# !usr/bin/env python
# -*- coding:utf-8 -*-
'''
Description :
Version : 1.0
Author : MrYXJ
Mail : yxj2017@gmail.com
Github : https://github.com/MrYxJ
Date : 2023-09-05 23:25:28
LastEditTime : 2023-09-10 17:09:08
Copyright (C) 2023 mryxj. All rights reserved.
'''
import gradio as gr
import pandas as pd
from model_utils import calculate_flops_in_hugging_space
from model_utils import get_mode_from_hf
def get_calclops_result(model_name: str,
input_shape: tuple,
access_token: str,
bp_factor: float,
output_unit: str):
if model_name == "0910":
return [f"# Happy Birthday to You 🎂🎂!", gr.update(visible=False), gr.update(visible=False)]
try:
input_shape = eval(input_shape)
if not isinstance(input_shape, tuple):
raise Exception("Not a tuple.")
except Exception as e:
raise gr.Error(f"Input shape must be a tuple.")
try:
bp_factor = float(bp_factor)
except Exception as e:
raise gr.Error(f"The Factor of Backward as multiple as Forward must be a float.")
if output_unit not in ["", "auto", "T", "G", "M", "K", "m", "u"]:
raise gr.Error(
f"The Output Unit only can be leaved blank or 'T'、'G'、'M'、'K'、'm'、'u'."
)
model = get_mode_from_hf(model_name=model_name,
library="auto",
access_token=access_token)
title = f"## Calculate FLOPs and Params of '{model_name}' "
data, return_print = calculate_flops_in_hugging_space(model_name=model_name,
empty_model=model,
access_token=access_token,
input_shape=input_shape,
bp_factor=bp_factor,
output_unit=output_unit)
return [title, gr.update(visible=True, value=pd.DataFrame(data)), gr.update(visible=True, value=return_print)]
with gr.Blocks() as demo:
with gr.Column():
gr.Markdown(
"""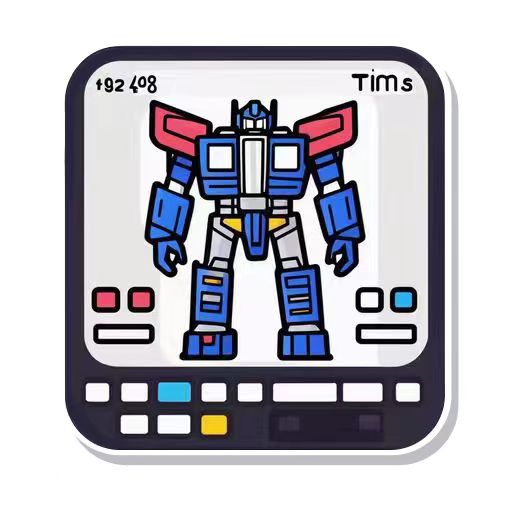
⛽️Model(Transformers) FLOPs and Parameter Calculator
This tool is based on the python package [calflops](https://pypi.org/project/calflops/) developed in 🤗Huggingface Space specifically for mainly transformers model to compute FLOPs and Parameters num more convenient.
And calflops is designed to compute the theoretical amount of FLOPs(floating-point operations)、MACs(multiply-add operations) and Parameters in all various neural networks, also including **Transformers(Bert、LlaMA etc Large Language Model) Models** as long as based on the Pytorch implementation,
, which also open source on github, welcome to [star🌟 and use🌟](https://github.com/MrYxJ/calculate-flops.pytorch).
This tool can support the calculation of FLOPs without downloading the parameters of the whole model to the local which is very friendly for large models, but at the same time, this method can only support forward propagation of the model in the `meta` device (Some model implemented operators are not supported on meta device).
Therefore, it is possible that the model operator cannot calculate FLOPs in this way because it does not support calculation on `meta` device, which is that you can also calculate FLOPs by ```pip install calflops``` and download the parameters of the full model to the local.
This tool also support to calculate the FLOPs of model once complete forward + backward pass. According to [epochai report](https://epochai.org/blog/backward-forward-FLOP-ratio), the backward pass of the model FLOPs is default **2** times as much computation as forward pass.
Considering the distributed training of large models, according to [paper](https://arxiv.org/pdf/2205.05198.pdf), if you use parameter activate the recalculation in order to save gpu memory which would make backward pass of models FLOPs would be **3** times as much computation as forward pass.
All in all, you can set the param `Factor of Backward as multiple as Forward` to determine how many times backward pass as much computation as forward pass. Meanwhile this tool supports the printing of FLOPS, Parameter calculation value and proportion of each submodule of the model, it is convient for users to understand the performance consumption of each part of the model and make targeted optimization.
To use this tool pass in the URL or model name on the 🤗HuggingFace Models that you want to calculate the FLOPs and Parameters, you can
select what input shape of model in one step、what times of model backward pass as much comsuption as forward pass and what unit of the calculation result. Finally, this tool is learned from anther good tool [model-memory-usage](https://huggingface.co/spaces/hf-accelerate/model-memory-usage) in the implementation process, 🙏thanks."""
)
out_text = gr.Markdown()
calculate_out = gr.DataFrame(
headers = ["Total Training Params", "Forward FLOPs", "Forward MACs", "Forward+Backward FLOPs", "Forward+Backward MACs"],
interactive=False,
visible=False
)
print_model = gr.Textbox(label=f"Print Each Modules FLOPs、Params", visible=False,
show_legend=True, show_copy_button=True)
with gr.Row():
inp = gr.Textbox(label="Model Name or URL", value="bert-base-cased")
access_token = gr.Textbox(label="API Token", placeholder="Optional (for gated models)")
with gr.Row():
input_shape = gr.Textbox(label="Input Shape, such: (batch, seq_len)", value="(1, 128)")
bp_factor = gr.Textbox(label="Factor of Backward as multiple as Forward", value="2.0")
output_unit = gr.Textbox(label="Outputs Unit, blank means not using unit", value="auto",
placeholder="Optional, leave blank or 'auto','T','G','M','K','m','u'.")
with gr.Row():
btn = gr.Button("Calculate FLOPs and Params")
post_to_hub = gr.Button(
value="Report results in this model repo's discussions!\n(Will open in a new tab)", visible=False
)
btn.click(
get_calclops_result,
inputs=[inp, input_shape, access_token, bp_factor, output_unit],
outputs=[out_text, calculate_out, print_model]
)
demo.launch()