# Comparing model predictions and ground truth labels with Rubrix and Hugging Face ## Build dataset You can skip this step if you run: ```python from datasets import load_dataset import rubrix as rb ds = rb.DatasetForTextClassification.from_datasets(load_dataset("rubrix/sst2_with_predictions", split="train")) ``` Otherwise, the following cell will run the pipeline over the training set and store labels and predictions. ```python from datasets import load_dataset from transformers import pipeline, AutoModelForSequenceClassification import rubrix as rb name = "distilbert-base-uncased-finetuned-sst-2-english" # Need to define id2label because surprisingly the pipeline has uppercase label names model = AutoModelForSequenceClassification.from_pretrained(name, id2label={0: 'negative', 1: 'positive'}) nlp = pipeline("sentiment-analysis", model=model, tokenizer=name, return_all_scores=True) dataset = load_dataset("glue", "sst2", split="train") # batch predict def predict(example): return {"prediction": nlp(example["sentence"])} # add predictions to the dataset dataset = dataset.map(predict, batched=True).rename_column("sentence", "text") # build rubrix dataset from hf dataset ds = rb.DatasetForTextClassification.from_datasets(dataset, annotation="label") ``` ```python # Install Rubrix and start exploring and sharing URLs with interesting subsets, etc. rb.log(ds, "sst2") ``` ```python ds.to_datasets().push_to_hub("rubrix/sst2_with_predictions") ``` Pushing dataset shards to the dataset hub: 0%| | 0/1 [00:00, ?it/s] ## Analize misspredictions and ambiguous labels ### With the UI With Rubrix's UI you can: - Combine filters and full-text/DSL queries to quickly find important samples - All URLs contain the state so you can share with collaborator and annotator specific dataset regions to work on. - Sort examples by score, as well as custom metadata fields. 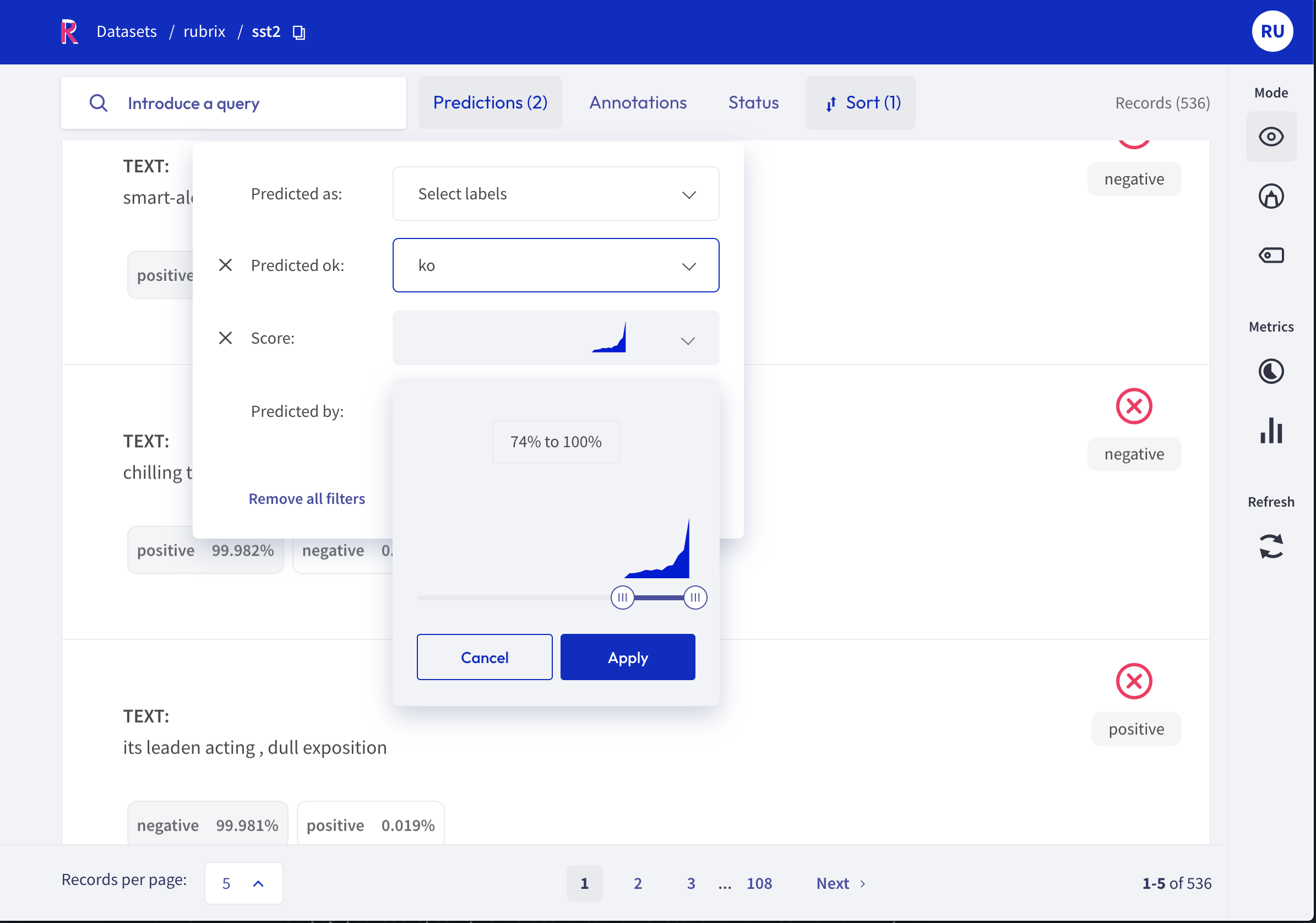 ### Programmatically Let's find all the wrong predictions from Python. This is useful for bulk operations (relabelling, discarding, etc.) as well as ```python import pandas as pd # Get dataset slice with wrong predictions df = rb.load("sst2", query="predicted:ko").to_pandas() # display first 20 examples with pd.option_context('display.max_colwidth', None): display(df[["text", "prediction", "annotation"]].head(20)) ```
text | prediction | annotation | |
---|---|---|---|
0 | this particular , anciently demanding métier | [(negative, 0.9386059045791626), (positive, 0.06139408051967621)] | positive |
1 | under our skin | [(positive, 0.7508484721183777), (negative, 0.24915160238742828)] | negative |
2 | evokes a palpable sense of disconnection , made all the more poignant by the incessant use of cell phones . | [(negative, 0.6634528636932373), (positive, 0.3365470767021179)] | positive |
3 | plays like a living-room war of the worlds , gaining most of its unsettling force from the suggested and the unknown . | [(positive, 0.9968075752258301), (negative, 0.003192420583218336)] | negative |
4 | into a pulpy concept that , in many other hands would be completely forgettable | [(positive, 0.6178210377693176), (negative, 0.3821789622306824)] | negative |
5 | transcends ethnic lines . | [(positive, 0.9758220314979553), (negative, 0.024177948012948036)] | negative |
6 | is barely | [(negative, 0.9922297596931458), (positive, 0.00777028314769268)] | positive |
7 | a pulpy concept that , in many other hands would be completely forgettable | [(negative, 0.9738760590553284), (positive, 0.026123959571123123)] | positive |
8 | of hollywood heart-string plucking | [(positive, 0.9889695644378662), (negative, 0.011030420660972595)] | negative |
9 | a minimalist beauty and the beast | [(positive, 0.9100378751754761), (negative, 0.08996208757162094)] | negative |
10 | the intimate , unguarded moments of folks who live in unusual homes -- | [(positive, 0.9967381358146667), (negative, 0.0032618637196719646)] | negative |
11 | steals the show | [(negative, 0.8031412363052368), (positive, 0.1968587338924408)] | positive |
12 | enough | [(positive, 0.7941301465034485), (negative, 0.2058698982000351)] | negative |
13 | accept it as life and | [(positive, 0.9987508058547974), (negative, 0.0012492131209000945)] | negative |
14 | this is the kind of movie that you only need to watch for about thirty seconds before you say to yourself , ` ah , yes , | [(negative, 0.7889454960823059), (positive, 0.21105451881885529)] | positive |
15 | plunges you into a reality that is , more often then not , difficult and sad , | [(positive, 0.967541515827179), (negative, 0.03245845437049866)] | negative |
16 | overcomes the script 's flaws and envelops the audience in his character 's anguish , anger and frustration . | [(positive, 0.9953157901763916), (negative, 0.004684178624302149)] | negative |
17 | troubled and determined homicide cop | [(negative, 0.6632784008979797), (positive, 0.33672159910202026)] | positive |
18 | human nature is a goofball movie , in the way that malkovich was , but it tries too hard | [(positive, 0.5959018468856812), (negative, 0.40409812331199646)] | negative |
19 | to watch too many barney videos | [(negative, 0.9909896850585938), (positive, 0.00901023019105196)] | positive |
text | prediction | annotation | |
---|---|---|---|
0 | plays like a living-room war of the worlds , gaining most of its unsettling force from the suggested and the unknown . | [(positive, 0.9968075752258301), (negative, 0.003192420583218336)] | negative |
1 | a minimalist beauty and the beast | [(positive, 0.9100378751754761), (negative, 0.08996208757162094)] | negative |
2 | accept it as life and | [(positive, 0.9987508058547974), (negative, 0.0012492131209000945)] | negative |
3 | plunges you into a reality that is , more often then not , difficult and sad , | [(positive, 0.967541515827179), (negative, 0.03245845437049866)] | negative |
4 | overcomes the script 's flaws and envelops the audience in his character 's anguish , anger and frustration . | [(positive, 0.9953157901763916), (negative, 0.004684178624302149)] | negative |
5 | and social commentary | [(positive, 0.7863275408744812), (negative, 0.2136724889278412)] | negative |
6 | we do n't get williams ' usual tear and a smile , just sneers and bile , and the spectacle is nothing short of refreshing . | [(positive, 0.9982783794403076), (negative, 0.0017216014675796032)] | negative |
7 | before pulling the plug on the conspirators and averting an american-russian armageddon | [(positive, 0.6992855072021484), (negative, 0.30071452260017395)] | negative |
8 | in tight pants and big tits | [(positive, 0.7850217819213867), (negative, 0.2149781733751297)] | negative |
9 | that it certainly does n't feel like a film that strays past the two and a half mark | [(positive, 0.6591460108757019), (negative, 0.3408539891242981)] | negative |
10 | actress-producer and writer | [(positive, 0.8167378306388855), (negative, 0.1832621842622757)] | negative |
11 | gives devastating testimony to both people 's capacity for evil and their heroic capacity for good . | [(positive, 0.8960123062133789), (negative, 0.10398765653371811)] | negative |
12 | deep into the girls ' confusion and pain as they struggle tragically to comprehend the chasm of knowledge that 's opened between them | [(positive, 0.9729612469673157), (negative, 0.027038726955652237)] | negative |
13 | a younger lad in zen and the art of getting laid in this prickly indie comedy of manners and misanthropy | [(positive, 0.9875985980033875), (negative, 0.012401451356709003)] | negative |
14 | get on a board and , uh , shred , | [(positive, 0.5352609753608704), (negative, 0.46473899483680725)] | negative |
15 | so preachy-keen and | [(positive, 0.9644021391868591), (negative, 0.035597823560237885)] | negative |
16 | there 's an admirable rigor to jimmy 's relentless anger , and to the script 's refusal of a happy ending , | [(positive, 0.9928517937660217), (negative, 0.007148175034672022)] | negative |
17 | ` christian bale 's quinn ( is ) a leather clad grunge-pirate with a hairdo like gandalf in a wind-tunnel and a simply astounding cor-blimey-luv-a-duck cockney accent . ' | [(positive, 0.9713286757469177), (negative, 0.028671346604824066)] | negative |
18 | passion , grief and fear | [(positive, 0.9849751591682434), (negative, 0.015024829655885696)] | negative |
19 | to keep the extremes of screwball farce and blood-curdling family intensity on one continuum | [(positive, 0.8838250637054443), (negative, 0.11617499589920044)] | negative |
text | prediction | annotation | |
---|---|---|---|
0 | plays like a living-room war of the worlds , gaining most of its unsettling force from the suggested and the unknown . | [(positive, 0.9968075752258301), (negative, 0.003192420583218336)] | negative |
1 | accept it as life and | [(positive, 0.9987508058547974), (negative, 0.0012492131209000945)] | negative |
2 | overcomes the script 's flaws and envelops the audience in his character 's anguish , anger and frustration . | [(positive, 0.9953157901763916), (negative, 0.004684178624302149)] | negative |
3 | will no doubt rally to its cause , trotting out threadbare standbys like ` masterpiece ' and ` triumph ' and all that malarkey , | [(negative, 0.9936562180519104), (positive, 0.006343740504235029)] | positive |
4 | we do n't get williams ' usual tear and a smile , just sneers and bile , and the spectacle is nothing short of refreshing . | [(positive, 0.9982783794403076), (negative, 0.0017216014675796032)] | negative |
5 | somehow manages to bring together kevin pollak , former wrestler chyna and dolly parton | [(negative, 0.9979034662246704), (positive, 0.002096540294587612)] | positive |
6 | there 's an admirable rigor to jimmy 's relentless anger , and to the script 's refusal of a happy ending , | [(positive, 0.9928517937660217), (negative, 0.007148175034672022)] | negative |
7 | the bottom line with nemesis is the same as it has been with all the films in the series : fans will undoubtedly enjoy it , and the uncommitted need n't waste their time on it | [(positive, 0.995850682258606), (negative, 0.004149340093135834)] | negative |
8 | is genial but never inspired , and little | [(negative, 0.9921030402183533), (positive, 0.007896988652646542)] | positive |
9 | heaped upon a project of such vast proportions need to reap more rewards than spiffy bluescreen technique and stylish weaponry . | [(negative, 0.9958089590072632), (positive, 0.004191054962575436)] | positive |
10 | than recommended -- as visually bland as a dentist 's waiting room , complete with soothing muzak and a cushion of predictable narrative rhythms | [(negative, 0.9988711476325989), (positive, 0.0011287889210507274)] | positive |
11 | spectacle and | [(positive, 0.9941601753234863), (negative, 0.005839805118739605)] | negative |
12 | groan and | [(negative, 0.9987359642982483), (positive, 0.0012639997294172645)] | positive |
13 | 're not likely to have seen before , but beneath the exotic surface ( and exotic dancing ) it 's surprisingly old-fashioned . | [(positive, 0.9908103942871094), (negative, 0.009189637377858162)] | negative |
14 | its metaphors are opaque enough to avoid didacticism , and | [(negative, 0.990602970123291), (positive, 0.00939704105257988)] | positive |
15 | by kevin bray , whose crisp framing , edgy camera work , and wholesale ineptitude with acting , tone and pace very obviously mark him as a video helmer making his feature debut | [(positive, 0.9973387122154236), (negative, 0.0026612314395606518)] | negative |
16 | evokes the frustration , the awkwardness and the euphoria of growing up , without relying on the usual tropes . | [(positive, 0.9989104270935059), (negative, 0.0010896018939092755)] | negative |
17 | , incoherence and sub-sophomoric | [(negative, 0.9962475895881653), (positive, 0.003752368036657572)] | positive |
18 | seems intimidated by both her subject matter and the period trappings of this debut venture into the heritage business . | [(negative, 0.9923072457313538), (positive, 0.007692818529903889)] | positive |
19 | despite downplaying her good looks , carries a little too much ai n't - she-cute baggage into her lead role as a troubled and determined homicide cop to quite pull off the heavy stuff . | [(negative, 0.9948075413703918), (positive, 0.005192441400140524)] | positive |
text | prediction | annotation | |
---|---|---|---|
0 | get on a board and , uh , shred , | [(positive, 0.5352609753608704), (negative, 0.46473899483680725)] | negative |
1 | is , truly and thankfully , a one-of-a-kind work | [(positive, 0.5819814801216125), (negative, 0.41801854968070984)] | negative |
2 | starts as a tart little lemon drop of a movie and | [(negative, 0.5641832947731018), (positive, 0.4358167052268982)] | positive |
3 | between flaccid satire and what | [(negative, 0.5532692074775696), (positive, 0.44673076272010803)] | positive |
4 | it certainly does n't feel like a film that strays past the two and a half mark | [(negative, 0.5386656522750854), (positive, 0.46133431792259216)] | positive |
5 | who liked there 's something about mary and both american pie movies | [(negative, 0.5086333751678467), (positive, 0.4913666248321533)] | positive |
6 | many good ideas as bad is the cold comfort that chin 's film serves up with style and empathy | [(positive, 0.557632327079773), (negative, 0.44236767292022705)] | negative |
7 | about its ideas and | [(positive, 0.518638551235199), (negative, 0.48136141896247864)] | negative |
8 | of a sick and evil woman | [(negative, 0.5554516315460205), (positive, 0.4445483684539795)] | positive |
9 | though this rude and crude film does deliver a few gut-busting laughs | [(positive, 0.5045541524887085), (negative, 0.4954459071159363)] | negative |
10 | to squeeze the action and our emotions into the all-too-familiar dramatic arc of the holocaust escape story | [(negative, 0.5050069093704224), (positive, 0.49499306082725525)] | positive |
11 | that throws a bunch of hot-button items in the viewer 's face and asks to be seen as hip , winking social commentary | [(negative, 0.5873904228210449), (positive, 0.41260960698127747)] | positive |
12 | 's soulful and unslick | [(positive, 0.5931627750396729), (negative, 0.40683719515800476)] | negative |