(maxlen=window_size)
+ self.total = 0.0
+ self.count = 0
+ self.fmt = fmt
+
+ def update(self, value, n=1):
+ self.deque.append(value)
+ self.count += n
+ self.total += value * n
+
+ def synchronize_between_processes(self):
+ """
+ Warning: does not synchronize the deque!
+ """
+ if not dist_utils.is_dist_avail_and_initialized():
+ return
+ t = torch.tensor([self.count, self.total], dtype=torch.float64, device="cuda")
+ dist.barrier()
+ dist.all_reduce(t)
+ t = t.tolist()
+ self.count = int(t[0])
+ self.total = t[1]
+
+ @property
+ def median(self):
+ d = torch.tensor(list(self.deque))
+ return d.median().item()
+
+ @property
+ def avg(self):
+ d = torch.tensor(list(self.deque), dtype=torch.float32)
+ return d.mean().item()
+
+ @property
+ def global_avg(self):
+ return self.total / self.count
+
+ @property
+ def max(self):
+ return max(self.deque)
+
+ @property
+ def value(self):
+ return self.deque[-1]
+
+ def __str__(self):
+ return self.fmt.format(
+ median=self.median,
+ avg=self.avg,
+ global_avg=self.global_avg,
+ max=self.max,
+ value=self.value,
+ )
+
+
+class MetricLogger(object):
+ def __init__(self, delimiter="\t"):
+ self.meters = defaultdict(SmoothedValue)
+ self.delimiter = delimiter
+
+ def update(self, **kwargs):
+ for k, v in kwargs.items():
+ if isinstance(v, torch.Tensor):
+ v = v.item()
+ assert isinstance(v, (float, int))
+ self.meters[k].update(v)
+
+ def __getattr__(self, attr):
+ if attr in self.meters:
+ return self.meters[attr]
+ if attr in self.__dict__:
+ return self.__dict__[attr]
+ raise AttributeError(
+ "'{}' object has no attribute '{}'".format(type(self).__name__, attr)
+ )
+
+ def __str__(self):
+ loss_str = []
+ for name, meter in self.meters.items():
+ loss_str.append("{}: {}".format(name, str(meter)))
+ return self.delimiter.join(loss_str)
+
+ def global_avg(self):
+ loss_str = []
+ for name, meter in self.meters.items():
+ loss_str.append("{}: {:.4f}".format(name, meter.global_avg))
+ return self.delimiter.join(loss_str)
+
+ def synchronize_between_processes(self):
+ for meter in self.meters.values():
+ meter.synchronize_between_processes()
+
+ def add_meter(self, name, meter):
+ self.meters[name] = meter
+
+ def log_every(self, iterable, print_freq, header=None):
+ i = 0
+ if not header:
+ header = ""
+ start_time = time.time()
+ end = time.time()
+ iter_time = SmoothedValue(fmt="{avg:.4f}")
+ data_time = SmoothedValue(fmt="{avg:.4f}")
+ space_fmt = ":" + str(len(str(len(iterable)))) + "d"
+ log_msg = [
+ header,
+ "[{0" + space_fmt + "}/{1}]",
+ "eta: {eta}",
+ "{meters}",
+ "time: {time}",
+ "data: {data}",
+ ]
+ if torch.cuda.is_available():
+ log_msg.append("max mem: {memory:.0f}")
+ log_msg = self.delimiter.join(log_msg)
+ MB = 1024.0 * 1024.0
+ for obj in iterable:
+ data_time.update(time.time() - end)
+ yield obj
+ iter_time.update(time.time() - end)
+ if i % print_freq == 0 or i == len(iterable) - 1:
+ eta_seconds = iter_time.global_avg * (len(iterable) - i)
+ eta_string = str(datetime.timedelta(seconds=int(eta_seconds)))
+ if torch.cuda.is_available():
+ print(
+ log_msg.format(
+ i,
+ len(iterable),
+ eta=eta_string,
+ meters=str(self),
+ time=str(iter_time),
+ data=str(data_time),
+ memory=torch.cuda.max_memory_allocated() / MB,
+ )
+ )
+ else:
+ print(
+ log_msg.format(
+ i,
+ len(iterable),
+ eta=eta_string,
+ meters=str(self),
+ time=str(iter_time),
+ data=str(data_time),
+ )
+ )
+ i += 1
+ end = time.time()
+ total_time = time.time() - start_time
+ total_time_str = str(datetime.timedelta(seconds=int(total_time)))
+ print(
+ "{} Total time: {} ({:.4f} s / it)".format(
+ header, total_time_str, total_time / len(iterable)
+ )
+ )
+
+
+class AttrDict(dict):
+ def __init__(self, *args, **kwargs):
+ super(AttrDict, self).__init__(*args, **kwargs)
+ self.__dict__ = self
+
+
+def setup_logger():
+ logging.basicConfig(
+ level=logging.INFO if dist_utils.is_main_process() else logging.WARN,
+ format="%(asctime)s [%(levelname)s] %(message)s",
+ handlers=[logging.StreamHandler()],
+ )
diff --git a/global_local/common/optims.py b/global_local/common/optims.py
new file mode 100644
index 0000000000000000000000000000000000000000..d5e1f5ad02618069c42e8987f0d473e068a5dbd7
--- /dev/null
+++ b/global_local/common/optims.py
@@ -0,0 +1,134 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import math
+
+from global_local.common.registry import registry
+from torch.optim.lr_scheduler import LambdaLR
+
+
+@registry.register_lr_scheduler("linear_warmup_step_lr")
+class LinearWarmupStepLRScheduler:
+ def __init__(
+ self,
+ optimizer,
+ max_epoch,
+ min_lr,
+ init_lr,
+ decay_rate=1,
+ warmup_start_lr=-1,
+ warmup_steps=0,
+ **kwargs
+ ):
+ self.optimizer = optimizer
+
+ self.max_epoch = max_epoch
+ self.min_lr = min_lr
+
+ self.decay_rate = decay_rate
+
+ self.init_lr = init_lr
+ self.warmup_steps = warmup_steps
+ self.warmup_start_lr = warmup_start_lr if warmup_start_lr >= 0 else init_lr
+
+ def step(self, cur_epoch, cur_step):
+ if cur_epoch == 0:
+ warmup_lr_schedule(
+ step=cur_step,
+ optimizer=self.optimizer,
+ max_step=self.warmup_steps,
+ init_lr=self.warmup_start_lr,
+ max_lr=self.init_lr,
+ )
+ else:
+ step_lr_schedule(
+ epoch=cur_epoch,
+ optimizer=self.optimizer,
+ init_lr=self.init_lr,
+ min_lr=self.min_lr,
+ decay_rate=self.decay_rate,
+ )
+
+
+@registry.register_lr_scheduler("linear_warmup_cosine_lr")
+class LinearWarmupCosineLRScheduler:
+ def __init__(
+ self,
+ optimizer,
+ max_epoch,
+ iters_per_epoch,
+ min_lr,
+ init_lr,
+ warmup_steps=0,
+ warmup_start_lr=-1,
+ **kwargs
+ ):
+ self.optimizer = optimizer
+
+ self.max_epoch = max_epoch
+ self.iters_per_epoch = iters_per_epoch
+ self.min_lr = min_lr
+
+ self.init_lr = init_lr
+ self.warmup_steps = warmup_steps
+ self.warmup_start_lr = warmup_start_lr if warmup_start_lr >= 0 else init_lr
+
+ def step(self, cur_epoch, cur_step):
+ total_cur_step = cur_epoch * self.iters_per_epoch + cur_step
+ if total_cur_step < self.warmup_steps:
+ warmup_lr_schedule(
+ step=cur_step,
+ optimizer=self.optimizer,
+ max_step=self.warmup_steps,
+ init_lr=self.warmup_start_lr,
+ max_lr=self.init_lr,
+ )
+ else:
+ cosine_lr_schedule(
+ epoch=total_cur_step,
+ optimizer=self.optimizer,
+ max_epoch=self.max_epoch * self.iters_per_epoch,
+ init_lr=self.init_lr,
+ min_lr=self.min_lr,
+ )
+
+
+def cosine_lr_schedule(optimizer, epoch, max_epoch, init_lr, min_lr):
+ """Decay the learning rate"""
+ lr = (init_lr - min_lr) * 0.5 * (
+ 1.0 + math.cos(math.pi * epoch / max_epoch)
+ ) + min_lr
+ for param_group in optimizer.param_groups:
+ param_group["lr"] = lr
+
+
+def warmup_lr_schedule(optimizer, step, max_step, init_lr, max_lr):
+ """Warmup the learning rate"""
+ lr = min(max_lr, init_lr + (max_lr - init_lr) * step / max(max_step, 1))
+ for param_group in optimizer.param_groups:
+ param_group["lr"] = lr
+
+
+def step_lr_schedule(optimizer, epoch, init_lr, min_lr, decay_rate):
+ """Decay the learning rate"""
+ lr = max(min_lr, init_lr * (decay_rate**epoch))
+ for param_group in optimizer.param_groups:
+ param_group["lr"] = lr
+
+def get_cosine_schedule_with_warmup(optimizer, num_warmup_steps, num_training_steps, num_cycles=0.5, last_epoch=-1):
+ """ Create a schedule with a learning rate that decreases following the
+ values of the cosine function between 0 and `pi * cycles` after a warmup
+ period during which it increases linearly between 0 and 1.
+ """
+
+ def lr_lambda(current_step):
+ if current_step < num_warmup_steps:
+ return float(current_step) / float(max(1, num_warmup_steps))
+ progress = float(current_step - num_warmup_steps) / float(max(1, num_training_steps - num_warmup_steps))
+ return max(0.0, 0.5 * (1.0 + math.cos(math.pi * float(num_cycles) * 2.0 * progress)))
+
+ return LambdaLR(optimizer, lr_lambda, last_epoch)
diff --git a/global_local/common/registry.py b/global_local/common/registry.py
new file mode 100644
index 0000000000000000000000000000000000000000..5c3fde8d691663a2cf16e001332ea20f380884ac
--- /dev/null
+++ b/global_local/common/registry.py
@@ -0,0 +1,329 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+
+class Registry:
+ mapping = {
+ "builder_name_mapping": {},
+ "task_name_mapping": {},
+ "processor_name_mapping": {},
+ "model_name_mapping": {},
+ "lr_scheduler_name_mapping": {},
+ "runner_name_mapping": {},
+ "state": {},
+ "paths": {},
+ }
+
+ @classmethod
+ def register_builder(cls, name):
+ r"""Register a dataset builder to registry with key 'name'
+
+ Args:
+ name: Key with which the builder will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ from video_llama.datasets.base_dataset_builder import BaseDatasetBuilder
+ """
+
+ def wrap(builder_cls):
+ from global_local.datasets.builders.base_dataset_builder import BaseDatasetBuilder
+
+ assert issubclass(
+ builder_cls, BaseDatasetBuilder
+ ), "All builders must inherit BaseDatasetBuilder class, found {}".format(
+ builder_cls
+ )
+ if name in cls.mapping["builder_name_mapping"]:
+ raise KeyError(
+ "Name '{}' already registered for {}.".format(
+ name, cls.mapping["builder_name_mapping"][name]
+ )
+ )
+ cls.mapping["builder_name_mapping"][name] = builder_cls
+ return builder_cls
+
+ return wrap
+
+ @classmethod
+ def register_task(cls, name):
+ r"""Register a task to registry with key 'name'
+
+ Args:
+ name: Key with which the task will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ """
+
+ def wrap(task_cls):
+ from global_local.tasks.base_task import BaseTask
+
+ assert issubclass(
+ task_cls, BaseTask
+ ), "All tasks must inherit BaseTask class"
+ if name in cls.mapping["task_name_mapping"]:
+ raise KeyError(
+ "Name '{}' already registered for {}.".format(
+ name, cls.mapping["task_name_mapping"][name]
+ )
+ )
+ cls.mapping["task_name_mapping"][name] = task_cls
+ return task_cls
+
+ return wrap
+
+ @classmethod
+ def register_model(cls, name):
+ r"""Register a task to registry with key 'name'
+
+ Args:
+ name: Key with which the task will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ """
+
+ def wrap(model_cls):
+ from global_local.models import BaseModel
+
+ assert issubclass(
+ model_cls, BaseModel
+ ), "All models must inherit BaseModel class"
+ if name in cls.mapping["model_name_mapping"]:
+ raise KeyError(
+ "Name '{}' already registered for {}.".format(
+ name, cls.mapping["model_name_mapping"][name]
+ )
+ )
+ cls.mapping["model_name_mapping"][name] = model_cls
+ return model_cls
+
+ return wrap
+
+ @classmethod
+ def register_processor(cls, name):
+ r"""Register a processor to registry with key 'name'
+
+ Args:
+ name: Key with which the task will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ """
+
+ def wrap(processor_cls):
+ from global_local.processors import BaseProcessor
+
+ assert issubclass(
+ processor_cls, BaseProcessor
+ ), "All processors must inherit BaseProcessor class"
+ if name in cls.mapping["processor_name_mapping"]:
+ raise KeyError(
+ "Name '{}' already registered for {}.".format(
+ name, cls.mapping["processor_name_mapping"][name]
+ )
+ )
+ cls.mapping["processor_name_mapping"][name] = processor_cls
+ return processor_cls
+
+ return wrap
+
+ @classmethod
+ def register_lr_scheduler(cls, name):
+ r"""Register a model to registry with key 'name'
+
+ Args:
+ name: Key with which the task will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ """
+
+ def wrap(lr_sched_cls):
+ if name in cls.mapping["lr_scheduler_name_mapping"]:
+ raise KeyError(
+ "Name '{}' already registered for {}.".format(
+ name, cls.mapping["lr_scheduler_name_mapping"][name]
+ )
+ )
+ cls.mapping["lr_scheduler_name_mapping"][name] = lr_sched_cls
+ return lr_sched_cls
+
+ return wrap
+
+ @classmethod
+ def register_runner(cls, name):
+ r"""Register a model to registry with key 'name'
+
+ Args:
+ name: Key with which the task will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ """
+
+ def wrap(runner_cls):
+ if name in cls.mapping["runner_name_mapping"]:
+ raise KeyError(
+ "Name '{}' already registered for {}.".format(
+ name, cls.mapping["runner_name_mapping"][name]
+ )
+ )
+ cls.mapping["runner_name_mapping"][name] = runner_cls
+ return runner_cls
+
+ return wrap
+
+ @classmethod
+ def register_path(cls, name, path):
+ r"""Register a path to registry with key 'name'
+
+ Args:
+ name: Key with which the path will be registered.
+
+ Usage:
+
+ from video_llama.common.registry import registry
+ """
+ assert isinstance(path, str), "All path must be str."
+ if name in cls.mapping["paths"]:
+ raise KeyError("Name '{}' already registered.".format(name))
+ cls.mapping["paths"][name] = path
+
+ @classmethod
+ def register(cls, name, obj):
+ r"""Register an item to registry with key 'name'
+
+ Args:
+ name: Key with which the item will be registered.
+
+ Usage::
+
+ from video_llama.common.registry import registry
+
+ registry.register("config", {})
+ """
+ path = name.split(".")
+ current = cls.mapping["state"]
+
+ for part in path[:-1]:
+ if part not in current:
+ current[part] = {}
+ current = current[part]
+
+ current[path[-1]] = obj
+
+ # @classmethod
+ # def get_trainer_class(cls, name):
+ # return cls.mapping["trainer_name_mapping"].get(name, None)
+
+ @classmethod
+ def get_builder_class(cls, name):
+ return cls.mapping["builder_name_mapping"].get(name, None)
+
+ @classmethod
+ def get_model_class(cls, name):
+ return cls.mapping["model_name_mapping"].get(name, None)
+
+ @classmethod
+ def get_task_class(cls, name):
+ return cls.mapping["task_name_mapping"].get(name, None)
+
+ @classmethod
+ def get_processor_class(cls, name):
+ return cls.mapping["processor_name_mapping"].get(name, None)
+
+ @classmethod
+ def get_lr_scheduler_class(cls, name):
+ return cls.mapping["lr_scheduler_name_mapping"].get(name, None)
+
+ @classmethod
+ def get_runner_class(cls, name):
+ return cls.mapping["runner_name_mapping"].get(name, None)
+
+ @classmethod
+ def list_runners(cls):
+ return sorted(cls.mapping["runner_name_mapping"].keys())
+
+ @classmethod
+ def list_models(cls):
+ return sorted(cls.mapping["model_name_mapping"].keys())
+
+ @classmethod
+ def list_tasks(cls):
+ return sorted(cls.mapping["task_name_mapping"].keys())
+
+ @classmethod
+ def list_processors(cls):
+ return sorted(cls.mapping["processor_name_mapping"].keys())
+
+ @classmethod
+ def list_lr_schedulers(cls):
+ return sorted(cls.mapping["lr_scheduler_name_mapping"].keys())
+
+ @classmethod
+ def list_datasets(cls):
+ return sorted(cls.mapping["builder_name_mapping"].keys())
+
+ @classmethod
+ def get_path(cls, name):
+ return cls.mapping["paths"].get(name, None)
+
+ @classmethod
+ def get(cls, name, default=None, no_warning=False):
+ r"""Get an item from registry with key 'name'
+
+ Args:
+ name (string): Key whose value needs to be retrieved.
+ default: If passed and key is not in registry, default value will
+ be returned with a warning. Default: None
+ no_warning (bool): If passed as True, warning when key doesn't exist
+ will not be generated. Useful for MMF's
+ internal operations. Default: False
+ """
+ original_name = name
+ name = name.split(".")
+ value = cls.mapping["state"]
+ for subname in name:
+ value = value.get(subname, default)
+ if value is default:
+ break
+
+ if (
+ "writer" in cls.mapping["state"]
+ and value == default
+ and no_warning is False
+ ):
+ cls.mapping["state"]["writer"].warning(
+ "Key {} is not present in registry, returning default value "
+ "of {}".format(original_name, default)
+ )
+ return value
+
+ @classmethod
+ def unregister(cls, name):
+ r"""Remove an item from registry with key 'name'
+
+ Args:
+ name: Key which needs to be removed.
+ Usage::
+
+ from mmf.common.registry import registry
+
+ config = registry.unregister("config")
+ """
+ return cls.mapping["state"].pop(name, None)
+
+
+registry = Registry()
diff --git a/global_local/common/utils.py b/global_local/common/utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..ab78ab89ec697a69bec9a1ecbed92d0d4b701b7b
--- /dev/null
+++ b/global_local/common/utils.py
@@ -0,0 +1,424 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import io
+import json
+import logging
+import os
+import pickle
+import re
+import shutil
+import urllib
+import urllib.error
+import urllib.request
+from typing import Optional
+from urllib.parse import urlparse
+
+import numpy as np
+import pandas as pd
+import yaml
+from iopath.common.download import download
+from iopath.common.file_io import file_lock, g_pathmgr
+from global_local.common.registry import registry
+from torch.utils.model_zoo import tqdm
+from torchvision.datasets.utils import (
+ check_integrity,
+ download_file_from_google_drive,
+ extract_archive,
+)
+
+
+def now():
+ from datetime import datetime
+
+ return datetime.now().strftime("%Y%m%d%H%M")[:-1]
+
+
+def is_url(url_or_filename):
+ parsed = urlparse(url_or_filename)
+ return parsed.scheme in ("http", "https")
+
+
+def get_cache_path(rel_path):
+ return os.path.expanduser(os.path.join(registry.get_path("cache_root"), rel_path))
+
+
+def get_abs_path(rel_path):
+ return os.path.join(registry.get_path("library_root"), rel_path)
+
+
+def load_json(filename):
+ with open(filename, "r") as f:
+ return json.load(f)
+
+
+# The following are adapted from torchvision and vissl
+# torchvision: https://github.com/pytorch/vision
+# vissl: https://github.com/facebookresearch/vissl/blob/main/vissl/utils/download.py
+
+
+def makedir(dir_path):
+ """
+ Create the directory if it does not exist.
+ """
+ is_success = False
+ try:
+ if not g_pathmgr.exists(dir_path):
+ g_pathmgr.mkdirs(dir_path)
+ is_success = True
+ except BaseException:
+ print(f"Error creating directory: {dir_path}")
+ return is_success
+
+
+def get_redirected_url(url: str):
+ """
+ Given a URL, returns the URL it redirects to or the
+ original URL in case of no indirection
+ """
+ import requests
+
+ with requests.Session() as session:
+ with session.get(url, stream=True, allow_redirects=True) as response:
+ if response.history:
+ return response.url
+ else:
+ return url
+
+
+def to_google_drive_download_url(view_url: str) -> str:
+ """
+ Utility function to transform a view URL of google drive
+ to a download URL for google drive
+ Example input:
+ https://drive.google.com/file/d/137RyRjvTBkBiIfeYBNZBtViDHQ6_Ewsp/view
+ Example output:
+ https://drive.google.com/uc?export=download&id=137RyRjvTBkBiIfeYBNZBtViDHQ6_Ewsp
+ """
+ splits = view_url.split("/")
+ assert splits[-1] == "view"
+ file_id = splits[-2]
+ return f"https://drive.google.com/uc?export=download&id={file_id}"
+
+
+def download_google_drive_url(url: str, output_path: str, output_file_name: str):
+ """
+ Download a file from google drive
+ Downloading an URL from google drive requires confirmation when
+ the file of the size is too big (google drive notifies that
+ anti-viral checks cannot be performed on such files)
+ """
+ import requests
+
+ with requests.Session() as session:
+
+ # First get the confirmation token and append it to the URL
+ with session.get(url, stream=True, allow_redirects=True) as response:
+ for k, v in response.cookies.items():
+ if k.startswith("download_warning"):
+ url = url + "&confirm=" + v
+
+ # Then download the content of the file
+ with session.get(url, stream=True, verify=True) as response:
+ makedir(output_path)
+ path = os.path.join(output_path, output_file_name)
+ total_size = int(response.headers.get("Content-length", 0))
+ with open(path, "wb") as file:
+ from tqdm import tqdm
+
+ with tqdm(total=total_size) as progress_bar:
+ for block in response.iter_content(
+ chunk_size=io.DEFAULT_BUFFER_SIZE
+ ):
+ file.write(block)
+ progress_bar.update(len(block))
+
+
+def _get_google_drive_file_id(url: str) -> Optional[str]:
+ parts = urlparse(url)
+
+ if re.match(r"(drive|docs)[.]google[.]com", parts.netloc) is None:
+ return None
+
+ match = re.match(r"/file/d/(?P[^/]*)", parts.path)
+ if match is None:
+ return None
+
+ return match.group("id")
+
+
+def _urlretrieve(url: str, filename: str, chunk_size: int = 1024) -> None:
+ with open(filename, "wb") as fh:
+ with urllib.request.urlopen(
+ urllib.request.Request(url, headers={"User-Agent": "vissl"})
+ ) as response:
+ with tqdm(total=response.length) as pbar:
+ for chunk in iter(lambda: response.read(chunk_size), ""):
+ if not chunk:
+ break
+ pbar.update(chunk_size)
+ fh.write(chunk)
+
+
+def download_url(
+ url: str,
+ root: str,
+ filename: Optional[str] = None,
+ md5: Optional[str] = None,
+) -> None:
+ """Download a file from a url and place it in root.
+ Args:
+ url (str): URL to download file from
+ root (str): Directory to place downloaded file in
+ filename (str, optional): Name to save the file under.
+ If None, use the basename of the URL.
+ md5 (str, optional): MD5 checksum of the download. If None, do not check
+ """
+ root = os.path.expanduser(root)
+ if not filename:
+ filename = os.path.basename(url)
+ fpath = os.path.join(root, filename)
+
+ makedir(root)
+
+ # check if file is already present locally
+ if check_integrity(fpath, md5):
+ print("Using downloaded and verified file: " + fpath)
+ return
+
+ # expand redirect chain if needed
+ url = get_redirected_url(url)
+
+ # check if file is located on Google Drive
+ file_id = _get_google_drive_file_id(url)
+ if file_id is not None:
+ return download_file_from_google_drive(file_id, root, filename, md5)
+
+ # download the file
+ try:
+ print("Downloading " + url + " to " + fpath)
+ _urlretrieve(url, fpath)
+ except (urllib.error.URLError, IOError) as e: # type: ignore[attr-defined]
+ if url[:5] == "https":
+ url = url.replace("https:", "http:")
+ print(
+ "Failed download. Trying https -> http instead."
+ " Downloading " + url + " to " + fpath
+ )
+ _urlretrieve(url, fpath)
+ else:
+ raise e
+
+ # check integrity of downloaded file
+ if not check_integrity(fpath, md5):
+ raise RuntimeError("File not found or corrupted.")
+
+
+def download_and_extract_archive(
+ url: str,
+ download_root: str,
+ extract_root: Optional[str] = None,
+ filename: Optional[str] = None,
+ md5: Optional[str] = None,
+ remove_finished: bool = False,
+) -> None:
+ download_root = os.path.expanduser(download_root)
+ if extract_root is None:
+ extract_root = download_root
+ if not filename:
+ filename = os.path.basename(url)
+
+ download_url(url, download_root, filename, md5)
+
+ archive = os.path.join(download_root, filename)
+ print("Extracting {} to {}".format(archive, extract_root))
+ extract_archive(archive, extract_root, remove_finished)
+
+
+def cache_url(url: str, cache_dir: str) -> str:
+ """
+ This implementation downloads the remote resource and caches it locally.
+ The resource will only be downloaded if not previously requested.
+ """
+ parsed_url = urlparse(url)
+ dirname = os.path.join(cache_dir, os.path.dirname(parsed_url.path.lstrip("/")))
+ makedir(dirname)
+ filename = url.split("/")[-1]
+ cached = os.path.join(dirname, filename)
+ with file_lock(cached):
+ if not os.path.isfile(cached):
+ logging.info(f"Downloading {url} to {cached} ...")
+ cached = download(url, dirname, filename=filename)
+ logging.info(f"URL {url} cached in {cached}")
+ return cached
+
+
+# TODO (prigoyal): convert this into RAII-style API
+def create_file_symlink(file1, file2):
+ """
+ Simply create the symlinks for a given file1 to file2.
+ Useful during model checkpointing to symlinks to the
+ latest successful checkpoint.
+ """
+ try:
+ if g_pathmgr.exists(file2):
+ g_pathmgr.rm(file2)
+ g_pathmgr.symlink(file1, file2)
+ except Exception as e:
+ logging.info(f"Could NOT create symlink. Error: {e}")
+
+
+def save_file(data, filename, append_to_json=True, verbose=True):
+ """
+ Common i/o utility to handle saving data to various file formats.
+ Supported:
+ .pkl, .pickle, .npy, .json
+ Specifically for .json, users have the option to either append (default)
+ or rewrite by passing in Boolean value to append_to_json.
+ """
+ if verbose:
+ logging.info(f"Saving data to file: {filename}")
+ file_ext = os.path.splitext(filename)[1]
+ if file_ext in [".pkl", ".pickle"]:
+ with g_pathmgr.open(filename, "wb") as fopen:
+ pickle.dump(data, fopen, pickle.HIGHEST_PROTOCOL)
+ elif file_ext == ".npy":
+ with g_pathmgr.open(filename, "wb") as fopen:
+ np.save(fopen, data)
+ elif file_ext == ".json":
+ if append_to_json:
+ with g_pathmgr.open(filename, "a") as fopen:
+ fopen.write(json.dumps(data, sort_keys=True) + "\n")
+ fopen.flush()
+ else:
+ with g_pathmgr.open(filename, "w") as fopen:
+ fopen.write(json.dumps(data, sort_keys=True) + "\n")
+ fopen.flush()
+ elif file_ext == ".yaml":
+ with g_pathmgr.open(filename, "w") as fopen:
+ dump = yaml.dump(data)
+ fopen.write(dump)
+ fopen.flush()
+ else:
+ raise Exception(f"Saving {file_ext} is not supported yet")
+
+ if verbose:
+ logging.info(f"Saved data to file: {filename}")
+
+
+def load_file(filename, mmap_mode=None, verbose=True, allow_pickle=False):
+ """
+ Common i/o utility to handle loading data from various file formats.
+ Supported:
+ .pkl, .pickle, .npy, .json
+ For the npy files, we support reading the files in mmap_mode.
+ If the mmap_mode of reading is not successful, we load data without the
+ mmap_mode.
+ """
+ if verbose:
+ logging.info(f"Loading data from file: {filename}")
+
+ file_ext = os.path.splitext(filename)[1]
+ if file_ext == ".txt":
+ with g_pathmgr.open(filename, "r") as fopen:
+ data = fopen.readlines()
+ elif file_ext in [".pkl", ".pickle"]:
+ with g_pathmgr.open(filename, "rb") as fopen:
+ data = pickle.load(fopen, encoding="latin1")
+ elif file_ext == ".npy":
+ if mmap_mode:
+ try:
+ with g_pathmgr.open(filename, "rb") as fopen:
+ data = np.load(
+ fopen,
+ allow_pickle=allow_pickle,
+ encoding="latin1",
+ mmap_mode=mmap_mode,
+ )
+ except ValueError as e:
+ logging.info(
+ f"Could not mmap {filename}: {e}. Trying without g_pathmgr"
+ )
+ data = np.load(
+ filename,
+ allow_pickle=allow_pickle,
+ encoding="latin1",
+ mmap_mode=mmap_mode,
+ )
+ logging.info("Successfully loaded without g_pathmgr")
+ except Exception:
+ logging.info("Could not mmap without g_pathmgr. Trying without mmap")
+ with g_pathmgr.open(filename, "rb") as fopen:
+ data = np.load(fopen, allow_pickle=allow_pickle, encoding="latin1")
+ else:
+ with g_pathmgr.open(filename, "rb") as fopen:
+ data = np.load(fopen, allow_pickle=allow_pickle, encoding="latin1")
+ elif file_ext == ".json":
+ with g_pathmgr.open(filename, "r") as fopen:
+ data = json.load(fopen)
+ elif file_ext == ".yaml":
+ with g_pathmgr.open(filename, "r") as fopen:
+ data = yaml.load(fopen, Loader=yaml.FullLoader)
+ elif file_ext == ".csv":
+ with g_pathmgr.open(filename, "r") as fopen:
+ data = pd.read_csv(fopen)
+ else:
+ raise Exception(f"Reading from {file_ext} is not supported yet")
+ return data
+
+
+def abspath(resource_path: str):
+ """
+ Make a path absolute, but take into account prefixes like
+ "http://" or "manifold://"
+ """
+ regex = re.compile(r"^\w+://")
+ if regex.match(resource_path) is None:
+ return os.path.abspath(resource_path)
+ else:
+ return resource_path
+
+
+def makedir(dir_path):
+ """
+ Create the directory if it does not exist.
+ """
+ is_success = False
+ try:
+ if not g_pathmgr.exists(dir_path):
+ g_pathmgr.mkdirs(dir_path)
+ is_success = True
+ except BaseException:
+ logging.info(f"Error creating directory: {dir_path}")
+ return is_success
+
+
+def is_url(input_url):
+ """
+ Check if an input string is a url. look for http(s):// and ignoring the case
+ """
+ is_url = re.match(r"^(?:http)s?://", input_url, re.IGNORECASE) is not None
+ return is_url
+
+
+def cleanup_dir(dir):
+ """
+ Utility for deleting a directory. Useful for cleaning the storage space
+ that contains various training artifacts like checkpoints, data etc.
+ """
+ if os.path.exists(dir):
+ logging.info(f"Deleting directory: {dir}")
+ shutil.rmtree(dir)
+ logging.info(f"Deleted contents of directory: {dir}")
+
+
+def get_file_size(filename):
+ """
+ Given a file, get the size of file in MB
+ """
+ size_in_mb = os.path.getsize(filename) / float(1024**2)
+ return size_in_mb
diff --git a/global_local/configs/datasets/cc_sbu/align.yaml b/global_local/configs/datasets/cc_sbu/align.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..180ea8ff0a3548219165e8864d4a59a951206298
--- /dev/null
+++ b/global_local/configs/datasets/cc_sbu/align.yaml
@@ -0,0 +1,5 @@
+datasets:
+ cc_sbu_align:
+ data_type: images
+ build_info:
+ storage: /path/to/cc_sbu_align_dataset
diff --git a/global_local/configs/datasets/cc_sbu/defaults.yaml b/global_local/configs/datasets/cc_sbu/defaults.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..359de601d2511acd6cac34e7ee1c20a1dfe9ae04
--- /dev/null
+++ b/global_local/configs/datasets/cc_sbu/defaults.yaml
@@ -0,0 +1,5 @@
+datasets:
+ cc_sbu:
+ data_type: images
+ build_info:
+ storage: /path/to/cc_sbu_dataset/{00000..00001}.tar
diff --git a/global_local/configs/datasets/instruct/llava_instruct.yaml b/global_local/configs/datasets/instruct/llava_instruct.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..0ec4a938e299f98f6d84104c909da210385003af
--- /dev/null
+++ b/global_local/configs/datasets/instruct/llava_instruct.yaml
@@ -0,0 +1,6 @@
+datasets:
+ llava_instruct:
+ data_type: image
+ build_info:
+ anno_dir: /path/llava_instruct_150k.json
+ videos_dir: /path/train2014/train2014/
diff --git a/global_local/configs/datasets/instruct/webvid_instruct.yaml b/global_local/configs/datasets/instruct/webvid_instruct.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..9619106ad8cb1000c3c40fa48672fb9247988d74
--- /dev/null
+++ b/global_local/configs/datasets/instruct/webvid_instruct.yaml
@@ -0,0 +1,6 @@
+datasets:
+ webvid_instruct:
+ data_type: image
+ build_info:
+ anno_dir: /path/webvid_align/videochat_instruct_11k.json
+ videos_dir: /path/webvid_align/videos/
diff --git a/global_local/configs/datasets/laion/defaults.yaml b/global_local/configs/datasets/laion/defaults.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..7dfff3ba891d96a136510f34b1dcf1b774705f4a
--- /dev/null
+++ b/global_local/configs/datasets/laion/defaults.yaml
@@ -0,0 +1,5 @@
+datasets:
+ laion:
+ data_type: images
+ build_info:
+ storage: path/laion/laion_dataset/{00000..00001}.tar
diff --git a/global_local/configs/datasets/webvid/defaults.yaml b/global_local/configs/datasets/webvid/defaults.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..046ae32dde61e2d79d1f519e1c8c653d8e2b5886
--- /dev/null
+++ b/global_local/configs/datasets/webvid/defaults.yaml
@@ -0,0 +1,6 @@
+datasets:
+ webvid:
+ data_type: video
+ build_info:
+ anno_dir: path/webvid/webvid_tain_data/annotations/
+ videos_dir: path//webvid/webvid_tain_data/videos/
diff --git a/global_local/configs/default.yaml b/global_local/configs/default.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..ff5a6a23fa2e3914938631b96c71fdf723dbbc10
--- /dev/null
+++ b/global_local/configs/default.yaml
@@ -0,0 +1,5 @@
+env:
+ # For default users
+ # cache_root: "cache"
+ # For internal use with persistent storage
+ cache_root: "/export/home/.cache/minigpt4"
diff --git a/global_local/configs/models/minigpt4.yaml b/global_local/configs/models/minigpt4.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..358c3f5f7b53251c607ea490ee262a890e531dc6
--- /dev/null
+++ b/global_local/configs/models/minigpt4.yaml
@@ -0,0 +1,33 @@
+model:
+ arch: mini_gpt4
+
+ # vit encoder
+ image_size: 224
+ drop_path_rate: 0
+ use_grad_checkpoint: False
+ vit_precision: "fp16"
+ freeze_vit: True
+ freeze_qformer: True
+
+ # Q-Former
+ num_query_token: 32
+
+ # Vicuna
+ llama_model: "ckpt/vicuna-13b/"
+
+ # generation configs
+ prompt: ""
+
+preprocess:
+ vis_processor:
+ train:
+ name: "blip2_image_train"
+ image_size: 224
+ eval:
+ name: "blip2_image_eval"
+ image_size: 224
+ text_processor:
+ train:
+ name: "blip_caption"
+ eval:
+ name: "blip_caption"
diff --git a/global_local/configs/models/video_llama.yaml b/global_local/configs/models/video_llama.yaml
new file mode 100644
index 0000000000000000000000000000000000000000..6d11b294b22f7945967c8f99a8ec584a299491d8
--- /dev/null
+++ b/global_local/configs/models/video_llama.yaml
@@ -0,0 +1,36 @@
+model:
+ arch: video_llama
+
+ # vit encoder
+ image_size: 224
+ drop_path_rate: 0
+ use_grad_checkpoint: False
+ vit_precision: "fp16"
+ freeze_vit: True
+ freeze_qformer: True
+
+ # Q-Former
+ num_query_token: 32
+
+ # Vicuna
+ llama_model: "/projectnb/ivc-ml/samarth/projects/misc/minigpt-4-chat-models/Llama-2-7b-chat-hf"
+
+ # generation configs
+ prompt: ""
+
+preprocess:
+ vis_processor:
+ train:
+ name: "alpro_video_train"
+ image_size: 224
+ n_frms: 8
+ eval:
+ name: "alpro_video_eval"
+ image_size: 224
+ n_frms: 8
+ text_processor:
+ train:
+ name: "blip_caption"
+ eval:
+ name: "blip_caption"
+
\ No newline at end of file
diff --git a/global_local/conversation/__init__.py b/global_local/conversation/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/global_local/conversation/__pycache__/__init__.cpython-39.pyc b/global_local/conversation/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..a7c0a55dc73ae095c320a9db5142218343aa1d91
Binary files /dev/null and b/global_local/conversation/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/conversation/__pycache__/conversation_video.cpython-39.pyc b/global_local/conversation/__pycache__/conversation_video.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..33a21b44bf29fb1d2047cfbc2f013830663077ec
Binary files /dev/null and b/global_local/conversation/__pycache__/conversation_video.cpython-39.pyc differ
diff --git a/global_local/conversation/conversation_video.py b/global_local/conversation/conversation_video.py
new file mode 100644
index 0000000000000000000000000000000000000000..68e030bd7204cfd0005c8f9663c953867da1455f
--- /dev/null
+++ b/global_local/conversation/conversation_video.py
@@ -0,0 +1,404 @@
+"""
+Conversation prompt template of Video-LLaMA.
+Adapted from: https://github.com/Vision-CAIR/MiniGPT-4/blob/main/minigpt4/conversation/conversation.py
+"""
+import argparse
+import time
+from PIL import Image
+import sys
+import os
+import torch
+from transformers import AutoTokenizer, AutoModelForCausalLM, LlamaTokenizer
+from transformers import StoppingCriteria, StoppingCriteriaList
+
+import dataclasses
+from enum import auto, Enum
+from typing import List, Tuple, Any
+import os
+import sys
+from global_local.common.registry import registry
+from global_local.processors.video_processor import ToTHWC,ToUint8,load_video
+from global_local.processors import Blip2ImageEvalProcessor
+
+#from video_llama.models.ImageBind.data import load_and_transform_audio_data
+class SeparatorStyle(Enum):
+ """Different separator style."""
+ SINGLE = auto()
+ TWO = auto()
+ LLAMA_2 = auto()
+
+
+@dataclasses.dataclass
+class Conversation:
+ """A class that keeps all conversation history."""
+ system: str
+ roles: List[str]
+ messages: List[List[str]]
+ offset: int
+ # system_img: List[Image.Image] = []
+ sep_style: SeparatorStyle = SeparatorStyle.SINGLE
+ sep: str = "###"
+ sep2: str = None
+
+ skip_next: bool = False
+ conv_id: Any = None
+
+ def get_prompt(self):
+ if self.sep_style == SeparatorStyle.SINGLE:
+ ret = self.system + self.sep
+ for role, message in self.messages:
+ if message:
+ ret += role + ": " + message + self.sep
+ else:
+ ret += role + ":"
+ return ret
+ elif self.sep_style == SeparatorStyle.TWO:
+ seps = [self.sep, self.sep2]
+ ret = self.system + seps[0]
+ for i, (role, message) in enumerate(self.messages):
+ if message:
+ ret += role + ": " + message + seps[i % 2]
+ else:
+ ret += role + ":"
+ return ret
+ elif self.sep_style == SeparatorStyle.LLAMA_2:
+ wrap_sys = lambda msg: f"<>\n{msg}\n<>\n\n"
+ wrap_inst = lambda msg: f"[INST] {msg} [/INST]"
+ ret = ""
+
+ for i, (role, message) in enumerate(self.messages):
+ if i == 0:
+ assert message, "first message should not be none"
+ assert role == self.roles[0], "first message should come from user"
+ if message:
+ if type(message) is tuple:
+ message, _, _ = message
+ if i == 0: message = wrap_sys(self.system) + message
+ if i % 2 == 0:
+ message = wrap_inst(message)
+ ret += self.sep + message
+ else:
+ ret += " " + message + " " + self.sep2
+ else:
+ ret += ""
+ ret = ret.lstrip(self.sep)
+ return ret
+ else:
+ raise ValueError(f"Invalid style: {self.sep_style}")
+
+ def append_message(self, role, message):
+ self.messages.append([role, message])
+
+ def to_gradio_chatbot(self):
+ ret = []
+ for i, (role, msg) in enumerate(self.messages[self.offset:]):
+ if i % 2 == 0:
+ ret.append([msg, None])
+ else:
+ ret[-1][-1] = msg
+ return ret
+
+ def copy(self):
+ return Conversation(
+ system=self.system,
+ # system_img=self.system_img,
+ roles=self.roles,
+ messages=[[x, y] for x, y in self.messages],
+ offset=self.offset,
+ sep_style=self.sep_style,
+ sep=self.sep,
+ sep2=self.sep2,
+ conv_id=self.conv_id)
+
+ def dict(self):
+ return {
+ "system": self.system,
+ # "system_img": self.system_img,
+ "roles": self.roles,
+ "messages": self.messages,
+ "offset": self.offset,
+ "sep": self.sep,
+ "sep2": self.sep2,
+ "conv_id": self.conv_id,
+ }
+
+
+class StoppingCriteriaSub(StoppingCriteria):
+
+ def __init__(self, stops=[], encounters=1):
+ super().__init__()
+ self.stops = stops
+
+ def __call__(self, input_ids: torch.LongTensor, scores: torch.FloatTensor):
+ for stop in self.stops:
+ if torch.all((stop == input_ids[0][-len(stop):])).item():
+ return True
+
+ return False
+
+
+CONV_VISION = Conversation(
+ system="Give the following image:
ImageContent. "
+ "You will be able to see the image once I provide it to you. Please answer my questions.",
+ roles=("Human", "Assistant"),
+ messages=[],
+ offset=0,
+ sep_style=SeparatorStyle.SINGLE,
+ sep="###",
+)
+
+default_conversation = Conversation(
+ system="",
+ roles=("Human", "Assistant"),
+ messages=[],
+ offset=0,
+ sep_style=SeparatorStyle.SINGLE,
+ sep="###",
+)
+conv_llava_llama_2 = Conversation(
+ system="You are a helpful language and vision assistant. "
+ "You are able to understand the visual content that the user provides, "
+ "and assist the user with a variety of tasks using natural language.",
+ roles=("USER", "ASSISTANT"),
+ messages=(),
+ offset=0,
+ sep_style=SeparatorStyle.LLAMA_2,
+ sep="",
+ sep2="",
+)
+class Chat:
+ def __init__(self, model, vis_processor, device='cuda:0'):
+ self.device = device
+ self.model = model
+ self.vis_processor = vis_processor
+ self.image_vis_processor = Blip2ImageEvalProcessor()
+ # stop_words_ids = [torch.tensor([835]).to(self.device),
+ # torch.tensor([2277, 29937]).to(self.device)] # '###' can be encoded in two different ways.
+ # self.stopping_criteria = StoppingCriteriaList([StoppingCriteriaSub(stops=stop_words_ids)])
+
+ self.num_frames_per_clip = 16
+ self.num_segments = 4
+
+ def ask(self, text, conv):
+ if len(conv.messages) > 0 and conv.messages[-1][0] == conv.roles[0] \
+ and ('' in conv.messages[-1][1] or '' in conv.messages[-1][1]): # last message is image.
+ conv.messages[-1][1] = ' '.join([conv.messages[-1][1], text])
+ else:
+ conv.append_message(conv.roles[0], text)
+
+ def answer(self, conv, img_list, max_new_tokens=300, num_beams=1, min_length=1, top_p=0.9,
+ repetition_penalty=1.0, length_penalty=1, temperature=1.0, max_length=2000):
+ conv.append_message(conv.roles[1], None)
+ embs = self.get_context_emb(conv, img_list)
+
+ current_max_len = embs.shape[1] + max_new_tokens
+ if current_max_len - max_length > 0:
+ print('Warning: The number of tokens in current conversation exceeds the max length. '
+ 'The model will not see the contexts outside the range.')
+ begin_idx = max(0, current_max_len - max_length)
+
+ embs = embs[:, begin_idx:]
+ if conv.sep =="###":
+ stop_words_ids = [torch.tensor([835]).to(self.device),
+ torch.tensor([2277, 29937]).to(self.device)] # '###' can be encoded in two different ways.
+ stopping_criteria = StoppingCriteriaList([StoppingCriteriaSub(stops=stop_words_ids)])
+ else:
+ stop_words_ids = [torch.tensor([2]).to(self.device)]
+ stopping_criteria = StoppingCriteriaList([StoppingCriteriaSub(stops=stop_words_ids)])
+
+ # stopping_criteria
+ outputs = self.model.llama_model.generate(
+ inputs_embeds=embs,
+ max_new_tokens=max_new_tokens,
+ stopping_criteria=stopping_criteria,
+ num_beams=num_beams,
+ do_sample=True,
+ min_length=min_length,
+ top_p=top_p,
+ repetition_penalty=repetition_penalty,
+ length_penalty=length_penalty,
+ temperature=temperature,
+ )
+ output_token = outputs[0]
+ if output_token[0] == 0: # the model might output a unknow token at the beginning. remove it
+ output_token = output_token[1:]
+ if output_token[0] == 1: # some users find that there is a start token at the beginning. remove it
+ output_token = output_token[1:]
+ output_text = self.model.llama_tokenizer.decode(output_token, add_special_tokens=False)
+ if conv.sep =="###":
+ output_text = output_text.split('###')[0] # remove the stop sign '###'
+ output_text = output_text.split('Assistant:')[-1].strip()
+ else:
+ output_text = output_text.split(conv.sep2)[0] # remove the stop sign '###'
+ output_text = output_text.split(conv.roles[1]+':')[-1].strip()
+ conv.messages[-1][1] = output_text
+ return output_text, output_token.cpu().numpy()
+
+ def upload_video(self, video_path, conv, img_list):
+
+ msg = ""
+ if isinstance(video_path, str): # is a video path
+ ext = os.path.splitext(video_path)[-1].lower()
+ print(video_path)
+ # image = self.vis_processor(image).unsqueeze(0).to(self.device)
+ video, msg = load_video(
+ video_path=video_path,
+ n_frms=8,
+ height=224,
+ width=224,
+ sampling ="uniform", return_msg = True
+ )
+ video = self.vis_processor.transform(video)
+ video = video.unsqueeze(0).to(self.device)
+ # print(image)
+ else:
+ raise NotImplementedError
+
+ try:
+ audio_flag = 1
+ audio = load_and_transform_audio_data([video_path],"cpu", clips_per_video=8)
+ audio = audio.to(self.device)
+ except :
+ print('no audio is found')
+ audio_flag = 0
+ finally:
+ if audio_flag == 1:
+ # image_emb, _ = self.model.encode_videoQformer_audiovideo(video,audio)
+ image_emb, _ = self.model.encode_videoQformer_visual(video)
+ audio_emb,_ = self.model.encode_audioQformer(audio)
+ img_list.append(audio_emb)
+ img_list.append(image_emb)
+ conv.system = ""
+ # conv.append_message(conv.roles[0], "The audio of this video is ")
+ conv.append_message(conv.roles[0], "Close your eyes, open your ears and you imagine only based on the sound that: . \
+ Close your ears, open your eyes and you see that . \
+ Now answer my question based on what you have just seen and heard.")
+
+ else: # only vison no audio
+ # conv.system = "You can understand the video that the user provides. Follow the instructions carefully and explain your answers in detail."
+ image_emb, _ = self.model.encode_videoQformer_visual(video)
+ img_list.append(image_emb)
+ conv.append_message(conv.roles[0], " "+ msg)
+ return "Received."
+
+ def upload_video_without_audio(self, video_path, conv, img_list):
+ msg = ""
+ if isinstance(video_path, str): # is a video path
+ ext = os.path.splitext(video_path)[-1].lower()
+ print(video_path)
+ # image = self.vis_processor(image).unsqueeze(0).to(self.device)
+ video, msg = load_video(
+ video_path=video_path,
+ n_frms=self.num_frames_per_clip*self.num_segments,
+ height=224,
+ width=224,
+ sampling ="uniform", return_msg = True
+ )
+
+ video = self.vis_processor.transform(video)
+ video = video.unsqueeze(0).to(self.device)
+ else:
+ raise NotImplementedError
+
+ # conv.system = "You can understand the video that the user provides. Follow the instructions carefully and explain your answers in detail."
+ #image_emb, _ = self.model.encode_videoQformer_visual(video)
+ image_emb, _ = self.process_video_frames(video)
+ img_list.append(image_emb)
+ conv.append_message(conv.roles[0], " "+ msg)
+
+ return "Received."
+
+ def process_video_frames(self, all_frames):
+ total_num_frames = self.num_frames_per_clip * self.num_segments
+ global_clip_indices = torch.linspace(0, total_num_frames-1, steps=self.num_frames_per_clip)
+ short_window_indices = torch.linspace(0, total_num_frames-1, steps=self.num_frames_per_clip * self.num_segments)
+
+ global_processed_frames = []
+ for i in global_clip_indices:
+ i = int(i)
+ curr = all_frames[:, :, i]
+ #curr = np.uint8(all_frames[i])
+ #curr = frame_transform(Image.fromarray(curr))
+ global_processed_frames.append(curr)
+ global_processed_frames = torch.stack(global_processed_frames, dim=2)
+
+ '''if len(global_processed_frames) < args.num_frames_per_clip:
+ diff = args.num_frames_per_clip - len(global_processed_frames)
+ pad = global_processed_frames[-1].unsqueeze(0).repeat(diff, 1, 1, 1)
+ global_processed_frames = torch.cat((global_processed_frames, pad), dim=0)'''
+
+ short_window_processed_frames = []
+ for i in short_window_indices:
+ i = int(i)
+ curr = all_frames[:, :, i]
+ #curr = np.uint8(all_frames[i])
+ #curr = frame_transform(Image.fromarray(curr))
+ short_window_processed_frames.append(curr)
+ short_window_processed_frames = torch.stack(short_window_processed_frames, dim=2)
+
+ '''if len(short_window_processed_frames) < args.num_frames_per_clip * args.num_segments:
+ diff = args.num_frames_per_clip * args.num_segments - len(short_window_processed_frames)
+ pad = short_window_processed_frames[-1].unsqueeze(0).repeat(diff, 1, 1, 1)
+ short_window_processed_frames = torch.cat((short_window_processed_frames, pad), dim=0)'''
+
+ global_attn_mask = torch.zeros((self.num_frames_per_clip))
+ global_attn_mask[:global_processed_frames.size(2)] = True
+
+ short_window_attn_mask = torch.zeros((self.num_frames_per_clip * self.num_segments))
+ short_window_attn_mask[:short_window_processed_frames.size(2)] = True
+
+ global_processed_frames = global_processed_frames.permute((0, 2, 1, 3, 4)).cuda()
+ short_window_processed_frames = short_window_processed_frames.permute((0, 2, 1, 3, 4)).cuda()
+ global_frame_attn_mask = global_attn_mask.unsqueeze(0).cuda()
+ segments_frame_attn_mask = short_window_attn_mask.unsqueeze(0).cuda()
+
+ with torch.no_grad():
+ samples = {'global_video': global_processed_frames, 'global_frame_attn_mask': global_frame_attn_mask, 'segments_video': short_window_processed_frames, 'segments_frame_attn_mask': segments_frame_attn_mask}
+ merged_video_embeds, merged_video_embeds_mask = self.model.compute_merged_video_embeds(samples)
+
+ return merged_video_embeds, merged_video_embeds_mask
+
+ def upload_img(self, image, conv, img_list):
+
+ msg = ""
+ if isinstance(image, str): # is a image path
+ raw_image = Image.open(image).convert('RGB') # 增加一个时间维度
+ image = self.image_vis_processor(raw_image).unsqueeze(0).unsqueeze(2).to(self.device)
+ elif isinstance(image, Image.Image):
+ raw_image = image
+ image = self.image_vis_processor(raw_image).unsqueeze(0).unsqueeze(2).to(self.device)
+ elif isinstance(image, torch.Tensor):
+ if len(image.shape) == 3:
+ image = image.unsqueeze(0)
+ image = image.to(self.device)
+ else:
+ raise NotImplementedError
+
+ image_emb, _ = self.model.encode_videoQformer_visual(image)
+ img_list.append(image_emb)
+ # Todo msg=""
+ conv.append_message(conv.roles[0], " "+ msg)
+
+ return "Received."
+
+ def get_context_emb(self, conv, img_list):
+ prompt = conv.get_prompt()
+ prompt_segs = prompt.split('')
+ assert len(prompt_segs) == len(img_list) + 1, "Unmatched numbers of image placeholders and images."
+ seg_tokens = [
+ self.model.llama_tokenizer(
+ seg, return_tensors="pt", add_special_tokens=i == 0).to(self.device).input_ids
+ # only add bos to the first seg
+ for i, seg in enumerate(prompt_segs)
+ ]
+ seg_embs = [self.model.llama_model.model.embed_tokens(seg_t) for seg_t in seg_tokens]
+ mixed_embs = [emb for pair in zip(seg_embs[:-1], img_list) for emb in pair] + [seg_embs[-1]]
+ mixed_embs = torch.cat(mixed_embs, dim=1)
+
+ return mixed_embs
+
+if __name__ =='__main__':
+ video_path = '/mnt/workspace/videoGPT/Video-LLaMA/examples/applausing.mp4'
+ # import torch.classes.torchaudio.ffmpeg_StreamReader
+ # ffmpeg_StreamReader(video_path)
+ load_and_transform_audio_data([video_path],"cpu", clips_per_video=8)
diff --git a/global_local/datasets/__init__.py b/global_local/datasets/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/global_local/datasets/__pycache__/__init__.cpython-39.pyc b/global_local/datasets/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..c20496685579817c40456fbc74c5b3ebe09bf6ab
Binary files /dev/null and b/global_local/datasets/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/datasets/__pycache__/data_utils.cpython-39.pyc b/global_local/datasets/__pycache__/data_utils.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..de49281c734e3e21d6e119fc159736d452c7ca81
Binary files /dev/null and b/global_local/datasets/__pycache__/data_utils.cpython-39.pyc differ
diff --git a/global_local/datasets/builders/__init__.py b/global_local/datasets/builders/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..14b373a8c1ea80464da7b34e2e4b7851ce0c3b5e
--- /dev/null
+++ b/global_local/datasets/builders/__init__.py
@@ -0,0 +1,77 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from global_local.datasets.builders.base_dataset_builder import load_dataset_config
+from global_local.datasets.builders.image_text_pair_builder import (
+ CCSBUBuilder,
+ LaionBuilder,
+ CCSBUAlignBuilder
+)
+from global_local.datasets.builders.video_caption_builder import WebvidBuilder
+from global_local.common.registry import registry
+from global_local.datasets.builders.instruct_builder import WebvidInstruct_Builder,LlavaInstruct_Builder
+__all__ = [
+ "CCSBUBuilder",
+ "LaionBuilder",
+ "CCSBUAlignBuilder",
+ "WebvidBuilder",
+ "LlavaInstruct_Builder",
+ "WebvidInstruct_Builder"
+
+]
+
+
+def load_dataset(name, cfg_path=None, vis_path=None, data_type=None):
+ """
+ Example
+
+ >>> dataset = load_dataset("coco_caption", cfg=None)
+ >>> splits = dataset.keys()
+ >>> print([len(dataset[split]) for split in splits])
+
+ """
+ if cfg_path is None:
+ cfg = None
+ else:
+ cfg = load_dataset_config(cfg_path)
+
+ try:
+ builder = registry.get_builder_class(name)(cfg)
+ except TypeError:
+ print(
+ f"Dataset {name} not found. Available datasets:\n"
+ + ", ".join([str(k) for k in dataset_zoo.get_names()])
+ )
+ exit(1)
+
+ if vis_path is not None:
+ if data_type is None:
+ # use default data type in the config
+ data_type = builder.config.data_type
+
+ assert (
+ data_type in builder.config.build_info
+ ), f"Invalid data_type {data_type} for {name}."
+
+ builder.config.build_info.get(data_type).storage = vis_path
+
+ dataset = builder.build_datasets()
+ return dataset
+
+
+class DatasetZoo:
+ def __init__(self) -> None:
+ self.dataset_zoo = {
+ k: list(v.DATASET_CONFIG_DICT.keys())
+ for k, v in sorted(registry.mapping["builder_name_mapping"].items())
+ }
+
+ def get_names(self):
+ return list(self.dataset_zoo.keys())
+
+
+dataset_zoo = DatasetZoo()
diff --git a/global_local/datasets/builders/__pycache__/__init__.cpython-39.pyc b/global_local/datasets/builders/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..c852b0feb52c9b1eed9f55f601b9c3d941929e13
Binary files /dev/null and b/global_local/datasets/builders/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/datasets/builders/__pycache__/base_dataset_builder.cpython-39.pyc b/global_local/datasets/builders/__pycache__/base_dataset_builder.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..938309931b854f8a4604a49d1743b0d1ef728eff
Binary files /dev/null and b/global_local/datasets/builders/__pycache__/base_dataset_builder.cpython-39.pyc differ
diff --git a/global_local/datasets/builders/__pycache__/image_text_pair_builder.cpython-39.pyc b/global_local/datasets/builders/__pycache__/image_text_pair_builder.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..f4b50dd18e1b732152405ec478885d62832cc404
Binary files /dev/null and b/global_local/datasets/builders/__pycache__/image_text_pair_builder.cpython-39.pyc differ
diff --git a/global_local/datasets/builders/__pycache__/instruct_builder.cpython-39.pyc b/global_local/datasets/builders/__pycache__/instruct_builder.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..422b2c724ab88283ace72cc2d4c7ca1cb249b0ca
Binary files /dev/null and b/global_local/datasets/builders/__pycache__/instruct_builder.cpython-39.pyc differ
diff --git a/global_local/datasets/builders/__pycache__/video_caption_builder.cpython-39.pyc b/global_local/datasets/builders/__pycache__/video_caption_builder.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..204ce1b709f42eebaffdcebb2a18267d8303be25
Binary files /dev/null and b/global_local/datasets/builders/__pycache__/video_caption_builder.cpython-39.pyc differ
diff --git a/global_local/datasets/builders/base_dataset_builder.py b/global_local/datasets/builders/base_dataset_builder.py
new file mode 100644
index 0000000000000000000000000000000000000000..dd8759f2636cf2b7f6be75b80526d017eaa30c61
--- /dev/null
+++ b/global_local/datasets/builders/base_dataset_builder.py
@@ -0,0 +1,236 @@
+"""
+ This file is from
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import logging
+import os
+import shutil
+import warnings
+
+from omegaconf import OmegaConf
+import torch.distributed as dist
+from torchvision.datasets.utils import download_url
+
+import global_local.common.utils as utils
+from global_local.common.dist_utils import is_dist_avail_and_initialized, is_main_process
+from global_local.common.registry import registry
+from global_local.processors.base_processor import BaseProcessor
+
+
+
+class BaseDatasetBuilder:
+ train_dataset_cls, eval_dataset_cls = None, None
+
+ def __init__(self, cfg=None):
+ super().__init__()
+
+ if cfg is None:
+ # help to create datasets from default config.
+ self.config = load_dataset_config(self.default_config_path())
+ elif isinstance(cfg, str):
+ self.config = load_dataset_config(cfg)
+ else:
+ # when called from task.build_dataset()
+ self.config = cfg
+
+ self.data_type = self.config.data_type
+
+ self.vis_processors = {"train": BaseProcessor(), "eval": BaseProcessor()}
+ self.text_processors = {"train": BaseProcessor(), "eval": BaseProcessor()}
+
+ def build_datasets(self):
+ # download, split, etc...
+ # only called on 1 GPU/TPU in distributed
+
+ if is_main_process():
+ self._download_data()
+
+ if is_dist_avail_and_initialized():
+ dist.barrier()
+
+ # at this point, all the annotations and image/videos should be all downloaded to the specified locations.
+ logging.info("Building datasets...")
+ datasets = self.build() # dataset['train'/'val'/'test']
+
+ return datasets
+
+ def build_processors(self):
+ vis_proc_cfg = self.config.get("vis_processor")
+ txt_proc_cfg = self.config.get("text_processor")
+
+ if vis_proc_cfg is not None:
+ vis_train_cfg = vis_proc_cfg.get("train")
+ vis_eval_cfg = vis_proc_cfg.get("eval")
+
+ self.vis_processors["train"] = self._build_proc_from_cfg(vis_train_cfg)
+ self.vis_processors["eval"] = self._build_proc_from_cfg(vis_eval_cfg)
+
+ if txt_proc_cfg is not None:
+ txt_train_cfg = txt_proc_cfg.get("train")
+ txt_eval_cfg = txt_proc_cfg.get("eval")
+
+ self.text_processors["train"] = self._build_proc_from_cfg(txt_train_cfg)
+ self.text_processors["eval"] = self._build_proc_from_cfg(txt_eval_cfg)
+
+ @staticmethod
+ def _build_proc_from_cfg(cfg):
+ return (
+ registry.get_processor_class(cfg.name).from_config(cfg)
+ if cfg is not None
+ else None
+ )
+
+ @classmethod
+ def default_config_path(cls, type="default"):
+ return utils.get_abs_path(cls.DATASET_CONFIG_DICT[type])
+
+ def _download_data(self):
+ self._download_ann()
+ self._download_vis()
+
+ def _download_ann(self):
+ """
+ Download annotation files if necessary.
+ All the vision-language datasets should have annotations of unified format.
+
+ storage_path can be:
+ (1) relative/absolute: will be prefixed with env.cache_root to make full path if relative.
+ (2) basename/dirname: will be suffixed with base name of URL if dirname is provided.
+
+ Local annotation paths should be relative.
+ """
+ anns = self.config.build_info.annotations
+
+ splits = anns.keys()
+
+ cache_root = registry.get_path("cache_root")
+
+ for split in splits:
+ info = anns[split]
+
+ urls, storage_paths = info.get("url", None), info.storage
+
+ if isinstance(urls, str):
+ urls = [urls]
+ if isinstance(storage_paths, str):
+ storage_paths = [storage_paths]
+
+ assert len(urls) == len(storage_paths)
+
+ for url_or_filename, storage_path in zip(urls, storage_paths):
+ # if storage_path is relative, make it full by prefixing with cache_root.
+ if not os.path.isabs(storage_path):
+ storage_path = os.path.join(cache_root, storage_path)
+
+ dirname = os.path.dirname(storage_path)
+ if not os.path.exists(dirname):
+ os.makedirs(dirname)
+
+ if os.path.isfile(url_or_filename):
+ src, dst = url_or_filename, storage_path
+ if not os.path.exists(dst):
+ shutil.copyfile(src=src, dst=dst)
+ else:
+ logging.info("Using existing file {}.".format(dst))
+ else:
+ if os.path.isdir(storage_path):
+ # if only dirname is provided, suffix with basename of URL.
+ raise ValueError(
+ "Expecting storage_path to be a file path, got directory {}".format(
+ storage_path
+ )
+ )
+ else:
+ filename = os.path.basename(storage_path)
+
+ download_url(url=url_or_filename, root=dirname, filename=filename)
+
+ def _download_vis(self):
+
+ storage_path = self.config.build_info.get(self.data_type).storage
+ storage_path = utils.get_cache_path(storage_path)
+
+ if not os.path.exists(storage_path):
+ warnings.warn(
+ f"""
+ The specified path {storage_path} for visual inputs does not exist.
+ Please provide a correct path to the visual inputs or
+ refer to datasets/download_scripts/README.md for downloading instructions.
+ """
+ )
+
+ def build(self):
+ """
+ Create by split datasets inheriting torch.utils.data.Datasets.
+
+ # build() can be dataset-specific. Overwrite to customize.
+ """
+ self.build_processors()
+
+ build_info = self.config.build_info
+
+ ann_info = build_info.annotations
+ vis_info = build_info.get(self.data_type)
+
+ datasets = dict()
+ for split in ann_info.keys():
+ if split not in ["train", "val", "test"]:
+ continue
+
+ is_train = split == "train"
+
+ # processors
+ vis_processor = (
+ self.vis_processors["train"]
+ if is_train
+ else self.vis_processors["eval"]
+ )
+ text_processor = (
+ self.text_processors["train"]
+ if is_train
+ else self.text_processors["eval"]
+ )
+
+ # annotation path
+ ann_paths = ann_info.get(split).storage
+ if isinstance(ann_paths, str):
+ ann_paths = [ann_paths]
+
+ abs_ann_paths = []
+ for ann_path in ann_paths:
+ if not os.path.isabs(ann_path):
+ ann_path = utils.get_cache_path(ann_path)
+ abs_ann_paths.append(ann_path)
+ ann_paths = abs_ann_paths
+
+ # visual data storage path
+ vis_path = os.path.join(vis_info.storage, split)
+
+ if not os.path.isabs(vis_path):
+ # vis_path = os.path.join(utils.get_cache_path(), vis_path)
+ vis_path = utils.get_cache_path(vis_path)
+
+ if not os.path.exists(vis_path):
+ warnings.warn("storage path {} does not exist.".format(vis_path))
+
+ # create datasets
+ dataset_cls = self.train_dataset_cls if is_train else self.eval_dataset_cls
+ datasets[split] = dataset_cls(
+ vis_processor=vis_processor,
+ text_processor=text_processor,
+ ann_paths=ann_paths,
+ vis_root=vis_path,
+ )
+
+ return datasets
+
+
+def load_dataset_config(cfg_path):
+ cfg = OmegaConf.load(cfg_path).datasets
+ cfg = cfg[list(cfg.keys())[0]]
+
+ return cfg
diff --git a/global_local/datasets/builders/image_text_pair_builder.py b/global_local/datasets/builders/image_text_pair_builder.py
new file mode 100644
index 0000000000000000000000000000000000000000..11d2887706e6ade56c8040095630f8a266566f1f
--- /dev/null
+++ b/global_local/datasets/builders/image_text_pair_builder.py
@@ -0,0 +1,106 @@
+import os
+import logging
+import warnings
+
+from global_local.common.registry import registry
+from global_local.datasets.builders.base_dataset_builder import BaseDatasetBuilder
+from global_local.datasets.datasets.laion_dataset import LaionDataset
+from global_local.datasets.datasets.cc_sbu_dataset import CCSBUDataset, CCSBUAlignDataset
+
+
+@registry.register_builder("cc_sbu")
+class CCSBUBuilder(BaseDatasetBuilder):
+ train_dataset_cls = CCSBUDataset
+
+ DATASET_CONFIG_DICT = {"default": "configs/datasets/cc_sbu/defaults.yaml"}
+
+ def _download_ann(self):
+ pass
+
+ def _download_vis(self):
+ pass
+
+ def build(self):
+ self.build_processors()
+
+ build_info = self.config.build_info
+
+ datasets = dict()
+ split = "train"
+
+ # create datasets
+ # [NOTE] return inner_datasets (wds.DataPipeline)
+ dataset_cls = self.train_dataset_cls
+ datasets[split] = dataset_cls(
+ vis_processor=self.vis_processors[split],
+ text_processor=self.text_processors[split],
+ location=build_info.storage,
+ ).inner_dataset
+
+ return datasets
+
+
+@registry.register_builder("laion")
+class LaionBuilder(BaseDatasetBuilder):
+ train_dataset_cls = LaionDataset
+
+ DATASET_CONFIG_DICT = {"default": "configs/datasets/laion/defaults.yaml"}
+
+ def _download_ann(self):
+ pass
+
+ def _download_vis(self):
+ pass
+
+ def build(self):
+ self.build_processors()
+
+ build_info = self.config.build_info
+
+ datasets = dict()
+ split = "train"
+
+ # create datasets
+ # [NOTE] return inner_datasets (wds.DataPipeline)
+ dataset_cls = self.train_dataset_cls
+ datasets[split] = dataset_cls(
+ vis_processor=self.vis_processors[split],
+ text_processor=self.text_processors[split],
+ location=build_info.storage,
+ ).inner_dataset
+
+ return datasets
+
+
+@registry.register_builder("cc_sbu_align")
+class CCSBUAlignBuilder(BaseDatasetBuilder):
+ train_dataset_cls = CCSBUAlignDataset
+
+ DATASET_CONFIG_DICT = {
+ "default": "configs/datasets/cc_sbu/align.yaml",
+ }
+
+ def build_datasets(self):
+ # at this point, all the annotations and image/videos should be all downloaded to the specified locations.
+ logging.info("Building datasets...")
+ self.build_processors()
+
+ build_info = self.config.build_info
+ storage_path = build_info.storage
+
+ datasets = dict()
+
+ if not os.path.exists(storage_path):
+ warnings.warn("storage path {} does not exist.".format(storage_path))
+
+ # create datasets
+ dataset_cls = self.train_dataset_cls
+ datasets['train'] = dataset_cls(
+ vis_processor=self.vis_processors["train"],
+ text_processor=self.text_processors["train"],
+ ann_paths=[os.path.join(storage_path, 'filter_cap.json')],
+ vis_root=os.path.join(storage_path, 'image'),
+ )
+
+ return datasets
+
diff --git a/global_local/datasets/builders/instruct_builder.py b/global_local/datasets/builders/instruct_builder.py
new file mode 100644
index 0000000000000000000000000000000000000000..2b963512da50c7c4de2e1f4c3ce4e09217d56529
--- /dev/null
+++ b/global_local/datasets/builders/instruct_builder.py
@@ -0,0 +1,78 @@
+import os
+import logging
+import warnings
+
+from global_local.common.registry import registry
+from global_local.datasets.builders.base_dataset_builder import BaseDatasetBuilder
+from global_local.datasets.datasets.laion_dataset import LaionDataset
+from global_local.datasets.datasets.llava_instruct_dataset import Instruct_Dataset
+from global_local.datasets.datasets.video_instruct_dataset import Video_Instruct_Dataset
+
+@registry.register_builder("instruct")
+class Instruct_Builder(BaseDatasetBuilder):
+ train_dataset_cls = Instruct_Dataset
+
+ DATASET_CONFIG_DICT = {"default": "configs/datasets/instruct/defaults.yaml"}
+
+ def _download_ann(self):
+ pass
+
+ def _download_vis(self):
+ pass
+
+ def build(self):
+ self.build_processors()
+ datasets = dict()
+ split = "train"
+
+ build_info = self.config.build_info
+ dataset_cls = self.train_dataset_cls
+ if self.config.num_video_query_token:
+ num_video_query_token = self.config.num_video_query_token
+ else:
+ num_video_query_token = 32
+
+ if self.config.tokenizer_name:
+ tokenizer_name = self.config.tokenizer_name
+ else:
+ tokenizer_name = '/mnt/workspace/ckpt/vicuna-13b/'
+
+
+ datasets[split] = dataset_cls(
+ vis_processor=self.vis_processors[split],
+ text_processor=self.text_processors[split],
+ vis_root=build_info.videos_dir,
+ ann_root=build_info.anno_dir,
+ num_video_query_token = num_video_query_token,
+ tokenizer_name = tokenizer_name,
+ data_type = self.config.data_type
+ )
+
+ return datasets
+
+@registry.register_builder("webvid_instruct")
+class WebvidInstruct_Builder(Instruct_Builder):
+ train_dataset_cls = Video_Instruct_Dataset
+
+ DATASET_CONFIG_DICT = {
+ "default": "configs/datasets/instruct/webvid_instruct.yaml",
+ }
+
+@registry.register_builder("webvid_instruct_zh")
+class WebvidInstruct_zh_Builder(Instruct_Builder):
+ train_dataset_cls = Video_Instruct_Dataset
+
+ DATASET_CONFIG_DICT = {
+ "default": "configs/datasets/instruct/webvid_instruct.yaml",
+ }
+
+
+
+@registry.register_builder("llava_instruct")
+class LlavaInstruct_Builder(Instruct_Builder):
+ train_dataset_cls = Instruct_Dataset
+
+ DATASET_CONFIG_DICT = {
+ "default": "configs/datasets/instruct/llava_instruct.yaml",
+ }
+
diff --git a/global_local/datasets/builders/video_caption_builder.py b/global_local/datasets/builders/video_caption_builder.py
new file mode 100644
index 0000000000000000000000000000000000000000..79654e317b81863536514db7fe9670ca36285d9b
--- /dev/null
+++ b/global_local/datasets/builders/video_caption_builder.py
@@ -0,0 +1,34 @@
+import os
+import logging
+import warnings
+
+from global_local.common.registry import registry
+from global_local.datasets.builders.base_dataset_builder import BaseDatasetBuilder
+from global_local.datasets.datasets.webvid_datasets import WebvidDataset
+
+@registry.register_builder("webvid")
+class WebvidBuilder(BaseDatasetBuilder):
+ train_dataset_cls = WebvidDataset
+ DATASET_CONFIG_DICT = {"default": "configs/datasets/webvid/defaults.yaml"}
+
+ def _download_ann(self):
+ pass
+
+ def _download_vis(self):
+ pass
+
+ def build(self):
+ self.build_processors()
+ datasets = dict()
+ split = "train"
+
+ build_info = self.config.build_info
+ dataset_cls = self.train_dataset_cls
+ datasets[split] = dataset_cls(
+ vis_processor=self.vis_processors[split],
+ text_processor=self.text_processors[split],
+ vis_root=build_info.videos_dir,
+ ann_root=build_info.anno_dir
+ )
+
+ return datasets
\ No newline at end of file
diff --git a/global_local/datasets/data_utils.py b/global_local/datasets/data_utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..6781ca7f8a4772246c40cc24a5e323b21a94938a
--- /dev/null
+++ b/global_local/datasets/data_utils.py
@@ -0,0 +1,196 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import gzip
+import logging
+import os
+import random as rnd
+import tarfile
+import zipfile
+import random
+from typing import List
+from tqdm import tqdm
+
+import decord
+from decord import VideoReader
+import webdataset as wds
+import numpy as np
+import torch
+from torch.utils.data.dataset import IterableDataset
+
+from global_local.common.registry import registry
+from global_local.datasets.datasets.base_dataset import ConcatDataset
+
+
+decord.bridge.set_bridge("torch")
+MAX_INT = registry.get("MAX_INT")
+
+
+class ChainDataset(wds.DataPipeline):
+ r"""Dataset for chaining multiple :class:`DataPipeline` s.
+
+ This class is useful to assemble different existing dataset streams. The
+ chaining operation is done on-the-fly, so concatenating large-scale
+ datasets with this class will be efficient.
+
+ Args:
+ datasets (iterable of IterableDataset): datasets to be chained together
+ """
+ def __init__(self, datasets: List[wds.DataPipeline]) -> None:
+ super().__init__()
+ self.datasets = datasets
+ self.prob = []
+ self.names = []
+ for dataset in self.datasets:
+ if hasattr(dataset, 'name'):
+ self.names.append(dataset.name)
+ else:
+ self.names.append('Unknown')
+ if hasattr(dataset, 'sample_ratio'):
+ self.prob.append(dataset.sample_ratio)
+ else:
+ self.prob.append(1)
+ logging.info("One of the datapipeline doesn't define ratio and set to 1 automatically.")
+
+ def __iter__(self):
+ datastreams = [iter(dataset) for dataset in self.datasets]
+ while True:
+ select_datastream = random.choices(datastreams, weights=self.prob, k=1)[0]
+ yield next(select_datastream)
+
+
+def apply_to_sample(f, sample):
+ if len(sample) == 0:
+ return {}
+
+ def _apply(x):
+ if torch.is_tensor(x):
+ return f(x)
+ elif isinstance(x, dict):
+ return {key: _apply(value) for key, value in x.items()}
+ elif isinstance(x, list):
+ return [_apply(x) for x in x]
+ else:
+ return x
+
+ return _apply(sample)
+
+
+def move_to_cuda(sample):
+ def _move_to_cuda(tensor):
+ return tensor.cuda()
+
+ return apply_to_sample(_move_to_cuda, sample)
+
+
+def prepare_sample(samples, cuda_enabled=True):
+ if cuda_enabled:
+ samples = move_to_cuda(samples)
+
+ # TODO fp16 support
+
+ return samples
+
+
+def reorg_datasets_by_split(datasets):
+ """
+ Organizes datasets by split.
+
+ Args:
+ datasets: dict of torch.utils.data.Dataset objects by name.
+
+ Returns:
+ Dict of datasets by split {split_name: List[Datasets]}.
+ """
+ # if len(datasets) == 1:
+ # return datasets[list(datasets.keys())[0]]
+ # else:
+ reorg_datasets = dict()
+
+ # reorganize by split
+ for _, dataset in datasets.items():
+ for split_name, dataset_split in dataset.items():
+ if split_name not in reorg_datasets:
+ reorg_datasets[split_name] = [dataset_split]
+ else:
+ reorg_datasets[split_name].append(dataset_split)
+
+ return reorg_datasets
+
+
+def concat_datasets(datasets):
+ """
+ Concatenates multiple datasets into a single dataset.
+
+ It supports may-style datasets and DataPipeline from WebDataset. Currently, does not support
+ generic IterableDataset because it requires creating separate samplers.
+
+ Now only supports conctenating training datasets and assuming validation and testing
+ have only a single dataset. This is because metrics should not be computed on the concatenated
+ datasets.
+
+ Args:
+ datasets: dict of torch.utils.data.Dataset objects by split.
+
+ Returns:
+ Dict of concatenated datasets by split, "train" is the concatenation of multiple datasets,
+ "val" and "test" remain the same.
+
+ If the input training datasets contain both map-style and DataPipeline datasets, returns
+ a tuple, where the first element is a concatenated map-style dataset and the second
+ element is a chained DataPipeline dataset.
+
+ """
+ # concatenate datasets in the same split
+ for split_name in datasets:
+ if split_name != "train":
+ assert (
+ len(datasets[split_name]) == 1
+ ), "Do not support multiple {} datasets.".format(split_name)
+ datasets[split_name] = datasets[split_name][0]
+ else:
+ iterable_datasets, map_datasets = [], []
+ for dataset in datasets[split_name]:
+ if isinstance(dataset, wds.DataPipeline):
+ logging.info(
+ "Dataset {} is IterableDataset, can't be concatenated.".format(
+ dataset
+ )
+ )
+ iterable_datasets.append(dataset)
+ elif isinstance(dataset, IterableDataset):
+ raise NotImplementedError(
+ "Do not support concatenation of generic IterableDataset."
+ )
+ else:
+ map_datasets.append(dataset)
+
+ # if len(iterable_datasets) > 0:
+ # concatenate map-style datasets and iterable-style datasets separately
+ if len(iterable_datasets) > 1:
+ chained_datasets = (
+ ChainDataset(iterable_datasets)
+ )
+ elif len(iterable_datasets) == 1:
+ chained_datasets = iterable_datasets[0]
+ else:
+ chained_datasets = None
+
+ concat_datasets = (
+ ConcatDataset(map_datasets) if len(map_datasets) > 0 else None
+ )
+
+ train_datasets = concat_datasets, chained_datasets
+ train_datasets = tuple([x for x in train_datasets if x is not None])
+ train_datasets = (
+ train_datasets[0] if len(train_datasets) == 1 else train_datasets
+ )
+
+ datasets[split_name] = train_datasets
+
+ return datasets
+
diff --git a/global_local/datasets/datasets/__init__.py b/global_local/datasets/datasets/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/global_local/datasets/datasets/__pycache__/__init__.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..f57d372eaaf6b224a99eb84762de7930a5515d2d
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/base_dataset.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/base_dataset.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..e47cf52003ee3f25b97deaf57adf135275ce609b
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/base_dataset.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/caption_datasets.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/caption_datasets.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..34e67429a9c8e770b5a211fbb614cf66e8cbe27b
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/caption_datasets.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/cc_sbu_dataset.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/cc_sbu_dataset.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..c29cc571308458961ed414457e309684f6e0ef11
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/cc_sbu_dataset.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/dataloader_utils.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/dataloader_utils.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..fe3d7ff7d8951d4db4182537c4d226998f8f4499
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/dataloader_utils.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/laion_dataset.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/laion_dataset.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..cc98f48d4eca37356152b59a0ed52ec0026a2857
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/laion_dataset.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/llava_instruct_dataset.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/llava_instruct_dataset.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..707449617bd5beb27720200c544ce5610db56b7f
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/llava_instruct_dataset.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/video_instruct_dataset.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/video_instruct_dataset.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..95aa055d472b8859505b479451add938d6da0eea
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/video_instruct_dataset.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/__pycache__/webvid_datasets.cpython-39.pyc b/global_local/datasets/datasets/__pycache__/webvid_datasets.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..477bb1e33127a704ad59af607b408f10b5d4c227
Binary files /dev/null and b/global_local/datasets/datasets/__pycache__/webvid_datasets.cpython-39.pyc differ
diff --git a/global_local/datasets/datasets/base_dataset.py b/global_local/datasets/datasets/base_dataset.py
new file mode 100644
index 0000000000000000000000000000000000000000..ae2a8d0e21370129c0182cddc427eb293bbe5982
--- /dev/null
+++ b/global_local/datasets/datasets/base_dataset.py
@@ -0,0 +1,68 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import json
+from typing import Iterable
+
+from torch.utils.data import Dataset, ConcatDataset
+from torch.utils.data.dataloader import default_collate
+
+
+class BaseDataset(Dataset):
+ def __init__(
+ self, vis_processor=None, text_processor=None, vis_root=None, ann_paths=[]
+ ):
+ """
+ vis_root (string): Root directory of images (e.g. coco/images/)
+ ann_root (string): directory to store the annotation file
+ """
+ self.vis_root = vis_root
+
+ self.annotation = []
+ for ann_path in ann_paths:
+ self.annotation.extend(json.load(open(ann_path, "r"))['annotations'])
+
+ self.vis_processor = vis_processor
+ self.text_processor = text_processor
+
+ self._add_instance_ids()
+
+ def __len__(self):
+ return len(self.annotation)
+
+ def collater(self, samples):
+ return default_collate(samples)
+
+ def set_processors(self, vis_processor, text_processor):
+ self.vis_processor = vis_processor
+ self.text_processor = text_processor
+
+ def _add_instance_ids(self, key="instance_id"):
+ for idx, ann in enumerate(self.annotation):
+ ann[key] = str(idx)
+
+
+class ConcatDataset(ConcatDataset):
+ def __init__(self, datasets: Iterable[Dataset]) -> None:
+ super().__init__(datasets)
+
+ def collater(self, samples):
+ # TODO For now only supports datasets with same underlying collater implementations
+
+ all_keys = set()
+ for s in samples:
+ all_keys.update(s)
+
+ shared_keys = all_keys
+ for s in samples:
+ shared_keys = shared_keys & set(s.keys())
+
+ samples_shared_keys = []
+ for s in samples:
+ samples_shared_keys.append({k: s[k] for k in s.keys() if k in shared_keys})
+
+ return self.datasets[0].collater(samples_shared_keys)
diff --git a/global_local/datasets/datasets/caption_datasets.py b/global_local/datasets/datasets/caption_datasets.py
new file mode 100644
index 0000000000000000000000000000000000000000..235f5e3710034996fbb6a0f9c582b66c199b58cb
--- /dev/null
+++ b/global_local/datasets/datasets/caption_datasets.py
@@ -0,0 +1,85 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import os
+from collections import OrderedDict
+
+from global_local.datasets.datasets.base_dataset import BaseDataset
+from PIL import Image
+
+
+class __DisplMixin:
+ def displ_item(self, index):
+ sample, ann = self.__getitem__(index), self.annotation[index]
+
+ return OrderedDict(
+ {
+ "file": ann["image"],
+ "caption": ann["caption"],
+ "image": sample["image"],
+ }
+ )
+
+
+class CaptionDataset(BaseDataset, __DisplMixin):
+ def __init__(self, vis_processor, text_processor, vis_root, ann_paths):
+ """
+ vis_root (string): Root directory of images (e.g. coco/images/)
+ ann_root (string): directory to store the annotation file
+ """
+ super().__init__(vis_processor, text_processor, vis_root, ann_paths)
+
+ self.img_ids = {}
+ n = 0
+ for ann in self.annotation:
+ img_id = ann["image_id"]
+ if img_id not in self.img_ids.keys():
+ self.img_ids[img_id] = n
+ n += 1
+
+ def __getitem__(self, index):
+
+ # TODO this assumes image input, not general enough
+ ann = self.annotation[index]
+
+ img_file = '{:0>12}.jpg'.format(ann["image_id"])
+ image_path = os.path.join(self.vis_root, img_file)
+ image = Image.open(image_path).convert("RGB")
+
+ image = self.vis_processor(image)
+ caption = self.text_processor(ann["caption"])
+
+ return {
+ "image": image,
+ "text_input": caption,
+ "image_id": self.img_ids[ann["image_id"]],
+ }
+
+
+class CaptionEvalDataset(BaseDataset, __DisplMixin):
+ def __init__(self, vis_processor, text_processor, vis_root, ann_paths):
+ """
+ vis_root (string): Root directory of images (e.g. coco/images/)
+ ann_root (string): directory to store the annotation file
+ split (string): val or test
+ """
+ super().__init__(vis_processor, text_processor, vis_root, ann_paths)
+
+ def __getitem__(self, index):
+
+ ann = self.annotation[index]
+
+ image_path = os.path.join(self.vis_root, ann["image"])
+ image = Image.open(image_path).convert("RGB")
+
+ image = self.vis_processor(image)
+
+ return {
+ "image": image,
+ "image_id": ann["image_id"],
+ "instance_id": ann["instance_id"],
+ }
diff --git a/global_local/datasets/datasets/cc_sbu_dataset.py b/global_local/datasets/datasets/cc_sbu_dataset.py
new file mode 100644
index 0000000000000000000000000000000000000000..cc9f64fc4819d64ce1f1b2b970b8c00c2c254a2e
--- /dev/null
+++ b/global_local/datasets/datasets/cc_sbu_dataset.py
@@ -0,0 +1,49 @@
+import os
+from PIL import Image
+import webdataset as wds
+from global_local.datasets.datasets.base_dataset import BaseDataset
+from global_local.datasets.datasets.caption_datasets import CaptionDataset
+
+
+class CCSBUDataset(BaseDataset):
+ def __init__(self, vis_processor, text_processor, location):
+ super().__init__(vis_processor=vis_processor, text_processor=text_processor)
+
+ self.inner_dataset = wds.DataPipeline(
+ wds.ResampledShards(location),
+ wds.tarfile_to_samples(handler=wds.warn_and_continue),
+ wds.shuffle(1000, handler=wds.warn_and_continue),
+ wds.decode("pilrgb", handler=wds.warn_and_continue),
+ wds.to_tuple("jpg", "json", handler=wds.warn_and_continue),
+ wds.map_tuple(self.vis_processor, handler=wds.warn_and_continue),
+ wds.map(self.to_dict, handler=wds.warn_and_continue),
+ )
+
+ def to_dict(self, sample):
+ return {
+ "image": sample[0],
+ "text_input": self.text_processor(sample[1]["caption"]),
+ "type":'image',
+ }
+
+
+class CCSBUAlignDataset(CaptionDataset):
+
+ def __getitem__(self, index):
+
+ # TODO this assumes image input, not general enough
+ ann = self.annotation[index]
+
+ img_file = '{}.jpg'.format(ann["image_id"])
+ image_path = os.path.join(self.vis_root, img_file)
+ image = Image.open(image_path).convert("RGB")
+
+ image = self.vis_processor(image)
+ caption = ann["caption"]
+
+ return {
+ "image": image,
+ "text_input": caption,
+ "image_id": self.img_ids[ann["image_id"]],
+ "type":'image',
+ }
\ No newline at end of file
diff --git a/global_local/datasets/datasets/dataloader_utils.py b/global_local/datasets/datasets/dataloader_utils.py
new file mode 100644
index 0000000000000000000000000000000000000000..fdff93597ba9add53a06a7cd30b62bd5bb971e8e
--- /dev/null
+++ b/global_local/datasets/datasets/dataloader_utils.py
@@ -0,0 +1,162 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import time
+import random
+import torch
+from global_local.datasets.data_utils import move_to_cuda
+from torch.utils.data import DataLoader
+
+
+class MultiIterLoader:
+ """
+ A simple wrapper for iterating over multiple iterators.
+
+ Args:
+ loaders (List[Loader]): List of Iterator loaders.
+ ratios (List[float]): List of ratios to sample from each loader. If None, all loaders are sampled uniformly.
+ """
+
+ def __init__(self, loaders, ratios=None):
+ # assert all loaders has __next__ method
+ for loader in loaders:
+ assert hasattr(
+ loader, "__next__"
+ ), "Loader {} has no __next__ method.".format(loader)
+
+ if ratios is None:
+ ratios = [1.0] * len(loaders)
+ else:
+ assert len(ratios) == len(loaders)
+ ratios = [float(ratio) / sum(ratios) for ratio in ratios]
+
+ self.loaders = loaders
+ self.ratios = ratios
+
+ def __next__(self):
+ # random sample from each loader by ratio
+ loader_idx = random.choices(range(len(self.loaders)), self.ratios, k=1)[0]
+ return next(self.loaders[loader_idx])
+
+
+class PrefetchLoader(object):
+ """
+ Modified from https://github.com/ChenRocks/UNITER.
+
+ overlap compute and cuda data transfer
+ (copied and then modified from nvidia apex)
+ """
+
+ def __init__(self, loader):
+ self.loader = loader
+ self.stream = torch.cuda.Stream()
+
+ def __iter__(self):
+ loader_it = iter(self.loader)
+ self.preload(loader_it)
+ batch = self.next(loader_it)
+ while batch is not None:
+ is_tuple = isinstance(batch, tuple)
+ if is_tuple:
+ task, batch = batch
+
+ if is_tuple:
+ yield task, batch
+ else:
+ yield batch
+ batch = self.next(loader_it)
+
+ def __len__(self):
+ return len(self.loader)
+
+ def preload(self, it):
+ try:
+ self.batch = next(it)
+ except StopIteration:
+ self.batch = None
+ return
+ # if record_stream() doesn't work, another option is to make sure
+ # device inputs are created on the main stream.
+ # self.next_input_gpu = torch.empty_like(self.next_input,
+ # device='cuda')
+ # self.next_target_gpu = torch.empty_like(self.next_target,
+ # device='cuda')
+ # Need to make sure the memory allocated for next_* is not still in use
+ # by the main stream at the time we start copying to next_*:
+ # self.stream.wait_stream(torch.cuda.current_stream())
+ with torch.cuda.stream(self.stream):
+ self.batch = move_to_cuda(self.batch)
+ # more code for the alternative if record_stream() doesn't work:
+ # copy_ will record the use of the pinned source tensor in this
+ # side stream.
+ # self.next_input_gpu.copy_(self.next_input, non_blocking=True)
+ # self.next_target_gpu.copy_(self.next_target, non_blocking=True)
+ # self.next_input = self.next_input_gpu
+ # self.next_target = self.next_target_gpu
+
+ def next(self, it):
+ torch.cuda.current_stream().wait_stream(self.stream)
+ batch = self.batch
+ if batch is not None:
+ record_cuda_stream(batch)
+ self.preload(it)
+ return batch
+
+ def __getattr__(self, name):
+ method = self.loader.__getattribute__(name)
+ return method
+
+
+def record_cuda_stream(batch):
+ if isinstance(batch, torch.Tensor):
+ batch.record_stream(torch.cuda.current_stream())
+ elif isinstance(batch, list) or isinstance(batch, tuple):
+ for t in batch:
+ record_cuda_stream(t)
+ elif isinstance(batch, dict):
+ for t in batch.values():
+ record_cuda_stream(t)
+ else:
+ pass
+
+
+class IterLoader:
+ """
+ A wrapper to convert DataLoader as an infinite iterator.
+
+ Modified from:
+ https://github.com/open-mmlab/mmcv/blob/master/mmcv/runner/iter_based_runner.py
+ """
+
+ def __init__(self, dataloader: DataLoader, use_distributed: bool = False):
+ self._dataloader = dataloader
+ self.iter_loader = iter(self._dataloader)
+ self._use_distributed = use_distributed
+ self._epoch = 0
+
+ @property
+ def epoch(self) -> int:
+ return self._epoch
+
+ def __next__(self):
+ try:
+ data = next(self.iter_loader)
+ except StopIteration:
+ self._epoch += 1
+ if hasattr(self._dataloader.sampler, "set_epoch") and self._use_distributed:
+ self._dataloader.sampler.set_epoch(self._epoch)
+ time.sleep(2) # Prevent possible deadlock during epoch transition
+ self.iter_loader = iter(self._dataloader)
+ data = next(self.iter_loader)
+
+ return data
+
+ def __iter__(self):
+ return self
+
+ def __len__(self):
+ return len(self._dataloader)
diff --git a/global_local/datasets/datasets/laion_dataset.py b/global_local/datasets/datasets/laion_dataset.py
new file mode 100644
index 0000000000000000000000000000000000000000..6f76d4926f1352ef68ade942e62382fc9b35bcc3
--- /dev/null
+++ b/global_local/datasets/datasets/laion_dataset.py
@@ -0,0 +1,31 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import webdataset as wds
+from global_local.datasets.datasets.base_dataset import BaseDataset
+
+
+class LaionDataset(BaseDataset):
+ def __init__(self, vis_processor, text_processor, location):
+ super().__init__(vis_processor=vis_processor, text_processor=text_processor)
+
+ self.inner_dataset = wds.DataPipeline(
+ wds.ResampledShards(location),
+ wds.tarfile_to_samples(handler=wds.warn_and_continue),
+ wds.shuffle(1000, handler=wds.warn_and_continue),
+ wds.decode("pilrgb", handler=wds.warn_and_continue),
+ wds.to_tuple("jpg", "json", handler=wds.warn_and_continue),
+ wds.map_tuple(self.vis_processor, handler=wds.warn_and_continue),
+ wds.map(self.to_dict, handler=wds.warn_and_continue),
+ )
+
+ def to_dict(self, sample):
+ return {
+ "image": sample[0],
+ "text_input": self.text_processor(sample[1]["caption"]),
+ }
+
diff --git a/global_local/datasets/datasets/llava_instruct_dataset.py b/global_local/datasets/datasets/llava_instruct_dataset.py
new file mode 100644
index 0000000000000000000000000000000000000000..f9bea8f9d6639563d433da6ed767ed6c7183167f
--- /dev/null
+++ b/global_local/datasets/datasets/llava_instruct_dataset.py
@@ -0,0 +1,312 @@
+import os
+from global_local.datasets.datasets.base_dataset import BaseDataset
+from global_local.datasets.datasets.caption_datasets import CaptionDataset
+import pandas as pd
+import decord
+from decord import VideoReader
+import random
+import torch
+from torch.utils.data.dataloader import default_collate
+from PIL import Image
+from typing import Dict, Optional, Sequence
+import transformers
+import pathlib
+import json
+from transformers import AutoTokenizer, AutoModelForCausalLM, LlamaTokenizer
+from global_local.conversation.conversation_video import Conversation,SeparatorStyle
+DEFAULT_IMAGE_PATCH_TOKEN = ''
+DEFAULT_IMAGE_TOKEN = ""
+import copy
+from global_local.processors import transforms_video,AlproVideoTrainProcessor
+IGNORE_INDEX = -100
+image_conversation = Conversation(
+ system="",
+ roles=("Human", "Assistant"),
+ messages=[],
+ offset=0,
+ sep_style=SeparatorStyle.SINGLE,
+ sep="###",
+)
+llama_v2_image_conversation = Conversation(
+ system=" ",
+ roles=("USER", "ASSISTANT"),
+ messages=(),
+ offset=0,
+ sep_style=SeparatorStyle.LLAMA_2,
+ sep="",
+ sep2="",
+)
+IGNORE_INDEX = -100
+
+class Instruct_Dataset(BaseDataset):
+ def __init__(self, vis_processor, text_processor, vis_root, ann_root,num_video_query_token=32,tokenizer_name = '/mnt/workspace/ckpt/vicuna-13b/',data_type = 'image', model_type='vicuna'):
+ """
+ vis_root (string): Root directory of Llava images (e.g. webvid_eval/video/)
+ ann_root (string): Root directory of video (e.g. webvid_eval/annotations/)
+ split (string): val or test
+ """
+ super().__init__(vis_processor=vis_processor, text_processor=text_processor)
+
+ data_path = pathlib.Path(ann_root)
+ with data_path.open(encoding='utf-8') as f:
+ self.annotation = json.load(f)
+
+ self.vis_root = vis_root
+ self.resize_size = 224
+ self.num_frm = 8
+ self.tokenizer = LlamaTokenizer.from_pretrained(tokenizer_name, use_fast=False)
+ self.tokenizer.pad_token = self.tokenizer.unk_token
+ self.tokenizer.add_tokens([DEFAULT_IMAGE_PATCH_TOKEN], special_tokens=True)
+ self.num_video_query_token = num_video_query_token
+ self.IMAGE_PATCH_TOKEN_ID = self.tokenizer.get_vocab()[DEFAULT_IMAGE_PATCH_TOKEN]
+
+ self.transform = AlproVideoTrainProcessor(
+ image_size=self.resize_size, n_frms = self.num_frm
+ ).transform
+ self.data_type = data_type
+ self.model_type = model_type
+
+ def _get_image_path(self, sample):
+ rel_video_fp ='COCO_train2014_' + sample['image']
+ full_video_fp = os.path.join(self.vis_root, rel_video_fp)
+ return full_video_fp
+
+ def __getitem__(self, index):
+ num_retries = 10 # skip error videos
+ for _ in range(num_retries):
+ try:
+ sample = self.annotation[index]
+
+ image_path = self._get_image_path(sample)
+ conversation_list = sample['conversations']
+ image = Image.open(image_path).convert("RGB")
+
+ image = self.vis_processor(image)
+ # text = self.text_processor(text)
+ sources = preprocess_multimodal(copy.deepcopy(conversation_list), None, cur_token_len=self.num_video_query_token)
+ if self.model_type =='vicuna':
+ data_dict = preprocess(
+ sources,
+ self.tokenizer)
+ elif self.model_type =='llama_v2':
+ data_dict = preprocess_for_llama_v2(
+ sources,
+ self.tokenizer)
+ else:
+ print('not support')
+ raise('not support')
+ data_dict = dict(input_ids=data_dict["input_ids"][0],
+ labels=data_dict["labels"][0])
+
+ # image exist in the data
+ data_dict['image'] = image
+ except:
+ print(f"Failed to load examples with image: {image_path}. "
+ f"Will randomly sample an example as a replacement.")
+ index = random.randint(0, len(self) - 1)
+ continue
+ break
+ else:
+ raise RuntimeError(f"Failed to fetch image after {num_retries} retries.")
+ # "image_id" is kept to stay compatible with the COCO evaluation format
+ return {
+ "image": image,
+ "text_input": data_dict["input_ids"],
+ "labels": data_dict["labels"],
+ "type":'image',
+ }
+
+ def __len__(self):
+ return len(self.annotation)
+
+ def collater(self, instances):
+ input_ids, labels = tuple([instance[key] for instance in instances]
+ for key in ("text_input", "labels"))
+ input_ids = torch.nn.utils.rnn.pad_sequence(
+ input_ids,
+ batch_first=True,
+ padding_value=self.tokenizer.pad_token_id)
+ labels = torch.nn.utils.rnn.pad_sequence(labels,
+ batch_first=True,
+ padding_value=IGNORE_INDEX)
+ batch = dict(
+ input_ids=input_ids,
+ labels=labels,
+ attention_mask=input_ids.ne(self.tokenizer.pad_token_id),
+ )
+
+ if 'image' in instances[0]:
+ images = [instance['image'] for instance in instances]
+ if all(x is not None and x.shape == images[0].shape for x in images):
+ batch['images'] = torch.stack(images)
+ else:
+ batch['images'] = images
+ batch['conv_type'] = 'multi'
+ return batch
+
+
+def preprocess_multimodal(
+ conversation_list: Sequence[str],
+ multimodal_cfg: dict,
+ cur_token_len: int,
+) -> Dict:
+ # 将conversational list中
+ is_multimodal = True
+ # image_token_len = multimodal_cfg['image_token_len']
+ image_token_len = cur_token_len
+
+ for sentence in conversation_list:
+ replace_token = ''+DEFAULT_IMAGE_PATCH_TOKEN * image_token_len+''
+ sentence["value"] = sentence["value"].replace(DEFAULT_IMAGE_TOKEN, replace_token)
+
+ return [conversation_list]
+
+def _add_speaker_and_signal(header, source, get_conversation=True):
+ """Add speaker and start/end signal on each round."""
+ BEGIN_SIGNAL = "###"
+ END_SIGNAL = "\n"
+ conversation = header
+ for sentence in source:
+ from_str = sentence["from"]
+ if from_str.lower() == "human":
+ from_str = image_conversation.roles[0]
+ elif from_str.lower() == "gpt":
+ from_str = image_conversation.roles[1]
+ else:
+ from_str = 'unknown'
+ sentence["value"] = (BEGIN_SIGNAL + from_str + ": " +
+ sentence["value"] + END_SIGNAL)
+ if get_conversation:
+ conversation += sentence["value"]
+ conversation += BEGIN_SIGNAL
+ return conversation
+
+def _tokenize_fn(strings: Sequence[str],
+ tokenizer: transformers.PreTrainedTokenizer) -> Dict:
+ """Tokenize a list of strings."""
+ tokenized_list = [
+ tokenizer(
+ text,
+ return_tensors="pt",
+ padding="longest",
+ max_length=512,
+ truncation=True,
+ ) for text in strings
+ ]
+ input_ids = labels = [
+ tokenized.input_ids[0] for tokenized in tokenized_list
+ ]
+ input_ids_lens = labels_lens = [
+ tokenized.input_ids.ne(tokenizer.pad_token_id).sum().item()
+ for tokenized in tokenized_list
+ ]
+ return dict(
+ input_ids=input_ids,
+ labels=labels,
+ input_ids_lens=input_ids_lens,
+ labels_lens=labels_lens,
+ )
+
+def preprocess(
+ sources: Sequence[str],
+ tokenizer: transformers.PreTrainedTokenizer,
+) -> Dict:
+ """
+ Given a list of sources, each is a conversation list. This transform:
+ 1. Add signal '### ' at the beginning each sentence, with end signal '\n';
+ 2. Concatenate conversations together;
+ 3. Tokenize the concatenated conversation;
+ 4. Make a deepcopy as the target. Mask human words with IGNORE_INDEX.
+ """
+ # add end signal and concatenate together
+ conversations = []
+ for source in sources:
+ header = f"{image_conversation.system}\n\n"
+ conversation = _add_speaker_and_signal(header, source)
+ conversations.append(conversation)
+ # tokenize conversations
+ conversations_tokenized = _tokenize_fn(conversations, tokenizer)
+ input_ids = conversations_tokenized["input_ids"]
+ targets = copy.deepcopy(input_ids)
+ for target, source in zip(targets, sources):
+ tokenized_lens = _tokenize_fn([header] + [s["value"] for s in source],
+ tokenizer)["input_ids_lens"]
+ speakers = [sentence["from"] for sentence in source]
+ _mask_targets(target, tokenized_lens, speakers)
+
+ return dict(input_ids=input_ids, labels=targets)
+
+def preprocess_for_llama_v2(
+ sources: Sequence[str],
+ tokenizer: transformers.PreTrainedTokenizer,
+) -> Dict:
+ """
+ Given a list of sources, each is a conversation list. This transform:
+ 1. Add signal '### ' at the beginning each sentence, with end signal '\n';
+ 2. Concatenate conversations together;
+ 3. Tokenize the concatenated conversation;
+ 4. Make a deepcopy as the target. Mask human words with IGNORE_INDEX.
+ """
+ # add end signal and concatenate together
+ conversations = []
+ conv = copy.deepcopy(llama_v2_image_conversation.copy())
+ roles = {"human": conv.roles[0], "gpt": conv.roles[1]}
+ for source in sources:
+ # [INST] <>\n{system_prompt}\n<>\n\n
+ header = f"[INST] <>\n{conv.system}\n>\n\n"
+
+ if roles[source[0]["from"]] != conv.roles[0]:
+ # Skip the first one if it is not from human
+ source = source[1:]
+ conv.messages = []
+ for j, sentence in enumerate(source):
+ role = roles[sentence["from"]]
+ assert role == conv.roles[j % 2]
+ conv.append_message(role, sentence["value"])
+ conversations.append(conv.get_prompt())
+
+ input_ids = tokenizer(
+ conversations,
+ return_tensors="pt",
+ padding="longest",
+ max_length=512,
+ truncation=True,
+ ).input_ids
+ targets = copy.deepcopy(input_ids)
+
+
+ sep = "[/INST] "
+ for conversation, target in zip(conversations, targets):
+ # total_len = int(target.ne(tokenizer.pad_token_id).sum())
+ rounds = conversation.split(conv.sep2)
+ cur_len = 1
+ target[:cur_len] = IGNORE_INDEX
+ for i, rou in enumerate(rounds):
+ if rou == "":
+ break
+
+ parts = rou.split(sep)
+ if len(parts) != 2:
+ break
+ parts[0] += sep
+
+
+ round_len = len(tokenizer(rou).input_ids)
+ instruction_len = len(tokenizer(parts[0]).input_ids) - 2 # 为什么减去2,speical token 的数目
+
+ target[cur_len : cur_len + instruction_len] = IGNORE_INDEX
+
+ cur_len += round_len
+ target[cur_len:] = IGNORE_INDEX
+
+ return dict(input_ids=input_ids, labels=targets)
+
+def _mask_targets(target, tokenized_lens, speakers):
+ # cur_idx = 0
+ cur_idx = tokenized_lens[0]
+ tokenized_lens = tokenized_lens[1:]
+ target[:cur_idx] = IGNORE_INDEX
+ for tokenized_len, speaker in zip(tokenized_lens, speakers):
+ if speaker == "human":
+ target[cur_idx+2:cur_idx + tokenized_len] = IGNORE_INDEX
+ cur_idx += tokenized_len
diff --git a/global_local/datasets/datasets/video_instruct_dataset.py b/global_local/datasets/datasets/video_instruct_dataset.py
new file mode 100644
index 0000000000000000000000000000000000000000..655df9dcda0097bed6c4842846cc505eb7ba1447
--- /dev/null
+++ b/global_local/datasets/datasets/video_instruct_dataset.py
@@ -0,0 +1,335 @@
+import os
+from global_local.datasets.datasets.base_dataset import BaseDataset
+from global_local.datasets.datasets.caption_datasets import CaptionDataset
+import pandas as pd
+import decord
+from decord import VideoReader
+import random
+import torch
+from torch.utils.data.dataloader import default_collate
+from PIL import Image
+from typing import Dict, Optional, Sequence
+import transformers
+import pathlib
+import json
+from transformers import AutoTokenizer, AutoModelForCausalLM, LlamaTokenizer
+import copy
+from global_local.processors import transforms_video,AlproVideoTrainProcessor
+from torchvision import transforms
+from global_local.processors.video_processor import ToTHWC,ToUint8,load_video
+from global_local.conversation.conversation_video import Conversation,SeparatorStyle
+
+DEFAULT_IMAGE_PATCH_TOKEN = ''
+video_conversation = Conversation(
+ system="",
+ roles=("Human", "Assistant"),
+ messages=[],
+ offset=0,
+ sep_style=SeparatorStyle.SINGLE,
+ sep="###",
+)
+llama_v2_video_conversation = Conversation(
+ system=" ",
+ roles=("USER", "ASSISTANT"),
+ messages=(),
+ offset=0,
+ sep_style=SeparatorStyle.LLAMA_2,
+ sep="",
+ sep2="",
+)
+IGNORE_INDEX = -100
+
+class Video_Instruct_Dataset(BaseDataset):
+ def __init__(self, vis_processor, text_processor, vis_root, ann_root,num_video_query_token=32,tokenizer_name = '/mnt/workspace/ckpt/vicuna-13b/',data_type = 'video', model_type='vicuna'):
+ """
+ vis_root (string): Root directory of Llava images (e.g. webvid_eval/video/)
+ ann_root (string): Root directory of video (e.g. webvid_eval/annotations/)
+ split (string): val or test
+ """
+ super().__init__(vis_processor=vis_processor, text_processor=text_processor)
+
+ data_path = pathlib.Path(ann_root)
+ with data_path.open(encoding='utf-8') as f:
+ self.annotation = json.load(f)
+
+ self.num_video_query_token = num_video_query_token
+ self.vis_root = vis_root
+ self.resize_size = 224
+ self.num_frm = 8
+ self.tokenizer = LlamaTokenizer.from_pretrained(tokenizer_name, use_fast=False)
+ self.tokenizer.pad_token = self.tokenizer.unk_token
+ self.tokenizer.add_tokens([DEFAULT_IMAGE_PATCH_TOKEN], special_tokens=True)
+ self.IMAGE_PATCH_TOKEN_ID = self.tokenizer.get_vocab()[DEFAULT_IMAGE_PATCH_TOKEN]
+
+ self.transform = AlproVideoTrainProcessor(
+ image_size=self.resize_size, n_frms = self.num_frm
+ ).transform
+ self.data_type = data_type
+ self.model_type = model_type
+
+ def _get_video_path(self, sample):
+ rel_video_fp = sample['video']
+ full_video_fp = os.path.join(self.vis_root, rel_video_fp)
+ return full_video_fp
+
+ def __getitem__(self, index):
+ num_retries = 10 # skip error videos
+ for _ in range(num_retries):
+ try:
+ sample = self.annotation[index]
+
+ video_path = self._get_video_path(sample)
+ conversation_list = sample['QA']
+
+ video, msg = load_video(
+ video_path=video_path,
+ n_frms=self.num_frm,
+ height=self.resize_size,
+ width=self.resize_size,
+ sampling ="uniform", return_msg = True
+ )
+ video = self.transform(video)
+ if 'cn' in self.data_type:
+ msg = ""
+ # 添加视频,以及msg到convsation list 0
+ sources = preprocess_multimodal(copy.deepcopy(conversation_list), None, cur_token_len=self.num_video_query_token,msg = msg)
+ new_sources = convert_source_vicuna_format(sources)
+
+ if self.model_type =='vicuna':
+ data_dict = preprocess(
+ new_sources,
+ self.tokenizer)
+ elif self.model_type =='llama_v2':
+ data_dict = preprocess_for_llama_v2(
+ new_sources,
+ self.tokenizer)
+ else:
+ print('not support')
+ raise('not support')
+ data_dict = dict(input_ids=data_dict["input_ids"][0],
+ labels=data_dict["labels"][0])
+ # image exist in the data
+ data_dict['image'] = video
+ except:
+ print(f"Failed to load examples with video: {video_path}. "
+ f"Will randomly sample an example as a replacement.")
+ index = random.randint(0, len(self) - 1)
+ continue
+ break
+ else:
+ raise RuntimeError(f"Failed to fetch video after {num_retries} retries.")
+ # "image_id" is kept to stay compatible with the COCO evaluation format
+ return {
+ "image": video,
+ "text_input": data_dict["input_ids"],
+ "labels": data_dict["labels"],
+ "type":'video',
+ }
+
+ def __len__(self):
+ return len(self.annotation)
+
+ def collater(self, instances):
+ input_ids, labels = tuple([instance[key] for instance in instances]
+ for key in ("text_input", "labels"))
+ input_ids = torch.nn.utils.rnn.pad_sequence(
+ input_ids,
+ batch_first=True,
+ padding_value=self.tokenizer.pad_token_id)
+ labels = torch.nn.utils.rnn.pad_sequence(labels,
+ batch_first=True,
+ padding_value=IGNORE_INDEX)
+ batch = dict(
+ input_ids=input_ids,
+ labels=labels,
+ attention_mask=input_ids.ne(self.tokenizer.pad_token_id),
+ )
+
+ if 'image' in instances[0]:
+ images = [instance['image'] for instance in instances]
+ if all(x is not None and x.shape == images[0].shape for x in images):
+ batch['images'] = torch.stack(images)
+ else:
+ batch['images'] = images
+ batch['conv_type'] = 'multi'
+ return batch
+
+def convert_source_vicuna_format(sources):
+ new_sources = []
+ for source in sources:
+ new_source = []
+ for i, sentence in enumerate(source):
+ role_0_msg = sentence['q']
+ role_1_msg = sentence['a']
+ new_source.append({
+ 'from':'human',
+ 'value': role_0_msg,
+ })
+ new_source.append({
+ 'from':'gpt',
+ 'value': role_1_msg,
+ })
+ new_sources.append(new_source)
+ return new_sources
+
+def preprocess_multimodal(
+ conversation_list: Sequence[str],
+ multimodal_cfg: dict,
+ cur_token_len: int,
+ msg=''
+) -> Dict:
+ # 将conversational list中
+ is_multimodal = True
+ # image_token_len = multimodal_cfg['image_token_len']
+ image_token_len = cur_token_len
+ conversation_list[0]["q"] = " " + msg + conversation_list[0]["q"]
+ return [conversation_list]
+
+def _add_speaker_and_signal(header, source, get_conversation=True):
+ """Add speaker and start/end signal on each round."""
+ BEGIN_SIGNAL = "###"
+ END_SIGNAL = "\n"
+ conversation = header
+ for sentence in source:
+ from_str = sentence["from"]
+ if from_str.lower() == "human":
+ from_str = video_conversation.roles[0]
+ elif from_str.lower() == "gpt":
+ from_str = video_conversation.roles[1]
+ else:
+ from_str = 'unknown'
+ sentence["value"] = (BEGIN_SIGNAL + from_str + ": " +
+ sentence["value"] + END_SIGNAL)
+ if get_conversation:
+ conversation += sentence["value"]
+ conversation += BEGIN_SIGNAL
+ return conversation
+
+def _tokenize_fn(strings: Sequence[str],
+ tokenizer: transformers.PreTrainedTokenizer) -> Dict:
+ """Tokenize a list of strings."""
+ tokenized_list = [
+ tokenizer(
+ text,
+ return_tensors="pt",
+ padding="longest",
+ max_length=512,
+ truncation=True,
+ ) for text in strings
+ ]
+ input_ids = labels = [
+ tokenized.input_ids[0] for tokenized in tokenized_list
+ ]
+ input_ids_lens = labels_lens = [
+ tokenized.input_ids.ne(tokenizer.pad_token_id).sum().item()
+ for tokenized in tokenized_list
+ ]
+ return dict(
+ input_ids=input_ids,
+ labels=labels,
+ input_ids_lens=input_ids_lens,
+ labels_lens=labels_lens,
+ )
+
+def preprocess(
+ sources: Sequence[str],
+ tokenizer: transformers.PreTrainedTokenizer,
+) -> Dict:
+ """
+ Given a list of sources, each is a conversation list. This transform:
+ 1. Add signal '### ' at the beginning each sentence, with end signal '\n';
+ 2. Concatenate conversations together;
+ 3. Tokenize the concatenated conversation;
+ 4. Make a deepcopy as the target. Mask human words with IGNORE_INDEX.
+ """
+ # add end signal and concatenate together
+ conversations = []
+ for source in sources:
+ header = f"{video_conversation.system}\n\n"
+ conversation = _add_speaker_and_signal(header, source)
+ conversations.append(conversation)
+ # tokenize conversations
+ conversations_tokenized = _tokenize_fn(conversations, tokenizer)
+ input_ids = conversations_tokenized["input_ids"]
+ targets = copy.deepcopy(input_ids)
+ for target, source in zip(targets, sources):
+ tokenized_lens = _tokenize_fn([header] + [s["value"] for s in source],
+ tokenizer)["input_ids_lens"]
+ speakers = [sentence["from"] for sentence in source]
+ _mask_targets(target, tokenized_lens, speakers)
+
+ return dict(input_ids=input_ids, labels=targets)
+
+def preprocess_for_llama_v2(
+ sources: Sequence[str],
+ tokenizer: transformers.PreTrainedTokenizer,
+) -> Dict:
+ """
+ Given a list of sources, each is a conversation list. This transform:
+ 1. Add signal '### ' at the beginning each sentence, with end signal '\n';
+ 2. Concatenate conversations together;
+ 3. Tokenize the concatenated conversation;
+ 4. Make a deepcopy as the target. Mask human words with IGNORE_INDEX.
+ """
+ # add end signal and concatenate together
+ conversations = []
+ conv = copy.deepcopy(video_conversation.copy())
+ roles = {"human": conv.roles[0], "gpt": conv.roles[1]}
+ for source in sources:
+ # [INST] <>\n{system_prompt}\n<>\n\n
+ header = f"[INST] <>\n{conv.system}\n>\n\n"
+
+ if roles[source[0]["from"]] != conv.roles[0]:
+ # Skip the first one if it is not from human
+ source = source[1:]
+ conv.messages = []
+ for j, sentence in enumerate(source):
+ role = roles[sentence["from"]]
+ assert role == conv.roles[j % 2]
+ conv.append_message(role, sentence["value"])
+ conversations.append(conv.get_prompt())
+
+ input_ids = tokenizer(
+ conversations,
+ return_tensors="pt",
+ padding="longest",
+ max_length=512,
+ truncation=True,
+ ).input_ids
+ targets = copy.deepcopy(input_ids)
+
+
+ sep = "[/INST] "
+ for conversation, target in zip(conversations, targets):
+ # total_len = int(target.ne(tokenizer.pad_token_id).sum())
+ rounds = conversation.split(conv.sep2)
+ cur_len = 1
+ target[:cur_len] = IGNORE_INDEX
+ for i, rou in enumerate(rounds):
+ if rou == "":
+ break
+
+ parts = rou.split(sep)
+ if len(parts) != 2:
+ break
+ parts[0] += sep
+
+
+ round_len = len(tokenizer(rou).input_ids)
+ instruction_len = len(tokenizer(parts[0]).input_ids) - 2 # 为什么减去2,speical token 的数目
+
+ target[cur_len : cur_len + instruction_len] = IGNORE_INDEX
+
+ cur_len += round_len
+ target[cur_len:] = IGNORE_INDEX
+
+ return dict(input_ids=input_ids, labels=targets)
+def _mask_targets(target, tokenized_lens, speakers):
+ # cur_idx = 0
+ cur_idx = tokenized_lens[0]
+ tokenized_lens = tokenized_lens[1:]
+ target[:cur_idx] = IGNORE_INDEX
+ for tokenized_len, speaker in zip(tokenized_lens, speakers):
+ if speaker == "human":
+ target[cur_idx+2:cur_idx + tokenized_len] = IGNORE_INDEX
+ cur_idx += tokenized_len
diff --git a/global_local/datasets/datasets/webvid_datasets.py b/global_local/datasets/datasets/webvid_datasets.py
new file mode 100644
index 0000000000000000000000000000000000000000..21df4c3764847f097b6111f286da915d7486e6d9
--- /dev/null
+++ b/global_local/datasets/datasets/webvid_datasets.py
@@ -0,0 +1,122 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import os
+from global_local.datasets.datasets.base_dataset import BaseDataset
+from global_local.datasets.datasets.caption_datasets import CaptionDataset
+import pandas as pd
+import decord
+from decord import VideoReader
+import random
+import torch
+from torch.utils.data.dataloader import default_collate
+class WebvidDataset(BaseDataset):
+ def __init__(self, vis_processor, text_processor, vis_root, ann_root):
+ """
+ vis_root (string): Root directory of video (e.g. webvid_eval/video/)
+ ann_root (string): Root directory of video (e.g. webvid_eval/annotations/)
+ split (string): val or test
+ """
+ super().__init__(vis_processor=vis_processor, text_processor=text_processor)
+
+
+ # 读取一个路径下所有的
+
+ ts_df = []
+ for file_name in os.listdir(ann_root):
+ if file_name.endswith('.csv'):
+ df = pd.read_csv(os.path.join(ann_root, file_name))
+ ts_df.append(df)
+
+ merged_df = pd.concat(ts_df)
+ self.annotation = merged_df
+ self.vis_root = vis_root
+ self.resize_size = 224
+ self.num_frm = 8
+ self.frm_sampling_strategy = 'headtail'
+
+ def _get_video_path(self, sample):
+ rel_video_fp = os.path.join(sample['page_dir'], str(sample['videoid']) + '.mp4')
+ full_video_fp = os.path.join(self.vis_root, rel_video_fp)
+ return full_video_fp
+
+ def __getitem__(self, index):
+ num_retries = 10 # skip error videos
+ for _ in range(num_retries):
+ sample = self.annotation.iloc[index]
+ sample_dict = sample.to_dict()
+ video_id = sample_dict['videoid']
+
+ if 'name' in sample_dict.keys():
+ text = sample_dict['name'].strip()
+ else:
+ raise NotImplementedError("Un-supported text annotation format.")
+
+ # fetch video
+ video_path = self._get_video_path(sample_dict)
+ # if os.path.exists(video_path):
+ try:
+ video = self.vis_processor(video_path)
+ except:
+ print(f"Failed to load examples with video: {video_path}. "
+ f"Will randomly sample an example as a replacement.")
+ index = random.randint(0, len(self) - 1)
+ continue
+ caption = self.text_processor(text)
+
+ # print(video.size())
+ if video is None or caption is None \
+ or video.size()!=torch.Size([3,self.vis_processor.n_frms,224,224]):
+ print(f"Failed to load examples with video: {video_path}. "
+ f"Will randomly sample an example as a replacement.")
+ index = random.randint(0, len(self) - 1)
+ continue
+ else:
+ break
+ else:
+ raise RuntimeError(f"Failed to fetch video after {num_retries} retries.")
+ # "image_id" is kept to stay compatible with the COCO evaluation format
+ return {
+ "image": video,
+ "text_input": caption,
+ "type":'video',
+ }
+
+ def __len__(self):
+ return len(self.annotation)
+
+ # def collater(self, samples):
+ # new_result = {}
+ # new_result['image'] = default_collate( [sample["image"] for sample in samples])
+ # new_result['text_input'] = default_collate( [sample["text_input"] for sample in samples])
+ # return new_result
+
+class WebvidDatasetEvalDataset(BaseDataset):
+ def __init__(self, vis_processor, text_processor, vis_root, ann_paths):
+ """
+ vis_root (string): Root directory of images (e.g. coco/images/)
+ ann_root (string): directory to store the annotation file
+ split (string): val or test
+ """
+ super().__init__(vis_processor, text_processor, vis_root, ann_paths)
+
+ def __getitem__(self, index):
+
+ ann = self.annotation[index]
+
+ vname = ann["video"]
+ video_path = os.path.join(self.vis_root, vname)
+
+ video = self.vis_processor(video_path)
+
+ return {
+ "video": video,
+ "image_id": ann["image_id"],
+ "instance_id": ann["instance_id"],
+ }
+
+
diff --git a/global_local/models/ImageBind/.assets/bird_audio.wav b/global_local/models/ImageBind/.assets/bird_audio.wav
new file mode 100644
index 0000000000000000000000000000000000000000..a98fc72b0df440fd10b3e54c87dfe0ffae0fa12e
Binary files /dev/null and b/global_local/models/ImageBind/.assets/bird_audio.wav differ
diff --git a/global_local/models/ImageBind/.assets/bird_image.jpg b/global_local/models/ImageBind/.assets/bird_image.jpg
new file mode 100644
index 0000000000000000000000000000000000000000..78b10ab1fe76f42e3dda1dc515e69312f02713d9
Binary files /dev/null and b/global_local/models/ImageBind/.assets/bird_image.jpg differ
diff --git a/global_local/models/ImageBind/.assets/car_audio.wav b/global_local/models/ImageBind/.assets/car_audio.wav
new file mode 100644
index 0000000000000000000000000000000000000000..b71b42a3a375b763521d08855f1a1eebb647a3d2
Binary files /dev/null and b/global_local/models/ImageBind/.assets/car_audio.wav differ
diff --git a/global_local/models/ImageBind/.assets/car_image.jpg b/global_local/models/ImageBind/.assets/car_image.jpg
new file mode 100644
index 0000000000000000000000000000000000000000..e33288eb765882c594f479bfb35d941fd51a19b1
Binary files /dev/null and b/global_local/models/ImageBind/.assets/car_image.jpg differ
diff --git a/global_local/models/ImageBind/.assets/dog_audio.wav b/global_local/models/ImageBind/.assets/dog_audio.wav
new file mode 100644
index 0000000000000000000000000000000000000000..71d69c77e92039d5906ed766d9c3ca4b181f9ffd
Binary files /dev/null and b/global_local/models/ImageBind/.assets/dog_audio.wav differ
diff --git a/global_local/models/ImageBind/.assets/dog_image.jpg b/global_local/models/ImageBind/.assets/dog_image.jpg
new file mode 100644
index 0000000000000000000000000000000000000000..a54bffa5c80869c6b96246ba29c9e2462c698e3b
Binary files /dev/null and b/global_local/models/ImageBind/.assets/dog_image.jpg differ
diff --git a/global_local/models/ImageBind/CODE_OF_CONDUCT.md b/global_local/models/ImageBind/CODE_OF_CONDUCT.md
new file mode 100644
index 0000000000000000000000000000000000000000..f913b6a55a6c5ab6e1224e11fc039c3d4c3b6283
--- /dev/null
+++ b/global_local/models/ImageBind/CODE_OF_CONDUCT.md
@@ -0,0 +1,80 @@
+# Code of Conduct
+
+## Our Pledge
+
+In the interest of fostering an open and welcoming environment, we as
+contributors and maintainers pledge to make participation in our project and
+our community a harassment-free experience for everyone, regardless of age, body
+size, disability, ethnicity, sex characteristics, gender identity and expression,
+level of experience, education, socio-economic status, nationality, personal
+appearance, race, religion, or sexual identity and orientation.
+
+## Our Standards
+
+Examples of behavior that contributes to creating a positive environment
+include:
+
+* Using welcoming and inclusive language
+* Being respectful of differing viewpoints and experiences
+* Gracefully accepting constructive criticism
+* Focusing on what is best for the community
+* Showing empathy towards other community members
+
+Examples of unacceptable behavior by participants include:
+
+* The use of sexualized language or imagery and unwelcome sexual attention or
+advances
+* Trolling, insulting/derogatory comments, and personal or political attacks
+* Public or private harassment
+* Publishing others' private information, such as a physical or electronic
+address, without explicit permission
+* Other conduct which could reasonably be considered inappropriate in a
+professional setting
+
+## Our Responsibilities
+
+Project maintainers are responsible for clarifying the standards of acceptable
+behavior and are expected to take appropriate and fair corrective action in
+response to any instances of unacceptable behavior.
+
+Project maintainers have the right and responsibility to remove, edit, or
+reject comments, commits, code, wiki edits, issues, and other contributions
+that are not aligned to this Code of Conduct, or to ban temporarily or
+permanently any contributor for other behaviors that they deem inappropriate,
+threatening, offensive, or harmful.
+
+## Scope
+
+This Code of Conduct applies within all project spaces, and it also applies when
+an individual is representing the project or its community in public spaces.
+Examples of representing a project or community include using an official
+project e-mail address, posting via an official social media account, or acting
+as an appointed representative at an online or offline event. Representation of
+a project may be further defined and clarified by project maintainers.
+
+This Code of Conduct also applies outside the project spaces when there is a
+reasonable belief that an individual's behavior may have a negative impact on
+the project or its community.
+
+## Enforcement
+
+Instances of abusive, harassing, or otherwise unacceptable behavior may be
+reported by contacting the project team at . All
+complaints will be reviewed and investigated and will result in a response that
+is deemed necessary and appropriate to the circumstances. The project team is
+obligated to maintain confidentiality with regard to the reporter of an incident.
+Further details of specific enforcement policies may be posted separately.
+
+Project maintainers who do not follow or enforce the Code of Conduct in good
+faith may face temporary or permanent repercussions as determined by other
+members of the project's leadership.
+
+## Attribution
+
+This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
+available at https://www.contributor-covenant.org/version/1/4/code-of-conduct.html
+
+[homepage]: https://www.contributor-covenant.org
+
+For answers to common questions about this code of conduct, see
+https://www.contributor-covenant.org/faq
\ No newline at end of file
diff --git a/global_local/models/ImageBind/CONTRIBUTING.md b/global_local/models/ImageBind/CONTRIBUTING.md
new file mode 100644
index 0000000000000000000000000000000000000000..63d0b751e8a00b606ddff92e2524faa3c90a63b0
--- /dev/null
+++ b/global_local/models/ImageBind/CONTRIBUTING.md
@@ -0,0 +1,31 @@
+# Contributing to ImageBind
+We want to make contributing to this project as easy and transparent as
+possible.
+
+## Pull Requests
+We actively welcome your pull requests.
+
+1. Fork the repo and create your branch from `main`.
+2. If you've added code that should be tested, add tests.
+3. If you've changed APIs, update the documentation.
+4. Ensure the test suite passes.
+5. Make sure your code lints.
+6. If you haven't already, complete the Contributor License Agreement ("CLA").
+
+## Contributor License Agreement ("CLA")
+In order to accept your pull request, we need you to submit a CLA. You only need
+to do this once to work on any of Meta's open source projects.
+
+Complete your CLA here:
+
+## Issues
+We use GitHub issues to track public bugs. Please ensure your description is
+clear and has sufficient instructions to be able to reproduce the issue.
+
+Meta has a [bounty program](https://www.facebook.com/whitehat/) for the safe
+disclosure of security bugs. In those cases, please go through the process
+outlined on that page and do not file a public issue.
+
+## License
+By contributing to Omnivore, you agree that your contributions will be licensed
+under the [LICENSE](LICENSE) file in the root directory of this source tree.
diff --git a/global_local/models/ImageBind/LICENSE b/global_local/models/ImageBind/LICENSE
new file mode 100644
index 0000000000000000000000000000000000000000..bfef380bf7d9cb74ec9ba533b37c3fbeef3bdc09
--- /dev/null
+++ b/global_local/models/ImageBind/LICENSE
@@ -0,0 +1,437 @@
+Attribution-NonCommercial-ShareAlike 4.0 International
+
+=======================================================================
+
+Creative Commons Corporation ("Creative Commons") is not a law firm and
+does not provide legal services or legal advice. Distribution of
+Creative Commons public licenses does not create a lawyer-client or
+other relationship. Creative Commons makes its licenses and related
+information available on an "as-is" basis. Creative Commons gives no
+warranties regarding its licenses, any material licensed under their
+terms and conditions, or any related information. Creative Commons
+disclaims all liability for damages resulting from their use to the
+fullest extent possible.
+
+Using Creative Commons Public Licenses
+
+Creative Commons public licenses provide a standard set of terms and
+conditions that creators and other rights holders may use to share
+original works of authorship and other material subject to copyright
+and certain other rights specified in the public license below. The
+following considerations are for informational purposes only, are not
+exhaustive, and do not form part of our licenses.
+
+ Considerations for licensors: Our public licenses are
+ intended for use by those authorized to give the public
+ permission to use material in ways otherwise restricted by
+ copyright and certain other rights. Our licenses are
+ irrevocable. Licensors should read and understand the terms
+ and conditions of the license they choose before applying it.
+ Licensors should also secure all rights necessary before
+ applying our licenses so that the public can reuse the
+ material as expected. Licensors should clearly mark any
+ material not subject to the license. This includes other CC-
+ licensed material, or material used under an exception or
+ limitation to copyright. More considerations for licensors:
+ wiki.creativecommons.org/Considerations_for_licensors
+
+ Considerations for the public: By using one of our public
+ licenses, a licensor grants the public permission to use the
+ licensed material under specified terms and conditions. If
+ the licensor's permission is not necessary for any reason--for
+ example, because of any applicable exception or limitation to
+ copyright--then that use is not regulated by the license. Our
+ licenses grant only permissions under copyright and certain
+ other rights that a licensor has authority to grant. Use of
+ the licensed material may still be restricted for other
+ reasons, including because others have copyright or other
+ rights in the material. A licensor may make special requests,
+ such as asking that all changes be marked or described.
+ Although not required by our licenses, you are encouraged to
+ respect those requests where reasonable. More considerations
+ for the public:
+ wiki.creativecommons.org/Considerations_for_licensees
+
+=======================================================================
+
+Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International
+Public License
+
+By exercising the Licensed Rights (defined below), You accept and agree
+to be bound by the terms and conditions of this Creative Commons
+Attribution-NonCommercial-ShareAlike 4.0 International Public License
+("Public License"). To the extent this Public License may be
+interpreted as a contract, You are granted the Licensed Rights in
+consideration of Your acceptance of these terms and conditions, and the
+Licensor grants You such rights in consideration of benefits the
+Licensor receives from making the Licensed Material available under
+these terms and conditions.
+
+
+Section 1 -- Definitions.
+
+ a. Adapted Material means material subject to Copyright and Similar
+ Rights that is derived from or based upon the Licensed Material
+ and in which the Licensed Material is translated, altered,
+ arranged, transformed, or otherwise modified in a manner requiring
+ permission under the Copyright and Similar Rights held by the
+ Licensor. For purposes of this Public License, where the Licensed
+ Material is a musical work, performance, or sound recording,
+ Adapted Material is always produced where the Licensed Material is
+ synched in timed relation with a moving image.
+
+ b. Adapter's License means the license You apply to Your Copyright
+ and Similar Rights in Your contributions to Adapted Material in
+ accordance with the terms and conditions of this Public License.
+
+ c. BY-NC-SA Compatible License means a license listed at
+ creativecommons.org/compatiblelicenses, approved by Creative
+ Commons as essentially the equivalent of this Public License.
+
+ d. Copyright and Similar Rights means copyright and/or similar rights
+ closely related to copyright including, without limitation,
+ performance, broadcast, sound recording, and Sui Generis Database
+ Rights, without regard to how the rights are labeled or
+ categorized. For purposes of this Public License, the rights
+ specified in Section 2(b)(1)-(2) are not Copyright and Similar
+ Rights.
+
+ e. Effective Technological Measures means those measures that, in the
+ absence of proper authority, may not be circumvented under laws
+ fulfilling obligations under Article 11 of the WIPO Copyright
+ Treaty adopted on December 20, 1996, and/or similar international
+ agreements.
+
+ f. Exceptions and Limitations means fair use, fair dealing, and/or
+ any other exception or limitation to Copyright and Similar Rights
+ that applies to Your use of the Licensed Material.
+
+ g. License Elements means the license attributes listed in the name
+ of a Creative Commons Public License. The License Elements of this
+ Public License are Attribution, NonCommercial, and ShareAlike.
+
+ h. Licensed Material means the artistic or literary work, database,
+ or other material to which the Licensor applied this Public
+ License.
+
+ i. Licensed Rights means the rights granted to You subject to the
+ terms and conditions of this Public License, which are limited to
+ all Copyright and Similar Rights that apply to Your use of the
+ Licensed Material and that the Licensor has authority to license.
+
+ j. Licensor means the individual(s) or entity(ies) granting rights
+ under this Public License.
+
+ k. NonCommercial means not primarily intended for or directed towards
+ commercial advantage or monetary compensation. For purposes of
+ this Public License, the exchange of the Licensed Material for
+ other material subject to Copyright and Similar Rights by digital
+ file-sharing or similar means is NonCommercial provided there is
+ no payment of monetary compensation in connection with the
+ exchange.
+
+ l. Share means to provide material to the public by any means or
+ process that requires permission under the Licensed Rights, such
+ as reproduction, public display, public performance, distribution,
+ dissemination, communication, or importation, and to make material
+ available to the public including in ways that members of the
+ public may access the material from a place and at a time
+ individually chosen by them.
+
+ m. Sui Generis Database Rights means rights other than copyright
+ resulting from Directive 96/9/EC of the European Parliament and of
+ the Council of 11 March 1996 on the legal protection of databases,
+ as amended and/or succeeded, as well as other essentially
+ equivalent rights anywhere in the world.
+
+ n. You means the individual or entity exercising the Licensed Rights
+ under this Public License. Your has a corresponding meaning.
+
+
+Section 2 -- Scope.
+
+ a. License grant.
+
+ 1. Subject to the terms and conditions of this Public License,
+ the Licensor hereby grants You a worldwide, royalty-free,
+ non-sublicensable, non-exclusive, irrevocable license to
+ exercise the Licensed Rights in the Licensed Material to:
+
+ a. reproduce and Share the Licensed Material, in whole or
+ in part, for NonCommercial purposes only; and
+
+ b. produce, reproduce, and Share Adapted Material for
+ NonCommercial purposes only.
+
+ 2. Exceptions and Limitations. For the avoidance of doubt, where
+ Exceptions and Limitations apply to Your use, this Public
+ License does not apply, and You do not need to comply with
+ its terms and conditions.
+
+ 3. Term. The term of this Public License is specified in Section
+ 6(a).
+
+ 4. Media and formats; technical modifications allowed. The
+ Licensor authorizes You to exercise the Licensed Rights in
+ all media and formats whether now known or hereafter created,
+ and to make technical modifications necessary to do so. The
+ Licensor waives and/or agrees not to assert any right or
+ authority to forbid You from making technical modifications
+ necessary to exercise the Licensed Rights, including
+ technical modifications necessary to circumvent Effective
+ Technological Measures. For purposes of this Public License,
+ simply making modifications authorized by this Section 2(a)
+ (4) never produces Adapted Material.
+
+ 5. Downstream recipients.
+
+ a. Offer from the Licensor -- Licensed Material. Every
+ recipient of the Licensed Material automatically
+ receives an offer from the Licensor to exercise the
+ Licensed Rights under the terms and conditions of this
+ Public License.
+
+ b. Additional offer from the Licensor -- Adapted Material.
+ Every recipient of Adapted Material from You
+ automatically receives an offer from the Licensor to
+ exercise the Licensed Rights in the Adapted Material
+ under the conditions of the Adapter's License You apply.
+
+ c. No downstream restrictions. You may not offer or impose
+ any additional or different terms or conditions on, or
+ apply any Effective Technological Measures to, the
+ Licensed Material if doing so restricts exercise of the
+ Licensed Rights by any recipient of the Licensed
+ Material.
+
+ 6. No endorsement. Nothing in this Public License constitutes or
+ may be construed as permission to assert or imply that You
+ are, or that Your use of the Licensed Material is, connected
+ with, or sponsored, endorsed, or granted official status by,
+ the Licensor or others designated to receive attribution as
+ provided in Section 3(a)(1)(A)(i).
+
+ b. Other rights.
+
+ 1. Moral rights, such as the right of integrity, are not
+ licensed under this Public License, nor are publicity,
+ privacy, and/or other similar personality rights; however, to
+ the extent possible, the Licensor waives and/or agrees not to
+ assert any such rights held by the Licensor to the limited
+ extent necessary to allow You to exercise the Licensed
+ Rights, but not otherwise.
+
+ 2. Patent and trademark rights are not licensed under this
+ Public License.
+
+ 3. To the extent possible, the Licensor waives any right to
+ collect royalties from You for the exercise of the Licensed
+ Rights, whether directly or through a collecting society
+ under any voluntary or waivable statutory or compulsory
+ licensing scheme. In all other cases the Licensor expressly
+ reserves any right to collect such royalties, including when
+ the Licensed Material is used other than for NonCommercial
+ purposes.
+
+
+Section 3 -- License Conditions.
+
+Your exercise of the Licensed Rights is expressly made subject to the
+following conditions.
+
+ a. Attribution.
+
+ 1. If You Share the Licensed Material (including in modified
+ form), You must:
+
+ a. retain the following if it is supplied by the Licensor
+ with the Licensed Material:
+
+ i. identification of the creator(s) of the Licensed
+ Material and any others designated to receive
+ attribution, in any reasonable manner requested by
+ the Licensor (including by pseudonym if
+ designated);
+
+ ii. a copyright notice;
+
+ iii. a notice that refers to this Public License;
+
+ iv. a notice that refers to the disclaimer of
+ warranties;
+
+ v. a URI or hyperlink to the Licensed Material to the
+ extent reasonably practicable;
+
+ b. indicate if You modified the Licensed Material and
+ retain an indication of any previous modifications; and
+
+ c. indicate the Licensed Material is licensed under this
+ Public License, and include the text of, or the URI or
+ hyperlink to, this Public License.
+
+ 2. You may satisfy the conditions in Section 3(a)(1) in any
+ reasonable manner based on the medium, means, and context in
+ which You Share the Licensed Material. For example, it may be
+ reasonable to satisfy the conditions by providing a URI or
+ hyperlink to a resource that includes the required
+ information.
+ 3. If requested by the Licensor, You must remove any of the
+ information required by Section 3(a)(1)(A) to the extent
+ reasonably practicable.
+
+ b. ShareAlike.
+
+ In addition to the conditions in Section 3(a), if You Share
+ Adapted Material You produce, the following conditions also apply.
+
+ 1. The Adapter's License You apply must be a Creative Commons
+ license with the same License Elements, this version or
+ later, or a BY-NC-SA Compatible License.
+
+ 2. You must include the text of, or the URI or hyperlink to, the
+ Adapter's License You apply. You may satisfy this condition
+ in any reasonable manner based on the medium, means, and
+ context in which You Share Adapted Material.
+
+ 3. You may not offer or impose any additional or different terms
+ or conditions on, or apply any Effective Technological
+ Measures to, Adapted Material that restrict exercise of the
+ rights granted under the Adapter's License You apply.
+
+
+Section 4 -- Sui Generis Database Rights.
+
+Where the Licensed Rights include Sui Generis Database Rights that
+apply to Your use of the Licensed Material:
+
+ a. for the avoidance of doubt, Section 2(a)(1) grants You the right
+ to extract, reuse, reproduce, and Share all or a substantial
+ portion of the contents of the database for NonCommercial purposes
+ only;
+
+ b. if You include all or a substantial portion of the database
+ contents in a database in which You have Sui Generis Database
+ Rights, then the database in which You have Sui Generis Database
+ Rights (but not its individual contents) is Adapted Material,
+ including for purposes of Section 3(b); and
+
+ c. You must comply with the conditions in Section 3(a) if You Share
+ all or a substantial portion of the contents of the database.
+
+For the avoidance of doubt, this Section 4 supplements and does not
+replace Your obligations under this Public License where the Licensed
+Rights include other Copyright and Similar Rights.
+
+
+Section 5 -- Disclaimer of Warranties and Limitation of Liability.
+
+ a. UNLESS OTHERWISE SEPARATELY UNDERTAKEN BY THE LICENSOR, TO THE
+ EXTENT POSSIBLE, THE LICENSOR OFFERS THE LICENSED MATERIAL AS-IS
+ AND AS-AVAILABLE, AND MAKES NO REPRESENTATIONS OR WARRANTIES OF
+ ANY KIND CONCERNING THE LICENSED MATERIAL, WHETHER EXPRESS,
+ IMPLIED, STATUTORY, OR OTHER. THIS INCLUDES, WITHOUT LIMITATION,
+ WARRANTIES OF TITLE, MERCHANTABILITY, FITNESS FOR A PARTICULAR
+ PURPOSE, NON-INFRINGEMENT, ABSENCE OF LATENT OR OTHER DEFECTS,
+ ACCURACY, OR THE PRESENCE OR ABSENCE OF ERRORS, WHETHER OR NOT
+ KNOWN OR DISCOVERABLE. WHERE DISCLAIMERS OF WARRANTIES ARE NOT
+ ALLOWED IN FULL OR IN PART, THIS DISCLAIMER MAY NOT APPLY TO YOU.
+
+ b. TO THE EXTENT POSSIBLE, IN NO EVENT WILL THE LICENSOR BE LIABLE
+ TO YOU ON ANY LEGAL THEORY (INCLUDING, WITHOUT LIMITATION,
+ NEGLIGENCE) OR OTHERWISE FOR ANY DIRECT, SPECIAL, INDIRECT,
+ INCIDENTAL, CONSEQUENTIAL, PUNITIVE, EXEMPLARY, OR OTHER LOSSES,
+ COSTS, EXPENSES, OR DAMAGES ARISING OUT OF THIS PUBLIC LICENSE OR
+ USE OF THE LICENSED MATERIAL, EVEN IF THE LICENSOR HAS BEEN
+ ADVISED OF THE POSSIBILITY OF SUCH LOSSES, COSTS, EXPENSES, OR
+ DAMAGES. WHERE A LIMITATION OF LIABILITY IS NOT ALLOWED IN FULL OR
+ IN PART, THIS LIMITATION MAY NOT APPLY TO YOU.
+
+ c. The disclaimer of warranties and limitation of liability provided
+ above shall be interpreted in a manner that, to the extent
+ possible, most closely approximates an absolute disclaimer and
+ waiver of all liability.
+
+
+Section 6 -- Term and Termination.
+
+ a. This Public License applies for the term of the Copyright and
+ Similar Rights licensed here. However, if You fail to comply with
+ this Public License, then Your rights under this Public License
+ terminate automatically.
+
+ b. Where Your right to use the Licensed Material has terminated under
+ Section 6(a), it reinstates:
+
+ 1. automatically as of the date the violation is cured, provided
+ it is cured within 30 days of Your discovery of the
+ violation; or
+
+ 2. upon express reinstatement by the Licensor.
+
+ For the avoidance of doubt, this Section 6(b) does not affect any
+ right the Licensor may have to seek remedies for Your violations
+ of this Public License.
+
+ c. For the avoidance of doubt, the Licensor may also offer the
+ Licensed Material under separate terms or conditions or stop
+ distributing the Licensed Material at any time; however, doing so
+ will not terminate this Public License.
+
+ d. Sections 1, 5, 6, 7, and 8 survive termination of this Public
+ License.
+
+
+Section 7 -- Other Terms and Conditions.
+
+ a. The Licensor shall not be bound by any additional or different
+ terms or conditions communicated by You unless expressly agreed.
+
+ b. Any arrangements, understandings, or agreements regarding the
+ Licensed Material not stated herein are separate from and
+ independent of the terms and conditions of this Public License.
+
+
+Section 8 -- Interpretation.
+
+ a. For the avoidance of doubt, this Public License does not, and
+ shall not be interpreted to, reduce, limit, restrict, or impose
+ conditions on any use of the Licensed Material that could lawfully
+ be made without permission under this Public License.
+
+ b. To the extent possible, if any provision of this Public License is
+ deemed unenforceable, it shall be automatically reformed to the
+ minimum extent necessary to make it enforceable. If the provision
+ cannot be reformed, it shall be severed from this Public License
+ without affecting the enforceability of the remaining terms and
+ conditions.
+
+ c. No term or condition of this Public License will be waived and no
+ failure to comply consented to unless expressly agreed to by the
+ Licensor.
+
+ d. Nothing in this Public License constitutes or may be interpreted
+ as a limitation upon, or waiver of, any privileges and immunities
+ that apply to the Licensor or You, including from the legal
+ processes of any jurisdiction or authority.
+
+=======================================================================
+
+Creative Commons is not a party to its public
+licenses. Notwithstanding, Creative Commons may elect to apply one of
+its public licenses to material it publishes and in those instances
+will be considered the “Licensor.” The text of the Creative Commons
+public licenses is dedicated to the public domain under the CC0 Public
+Domain Dedication. Except for the limited purpose of indicating that
+material is shared under a Creative Commons public license or as
+otherwise permitted by the Creative Commons policies published at
+creativecommons.org/policies, Creative Commons does not authorize the
+use of the trademark "Creative Commons" or any other trademark or logo
+of Creative Commons without its prior written consent including,
+without limitation, in connection with any unauthorized modifications
+to any of its public licenses or any other arrangements,
+understandings, or agreements concerning use of licensed material. For
+the avoidance of doubt, this paragraph does not form part of the
+public licenses.
+
+Creative Commons may be contacted at creativecommons.org.
\ No newline at end of file
diff --git a/global_local/models/ImageBind/README.md b/global_local/models/ImageBind/README.md
new file mode 100644
index 0000000000000000000000000000000000000000..028fa988bb6cd9843aec9454636e1541b53680e7
--- /dev/null
+++ b/global_local/models/ImageBind/README.md
@@ -0,0 +1,155 @@
+# ImageBind: One Embedding Space To Bind Them All
+
+**[FAIR, Meta AI](https://ai.facebook.com/research/)**
+
+Rohit Girdhar*,
+Alaaeldin El-Nouby*,
+Zhuang Liu,
+Mannat Singh,
+Kalyan Vasudev Alwala,
+Armand Joulin,
+Ishan Misra*
+
+To appear at CVPR 2023 (*Highlighted paper*)
+
+[[`Paper`](https://facebookresearch.github.io/ImageBind/paper)] [[`Blog`](https://ai.facebook.com/blog/imagebind-six-modalities-binding-ai/)] [[`Demo`](https://imagebind.metademolab.com/)] [[`Supplementary Video`](https://dl.fbaipublicfiles.com/imagebind/imagebind_video.mp4)] [[`BibTex`](#citing-imagebind)]
+
+PyTorch implementation and pretrained models for ImageBind. For details, see the paper: **[ImageBind: One Embedding Space To Bind Them All](https://facebookresearch.github.io/ImageBind/paper)**.
+
+ImageBind learns a joint embedding across six different modalities - images, text, audio, depth, thermal, and IMU data. It enables novel emergent applications ‘out-of-the-box’ including cross-modal retrieval, composing modalities with arithmetic, cross-modal detection and generation.
+
+
+
+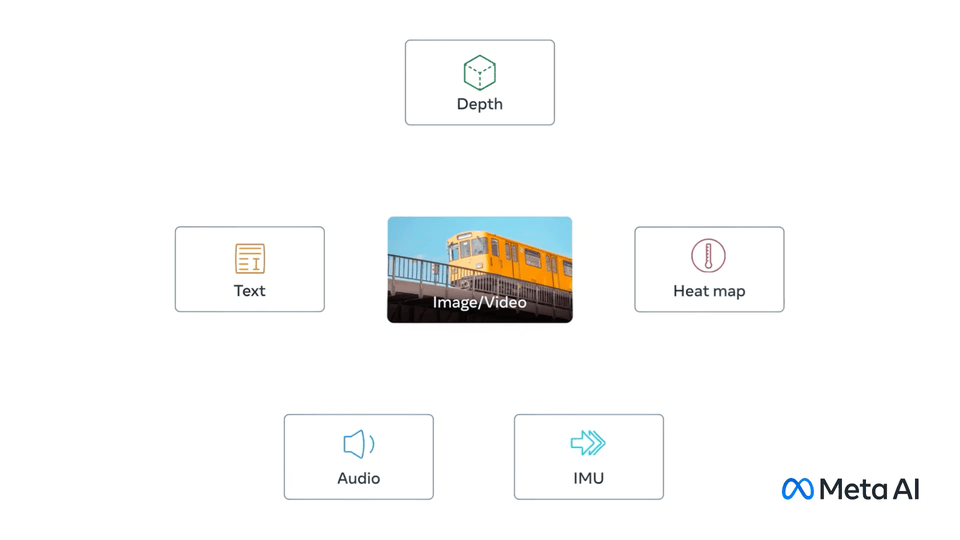
+
+## ImageBind model
+
+Emergent zero-shot classification performance.
+
+
+
+ Model |
+ IN1k |
+ K400 |
+ NYU-D |
+ ESC |
+ LLVIP |
+ Ego4D |
+ download |
+
+
+ imagebind_huge |
+ 77.7 |
+ 50.0 |
+ 54.0 |
+ 66.9 |
+ 63.4 |
+ 25.0 |
+ checkpoint |
+
+
+
+
+## Usage
+
+Install pytorch 1.13+ and other 3rd party dependencies.
+
+```shell
+conda create --name imagebind python=3.8 -y
+conda activate imagebind
+
+pip install -r requirements.txt
+```
+
+For windows users, you might need to install `soundfile` for reading/writing audio files. (Thanks @congyue1977)
+
+```
+pip install soundfile
+```
+
+
+Extract and compare features across modalities (e.g. Image, Text and Audio).
+
+```python
+import data
+import torch
+from models import imagebind_model
+from models.imagebind_model import ModalityType
+
+text_list=["A dog.", "A car", "A bird"]
+image_paths=[".assets/dog_image.jpg", ".assets/car_image.jpg", ".assets/bird_image.jpg"]
+audio_paths=[".assets/dog_audio.wav", ".assets/car_audio.wav", ".assets/bird_audio.wav"]
+
+device = "cuda:0" if torch.cuda.is_available() else "cpu"
+
+# Instantiate model
+model = imagebind_model.imagebind_huge(pretrained=True)
+model.eval()
+model.to(device)
+
+# Load data
+inputs = {
+ ModalityType.TEXT: data.load_and_transform_text(text_list, device),
+ ModalityType.VISION: data.load_and_transform_vision_data(image_paths, device),
+ ModalityType.AUDIO: data.load_and_transform_audio_data(audio_paths, device),
+}
+
+with torch.no_grad():
+ embeddings = model(inputs)
+
+print(
+ "Vision x Text: ",
+ torch.softmax(embeddings[ModalityType.VISION] @ embeddings[ModalityType.TEXT].T, dim=-1),
+)
+print(
+ "Audio x Text: ",
+ torch.softmax(embeddings[ModalityType.AUDIO] @ embeddings[ModalityType.TEXT].T, dim=-1),
+)
+print(
+ "Vision x Audio: ",
+ torch.softmax(embeddings[ModalityType.VISION] @ embeddings[ModalityType.AUDIO].T, dim=-1),
+)
+
+# Expected output:
+#
+# Vision x Text:
+# tensor([[9.9761e-01, 2.3694e-03, 1.8612e-05],
+# [3.3836e-05, 9.9994e-01, 2.4118e-05],
+# [4.7997e-05, 1.3496e-02, 9.8646e-01]])
+#
+# Audio x Text:
+# tensor([[1., 0., 0.],
+# [0., 1., 0.],
+# [0., 0., 1.]])
+#
+# Vision x Audio:
+# tensor([[0.8070, 0.1088, 0.0842],
+# [0.1036, 0.7884, 0.1079],
+# [0.0018, 0.0022, 0.9960]])
+
+```
+
+## Model card
+Please see the [model card](model_card.md) for details.
+
+## License
+
+ImageBind code and model weights are released under the CC-BY-NC 4.0 license. See [LICENSE](LICENSE) for additional details.
+
+## Contributing
+
+See [contributing](CONTRIBUTING.md) and the [code of conduct](CODE_OF_CONDUCT.md).
+
+## Citing ImageBind
+
+If you find this repository useful, please consider giving a star :star: and citation
+
+```
+@inproceedings{girdhar2023imagebind,
+ title={ImageBind: One Embedding Space To Bind Them All},
+ author={Girdhar, Rohit and El-Nouby, Alaaeldin and Liu, Zhuang
+and Singh, Mannat and Alwala, Kalyan Vasudev and Joulin, Armand and Misra, Ishan},
+ booktitle={CVPR},
+ year={2023}
+}
+```
diff --git a/global_local/models/ImageBind/__pycache__/data.cpython-39.pyc b/global_local/models/ImageBind/__pycache__/data.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..563b024ba8f422a535f4a4fa8dc6249194b78b22
Binary files /dev/null and b/global_local/models/ImageBind/__pycache__/data.cpython-39.pyc differ
diff --git a/global_local/models/ImageBind/bpe/bpe_simple_vocab_16e6.txt.gz b/global_local/models/ImageBind/bpe/bpe_simple_vocab_16e6.txt.gz
new file mode 100644
index 0000000000000000000000000000000000000000..36a15856e00a06a9fbed8cdd34d2393fea4a3113
--- /dev/null
+++ b/global_local/models/ImageBind/bpe/bpe_simple_vocab_16e6.txt.gz
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:924691ac288e54409236115652ad4aa250f48203de50a9e4722a6ecd48d6804a
+size 1356917
diff --git a/global_local/models/ImageBind/data.py b/global_local/models/ImageBind/data.py
new file mode 100644
index 0000000000000000000000000000000000000000..993ff696bd98f1b380f2e9537b3f70ca38501f22
--- /dev/null
+++ b/global_local/models/ImageBind/data.py
@@ -0,0 +1,338 @@
+#!/usr/bin/env python3
+# Portions Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+import logging
+import math
+
+import torch
+import torch.nn as nn
+import torchaudio
+from PIL import Image
+from pytorchvideo import transforms as pv_transforms
+from pytorchvideo.data.clip_sampling import ConstantClipsPerVideoSampler
+from pytorchvideo.data.encoded_video import EncodedVideo
+from torchvision import transforms
+from torchvision.transforms._transforms_video import NormalizeVideo
+
+from .models.multimodal_preprocessors import SimpleTokenizer
+
+DEFAULT_AUDIO_FRAME_SHIFT_MS = 10 # in milliseconds
+
+BPE_PATH = "bpe/bpe_simple_vocab_16e6.txt.gz"
+
+
+def waveform2melspec(waveform, sample_rate, num_mel_bins, target_length):
+ # Based on https://github.com/YuanGongND/ast/blob/d7d8b4b8e06cdaeb6c843cdb38794c1c7692234c/src/dataloader.py#L102
+ waveform -= waveform.mean()
+ fbank = torchaudio.compliance.kaldi.fbank(
+ waveform,
+ htk_compat=True,
+ sample_frequency=sample_rate,
+ use_energy=False,
+ window_type="hanning",
+ num_mel_bins=num_mel_bins,
+ dither=0.0,
+ frame_length=25,
+ frame_shift=DEFAULT_AUDIO_FRAME_SHIFT_MS,
+ )
+ # Convert to [mel_bins, num_frames] shape
+ fbank = fbank.transpose(0, 1)
+ # Pad to target_length
+ n_frames = fbank.size(1)
+ p = target_length - n_frames
+ # if p is too large (say >20%), flash a warning
+ if abs(p) / n_frames > 0.2:
+ logging.warning(
+ "Large gap between audio n_frames(%d) and "
+ "target_length (%d). Is the audio_target_length "
+ "setting correct?",
+ n_frames,
+ target_length,
+ )
+ # cut and pad
+ if p > 0:
+ fbank = torch.nn.functional.pad(fbank, (0, p), mode="constant", value=0)
+ elif p < 0:
+ fbank = fbank[:, 0:target_length]
+ # Convert to [1, mel_bins, num_frames] shape, essentially like a 1
+ # channel image
+ fbank = fbank.unsqueeze(0)
+ return fbank
+
+
+def get_clip_timepoints(clip_sampler, duration):
+ # Read out all clips in this video
+ all_clips_timepoints = []
+ is_last_clip = False
+ end = 0.0
+ while not is_last_clip:
+ start, end, _, _, is_last_clip = clip_sampler(end, duration, annotation=None)
+ all_clips_timepoints.append((start, end))
+ return all_clips_timepoints
+
+
+def load_and_transform_vision_data(image_paths, device):
+ if image_paths is None:
+ return None
+
+ image_ouputs = []
+ for image_path in image_paths:
+ data_transform = transforms.Compose(
+ [
+ transforms.Resize(
+ 224, interpolation=transforms.InterpolationMode.BICUBIC
+ ),
+ transforms.CenterCrop(224),
+ transforms.ToTensor(),
+ transforms.Normalize(
+ mean=(0.48145466, 0.4578275, 0.40821073),
+ std=(0.26862954, 0.26130258, 0.27577711),
+ ),
+ ]
+ )
+ with open(image_path, "rb") as fopen:
+ image = Image.open(fopen).convert("RGB")
+
+ image = data_transform(image).to(device)
+ image_ouputs.append(image)
+ return torch.stack(image_ouputs, dim=0)
+
+
+def load_and_transform_text(text, device):
+ if text is None:
+ return None
+ tokenizer = SimpleTokenizer(bpe_path=BPE_PATH)
+ tokens = [tokenizer(t).unsqueeze(0).to(device) for t in text]
+ tokens = torch.cat(tokens, dim=0)
+ return tokens
+
+
+def load_and_transform_audio_data(
+ audio_paths,
+ device,
+ num_mel_bins=128,
+ target_length=204,
+ sample_rate=16000,
+ clip_duration=2,
+ clips_per_video=3,
+ mean=-4.268,
+ std=9.138,
+):
+ if audio_paths is None:
+ return None
+
+ audio_outputs = []
+ clip_sampler = ConstantClipsPerVideoSampler(
+ clip_duration=clip_duration, clips_per_video=clips_per_video
+ )
+
+ for audio_path in audio_paths:
+ waveform, sr = torchaudio.load(audio_path)
+ if sample_rate != sr:
+ waveform = torchaudio.functional.resample(
+ waveform, orig_freq=sr, new_freq=sample_rate
+ )
+ all_clips_timepoints = get_clip_timepoints(
+ clip_sampler, waveform.size(1) / sample_rate
+ )
+ all_clips = []
+ for clip_timepoints in all_clips_timepoints:
+ waveform_clip = waveform[
+ :,
+ int(clip_timepoints[0] * sample_rate) : int(
+ clip_timepoints[1] * sample_rate
+ ),
+ ]
+ waveform_melspec = waveform2melspec(
+ waveform_clip, sample_rate, num_mel_bins, target_length
+ )
+ all_clips.append(waveform_melspec)
+
+ normalize = transforms.Normalize(mean=mean, std=std)
+ all_clips = [normalize(ac).to(device) for ac in all_clips]
+
+ all_clips = torch.stack(all_clips, dim=0)
+ audio_outputs.append(all_clips)
+
+ return torch.stack(audio_outputs, dim=0)
+
+
+def crop_boxes(boxes, x_offset, y_offset):
+ """
+ Perform crop on the bounding boxes given the offsets.
+ Args:
+ boxes (ndarray or None): bounding boxes to perform crop. The dimension
+ is `num boxes` x 4.
+ x_offset (int): cropping offset in the x axis.
+ y_offset (int): cropping offset in the y axis.
+ Returns:
+ cropped_boxes (ndarray or None): the cropped boxes with dimension of
+ `num boxes` x 4.
+ """
+ cropped_boxes = boxes.copy()
+ cropped_boxes[:, [0, 2]] = boxes[:, [0, 2]] - x_offset
+ cropped_boxes[:, [1, 3]] = boxes[:, [1, 3]] - y_offset
+
+ return cropped_boxes
+
+
+def uniform_crop(images, size, spatial_idx, boxes=None, scale_size=None):
+ """
+ Perform uniform spatial sampling on the images and corresponding boxes.
+ Args:
+ images (tensor): images to perform uniform crop. The dimension is
+ `num frames` x `channel` x `height` x `width`.
+ size (int): size of height and weight to crop the images.
+ spatial_idx (int): 0, 1, or 2 for left, center, and right crop if width
+ is larger than height. Or 0, 1, or 2 for top, center, and bottom
+ crop if height is larger than width.
+ boxes (ndarray or None): optional. Corresponding boxes to images.
+ Dimension is `num boxes` x 4.
+ scale_size (int): optinal. If not None, resize the images to scale_size before
+ performing any crop.
+ Returns:
+ cropped (tensor): images with dimension of
+ `num frames` x `channel` x `size` x `size`.
+ cropped_boxes (ndarray or None): the cropped boxes with dimension of
+ `num boxes` x 4.
+ """
+ assert spatial_idx in [0, 1, 2]
+ ndim = len(images.shape)
+ if ndim == 3:
+ images = images.unsqueeze(0)
+ height = images.shape[2]
+ width = images.shape[3]
+
+ if scale_size is not None:
+ if width <= height:
+ width, height = scale_size, int(height / width * scale_size)
+ else:
+ width, height = int(width / height * scale_size), scale_size
+ images = torch.nn.functional.interpolate(
+ images,
+ size=(height, width),
+ mode="bilinear",
+ align_corners=False,
+ )
+
+ y_offset = int(math.ceil((height - size) / 2))
+ x_offset = int(math.ceil((width - size) / 2))
+
+ if height > width:
+ if spatial_idx == 0:
+ y_offset = 0
+ elif spatial_idx == 2:
+ y_offset = height - size
+ else:
+ if spatial_idx == 0:
+ x_offset = 0
+ elif spatial_idx == 2:
+ x_offset = width - size
+ cropped = images[:, :, y_offset : y_offset + size, x_offset : x_offset + size]
+ cropped_boxes = crop_boxes(boxes, x_offset, y_offset) if boxes is not None else None
+ if ndim == 3:
+ cropped = cropped.squeeze(0)
+ return cropped, cropped_boxes
+
+
+class SpatialCrop(nn.Module):
+ """
+ Convert the video into 3 smaller clips spatially. Must be used after the
+ temporal crops to get spatial crops, and should be used with
+ -2 in the spatial crop at the slowfast augmentation stage (so full
+ frames are passed in here). Will return a larger list with the
+ 3x spatial crops as well.
+ """
+
+ def __init__(self, crop_size: int = 224, num_crops: int = 3):
+ super().__init__()
+ self.crop_size = crop_size
+ if num_crops == 3:
+ self.crops_to_ext = [0, 1, 2]
+ self.flipped_crops_to_ext = []
+ elif num_crops == 1:
+ self.crops_to_ext = [1]
+ self.flipped_crops_to_ext = []
+ else:
+ raise NotImplementedError("Nothing else supported yet")
+
+ def forward(self, videos):
+ """
+ Args:
+ videos: A list of C, T, H, W videos.
+ Returns:
+ videos: A list with 3x the number of elements. Each video converted
+ to C, T, H', W' by spatial cropping.
+ """
+ assert isinstance(videos, list), "Must be a list of videos after temporal crops"
+ assert all([video.ndim == 4 for video in videos]), "Must be (C,T,H,W)"
+ res = []
+ for video in videos:
+ for spatial_idx in self.crops_to_ext:
+ res.append(uniform_crop(video, self.crop_size, spatial_idx)[0])
+ if not self.flipped_crops_to_ext:
+ continue
+ flipped_video = transforms.functional.hflip(video)
+ for spatial_idx in self.flipped_crops_to_ext:
+ res.append(uniform_crop(flipped_video, self.crop_size, spatial_idx)[0])
+ return res
+
+
+def load_and_transform_video_data(
+ video_paths,
+ device,
+ clip_duration=2,
+ clips_per_video=5,
+ sample_rate=16000,
+):
+ if video_paths is None:
+ return None
+
+ video_outputs = []
+ video_transform = transforms.Compose(
+ [
+ pv_transforms.ShortSideScale(224),
+ NormalizeVideo(
+ mean=(0.48145466, 0.4578275, 0.40821073),
+ std=(0.26862954, 0.26130258, 0.27577711),
+ ),
+ ]
+ )
+
+ clip_sampler = ConstantClipsPerVideoSampler(
+ clip_duration=clip_duration, clips_per_video=clips_per_video
+ )
+ frame_sampler = pv_transforms.UniformTemporalSubsample(num_samples=clip_duration)
+
+ for video_path in video_paths:
+ video = EncodedVideo.from_path(
+ video_path,
+ decoder="decord",
+ decode_audio=False,
+ **{"sample_rate": sample_rate},
+ )
+
+ all_clips_timepoints = get_clip_timepoints(clip_sampler, video.duration)
+
+ all_video = []
+ for clip_timepoints in all_clips_timepoints:
+ # Read the clip, get frames
+ clip = video.get_clip(clip_timepoints[0], clip_timepoints[1])
+ if clip is None:
+ raise ValueError("No clip found")
+ video_clip = frame_sampler(clip["video"])
+ video_clip = video_clip / 255.0 # since this is float, need 0-1
+
+ all_video.append(video_clip)
+
+ all_video = [video_transform(clip) for clip in all_video]
+ all_video = SpatialCrop(224, num_crops=3)(all_video)
+
+ all_video = torch.stack(all_video, dim=0)
+ video_outputs.append(all_video)
+
+ return torch.stack(video_outputs, dim=0).to(device)
diff --git a/global_local/models/ImageBind/model_card.md b/global_local/models/ImageBind/model_card.md
new file mode 100644
index 0000000000000000000000000000000000000000..c7bb26500b6590b64ffa6350f37be80dc88612d8
--- /dev/null
+++ b/global_local/models/ImageBind/model_card.md
@@ -0,0 +1,94 @@
+# Model Card for ImageBind
+
+Multimodal joint embedding model for image/video, text, audio, depth, IMU, and thermal images.
+Input any of the six modalities and get the same sized embedding that can be used for cross-modal and multimodal tasks.
+
+# Model Details
+
+## Model Description
+
+
+Multimodal joint embedding model for image/video, text, audio, depth, IMU, and thermal images
+
+- **Developed by:** Meta AI
+- **Model type:** Multimodal model
+- **Language(s) (NLP):** en
+- **License:** CC BY-NC-SA 4.0
+- **Resources for more information:**
+ - [GitHub Repo](https://github.com/facebookresearch/ImageBind)
+
+
+# Uses
+
+
+This model is intended only for research purposes. It provides a joint embedding space for different modalities -- image/video, text, audio, depth, IMU and thermal images.
+We hope that these joint embeddings can be used for a variety of different cross-modal research, e.g., cross-modal retrieval and combining embeddings from different modalities.
+
+## Out-of-Scope Use
+
+
+
+
+This model is *NOT* intended to be used in any real world application -- commercial or otherwise.
+It may produce harmful associations with different inputs.
+The model needs to be investigated and likely re-trained on specific data for any such application.
+The model is expected to work better on web-based visual data since it was trained on such data.
+The text encoder is likely to work only on English language text because of the underlying training datasets.
+
+# Bias, Risks, and Limitations
+
+
+Open-domain joint embedding models are prone to producing specific biases, e.g., study from [CLIP](https://github.com/openai/CLIP/blob/main/model-card.md#bias-and-fairness).
+Since our model uses such models as initialization, it will exhibit such biases too.
+Moreover, for learning joint embeddings for other modalities such as audio, thermal, depth, and IMU we leverage datasets that are relatively small. These joint embeddings are thus limited to the concepts present in the datasets. For example, the thermal datasets we used are limited to outdoor street scenes, while the depth datasets are limited to indoor scenes.
+
+
+
+# Training Details
+
+## Training Data
+
+
+
+ImageBind uses image-paired data for training -- (image, X) where X is one of text, audio, depth, IMU or thermal data.
+In particular, we initialize and freeze the image and text encoders using an OpenCLIP ViT-H encoder.
+We train audio embeddings using Audioset, depth embeddings using the SUN RGB-D dataset, IMU using the Ego4D dataset and thermal embeddings using the LLVIP dataset.
+We provide the exact training data details in the paper.
+
+
+## Training Procedure
+
+
+Please refer to the research paper and github repo for exact details on this.
+
+# Evaluation
+
+## Testing Data, Factors & Metrics
+
+We evaluate the model on a variety of different classification benchmarks for each modality.
+The evaluation details are presented in the paper.
+The models performance is measured using standard classification metrics such as accuracy and mAP.
+
+# Citation
+
+
+
+**BibTeX:**
+```
+@inproceedings{girdhar2023imagebind,
+ title={ImageBind: One Embedding Space To Bind Them All},
+ author={Girdhar, Rohit and El-Nouby, Alaaeldin and Liu, Zhuang
+and Singh, Mannat and Alwala, Kalyan Vasudev and Joulin, Armand and Misra, Ishan},
+ booktitle={CVPR},
+ year={2023}
+}
+```
+
+
+# Model Card Contact
+
+Please reach out to the authors at: rgirdhar@meta.com imisra@meta.com alaaelnouby@gmail.com
+
+# How to Get Started with the Model
+
+Our github repo provides a simple example to extract embeddings from images, audio etc.
diff --git a/global_local/models/ImageBind/models/__init__.py b/global_local/models/ImageBind/models/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/global_local/models/ImageBind/models/__pycache__/__init__.cpython-39.pyc b/global_local/models/ImageBind/models/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..997781ed79fde784530d4b7beca027d3b26b9693
Binary files /dev/null and b/global_local/models/ImageBind/models/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/models/ImageBind/models/__pycache__/helpers.cpython-39.pyc b/global_local/models/ImageBind/models/__pycache__/helpers.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..752caac95ac71293da47168833122a0dd9ebae65
Binary files /dev/null and b/global_local/models/ImageBind/models/__pycache__/helpers.cpython-39.pyc differ
diff --git a/global_local/models/ImageBind/models/__pycache__/imagebind_model.cpython-39.pyc b/global_local/models/ImageBind/models/__pycache__/imagebind_model.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..9b5ac3d19511aa623b9a072c8f08bd88b230b872
Binary files /dev/null and b/global_local/models/ImageBind/models/__pycache__/imagebind_model.cpython-39.pyc differ
diff --git a/global_local/models/ImageBind/models/__pycache__/multimodal_preprocessors.cpython-39.pyc b/global_local/models/ImageBind/models/__pycache__/multimodal_preprocessors.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..e41673acb4fe461465aaae4d1ac3400c9eeffa69
Binary files /dev/null and b/global_local/models/ImageBind/models/__pycache__/multimodal_preprocessors.cpython-39.pyc differ
diff --git a/global_local/models/ImageBind/models/__pycache__/transformer.cpython-39.pyc b/global_local/models/ImageBind/models/__pycache__/transformer.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..b060791093e8aef5259fb54a3f4b366c11276b3b
Binary files /dev/null and b/global_local/models/ImageBind/models/__pycache__/transformer.cpython-39.pyc differ
diff --git a/global_local/models/ImageBind/models/helpers.py b/global_local/models/ImageBind/models/helpers.py
new file mode 100644
index 0000000000000000000000000000000000000000..71abe9b1fc32ed22ba46ea89ae7439d4ea49afca
--- /dev/null
+++ b/global_local/models/ImageBind/models/helpers.py
@@ -0,0 +1,140 @@
+#!/usr/bin/env python3
+# Portions Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+
+import einops
+import numpy as np
+import torch
+import torch.nn as nn
+
+
+class Normalize(nn.Module):
+ def __init__(self, dim: int) -> None:
+ super().__init__()
+ self.dim = dim
+
+ def forward(self, x):
+ return torch.nn.functional.normalize(x, dim=self.dim, p=2)
+
+
+class LearnableLogitScaling(nn.Module):
+ def __init__(
+ self,
+ logit_scale_init: float = 1 / 0.07,
+ learnable: bool = True,
+ max_logit_scale: float = 100,
+ ) -> None:
+ super().__init__()
+ self.max_logit_scale = max_logit_scale
+ self.logit_scale_init = logit_scale_init
+ self.learnable = learnable
+ log_logit_scale = torch.ones([]) * np.log(self.logit_scale_init)
+ if learnable:
+ self.log_logit_scale = nn.Parameter(log_logit_scale)
+ else:
+ self.register_buffer("log_logit_scale", log_logit_scale)
+
+ def forward(self, x):
+ return torch.clip(self.log_logit_scale.exp(), max=self.max_logit_scale) * x
+
+ def extra_repr(self):
+ st = f"logit_scale_init={self.logit_scale_init},learnable={self.learnable}," \
+ f" max_logit_scale={self.max_logit_scale}"
+ return st
+
+
+class EinOpsRearrange(nn.Module):
+ def __init__(self, rearrange_expr: str, **kwargs) -> None:
+ super().__init__()
+ self.rearrange_expr = rearrange_expr
+ self.kwargs = kwargs
+
+ def forward(self, x):
+ assert isinstance(x, torch.Tensor)
+ return einops.rearrange(x, self.rearrange_expr, **self.kwargs)
+
+
+class VerboseNNModule(nn.Module):
+ """
+ Wrapper around nn.Module that prints registered buffers and parameter names.
+ """
+
+ @staticmethod
+ def get_readable_tensor_repr(name: str, tensor: torch.Tensor) -> str:
+ st = (
+ "("
+ + name
+ + "): "
+ + "tensor("
+ + str(tuple(tensor[1].shape))
+ + ", requires_grad="
+ + str(tensor[1].requires_grad)
+ + ")\n"
+ )
+ return st
+
+ def extra_repr(self) -> str:
+ named_modules = set()
+ for p in self.named_modules():
+ named_modules.update([p[0]])
+ named_modules = list(named_modules)
+
+ string_repr = ""
+ for p in self.named_parameters():
+ name = p[0].split(".")[0]
+ if name not in named_modules:
+ string_repr += self.get_readable_tensor_repr(name, p)
+
+ for p in self.named_buffers():
+ name = p[0].split(".")[0]
+ string_repr += self.get_readable_tensor_repr(name, p)
+
+ return string_repr
+
+
+def cast_if_src_dtype(
+ tensor: torch.Tensor, src_dtype: torch.dtype, tgt_dtype: torch.dtype
+):
+ updated = False
+ if tensor.dtype == src_dtype:
+ tensor = tensor.to(dtype=tgt_dtype)
+ updated = True
+ return tensor, updated
+
+
+class QuickGELU(nn.Module):
+ # From https://github.com/openai/CLIP/blob/d50d76daa670286dd6cacf3bcd80b5e4823fc8e1/clip/model.py#L166
+ def forward(self, x: torch.Tensor):
+ return x * torch.sigmoid(1.702 * x)
+
+
+class SelectElement(nn.Module):
+ def __init__(self, index) -> None:
+ super().__init__()
+ self.index = index
+
+ def forward(self, x):
+ assert x.ndim >= 3
+ return x[:, self.index, ...]
+
+
+class SelectEOSAndProject(nn.Module):
+ """
+ Text Pooling used in OpenCLIP
+ """
+
+ def __init__(self, proj: nn.Module) -> None:
+ super().__init__()
+ self.proj = proj
+
+ def forward(self, x, seq_len):
+ assert x.ndim == 3
+ # x is of shape B x L x D
+ # take features from the eot embedding (eot_token is the highest number in each sequence)
+ x = x[torch.arange(x.shape[0]), seq_len]
+ x = self.proj(x)
+ return x
diff --git a/global_local/models/ImageBind/models/imagebind_model.py b/global_local/models/ImageBind/models/imagebind_model.py
new file mode 100644
index 0000000000000000000000000000000000000000..4430d2ea7a0acb19ca0bdf16dfebfc252164cccd
--- /dev/null
+++ b/global_local/models/ImageBind/models/imagebind_model.py
@@ -0,0 +1,541 @@
+#!/usr/bin/env python3
+# Portions Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+
+import os
+from functools import partial
+from types import SimpleNamespace
+
+import torch
+import torch.nn as nn
+
+from .helpers import (EinOpsRearrange, LearnableLogitScaling, Normalize,
+ SelectElement, SelectEOSAndProject)
+from .multimodal_preprocessors import (AudioPreprocessor,
+ IMUPreprocessor, PadIm2Video,
+ PatchEmbedGeneric,
+ RGBDTPreprocessor,
+ SpatioTemporalPosEmbeddingHelper,
+ TextPreprocessor,
+ ThermalPreprocessor)
+from .transformer import MultiheadAttention, SimpleTransformer
+
+ModalityType = SimpleNamespace(
+ VISION="vision",
+ TEXT="text",
+ AUDIO="audio",
+ THERMAL="thermal",
+ DEPTH="depth",
+ IMU="imu",
+)
+
+
+class ImageBindModel(nn.Module):
+ def __init__(
+ self,
+ video_frames=2,
+ kernel_size=(2, 14, 14),
+ audio_kernel_size=16,
+ audio_stride=10,
+ out_embed_dim=768,
+ vision_embed_dim=1024,
+ vision_num_blocks=24,
+ vision_num_heads=16,
+ audio_embed_dim=768,
+ audio_num_blocks=12,
+ audio_num_heads=12,
+ audio_num_mel_bins=128,
+ audio_target_len=204,
+ audio_drop_path=0.1,
+ text_embed_dim=768,
+ text_num_blocks=12,
+ text_num_heads=12,
+ depth_embed_dim=384,
+ depth_kernel_size=16,
+ depth_num_blocks=12,
+ depth_num_heads=8,
+ depth_drop_path=0.0,
+ thermal_embed_dim=768,
+ thermal_kernel_size=16,
+ thermal_num_blocks=12,
+ thermal_num_heads=12,
+ thermal_drop_path=0.0,
+ imu_embed_dim=512,
+ imu_kernel_size=8,
+ imu_num_blocks=6,
+ imu_num_heads=8,
+ imu_drop_path=0.7,
+ ):
+ super().__init__()
+
+ self.modality_preprocessors = self._create_modality_preprocessors(
+ video_frames,
+ vision_embed_dim,
+ kernel_size,
+ text_embed_dim,
+ audio_embed_dim,
+ audio_kernel_size,
+ audio_stride,
+ audio_num_mel_bins,
+ audio_target_len,
+ depth_embed_dim,
+ depth_kernel_size,
+ thermal_embed_dim,
+ thermal_kernel_size,
+ imu_embed_dim,
+ )
+
+ self.modality_trunks = self._create_modality_trunks(
+ vision_embed_dim,
+ vision_num_blocks,
+ vision_num_heads,
+ text_embed_dim,
+ text_num_blocks,
+ text_num_heads,
+ audio_embed_dim,
+ audio_num_blocks,
+ audio_num_heads,
+ audio_drop_path,
+ depth_embed_dim,
+ depth_num_blocks,
+ depth_num_heads,
+ depth_drop_path,
+ thermal_embed_dim,
+ thermal_num_blocks,
+ thermal_num_heads,
+ thermal_drop_path,
+ imu_embed_dim,
+ imu_num_blocks,
+ imu_num_heads,
+ imu_drop_path,
+ )
+
+ self.modality_heads = self._create_modality_heads(
+ out_embed_dim,
+ vision_embed_dim,
+ text_embed_dim,
+ audio_embed_dim,
+ depth_embed_dim,
+ thermal_embed_dim,
+ imu_embed_dim,
+ )
+
+ self.modality_postprocessors = self._create_modality_postprocessors(
+ out_embed_dim
+ )
+
+ def _create_modality_preprocessors(
+ self,
+ video_frames=2,
+ vision_embed_dim=1024,
+ kernel_size=(2, 14, 14),
+ text_embed_dim=768,
+ audio_embed_dim=768,
+ audio_kernel_size=16,
+ audio_stride=10,
+ audio_num_mel_bins=128,
+ audio_target_len=204,
+ depth_embed_dim=768,
+ depth_kernel_size=16,
+ thermal_embed_dim=768,
+ thermal_kernel_size=16,
+ imu_embed_dim=512,
+ ):
+ rgbt_stem = PatchEmbedGeneric(
+ proj_stem=[
+ PadIm2Video(pad_type="repeat", ntimes=2),
+ nn.Conv3d(
+ in_channels=3,
+ kernel_size=kernel_size,
+ out_channels=vision_embed_dim,
+ stride=kernel_size,
+ bias=False,
+ ),
+ ]
+ )
+ rgbt_preprocessor = RGBDTPreprocessor(
+ img_size=[3, video_frames, 224, 224],
+ num_cls_tokens=1,
+ pos_embed_fn=partial(SpatioTemporalPosEmbeddingHelper, learnable=True),
+ rgbt_stem=rgbt_stem,
+ depth_stem=None,
+ )
+
+ text_preprocessor = TextPreprocessor(
+ context_length=77,
+ vocab_size=49408,
+ embed_dim=text_embed_dim,
+ causal_masking=True,
+ )
+
+ audio_stem = PatchEmbedGeneric(
+ proj_stem=[
+ nn.Conv2d(
+ in_channels=1,
+ kernel_size=audio_kernel_size,
+ stride=audio_stride,
+ out_channels=audio_embed_dim,
+ bias=False,
+ ),
+ ],
+ norm_layer=nn.LayerNorm(normalized_shape=audio_embed_dim),
+ )
+ audio_preprocessor = AudioPreprocessor(
+ img_size=[1, audio_num_mel_bins, audio_target_len],
+ num_cls_tokens=1,
+ pos_embed_fn=partial(SpatioTemporalPosEmbeddingHelper, learnable=True),
+ audio_stem=audio_stem,
+ )
+
+ depth_stem = PatchEmbedGeneric(
+ [
+ nn.Conv2d(
+ kernel_size=depth_kernel_size,
+ in_channels=1,
+ out_channels=depth_embed_dim,
+ stride=depth_kernel_size,
+ bias=False,
+ ),
+ ],
+ norm_layer=nn.LayerNorm(normalized_shape=depth_embed_dim),
+ )
+
+ depth_preprocessor = RGBDTPreprocessor(
+ img_size=[1, 224, 224],
+ num_cls_tokens=1,
+ pos_embed_fn=partial(SpatioTemporalPosEmbeddingHelper, learnable=True),
+ rgbt_stem=None,
+ depth_stem=depth_stem,
+ )
+
+ thermal_stem = PatchEmbedGeneric(
+ [
+ nn.Conv2d(
+ kernel_size=thermal_kernel_size,
+ in_channels=1,
+ out_channels=thermal_embed_dim,
+ stride=thermal_kernel_size,
+ bias=False,
+ ),
+ ],
+ norm_layer=nn.LayerNorm(normalized_shape=thermal_embed_dim),
+ )
+ thermal_preprocessor = ThermalPreprocessor(
+ img_size=[1, 224, 224],
+ num_cls_tokens=1,
+ pos_embed_fn=partial(SpatioTemporalPosEmbeddingHelper, learnable=True),
+ thermal_stem=thermal_stem,
+ )
+
+ imu_stem = PatchEmbedGeneric(
+ [
+ nn.Linear(
+ in_features=48,
+ out_features=imu_embed_dim,
+ bias=False,
+ ),
+ ],
+ norm_layer=nn.LayerNorm(normalized_shape=imu_embed_dim),
+ )
+
+ imu_preprocessor = IMUPreprocessor(
+ img_size=[6, 2000],
+ num_cls_tokens=1,
+ kernel_size=8,
+ embed_dim=imu_embed_dim,
+ pos_embed_fn=partial(SpatioTemporalPosEmbeddingHelper, learnable=True),
+ imu_stem=imu_stem,
+ )
+
+ modality_preprocessors = {
+ ModalityType.VISION: rgbt_preprocessor,
+ ModalityType.TEXT: text_preprocessor,
+ ModalityType.AUDIO: audio_preprocessor,
+ ModalityType.DEPTH: depth_preprocessor,
+ ModalityType.THERMAL: thermal_preprocessor,
+ ModalityType.IMU: imu_preprocessor,
+ }
+
+ return nn.ModuleDict(modality_preprocessors)
+
+ def _create_modality_trunks(
+ self,
+ vision_embed_dim=1024,
+ vision_num_blocks=24,
+ vision_num_heads=16,
+ text_embed_dim=768,
+ text_num_blocks=12,
+ text_num_heads=12,
+ audio_embed_dim=768,
+ audio_num_blocks=12,
+ audio_num_heads=12,
+ audio_drop_path=0.0,
+ depth_embed_dim=768,
+ depth_num_blocks=12,
+ depth_num_heads=12,
+ depth_drop_path=0.0,
+ thermal_embed_dim=768,
+ thermal_num_blocks=12,
+ thermal_num_heads=12,
+ thermal_drop_path=0.0,
+ imu_embed_dim=512,
+ imu_num_blocks=6,
+ imu_num_heads=8,
+ imu_drop_path=0.7,
+ ):
+ def instantiate_trunk(
+ embed_dim, num_blocks, num_heads, pre_transformer_ln, add_bias_kv, drop_path
+ ):
+ return SimpleTransformer(
+ embed_dim=embed_dim,
+ num_blocks=num_blocks,
+ ffn_dropout_rate=0.0,
+ drop_path_rate=drop_path,
+ attn_target=partial(
+ MultiheadAttention,
+ embed_dim=embed_dim,
+ num_heads=num_heads,
+ bias=True,
+ add_bias_kv=add_bias_kv,
+ ),
+ pre_transformer_layer=nn.Sequential(
+ nn.LayerNorm(embed_dim, eps=1e-6)
+ if pre_transformer_ln
+ else nn.Identity(),
+ EinOpsRearrange("b l d -> l b d"),
+ ),
+ post_transformer_layer=EinOpsRearrange("l b d -> b l d"),
+ )
+
+ modality_trunks = {}
+ modality_trunks[ModalityType.VISION] = instantiate_trunk(
+ vision_embed_dim,
+ vision_num_blocks,
+ vision_num_heads,
+ pre_transformer_ln=True,
+ add_bias_kv=False,
+ drop_path=0.0,
+ )
+ modality_trunks[ModalityType.TEXT] = instantiate_trunk(
+ text_embed_dim,
+ text_num_blocks,
+ text_num_heads,
+ pre_transformer_ln=False,
+ add_bias_kv=False,
+ drop_path=0.0,
+ )
+ modality_trunks[ModalityType.AUDIO] = instantiate_trunk(
+ audio_embed_dim,
+ audio_num_blocks,
+ audio_num_heads,
+ pre_transformer_ln=False,
+ add_bias_kv=True,
+ drop_path=audio_drop_path,
+ )
+ modality_trunks[ModalityType.DEPTH] = instantiate_trunk(
+ depth_embed_dim,
+ depth_num_blocks,
+ depth_num_heads,
+ pre_transformer_ln=False,
+ add_bias_kv=True,
+ drop_path=depth_drop_path,
+ )
+ modality_trunks[ModalityType.THERMAL] = instantiate_trunk(
+ thermal_embed_dim,
+ thermal_num_blocks,
+ thermal_num_heads,
+ pre_transformer_ln=False,
+ add_bias_kv=True,
+ drop_path=thermal_drop_path,
+ )
+ modality_trunks[ModalityType.IMU] = instantiate_trunk(
+ imu_embed_dim,
+ imu_num_blocks,
+ imu_num_heads,
+ pre_transformer_ln=False,
+ add_bias_kv=True,
+ drop_path=imu_drop_path,
+ )
+
+ return nn.ModuleDict(modality_trunks)
+
+ def _create_modality_heads(
+ self,
+ out_embed_dim,
+ vision_embed_dim,
+ text_embed_dim,
+ audio_embed_dim,
+ depth_embed_dim,
+ thermal_embed_dim,
+ imu_embed_dim,
+ ):
+ modality_heads = {}
+
+ modality_heads[ModalityType.VISION] = nn.Sequential(
+ nn.LayerNorm(normalized_shape=vision_embed_dim, eps=1e-6),
+ SelectElement(index=0),
+ nn.Linear(vision_embed_dim, out_embed_dim, bias=False),
+ )
+
+ modality_heads[ModalityType.TEXT] = SelectEOSAndProject(
+ proj=nn.Sequential(
+ nn.LayerNorm(normalized_shape=text_embed_dim, eps=1e-6),
+ nn.Linear(text_embed_dim, out_embed_dim, bias=False),
+ )
+ )
+
+ modality_heads[ModalityType.AUDIO] = nn.Sequential(
+ nn.LayerNorm(normalized_shape=audio_embed_dim, eps=1e-6),
+ SelectElement(index=0),
+ nn.Linear(audio_embed_dim, out_embed_dim, bias=False),
+ )
+
+ modality_heads[ModalityType.DEPTH] = nn.Sequential(
+ nn.LayerNorm(normalized_shape=depth_embed_dim, eps=1e-6),
+ SelectElement(index=0),
+ nn.Linear(depth_embed_dim, out_embed_dim, bias=False),
+ )
+
+ modality_heads[ModalityType.THERMAL] = nn.Sequential(
+ nn.LayerNorm(normalized_shape=thermal_embed_dim, eps=1e-6),
+ SelectElement(index=0),
+ nn.Linear(thermal_embed_dim, out_embed_dim, bias=False),
+ )
+
+ modality_heads[ModalityType.IMU] = nn.Sequential(
+ nn.LayerNorm(normalized_shape=imu_embed_dim, eps=1e-6),
+ SelectElement(index=0),
+ nn.Dropout(p=0.5),
+ nn.Linear(imu_embed_dim, out_embed_dim, bias=False),
+ )
+
+ return nn.ModuleDict(modality_heads)
+
+ def _create_modality_postprocessors(self, out_embed_dim):
+ modality_postprocessors = {}
+
+ modality_postprocessors[ModalityType.VISION] = Normalize(dim=-1)
+ modality_postprocessors[ModalityType.TEXT] = nn.Sequential(
+ Normalize(dim=-1), LearnableLogitScaling(learnable=True)
+ )
+ modality_postprocessors[ModalityType.AUDIO] = nn.Sequential(
+ Normalize(dim=-1),
+ LearnableLogitScaling(logit_scale_init=20.0, learnable=False),
+ )
+ modality_postprocessors[ModalityType.DEPTH] = nn.Sequential(
+ Normalize(dim=-1),
+ LearnableLogitScaling(logit_scale_init=5.0, learnable=False),
+ )
+ modality_postprocessors[ModalityType.THERMAL] = nn.Sequential(
+ Normalize(dim=-1),
+ LearnableLogitScaling(logit_scale_init=10.0, learnable=False),
+ )
+ modality_postprocessors[ModalityType.IMU] = nn.Sequential(
+ Normalize(dim=-1),
+ LearnableLogitScaling(logit_scale_init=5.0, learnable=False),
+ )
+
+ return nn.ModuleDict(modality_postprocessors)
+
+ def forward(self, inputs):
+ outputs = {}
+ for modality_key, modality_value in inputs.items():
+ reduce_list = (
+ modality_value.ndim >= 5
+ ) # Audio and Video inputs consist of multiple clips
+ if reduce_list:
+ B, S = modality_value.shape[:2]
+ modality_value = modality_value.reshape(
+ B * S, *modality_value.shape[2:]
+ )
+
+ if modality_value is not None:
+ modality_value = self.modality_preprocessors[modality_key](
+ **{modality_key: modality_value}
+ )
+ trunk_inputs = modality_value["trunk"]
+ head_inputs = modality_value["head"]
+ modality_value = self.modality_trunks[modality_key](**trunk_inputs)
+ modality_value = self.modality_heads[modality_key](
+ modality_value, **head_inputs
+ )
+ modality_value = self.modality_postprocessors[modality_key](
+ modality_value
+ )
+
+ if reduce_list:
+ modality_value = modality_value.reshape(B, S, -1)
+ modality_value = modality_value.mean(dim=1)
+
+ outputs[modality_key] = modality_value
+ # modality_heads normalize 后768->linear 1024 ->
+ return outputs
+ def get_audio_feature(self, inputs, modality_type):
+ modality_value = inputs
+ modality_key = modality_type
+ reduce_list = (
+ modality_value.ndim >= 5
+ ) # Audio and Video inputs consist of multiple clips
+ if reduce_list:
+ B, S = modality_value.shape[:2]
+ modality_value = modality_value.reshape(
+ B * S, *modality_value.shape[2:]
+ )
+
+ if modality_value is not None:
+ modality_value = self.modality_preprocessors[modality_key](
+ **{modality_key: modality_value}
+ )
+ trunk_inputs = modality_value["trunk"]
+ head_inputs = modality_value["head"]
+ modality_value = self.modality_trunks[modality_key](**trunk_inputs)
+
+ audio_feature = self.modality_heads[modality_key][:-1](
+ modality_value, **head_inputs
+ )
+ modality_value = self.modality_heads[modality_key][-1:](
+ audio_feature, **head_inputs
+ )
+ modality_value = self.modality_postprocessors[modality_key](
+ modality_value
+ )
+
+ if reduce_list:
+ audio_feature = audio_feature.reshape(B, S, -1)
+ modality_value = modality_value.reshape(B, S, -1)
+ # modality_heads
+ return audio_feature, modality_value
+
+
+def imagebind_huge(pretrained=False):
+ model = ImageBindModel(
+ vision_embed_dim=1280,
+ vision_num_blocks=32,
+ vision_num_heads=16,
+ text_embed_dim=1024,
+ text_num_blocks=24,
+ text_num_heads=16,
+ out_embed_dim=1024,
+ audio_drop_path=0.1,
+ imu_drop_path=0.7,
+ )
+
+ if pretrained:
+ if not os.path.exists(".checkpoints/imagebind_huge.pth"):
+ print(
+ "Downloading imagebind weights to .checkpoints/imagebind_huge.pth ..."
+ )
+ os.makedirs(".checkpoints", exist_ok=True)
+ torch.hub.download_url_to_file(
+ "https://dl.fbaipublicfiles.com/imagebind/imagebind_huge.pth",
+ ".checkpoints/imagebind_huge.pth",
+ progress=True,
+ )
+
+ model.load_state_dict(torch.load(".checkpoints/imagebind_huge.pth"))
+
+ return model,1024
diff --git a/global_local/models/ImageBind/models/multimodal_preprocessors.py b/global_local/models/ImageBind/models/multimodal_preprocessors.py
new file mode 100644
index 0000000000000000000000000000000000000000..768c5b9c4f3f9b17b04ee41fec7ca2d99c15335e
--- /dev/null
+++ b/global_local/models/ImageBind/models/multimodal_preprocessors.py
@@ -0,0 +1,685 @@
+#!/usr/bin/env python3
+# Portions Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+import gzip
+import html
+import io
+import math
+from functools import lru_cache
+from typing import Callable, List, Optional, Tuple
+
+import ftfy
+import numpy as np
+import regex as re
+import torch
+import torch.nn as nn
+from iopath.common.file_io import g_pathmgr
+from timm.models.layers import trunc_normal_
+
+from .helpers import VerboseNNModule, cast_if_src_dtype
+
+
+def get_sinusoid_encoding_table(n_position, d_hid):
+ """Sinusoid position encoding table"""
+
+ # TODO: make it with torch instead of numpy
+ def get_position_angle_vec(position):
+ return [
+ position / np.power(10000, 2 * (hid_j // 2) / d_hid)
+ for hid_j in range(d_hid)
+ ]
+
+ sinusoid_table = np.array(
+ [get_position_angle_vec(pos_i) for pos_i in range(n_position)]
+ )
+ sinusoid_table[:, 0::2] = np.sin(sinusoid_table[:, 0::2]) # dim 2i
+ sinusoid_table[:, 1::2] = np.cos(sinusoid_table[:, 1::2]) # dim 2i+1
+
+ return torch.FloatTensor(sinusoid_table).unsqueeze(0)
+
+
+def interpolate_pos_encoding_2d(target_spatial_size, pos_embed):
+ N = pos_embed.shape[1]
+ if N == target_spatial_size:
+ return pos_embed
+ dim = pos_embed.shape[-1]
+ # nn.functional.interpolate doesn't work with bfloat16 so we cast to float32
+ pos_embed, updated = cast_if_src_dtype(pos_embed, torch.bfloat16, torch.float32)
+ pos_embed = nn.functional.interpolate(
+ pos_embed.reshape(1, int(math.sqrt(N)), int(math.sqrt(N)), dim).permute(
+ 0, 3, 1, 2
+ ),
+ scale_factor=math.sqrt(target_spatial_size / N),
+ mode="bicubic",
+ )
+ if updated:
+ pos_embed, _ = cast_if_src_dtype(pos_embed, torch.float32, torch.bfloat16)
+ pos_embed = pos_embed.permute(0, 2, 3, 1).view(1, -1, dim)
+ return pos_embed
+
+
+def interpolate_pos_encoding(
+ npatch_per_img,
+ pos_embed,
+ patches_layout,
+ input_shape=None,
+ first_patch_idx=1,
+):
+ assert first_patch_idx == 0 or first_patch_idx == 1, "there is 1 CLS token or none"
+ N = pos_embed.shape[1] - first_patch_idx # since it's 1 if cls_token exists
+ if npatch_per_img == N:
+ return pos_embed
+
+ assert (
+ patches_layout[-1] == patches_layout[-2]
+ ), "Interpolation of pos embed not supported for non-square layouts"
+
+ class_emb = pos_embed[:, :first_patch_idx]
+ pos_embed = pos_embed[:, first_patch_idx:]
+
+ if input_shape is None or patches_layout[0] == 1:
+ # simple 2D pos embedding, no temporal component
+ pos_embed = interpolate_pos_encoding_2d(npatch_per_img, pos_embed)
+ elif patches_layout[0] > 1:
+ # pos embed has a temporal component
+ assert len(input_shape) == 4, "temporal interpolation not supported"
+ # we only support 2D interpolation in this case
+ num_frames = patches_layout[0]
+ num_spatial_tokens = patches_layout[1] * patches_layout[2]
+ pos_embed = pos_embed.view(1, num_frames, num_spatial_tokens, -1)
+ # interpolate embedding for zeroth frame
+ pos_embed = interpolate_pos_encoding_2d(
+ npatch_per_img, pos_embed[0, 0, ...].unsqueeze(0)
+ )
+ else:
+ raise ValueError("This type of interpolation isn't implemented")
+
+ return torch.cat((class_emb, pos_embed), dim=1)
+
+
+def _get_pos_embedding(
+ npatch_per_img,
+ pos_embed,
+ patches_layout,
+ input_shape,
+ first_patch_idx=1,
+):
+ pos_embed = interpolate_pos_encoding(
+ npatch_per_img,
+ pos_embed,
+ patches_layout,
+ input_shape=input_shape,
+ first_patch_idx=first_patch_idx,
+ )
+ return pos_embed
+
+
+class PatchEmbedGeneric(nn.Module):
+ """
+ PatchEmbed from Hydra
+ """
+
+ def __init__(self, proj_stem, norm_layer: Optional[nn.Module] = None):
+ super().__init__()
+
+ if len(proj_stem) > 1:
+ self.proj = nn.Sequential(*proj_stem)
+ else:
+ # Special case to be able to load pre-trained models that were
+ # trained with a standard stem
+ self.proj = proj_stem[0]
+ self.norm_layer = norm_layer
+
+ def get_patch_layout(self, img_size):
+ with torch.no_grad():
+ dummy_img = torch.zeros(
+ [
+ 1,
+ ]
+ + img_size
+ )
+ dummy_out = self.proj(dummy_img)
+ embed_dim = dummy_out.shape[1]
+ patches_layout = tuple(dummy_out.shape[2:])
+ num_patches = np.prod(patches_layout)
+ return patches_layout, num_patches, embed_dim
+
+ def forward(self, x):
+ x = self.proj(x)
+ # B C (T) H W -> B (T)HW C
+ x = x.flatten(2).transpose(1, 2)
+ if self.norm_layer is not None:
+ x = self.norm_layer(x)
+ return x
+
+
+class SpatioTemporalPosEmbeddingHelper(VerboseNNModule):
+ def __init__(
+ self,
+ patches_layout: List,
+ num_patches: int,
+ num_cls_tokens: int,
+ embed_dim: int,
+ learnable: bool,
+ ) -> None:
+ super().__init__()
+ self.num_cls_tokens = num_cls_tokens
+ self.patches_layout = patches_layout
+ self.num_patches = num_patches
+ self.num_tokens = num_cls_tokens + num_patches
+ self.learnable = learnable
+ if self.learnable:
+ self.pos_embed = nn.Parameter(torch.zeros(1, self.num_tokens, embed_dim))
+ trunc_normal_(self.pos_embed, std=0.02)
+ else:
+ self.register_buffer(
+ "pos_embed", get_sinusoid_encoding_table(self.num_tokens, embed_dim)
+ )
+
+ def get_pos_embedding(self, vision_input, all_vision_tokens):
+ input_shape = vision_input.shape
+ pos_embed = _get_pos_embedding(
+ all_vision_tokens.size(1) - self.num_cls_tokens,
+ pos_embed=self.pos_embed,
+ patches_layout=self.patches_layout,
+ input_shape=input_shape,
+ first_patch_idx=self.num_cls_tokens,
+ )
+ return pos_embed
+
+
+class RGBDTPreprocessor(VerboseNNModule):
+ def __init__(
+ self,
+ rgbt_stem: PatchEmbedGeneric,
+ depth_stem: Optional[PatchEmbedGeneric],
+ img_size: Tuple = (3, 224, 224),
+ num_cls_tokens: int = 1,
+ pos_embed_fn: Optional[Callable] = None,
+ use_type_embed: bool = False,
+ init_param_style: str = "openclip",
+ ) -> None:
+ super().__init__()
+ stem = rgbt_stem if rgbt_stem is not None else depth_stem
+ (
+ self.patches_layout,
+ self.num_patches,
+ self.embed_dim,
+ ) = stem.get_patch_layout(img_size)
+ self.rgbt_stem = rgbt_stem
+ self.depth_stem = depth_stem
+ self.use_pos_embed = pos_embed_fn is not None
+ self.use_type_embed = use_type_embed
+ self.num_cls_tokens = num_cls_tokens
+
+ if self.use_pos_embed:
+ self.pos_embedding_helper = pos_embed_fn(
+ patches_layout=self.patches_layout,
+ num_cls_tokens=num_cls_tokens,
+ num_patches=self.num_patches,
+ embed_dim=self.embed_dim,
+ )
+ if self.num_cls_tokens > 0:
+ self.cls_token = nn.Parameter(
+ torch.zeros(1, self.num_cls_tokens, self.embed_dim)
+ )
+ if self.use_type_embed:
+ self.type_embed = nn.Parameter(torch.zeros(1, 1, self.embed_dim))
+
+ self.init_parameters(init_param_style)
+
+ @torch.no_grad()
+ def init_parameters(self, init_param_style):
+ if init_param_style == "openclip":
+ # OpenCLIP style initialization
+ scale = self.embed_dim**-0.5
+ if self.use_pos_embed:
+ nn.init.normal_(self.pos_embedding_helper.pos_embed)
+ self.pos_embedding_helper.pos_embed *= scale
+
+ if self.num_cls_tokens > 0:
+ nn.init.normal_(self.cls_token)
+ self.cls_token *= scale
+ elif init_param_style == "vit":
+ self.cls_token.data.fill_(0)
+ else:
+ raise ValueError(f"Unknown init {init_param_style}")
+
+ if self.use_type_embed:
+ nn.init.normal_(self.type_embed)
+
+ def tokenize_input_and_cls_pos(self, input, stem, mask):
+ # tokens is of shape B x L x D
+ tokens = stem(input)
+ assert tokens.ndim == 3
+ assert tokens.shape[2] == self.embed_dim
+ B = tokens.shape[0]
+ if self.num_cls_tokens > 0:
+ class_tokens = self.cls_token.expand(
+ B, -1, -1
+ ) # stole class_tokens impl from Phil Wang, thanks
+ tokens = torch.cat((class_tokens, tokens), dim=1)
+ if self.use_pos_embed:
+ pos_embed = self.pos_embedding_helper.get_pos_embedding(input, tokens)
+ tokens = tokens + pos_embed
+ if self.use_type_embed:
+ tokens = tokens + self.type_embed.expand(B, -1, -1)
+ return tokens
+
+ def forward(self, vision=None, depth=None, patch_mask=None):
+ if patch_mask is not None:
+ raise NotImplementedError()
+
+ if vision is not None:
+ vision_tokens = self.tokenize_input_and_cls_pos(
+ vision, self.rgbt_stem, patch_mask
+ )
+
+ if depth is not None:
+ depth_tokens = self.tokenize_input_and_cls_pos(
+ depth, self.depth_stem, patch_mask
+ )
+
+ # aggregate tokens
+ if vision is not None and depth is not None:
+ final_tokens = vision_tokens + depth_tokens
+ else:
+ final_tokens = vision_tokens if vision is not None else depth_tokens
+ return_dict = {
+ "trunk": {
+ "tokens": final_tokens,
+ },
+ "head": {},
+ }
+ return return_dict
+
+
+class AudioPreprocessor(RGBDTPreprocessor):
+ def __init__(self, audio_stem: PatchEmbedGeneric, **kwargs) -> None:
+ super().__init__(rgbt_stem=audio_stem, depth_stem=None, **kwargs)
+
+ def forward(self, audio=None):
+ return super().forward(vision=audio)
+
+
+class ThermalPreprocessor(RGBDTPreprocessor):
+ def __init__(self, thermal_stem: PatchEmbedGeneric, **kwargs) -> None:
+ super().__init__(rgbt_stem=thermal_stem, depth_stem=None, **kwargs)
+
+ def forward(self, thermal=None):
+ return super().forward(vision=thermal)
+
+
+def build_causal_attention_mask(context_length):
+ # lazily create causal attention mask, with full attention between the vision tokens
+ # pytorch uses additive attention mask; fill with -inf
+ mask = torch.empty(context_length, context_length, requires_grad=False)
+ mask.fill_(float("-inf"))
+ mask.triu_(1) # zero out the lower diagonal
+ return mask
+
+
+class TextPreprocessor(VerboseNNModule):
+ def __init__(
+ self,
+ vocab_size: int,
+ context_length: int,
+ embed_dim: int,
+ causal_masking: bool,
+ supply_seq_len_to_head: bool = True,
+ num_cls_tokens: int = 0,
+ init_param_style: str = "openclip",
+ ) -> None:
+ super().__init__()
+ self.vocab_size = vocab_size
+ self.context_length = context_length
+ self.token_embedding = nn.Embedding(vocab_size, embed_dim)
+ self.pos_embed = nn.Parameter(
+ torch.empty(1, self.context_length + num_cls_tokens, embed_dim)
+ )
+ self.causal_masking = causal_masking
+ if self.causal_masking:
+ mask = build_causal_attention_mask(self.context_length)
+ # register the mask as a buffer so it can be moved to the right device
+ self.register_buffer("mask", mask)
+
+ self.supply_seq_len_to_head = supply_seq_len_to_head
+ self.num_cls_tokens = num_cls_tokens
+ self.embed_dim = embed_dim
+ if num_cls_tokens > 0:
+ assert self.causal_masking is False, "Masking + CLS token isn't implemented"
+ self.cls_token = nn.Parameter(
+ torch.zeros(1, self.num_cls_tokens, embed_dim)
+ )
+
+ self.init_parameters(init_param_style)
+
+ @torch.no_grad()
+ def init_parameters(self, init_param_style="openclip"):
+ # OpenCLIP style initialization
+ nn.init.normal_(self.token_embedding.weight, std=0.02)
+ nn.init.normal_(self.pos_embed, std=0.01)
+
+ if init_param_style == "openclip":
+ # OpenCLIP style initialization
+ scale = self.embed_dim**-0.5
+ if self.num_cls_tokens > 0:
+ nn.init.normal_(self.cls_token)
+ self.cls_token *= scale
+ elif init_param_style == "vit":
+ self.cls_token.data.fill_(0)
+ else:
+ raise ValueError(f"Unknown init {init_param_style}")
+
+ def forward(self, text):
+ # text tokens are of shape B x L x D
+ text_tokens = self.token_embedding(text)
+ # concat CLS tokens if any
+ if self.num_cls_tokens > 0:
+ B = text_tokens.shape[0]
+ class_tokens = self.cls_token.expand(
+ B, -1, -1
+ ) # stole class_tokens impl from Phil Wang, thanks
+ text_tokens = torch.cat((class_tokens, text_tokens), dim=1)
+ text_tokens = text_tokens + self.pos_embed
+ return_dict = {
+ "trunk": {
+ "tokens": text_tokens,
+ },
+ "head": {},
+ }
+ # Compute sequence length after adding CLS tokens
+ if self.supply_seq_len_to_head:
+ text_lengths = text.argmax(dim=-1)
+ return_dict["head"] = {
+ "seq_len": text_lengths,
+ }
+ if self.causal_masking:
+ return_dict["trunk"].update({"attn_mask": self.mask})
+ return return_dict
+
+
+class Im2Video(nn.Module):
+ """Convert an image into a trivial video."""
+
+ def __init__(self, time_dim=2):
+ super().__init__()
+ self.time_dim = time_dim
+
+ def forward(self, x):
+ if x.ndim == 4:
+ # B, C, H, W -> B, C, T, H, W
+ return x.unsqueeze(self.time_dim)
+ elif x.ndim == 5:
+ return x
+ else:
+ raise ValueError(f"Dimension incorrect {x.shape}")
+
+
+class PadIm2Video(Im2Video):
+ def __init__(self, ntimes, pad_type, time_dim=2):
+ super().__init__(time_dim=time_dim)
+ assert ntimes > 0
+ assert pad_type in ["zero", "repeat"]
+ self.ntimes = ntimes
+ self.pad_type = pad_type
+
+ def forward(self, x):
+ x = super().forward(x)
+ if x.shape[self.time_dim] == 1:
+ if self.pad_type == "repeat":
+ new_shape = [1] * len(x.shape)
+ new_shape[self.time_dim] = self.ntimes
+ x = x.repeat(new_shape)
+ elif self.pad_type == "zero":
+ padarg = [0, 0] * len(x.shape)
+ padarg[2 * self.time_dim + 1] = self.ntimes - x.shape[self.time_dim]
+ x = nn.functional.pad(x, padarg)
+ return x
+
+
+# Modified from github.com/openai/CLIP
+@lru_cache()
+def bytes_to_unicode():
+ """
+ Returns list of utf-8 byte and a corresponding list of unicode strings.
+ The reversible bpe codes work on unicode strings.
+ This means you need a large # of unicode characters in your vocab if you want to avoid UNKs.
+ When you're at something like a 10B token dataset you end up needing around 5K for decent coverage.
+ This is a signficant percentage of your normal, say, 32K bpe vocab.
+ To avoid that, we want lookup tables between utf-8 bytes and unicode strings.
+ And avoids mapping to whitespace/control characters the bpe code barfs on.
+ """
+ bs = (
+ list(range(ord("!"), ord("~") + 1))
+ + list(range(ord("¡"), ord("¬") + 1))
+ + list(range(ord("®"), ord("ÿ") + 1))
+ )
+ cs = bs[:]
+ n = 0
+ for b in range(2**8):
+ if b not in bs:
+ bs.append(b)
+ cs.append(2**8 + n)
+ n += 1
+ cs = [chr(n) for n in cs]
+ return dict(zip(bs, cs))
+
+
+def get_pairs(word):
+ """Return set of symbol pairs in a word.
+ Word is represented as tuple of symbols (symbols being variable-length strings).
+ """
+ pairs = set()
+ prev_char = word[0]
+ for char in word[1:]:
+ pairs.add((prev_char, char))
+ prev_char = char
+ return pairs
+
+
+def basic_clean(text):
+ text = ftfy.fix_text(text)
+ text = html.unescape(html.unescape(text))
+ return text.strip()
+
+
+def whitespace_clean(text):
+ text = re.sub(r"\s+", " ", text)
+ text = text.strip()
+ return text
+
+
+class SimpleTokenizer(object):
+ def __init__(self, bpe_path: str, context_length=77):
+ self.byte_encoder = bytes_to_unicode()
+ self.byte_decoder = {v: k for k, v in self.byte_encoder.items()}
+
+ with g_pathmgr.open(bpe_path, "rb") as fh:
+ bpe_bytes = io.BytesIO(fh.read())
+ merges: List[str] = gzip.open(bpe_bytes).read().decode("utf-8").split("\n")
+ merges = merges[1 : 49152 - 256 - 2 + 1]
+ merges: List[Tuple[str, ...]] = [tuple(merge.split()) for merge in merges]
+ vocab = list(bytes_to_unicode().values())
+ vocab = vocab + [v + "" for v in vocab]
+ for merge in merges:
+ vocab.append("".join(merge))
+ vocab.extend(["<|startoftext|>", "<|endoftext|>"])
+ self.encoder = dict(zip(vocab, range(len(vocab))))
+ self.decoder = {v: k for k, v in self.encoder.items()}
+ self.bpe_ranks = dict(zip(merges, range(len(merges))))
+ self.cache = {
+ "<|startoftext|>": "<|startoftext|>",
+ "<|endoftext|>": "<|endoftext|>",
+ }
+ self.pat = re.compile(
+ r"""<\|startoftext\|>|<\|endoftext\|>|'s|'t|'re|'ve|'m|'ll|'d|[\p{L}]+|[\p{N}]|[^\s\p{L}\p{N}]+""",
+ re.IGNORECASE,
+ )
+ self.context_length = context_length
+
+ def bpe(self, token):
+ if token in self.cache:
+ return self.cache[token]
+ word = tuple(token[:-1]) + (token[-1] + "",)
+ pairs = get_pairs(word)
+
+ if not pairs:
+ return token + ""
+
+ while True:
+ bigram = min(pairs, key=lambda pair: self.bpe_ranks.get(pair, float("inf")))
+ if bigram not in self.bpe_ranks:
+ break
+ first, second = bigram
+ new_word = []
+ i = 0
+ while i < len(word):
+ try:
+ j = word.index(first, i)
+ new_word.extend(word[i:j])
+ i = j
+ except:
+ new_word.extend(word[i:])
+ break
+
+ if word[i] == first and i < len(word) - 1 and word[i + 1] == second:
+ new_word.append(first + second)
+ i += 2
+ else:
+ new_word.append(word[i])
+ i += 1
+ new_word = tuple(new_word)
+ word = new_word
+ if len(word) == 1:
+ break
+ else:
+ pairs = get_pairs(word)
+ word = " ".join(word)
+ self.cache[token] = word
+ return word
+
+ def encode(self, text):
+ bpe_tokens = []
+ text = whitespace_clean(basic_clean(text)).lower()
+ for token in re.findall(self.pat, text):
+ token = "".join(self.byte_encoder[b] for b in token.encode("utf-8"))
+ bpe_tokens.extend(
+ self.encoder[bpe_token] for bpe_token in self.bpe(token).split(" ")
+ )
+ return bpe_tokens
+
+ def decode(self, tokens):
+ text = "".join([self.decoder[token] for token in tokens])
+ text = (
+ bytearray([self.byte_decoder[c] for c in text])
+ .decode("utf-8", errors="replace")
+ .replace("", " ")
+ )
+ return text
+
+ def __call__(self, texts, context_length=None):
+ if not context_length:
+ context_length = self.context_length
+
+ if isinstance(texts, str):
+ texts = [texts]
+
+ sot_token = self.encoder["<|startoftext|>"]
+ eot_token = self.encoder["<|endoftext|>"]
+ all_tokens = [[sot_token] + self.encode(text) + [eot_token] for text in texts]
+ result = torch.zeros(len(all_tokens), context_length, dtype=torch.long)
+
+ for i, tokens in enumerate(all_tokens):
+ tokens = tokens[:context_length]
+ result[i, : len(tokens)] = torch.tensor(tokens)
+
+ if len(result) == 1:
+ return result[0]
+ return result
+
+
+class IMUPreprocessor(VerboseNNModule):
+ def __init__(
+ self,
+ kernel_size: int,
+ imu_stem: PatchEmbedGeneric,
+ embed_dim: int,
+ img_size: Tuple = (6, 2000),
+ num_cls_tokens: int = 1,
+ pos_embed_fn: Optional[Callable] = None,
+ init_param_style: str = "openclip",
+ ) -> None:
+ super().__init__()
+ self.imu_stem = imu_stem
+ self.embed_dim = embed_dim
+ self.use_pos_embed = pos_embed_fn is not None
+ self.num_cls_tokens = num_cls_tokens
+ self.kernel_size = kernel_size
+ self.pos_embed = nn.Parameter(
+ torch.empty(1, (img_size[1] // kernel_size) + num_cls_tokens, embed_dim)
+ )
+
+ if self.num_cls_tokens > 0:
+ self.cls_token = nn.Parameter(
+ torch.zeros(1, self.num_cls_tokens, self.embed_dim)
+ )
+
+ self.init_parameters(init_param_style)
+
+ @torch.no_grad()
+ def init_parameters(self, init_param_style):
+ nn.init.normal_(self.pos_embed, std=0.01)
+
+ if init_param_style == "openclip":
+ # OpenCLIP style initialization
+ scale = self.embed_dim**-0.5
+
+ if self.num_cls_tokens > 0:
+ nn.init.normal_(self.cls_token)
+ self.cls_token *= scale
+ elif init_param_style == "vit":
+ self.cls_token.data.fill_(0)
+ else:
+ raise ValueError(f"Unknown init {init_param_style}")
+
+ def tokenize_input_and_cls_pos(self, input, stem):
+ # tokens is of shape B x L x D
+ tokens = stem.norm_layer(stem.proj(input))
+ assert tokens.ndim == 3
+ assert tokens.shape[2] == self.embed_dim
+ B = tokens.shape[0]
+ if self.num_cls_tokens > 0:
+ class_tokens = self.cls_token.expand(
+ B, -1, -1
+ ) # stole class_tokens impl from Phil Wang, thanks
+ tokens = torch.cat((class_tokens, tokens), dim=1)
+ if self.use_pos_embed:
+ tokens = tokens + self.pos_embed
+ return tokens
+
+ def forward(self, imu):
+ # Patchify
+ imu = imu.unfold(
+ -1,
+ self.kernel_size,
+ self.kernel_size,
+ ).permute(0, 2, 1, 3)
+ imu = imu.reshape(imu.size(0), imu.size(1), -1)
+
+ imu_tokens = self.tokenize_input_and_cls_pos(
+ imu,
+ self.imu_stem,
+ )
+
+ return_dict = {
+ "trunk": {
+ "tokens": imu_tokens,
+ },
+ "head": {},
+ }
+ return return_dict
diff --git a/global_local/models/ImageBind/models/transformer.py b/global_local/models/ImageBind/models/transformer.py
new file mode 100644
index 0000000000000000000000000000000000000000..6224faf89d620de010d148bd50dae85176995031
--- /dev/null
+++ b/global_local/models/ImageBind/models/transformer.py
@@ -0,0 +1,280 @@
+#!/usr/bin/env python3
+# Portions Copyright (c) Meta Platforms, Inc. and affiliates.
+# All rights reserved.
+
+# This source code is licensed under the license found in the
+# LICENSE file in the root directory of this source tree.
+
+# Code modified from
+# https://github.com/rwightman/pytorch-image-models/blob/master/timm/models/vision_transformer.py ;
+# https://github.com/facebookresearch/deit/blob/main/models.py
+# and https://github.com/facebookresearch/vissl/blob/main/vissl/models/trunks/vision_transformer.py
+
+
+from functools import partial
+from typing import Callable, List, Optional
+
+import torch
+import torch.nn as nn
+import torch.utils.checkpoint as checkpoint
+from timm.models.layers import DropPath, trunc_normal_
+
+
+class Attention(nn.Module):
+ def __init__(
+ self,
+ dim,
+ num_heads=8,
+ qkv_bias=False,
+ qk_scale=None,
+ attn_drop=0.0,
+ proj_drop=0.0,
+ ):
+ super().__init__()
+ self.num_heads = num_heads
+ head_dim = dim // num_heads
+ # NOTE scale factor was wrong in my original version,
+ # can set manually to be compat with prev weights
+ self.scale = qk_scale or head_dim**-0.5
+
+ self.qkv = nn.Linear(dim, dim * 3, bias=qkv_bias)
+ self.attn_drop = nn.Dropout(attn_drop)
+ self.proj = nn.Linear(dim, dim)
+ self.proj_drop = nn.Dropout(proj_drop)
+
+ def forward(self, x):
+ B, N, C = x.shape
+ qkv = (
+ self.qkv(x)
+ .reshape(B, N, 3, self.num_heads, C // self.num_heads)
+ .permute(2, 0, 3, 1, 4)
+ )
+ q, k, v = (
+ qkv[0],
+ qkv[1],
+ qkv[2],
+ ) # make torchscript happy (cannot use tensor as tuple)
+
+ attn = (q @ k.transpose(-2, -1)) * self.scale
+ attn = attn.softmax(dim=-1)
+ attn = self.attn_drop(attn)
+
+ x = (attn @ v).transpose(1, 2).reshape(B, N, C)
+ x = self.proj(x)
+ x = self.proj_drop(x)
+ return x
+
+
+class Mlp(nn.Module):
+ def __init__(
+ self,
+ in_features,
+ hidden_features=None,
+ out_features=None,
+ act_layer=nn.GELU,
+ drop=0.0,
+ ):
+ super().__init__()
+ out_features = out_features or in_features
+ hidden_features = hidden_features or in_features
+ self.fc1 = nn.Linear(in_features, hidden_features)
+ self.act = act_layer()
+ self.fc2 = nn.Linear(hidden_features, out_features)
+ self.drop = nn.Dropout(drop)
+
+ def forward(self, x):
+ x = self.fc1(x)
+ x = self.act(x)
+ x = self.drop(x)
+ x = self.fc2(x)
+ x = self.drop(x)
+ return x
+
+
+class MultiheadAttention(nn.MultiheadAttention):
+ def forward(self, x: torch.Tensor, attn_mask: torch.Tensor):
+ return super().forward(x, x, x, need_weights=False, attn_mask=attn_mask)[0]
+
+
+class ViTAttention(Attention):
+ def forward(self, x: torch.Tensor, attn_mask: torch.Tensor):
+ assert attn_mask is None
+ return super().forward(x)
+
+
+class BlockWithMasking(nn.Module):
+ def __init__(
+ self,
+ dim: int,
+ attn_target: Callable,
+ mlp_ratio: int = 4,
+ act_layer: Callable = nn.GELU,
+ norm_layer: Callable = nn.LayerNorm,
+ ffn_dropout_rate: float = 0.0,
+ drop_path: float = 0.0,
+ layer_scale_type: Optional[str] = None,
+ layer_scale_init_value: float = 1e-4,
+ ):
+ super().__init__()
+
+ assert not isinstance(
+ attn_target, nn.Module
+ ), "attn_target should be a Callable. Otherwise attn_target is shared across blocks!"
+ self.attn = attn_target()
+ if drop_path > 0.0:
+ self.drop_path = DropPath(drop_path)
+ else:
+ self.drop_path = nn.Identity()
+ self.norm_1 = norm_layer(dim)
+ mlp_hidden_dim = int(mlp_ratio * dim)
+ self.mlp = Mlp(
+ in_features=dim,
+ hidden_features=mlp_hidden_dim,
+ act_layer=act_layer,
+ drop=ffn_dropout_rate,
+ )
+ self.norm_2 = norm_layer(dim)
+ self.layer_scale_type = layer_scale_type
+ if self.layer_scale_type is not None:
+ assert self.layer_scale_type in [
+ "per_channel",
+ "scalar",
+ ], f"Found Layer scale type {self.layer_scale_type}"
+ if self.layer_scale_type == "per_channel":
+ # one gamma value per channel
+ gamma_shape = [1, 1, dim]
+ elif self.layer_scale_type == "scalar":
+ # single gamma value for all channels
+ gamma_shape = [1, 1, 1]
+ # two gammas: for each part of the fwd in the encoder
+ self.layer_scale_gamma1 = nn.Parameter(
+ torch.ones(size=gamma_shape) * layer_scale_init_value,
+ requires_grad=True,
+ )
+ self.layer_scale_gamma2 = nn.Parameter(
+ torch.ones(size=gamma_shape) * layer_scale_init_value,
+ requires_grad=True,
+ )
+
+ def forward(self, x: torch.Tensor, attn_mask: torch.Tensor):
+ if self.layer_scale_type is None:
+ x = x + self.drop_path(self.attn(self.norm_1(x), attn_mask))
+ x = x + self.drop_path(self.mlp(self.norm_2(x)))
+ else:
+ x = (
+ x
+ + self.drop_path(self.attn(self.norm_1(x), attn_mask))
+ * self.layer_scale_gamma1
+ )
+ x = x + self.drop_path(self.mlp(self.norm_2(x))) * self.layer_scale_gamma2
+ return x
+
+
+_LAYER_NORM = partial(nn.LayerNorm, eps=1e-6)
+
+
+class SimpleTransformer(nn.Module):
+ def __init__(
+ self,
+ attn_target: Callable,
+ embed_dim: int,
+ num_blocks: int,
+ block: Callable = BlockWithMasking,
+ pre_transformer_layer: Optional[Callable] = None,
+ post_transformer_layer: Optional[Callable] = None,
+ drop_path_rate: float = 0.0,
+ drop_path_type: str = "progressive",
+ norm_layer: Callable = _LAYER_NORM,
+ mlp_ratio: int = 4,
+ ffn_dropout_rate: float = 0.0,
+ layer_scale_type: Optional[str] = None, # from cait; possible values are None, "per_channel", "scalar"
+ layer_scale_init_value: float = 1e-4, # from cait; float
+ weight_init_style: str = "jax", # possible values jax or pytorch
+ ):
+ """
+ Simple Transformer with the following features
+ 1. Supports masked attention
+ 2. Supports DropPath
+ 3. Supports LayerScale
+ 4. Supports Dropout in Attention and FFN
+ 5. Makes few assumptions about the input except that it is a Tensor
+ """
+ super().__init__()
+ self.pre_transformer_layer = pre_transformer_layer
+ if drop_path_type == "progressive":
+ dpr = [x.item() for x in torch.linspace(0, drop_path_rate, num_blocks)]
+ elif drop_path_type == "uniform":
+ dpr = [drop_path_rate for i in range(num_blocks)]
+ else:
+ raise ValueError(f"Unknown drop_path_type: {drop_path_type}")
+
+ self.blocks = nn.Sequential(
+ *[
+ block(
+ dim=embed_dim,
+ attn_target=attn_target,
+ mlp_ratio=mlp_ratio,
+ ffn_dropout_rate=ffn_dropout_rate,
+ drop_path=dpr[i],
+ norm_layer=norm_layer,
+ layer_scale_type=layer_scale_type,
+ layer_scale_init_value=layer_scale_init_value,
+ )
+ for i in range(num_blocks)
+ ]
+ )
+ self.post_transformer_layer = post_transformer_layer
+ self.weight_init_style = weight_init_style
+ self.apply(self._init_weights)
+
+ def _init_weights(self, m):
+ if isinstance(m, nn.Linear):
+ if self.weight_init_style == "jax":
+ # Based on MAE and official Jax ViT implementation
+ torch.nn.init.xavier_uniform_(m.weight)
+ elif self.weight_init_style == "pytorch":
+ # PyTorch ViT uses trunc_normal_
+ trunc_normal_(m.weight, std=0.02)
+
+ if m.bias is not None:
+ nn.init.constant_(m.bias, 0)
+ elif isinstance(m, (nn.LayerNorm)):
+ nn.init.constant_(m.bias, 0)
+ nn.init.constant_(m.weight, 1.0)
+
+ def forward(
+ self,
+ tokens: torch.Tensor,
+ attn_mask: torch.Tensor = None,
+ use_checkpoint: bool = False,
+ checkpoint_every_n: int = 1,
+ checkpoint_blk_ids: Optional[List[int]] = None,
+ ):
+ """
+ Inputs
+ - tokens: data of shape N x L x D (or L x N x D depending on the attention implementation)
+ - attn: mask of shape L x L
+
+ Output
+ - x: data of shape N x L x D (or L x N x D depending on the attention implementation)
+ """
+ if self.pre_transformer_layer:
+ tokens = self.pre_transformer_layer(tokens)
+ if use_checkpoint and checkpoint_blk_ids is None:
+ checkpoint_blk_ids = [
+ blk_id
+ for blk_id in range(len(self.blocks))
+ if blk_id % checkpoint_every_n == 0
+ ]
+ if checkpoint_blk_ids:
+ checkpoint_blk_ids = set(checkpoint_blk_ids)
+ for blk_id, blk in enumerate(self.blocks):
+ if use_checkpoint and blk_id in checkpoint_blk_ids:
+ tokens = checkpoint.checkpoint(
+ blk, tokens, attn_mask, use_reentrant=False
+ )
+ else:
+ tokens = blk(tokens, attn_mask=attn_mask)
+ if self.post_transformer_layer:
+ tokens = self.post_transformer_layer(tokens)
+ return tokens
diff --git a/global_local/models/ImageBind/requirements.txt b/global_local/models/ImageBind/requirements.txt
new file mode 100644
index 0000000000000000000000000000000000000000..d35cb65aedcc46b805aac9328ca2e0e246a76dae
--- /dev/null
+++ b/global_local/models/ImageBind/requirements.txt
@@ -0,0 +1,17 @@
+--extra-index-url https://download.pytorch.org/whl/cu113
+torch==1.13.0
+torchvision==0.14.0
+torchaudio==0.13.0
+pytorchvideo @ git+https://github.com/facebookresearch/pytorchvideo.git@28fe037d212663c6a24f373b94cc5d478c8c1a1d
+timm==0.6.7
+ftfy
+regex
+einops
+fvcore
+decord==0.6.0
+iopath
+numpy
+matplotlib
+types-regex
+mayavi
+cartopy
diff --git a/global_local/models/Qformer.py b/global_local/models/Qformer.py
new file mode 100644
index 0000000000000000000000000000000000000000..4902165ec6574d89f04cbeb2141b018278324ca6
--- /dev/null
+++ b/global_local/models/Qformer.py
@@ -0,0 +1,1217 @@
+"""
+Adapted from salesforce@LAVIS. Below is the original copyright:
+ * Copyright (c) 2023, salesforce.com, inc.
+ * All rights reserved.
+ * SPDX-License-Identifier: BSD-3-Clause
+ * For full license text, see LICENSE.txt file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+ * By Junnan Li
+ * Based on huggingface code base
+ * https://github.com/huggingface/transformers/blob/v4.15.0/src/transformers/models/bert
+"""
+
+import math
+import os
+import warnings
+from dataclasses import dataclass
+from typing import Optional, Tuple, Dict, Any
+
+import torch
+from torch import Tensor, device, dtype, nn
+import torch.utils.checkpoint
+from torch import nn
+from torch.nn import CrossEntropyLoss
+import torch.nn.functional as F
+
+from transformers.activations import ACT2FN
+from transformers.file_utils import (
+ ModelOutput,
+)
+from transformers.modeling_outputs import (
+ BaseModelOutputWithPastAndCrossAttentions,
+ BaseModelOutputWithPoolingAndCrossAttentions,
+ CausalLMOutputWithCrossAttentions,
+ MaskedLMOutput,
+ MultipleChoiceModelOutput,
+ NextSentencePredictorOutput,
+ QuestionAnsweringModelOutput,
+ SequenceClassifierOutput,
+ TokenClassifierOutput,
+)
+from transformers.modeling_utils import (
+ PreTrainedModel,
+ apply_chunking_to_forward,
+ find_pruneable_heads_and_indices,
+ prune_linear_layer,
+)
+from transformers.utils import logging
+from transformers.models.bert.configuration_bert import BertConfig
+
+logger = logging.get_logger(__name__)
+
+
+class BertEmbeddings(nn.Module):
+ """Construct the embeddings from word and position embeddings."""
+
+ def __init__(self, config):
+ super().__init__()
+ self.word_embeddings = nn.Embedding(
+ config.vocab_size, config.hidden_size, padding_idx=config.pad_token_id
+ )
+ self.position_embeddings = nn.Embedding(
+ config.max_position_embeddings, config.hidden_size
+ )
+
+ # self.LayerNorm is not snake-cased to stick with TensorFlow model variable name and be able to load
+ # any TensorFlow checkpoint file
+ self.LayerNorm = nn.LayerNorm(config.hidden_size, eps=config.layer_norm_eps)
+ self.dropout = nn.Dropout(config.hidden_dropout_prob)
+
+ # position_ids (1, len position emb) is contiguous in memory and exported when serialized
+ self.register_buffer(
+ "position_ids", torch.arange(config.max_position_embeddings).expand((1, -1))
+ )
+ self.position_embedding_type = getattr(
+ config, "position_embedding_type", "absolute"
+ )
+
+ self.config = config
+
+ def forward(
+ self,
+ input_ids=None,
+ position_ids=None,
+ query_embeds=None,
+ past_key_values_length=0,
+ ):
+ if input_ids is not None:
+ seq_length = input_ids.size()[1]
+ else:
+ seq_length = 0
+
+ if position_ids is None:
+ position_ids = self.position_ids[
+ :, past_key_values_length : seq_length + past_key_values_length
+ ].clone()
+
+ if input_ids is not None:
+ embeddings = self.word_embeddings(input_ids)
+ if self.position_embedding_type == "absolute":
+ position_embeddings = self.position_embeddings(position_ids)
+ embeddings = embeddings + position_embeddings
+
+ if query_embeds is not None:
+ embeddings = torch.cat((query_embeds, embeddings), dim=1)
+ else:
+ embeddings = query_embeds
+
+ embeddings = self.LayerNorm(embeddings)
+ embeddings = self.dropout(embeddings)
+ return embeddings
+
+
+class BertSelfAttention(nn.Module):
+ def __init__(self, config, is_cross_attention):
+ super().__init__()
+ self.config = config
+ if config.hidden_size % config.num_attention_heads != 0 and not hasattr(
+ config, "embedding_size"
+ ):
+ raise ValueError(
+ "The hidden size (%d) is not a multiple of the number of attention "
+ "heads (%d)" % (config.hidden_size, config.num_attention_heads)
+ )
+
+ self.num_attention_heads = config.num_attention_heads
+ self.attention_head_size = int(config.hidden_size / config.num_attention_heads)
+ self.all_head_size = self.num_attention_heads * self.attention_head_size
+
+ self.query = nn.Linear(config.hidden_size, self.all_head_size)
+ if is_cross_attention:
+ self.key = nn.Linear(config.encoder_width, self.all_head_size)
+ self.value = nn.Linear(config.encoder_width, self.all_head_size)
+ else:
+ self.key = nn.Linear(config.hidden_size, self.all_head_size)
+ self.value = nn.Linear(config.hidden_size, self.all_head_size)
+
+ self.dropout = nn.Dropout(config.attention_probs_dropout_prob)
+ self.position_embedding_type = getattr(
+ config, "position_embedding_type", "absolute"
+ )
+ if (
+ self.position_embedding_type == "relative_key"
+ or self.position_embedding_type == "relative_key_query"
+ ):
+ self.max_position_embeddings = config.max_position_embeddings
+ self.distance_embedding = nn.Embedding(
+ 2 * config.max_position_embeddings - 1, self.attention_head_size
+ )
+ self.save_attention = False
+
+ def save_attn_gradients(self, attn_gradients):
+ self.attn_gradients = attn_gradients
+
+ def get_attn_gradients(self):
+ return self.attn_gradients
+
+ def save_attention_map(self, attention_map):
+ self.attention_map = attention_map
+
+ def get_attention_map(self):
+ return self.attention_map
+
+ def transpose_for_scores(self, x):
+ new_x_shape = x.size()[:-1] + (
+ self.num_attention_heads,
+ self.attention_head_size,
+ )
+ x = x.view(*new_x_shape)
+ return x.permute(0, 2, 1, 3)
+
+ def forward(
+ self,
+ hidden_states,
+ attention_mask=None,
+ head_mask=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ past_key_value=None,
+ output_attentions=False,
+ ):
+
+ # If this is instantiated as a cross-attention module, the keys
+ # and values come from an encoder; the attention mask needs to be
+ # such that the encoder's padding tokens are not attended to.
+ is_cross_attention = encoder_hidden_states is not None
+
+ if is_cross_attention:
+ key_layer = self.transpose_for_scores(self.key(encoder_hidden_states))
+ value_layer = self.transpose_for_scores(self.value(encoder_hidden_states))
+ attention_mask = encoder_attention_mask
+ elif past_key_value is not None:
+ key_layer = self.transpose_for_scores(self.key(hidden_states))
+ value_layer = self.transpose_for_scores(self.value(hidden_states))
+ key_layer = torch.cat([past_key_value[0], key_layer], dim=2)
+ value_layer = torch.cat([past_key_value[1], value_layer], dim=2)
+ else:
+ key_layer = self.transpose_for_scores(self.key(hidden_states))
+ value_layer = self.transpose_for_scores(self.value(hidden_states))
+
+ mixed_query_layer = self.query(hidden_states)
+
+ query_layer = self.transpose_for_scores(mixed_query_layer)
+
+ past_key_value = (key_layer, value_layer)
+
+ # Take the dot product between "query" and "key" to get the raw attention scores.
+ attention_scores = torch.matmul(query_layer, key_layer.transpose(-1, -2))
+
+ if (
+ self.position_embedding_type == "relative_key"
+ or self.position_embedding_type == "relative_key_query"
+ ):
+ seq_length = hidden_states.size()[1]
+ position_ids_l = torch.arange(
+ seq_length, dtype=torch.long, device=hidden_states.device
+ ).view(-1, 1)
+ position_ids_r = torch.arange(
+ seq_length, dtype=torch.long, device=hidden_states.device
+ ).view(1, -1)
+ distance = position_ids_l - position_ids_r
+ positional_embedding = self.distance_embedding(
+ distance + self.max_position_embeddings - 1
+ )
+ positional_embedding = positional_embedding.to(
+ dtype=query_layer.dtype
+ ) # fp16 compatibility
+
+ if self.position_embedding_type == "relative_key":
+ relative_position_scores = torch.einsum(
+ "bhld,lrd->bhlr", query_layer, positional_embedding
+ )
+ attention_scores = attention_scores + relative_position_scores
+ elif self.position_embedding_type == "relative_key_query":
+ relative_position_scores_query = torch.einsum(
+ "bhld,lrd->bhlr", query_layer, positional_embedding
+ )
+ relative_position_scores_key = torch.einsum(
+ "bhrd,lrd->bhlr", key_layer, positional_embedding
+ )
+ attention_scores = (
+ attention_scores
+ + relative_position_scores_query
+ + relative_position_scores_key
+ )
+
+ attention_scores = attention_scores / math.sqrt(self.attention_head_size)
+ if attention_mask is not None:
+ # Apply the attention mask is (precomputed for all layers in BertModel forward() function)
+ attention_scores = attention_scores + attention_mask
+
+ # Normalize the attention scores to probabilities.
+ attention_probs = nn.Softmax(dim=-1)(attention_scores)
+
+ if is_cross_attention and self.save_attention:
+ self.save_attention_map(attention_probs)
+ attention_probs.register_hook(self.save_attn_gradients)
+
+ # This is actually dropping out entire tokens to attend to, which might
+ # seem a bit unusual, but is taken from the original Transformer paper.
+ attention_probs_dropped = self.dropout(attention_probs)
+
+ # Mask heads if we want to
+ if head_mask is not None:
+ attention_probs_dropped = attention_probs_dropped * head_mask
+
+ context_layer = torch.matmul(attention_probs_dropped, value_layer)
+
+ context_layer = context_layer.permute(0, 2, 1, 3).contiguous()
+ new_context_layer_shape = context_layer.size()[:-2] + (self.all_head_size,)
+ context_layer = context_layer.view(*new_context_layer_shape)
+
+ outputs = (
+ (context_layer, attention_probs) if output_attentions else (context_layer,)
+ )
+
+ outputs = outputs + (past_key_value,)
+ return outputs
+
+
+class BertSelfOutput(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.dense = nn.Linear(config.hidden_size, config.hidden_size)
+ self.LayerNorm = nn.LayerNorm(config.hidden_size, eps=config.layer_norm_eps)
+ self.dropout = nn.Dropout(config.hidden_dropout_prob)
+
+ def forward(self, hidden_states, input_tensor):
+ hidden_states = self.dense(hidden_states)
+ hidden_states = self.dropout(hidden_states)
+ hidden_states = self.LayerNorm(hidden_states + input_tensor)
+ return hidden_states
+
+
+class BertAttention(nn.Module):
+ def __init__(self, config, is_cross_attention=False):
+ super().__init__()
+ self.self = BertSelfAttention(config, is_cross_attention)
+ self.output = BertSelfOutput(config)
+ self.pruned_heads = set()
+
+ def prune_heads(self, heads):
+ if len(heads) == 0:
+ return
+ heads, index = find_pruneable_heads_and_indices(
+ heads,
+ self.self.num_attention_heads,
+ self.self.attention_head_size,
+ self.pruned_heads,
+ )
+
+ # Prune linear layers
+ self.self.query = prune_linear_layer(self.self.query, index)
+ self.self.key = prune_linear_layer(self.self.key, index)
+ self.self.value = prune_linear_layer(self.self.value, index)
+ self.output.dense = prune_linear_layer(self.output.dense, index, dim=1)
+
+ # Update hyper params and store pruned heads
+ self.self.num_attention_heads = self.self.num_attention_heads - len(heads)
+ self.self.all_head_size = (
+ self.self.attention_head_size * self.self.num_attention_heads
+ )
+ self.pruned_heads = self.pruned_heads.union(heads)
+
+ def forward(
+ self,
+ hidden_states,
+ attention_mask=None,
+ head_mask=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ past_key_value=None,
+ output_attentions=False,
+ ):
+ self_outputs = self.self(
+ hidden_states,
+ attention_mask,
+ head_mask,
+ encoder_hidden_states,
+ encoder_attention_mask,
+ past_key_value,
+ output_attentions,
+ )
+ attention_output = self.output(self_outputs[0], hidden_states)
+
+ outputs = (attention_output,) + self_outputs[
+ 1:
+ ] # add attentions if we output them
+ return outputs
+
+
+class BertIntermediate(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.dense = nn.Linear(config.hidden_size, config.intermediate_size)
+ if isinstance(config.hidden_act, str):
+ self.intermediate_act_fn = ACT2FN[config.hidden_act]
+ else:
+ self.intermediate_act_fn = config.hidden_act
+
+ def forward(self, hidden_states):
+ hidden_states = self.dense(hidden_states)
+ hidden_states = self.intermediate_act_fn(hidden_states)
+ return hidden_states
+
+
+class BertOutput(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.dense = nn.Linear(config.intermediate_size, config.hidden_size)
+ self.LayerNorm = nn.LayerNorm(config.hidden_size, eps=config.layer_norm_eps)
+ self.dropout = nn.Dropout(config.hidden_dropout_prob)
+
+ def forward(self, hidden_states, input_tensor):
+ hidden_states = self.dense(hidden_states)
+ hidden_states = self.dropout(hidden_states)
+ hidden_states = self.LayerNorm(hidden_states + input_tensor)
+ return hidden_states
+
+
+class BertLayer(nn.Module):
+ def __init__(self, config, layer_num):
+ super().__init__()
+ self.config = config
+ self.chunk_size_feed_forward = config.chunk_size_feed_forward
+ self.seq_len_dim = 1
+ self.attention = BertAttention(config)
+ self.layer_num = layer_num
+ if (
+ self.config.add_cross_attention
+ and layer_num % self.config.cross_attention_freq == 0
+ ):
+ self.crossattention = BertAttention(
+ config, is_cross_attention=self.config.add_cross_attention
+ )
+ self.has_cross_attention = True
+ else:
+ self.has_cross_attention = False
+ self.intermediate = BertIntermediate(config)
+ self.output = BertOutput(config)
+
+ self.intermediate_query = BertIntermediate(config)
+ self.output_query = BertOutput(config)
+
+ def forward(
+ self,
+ hidden_states,
+ attention_mask=None,
+ head_mask=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ past_key_value=None,
+ output_attentions=False,
+ query_length=0,
+ ):
+ # decoder uni-directional self-attention cached key/values tuple is at positions 1,2
+ self_attn_past_key_value = (
+ past_key_value[:2] if past_key_value is not None else None
+ )
+ self_attention_outputs = self.attention(
+ hidden_states,
+ attention_mask,
+ head_mask,
+ output_attentions=output_attentions,
+ past_key_value=self_attn_past_key_value,
+ )
+ attention_output = self_attention_outputs[0]
+ outputs = self_attention_outputs[1:-1]
+
+ present_key_value = self_attention_outputs[-1]
+
+ if query_length > 0:
+ query_attention_output = attention_output[:, :query_length, :]
+
+ if self.has_cross_attention:
+ assert (
+ encoder_hidden_states is not None
+ ), "encoder_hidden_states must be given for cross-attention layers"
+ cross_attention_outputs = self.crossattention(
+ query_attention_output,
+ attention_mask,
+ head_mask,
+ encoder_hidden_states,
+ encoder_attention_mask,
+ output_attentions=output_attentions,
+ )
+ query_attention_output = cross_attention_outputs[0]
+ outputs = (
+ outputs + cross_attention_outputs[1:-1]
+ ) # add cross attentions if we output attention weights
+
+ layer_output = apply_chunking_to_forward(
+ self.feed_forward_chunk_query,
+ self.chunk_size_feed_forward,
+ self.seq_len_dim,
+ query_attention_output,
+ )
+ if attention_output.shape[1] > query_length:
+ layer_output_text = apply_chunking_to_forward(
+ self.feed_forward_chunk,
+ self.chunk_size_feed_forward,
+ self.seq_len_dim,
+ attention_output[:, query_length:, :],
+ )
+ layer_output = torch.cat([layer_output, layer_output_text], dim=1)
+ else:
+ layer_output = apply_chunking_to_forward(
+ self.feed_forward_chunk,
+ self.chunk_size_feed_forward,
+ self.seq_len_dim,
+ attention_output,
+ )
+ outputs = (layer_output,) + outputs
+
+ outputs = outputs + (present_key_value,)
+
+ return outputs
+
+ def feed_forward_chunk(self, attention_output):
+ intermediate_output = self.intermediate(attention_output)
+ layer_output = self.output(intermediate_output, attention_output)
+ return layer_output
+
+ def feed_forward_chunk_query(self, attention_output):
+ intermediate_output = self.intermediate_query(attention_output)
+ layer_output = self.output_query(intermediate_output, attention_output)
+ return layer_output
+
+
+class BertEncoder(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.config = config
+ self.layer = nn.ModuleList(
+ [BertLayer(config, i) for i in range(config.num_hidden_layers)]
+ )
+
+ def forward(
+ self,
+ hidden_states,
+ attention_mask=None,
+ head_mask=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ past_key_values=None,
+ use_cache=None,
+ output_attentions=False,
+ output_hidden_states=False,
+ return_dict=True,
+ query_length=0,
+ ):
+ all_hidden_states = () if output_hidden_states else None
+ all_self_attentions = () if output_attentions else None
+ all_cross_attentions = (
+ () if output_attentions and self.config.add_cross_attention else None
+ )
+
+ next_decoder_cache = () if use_cache else None
+
+ for i in range(self.config.num_hidden_layers):
+ layer_module = self.layer[i]
+ if output_hidden_states:
+ all_hidden_states = all_hidden_states + (hidden_states,)
+
+ layer_head_mask = head_mask[i] if head_mask is not None else None
+ past_key_value = past_key_values[i] if past_key_values is not None else None
+
+ if getattr(self.config, "gradient_checkpointing", False) and self.training:
+
+ if use_cache:
+ logger.warn(
+ "`use_cache=True` is incompatible with gradient checkpointing. Setting `use_cache=False`..."
+ )
+ use_cache = False
+
+ def create_custom_forward(module):
+ def custom_forward(*inputs):
+ return module(
+ *inputs, past_key_value, output_attentions, query_length
+ )
+
+ return custom_forward
+
+ layer_outputs = torch.utils.checkpoint.checkpoint(
+ create_custom_forward(layer_module),
+ hidden_states,
+ attention_mask,
+ layer_head_mask,
+ encoder_hidden_states,
+ encoder_attention_mask,
+ )
+ else:
+ layer_outputs = layer_module(
+ hidden_states,
+ attention_mask,
+ layer_head_mask,
+ encoder_hidden_states,
+ encoder_attention_mask,
+ past_key_value,
+ output_attentions,
+ query_length,
+ )
+
+ hidden_states = layer_outputs[0]
+ if use_cache:
+ next_decoder_cache += (layer_outputs[-1],)
+ if output_attentions:
+ all_self_attentions = all_self_attentions + (layer_outputs[1],)
+ all_cross_attentions = all_cross_attentions + (layer_outputs[2],)
+
+ if output_hidden_states:
+ all_hidden_states = all_hidden_states + (hidden_states,)
+
+ if not return_dict:
+ return tuple(
+ v
+ for v in [
+ hidden_states,
+ next_decoder_cache,
+ all_hidden_states,
+ all_self_attentions,
+ all_cross_attentions,
+ ]
+ if v is not None
+ )
+ return BaseModelOutputWithPastAndCrossAttentions(
+ last_hidden_state=hidden_states,
+ past_key_values=next_decoder_cache,
+ hidden_states=all_hidden_states,
+ attentions=all_self_attentions,
+ cross_attentions=all_cross_attentions,
+ )
+
+
+class BertPooler(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.dense = nn.Linear(config.hidden_size, config.hidden_size)
+ self.activation = nn.Tanh()
+
+ def forward(self, hidden_states):
+ # We "pool" the model by simply taking the hidden state corresponding
+ # to the first token.
+ first_token_tensor = hidden_states[:, 0]
+ pooled_output = self.dense(first_token_tensor)
+ pooled_output = self.activation(pooled_output)
+ return pooled_output
+
+
+class BertPredictionHeadTransform(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.dense = nn.Linear(config.hidden_size, config.hidden_size)
+ if isinstance(config.hidden_act, str):
+ self.transform_act_fn = ACT2FN[config.hidden_act]
+ else:
+ self.transform_act_fn = config.hidden_act
+ self.LayerNorm = nn.LayerNorm(config.hidden_size, eps=config.layer_norm_eps)
+
+ def forward(self, hidden_states):
+ hidden_states = self.dense(hidden_states)
+ hidden_states = self.transform_act_fn(hidden_states)
+ hidden_states = self.LayerNorm(hidden_states)
+ return hidden_states
+
+
+class BertLMPredictionHead(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.transform = BertPredictionHeadTransform(config)
+
+ # The output weights are the same as the input embeddings, but there is
+ # an output-only bias for each token.
+ self.decoder = nn.Linear(config.hidden_size, config.vocab_size, bias=False)
+
+ self.bias = nn.Parameter(torch.zeros(config.vocab_size))
+
+ # Need a link between the two variables so that the bias is correctly resized with `resize_token_embeddings`
+ self.decoder.bias = self.bias
+
+ def forward(self, hidden_states):
+ hidden_states = self.transform(hidden_states)
+ hidden_states = self.decoder(hidden_states)
+ return hidden_states
+
+
+class BertOnlyMLMHead(nn.Module):
+ def __init__(self, config):
+ super().__init__()
+ self.predictions = BertLMPredictionHead(config)
+
+ def forward(self, sequence_output):
+ prediction_scores = self.predictions(sequence_output)
+ return prediction_scores
+
+
+class BertPreTrainedModel(PreTrainedModel):
+ """
+ An abstract class to handle weights initialization and a simple interface for downloading and loading pretrained
+ models.
+ """
+
+ config_class = BertConfig
+ base_model_prefix = "bert"
+ _keys_to_ignore_on_load_missing = [r"position_ids"]
+
+ def _init_weights(self, module):
+ """Initialize the weights"""
+ if isinstance(module, (nn.Linear, nn.Embedding)):
+ # Slightly different from the TF version which uses truncated_normal for initialization
+ # cf https://github.com/pytorch/pytorch/pull/5617
+ module.weight.data.normal_(mean=0.0, std=self.config.initializer_range)
+ elif isinstance(module, nn.LayerNorm):
+ module.bias.data.zero_()
+ module.weight.data.fill_(1.0)
+ if isinstance(module, nn.Linear) and module.bias is not None:
+ module.bias.data.zero_()
+
+
+class BertModel(BertPreTrainedModel):
+ """
+ The model can behave as an encoder (with only self-attention) as well as a decoder, in which case a layer of
+ cross-attention is added between the self-attention layers, following the architecture described in `Attention is
+ all you need `__ by Ashish Vaswani, Noam Shazeer, Niki Parmar, Jakob Uszkoreit,
+ Llion Jones, Aidan N. Gomez, Lukasz Kaiser and Illia Polosukhin.
+ argument and :obj:`add_cross_attention` set to :obj:`True`; an :obj:`encoder_hidden_states` is then expected as an
+ input to the forward pass.
+ """
+
+ def __init__(self, config, add_pooling_layer=False):
+ super().__init__(config)
+ self.config = config
+
+ self.embeddings = BertEmbeddings(config)
+
+ self.encoder = BertEncoder(config)
+
+ self.pooler = BertPooler(config) if add_pooling_layer else None
+
+ self.init_weights()
+
+ def get_input_embeddings(self):
+ return self.embeddings.word_embeddings
+
+ def set_input_embeddings(self, value):
+ self.embeddings.word_embeddings = value
+
+ def _prune_heads(self, heads_to_prune):
+ """
+ Prunes heads of the model. heads_to_prune: dict of {layer_num: list of heads to prune in this layer} See base
+ class PreTrainedModel
+ """
+ for layer, heads in heads_to_prune.items():
+ self.encoder.layer[layer].attention.prune_heads(heads)
+
+ def get_extended_attention_mask(
+ self,
+ attention_mask: Tensor,
+ input_shape: Tuple[int],
+ device: device,
+ is_decoder: bool,
+ has_query: bool = False,
+ ) -> Tensor:
+ """
+ Makes broadcastable attention and causal masks so that future and masked tokens are ignored.
+
+ Arguments:
+ attention_mask (:obj:`torch.Tensor`):
+ Mask with ones indicating tokens to attend to, zeros for tokens to ignore.
+ input_shape (:obj:`Tuple[int]`):
+ The shape of the input to the model.
+ device: (:obj:`torch.device`):
+ The device of the input to the model.
+
+ Returns:
+ :obj:`torch.Tensor` The extended attention mask, with a the same dtype as :obj:`attention_mask.dtype`.
+ """
+ # We can provide a self-attention mask of dimensions [batch_size, from_seq_length, to_seq_length]
+ # ourselves in which case we just need to make it broadcastable to all heads.
+ if attention_mask.dim() == 3:
+ extended_attention_mask = attention_mask[:, None, :, :]
+ elif attention_mask.dim() == 2:
+ # Provided a padding mask of dimensions [batch_size, seq_length]
+ # - if the model is a decoder, apply a causal mask in addition to the padding mask
+ # - if the model is an encoder, make the mask broadcastable to [batch_size, num_heads, seq_length, seq_length]
+ if is_decoder:
+ batch_size, seq_length = input_shape
+
+ seq_ids = torch.arange(seq_length, device=device)
+ causal_mask = (
+ seq_ids[None, None, :].repeat(batch_size, seq_length, 1)
+ <= seq_ids[None, :, None]
+ )
+
+ # add a prefix ones mask to the causal mask
+ # causal and attention masks must have same type with pytorch version < 1.3
+ causal_mask = causal_mask.to(attention_mask.dtype)
+
+ if causal_mask.shape[1] < attention_mask.shape[1]:
+ prefix_seq_len = attention_mask.shape[1] - causal_mask.shape[1]
+ if has_query: # UniLM style attention mask
+ causal_mask = torch.cat(
+ [
+ torch.zeros(
+ (batch_size, prefix_seq_len, seq_length),
+ device=device,
+ dtype=causal_mask.dtype,
+ ),
+ causal_mask,
+ ],
+ axis=1,
+ )
+ causal_mask = torch.cat(
+ [
+ torch.ones(
+ (batch_size, causal_mask.shape[1], prefix_seq_len),
+ device=device,
+ dtype=causal_mask.dtype,
+ ),
+ causal_mask,
+ ],
+ axis=-1,
+ )
+ extended_attention_mask = (
+ causal_mask[:, None, :, :] * attention_mask[:, None, None, :]
+ )
+ else:
+ extended_attention_mask = attention_mask[:, None, None, :]
+ else:
+ raise ValueError(
+ "Wrong shape for input_ids (shape {}) or attention_mask (shape {})".format(
+ input_shape, attention_mask.shape
+ )
+ )
+
+ # Since attention_mask is 1.0 for positions we want to attend and 0.0 for
+ # masked positions, this operation will create a tensor which is 0.0 for
+ # positions we want to attend and -10000.0 for masked positions.
+ # Since we are adding it to the raw scores before the softmax, this is
+ # effectively the same as removing these entirely.
+ extended_attention_mask = extended_attention_mask.to(
+ dtype=self.dtype
+ ) # fp16 compatibility
+ extended_attention_mask = (1.0 - extended_attention_mask) * -10000.0
+ return extended_attention_mask
+
+ def forward(
+ self,
+ input_ids=None,
+ attention_mask=None,
+ position_ids=None,
+ head_mask=None,
+ query_embeds=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ past_key_values=None,
+ use_cache=None,
+ output_attentions=None,
+ output_hidden_states=None,
+ return_dict=None,
+ is_decoder=False,
+ ):
+ r"""
+ encoder_hidden_states (:obj:`torch.FloatTensor` of shape :obj:`(batch_size, sequence_length, hidden_size)`, `optional`):
+ Sequence of hidden-states at the output of the last layer of the encoder. Used in the cross-attention if
+ the model is configured as a decoder.
+ encoder_attention_mask (:obj:`torch.FloatTensor` of shape :obj:`(batch_size, sequence_length)`, `optional`):
+ Mask to avoid performing attention on the padding token indices of the encoder input. This mask is used in
+ the cross-attention if the model is configured as a decoder. Mask values selected in ``[0, 1]``:
+ - 1 for tokens that are **not masked**,
+ - 0 for tokens that are **masked**.
+ past_key_values (:obj:`tuple(tuple(torch.FloatTensor))` of length :obj:`config.n_layers` with each tuple having 4 tensors of shape :obj:`(batch_size, num_heads, sequence_length - 1, embed_size_per_head)`):
+ Contains precomputed key and value hidden states of the attention blocks. Can be used to speed up decoding.
+ If :obj:`past_key_values` are used, the user can optionally input only the last :obj:`decoder_input_ids`
+ (those that don't have their past key value states given to this model) of shape :obj:`(batch_size, 1)`
+ instead of all :obj:`decoder_input_ids` of shape :obj:`(batch_size, sequence_length)`.
+ use_cache (:obj:`bool`, `optional`):
+ If set to :obj:`True`, :obj:`past_key_values` key value states are returned and can be used to speed up
+ decoding (see :obj:`past_key_values`).
+ """
+ output_attentions = (
+ output_attentions
+ if output_attentions is not None
+ else self.config.output_attentions
+ )
+ output_hidden_states = (
+ output_hidden_states
+ if output_hidden_states is not None
+ else self.config.output_hidden_states
+ )
+ return_dict = (
+ return_dict if return_dict is not None else self.config.use_return_dict
+ )
+
+ # use_cache = use_cache if use_cache is not None else self.config.use_cache
+
+ if input_ids is None:
+ assert (
+ query_embeds is not None
+ ), "You have to specify query_embeds when input_ids is None"
+
+ # past_key_values_length
+ past_key_values_length = (
+ past_key_values[0][0].shape[2] - self.config.query_length
+ if past_key_values is not None
+ else 0
+ )
+
+ query_length = query_embeds.shape[1] if query_embeds is not None else 0
+
+ embedding_output = self.embeddings(
+ input_ids=input_ids,
+ position_ids=position_ids,
+ query_embeds=query_embeds,
+ past_key_values_length=past_key_values_length,
+ )
+
+ input_shape = embedding_output.size()[:-1]
+ batch_size, seq_length = input_shape
+ device = embedding_output.device
+
+ if attention_mask is None:
+ attention_mask = torch.ones(
+ ((batch_size, seq_length + past_key_values_length)), device=device
+ )
+
+ # We can provide a self-attention mask of dimensions [batch_size, from_seq_length, to_seq_length]
+ # ourselves in which case we just need to make it broadcastable to all heads.
+ if is_decoder:
+ extended_attention_mask = self.get_extended_attention_mask(
+ attention_mask,
+ input_ids.shape,
+ device,
+ is_decoder,
+ has_query=(query_embeds is not None),
+ )
+ else:
+ extended_attention_mask = self.get_extended_attention_mask(
+ attention_mask, input_shape, device, is_decoder
+ )
+
+ # If a 2D or 3D attention mask is provided for the cross-attention
+ # we need to make broadcastable to [batch_size, num_heads, seq_length, seq_length]
+ if encoder_hidden_states is not None:
+ if type(encoder_hidden_states) == list:
+ encoder_batch_size, encoder_sequence_length, _ = encoder_hidden_states[
+ 0
+ ].size()
+ else:
+ (
+ encoder_batch_size,
+ encoder_sequence_length,
+ _,
+ ) = encoder_hidden_states.size()
+ encoder_hidden_shape = (encoder_batch_size, encoder_sequence_length)
+
+ if type(encoder_attention_mask) == list:
+ encoder_extended_attention_mask = [
+ self.invert_attention_mask(mask) for mask in encoder_attention_mask
+ ]
+ elif encoder_attention_mask is None:
+ encoder_attention_mask = torch.ones(encoder_hidden_shape, device=device)
+ encoder_extended_attention_mask = self.invert_attention_mask(
+ encoder_attention_mask
+ )
+ else:
+ encoder_extended_attention_mask = self.invert_attention_mask(
+ encoder_attention_mask
+ )
+ else:
+ encoder_extended_attention_mask = None
+
+ # Prepare head mask if needed
+ # 1.0 in head_mask indicate we keep the head
+ # attention_probs has shape bsz x n_heads x N x N
+ # input head_mask has shape [num_heads] or [num_hidden_layers x num_heads]
+ # and head_mask is converted to shape [num_hidden_layers x batch x num_heads x seq_length x seq_length]
+ head_mask = self.get_head_mask(head_mask, self.config.num_hidden_layers)
+
+ encoder_outputs = self.encoder(
+ embedding_output,
+ attention_mask=extended_attention_mask,
+ head_mask=head_mask,
+ encoder_hidden_states=encoder_hidden_states,
+ encoder_attention_mask=encoder_extended_attention_mask,
+ past_key_values=past_key_values,
+ use_cache=use_cache,
+ output_attentions=output_attentions,
+ output_hidden_states=output_hidden_states,
+ return_dict=return_dict,
+ query_length=query_length,
+ )
+ sequence_output = encoder_outputs[0]
+ pooled_output = (
+ self.pooler(sequence_output) if self.pooler is not None else None
+ )
+
+ if not return_dict:
+ return (sequence_output, pooled_output) + encoder_outputs[1:]
+
+ return BaseModelOutputWithPoolingAndCrossAttentions(
+ last_hidden_state=sequence_output,
+ pooler_output=pooled_output,
+ past_key_values=encoder_outputs.past_key_values,
+ hidden_states=encoder_outputs.hidden_states,
+ attentions=encoder_outputs.attentions,
+ cross_attentions=encoder_outputs.cross_attentions,
+ )
+
+
+class BertLMHeadModel(BertPreTrainedModel):
+
+ _keys_to_ignore_on_load_unexpected = [r"pooler"]
+ _keys_to_ignore_on_load_missing = [r"position_ids", r"predictions.decoder.bias"]
+
+ def __init__(self, config):
+ super().__init__(config)
+
+ self.bert = BertModel(config, add_pooling_layer=False)
+ self.cls = BertOnlyMLMHead(config)
+
+ self.init_weights()
+
+ def get_output_embeddings(self):
+ return self.cls.predictions.decoder
+
+ def set_output_embeddings(self, new_embeddings):
+ self.cls.predictions.decoder = new_embeddings
+
+ def forward(
+ self,
+ input_ids=None,
+ attention_mask=None,
+ position_ids=None,
+ head_mask=None,
+ query_embeds=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ labels=None,
+ past_key_values=None,
+ use_cache=True,
+ output_attentions=None,
+ output_hidden_states=None,
+ return_dict=None,
+ return_logits=False,
+ is_decoder=True,
+ reduction="mean",
+ ):
+ r"""
+ encoder_hidden_states (:obj:`torch.FloatTensor` of shape :obj:`(batch_size, sequence_length, hidden_size)`, `optional`):
+ Sequence of hidden-states at the output of the last layer of the encoder. Used in the cross-attention if
+ the model is configured as a decoder.
+ encoder_attention_mask (:obj:`torch.FloatTensor` of shape :obj:`(batch_size, sequence_length)`, `optional`):
+ Mask to avoid performing attention on the padding token indices of the encoder input. This mask is used in
+ the cross-attention if the model is configured as a decoder. Mask values selected in ``[0, 1]``:
+ - 1 for tokens that are **not masked**,
+ - 0 for tokens that are **masked**.
+ labels (:obj:`torch.LongTensor` of shape :obj:`(batch_size, sequence_length)`, `optional`):
+ Labels for computing the left-to-right language modeling loss (next word prediction). Indices should be in
+ ``[-100, 0, ..., config.vocab_size]`` (see ``input_ids`` docstring) Tokens with indices set to ``-100`` are
+ ignored (masked), the loss is only computed for the tokens with labels n ``[0, ..., config.vocab_size]``
+ past_key_values (:obj:`tuple(tuple(torch.FloatTensor))` of length :obj:`config.n_layers` with each tuple having 4 tensors of shape :obj:`(batch_size, num_heads, sequence_length - 1, embed_size_per_head)`):
+ Contains precomputed key and value hidden states of the attention blocks. Can be used to speed up decoding.
+ If :obj:`past_key_values` are used, the user can optionally input only the last :obj:`decoder_input_ids`
+ (those that don't have their past key value states given to this model) of shape :obj:`(batch_size, 1)`
+ instead of all :obj:`decoder_input_ids` of shape :obj:`(batch_size, sequence_length)`.
+ use_cache (:obj:`bool`, `optional`):
+ If set to :obj:`True`, :obj:`past_key_values` key value states are returned and can be used to speed up
+ decoding (see :obj:`past_key_values`).
+ Returns:
+ Example::
+ >>> from transformers import BertTokenizer, BertLMHeadModel, BertConfig
+ >>> import torch
+ >>> tokenizer = BertTokenizer.from_pretrained('bert-base-cased')
+ >>> config = BertConfig.from_pretrained("bert-base-cased")
+ >>> model = BertLMHeadModel.from_pretrained('bert-base-cased', config=config)
+ >>> inputs = tokenizer("Hello, my dog is cute", return_tensors="pt")
+ >>> outputs = model(**inputs)
+ >>> prediction_logits = outputs.logits
+ """
+ return_dict = (
+ return_dict if return_dict is not None else self.config.use_return_dict
+ )
+ if labels is not None:
+ use_cache = False
+ if past_key_values is not None:
+ query_embeds = None
+
+ outputs = self.bert(
+ input_ids,
+ attention_mask=attention_mask,
+ position_ids=position_ids,
+ head_mask=head_mask,
+ query_embeds=query_embeds,
+ encoder_hidden_states=encoder_hidden_states,
+ encoder_attention_mask=encoder_attention_mask,
+ past_key_values=past_key_values,
+ use_cache=use_cache,
+ output_attentions=output_attentions,
+ output_hidden_states=output_hidden_states,
+ return_dict=return_dict,
+ is_decoder=is_decoder,
+ )
+
+ sequence_output = outputs[0]
+ if query_embeds is not None:
+ sequence_output = outputs[0][:, query_embeds.shape[1] :, :]
+
+ prediction_scores = self.cls(sequence_output)
+
+ if return_logits:
+ return prediction_scores[:, :-1, :].contiguous()
+
+ lm_loss = None
+ if labels is not None:
+ # we are doing next-token prediction; shift prediction scores and input ids by one
+ shifted_prediction_scores = prediction_scores[:, :-1, :].contiguous()
+ labels = labels[:, 1:].contiguous()
+ loss_fct = CrossEntropyLoss(reduction=reduction, label_smoothing=0.1)
+ lm_loss = loss_fct(
+ shifted_prediction_scores.view(-1, self.config.vocab_size),
+ labels.view(-1),
+ )
+ if reduction == "none":
+ lm_loss = lm_loss.view(prediction_scores.size(0), -1).sum(1)
+
+ if not return_dict:
+ output = (prediction_scores,) + outputs[2:]
+ return ((lm_loss,) + output) if lm_loss is not None else output
+
+ return CausalLMOutputWithCrossAttentions(
+ loss=lm_loss,
+ logits=prediction_scores,
+ past_key_values=outputs.past_key_values,
+ hidden_states=outputs.hidden_states,
+ attentions=outputs.attentions,
+ cross_attentions=outputs.cross_attentions,
+ )
+
+ def prepare_inputs_for_generation(
+ self, input_ids, query_embeds, past=None, attention_mask=None, **model_kwargs
+ ):
+ # if model is used as a decoder in encoder-decoder model, the decoder attention mask is created on the fly
+ if attention_mask is None:
+ attention_mask = input_ids.new_ones(input_ids.shape)
+ query_mask = input_ids.new_ones(query_embeds.shape[:-1])
+ attention_mask = torch.cat([query_mask, attention_mask], dim=-1)
+
+ # cut decoder_input_ids if past is used
+ if past is not None:
+ input_ids = input_ids[:, -1:]
+
+ return {
+ "input_ids": input_ids,
+ "query_embeds": query_embeds,
+ "attention_mask": attention_mask,
+ "past_key_values": past,
+ "encoder_hidden_states": model_kwargs.get("encoder_hidden_states", None),
+ "encoder_attention_mask": model_kwargs.get("encoder_attention_mask", None),
+ "is_decoder": True,
+ }
+
+ def _reorder_cache(self, past, beam_idx):
+ reordered_past = ()
+ for layer_past in past:
+ reordered_past += (
+ tuple(
+ past_state.index_select(0, beam_idx) for past_state in layer_past
+ ),
+ )
+ return reordered_past
+
+
+class BertForMaskedLM(BertPreTrainedModel):
+
+ _keys_to_ignore_on_load_unexpected = [r"pooler"]
+ _keys_to_ignore_on_load_missing = [r"position_ids", r"predictions.decoder.bias"]
+
+ def __init__(self, config):
+ super().__init__(config)
+
+ self.bert = BertModel(config, add_pooling_layer=False)
+ self.cls = BertOnlyMLMHead(config)
+
+ self.init_weights()
+
+ def get_output_embeddings(self):
+ return self.cls.predictions.decoder
+
+ def set_output_embeddings(self, new_embeddings):
+ self.cls.predictions.decoder = new_embeddings
+
+ def forward(
+ self,
+ input_ids=None,
+ attention_mask=None,
+ position_ids=None,
+ head_mask=None,
+ query_embeds=None,
+ encoder_hidden_states=None,
+ encoder_attention_mask=None,
+ labels=None,
+ output_attentions=None,
+ output_hidden_states=None,
+ return_dict=None,
+ return_logits=False,
+ is_decoder=False,
+ ):
+ r"""
+ labels (:obj:`torch.LongTensor` of shape :obj:`(batch_size, sequence_length)`, `optional`):
+ Labels for computing the masked language modeling loss. Indices should be in ``[-100, 0, ...,
+ config.vocab_size]`` (see ``input_ids`` docstring) Tokens with indices set to ``-100`` are ignored
+ (masked), the loss is only computed for the tokens with labels in ``[0, ..., config.vocab_size]``
+ """
+
+ return_dict = (
+ return_dict if return_dict is not None else self.config.use_return_dict
+ )
+
+ outputs = self.bert(
+ input_ids,
+ attention_mask=attention_mask,
+ position_ids=position_ids,
+ head_mask=head_mask,
+ query_embeds=query_embeds,
+ encoder_hidden_states=encoder_hidden_states,
+ encoder_attention_mask=encoder_attention_mask,
+ output_attentions=output_attentions,
+ output_hidden_states=output_hidden_states,
+ return_dict=return_dict,
+ is_decoder=is_decoder,
+ )
+
+ if query_embeds is not None:
+ sequence_output = outputs[0][:, query_embeds.shape[1] :, :]
+ prediction_scores = self.cls(sequence_output)
+
+ if return_logits:
+ return prediction_scores
+
+ masked_lm_loss = None
+ if labels is not None:
+ loss_fct = CrossEntropyLoss() # -100 index = padding token
+ masked_lm_loss = loss_fct(
+ prediction_scores.view(-1, self.config.vocab_size), labels.view(-1)
+ )
+
+ if not return_dict:
+ output = (prediction_scores,) + outputs[2:]
+ return (
+ ((masked_lm_loss,) + output) if masked_lm_loss is not None else output
+ )
+
+ return MaskedLMOutput(
+ loss=masked_lm_loss,
+ logits=prediction_scores,
+ hidden_states=outputs.hidden_states,
+ attentions=outputs.attentions,
+ )
diff --git a/global_local/models/__init__.py b/global_local/models/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..ac338d1edce580b3ca4f52fdd8a65a0ebbb2b32d
--- /dev/null
+++ b/global_local/models/__init__.py
@@ -0,0 +1,201 @@
+"""
+Adapted from salesforce@LAVIS Vision-CAIR@MiniGPT-4. Below is the original copyright:
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import logging
+import torch
+from omegaconf import OmegaConf
+
+from global_local.common.registry import registry
+from global_local.models.base_model import BaseModel
+from global_local.models.blip2 import Blip2Base
+from global_local.models.video_instruction_ft_model import VideoInstructionFTLLAMA
+from global_local.processors.base_processor import BaseProcessor
+
+
+__all__ = [
+ "load_model",
+ "BaseModel",
+ "Blip2Base",
+ "VideoInstructionFTLLAMA"
+]
+
+
+def load_model(name, model_type, is_eval=False, device="cpu", checkpoint=None):
+ """
+ Load supported models.
+
+ To list all available models and types in registry:
+ >>> from video_llama.models import model_zoo
+ >>> print(model_zoo)
+
+ Args:
+ name (str): name of the model.
+ model_type (str): type of the model.
+ is_eval (bool): whether the model is in eval mode. Default: False.
+ device (str): device to use. Default: "cpu".
+ checkpoint (str): path or to checkpoint. Default: None.
+ Note that expecting the checkpoint to have the same keys in state_dict as the model.
+
+ Returns:
+ model (torch.nn.Module): model.
+ """
+
+ model = registry.get_model_class(name).from_pretrained(model_type=model_type)
+
+ if checkpoint is not None:
+ model.load_checkpoint(checkpoint)
+
+ if is_eval:
+ model.eval()
+
+ if device == "cpu":
+ model = model.float()
+
+ return model.to(device)
+
+
+def load_preprocess(config):
+ """
+ Load preprocessor configs and construct preprocessors.
+
+ If no preprocessor is specified, return BaseProcessor, which does not do any preprocessing.
+
+ Args:
+ config (dict): preprocessor configs.
+
+ Returns:
+ vis_processors (dict): preprocessors for visual inputs.
+ txt_processors (dict): preprocessors for text inputs.
+
+ Key is "train" or "eval" for processors used in training and evaluation respectively.
+ """
+
+ def _build_proc_from_cfg(cfg):
+ return (
+ registry.get_processor_class(cfg.name).from_config(cfg)
+ if cfg is not None
+ else BaseProcessor()
+ )
+
+ vis_processors = dict()
+ txt_processors = dict()
+
+ vis_proc_cfg = config.get("vis_processor")
+ txt_proc_cfg = config.get("text_processor")
+
+ if vis_proc_cfg is not None:
+ vis_train_cfg = vis_proc_cfg.get("train")
+ vis_eval_cfg = vis_proc_cfg.get("eval")
+ else:
+ vis_train_cfg = None
+ vis_eval_cfg = None
+
+ vis_processors["train"] = _build_proc_from_cfg(vis_train_cfg)
+ vis_processors["eval"] = _build_proc_from_cfg(vis_eval_cfg)
+
+ if txt_proc_cfg is not None:
+ txt_train_cfg = txt_proc_cfg.get("train")
+ txt_eval_cfg = txt_proc_cfg.get("eval")
+ else:
+ txt_train_cfg = None
+ txt_eval_cfg = None
+
+ txt_processors["train"] = _build_proc_from_cfg(txt_train_cfg)
+ txt_processors["eval"] = _build_proc_from_cfg(txt_eval_cfg)
+
+ return vis_processors, txt_processors
+
+
+def load_model_and_preprocess(name, model_type, is_eval=False, device="cpu"):
+ """
+ Load model and its related preprocessors.
+
+ List all available models and types in registry:
+ >>> from video_llama.models import model_zoo
+ >>> print(model_zoo)
+
+ Args:
+ name (str): name of the model.
+ model_type (str): type of the model.
+ is_eval (bool): whether the model is in eval mode. Default: False.
+ device (str): device to use. Default: "cpu".
+
+ Returns:
+ model (torch.nn.Module): model.
+ vis_processors (dict): preprocessors for visual inputs.
+ txt_processors (dict): preprocessors for text inputs.
+ """
+ model_cls = registry.get_model_class(name)
+
+ # load model
+ model = model_cls.from_pretrained(model_type=model_type)
+
+ if is_eval:
+ model.eval()
+
+ # load preprocess
+ cfg = OmegaConf.load(model_cls.default_config_path(model_type))
+ if cfg is not None:
+ preprocess_cfg = cfg.preprocess
+
+ vis_processors, txt_processors = load_preprocess(preprocess_cfg)
+ else:
+ vis_processors, txt_processors = None, None
+ logging.info(
+ f"""No default preprocess for model {name} ({model_type}).
+ This can happen if the model is not finetuned on downstream datasets,
+ or it is not intended for direct use without finetuning.
+ """
+ )
+
+ if device == "cpu" or device == torch.device("cpu"):
+ model = model.float()
+
+ return model.to(device), vis_processors, txt_processors
+
+
+class ModelZoo:
+ """
+ A utility class to create string representation of available model architectures and types.
+
+ >>> from video_llama.models import model_zoo
+ >>> # list all available models
+ >>> print(model_zoo)
+ >>> # show total number of models
+ >>> print(len(model_zoo))
+ """
+
+ def __init__(self) -> None:
+ self.model_zoo = {
+ k: list(v.PRETRAINED_MODEL_CONFIG_DICT.keys())
+ for k, v in registry.mapping["model_name_mapping"].items()
+ }
+
+ def __str__(self) -> str:
+ return (
+ "=" * 50
+ + "\n"
+ + f"{'Architectures':<30} {'Types'}\n"
+ + "=" * 50
+ + "\n"
+ + "\n".join(
+ [
+ f"{name:<30} {', '.join(types)}"
+ for name, types in self.model_zoo.items()
+ ]
+ )
+ )
+
+ def __iter__(self):
+ return iter(self.model_zoo.items())
+
+ def __len__(self):
+ return sum([len(v) for v in self.model_zoo.values()])
+
+
+model_zoo = ModelZoo()
diff --git a/global_local/models/__pycache__/Qformer.cpython-39.pyc b/global_local/models/__pycache__/Qformer.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..8bf180b0f6b67ecd63ef5ce13f25abaaf8e13ba7
Binary files /dev/null and b/global_local/models/__pycache__/Qformer.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/__init__.cpython-39.pyc b/global_local/models/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..2bc73f6a078a70ae3e69083af4e2dcdb4338db6f
Binary files /dev/null and b/global_local/models/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/base_model.cpython-39.pyc b/global_local/models/__pycache__/base_model.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..55622162f504ce96b4b49948a07a66cba666ebb7
Binary files /dev/null and b/global_local/models/__pycache__/base_model.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/blip2.cpython-39.pyc b/global_local/models/__pycache__/blip2.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..579dc211abc58dfe25c39562f3955df710f0e0b2
Binary files /dev/null and b/global_local/models/__pycache__/blip2.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/eva_vit.cpython-39.pyc b/global_local/models/__pycache__/eva_vit.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..b6097213cfe5ad0e480e60c7247b1fedb112806d
Binary files /dev/null and b/global_local/models/__pycache__/eva_vit.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/modeling_llama.cpython-39.pyc b/global_local/models/__pycache__/modeling_llama.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..87fc112fff07de87d5b3dd02bd14dce1b313d597
Binary files /dev/null and b/global_local/models/__pycache__/modeling_llama.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/moviechat.cpython-39.pyc b/global_local/models/__pycache__/moviechat.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..08d67bae2afd59096e4507084b935f3ae7c1556e
Binary files /dev/null and b/global_local/models/__pycache__/moviechat.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/video_agg_model.cpython-39.pyc b/global_local/models/__pycache__/video_agg_model.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..fcb9e481519b939e29176f9d260d5386bf943213
Binary files /dev/null and b/global_local/models/__pycache__/video_agg_model.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/video_goal_inference_model.cpython-39.pyc b/global_local/models/__pycache__/video_goal_inference_model.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..2c52e9404d91e66c56be3c73ed8ebea97135904d
Binary files /dev/null and b/global_local/models/__pycache__/video_goal_inference_model.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/video_instruction_ft_model.cpython-39.pyc b/global_local/models/__pycache__/video_instruction_ft_model.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..1c4853dd0540fc22b8c65c6cb064f601643e11d2
Binary files /dev/null and b/global_local/models/__pycache__/video_instruction_ft_model.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/video_llama.cpython-39.pyc b/global_local/models/__pycache__/video_llama.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..822b60c36fa55ccc460001c2e8f47d4ae67108c8
Binary files /dev/null and b/global_local/models/__pycache__/video_llama.cpython-39.pyc differ
diff --git a/global_local/models/__pycache__/video_llama_captioning.cpython-39.pyc b/global_local/models/__pycache__/video_llama_captioning.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..b0c6e07e14a12e2e0615dbe96b829270566ba1ec
Binary files /dev/null and b/global_local/models/__pycache__/video_llama_captioning.cpython-39.pyc differ
diff --git a/global_local/models/base_model.py b/global_local/models/base_model.py
new file mode 100644
index 0000000000000000000000000000000000000000..31068e52b4ec5343114d98a6c07584ae14890a2d
--- /dev/null
+++ b/global_local/models/base_model.py
@@ -0,0 +1,248 @@
+"""
+Adapted from salesforce@LAVIS. Below is the original copyright:
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import logging
+import os
+
+import numpy as np
+import torch
+import torch.nn as nn
+from global_local.common.dist_utils import download_cached_file, is_dist_avail_and_initialized
+from global_local.common.utils import get_abs_path, is_url
+from omegaconf import OmegaConf
+
+
+class BaseModel(nn.Module):
+ """Base class for models."""
+
+ def __init__(self):
+ super().__init__()
+
+ @property
+ def device(self):
+ return list(self.parameters())[0].device
+
+ def load_checkpoint(self, url_or_filename):
+ """
+ Load from a finetuned checkpoint.
+
+ This should expect no mismatch in the model keys and the checkpoint keys.
+ """
+
+ if is_url(url_or_filename):
+ cached_file = download_cached_file(
+ url_or_filename, check_hash=False, progress=True
+ )
+ checkpoint = torch.load(cached_file, map_location="cpu")
+ elif os.path.isfile(url_or_filename):
+ checkpoint = torch.load(url_or_filename, map_location="cpu")
+ else:
+ raise RuntimeError("checkpoint url or path is invalid")
+
+ if "model" in checkpoint.keys():
+ state_dict = checkpoint["model"]
+ else:
+ state_dict = checkpoint
+
+ msg = self.load_state_dict(state_dict, strict=False)
+
+ logging.info("Missing keys {}".format(msg.missing_keys))
+ logging.info("load checkpoint from %s" % url_or_filename)
+
+ return msg
+
+ @classmethod
+ def from_pretrained(cls, model_type):
+ """
+ Build a pretrained model from default configuration file, specified by model_type.
+
+ Args:
+ - model_type (str): model type, specifying architecture and checkpoints.
+
+ Returns:
+ - model (nn.Module): pretrained or finetuned model, depending on the configuration.
+ """
+ model_cfg = OmegaConf.load(cls.default_config_path(model_type)).model
+ model = cls.from_config(model_cfg)
+
+ return model
+
+ @classmethod
+ def default_config_path(cls, model_type):
+ assert (
+ model_type in cls.PRETRAINED_MODEL_CONFIG_DICT
+ ), "Unknown model type {}".format(model_type)
+ return get_abs_path(cls.PRETRAINED_MODEL_CONFIG_DICT[model_type])
+
+ def load_checkpoint_from_config(self, cfg, **kwargs):
+ """
+ Load checkpoint as specified in the config file.
+
+ If load_finetuned is True, load the finetuned model; otherwise, load the pretrained model.
+ When loading the pretrained model, each task-specific architecture may define their
+ own load_from_pretrained() method.
+ """
+ load_finetuned = cfg.get("load_finetuned", True)
+ if load_finetuned:
+ finetune_path = cfg.get("finetuned", None)
+ assert (
+ finetune_path is not None
+ ), "Found load_finetuned is True, but finetune_path is None."
+ self.load_checkpoint(url_or_filename=finetune_path)
+ else:
+ # load pre-trained weights
+ pretrain_path = cfg.get("pretrained", None)
+ assert "Found load_finetuned is False, but pretrain_path is None."
+ self.load_from_pretrained(url_or_filename=pretrain_path, **kwargs)
+
+ def before_evaluation(self, **kwargs):
+ pass
+
+ def show_n_params(self, return_str=True):
+ tot = 0
+ for p in self.parameters():
+ w = 1
+ for x in p.shape:
+ w *= x
+ tot += w
+ if return_str:
+ if tot >= 1e6:
+ return "{:.1f}M".format(tot / 1e6)
+ else:
+ return "{:.1f}K".format(tot / 1e3)
+ else:
+ return tot
+
+
+class BaseEncoder(nn.Module):
+ """
+ Base class for primitive encoders, such as ViT, TimeSformer, etc.
+ """
+
+ def __init__(self):
+ super().__init__()
+
+ def forward_features(self, samples, **kwargs):
+ raise NotImplementedError
+
+ @property
+ def device(self):
+ return list(self.parameters())[0].device
+
+
+class SharedQueueMixin:
+ @torch.no_grad()
+ def _dequeue_and_enqueue(self, image_feat, text_feat, idxs=None):
+ # gather keys before updating queue
+ image_feats = concat_all_gather(image_feat)
+ text_feats = concat_all_gather(text_feat)
+
+ batch_size = image_feats.shape[0]
+
+ ptr = int(self.queue_ptr)
+ assert self.queue_size % batch_size == 0 # for simplicity
+
+ # replace the keys at ptr (dequeue and enqueue)
+ self.image_queue[:, ptr : ptr + batch_size] = image_feats.T
+ self.text_queue[:, ptr : ptr + batch_size] = text_feats.T
+
+ if idxs is not None:
+ idxs = concat_all_gather(idxs)
+ self.idx_queue[:, ptr : ptr + batch_size] = idxs.T
+
+ ptr = (ptr + batch_size) % self.queue_size # move pointer
+ self.queue_ptr[0] = ptr
+
+
+class MomentumDistilationMixin:
+ @torch.no_grad()
+ def copy_params(self):
+ for model_pair in self.model_pairs:
+ for param, param_m in zip(
+ model_pair[0].parameters(), model_pair[1].parameters()
+ ):
+ param_m.data.copy_(param.data) # initialize
+ param_m.requires_grad = False # not update by gradient
+
+ @torch.no_grad()
+ def _momentum_update(self):
+ for model_pair in self.model_pairs:
+ for param, param_m in zip(
+ model_pair[0].parameters(), model_pair[1].parameters()
+ ):
+ param_m.data = param_m.data * self.momentum + param.data * (
+ 1.0 - self.momentum
+ )
+
+
+class GatherLayer(torch.autograd.Function):
+ """
+ Gather tensors from all workers with support for backward propagation:
+ This implementation does not cut the gradients as torch.distributed.all_gather does.
+ """
+
+ @staticmethod
+ def forward(ctx, x):
+ output = [
+ torch.zeros_like(x) for _ in range(torch.distributed.get_world_size())
+ ]
+ torch.distributed.all_gather(output, x)
+ return tuple(output)
+
+ @staticmethod
+ def backward(ctx, *grads):
+ all_gradients = torch.stack(grads)
+ torch.distributed.all_reduce(all_gradients)
+ return all_gradients[torch.distributed.get_rank()]
+
+
+def all_gather_with_grad(tensors):
+ """
+ Performs all_gather operation on the provided tensors.
+ Graph remains connected for backward grad computation.
+ """
+ # Queue the gathered tensors
+ world_size = torch.distributed.get_world_size()
+ # There is no need for reduction in the single-proc case
+ if world_size == 1:
+ return tensors
+
+ # tensor_all = GatherLayer.apply(tensors)
+ tensor_all = GatherLayer.apply(tensors)
+
+ return torch.cat(tensor_all, dim=0)
+
+
+@torch.no_grad()
+def concat_all_gather(tensor):
+ """
+ Performs all_gather operation on the provided tensors.
+ *** Warning ***: torch.distributed.all_gather has no gradient.
+ """
+ # if use distributed training
+ if not is_dist_avail_and_initialized():
+ return tensor
+
+ tensors_gather = [
+ torch.ones_like(tensor) for _ in range(torch.distributed.get_world_size())
+ ]
+ torch.distributed.all_gather(tensors_gather, tensor, async_op=False)
+
+ output = torch.cat(tensors_gather, dim=0)
+ return output
+
+
+def tile(x, dim, n_tile):
+ init_dim = x.size(dim)
+ repeat_idx = [1] * x.dim()
+ repeat_idx[dim] = n_tile
+ x = x.repeat(*(repeat_idx))
+ order_index = torch.LongTensor(
+ np.concatenate([init_dim * np.arange(n_tile) + i for i in range(init_dim)])
+ )
+ return torch.index_select(x, dim, order_index.to(x.device))
diff --git a/global_local/models/blip2.py b/global_local/models/blip2.py
new file mode 100644
index 0000000000000000000000000000000000000000..1935c584cbc4508de5e85bf891d882a42ff054ea
--- /dev/null
+++ b/global_local/models/blip2.py
@@ -0,0 +1,222 @@
+"""
+Adapted from salesforce@LAVIS. Below is the original copyright:
+ Copyright (c) 2023, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+import contextlib
+import logging
+import os
+import time
+import datetime
+
+import torch
+import torch.nn as nn
+import torch.distributed as dist
+import torch.nn.functional as F
+
+import global_local.common.dist_utils as dist_utils
+from global_local.common.dist_utils import download_cached_file
+from global_local.common.utils import is_url
+from global_local.common.logger import MetricLogger
+from global_local.models.base_model import BaseModel
+from global_local.models.Qformer import BertConfig, BertLMHeadModel
+from global_local.models.eva_vit import create_eva_vit_g
+from transformers import BertTokenizer
+
+
+class Blip2Base(BaseModel):
+ @classmethod
+ def init_tokenizer(cls):
+ tokenizer = BertTokenizer.from_pretrained("bert-base-uncased")
+ tokenizer.add_special_tokens({"bos_token": "[DEC]"})
+ return tokenizer
+
+ def maybe_autocast(self, dtype=torch.float16):
+ # if on cpu, don't use autocast
+ # if on gpu, use autocast with dtype if provided, otherwise use torch.float16
+ enable_autocast = self.device != torch.device("cpu")
+
+ if enable_autocast:
+ return torch.cuda.amp.autocast(dtype=dtype)
+ else:
+ return contextlib.nullcontext()
+
+ @classmethod
+ def init_Qformer(cls, num_query_token, vision_width, cross_attention_freq=2):
+ encoder_config = BertConfig.from_pretrained("bert-base-uncased")
+ encoder_config.encoder_width = vision_width
+ # insert cross-attention layer every other block
+ encoder_config.add_cross_attention = True
+ encoder_config.cross_attention_freq = cross_attention_freq
+ encoder_config.query_length = num_query_token
+ Qformer = BertLMHeadModel(config=encoder_config)
+ query_tokens = nn.Parameter(
+ torch.zeros(1, num_query_token, encoder_config.hidden_size)
+ )
+ query_tokens.data.normal_(mean=0.0, std=encoder_config.initializer_range)
+ return Qformer, query_tokens
+
+ @classmethod
+ def init_vision_encoder(
+ cls, model_name, img_size, drop_path_rate, use_grad_checkpoint, precision
+ ):
+ assert model_name == "eva_clip_g", "vit model must be eva_clip_g for current version of MiniGPT-4"
+ visual_encoder = create_eva_vit_g(
+ img_size, drop_path_rate, use_grad_checkpoint, precision
+ )
+
+ ln_vision = LayerNorm(visual_encoder.num_features)
+ return visual_encoder, ln_vision
+
+ def load_from_pretrained(self, url_or_filename):
+ if is_url(url_or_filename):
+ cached_file = download_cached_file(
+ url_or_filename, check_hash=False, progress=True
+ )
+ checkpoint = torch.load(cached_file, map_location="cpu")
+ elif os.path.isfile(url_or_filename):
+ checkpoint = torch.load(url_or_filename, map_location="cpu")
+ else:
+ raise RuntimeError("checkpoint url or path is invalid")
+
+ state_dict = checkpoint["model"]
+
+ msg = self.load_state_dict(state_dict, strict=False)
+
+ # logging.info("Missing keys {}".format(msg.missing_keys))
+ logging.info("load checkpoint from %s" % url_or_filename)
+
+ return msg
+
+
+def disabled_train(self, mode=True):
+ """Overwrite model.train with this function to make sure train/eval mode
+ does not change anymore."""
+ return self
+
+
+class LayerNorm(nn.LayerNorm):
+ """Subclass torch's LayerNorm to handle fp16."""
+
+ def forward(self, x: torch.Tensor):
+ orig_type = x.dtype
+ ret = super().forward(x.type(torch.float32))
+ return ret.type(orig_type)
+
+
+def compute_sim_matrix(model, data_loader, **kwargs):
+ k_test = kwargs.pop("k_test")
+
+ metric_logger = MetricLogger(delimiter=" ")
+ header = "Evaluation:"
+
+ logging.info("Computing features for evaluation...")
+ start_time = time.time()
+
+ texts = data_loader.dataset.text
+ num_text = len(texts)
+ text_bs = 256
+ text_ids = []
+ text_embeds = []
+ text_atts = []
+ for i in range(0, num_text, text_bs):
+ text = texts[i : min(num_text, i + text_bs)]
+ text_input = model.tokenizer(
+ text,
+ padding="max_length",
+ truncation=True,
+ max_length=35,
+ return_tensors="pt",
+ ).to(model.device)
+ text_feat = model.forward_text(text_input)
+ text_embed = F.normalize(model.text_proj(text_feat))
+ text_embeds.append(text_embed)
+ text_ids.append(text_input.input_ids)
+ text_atts.append(text_input.attention_mask)
+
+ text_embeds = torch.cat(text_embeds, dim=0)
+ text_ids = torch.cat(text_ids, dim=0)
+ text_atts = torch.cat(text_atts, dim=0)
+
+ vit_feats = []
+ image_embeds = []
+ for samples in data_loader:
+ image = samples["image"]
+
+ image = image.to(model.device)
+ image_feat, vit_feat = model.forward_image(image)
+ image_embed = model.vision_proj(image_feat)
+ image_embed = F.normalize(image_embed, dim=-1)
+
+ vit_feats.append(vit_feat.cpu())
+ image_embeds.append(image_embed)
+
+ vit_feats = torch.cat(vit_feats, dim=0)
+ image_embeds = torch.cat(image_embeds, dim=0)
+
+ sims_matrix = []
+ for image_embed in image_embeds:
+ sim_q2t = image_embed @ text_embeds.t()
+ sim_i2t, _ = sim_q2t.max(0)
+ sims_matrix.append(sim_i2t)
+ sims_matrix = torch.stack(sims_matrix, dim=0)
+
+ score_matrix_i2t = torch.full(
+ (len(data_loader.dataset.image), len(texts)), -100.0
+ ).to(model.device)
+
+ num_tasks = dist_utils.get_world_size()
+ rank = dist_utils.get_rank()
+ step = sims_matrix.size(0) // num_tasks + 1
+ start = rank * step
+ end = min(sims_matrix.size(0), start + step)
+
+ for i, sims in enumerate(
+ metric_logger.log_every(sims_matrix[start:end], 50, header)
+ ):
+ topk_sim, topk_idx = sims.topk(k=k_test, dim=0)
+ image_inputs = vit_feats[start + i].repeat(k_test, 1, 1).to(model.device)
+ score = model.compute_itm(
+ image_inputs=image_inputs,
+ text_ids=text_ids[topk_idx],
+ text_atts=text_atts[topk_idx],
+ ).float()
+ score_matrix_i2t[start + i, topk_idx] = score + topk_sim
+
+ sims_matrix = sims_matrix.t()
+ score_matrix_t2i = torch.full(
+ (len(texts), len(data_loader.dataset.image)), -100.0
+ ).to(model.device)
+
+ step = sims_matrix.size(0) // num_tasks + 1
+ start = rank * step
+ end = min(sims_matrix.size(0), start + step)
+
+ for i, sims in enumerate(
+ metric_logger.log_every(sims_matrix[start:end], 50, header)
+ ):
+ topk_sim, topk_idx = sims.topk(k=k_test, dim=0)
+ image_inputs = vit_feats[topk_idx.cpu()].to(model.device)
+ score = model.compute_itm(
+ image_inputs=image_inputs,
+ text_ids=text_ids[start + i].repeat(k_test, 1),
+ text_atts=text_atts[start + i].repeat(k_test, 1),
+ ).float()
+ score_matrix_t2i[start + i, topk_idx] = score + topk_sim
+
+ if dist_utils.is_dist_avail_and_initialized():
+ dist.barrier()
+ torch.distributed.all_reduce(
+ score_matrix_i2t, op=torch.distributed.ReduceOp.SUM
+ )
+ torch.distributed.all_reduce(
+ score_matrix_t2i, op=torch.distributed.ReduceOp.SUM
+ )
+
+ total_time = time.time() - start_time
+ total_time_str = str(datetime.timedelta(seconds=int(total_time)))
+ logging.info("Evaluation time {}".format(total_time_str))
+
+ return score_matrix_i2t.cpu().numpy(), score_matrix_t2i.cpu().numpy()
diff --git a/global_local/models/blip2_outputs.py b/global_local/models/blip2_outputs.py
new file mode 100644
index 0000000000000000000000000000000000000000..92d83a0556e6c5c3c0a603279f318605ae25d6d5
--- /dev/null
+++ b/global_local/models/blip2_outputs.py
@@ -0,0 +1,111 @@
+"""
+Adapted from salesforce@LAVIS. Below is the original copyright:
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from dataclasses import dataclass
+from typing import Optional
+
+import torch
+from transformers.modeling_outputs import (
+ ModelOutput,
+ BaseModelOutputWithPoolingAndCrossAttentions,
+ CausalLMOutputWithCrossAttentions,
+)
+
+
+@dataclass
+class BlipSimilarity(ModelOutput):
+ sim_i2t: torch.FloatTensor = None
+ sim_t2i: torch.FloatTensor = None
+
+ sim_i2t_m: Optional[torch.FloatTensor] = None
+ sim_t2i_m: Optional[torch.FloatTensor] = None
+
+ sim_i2t_targets: Optional[torch.FloatTensor] = None
+ sim_t2i_targets: Optional[torch.FloatTensor] = None
+
+
+@dataclass
+class BlipIntermediateOutput(ModelOutput):
+ """
+ Data class for intermediate outputs of BLIP models.
+
+ image_embeds (torch.FloatTensor): Image embeddings, shape (batch_size, num_patches, embed_dim).
+ text_embeds (torch.FloatTensor): Text embeddings, shape (batch_size, seq_len, embed_dim).
+
+ image_embeds_m (torch.FloatTensor): Image embeddings from momentum visual encoder, shape (batch_size, num_patches, embed_dim).
+ text_embeds_m (torch.FloatTensor): Text embeddings from momentum text encoder, shape (batch_size, seq_len, embed_dim).
+
+ encoder_output (BaseModelOutputWithPoolingAndCrossAttentions): output from the image-grounded text encoder.
+ encoder_output_neg (BaseModelOutputWithPoolingAndCrossAttentions): output from the image-grounded text encoder for negative pairs.
+
+ decoder_output (CausalLMOutputWithCrossAttentions): output from the image-grounded text decoder.
+ decoder_labels (torch.LongTensor): labels for the captioning loss.
+
+ itm_logits (torch.FloatTensor): logits for the image-text matching loss, shape (batch_size * 3, 2).
+ itm_labels (torch.LongTensor): labels for the image-text matching loss, shape (batch_size * 3,)
+
+ """
+
+ # uni-modal features
+ image_embeds: torch.FloatTensor = None
+ text_embeds: Optional[torch.FloatTensor] = None
+
+ image_embeds_m: Optional[torch.FloatTensor] = None
+ text_embeds_m: Optional[torch.FloatTensor] = None
+
+ # intermediate outputs of multimodal encoder
+ encoder_output: Optional[BaseModelOutputWithPoolingAndCrossAttentions] = None
+ encoder_output_neg: Optional[BaseModelOutputWithPoolingAndCrossAttentions] = None
+
+ itm_logits: Optional[torch.FloatTensor] = None
+ itm_labels: Optional[torch.LongTensor] = None
+
+ # intermediate outputs of multimodal decoder
+ decoder_output: Optional[CausalLMOutputWithCrossAttentions] = None
+ decoder_labels: Optional[torch.LongTensor] = None
+
+
+@dataclass
+class BlipOutput(ModelOutput):
+ # some finetuned models (e.g. BlipVQA) do not compute similarity, thus optional.
+ sims: Optional[BlipSimilarity] = None
+
+ intermediate_output: BlipIntermediateOutput = None
+
+ loss: Optional[torch.FloatTensor] = None
+
+ loss_itc: Optional[torch.FloatTensor] = None
+
+ loss_itm: Optional[torch.FloatTensor] = None
+
+ loss_lm: Optional[torch.FloatTensor] = None
+
+
+@dataclass
+class BlipOutputFeatures(ModelOutput):
+ """
+ Data class of features from BlipFeatureExtractor.
+
+ Args:
+ image_embeds: (torch.FloatTensor) of shape (batch_size, num_patches+1, embed_dim), optional
+ image_features: (torch.FloatTensor) of shape (batch_size, num_patches+1, feature_dim), optional
+ text_embeds: (torch.FloatTensor) of shape (batch_size, sequence_length+1, embed_dim), optional
+ text_features: (torch.FloatTensor) of shape (batch_size, sequence_length+1, feature_dim), optional
+
+ The first embedding or feature is for the [CLS] token.
+
+ Features are obtained by projecting the corresponding embedding into a normalized low-dimensional space.
+ """
+
+ image_embeds: Optional[torch.FloatTensor] = None
+ image_embeds_proj: Optional[torch.FloatTensor] = None
+
+ text_embeds: Optional[torch.FloatTensor] = None
+ text_embeds_proj: Optional[torch.FloatTensor] = None
+
+ multimodal_embeds: Optional[torch.FloatTensor] = None
diff --git a/global_local/models/eva_vit.py b/global_local/models/eva_vit.py
new file mode 100644
index 0000000000000000000000000000000000000000..abc1cda9fe26c4c184a627e8b4835d3860343f95
--- /dev/null
+++ b/global_local/models/eva_vit.py
@@ -0,0 +1,505 @@
+# Based on EVA, BEIT, timm and DeiT code bases
+# https://github.com/baaivision/EVA
+# https://github.com/rwightman/pytorch-image-models/tree/master/timm
+# https://github.com/microsoft/unilm/tree/master/beit
+# https://github.com/facebookresearch/deit/
+# https://github.com/facebookresearch/dino
+# --------------------------------------------------------'
+import math
+from functools import partial
+
+import torch
+import torch.nn as nn
+import torch.nn.functional as F
+import torch.utils.checkpoint as checkpoint
+from timm.models.layers import drop_path, to_2tuple, trunc_normal_
+from timm.models.registry import register_model
+
+from global_local.common.dist_utils import download_cached_file
+
+def _cfg(url='', **kwargs):
+ return {
+ 'url': url,
+ 'num_classes': 1000, 'input_size': (3, 224, 224), 'pool_size': None,
+ 'crop_pct': .9, 'interpolation': 'bicubic',
+ 'mean': (0.5, 0.5, 0.5), 'std': (0.5, 0.5, 0.5),
+ **kwargs
+ }
+
+
+class DropPath(nn.Module):
+ """Drop paths (Stochastic Depth) per sample (when applied in main path of residual blocks).
+ """
+ def __init__(self, drop_prob=None):
+ super(DropPath, self).__init__()
+ self.drop_prob = drop_prob
+
+ def forward(self, x):
+ return drop_path(x, self.drop_prob, self.training)
+
+ def extra_repr(self) -> str:
+ return 'p={}'.format(self.drop_prob)
+
+
+class Mlp(nn.Module):
+ def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.):
+ super().__init__()
+ out_features = out_features or in_features
+ hidden_features = hidden_features or in_features
+ self.fc1 = nn.Linear(in_features, hidden_features)
+ self.act = act_layer()
+ self.fc2 = nn.Linear(hidden_features, out_features)
+ self.drop = nn.Dropout(drop)
+
+ def forward(self, x):
+ x = self.fc1(x)
+ x = self.act(x)
+ # x = self.drop(x)
+ # commit this for the orignal BERT implement
+ x = self.fc2(x)
+ x = self.drop(x)
+ return x
+
+
+class Attention(nn.Module):
+ def __init__(
+ self, dim, num_heads=8, qkv_bias=False, qk_scale=None, attn_drop=0.,
+ proj_drop=0., window_size=None, attn_head_dim=None):
+ super().__init__()
+ self.num_heads = num_heads
+ head_dim = dim // num_heads
+ if attn_head_dim is not None:
+ head_dim = attn_head_dim
+ all_head_dim = head_dim * self.num_heads
+ self.scale = qk_scale or head_dim ** -0.5
+
+ self.qkv = nn.Linear(dim, all_head_dim * 3, bias=False)
+ if qkv_bias:
+ self.q_bias = nn.Parameter(torch.zeros(all_head_dim))
+ self.v_bias = nn.Parameter(torch.zeros(all_head_dim))
+ else:
+ self.q_bias = None
+ self.v_bias = None
+
+ if window_size:
+ self.window_size = window_size
+ self.num_relative_distance = (2 * window_size[0] - 1) * (2 * window_size[1] - 1) + 3
+ self.relative_position_bias_table = nn.Parameter(
+ torch.zeros(self.num_relative_distance, num_heads)) # 2*Wh-1 * 2*Ww-1, nH
+ # cls to token & token 2 cls & cls to cls
+
+ # get pair-wise relative position index for each token inside the window
+ coords_h = torch.arange(window_size[0])
+ coords_w = torch.arange(window_size[1])
+ coords = torch.stack(torch.meshgrid([coords_h, coords_w])) # 2, Wh, Ww
+ coords_flatten = torch.flatten(coords, 1) # 2, Wh*Ww
+ relative_coords = coords_flatten[:, :, None] - coords_flatten[:, None, :] # 2, Wh*Ww, Wh*Ww
+ relative_coords = relative_coords.permute(1, 2, 0).contiguous() # Wh*Ww, Wh*Ww, 2
+ relative_coords[:, :, 0] += window_size[0] - 1 # shift to start from 0
+ relative_coords[:, :, 1] += window_size[1] - 1
+ relative_coords[:, :, 0] *= 2 * window_size[1] - 1
+ relative_position_index = \
+ torch.zeros(size=(window_size[0] * window_size[1] + 1, ) * 2, dtype=relative_coords.dtype)
+ relative_position_index[1:, 1:] = relative_coords.sum(-1) # Wh*Ww, Wh*Ww
+ relative_position_index[0, 0:] = self.num_relative_distance - 3
+ relative_position_index[0:, 0] = self.num_relative_distance - 2
+ relative_position_index[0, 0] = self.num_relative_distance - 1
+
+ self.register_buffer("relative_position_index", relative_position_index)
+ else:
+ self.window_size = None
+ self.relative_position_bias_table = None
+ self.relative_position_index = None
+
+ self.attn_drop = nn.Dropout(attn_drop)
+ self.proj = nn.Linear(all_head_dim, dim)
+ self.proj_drop = nn.Dropout(proj_drop)
+
+ def forward(self, x, rel_pos_bias=None):
+ B, N, C = x.shape
+ qkv_bias = None
+ if self.q_bias is not None:
+ qkv_bias = torch.cat((self.q_bias, torch.zeros_like(self.v_bias, requires_grad=False), self.v_bias))
+ # qkv = self.qkv(x).reshape(B, N, 3, self.num_heads, C // self.num_heads).permute(2, 0, 3, 1, 4)
+ qkv = F.linear(input=x, weight=self.qkv.weight, bias=qkv_bias)
+ qkv = qkv.reshape(B, N, 3, self.num_heads, -1).permute(2, 0, 3, 1, 4)
+ q, k, v = qkv[0], qkv[1], qkv[2] # make torchscript happy (cannot use tensor as tuple)
+
+ q = q * self.scale
+ attn = (q @ k.transpose(-2, -1))
+
+ if self.relative_position_bias_table is not None:
+ relative_position_bias = \
+ self.relative_position_bias_table[self.relative_position_index.view(-1)].view(
+ self.window_size[0] * self.window_size[1] + 1,
+ self.window_size[0] * self.window_size[1] + 1, -1) # Wh*Ww,Wh*Ww,nH
+ relative_position_bias = relative_position_bias.permute(2, 0, 1).contiguous() # nH, Wh*Ww, Wh*Ww
+ attn = attn + relative_position_bias.unsqueeze(0)
+
+ if rel_pos_bias is not None:
+ attn = attn + rel_pos_bias
+
+ attn = attn.softmax(dim=-1)
+ attn = self.attn_drop(attn)
+
+ x = (attn @ v).transpose(1, 2).reshape(B, N, -1)
+ x = self.proj(x)
+ x = self.proj_drop(x)
+ return x
+
+ def get_attn_weights(self, x, rel_pos_bias=None):
+ B, N, C = x.shape
+ qkv_bias = None
+ if self.q_bias is not None:
+ qkv_bias = torch.cat((self.q_bias, torch.zeros_like(self.v_bias, requires_grad=False), self.v_bias))
+ # qkv = self.qkv(x).reshape(B, N, 3, self.num_heads, C // self.num_heads).permute(2, 0, 3, 1, 4)
+ qkv = F.linear(input=x, weight=self.qkv.weight, bias=qkv_bias)
+ qkv = qkv.reshape(B, N, 3, self.num_heads, -1).permute(2, 0, 3, 1, 4)
+ q, k, v = qkv[0], qkv[1], qkv[2] # make torchscript happy (cannot use tensor as tuple)
+
+ q = q * self.scale
+ attn = (q @ k.transpose(-2, -1))
+
+ if self.relative_position_bias_table is not None:
+ relative_position_bias = \
+ self.relative_position_bias_table[self.relative_position_index.view(-1)].view(
+ self.window_size[0] * self.window_size[1] + 1,
+ self.window_size[0] * self.window_size[1] + 1, -1) # Wh*Ww,Wh*Ww,nH
+ relative_position_bias = relative_position_bias.permute(2, 0, 1).contiguous() # nH, Wh*Ww, Wh*Ww
+ attn = attn + relative_position_bias.unsqueeze(0)
+
+ if rel_pos_bias is not None:
+ attn = attn + rel_pos_bias
+
+ normalized_attn = attn.softmax(dim=-1)
+ attn = self.attn_drop(normalized_attn)
+
+ x = (attn @ v).transpose(1, 2).reshape(B, N, -1)
+ x = self.proj(x)
+ x = self.proj_drop(x)
+ return x, normalized_attn
+
+
+class Block(nn.Module):
+
+ def __init__(self, dim, num_heads, mlp_ratio=4., qkv_bias=False, qk_scale=None, drop=0., attn_drop=0.,
+ drop_path=0., init_values=None, act_layer=nn.GELU, norm_layer=nn.LayerNorm,
+ window_size=None, attn_head_dim=None):
+ super().__init__()
+ self.norm1 = norm_layer(dim)
+ self.attn = Attention(
+ dim, num_heads=num_heads, qkv_bias=qkv_bias, qk_scale=qk_scale,
+ attn_drop=attn_drop, proj_drop=drop, window_size=window_size, attn_head_dim=attn_head_dim)
+ # NOTE: drop path for stochastic depth, we shall see if this is better than dropout here
+ self.drop_path = DropPath(drop_path) if drop_path > 0. else nn.Identity()
+ self.norm2 = norm_layer(dim)
+ mlp_hidden_dim = int(dim * mlp_ratio)
+ self.mlp = Mlp(in_features=dim, hidden_features=mlp_hidden_dim, act_layer=act_layer, drop=drop)
+
+ if init_values is not None and init_values > 0:
+ self.gamma_1 = nn.Parameter(init_values * torch.ones((dim)),requires_grad=True)
+ self.gamma_2 = nn.Parameter(init_values * torch.ones((dim)),requires_grad=True)
+ else:
+ self.gamma_1, self.gamma_2 = None, None
+
+ def forward(self, x, rel_pos_bias=None):
+ if self.gamma_1 is None:
+ x = x + self.drop_path(self.attn(self.norm1(x), rel_pos_bias=rel_pos_bias))
+ x = x + self.drop_path(self.mlp(self.norm2(x)))
+ else:
+ x = x + self.drop_path(self.gamma_1 * self.attn(self.norm1(x), rel_pos_bias=rel_pos_bias))
+ x = x + self.drop_path(self.gamma_2 * self.mlp(self.norm2(x)))
+ return x
+
+ def get_attn_weights(self, x, rel_pos_bias=None):
+ if self.gamma_1 is None:
+ tmp, tmp_attn = self.attn.get_attn_weights(self.norm1(x), rel_pos_bias=rel_pos_bias)
+ x = x + self.drop_path(tmp)
+ x = x + self.drop_path(self.mlp(self.norm2(x)))
+ else:
+ tmp, tmp_attn = self.attn.get_attn_weights(self.norm1(x), rel_pos_bias=rel_pos_bias)
+ x = x + self.drop_path(self.gamma_1 * tmp)
+ x = x + self.drop_path(self.gamma_2 * self.mlp(self.norm2(x)))
+ return x, tmp_attn
+
+
+class PatchEmbed(nn.Module):
+ """ Image to Patch Embedding
+ """
+ def __init__(self, img_size=224, patch_size=16, in_chans=3, embed_dim=768):
+ super().__init__()
+ img_size = to_2tuple(img_size)
+ patch_size = to_2tuple(patch_size)
+ num_patches = (img_size[1] // patch_size[1]) * (img_size[0] // patch_size[0])
+ self.patch_shape = (img_size[0] // patch_size[0], img_size[1] // patch_size[1])
+ self.img_size = img_size
+ self.patch_size = patch_size
+ self.num_patches = num_patches
+
+ self.proj = nn.Conv2d(in_chans, embed_dim, kernel_size=patch_size, stride=patch_size)
+
+ def forward(self, x, **kwargs):
+ B, C, H, W = x.shape
+ # FIXME look at relaxing size constraints
+ assert H == self.img_size[0] and W == self.img_size[1], \
+ f"Input image size ({H}*{W}) doesn't match model ({self.img_size[0]}*{self.img_size[1]})."
+ x = self.proj(x).flatten(2).transpose(1, 2)
+ return x
+
+
+class RelativePositionBias(nn.Module):
+
+ def __init__(self, window_size, num_heads):
+ super().__init__()
+ self.window_size = window_size
+ self.num_relative_distance = (2 * window_size[0] - 1) * (2 * window_size[1] - 1) + 3
+ self.relative_position_bias_table = nn.Parameter(
+ torch.zeros(self.num_relative_distance, num_heads)) # 2*Wh-1 * 2*Ww-1, nH
+ # cls to token & token 2 cls & cls to cls
+
+ # get pair-wise relative position index for each token inside the window
+ coords_h = torch.arange(window_size[0])
+ coords_w = torch.arange(window_size[1])
+ coords = torch.stack(torch.meshgrid([coords_h, coords_w])) # 2, Wh, Ww
+ coords_flatten = torch.flatten(coords, 1) # 2, Wh*Ww
+ relative_coords = coords_flatten[:, :, None] - coords_flatten[:, None, :] # 2, Wh*Ww, Wh*Ww
+ relative_coords = relative_coords.permute(1, 2, 0).contiguous() # Wh*Ww, Wh*Ww, 2
+ relative_coords[:, :, 0] += window_size[0] - 1 # shift to start from 0
+ relative_coords[:, :, 1] += window_size[1] - 1
+ relative_coords[:, :, 0] *= 2 * window_size[1] - 1
+ relative_position_index = \
+ torch.zeros(size=(window_size[0] * window_size[1] + 1,) * 2, dtype=relative_coords.dtype)
+ relative_position_index[1:, 1:] = relative_coords.sum(-1) # Wh*Ww, Wh*Ww
+ relative_position_index[0, 0:] = self.num_relative_distance - 3
+ relative_position_index[0:, 0] = self.num_relative_distance - 2
+ relative_position_index[0, 0] = self.num_relative_distance - 1
+
+ self.register_buffer("relative_position_index", relative_position_index)
+
+ # trunc_normal_(self.relative_position_bias_table, std=.02)
+
+ def forward(self):
+ relative_position_bias = \
+ self.relative_position_bias_table[self.relative_position_index.view(-1)].view(
+ self.window_size[0] * self.window_size[1] + 1,
+ self.window_size[0] * self.window_size[1] + 1, -1) # Wh*Ww,Wh*Ww,nH
+ return relative_position_bias.permute(2, 0, 1).contiguous() # nH, Wh*Ww, Wh*Ww
+
+
+class VisionTransformer(nn.Module):
+ """ Vision Transformer with support for patch or hybrid CNN input stage
+ """
+ def __init__(self, img_size=224, patch_size=16, in_chans=3, num_classes=1000, embed_dim=768, depth=12,
+ num_heads=12, mlp_ratio=4., qkv_bias=False, qk_scale=None, drop_rate=0., attn_drop_rate=0.,
+ drop_path_rate=0., norm_layer=nn.LayerNorm, init_values=None,
+ use_abs_pos_emb=True, use_rel_pos_bias=False, use_shared_rel_pos_bias=False,
+ use_mean_pooling=True, init_scale=0.001, use_checkpoint=False):
+ super().__init__()
+ self.image_size = img_size
+ self.num_classes = num_classes
+ self.num_features = self.embed_dim = embed_dim # num_features for consistency with other models
+
+ self.patch_embed = PatchEmbed(
+ img_size=img_size, patch_size=patch_size, in_chans=in_chans, embed_dim=embed_dim)
+ num_patches = self.patch_embed.num_patches
+
+ self.cls_token = nn.Parameter(torch.zeros(1, 1, embed_dim))
+ if use_abs_pos_emb:
+ self.pos_embed = nn.Parameter(torch.zeros(1, num_patches + 1, embed_dim))
+ else:
+ self.pos_embed = None
+ self.pos_drop = nn.Dropout(p=drop_rate)
+
+ if use_shared_rel_pos_bias:
+ self.rel_pos_bias = RelativePositionBias(window_size=self.patch_embed.patch_shape, num_heads=num_heads)
+ else:
+ self.rel_pos_bias = None
+ self.use_checkpoint = use_checkpoint
+
+ dpr = [x.item() for x in torch.linspace(0, drop_path_rate, depth)] # stochastic depth decay rule
+ self.use_rel_pos_bias = use_rel_pos_bias
+ self.blocks = nn.ModuleList([
+ Block(
+ dim=embed_dim, num_heads=num_heads, mlp_ratio=mlp_ratio, qkv_bias=qkv_bias, qk_scale=qk_scale,
+ drop=drop_rate, attn_drop=attn_drop_rate, drop_path=dpr[i], norm_layer=norm_layer,
+ init_values=init_values, window_size=self.patch_embed.patch_shape if use_rel_pos_bias else None)
+ for i in range(depth)])
+# self.norm = nn.Identity() if use_mean_pooling else norm_layer(embed_dim)
+# self.fc_norm = norm_layer(embed_dim) if use_mean_pooling else None
+# self.head = nn.Linear(embed_dim, num_classes) if num_classes > 0 else nn.Identity()
+
+ if self.pos_embed is not None:
+ trunc_normal_(self.pos_embed, std=.02)
+ trunc_normal_(self.cls_token, std=.02)
+ # trunc_normal_(self.mask_token, std=.02)
+# if isinstance(self.head, nn.Linear):
+# trunc_normal_(self.head.weight, std=.02)
+ self.apply(self._init_weights)
+ self.fix_init_weight()
+# if isinstance(self.head, nn.Linear):
+# self.head.weight.data.mul_(init_scale)
+# self.head.bias.data.mul_(init_scale)
+
+ def fix_init_weight(self):
+ def rescale(param, layer_id):
+ param.div_(math.sqrt(2.0 * layer_id))
+
+ for layer_id, layer in enumerate(self.blocks):
+ rescale(layer.attn.proj.weight.data, layer_id + 1)
+ rescale(layer.mlp.fc2.weight.data, layer_id + 1)
+
+ def _init_weights(self, m):
+ if isinstance(m, nn.Linear):
+ trunc_normal_(m.weight, std=.02)
+ if isinstance(m, nn.Linear) and m.bias is not None:
+ nn.init.constant_(m.bias, 0)
+ elif isinstance(m, nn.LayerNorm):
+ nn.init.constant_(m.bias, 0)
+ nn.init.constant_(m.weight, 1.0)
+
+ def get_classifier(self):
+ return self.head
+
+ def reset_classifier(self, num_classes, global_pool=''):
+ self.num_classes = num_classes
+ self.head = nn.Linear(self.embed_dim, num_classes) if num_classes > 0 else nn.Identity()
+
+ def forward_features(self, x):
+ x = self.patch_embed(x)
+ batch_size, seq_len, _ = x.size()
+
+ cls_tokens = self.cls_token.expand(batch_size, -1, -1) # stole cls_tokens impl from Phil Wang, thanks
+ x = torch.cat((cls_tokens, x), dim=1)
+ if self.pos_embed is not None:
+ x = x + self.pos_embed
+ x = self.pos_drop(x)
+
+ rel_pos_bias = self.rel_pos_bias() if self.rel_pos_bias is not None else None
+ for blk in self.blocks:
+ if self.use_checkpoint:
+ x = checkpoint.checkpoint(blk, x, rel_pos_bias)
+ else:
+ x = blk(x, rel_pos_bias)
+ return x
+# x = self.norm(x)
+
+# if self.fc_norm is not None:
+# t = x[:, 1:, :]
+# return self.fc_norm(t.mean(1))
+# else:
+# return x[:, 0]
+
+ def forward(self, x):
+ x = self.forward_features(x)
+# x = self.head(x)
+ return x
+
+ def get_intermediate_layers(self, x):
+ x = self.patch_embed(x)
+ batch_size, seq_len, _ = x.size()
+
+ cls_tokens = self.cls_token.expand(batch_size, -1, -1) # stole cls_tokens impl from Phil Wang, thanks
+ x = torch.cat((cls_tokens, x), dim=1)
+ if self.pos_embed is not None:
+ x = x + self.pos_embed
+ x = self.pos_drop(x)
+
+ features = []
+ rel_pos_bias = self.rel_pos_bias() if self.rel_pos_bias is not None else None
+ for blk in self.blocks:
+ x = blk(x, rel_pos_bias)
+ features.append(x)
+
+ return features
+
+ def get_attn_weights(self, x):
+ x = self.patch_embed(x)
+ batch_size, seq_len, _ = x.size()
+
+ cls_tokens = self.cls_token.expand(batch_size, -1, -1) # stole cls_tokens impl from Phil Wang, thanks
+ x = torch.cat((cls_tokens, x), dim=1)
+ if self.pos_embed is not None:
+ x = x + self.pos_embed
+ x = self.pos_drop(x)
+
+ rel_pos_bias = self.rel_pos_bias() if self.rel_pos_bias is not None else None
+ all_attn = []
+ for blk in self.blocks:
+ if self.use_checkpoint:
+ x, x_attn = checkpoint.checkpoint(blk, x, rel_pos_bias)
+ else:
+ x, x_attn = blk.get_attn_weights(x, rel_pos_bias)
+ all_attn.append(x_attn)
+
+ return x, all_attn
+
+def interpolate_pos_embed(model, checkpoint_model):
+ if 'pos_embed' in checkpoint_model:
+ pos_embed_checkpoint = checkpoint_model['pos_embed'].float()
+ embedding_size = pos_embed_checkpoint.shape[-1]
+ num_patches = model.patch_embed.num_patches
+ num_extra_tokens = model.pos_embed.shape[-2] - num_patches
+ # height (== width) for the checkpoint position embedding
+ orig_size = int((pos_embed_checkpoint.shape[-2] - num_extra_tokens) ** 0.5)
+ # height (== width) for the new position embedding
+ new_size = int(num_patches ** 0.5)
+ # class_token and dist_token are kept unchanged
+ if orig_size != new_size:
+ print("Position interpolate from %dx%d to %dx%d" % (orig_size, orig_size, new_size, new_size))
+ extra_tokens = pos_embed_checkpoint[:, :num_extra_tokens]
+ # only the position tokens are interpolated
+ pos_tokens = pos_embed_checkpoint[:, num_extra_tokens:]
+ pos_tokens = pos_tokens.reshape(-1, orig_size, orig_size, embedding_size).permute(0, 3, 1, 2)
+ pos_tokens = torch.nn.functional.interpolate(
+ pos_tokens, size=(new_size, new_size), mode='bicubic', align_corners=False)
+ pos_tokens = pos_tokens.permute(0, 2, 3, 1).flatten(1, 2)
+ new_pos_embed = torch.cat((extra_tokens, pos_tokens), dim=1)
+ checkpoint_model['pos_embed'] = new_pos_embed
+
+
+def convert_weights_to_fp16(model: nn.Module):
+ """Convert applicable model parameters to fp16"""
+
+ def _convert_weights_to_fp16(l):
+ if isinstance(l, (nn.Conv1d, nn.Conv2d, nn.Linear)):
+ l.weight.data = l.weight.data.half()
+ if l.bias is not None:
+ l.bias.data = l.bias.data.half()
+
+# if isinstance(l, (nn.MultiheadAttention, Attention)):
+# for attr in [*[f"{s}_proj_weight" for s in ["in", "q", "k", "v"]], "in_proj_bias", "bias_k", "bias_v"]:
+# tensor = getattr(l, attr)
+# if tensor is not None:
+# tensor.data = tensor.data.half()
+
+ model.apply(_convert_weights_to_fp16)
+
+
+def create_eva_vit_g(img_size=224,drop_path_rate=0.4,use_checkpoint=False,precision="fp16"):
+ model = VisionTransformer(
+ img_size=img_size,
+ patch_size=14,
+ use_mean_pooling=False,
+ embed_dim=1408,
+ depth=39,
+ num_heads=1408//88,
+ mlp_ratio=4.3637,
+ qkv_bias=True,
+ drop_path_rate=drop_path_rate,
+ norm_layer=partial(nn.LayerNorm, eps=1e-6),
+ use_checkpoint=use_checkpoint,
+ )
+ url = "https://storage.googleapis.com/sfr-vision-language-research/LAVIS/models/BLIP2/eva_vit_g.pth"
+ cached_file = download_cached_file(
+ url, check_hash=False, progress=True
+ )
+ state_dict = torch.load(cached_file, map_location="cpu")
+ interpolate_pos_embed(model,state_dict)
+
+ incompatible_keys = model.load_state_dict(state_dict, strict=False)
+# print(incompatible_keys)
+
+ if precision == "fp16":
+# model.to("cuda")
+ convert_weights_to_fp16(model)
+ return model
\ No newline at end of file
diff --git a/global_local/models/modeling_llama.py b/global_local/models/modeling_llama.py
new file mode 100644
index 0000000000000000000000000000000000000000..4a608a3f1c04b653d384e3d61974a6a1718cc83e
--- /dev/null
+++ b/global_local/models/modeling_llama.py
@@ -0,0 +1,767 @@
+# This script is based on https://github.com/huggingface/transformers/blob/main/src/transformers/models/llama/modeling_llama.py
+
+""" PyTorch LLaMA model."""
+import math
+from typing import List, Optional, Tuple, Union
+
+import torch
+import torch.utils.checkpoint
+from torch import nn
+from torch.nn import BCEWithLogitsLoss, CrossEntropyLoss, MSELoss
+
+from transformers.activations import ACT2FN
+from transformers.modeling_outputs import BaseModelOutputWithPast, CausalLMOutputWithPast, SequenceClassifierOutputWithPast
+from transformers.modeling_utils import PreTrainedModel
+from transformers.utils import add_start_docstrings, add_start_docstrings_to_model_forward, logging, replace_return_docstrings
+from transformers.models.llama.configuration_llama import LlamaConfig
+
+
+logger = logging.get_logger(__name__)
+
+_CONFIG_FOR_DOC = "LlamaConfig"
+
+
+# Copied from transformers.models.bart.modeling_bart._make_causal_mask
+def _make_causal_mask(
+ input_ids_shape: torch.Size, dtype: torch.dtype, device: torch.device, past_key_values_length: int = 0
+):
+ """
+ Make causal mask used for bi-directional self-attention.
+ """
+ bsz, tgt_len = input_ids_shape
+ mask = torch.full((tgt_len, tgt_len), torch.tensor(torch.finfo(dtype).min, device=device), device=device)
+ mask_cond = torch.arange(mask.size(-1), device=device)
+ mask.masked_fill_(mask_cond < (mask_cond + 1).view(mask.size(-1), 1), 0)
+ mask = mask.to(dtype)
+
+ if past_key_values_length > 0:
+ mask = torch.cat([torch.zeros(tgt_len, past_key_values_length, dtype=dtype, device=device), mask], dim=-1)
+ return mask[None, None, :, :].expand(bsz, 1, tgt_len, tgt_len + past_key_values_length)
+
+
+# Copied from transformers.models.bart.modeling_bart._expand_mask
+def _expand_mask(mask: torch.Tensor, dtype: torch.dtype, tgt_len: Optional[int] = None):
+ """
+ Expands attention_mask from `[bsz, seq_len]` to `[bsz, 1, tgt_seq_len, src_seq_len]`.
+ """
+ bsz, src_len = mask.size()
+ tgt_len = tgt_len if tgt_len is not None else src_len
+
+ expanded_mask = mask[:, None, None, :].expand(bsz, 1, tgt_len, src_len).to(dtype)
+
+ inverted_mask = 1.0 - expanded_mask
+
+ return inverted_mask.masked_fill(inverted_mask.to(torch.bool), torch.finfo(dtype).min)
+
+
+class LlamaRMSNorm(nn.Module):
+ def __init__(self, hidden_size, eps=1e-6):
+ """
+ LlamaRMSNorm is equivalent to T5LayerNorm
+ """
+ super().__init__()
+ self.weight = nn.Parameter(torch.ones(hidden_size))
+ self.variance_epsilon = eps
+
+ def forward(self, hidden_states):
+ variance = hidden_states.to(torch.float32).pow(2).mean(-1, keepdim=True)
+ hidden_states = hidden_states * torch.rsqrt(variance + self.variance_epsilon)
+
+ # convert into half-precision if necessary
+ if self.weight.dtype in [torch.float16, torch.bfloat16]:
+ hidden_states = hidden_states.to(self.weight.dtype)
+
+ return self.weight * hidden_states
+
+
+class LlamaRotaryEmbedding(torch.nn.Module):
+ def __init__(self, dim, max_position_embeddings=2048, base=10000, device=None):
+ super().__init__()
+ inv_freq = 1.0 / (base ** (torch.arange(0, dim, 2).float().to(device) / dim))
+ self.register_buffer("inv_freq", inv_freq)
+
+ # Build here to make `torch.jit.trace` work.
+ self.max_seq_len_cached = max_position_embeddings
+ t = torch.arange(self.max_seq_len_cached, device=self.inv_freq.device, dtype=self.inv_freq.dtype)
+ freqs = torch.einsum("i,j->ij", t, self.inv_freq)
+ # Different from paper, but it uses a different permutation in order to obtain the same calculation
+ emb = torch.cat((freqs, freqs), dim=-1)
+ self.register_buffer("cos_cached", emb.cos()[None, None, :, :], persistent=False)
+ self.register_buffer("sin_cached", emb.sin()[None, None, :, :], persistent=False)
+
+ def forward(self, x, seq_len=None):
+ # x: [bs, num_attention_heads, seq_len, head_size]
+ # This `if` block is unlikely to be run after we build sin/cos in `__init__`. Keep the logic here just in case.
+ if seq_len > self.max_seq_len_cached:
+ self.max_seq_len_cached = seq_len
+ t = torch.arange(self.max_seq_len_cached, device=x.device, dtype=self.inv_freq.dtype)
+ freqs = torch.einsum("i,j->ij", t, self.inv_freq)
+ # Different from paper, but it uses a different permutation in order to obtain the same calculation
+ emb = torch.cat((freqs, freqs), dim=-1).to(x.device)
+ self.register_buffer("cos_cached", emb.cos()[None, None, :, :], persistent=False)
+ self.register_buffer("sin_cached", emb.sin()[None, None, :, :], persistent=False)
+ return (
+ self.cos_cached[:, :, :seq_len, ...].to(dtype=x.dtype),
+ self.sin_cached[:, :, :seq_len, ...].to(dtype=x.dtype),
+ )
+
+
+def rotate_half(x):
+ """Rotates half the hidden dims of the input."""
+ x1 = x[..., : x.shape[-1] // 2]
+ x2 = x[..., x.shape[-1] // 2 :]
+ return torch.cat((-x2, x1), dim=-1)
+
+
+def apply_rotary_pos_emb(q, k, cos, sin, position_ids):
+ gather_indices = position_ids[:, None, :, None] # [bs, 1, seq_len, 1]
+ gather_indices = gather_indices.repeat(1, cos.shape[1], 1, cos.shape[3])
+ cos = torch.gather(cos.repeat(gather_indices.shape[0], 1, 1, 1), 2, gather_indices)
+ sin = torch.gather(sin.repeat(gather_indices.shape[0], 1, 1, 1), 2, gather_indices)
+ q_embed = (q * cos) + (rotate_half(q) * sin)
+ k_embed = (k * cos) + (rotate_half(k) * sin)
+ return q_embed, k_embed
+
+
+class LlamaMLP(nn.Module):
+ def __init__(
+ self,
+ hidden_size: int,
+ intermediate_size: int,
+ hidden_act: str,
+ ):
+ super().__init__()
+ self.gate_proj = nn.Linear(hidden_size, intermediate_size, bias=False)
+ self.down_proj = nn.Linear(intermediate_size, hidden_size, bias=False)
+ self.up_proj = nn.Linear(hidden_size, intermediate_size, bias=False)
+ self.act_fn = ACT2FN[hidden_act]
+
+ def forward(self, x):
+ return self.down_proj(self.act_fn(self.gate_proj(x)) * self.up_proj(x))
+
+
+class LlamaAttention(nn.Module):
+ """Multi-headed attention from 'Attention Is All You Need' paper"""
+
+ def __init__(self, config: LlamaConfig):
+ super().__init__()
+ self.config = config
+ self.hidden_size = config.hidden_size
+ self.num_heads = config.num_attention_heads
+ self.head_dim = self.hidden_size // self.num_heads
+ self.max_position_embeddings = config.max_position_embeddings
+
+ if (self.head_dim * self.num_heads) != self.hidden_size:
+ raise ValueError(
+ f"hidden_size must be divisible by num_heads (got `hidden_size`: {self.hidden_size}"
+ f" and `num_heads`: {self.num_heads})."
+ )
+ self.q_proj = nn.Linear(self.hidden_size, self.num_heads * self.head_dim, bias=False)
+ self.k_proj = nn.Linear(self.hidden_size, self.num_heads * self.head_dim, bias=False)
+ self.v_proj = nn.Linear(self.hidden_size, self.num_heads * self.head_dim, bias=False)
+ self.o_proj = nn.Linear(self.num_heads * self.head_dim, self.hidden_size, bias=False)
+ self.rotary_emb = LlamaRotaryEmbedding(self.head_dim, max_position_embeddings=self.max_position_embeddings)
+
+ def _shape(self, tensor: torch.Tensor, seq_len: int, bsz: int):
+ return tensor.view(bsz, seq_len, self.num_heads, self.head_dim).transpose(1, 2).contiguous()
+
+ def forward(
+ self,
+ hidden_states: torch.Tensor,
+ attention_mask: Optional[torch.Tensor] = None,
+ position_ids: Optional[torch.LongTensor] = None,
+ past_key_value: Optional[Tuple[torch.Tensor]] = None,
+ output_attentions: bool = False,
+ use_cache: bool = False,
+ ) -> Tuple[torch.Tensor, Optional[torch.Tensor], Optional[Tuple[torch.Tensor]]]:
+ bsz, q_len, _ = hidden_states.size()
+
+ query_states = self.q_proj(hidden_states).view(bsz, q_len, self.num_heads, self.head_dim).transpose(1, 2)
+ key_states = self.k_proj(hidden_states).view(bsz, q_len, self.num_heads, self.head_dim).transpose(1, 2)
+ value_states = self.v_proj(hidden_states).view(bsz, q_len, self.num_heads, self.head_dim).transpose(1, 2)
+
+ kv_seq_len = key_states.shape[-2]
+ if past_key_value is not None:
+ kv_seq_len += past_key_value[0].shape[-2]
+ cos, sin = self.rotary_emb(value_states, seq_len=kv_seq_len)
+ query_states, key_states = apply_rotary_pos_emb(query_states, key_states, cos, sin, position_ids)
+ # [bsz, nh, t, hd]
+
+ if past_key_value is not None:
+ # reuse k, v, self_attention
+ key_states = torch.cat([past_key_value[0], key_states], dim=2)
+ value_states = torch.cat([past_key_value[1], value_states], dim=2)
+
+ past_key_value = (key_states, value_states) if use_cache else None
+
+ attn_weights = torch.matmul(query_states, key_states.transpose(2, 3)) / math.sqrt(self.head_dim)
+
+ if attn_weights.size() != (bsz, self.num_heads, q_len, kv_seq_len):
+ raise ValueError(
+ f"Attention weights should be of size {(bsz * self.num_heads, q_len, kv_seq_len)}, but is"
+ f" {attn_weights.size()}"
+ )
+
+ if attention_mask is not None:
+ if attention_mask.size() != (bsz, 1, q_len, kv_seq_len):
+ raise ValueError(
+ f"Attention mask should be of size {(bsz, 1, q_len, kv_seq_len)}, but is {attention_mask.size()}"
+ )
+ attn_weights = attn_weights + attention_mask
+ attn_weights = torch.max(attn_weights, torch.tensor(torch.finfo(attn_weights.dtype).min))
+
+ # upcast attention to fp32
+ attn_weights = nn.functional.softmax(attn_weights, dim=-1, dtype=torch.float32).to(query_states.dtype)
+ attn_output = torch.matmul(attn_weights, value_states)
+
+ if attn_output.size() != (bsz, self.num_heads, q_len, self.head_dim):
+ raise ValueError(
+ f"`attn_output` should be of size {(bsz, self.num_heads, q_len, self.head_dim)}, but is"
+ f" {attn_output.size()}"
+ )
+
+ attn_output = attn_output.transpose(1, 2)
+ attn_output = attn_output.reshape(bsz, q_len, self.hidden_size)
+
+ attn_output = self.o_proj(attn_output)
+
+ if not output_attentions:
+ attn_weights = None
+
+ return attn_output, attn_weights, past_key_value
+
+
+class LlamaDecoderLayer(nn.Module):
+ def __init__(self, config: LlamaConfig):
+ super().__init__()
+ self.hidden_size = config.hidden_size
+ self.self_attn = LlamaAttention(config=config)
+ self.mlp = LlamaMLP(
+ hidden_size=self.hidden_size,
+ intermediate_size=config.intermediate_size,
+ hidden_act=config.hidden_act,
+ )
+ self.input_layernorm = LlamaRMSNorm(config.hidden_size, eps=config.rms_norm_eps)
+ self.post_attention_layernorm = LlamaRMSNorm(config.hidden_size, eps=config.rms_norm_eps)
+
+ def forward(
+ self,
+ hidden_states: torch.Tensor,
+ attention_mask: Optional[torch.Tensor] = None,
+ position_ids: Optional[torch.LongTensor] = None,
+ past_key_value: Optional[Tuple[torch.Tensor]] = None,
+ output_attentions: Optional[bool] = False,
+ use_cache: Optional[bool] = False,
+ ) -> Tuple[torch.FloatTensor, Optional[Tuple[torch.FloatTensor, torch.FloatTensor]]]:
+ """
+ Args:
+ hidden_states (`torch.FloatTensor`): input to the layer of shape `(batch, seq_len, embed_dim)`
+ attention_mask (`torch.FloatTensor`, *optional*): attention mask of size
+ `(batch, 1, tgt_len, src_len)` where padding elements are indicated by very large negative values.
+ output_attentions (`bool`, *optional*):
+ Whether or not to return the attentions tensors of all attention layers. See `attentions` under
+ returned tensors for more detail.
+ use_cache (`bool`, *optional*):
+ If set to `True`, `past_key_values` key value states are returned and can be used to speed up decoding
+ (see `past_key_values`).
+ past_key_value (`Tuple(torch.FloatTensor)`, *optional*): cached past key and value projection states
+ """
+
+ residual = hidden_states
+
+ hidden_states = self.input_layernorm(hidden_states)
+
+ # Self Attention
+ hidden_states, self_attn_weights, present_key_value = self.self_attn(
+ hidden_states=hidden_states,
+ attention_mask=attention_mask,
+ position_ids=position_ids,
+ past_key_value=past_key_value,
+ output_attentions=output_attentions,
+ use_cache=use_cache,
+ )
+ hidden_states = residual + hidden_states
+
+ # Fully Connected
+ residual = hidden_states
+ hidden_states = self.post_attention_layernorm(hidden_states)
+ hidden_states = self.mlp(hidden_states)
+ hidden_states = residual + hidden_states
+
+ outputs = (hidden_states,)
+
+ if output_attentions:
+ outputs += (self_attn_weights,)
+
+ if use_cache:
+ outputs += (present_key_value,)
+
+ return outputs
+
+
+LLAMA_START_DOCSTRING = r"""
+ This model inherits from [`PreTrainedModel`]. Check the superclass documentation for the generic methods the
+ library implements for all its model (such as downloading or saving, resizing the input embeddings, pruning heads
+ etc.)
+
+ This model is also a PyTorch [torch.nn.Module](https://pytorch.org/docs/stable/nn.html#torch.nn.Module) subclass.
+ Use it as a regular PyTorch Module and refer to the PyTorch documentation for all matter related to general usage
+ and behavior.
+
+ Parameters:
+ config ([`LlamaConfig`]):
+ Model configuration class with all the parameters of the model. Initializing with a config file does not
+ load the weights associated with the model, only the configuration. Check out the
+ [`~PreTrainedModel.from_pretrained`] method to load the model weights.
+"""
+
+
+@add_start_docstrings(
+ "The bare LLaMA Model outputting raw hidden-states without any specific head on top.",
+ LLAMA_START_DOCSTRING,
+)
+class LlamaPreTrainedModel(PreTrainedModel):
+ config_class = LlamaConfig
+ base_model_prefix = "model"
+ supports_gradient_checkpointing = True
+ _no_split_modules = ["LlamaDecoderLayer"]
+ _keys_to_ignore_on_load_unexpected = [r"decoder\.version"]
+
+ def _init_weights(self, module):
+ std = self.config.initializer_range
+ if isinstance(module, nn.Linear):
+ module.weight.data.normal_(mean=0.0, std=std)
+ if module.bias is not None:
+ module.bias.data.zero_()
+ elif isinstance(module, nn.Embedding):
+ module.weight.data.normal_(mean=0.0, std=std)
+ if module.padding_idx is not None:
+ module.weight.data[module.padding_idx].zero_()
+
+ def _set_gradient_checkpointing(self, module, value=False):
+ if isinstance(module, LlamaModel):
+ module.gradient_checkpointing = value
+
+
+LLAMA_INPUTS_DOCSTRING = r"""
+ Args:
+ input_ids (`torch.LongTensor` of shape `(batch_size, sequence_length)`):
+ Indices of input sequence tokens in the vocabulary. Padding will be ignored by default should you provide
+ it.
+
+ Indices can be obtained using [`AutoTokenizer`]. See [`PreTrainedTokenizer.encode`] and
+ [`PreTrainedTokenizer.__call__`] for details.
+
+ [What are input IDs?](../glossary#input-ids)
+ attention_mask (`torch.Tensor` of shape `(batch_size, sequence_length)`, *optional*):
+ Mask to avoid performing attention on padding token indices. Mask values selected in `[0, 1]`:
+
+ - 1 for tokens that are **not masked**,
+ - 0 for tokens that are **masked**.
+
+ [What are attention masks?](../glossary#attention-mask)
+
+ Indices can be obtained using [`AutoTokenizer`]. See [`PreTrainedTokenizer.encode`] and
+ [`PreTrainedTokenizer.__call__`] for details.
+
+ If `past_key_values` is used, optionally only the last `decoder_input_ids` have to be input (see
+ `past_key_values`).
+
+ If you want to change padding behavior, you should read [`modeling_opt._prepare_decoder_attention_mask`]
+ and modify to your needs. See diagram 1 in [the paper](https://arxiv.org/abs/1910.13461) for more
+ information on the default strategy.
+
+ - 1 indicates the head is **not masked**,
+ - 0 indicates the head is **masked**.
+ position_ids (`torch.LongTensor` of shape `(batch_size, sequence_length)`, *optional*):
+ Indices of positions of each input sequence tokens in the position embeddings. Selected in the range `[0,
+ config.n_positions - 1]`.
+
+ [What are position IDs?](../glossary#position-ids)
+ past_key_values (`tuple(tuple(torch.FloatTensor))`, *optional*, returned when `use_cache=True` is passed or when `config.use_cache=True`):
+ Tuple of `tuple(torch.FloatTensor)` of length `config.n_layers`, with each tuple having 2 tensors of shape
+ `(batch_size, num_heads, sequence_length, embed_size_per_head)`) and 2 additional tensors of shape
+ `(batch_size, num_heads, encoder_sequence_length, embed_size_per_head)`.
+
+ Contains pre-computed hidden-states (key and values in the self-attention blocks and in the cross-attention
+ blocks) that can be used (see `past_key_values` input) to speed up sequential decoding.
+
+ If `past_key_values` are used, the user can optionally input only the last `decoder_input_ids` (those that
+ don't have their past key value states given to this model) of shape `(batch_size, 1)` instead of all
+ `decoder_input_ids` of shape `(batch_size, sequence_length)`.
+ inputs_embeds (`torch.FloatTensor` of shape `(batch_size, sequence_length, hidden_size)`, *optional*):
+ Optionally, instead of passing `input_ids` you can choose to directly pass an embedded representation. This
+ is useful if you want more control over how to convert `input_ids` indices into associated vectors than the
+ model's internal embedding lookup matrix.
+ use_cache (`bool`, *optional*):
+ If set to `True`, `past_key_values` key value states are returned and can be used to speed up decoding (see
+ `past_key_values`).
+ output_attentions (`bool`, *optional*):
+ Whether or not to return the attentions tensors of all attention layers. See `attentions` under returned
+ tensors for more detail.
+ output_hidden_states (`bool`, *optional*):
+ Whether or not to return the hidden states of all layers. See `hidden_states` under returned tensors for
+ more detail.
+ return_dict (`bool`, *optional*):
+ Whether or not to return a [`~utils.ModelOutput`] instead of a plain tuple.
+"""
+
+
+@add_start_docstrings(
+ "The bare LLaMA Model outputting raw hidden-states without any specific head on top.",
+ LLAMA_START_DOCSTRING,
+)
+class LlamaModel(LlamaPreTrainedModel):
+ """
+ Transformer decoder consisting of *config.num_hidden_layers* layers. Each layer is a [`LlamaDecoderLayer`]
+
+ Args:
+ config: LlamaConfig
+ """
+
+ def __init__(self, config: LlamaConfig):
+ super().__init__(config)
+ self.padding_idx = config.pad_token_id
+ self.vocab_size = config.vocab_size
+
+ self.embed_tokens = nn.Embedding(config.vocab_size, config.hidden_size, self.padding_idx)
+ self.layers = nn.ModuleList([LlamaDecoderLayer(config) for _ in range(config.num_hidden_layers)])
+ self.norm = LlamaRMSNorm(config.hidden_size, eps=config.rms_norm_eps)
+
+ self.gradient_checkpointing = False
+ # Initialize weights and apply final processing
+ self.post_init()
+
+ def get_input_embeddings(self):
+ return self.embed_tokens
+
+ def set_input_embeddings(self, value):
+ self.embed_tokens = value
+
+ # Copied from transformers.models.bart.modeling_bart.BartDecoder._prepare_decoder_attention_mask
+ def _prepare_decoder_attention_mask(self, attention_mask, input_shape, inputs_embeds, past_key_values_length):
+ # create causal mask
+ # [bsz, seq_len] -> [bsz, 1, tgt_seq_len, src_seq_len]
+ combined_attention_mask = None
+ if input_shape[-1] > 1:
+ combined_attention_mask = _make_causal_mask(
+ input_shape,
+ inputs_embeds.dtype,
+ device=inputs_embeds.device,
+ past_key_values_length=past_key_values_length,
+ )
+
+ if attention_mask is not None:
+ # [bsz, seq_len] -> [bsz, 1, tgt_seq_len, src_seq_len]
+ expanded_attn_mask = _expand_mask(attention_mask, inputs_embeds.dtype, tgt_len=input_shape[-1]).to(
+ inputs_embeds.device
+ )
+ combined_attention_mask = (
+ expanded_attn_mask if combined_attention_mask is None else expanded_attn_mask + combined_attention_mask
+ )
+
+ return combined_attention_mask
+
+ @add_start_docstrings_to_model_forward(LLAMA_INPUTS_DOCSTRING)
+ def forward(
+ self,
+ input_ids: torch.LongTensor = None,
+ attention_mask: Optional[torch.Tensor] = None,
+ position_ids: Optional[torch.LongTensor] = None,
+ past_key_values: Optional[List[torch.FloatTensor]] = None,
+ inputs_embeds: Optional[torch.FloatTensor] = None,
+ query_embeds: Optional[torch.FloatTensor] = None,
+ use_cache: Optional[bool] = None,
+ output_attentions: Optional[bool] = None,
+ output_hidden_states: Optional[bool] = None,
+ return_dict: Optional[bool] = None,
+ ) -> Union[Tuple, BaseModelOutputWithPast]:
+ output_attentions = output_attentions if output_attentions is not None else self.config.output_attentions
+ output_hidden_states = (
+ output_hidden_states if output_hidden_states is not None else self.config.output_hidden_states
+ )
+ use_cache = use_cache if use_cache is not None else self.config.use_cache
+
+ return_dict = return_dict if return_dict is not None else self.config.use_return_dict
+
+ # retrieve input_ids and inputs_embeds
+ if input_ids is not None and inputs_embeds is not None:
+ raise ValueError("You cannot specify both decoder_input_ids and decoder_inputs_embeds at the same time")
+ elif input_ids is not None:
+ batch_size, seq_length = input_ids.shape
+ elif inputs_embeds is not None:
+ batch_size, seq_length, _ = inputs_embeds.shape
+ else:
+ raise ValueError("You have to specify either decoder_input_ids or decoder_inputs_embeds")
+
+ if inputs_embeds is None:
+ inputs_embeds = self.embed_tokens(input_ids)
+ if query_embeds is not None:
+ inputs_embeds = torch.cat([query_embeds, inputs_embeds], dim=1)
+ batch_size, seq_length, _ = inputs_embeds.shape
+
+ seq_length_with_past = seq_length
+ past_key_values_length = 0
+
+ if past_key_values is not None:
+ past_key_values_length = past_key_values[0][0].shape[2]
+ seq_length_with_past = seq_length_with_past + past_key_values_length
+
+ if position_ids is None:
+ device = input_ids.device if input_ids is not None else inputs_embeds.device
+ position_ids = torch.arange(
+ past_key_values_length, seq_length + past_key_values_length, dtype=torch.long, device=device
+ )
+ position_ids = position_ids.unsqueeze(0).view(-1, seq_length)
+ else:
+ position_ids = position_ids.view(-1, seq_length).long()
+
+ # embed positions
+ if attention_mask is None:
+ attention_mask = torch.ones(
+ (batch_size, seq_length_with_past), dtype=torch.bool, device=inputs_embeds.device
+ )
+ attention_mask = self._prepare_decoder_attention_mask(
+ attention_mask, (batch_size, seq_length), inputs_embeds, past_key_values_length
+ )
+
+ hidden_states = inputs_embeds
+
+ if self.gradient_checkpointing and self.training:
+ if use_cache:
+ logger.warning_once(
+ "`use_cache=True` is incompatible with gradient checkpointing. Setting `use_cache=False`..."
+ )
+ use_cache = False
+
+ # decoder layers
+ all_hidden_states = () if output_hidden_states else None
+ all_self_attns = () if output_attentions else None
+ next_decoder_cache = () if use_cache else None
+
+ for idx, decoder_layer in enumerate(self.layers):
+ if output_hidden_states:
+ all_hidden_states += (hidden_states,)
+
+ past_key_value = past_key_values[idx] if past_key_values is not None else None
+
+ if self.gradient_checkpointing and self.training:
+
+ def create_custom_forward(module):
+ def custom_forward(*inputs):
+ # None for past_key_value
+ return module(*inputs, output_attentions, None)
+
+ return custom_forward
+
+ layer_outputs = torch.utils.checkpoint.checkpoint(
+ create_custom_forward(decoder_layer),
+ hidden_states,
+ attention_mask,
+ position_ids,
+ None,
+ )
+ else:
+ layer_outputs = decoder_layer(
+ hidden_states,
+ attention_mask=attention_mask,
+ position_ids=position_ids,
+ past_key_value=past_key_value,
+ output_attentions=output_attentions,
+ use_cache=use_cache,
+ )
+
+ hidden_states = layer_outputs[0]
+
+ if use_cache:
+ next_decoder_cache += (layer_outputs[2 if output_attentions else 1],)
+
+ if output_attentions:
+ all_self_attns += (layer_outputs[1],)
+
+ hidden_states = self.norm(hidden_states)
+
+ # add hidden states from the last decoder layer
+ if output_hidden_states:
+ all_hidden_states += (hidden_states,)
+
+ next_cache = next_decoder_cache if use_cache else None
+ if not return_dict:
+ return tuple(v for v in [hidden_states, next_cache, all_hidden_states, all_self_attns] if v is not None)
+ return BaseModelOutputWithPast(
+ last_hidden_state=hidden_states,
+ past_key_values=next_cache,
+ hidden_states=all_hidden_states,
+ attentions=all_self_attns,
+ )
+
+
+class LlamaForCausalLM(LlamaPreTrainedModel):
+ def __init__(self, config):
+ super().__init__(config)
+ self.model = LlamaModel(config)
+
+ self.lm_head = nn.Linear(config.hidden_size, config.vocab_size, bias=False)
+
+ # Initialize weights and apply final processing
+ self.post_init()
+
+ def get_input_embeddings(self):
+ return self.model.embed_tokens
+
+ def set_input_embeddings(self, value):
+ self.model.embed_tokens = value
+
+ def get_output_embeddings(self):
+ return self.lm_head
+
+ def set_output_embeddings(self, new_embeddings):
+ self.lm_head = new_embeddings
+
+ def set_decoder(self, decoder):
+ self.model = decoder
+
+ def get_decoder(self):
+ return self.model
+
+ @add_start_docstrings_to_model_forward(LLAMA_INPUTS_DOCSTRING)
+ @replace_return_docstrings(output_type=CausalLMOutputWithPast, config_class=_CONFIG_FOR_DOC)
+ def forward(
+ self,
+ input_ids: torch.LongTensor = None,
+ attention_mask: Optional[torch.Tensor] = None,
+ position_ids: Optional[torch.LongTensor] = None,
+ past_key_values: Optional[List[torch.FloatTensor]] = None,
+ inputs_embeds: Optional[torch.FloatTensor] = None,
+ query_embeds: Optional[torch.FloatTensor] = None,
+ labels: Optional[torch.LongTensor] = None,
+ use_cache: Optional[bool] = None,
+ output_attentions: Optional[bool] = None,
+ output_hidden_states: Optional[bool] = None,
+ return_dict: Optional[bool] = None,
+ value_forward: Optional[bool] = False,
+ no_average: Optional[bool] = False,
+ ) -> Union[Tuple, CausalLMOutputWithPast]:
+ r"""
+ Args:
+ labels (`torch.LongTensor` of shape `(batch_size, sequence_length)`, *optional*):
+ Labels for computing the masked language modeling loss. Indices should either be in `[0, ...,
+ config.vocab_size]` or -100 (see `input_ids` docstring). Tokens with indices set to `-100` are ignored
+ (masked), the loss is only computed for the tokens with labels in `[0, ..., config.vocab_size]`.
+
+ Returns:
+
+ Example:
+
+ ```python
+ >>> from transformers import AutoTokenizer, LlamaForCausalLM
+
+ >>> model = LlamaForCausalLM.from_pretrained(PATH_TO_CONVERTED_WEIGHTS)
+ >>> tokenizer = AutoTokenizer.from_pretrained(PATH_TO_CONVERTED_TOKENIZER)
+
+ >>> prompt = "Hey, are you consciours? Can you talk to me?"
+ >>> inputs = tokenizer(prompt, return_tensors="pt")
+
+ >>> # Generate
+ >>> generate_ids = model.generate(inputs.input_ids, max_length=30)
+ >>> tokenizer.batch_decode(generate_ids, skip_special_tokens=True, clean_up_tokenization_spaces=False)[0]
+ "Hey, are you consciours? Can you talk to me?\nI'm not consciours, but I can talk to you."
+ ```"""
+
+ output_attentions = output_attentions if output_attentions is not None else self.config.output_attentions
+ output_hidden_states = (
+ output_hidden_states if output_hidden_states is not None else self.config.output_hidden_states
+ )
+ return_dict = return_dict if return_dict is not None else self.config.use_return_dict
+
+ # decoder outputs consists of (dec_features, layer_state, dec_hidden, dec_attn)
+ outputs = self.model(
+ input_ids=input_ids,
+ attention_mask=attention_mask,
+ position_ids=position_ids,
+ past_key_values=past_key_values,
+ inputs_embeds=inputs_embeds,
+ query_embeds=query_embeds,
+ use_cache=use_cache,
+ output_attentions=output_attentions,
+ output_hidden_states=output_hidden_states,
+ return_dict=return_dict,
+ )
+
+ hidden_states = outputs[0]
+
+ if value_forward:
+ return hidden_states
+
+ logits = self.lm_head(hidden_states)
+
+ loss = None
+ if labels is not None:
+ # Shift so that tokens < n predict n
+ shift_logits = logits[..., :-1, :].contiguous()
+ shift_labels = labels[..., 1:].contiguous()
+ # Flatten the tokens
+ if no_average:
+ loss_fct = CrossEntropyLoss(reduction='none')
+ else:
+ loss_fct = CrossEntropyLoss()
+ shift_logits = shift_logits.view(-1, self.config.vocab_size)
+ shift_labels = shift_labels.view(-1)
+ # Enable model parallelism
+ shift_labels = shift_labels.to(shift_logits.device)
+ loss = loss_fct(shift_logits, shift_labels)
+
+ if no_average:
+ loss = loss.view(logits[..., :-1, :].size(0), logits[..., :-1, :].size(1))
+
+ if not return_dict:
+ output = (logits,) + outputs[1:]
+ return (loss,) + output if loss is not None else output
+
+ return CausalLMOutputWithPast(
+ loss=loss,
+ logits=logits,
+ past_key_values=outputs.past_key_values,
+ hidden_states=outputs.hidden_states,
+ attentions=outputs.attentions,
+ )
+
+ def prepare_inputs_for_generation(
+ self, input_ids, query_embeds=None, past_key_values=None, attention_mask=None, inputs_embeds=None, **kwargs
+ ):
+ if past_key_values:
+ input_ids = input_ids[:, -1:]
+
+ position_ids = kwargs.get("position_ids", None)
+ if attention_mask is not None and position_ids is None:
+ # create position_ids on the fly for batch generation
+ position_ids = attention_mask.long().cumsum(-1) - 1
+ position_ids.masked_fill_(attention_mask == 0, 1)
+ if past_key_values:
+ position_ids = position_ids[:, -1].unsqueeze(-1)
+ query_embeds = None
+
+ # if `inputs_embeds` are passed, we only want to use them in the 1st generation step
+ if inputs_embeds is not None and past_key_values is None:
+ model_inputs = {"inputs_embeds": inputs_embeds}
+ else:
+ model_inputs = {"input_ids": input_ids}
+
+ model_inputs.update(
+ {
+ "position_ids": position_ids,
+ "query_embeds": query_embeds,
+ "past_key_values": past_key_values,
+ "use_cache": kwargs.get("use_cache"),
+ "attention_mask": attention_mask,
+ }
+ )
+ return model_inputs
+
+ @staticmethod
+ def _reorder_cache(past_key_values, beam_idx):
+ reordered_past = ()
+ for layer_past in past_key_values:
+ reordered_past += (tuple(past_state.index_select(0, beam_idx) for past_state in layer_past),)
+ return reordered_past
+
diff --git a/global_local/models/video_instruction_ft_model.py b/global_local/models/video_instruction_ft_model.py
new file mode 100644
index 0000000000000000000000000000000000000000..e2e43e27658cb6ee12c5b1994448564848fdd13c
--- /dev/null
+++ b/global_local/models/video_instruction_ft_model.py
@@ -0,0 +1,1395 @@
+import logging
+import random
+import sys
+
+import torch
+from torch.cuda.amp import autocast as autocast
+import torch.nn as nn
+import torch.nn.functional as F
+
+from global_local.common.registry import registry
+from global_local.models.blip2 import Blip2Base, disabled_train
+from global_local.models.modeling_llama import LlamaForCausalLM
+from transformers import LlamaTokenizer,BertConfig
+import einops
+import copy
+from global_local.models.Qformer import BertConfig, BertLMHeadModel
+from global_local.models.ImageBind.models.imagebind_model import ImageBindModel,ModalityType
+from global_local.models.ImageBind.models import imagebind_model
+# from flamingo_pytorch import PerceiverResampler
+@registry.register_model("video_instruction_llama")
+class VideoInstructionFTLLAMA(Blip2Base):
+ """
+ BLIP2 GPT-LLAMA model.
+ """
+
+ PRETRAINED_MODEL_CONFIG_DICT = {
+ "pretrain_vicuna": "configs/models/video_llama.yaml",
+ "pretrain_llama_v2": "configs/models/video_llama.yaml",
+ }
+
+ @classmethod
+ def init_video_Qformer(cls, num_query_token, vision_width,num_hidden_layers =2):
+ encoder_config = BertConfig.from_pretrained("bert-base-uncased")
+ encoder_config.num_hidden_layers = num_hidden_layers
+ encoder_config.encoder_width = vision_width
+ # insert cross-attention layer every other block
+ encoder_config.add_cross_attention = True
+ encoder_config.cross_attention_freq = 1
+ encoder_config.query_length = num_query_token
+ Qformer = BertLMHeadModel(config=encoder_config)
+ query_tokens = nn.Parameter(
+ torch.zeros(1, num_query_token, encoder_config.hidden_size)
+ )
+ query_tokens.data.normal_(mean=0.0, std=encoder_config.initializer_range)
+ return Qformer, query_tokens
+
+ def __init__(
+ self,
+ vit_model="eva_clip_g",
+ q_former_model="https://storage.googleapis.com/sfr-vision-language-research/LAVIS/models/BLIP2/blip2_pretrained_flant5xxl.pth",
+ img_size=224,
+ drop_path_rate=0,
+ use_grad_checkpoint=False,
+ vit_precision="fp16",
+ freeze_vit=True,
+ freeze_qformer=True,
+ num_query_token=32,
+ llama_model="",
+ prompt_path="",
+ prompt_template="",
+ max_txt_len=128,
+ end_sym='\n',
+ low_resource=False, # use 8 bit and put vit in cpu
+ device_8bit=0, # the device of 8bit model should be set when loading and cannot be changed anymore.
+
+ frozen_llama_proj=True,
+ frozen_video_Qformer=True,
+ frozen_audio_Qformer=True,
+
+ llama_proj_model='',
+ fusion_header_type= "seqTransf",
+ max_frame_pos= 32,
+ fusion_head_layers = 2,
+ num_video_query_token = 32,
+ num_audio_query_token = 8,
+ imagebind_ckpt_path = '/mnt/workspace/ckpt',
+ equip_audio_branch = True
+ ):
+ super().__init__()
+
+ self.tokenizer = self.init_tokenizer()
+ self.low_resource = low_resource
+
+ print('Loading VIT')
+ self.visual_encoder, self.ln_vision = self.init_vision_encoder(
+ vit_model, img_size, drop_path_rate, use_grad_checkpoint, vit_precision
+ )
+ if freeze_vit:
+ for name, param in self.visual_encoder.named_parameters():
+ param.requires_grad = False
+ self.visual_encoder = self.visual_encoder.eval()
+ self.visual_encoder.train = disabled_train
+ for name, param in self.ln_vision.named_parameters():
+ param.requires_grad = False
+ self.ln_vision = self.ln_vision.eval()
+ self.ln_vision.train = disabled_train
+ logging.info("freeze vision encoder")
+ print('Loading VIT Done')
+
+ print('Loading Q-Former')
+ self.Qformer, self.query_tokens = self.init_Qformer(
+ num_query_token, self.visual_encoder.num_features
+ )
+ self.Qformer.cls = None
+ self.Qformer.bert.embeddings.word_embeddings = None
+ self.Qformer.bert.embeddings.position_embeddings = None
+ for layer in self.Qformer.bert.encoder.layer:
+ layer.output = None
+ layer.intermediate = None
+ self.load_from_pretrained(url_or_filename=q_former_model)
+
+ if freeze_qformer:
+ for name, param in self.Qformer.named_parameters():
+ param.requires_grad = False
+ self.Qformer = self.Qformer.eval()
+ self.Qformer.train = disabled_train
+ self.query_tokens.requires_grad = False
+ logging.info("freeze Qformer")
+ logging.info('Loading Q-Former Done')
+
+ logging.info('Loading LLAMA Tokenizer')
+ self.llama_tokenizer = LlamaTokenizer.from_pretrained(llama_model, use_fast=False)
+ if self.llama_tokenizer.pad_token is None:
+ self.llama_tokenizer.pad_token = self.llama_tokenizer.unk_token
+ DEFAULT_IMAGE_PATCH_TOKEN = ''
+ DEFAULT_AUDIO_PATCH_TOKEN = ''
+ self.llama_tokenizer.add_tokens([DEFAULT_IMAGE_PATCH_TOKEN], special_tokens=True)
+ self.llama_tokenizer.add_tokens([DEFAULT_AUDIO_PATCH_TOKEN], special_tokens=True)
+
+ self.IMAGE_PATCH_TOKEN_ID = self.llama_tokenizer.get_vocab()[DEFAULT_IMAGE_PATCH_TOKEN]
+ self.AUDIO_PATCH_TOKEN_ID = self.llama_tokenizer.get_vocab()[DEFAULT_AUDIO_PATCH_TOKEN]
+
+ logging.info('Loading LLAMA Model')
+ if self.low_resource:
+ self.llama_model = LlamaForCausalLM.from_pretrained(
+ llama_model,
+ torch_dtype=torch.bfloat16,
+ load_in_8bit=True,
+ device_map={'': device_8bit}
+ )
+ else:
+ self.llama_model = LlamaForCausalLM.from_pretrained(
+ llama_model,
+ torch_dtype=torch.bfloat16,
+ )
+
+ for name, param in self.llama_model.named_parameters():
+ param.requires_grad = False
+ logging.info('Loading LLAMA Done')
+
+
+ logging.info('Loading LLAMA proj')
+ self.llama_proj = nn.Linear(
+ self.Qformer.config.hidden_size, self.llama_model.config.hidden_size
+ )
+ if llama_proj_model:
+ print("load llama proj weight: {}".format(llama_proj_model))
+ llama_proj_weight = torch.load(llama_proj_model, map_location="cpu")
+ msg = self.load_state_dict(llama_proj_weight['model'], strict=False)
+
+ if frozen_llama_proj:
+ # todo frozen llama_proj
+ for name, param in self.llama_proj.named_parameters():
+ param.requires_grad = False
+ logging.info('LLAMA proj is frozen')
+ else:
+ for name, param in self.llama_proj.named_parameters():
+ param.requires_grad = True
+ logging.info('LLAMA proj is not frozen')
+
+ logging.info('Loading llama_proj Done')
+
+ self.max_txt_len = max_txt_len
+ self.end_sym = end_sym
+
+ if prompt_path:
+ with open(prompt_path, 'r') as f:
+ raw_prompts = f.read().splitlines()
+ filted_prompts = [raw_prompt for raw_prompt in raw_prompts if "" in raw_prompt]
+ self.prompt_list = [prompt_template.format(p) for p in filted_prompts]
+ print('Load {} training prompts'.format(len(self.prompt_list)))
+ print('Prompt Example \n{}'.format(random.choice(self.prompt_list)))
+ else:
+ self.prompt_list = []
+
+ self.video_frame_position_embedding = nn.Embedding(max_frame_pos, self.Qformer.config.hidden_size)
+
+ self.num_video_query_token = num_video_query_token
+ self.video_Qformer,self.video_query_tokens = self.init_video_Qformer(num_query_token = num_video_query_token,\
+ vision_width=self.Qformer.config.hidden_size, num_hidden_layers =2)
+
+ self.video_Qformer.cls = None
+ self.video_Qformer.bert.embeddings.word_embeddings = None
+ self.video_Qformer.bert.embeddings.position_embeddings = None
+ for layer in self.video_Qformer.bert.encoder.layer:
+ layer.output = None
+ layer.intermediate = None
+
+
+ if frozen_video_Qformer:
+ # todo frozen llama_proj
+ for name, param in self.video_Qformer.named_parameters():
+ param.requires_grad = False
+ for name, param in self.video_frame_position_embedding.named_parameters():
+ param.requires_grad = False
+ self.video_query_tokens.requires_grad = False
+
+ logging.info('video_Qformer is frozen')
+ else:
+ for name, param in self.video_Qformer.named_parameters():
+ param.requires_grad = True
+ for name, param in self.video_frame_position_embedding.named_parameters():
+ param.requires_grad = True
+ self.video_query_tokens.requires_grad = True
+ logging.info('video_Qformer is not frozen')
+
+ if frozen_video_Qformer and (not frozen_audio_Qformer):
+ self.train_flag = 1 # 只训练audio_Qformer
+ elif not(frozen_video_Qformer) and frozen_audio_Qformer:
+ self.train_flag = 0 # 训练video_Qformer
+ elif not(frozen_video_Qformer) and not(frozen_audio_Qformer):
+ self.train_flag = 2 # video_Qformer and AL trained
+ else:
+ self.train_flag = 3
+
+ if equip_audio_branch:
+ print (f'Initializing audio encoder from {imagebind_ckpt_path} ...')
+ self.audio_encoder,self.audio_hidden_size = \
+ imagebind_model.imagebind_huge()
+ self.audio_encoder.load_state_dict(torch.load("{}/imagebind_huge.pth".format(imagebind_ckpt_path)))
+ # free vision encoder
+ for name, param in self.audio_encoder.named_parameters():
+ param.requires_grad = False
+ self.audio_encoder.eval()
+ print ('audio encoder initialized.')
+
+ self.num_audio_query_token = num_audio_query_token
+ self.audio_Qformer,self.audio_query_tokens = self.init_video_Qformer(num_query_token = self.num_audio_query_token,\
+ vision_width=self.audio_hidden_size, num_hidden_layers =2)
+ self.audio_Qformer.cls = None
+ self.audio_Qformer.bert.embeddings.word_embeddings = None
+ self.audio_Qformer.bert.embeddings.position_embeddings = None
+ for layer in self.audio_Qformer.bert.encoder.layer:
+ layer.output = None
+ layer.intermediate = None
+ self.audio_llama_proj = nn.Linear(
+ self.audio_Qformer.config.hidden_size, self.llama_model.config.hidden_size
+ )
+ self.audio_position_embedding = nn.Embedding(8, self.audio_hidden_size)
+
+ if frozen_audio_Qformer:
+ # todo frozen llama_proj
+ for name, param in self.audio_Qformer.named_parameters():
+ param.requires_grad = False
+ self.audio_query_tokens.requires_grad = False
+ for name, param in self.audio_llama_proj.named_parameters():
+ param.requires_grad = False
+ for name, param in self.audio_position_embedding.named_parameters():
+ param.requires_grad = False
+ logging.info('audio_Qformer and audio-LLAMA proj is frozen')
+ else:
+ for name, param in self.audio_Qformer.named_parameters():
+ param.requires_grad = True
+ self.audio_query_tokens.requires_grad = True
+ for name, param in self.audio_llama_proj.named_parameters():
+ param.requires_grad = True
+ for name, param in self.audio_position_embedding.named_parameters():
+ param.requires_grad = True
+ logging.info('audio_Qformer is not frozen')
+
+ # initialize additional arguments
+ self.pos_extending_factor = None
+
+ self.prompt = '[INST] %s [/INST]'
+
+ def vit_to_cpu(self):
+ self.ln_vision.to("cpu")
+ self.ln_vision.float()
+ self.visual_encoder.to("cpu")
+ self.visual_encoder.float()
+
+ def initialize_visual_agg_function(self):
+ if self.hierarchical_agg_function == 'without-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned':
+ self.video_global_proj = nn.Linear(self.Qformer.config.hidden_size, self.llama_model.config.hidden_size)
+ self.video_global_proj.load_state_dict(self.llama_proj.state_dict())
+
+ for name, param in self.video_global_proj.named_parameters():
+ param.requires_grad = True
+
+ if 'without-top' not in self.hierarchical_agg_function:
+ self.global_region_prompts = nn.Parameter(torch.zeros(1, self.video_query_tokens.size(1), self.video_query_tokens.size(-1)))
+ self.global_region_prompts.data = self.video_query_tokens.data.clone()
+ self.global_region_prompts.requires_grad = True
+
+ self.segment_region_prompts = nn.Parameter(torch.zeros(1, self.video_query_tokens.size(1), self.video_query_tokens.size(-1)))
+ self.segment_region_prompts.data = self.video_query_tokens.data.clone()
+ self.segment_region_prompts.requires_grad = True
+
+ if 'region-prompts' in self.hierarchical_agg_function:
+ self.segment_attn_queries = nn.Parameter(torch.zeros(1, self.num_segments, self.video_query_tokens.size(-1)))
+ self.segment_attn_queries.data = self.video_query_tokens.data[:, :self.num_segments].clone()
+ self.segment_attn_queries.requires_grad = True
+
+ if 'spatiotemporal-prompts' in self.hierarchical_agg_function:
+ if 'full-dis-spatiotemporal' in self.hierarchical_agg_function:
+ self.spatial_segment_prompts = nn.Parameter(torch.zeros(1, self.video_query_tokens.size(1), self.video_query_tokens.size(-1)))
+ self.spatial_segment_prompts.data = self.video_query_tokens.data.clone()
+ self.spatial_segment_prompts.requires_grad = True
+
+ self.temporal_segment_prompts = nn.Parameter(torch.zeros(1, self.num_segments, self.video_query_tokens.size(-1)))
+ self.temporal_segment_prompts.data = self.video_query_tokens.data.clone().mean(1, keepdim=True).repeat(1, self.num_segments, 1)
+ self.temporal_segment_prompts.requires_grad = True
+
+ elif 'full-spatiotemporal' not in self.hierarchical_agg_function:
+ self.temporal_segment_prompts = nn.Parameter(torch.zeros(1, self.num_segments, self.video_query_tokens.size(-1)))
+ self.temporal_segment_prompts.data = self.video_query_tokens.data[:, :self.num_segments].clone()
+ self.temporal_segment_prompts.requires_grad = True
+
+ self.spatial_segment_prompts = nn.Parameter(torch.zeros(1, self.video_query_tokens.size(1), self.video_query_tokens.size(-1)))
+ self.spatial_segment_prompts.data = self.video_query_tokens.data.clone()
+ self.spatial_segment_prompts.requires_grad = True
+ else:
+ self.spatial_segment_prompts = nn.Parameter(torch.zeros(self.num_segments, self.video_query_tokens.size(1), self.video_query_tokens.size(-1)))
+ self.spatial_segment_prompts.data = self.video_query_tokens.data.clone().repeat(self.num_segments, 1, 1)
+ self.spatial_segment_prompts.requires_grad = True
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ self.global_to_segment_prompts = nn.Parameter(torch.zeros(1, self.video_query_tokens.size(1), self.video_query_tokens.size(-1)))
+ self.global_to_segment_prompts.data = self.video_query_tokens.data.clone()
+ self.global_to_segment_prompts.requires_grad = True
+
+ if 'proj-' in self.hierarchical_agg_function:
+ self.global_frame_proj = nn.Linear(self.Qformer.config.hidden_size, self.Qformer.config.hidden_size)
+ self.global_segment_proj = nn.Linear(self.Qformer.config.hidden_size, self.Qformer.config.hidden_size)
+
+ if '-learned' in self.hierarchical_agg_function:
+ if self.global_region_embed_weight is None:
+ self.global_region_embed_weight = nn.Parameter(data=torch.rand(1))
+ else:
+ self.global_region_embed_weight = nn.Parameter(data=torch.Tensor([self.global_region_embed_weight]))
+ self.global_region_embed_weight.requires_grad = True
+
+ for k, v in self.named_parameters():
+ if 'video_global_proj' not in k:
+ v.requires_grad = False
+
+ return
+
+ def encode_videoQformer_visual(self, image, frame_attn_mask, global_video=True):
+ device = image.device
+
+ # input shape b,t,c,h,w
+ batch_size, time_length, _, _, _ = image.size()
+
+ image = einops.rearrange(image, 'b t c h w -> (b t) c h w')
+
+ with self.maybe_autocast():
+ # embed image features with blip2, out: (b t) q h
+ image_embeds = self.ln_vision(self.visual_encoder(image)).to(device)
+ image_atts = torch.ones(image_embeds.size()[:-1], dtype=torch.long).to(device)
+
+ query_tokens = self.query_tokens.expand(image_embeds.shape[0], -1, -1)
+
+ query_output = self.Qformer.bert(
+ query_embeds=query_tokens,
+ encoder_hidden_states=image_embeds,
+ encoder_attention_mask=image_atts,
+ return_dict=True,
+ )
+
+ # add frame_pos embedding
+ position_ids = torch.arange(time_length, dtype=torch.long, device=query_tokens.device)
+ position_ids = position_ids.unsqueeze(0).expand(batch_size, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ q_hidden_state = query_output.last_hidden_state
+
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ frame_hidden_state = einops.rearrange(q_hidden_state, '(b t) q h -> b t q h',b=batch_size,t=time_length)
+ frame_hidden_state = frame_position_embeddings + frame_hidden_state
+
+ # frame attention
+ frame_hidden_state = einops.rearrange(frame_hidden_state, 'b t q h -> b (t q) h',b=batch_size,t=time_length)
+ frame_atts = torch.ones(frame_hidden_state.size()[:-1], dtype=torch.long).to(device)
+ video_query_tokens = self.video_query_tokens.expand(frame_hidden_state.shape[0], -1, -1)
+
+ frame_attn_mask = frame_attn_mask.unsqueeze(-1).repeat(1, 1, q_hidden_state.size(1))
+ frame_attn_mask = frame_attn_mask.view(frame_attn_mask.size(0), -1)
+ frame_atts = frame_atts * frame_attn_mask
+
+ video_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=frame_hidden_state,
+ encoder_attention_mask=frame_atts,
+ return_dict=True,
+ )
+ video_hidden = video_query_output.last_hidden_state
+ atts_llama = torch.ones(video_hidden.size()[:-1], dtype=torch.long).to(image_embeds.device)
+ return video_hidden, atts_llama
+
+ def encode_frame_level_visual(self, image, frame_attn_mask, return_attn=False):
+ device = image.device
+
+
+ # input shape b,t,c,h,w
+ batch_size, time_length, _, _, _ = image.size()
+ image = einops.rearrange(image, 'b t c h w -> (b t) c h w')
+
+ with self.maybe_autocast():
+ # embed image features with blip2, out: (b t) q h
+ image_embeds = self.ln_vision(self.visual_encoder(image)).to(device)
+ image_atts = torch.ones(image_embeds.size()[:-1], dtype=torch.long).to(device)
+
+ query_tokens = self.query_tokens.expand(image_embeds.shape[0], -1, -1)
+ query_output = self.Qformer.bert(
+ query_embeds=query_tokens,
+ encoder_hidden_states=image_embeds,
+ encoder_attention_mask=image_atts,
+ output_attentions=return_attn,
+ return_dict=True,
+ )
+
+ q_hidden_state = query_output.last_hidden_state
+ frame_hidden_state = einops.rearrange(q_hidden_state, '(b t) q h -> b t q h',b=batch_size,t=time_length)
+ frame_atts = torch.ones(frame_hidden_state.size()[:-1], dtype=torch.long).to(device)
+
+ if return_attn:
+ with self.maybe_autocast():
+ _, frame_patch_attn = self.visual_encoder.get_attn_weights(image)
+ return frame_hidden_state, frame_atts, query_output['attentions'], query_output['cross_attentions'], frame_patch_attn
+ else:
+ return frame_hidden_state, frame_atts
+
+
+ def prompt_wrap(self, img_embeds, atts_img, prompt):
+ if prompt:
+ batch_size = img_embeds.shape[0]
+ # print(prompt)
+ p_before, p_after = self.prompt.split('')
+ tmp = p_after.split('%s')
+ p_video_end = tmp[0]
+ p_inst_end = tmp[1]
+ p_before_tokens = self.llama_tokenizer(
+ p_before, return_tensors="pt", add_special_tokens=False).to(img_embeds.device)
+ p_video_end_tokens = self.llama_tokenizer(
+ p_video_end, return_tensors="pt", add_special_tokens=False).to(img_embeds.device)
+ p_inst_end_tokens = self.llama_tokenizer(
+ p_inst_end, return_tensors="pt", add_special_tokens=False).to(img_embeds.device)
+
+ prompt_tokens = self.llama_tokenizer(
+ prompt,
+ return_tensors="pt",
+ padding="longest",
+ truncation=True,
+ max_length=self.max_txt_len,
+ add_special_tokens=False
+ ).to(img_embeds.device)
+
+ p_before_embeds = self.llama_model.model.embed_tokens(p_before_tokens.input_ids).expand(batch_size, -1, -1)
+ p_video_end_embeds = self.llama_model.model.embed_tokens(p_video_end_tokens.input_ids).expand(batch_size, -1, -1)
+ p_inst_end_embeds = self.llama_model.model.embed_tokens(p_inst_end_tokens.input_ids).expand(batch_size, -1, -1)
+ p_tokens_embeds = self.llama_model.model.embed_tokens(prompt_tokens.input_ids)
+ wrapped_img_embeds = torch.cat([p_before_embeds, img_embeds, p_video_end_embeds, p_tokens_embeds, p_inst_end_embeds], dim=1)
+
+ p_before_attn = p_before_tokens.attention_mask.expand(batch_size, -1)
+ p_video_end_attn = p_video_end_tokens.attention_mask.expand(batch_size, -1)
+ p_inst_end_attn = p_inst_end_tokens.attention_mask.expand(batch_size, -1)
+ p_tokens_attn = prompt_tokens.attention_mask
+ wrapped_atts_img = torch.cat([p_before_attn, atts_img, p_video_end_attn, p_tokens_attn, p_inst_end_attn], dim=1)
+ return wrapped_img_embeds, wrapped_atts_img
+ else:
+ return img_embeds, atts_img
+ # input audio shape [b t c h w]
+ def encode_audioQformer(self, audio,modality_type=ModalityType.AUDIO):
+ device = audio.device
+ with self.maybe_autocast():
+ audio_feature, audio_imagebind_finalout = self.audio_encoder.get_audio_feature(audio,modality_type=modality_type)
+ batch_size,time_length = audio.size()[:2]
+
+
+ position_ids = torch.arange(time_length, dtype=torch.long, device=device)
+ position_ids = position_ids.unsqueeze(0).expand(batch_size, -1)
+
+ audio_position_embeddings = self.audio_position_embedding(position_ids)
+ audio_imagebind_finalout = audio_imagebind_finalout + audio_position_embeddings
+
+ audio_query_tokens = self.audio_query_tokens.expand(audio_imagebind_finalout.shape[0], -1, -1)
+ frame_atts = torch.ones(audio_imagebind_finalout.size()[:-1], dtype=torch.long).to(device)
+
+ audio_query_output = self.audio_Qformer.bert(
+ query_embeds=audio_query_tokens, #[32,768]
+ encoder_hidden_states=audio_imagebind_finalout,
+ encoder_attention_mask=frame_atts,
+ return_dict=True,
+ )
+ audio_hidden = audio_query_output.last_hidden_state
+
+ inputs_llama = self.audio_llama_proj(audio_hidden)
+ atts_llama = torch.ones(inputs_llama.size()[:-1], dtype=torch.long).to(device)
+
+ return inputs_llama, atts_llama
+
+ def encode_videoQformer_audiovideo(self, image, audio):
+ device = image.device
+
+ # input shape b,c,t,h,w
+ batch_size,_,time_length,_,_ = image.size()
+ image = einops.rearrange(image, 'b c t h w -> (b t) c h w')
+ with self.maybe_autocast():
+ # embed image features with blip2, out: (b t) q h
+ image_embeds = self.ln_vision(self.visual_encoder(image)).to(device)
+ image_atts = torch.ones(image_embeds.size()[:-1], dtype=torch.long).to(device)
+
+ query_tokens = self.query_tokens.expand(image_embeds.shape[0], -1, -1)
+ query_output = self.Qformer.bert(
+ query_embeds=query_tokens,
+ encoder_hidden_states=image_embeds,
+ encoder_attention_mask=image_atts,
+ return_dict=True,
+ )
+
+ # add frame_pos embedding
+ position_ids = torch.arange(time_length, dtype=torch.long, device=query_tokens.device)
+ position_ids = position_ids.unsqueeze(0).expand(batch_size, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ q_hidden_state = query_output.last_hidden_state
+
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ frame_hidden_state = einops.rearrange(q_hidden_state, '(b t) q h -> b t q h',b=batch_size,t=time_length)
+ frame_hidden_state = frame_position_embeddings + frame_hidden_state
+
+ # encode audio
+ audio_feature, audio_imagebind_finalout = self.audio_encoder.get_audio_feature(audio,modality_type=ModalityType.AUDIO) # [batch,8*1,768] 8*32, 768
+ audio_frame_position_embeddings = frame_position_embeddings.squeeze(-2)
+ audio_feature = audio_feature + audio_frame_position_embeddings
+
+ # frame attention a
+ frame_hidden_state = einops.rearrange(frame_hidden_state, 'b t q h -> b (t q) h',b=batch_size,t=time_length)
+ frame_hidden_state = torch.cat([frame_hidden_state,audio_feature],dim = 1)
+ video_query_tokens = self.video_query_tokens.expand(frame_hidden_state.shape[0], -1, -1)
+ frame_atts = torch.ones(frame_hidden_state.size()[:-1], dtype=torch.long).to(device)
+
+ video_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens, #[32,768]
+ encoder_hidden_states=frame_hidden_state,
+ encoder_attention_mask=frame_atts,
+ return_dict=True,
+ )
+ video_hidden = video_query_output.last_hidden_state
+
+ inputs_llama = self.llama_proj(video_hidden)
+ atts_llama = torch.ones(inputs_llama.size()[:-1], dtype=torch.long).to(image_embeds.device)
+
+ return inputs_llama, atts_llama
+
+ def forward(self, samples):
+
+ global_video = samples['global_video'].cuda()
+ global_frame_attn_mask = samples['global_frame_attn_mask'].cuda()
+ segments_video = samples['segments_video'].cuda()
+ segments_frame_attn_mask = samples['segments_frame_attn_mask'].cuda()
+ #text = samples['text']
+ text_question = samples['text_question']
+ text_answer = samples['text_answer']
+
+ batch_size = global_video.size(0)
+
+ global_video_embeds, global_video_embeds_mask = self.encode_videoQformer_visual(global_video, global_frame_attn_mask)
+ segments_video = segments_video.view(-1, self.num_frames_per_clip, segments_video.size(-3), segments_video.size(-2), segments_video.size(-1))
+ segments_frame_attn_mask = segments_frame_attn_mask.view(-1, self.num_frames_per_clip)
+
+ segments_video_embeds, segments_video_embeds_mask = self.encode_frame_level_visual(segments_video, segments_frame_attn_mask)
+ segments_video_embeds = segments_video_embeds.view(-1, self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(-1, self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ if self.hierarchical_agg_function == 'without-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned':
+ # add segment pos embedding
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+ num_region_queries = video_query_tokens.size(1)
+
+ # add short video segment prompts
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_context = global_video_embeds + curr_segment_query_tokens
+
+ global_context = global_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+
+ video_query_tokens = torch.cat([video_query_tokens, global_context], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_segment_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_segment_embeds = global_region_segment_embeds.view(batch_size, self.num_segments, global_region_segment_embeds.size(-2), global_region_segment_embeds.size(-1))
+
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(global_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + global_region_segment_embeds
+
+ segment_temporal_context = segments_hidden_state.mean(1)
+ segment_spatial_context = segments_hidden_state.mean(2)
+
+ if 'spatiotemporal-prompts' in self.hierarchical_agg_function:
+
+ if 'full-dis-spatiotemporal' in self.hierarchical_agg_function:
+ temporal_context_prompts = self.temporal_segment_prompts.unsqueeze(-2).expand(global_video_embeds.shape[0], -1, segments_hidden_state.size(-2), -1)
+ spatial_context_prompts = self.spatial_segment_prompts.unsqueeze(0).expand(global_video_embeds.shape[0], self.num_segments, -1, -1)
+
+ final_context = segments_hidden_state + temporal_context_prompts + spatial_context_prompts
+ final_context = final_context.view(final_context.size(0), -1, final_context.size(-1))
+
+ if 'without' in self.hierarchical_agg_function and 'full-dis-spatiotemporal' in self.hierarchical_agg_function:
+ final_top_down_context = final_context
+
+ final_top_down_context_mask = torch.ones(final_top_down_context.size()[:-1], dtype=torch.long).to(final_top_down_context.device)
+
+
+ if 'without-top' in self.hierarchical_agg_function:
+ merged_query_tokens = self.video_query_tokens.expand(len(global_video_embeds), -1, -1)
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ global_to_segment_prompts = self.global_to_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_to_segment_context = global_video_embeds + global_to_segment_prompts
+
+ merged_query_tokens = torch.cat([merged_query_tokens, global_to_segment_context], dim=1)
+
+ global_region_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=final_top_down_context,
+ encoder_attention_mask=final_top_down_context_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_output.last_hidden_state
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ global_region_merged_embeds = global_region_merged_embeds[:, :num_region_queries]
+
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+
+ merged_video_embeds = self.llama_proj(merged_video_embeds)
+ merged_video_embeds = merged_video_embeds + self.global_region_embed_weight * global_region_merged_embeds
+
+ merged_video_embeds, merged_atts_video = self.prompt_wrap(merged_video_embeds, merged_video_embeds_mask, text_question)
+ to_regress_tokens = self.llama_tokenizer(
+ text_answer,
+ return_tensors="pt",
+ padding="longest",
+ truncation=True,
+ #max_length=self.max_txt_len,
+ add_special_tokens=False
+ ).to(global_video.device)
+
+ targets = to_regress_tokens.input_ids.masked_fill(
+ to_regress_tokens.input_ids == self.llama_tokenizer.pad_token_id, -100
+ )
+
+ empty_targets = (
+ torch.ones([merged_atts_video.shape[0], merged_atts_video.shape[1]+1],
+ dtype=torch.long).to(global_video.device).fill_(-100) # plus one for bos
+ )
+
+ targets = torch.cat([empty_targets, targets], dim=1)
+
+ batch_size = merged_video_embeds.shape[0]
+ bos = torch.ones([batch_size, 1],
+ dtype=to_regress_tokens.input_ids.dtype,
+ device=to_regress_tokens.input_ids.device) * self.llama_tokenizer.bos_token_id
+ bos_embeds = self.llama_model.model.embed_tokens(bos)
+ atts_bos = merged_atts_video[:, :1]
+
+ to_regress_embeds = self.llama_model.model.embed_tokens(to_regress_tokens.input_ids)
+ inputs_embeds = torch.cat([bos_embeds, merged_video_embeds, to_regress_embeds], dim=1)
+ attention_mask = torch.cat([atts_bos, merged_atts_video, to_regress_tokens.attention_mask], dim=1)
+
+ with self.maybe_autocast():
+ outputs = self.llama_model(
+ inputs_embeds=inputs_embeds,
+ attention_mask=attention_mask,
+ return_dict=True,
+ labels=targets,
+ )
+ loss = outputs.loss
+
+ return {"loss": loss}
+
+ def compute_merged_video_embeds(self, samples):
+
+ global_video = samples['global_video'].cuda()
+ global_frame_attn_mask = samples['global_frame_attn_mask'].cuda()
+ segments_video = samples['segments_video'].cuda()
+ segments_frame_attn_mask = samples['segments_frame_attn_mask'].cuda()
+
+ global_video_embeds, global_video_embeds_mask = self.encode_videoQformer_visual(global_video, global_frame_attn_mask)
+
+ segments_video = segments_video.view(-1, self.num_frames_per_clip, segments_video.size(-3), segments_video.size(-2), segments_video.size(-1))
+ segments_frame_attn_mask = segments_frame_attn_mask.view(-1, self.num_frames_per_clip)
+
+ if 'early-attn' not in self.hierarchical_agg_function:
+ segments_video_embeds, segments_video_embeds_mask = self.encode_videoQformer_visual(segments_video, segments_frame_attn_mask, global_video=False)
+
+ segments_video_embeds = segments_video_embeds.view(-1, self.num_segments, segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(-1, self.num_segments, segments_video_embeds_mask.size(-1))
+ else:
+ segments_video_embeds, segments_video_embeds_mask = self.encode_frame_level_visual(segments_video, segments_frame_attn_mask)
+ segments_video_embeds = segments_video_embeds.view(-1, self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(-1, self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ ############################################################################################
+
+ batch_size = global_video_embeds.size(0)
+
+ if self.hierarchical_agg_function == 'average':
+ segments_video_embeds = segments_video_embeds.mean(1, keepdim=True)
+ merged_video_embeds = torch.cat([global_video_embeds.unsqueeze(1), segments_video_embeds], dim=1)
+ merged_video_embeds = merged_video_embeds.mean(1)
+ merged_video_embeds_mask = segments_video_embeds_mask[:, 0]
+ elif self.hierarchical_agg_function == 'global-region':
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(segments_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + segments_video_embeds
+ segments_hidden_state = einops.rearrange(segments_hidden_state, 'b t q h -> b (t q) h',b=len(segments_hidden_state),t=self.num_segments)
+
+ merged_query_output = self.video_Qformer.bert(
+ query_embeds=global_video_embeds,
+ encoder_hidden_states=segments_hidden_state,
+ encoder_attention_mask=segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1),
+ #output_attentions=True,
+ return_dict=True,
+ )
+ merged_video_embeds = merged_query_output.last_hidden_state
+ merged_video_embeds_mask = segments_video_embeds_mask[:, 0]
+ elif self.hierarchical_agg_function == 'global-region-prompts':
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(segments_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + segments_video_embeds
+ segments_hidden_state = einops.rearrange(segments_hidden_state, 'b t q h -> b (t q) h',b=len(segments_hidden_state),t=self.num_segments)
+ segments_attn_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+ segments_hidden_state = torch.cat([global_video_embeds, segments_hidden_state], dim=1)
+ segments_attn_mask = torch.cat([global_video_embeds_mask, segments_attn_mask], dim=1)
+
+ merged_query_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=segments_hidden_state,
+ encoder_attention_mask=segments_attn_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ merged_video_embeds = merged_query_output.last_hidden_state
+ merged_video_embeds_mask = segments_video_embeds_mask[:, 0]
+ elif self.hierarchical_agg_function == 'global-region-prompts-attn':
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(segments_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + segments_video_embeds
+ segments_hidden_state = einops.rearrange(segments_hidden_state, 'b t q h -> b (t q) h',b=len(segments_hidden_state),t=self.num_segments)
+ segments_attn_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+ segments_hidden_state = torch.cat([global_video_embeds, segments_hidden_state], dim=1)
+ segments_attn_mask = torch.cat([global_video_embeds_mask, segments_attn_mask], dim=1)
+
+ merged_query_output = self.global_region_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=segments_hidden_state,
+ encoder_attention_mask=segments_attn_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+
+ merged_video_embeds = merged_query_output.last_hidden_state
+ merged_video_embeds_mask = segments_video_embeds_mask[:, 0]
+ elif self.hierarchical_agg_function == 'average-linear' or self.hierarchical_agg_function == 'average-linear-learned':
+ segments_video_embeds = segments_video_embeds.mean(1)
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = segments_video_embeds_mask[:, 0]
+
+ global_region_merged_embeds = self.video_global_proj(segments_video_embeds)
+ elif self.hierarchical_agg_function == 'global-region-prompts-linear':
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(segments_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + segments_video_embeds
+ segments_hidden_state = einops.rearrange(segments_hidden_state, 'b t q h -> b (t q) h',b=len(segments_hidden_state),t=self.num_segments)
+ segments_attn_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+ segments_hidden_state = torch.cat([global_video_embeds, segments_hidden_state], dim=1)
+ segments_attn_mask = torch.cat([global_video_embeds_mask, segments_attn_mask], dim=1)
+
+ global_region_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=segments_hidden_state,
+ encoder_attention_mask=segments_attn_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_output.last_hidden_state
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+ elif self.hierarchical_agg_function == 'global-to-region-early-attn-linear' or self.hierarchical_agg_function == 'global-to-region-early-attn-linear-learned':
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+
+ global_context = global_video_embeds.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+
+ num_region_queries = video_query_tokens.size(1)
+ video_query_tokens = torch.cat([video_query_tokens, global_context], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_merged_embeds = global_region_merged_embeds.view(batch_size, self.num_segments, global_region_merged_embeds.size(-2), global_region_merged_embeds.size(-1))
+ global_region_merged_embeds = global_region_merged_embeds.mean(1)
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+ elif self.hierarchical_agg_function == 'global-prompts-region-prompts-early-attn-linear' or self.hierarchical_agg_function == 'global-prompts-region-prompts-early-attn-linear-weighted' or self.hierarchical_agg_function == 'global-prompts-region-prompts-early-attn-linear-learned' or self.hierarchical_agg_function == 'global-prompts-region-prompts-segment-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'global-prompts-region-prompts-region-attn-segment-attn-early-attn-linear-learned':
+ # add segment pos embedding
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+ num_region_queries = video_query_tokens.size(1)
+
+ # add short video segment prompts
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_context = global_video_embeds + curr_segment_query_tokens
+ global_context = global_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+
+ if 'region-attn' in self.hierarchical_agg_function:
+ region_context = segments_video_embeds.view(batch_size, self.num_segments, -1, segments_video_embeds.size(-1)).mean(1)
+ region_context = region_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ region_context = region_context.view(-1, region_context.size(-2), region_context.size(-1))
+ region_attn_queries = self.region_attn_queries.expand(segments_video_embeds.shape[0], -1, -1, -1)
+ region_attn_queries = region_attn_queries.view(region_attn_queries.size(0), -1, region_attn_queries.size(-1))
+ region_context = region_context + region_attn_queries
+ else:
+ region_context = None
+
+ if 'segment-attn' in self.hierarchical_agg_function:
+ segment_attn_queries = self.segment_attn_queries.expand(global_video_embeds.shape[0], -1, -1)
+ segment_context = segments_video_embeds.mean(1)
+ segment_context = segment_context.view(batch_size, self.num_segments, segment_context.size(-1))
+ segment_context = segment_context + segment_attn_queries
+ segment_context = segment_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ segment_context = segment_context.view(-1, segment_context.size(-2), segment_context.size(-1))
+
+ if region_context is not None:
+ video_query_tokens = torch.cat([video_query_tokens, region_context, segment_context, global_context], dim=1)
+ else:
+ video_query_tokens = torch.cat([video_query_tokens, segment_context, global_context], dim=1)
+ else:
+ video_query_tokens = torch.cat([video_query_tokens, global_context], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_segment_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_segment_embeds = global_region_segment_embeds.view(batch_size, self.num_segments, global_region_segment_embeds.size(-2), global_region_segment_embeds.size(-1))
+
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(global_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + global_region_segment_embeds
+ segments_hidden_state = einops.rearrange(segments_hidden_state, 'b t q h -> b (t q) h',b=len(segments_hidden_state),t=self.num_segments)
+ #segments_attn_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+
+ segments_attn_mask = torch.ones(segments_hidden_state.size()[:-1], dtype=torch.long).to(segments_hidden_state.device)
+
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+ segments_hidden_state = torch.cat([global_video_embeds, segments_hidden_state], dim=1)
+ segments_attn_mask = torch.cat([global_video_embeds_mask, segments_attn_mask], dim=1)
+
+ global_region_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=segments_hidden_state,
+ encoder_attention_mask=segments_attn_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_output.last_hidden_state
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+ elif self.hierarchical_agg_function == 'global-to-region-prompts-early-attn-linear' or self.hierarchical_agg_function == 'global-to-region-prompts-early-attn-linear-learned':
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_context = global_video_embeds + curr_segment_query_tokens
+
+ global_context = global_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+
+ num_region_queries = video_query_tokens.size(1)
+ video_query_tokens = torch.cat([video_query_tokens, global_context], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_merged_embeds = global_region_merged_embeds.view(batch_size, self.num_segments, global_region_merged_embeds.size(-2), global_region_merged_embeds.size(-1))
+ global_region_merged_embeds = global_region_merged_embeds.mean(1)
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+
+ elif self.hierarchical_agg_function == 'top-down-context-segment-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'top-down-context-region-attn-segment-attn-early-attn-linear-learned':
+ # add segment pos embedding
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+ num_region_queries = video_query_tokens.size(1)
+
+ # add short video segment prompts
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_context = global_video_embeds + curr_segment_query_tokens
+
+ global_context = global_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+ global_context_attn_mask = global_video_embeds_mask.unsqueeze(1).repeat(1, self.num_segments, 1)
+ global_context_attn_mask = global_context_attn_mask.view(-1, global_context_attn_mask.size(-1))
+
+ segment_attn_queries = self.segment_attn_queries.expand(global_video_embeds.shape[0], -1, -1)
+ segment_context = segments_video_embeds.mean(1)
+ segment_context = segment_context.view(batch_size, self.num_segments, segment_context.size(-1))
+ segment_context = segment_context + segment_attn_queries
+ segment_context = segment_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ segment_context = segment_context.view(-1, segment_context.size(-2), segment_context.size(-1))
+ segment_context_attn_mask = torch.ones(segment_context.size()[:-1], dtype=torch.long).to(segment_context.device)
+
+ if 'region-attn' in self.hierarchical_agg_function:
+ region_context = segments_video_embeds.view(batch_size, self.num_segments, -1, segments_video_embeds.size(-1)).mean(1)
+ region_context = region_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ region_context = region_context.view(-1, region_context.size(-2), region_context.size(-1))
+ region_attn_queries = self.region_attn_queries.expand(segments_video_embeds.shape[0], -1, -1, -1)
+ region_attn_queries = region_attn_queries.view(region_attn_queries.size(0), -1, region_attn_queries.size(-1))
+ region_context = region_context + region_attn_queries
+
+ region_context_attn_mask = segments_video_embeds_mask.clone()
+
+ segments_video_embeds = torch.cat([segments_video_embeds, region_context, segment_context, global_context], dim=1)
+ segments_video_embeds_mask = torch.cat([segments_video_embeds_mask, region_context_attn_mask, segment_context_attn_mask, global_context_attn_mask], dim=1)
+ else:
+ segments_video_embeds = torch.cat([segments_video_embeds, segment_context, global_context], dim=1)
+ segments_video_embeds_mask = torch.cat([segments_video_embeds_mask, segment_context_attn_mask, global_context_attn_mask], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_segment_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_segment_embeds = global_region_segment_embeds.view(batch_size, self.num_segments, global_region_segment_embeds.size(-2), global_region_segment_embeds.size(-1))
+
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(global_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + global_region_segment_embeds
+ segments_hidden_state = einops.rearrange(segments_hidden_state, 'b t q h -> b (t q) h',b=len(segments_hidden_state),t=self.num_segments)
+ #segments_attn_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+
+ segments_attn_mask = torch.ones(segments_hidden_state.size()[:-1], dtype=torch.long).to(segments_hidden_state.device)
+
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+ segments_hidden_state = torch.cat([global_video_embeds, segments_hidden_state], dim=1)
+ segments_attn_mask = torch.cat([global_video_embeds_mask, segments_attn_mask], dim=1)
+
+ global_region_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=segments_hidden_state,
+ encoder_attention_mask=segments_attn_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_output.last_hidden_state
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+ elif self.hierarchical_agg_function == 'global-prompts-region-prompts-segment-spatiotemporal-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'global-prompts-region-prompts-segment-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'global-prompts-region-segment-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'without-global-prompts-region-segment-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'without-top-global-prompts-region-segment-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'without-top-global-prompts-region-segment-full-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'without-top-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'without-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned' or self.hierarchical_agg_function == 'proj-without-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned':
+ # add segment pos embedding
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+ num_region_queries = video_query_tokens.size(1)
+
+ # add short video segment prompts
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_context = global_video_embeds + curr_segment_query_tokens
+
+ global_context = global_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+
+ if 'proj-' in self.hierarchical_agg_function:
+ global_context = self.global_frame_proj(global_context)
+
+ if 'region-prompts' in self.hierarchical_agg_function:
+ segment_attn_queries = self.segment_attn_queries.expand(global_video_embeds.shape[0], -1, -1)
+ segment_context = segments_video_embeds.mean(1)
+
+ segment_context = segment_context.view(batch_size, self.num_segments, segment_context.size(-1))
+ segment_context = segment_context + segment_attn_queries
+
+ segment_context = segment_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+
+ segment_context = segment_context.view(-1, segment_context.size(-2), segment_context.size(-1))
+
+ video_query_tokens = torch.cat([video_query_tokens, segment_context, global_context], dim=1)
+ else:
+ video_query_tokens = torch.cat([video_query_tokens, global_context], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_segment_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_segment_embeds = global_region_segment_embeds.view(batch_size, self.num_segments, global_region_segment_embeds.size(-2), global_region_segment_embeds.size(-1))
+
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(global_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + global_region_segment_embeds
+
+ segment_temporal_context = segments_hidden_state.mean(1)
+ segment_spatial_context = segments_hidden_state.mean(2)
+
+ if 'spatiotemporal-prompts' in self.hierarchical_agg_function:
+
+ if 'full-dis-spatiotemporal' in self.hierarchical_agg_function:
+ temporal_context_prompts = self.temporal_segment_prompts.unsqueeze(-2).expand(global_video_embeds.shape[0], -1, segments_hidden_state.size(-2), -1)
+ spatial_context_prompts = self.spatial_segment_prompts.unsqueeze(0).expand(global_video_embeds.shape[0], self.num_segments, -1, -1)
+
+ final_context = segments_hidden_state + temporal_context_prompts + spatial_context_prompts
+ final_context = final_context.view(final_context.size(0), -1, final_context.size(-1))
+
+ elif 'full-spatiotemporal' not in self.hierarchical_agg_function:
+ temporal_context_prompts = self.temporal_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ spatial_context_prompts = self.spatial_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+
+ segment_temporal_context = segment_temporal_context + spatial_context_prompts
+ segment_spatial_context = segment_spatial_context + temporal_context_prompts
+ else:
+ spatial_context_prompts = self.spatial_segment_prompts.unsqueeze(0).expand(global_video_embeds.shape[0], -1, -1, -1)
+ segment_spatial_context = segments_hidden_state + spatial_context_prompts
+ segment_spatial_context = segment_spatial_context.view(segment_spatial_context.size(0), -1, segment_spatial_context.size(-1))
+
+ if 'without' in self.hierarchical_agg_function and 'full-dis-spatiotemporal' in self.hierarchical_agg_function:
+ final_top_down_context = final_context
+ elif 'without' in self.hierarchical_agg_function and 'full-spatiotemporal' not in self.hierarchical_agg_function:
+ final_top_down_context = torch.cat([segment_temporal_context, segment_spatial_context], dim=1)
+ elif 'without' in self.hierarchical_agg_function and 'full-spatiotemporal' in self.hierarchical_agg_function:
+ final_top_down_context = segment_spatial_context
+ else:
+ final_top_down_context = torch.cat([global_video_embeds, segment_temporal_context, segment_spatial_context], dim=1)
+ final_top_down_context_mask = torch.ones(final_top_down_context.size()[:-1], dtype=torch.long).to(final_top_down_context.device)
+
+ if 'without-top' in self.hierarchical_agg_function:
+ merged_query_tokens = self.video_query_tokens.expand(len(global_video_embeds), -1, -1)
+ else:
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ global_to_segment_prompts = self.global_to_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_to_segment_context = global_video_embeds + global_to_segment_prompts
+
+ if 'proj-' in self.hierarchical_agg_function:
+ global_to_segment_context = self.global_segment_proj(global_to_segment_context)
+
+ merged_query_tokens = torch.cat([merged_query_tokens, global_to_segment_context], dim=1)
+
+ global_region_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=final_top_down_context,
+ encoder_attention_mask=final_top_down_context_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_output.last_hidden_state
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ global_region_merged_embeds = global_region_merged_embeds[:, :num_region_queries]
+
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+ elif self.hierarchical_agg_function == 'without-context-top-final-global-prompts-region-segment-full-dis-spatiotemporal-prompts-attn-early-attn-linear-learned':
+ # add segment pos embedding
+ position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(batch_size*self.num_segments, segments_video_embeds.size(-3), segments_video_embeds.size(-2), segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(batch_size*self.num_segments, segments_video_embeds_mask.size(-2), segments_video_embeds_mask.size(-1))
+
+ position_ids = position_ids.unsqueeze(0).expand(batch_size*self.num_segments, -1)
+ frame_position_embeddings = self.video_frame_position_embedding(position_ids)
+ frame_position_embeddings = frame_position_embeddings.unsqueeze(-2)
+ segments_video_embeds = frame_position_embeddings + segments_video_embeds
+ segments_video_embeds_mask = segments_video_embeds_mask * segments_frame_attn_mask.unsqueeze(-1)
+
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+ video_query_tokens = self.video_query_tokens.expand(segments_video_embeds.shape[0], -1, -1)
+ num_region_queries = video_query_tokens.size(1)
+
+ # add short video segment prompts
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_context = curr_segment_query_tokens
+
+ global_context = global_context.unsqueeze(1).repeat(1, self.num_segments, 1, 1)
+
+ global_context = global_context.view(-1, global_context.size(-2), global_context.size(-1))
+
+ video_query_tokens = torch.cat([video_query_tokens, global_context], dim=1)
+
+ global_region_query_output = self.video_Qformer.bert(
+ query_embeds=video_query_tokens,
+ encoder_hidden_states=segments_video_embeds,
+ encoder_attention_mask=segments_video_embeds_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_segment_embeds = global_region_query_output.last_hidden_state[:, :num_region_queries]
+ global_region_segment_embeds = global_region_segment_embeds.view(batch_size, self.num_segments, global_region_segment_embeds.size(-2), global_region_segment_embeds.size(-1))
+
+ # add segment pos embedding
+ position_ids = torch.arange(self.num_segments, dtype=torch.long, device=segments_video_embeds.device)
+ position_ids = position_ids.unsqueeze(0).expand(len(global_video_embeds), -1)
+ segments_position_embeddings = self.video_frame_position_embedding(position_ids)
+
+ segments_position_embeddings = segments_position_embeddings.unsqueeze(-2)
+ segments_hidden_state = segments_position_embeddings + global_region_segment_embeds
+
+ segment_temporal_context = segments_hidden_state.mean(1)
+ segment_spatial_context = segments_hidden_state.mean(2)
+
+ temporal_context_prompts = self.temporal_segment_prompts.unsqueeze(-2).expand(global_video_embeds.shape[0], -1, segments_hidden_state.size(-2), -1)
+ spatial_context_prompts = self.spatial_segment_prompts.unsqueeze(0).expand(global_video_embeds.shape[0], self.num_segments, -1, -1)
+ final_context = segments_hidden_state + temporal_context_prompts + spatial_context_prompts
+ final_context = final_context.view(final_context.size(0), -1, final_context.size(-1))
+
+ final_top_down_context = final_context
+ final_top_down_context_mask = torch.ones(final_top_down_context.size()[:-1], dtype=torch.long).to(final_top_down_context.device)
+
+ if 'without-top' in self.hierarchical_agg_function:
+ merged_query_tokens = self.video_query_tokens.expand(len(global_video_embeds), -1, -1)
+ else:
+ merged_query_tokens = self.global_region_prompts.expand(len(global_video_embeds), -1, -1)
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ global_to_segment_prompts = self.global_to_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_to_segment_context = global_to_segment_prompts
+ merged_query_tokens = torch.cat([merged_query_tokens, global_to_segment_context], dim=1)
+
+ global_region_output = self.video_Qformer.bert(
+ query_embeds=merged_query_tokens,
+ encoder_hidden_states=final_top_down_context,
+ encoder_attention_mask=final_top_down_context_mask,
+ #output_attentions=True,
+ return_dict=True,
+ )
+ global_region_merged_embeds = global_region_output.last_hidden_state
+
+ if 'final-global-prompts' in self.hierarchical_agg_function:
+ global_region_merged_embeds = global_region_merged_embeds[:, :num_region_queries]
+
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask
+
+ elif self.hierarchical_agg_function == 'ablation-concat-linear':
+ #position_ids = torch.arange(segments_video_embeds.size(2), dtype=torch.long, device=self.video_query_tokens.device)
+ segments_video_embeds = segments_video_embeds.view(segments_video_embeds.size(0), -1, segments_video_embeds.size(-1))
+ segments_video_embeds_mask = segments_video_embeds_mask.view(segments_video_embeds_mask.size(0), -1)
+
+ curr_segment_query_tokens = self.segment_region_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ temporal_context_prompts = self.temporal_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ spatial_context_prompts = self.spatial_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+ global_to_segment_prompts = self.global_to_segment_prompts.expand(global_video_embeds.shape[0], -1, -1)
+
+ global_region_merged_embeds = torch.cat([curr_segment_query_tokens, temporal_context_prompts, spatial_context_prompts, global_to_segment_prompts], dim=1)
+
+ global_region_merged_embeds = self.video_global_proj(global_region_merged_embeds)
+ merged_video_embeds = global_video_embeds
+ merged_video_embeds_mask = global_video_embeds_mask[:, 0:1].expand(-1, global_region_merged_embeds.size(1)+global_video_embeds_mask.size(1))
+
+ merged_video_embeds = self.llama_proj(merged_video_embeds)
+ merged_video_embeds = merged_video_embeds + self.global_region_embed_weight * global_region_merged_embeds
+
+ return merged_video_embeds, merged_video_embeds_mask
+
+ @classmethod
+ def from_config(cls, cfg):
+ vit_model = cfg.get("vit_model", "eva_clip_g")
+ q_former_model = cfg.get("q_former_model", "https://storage.googleapis.com/sfr-vision-language-research/LAVIS/models/BLIP2/blip2_pretrained_flant5xxl.pth")
+ img_size = cfg.get("image_size")
+ num_query_token = cfg.get("num_query_token")
+ llama_model = cfg.get("llama_model")
+
+ drop_path_rate = cfg.get("drop_path_rate", 0)
+ use_grad_checkpoint = cfg.get("use_grad_checkpoint", False)
+ vit_precision = cfg.get("vit_precision", "fp16")
+ freeze_vit = cfg.get("freeze_vit", True)
+ freeze_qformer = cfg.get("freeze_qformer", True)
+ low_resource = cfg.get("low_resource", False)
+ device_8bit = cfg.get("device_8bit", 0)
+
+ prompt_path = cfg.get("prompt_path", "")
+ prompt_template = cfg.get("prompt_template", "")
+ max_txt_len = cfg.get("max_txt_len", 32)
+ end_sym = cfg.get("end_sym", '\n')
+
+ frozen_llama_proj = cfg.get("frozen_llama_proj", True)
+ frozen_video_Qformer = cfg.get("frozen_video_Qformer", True)
+ frozen_audio_Qformer = cfg.get("frozen_audio_Qformer", True)
+
+ llama_proj_model = cfg.get("llama_proj_model", '')
+
+ fusion_header_type = cfg.get("fusion_header_type", 'seqTransf')
+ max_frame_pos = cfg.get("max_frame_pos", 32)
+ fusion_head_layers = cfg.get("fusion_head_layers", 2)
+ num_video_query_token = cfg.get("num_video_query_token", 32)
+
+ equip_audio_branch= cfg.get("equip_audio_branch", True)
+ num_audio_query_token = cfg.get("num_audio_query_token", 8)
+ imagebind_ckpt_path = cfg.get("imagebind_ckpt_path", '/mnt/workspace/ckpt')
+ model = cls(
+ vit_model=vit_model,
+ q_former_model=q_former_model,
+ img_size=img_size,
+ drop_path_rate=drop_path_rate,
+ use_grad_checkpoint=use_grad_checkpoint,
+ vit_precision=vit_precision,
+ freeze_vit=freeze_vit,
+ freeze_qformer=freeze_qformer,
+ num_query_token=num_query_token,
+ llama_model=llama_model,
+ prompt_path=prompt_path,
+ prompt_template=prompt_template,
+ max_txt_len=max_txt_len,
+ end_sym=end_sym,
+ low_resource=low_resource,
+ device_8bit=device_8bit,
+ fusion_header_type=fusion_header_type,
+ max_frame_pos=max_frame_pos,
+ fusion_head_layers=fusion_head_layers,
+ frozen_llama_proj=frozen_llama_proj,
+ frozen_video_Qformer=frozen_video_Qformer,
+ frozen_audio_Qformer=frozen_audio_Qformer,
+ num_video_query_token=num_video_query_token,
+ num_audio_query_token = num_audio_query_token,
+ imagebind_ckpt_path = imagebind_ckpt_path,
+ equip_audio_branch = equip_audio_branch,
+ llama_proj_model = llama_proj_model
+ )
+
+ ckpt_path = cfg.get("ckpt", "") # load weights of MiniGPT-4
+ if ckpt_path:
+ print("Load first Checkpoint: {}".format(ckpt_path))
+ ckpt = torch.load(ckpt_path, map_location="cpu")
+ msg = model.load_state_dict(ckpt['model'], strict=False)
+ ckpt_path_2 = cfg.get("ckpt_2", "")
+ if ckpt_path_2:
+ print("Load second Checkpoint: {}".format(ckpt_path_2))
+ ckpt = torch.load(ckpt_path_2, map_location="cpu")
+ msg = model.load_state_dict(ckpt['model'], strict=False)
+ return model
diff --git a/global_local/processors/.ipynb_checkpoints/video_processor-checkpoint.py b/global_local/processors/.ipynb_checkpoints/video_processor-checkpoint.py
new file mode 100644
index 0000000000000000000000000000000000000000..ac0f3a9d56aef53de0ee9f2ee3d6d2cda0ea1674
--- /dev/null
+++ b/global_local/processors/.ipynb_checkpoints/video_processor-checkpoint.py
@@ -0,0 +1,243 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import torch
+from video_llama.common.registry import registry
+from decord import VideoReader
+import decord
+import numpy as np
+from video_llama.processors import transforms_video
+from video_llama.processors.base_processor import BaseProcessor
+from video_llama.processors.randaugment import VideoRandomAugment
+from video_llama.processors import functional_video as F
+from omegaconf import OmegaConf
+from torchvision import transforms
+import random as rnd
+MAX_INT = registry.get("MAX_INT")
+
+def load_video(video_path, n_frms=MAX_INT, height=-1, width=-1, sampling="uniform"):
+ vr = VideoReader(uri=video_path, height=height, width=width)
+
+ vlen = len(vr)
+ start, end = 0, vlen
+
+ n_frms = min(n_frms, vlen)
+
+ if sampling == "uniform":
+ indices = np.arange(start, end, vlen / n_frms).astype(int).tolist()
+ elif sampling == "headtail":
+ indices_h = sorted(rnd.sample(range(vlen // 2), n_frms // 2))
+ indices_t = sorted(rnd.sample(range(vlen // 2, vlen), n_frms // 2))
+ indices = indices_h + indices_t
+ else:
+ raise NotImplementedError
+
+ # get_batch -> T, H, W, C
+ print(video_path)
+ print(indices)
+ print(vr.get_batch(indices))
+
+ frms = vr.get_batch(indices).permute(3, 0, 1, 2).float() # (C, T, H, W)
+ # print(111)
+ return frms
+
+class AlproVideoBaseProcessor(BaseProcessor):
+ def __init__(self, mean=None, std=None, n_frms=MAX_INT):
+ if mean is None:
+ mean = (0.48145466, 0.4578275, 0.40821073)
+ if std is None:
+ std = (0.26862954, 0.26130258, 0.27577711)
+
+ self.normalize = transforms_video.NormalizeVideo(mean, std)
+
+ self.n_frms = n_frms
+
+
+class ToUint8(object):
+ def __init__(self):
+ pass
+
+ def __call__(self, tensor):
+ return tensor.to(torch.uint8)
+
+ def __repr__(self):
+ return self.__class__.__name__
+
+
+class ToTHWC(object):
+ """
+ Args:
+ clip (torch.tensor, dtype=torch.uint8): Size is (C, T, H, W)
+ Return:
+ clip (torch.tensor, dtype=torch.float): Size is (T, H, W, C)
+ """
+
+ def __init__(self):
+ pass
+
+ def __call__(self, tensor):
+ return tensor.permute(1, 2, 3, 0)
+
+ def __repr__(self):
+ return self.__class__.__name__
+
+
+class ResizeVideo(object):
+ def __init__(self, target_size, interpolation_mode="bilinear"):
+ self.target_size = target_size
+ self.interpolation_mode = interpolation_mode
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: central cropping of video clip. Size is
+ (C, T, crop_size, crop_size)
+ """
+ return F.resize(clip, self.target_size, self.interpolation_mode)
+
+ def __repr__(self):
+ return self.__class__.__name__ + "(resize_size={0})".format(self.target_size)
+
+
+@registry.register_processor("alpro_video_train")
+class AlproVideoTrainProcessor(AlproVideoBaseProcessor):
+ def __init__(
+ self,
+ image_size=384,
+ mean=None,
+ std=None,
+ min_scale=0.5,
+ max_scale=1.0,
+ n_frms=MAX_INT,
+ ):
+ super().__init__(mean=mean, std=std, n_frms=n_frms)
+
+ self.image_size = image_size
+
+ self.transform = transforms.Compose(
+ [
+ # Video size is (C, T, H, W)
+ transforms_video.RandomResizedCropVideo(
+ image_size,
+ scale=(min_scale, max_scale),
+ interpolation_mode="bicubic",
+ ),
+ transforms_video.RandomHorizontalFlipVideo(),
+ ToTHWC(), # C, T, H, W -> T, H, W, C
+ VideoRandomAugment(
+ 2,
+ 5,
+ augs=[
+ "Identity",
+ "AutoContrast",
+ "Brightness",
+ "Sharpness",
+ "Equalize",
+ "ShearX",
+ "ShearY",
+ "TranslateX",
+ "TranslateY",
+ "Rotate",
+ ],
+ ),
+ ToUint8(),
+ transforms_video.ToTensorVideo(), # T, H, W, C -> C, T, H, W
+ self.normalize,
+ ]
+ )
+
+ def __call__(self, vpath):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: video clip after transforms. Size is (C, T, size, size).
+ """
+ clip = load_video(
+ video_path=vpath,
+ n_frms=self.n_frms,
+ height=self.image_size,
+ width=self.image_size,
+ sampling="headtail",
+ )
+
+ return self.transform(clip)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ image_size = cfg.get("image_size", 256)
+
+ mean = cfg.get("mean", None)
+ std = cfg.get("std", None)
+
+ min_scale = cfg.get("min_scale", 0.5)
+ max_scale = cfg.get("max_scale", 1.0)
+
+ n_frms = cfg.get("n_frms", MAX_INT)
+
+ return cls(
+ image_size=image_size,
+ mean=mean,
+ std=std,
+ min_scale=min_scale,
+ max_scale=max_scale,
+ n_frms=n_frms,
+ )
+
+
+@registry.register_processor("alpro_video_eval")
+class AlproVideoEvalProcessor(AlproVideoBaseProcessor):
+ def __init__(self, image_size=256, mean=None, std=None, n_frms=MAX_INT):
+ super().__init__(mean=mean, std=std, n_frms=n_frms)
+
+ self.image_size = image_size
+
+ # Input video size is (C, T, H, W)
+ self.transform = transforms.Compose(
+ [
+ # frames will be resized during decord loading.
+ ToUint8(), # C, T, H, W
+ ToTHWC(), # T, H, W, C
+ transforms_video.ToTensorVideo(), # C, T, H, W
+ self.normalize, # C, T, H, W
+ ]
+ )
+
+ def __call__(self, vpath):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: video clip after transforms. Size is (C, T, size, size).
+ """
+ clip = load_video(
+ video_path=vpath,
+ n_frms=self.n_frms,
+ height=self.image_size,
+ width=self.image_size,
+ )
+
+ return self.transform(clip)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ image_size = cfg.get("image_size", 256)
+
+ mean = cfg.get("mean", None)
+ std = cfg.get("std", None)
+
+ n_frms = cfg.get("n_frms", MAX_INT)
+
+ return cls(image_size=image_size, mean=mean, std=std, n_frms=n_frms)
diff --git a/global_local/processors/__init__.py b/global_local/processors/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..8123a0fb1396d094754b509953aa711baffa8b5e
--- /dev/null
+++ b/global_local/processors/__init__.py
@@ -0,0 +1,38 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from global_local.processors.base_processor import BaseProcessor
+from global_local.processors.blip_processors import (
+ Blip2ImageTrainProcessor,
+ Blip2ImageEvalProcessor,
+ BlipCaptionProcessor,
+)
+from global_local.processors.video_processor import (
+ AlproVideoTrainProcessor,
+ AlproVideoEvalProcessor
+)
+from global_local.common.registry import registry
+
+__all__ = [
+ "BaseProcessor",
+ "Blip2ImageTrainProcessor",
+ "Blip2ImageEvalProcessor",
+ "BlipCaptionProcessor",
+ "AlproVideoTrainProcessor",
+ "AlproVideoEvalProcessor",
+]
+
+
+def load_processor(name, cfg=None):
+ """
+ Example
+
+ >>> processor = load_processor("alpro_video_train", cfg=None)
+ """
+ processor = registry.get_processor_class(name).from_config(cfg)
+
+ return processor
diff --git a/global_local/processors/__pycache__/__init__.cpython-39.pyc b/global_local/processors/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..b350fdf1af78216f66d20ab8ac285958e1dc0623
Binary files /dev/null and b/global_local/processors/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/processors/__pycache__/base_processor.cpython-39.pyc b/global_local/processors/__pycache__/base_processor.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..68add452c759c1b57df871f3791e5da9c565c676
Binary files /dev/null and b/global_local/processors/__pycache__/base_processor.cpython-39.pyc differ
diff --git a/global_local/processors/__pycache__/blip_processors.cpython-39.pyc b/global_local/processors/__pycache__/blip_processors.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..a8311868b7e1d537854cd6e846ce52c4940f4fba
Binary files /dev/null and b/global_local/processors/__pycache__/blip_processors.cpython-39.pyc differ
diff --git a/global_local/processors/__pycache__/functional_video.cpython-39.pyc b/global_local/processors/__pycache__/functional_video.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..69a24c7ce676ec370ae15c03c6248d510b401e36
Binary files /dev/null and b/global_local/processors/__pycache__/functional_video.cpython-39.pyc differ
diff --git a/global_local/processors/__pycache__/randaugment.cpython-39.pyc b/global_local/processors/__pycache__/randaugment.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..7937f9c4211603ad85015ca01da844b27f48dcc1
Binary files /dev/null and b/global_local/processors/__pycache__/randaugment.cpython-39.pyc differ
diff --git a/global_local/processors/__pycache__/transforms_video.cpython-39.pyc b/global_local/processors/__pycache__/transforms_video.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..a0597a6de7feedb453e22a9988bd5f70db0e5f9c
Binary files /dev/null and b/global_local/processors/__pycache__/transforms_video.cpython-39.pyc differ
diff --git a/global_local/processors/__pycache__/video_processor.cpython-39.pyc b/global_local/processors/__pycache__/video_processor.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..ef420b07ac108eadb5573e45e54fafa2e3ff7cf2
Binary files /dev/null and b/global_local/processors/__pycache__/video_processor.cpython-39.pyc differ
diff --git a/global_local/processors/base_processor.py b/global_local/processors/base_processor.py
new file mode 100644
index 0000000000000000000000000000000000000000..39b33cdf8fcd97cfd3e4a5fbece6593357af9d41
--- /dev/null
+++ b/global_local/processors/base_processor.py
@@ -0,0 +1,26 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from omegaconf import OmegaConf
+
+
+class BaseProcessor:
+ def __init__(self):
+ self.transform = lambda x: x
+ return
+
+ def __call__(self, item):
+ return self.transform(item)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ return cls()
+
+ def build(self, **kwargs):
+ cfg = OmegaConf.create(kwargs)
+
+ return self.from_config(cfg)
diff --git a/global_local/processors/blip_processors.py b/global_local/processors/blip_processors.py
new file mode 100644
index 0000000000000000000000000000000000000000..6b26ecb825dea1147320e1e923b5bd38e6102b9c
--- /dev/null
+++ b/global_local/processors/blip_processors.py
@@ -0,0 +1,142 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import re
+
+from global_local.common.registry import registry
+from global_local.processors.base_processor import BaseProcessor
+from global_local.processors.randaugment import RandomAugment
+from omegaconf import OmegaConf
+from torchvision import transforms
+from torchvision.transforms.functional import InterpolationMode
+
+
+class BlipImageBaseProcessor(BaseProcessor):
+ def __init__(self, mean=None, std=None):
+ if mean is None:
+ mean = (0.48145466, 0.4578275, 0.40821073)
+ if std is None:
+ std = (0.26862954, 0.26130258, 0.27577711)
+
+ self.normalize = transforms.Normalize(mean, std)
+
+
+@registry.register_processor("blip_caption")
+class BlipCaptionProcessor(BaseProcessor):
+ def __init__(self, prompt="", max_words=50):
+ self.prompt = prompt
+ self.max_words = max_words
+
+ def __call__(self, caption):
+ caption = self.prompt + self.pre_caption(caption)
+
+ return caption
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ prompt = cfg.get("prompt", "")
+ max_words = cfg.get("max_words", 50)
+
+ return cls(prompt=prompt, max_words=max_words)
+
+ def pre_caption(self, caption):
+ caption = re.sub(
+ r"([.!\"()*#:;~])",
+ " ",
+ caption.lower(),
+ )
+ caption = re.sub(
+ r"\s{2,}",
+ " ",
+ caption,
+ )
+ caption = caption.rstrip("\n")
+ caption = caption.strip(" ")
+
+ # truncate caption
+ caption_words = caption.split(" ")
+ if len(caption_words) > self.max_words:
+ caption = " ".join(caption_words[: self.max_words])
+
+ return caption
+
+
+@registry.register_processor("blip2_image_train")
+class Blip2ImageTrainProcessor(BlipImageBaseProcessor):
+ def __init__(self, image_size=224, mean=None, std=None, min_scale=0.5, max_scale=1.0):
+ super().__init__(mean=mean, std=std)
+
+ self.transform = transforms.Compose(
+ [
+ transforms.RandomResizedCrop(
+ image_size,
+ scale=(min_scale, max_scale),
+ interpolation=InterpolationMode.BICUBIC,
+ ),
+ transforms.ToTensor(),
+ self.normalize,
+ ]
+ )
+
+ def __call__(self, item):
+ return self.transform(item)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ image_size = cfg.get("image_size", 224)
+
+ mean = cfg.get("mean", None)
+ std = cfg.get("std", None)
+
+ min_scale = cfg.get("min_scale", 0.5)
+ max_scale = cfg.get("max_scale", 1.0)
+
+ return cls(
+ image_size=image_size,
+ mean=mean,
+ std=std,
+ min_scale=min_scale,
+ max_scale=max_scale,
+ )
+
+
+@registry.register_processor("blip2_image_eval")
+class Blip2ImageEvalProcessor(BlipImageBaseProcessor):
+ def __init__(self, image_size=224, mean=None, std=None):
+ super().__init__(mean=mean, std=std)
+
+ self.transform = transforms.Compose(
+ [
+ transforms.Resize(
+ (image_size, image_size), interpolation=InterpolationMode.BICUBIC
+ ),
+ transforms.ToTensor(),
+ self.normalize,
+ ]
+ )
+
+ def __call__(self, item):
+ return self.transform(item)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ image_size = cfg.get("image_size", 224)
+
+ mean = cfg.get("mean", None)
+ std = cfg.get("std", None)
+
+ return cls(image_size=image_size, mean=mean, std=std)
+
diff --git a/global_local/processors/functional_video.py b/global_local/processors/functional_video.py
new file mode 100644
index 0000000000000000000000000000000000000000..597a29315d4e1a575e7209edb0618eeaf4fc024a
--- /dev/null
+++ b/global_local/processors/functional_video.py
@@ -0,0 +1,121 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import warnings
+
+import torch
+
+
+def _is_tensor_video_clip(clip):
+ if not torch.is_tensor(clip):
+ raise TypeError("clip should be Tensor. Got %s" % type(clip))
+
+ if not clip.ndimension() == 4:
+ raise ValueError("clip should be 4D. Got %dD" % clip.dim())
+
+ return True
+
+
+def crop(clip, i, j, h, w):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ """
+ if len(clip.size()) != 4:
+ raise ValueError("clip should be a 4D tensor")
+ return clip[..., i : i + h, j : j + w]
+
+
+def resize(clip, target_size, interpolation_mode):
+ if len(target_size) != 2:
+ raise ValueError(
+ f"target size should be tuple (height, width), instead got {target_size}"
+ )
+ return torch.nn.functional.interpolate(
+ clip, size=target_size, mode=interpolation_mode, align_corners=False
+ )
+
+
+def resized_crop(clip, i, j, h, w, size, interpolation_mode="bilinear"):
+ """
+ Do spatial cropping and resizing to the video clip
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ i (int): i in (i,j) i.e coordinates of the upper left corner.
+ j (int): j in (i,j) i.e coordinates of the upper left corner.
+ h (int): Height of the cropped region.
+ w (int): Width of the cropped region.
+ size (tuple(int, int)): height and width of resized clip
+ Returns:
+ clip (torch.tensor): Resized and cropped clip. Size is (C, T, H, W)
+ """
+ if not _is_tensor_video_clip(clip):
+ raise ValueError("clip should be a 4D torch.tensor")
+ clip = crop(clip, i, j, h, w)
+ clip = resize(clip, size, interpolation_mode)
+ return clip
+
+
+def center_crop(clip, crop_size):
+ if not _is_tensor_video_clip(clip):
+ raise ValueError("clip should be a 4D torch.tensor")
+ h, w = clip.size(-2), clip.size(-1)
+ th, tw = crop_size
+ if h < th or w < tw:
+ raise ValueError("height and width must be no smaller than crop_size")
+
+ i = int(round((h - th) / 2.0))
+ j = int(round((w - tw) / 2.0))
+ return crop(clip, i, j, th, tw)
+
+
+def to_tensor(clip):
+ """
+ Convert tensor data type from uint8 to float, divide value by 255.0 and
+ permute the dimensions of clip tensor
+ Args:
+ clip (torch.tensor, dtype=torch.uint8): Size is (T, H, W, C)
+ Return:
+ clip (torch.tensor, dtype=torch.float): Size is (C, T, H, W)
+ """
+ _is_tensor_video_clip(clip)
+ if not clip.dtype == torch.uint8:
+ raise TypeError(
+ "clip tensor should have data type uint8. Got %s" % str(clip.dtype)
+ )
+ return clip.float().permute(3, 0, 1, 2) / 255.0
+
+
+def normalize(clip, mean, std, inplace=False):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be normalized. Size is (C, T, H, W)
+ mean (tuple): pixel RGB mean. Size is (3)
+ std (tuple): pixel standard deviation. Size is (3)
+ Returns:
+ normalized clip (torch.tensor): Size is (C, T, H, W)
+ """
+ if not _is_tensor_video_clip(clip):
+ raise ValueError("clip should be a 4D torch.tensor")
+ if not inplace:
+ clip = clip.clone()
+ mean = torch.as_tensor(mean, dtype=clip.dtype, device=clip.device)
+ std = torch.as_tensor(std, dtype=clip.dtype, device=clip.device)
+ clip.sub_(mean[:, None, None, None]).div_(std[:, None, None, None])
+ return clip
+
+
+def hflip(clip):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be normalized. Size is (C, T, H, W)
+ Returns:
+ flipped clip (torch.tensor): Size is (C, T, H, W)
+ """
+ if not _is_tensor_video_clip(clip):
+ raise ValueError("clip should be a 4D torch.tensor")
+ return clip.flip(-1)
diff --git a/global_local/processors/randaugment.py b/global_local/processors/randaugment.py
new file mode 100644
index 0000000000000000000000000000000000000000..7034a49ad5fc63b97910790017432617ff4c6d7b
--- /dev/null
+++ b/global_local/processors/randaugment.py
@@ -0,0 +1,398 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import cv2
+import numpy as np
+
+import torch
+
+
+## aug functions
+def identity_func(img):
+ return img
+
+
+def autocontrast_func(img, cutoff=0):
+ """
+ same output as PIL.ImageOps.autocontrast
+ """
+ n_bins = 256
+
+ def tune_channel(ch):
+ n = ch.size
+ cut = cutoff * n // 100
+ if cut == 0:
+ high, low = ch.max(), ch.min()
+ else:
+ hist = cv2.calcHist([ch], [0], None, [n_bins], [0, n_bins])
+ low = np.argwhere(np.cumsum(hist) > cut)
+ low = 0 if low.shape[0] == 0 else low[0]
+ high = np.argwhere(np.cumsum(hist[::-1]) > cut)
+ high = n_bins - 1 if high.shape[0] == 0 else n_bins - 1 - high[0]
+ if high <= low:
+ table = np.arange(n_bins)
+ else:
+ scale = (n_bins - 1) / (high - low)
+ offset = -low * scale
+ table = np.arange(n_bins) * scale + offset
+ table[table < 0] = 0
+ table[table > n_bins - 1] = n_bins - 1
+ table = table.clip(0, 255).astype(np.uint8)
+ return table[ch]
+
+ channels = [tune_channel(ch) for ch in cv2.split(img)]
+ out = cv2.merge(channels)
+ return out
+
+
+def equalize_func(img):
+ """
+ same output as PIL.ImageOps.equalize
+ PIL's implementation is different from cv2.equalize
+ """
+ n_bins = 256
+
+ def tune_channel(ch):
+ hist = cv2.calcHist([ch], [0], None, [n_bins], [0, n_bins])
+ non_zero_hist = hist[hist != 0].reshape(-1)
+ step = np.sum(non_zero_hist[:-1]) // (n_bins - 1)
+ if step == 0:
+ return ch
+ n = np.empty_like(hist)
+ n[0] = step // 2
+ n[1:] = hist[:-1]
+ table = (np.cumsum(n) // step).clip(0, 255).astype(np.uint8)
+ return table[ch]
+
+ channels = [tune_channel(ch) for ch in cv2.split(img)]
+ out = cv2.merge(channels)
+ return out
+
+
+def rotate_func(img, degree, fill=(0, 0, 0)):
+ """
+ like PIL, rotate by degree, not radians
+ """
+ H, W = img.shape[0], img.shape[1]
+ center = W / 2, H / 2
+ M = cv2.getRotationMatrix2D(center, degree, 1)
+ out = cv2.warpAffine(img, M, (W, H), borderValue=fill)
+ return out
+
+
+def solarize_func(img, thresh=128):
+ """
+ same output as PIL.ImageOps.posterize
+ """
+ table = np.array([el if el < thresh else 255 - el for el in range(256)])
+ table = table.clip(0, 255).astype(np.uint8)
+ out = table[img]
+ return out
+
+
+def color_func(img, factor):
+ """
+ same output as PIL.ImageEnhance.Color
+ """
+ ## implementation according to PIL definition, quite slow
+ # degenerate = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)[:, :, np.newaxis]
+ # out = blend(degenerate, img, factor)
+ # M = (
+ # np.eye(3) * factor
+ # + np.float32([0.114, 0.587, 0.299]).reshape(3, 1) * (1. - factor)
+ # )[np.newaxis, np.newaxis, :]
+ M = np.float32(
+ [[0.886, -0.114, -0.114], [-0.587, 0.413, -0.587], [-0.299, -0.299, 0.701]]
+ ) * factor + np.float32([[0.114], [0.587], [0.299]])
+ out = np.matmul(img, M).clip(0, 255).astype(np.uint8)
+ return out
+
+
+def contrast_func(img, factor):
+ """
+ same output as PIL.ImageEnhance.Contrast
+ """
+ mean = np.sum(np.mean(img, axis=(0, 1)) * np.array([0.114, 0.587, 0.299]))
+ table = (
+ np.array([(el - mean) * factor + mean for el in range(256)])
+ .clip(0, 255)
+ .astype(np.uint8)
+ )
+ out = table[img]
+ return out
+
+
+def brightness_func(img, factor):
+ """
+ same output as PIL.ImageEnhance.Contrast
+ """
+ table = (np.arange(256, dtype=np.float32) * factor).clip(0, 255).astype(np.uint8)
+ out = table[img]
+ return out
+
+
+def sharpness_func(img, factor):
+ """
+ The differences the this result and PIL are all on the 4 boundaries, the center
+ areas are same
+ """
+ kernel = np.ones((3, 3), dtype=np.float32)
+ kernel[1][1] = 5
+ kernel /= 13
+ degenerate = cv2.filter2D(img, -1, kernel)
+ if factor == 0.0:
+ out = degenerate
+ elif factor == 1.0:
+ out = img
+ else:
+ out = img.astype(np.float32)
+ degenerate = degenerate.astype(np.float32)[1:-1, 1:-1, :]
+ out[1:-1, 1:-1, :] = degenerate + factor * (out[1:-1, 1:-1, :] - degenerate)
+ out = out.astype(np.uint8)
+ return out
+
+
+def shear_x_func(img, factor, fill=(0, 0, 0)):
+ H, W = img.shape[0], img.shape[1]
+ M = np.float32([[1, factor, 0], [0, 1, 0]])
+ out = cv2.warpAffine(
+ img, M, (W, H), borderValue=fill, flags=cv2.INTER_LINEAR
+ ).astype(np.uint8)
+ return out
+
+
+def translate_x_func(img, offset, fill=(0, 0, 0)):
+ """
+ same output as PIL.Image.transform
+ """
+ H, W = img.shape[0], img.shape[1]
+ M = np.float32([[1, 0, -offset], [0, 1, 0]])
+ out = cv2.warpAffine(
+ img, M, (W, H), borderValue=fill, flags=cv2.INTER_LINEAR
+ ).astype(np.uint8)
+ return out
+
+
+def translate_y_func(img, offset, fill=(0, 0, 0)):
+ """
+ same output as PIL.Image.transform
+ """
+ H, W = img.shape[0], img.shape[1]
+ M = np.float32([[1, 0, 0], [0, 1, -offset]])
+ out = cv2.warpAffine(
+ img, M, (W, H), borderValue=fill, flags=cv2.INTER_LINEAR
+ ).astype(np.uint8)
+ return out
+
+
+def posterize_func(img, bits):
+ """
+ same output as PIL.ImageOps.posterize
+ """
+ out = np.bitwise_and(img, np.uint8(255 << (8 - bits)))
+ return out
+
+
+def shear_y_func(img, factor, fill=(0, 0, 0)):
+ H, W = img.shape[0], img.shape[1]
+ M = np.float32([[1, 0, 0], [factor, 1, 0]])
+ out = cv2.warpAffine(
+ img, M, (W, H), borderValue=fill, flags=cv2.INTER_LINEAR
+ ).astype(np.uint8)
+ return out
+
+
+def cutout_func(img, pad_size, replace=(0, 0, 0)):
+ replace = np.array(replace, dtype=np.uint8)
+ H, W = img.shape[0], img.shape[1]
+ rh, rw = np.random.random(2)
+ pad_size = pad_size // 2
+ ch, cw = int(rh * H), int(rw * W)
+ x1, x2 = max(ch - pad_size, 0), min(ch + pad_size, H)
+ y1, y2 = max(cw - pad_size, 0), min(cw + pad_size, W)
+ out = img.copy()
+ out[x1:x2, y1:y2, :] = replace
+ return out
+
+
+### level to args
+def enhance_level_to_args(MAX_LEVEL):
+ def level_to_args(level):
+ return ((level / MAX_LEVEL) * 1.8 + 0.1,)
+
+ return level_to_args
+
+
+def shear_level_to_args(MAX_LEVEL, replace_value):
+ def level_to_args(level):
+ level = (level / MAX_LEVEL) * 0.3
+ if np.random.random() > 0.5:
+ level = -level
+ return (level, replace_value)
+
+ return level_to_args
+
+
+def translate_level_to_args(translate_const, MAX_LEVEL, replace_value):
+ def level_to_args(level):
+ level = (level / MAX_LEVEL) * float(translate_const)
+ if np.random.random() > 0.5:
+ level = -level
+ return (level, replace_value)
+
+ return level_to_args
+
+
+def cutout_level_to_args(cutout_const, MAX_LEVEL, replace_value):
+ def level_to_args(level):
+ level = int((level / MAX_LEVEL) * cutout_const)
+ return (level, replace_value)
+
+ return level_to_args
+
+
+def solarize_level_to_args(MAX_LEVEL):
+ def level_to_args(level):
+ level = int((level / MAX_LEVEL) * 256)
+ return (level,)
+
+ return level_to_args
+
+
+def none_level_to_args(level):
+ return ()
+
+
+def posterize_level_to_args(MAX_LEVEL):
+ def level_to_args(level):
+ level = int((level / MAX_LEVEL) * 4)
+ return (level,)
+
+ return level_to_args
+
+
+def rotate_level_to_args(MAX_LEVEL, replace_value):
+ def level_to_args(level):
+ level = (level / MAX_LEVEL) * 30
+ if np.random.random() < 0.5:
+ level = -level
+ return (level, replace_value)
+
+ return level_to_args
+
+
+func_dict = {
+ "Identity": identity_func,
+ "AutoContrast": autocontrast_func,
+ "Equalize": equalize_func,
+ "Rotate": rotate_func,
+ "Solarize": solarize_func,
+ "Color": color_func,
+ "Contrast": contrast_func,
+ "Brightness": brightness_func,
+ "Sharpness": sharpness_func,
+ "ShearX": shear_x_func,
+ "TranslateX": translate_x_func,
+ "TranslateY": translate_y_func,
+ "Posterize": posterize_func,
+ "ShearY": shear_y_func,
+}
+
+translate_const = 10
+MAX_LEVEL = 10
+replace_value = (128, 128, 128)
+arg_dict = {
+ "Identity": none_level_to_args,
+ "AutoContrast": none_level_to_args,
+ "Equalize": none_level_to_args,
+ "Rotate": rotate_level_to_args(MAX_LEVEL, replace_value),
+ "Solarize": solarize_level_to_args(MAX_LEVEL),
+ "Color": enhance_level_to_args(MAX_LEVEL),
+ "Contrast": enhance_level_to_args(MAX_LEVEL),
+ "Brightness": enhance_level_to_args(MAX_LEVEL),
+ "Sharpness": enhance_level_to_args(MAX_LEVEL),
+ "ShearX": shear_level_to_args(MAX_LEVEL, replace_value),
+ "TranslateX": translate_level_to_args(translate_const, MAX_LEVEL, replace_value),
+ "TranslateY": translate_level_to_args(translate_const, MAX_LEVEL, replace_value),
+ "Posterize": posterize_level_to_args(MAX_LEVEL),
+ "ShearY": shear_level_to_args(MAX_LEVEL, replace_value),
+}
+
+
+class RandomAugment(object):
+ def __init__(self, N=2, M=10, isPIL=False, augs=[]):
+ self.N = N
+ self.M = M
+ self.isPIL = isPIL
+ if augs:
+ self.augs = augs
+ else:
+ self.augs = list(arg_dict.keys())
+
+ def get_random_ops(self):
+ sampled_ops = np.random.choice(self.augs, self.N)
+ return [(op, 0.5, self.M) for op in sampled_ops]
+
+ def __call__(self, img):
+ if self.isPIL:
+ img = np.array(img)
+ ops = self.get_random_ops()
+ for name, prob, level in ops:
+ if np.random.random() > prob:
+ continue
+ args = arg_dict[name](level)
+ img = func_dict[name](img, *args)
+ return img
+
+
+class VideoRandomAugment(object):
+ def __init__(self, N=2, M=10, p=0.0, tensor_in_tensor_out=True, augs=[]):
+ self.N = N
+ self.M = M
+ self.p = p
+ self.tensor_in_tensor_out = tensor_in_tensor_out
+ if augs:
+ self.augs = augs
+ else:
+ self.augs = list(arg_dict.keys())
+
+ def get_random_ops(self):
+ sampled_ops = np.random.choice(self.augs, self.N, replace=False)
+ return [(op, self.M) for op in sampled_ops]
+
+ def __call__(self, frames):
+ assert (
+ frames.shape[-1] == 3
+ ), "Expecting last dimension for 3-channels RGB (b, h, w, c)."
+
+ if self.tensor_in_tensor_out:
+ frames = frames.numpy().astype(np.uint8)
+
+ num_frames = frames.shape[0]
+
+ ops = num_frames * [self.get_random_ops()]
+ apply_or_not = num_frames * [np.random.random(size=self.N) > self.p]
+
+ frames = torch.stack(
+ list(map(self._aug, frames, ops, apply_or_not)), dim=0
+ ).float()
+
+ return frames
+
+ def _aug(self, img, ops, apply_or_not):
+ for i, (name, level) in enumerate(ops):
+ if not apply_or_not[i]:
+ continue
+ args = arg_dict[name](level)
+ img = func_dict[name](img, *args)
+ return torch.from_numpy(img)
+
+
+if __name__ == "__main__":
+ a = RandomAugment()
+ img = np.random.randn(32, 32, 3)
+ a(img)
diff --git a/global_local/processors/transforms_video.py b/global_local/processors/transforms_video.py
new file mode 100644
index 0000000000000000000000000000000000000000..2e737d61624a1593d0092946ff4768a91718e2ef
--- /dev/null
+++ b/global_local/processors/transforms_video.py
@@ -0,0 +1,179 @@
+#!/usr/bin/env python3
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+
+import numbers
+import random
+
+from torchvision.transforms import (
+ RandomCrop,
+ RandomResizedCrop,
+)
+
+import global_local.processors.functional_video as F
+
+
+__all__ = [
+ "RandomCropVideo",
+ "RandomResizedCropVideo",
+ "CenterCropVideo",
+ "NormalizeVideo",
+ "ToTensorVideo",
+ "RandomHorizontalFlipVideo",
+]
+
+
+class RandomCropVideo(RandomCrop):
+ def __init__(self, size):
+ if isinstance(size, numbers.Number):
+ self.size = (int(size), int(size))
+ else:
+ self.size = size
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: randomly cropped/resized video clip.
+ size is (C, T, OH, OW)
+ """
+ i, j, h, w = self.get_params(clip, self.size)
+ return F.crop(clip, i, j, h, w)
+
+ def __repr__(self) -> str:
+ return f"{self.__class__.__name__}(size={self.size})"
+
+
+class RandomResizedCropVideo(RandomResizedCrop):
+ def __init__(
+ self,
+ size,
+ scale=(0.08, 1.0),
+ ratio=(3.0 / 4.0, 4.0 / 3.0),
+ interpolation_mode="bilinear",
+ ):
+ if isinstance(size, tuple):
+ if len(size) != 2:
+ raise ValueError(
+ f"size should be tuple (height, width), instead got {size}"
+ )
+ self.size = size
+ else:
+ self.size = (size, size)
+
+ self.interpolation_mode = interpolation_mode
+ self.scale = scale
+ self.ratio = ratio
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: randomly cropped/resized video clip.
+ size is (C, T, H, W)
+ """
+ i, j, h, w = self.get_params(clip, self.scale, self.ratio)
+ return F.resized_crop(clip, i, j, h, w, self.size, self.interpolation_mode)
+
+ def __repr__(self) -> str:
+ return f"{self.__class__.__name__}(size={self.size}, interpolation_mode={self.interpolation_mode}, scale={self.scale}, ratio={self.ratio})"
+
+
+class CenterCropVideo:
+ def __init__(self, crop_size):
+ if isinstance(crop_size, numbers.Number):
+ self.crop_size = (int(crop_size), int(crop_size))
+ else:
+ self.crop_size = crop_size
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: central cropping of video clip. Size is
+ (C, T, crop_size, crop_size)
+ """
+ return F.center_crop(clip, self.crop_size)
+
+ def __repr__(self) -> str:
+ return f"{self.__class__.__name__}(crop_size={self.crop_size})"
+
+
+class NormalizeVideo:
+ """
+ Normalize the video clip by mean subtraction and division by standard deviation
+ Args:
+ mean (3-tuple): pixel RGB mean
+ std (3-tuple): pixel RGB standard deviation
+ inplace (boolean): whether do in-place normalization
+ """
+
+ def __init__(self, mean, std, inplace=False):
+ self.mean = mean
+ self.std = std
+ self.inplace = inplace
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): video clip to be normalized. Size is (C, T, H, W)
+ """
+ return F.normalize(clip, self.mean, self.std, self.inplace)
+
+ def __repr__(self) -> str:
+ return f"{self.__class__.__name__}(mean={self.mean}, std={self.std}, inplace={self.inplace})"
+
+
+class ToTensorVideo:
+ """
+ Convert tensor data type from uint8 to float, divide value by 255.0 and
+ permute the dimensions of clip tensor
+ """
+
+ def __init__(self):
+ pass
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor, dtype=torch.uint8): Size is (T, H, W, C)
+ Return:
+ clip (torch.tensor, dtype=torch.float): Size is (C, T, H, W)
+ """
+ return F.to_tensor(clip)
+
+ def __repr__(self) -> str:
+ return self.__class__.__name__
+
+
+class RandomHorizontalFlipVideo:
+ """
+ Flip the video clip along the horizonal direction with a given probability
+ Args:
+ p (float): probability of the clip being flipped. Default value is 0.5
+ """
+
+ def __init__(self, p=0.5):
+ self.p = p
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): Size is (C, T, H, W)
+ Return:
+ clip (torch.tensor): Size is (C, T, H, W)
+ """
+ if random.random() < self.p:
+ clip = F.hflip(clip)
+ return clip
+
+ def __repr__(self) -> str:
+ return f"{self.__class__.__name__}(p={self.p})"
diff --git a/global_local/processors/video_processor.py b/global_local/processors/video_processor.py
new file mode 100644
index 0000000000000000000000000000000000000000..80dd863b4c8a87f058e85ff8ec8d0025531ed118
--- /dev/null
+++ b/global_local/processors/video_processor.py
@@ -0,0 +1,237 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import torch
+from global_local.common.registry import registry
+from decord import VideoReader
+import decord
+import numpy as np
+from global_local.processors import transforms_video
+from global_local.processors.base_processor import BaseProcessor
+from global_local.processors.randaugment import VideoRandomAugment
+from global_local.processors import functional_video as F
+from omegaconf import OmegaConf
+from torchvision import transforms
+import random as rnd
+
+
+MAX_INT = registry.get("MAX_INT")
+decord.bridge.set_bridge("torch")
+
+def load_video(video_path, n_frms=MAX_INT, height=-1, width=-1, sampling="uniform", return_msg = False):
+ decord.bridge.set_bridge("torch")
+ vr = VideoReader(uri=video_path, height=height, width=width)
+
+ vlen = len(vr)
+ start, end = 0, vlen
+
+ n_frms = min(n_frms, vlen)
+
+ if sampling == "uniform":
+ indices = np.arange(start, end, vlen / n_frms).astype(int).tolist()
+ elif sampling == "headtail":
+ indices_h = sorted(rnd.sample(range(vlen // 2), n_frms // 2))
+ indices_t = sorted(rnd.sample(range(vlen // 2, vlen), n_frms // 2))
+ indices = indices_h + indices_t
+ else:
+ raise NotImplementedError
+
+ # get_batch -> T, H, W, C
+ temp_frms = vr.get_batch(indices)
+ # print(type(temp_frms))
+ tensor_frms = torch.from_numpy(temp_frms) if type(temp_frms) is not torch.Tensor else temp_frms
+ frms = tensor_frms.permute(3, 0, 1, 2).float() # (C, T, H, W)
+
+ if not return_msg:
+ return frms
+
+ fps = float(vr.get_avg_fps())
+ sec = ", ".join([str(round(f / fps, 1)) for f in indices])
+ # " " should be added in the start and end
+ msg = f"The video contains {len(indices)} frames sampled at {sec} seconds. "
+ return frms, msg
+
+
+class AlproVideoBaseProcessor(BaseProcessor):
+ def __init__(self, mean=None, std=None, n_frms=MAX_INT):
+ if mean is None:
+ mean = (0.48145466, 0.4578275, 0.40821073)
+ if std is None:
+ std = (0.26862954, 0.26130258, 0.27577711)
+
+ self.normalize = transforms_video.NormalizeVideo(mean, std)
+
+ self.n_frms = n_frms
+
+
+class ToUint8(object):
+ def __init__(self):
+ pass
+
+ def __call__(self, tensor):
+ return tensor.to(torch.uint8)
+
+ def __repr__(self):
+ return self.__class__.__name__
+
+
+class ToTHWC(object):
+ """
+ Args:
+ clip (torch.tensor, dtype=torch.uint8): Size is (C, T, H, W)
+ Return:
+ clip (torch.tensor, dtype=torch.float): Size is (T, H, W, C)
+ """
+
+ def __init__(self):
+ pass
+
+ def __call__(self, tensor):
+ return tensor.permute(1, 2, 3, 0)
+
+ def __repr__(self):
+ return self.__class__.__name__
+
+
+class ResizeVideo(object):
+ def __init__(self, target_size, interpolation_mode="bilinear"):
+ self.target_size = target_size
+ self.interpolation_mode = interpolation_mode
+
+ def __call__(self, clip):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: central cropping of video clip. Size is
+ (C, T, crop_size, crop_size)
+ """
+ return F.resize(clip, self.target_size, self.interpolation_mode)
+
+ def __repr__(self):
+ return self.__class__.__name__ + "(resize_size={0})".format(self.target_size)
+
+
+@registry.register_processor("alpro_video_train")
+class AlproVideoTrainProcessor(AlproVideoBaseProcessor):
+ def __init__(
+ self,
+ image_size=384,
+ mean=None,
+ std=None,
+ min_scale=0.5,
+ max_scale=1.0,
+ n_frms=MAX_INT,
+ ):
+ super().__init__(mean=mean, std=std, n_frms=n_frms)
+
+ self.image_size = image_size
+
+ self.transform = transforms.Compose(
+ [
+ # Video size is (C, T, H, W)
+ transforms_video.RandomResizedCropVideo(
+ image_size,
+ scale=(min_scale, max_scale),
+ interpolation_mode="bicubic",
+ ),
+ ToTHWC(), # C, T, H, W -> T, H, W, C
+ ToUint8(),
+ transforms_video.ToTensorVideo(), # T, H, W, C -> C, T, H, W
+ self.normalize,
+ ]
+ )
+
+ def __call__(self, vpath):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: video clip after transforms. Size is (C, T, size, size).
+ """
+ clip = load_video(
+ video_path=vpath,
+ n_frms=self.n_frms,
+ height=self.image_size,
+ width=self.image_size,
+ sampling="headtail",
+ )
+
+ return self.transform(clip)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ image_size = cfg.get("image_size", 256)
+
+ mean = cfg.get("mean", None)
+ std = cfg.get("std", None)
+
+ min_scale = cfg.get("min_scale", 0.5)
+ max_scale = cfg.get("max_scale", 1.0)
+
+ n_frms = cfg.get("n_frms", MAX_INT)
+
+ return cls(
+ image_size=image_size,
+ mean=mean,
+ std=std,
+ min_scale=min_scale,
+ max_scale=max_scale,
+ n_frms=n_frms,
+ )
+
+
+@registry.register_processor("alpro_video_eval")
+class AlproVideoEvalProcessor(AlproVideoBaseProcessor):
+ def __init__(self, image_size=256, mean=None, std=None, n_frms=MAX_INT):
+ super().__init__(mean=mean, std=std, n_frms=n_frms)
+
+ self.image_size = image_size
+
+ # Input video size is (C, T, H, W)
+ self.transform = transforms.Compose(
+ [
+ # frames will be resized during decord loading.
+ ToUint8(), # C, T, H, W
+ ToTHWC(), # T, H, W, C
+ transforms_video.ToTensorVideo(), # C, T, H, W
+ self.normalize, # C, T, H, W
+ ]
+ )
+
+ def __call__(self, vpath):
+ """
+ Args:
+ clip (torch.tensor): Video clip to be cropped. Size is (C, T, H, W)
+ Returns:
+ torch.tensor: video clip after transforms. Size is (C, T, size, size).
+ """
+ clip = load_video(
+ video_path=vpath,
+ n_frms=self.n_frms,
+ height=self.image_size,
+ width=self.image_size,
+ )
+
+ return self.transform(clip)
+
+ @classmethod
+ def from_config(cls, cfg=None):
+ if cfg is None:
+ cfg = OmegaConf.create()
+
+ image_size = cfg.get("image_size", 256)
+
+ mean = cfg.get("mean", None)
+ std = cfg.get("std", None)
+
+ n_frms = cfg.get("n_frms", MAX_INT)
+
+ return cls(image_size=image_size, mean=mean, std=std, n_frms=n_frms)
diff --git a/global_local/runners/__init__.py b/global_local/runners/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..b7cd263a0bac2fe331e221dee247d79e573d7ca0
--- /dev/null
+++ b/global_local/runners/__init__.py
@@ -0,0 +1,10 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from global_local.runners.runner_base import RunnerBase
+
+__all__ = ["RunnerBase"]
diff --git a/global_local/runners/__pycache__/__init__.cpython-39.pyc b/global_local/runners/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..77e5c2f224fb068d5605f0d43ff4c96c9cd458e8
Binary files /dev/null and b/global_local/runners/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/runners/__pycache__/runner_base.cpython-39.pyc b/global_local/runners/__pycache__/runner_base.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..282221482ef8562736ae6bd1ba143141363a9cf6
Binary files /dev/null and b/global_local/runners/__pycache__/runner_base.cpython-39.pyc differ
diff --git a/global_local/runners/runner_base.py b/global_local/runners/runner_base.py
new file mode 100644
index 0000000000000000000000000000000000000000..378551d887932447a559ae0988b579dd82b764ec
--- /dev/null
+++ b/global_local/runners/runner_base.py
@@ -0,0 +1,658 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import datetime
+import json
+import logging
+import os
+import time
+from pathlib import Path
+
+import torch
+import torch.distributed as dist
+import webdataset as wds
+from global_local.common.dist_utils import (
+ download_cached_file,
+ get_rank,
+ get_world_size,
+ is_main_process,
+ main_process,
+)
+from global_local.common.registry import registry
+from global_local.common.utils import is_url
+from global_local.datasets.data_utils import concat_datasets, reorg_datasets_by_split, ChainDataset
+from global_local.datasets.datasets.dataloader_utils import (
+ IterLoader,
+ MultiIterLoader,
+ PrefetchLoader,
+)
+from torch.nn.parallel import DistributedDataParallel as DDP
+from torch.utils.data import DataLoader, DistributedSampler
+
+
+@registry.register_runner("runner_base")
+class RunnerBase:
+ """
+ A runner class to train and evaluate a model given a task and datasets.
+
+ The runner uses pytorch distributed data parallel by default. Future release
+ will support other distributed frameworks.
+ """
+
+ def __init__(self, cfg, task, model, datasets, job_id):
+ self.config = cfg
+ self.job_id = job_id
+
+ self.task = task
+ self.datasets = datasets
+
+ self._model = model
+
+ self._wrapped_model = None
+ self._device = None
+ self._optimizer = None
+ self._scaler = None
+ self._dataloaders = None
+ self._lr_sched = None
+
+ self.start_epoch = 0
+
+ # self.setup_seeds()
+ self.setup_output_dir()
+
+ @property
+ def device(self):
+ if self._device is None:
+ self._device = torch.device(self.config.run_cfg.device)
+
+ return self._device
+
+ @property
+ def use_distributed(self):
+ return self.config.run_cfg.distributed
+
+ @property
+ def model(self):
+ """
+ A property to get the DDP-wrapped model on the device.
+ """
+ # move model to device
+ if self._model.device != self.device:
+ self._model = self._model.to(self.device)
+
+ # distributed training wrapper
+ if self.use_distributed:
+ if self._wrapped_model is None:
+ self._wrapped_model = DDP(
+ self._model, device_ids=[self.config.run_cfg.gpu]
+ )
+ else:
+ self._wrapped_model = self._model
+
+ return self._wrapped_model
+
+ @property
+ def optimizer(self):
+ # TODO make optimizer class and configurations
+ if self._optimizer is None:
+ num_parameters = 0
+ p_wd, p_non_wd = [], []
+ for n, p in self.model.named_parameters():
+ if not p.requires_grad:
+ continue # frozen weights
+ print(n)
+ if p.ndim < 2 or "bias" in n or "ln" in n or "bn" in n:
+ p_non_wd.append(p)
+ else:
+ p_wd.append(p)
+ num_parameters += p.data.nelement()
+ logging.info("number of trainable parameters: %d" % num_parameters)
+ optim_params = [
+ {
+ "params": p_wd,
+ "weight_decay": float(self.config.run_cfg.weight_decay),
+ },
+ {"params": p_non_wd, "weight_decay": 0},
+ ]
+ beta2 = self.config.run_cfg.get("beta2", 0.999)
+ self._optimizer = torch.optim.AdamW(
+ optim_params,
+ lr=float(self.config.run_cfg.init_lr),
+ weight_decay=float(self.config.run_cfg.weight_decay),
+ betas=(0.9, beta2),
+ )
+
+ return self._optimizer
+
+ @property
+ def scaler(self):
+ amp = self.config.run_cfg.get("amp", False)
+
+ if amp:
+ if self._scaler is None:
+ self._scaler = torch.cuda.amp.GradScaler()
+
+ return self._scaler
+
+ @property
+ def lr_scheduler(self):
+ """
+ A property to get and create learning rate scheduler by split just in need.
+ """
+ if self._lr_sched is None:
+ lr_sched_cls = registry.get_lr_scheduler_class(self.config.run_cfg.lr_sched)
+
+ # max_epoch = self.config.run_cfg.max_epoch
+ max_epoch = self.max_epoch
+ # min_lr = self.config.run_cfg.min_lr
+ min_lr = self.min_lr
+ # init_lr = self.config.run_cfg.init_lr
+ init_lr = self.init_lr
+
+ # optional parameters
+ decay_rate = self.config.run_cfg.get("lr_decay_rate", None)
+ warmup_start_lr = self.config.run_cfg.get("warmup_lr", -1)
+ warmup_steps = self.config.run_cfg.get("warmup_steps", 0)
+ iters_per_epoch = self.config.run_cfg.get("iters_per_epoch", None)
+
+ if iters_per_epoch is None:
+ try:
+ iters_per_epoch = len(self.dataloaders['train'])
+ except (AttributeError, TypeError):
+ iters_per_epoch = 10000
+
+ self._lr_sched = lr_sched_cls(
+ optimizer=self.optimizer,
+ max_epoch=max_epoch,
+ iters_per_epoch=iters_per_epoch,
+ min_lr=min_lr,
+ init_lr=init_lr,
+ decay_rate=decay_rate,
+ warmup_start_lr=warmup_start_lr,
+ warmup_steps=warmup_steps,
+ )
+
+ return self._lr_sched
+
+ @property
+ def dataloaders(self) -> dict:
+ """
+ A property to get and create dataloaders by split just in need.
+
+ If no train_dataset_ratio is provided, concatenate map-style datasets and
+ chain wds.DataPipe datasets separately. Training set becomes a tuple
+ (ConcatDataset, ChainDataset), both are optional but at least one of them is
+ required. The resultant ConcatDataset and ChainDataset will be sampled evenly.
+
+ If train_dataset_ratio is provided, create a MultiIterLoader to sample
+ each dataset by ratios during training.
+
+ Currently do not support multiple datasets for validation and test.
+
+ Returns:
+ dict: {split_name: (tuples of) dataloader}
+ """
+ if self._dataloaders is None:
+
+ # concatenate map-style datasets and chain wds.DataPipe datasets separately
+ # training set becomes a tuple (ConcatDataset, ChainDataset), both are
+ # optional but at least one of them is required. The resultant ConcatDataset
+ # and ChainDataset will be sampled evenly.
+ logging.info(
+ "dataset_ratios not specified, datasets will be concatenated (map-style datasets) or chained (webdataset.DataPipeline)."
+ )
+
+ datasets = reorg_datasets_by_split(self.datasets)
+ self.datasets = datasets
+ # self.datasets = concat_datasets(datasets)
+
+ # print dataset statistics after concatenation/chaining
+ for split_name in self.datasets:
+ if isinstance(self.datasets[split_name], tuple) or isinstance(
+ self.datasets[split_name], list
+ ):
+ # mixed wds.DataPipeline and torch.utils.data.Dataset
+ num_records = sum(
+ [
+ len(d)
+ if not type(d) in [wds.DataPipeline, ChainDataset]
+ else 0
+ for d in self.datasets[split_name]
+ ]
+ )
+
+ else:
+ if hasattr(self.datasets[split_name], "__len__"):
+ # a single map-style dataset
+ num_records = len(self.datasets[split_name])
+ else:
+ # a single wds.DataPipeline
+ num_records = -1
+ logging.info(
+ "Only a single wds.DataPipeline dataset, no __len__ attribute."
+ )
+
+ if num_records >= 0:
+ logging.info(
+ "Loaded {} records for {} split from the dataset.".format(
+ num_records, split_name
+ )
+ )
+
+ # create dataloaders
+ split_names = sorted(self.datasets.keys())
+
+ datasets = [self.datasets[split] for split in split_names]
+ is_trains = [split in self.train_splits for split in split_names]
+
+ batch_sizes = [
+ self.config.run_cfg.batch_size_train
+ if split == "train"
+ else self.config.run_cfg.batch_size_eval
+ for split in split_names
+ ]
+
+ collate_fns = []
+ for dataset in datasets:
+ if isinstance(dataset, tuple) or isinstance(dataset, list):
+ collate_fns.append([getattr(d, "collater", None) for d in dataset])
+ else:
+ collate_fns.append(getattr(dataset, "collater", None))
+
+ dataloaders = self.create_loaders(
+ datasets=datasets,
+ num_workers=self.config.run_cfg.num_workers,
+ batch_sizes=batch_sizes,
+ is_trains=is_trains,
+ collate_fns=collate_fns,
+ )
+
+ self._dataloaders = {k: v for k, v in zip(split_names, dataloaders)}
+
+ return self._dataloaders
+
+ @property
+ def cuda_enabled(self):
+ return self.device.type == "cuda"
+
+ @property
+ def max_epoch(self):
+ return int(self.config.run_cfg.max_epoch)
+
+ @property
+ def log_freq(self):
+ log_freq = self.config.run_cfg.get("log_freq", 50)
+ return int(log_freq)
+
+ @property
+ def init_lr(self):
+ return float(self.config.run_cfg.init_lr)
+
+ @property
+ def min_lr(self):
+ return float(self.config.run_cfg.min_lr)
+
+ @property
+ def accum_grad_iters(self):
+ return int(self.config.run_cfg.get("accum_grad_iters", 1))
+
+ @property
+ def valid_splits(self):
+ valid_splits = self.config.run_cfg.get("valid_splits", [])
+
+ if len(valid_splits) == 0:
+ logging.info("No validation splits found.")
+
+ return valid_splits
+
+ @property
+ def test_splits(self):
+ test_splits = self.config.run_cfg.get("test_splits", [])
+
+ return test_splits
+
+ @property
+ def train_splits(self):
+ train_splits = self.config.run_cfg.get("train_splits", [])
+
+ if len(train_splits) == 0:
+ logging.info("Empty train splits.")
+
+ return train_splits
+
+ @property
+ def evaluate_only(self):
+ """
+ Set to True to skip training.
+ """
+ return self.config.run_cfg.evaluate
+
+ @property
+ def use_dist_eval_sampler(self):
+ return self.config.run_cfg.get("use_dist_eval_sampler", True)
+
+ @property
+ def resume_ckpt_path(self):
+ return self.config.run_cfg.get("resume_ckpt_path", None)
+
+ @property
+ def train_loader(self):
+ train_dataloader = self.dataloaders["train"]
+
+ return train_dataloader
+
+ def setup_output_dir(self):
+ lib_root = Path(registry.get_path("library_root"))
+
+ output_dir = lib_root / self.config.run_cfg.output_dir / self.job_id
+ result_dir = output_dir / "result"
+
+ output_dir.mkdir(parents=True, exist_ok=True)
+ result_dir.mkdir(parents=True, exist_ok=True)
+
+ registry.register_path("result_dir", str(result_dir))
+ registry.register_path("output_dir", str(output_dir))
+
+ self.result_dir = result_dir
+ self.output_dir = output_dir
+
+ def train(self):
+ start_time = time.time()
+ best_agg_metric = 0
+ best_epoch = 0
+
+ self.log_config()
+
+ # resume from checkpoint if specified
+ if not self.evaluate_only and self.resume_ckpt_path is not None:
+ self._load_checkpoint(self.resume_ckpt_path)
+
+ for cur_epoch in range(self.start_epoch, self.max_epoch):
+ # training phase
+ if not self.evaluate_only:
+ logging.info("Start training")
+ train_stats = self.train_epoch(cur_epoch)
+ self.log_stats(split_name="train", stats=train_stats)
+
+ # evaluation phase
+ if len(self.valid_splits) > 0:
+ for split_name in self.valid_splits:
+ logging.info("Evaluating on {}.".format(split_name))
+
+ val_log = self.eval_epoch(
+ split_name=split_name, cur_epoch=cur_epoch
+ )
+ if val_log is not None:
+ if is_main_process():
+ assert (
+ "agg_metrics" in val_log
+ ), "No agg_metrics found in validation log."
+
+ agg_metrics = val_log["agg_metrics"]
+ if agg_metrics > best_agg_metric and split_name == "val":
+ best_epoch, best_agg_metric = cur_epoch, agg_metrics
+
+ self._save_checkpoint(cur_epoch, is_best=True)
+
+ val_log.update({"best_epoch": best_epoch})
+ self.log_stats(val_log, split_name)
+
+ else:
+ # if no validation split is provided, we just save the checkpoint at the end of each epoch.
+ if not self.evaluate_only:
+ self._save_checkpoint(cur_epoch, is_best=False)
+
+ if self.evaluate_only:
+ break
+
+ if self.config.run_cfg.distributed:
+ dist.barrier()
+
+ # testing phase
+ test_epoch = "best" if len(self.valid_splits) > 0 else cur_epoch
+ self.evaluate(cur_epoch=test_epoch, skip_reload=self.evaluate_only)
+
+ total_time = time.time() - start_time
+ total_time_str = str(datetime.timedelta(seconds=int(total_time)))
+ logging.info("Training time {}".format(total_time_str))
+
+ def evaluate(self, cur_epoch="best", skip_reload=False):
+ test_logs = dict()
+
+ if len(self.test_splits) > 0:
+ for split_name in self.test_splits:
+ test_logs[split_name] = self.eval_epoch(
+ split_name=split_name, cur_epoch=cur_epoch, skip_reload=skip_reload
+ )
+
+ return test_logs
+
+ def train_epoch(self, epoch):
+ # train
+ self.model.train()
+
+ return self.task.train_epoch(
+ epoch=epoch,
+ model=self.model,
+ data_loader=self.train_loader,
+ optimizer=self.optimizer,
+ scaler=self.scaler,
+ lr_scheduler=self.lr_scheduler,
+ cuda_enabled=self.cuda_enabled,
+ log_freq=self.log_freq,
+ accum_grad_iters=self.accum_grad_iters,
+ )
+
+ @torch.no_grad()
+ def eval_epoch(self, split_name, cur_epoch, skip_reload=False):
+ """
+ Evaluate the model on a given split.
+
+ Args:
+ split_name (str): name of the split to evaluate on.
+ cur_epoch (int): current epoch.
+ skip_reload_best (bool): whether to skip reloading the best checkpoint.
+ During training, we will reload the best checkpoint for validation.
+ During testing, we will use provided weights and skip reloading the best checkpoint .
+ """
+ data_loader = self.dataloaders.get(split_name, None)
+ assert data_loader, "data_loader for split {} is None.".format(split_name)
+
+ # TODO In validation, you need to compute loss as well as metrics
+ # TODO consider moving to model.before_evaluation()
+ model = self.unwrap_dist_model(self.model)
+ if not skip_reload and cur_epoch == "best":
+ model = self._reload_best_model(model)
+ model.eval()
+
+ self.task.before_evaluation(
+ model=model,
+ dataset=self.datasets[split_name],
+ )
+ results = self.task.evaluation(model, data_loader)
+
+ if results is not None:
+ return self.task.after_evaluation(
+ val_result=results,
+ split_name=split_name,
+ epoch=cur_epoch,
+ )
+
+ def unwrap_dist_model(self, model):
+ if self.use_distributed:
+ return model.module
+ else:
+ return model
+
+ def create_loaders(
+ self,
+ datasets,
+ num_workers,
+ batch_sizes,
+ is_trains,
+ collate_fns,
+ dataset_ratios=None,
+ ):
+ """
+ Create dataloaders for training and validation.
+ """
+
+ def _create_loader(dataset, num_workers, bsz, is_train, collate_fn):
+ # create a single dataloader for each split
+ if isinstance(dataset, ChainDataset) or isinstance(
+ dataset, wds.DataPipeline
+ ):
+ # wds.WebdDataset instance are chained together
+ # webdataset.DataPipeline has its own sampler and collate_fn
+ loader = iter(
+ DataLoader(
+ dataset,
+ batch_size=bsz,
+ num_workers=num_workers,
+ pin_memory=True,
+ )
+ )
+ else:
+ # map-style dataset are concatenated together
+ # setup distributed sampler
+ if self.use_distributed:
+ sampler = DistributedSampler(
+ dataset,
+ shuffle=is_train,
+ num_replicas=get_world_size(),
+ rank=get_rank(),
+ )
+ if not self.use_dist_eval_sampler:
+ # e.g. retrieval evaluation
+ sampler = sampler if is_train else None
+ else:
+ sampler = None
+
+ loader = DataLoader(
+ dataset,
+ batch_size=bsz,
+ num_workers=num_workers,
+ pin_memory=True,
+ sampler=sampler,
+ shuffle=sampler is None and is_train,
+ collate_fn=collate_fn,
+ drop_last=True if is_train else False,
+ )
+ loader = PrefetchLoader(loader)
+
+ if is_train:
+ loader = IterLoader(loader, use_distributed=self.use_distributed)
+
+ return loader
+
+ loaders = []
+
+ for dataset, bsz, is_train, collate_fn in zip(
+ datasets, batch_sizes, is_trains, collate_fns
+ ):
+ if isinstance(dataset, list) or isinstance(dataset, tuple):
+ if hasattr(dataset[0], 'sample_ratio') and dataset_ratios is None:
+ dataset_ratios = [d.sample_ratio for d in dataset]
+ loader = MultiIterLoader(
+ loaders=[
+ _create_loader(d, num_workers, bsz, is_train, collate_fn[i])
+ for i, d in enumerate(dataset)
+ ],
+ ratios=dataset_ratios,
+ )
+ else:
+ loader = _create_loader(dataset, num_workers, bsz, is_train, collate_fn)
+
+ loaders.append(loader)
+
+ return loaders
+
+ @main_process
+ def _save_checkpoint(self, cur_epoch, is_best=False):
+ """
+ Save the checkpoint at the current epoch.
+ """
+ model_no_ddp = self.unwrap_dist_model(self.model)
+ param_grad_dic = {
+ k: v.requires_grad for (k, v) in model_no_ddp.named_parameters()
+ }
+ state_dict = model_no_ddp.state_dict()
+ for k in list(state_dict.keys()):
+ if k in param_grad_dic.keys() and not param_grad_dic[k]:
+ # delete parameters that do not require gradient
+ del state_dict[k]
+ save_obj = {
+ "model": state_dict,
+ "optimizer": self.optimizer.state_dict(),
+ "config": self.config.to_dict(),
+ "scaler": self.scaler.state_dict() if self.scaler else None,
+ "epoch": cur_epoch,
+ }
+ save_to = os.path.join(
+ self.output_dir,
+ "checkpoint_{}.pth".format("best" if is_best else cur_epoch),
+ )
+ logging.info("Saving checkpoint at epoch {} to {}.".format(cur_epoch, save_to))
+ torch.save(save_obj, save_to)
+
+ def _reload_best_model(self, model):
+ """
+ Load the best checkpoint for evaluation.
+ """
+ checkpoint_path = os.path.join(self.output_dir, "checkpoint_best.pth")
+
+ logging.info("Loading checkpoint from {}.".format(checkpoint_path))
+ checkpoint = torch.load(checkpoint_path, map_location="cpu")
+ try:
+ model.load_state_dict(checkpoint["model"])
+ except RuntimeError as e:
+ logging.warning(
+ """
+ Key mismatch when loading checkpoint. This is expected if only part of the model is saved.
+ Trying to load the model with strict=False.
+ """
+ )
+ model.load_state_dict(checkpoint["model"], strict=False)
+ return model
+
+ def _load_checkpoint(self, url_or_filename):
+ """
+ Resume from a checkpoint.
+ """
+ if is_url(url_or_filename):
+ cached_file = download_cached_file(
+ url_or_filename, check_hash=False, progress=True
+ )
+ checkpoint = torch.load(cached_file, map_location=self.device, strict=False)
+ elif os.path.isfile(url_or_filename):
+ checkpoint = torch.load(url_or_filename, map_location=self.device, strict=False)
+ else:
+ raise RuntimeError("checkpoint url or path is invalid")
+
+ state_dict = checkpoint["model"]
+ self.unwrap_dist_model(self.model).load_state_dict(state_dict)
+
+ self.optimizer.load_state_dict(checkpoint["optimizer"])
+ if self.scaler and "scaler" in checkpoint:
+ self.scaler.load_state_dict(checkpoint["scaler"])
+
+ self.start_epoch = checkpoint["epoch"] + 1
+ logging.info("Resume checkpoint from {}".format(url_or_filename))
+
+ @main_process
+ def log_stats(self, stats, split_name):
+ if isinstance(stats, dict):
+ log_stats = {**{f"{split_name}_{k}": v for k, v in stats.items()}}
+ with open(os.path.join(self.output_dir, "log.txt"), "a") as f:
+ f.write(json.dumps(log_stats) + "\n")
+ elif isinstance(stats, list):
+ pass
+
+ @main_process
+ def log_config(self):
+ with open(os.path.join(self.output_dir, "log.txt"), "a") as f:
+ f.write(json.dumps(self.config.to_dict(), indent=4) + "\n")
diff --git a/global_local/runners/test.py b/global_local/runners/test.py
new file mode 100644
index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391
diff --git a/global_local/tasks/__init__.py b/global_local/tasks/__init__.py
new file mode 100644
index 0000000000000000000000000000000000000000..ab75be16c5054cc75753a43482a776cc3564026b
--- /dev/null
+++ b/global_local/tasks/__init__.py
@@ -0,0 +1,28 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from global_local.common.registry import registry
+from global_local.tasks.base_task import BaseTask
+from global_local.tasks.image_text_pretrain import ImageTextPretrainTask
+from global_local.tasks.video_text_pretrain import VideoTextPretrainTask
+
+
+def setup_task(cfg):
+ assert "task" in cfg.run_cfg, "Task name must be provided."
+
+ task_name = cfg.run_cfg.task
+ task = registry.get_task_class(task_name).setup_task(cfg=cfg)
+ assert task is not None, "Task {} not properly registered.".format(task_name)
+
+ return task
+
+
+__all__ = [
+ "BaseTask",
+ "ImageTextPretrainTask",
+ "VideoTextPretrainTask"
+]
diff --git a/global_local/tasks/__pycache__/__init__.cpython-39.pyc b/global_local/tasks/__pycache__/__init__.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..0e744db3cce3bde426a7ec0a78a094eb5fc5ac7f
Binary files /dev/null and b/global_local/tasks/__pycache__/__init__.cpython-39.pyc differ
diff --git a/global_local/tasks/__pycache__/base_task.cpython-39.pyc b/global_local/tasks/__pycache__/base_task.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..70860c456f014a4902e8bcb4f32c45a8d1494c5d
Binary files /dev/null and b/global_local/tasks/__pycache__/base_task.cpython-39.pyc differ
diff --git a/global_local/tasks/__pycache__/image_text_pretrain.cpython-39.pyc b/global_local/tasks/__pycache__/image_text_pretrain.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..4d746bf57b2a84bf327b7649eeaac97dec3b20ca
Binary files /dev/null and b/global_local/tasks/__pycache__/image_text_pretrain.cpython-39.pyc differ
diff --git a/global_local/tasks/__pycache__/video_text_pretrain.cpython-39.pyc b/global_local/tasks/__pycache__/video_text_pretrain.cpython-39.pyc
new file mode 100644
index 0000000000000000000000000000000000000000..865b05c2f6044698011e99dd0884e59959ca6490
Binary files /dev/null and b/global_local/tasks/__pycache__/video_text_pretrain.cpython-39.pyc differ
diff --git a/global_local/tasks/base_task.py b/global_local/tasks/base_task.py
new file mode 100644
index 0000000000000000000000000000000000000000..6f18d48d9e074d3aea37f0bcc473c6ffcf70fa22
--- /dev/null
+++ b/global_local/tasks/base_task.py
@@ -0,0 +1,286 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+import logging
+import os
+
+import torch
+import torch.distributed as dist
+from global_local.common.dist_utils import get_rank, get_world_size, is_main_process, is_dist_avail_and_initialized
+from global_local.common.logger import MetricLogger, SmoothedValue
+from global_local.common.registry import registry
+from global_local.datasets.data_utils import prepare_sample
+
+
+class BaseTask:
+ def __init__(self, **kwargs):
+ super().__init__()
+
+ self.inst_id_key = "instance_id"
+
+ @classmethod
+ def setup_task(cls, **kwargs):
+ return cls()
+
+ def build_model(self, cfg):
+ model_config = cfg.model_cfg
+
+ model_cls = registry.get_model_class(model_config.arch)
+ return model_cls.from_config(model_config)
+
+ def build_datasets(self, cfg):
+ """
+ Build a dictionary of datasets, keyed by split 'train', 'valid', 'test'.
+ Download dataset and annotations automatically if not exist.
+
+ Args:
+ cfg (common.config.Config): _description_
+
+ Returns:
+ dict: Dictionary of torch.utils.data.Dataset objects by split.
+ """
+
+ datasets = dict()
+
+ datasets_config = cfg.datasets_cfg
+
+ assert len(datasets_config) > 0, "At least one dataset has to be specified."
+
+ for name in datasets_config:
+ dataset_config = datasets_config[name]
+
+ builder = registry.get_builder_class(name)(dataset_config)
+ dataset = builder.build_datasets()
+
+ dataset['train'].name = name
+ if 'sample_ratio' in dataset_config:
+ dataset['train'].sample_ratio = dataset_config.sample_ratio
+
+ datasets[name] = dataset
+
+ return datasets
+
+ def train_step(self, model, samples):
+ loss = model(samples)["loss"]
+ return loss
+
+ def valid_step(self, model, samples):
+ raise NotImplementedError
+
+ def before_evaluation(self, model, dataset, **kwargs):
+ model.before_evaluation(dataset=dataset, task_type=type(self))
+
+ def after_evaluation(self, **kwargs):
+ pass
+
+ def inference_step(self):
+ raise NotImplementedError
+
+ def evaluation(self, model, data_loader, cuda_enabled=True):
+ metric_logger = MetricLogger(delimiter=" ")
+ header = "Evaluation"
+ # TODO make it configurable
+ print_freq = 10
+
+ results = []
+
+ for samples in metric_logger.log_every(data_loader, print_freq, header):
+ samples = prepare_sample(samples, cuda_enabled=cuda_enabled)
+
+ eval_output = self.valid_step(model=model, samples=samples)
+ results.extend(eval_output)
+
+ if is_dist_avail_and_initialized():
+ dist.barrier()
+
+ return results
+
+ def train_epoch(
+ self,
+ epoch,
+ model,
+ data_loader,
+ optimizer,
+ lr_scheduler,
+ scaler=None,
+ cuda_enabled=False,
+ log_freq=50,
+ accum_grad_iters=1,
+ ):
+ return self._train_inner_loop(
+ epoch=epoch,
+ iters_per_epoch=lr_scheduler.iters_per_epoch,
+ model=model,
+ data_loader=data_loader,
+ optimizer=optimizer,
+ scaler=scaler,
+ lr_scheduler=lr_scheduler,
+ log_freq=log_freq,
+ cuda_enabled=cuda_enabled,
+ accum_grad_iters=accum_grad_iters,
+ )
+
+ def train_iters(
+ self,
+ epoch,
+ start_iters,
+ iters_per_inner_epoch,
+ model,
+ data_loader,
+ optimizer,
+ lr_scheduler,
+ scaler=None,
+ cuda_enabled=False,
+ log_freq=50,
+ accum_grad_iters=1,
+ ):
+ return self._train_inner_loop(
+ epoch=epoch,
+ start_iters=start_iters,
+ iters_per_epoch=iters_per_inner_epoch,
+ model=model,
+ data_loader=data_loader,
+ optimizer=optimizer,
+ scaler=scaler,
+ lr_scheduler=lr_scheduler,
+ log_freq=log_freq,
+ cuda_enabled=cuda_enabled,
+ accum_grad_iters=accum_grad_iters,
+ )
+
+ def _train_inner_loop(
+ self,
+ epoch,
+ iters_per_epoch,
+ model,
+ data_loader,
+ optimizer,
+ lr_scheduler,
+ scaler=None,
+ start_iters=None,
+ log_freq=50,
+ cuda_enabled=False,
+ accum_grad_iters=1,
+ ):
+ """
+ An inner training loop compatible with both epoch-based and iter-based training.
+
+ When using epoch-based, training stops after one epoch; when using iter-based,
+ training stops after #iters_per_epoch iterations.
+ """
+ use_amp = scaler is not None
+
+ if not hasattr(data_loader, "__next__"):
+ # convert to iterator if not already
+ data_loader = iter(data_loader)
+
+ metric_logger = MetricLogger(delimiter=" ")
+ metric_logger.add_meter("lr", SmoothedValue(window_size=1, fmt="{value:.6f}"))
+ metric_logger.add_meter("loss", SmoothedValue(window_size=1, fmt="{value:.4f}"))
+
+ # if iter-based runner, schedule lr based on inner epoch.
+ logging.info(
+ "Start training epoch {}, {} iters per inner epoch.".format(
+ epoch, iters_per_epoch
+ )
+ )
+ header = "Train: data epoch: [{}]".format(epoch)
+ if start_iters is None:
+ # epoch-based runner
+ inner_epoch = epoch
+ else:
+ # In iter-based runner, we schedule the learning rate based on iterations.
+ inner_epoch = start_iters // iters_per_epoch
+ header = header + "; inner epoch [{}]".format(inner_epoch)
+
+ for i in metric_logger.log_every(range(iters_per_epoch), log_freq, header):
+ # if using iter-based runner, we stop after iters_per_epoch iterations.
+ if i >= iters_per_epoch:
+ break
+
+ samples = next(data_loader)
+
+ samples = prepare_sample(samples, cuda_enabled=cuda_enabled)
+ samples.update(
+ {
+ "epoch": inner_epoch,
+ "num_iters_per_epoch": iters_per_epoch,
+ "iters": i,
+ }
+ )
+
+ lr_scheduler.step(cur_epoch=inner_epoch, cur_step=i)
+
+ with torch.cuda.amp.autocast(enabled=use_amp):
+ loss = self.train_step(model=model, samples=samples)
+
+ # after_train_step()
+ if use_amp:
+ scaler.scale(loss).backward()
+ else:
+ loss.backward()
+
+ # update gradients every accum_grad_iters iterations
+ if (i + 1) % accum_grad_iters == 0:
+ if use_amp:
+ scaler.step(optimizer)
+ scaler.update()
+ else:
+ optimizer.step()
+ optimizer.zero_grad()
+
+ metric_logger.update(loss=loss.item())
+ metric_logger.update(lr=optimizer.param_groups[0]["lr"])
+
+ # after train_epoch()
+ # gather the stats from all processes
+ metric_logger.synchronize_between_processes()
+ logging.info("Averaged stats: " + str(metric_logger.global_avg()))
+ return {
+ k: "{:.3f}".format(meter.global_avg)
+ for k, meter in metric_logger.meters.items()
+ }
+
+ @staticmethod
+ def save_result(result, result_dir, filename, remove_duplicate=""):
+ import json
+
+ result_file = os.path.join(
+ result_dir, "%s_rank%d.json" % (filename, get_rank())
+ )
+ final_result_file = os.path.join(result_dir, "%s.json" % filename)
+
+ json.dump(result, open(result_file, "w"))
+
+ if is_dist_avail_and_initialized():
+ dist.barrier()
+
+ if is_main_process():
+ logging.warning("rank %d starts merging results." % get_rank())
+ # combine results from all processes
+ result = []
+
+ for rank in range(get_world_size()):
+ result_file = os.path.join(
+ result_dir, "%s_rank%d.json" % (filename, rank)
+ )
+ res = json.load(open(result_file, "r"))
+ result += res
+
+ if remove_duplicate:
+ result_new = []
+ id_list = []
+ for res in result:
+ if res[remove_duplicate] not in id_list:
+ id_list.append(res[remove_duplicate])
+ result_new.append(res)
+ result = result_new
+
+ json.dump(result, open(final_result_file, "w"))
+ print("result file saved to %s" % final_result_file)
+
+ return final_result_file
diff --git a/global_local/tasks/image_text_pretrain.py b/global_local/tasks/image_text_pretrain.py
new file mode 100644
index 0000000000000000000000000000000000000000..7eb66dba502935f5fc3f48f14e8490a987cc8c40
--- /dev/null
+++ b/global_local/tasks/image_text_pretrain.py
@@ -0,0 +1,18 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from global_local.common.registry import registry
+from global_local.tasks.base_task import BaseTask
+
+
+@registry.register_task("image_text_pretrain")
+class ImageTextPretrainTask(BaseTask):
+ def __init__(self):
+ super().__init__()
+
+ def evaluation(self, model, data_loader, cuda_enabled=True):
+ pass
diff --git a/global_local/tasks/video_text_pretrain.py b/global_local/tasks/video_text_pretrain.py
new file mode 100644
index 0000000000000000000000000000000000000000..d9e8f8f7e26bdb6597318ac238a4ddf4c60ec235
--- /dev/null
+++ b/global_local/tasks/video_text_pretrain.py
@@ -0,0 +1,18 @@
+"""
+ Copyright (c) 2022, salesforce.com, inc.
+ All rights reserved.
+ SPDX-License-Identifier: BSD-3-Clause
+ For full license text, see the LICENSE_Lavis file in the repo root or https://opensource.org/licenses/BSD-3-Clause
+"""
+
+from global_local.common.registry import registry
+from global_local.tasks.base_task import BaseTask
+
+
+@registry.register_task("video_text_pretrain")
+class VideoTextPretrainTask(BaseTask):
+ def __init__(self):
+ super().__init__()
+
+ def evaluation(self, model, data_loader, cuda_enabled=True):
+ pass
diff --git a/prompts/alignment_image.txt b/prompts/alignment_image.txt
new file mode 100644
index 0000000000000000000000000000000000000000..0bc3d22ad8a3ff20180a87bd49d151e5a30553a6
--- /dev/null
+++ b/prompts/alignment_image.txt
@@ -0,0 +1,4 @@
+ Describe this image in detail.
+ Take a look at this image and describe what you notice.
+ Please provide a detailed description of the picture.
+ Could you describe the contents of this image for me?
\ No newline at end of file
diff --git a/requirements.txt b/requirements.txt
new file mode 100644
index 0000000000000000000000000000000000000000..9149ac78cda844f0abd40246abd6627116534b91
--- /dev/null
+++ b/requirements.txt
@@ -0,0 +1,13 @@
+--extra-index-url https://download.pytorch.org/whl/cu118
+torch==2.0.1
+torchvision==0.15.2
+transformers==4.33.3
+tqdm
+decord
+timm
+einops
+opencv_python
+torchvision
+
+salesforce-lavis
+accelerate
\ No newline at end of file