{ "cells": [ { "cell_type": "markdown", "id": "8c68f03e-620c-46a9-a7ec-a6cde27043cd", "metadata": {}, "source": [ "# Hugging Face Spaces From A Notebook\n", "\n", "> A demo of using nbdev with Hugging Face Spaces" ] }, { "cell_type": "markdown", "id": "96483373-4ae1-49b2-85ed-ceee8456df19", "metadata": {}, "source": [ "## 1. Create a Gradio-enabled Space on Hugging Face\n", "\n", "The first step is to create a space and select the appropriate sdk (which is Gradio in this example), per [these instructions](https://huggingface.co/docs/hub/spaces-overview#creating-a-new-space):" ] }, { "cell_type": "markdown", "id": "b34d7ec6-69b8-48c4-a68b-fad6db3c2fab", "metadata": {}, "source": [ "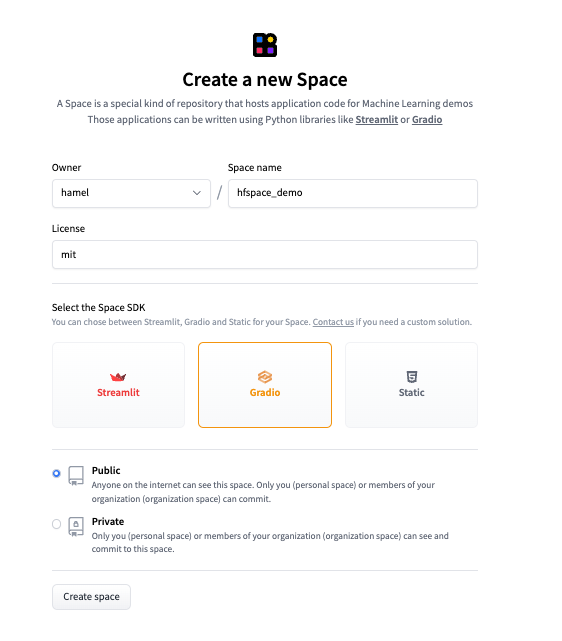" ] }, { "cell_type": "markdown", "id": "c25e8e7a-52d9-4305-a107-ba03e3d6a5f3", "metadata": {}, "source": [ "After you are done creating the space, **clone the repo per the instructions provided in the app.** In this example, I ran the command `git clone https://huggingface.co/spaces/hamel/hfspace_demo`." ] }, { "cell_type": "markdown", "id": "ff26114c-329b-4a97-98b5-c652554b0114", "metadata": {}, "source": [ "## 2. Make an app with Gradio" ] }, { "cell_type": "markdown", "id": "14a884fc-36e2-43ec-8e42-ca2903aaa4de", "metadata": {}, "source": [ "Below, we will create a [gradio](https://gradio.app/) app in a notebook and show you how to deploy it to [Hugging Face Spaces](https://huggingface.co/docs/hub/spaces).\n", "\n", "First, lets specify the libraries we need, which in this case are `gradio` and `fastcore`:" ] }, { "cell_type": "code", "execution_count": null, "id": "e5e5d597-19ad-46e5-81ad-8f646d8a1c21", "metadata": {}, "outputs": [], "source": [ "#|export\n", "import gradio as gr\n", "from fastcore.net import urljson, HTTPError" ] }, { "cell_type": "code", "execution_count": null, "id": "38a4389f-ef53-4626-a6f5-a859354f854b", "metadata": {}, "outputs": [], "source": [ "#|export\n", "def size(repo:str):\n", " \"Returns the size in GB of a HuggingFace Dataset.\"\n", " url = f'https://huggingface.co/api/datasets/{repo}'\n", " try: resp = urljson(f'{url}/treesize/main')\n", " except HTTPError: return f'Did not find repo: {url}'\n", " gb = resp['size'] / 1e9\n", " return f'{gb:.2f} GB'" ] }, { "cell_type": "markdown", "id": "9ff9f84d-7744-46ad-80ed-2cf1fa6d0643", "metadata": {}, "source": [ "`size` take as an input a [Hugging Face Dataset](https://huggingface.co/docs/datasets/index) repo and returns the total size in GB of the data.\n", "\n", "For example, we can check the size of [tglcourse/CelebA-faces-cropped-128](https://huggingface.co/datasets/tglcourse/CelebA-faces-cropped-128) like so:" ] }, { "cell_type": "code", "execution_count": null, "id": "95bc32b8-d8ff-4761-a2d7-0880c51d0a42", "metadata": {}, "outputs": [ { "data": { "text/plain": [ "'5.49 GB'" ] }, "execution_count": null, "metadata": {}, "output_type": "execute_result" } ], "source": [ "size(\"tglcourse/CelebA-faces-cropped-128\")" ] }, { "cell_type": "markdown", "id": "cb13747b-ea48-4146-846d-deb9e855d32d", "metadata": {}, "source": [ "You can construct a simple UI with the `gradio.interface` and then call the `launch` method of that interface to display a preview in a notebook. This is a great way to test your app to see if it works" ] }, { "cell_type": "code", "execution_count": null, "id": "7b20e2a1-b622-4970-9069-0202ce10a2ce", "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Running on local URL: http://127.0.0.1:7860\n", "\n", "To create a public link, set `share=True` in `launch()`.\n" ] }, { "data": { "text/html": [ "
" ], "text/plain": [ "